something parses to map["ns:key"]interface{}{"something"}
- - xmlns:ns="http://myns.com/ns" parses to map["xmlns:ns"]interface{}{"http://myns.com/ns"}
-
- Both
-
- - By default, "Nan", "Inf", and "-Inf" values are not cast to float64. If you want them
- to be cast, set a flag to cast them using CastNanInf(true).
-
-XML ENCODING CONVENTIONS
-
- - 'nil' Map values, which may represent 'null' JSON values, are encoded as "".
- NOTE: the operation is not symmetric as "" elements are decoded as 'tag:""' Map values,
- which, then, encode in JSON as '"tag":""' values..
- - ALSO: there is no guarantee that the encoded XML doc will be the same as the decoded one. (Go
- randomizes the walk through map[string]interface{} values.) If you plan to re-encode the
- Map value to XML and want the same sequencing of elements look at NewMapXmlSeq() and
- mv.XmlSeq() - these try to preserve the element sequencing but with added complexity when
- working with the Map representation.
-
-*/
-package mxj
diff --git a/vendor/github.com/clbanning/mxj/escapechars.go b/vendor/github.com/clbanning/mxj/escapechars.go
deleted file mode 100644
index bee0442c..00000000
--- a/vendor/github.com/clbanning/mxj/escapechars.go
+++ /dev/null
@@ -1,54 +0,0 @@
-// Copyright 2016 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-package mxj
-
-import (
- "bytes"
-)
-
-var xmlEscapeChars bool
-
-// XMLEscapeChars(true) forces escaping invalid characters in attribute and element values.
-// NOTE: this is brute force with NO interrogation of '&' being escaped already; if it is
-// then '&' will be re-escaped as '&'.
-//
-/*
- The values are:
- " "
- ' '
- < <
- > >
- & &
-*/
-func XMLEscapeChars(b bool) {
- xmlEscapeChars = b
-}
-
-// Scan for '&' first, since 's' may contain "&" that is parsed to "&"
-// - or "<" that is parsed to "<".
-var escapechars = [][2][]byte{
- {[]byte(`&`), []byte(`&`)},
- {[]byte(`<`), []byte(`<`)},
- {[]byte(`>`), []byte(`>`)},
- {[]byte(`"`), []byte(`"`)},
- {[]byte(`'`), []byte(`'`)},
-}
-
-func escapeChars(s string) string {
- if len(s) == 0 {
- return s
- }
-
- b := []byte(s)
- for _, v := range escapechars {
- n := bytes.Count(b, v[0])
- if n == 0 {
- continue
- }
- b = bytes.Replace(b, v[0], v[1], n)
- }
- return string(b)
-}
-
diff --git a/vendor/github.com/clbanning/mxj/exists.go b/vendor/github.com/clbanning/mxj/exists.go
deleted file mode 100644
index 2fb3084b..00000000
--- a/vendor/github.com/clbanning/mxj/exists.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package mxj
-
-// Checks whether the path exists
-func (mv Map) Exists(path string, subkeys ...string) bool {
- v, err := mv.ValuesForPath(path, subkeys...)
- return err == nil && len(v) > 0
-}
diff --git a/vendor/github.com/clbanning/mxj/files.go b/vendor/github.com/clbanning/mxj/files.go
deleted file mode 100644
index 27e06e1e..00000000
--- a/vendor/github.com/clbanning/mxj/files.go
+++ /dev/null
@@ -1,287 +0,0 @@
-package mxj
-
-import (
- "fmt"
- "io"
- "os"
-)
-
-type Maps []Map
-
-func NewMaps() Maps {
- return make(Maps, 0)
-}
-
-type MapRaw struct {
- M Map
- R []byte
-}
-
-// NewMapsFromXmlFile - creates an array from a file of JSON values.
-func NewMapsFromJsonFile(name string) (Maps, error) {
- fi, err := os.Stat(name)
- if err != nil {
- return nil, err
- }
- if !fi.Mode().IsRegular() {
- return nil, fmt.Errorf("file %s is not a regular file", name)
- }
-
- fh, err := os.Open(name)
- if err != nil {
- return nil, err
- }
- defer fh.Close()
-
- am := make([]Map, 0)
- for {
- m, raw, err := NewMapJsonReaderRaw(fh)
- if err != nil && err != io.EOF {
- return am, fmt.Errorf("error: %s - reading: %s", err.Error(), string(raw))
- }
- if len(m) > 0 {
- am = append(am, m)
- }
- if err == io.EOF {
- break
- }
- }
- return am, nil
-}
-
-// ReadMapsFromJsonFileRaw - creates an array of MapRaw from a file of JSON values.
-func NewMapsFromJsonFileRaw(name string) ([]MapRaw, error) {
- fi, err := os.Stat(name)
- if err != nil {
- return nil, err
- }
- if !fi.Mode().IsRegular() {
- return nil, fmt.Errorf("file %s is not a regular file", name)
- }
-
- fh, err := os.Open(name)
- if err != nil {
- return nil, err
- }
- defer fh.Close()
-
- am := make([]MapRaw, 0)
- for {
- mr := new(MapRaw)
- mr.M, mr.R, err = NewMapJsonReaderRaw(fh)
- if err != nil && err != io.EOF {
- return am, fmt.Errorf("error: %s - reading: %s", err.Error(), string(mr.R))
- }
- if len(mr.M) > 0 {
- am = append(am, *mr)
- }
- if err == io.EOF {
- break
- }
- }
- return am, nil
-}
-
-// NewMapsFromXmlFile - creates an array from a file of XML values.
-func NewMapsFromXmlFile(name string) (Maps, error) {
- fi, err := os.Stat(name)
- if err != nil {
- return nil, err
- }
- if !fi.Mode().IsRegular() {
- return nil, fmt.Errorf("file %s is not a regular file", name)
- }
-
- fh, err := os.Open(name)
- if err != nil {
- return nil, err
- }
- defer fh.Close()
-
- am := make([]Map, 0)
- for {
- m, raw, err := NewMapXmlReaderRaw(fh)
- if err != nil && err != io.EOF {
- return am, fmt.Errorf("error: %s - reading: %s", err.Error(), string(raw))
- }
- if len(m) > 0 {
- am = append(am, m)
- }
- if err == io.EOF {
- break
- }
- }
- return am, nil
-}
-
-// NewMapsFromXmlFileRaw - creates an array of MapRaw from a file of XML values.
-// NOTE: the slice with the raw XML is clean with no extra capacity - unlike NewMapXmlReaderRaw().
-// It is slow at parsing a file from disk and is intended for relatively small utility files.
-func NewMapsFromXmlFileRaw(name string) ([]MapRaw, error) {
- fi, err := os.Stat(name)
- if err != nil {
- return nil, err
- }
- if !fi.Mode().IsRegular() {
- return nil, fmt.Errorf("file %s is not a regular file", name)
- }
-
- fh, err := os.Open(name)
- if err != nil {
- return nil, err
- }
- defer fh.Close()
-
- am := make([]MapRaw, 0)
- for {
- mr := new(MapRaw)
- mr.M, mr.R, err = NewMapXmlReaderRaw(fh)
- if err != nil && err != io.EOF {
- return am, fmt.Errorf("error: %s - reading: %s", err.Error(), string(mr.R))
- }
- if len(mr.M) > 0 {
- am = append(am, *mr)
- }
- if err == io.EOF {
- break
- }
- }
- return am, nil
-}
-
-// ------------------------ Maps writing -------------------------
-// These are handy-dandy methods for dumping configuration data, etc.
-
-// JsonString - analogous to mv.Json()
-func (mvs Maps) JsonString(safeEncoding ...bool) (string, error) {
- var s string
- for _, v := range mvs {
- j, err := v.Json()
- if err != nil {
- return s, err
- }
- s += string(j)
- }
- return s, nil
-}
-
-// JsonStringIndent - analogous to mv.JsonIndent()
-func (mvs Maps) JsonStringIndent(prefix, indent string, safeEncoding ...bool) (string, error) {
- var s string
- var haveFirst bool
- for _, v := range mvs {
- j, err := v.JsonIndent(prefix, indent)
- if err != nil {
- return s, err
- }
- if haveFirst {
- s += "\n"
- } else {
- haveFirst = true
- }
- s += string(j)
- }
- return s, nil
-}
-
-// XmlString - analogous to mv.Xml()
-func (mvs Maps) XmlString() (string, error) {
- var s string
- for _, v := range mvs {
- x, err := v.Xml()
- if err != nil {
- return s, err
- }
- s += string(x)
- }
- return s, nil
-}
-
-// XmlStringIndent - analogous to mv.XmlIndent()
-func (mvs Maps) XmlStringIndent(prefix, indent string) (string, error) {
- var s string
- for _, v := range mvs {
- x, err := v.XmlIndent(prefix, indent)
- if err != nil {
- return s, err
- }
- s += string(x)
- }
- return s, nil
-}
-
-// JsonFile - write Maps to named file as JSON
-// Note: the file will be created, if necessary; if it exists it will be truncated.
-// If you need to append to a file, open it and use JsonWriter method.
-func (mvs Maps) JsonFile(file string, safeEncoding ...bool) error {
- var encoding bool
- if len(safeEncoding) == 1 {
- encoding = safeEncoding[0]
- }
- s, err := mvs.JsonString(encoding)
- if err != nil {
- return err
- }
- fh, err := os.Create(file)
- if err != nil {
- return err
- }
- defer fh.Close()
- fh.WriteString(s)
- return nil
-}
-
-// JsonFileIndent - write Maps to named file as pretty JSON
-// Note: the file will be created, if necessary; if it exists it will be truncated.
-// If you need to append to a file, open it and use JsonIndentWriter method.
-func (mvs Maps) JsonFileIndent(file, prefix, indent string, safeEncoding ...bool) error {
- var encoding bool
- if len(safeEncoding) == 1 {
- encoding = safeEncoding[0]
- }
- s, err := mvs.JsonStringIndent(prefix, indent, encoding)
- if err != nil {
- return err
- }
- fh, err := os.Create(file)
- if err != nil {
- return err
- }
- defer fh.Close()
- fh.WriteString(s)
- return nil
-}
-
-// XmlFile - write Maps to named file as XML
-// Note: the file will be created, if necessary; if it exists it will be truncated.
-// If you need to append to a file, open it and use XmlWriter method.
-func (mvs Maps) XmlFile(file string) error {
- s, err := mvs.XmlString()
- if err != nil {
- return err
- }
- fh, err := os.Create(file)
- if err != nil {
- return err
- }
- defer fh.Close()
- fh.WriteString(s)
- return nil
-}
-
-// XmlFileIndent - write Maps to named file as pretty XML
-// Note: the file will be created,if necessary; if it exists it will be truncated.
-// If you need to append to a file, open it and use XmlIndentWriter method.
-func (mvs Maps) XmlFileIndent(file, prefix, indent string) error {
- s, err := mvs.XmlStringIndent(prefix, indent)
- if err != nil {
- return err
- }
- fh, err := os.Create(file)
- if err != nil {
- return err
- }
- defer fh.Close()
- fh.WriteString(s)
- return nil
-}
diff --git a/vendor/github.com/clbanning/mxj/files_test.badjson b/vendor/github.com/clbanning/mxj/files_test.badjson
deleted file mode 100644
index d1872004..00000000
--- a/vendor/github.com/clbanning/mxj/files_test.badjson
+++ /dev/null
@@ -1,2 +0,0 @@
-{ "this":"is", "a":"test", "file":"for", "files_test.go":"case" }
-{ "with":"some", "bad":JSON, "in":"it" }
diff --git a/vendor/github.com/clbanning/mxj/files_test.badxml b/vendor/github.com/clbanning/mxj/files_test.badxml
deleted file mode 100644
index 4736ef97..00000000
--- a/vendor/github.com/clbanning/mxj/files_test.badxml
+++ /dev/null
@@ -1,9 +0,0 @@
-
- test
- for files.go
-
-
- some
- doc
- test case
-
diff --git a/vendor/github.com/clbanning/mxj/files_test.json b/vendor/github.com/clbanning/mxj/files_test.json
deleted file mode 100644
index e9a3ddf4..00000000
--- a/vendor/github.com/clbanning/mxj/files_test.json
+++ /dev/null
@@ -1,2 +0,0 @@
-{ "this":"is", "a":"test", "file":"for", "files_test.go":"case" }
-{ "with":"just", "two":2, "JSON":"values", "true":true }
diff --git a/vendor/github.com/clbanning/mxj/files_test.xml b/vendor/github.com/clbanning/mxj/files_test.xml
deleted file mode 100644
index 65cf021f..00000000
--- a/vendor/github.com/clbanning/mxj/files_test.xml
+++ /dev/null
@@ -1,9 +0,0 @@
-
- test
- for files.go
-
-
- some
- doc
- test case
-
diff --git a/vendor/github.com/clbanning/mxj/files_test_dup.json b/vendor/github.com/clbanning/mxj/files_test_dup.json
deleted file mode 100644
index 2becb6a4..00000000
--- a/vendor/github.com/clbanning/mxj/files_test_dup.json
+++ /dev/null
@@ -1 +0,0 @@
-{"a":"test","file":"for","files_test.go":"case","this":"is"}{"JSON":"values","true":true,"two":2,"with":"just"}
\ No newline at end of file
diff --git a/vendor/github.com/clbanning/mxj/files_test_dup.xml b/vendor/github.com/clbanning/mxj/files_test_dup.xml
deleted file mode 100644
index f68d22e2..00000000
--- a/vendor/github.com/clbanning/mxj/files_test_dup.xml
+++ /dev/null
@@ -1 +0,0 @@
-for files.gotestdoctest casesome
\ No newline at end of file
diff --git a/vendor/github.com/clbanning/mxj/files_test_indent.json b/vendor/github.com/clbanning/mxj/files_test_indent.json
deleted file mode 100644
index 6fde1563..00000000
--- a/vendor/github.com/clbanning/mxj/files_test_indent.json
+++ /dev/null
@@ -1,12 +0,0 @@
-{
- "a": "test",
- "file": "for",
- "files_test.go": "case",
- "this": "is"
-}
-{
- "JSON": "values",
- "true": true,
- "two": 2,
- "with": "just"
-}
\ No newline at end of file
diff --git a/vendor/github.com/clbanning/mxj/files_test_indent.xml b/vendor/github.com/clbanning/mxj/files_test_indent.xml
deleted file mode 100644
index 8c91a1dc..00000000
--- a/vendor/github.com/clbanning/mxj/files_test_indent.xml
+++ /dev/null
@@ -1,8 +0,0 @@
-
- for files.go
- test
-
- doc
- test case
- some
-
\ No newline at end of file
diff --git a/vendor/github.com/clbanning/mxj/gob.go b/vendor/github.com/clbanning/mxj/gob.go
deleted file mode 100644
index d56c2fd6..00000000
--- a/vendor/github.com/clbanning/mxj/gob.go
+++ /dev/null
@@ -1,35 +0,0 @@
-// gob.go - Encode/Decode a Map into a gob object.
-
-package mxj
-
-import (
- "bytes"
- "encoding/gob"
-)
-
-// NewMapGob returns a Map value for a gob object that has been
-// encoded from a map[string]interface{} (or compatible type) value.
-// It is intended to provide symmetric handling of Maps that have
-// been encoded using mv.Gob.
-func NewMapGob(gobj []byte) (Map, error) {
- m := make(map[string]interface{}, 0)
- if len(gobj) == 0 {
- return m, nil
- }
- r := bytes.NewReader(gobj)
- dec := gob.NewDecoder(r)
- if err := dec.Decode(&m); err != nil {
- return m, err
- }
- return m, nil
-}
-
-// Gob returns a gob-encoded value for the Map 'mv'.
-func (mv Map) Gob() ([]byte, error) {
- var buf bytes.Buffer
- enc := gob.NewEncoder(&buf)
- if err := enc.Encode(map[string]interface{}(mv)); err != nil {
- return nil, err
- }
- return buf.Bytes(), nil
-}
diff --git a/vendor/github.com/clbanning/mxj/json.go b/vendor/github.com/clbanning/mxj/json.go
deleted file mode 100644
index eb2c05a1..00000000
--- a/vendor/github.com/clbanning/mxj/json.go
+++ /dev/null
@@ -1,323 +0,0 @@
-// Copyright 2012-2014 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-package mxj
-
-import (
- "bytes"
- "encoding/json"
- "fmt"
- "io"
- "time"
-)
-
-// ------------------------------ write JSON -----------------------
-
-// Just a wrapper on json.Marshal.
-// If option safeEncoding is'true' then safe encoding of '<', '>' and '&'
-// is preserved. (see encoding/json#Marshal, encoding/json#Encode)
-func (mv Map) Json(safeEncoding ...bool) ([]byte, error) {
- var s bool
- if len(safeEncoding) == 1 {
- s = safeEncoding[0]
- }
-
- b, err := json.Marshal(mv)
-
- if !s {
- b = bytes.Replace(b, []byte("\\u003c"), []byte("<"), -1)
- b = bytes.Replace(b, []byte("\\u003e"), []byte(">"), -1)
- b = bytes.Replace(b, []byte("\\u0026"), []byte("&"), -1)
- }
- return b, err
-}
-
-// Just a wrapper on json.MarshalIndent.
-// If option safeEncoding is'true' then safe encoding of '<' , '>' and '&'
-// is preserved. (see encoding/json#Marshal, encoding/json#Encode)
-func (mv Map) JsonIndent(prefix, indent string, safeEncoding ...bool) ([]byte, error) {
- var s bool
- if len(safeEncoding) == 1 {
- s = safeEncoding[0]
- }
-
- b, err := json.MarshalIndent(mv, prefix, indent)
- if !s {
- b = bytes.Replace(b, []byte("\\u003c"), []byte("<"), -1)
- b = bytes.Replace(b, []byte("\\u003e"), []byte(">"), -1)
- b = bytes.Replace(b, []byte("\\u0026"), []byte("&"), -1)
- }
- return b, err
-}
-
-// The following implementation is provided for symmetry with NewMapJsonReader[Raw]
-// The names will also provide a key for the number of return arguments.
-
-// Writes the Map as JSON on the Writer.
-// If 'safeEncoding' is 'true', then "safe" encoding of '<', '>' and '&' is preserved.
-func (mv Map) JsonWriter(jsonWriter io.Writer, safeEncoding ...bool) error {
- b, err := mv.Json(safeEncoding...)
- if err != nil {
- return err
- }
-
- _, err = jsonWriter.Write(b)
- return err
-}
-
-// Writes the Map as JSON on the Writer. []byte is the raw JSON that was written.
-// If 'safeEncoding' is 'true', then "safe" encoding of '<', '>' and '&' is preserved.
-func (mv Map) JsonWriterRaw(jsonWriter io.Writer, safeEncoding ...bool) ([]byte, error) {
- b, err := mv.Json(safeEncoding...)
- if err != nil {
- return b, err
- }
-
- _, err = jsonWriter.Write(b)
- return b, err
-}
-
-// Writes the Map as pretty JSON on the Writer.
-// If 'safeEncoding' is 'true', then "safe" encoding of '<', '>' and '&' is preserved.
-func (mv Map) JsonIndentWriter(jsonWriter io.Writer, prefix, indent string, safeEncoding ...bool) error {
- b, err := mv.JsonIndent(prefix, indent, safeEncoding...)
- if err != nil {
- return err
- }
-
- _, err = jsonWriter.Write(b)
- return err
-}
-
-// Writes the Map as pretty JSON on the Writer. []byte is the raw JSON that was written.
-// If 'safeEncoding' is 'true', then "safe" encoding of '<', '>' and '&' is preserved.
-func (mv Map) JsonIndentWriterRaw(jsonWriter io.Writer, prefix, indent string, safeEncoding ...bool) ([]byte, error) {
- b, err := mv.JsonIndent(prefix, indent, safeEncoding...)
- if err != nil {
- return b, err
- }
-
- _, err = jsonWriter.Write(b)
- return b, err
-}
-
-// --------------------------- read JSON -----------------------------
-
-// Decode numericvalues as json.Number type Map values - see encoding/json#Number.
-// NOTE: this is for decoding JSON into a Map with NewMapJson(), NewMapJsonReader(),
-// etc.; it does not affect NewMapXml(), etc. The XML encoders mv.Xml() and mv.XmlIndent()
-// do recognize json.Number types; a JSON object can be decoded to a Map with json.Number
-// value types and the resulting Map can be correctly encoded into a XML object.
-var JsonUseNumber bool
-
-// Just a wrapper on json.Unmarshal
-// Converting JSON to XML is a simple as:
-// ...
-// mapVal, merr := mxj.NewMapJson(jsonVal)
-// if merr != nil {
-// // handle error
-// }
-// xmlVal, xerr := mapVal.Xml()
-// if xerr != nil {
-// // handle error
-// }
-// NOTE: as a special case, passing a list, e.g., [{"some-null-value":"", "a-non-null-value":"bar"}],
-// will be interpreted as having the root key 'object' prepended - {"object":[ ... ]} - to unmarshal to a Map.
-// See mxj/j2x/j2x_test.go.
-func NewMapJson(jsonVal []byte) (Map, error) {
- // empty or nil begets empty
- if len(jsonVal) == 0 {
- m := make(map[string]interface{}, 0)
- return m, nil
- }
- // handle a goofy case ...
- if jsonVal[0] == '[' {
- jsonVal = []byte(`{"object":` + string(jsonVal) + `}`)
- }
- m := make(map[string]interface{})
- // err := json.Unmarshal(jsonVal, &m)
- buf := bytes.NewReader(jsonVal)
- dec := json.NewDecoder(buf)
- if JsonUseNumber {
- dec.UseNumber()
- }
- err := dec.Decode(&m)
- return m, err
-}
-
-// Retrieve a Map value from an io.Reader.
-// NOTE: The raw JSON off the reader is buffered to []byte using a ByteReader. If the io.Reader is an
-// os.File, there may be significant performance impact. If the io.Reader is wrapping a []byte
-// value in-memory, however, such as http.Request.Body you CAN use it to efficiently unmarshal
-// a JSON object.
-func NewMapJsonReader(jsonReader io.Reader) (Map, error) {
- jb, err := getJson(jsonReader)
- if err != nil || len(*jb) == 0 {
- return nil, err
- }
-
- // Unmarshal the 'presumed' JSON string
- return NewMapJson(*jb)
-}
-
-// Retrieve a Map value and raw JSON - []byte - from an io.Reader.
-// NOTE: The raw JSON off the reader is buffered to []byte using a ByteReader. If the io.Reader is an
-// os.File, there may be significant performance impact. If the io.Reader is wrapping a []byte
-// value in-memory, however, such as http.Request.Body you CAN use it to efficiently unmarshal
-// a JSON object and retrieve the raw JSON in a single call.
-func NewMapJsonReaderRaw(jsonReader io.Reader) (Map, []byte, error) {
- jb, err := getJson(jsonReader)
- if err != nil || len(*jb) == 0 {
- return nil, *jb, err
- }
-
- // Unmarshal the 'presumed' JSON string
- m, merr := NewMapJson(*jb)
- return m, *jb, merr
-}
-
-// Pull the next JSON string off the stream: just read from first '{' to its closing '}'.
-// Returning a pointer to the slice saves 16 bytes - maybe unnecessary, but internal to package.
-func getJson(rdr io.Reader) (*[]byte, error) {
- bval := make([]byte, 1)
- jb := make([]byte, 0)
- var inQuote, inJson bool
- var parenCnt int
- var previous byte
-
- // scan the input for a matched set of {...}
- // json.Unmarshal will handle syntax checking.
- for {
- _, err := rdr.Read(bval)
- if err != nil {
- if err == io.EOF && inJson && parenCnt > 0 {
- return &jb, fmt.Errorf("no closing } for JSON string: %s", string(jb))
- }
- return &jb, err
- }
- switch bval[0] {
- case '{':
- if !inQuote {
- parenCnt++
- inJson = true
- }
- case '}':
- if !inQuote {
- parenCnt--
- }
- if parenCnt < 0 {
- return nil, fmt.Errorf("closing } without opening {: %s", string(jb))
- }
- case '"':
- if inQuote {
- if previous == '\\' {
- break
- }
- inQuote = false
- } else {
- inQuote = true
- }
- case '\n', '\r', '\t', ' ':
- if !inQuote {
- continue
- }
- }
- if inJson {
- jb = append(jb, bval[0])
- if parenCnt == 0 {
- break
- }
- }
- previous = bval[0]
- }
-
- return &jb, nil
-}
-
-// ------------------------------- JSON Reader handler via Map values -----------------------
-
-// Default poll delay to keep Handler from spinning on an open stream
-// like sitting on os.Stdin waiting for imput.
-var jhandlerPollInterval = time.Duration(1e6)
-
-// While unnecessary, we make HandleJsonReader() have the same signature as HandleXmlReader().
-// This avoids treating one or other as a special case and discussing the underlying stdlib logic.
-
-// Bulk process JSON using handlers that process a Map value.
-// 'rdr' is an io.Reader for the JSON (stream).
-// 'mapHandler' is the Map processing handler. Return of 'false' stops io.Reader processing.
-// 'errHandler' is the error processor. Return of 'false' stops io.Reader processing and returns the error.
-// Note: mapHandler() and errHandler() calls are blocking, so reading and processing of messages is serialized.
-// This means that you can stop reading the file on error or after processing a particular message.
-// To have reading and handling run concurrently, pass argument to a go routine in handler and return 'true'.
-func HandleJsonReader(jsonReader io.Reader, mapHandler func(Map) bool, errHandler func(error) bool) error {
- var n int
- for {
- m, merr := NewMapJsonReader(jsonReader)
- n++
-
- // handle error condition with errhandler
- if merr != nil && merr != io.EOF {
- merr = fmt.Errorf("[jsonReader: %d] %s", n, merr.Error())
- if ok := errHandler(merr); !ok {
- // caused reader termination
- return merr
- }
- continue
- }
-
- // pass to maphandler
- if len(m) != 0 {
- if ok := mapHandler(m); !ok {
- break
- }
- } else if merr != io.EOF {
- <-time.After(jhandlerPollInterval)
- }
-
- if merr == io.EOF {
- break
- }
- }
- return nil
-}
-
-// Bulk process JSON using handlers that process a Map value and the raw JSON.
-// 'rdr' is an io.Reader for the JSON (stream).
-// 'mapHandler' is the Map and raw JSON - []byte - processor. Return of 'false' stops io.Reader processing.
-// 'errHandler' is the error and raw JSON processor. Return of 'false' stops io.Reader processing and returns the error.
-// Note: mapHandler() and errHandler() calls are blocking, so reading and processing of messages is serialized.
-// This means that you can stop reading the file on error or after processing a particular message.
-// To have reading and handling run concurrently, pass argument(s) to a go routine in handler and return 'true'.
-func HandleJsonReaderRaw(jsonReader io.Reader, mapHandler func(Map, []byte) bool, errHandler func(error, []byte) bool) error {
- var n int
- for {
- m, raw, merr := NewMapJsonReaderRaw(jsonReader)
- n++
-
- // handle error condition with errhandler
- if merr != nil && merr != io.EOF {
- merr = fmt.Errorf("[jsonReader: %d] %s", n, merr.Error())
- if ok := errHandler(merr, raw); !ok {
- // caused reader termination
- return merr
- }
- continue
- }
-
- // pass to maphandler
- if len(m) != 0 {
- if ok := mapHandler(m, raw); !ok {
- break
- }
- } else if merr != io.EOF {
- <-time.After(jhandlerPollInterval)
- }
-
- if merr == io.EOF {
- break
- }
- }
- return nil
-}
diff --git a/vendor/github.com/clbanning/mxj/keyvalues.go b/vendor/github.com/clbanning/mxj/keyvalues.go
deleted file mode 100644
index 0b244c87..00000000
--- a/vendor/github.com/clbanning/mxj/keyvalues.go
+++ /dev/null
@@ -1,671 +0,0 @@
-// Copyright 2012-2014 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// keyvalues.go: Extract values from an arbitrary XML doc. Tag path can include wildcard characters.
-
-package mxj
-
-import (
- "errors"
- "fmt"
- "strconv"
- "strings"
-)
-
-// ----------------------------- get everything FOR a single key -------------------------
-
-const (
- minArraySize = 32
-)
-
-var defaultArraySize int = minArraySize
-
-// Adjust the buffers for expected number of values to return from ValuesForKey() and ValuesForPath().
-// This can have the effect of significantly reducing memory allocation-copy functions for large data sets.
-// Returns the initial buffer size.
-func SetArraySize(size int) int {
- if size > minArraySize {
- defaultArraySize = size
- } else {
- defaultArraySize = minArraySize
- }
- return defaultArraySize
-}
-
-// Return all values in Map, 'mv', associated with a 'key'. If len(returned_values) == 0, then no match.
-// On error, the returned slice is 'nil'. NOTE: 'key' can be wildcard, "*".
-// 'subkeys' (optional) are "key:val[:type]" strings representing attributes or elements in a list.
-// - By default 'val' is of type string. "key:val:bool" and "key:val:float" to coerce them.
-// - For attributes prefix the label with a hyphen, '-', e.g., "-seq:3".
-// - If the 'key' refers to a list, then "key:value" could select a list member of the list.
-// - The subkey can be wildcarded - "key:*" - to require that it's there with some value.
-// - If a subkey is preceeded with the '!' character, the key:value[:type] entry is treated as an
-// exclusion critera - e.g., "!author:William T. Gaddis".
-// - If val contains ":" symbol, use SetFieldSeparator to a unused symbol, perhaps "|".
-func (mv Map) ValuesForKey(key string, subkeys ...string) ([]interface{}, error) {
- m := map[string]interface{}(mv)
- var subKeyMap map[string]interface{}
- if len(subkeys) > 0 {
- var err error
- subKeyMap, err = getSubKeyMap(subkeys...)
- if err != nil {
- return nil, err
- }
- }
-
- ret := make([]interface{}, 0, defaultArraySize)
- var cnt int
- hasKey(m, key, &ret, &cnt, subKeyMap)
- return ret[:cnt], nil
-}
-
-var KeyNotExistError = errors.New("Key does not exist")
-
-// ValueForKey is a wrapper on ValuesForKey. It returns the first member of []interface{}, if any.
-// If there is no value, "nil, nil" is returned.
-func (mv Map) ValueForKey(key string, subkeys ...string) (interface{}, error) {
- vals, err := mv.ValuesForKey(key, subkeys...)
- if err != nil {
- return nil, err
- }
- if len(vals) == 0 {
- return nil, KeyNotExistError
- }
- return vals[0], nil
-}
-
-// hasKey - if the map 'key' exists append it to array
-// if it doesn't do nothing except scan array and map values
-func hasKey(iv interface{}, key string, ret *[]interface{}, cnt *int, subkeys map[string]interface{}) {
- // func hasKey(iv interface{}, key string, ret *[]interface{}, subkeys map[string]interface{}) {
- switch iv.(type) {
- case map[string]interface{}:
- vv := iv.(map[string]interface{})
- // see if the current value is of interest
- if v, ok := vv[key]; ok {
- switch v.(type) {
- case map[string]interface{}:
- if hasSubKeys(v, subkeys) {
- *ret = append(*ret, v)
- *cnt++
- }
- case []interface{}:
- for _, av := range v.([]interface{}) {
- if hasSubKeys(av, subkeys) {
- *ret = append(*ret, av)
- *cnt++
- }
- }
- default:
- if len(subkeys) == 0 {
- *ret = append(*ret, v)
- *cnt++
- }
- }
- }
-
- // wildcard case
- if key == "*" {
- for _, v := range vv {
- switch v.(type) {
- case map[string]interface{}:
- if hasSubKeys(v, subkeys) {
- *ret = append(*ret, v)
- *cnt++
- }
- case []interface{}:
- for _, av := range v.([]interface{}) {
- if hasSubKeys(av, subkeys) {
- *ret = append(*ret, av)
- *cnt++
- }
- }
- default:
- if len(subkeys) == 0 {
- *ret = append(*ret, v)
- *cnt++
- }
- }
- }
- }
-
- // scan the rest
- for _, v := range vv {
- hasKey(v, key, ret, cnt, subkeys)
- }
- case []interface{}:
- for _, v := range iv.([]interface{}) {
- hasKey(v, key, ret, cnt, subkeys)
- }
- }
-}
-
-// ----------------------- get everything for a node in the Map ---------------------------
-
-// Allow indexed arrays in "path" specification. (Request from Abhijit Kadam - abhijitk100@gmail.com.)
-// 2014.04.28 - implementation note.
-// Implemented as a wrapper of (old)ValuesForPath() because we need look-ahead logic to handle expansion
-// of wildcards and unindexed arrays. Embedding such logic into valuesForKeyPath() would have made the
-// code much more complicated; this wrapper is straightforward, easy to debug, and doesn't add significant overhead.
-
-// Retrieve all values for a path from the Map. If len(returned_values) == 0, then no match.
-// On error, the returned array is 'nil'.
-// 'path' is a dot-separated path of key values.
-// - If a node in the path is '*', then everything beyond is walked.
-// - 'path' can contain indexed array references, such as, "*.data[1]" and "msgs[2].data[0].field" -
-// even "*[2].*[0].field".
-// 'subkeys' (optional) are "key:val[:type]" strings representing attributes or elements in a list.
-// - By default 'val' is of type string. "key:val:bool" and "key:val:float" to coerce them.
-// - For attributes prefix the label with a hyphen, '-', e.g., "-seq:3".
-// - If the 'path' refers to a list, then "tag:value" would return member of the list.
-// - The subkey can be wildcarded - "key:*" - to require that it's there with some value.
-// - If a subkey is preceeded with the '!' character, the key:value[:type] entry is treated as an
-// exclusion critera - e.g., "!author:William T. Gaddis".
-// - If val contains ":" symbol, use SetFieldSeparator to a unused symbol, perhaps "|".
-func (mv Map) ValuesForPath(path string, subkeys ...string) ([]interface{}, error) {
- // If there are no array indexes in path, use legacy ValuesForPath() logic.
- if strings.Index(path, "[") < 0 {
- return mv.oldValuesForPath(path, subkeys...)
- }
-
- var subKeyMap map[string]interface{}
- if len(subkeys) > 0 {
- var err error
- subKeyMap, err = getSubKeyMap(subkeys...)
- if err != nil {
- return nil, err
- }
- }
-
- keys, kerr := parsePath(path)
- if kerr != nil {
- return nil, kerr
- }
-
- vals, verr := valuesForArray(keys, mv)
- if verr != nil {
- return nil, verr // Vals may be nil, but return empty array.
- }
-
- // Need to handle subkeys ... only return members of vals that satisfy conditions.
- retvals := make([]interface{}, 0)
- for _, v := range vals {
- if hasSubKeys(v, subKeyMap) {
- retvals = append(retvals, v)
- }
- }
- return retvals, nil
-}
-
-func valuesForArray(keys []*key, m Map) ([]interface{}, error) {
- var tmppath string
- var haveFirst bool
- var vals []interface{}
- var verr error
-
- lastkey := len(keys) - 1
- for i := 0; i <= lastkey; i++ {
- if !haveFirst {
- tmppath = keys[i].name
- haveFirst = true
- } else {
- tmppath += "." + keys[i].name
- }
-
- // Look-ahead: explode wildcards and unindexed arrays.
- // Need to handle un-indexed list recursively:
- // e.g., path is "stuff.data[0]" rather than "stuff[0].data[0]".
- // Need to treat it as "stuff[0].data[0]", "stuff[1].data[0]", ...
- if !keys[i].isArray && i < lastkey && keys[i+1].isArray {
- // Can't pass subkeys because we may not be at literal end of path.
- vv, vverr := m.oldValuesForPath(tmppath)
- if vverr != nil {
- return nil, vverr
- }
- for _, v := range vv {
- // See if we can walk the value.
- am, ok := v.(map[string]interface{})
- if !ok {
- continue
- }
- // Work the backend.
- nvals, nvalserr := valuesForArray(keys[i+1:], Map(am))
- if nvalserr != nil {
- return nil, nvalserr
- }
- vals = append(vals, nvals...)
- }
- break // have recursed the whole path - return
- }
-
- if keys[i].isArray || i == lastkey {
- // Don't pass subkeys because may not be at literal end of path.
- vals, verr = m.oldValuesForPath(tmppath)
- } else {
- continue
- }
- if verr != nil {
- return nil, verr
- }
-
- if i == lastkey && !keys[i].isArray {
- break
- }
-
- // Now we're looking at an array - supposedly.
- // Is index in range of vals?
- if len(vals) <= keys[i].position {
- vals = nil
- break
- }
-
- // Return the array member of interest, if at end of path.
- if i == lastkey {
- vals = vals[keys[i].position:(keys[i].position + 1)]
- break
- }
-
- // Extract the array member of interest.
- am := vals[keys[i].position:(keys[i].position + 1)]
-
- // must be a map[string]interface{} value so we can keep walking the path
- amm, ok := am[0].(map[string]interface{})
- if !ok {
- vals = nil
- break
- }
-
- m = Map(amm)
- haveFirst = false
- }
-
- return vals, nil
-}
-
-type key struct {
- name string
- isArray bool
- position int
-}
-
-func parsePath(s string) ([]*key, error) {
- keys := strings.Split(s, ".")
-
- ret := make([]*key, 0)
-
- for i := 0; i < len(keys); i++ {
- if keys[i] == "" {
- continue
- }
-
- newkey := new(key)
- if strings.Index(keys[i], "[") < 0 {
- newkey.name = keys[i]
- ret = append(ret, newkey)
- continue
- }
-
- p := strings.Split(keys[i], "[")
- newkey.name = p[0]
- p = strings.Split(p[1], "]")
- if p[0] == "" { // no right bracket
- return nil, fmt.Errorf("no right bracket on key index: %s", keys[i])
- }
- // convert p[0] to a int value
- pos, nerr := strconv.ParseInt(p[0], 10, 32)
- if nerr != nil {
- return nil, fmt.Errorf("cannot convert index to int value: %s", p[0])
- }
- newkey.position = int(pos)
- newkey.isArray = true
- ret = append(ret, newkey)
- }
-
- return ret, nil
-}
-
-// legacy ValuesForPath() - now wrapped to handle special case of indexed arrays in 'path'.
-func (mv Map) oldValuesForPath(path string, subkeys ...string) ([]interface{}, error) {
- m := map[string]interface{}(mv)
- var subKeyMap map[string]interface{}
- if len(subkeys) > 0 {
- var err error
- subKeyMap, err = getSubKeyMap(subkeys...)
- if err != nil {
- return nil, err
- }
- }
-
- keys := strings.Split(path, ".")
- if keys[len(keys)-1] == "" {
- keys = keys[:len(keys)-1]
- }
- ivals := make([]interface{}, 0, defaultArraySize)
- var cnt int
- valuesForKeyPath(&ivals, &cnt, m, keys, subKeyMap)
- return ivals[:cnt], nil
-}
-
-func valuesForKeyPath(ret *[]interface{}, cnt *int, m interface{}, keys []string, subkeys map[string]interface{}) {
- lenKeys := len(keys)
-
- // load 'm' values into 'ret'
- // expand any lists
- if lenKeys == 0 {
- switch m.(type) {
- case map[string]interface{}:
- if subkeys != nil {
- if ok := hasSubKeys(m, subkeys); !ok {
- return
- }
- }
- *ret = append(*ret, m)
- *cnt++
- case []interface{}:
- for i, v := range m.([]interface{}) {
- if subkeys != nil {
- if ok := hasSubKeys(v, subkeys); !ok {
- continue // only load list members with subkeys
- }
- }
- *ret = append(*ret, (m.([]interface{}))[i])
- *cnt++
- }
- default:
- if subkeys != nil {
- return // must be map[string]interface{} if there are subkeys
- }
- *ret = append(*ret, m)
- *cnt++
- }
- return
- }
-
- // key of interest
- key := keys[0]
- switch key {
- case "*": // wildcard - scan all values
- switch m.(type) {
- case map[string]interface{}:
- for _, v := range m.(map[string]interface{}) {
- // valuesForKeyPath(ret, v, keys[1:], subkeys)
- valuesForKeyPath(ret, cnt, v, keys[1:], subkeys)
- }
- case []interface{}:
- for _, v := range m.([]interface{}) {
- switch v.(type) {
- // flatten out a list of maps - keys are processed
- case map[string]interface{}:
- for _, vv := range v.(map[string]interface{}) {
- // valuesForKeyPath(ret, vv, keys[1:], subkeys)
- valuesForKeyPath(ret, cnt, vv, keys[1:], subkeys)
- }
- default:
- // valuesForKeyPath(ret, v, keys[1:], subkeys)
- valuesForKeyPath(ret, cnt, v, keys[1:], subkeys)
- }
- }
- }
- default: // key - must be map[string]interface{}
- switch m.(type) {
- case map[string]interface{}:
- if v, ok := m.(map[string]interface{})[key]; ok {
- // valuesForKeyPath(ret, v, keys[1:], subkeys)
- valuesForKeyPath(ret, cnt, v, keys[1:], subkeys)
- }
- case []interface{}: // may be buried in list
- for _, v := range m.([]interface{}) {
- switch v.(type) {
- case map[string]interface{}:
- if vv, ok := v.(map[string]interface{})[key]; ok {
- // valuesForKeyPath(ret, vv, keys[1:], subkeys)
- valuesForKeyPath(ret, cnt, vv, keys[1:], subkeys)
- }
- }
- }
- }
- }
-}
-
-// hasSubKeys() - interface{} equality works for string, float64, bool
-// 'v' must be a map[string]interface{} value to have subkeys
-// 'a' can have k:v pairs with v.(string) == "*", which is treated like a wildcard.
-func hasSubKeys(v interface{}, subkeys map[string]interface{}) bool {
- if len(subkeys) == 0 {
- return true
- }
-
- switch v.(type) {
- case map[string]interface{}:
- // do all subKey name:value pairs match?
- mv := v.(map[string]interface{})
- for skey, sval := range subkeys {
- isNotKey := false
- if skey[:1] == "!" { // a NOT-key
- skey = skey[1:]
- isNotKey = true
- }
- vv, ok := mv[skey]
- if !ok { // key doesn't exist
- if isNotKey { // key not there, but that's what we want
- if kv, ok := sval.(string); ok && kv == "*" {
- continue
- }
- }
- return false
- }
- // wildcard check
- if kv, ok := sval.(string); ok && kv == "*" {
- if isNotKey { // key is there, and we don't want it
- return false
- }
- continue
- }
- switch sval.(type) {
- case string:
- if s, ok := vv.(string); ok && s == sval.(string) {
- if isNotKey {
- return false
- }
- continue
- }
- case bool:
- if b, ok := vv.(bool); ok && b == sval.(bool) {
- if isNotKey {
- return false
- }
- continue
- }
- case float64:
- if f, ok := vv.(float64); ok && f == sval.(float64) {
- if isNotKey {
- return false
- }
- continue
- }
- }
- // key there but didn't match subkey value
- if isNotKey { // that's what we want
- continue
- }
- return false
- }
- // all subkeys matched
- return true
- }
-
- // not a map[string]interface{} value, can't have subkeys
- return false
-}
-
-// Generate map of key:value entries as map[string]string.
-// 'kv' arguments are "name:value" pairs: attribute keys are designated with prepended hyphen, '-'.
-// If len(kv) == 0, the return is (nil, nil).
-func getSubKeyMap(kv ...string) (map[string]interface{}, error) {
- if len(kv) == 0 {
- return nil, nil
- }
- m := make(map[string]interface{}, 0)
- for _, v := range kv {
- vv := strings.Split(v, fieldSep)
- switch len(vv) {
- case 2:
- m[vv[0]] = interface{}(vv[1])
- case 3:
- switch vv[2] {
- case "string", "char", "text":
- m[vv[0]] = interface{}(vv[1])
- case "bool", "boolean":
- // ParseBool treats "1"==true & "0"==false
- b, err := strconv.ParseBool(vv[1])
- if err != nil {
- return nil, fmt.Errorf("can't convert subkey value to bool: %s", vv[1])
- }
- m[vv[0]] = interface{}(b)
- case "float", "float64", "num", "number", "numeric":
- f, err := strconv.ParseFloat(vv[1], 64)
- if err != nil {
- return nil, fmt.Errorf("can't convert subkey value to float: %s", vv[1])
- }
- m[vv[0]] = interface{}(f)
- default:
- return nil, fmt.Errorf("unknown subkey conversion spec: %s", v)
- }
- default:
- return nil, fmt.Errorf("unknown subkey spec: %s", v)
- }
- }
- return m, nil
-}
-
-// ------------------------------- END of valuesFor ... ----------------------------
-
-// ----------------------- locate where a key value is in the tree -------------------
-
-//----------------------------- find all paths to a key --------------------------------
-
-// Get all paths through Map, 'mv', (in dot-notation) that terminate with the specified key.
-// Results can be used with ValuesForPath.
-func (mv Map) PathsForKey(key string) []string {
- m := map[string]interface{}(mv)
- breadbasket := make(map[string]bool, 0)
- breadcrumbs := ""
-
- hasKeyPath(breadcrumbs, m, key, breadbasket)
- if len(breadbasket) == 0 {
- return nil
- }
-
- // unpack map keys to return
- res := make([]string, len(breadbasket))
- var i int
- for k := range breadbasket {
- res[i] = k
- i++
- }
-
- return res
-}
-
-// Extract the shortest path from all possible paths - from PathsForKey() - in Map, 'mv'..
-// Paths are strings using dot-notation.
-func (mv Map) PathForKeyShortest(key string) string {
- paths := mv.PathsForKey(key)
-
- lp := len(paths)
- if lp == 0 {
- return ""
- }
- if lp == 1 {
- return paths[0]
- }
-
- shortest := paths[0]
- shortestLen := len(strings.Split(shortest, "."))
-
- for i := 1; i < len(paths); i++ {
- vlen := len(strings.Split(paths[i], "."))
- if vlen < shortestLen {
- shortest = paths[i]
- shortestLen = vlen
- }
- }
-
- return shortest
-}
-
-// hasKeyPath - if the map 'key' exists append it to KeyPath.path and increment KeyPath.depth
-// This is really just a breadcrumber that saves all trails that hit the prescribed 'key'.
-func hasKeyPath(crumbs string, iv interface{}, key string, basket map[string]bool) {
- switch iv.(type) {
- case map[string]interface{}:
- vv := iv.(map[string]interface{})
- if _, ok := vv[key]; ok {
- // create a new breadcrumb, intialized with the one we have
- var nbc string
- if crumbs == "" {
- nbc = key
- } else {
- nbc = crumbs + "." + key
- }
- basket[nbc] = true
- }
- // walk on down the path, key could occur again at deeper node
- for k, v := range vv {
- // create a new breadcrumb, intialized with the one we have
- var nbc string
- if crumbs == "" {
- nbc = k
- } else {
- nbc = crumbs + "." + k
- }
- hasKeyPath(nbc, v, key, basket)
- }
- case []interface{}:
- // crumb-trail doesn't change, pass it on
- for _, v := range iv.([]interface{}) {
- hasKeyPath(crumbs, v, key, basket)
- }
- }
-}
-
-var PathNotExistError = errors.New("Path does not exist")
-
-// ValueForPath wrap ValuesFor Path and returns the first value returned.
-// If no value is found it returns 'nil' and PathNotExistError.
-func (mv Map) ValueForPath(path string) (interface{}, error) {
- vals, err := mv.ValuesForPath(path)
- if err != nil {
- return nil, err
- }
- if len(vals) == 0 {
- return nil, PathNotExistError
- }
- return vals[0], nil
-}
-
-// Returns the first found value for the path as a string.
-func (mv Map) ValueForPathString(path string) (string, error) {
- vals, err := mv.ValuesForPath(path)
- if err != nil {
- return "", err
- }
- if len(vals) == 0 {
- return "", errors.New("ValueForPath: path not found")
- }
- val := vals[0]
- switch str := val.(type) {
- case string:
- return str, nil
- default:
- return "", fmt.Errorf("ValueForPath: unsupported type: %T", str)
- }
-}
-
-// Returns the first found value for the path as a string.
-// If the path is not found then it returns an empty string.
-func (mv Map) ValueOrEmptyForPathString(path string) string {
- str, _ := mv.ValueForPathString(path)
- return str
-}
diff --git a/vendor/github.com/clbanning/mxj/leafnode.go b/vendor/github.com/clbanning/mxj/leafnode.go
deleted file mode 100644
index cf413ebd..00000000
--- a/vendor/github.com/clbanning/mxj/leafnode.go
+++ /dev/null
@@ -1,112 +0,0 @@
-package mxj
-
-// leafnode.go - return leaf nodes with paths and values for the Map
-// inspired by: https://groups.google.com/forum/#!topic/golang-nuts/3JhuVKRuBbw
-
-import (
- "strconv"
- "strings"
-)
-
-const (
- NoAttributes = true // suppress LeafNode values that are attributes
-)
-
-// LeafNode - a terminal path value in a Map.
-// For XML Map values it represents an attribute or simple element value - of type
-// string unless Map was created using Cast flag. For JSON Map values it represents
-// a string, numeric, boolean, or null value.
-type LeafNode struct {
- Path string // a dot-notation representation of the path with array subscripting
- Value interface{} // the value at the path termination
-}
-
-// LeafNodes - returns an array of all LeafNode values for the Map.
-// The option no_attr argument suppresses attribute values (keys with prepended hyphen, '-')
-// as well as the "#text" key for the associated simple element value.
-//
-// PrependAttrWithHypen(false) will result in attributes having .attr-name as
-// terminal node in 'path' while the path for the element value, itself, will be
-// the base path w/o "#text".
-//
-// LeafUseDotNotation(true) causes list members to be identified using ".N" syntax
-// rather than "[N]" syntax.
-func (mv Map) LeafNodes(no_attr ...bool) []LeafNode {
- var a bool
- if len(no_attr) == 1 {
- a = no_attr[0]
- }
-
- l := make([]LeafNode, 0)
- getLeafNodes("", "", map[string]interface{}(mv), &l, a)
- return l
-}
-
-func getLeafNodes(path, node string, mv interface{}, l *[]LeafNode, noattr bool) {
- // if stripping attributes, then also strip "#text" key
- if !noattr || node != "#text" {
- if path != "" && node[:1] != "[" {
- path += "."
- }
- path += node
- }
- switch mv.(type) {
- case map[string]interface{}:
- for k, v := range mv.(map[string]interface{}) {
- // if noattr && k[:1] == "-" {
- if noattr && len(attrPrefix) > 0 && strings.Index(k, attrPrefix) == 0 {
- continue
- }
- getLeafNodes(path, k, v, l, noattr)
- }
- case []interface{}:
- for i, v := range mv.([]interface{}) {
- if useDotNotation {
- getLeafNodes(path, strconv.Itoa(i), v, l, noattr)
- } else {
- getLeafNodes(path, "["+strconv.Itoa(i)+"]", v, l, noattr)
- }
- }
- default:
- // can't walk any further, so create leaf
- n := LeafNode{path, mv}
- *l = append(*l, n)
- }
-}
-
-// LeafPaths - all paths that terminate in LeafNode values.
-func (mv Map) LeafPaths(no_attr ...bool) []string {
- ln := mv.LeafNodes()
- ss := make([]string, len(ln))
- for i := 0; i < len(ln); i++ {
- ss[i] = ln[i].Path
- }
- return ss
-}
-
-// LeafValues - all terminal values in the Map.
-func (mv Map) LeafValues(no_attr ...bool) []interface{} {
- ln := mv.LeafNodes()
- vv := make([]interface{}, len(ln))
- for i := 0; i < len(ln); i++ {
- vv[i] = ln[i].Value
- }
- return vv
-}
-
-// ====================== utilities ======================
-
-// https://groups.google.com/forum/#!topic/golang-nuts/pj0C5IrZk4I
-var useDotNotation bool
-
-// LeafUseDotNotation sets a flag that list members in LeafNode paths
-// should be identified using ".N" syntax rather than the default "[N]"
-// syntax. Calling LeafUseDotNotation with no arguments toggles the
-// flag on/off; otherwise, the argument sets the flag value 'true'/'false'.
-func LeafUseDotNotation(b ...bool) {
- if len(b) == 0 {
- useDotNotation = !useDotNotation
- return
- }
- useDotNotation = b[0]
-}
diff --git a/vendor/github.com/clbanning/mxj/misc.go b/vendor/github.com/clbanning/mxj/misc.go
deleted file mode 100644
index 5b4fab21..00000000
--- a/vendor/github.com/clbanning/mxj/misc.go
+++ /dev/null
@@ -1,86 +0,0 @@
-// Copyright 2016 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// misc.go - mimic functions (+others) called out in:
-// https://groups.google.com/forum/#!topic/golang-nuts/jm_aGsJNbdQ
-// Primarily these methods let you retrive XML structure information.
-
-package mxj
-
-import (
- "fmt"
- "sort"
- "strings"
-)
-
-// Return the root element of the Map. If there is not a single key in Map,
-// then an error is returned.
-func (mv Map) Root() (string, error) {
- mm := map[string]interface{}(mv)
- if len(mm) != 1 {
- return "", fmt.Errorf("Map does not have singleton root. Len: %d.", len(mm))
- }
- for k, _ := range mm {
- return k, nil
- }
- return "", nil
-}
-
-// If the path is an element with sub-elements, return a list of the sub-element
-// keys. (The list is alphabeticly sorted.) NOTE: Map keys that are prefixed with
-// '-', a hyphen, are considered attributes; see m.Attributes(path).
-func (mv Map) Elements(path string) ([]string, error) {
- e, err := mv.ValueForPath(path)
- if err != nil {
- return nil, err
- }
- switch e.(type) {
- case map[string]interface{}:
- ee := e.(map[string]interface{})
- elems := make([]string, len(ee))
- var i int
- for k, _ := range ee {
- if len(attrPrefix) > 0 && strings.Index(k, attrPrefix) == 0 {
- continue // skip attributes
- }
- elems[i] = k
- i++
- }
- elems = elems[:i]
- // alphabetic sort keeps things tidy
- sort.Strings(elems)
- return elems, nil
- }
- return nil, fmt.Errorf("no elements for path: %s", path)
-}
-
-// If the path is an element with attributes, return a list of the attribute
-// keys. (The list is alphabeticly sorted.) NOTE: Map keys that are not prefixed with
-// '-', a hyphen, are not treated as attributes; see m.Elements(path). Also, if the
-// attribute prefix is "" - SetAttrPrefix("") or PrependAttrWithHyphen(false) - then
-// there are no identifiable attributes.
-func (mv Map) Attributes(path string) ([]string, error) {
- a, err := mv.ValueForPath(path)
- if err != nil {
- return nil, err
- }
- switch a.(type) {
- case map[string]interface{}:
- aa := a.(map[string]interface{})
- attrs := make([]string, len(aa))
- var i int
- for k, _ := range aa {
- if len(attrPrefix) == 0 || strings.Index(k, attrPrefix) != 0 {
- continue // skip non-attributes
- }
- attrs[i] = k[len(attrPrefix):]
- i++
- }
- attrs = attrs[:i]
- // alphabetic sort keeps things tidy
- sort.Strings(attrs)
- return attrs, nil
- }
- return nil, fmt.Errorf("no attributes for path: %s", path)
-}
diff --git a/vendor/github.com/clbanning/mxj/mxj.go b/vendor/github.com/clbanning/mxj/mxj.go
deleted file mode 100644
index f0592f06..00000000
--- a/vendor/github.com/clbanning/mxj/mxj.go
+++ /dev/null
@@ -1,128 +0,0 @@
-// mxj - A collection of map[string]interface{} and associated XML and JSON utilities.
-// Copyright 2012-2014 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-package mxj
-
-import (
- "fmt"
- "sort"
-)
-
-const (
- Cast = true // for clarity - e.g., mxj.NewMapXml(doc, mxj.Cast)
- SafeEncoding = true // ditto - e.g., mv.Json(mxj.SafeEncoding)
-)
-
-type Map map[string]interface{}
-
-// Allocate a Map.
-func New() Map {
- m := make(map[string]interface{}, 0)
- return m
-}
-
-// Cast a Map to map[string]interface{}
-func (mv Map) Old() map[string]interface{} {
- return mv
-}
-
-// Return a copy of mv as a newly allocated Map. If the Map only contains string,
-// numeric, map[string]interface{}, and []interface{} values, then it can be thought
-// of as a "deep copy." Copying a structure (or structure reference) value is subject
-// to the noted restrictions.
-// NOTE: If 'mv' includes structure values with, possibly, JSON encoding tags
-// then only public fields of the structure are in the new Map - and with
-// keys that conform to any encoding tag instructions. The structure itself will
-// be represented as a map[string]interface{} value.
-func (mv Map) Copy() (Map, error) {
- // this is the poor-man's deep copy
- // not efficient, but it works
- j, jerr := mv.Json()
- // must handle, we don't know how mv got built
- if jerr != nil {
- return nil, jerr
- }
- return NewMapJson(j)
-}
-
-// --------------- StringIndent ... from x2j.WriteMap -------------
-
-// Pretty print a Map.
-func (mv Map) StringIndent(offset ...int) string {
- return writeMap(map[string]interface{}(mv), true, true, offset...)
-}
-
-// Pretty print a Map without the value type information - just key:value entries.
-func (mv Map) StringIndentNoTypeInfo(offset ...int) string {
- return writeMap(map[string]interface{}(mv), false, true, offset...)
-}
-
-// writeMap - dumps the map[string]interface{} for examination.
-// 'typeInfo' causes value type to be printed.
-// 'offset' is initial indentation count; typically: Write(m).
-func writeMap(m interface{}, typeInfo, root bool, offset ...int) string {
- var indent int
- if len(offset) == 1 {
- indent = offset[0]
- }
-
- var s string
- switch m.(type) {
- case []interface{}:
- if typeInfo {
- s += "[[]interface{}]"
- }
- for _, v := range m.([]interface{}) {
- s += "\n"
- for i := 0; i < indent; i++ {
- s += " "
- }
- s += writeMap(v, typeInfo, false, indent+1)
- }
- case map[string]interface{}:
- list := make([][2]string, len(m.(map[string]interface{})))
- var n int
- for k, v := range m.(map[string]interface{}) {
- list[n][0] = k
- list[n][1] = writeMap(v, typeInfo, false, indent+1)
- n++
- }
- sort.Sort(mapList(list))
- for _, v := range list {
- if root {
- root = false
- } else {
- s += "\n"
- }
- for i := 0; i < indent; i++ {
- s += " "
- }
- s += v[0] + " : " + v[1]
- }
- default:
- if typeInfo {
- s += fmt.Sprintf("[%T] %+v", m, m)
- } else {
- s += fmt.Sprintf("%+v", m)
- }
- }
- return s
-}
-
-// ======================== utility ===============
-
-type mapList [][2]string
-
-func (ml mapList) Len() int {
- return len(ml)
-}
-
-func (ml mapList) Swap(i, j int) {
- ml[i], ml[j] = ml[j], ml[i]
-}
-
-func (ml mapList) Less(i, j int) bool {
- return ml[i][0] <= ml[j][0]
-}
diff --git a/vendor/github.com/clbanning/mxj/newmap.go b/vendor/github.com/clbanning/mxj/newmap.go
deleted file mode 100644
index b2939490..00000000
--- a/vendor/github.com/clbanning/mxj/newmap.go
+++ /dev/null
@@ -1,184 +0,0 @@
-// mxj - A collection of map[string]interface{} and associated XML and JSON utilities.
-// Copyright 2012-2014, 2018 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// remap.go - build a new Map from the current Map based on keyOld:keyNew mapppings
-// keys can use dot-notation, keyOld can use wildcard, '*'
-//
-// Computational strategy -
-// Using the key path - []string - traverse a new map[string]interface{} and
-// insert the oldVal as the newVal when we arrive at the end of the path.
-// If the type at the end is nil, then that is newVal
-// If the type at the end is a singleton (string, float64, bool) an array is created.
-// If the type at the end is an array, newVal is just appended.
-// If the type at the end is a map, it is inserted if possible or the map value
-// is converted into an array if necessary.
-
-package mxj
-
-import (
- "errors"
- "strings"
-)
-
-// (Map)NewMap - create a new Map from data in the current Map.
-// 'keypairs' are key mappings "oldKey:newKey" and specify that the current value of 'oldKey'
-// should be the value for 'newKey' in the returned Map.
-// - 'oldKey' supports dot-notation as described for (Map)ValuesForPath()
-// - 'newKey' supports dot-notation but with no wildcards, '*', or indexed arrays
-// - "oldKey" is shorthand for the keypair value "oldKey:oldKey"
-// - "oldKey:" and ":newKey" are invalid keypair values
-// - if 'oldKey' does not exist in the current Map, it is not written to the new Map.
-// "null" is not supported unless it is the current Map.
-// - see newmap_test.go for several syntax examples
-// - mv.NewMap() == mxj.New()
-//
-// NOTE: "examples/partial.go" shows how to create arbitrary sub-docs of an XML doc.
-func (mv Map) NewMap(keypairs ...string) (Map, error) {
- n := make(map[string]interface{}, 0)
- if len(keypairs) == 0 {
- return n, nil
- }
-
- // loop through the pairs
- var oldKey, newKey string
- var path []string
- for _, v := range keypairs {
- if len(v) == 0 {
- continue // just skip over empty keypair arguments
- }
-
- // initialize oldKey, newKey and check
- vv := strings.Split(v, ":")
- if len(vv) > 2 {
- return n, errors.New("oldKey:newKey keypair value not valid - " + v)
- }
- if len(vv) == 1 {
- oldKey, newKey = vv[0], vv[0]
- } else {
- oldKey, newKey = vv[0], vv[1]
- }
- strings.TrimSpace(oldKey)
- strings.TrimSpace(newKey)
- if i := strings.Index(newKey, "*"); i > -1 {
- return n, errors.New("newKey value cannot contain wildcard character - " + v)
- }
- if i := strings.Index(newKey, "["); i > -1 {
- return n, errors.New("newKey value cannot contain indexed arrays - " + v)
- }
- if oldKey == "" || newKey == "" {
- return n, errors.New("oldKey or newKey is not specified - " + v)
- }
-
- // get oldKey value
- oldVal, err := mv.ValuesForPath(oldKey)
- if err != nil {
- return n, err
- }
- if len(oldVal) == 0 {
- continue // oldKey has no value, may not exist in mv
- }
-
- // break down path
- path = strings.Split(newKey, ".")
- if path[len(path)-1] == "" { // ignore a trailing dot in newKey spec
- path = path[:len(path)-1]
- }
-
- addNewVal(&n, path, oldVal)
- }
-
- return n, nil
-}
-
-// navigate 'n' to end of path and add val
-func addNewVal(n *map[string]interface{}, path []string, val []interface{}) {
- // newVal - either singleton or array
- var newVal interface{}
- if len(val) == 1 {
- newVal = val[0] // is type interface{}
- } else {
- newVal = interface{}(val)
- }
-
- // walk to the position of interest, create it if necessary
- m := (*n) // initialize map walker
- var k string // key for m
- lp := len(path) - 1 // when to stop looking
- for i := 0; i < len(path); i++ {
- k = path[i]
- if i == lp {
- break
- }
- var nm map[string]interface{} // holds position of next-map
- switch m[k].(type) {
- case nil: // need a map for next node in path, so go there
- nm = make(map[string]interface{}, 0)
- m[k] = interface{}(nm)
- m = m[k].(map[string]interface{})
- case map[string]interface{}:
- // OK - got somewhere to walk to, go there
- m = m[k].(map[string]interface{})
- case []interface{}:
- // add a map and nm points to new map unless there's already
- // a map in the array, then nm points there
- // The placement of the next value in the array is dependent
- // on the sequence of members - could land on a map or a nil
- // value first. TODO: how to test this.
- a := make([]interface{}, 0)
- var foundmap bool
- for _, vv := range m[k].([]interface{}) {
- switch vv.(type) {
- case nil: // doesn't appear that this occurs, need a test case
- if foundmap { // use the first one in array
- a = append(a, vv)
- continue
- }
- nm = make(map[string]interface{}, 0)
- a = append(a, interface{}(nm))
- foundmap = true
- case map[string]interface{}:
- if foundmap { // use the first one in array
- a = append(a, vv)
- continue
- }
- nm = vv.(map[string]interface{})
- a = append(a, vv)
- foundmap = true
- default:
- a = append(a, vv)
- }
- }
- // no map found in array
- if !foundmap {
- nm = make(map[string]interface{}, 0)
- a = append(a, interface{}(nm))
- }
- m[k] = interface{}(a) // must insert in map
- m = nm
- default: // it's a string, float, bool, etc.
- aa := make([]interface{}, 0)
- nm = make(map[string]interface{}, 0)
- aa = append(aa, m[k], nm)
- m[k] = interface{}(aa)
- m = nm
- }
- }
-
- // value is nil, array or a singleton of some kind
- // initially m.(type) == map[string]interface{}
- v := m[k]
- switch v.(type) {
- case nil: // initialized
- m[k] = newVal
- case []interface{}:
- a := m[k].([]interface{})
- a = append(a, newVal)
- m[k] = interface{}(a)
- default: // v exists:string, float64, bool, map[string]interface, etc.
- a := make([]interface{}, 0)
- a = append(a, v, newVal)
- m[k] = interface{}(a)
- }
-}
diff --git a/vendor/github.com/clbanning/mxj/readme.md b/vendor/github.com/clbanning/mxj/readme.md
deleted file mode 100644
index 6bb21dca..00000000
--- a/vendor/github.com/clbanning/mxj/readme.md
+++ /dev/null
@@ -1,179 +0,0 @@
-mxj - to/from maps, XML and JSON
-Decode/encode XML to/from map[string]interface{} (or JSON) values, and extract/modify values from maps by key or key-path, including wildcards.
-
-mxj supplants the legacy x2j and j2x packages. If you want the old syntax, use mxj/x2j and mxj/j2x packages.
-
-Related Packages
-
-https://github.com/clbanning/checkxml provides functions for validating XML data.
-
-Refactor Decoder - 2015.11.15
-For over a year I've wanted to refactor the XML-to-map[string]interface{} decoder to make it more performant. I recently took the time to do that, since we were using github.com/clbanning/mxj in a production system that could be deployed on a Raspberry Pi. Now the decoder is comparable to the stdlib JSON-to-map[string]interface{} decoder in terms of its additional processing overhead relative to decoding to a structure value. As shown by:
-
- BenchmarkNewMapXml-4 100000 18043 ns/op
- BenchmarkNewStructXml-4 100000 14892 ns/op
- BenchmarkNewMapJson-4 300000 4633 ns/op
- BenchmarkNewStructJson-4 300000 3427 ns/op
- BenchmarkNewMapXmlBooks-4 20000 82850 ns/op
- BenchmarkNewStructXmlBooks-4 20000 67822 ns/op
- BenchmarkNewMapJsonBooks-4 100000 17222 ns/op
- BenchmarkNewStructJsonBooks-4 100000 15309 ns/op
-
-Notices
-
- 2018.04.18: mv.Xml/mv.XmlIndent encodes non-map[string]interface{} map values - map[string]string, map[int]uint, etc.
- 2018.03.29: mv.Gob/NewMapGob support gob encoding/decoding of Maps.
- 2018.03.26: Added mxj/x2j-wrapper sub-package for migrating from legacy x2j package.
- 2017.02.22: LeafNode paths can use ".N" syntax rather than "[N]" for list member indexing.
- 2017.02.10: SetFieldSeparator changes field separator for args in UpdateValuesForPath, ValuesFor... methods.
- 2017.02.06: Support XMPP stream processing - HandleXMPPStreamTag().
- 2016.11.07: Preserve name space prefix syntax in XmlSeq parser - NewMapXmlSeq(), etc.
- 2016.06.25: Support overriding default XML attribute prefix, "-", in Map keys - SetAttrPrefix().
- 2016.05.26: Support customization of xml.Decoder by exposing CustomDecoder variable.
- 2016.03.19: Escape invalid chars when encoding XML attribute and element values - XMLEscapeChars().
- 2016.03.02: By default decoding XML with float64 and bool value casting will not cast "NaN", "Inf", and "-Inf".
- To cast them to float64, first set flag with CastNanInf(true).
- 2016.02.22: New mv.Root(), mv.Elements(), mv.Attributes methods let you examine XML document structure.
- 2016.02.16: Add CoerceKeysToLower() option to handle tags with mixed capitalization.
- 2016.02.12: Seek for first xml.StartElement token; only return error if io.EOF is reached first (handles BOM).
- 2015.12.02: XML decoding/encoding that preserves original structure of document. See NewMapXmlSeq()
- and mv.XmlSeq() / mv.XmlSeqIndent().
- 2015-05-20: New: mv.StringIndentNoTypeInfo().
- Also, alphabetically sort map[string]interface{} values by key to prettify output for mv.Xml(),
- mv.XmlIndent(), mv.StringIndent(), mv.StringIndentNoTypeInfo().
- 2014-11-09: IncludeTagSeqNum() adds "_seq" key with XML doc positional information.
- (NOTE: PreserveXmlList() is similar and will be here soon.)
- 2014-09-18: inspired by NYTimes fork, added PrependAttrWithHyphen() to allow stripping hyphen from attribute tag.
- 2014-08-02: AnyXml() and AnyXmlIndent() will try to marshal arbitrary values to XML.
- 2014-04-28: ValuesForPath() and NewMap() now accept path with indexed array references.
-
-Basic Unmarshal XML to map[string]interface{}
-type Map map[string]interface{}
-
-Create a `Map` value, 'mv', from any `map[string]interface{}` value, 'v':
-mv := Map(v)
-
-Unmarshal / marshal XML as a `Map` value, 'mv':
-mv, err := NewMapXml(xmlValue) // unmarshal
-xmlValue, err := mv.Xml() // marshal
-
-Unmarshal XML from an `io.Reader` as a `Map` value, 'mv':
-mv, err := NewMapXmlReader(xmlReader) // repeated calls, as with an os.File Reader, will process stream
-mv, raw, err := NewMapXmlReaderRaw(xmlReader) // 'raw' is the raw XML that was decoded
-
-Marshal `Map` value, 'mv', to an XML Writer (`io.Writer`):
-err := mv.XmlWriter(xmlWriter)
-raw, err := mv.XmlWriterRaw(xmlWriter) // 'raw' is the raw XML that was written on xmlWriter
-
-Also, for prettified output:
-xmlValue, err := mv.XmlIndent(prefix, indent, ...)
-err := mv.XmlIndentWriter(xmlWriter, prefix, indent, ...)
-raw, err := mv.XmlIndentWriterRaw(xmlWriter, prefix, indent, ...)
-
-Bulk process XML with error handling (note: handlers must return a boolean value):
-err := HandleXmlReader(xmlReader, mapHandler(Map), errHandler(error))
-err := HandleXmlReaderRaw(xmlReader, mapHandler(Map, []byte), errHandler(error, []byte))
-
-Converting XML to JSON: see Examples for `NewMapXml` and `HandleXmlReader`.
-
-There are comparable functions and methods for JSON processing.
-
-Arbitrary structure values can be decoded to / encoded from `Map` values:
-mv, err := NewMapStruct(structVal)
-err := mv.Struct(structPointer)
-
-Extract / modify Map values
-To work with XML tag values, JSON or Map key values or structure field values, decode the XML, JSON
-or structure to a `Map` value, 'mv', or cast a `map[string]interface{}` value to a `Map` value, 'mv', then:
-paths := mv.PathsForKey(key)
-path := mv.PathForKeyShortest(key)
-values, err := mv.ValuesForKey(key, subkeys)
-values, err := mv.ValuesForPath(path, subkeys)
-count, err := mv.UpdateValuesForPath(newVal, path, subkeys)
-
-Get everything at once, irrespective of path depth:
-leafnodes := mv.LeafNodes()
-leafvalues := mv.LeafValues()
-
-A new `Map` with whatever keys are desired can be created from the current `Map` and then encoded in XML
-or JSON. (Note: keys can use dot-notation.)
-newMap, err := mv.NewMap("oldKey_1:newKey_1", "oldKey_2:newKey_2", ..., "oldKey_N:newKey_N")
-newMap, err := mv.NewMap("oldKey1", "oldKey3", "oldKey5") // a subset of 'mv'; see "examples/partial.go"
-newXml, err := newMap.Xml() // for example
-newJson, err := newMap.Json() // ditto
-
-Usage
-
-The package is fairly well [self-documented with examples](http://godoc.org/github.com/clbanning/mxj).
-
-Also, the subdirectory "examples" contains a wide range of examples, several taken from golang-nuts discussions.
-
-XML parsing conventions
-
-Using NewMapXml()
-
- - Attributes are parsed to `map[string]interface{}` values by prefixing a hyphen, `-`,
- to the attribute label. (Unless overridden by `PrependAttrWithHyphen(false)` or
- `SetAttrPrefix()`.)
- - If the element is a simple element and has attributes, the element value
- is given the key `#text` for its `map[string]interface{}` representation. (See
- the 'atomFeedString.xml' test data, below.)
- - XML comments, directives, and process instructions are ignored.
- - If CoerceKeysToLower() has been called, then the resultant keys will be lower case.
-
-Using NewMapXmlSeq()
-
- - Attributes are parsed to `map["#attr"]map[]map[string]interface{}`values
- where the `` value has "#text" and "#seq" keys - the "#text" key holds the
- value for ``.
- - All elements, except for the root, have a "#seq" key.
- - Comments, directives, and process instructions are unmarshalled into the Map using the
- keys "#comment", "#directive", and "#procinst", respectively. (See documentation for more
- specifics.)
- - Name space syntax is preserved:
- - `something` parses to `map["ns:key"]interface{}{"something"}`
- - `xmlns:ns="http://myns.com/ns"` parses to `map["xmlns:ns"]interface{}{"http://myns.com/ns"}`
-
-Both
-
- - By default, "Nan", "Inf", and "-Inf" values are not cast to float64. If you want them
- to be cast, set a flag to cast them using CastNanInf(true).
-
-XML encoding conventions
-
- - 'nil' `Map` values, which may represent 'null' JSON values, are encoded as ``.
- NOTE: the operation is not symmetric as `` elements are decoded as `tag:""` `Map` values,
- which, then, encode in JSON as `"tag":""` values.
- - ALSO: there is no guarantee that the encoded XML doc will be the same as the decoded one. (Go
- randomizes the walk through map[string]interface{} values.) If you plan to re-encode the
- Map value to XML and want the same sequencing of elements look at NewMapXmlSeq() and
- mv.XmlSeq() - these try to preserve the element sequencing but with added complexity when
- working with the Map representation.
-
-Running "go test"
-
-Because there are no guarantees on the sequence map elements are retrieved, the tests have been
-written for visual verification in most cases. One advantage is that you can easily use the
-output from running "go test" as examples of calling the various functions and methods.
-
-Motivation
-
-I make extensive use of JSON for messaging and typically unmarshal the messages into
-`map[string]interface{}` values. This is easily done using `json.Unmarshal` from the
-standard Go libraries. Unfortunately, many legacy solutions use structured
-XML messages; in those environments the applications would have to be refactored to
-interoperate with my components.
-
-The better solution is to just provide an alternative HTTP handler that receives
-XML messages and parses it into a `map[string]interface{}` value and then reuse
-all the JSON-based code. The Go `xml.Unmarshal()` function does not provide the same
-option of unmarshaling XML messages into `map[string]interface{}` values. So I wrote
-a couple of small functions to fill this gap and released them as the x2j package.
-
-Over the next year and a half additional features were added, and the companion j2x
-package was released to address XML encoding of arbitrary JSON and `map[string]interface{}`
-values. As part of a refactoring of our production system and looking at how we had been
-using the x2j and j2x packages we found that we rarely performed direct XML-to-JSON or
-JSON-to_XML conversion and that working with the XML or JSON as `map[string]interface{}`
-values was the primary value. Thus, everything was refactored into the mxj package.
-
diff --git a/vendor/github.com/clbanning/mxj/remove.go b/vendor/github.com/clbanning/mxj/remove.go
deleted file mode 100644
index 8362ab17..00000000
--- a/vendor/github.com/clbanning/mxj/remove.go
+++ /dev/null
@@ -1,37 +0,0 @@
-package mxj
-
-import "strings"
-
-// Removes the path.
-func (mv Map) Remove(path string) error {
- m := map[string]interface{}(mv)
- return remove(m, path)
-}
-
-func remove(m interface{}, path string) error {
- val, err := prevValueByPath(m, path)
- if err != nil {
- return err
- }
-
- lastKey := lastKey(path)
- delete(val, lastKey)
-
- return nil
-}
-
-// returns the last key of the path.
-// lastKey("a.b.c") would had returned "c"
-func lastKey(path string) string {
- keys := strings.Split(path, ".")
- key := keys[len(keys)-1]
- return key
-}
-
-// returns the path without the last key
-// parentPath("a.b.c") whould had returned "a.b"
-func parentPath(path string) string {
- keys := strings.Split(path, ".")
- parentPath := strings.Join(keys[0:len(keys)-1], ".")
- return parentPath
-}
diff --git a/vendor/github.com/clbanning/mxj/rename.go b/vendor/github.com/clbanning/mxj/rename.go
deleted file mode 100644
index e95a9639..00000000
--- a/vendor/github.com/clbanning/mxj/rename.go
+++ /dev/null
@@ -1,54 +0,0 @@
-package mxj
-
-import (
- "errors"
- "strings"
-)
-
-// RenameKey renames a key in a Map.
-// It works only for nested maps. It doesn't work for cases when it buried in a list.
-func (mv Map) RenameKey(path string, newName string) error {
- if !mv.Exists(path) {
- return errors.New("RenameKey: path not found: " + path)
- }
- if mv.Exists(parentPath(path) + "." + newName) {
- return errors.New("RenameKey: key already exists: " + newName)
- }
-
- m := map[string]interface{}(mv)
- return renameKey(m, path, newName)
-}
-
-func renameKey(m interface{}, path string, newName string) error {
- val, err := prevValueByPath(m, path)
- if err != nil {
- return err
- }
-
- oldName := lastKey(path)
- val[newName] = val[oldName]
- delete(val, oldName)
-
- return nil
-}
-
-// returns a value which contains a last key in the path
-// For example: prevValueByPath("a.b.c", {a{b{c: 3}}}) returns {c: 3}
-func prevValueByPath(m interface{}, path string) (map[string]interface{}, error) {
- keys := strings.Split(path, ".")
-
- switch mValue := m.(type) {
- case map[string]interface{}:
- for key, value := range mValue {
- if key == keys[0] {
- if len(keys) == 1 {
- return mValue, nil
- } else {
- // keep looking for the full path to the key
- return prevValueByPath(value, strings.Join(keys[1:], "."))
- }
- }
- }
- }
- return nil, errors.New("prevValueByPath: didn't find path – " + path)
-}
diff --git a/vendor/github.com/clbanning/mxj/set.go b/vendor/github.com/clbanning/mxj/set.go
deleted file mode 100644
index a297fc38..00000000
--- a/vendor/github.com/clbanning/mxj/set.go
+++ /dev/null
@@ -1,26 +0,0 @@
-package mxj
-
-import (
- "strings"
-)
-
-// Sets the value for the path
-func (mv Map) SetValueForPath(value interface{}, path string) error {
- pathAry := strings.Split(path, ".")
- parentPathAry := pathAry[0 : len(pathAry)-1]
- parentPath := strings.Join(parentPathAry, ".")
-
- val, err := mv.ValueForPath(parentPath)
- if err != nil {
- return err
- }
- if val == nil {
- return nil // we just ignore the request if there's no val
- }
-
- key := pathAry[len(pathAry)-1]
- cVal := val.(map[string]interface{})
- cVal[key] = value
-
- return nil
-}
diff --git a/vendor/github.com/clbanning/mxj/setfieldsep.go b/vendor/github.com/clbanning/mxj/setfieldsep.go
deleted file mode 100644
index b70715eb..00000000
--- a/vendor/github.com/clbanning/mxj/setfieldsep.go
+++ /dev/null
@@ -1,20 +0,0 @@
-package mxj
-
-// Per: https://github.com/clbanning/mxj/issues/37#issuecomment-278651862
-var fieldSep string = ":"
-
-// SetFieldSeparator changes the default field separator, ":", for the
-// newVal argument in mv.UpdateValuesForPath and the optional 'subkey' arguments
-// in mv.ValuesForKey and mv.ValuesForPath.
-//
-// E.g., if the newVal value is "http://blah/blah", setting the field separator
-// to "|" will allow the newVal specification, "|http://blah/blah" to parse
-// properly. If called with no argument or an empty string value, the field
-// separator is set to the default, ":".
-func SetFieldSeparator(s ...string) {
- if len(s) == 0 || s[0] == "" {
- fieldSep = ":" // the default
- return
- }
- fieldSep = s[0]
-}
diff --git a/vendor/github.com/clbanning/mxj/songtext.xml b/vendor/github.com/clbanning/mxj/songtext.xml
deleted file mode 100644
index 8c0f2bec..00000000
--- a/vendor/github.com/clbanning/mxj/songtext.xml
+++ /dev/null
@@ -1,29 +0,0 @@
-
- help me!
-
-
-
- Henry was a renegade
- Didn't like to play it safe
- One component at a time
- There's got to be a better way
- Oh, people came from miles around
- Searching for a steady job
- Welcome to the Motor Town
- Booming like an atom bomb
-
-
- Oh, Henry was the end of the story
- Then everything went wrong
- And we'll return it to its former glory
- But it just takes so long
-
-
-
- It's going to take a long time
- It's going to take it, but we'll make it one day
- It's going to take a long time
- It's going to take it, but we'll make it one day
-
-
-
diff --git a/vendor/github.com/clbanning/mxj/strict.go b/vendor/github.com/clbanning/mxj/strict.go
deleted file mode 100644
index 1e769560..00000000
--- a/vendor/github.com/clbanning/mxj/strict.go
+++ /dev/null
@@ -1,30 +0,0 @@
-// Copyright 2016 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// strict.go actually addresses setting xml.Decoder attribute
-// values. This'll let you parse non-standard XML.
-
-package mxj
-
-import (
- "encoding/xml"
-)
-
-// CustomDecoder can be used to specify xml.Decoder attribute
-// values, e.g., Strict:false, to be used. By default CustomDecoder
-// is nil. If CustomeDecoder != nil, then mxj.XmlCharsetReader variable is
-// ignored and must be set as part of the CustomDecoder value, if needed.
-// Usage:
-// mxj.CustomDecoder = &xml.Decoder{Strict:false}
-var CustomDecoder *xml.Decoder
-
-// useCustomDecoder copy over public attributes from customDecoder
-func useCustomDecoder(d *xml.Decoder) {
- d.Strict = CustomDecoder.Strict
- d.AutoClose = CustomDecoder.AutoClose
- d.Entity = CustomDecoder.Entity
- d.CharsetReader = CustomDecoder.CharsetReader
- d.DefaultSpace = CustomDecoder.DefaultSpace
-}
-
diff --git a/vendor/github.com/clbanning/mxj/struct.go b/vendor/github.com/clbanning/mxj/struct.go
deleted file mode 100644
index 9be636cd..00000000
--- a/vendor/github.com/clbanning/mxj/struct.go
+++ /dev/null
@@ -1,54 +0,0 @@
-// Copyright 2012-2017 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-package mxj
-
-import (
- "encoding/json"
- "errors"
- "reflect"
-
- // "github.com/fatih/structs"
-)
-
-// Create a new Map value from a structure. Error returned if argument is not a structure.
-// Only public structure fields are decoded in the Map value. See github.com/fatih/structs#Map
-// for handling of "structs" tags.
-
-// DEPRECATED - import github.com/fatih/structs and cast result of structs.Map to mxj.Map.
-// import "github.com/fatih/structs"
-// ...
-// sm, err := structs.Map()
-// if err != nil {
-// // handle error
-// }
-// m := mxj.Map(sm)
-// Alernatively uncomment the old source and import in struct.go.
-func NewMapStruct(structVal interface{}) (Map, error) {
- return nil, errors.New("deprecated - see package documentation")
- /*
- if !structs.IsStruct(structVal) {
- return nil, errors.New("NewMapStruct() error: argument is not type Struct")
- }
- return structs.Map(structVal), nil
- */
-}
-
-// Marshal a map[string]interface{} into a structure referenced by 'structPtr'. Error returned
-// if argument is not a pointer or if json.Unmarshal returns an error.
-// json.Unmarshal structure encoding rules are followed to encode public structure fields.
-func (mv Map) Struct(structPtr interface{}) error {
- // should check that we're getting a pointer.
- if reflect.ValueOf(structPtr).Kind() != reflect.Ptr {
- return errors.New("mv.Struct() error: argument is not type Ptr")
- }
-
- m := map[string]interface{}(mv)
- j, err := json.Marshal(m)
- if err != nil {
- return err
- }
-
- return json.Unmarshal(j, structPtr)
-}
diff --git a/vendor/github.com/clbanning/mxj/updatevalues.go b/vendor/github.com/clbanning/mxj/updatevalues.go
deleted file mode 100644
index 46779f4f..00000000
--- a/vendor/github.com/clbanning/mxj/updatevalues.go
+++ /dev/null
@@ -1,256 +0,0 @@
-// Copyright 2012-2014, 2017 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// updatevalues.go - modify a value based on path and possibly sub-keys
-// TODO(clb): handle simple elements with attributes and NewMapXmlSeq Map values.
-
-package mxj
-
-import (
- "fmt"
- "strconv"
- "strings"
-)
-
-// Update value based on path and possible sub-key values.
-// A count of the number of values changed and any error are returned.
-// If the count == 0, then no path (and subkeys) matched.
-// 'newVal' can be a Map or map[string]interface{} value with a single 'key' that is the key to be modified
-// or a string value "key:value[:type]" where type is "bool" or "num" to cast the value.
-// 'path' is dot-notation list of keys to traverse; last key in path can be newVal key
-// NOTE: 'path' spec does not currently support indexed array references.
-// 'subkeys' are "key:value[:type]" entries that must match for path node
-// The subkey can be wildcarded - "key:*" - to require that it's there with some value.
-// If a subkey is preceeded with the '!' character, the key:value[:type] entry is treated as an
-// exclusion critera - e.g., "!author:William T. Gaddis".
-//
-// NOTES:
-// 1. Simple elements with attributes need a path terminated as ".#text" to modify the actual value.
-// 2. Values in Maps created using NewMapXmlSeq are map[string]interface{} values with a "#text" key.
-// 3. If values in 'newVal' or 'subkeys' args contain ":", use SetFieldSeparator to an unused symbol,
-// perhaps "|".
-func (mv Map) UpdateValuesForPath(newVal interface{}, path string, subkeys ...string) (int, error) {
- m := map[string]interface{}(mv)
-
- // extract the subkeys
- var subKeyMap map[string]interface{}
- if len(subkeys) > 0 {
- var err error
- subKeyMap, err = getSubKeyMap(subkeys...)
- if err != nil {
- return 0, err
- }
- }
-
- // extract key and value from newVal
- var key string
- var val interface{}
- switch newVal.(type) {
- case map[string]interface{}, Map:
- switch newVal.(type) { // "fallthrough is not permitted in type switch" (Spec)
- case Map:
- newVal = newVal.(Map).Old()
- }
- if len(newVal.(map[string]interface{})) != 1 {
- return 0, fmt.Errorf("newVal map can only have len == 1 - %+v", newVal)
- }
- for key, val = range newVal.(map[string]interface{}) {
- }
- case string: // split it as a key:value pair
- ss := strings.Split(newVal.(string), fieldSep)
- n := len(ss)
- if n < 2 || n > 3 {
- return 0, fmt.Errorf("unknown newVal spec - %+v", newVal)
- }
- key = ss[0]
- if n == 2 {
- val = interface{}(ss[1])
- } else if n == 3 {
- switch ss[2] {
- case "bool", "boolean":
- nv, err := strconv.ParseBool(ss[1])
- if err != nil {
- return 0, fmt.Errorf("can't convert newVal to bool - %+v", newVal)
- }
- val = interface{}(nv)
- case "num", "numeric", "float", "int":
- nv, err := strconv.ParseFloat(ss[1], 64)
- if err != nil {
- return 0, fmt.Errorf("can't convert newVal to float64 - %+v", newVal)
- }
- val = interface{}(nv)
- default:
- return 0, fmt.Errorf("unknown type for newVal value - %+v", newVal)
- }
- }
- default:
- return 0, fmt.Errorf("invalid newVal type - %+v", newVal)
- }
-
- // parse path
- keys := strings.Split(path, ".")
-
- var count int
- updateValuesForKeyPath(key, val, m, keys, subKeyMap, &count)
-
- return count, nil
-}
-
-// navigate the path
-func updateValuesForKeyPath(key string, value interface{}, m interface{}, keys []string, subkeys map[string]interface{}, cnt *int) {
- // ----- at end node: looking at possible node to get 'key' ----
- if len(keys) == 1 {
- updateValue(key, value, m, keys[0], subkeys, cnt)
- return
- }
-
- // ----- here we are navigating the path thru the penultimate node --------
- // key of interest is keys[0] - the next in the path
- switch keys[0] {
- case "*": // wildcard - scan all values
- switch m.(type) {
- case map[string]interface{}:
- for _, v := range m.(map[string]interface{}) {
- updateValuesForKeyPath(key, value, v, keys[1:], subkeys, cnt)
- }
- case []interface{}:
- for _, v := range m.([]interface{}) {
- switch v.(type) {
- // flatten out a list of maps - keys are processed
- case map[string]interface{}:
- for _, vv := range v.(map[string]interface{}) {
- updateValuesForKeyPath(key, value, vv, keys[1:], subkeys, cnt)
- }
- default:
- updateValuesForKeyPath(key, value, v, keys[1:], subkeys, cnt)
- }
- }
- }
- default: // key - must be map[string]interface{}
- switch m.(type) {
- case map[string]interface{}:
- if v, ok := m.(map[string]interface{})[keys[0]]; ok {
- updateValuesForKeyPath(key, value, v, keys[1:], subkeys, cnt)
- }
- case []interface{}: // may be buried in list
- for _, v := range m.([]interface{}) {
- switch v.(type) {
- case map[string]interface{}:
- if vv, ok := v.(map[string]interface{})[keys[0]]; ok {
- updateValuesForKeyPath(key, value, vv, keys[1:], subkeys, cnt)
- }
- }
- }
- }
- }
-}
-
-// change value if key and subkeys are present
-func updateValue(key string, value interface{}, m interface{}, keys0 string, subkeys map[string]interface{}, cnt *int) {
- // there are two possible options for the value of 'keys0': map[string]interface, []interface{}
- // and 'key' is a key in the map or is a key in a map in a list.
- switch m.(type) {
- case map[string]interface{}: // gotta have the last key
- if keys0 == "*" {
- for k := range m.(map[string]interface{}) {
- updateValue(key, value, m, k, subkeys, cnt)
- }
- return
- }
- endVal, _ := m.(map[string]interface{})[keys0]
-
- // if newV key is the end of path, replace the value for path-end
- // may be []interface{} - means replace just an entry w/ subkeys
- // otherwise replace the keys0 value if subkeys are there
- // NOTE: this will replace the subkeys, also
- if key == keys0 {
- switch endVal.(type) {
- case map[string]interface{}:
- if hasSubKeys(m, subkeys) {
- (m.(map[string]interface{}))[keys0] = value
- (*cnt)++
- }
- case []interface{}:
- // without subkeys can't select list member to modify
- // so key:value spec is it ...
- if hasSubKeys(m, subkeys) {
- (m.(map[string]interface{}))[keys0] = value
- (*cnt)++
- break
- }
- nv := make([]interface{}, 0)
- var valmodified bool
- for _, v := range endVal.([]interface{}) {
- // check entry subkeys
- if hasSubKeys(v, subkeys) {
- // replace v with value
- nv = append(nv, value)
- valmodified = true
- (*cnt)++
- continue
- }
- nv = append(nv, v)
- }
- if valmodified {
- (m.(map[string]interface{}))[keys0] = interface{}(nv)
- }
- default: // anything else is a strict replacement
- if hasSubKeys(m, subkeys) {
- (m.(map[string]interface{}))[keys0] = value
- (*cnt)++
- }
- }
- return
- }
-
- // so value is for an element of endVal
- // if endVal is a map then 'key' must be there w/ subkeys
- // if endVal is a list then 'key' must be in a list member w/ subkeys
- switch endVal.(type) {
- case map[string]interface{}:
- if !hasSubKeys(endVal, subkeys) {
- return
- }
- if _, ok := (endVal.(map[string]interface{}))[key]; ok {
- (endVal.(map[string]interface{}))[key] = value
- (*cnt)++
- }
- case []interface{}: // keys0 points to a list, check subkeys
- for _, v := range endVal.([]interface{}) {
- // got to be a map so we can replace value for 'key'
- vv, vok := v.(map[string]interface{})
- if !vok {
- continue
- }
- if _, ok := vv[key]; !ok {
- continue
- }
- if !hasSubKeys(vv, subkeys) {
- continue
- }
- vv[key] = value
- (*cnt)++
- }
- }
- case []interface{}: // key may be in a list member
- // don't need to handle keys0 == "*"; we're looking at everything, anyway.
- for _, v := range m.([]interface{}) {
- // only map values - we're looking for 'key'
- mm, ok := v.(map[string]interface{})
- if !ok {
- continue
- }
- if _, ok := mm[key]; !ok {
- continue
- }
- if !hasSubKeys(mm, subkeys) {
- continue
- }
- mm[key] = value
- (*cnt)++
- }
- }
-
- // return
-}
diff --git a/vendor/github.com/clbanning/mxj/xml.go b/vendor/github.com/clbanning/mxj/xml.go
deleted file mode 100644
index fac0f1d3..00000000
--- a/vendor/github.com/clbanning/mxj/xml.go
+++ /dev/null
@@ -1,1139 +0,0 @@
-// Copyright 2012-2016 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// xml.go - basically the core of X2j for map[string]interface{} values.
-// NewMapXml, NewMapXmlReader, mv.Xml, mv.XmlWriter
-// see x2j and j2x for wrappers to provide end-to-end transformation of XML and JSON messages.
-
-package mxj
-
-import (
- "bytes"
- "encoding/json"
- "encoding/xml"
- "errors"
- "fmt"
- "io"
- "reflect"
- "sort"
- "strconv"
- "strings"
- "time"
-)
-
-// ------------------- NewMapXml & NewMapXmlReader ... -------------------------
-
-// If XmlCharsetReader != nil, it will be used to decode the XML, if required.
-// Note: if CustomDecoder != nil, then XmlCharsetReader is ignored;
-// set the CustomDecoder attribute instead.
-// import (
-// charset "code.google.com/p/go-charset/charset"
-// github.com/clbanning/mxj
-// )
-// ...
-// mxj.XmlCharsetReader = charset.NewReader
-// m, merr := mxj.NewMapXml(xmlValue)
-var XmlCharsetReader func(charset string, input io.Reader) (io.Reader, error)
-
-// NewMapXml - convert a XML doc into a Map
-// (This is analogous to unmarshalling a JSON string to map[string]interface{} using json.Unmarshal().)
-// If the optional argument 'cast' is 'true', then values will be converted to boolean or float64 if possible.
-//
-// Converting XML to JSON is a simple as:
-// ...
-// mapVal, merr := mxj.NewMapXml(xmlVal)
-// if merr != nil {
-// // handle error
-// }
-// jsonVal, jerr := mapVal.Json()
-// if jerr != nil {
-// // handle error
-// }
-//
-// NOTES:
-// 1. The 'xmlVal' will be parsed looking for an xml.StartElement, so BOM and other
-// extraneous xml.CharData will be ignored unless io.EOF is reached first.
-// 2. If CoerceKeysToLower() has been called, then all key values will be lower case.
-// 3. If CoerceKeysToSnakeCase() has been called, then all key values will be converted to snake case.
-func NewMapXml(xmlVal []byte, cast ...bool) (Map, error) {
- var r bool
- if len(cast) == 1 {
- r = cast[0]
- }
- return xmlToMap(xmlVal, r)
-}
-
-// Get next XML doc from an io.Reader as a Map value. Returns Map value.
-// NOTES:
-// 1. The 'xmlReader' will be parsed looking for an xml.StartElement, so BOM and other
-// extraneous xml.CharData will be ignored unless io.EOF is reached first.
-// 2. If CoerceKeysToLower() has been called, then all key values will be lower case.
-// 3. If CoerceKeysToSnakeCase() has been called, then all key values will be converted to snake case.
-func NewMapXmlReader(xmlReader io.Reader, cast ...bool) (Map, error) {
- var r bool
- if len(cast) == 1 {
- r = cast[0]
- }
-
- // We need to put an *os.File reader in a ByteReader or the xml.NewDecoder
- // will wrap it in a bufio.Reader and seek on the file beyond where the
- // xml.Decoder parses!
- if _, ok := xmlReader.(io.ByteReader); !ok {
- xmlReader = myByteReader(xmlReader) // see code at EOF
- }
-
- // build the map
- return xmlReaderToMap(xmlReader, r)
-}
-
-// Get next XML doc from an io.Reader as a Map value. Returns Map value and slice with the raw XML.
-// NOTES:
-// 1. Due to the implementation of xml.Decoder, the raw XML off the reader is buffered to []byte
-// using a ByteReader. If the io.Reader is an os.File, there may be significant performance impact.
-// See the examples - getmetrics1.go through getmetrics4.go - for comparative use cases on a large
-// data set. If the io.Reader is wrapping a []byte value in-memory, however, such as http.Request.Body
-// you CAN use it to efficiently unmarshal a XML doc and retrieve the raw XML in a single call.
-// 2. The 'raw' return value may be larger than the XML text value.
-// 3. The 'xmlReader' will be parsed looking for an xml.StartElement, so BOM and other
-// extraneous xml.CharData will be ignored unless io.EOF is reached first.
-// 4. If CoerceKeysToLower() has been called, then all key values will be lower case.
-// 5. If CoerceKeysToSnakeCase() has been called, then all key values will be converted to snake case.
-func NewMapXmlReaderRaw(xmlReader io.Reader, cast ...bool) (Map, []byte, error) {
- var r bool
- if len(cast) == 1 {
- r = cast[0]
- }
- // create TeeReader so we can retrieve raw XML
- buf := make([]byte, 0)
- wb := bytes.NewBuffer(buf)
- trdr := myTeeReader(xmlReader, wb) // see code at EOF
-
- m, err := xmlReaderToMap(trdr, r)
-
- // retrieve the raw XML that was decoded
- b := wb.Bytes()
-
- if err != nil {
- return nil, b, err
- }
-
- return m, b, nil
-}
-
-// xmlReaderToMap() - parse a XML io.Reader to a map[string]interface{} value
-func xmlReaderToMap(rdr io.Reader, r bool) (map[string]interface{}, error) {
- // parse the Reader
- p := xml.NewDecoder(rdr)
- if CustomDecoder != nil {
- useCustomDecoder(p)
- } else {
- p.CharsetReader = XmlCharsetReader
- }
- return xmlToMapParser("", nil, p, r)
-}
-
-// xmlToMap - convert a XML doc into map[string]interface{} value
-func xmlToMap(doc []byte, r bool) (map[string]interface{}, error) {
- b := bytes.NewReader(doc)
- p := xml.NewDecoder(b)
- if CustomDecoder != nil {
- useCustomDecoder(p)
- } else {
- p.CharsetReader = XmlCharsetReader
- }
- return xmlToMapParser("", nil, p, r)
-}
-
-// ===================================== where the work happens =============================
-
-// PrependAttrWithHyphen. Prepend attribute tags with a hyphen.
-// Default is 'true'. (Not applicable to NewMapXmlSeq(), mv.XmlSeq(), etc.)
-// Note:
-// If 'false', unmarshaling and marshaling is not symmetric. Attributes will be
-// marshal'd as attr and may be part of a list.
-func PrependAttrWithHyphen(v bool) {
- if v {
- attrPrefix = "-"
- lenAttrPrefix = len(attrPrefix)
- return
- }
- attrPrefix = ""
- lenAttrPrefix = len(attrPrefix)
-}
-
-// Include sequence id with inner tags. - per Sean Murphy, murphysean84@gmail.com.
-var includeTagSeqNum bool
-
-// IncludeTagSeqNum - include a "_seq":N key:value pair with each inner tag, denoting
-// its position when parsed. This is of limited usefulness, since list values cannot
-// be tagged with "_seq" without changing their depth in the Map.
-// So THIS SHOULD BE USED WITH CAUTION - see the test cases. Here's a sample of what
-// you get.
-/*
-
-
-
-
- hello
-
-
- parses as:
-
- {
- Obj:{
- "-c":"la",
- "-h":"da",
- "-x":"dee",
- "intObj":[
- {
- "-id"="3",
- "_seq":"0" // if mxj.Cast is passed, then: "_seq":0
- },
- {
- "-id"="2",
- "_seq":"2"
- }],
- "intObj1":{
- "-id":"1",
- "_seq":"1"
- },
- "StrObj":{
- "#text":"hello", // simple element value gets "#text" tag
- "_seq":"3"
- }
- }
- }
-*/
-func IncludeTagSeqNum(b bool) {
- includeTagSeqNum = b
-}
-
-// all keys will be "lower case"
-var lowerCase bool
-
-// Coerce all tag values to keys in lower case. This is useful if you've got sources with variable
-// tag capitalization, and you want to use m.ValuesForKeys(), etc., with the key or path spec
-// in lower case.
-// CoerceKeysToLower() will toggle the coercion flag true|false - on|off
-// CoerceKeysToLower(true|false) will set the coercion flag on|off
-//
-// NOTE: only recognized by NewMapXml, NewMapXmlReader, and NewMapXmlReaderRaw functions as well as
-// the associated HandleXmlReader and HandleXmlReaderRaw.
-func CoerceKeysToLower(b ...bool) {
- if len(b) == 0 {
- lowerCase = !lowerCase
- } else if len(b) == 1 {
- lowerCase = b[0]
- }
-}
-
-// 25jun16: Allow user to specify the "prefix" character for XML attribute key labels.
-// We do this by replacing '`' constant with attrPrefix var, replacing useHyphen with attrPrefix = "",
-// and adding a SetAttrPrefix(s string) function.
-
-var attrPrefix string = `-` // the default
-var lenAttrPrefix int = 1 // the default
-
-// SetAttrPrefix changes the default, "-", to the specified value, s.
-// SetAttrPrefix("") is the same as PrependAttrWithHyphen(false).
-// (Not applicable for NewMapXmlSeq(), mv.XmlSeq(), etc.)
-func SetAttrPrefix(s string) {
- attrPrefix = s
- lenAttrPrefix = len(attrPrefix)
-}
-
-// 18jan17: Allows user to specify if the map keys should be in snake case instead
-// of the default hyphenated notation.
-var snakeCaseKeys bool
-
-// CoerceKeysToSnakeCase changes the default, false, to the specified value, b.
-// Note: the attribute prefix will be a hyphen, '-', or what ever string value has
-// been specified using SetAttrPrefix.
-func CoerceKeysToSnakeCase(b ...bool) {
- if len(b) == 0 {
- snakeCaseKeys = !snakeCaseKeys
- } else if len(b) == 1 {
- snakeCaseKeys = b[0]
- }
-}
-
-// 05feb17: support processing XMPP streams (issue #36)
-var handleXMPPStreamTag bool
-
-// HandleXMPPStreamTag causes decoder to parse XMPP elements.
-// If called with no argument, XMPP stream element handling is toggled on/off.
-// (See xmppStream_test.go for example.)
-// If called with NewMapXml, NewMapXmlReader, New MapXmlReaderRaw the "stream"
-// element will be returned as:
-// map["stream"]interface{}{map[-]interface{}}.
-// If called with NewMapSeq, NewMapSeqReader, NewMapSeqReaderRaw the "stream"
-// element will be returned as:
-// map["stream:stream"]interface{}{map["#attr"]interface{}{map[string]interface{}}}
-// where the "#attr" values have "#text" and "#seq" keys. (See NewMapXmlSeq.)
-func HandleXMPPStreamTag(b ...bool) {
- if len(b) == 0 {
- handleXMPPStreamTag = !handleXMPPStreamTag
- } else if len(b) == 1 {
- handleXMPPStreamTag = b[0]
- }
-}
-
-// 21jan18 - decode all values as map["#text":value] (issue #56)
-var decodeSimpleValuesAsMap bool
-
-// DecodeSimpleValuesAsMap forces all values to be decoded as map["#text":].
-// If called with no argument, the decoding is toggled on/off.
-//
-// By default the NewMapXml functions decode simple values without attributes as
-// map[:]. This function causes simple values without attributes to be
-// decoded the same as simple values with attributes - map[:map["#text":]].
-func DecodeSimpleValuesAsMap(b ...bool) {
- if len(b) == 0 {
- decodeSimpleValuesAsMap = !decodeSimpleValuesAsMap
- } else if len(b) == 1 {
- decodeSimpleValuesAsMap = b[0]
- }
-}
-
-// xmlToMapParser (2015.11.12) - load a 'clean' XML doc into a map[string]interface{} directly.
-// A refactoring of xmlToTreeParser(), markDuplicate() and treeToMap() - here, all-in-one.
-// We've removed the intermediate *node tree with the allocation and subsequent rescanning.
-func xmlToMapParser(skey string, a []xml.Attr, p *xml.Decoder, r bool) (map[string]interface{}, error) {
- if lowerCase {
- skey = strings.ToLower(skey)
- }
- if snakeCaseKeys {
- skey = strings.Replace(skey, "-", "_", -1)
- }
-
- // NOTE: all attributes and sub-elements parsed into 'na', 'na' is returned as value for 'skey' in 'n'.
- // Unless 'skey' is a simple element w/o attributes, in which case the xml.CharData value is the value.
- var n, na map[string]interface{}
- var seq int // for includeTagSeqNum
-
- // Allocate maps and load attributes, if any.
- // NOTE: on entry from NewMapXml(), etc., skey=="", and we fall through
- // to get StartElement then recurse with skey==xml.StartElement.Name.Local
- // where we begin allocating map[string]interface{} values 'n' and 'na'.
- if skey != "" {
- n = make(map[string]interface{}) // old n
- na = make(map[string]interface{}) // old n.nodes
- if len(a) > 0 {
- for _, v := range a {
- if snakeCaseKeys {
- v.Name.Local = strings.Replace(v.Name.Local, "-", "_", -1)
- }
- var key string
- key = attrPrefix + v.Name.Local
- if lowerCase {
- key = strings.ToLower(key)
- }
- na[key] = cast(v.Value, r)
- }
- }
- }
- // Return XMPP message.
- if handleXMPPStreamTag && skey == "stream" {
- n[skey] = na
- return n, nil
- }
-
- for {
- t, err := p.Token()
- if err != nil {
- if err != io.EOF {
- return nil, errors.New("xml.Decoder.Token() - " + err.Error())
- }
- return nil, err
- }
- switch t.(type) {
- case xml.StartElement:
- tt := t.(xml.StartElement)
-
- // First call to xmlToMapParser() doesn't pass xml.StartElement - the map key.
- // So when the loop is first entered, the first token is the root tag along
- // with any attributes, which we process here.
- //
- // Subsequent calls to xmlToMapParser() will pass in tag+attributes for
- // processing before getting the next token which is the element value,
- // which is done above.
- if skey == "" {
- return xmlToMapParser(tt.Name.Local, tt.Attr, p, r)
- }
-
- // If not initializing the map, parse the element.
- // len(nn) == 1, necessarily - it is just an 'n'.
- nn, err := xmlToMapParser(tt.Name.Local, tt.Attr, p, r)
- if err != nil {
- return nil, err
- }
-
- // The nn map[string]interface{} value is a na[nn_key] value.
- // We need to see if nn_key already exists - means we're parsing a list.
- // This may require converting na[nn_key] value into []interface{} type.
- // First, extract the key:val for the map - it's a singleton.
- // Note:
- // * if CoerceKeysToLower() called, then key will be lower case.
- // * if CoerceKeysToSnakeCase() called, then key will be converted to snake case.
- var key string
- var val interface{}
- for key, val = range nn {
- break
- }
-
- // IncludeTagSeqNum requests that the element be augmented with a "_seq" sub-element.
- // In theory, we don't need this if len(na) == 1. But, we don't know what might
- // come next - we're only parsing forward. So if you ask for 'includeTagSeqNum' you
- // get it on every element. (Personally, I never liked this, but I added it on request
- // and did get a $50 Amazon gift card in return - now we support it for backwards compatibility!)
- if includeTagSeqNum {
- switch val.(type) {
- case []interface{}:
- // noop - There's no clean way to handle this w/o changing message structure.
- case map[string]interface{}:
- val.(map[string]interface{})["_seq"] = seq // will overwrite an "_seq" XML tag
- seq++
- case interface{}: // a non-nil simple element: string, float64, bool
- v := map[string]interface{}{"#text": val}
- v["_seq"] = seq
- seq++
- val = v
- }
- }
-
- // 'na' holding sub-elements of n.
- // See if 'key' already exists.
- // If 'key' exists, then this is a list, if not just add key:val to na.
- if v, ok := na[key]; ok {
- var a []interface{}
- switch v.(type) {
- case []interface{}:
- a = v.([]interface{})
- default: // anything else - note: v.(type) != nil
- a = []interface{}{v}
- }
- a = append(a, val)
- na[key] = a
- } else {
- na[key] = val // save it as a singleton
- }
- case xml.EndElement:
- // len(n) > 0 if this is a simple element w/o xml.Attrs - see xml.CharData case.
- if len(n) == 0 {
- // If len(na)==0 we have an empty element == "";
- // it has no xml.Attr nor xml.CharData.
- // Note: in original node-tree parser, val defaulted to "";
- // so we always had the default if len(node.nodes) == 0.
- if len(na) > 0 {
- n[skey] = na
- } else {
- n[skey] = "" // empty element
- }
- }
- return n, nil
- case xml.CharData:
- // clean up possible noise
- tt := strings.Trim(string(t.(xml.CharData)), "\t\r\b\n ")
- if len(tt) > 0 {
- if len(na) > 0 || decodeSimpleValuesAsMap {
- na["#text"] = cast(tt, r)
- } else if skey != "" {
- n[skey] = cast(tt, r)
- } else {
- // per Adrian (http://www.adrianlungu.com/) catch stray text
- // in decoder stream -
- // https://github.com/clbanning/mxj/pull/14#issuecomment-182816374
- // NOTE: CharSetReader must be set to non-UTF-8 CharSet or you'll get
- // a p.Token() decoding error when the BOM is UTF-16 or UTF-32.
- continue
- }
- }
- default:
- // noop
- }
- }
-}
-
-var castNanInf bool
-
-// Cast "Nan", "Inf", "-Inf" XML values to 'float64'.
-// By default, these values will be decoded as 'string'.
-func CastNanInf(b bool) {
- castNanInf = b
-}
-
-// cast - try to cast string values to bool or float64
-func cast(s string, r bool) interface{} {
- if r {
- // handle nan and inf
- if !castNanInf {
- switch strings.ToLower(s) {
- case "nan", "inf", "-inf":
- return s
- }
- }
-
- // handle numeric strings ahead of boolean
- if f, err := strconv.ParseFloat(s, 64); err == nil {
- return f
- }
- // ParseBool treats "1"==true & "0"==false, we've already scanned those
- // values as float64. See if value has 't' or 'f' as initial screen to
- // minimize calls to ParseBool; also, see if len(s) < 6.
- if len(s) > 0 && len(s) < 6 {
- switch s[:1] {
- case "t", "T", "f", "F":
- if b, err := strconv.ParseBool(s); err == nil {
- return b
- }
- }
- }
- }
- return s
-}
-
-// ------------------ END: NewMapXml & NewMapXmlReader -------------------------
-
-// ------------------ mv.Xml & mv.XmlWriter - from j2x ------------------------
-
-const (
- DefaultRootTag = "doc"
-)
-
-var useGoXmlEmptyElemSyntax bool
-
-// XmlGoEmptyElemSyntax() - rather than .
-// Go's encoding/xml package marshals empty XML elements as . By default this package
-// encodes empty elements as . If you're marshaling Map values that include structures
-// (which are passed to xml.Marshal for encoding), this will let you conform to the standard package.
-func XmlGoEmptyElemSyntax() {
- useGoXmlEmptyElemSyntax = true
-}
-
-// XmlDefaultEmptyElemSyntax() - rather than .
-// Return XML encoding for empty elements to the default package setting.
-// Reverses effect of XmlGoEmptyElemSyntax().
-func XmlDefaultEmptyElemSyntax() {
- useGoXmlEmptyElemSyntax = false
-}
-
-// Encode a Map as XML. The companion of NewMapXml().
-// The following rules apply.
-// - The key label "#text" is treated as the value for a simple element with attributes.
-// - Map keys that begin with a hyphen, '-', are interpreted as attributes.
-// It is an error if the attribute doesn't have a []byte, string, number, or boolean value.
-// - Map value type encoding:
-// > string, bool, float64, int, int32, int64, float32: per "%v" formating
-// > []bool, []uint8: by casting to string
-// > structures, etc.: handed to xml.Marshal() - if there is an error, the element
-// value is "UNKNOWN"
-// - Elements with only attribute values or are null are terminated using "/>".
-// - If len(mv) == 1 and no rootTag is provided, then the map key is used as the root tag, possible.
-// Thus, `{ "key":"value" }` encodes as "value".
-// - To encode empty elements in a syntax consistent with encoding/xml call UseGoXmlEmptyElementSyntax().
-// The attributes tag=value pairs are alphabetized by "tag". Also, when encoding map[string]interface{} values -
-// complex elements, etc. - the key:value pairs are alphabetized by key so the resulting tags will appear sorted.
-func (mv Map) Xml(rootTag ...string) ([]byte, error) {
- m := map[string]interface{}(mv)
- var err error
- s := new(string)
- p := new(pretty) // just a stub
-
- if len(m) == 1 && len(rootTag) == 0 {
- for key, value := range m {
- // if it an array, see if all values are map[string]interface{}
- // we force a new root tag if we'll end up with no key:value in the list
- // so: key:[string_val, bool:true] --> string_valtrue
- switch value.(type) {
- case []interface{}:
- for _, v := range value.([]interface{}) {
- switch v.(type) {
- case map[string]interface{}: // noop
- default: // anything else
- err = mapToXmlIndent(false, s, DefaultRootTag, m, p)
- goto done
- }
- }
- }
- err = mapToXmlIndent(false, s, key, value, p)
- }
- } else if len(rootTag) == 1 {
- err = mapToXmlIndent(false, s, rootTag[0], m, p)
- } else {
- err = mapToXmlIndent(false, s, DefaultRootTag, m, p)
- }
-done:
- return []byte(*s), err
-}
-
-// The following implementation is provided only for symmetry with NewMapXmlReader[Raw]
-// The names will also provide a key for the number of return arguments.
-
-// Writes the Map as XML on the Writer.
-// See Xml() for encoding rules.
-func (mv Map) XmlWriter(xmlWriter io.Writer, rootTag ...string) error {
- x, err := mv.Xml(rootTag...)
- if err != nil {
- return err
- }
-
- _, err = xmlWriter.Write(x)
- return err
-}
-
-// Writes the Map as XML on the Writer. []byte is the raw XML that was written.
-// See Xml() for encoding rules.
-func (mv Map) XmlWriterRaw(xmlWriter io.Writer, rootTag ...string) ([]byte, error) {
- x, err := mv.Xml(rootTag...)
- if err != nil {
- return x, err
- }
-
- _, err = xmlWriter.Write(x)
- return x, err
-}
-
-// Writes the Map as pretty XML on the Writer.
-// See Xml() for encoding rules.
-func (mv Map) XmlIndentWriter(xmlWriter io.Writer, prefix, indent string, rootTag ...string) error {
- x, err := mv.XmlIndent(prefix, indent, rootTag...)
- if err != nil {
- return err
- }
-
- _, err = xmlWriter.Write(x)
- return err
-}
-
-// Writes the Map as pretty XML on the Writer. []byte is the raw XML that was written.
-// See Xml() for encoding rules.
-func (mv Map) XmlIndentWriterRaw(xmlWriter io.Writer, prefix, indent string, rootTag ...string) ([]byte, error) {
- x, err := mv.XmlIndent(prefix, indent, rootTag...)
- if err != nil {
- return x, err
- }
-
- _, err = xmlWriter.Write(x)
- return x, err
-}
-
-// -------------------- END: mv.Xml & mv.XmlWriter -------------------------------
-
-// -------------- Handle XML stream by processing Map value --------------------
-
-// Default poll delay to keep Handler from spinning on an open stream
-// like sitting on os.Stdin waiting for imput.
-var xhandlerPollInterval = time.Millisecond
-
-// Bulk process XML using handlers that process a Map value.
-// 'rdr' is an io.Reader for XML (stream)
-// 'mapHandler' is the Map processor. Return of 'false' stops io.Reader processing.
-// 'errHandler' is the error processor. Return of 'false' stops io.Reader processing and returns the error.
-// Note: mapHandler() and errHandler() calls are blocking, so reading and processing of messages is serialized.
-// This means that you can stop reading the file on error or after processing a particular message.
-// To have reading and handling run concurrently, pass argument to a go routine in handler and return 'true'.
-func HandleXmlReader(xmlReader io.Reader, mapHandler func(Map) bool, errHandler func(error) bool) error {
- var n int
- for {
- m, merr := NewMapXmlReader(xmlReader)
- n++
-
- // handle error condition with errhandler
- if merr != nil && merr != io.EOF {
- merr = fmt.Errorf("[xmlReader: %d] %s", n, merr.Error())
- if ok := errHandler(merr); !ok {
- // caused reader termination
- return merr
- }
- continue
- }
-
- // pass to maphandler
- if len(m) != 0 {
- if ok := mapHandler(m); !ok {
- break
- }
- } else if merr != io.EOF {
- time.Sleep(xhandlerPollInterval)
- }
-
- if merr == io.EOF {
- break
- }
- }
- return nil
-}
-
-// Bulk process XML using handlers that process a Map value and the raw XML.
-// 'rdr' is an io.Reader for XML (stream)
-// 'mapHandler' is the Map and raw XML - []byte - processor. Return of 'false' stops io.Reader processing.
-// 'errHandler' is the error and raw XML processor. Return of 'false' stops io.Reader processing and returns the error.
-// Note: mapHandler() and errHandler() calls are blocking, so reading and processing of messages is serialized.
-// This means that you can stop reading the file on error or after processing a particular message.
-// To have reading and handling run concurrently, pass argument(s) to a go routine in handler and return 'true'.
-// See NewMapXmlReaderRaw for comment on performance associated with retrieving raw XML from a Reader.
-func HandleXmlReaderRaw(xmlReader io.Reader, mapHandler func(Map, []byte) bool, errHandler func(error, []byte) bool) error {
- var n int
- for {
- m, raw, merr := NewMapXmlReaderRaw(xmlReader)
- n++
-
- // handle error condition with errhandler
- if merr != nil && merr != io.EOF {
- merr = fmt.Errorf("[xmlReader: %d] %s", n, merr.Error())
- if ok := errHandler(merr, raw); !ok {
- // caused reader termination
- return merr
- }
- continue
- }
-
- // pass to maphandler
- if len(m) != 0 {
- if ok := mapHandler(m, raw); !ok {
- break
- }
- } else if merr != io.EOF {
- time.Sleep(xhandlerPollInterval)
- }
-
- if merr == io.EOF {
- break
- }
- }
- return nil
-}
-
-// ----------------- END: Handle XML stream by processing Map value --------------
-
-// -------- a hack of io.TeeReader ... need one that's an io.ByteReader for xml.NewDecoder() ----------
-
-// This is a clone of io.TeeReader with the additional method t.ReadByte().
-// Thus, this TeeReader is also an io.ByteReader.
-// This is necessary because xml.NewDecoder uses a ByteReader not a Reader. It appears to have been written
-// with bufio.Reader or bytes.Reader in mind ... not a generic io.Reader, which doesn't have to have ReadByte()..
-// If NewDecoder is passed a Reader that does not satisfy ByteReader() it wraps the Reader with
-// bufio.NewReader and uses ReadByte rather than Read that runs the TeeReader pipe logic.
-
-type teeReader struct {
- r io.Reader
- w io.Writer
- b []byte
-}
-
-func myTeeReader(r io.Reader, w io.Writer) io.Reader {
- b := make([]byte, 1)
- return &teeReader{r, w, b}
-}
-
-// need for io.Reader - but we don't use it ...
-func (t *teeReader) Read(p []byte) (int, error) {
- return 0, nil
-}
-
-func (t *teeReader) ReadByte() (byte, error) {
- n, err := t.r.Read(t.b)
- if n > 0 {
- if _, err := t.w.Write(t.b[:1]); err != nil {
- return t.b[0], err
- }
- }
- return t.b[0], err
-}
-
-// For use with NewMapXmlReader & NewMapXmlSeqReader.
-type byteReader struct {
- r io.Reader
- b []byte
-}
-
-func myByteReader(r io.Reader) io.Reader {
- b := make([]byte, 1)
- return &byteReader{r, b}
-}
-
-// Need for io.Reader interface ...
-// Needed if reading a malformed http.Request.Body - issue #38.
-func (b *byteReader) Read(p []byte) (int, error) {
- return b.r.Read(p)
-}
-
-func (b *byteReader) ReadByte() (byte, error) {
- _, err := b.r.Read(b.b)
- if len(b.b) > 0 {
- return b.b[0], err
- }
- var c byte
- return c, err
-}
-
-// ----------------------- END: io.TeeReader hack -----------------------------------
-
-// ---------------------- XmlIndent - from j2x package ----------------------------
-
-// Encode a map[string]interface{} as a pretty XML string.
-// See Xml for encoding rules.
-func (mv Map) XmlIndent(prefix, indent string, rootTag ...string) ([]byte, error) {
- m := map[string]interface{}(mv)
-
- var err error
- s := new(string)
- p := new(pretty)
- p.indent = indent
- p.padding = prefix
-
- if len(m) == 1 && len(rootTag) == 0 {
- // this can extract the key for the single map element
- // use it if it isn't a key for a list
- for key, value := range m {
- if _, ok := value.([]interface{}); ok {
- err = mapToXmlIndent(true, s, DefaultRootTag, m, p)
- } else {
- err = mapToXmlIndent(true, s, key, value, p)
- }
- }
- } else if len(rootTag) == 1 {
- err = mapToXmlIndent(true, s, rootTag[0], m, p)
- } else {
- err = mapToXmlIndent(true, s, DefaultRootTag, m, p)
- }
- return []byte(*s), err
-}
-
-type pretty struct {
- indent string
- cnt int
- padding string
- mapDepth int
- start int
-}
-
-func (p *pretty) Indent() {
- p.padding += p.indent
- p.cnt++
-}
-
-func (p *pretty) Outdent() {
- if p.cnt > 0 {
- p.padding = p.padding[:len(p.padding)-len(p.indent)]
- p.cnt--
- }
-}
-
-// where the work actually happens
-// returns an error if an attribute is not atomic
-func mapToXmlIndent(doIndent bool, s *string, key string, value interface{}, pp *pretty) error {
- var endTag bool
- var isSimple bool
- var elen int
- p := &pretty{pp.indent, pp.cnt, pp.padding, pp.mapDepth, pp.start}
-
- // per issue #48, 18apr18 - try and coerce maps to map[string]interface{}
- // Don't need for mapToXmlSeqIndent, since maps there are decoded by NewMapXmlSeq().
- if reflect.ValueOf(value).Kind() == reflect.Map {
- switch value.(type) {
- case map[string]interface{}:
- default:
- val := make(map[string]interface{})
- vv := reflect.ValueOf(value)
- keys := vv.MapKeys()
- for _, k := range keys {
- val[fmt.Sprint(k)] = vv.MapIndex(k).Interface()
- }
- value = val
- }
- }
-
- switch value.(type) {
- // special handling of []interface{} values when len(value) == 0
- case map[string]interface{}, []byte, string, float64, bool, int, int32, int64, float32, json.Number:
- if doIndent {
- *s += p.padding
- }
- *s += `<` + key
- }
- switch value.(type) {
- case map[string]interface{}:
- vv := value.(map[string]interface{})
- lenvv := len(vv)
- // scan out attributes - attribute keys have prepended attrPrefix
- attrlist := make([][2]string, len(vv))
- var n int
- var ss string
- for k, v := range vv {
- if lenAttrPrefix > 0 && lenAttrPrefix < len(k) && k[:lenAttrPrefix] == attrPrefix {
- switch v.(type) {
- case string:
- if xmlEscapeChars {
- ss = escapeChars(v.(string))
- } else {
- ss = v.(string)
- }
- attrlist[n][0] = k[lenAttrPrefix:]
- attrlist[n][1] = ss
- case float64, bool, int, int32, int64, float32, json.Number:
- attrlist[n][0] = k[lenAttrPrefix:]
- attrlist[n][1] = fmt.Sprintf("%v", v)
- case []byte:
- if xmlEscapeChars {
- ss = escapeChars(string(v.([]byte)))
- } else {
- ss = string(v.([]byte))
- }
- attrlist[n][0] = k[lenAttrPrefix:]
- attrlist[n][1] = ss
- default:
- return fmt.Errorf("invalid attribute value for: %s:<%T>", k, v)
- }
- n++
- }
- }
- if n > 0 {
- attrlist = attrlist[:n]
- sort.Sort(attrList(attrlist))
- for _, v := range attrlist {
- *s += ` ` + v[0] + `="` + v[1] + `"`
- }
- }
- // only attributes?
- if n == lenvv {
- if useGoXmlEmptyElemSyntax {
- *s += `` + key + ">"
- } else {
- *s += `/>`
- }
- break
- }
-
- // simple element? Note: '#text" is an invalid XML tag.
- if v, ok := vv["#text"]; ok && n+1 == lenvv {
- switch v.(type) {
- case string:
- if xmlEscapeChars {
- v = escapeChars(v.(string))
- } else {
- v = v.(string)
- }
- case []byte:
- if xmlEscapeChars {
- v = escapeChars(string(v.([]byte)))
- }
- }
- *s += ">" + fmt.Sprintf("%v", v)
- endTag = true
- elen = 1
- isSimple = true
- break
- } else if ok {
- // Handle edge case where simple element with attributes
- // is unmarshal'd using NewMapXml() where attribute prefix
- // has been set to "".
- // TODO(clb): should probably scan all keys for invalid chars.
- return fmt.Errorf("invalid attribute key label: #text - due to attributes not being prefixed")
- }
-
- // close tag with possible attributes
- *s += ">"
- if doIndent {
- *s += "\n"
- }
- // something more complex
- p.mapDepth++
- // extract the map k:v pairs and sort on key
- elemlist := make([][2]interface{}, len(vv))
- n = 0
- for k, v := range vv {
- if lenAttrPrefix > 0 && lenAttrPrefix < len(k) && k[:lenAttrPrefix] == attrPrefix {
- continue
- }
- elemlist[n][0] = k
- elemlist[n][1] = v
- n++
- }
- elemlist = elemlist[:n]
- sort.Sort(elemList(elemlist))
- var i int
- for _, v := range elemlist {
- switch v[1].(type) {
- case []interface{}:
- default:
- if i == 0 && doIndent {
- p.Indent()
- }
- }
- i++
- if err := mapToXmlIndent(doIndent, s, v[0].(string), v[1], p); err != nil {
- return err
- }
- switch v[1].(type) {
- case []interface{}: // handled in []interface{} case
- default:
- if doIndent {
- p.Outdent()
- }
- }
- i--
- }
- p.mapDepth--
- endTag = true
- elen = 1 // we do have some content ...
- case []interface{}:
- // special case - found during implementing Issue #23
- if len(value.([]interface{})) == 0 {
- if doIndent {
- *s += p.padding + p.indent
- }
- *s += "<" + key
- elen = 0
- endTag = true
- break
- }
- for _, v := range value.([]interface{}) {
- if doIndent {
- p.Indent()
- }
- if err := mapToXmlIndent(doIndent, s, key, v, p); err != nil {
- return err
- }
- if doIndent {
- p.Outdent()
- }
- }
- return nil
- case []string:
- // This was added by https://github.com/slotix ... not a type that
- // would be encountered if mv generated from NewMapXml, NewMapJson.
- // Could be encountered in AnyXml(), so we'll let it stay, though
- // it should be merged with case []interface{}, above.
- //quick fix for []string type
- //[]string should be treated exaclty as []interface{}
- if len(value.([]string)) == 0 {
- if doIndent {
- *s += p.padding + p.indent
- }
- *s += "<" + key
- elen = 0
- endTag = true
- break
- }
- for _, v := range value.([]string) {
- if doIndent {
- p.Indent()
- }
- if err := mapToXmlIndent(doIndent, s, key, v, p); err != nil {
- return err
- }
- if doIndent {
- p.Outdent()
- }
- }
- return nil
- case nil:
- // terminate the tag
- if doIndent {
- *s += p.padding
- }
- *s += "<" + key
- endTag, isSimple = true, true
- break
- default: // handle anything - even goofy stuff
- elen = 0
- switch value.(type) {
- case string:
- v := value.(string)
- if xmlEscapeChars {
- v = escapeChars(v)
- }
- elen = len(v)
- if elen > 0 {
- *s += ">" + v
- }
- case float64, bool, int, int32, int64, float32, json.Number:
- v := fmt.Sprintf("%v", value)
- elen = len(v) // always > 0
- *s += ">" + v
- case []byte: // NOTE: byte is just an alias for uint8
- // similar to how xml.Marshal handles []byte structure members
- v := string(value.([]byte))
- if xmlEscapeChars {
- v = escapeChars(v)
- }
- elen = len(v)
- if elen > 0 {
- *s += ">" + v
- }
- default:
- var v []byte
- var err error
- if doIndent {
- v, err = xml.MarshalIndent(value, p.padding, p.indent)
- } else {
- v, err = xml.Marshal(value)
- }
- if err != nil {
- *s += ">UNKNOWN"
- } else {
- elen = len(v)
- if elen > 0 {
- *s += string(v)
- }
- }
- }
- isSimple = true
- endTag = true
- }
- if endTag {
- if doIndent {
- if !isSimple {
- *s += p.padding
- }
- }
- if elen > 0 || useGoXmlEmptyElemSyntax {
- if elen == 0 {
- *s += ">"
- }
- *s += `` + key + ">"
- } else {
- *s += `/>`
- }
- }
- if doIndent {
- if p.cnt > p.start {
- *s += "\n"
- }
- p.Outdent()
- }
-
- return nil
-}
-
-// ============================ sort interface implementation =================
-
-type attrList [][2]string
-
-func (a attrList) Len() int {
- return len(a)
-}
-
-func (a attrList) Swap(i, j int) {
- a[i], a[j] = a[j], a[i]
-}
-
-func (a attrList) Less(i, j int) bool {
- return a[i][0] <= a[j][0]
-}
-
-type elemList [][2]interface{}
-
-func (e elemList) Len() int {
- return len(e)
-}
-
-func (e elemList) Swap(i, j int) {
- e[i], e[j] = e[j], e[i]
-}
-
-func (e elemList) Less(i, j int) bool {
- return e[i][0].(string) <= e[j][0].(string)
-}
diff --git a/vendor/github.com/clbanning/mxj/xmlseq.go b/vendor/github.com/clbanning/mxj/xmlseq.go
deleted file mode 100644
index 6be73ae6..00000000
--- a/vendor/github.com/clbanning/mxj/xmlseq.go
+++ /dev/null
@@ -1,828 +0,0 @@
-// Copyright 2012-2016 Charles Banning. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file
-
-// xmlseq.go - version of xml.go with sequence # injection on Decoding and sorting on Encoding.
-// Also, handles comments, directives and process instructions.
-
-package mxj
-
-import (
- "bytes"
- "encoding/xml"
- "errors"
- "fmt"
- "io"
- "sort"
- "strings"
-)
-
-var NoRoot = errors.New("no root key")
-var NO_ROOT = NoRoot // maintain backwards compatibility
-
-// ------------------- NewMapXmlSeq & NewMapXmlSeqReader ... -------------------------
-
-// This is only useful if you want to re-encode the Map as XML using mv.XmlSeq(), etc., to preserve the original structure.
-// The xml.Decoder.RawToken method is used to parse the XML, so there is no checking for appropriate xml.EndElement values;
-// thus, it is assumed that the XML is valid.
-//
-// NewMapXmlSeq - convert a XML doc into a Map with elements id'd with decoding sequence int - #seq.
-// If the optional argument 'cast' is 'true', then values will be converted to boolean or float64 if possible.
-// NOTE: "#seq" key/value pairs are removed on encoding with mv.XmlSeq() / mv.XmlSeqIndent().
-// • attributes are a map - map["#attr"]map["attr_key"]map[string]interface{}{"#text":, "#seq":}
-// • all simple elements are decoded as map["#text"]interface{} with a "#seq" k:v pair, as well.
-// • lists always decode as map["list_tag"][]map[string]interface{} where the array elements are maps that
-// include a "#seq" k:v pair based on sequence they are decoded. Thus, XML like:
-//
-// value 1
-// value 2
-// value 3
-//
-// is decoded as:
-// doc :
-// ltag :[[]interface{}]
-// [item: 0]
-// #seq :[int] 0
-// #text :[string] value 1
-// [item: 1]
-// #seq :[int] 2
-// #text :[string] value 3
-// newtag :
-// #seq :[int] 1
-// #text :[string] value 2
-// It will encode in proper sequence even though the Map representation merges all "ltag" elements in an array.
-// • comments - "" - are decoded as map["#comment"]map["#text"]"cmnt_text" with a "#seq" k:v pair.
-// • directives - "" - are decoded as map["#directive"]map[#text"]"directive_text" with a "#seq" k:v pair.
-// • process instructions - "" - are decoded as map["#procinst"]interface{} where the #procinst value
-// is of map[string]interface{} type with the following keys: #target, #inst, and #seq.
-// • comments, directives, and procinsts that are NOT part of a document with a root key will be returned as
-// map[string]interface{} and the error value 'NoRoot'.
-// • note: ": tag preserve the
-// ":" notation rather than stripping it as with NewMapXml().
-// 2. Attribute keys for name space prefix declarations preserve "xmlns:" notation.
-func NewMapXmlSeq(xmlVal []byte, cast ...bool) (Map, error) {
- var r bool
- if len(cast) == 1 {
- r = cast[0]
- }
- return xmlSeqToMap(xmlVal, r)
-}
-
-// This is only useful if you want to re-encode the Map as XML using mv.XmlSeq(), etc., to preserve the original structure.
-//
-// Get next XML doc from an io.Reader as a Map value. Returns Map value.
-// NOTES:
-// 1. The 'xmlReader' will be parsed looking for an xml.StartElement, xml.Comment, etc., so BOM and other
-// extraneous xml.CharData will be ignored unless io.EOF is reached first.
-// 2. CoerceKeysToLower() is NOT recognized, since the intent here is to eventually call m.XmlSeq() to
-// re-encode the message in its original structure.
-// 3. If CoerceKeysToSnakeCase() has been called, then all key values will be converted to snake case.
-func NewMapXmlSeqReader(xmlReader io.Reader, cast ...bool) (Map, error) {
- var r bool
- if len(cast) == 1 {
- r = cast[0]
- }
-
- // We need to put an *os.File reader in a ByteReader or the xml.NewDecoder
- // will wrap it in a bufio.Reader and seek on the file beyond where the
- // xml.Decoder parses!
- if _, ok := xmlReader.(io.ByteReader); !ok {
- xmlReader = myByteReader(xmlReader) // see code at EOF
- }
-
- // build the map
- return xmlSeqReaderToMap(xmlReader, r)
-}
-
-// This is only useful if you want to re-encode the Map as XML using mv.XmlSeq(), etc., to preserve the original structure.
-//
-// Get next XML doc from an io.Reader as a Map value. Returns Map value and slice with the raw XML.
-// NOTES:
-// 1. Due to the implementation of xml.Decoder, the raw XML off the reader is buffered to []byte
-// using a ByteReader. If the io.Reader is an os.File, there may be significant performance impact.
-// See the examples - getmetrics1.go through getmetrics4.go - for comparative use cases on a large
-// data set. If the io.Reader is wrapping a []byte value in-memory, however, such as http.Request.Body
-// you CAN use it to efficiently unmarshal a XML doc and retrieve the raw XML in a single call.
-// 2. The 'raw' return value may be larger than the XML text value.
-// 3. The 'xmlReader' will be parsed looking for an xml.StartElement, xml.Comment, etc., so BOM and other
-// extraneous xml.CharData will be ignored unless io.EOF is reached first.
-// 4. CoerceKeysToLower() is NOT recognized, since the intent here is to eventually call m.XmlSeq() to
-// re-encode the message in its original structure.
-// 5. If CoerceKeysToSnakeCase() has been called, then all key values will be converted to snake case.
-func NewMapXmlSeqReaderRaw(xmlReader io.Reader, cast ...bool) (Map, []byte, error) {
- var r bool
- if len(cast) == 1 {
- r = cast[0]
- }
- // create TeeReader so we can retrieve raw XML
- buf := make([]byte, 0)
- wb := bytes.NewBuffer(buf)
- trdr := myTeeReader(xmlReader, wb)
-
- m, err := xmlSeqReaderToMap(trdr, r)
-
- // retrieve the raw XML that was decoded
- b := wb.Bytes()
-
- // err may be NoRoot
- return m, b, err
-}
-
-// xmlSeqReaderToMap() - parse a XML io.Reader to a map[string]interface{} value
-func xmlSeqReaderToMap(rdr io.Reader, r bool) (map[string]interface{}, error) {
- // parse the Reader
- p := xml.NewDecoder(rdr)
- if CustomDecoder != nil {
- useCustomDecoder(p)
- } else {
- p.CharsetReader = XmlCharsetReader
- }
- return xmlSeqToMapParser("", nil, p, r)
-}
-
-// xmlSeqToMap - convert a XML doc into map[string]interface{} value
-func xmlSeqToMap(doc []byte, r bool) (map[string]interface{}, error) {
- b := bytes.NewReader(doc)
- p := xml.NewDecoder(b)
- if CustomDecoder != nil {
- useCustomDecoder(p)
- } else {
- p.CharsetReader = XmlCharsetReader
- }
- return xmlSeqToMapParser("", nil, p, r)
-}
-
-// ===================================== where the work happens =============================
-
-// xmlSeqToMapParser - load a 'clean' XML doc into a map[string]interface{} directly.
-// Add #seq tag value for each element decoded - to be used for Encoding later.
-func xmlSeqToMapParser(skey string, a []xml.Attr, p *xml.Decoder, r bool) (map[string]interface{}, error) {
- if snakeCaseKeys {
- skey = strings.Replace(skey, "-", "_", -1)
- }
-
- // NOTE: all attributes and sub-elements parsed into 'na', 'na' is returned as value for 'skey' in 'n'.
- var n, na map[string]interface{}
- var seq int // for including seq num when decoding
-
- // Allocate maps and load attributes, if any.
- // NOTE: on entry from NewMapXml(), etc., skey=="", and we fall through
- // to get StartElement then recurse with skey==xml.StartElement.Name.Local
- // where we begin allocating map[string]interface{} values 'n' and 'na'.
- if skey != "" {
- // 'n' only needs one slot - save call to runtime•hashGrow()
- // 'na' we don't know
- n = make(map[string]interface{}, 1)
- na = make(map[string]interface{})
- if len(a) > 0 {
- // xml.Attr is decoded into: map["#attr"]map[]interface{}
- // where interface{} is map[string]interface{}{"#text":, "#seq":}
- aa := make(map[string]interface{}, len(a))
- for i, v := range a {
- if snakeCaseKeys {
- v.Name.Local = strings.Replace(v.Name.Local, "-", "_", -1)
- }
- if len(v.Name.Space) > 0 {
- aa[v.Name.Space+`:`+v.Name.Local] = map[string]interface{}{"#text": cast(v.Value, r), "#seq": i}
- } else {
- aa[v.Name.Local] = map[string]interface{}{"#text": cast(v.Value, r), "#seq": i}
- }
- }
- na["#attr"] = aa
- }
- }
-
- // Return XMPP message.
- if handleXMPPStreamTag && skey == "stream:stream" {
- n[skey] = na
- return n, nil
- }
-
- for {
- t, err := p.RawToken()
- if err != nil {
- if err != io.EOF {
- return nil, errors.New("xml.Decoder.Token() - " + err.Error())
- }
- return nil, err
- }
- switch t.(type) {
- case xml.StartElement:
- tt := t.(xml.StartElement)
-
- // First call to xmlSeqToMapParser() doesn't pass xml.StartElement - the map key.
- // So when the loop is first entered, the first token is the root tag along
- // with any attributes, which we process here.
- //
- // Subsequent calls to xmlSeqToMapParser() will pass in tag+attributes for
- // processing before getting the next token which is the element value,
- // which is done above.
- if skey == "" {
- if len(tt.Name.Space) > 0 {
- return xmlSeqToMapParser(tt.Name.Space+`:`+tt.Name.Local, tt.Attr, p, r)
- } else {
- return xmlSeqToMapParser(tt.Name.Local, tt.Attr, p, r)
- }
- }
-
- // If not initializing the map, parse the element.
- // len(nn) == 1, necessarily - it is just an 'n'.
- var nn map[string]interface{}
- if len(tt.Name.Space) > 0 {
- nn, err = xmlSeqToMapParser(tt.Name.Space+`:`+tt.Name.Local, tt.Attr, p, r)
- } else {
- nn, err = xmlSeqToMapParser(tt.Name.Local, tt.Attr, p, r)
- }
- if err != nil {
- return nil, err
- }
-
- // The nn map[string]interface{} value is a na[nn_key] value.
- // We need to see if nn_key already exists - means we're parsing a list.
- // This may require converting na[nn_key] value into []interface{} type.
- // First, extract the key:val for the map - it's a singleton.
- var key string
- var val interface{}
- for key, val = range nn {
- break
- }
-
- // add "#seq" k:v pair -
- // Sequence number included even in list elements - this should allow us
- // to properly resequence even something goofy like:
- // item 1
- // item 2
- // item 3
- // where all the "list" subelements are decoded into an array.
- switch val.(type) {
- case map[string]interface{}:
- val.(map[string]interface{})["#seq"] = seq
- seq++
- case interface{}: // a non-nil simple element: string, float64, bool
- v := map[string]interface{}{"#text": val, "#seq": seq}
- seq++
- val = v
- }
-
- // 'na' holding sub-elements of n.
- // See if 'key' already exists.
- // If 'key' exists, then this is a list, if not just add key:val to na.
- if v, ok := na[key]; ok {
- var a []interface{}
- switch v.(type) {
- case []interface{}:
- a = v.([]interface{})
- default: // anything else - note: v.(type) != nil
- a = []interface{}{v}
- }
- a = append(a, val)
- na[key] = a
- } else {
- na[key] = val // save it as a singleton
- }
- case xml.EndElement:
- if skey != "" {
- tt := t.(xml.EndElement)
- if snakeCaseKeys {
- tt.Name.Local = strings.Replace(tt.Name.Local, "-", "_", -1)
- }
- var name string
- if len(tt.Name.Space) > 0 {
- name = tt.Name.Space + `:` + tt.Name.Local
- } else {
- name = tt.Name.Local
- }
- if skey != name {
- return nil, fmt.Errorf("element %s not properly terminated, got %s at #%d",
- skey, name, p.InputOffset())
- }
- }
- // len(n) > 0 if this is a simple element w/o xml.Attrs - see xml.CharData case.
- if len(n) == 0 {
- // If len(na)==0 we have an empty element == "";
- // it has no xml.Attr nor xml.CharData.
- // Empty element content will be map["etag"]map["#text"]""
- // after #seq injection - map["etag"]map["#seq"]seq - after return.
- if len(na) > 0 {
- n[skey] = na
- } else {
- n[skey] = "" // empty element
- }
- }
- return n, nil
- case xml.CharData:
- // clean up possible noise
- tt := strings.Trim(string(t.(xml.CharData)), "\t\r\b\n ")
- if skey == "" {
- // per Adrian (http://www.adrianlungu.com/) catch stray text
- // in decoder stream -
- // https://github.com/clbanning/mxj/pull/14#issuecomment-182816374
- // NOTE: CharSetReader must be set to non-UTF-8 CharSet or you'll get
- // a p.Token() decoding error when the BOM is UTF-16 or UTF-32.
- continue
- }
- if len(tt) > 0 {
- // every simple element is a #text and has #seq associated with it
- na["#text"] = cast(tt, r)
- na["#seq"] = seq
- seq++
- }
- case xml.Comment:
- if n == nil { // no root 'key'
- n = map[string]interface{}{"#comment": string(t.(xml.Comment))}
- return n, NoRoot
- }
- cm := make(map[string]interface{}, 2)
- cm["#text"] = string(t.(xml.Comment))
- cm["#seq"] = seq
- seq++
- na["#comment"] = cm
- case xml.Directive:
- if n == nil { // no root 'key'
- n = map[string]interface{}{"#directive": string(t.(xml.Directive))}
- return n, NoRoot
- }
- dm := make(map[string]interface{}, 2)
- dm["#text"] = string(t.(xml.Directive))
- dm["#seq"] = seq
- seq++
- na["#directive"] = dm
- case xml.ProcInst:
- if n == nil {
- na = map[string]interface{}{"#target": t.(xml.ProcInst).Target, "#inst": string(t.(xml.ProcInst).Inst)}
- n = map[string]interface{}{"#procinst": na}
- return n, NoRoot
- }
- pm := make(map[string]interface{}, 3)
- pm["#target"] = t.(xml.ProcInst).Target
- pm["#inst"] = string(t.(xml.ProcInst).Inst)
- pm["#seq"] = seq
- seq++
- na["#procinst"] = pm
- default:
- // noop - shouldn't ever get here, now, since we handle all token types
- }
- }
-}
-
-// ------------------ END: NewMapXml & NewMapXmlReader -------------------------
-
-// --------------------- mv.XmlSeq & mv.XmlSeqWriter -------------------------
-
-// This should ONLY be used on Map values that were decoded using NewMapXmlSeq() & co.
-//
-// Encode a Map as XML with elements sorted on #seq. The companion of NewMapXmlSeq().
-// The following rules apply.
-// - The key label "#text" is treated as the value for a simple element with attributes.
-// - The "#seq" key is used to seqence the subelements or attributes but is ignored for writing.
-// - The "#attr" map key identifies the map of attribute map[string]interface{} values with "#text" key.
-// - The "#comment" map key identifies a comment in the value "#text" map entry - .
-// - The "#directive" map key identifies a directive in the value "#text" map entry - .
-// - The "#procinst" map key identifies a process instruction in the value "#target" and "#inst"
-// map entries - .
-// - Value type encoding:
-// > string, bool, float64, int, int32, int64, float32: per "%v" formating
-// > []bool, []uint8: by casting to string
-// > structures, etc.: handed to xml.Marshal() - if there is an error, the element
-// value is "UNKNOWN"
-// - Elements with only attribute values or are null are terminated using "/>" unless XmlGoEmptyElemSystax() called.
-// - If len(mv) == 1 and no rootTag is provided, then the map key is used as the root tag, possible.
-// Thus, `{ "key":"value" }` encodes as "value".
-func (mv Map) XmlSeq(rootTag ...string) ([]byte, error) {
- m := map[string]interface{}(mv)
- var err error
- s := new(string)
- p := new(pretty) // just a stub
-
- if len(m) == 1 && len(rootTag) == 0 {
- for key, value := range m {
- // if it's an array, see if all values are map[string]interface{}
- // we force a new root tag if we'll end up with no key:value in the list
- // so: key:[string_val, bool:true] --> string_valtrue
- switch value.(type) {
- case []interface{}:
- for _, v := range value.([]interface{}) {
- switch v.(type) {
- case map[string]interface{}: // noop
- default: // anything else
- err = mapToXmlSeqIndent(false, s, DefaultRootTag, m, p)
- goto done
- }
- }
- }
- err = mapToXmlSeqIndent(false, s, key, value, p)
- }
- } else if len(rootTag) == 1 {
- err = mapToXmlSeqIndent(false, s, rootTag[0], m, p)
- } else {
- err = mapToXmlSeqIndent(false, s, DefaultRootTag, m, p)
- }
-done:
- return []byte(*s), err
-}
-
-// The following implementation is provided only for symmetry with NewMapXmlReader[Raw]
-// The names will also provide a key for the number of return arguments.
-
-// This should ONLY be used on Map values that were decoded using NewMapXmlSeq() & co.
-//
-// Writes the Map as XML on the Writer.
-// See XmlSeq() for encoding rules.
-func (mv Map) XmlSeqWriter(xmlWriter io.Writer, rootTag ...string) error {
- x, err := mv.XmlSeq(rootTag...)
- if err != nil {
- return err
- }
-
- _, err = xmlWriter.Write(x)
- return err
-}
-
-// This should ONLY be used on Map values that were decoded using NewMapXmlSeq() & co.
-//
-// Writes the Map as XML on the Writer. []byte is the raw XML that was written.
-// See XmlSeq() for encoding rules.
-func (mv Map) XmlSeqWriterRaw(xmlWriter io.Writer, rootTag ...string) ([]byte, error) {
- x, err := mv.XmlSeq(rootTag...)
- if err != nil {
- return x, err
- }
-
- _, err = xmlWriter.Write(x)
- return x, err
-}
-
-// This should ONLY be used on Map values that were decoded using NewMapXmlSeq() & co.
-//
-// Writes the Map as pretty XML on the Writer.
-// See Xml() for encoding rules.
-func (mv Map) XmlSeqIndentWriter(xmlWriter io.Writer, prefix, indent string, rootTag ...string) error {
- x, err := mv.XmlSeqIndent(prefix, indent, rootTag...)
- if err != nil {
- return err
- }
-
- _, err = xmlWriter.Write(x)
- return err
-}
-
-// This should ONLY be used on Map values that were decoded using NewMapXmlSeq() & co.
-//
-// Writes the Map as pretty XML on the Writer. []byte is the raw XML that was written.
-// See XmlSeq() for encoding rules.
-func (mv Map) XmlSeqIndentWriterRaw(xmlWriter io.Writer, prefix, indent string, rootTag ...string) ([]byte, error) {
- x, err := mv.XmlSeqIndent(prefix, indent, rootTag...)
- if err != nil {
- return x, err
- }
-
- _, err = xmlWriter.Write(x)
- return x, err
-}
-
-// -------------------- END: mv.Xml & mv.XmlWriter -------------------------------
-
-// ---------------------- XmlSeqIndent ----------------------------
-
-// This should ONLY be used on Map values that were decoded using NewMapXmlSeq() & co.
-//
-// Encode a map[string]interface{} as a pretty XML string.
-// See mv.XmlSeq() for encoding rules.
-func (mv Map) XmlSeqIndent(prefix, indent string, rootTag ...string) ([]byte, error) {
- m := map[string]interface{}(mv)
-
- var err error
- s := new(string)
- p := new(pretty)
- p.indent = indent
- p.padding = prefix
-
- if len(m) == 1 && len(rootTag) == 0 {
- // this can extract the key for the single map element
- // use it if it isn't a key for a list
- for key, value := range m {
- if _, ok := value.([]interface{}); ok {
- err = mapToXmlSeqIndent(true, s, DefaultRootTag, m, p)
- } else {
- err = mapToXmlSeqIndent(true, s, key, value, p)
- }
- }
- } else if len(rootTag) == 1 {
- err = mapToXmlSeqIndent(true, s, rootTag[0], m, p)
- } else {
- err = mapToXmlSeqIndent(true, s, DefaultRootTag, m, p)
- }
- return []byte(*s), err
-}
-
-// where the work actually happens
-// returns an error if an attribute is not atomic
-func mapToXmlSeqIndent(doIndent bool, s *string, key string, value interface{}, pp *pretty) error {
- var endTag bool
- var isSimple bool
- var noEndTag bool
- var elen int
- var ss string
- p := &pretty{pp.indent, pp.cnt, pp.padding, pp.mapDepth, pp.start}
-
- switch value.(type) {
- case map[string]interface{}, []byte, string, float64, bool, int, int32, int64, float32:
- if doIndent {
- *s += p.padding
- }
- if key != "#comment" && key != "#directive" && key != "#procinst" {
- *s += `<` + key
- }
- }
- switch value.(type) {
- case map[string]interface{}:
- val := value.(map[string]interface{})
-
- if key == "#comment" {
- *s += ``
- noEndTag = true
- break
- }
-
- if key == "#directive" {
- *s += ``
- noEndTag = true
- break
- }
-
- if key == "#procinst" {
- *s += `` + val["#target"].(string) + ` ` + val["#inst"].(string) + `?>`
- noEndTag = true
- break
- }
-
- haveAttrs := false
- // process attributes first
- if v, ok := val["#attr"].(map[string]interface{}); ok {
- // First, unroll the map[string]interface{} into a []keyval array.
- // Then sequence it.
- kv := make([]keyval, len(v))
- n := 0
- for ak, av := range v {
- kv[n] = keyval{ak, av}
- n++
- }
- sort.Sort(elemListSeq(kv))
- // Now encode the attributes in original decoding sequence, using keyval array.
- for _, a := range kv {
- vv := a.v.(map[string]interface{})
- switch vv["#text"].(type) {
- case string:
- if xmlEscapeChars {
- ss = escapeChars(vv["#text"].(string))
- } else {
- ss = vv["#text"].(string)
- }
- *s += ` ` + a.k + `="` + ss + `"`
- case float64, bool, int, int32, int64, float32:
- *s += ` ` + a.k + `="` + fmt.Sprintf("%v", vv["#text"]) + `"`
- case []byte:
- if xmlEscapeChars {
- ss = escapeChars(string(vv["#text"].([]byte)))
- } else {
- ss = string(vv["#text"].([]byte))
- }
- *s += ` ` + a.k + `="` + ss + `"`
- default:
- return fmt.Errorf("invalid attribute value for: %s", a.k)
- }
- }
- haveAttrs = true
- }
-
- // simple element?
- // every map value has, at least, "#seq" and, perhaps, "#text" and/or "#attr"
- _, seqOK := val["#seq"] // have key
- if v, ok := val["#text"]; ok && ((len(val) == 3 && haveAttrs) || (len(val) == 2 && !haveAttrs)) && seqOK {
- if stmp, ok := v.(string); ok && stmp != "" {
- if xmlEscapeChars {
- stmp = escapeChars(stmp)
- }
- *s += ">" + stmp
- endTag = true
- elen = 1
- }
- isSimple = true
- break
- } else if !ok && ((len(val) == 2 && haveAttrs) || (len(val) == 1 && !haveAttrs)) && seqOK {
- // here no #text but have #seq or #seq+#attr
- endTag = false
- break
- }
-
- // we now need to sequence everything except attributes
- // 'kv' will hold everything that needs to be written
- kv := make([]keyval, 0)
- for k, v := range val {
- if k == "#attr" { // already processed
- continue
- }
- if k == "#seq" { // ignore - just for sorting
- continue
- }
- switch v.(type) {
- case []interface{}:
- // unwind the array as separate entries
- for _, vv := range v.([]interface{}) {
- kv = append(kv, keyval{k, vv})
- }
- default:
- kv = append(kv, keyval{k, v})
- }
- }
-
- // close tag with possible attributes
- *s += ">"
- if doIndent {
- *s += "\n"
- }
- // something more complex
- p.mapDepth++
- sort.Sort(elemListSeq(kv))
- i := 0
- for _, v := range kv {
- switch v.v.(type) {
- case []interface{}:
- default:
- if i == 0 && doIndent {
- p.Indent()
- }
- }
- i++
- if err := mapToXmlSeqIndent(doIndent, s, v.k, v.v, p); err != nil {
- return err
- }
- switch v.v.(type) {
- case []interface{}: // handled in []interface{} case
- default:
- if doIndent {
- p.Outdent()
- }
- }
- i--
- }
- p.mapDepth--
- endTag = true
- elen = 1 // we do have some content other than attrs
- case []interface{}:
- for _, v := range value.([]interface{}) {
- if doIndent {
- p.Indent()
- }
- if err := mapToXmlSeqIndent(doIndent, s, key, v, p); err != nil {
- return err
- }
- if doIndent {
- p.Outdent()
- }
- }
- return nil
- case nil:
- // terminate the tag
- if doIndent {
- *s += p.padding
- }
- *s += "<" + key
- endTag, isSimple = true, true
- break
- default: // handle anything - even goofy stuff
- elen = 0
- switch value.(type) {
- case string:
- if xmlEscapeChars {
- ss = escapeChars(value.(string))
- } else {
- ss = value.(string)
- }
- elen = len(ss)
- if elen > 0 {
- *s += ">" + ss
- }
- case float64, bool, int, int32, int64, float32:
- v := fmt.Sprintf("%v", value)
- elen = len(v)
- if elen > 0 {
- *s += ">" + v
- }
- case []byte: // NOTE: byte is just an alias for uint8
- // similar to how xml.Marshal handles []byte structure members
- if xmlEscapeChars {
- ss = escapeChars(string(value.([]byte)))
- } else {
- ss = string(value.([]byte))
- }
- elen = len(ss)
- if elen > 0 {
- *s += ">" + ss
- }
- default:
- var v []byte
- var err error
- if doIndent {
- v, err = xml.MarshalIndent(value, p.padding, p.indent)
- } else {
- v, err = xml.Marshal(value)
- }
- if err != nil {
- *s += ">UNKNOWN"
- } else {
- elen = len(v)
- if elen > 0 {
- *s += string(v)
- }
- }
- }
- isSimple = true
- endTag = true
- }
- if endTag && !noEndTag {
- if doIndent {
- if !isSimple {
- *s += p.padding
- }
- }
- switch value.(type) {
- case map[string]interface{}, []byte, string, float64, bool, int, int32, int64, float32:
- if elen > 0 || useGoXmlEmptyElemSyntax {
- if elen == 0 {
- *s += ">"
- }
- *s += `` + key + ">"
- } else {
- *s += `/>`
- }
- }
- } else if !noEndTag {
- if useGoXmlEmptyElemSyntax {
- *s += `` + key + ">"
- // *s += ">" + key + ">"
- } else {
- *s += "/>"
- }
- }
- if doIndent {
- if p.cnt > p.start {
- *s += "\n"
- }
- p.Outdent()
- }
-
- return nil
-}
-
-// the element sort implementation
-
-type keyval struct {
- k string
- v interface{}
-}
-type elemListSeq []keyval
-
-func (e elemListSeq) Len() int {
- return len(e)
-}
-
-func (e elemListSeq) Swap(i, j int) {
- e[i], e[j] = e[j], e[i]
-}
-
-func (e elemListSeq) Less(i, j int) bool {
- var iseq, jseq int
- var ok bool
- if iseq, ok = e[i].v.(map[string]interface{})["#seq"].(int); !ok {
- iseq = 9999999
- }
-
- if jseq, ok = e[j].v.(map[string]interface{})["#seq"].(int); !ok {
- jseq = 9999999
- }
-
- return iseq <= jseq
-}
-
-// =============== https://groups.google.com/forum/#!topic/golang-nuts/lHPOHD-8qio
-
-// BeautifyXml (re)formats an XML doc similar to Map.XmlIndent().
-func BeautifyXml(b []byte, prefix, indent string) ([]byte, error) {
- x, err := NewMapXmlSeq(b)
- if err != nil {
- return nil, err
- }
- return x.XmlSeqIndent(prefix, indent)
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/.editorconfig b/vendor/github.com/fsnotify/fsnotify/.editorconfig
deleted file mode 100644
index fad89585..00000000
--- a/vendor/github.com/fsnotify/fsnotify/.editorconfig
+++ /dev/null
@@ -1,12 +0,0 @@
-root = true
-
-[*.go]
-indent_style = tab
-indent_size = 4
-insert_final_newline = true
-
-[*.{yml,yaml}]
-indent_style = space
-indent_size = 2
-insert_final_newline = true
-trim_trailing_whitespace = true
diff --git a/vendor/github.com/fsnotify/fsnotify/.gitattributes b/vendor/github.com/fsnotify/fsnotify/.gitattributes
deleted file mode 100644
index 32f1001b..00000000
--- a/vendor/github.com/fsnotify/fsnotify/.gitattributes
+++ /dev/null
@@ -1 +0,0 @@
-go.sum linguist-generated
diff --git a/vendor/github.com/fsnotify/fsnotify/.gitignore b/vendor/github.com/fsnotify/fsnotify/.gitignore
deleted file mode 100644
index 1d89d85c..00000000
--- a/vendor/github.com/fsnotify/fsnotify/.gitignore
+++ /dev/null
@@ -1,6 +0,0 @@
-# go test -c output
-*.test
-*.test.exe
-
-# Output of go build ./cmd/fsnotify
-/fsnotify
diff --git a/vendor/github.com/fsnotify/fsnotify/.mailmap b/vendor/github.com/fsnotify/fsnotify/.mailmap
deleted file mode 100644
index a04f2907..00000000
--- a/vendor/github.com/fsnotify/fsnotify/.mailmap
+++ /dev/null
@@ -1,2 +0,0 @@
-Chris Howey
-Nathan Youngman <4566+nathany@users.noreply.github.com>
diff --git a/vendor/github.com/fsnotify/fsnotify/CHANGELOG.md b/vendor/github.com/fsnotify/fsnotify/CHANGELOG.md
deleted file mode 100644
index 77f9593b..00000000
--- a/vendor/github.com/fsnotify/fsnotify/CHANGELOG.md
+++ /dev/null
@@ -1,470 +0,0 @@
-# Changelog
-
-All notable changes to this project will be documented in this file.
-
-The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/),
-and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
-
-## [Unreleased]
-
-Nothing yet.
-
-## [1.6.0] - 2022-10-13
-
-This version of fsnotify needs Go 1.16 (this was already the case since 1.5.1,
-but not documented). It also increases the minimum Linux version to 2.6.32.
-
-### Additions
-
-- all: add `Event.Has()` and `Op.Has()` ([#477])
-
- This makes checking events a lot easier; for example:
-
- if event.Op&Write == Write && !(event.Op&Remove == Remove) {
- }
-
- Becomes:
-
- if event.Has(Write) && !event.Has(Remove) {
- }
-
-- all: add cmd/fsnotify ([#463])
-
- A command-line utility for testing and some examples.
-
-### Changes and fixes
-
-- inotify: don't ignore events for files that don't exist ([#260], [#470])
-
- Previously the inotify watcher would call `os.Lstat()` to check if a file
- still exists before emitting events.
-
- This was inconsistent with other platforms and resulted in inconsistent event
- reporting (e.g. when a file is quickly removed and re-created), and generally
- a source of confusion. It was added in 2013 to fix a memory leak that no
- longer exists.
-
-- all: return `ErrNonExistentWatch` when `Remove()` is called on a path that's
- not watched ([#460])
-
-- inotify: replace epoll() with non-blocking inotify ([#434])
-
- Non-blocking inotify was not generally available at the time this library was
- written in 2014, but now it is. As a result, the minimum Linux version is
- bumped from 2.6.27 to 2.6.32. This hugely simplifies the code and is faster.
-
-- kqueue: don't check for events every 100ms ([#480])
-
- The watcher would wake up every 100ms, even when there was nothing to do. Now
- it waits until there is something to do.
-
-- macos: retry opening files on EINTR ([#475])
-
-- kqueue: skip unreadable files ([#479])
-
- kqueue requires a file descriptor for every file in a directory; this would
- fail if a file was unreadable by the current user. Now these files are simply
- skipped.
-
-- windows: fix renaming a watched directory if the parent is also watched ([#370])
-
-- windows: increase buffer size from 4K to 64K ([#485])
-
-- windows: close file handle on Remove() ([#288])
-
-- kqueue: put pathname in the error if watching a file fails ([#471])
-
-- inotify, windows: calling Close() more than once could race ([#465])
-
-- kqueue: improve Close() performance ([#233])
-
-- all: various documentation additions and clarifications.
-
-[#233]: https://github.com/fsnotify/fsnotify/pull/233
-[#260]: https://github.com/fsnotify/fsnotify/pull/260
-[#288]: https://github.com/fsnotify/fsnotify/pull/288
-[#370]: https://github.com/fsnotify/fsnotify/pull/370
-[#434]: https://github.com/fsnotify/fsnotify/pull/434
-[#460]: https://github.com/fsnotify/fsnotify/pull/460
-[#463]: https://github.com/fsnotify/fsnotify/pull/463
-[#465]: https://github.com/fsnotify/fsnotify/pull/465
-[#470]: https://github.com/fsnotify/fsnotify/pull/470
-[#471]: https://github.com/fsnotify/fsnotify/pull/471
-[#475]: https://github.com/fsnotify/fsnotify/pull/475
-[#477]: https://github.com/fsnotify/fsnotify/pull/477
-[#479]: https://github.com/fsnotify/fsnotify/pull/479
-[#480]: https://github.com/fsnotify/fsnotify/pull/480
-[#485]: https://github.com/fsnotify/fsnotify/pull/485
-
-## [1.5.4] - 2022-04-25
-
-* Windows: add missing defer to `Watcher.WatchList` [#447](https://github.com/fsnotify/fsnotify/pull/447)
-* go.mod: use latest x/sys [#444](https://github.com/fsnotify/fsnotify/pull/444)
-* Fix compilation for OpenBSD [#443](https://github.com/fsnotify/fsnotify/pull/443)
-
-## [1.5.3] - 2022-04-22
-
-* This version is retracted. An incorrect branch is published accidentally [#445](https://github.com/fsnotify/fsnotify/issues/445)
-
-## [1.5.2] - 2022-04-21
-
-* Add a feature to return the directories and files that are being monitored [#374](https://github.com/fsnotify/fsnotify/pull/374)
-* Fix potential crash on windows if `raw.FileNameLength` exceeds `syscall.MAX_PATH` [#361](https://github.com/fsnotify/fsnotify/pull/361)
-* Allow build on unsupported GOOS [#424](https://github.com/fsnotify/fsnotify/pull/424)
-* Don't set `poller.fd` twice in `newFdPoller` [#406](https://github.com/fsnotify/fsnotify/pull/406)
-* fix go vet warnings: call to `(*T).Fatalf` from a non-test goroutine [#416](https://github.com/fsnotify/fsnotify/pull/416)
-
-## [1.5.1] - 2021-08-24
-
-* Revert Add AddRaw to not follow symlinks [#394](https://github.com/fsnotify/fsnotify/pull/394)
-
-## [1.5.0] - 2021-08-20
-
-* Go: Increase minimum required version to Go 1.12 [#381](https://github.com/fsnotify/fsnotify/pull/381)
-* Feature: Add AddRaw method which does not follow symlinks when adding a watch [#289](https://github.com/fsnotify/fsnotify/pull/298)
-* Windows: Follow symlinks by default like on all other systems [#289](https://github.com/fsnotify/fsnotify/pull/289)
-* CI: Use GitHub Actions for CI and cover go 1.12-1.17
- [#378](https://github.com/fsnotify/fsnotify/pull/378)
- [#381](https://github.com/fsnotify/fsnotify/pull/381)
- [#385](https://github.com/fsnotify/fsnotify/pull/385)
-* Go 1.14+: Fix unsafe pointer conversion [#325](https://github.com/fsnotify/fsnotify/pull/325)
-
-## [1.4.9] - 2020-03-11
-
-* Move example usage to the readme #329. This may resolve #328.
-
-## [1.4.8] - 2020-03-10
-
-* CI: test more go versions (@nathany 1d13583d846ea9d66dcabbfefbfb9d8e6fb05216)
-* Tests: Queued inotify events could have been read by the test before max_queued_events was hit (@matthias-stone #265)
-* Tests: t.Fatalf -> t.Errorf in go routines (@gdey #266)
-* CI: Less verbosity (@nathany #267)
-* Tests: Darwin: Exchangedata is deprecated on 10.13 (@nathany #267)
-* Tests: Check if channels are closed in the example (@alexeykazakov #244)
-* CI: Only run golint on latest version of go and fix issues (@cpuguy83 #284)
-* CI: Add windows to travis matrix (@cpuguy83 #284)
-* Docs: Remover appveyor badge (@nathany 11844c0959f6fff69ba325d097fce35bd85a8e93)
-* Linux: create epoll and pipe fds with close-on-exec (@JohannesEbke #219)
-* Linux: open files with close-on-exec (@linxiulei #273)
-* Docs: Plan to support fanotify (@nathany ab058b44498e8b7566a799372a39d150d9ea0119 )
-* Project: Add go.mod (@nathany #309)
-* Project: Revise editor config (@nathany #309)
-* Project: Update copyright for 2019 (@nathany #309)
-* CI: Drop go1.8 from CI matrix (@nathany #309)
-* Docs: Updating the FAQ section for supportability with NFS & FUSE filesystems (@Pratik32 4bf2d1fec78374803a39307bfb8d340688f4f28e )
-
-## [1.4.7] - 2018-01-09
-
-* BSD/macOS: Fix possible deadlock on closing the watcher on kqueue (thanks @nhooyr and @glycerine)
-* Tests: Fix missing verb on format string (thanks @rchiossi)
-* Linux: Fix deadlock in Remove (thanks @aarondl)
-* Linux: Watch.Add improvements (avoid race, fix consistency, reduce garbage) (thanks @twpayne)
-* Docs: Moved FAQ into the README (thanks @vahe)
-* Linux: Properly handle inotify's IN_Q_OVERFLOW event (thanks @zeldovich)
-* Docs: replace references to OS X with macOS
-
-## [1.4.2] - 2016-10-10
-
-* Linux: use InotifyInit1 with IN_CLOEXEC to stop leaking a file descriptor to a child process when using fork/exec [#178](https://github.com/fsnotify/fsnotify/pull/178) (thanks @pattyshack)
-
-## [1.4.1] - 2016-10-04
-
-* Fix flaky inotify stress test on Linux [#177](https://github.com/fsnotify/fsnotify/pull/177) (thanks @pattyshack)
-
-## [1.4.0] - 2016-10-01
-
-* add a String() method to Event.Op [#165](https://github.com/fsnotify/fsnotify/pull/165) (thanks @oozie)
-
-## [1.3.1] - 2016-06-28
-
-* Windows: fix for double backslash when watching the root of a drive [#151](https://github.com/fsnotify/fsnotify/issues/151) (thanks @brunoqc)
-
-## [1.3.0] - 2016-04-19
-
-* Support linux/arm64 by [patching](https://go-review.googlesource.com/#/c/21971/) x/sys/unix and switching to to it from syscall (thanks @suihkulokki) [#135](https://github.com/fsnotify/fsnotify/pull/135)
-
-## [1.2.10] - 2016-03-02
-
-* Fix golint errors in windows.go [#121](https://github.com/fsnotify/fsnotify/pull/121) (thanks @tiffanyfj)
-
-## [1.2.9] - 2016-01-13
-
-kqueue: Fix logic for CREATE after REMOVE [#111](https://github.com/fsnotify/fsnotify/pull/111) (thanks @bep)
-
-## [1.2.8] - 2015-12-17
-
-* kqueue: fix race condition in Close [#105](https://github.com/fsnotify/fsnotify/pull/105) (thanks @djui for reporting the issue and @ppknap for writing a failing test)
-* inotify: fix race in test
-* enable race detection for continuous integration (Linux, Mac, Windows)
-
-## [1.2.5] - 2015-10-17
-
-* inotify: use epoll_create1 for arm64 support (requires Linux 2.6.27 or later) [#100](https://github.com/fsnotify/fsnotify/pull/100) (thanks @suihkulokki)
-* inotify: fix path leaks [#73](https://github.com/fsnotify/fsnotify/pull/73) (thanks @chamaken)
-* kqueue: watch for rename events on subdirectories [#83](https://github.com/fsnotify/fsnotify/pull/83) (thanks @guotie)
-* kqueue: avoid infinite loops from symlinks cycles [#101](https://github.com/fsnotify/fsnotify/pull/101) (thanks @illicitonion)
-
-## [1.2.1] - 2015-10-14
-
-* kqueue: don't watch named pipes [#98](https://github.com/fsnotify/fsnotify/pull/98) (thanks @evanphx)
-
-## [1.2.0] - 2015-02-08
-
-* inotify: use epoll to wake up readEvents [#66](https://github.com/fsnotify/fsnotify/pull/66) (thanks @PieterD)
-* inotify: closing watcher should now always shut down goroutine [#63](https://github.com/fsnotify/fsnotify/pull/63) (thanks @PieterD)
-* kqueue: close kqueue after removing watches, fixes [#59](https://github.com/fsnotify/fsnotify/issues/59)
-
-## [1.1.1] - 2015-02-05
-
-* inotify: Retry read on EINTR [#61](https://github.com/fsnotify/fsnotify/issues/61) (thanks @PieterD)
-
-## [1.1.0] - 2014-12-12
-
-* kqueue: rework internals [#43](https://github.com/fsnotify/fsnotify/pull/43)
- * add low-level functions
- * only need to store flags on directories
- * less mutexes [#13](https://github.com/fsnotify/fsnotify/issues/13)
- * done can be an unbuffered channel
- * remove calls to os.NewSyscallError
-* More efficient string concatenation for Event.String() [#52](https://github.com/fsnotify/fsnotify/pull/52) (thanks @mdlayher)
-* kqueue: fix regression in rework causing subdirectories to be watched [#48](https://github.com/fsnotify/fsnotify/issues/48)
-* kqueue: cleanup internal watch before sending remove event [#51](https://github.com/fsnotify/fsnotify/issues/51)
-
-## [1.0.4] - 2014-09-07
-
-* kqueue: add dragonfly to the build tags.
-* Rename source code files, rearrange code so exported APIs are at the top.
-* Add done channel to example code. [#37](https://github.com/fsnotify/fsnotify/pull/37) (thanks @chenyukang)
-
-## [1.0.3] - 2014-08-19
-
-* [Fix] Windows MOVED_TO now translates to Create like on BSD and Linux. [#36](https://github.com/fsnotify/fsnotify/issues/36)
-
-## [1.0.2] - 2014-08-17
-
-* [Fix] Missing create events on macOS. [#14](https://github.com/fsnotify/fsnotify/issues/14) (thanks @zhsso)
-* [Fix] Make ./path and path equivalent. (thanks @zhsso)
-
-## [1.0.0] - 2014-08-15
-
-* [API] Remove AddWatch on Windows, use Add.
-* Improve documentation for exported identifiers. [#30](https://github.com/fsnotify/fsnotify/issues/30)
-* Minor updates based on feedback from golint.
-
-## dev / 2014-07-09
-
-* Moved to [github.com/fsnotify/fsnotify](https://github.com/fsnotify/fsnotify).
-* Use os.NewSyscallError instead of returning errno (thanks @hariharan-uno)
-
-## dev / 2014-07-04
-
-* kqueue: fix incorrect mutex used in Close()
-* Update example to demonstrate usage of Op.
-
-## dev / 2014-06-28
-
-* [API] Don't set the Write Op for attribute notifications [#4](https://github.com/fsnotify/fsnotify/issues/4)
-* Fix for String() method on Event (thanks Alex Brainman)
-* Don't build on Plan 9 or Solaris (thanks @4ad)
-
-## dev / 2014-06-21
-
-* Events channel of type Event rather than *Event.
-* [internal] use syscall constants directly for inotify and kqueue.
-* [internal] kqueue: rename events to kevents and fileEvent to event.
-
-## dev / 2014-06-19
-
-* Go 1.3+ required on Windows (uses syscall.ERROR_MORE_DATA internally).
-* [internal] remove cookie from Event struct (unused).
-* [internal] Event struct has the same definition across every OS.
-* [internal] remove internal watch and removeWatch methods.
-
-## dev / 2014-06-12
-
-* [API] Renamed Watch() to Add() and RemoveWatch() to Remove().
-* [API] Pluralized channel names: Events and Errors.
-* [API] Renamed FileEvent struct to Event.
-* [API] Op constants replace methods like IsCreate().
-
-## dev / 2014-06-12
-
-* Fix data race on kevent buffer (thanks @tilaks) [#98](https://github.com/howeyc/fsnotify/pull/98)
-
-## dev / 2014-05-23
-
-* [API] Remove current implementation of WatchFlags.
- * current implementation doesn't take advantage of OS for efficiency
- * provides little benefit over filtering events as they are received, but has extra bookkeeping and mutexes
- * no tests for the current implementation
- * not fully implemented on Windows [#93](https://github.com/howeyc/fsnotify/issues/93#issuecomment-39285195)
-
-## [0.9.3] - 2014-12-31
-
-* kqueue: cleanup internal watch before sending remove event [#51](https://github.com/fsnotify/fsnotify/issues/51)
-
-## [0.9.2] - 2014-08-17
-
-* [Backport] Fix missing create events on macOS. [#14](https://github.com/fsnotify/fsnotify/issues/14) (thanks @zhsso)
-
-## [0.9.1] - 2014-06-12
-
-* Fix data race on kevent buffer (thanks @tilaks) [#98](https://github.com/howeyc/fsnotify/pull/98)
-
-## [0.9.0] - 2014-01-17
-
-* IsAttrib() for events that only concern a file's metadata [#79][] (thanks @abustany)
-* [Fix] kqueue: fix deadlock [#77][] (thanks @cespare)
-* [NOTICE] Development has moved to `code.google.com/p/go.exp/fsnotify` in preparation for inclusion in the Go standard library.
-
-## [0.8.12] - 2013-11-13
-
-* [API] Remove FD_SET and friends from Linux adapter
-
-## [0.8.11] - 2013-11-02
-
-* [Doc] Add Changelog [#72][] (thanks @nathany)
-* [Doc] Spotlight and double modify events on macOS [#62][] (reported by @paulhammond)
-
-## [0.8.10] - 2013-10-19
-
-* [Fix] kqueue: remove file watches when parent directory is removed [#71][] (reported by @mdwhatcott)
-* [Fix] kqueue: race between Close and readEvents [#70][] (reported by @bernerdschaefer)
-* [Doc] specify OS-specific limits in README (thanks @debrando)
-
-## [0.8.9] - 2013-09-08
-
-* [Doc] Contributing (thanks @nathany)
-* [Doc] update package path in example code [#63][] (thanks @paulhammond)
-* [Doc] GoCI badge in README (Linux only) [#60][]
-* [Doc] Cross-platform testing with Vagrant [#59][] (thanks @nathany)
-
-## [0.8.8] - 2013-06-17
-
-* [Fix] Windows: handle `ERROR_MORE_DATA` on Windows [#49][] (thanks @jbowtie)
-
-## [0.8.7] - 2013-06-03
-
-* [API] Make syscall flags internal
-* [Fix] inotify: ignore event changes
-* [Fix] race in symlink test [#45][] (reported by @srid)
-* [Fix] tests on Windows
-* lower case error messages
-
-## [0.8.6] - 2013-05-23
-
-* kqueue: Use EVT_ONLY flag on Darwin
-* [Doc] Update README with full example
-
-## [0.8.5] - 2013-05-09
-
-* [Fix] inotify: allow monitoring of "broken" symlinks (thanks @tsg)
-
-## [0.8.4] - 2013-04-07
-
-* [Fix] kqueue: watch all file events [#40][] (thanks @ChrisBuchholz)
-
-## [0.8.3] - 2013-03-13
-
-* [Fix] inoitfy/kqueue memory leak [#36][] (reported by @nbkolchin)
-* [Fix] kqueue: use fsnFlags for watching a directory [#33][] (reported by @nbkolchin)
-
-## [0.8.2] - 2013-02-07
-
-* [Doc] add Authors
-* [Fix] fix data races for map access [#29][] (thanks @fsouza)
-
-## [0.8.1] - 2013-01-09
-
-* [Fix] Windows path separators
-* [Doc] BSD License
-
-## [0.8.0] - 2012-11-09
-
-* kqueue: directory watching improvements (thanks @vmirage)
-* inotify: add `IN_MOVED_TO` [#25][] (requested by @cpisto)
-* [Fix] kqueue: deleting watched directory [#24][] (reported by @jakerr)
-
-## [0.7.4] - 2012-10-09
-
-* [Fix] inotify: fixes from https://codereview.appspot.com/5418045/ (ugorji)
-* [Fix] kqueue: preserve watch flags when watching for delete [#21][] (reported by @robfig)
-* [Fix] kqueue: watch the directory even if it isn't a new watch (thanks @robfig)
-* [Fix] kqueue: modify after recreation of file
-
-## [0.7.3] - 2012-09-27
-
-* [Fix] kqueue: watch with an existing folder inside the watched folder (thanks @vmirage)
-* [Fix] kqueue: no longer get duplicate CREATE events
-
-## [0.7.2] - 2012-09-01
-
-* kqueue: events for created directories
-
-## [0.7.1] - 2012-07-14
-
-* [Fix] for renaming files
-
-## [0.7.0] - 2012-07-02
-
-* [Feature] FSNotify flags
-* [Fix] inotify: Added file name back to event path
-
-## [0.6.0] - 2012-06-06
-
-* kqueue: watch files after directory created (thanks @tmc)
-
-## [0.5.1] - 2012-05-22
-
-* [Fix] inotify: remove all watches before Close()
-
-## [0.5.0] - 2012-05-03
-
-* [API] kqueue: return errors during watch instead of sending over channel
-* kqueue: match symlink behavior on Linux
-* inotify: add `DELETE_SELF` (requested by @taralx)
-* [Fix] kqueue: handle EINTR (reported by @robfig)
-* [Doc] Godoc example [#1][] (thanks @davecheney)
-
-## [0.4.0] - 2012-03-30
-
-* Go 1 released: build with go tool
-* [Feature] Windows support using winfsnotify
-* Windows does not have attribute change notifications
-* Roll attribute notifications into IsModify
-
-## [0.3.0] - 2012-02-19
-
-* kqueue: add files when watch directory
-
-## [0.2.0] - 2011-12-30
-
-* update to latest Go weekly code
-
-## [0.1.0] - 2011-10-19
-
-* kqueue: add watch on file creation to match inotify
-* kqueue: create file event
-* inotify: ignore `IN_IGNORED` events
-* event String()
-* linux: common FileEvent functions
-* initial commit
-
-[#79]: https://github.com/howeyc/fsnotify/pull/79
-[#77]: https://github.com/howeyc/fsnotify/pull/77
-[#72]: https://github.com/howeyc/fsnotify/issues/72
-[#71]: https://github.com/howeyc/fsnotify/issues/71
-[#70]: https://github.com/howeyc/fsnotify/issues/70
-[#63]: https://github.com/howeyc/fsnotify/issues/63
-[#62]: https://github.com/howeyc/fsnotify/issues/62
-[#60]: https://github.com/howeyc/fsnotify/issues/60
-[#59]: https://github.com/howeyc/fsnotify/issues/59
-[#49]: https://github.com/howeyc/fsnotify/issues/49
-[#45]: https://github.com/howeyc/fsnotify/issues/45
-[#40]: https://github.com/howeyc/fsnotify/issues/40
-[#36]: https://github.com/howeyc/fsnotify/issues/36
-[#33]: https://github.com/howeyc/fsnotify/issues/33
-[#29]: https://github.com/howeyc/fsnotify/issues/29
-[#25]: https://github.com/howeyc/fsnotify/issues/25
-[#24]: https://github.com/howeyc/fsnotify/issues/24
-[#21]: https://github.com/howeyc/fsnotify/issues/21
diff --git a/vendor/github.com/fsnotify/fsnotify/CONTRIBUTING.md b/vendor/github.com/fsnotify/fsnotify/CONTRIBUTING.md
deleted file mode 100644
index ea379759..00000000
--- a/vendor/github.com/fsnotify/fsnotify/CONTRIBUTING.md
+++ /dev/null
@@ -1,26 +0,0 @@
-Thank you for your interest in contributing to fsnotify! We try to review and
-merge PRs in a reasonable timeframe, but please be aware that:
-
-- To avoid "wasted" work, please discus changes on the issue tracker first. You
- can just send PRs, but they may end up being rejected for one reason or the
- other.
-
-- fsnotify is a cross-platform library, and changes must work reasonably well on
- all supported platforms.
-
-- Changes will need to be compatible; old code should still compile, and the
- runtime behaviour can't change in ways that are likely to lead to problems for
- users.
-
-Testing
--------
-Just `go test ./...` runs all the tests; the CI runs this on all supported
-platforms. Testing different platforms locally can be done with something like
-[goon] or [Vagrant], but this isn't super-easy to set up at the moment.
-
-Use the `-short` flag to make the "stress test" run faster.
-
-
-[goon]: https://github.com/arp242/goon
-[Vagrant]: https://www.vagrantup.com/
-[integration_test.go]: /integration_test.go
diff --git a/vendor/github.com/fsnotify/fsnotify/LICENSE b/vendor/github.com/fsnotify/fsnotify/LICENSE
deleted file mode 100644
index fb03ade7..00000000
--- a/vendor/github.com/fsnotify/fsnotify/LICENSE
+++ /dev/null
@@ -1,25 +0,0 @@
-Copyright © 2012 The Go Authors. All rights reserved.
-Copyright © fsnotify Authors. All rights reserved.
-
-Redistribution and use in source and binary forms, with or without modification,
-are permitted provided that the following conditions are met:
-
-* Redistributions of source code must retain the above copyright notice, this
- list of conditions and the following disclaimer.
-* Redistributions in binary form must reproduce the above copyright notice, this
- list of conditions and the following disclaimer in the documentation and/or
- other materials provided with the distribution.
-* Neither the name of Google Inc. nor the names of its contributors may be used
- to endorse or promote products derived from this software without specific
- prior written permission.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
-ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
-WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
-ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
-(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
-LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON
-ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
-SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/fsnotify/fsnotify/README.md b/vendor/github.com/fsnotify/fsnotify/README.md
deleted file mode 100644
index d4e6080f..00000000
--- a/vendor/github.com/fsnotify/fsnotify/README.md
+++ /dev/null
@@ -1,161 +0,0 @@
-fsnotify is a Go library to provide cross-platform filesystem notifications on
-Windows, Linux, macOS, and BSD systems.
-
-Go 1.16 or newer is required; the full documentation is at
-https://pkg.go.dev/github.com/fsnotify/fsnotify
-
-**It's best to read the documentation at pkg.go.dev, as it's pinned to the last
-released version, whereas this README is for the last development version which
-may include additions/changes.**
-
----
-
-Platform support:
-
-| Adapter | OS | Status |
-| --------------------- | ---------------| -------------------------------------------------------------|
-| inotify | Linux 2.6.32+ | Supported |
-| kqueue | BSD, macOS | Supported |
-| ReadDirectoryChangesW | Windows | Supported |
-| FSEvents | macOS | [Planned](https://github.com/fsnotify/fsnotify/issues/11) |
-| FEN | Solaris 11 | [In Progress](https://github.com/fsnotify/fsnotify/pull/371) |
-| fanotify | Linux 5.9+ | [Maybe](https://github.com/fsnotify/fsnotify/issues/114) |
-| USN Journals | Windows | [Maybe](https://github.com/fsnotify/fsnotify/issues/53) |
-| Polling | *All* | [Maybe](https://github.com/fsnotify/fsnotify/issues/9) |
-
-Linux and macOS should include Android and iOS, but these are currently untested.
-
-Usage
------
-A basic example:
-
-```go
-package main
-
-import (
- "log"
-
- "github.com/fsnotify/fsnotify"
-)
-
-func main() {
- // Create new watcher.
- watcher, err := fsnotify.NewWatcher()
- if err != nil {
- log.Fatal(err)
- }
- defer watcher.Close()
-
- // Start listening for events.
- go func() {
- for {
- select {
- case event, ok := <-watcher.Events:
- if !ok {
- return
- }
- log.Println("event:", event)
- if event.Has(fsnotify.Write) {
- log.Println("modified file:", event.Name)
- }
- case err, ok := <-watcher.Errors:
- if !ok {
- return
- }
- log.Println("error:", err)
- }
- }
- }()
-
- // Add a path.
- err = watcher.Add("/tmp")
- if err != nil {
- log.Fatal(err)
- }
-
- // Block main goroutine forever.
- <-make(chan struct{})
-}
-```
-
-Some more examples can be found in [cmd/fsnotify](cmd/fsnotify), which can be
-run with:
-
- % go run ./cmd/fsnotify
-
-FAQ
----
-### Will a file still be watched when it's moved to another directory?
-No, not unless you are watching the location it was moved to.
-
-### Are subdirectories watched too?
-No, you must add watches for any directory you want to watch (a recursive
-watcher is on the roadmap: [#18]).
-
-[#18]: https://github.com/fsnotify/fsnotify/issues/18
-
-### Do I have to watch the Error and Event channels in a goroutine?
-As of now, yes (you can read both channels in the same goroutine using `select`,
-you don't need a separate goroutine for both channels; see the example).
-
-### Why don't notifications work with NFS, SMB, FUSE, /proc, or /sys?
-fsnotify requires support from underlying OS to work. The current NFS and SMB
-protocols does not provide network level support for file notifications, and
-neither do the /proc and /sys virtual filesystems.
-
-This could be fixed with a polling watcher ([#9]), but it's not yet implemented.
-
-[#9]: https://github.com/fsnotify/fsnotify/issues/9
-
-Platform-specific notes
------------------------
-### Linux
-When a file is removed a REMOVE event won't be emitted until all file
-descriptors are closed; it will emit a CHMOD instead:
-
- fp := os.Open("file")
- os.Remove("file") // CHMOD
- fp.Close() // REMOVE
-
-This is the event that inotify sends, so not much can be changed about this.
-
-The `fs.inotify.max_user_watches` sysctl variable specifies the upper limit for
-the number of watches per user, and `fs.inotify.max_user_instances` specifies
-the maximum number of inotify instances per user. Every Watcher you create is an
-"instance", and every path you add is a "watch".
-
-These are also exposed in `/proc` as `/proc/sys/fs/inotify/max_user_watches` and
-`/proc/sys/fs/inotify/max_user_instances`
-
-To increase them you can use `sysctl` or write the value to proc file:
-
- # The default values on Linux 5.18
- sysctl fs.inotify.max_user_watches=124983
- sysctl fs.inotify.max_user_instances=128
-
-To make the changes persist on reboot edit `/etc/sysctl.conf` or
-`/usr/lib/sysctl.d/50-default.conf` (details differ per Linux distro; check your
-distro's documentation):
-
- fs.inotify.max_user_watches=124983
- fs.inotify.max_user_instances=128
-
-Reaching the limit will result in a "no space left on device" or "too many open
-files" error.
-
-### kqueue (macOS, all BSD systems)
-kqueue requires opening a file descriptor for every file that's being watched;
-so if you're watching a directory with five files then that's six file
-descriptors. You will run in to your system's "max open files" limit faster on
-these platforms.
-
-The sysctl variables `kern.maxfiles` and `kern.maxfilesperproc` can be used to
-control the maximum number of open files.
-
-### macOS
-Spotlight indexing on macOS can result in multiple events (see [#15]). A temporary
-workaround is to add your folder(s) to the *Spotlight Privacy settings* until we
-have a native FSEvents implementation (see [#11]).
-
-[#11]: https://github.com/fsnotify/fsnotify/issues/11
-[#15]: https://github.com/fsnotify/fsnotify/issues/15
diff --git a/vendor/github.com/fsnotify/fsnotify/backend_fen.go b/vendor/github.com/fsnotify/fsnotify/backend_fen.go
deleted file mode 100644
index 1a95ad8e..00000000
--- a/vendor/github.com/fsnotify/fsnotify/backend_fen.go
+++ /dev/null
@@ -1,162 +0,0 @@
-//go:build solaris
-// +build solaris
-
-package fsnotify
-
-import (
- "errors"
-)
-
-// Watcher watches a set of paths, delivering events on a channel.
-//
-// A watcher should not be copied (e.g. pass it by pointer, rather than by
-// value).
-//
-// # Linux notes
-//
-// When a file is removed a Remove event won't be emitted until all file
-// descriptors are closed, and deletes will always emit a Chmod. For example:
-//
-// fp := os.Open("file")
-// os.Remove("file") // Triggers Chmod
-// fp.Close() // Triggers Remove
-//
-// This is the event that inotify sends, so not much can be changed about this.
-//
-// The fs.inotify.max_user_watches sysctl variable specifies the upper limit
-// for the number of watches per user, and fs.inotify.max_user_instances
-// specifies the maximum number of inotify instances per user. Every Watcher you
-// create is an "instance", and every path you add is a "watch".
-//
-// These are also exposed in /proc as /proc/sys/fs/inotify/max_user_watches and
-// /proc/sys/fs/inotify/max_user_instances
-//
-// To increase them you can use sysctl or write the value to the /proc file:
-//
-// # Default values on Linux 5.18
-// sysctl fs.inotify.max_user_watches=124983
-// sysctl fs.inotify.max_user_instances=128
-//
-// To make the changes persist on reboot edit /etc/sysctl.conf or
-// /usr/lib/sysctl.d/50-default.conf (details differ per Linux distro; check
-// your distro's documentation):
-//
-// fs.inotify.max_user_watches=124983
-// fs.inotify.max_user_instances=128
-//
-// Reaching the limit will result in a "no space left on device" or "too many open
-// files" error.
-//
-// # kqueue notes (macOS, BSD)
-//
-// kqueue requires opening a file descriptor for every file that's being watched;
-// so if you're watching a directory with five files then that's six file
-// descriptors. You will run in to your system's "max open files" limit faster on
-// these platforms.
-//
-// The sysctl variables kern.maxfiles and kern.maxfilesperproc can be used to
-// control the maximum number of open files, as well as /etc/login.conf on BSD
-// systems.
-//
-// # macOS notes
-//
-// Spotlight indexing on macOS can result in multiple events (see [#15]). A
-// temporary workaround is to add your folder(s) to the "Spotlight Privacy
-// Settings" until we have a native FSEvents implementation (see [#11]).
-//
-// [#11]: https://github.com/fsnotify/fsnotify/issues/11
-// [#15]: https://github.com/fsnotify/fsnotify/issues/15
-type Watcher struct {
- // Events sends the filesystem change events.
- //
- // fsnotify can send the following events; a "path" here can refer to a
- // file, directory, symbolic link, or special file like a FIFO.
- //
- // fsnotify.Create A new path was created; this may be followed by one
- // or more Write events if data also gets written to a
- // file.
- //
- // fsnotify.Remove A path was removed.
- //
- // fsnotify.Rename A path was renamed. A rename is always sent with the
- // old path as Event.Name, and a Create event will be
- // sent with the new name. Renames are only sent for
- // paths that are currently watched; e.g. moving an
- // unmonitored file into a monitored directory will
- // show up as just a Create. Similarly, renaming a file
- // to outside a monitored directory will show up as
- // only a Rename.
- //
- // fsnotify.Write A file or named pipe was written to. A Truncate will
- // also trigger a Write. A single "write action"
- // initiated by the user may show up as one or multiple
- // writes, depending on when the system syncs things to
- // disk. For example when compiling a large Go program
- // you may get hundreds of Write events, so you
- // probably want to wait until you've stopped receiving
- // them (see the dedup example in cmd/fsnotify).
- //
- // fsnotify.Chmod Attributes were changed. On Linux this is also sent
- // when a file is removed (or more accurately, when a
- // link to an inode is removed). On kqueue it's sent
- // and on kqueue when a file is truncated. On Windows
- // it's never sent.
- Events chan Event
-
- // Errors sends any errors.
- Errors chan error
-}
-
-// NewWatcher creates a new Watcher.
-func NewWatcher() (*Watcher, error) {
- return nil, errors.New("FEN based watcher not yet supported for fsnotify\n")
-}
-
-// Close removes all watches and closes the events channel.
-func (w *Watcher) Close() error {
- return nil
-}
-
-// Add starts monitoring the path for changes.
-//
-// A path can only be watched once; attempting to watch it more than once will
-// return an error. Paths that do not yet exist on the filesystem cannot be
-// added. A watch will be automatically removed if the path is deleted.
-//
-// A path will remain watched if it gets renamed to somewhere else on the same
-// filesystem, but the monitor will get removed if the path gets deleted and
-// re-created, or if it's moved to a different filesystem.
-//
-// Notifications on network filesystems (NFS, SMB, FUSE, etc.) or special
-// filesystems (/proc, /sys, etc.) generally don't work.
-//
-// # Watching directories
-//
-// All files in a directory are monitored, including new files that are created
-// after the watcher is started. Subdirectories are not watched (i.e. it's
-// non-recursive).
-//
-// # Watching files
-//
-// Watching individual files (rather than directories) is generally not
-// recommended as many tools update files atomically. Instead of "just" writing
-// to the file a temporary file will be written to first, and if successful the
-// temporary file is moved to to destination removing the original, or some
-// variant thereof. The watcher on the original file is now lost, as it no
-// longer exists.
-//
-// Instead, watch the parent directory and use Event.Name to filter out files
-// you're not interested in. There is an example of this in [cmd/fsnotify/file.go].
-func (w *Watcher) Add(name string) error {
- return nil
-}
-
-// Remove stops monitoring the path for changes.
-//
-// Directories are always removed non-recursively. For example, if you added
-// /tmp/dir and /tmp/dir/subdir then you will need to remove both.
-//
-// Removing a path that has not yet been added returns [ErrNonExistentWatch].
-func (w *Watcher) Remove(name string) error {
- return nil
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/backend_inotify.go b/vendor/github.com/fsnotify/fsnotify/backend_inotify.go
deleted file mode 100644
index 54c77fbb..00000000
--- a/vendor/github.com/fsnotify/fsnotify/backend_inotify.go
+++ /dev/null
@@ -1,459 +0,0 @@
-//go:build linux
-// +build linux
-
-package fsnotify
-
-import (
- "errors"
- "fmt"
- "io"
- "os"
- "path/filepath"
- "strings"
- "sync"
- "unsafe"
-
- "golang.org/x/sys/unix"
-)
-
-// Watcher watches a set of paths, delivering events on a channel.
-//
-// A watcher should not be copied (e.g. pass it by pointer, rather than by
-// value).
-//
-// # Linux notes
-//
-// When a file is removed a Remove event won't be emitted until all file
-// descriptors are closed, and deletes will always emit a Chmod. For example:
-//
-// fp := os.Open("file")
-// os.Remove("file") // Triggers Chmod
-// fp.Close() // Triggers Remove
-//
-// This is the event that inotify sends, so not much can be changed about this.
-//
-// The fs.inotify.max_user_watches sysctl variable specifies the upper limit
-// for the number of watches per user, and fs.inotify.max_user_instances
-// specifies the maximum number of inotify instances per user. Every Watcher you
-// create is an "instance", and every path you add is a "watch".
-//
-// These are also exposed in /proc as /proc/sys/fs/inotify/max_user_watches and
-// /proc/sys/fs/inotify/max_user_instances
-//
-// To increase them you can use sysctl or write the value to the /proc file:
-//
-// # Default values on Linux 5.18
-// sysctl fs.inotify.max_user_watches=124983
-// sysctl fs.inotify.max_user_instances=128
-//
-// To make the changes persist on reboot edit /etc/sysctl.conf or
-// /usr/lib/sysctl.d/50-default.conf (details differ per Linux distro; check
-// your distro's documentation):
-//
-// fs.inotify.max_user_watches=124983
-// fs.inotify.max_user_instances=128
-//
-// Reaching the limit will result in a "no space left on device" or "too many open
-// files" error.
-//
-// # kqueue notes (macOS, BSD)
-//
-// kqueue requires opening a file descriptor for every file that's being watched;
-// so if you're watching a directory with five files then that's six file
-// descriptors. You will run in to your system's "max open files" limit faster on
-// these platforms.
-//
-// The sysctl variables kern.maxfiles and kern.maxfilesperproc can be used to
-// control the maximum number of open files, as well as /etc/login.conf on BSD
-// systems.
-//
-// # macOS notes
-//
-// Spotlight indexing on macOS can result in multiple events (see [#15]). A
-// temporary workaround is to add your folder(s) to the "Spotlight Privacy
-// Settings" until we have a native FSEvents implementation (see [#11]).
-//
-// [#11]: https://github.com/fsnotify/fsnotify/issues/11
-// [#15]: https://github.com/fsnotify/fsnotify/issues/15
-type Watcher struct {
- // Events sends the filesystem change events.
- //
- // fsnotify can send the following events; a "path" here can refer to a
- // file, directory, symbolic link, or special file like a FIFO.
- //
- // fsnotify.Create A new path was created; this may be followed by one
- // or more Write events if data also gets written to a
- // file.
- //
- // fsnotify.Remove A path was removed.
- //
- // fsnotify.Rename A path was renamed. A rename is always sent with the
- // old path as Event.Name, and a Create event will be
- // sent with the new name. Renames are only sent for
- // paths that are currently watched; e.g. moving an
- // unmonitored file into a monitored directory will
- // show up as just a Create. Similarly, renaming a file
- // to outside a monitored directory will show up as
- // only a Rename.
- //
- // fsnotify.Write A file or named pipe was written to. A Truncate will
- // also trigger a Write. A single "write action"
- // initiated by the user may show up as one or multiple
- // writes, depending on when the system syncs things to
- // disk. For example when compiling a large Go program
- // you may get hundreds of Write events, so you
- // probably want to wait until you've stopped receiving
- // them (see the dedup example in cmd/fsnotify).
- //
- // fsnotify.Chmod Attributes were changed. On Linux this is also sent
- // when a file is removed (or more accurately, when a
- // link to an inode is removed). On kqueue it's sent
- // and on kqueue when a file is truncated. On Windows
- // it's never sent.
- Events chan Event
-
- // Errors sends any errors.
- Errors chan error
-
- // Store fd here as os.File.Read() will no longer return on close after
- // calling Fd(). See: https://github.com/golang/go/issues/26439
- fd int
- mu sync.Mutex // Map access
- inotifyFile *os.File
- watches map[string]*watch // Map of inotify watches (key: path)
- paths map[int]string // Map of watched paths (key: watch descriptor)
- done chan struct{} // Channel for sending a "quit message" to the reader goroutine
- doneResp chan struct{} // Channel to respond to Close
-}
-
-// NewWatcher creates a new Watcher.
-func NewWatcher() (*Watcher, error) {
- // Create inotify fd
- // Need to set the FD to nonblocking mode in order for SetDeadline methods to work
- // Otherwise, blocking i/o operations won't terminate on close
- fd, errno := unix.InotifyInit1(unix.IN_CLOEXEC | unix.IN_NONBLOCK)
- if fd == -1 {
- return nil, errno
- }
-
- w := &Watcher{
- fd: fd,
- inotifyFile: os.NewFile(uintptr(fd), ""),
- watches: make(map[string]*watch),
- paths: make(map[int]string),
- Events: make(chan Event),
- Errors: make(chan error),
- done: make(chan struct{}),
- doneResp: make(chan struct{}),
- }
-
- go w.readEvents()
- return w, nil
-}
-
-// Returns true if the event was sent, or false if watcher is closed.
-func (w *Watcher) sendEvent(e Event) bool {
- select {
- case w.Events <- e:
- return true
- case <-w.done:
- }
- return false
-}
-
-// Returns true if the error was sent, or false if watcher is closed.
-func (w *Watcher) sendError(err error) bool {
- select {
- case w.Errors <- err:
- return true
- case <-w.done:
- return false
- }
-}
-
-func (w *Watcher) isClosed() bool {
- select {
- case <-w.done:
- return true
- default:
- return false
- }
-}
-
-// Close removes all watches and closes the events channel.
-func (w *Watcher) Close() error {
- w.mu.Lock()
- if w.isClosed() {
- w.mu.Unlock()
- return nil
- }
-
- // Send 'close' signal to goroutine, and set the Watcher to closed.
- close(w.done)
- w.mu.Unlock()
-
- // Causes any blocking reads to return with an error, provided the file
- // still supports deadline operations.
- err := w.inotifyFile.Close()
- if err != nil {
- return err
- }
-
- // Wait for goroutine to close
- <-w.doneResp
-
- return nil
-}
-
-// Add starts monitoring the path for changes.
-//
-// A path can only be watched once; attempting to watch it more than once will
-// return an error. Paths that do not yet exist on the filesystem cannot be
-// added. A watch will be automatically removed if the path is deleted.
-//
-// A path will remain watched if it gets renamed to somewhere else on the same
-// filesystem, but the monitor will get removed if the path gets deleted and
-// re-created, or if it's moved to a different filesystem.
-//
-// Notifications on network filesystems (NFS, SMB, FUSE, etc.) or special
-// filesystems (/proc, /sys, etc.) generally don't work.
-//
-// # Watching directories
-//
-// All files in a directory are monitored, including new files that are created
-// after the watcher is started. Subdirectories are not watched (i.e. it's
-// non-recursive).
-//
-// # Watching files
-//
-// Watching individual files (rather than directories) is generally not
-// recommended as many tools update files atomically. Instead of "just" writing
-// to the file a temporary file will be written to first, and if successful the
-// temporary file is moved to to destination removing the original, or some
-// variant thereof. The watcher on the original file is now lost, as it no
-// longer exists.
-//
-// Instead, watch the parent directory and use Event.Name to filter out files
-// you're not interested in. There is an example of this in [cmd/fsnotify/file.go].
-func (w *Watcher) Add(name string) error {
- name = filepath.Clean(name)
- if w.isClosed() {
- return errors.New("inotify instance already closed")
- }
-
- var flags uint32 = unix.IN_MOVED_TO | unix.IN_MOVED_FROM |
- unix.IN_CREATE | unix.IN_ATTRIB | unix.IN_MODIFY |
- unix.IN_MOVE_SELF | unix.IN_DELETE | unix.IN_DELETE_SELF
-
- w.mu.Lock()
- defer w.mu.Unlock()
- watchEntry := w.watches[name]
- if watchEntry != nil {
- flags |= watchEntry.flags | unix.IN_MASK_ADD
- }
- wd, errno := unix.InotifyAddWatch(w.fd, name, flags)
- if wd == -1 {
- return errno
- }
-
- if watchEntry == nil {
- w.watches[name] = &watch{wd: uint32(wd), flags: flags}
- w.paths[wd] = name
- } else {
- watchEntry.wd = uint32(wd)
- watchEntry.flags = flags
- }
-
- return nil
-}
-
-// Remove stops monitoring the path for changes.
-//
-// Directories are always removed non-recursively. For example, if you added
-// /tmp/dir and /tmp/dir/subdir then you will need to remove both.
-//
-// Removing a path that has not yet been added returns [ErrNonExistentWatch].
-func (w *Watcher) Remove(name string) error {
- name = filepath.Clean(name)
-
- // Fetch the watch.
- w.mu.Lock()
- defer w.mu.Unlock()
- watch, ok := w.watches[name]
-
- // Remove it from inotify.
- if !ok {
- return fmt.Errorf("%w: %s", ErrNonExistentWatch, name)
- }
-
- // We successfully removed the watch if InotifyRmWatch doesn't return an
- // error, we need to clean up our internal state to ensure it matches
- // inotify's kernel state.
- delete(w.paths, int(watch.wd))
- delete(w.watches, name)
-
- // inotify_rm_watch will return EINVAL if the file has been deleted;
- // the inotify will already have been removed.
- // watches and pathes are deleted in ignoreLinux() implicitly and asynchronously
- // by calling inotify_rm_watch() below. e.g. readEvents() goroutine receives IN_IGNORE
- // so that EINVAL means that the wd is being rm_watch()ed or its file removed
- // by another thread and we have not received IN_IGNORE event.
- success, errno := unix.InotifyRmWatch(w.fd, watch.wd)
- if success == -1 {
- // TODO: Perhaps it's not helpful to return an error here in every case;
- // The only two possible errors are:
- //
- // - EBADF, which happens when w.fd is not a valid file descriptor
- // of any kind.
- // - EINVAL, which is when fd is not an inotify descriptor or wd
- // is not a valid watch descriptor. Watch descriptors are
- // invalidated when they are removed explicitly or implicitly;
- // explicitly by inotify_rm_watch, implicitly when the file they
- // are watching is deleted.
- return errno
- }
-
- return nil
-}
-
-// WatchList returns all paths added with [Add] (and are not yet removed).
-func (w *Watcher) WatchList() []string {
- w.mu.Lock()
- defer w.mu.Unlock()
-
- entries := make([]string, 0, len(w.watches))
- for pathname := range w.watches {
- entries = append(entries, pathname)
- }
-
- return entries
-}
-
-type watch struct {
- wd uint32 // Watch descriptor (as returned by the inotify_add_watch() syscall)
- flags uint32 // inotify flags of this watch (see inotify(7) for the list of valid flags)
-}
-
-// readEvents reads from the inotify file descriptor, converts the
-// received events into Event objects and sends them via the Events channel
-func (w *Watcher) readEvents() {
- defer func() {
- close(w.doneResp)
- close(w.Errors)
- close(w.Events)
- }()
-
- var (
- buf [unix.SizeofInotifyEvent * 4096]byte // Buffer for a maximum of 4096 raw events
- errno error // Syscall errno
- )
- for {
- // See if we have been closed.
- if w.isClosed() {
- return
- }
-
- n, err := w.inotifyFile.Read(buf[:])
- switch {
- case errors.Unwrap(err) == os.ErrClosed:
- return
- case err != nil:
- if !w.sendError(err) {
- return
- }
- continue
- }
-
- if n < unix.SizeofInotifyEvent {
- var err error
- if n == 0 {
- // If EOF is received. This should really never happen.
- err = io.EOF
- } else if n < 0 {
- // If an error occurred while reading.
- err = errno
- } else {
- // Read was too short.
- err = errors.New("notify: short read in readEvents()")
- }
- if !w.sendError(err) {
- return
- }
- continue
- }
-
- var offset uint32
- // We don't know how many events we just read into the buffer
- // While the offset points to at least one whole event...
- for offset <= uint32(n-unix.SizeofInotifyEvent) {
- var (
- // Point "raw" to the event in the buffer
- raw = (*unix.InotifyEvent)(unsafe.Pointer(&buf[offset]))
- mask = uint32(raw.Mask)
- nameLen = uint32(raw.Len)
- )
-
- if mask&unix.IN_Q_OVERFLOW != 0 {
- if !w.sendError(ErrEventOverflow) {
- return
- }
- }
-
- // If the event happened to the watched directory or the watched file, the kernel
- // doesn't append the filename to the event, but we would like to always fill the
- // the "Name" field with a valid filename. We retrieve the path of the watch from
- // the "paths" map.
- w.mu.Lock()
- name, ok := w.paths[int(raw.Wd)]
- // IN_DELETE_SELF occurs when the file/directory being watched is removed.
- // This is a sign to clean up the maps, otherwise we are no longer in sync
- // with the inotify kernel state which has already deleted the watch
- // automatically.
- if ok && mask&unix.IN_DELETE_SELF == unix.IN_DELETE_SELF {
- delete(w.paths, int(raw.Wd))
- delete(w.watches, name)
- }
- w.mu.Unlock()
-
- if nameLen > 0 {
- // Point "bytes" at the first byte of the filename
- bytes := (*[unix.PathMax]byte)(unsafe.Pointer(&buf[offset+unix.SizeofInotifyEvent]))[:nameLen:nameLen]
- // The filename is padded with NULL bytes. TrimRight() gets rid of those.
- name += "/" + strings.TrimRight(string(bytes[0:nameLen]), "\000")
- }
-
- event := w.newEvent(name, mask)
-
- // Send the events that are not ignored on the events channel
- if mask&unix.IN_IGNORED == 0 {
- if !w.sendEvent(event) {
- return
- }
- }
-
- // Move to the next event in the buffer
- offset += unix.SizeofInotifyEvent + nameLen
- }
- }
-}
-
-// newEvent returns an platform-independent Event based on an inotify mask.
-func (w *Watcher) newEvent(name string, mask uint32) Event {
- e := Event{Name: name}
- if mask&unix.IN_CREATE == unix.IN_CREATE || mask&unix.IN_MOVED_TO == unix.IN_MOVED_TO {
- e.Op |= Create
- }
- if mask&unix.IN_DELETE_SELF == unix.IN_DELETE_SELF || mask&unix.IN_DELETE == unix.IN_DELETE {
- e.Op |= Remove
- }
- if mask&unix.IN_MODIFY == unix.IN_MODIFY {
- e.Op |= Write
- }
- if mask&unix.IN_MOVE_SELF == unix.IN_MOVE_SELF || mask&unix.IN_MOVED_FROM == unix.IN_MOVED_FROM {
- e.Op |= Rename
- }
- if mask&unix.IN_ATTRIB == unix.IN_ATTRIB {
- e.Op |= Chmod
- }
- return e
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/backend_kqueue.go b/vendor/github.com/fsnotify/fsnotify/backend_kqueue.go
deleted file mode 100644
index 29087469..00000000
--- a/vendor/github.com/fsnotify/fsnotify/backend_kqueue.go
+++ /dev/null
@@ -1,707 +0,0 @@
-//go:build freebsd || openbsd || netbsd || dragonfly || darwin
-// +build freebsd openbsd netbsd dragonfly darwin
-
-package fsnotify
-
-import (
- "errors"
- "fmt"
- "io/ioutil"
- "os"
- "path/filepath"
- "sync"
-
- "golang.org/x/sys/unix"
-)
-
-// Watcher watches a set of paths, delivering events on a channel.
-//
-// A watcher should not be copied (e.g. pass it by pointer, rather than by
-// value).
-//
-// # Linux notes
-//
-// When a file is removed a Remove event won't be emitted until all file
-// descriptors are closed, and deletes will always emit a Chmod. For example:
-//
-// fp := os.Open("file")
-// os.Remove("file") // Triggers Chmod
-// fp.Close() // Triggers Remove
-//
-// This is the event that inotify sends, so not much can be changed about this.
-//
-// The fs.inotify.max_user_watches sysctl variable specifies the upper limit
-// for the number of watches per user, and fs.inotify.max_user_instances
-// specifies the maximum number of inotify instances per user. Every Watcher you
-// create is an "instance", and every path you add is a "watch".
-//
-// These are also exposed in /proc as /proc/sys/fs/inotify/max_user_watches and
-// /proc/sys/fs/inotify/max_user_instances
-//
-// To increase them you can use sysctl or write the value to the /proc file:
-//
-// # Default values on Linux 5.18
-// sysctl fs.inotify.max_user_watches=124983
-// sysctl fs.inotify.max_user_instances=128
-//
-// To make the changes persist on reboot edit /etc/sysctl.conf or
-// /usr/lib/sysctl.d/50-default.conf (details differ per Linux distro; check
-// your distro's documentation):
-//
-// fs.inotify.max_user_watches=124983
-// fs.inotify.max_user_instances=128
-//
-// Reaching the limit will result in a "no space left on device" or "too many open
-// files" error.
-//
-// # kqueue notes (macOS, BSD)
-//
-// kqueue requires opening a file descriptor for every file that's being watched;
-// so if you're watching a directory with five files then that's six file
-// descriptors. You will run in to your system's "max open files" limit faster on
-// these platforms.
-//
-// The sysctl variables kern.maxfiles and kern.maxfilesperproc can be used to
-// control the maximum number of open files, as well as /etc/login.conf on BSD
-// systems.
-//
-// # macOS notes
-//
-// Spotlight indexing on macOS can result in multiple events (see [#15]). A
-// temporary workaround is to add your folder(s) to the "Spotlight Privacy
-// Settings" until we have a native FSEvents implementation (see [#11]).
-//
-// [#11]: https://github.com/fsnotify/fsnotify/issues/11
-// [#15]: https://github.com/fsnotify/fsnotify/issues/15
-type Watcher struct {
- // Events sends the filesystem change events.
- //
- // fsnotify can send the following events; a "path" here can refer to a
- // file, directory, symbolic link, or special file like a FIFO.
- //
- // fsnotify.Create A new path was created; this may be followed by one
- // or more Write events if data also gets written to a
- // file.
- //
- // fsnotify.Remove A path was removed.
- //
- // fsnotify.Rename A path was renamed. A rename is always sent with the
- // old path as Event.Name, and a Create event will be
- // sent with the new name. Renames are only sent for
- // paths that are currently watched; e.g. moving an
- // unmonitored file into a monitored directory will
- // show up as just a Create. Similarly, renaming a file
- // to outside a monitored directory will show up as
- // only a Rename.
- //
- // fsnotify.Write A file or named pipe was written to. A Truncate will
- // also trigger a Write. A single "write action"
- // initiated by the user may show up as one or multiple
- // writes, depending on when the system syncs things to
- // disk. For example when compiling a large Go program
- // you may get hundreds of Write events, so you
- // probably want to wait until you've stopped receiving
- // them (see the dedup example in cmd/fsnotify).
- //
- // fsnotify.Chmod Attributes were changed. On Linux this is also sent
- // when a file is removed (or more accurately, when a
- // link to an inode is removed). On kqueue it's sent
- // and on kqueue when a file is truncated. On Windows
- // it's never sent.
- Events chan Event
-
- // Errors sends any errors.
- Errors chan error
-
- done chan struct{}
- kq int // File descriptor (as returned by the kqueue() syscall).
- closepipe [2]int // Pipe used for closing.
- mu sync.Mutex // Protects access to watcher data
- watches map[string]int // Watched file descriptors (key: path).
- watchesByDir map[string]map[int]struct{} // Watched file descriptors indexed by the parent directory (key: dirname(path)).
- userWatches map[string]struct{} // Watches added with Watcher.Add()
- dirFlags map[string]uint32 // Watched directories to fflags used in kqueue.
- paths map[int]pathInfo // File descriptors to path names for processing kqueue events.
- fileExists map[string]struct{} // Keep track of if we know this file exists (to stop duplicate create events).
- isClosed bool // Set to true when Close() is first called
-}
-
-type pathInfo struct {
- name string
- isDir bool
-}
-
-// NewWatcher creates a new Watcher.
-func NewWatcher() (*Watcher, error) {
- kq, closepipe, err := newKqueue()
- if err != nil {
- return nil, err
- }
-
- w := &Watcher{
- kq: kq,
- closepipe: closepipe,
- watches: make(map[string]int),
- watchesByDir: make(map[string]map[int]struct{}),
- dirFlags: make(map[string]uint32),
- paths: make(map[int]pathInfo),
- fileExists: make(map[string]struct{}),
- userWatches: make(map[string]struct{}),
- Events: make(chan Event),
- Errors: make(chan error),
- done: make(chan struct{}),
- }
-
- go w.readEvents()
- return w, nil
-}
-
-// newKqueue creates a new kernel event queue and returns a descriptor.
-//
-// This registers a new event on closepipe, which will trigger an event when
-// it's closed. This way we can use kevent() without timeout/polling; without
-// the closepipe, it would block forever and we wouldn't be able to stop it at
-// all.
-func newKqueue() (kq int, closepipe [2]int, err error) {
- kq, err = unix.Kqueue()
- if kq == -1 {
- return kq, closepipe, err
- }
-
- // Register the close pipe.
- err = unix.Pipe(closepipe[:])
- if err != nil {
- unix.Close(kq)
- return kq, closepipe, err
- }
-
- // Register changes to listen on the closepipe.
- changes := make([]unix.Kevent_t, 1)
- // SetKevent converts int to the platform-specific types.
- unix.SetKevent(&changes[0], closepipe[0], unix.EVFILT_READ,
- unix.EV_ADD|unix.EV_ENABLE|unix.EV_ONESHOT)
-
- ok, err := unix.Kevent(kq, changes, nil, nil)
- if ok == -1 {
- unix.Close(kq)
- unix.Close(closepipe[0])
- unix.Close(closepipe[1])
- return kq, closepipe, err
- }
- return kq, closepipe, nil
-}
-
-// Returns true if the event was sent, or false if watcher is closed.
-func (w *Watcher) sendEvent(e Event) bool {
- select {
- case w.Events <- e:
- return true
- case <-w.done:
- }
- return false
-}
-
-// Returns true if the error was sent, or false if watcher is closed.
-func (w *Watcher) sendError(err error) bool {
- select {
- case w.Errors <- err:
- return true
- case <-w.done:
- }
- return false
-}
-
-// Close removes all watches and closes the events channel.
-func (w *Watcher) Close() error {
- w.mu.Lock()
- if w.isClosed {
- w.mu.Unlock()
- return nil
- }
- w.isClosed = true
-
- // copy paths to remove while locked
- pathsToRemove := make([]string, 0, len(w.watches))
- for name := range w.watches {
- pathsToRemove = append(pathsToRemove, name)
- }
- w.mu.Unlock() // Unlock before calling Remove, which also locks
- for _, name := range pathsToRemove {
- w.Remove(name)
- }
-
- // Send "quit" message to the reader goroutine.
- unix.Close(w.closepipe[1])
- close(w.done)
-
- return nil
-}
-
-// Add starts monitoring the path for changes.
-//
-// A path can only be watched once; attempting to watch it more than once will
-// return an error. Paths that do not yet exist on the filesystem cannot be
-// added. A watch will be automatically removed if the path is deleted.
-//
-// A path will remain watched if it gets renamed to somewhere else on the same
-// filesystem, but the monitor will get removed if the path gets deleted and
-// re-created, or if it's moved to a different filesystem.
-//
-// Notifications on network filesystems (NFS, SMB, FUSE, etc.) or special
-// filesystems (/proc, /sys, etc.) generally don't work.
-//
-// # Watching directories
-//
-// All files in a directory are monitored, including new files that are created
-// after the watcher is started. Subdirectories are not watched (i.e. it's
-// non-recursive).
-//
-// # Watching files
-//
-// Watching individual files (rather than directories) is generally not
-// recommended as many tools update files atomically. Instead of "just" writing
-// to the file a temporary file will be written to first, and if successful the
-// temporary file is moved to to destination removing the original, or some
-// variant thereof. The watcher on the original file is now lost, as it no
-// longer exists.
-//
-// Instead, watch the parent directory and use Event.Name to filter out files
-// you're not interested in. There is an example of this in [cmd/fsnotify/file.go].
-func (w *Watcher) Add(name string) error {
- w.mu.Lock()
- w.userWatches[name] = struct{}{}
- w.mu.Unlock()
- _, err := w.addWatch(name, noteAllEvents)
- return err
-}
-
-// Remove stops monitoring the path for changes.
-//
-// Directories are always removed non-recursively. For example, if you added
-// /tmp/dir and /tmp/dir/subdir then you will need to remove both.
-//
-// Removing a path that has not yet been added returns [ErrNonExistentWatch].
-func (w *Watcher) Remove(name string) error {
- name = filepath.Clean(name)
- w.mu.Lock()
- watchfd, ok := w.watches[name]
- w.mu.Unlock()
- if !ok {
- return fmt.Errorf("%w: %s", ErrNonExistentWatch, name)
- }
-
- err := w.register([]int{watchfd}, unix.EV_DELETE, 0)
- if err != nil {
- return err
- }
-
- unix.Close(watchfd)
-
- w.mu.Lock()
- isDir := w.paths[watchfd].isDir
- delete(w.watches, name)
- delete(w.userWatches, name)
-
- parentName := filepath.Dir(name)
- delete(w.watchesByDir[parentName], watchfd)
-
- if len(w.watchesByDir[parentName]) == 0 {
- delete(w.watchesByDir, parentName)
- }
-
- delete(w.paths, watchfd)
- delete(w.dirFlags, name)
- delete(w.fileExists, name)
- w.mu.Unlock()
-
- // Find all watched paths that are in this directory that are not external.
- if isDir {
- var pathsToRemove []string
- w.mu.Lock()
- for fd := range w.watchesByDir[name] {
- path := w.paths[fd]
- if _, ok := w.userWatches[path.name]; !ok {
- pathsToRemove = append(pathsToRemove, path.name)
- }
- }
- w.mu.Unlock()
- for _, name := range pathsToRemove {
- // Since these are internal, not much sense in propagating error
- // to the user, as that will just confuse them with an error about
- // a path they did not explicitly watch themselves.
- w.Remove(name)
- }
- }
-
- return nil
-}
-
-// WatchList returns all paths added with [Add] (and are not yet removed).
-func (w *Watcher) WatchList() []string {
- w.mu.Lock()
- defer w.mu.Unlock()
-
- entries := make([]string, 0, len(w.userWatches))
- for pathname := range w.userWatches {
- entries = append(entries, pathname)
- }
-
- return entries
-}
-
-// Watch all events (except NOTE_EXTEND, NOTE_LINK, NOTE_REVOKE)
-const noteAllEvents = unix.NOTE_DELETE | unix.NOTE_WRITE | unix.NOTE_ATTRIB | unix.NOTE_RENAME
-
-// addWatch adds name to the watched file set.
-// The flags are interpreted as described in kevent(2).
-// Returns the real path to the file which was added, if any, which may be different from the one passed in the case of symlinks.
-func (w *Watcher) addWatch(name string, flags uint32) (string, error) {
- var isDir bool
- // Make ./name and name equivalent
- name = filepath.Clean(name)
-
- w.mu.Lock()
- if w.isClosed {
- w.mu.Unlock()
- return "", errors.New("kevent instance already closed")
- }
- watchfd, alreadyWatching := w.watches[name]
- // We already have a watch, but we can still override flags.
- if alreadyWatching {
- isDir = w.paths[watchfd].isDir
- }
- w.mu.Unlock()
-
- if !alreadyWatching {
- fi, err := os.Lstat(name)
- if err != nil {
- return "", err
- }
-
- // Don't watch sockets or named pipes
- if (fi.Mode()&os.ModeSocket == os.ModeSocket) || (fi.Mode()&os.ModeNamedPipe == os.ModeNamedPipe) {
- return "", nil
- }
-
- // Follow Symlinks
- //
- // Linux can add unresolvable symlinks to the watch list without issue,
- // and Windows can't do symlinks period. To maintain consistency, we
- // will act like everything is fine if the link can't be resolved.
- // There will simply be no file events for broken symlinks. Hence the
- // returns of nil on errors.
- if fi.Mode()&os.ModeSymlink == os.ModeSymlink {
- name, err = filepath.EvalSymlinks(name)
- if err != nil {
- return "", nil
- }
-
- w.mu.Lock()
- _, alreadyWatching = w.watches[name]
- w.mu.Unlock()
-
- if alreadyWatching {
- return name, nil
- }
-
- fi, err = os.Lstat(name)
- if err != nil {
- return "", nil
- }
- }
-
- // Retry on EINTR; open() can return EINTR in practice on macOS.
- // See #354, and go issues 11180 and 39237.
- for {
- watchfd, err = unix.Open(name, openMode, 0)
- if err == nil {
- break
- }
- if errors.Is(err, unix.EINTR) {
- continue
- }
-
- return "", err
- }
-
- isDir = fi.IsDir()
- }
-
- err := w.register([]int{watchfd}, unix.EV_ADD|unix.EV_CLEAR|unix.EV_ENABLE, flags)
- if err != nil {
- unix.Close(watchfd)
- return "", err
- }
-
- if !alreadyWatching {
- w.mu.Lock()
- parentName := filepath.Dir(name)
- w.watches[name] = watchfd
-
- watchesByDir, ok := w.watchesByDir[parentName]
- if !ok {
- watchesByDir = make(map[int]struct{}, 1)
- w.watchesByDir[parentName] = watchesByDir
- }
- watchesByDir[watchfd] = struct{}{}
-
- w.paths[watchfd] = pathInfo{name: name, isDir: isDir}
- w.mu.Unlock()
- }
-
- if isDir {
- // Watch the directory if it has not been watched before,
- // or if it was watched before, but perhaps only a NOTE_DELETE (watchDirectoryFiles)
- w.mu.Lock()
-
- watchDir := (flags&unix.NOTE_WRITE) == unix.NOTE_WRITE &&
- (!alreadyWatching || (w.dirFlags[name]&unix.NOTE_WRITE) != unix.NOTE_WRITE)
- // Store flags so this watch can be updated later
- w.dirFlags[name] = flags
- w.mu.Unlock()
-
- if watchDir {
- if err := w.watchDirectoryFiles(name); err != nil {
- return "", err
- }
- }
- }
- return name, nil
-}
-
-// readEvents reads from kqueue and converts the received kevents into
-// Event values that it sends down the Events channel.
-func (w *Watcher) readEvents() {
- defer func() {
- err := unix.Close(w.kq)
- if err != nil {
- w.Errors <- err
- }
- unix.Close(w.closepipe[0])
- close(w.Events)
- close(w.Errors)
- }()
-
- eventBuffer := make([]unix.Kevent_t, 10)
- for closed := false; !closed; {
- kevents, err := w.read(eventBuffer)
- // EINTR is okay, the syscall was interrupted before timeout expired.
- if err != nil && err != unix.EINTR {
- if !w.sendError(fmt.Errorf("fsnotify.readEvents: %w", err)) {
- closed = true
- }
- continue
- }
-
- // Flush the events we received to the Events channel
- for _, kevent := range kevents {
- var (
- watchfd = int(kevent.Ident)
- mask = uint32(kevent.Fflags)
- )
-
- // Shut down the loop when the pipe is closed, but only after all
- // other events have been processed.
- if watchfd == w.closepipe[0] {
- closed = true
- continue
- }
-
- w.mu.Lock()
- path := w.paths[watchfd]
- w.mu.Unlock()
-
- event := w.newEvent(path.name, mask)
-
- if path.isDir && !event.Has(Remove) {
- // Double check to make sure the directory exists. This can
- // happen when we do a rm -fr on a recursively watched folders
- // and we receive a modification event first but the folder has
- // been deleted and later receive the delete event.
- if _, err := os.Lstat(event.Name); os.IsNotExist(err) {
- event.Op |= Remove
- }
- }
-
- if event.Has(Rename) || event.Has(Remove) {
- w.Remove(event.Name)
- w.mu.Lock()
- delete(w.fileExists, event.Name)
- w.mu.Unlock()
- }
-
- if path.isDir && event.Has(Write) && !event.Has(Remove) {
- w.sendDirectoryChangeEvents(event.Name)
- } else {
- if !w.sendEvent(event) {
- closed = true
- continue
- }
- }
-
- if event.Has(Remove) {
- // Look for a file that may have overwritten this.
- // For example, mv f1 f2 will delete f2, then create f2.
- if path.isDir {
- fileDir := filepath.Clean(event.Name)
- w.mu.Lock()
- _, found := w.watches[fileDir]
- w.mu.Unlock()
- if found {
- // make sure the directory exists before we watch for changes. When we
- // do a recursive watch and perform rm -fr, the parent directory might
- // have gone missing, ignore the missing directory and let the
- // upcoming delete event remove the watch from the parent directory.
- if _, err := os.Lstat(fileDir); err == nil {
- w.sendDirectoryChangeEvents(fileDir)
- }
- }
- } else {
- filePath := filepath.Clean(event.Name)
- if fileInfo, err := os.Lstat(filePath); err == nil {
- w.sendFileCreatedEventIfNew(filePath, fileInfo)
- }
- }
- }
- }
- }
-}
-
-// newEvent returns an platform-independent Event based on kqueue Fflags.
-func (w *Watcher) newEvent(name string, mask uint32) Event {
- e := Event{Name: name}
- if mask&unix.NOTE_DELETE == unix.NOTE_DELETE {
- e.Op |= Remove
- }
- if mask&unix.NOTE_WRITE == unix.NOTE_WRITE {
- e.Op |= Write
- }
- if mask&unix.NOTE_RENAME == unix.NOTE_RENAME {
- e.Op |= Rename
- }
- if mask&unix.NOTE_ATTRIB == unix.NOTE_ATTRIB {
- e.Op |= Chmod
- }
- return e
-}
-
-// watchDirectoryFiles to mimic inotify when adding a watch on a directory
-func (w *Watcher) watchDirectoryFiles(dirPath string) error {
- // Get all files
- files, err := ioutil.ReadDir(dirPath)
- if err != nil {
- return err
- }
-
- for _, fileInfo := range files {
- path := filepath.Join(dirPath, fileInfo.Name())
-
- cleanPath, err := w.internalWatch(path, fileInfo)
- if err != nil {
- // No permission to read the file; that's not a problem: just skip.
- // But do add it to w.fileExists to prevent it from being picked up
- // as a "new" file later (it still shows up in the directory
- // listing).
- switch {
- case errors.Is(err, unix.EACCES) || errors.Is(err, unix.EPERM):
- cleanPath = filepath.Clean(path)
- default:
- return fmt.Errorf("%q: %w", filepath.Join(dirPath, fileInfo.Name()), err)
- }
- }
-
- w.mu.Lock()
- w.fileExists[cleanPath] = struct{}{}
- w.mu.Unlock()
- }
-
- return nil
-}
-
-// Search the directory for new files and send an event for them.
-//
-// This functionality is to have the BSD watcher match the inotify, which sends
-// a create event for files created in a watched directory.
-func (w *Watcher) sendDirectoryChangeEvents(dir string) {
- // Get all files
- files, err := ioutil.ReadDir(dir)
- if err != nil {
- if !w.sendError(fmt.Errorf("fsnotify.sendDirectoryChangeEvents: %w", err)) {
- return
- }
- }
-
- // Search for new files
- for _, fi := range files {
- err := w.sendFileCreatedEventIfNew(filepath.Join(dir, fi.Name()), fi)
- if err != nil {
- return
- }
- }
-}
-
-// sendFileCreatedEvent sends a create event if the file isn't already being tracked.
-func (w *Watcher) sendFileCreatedEventIfNew(filePath string, fileInfo os.FileInfo) (err error) {
- w.mu.Lock()
- _, doesExist := w.fileExists[filePath]
- w.mu.Unlock()
- if !doesExist {
- if !w.sendEvent(Event{Name: filePath, Op: Create}) {
- return
- }
- }
-
- // like watchDirectoryFiles (but without doing another ReadDir)
- filePath, err = w.internalWatch(filePath, fileInfo)
- if err != nil {
- return err
- }
-
- w.mu.Lock()
- w.fileExists[filePath] = struct{}{}
- w.mu.Unlock()
-
- return nil
-}
-
-func (w *Watcher) internalWatch(name string, fileInfo os.FileInfo) (string, error) {
- if fileInfo.IsDir() {
- // mimic Linux providing delete events for subdirectories
- // but preserve the flags used if currently watching subdirectory
- w.mu.Lock()
- flags := w.dirFlags[name]
- w.mu.Unlock()
-
- flags |= unix.NOTE_DELETE | unix.NOTE_RENAME
- return w.addWatch(name, flags)
- }
-
- // watch file to mimic Linux inotify
- return w.addWatch(name, noteAllEvents)
-}
-
-// Register events with the queue.
-func (w *Watcher) register(fds []int, flags int, fflags uint32) error {
- changes := make([]unix.Kevent_t, len(fds))
- for i, fd := range fds {
- // SetKevent converts int to the platform-specific types.
- unix.SetKevent(&changes[i], fd, unix.EVFILT_VNODE, flags)
- changes[i].Fflags = fflags
- }
-
- // Register the events.
- success, err := unix.Kevent(w.kq, changes, nil, nil)
- if success == -1 {
- return err
- }
- return nil
-}
-
-// read retrieves pending events, or waits until an event occurs.
-func (w *Watcher) read(events []unix.Kevent_t) ([]unix.Kevent_t, error) {
- n, err := unix.Kevent(w.kq, nil, events, nil)
- if err != nil {
- return nil, err
- }
- return events[0:n], nil
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/backend_other.go b/vendor/github.com/fsnotify/fsnotify/backend_other.go
deleted file mode 100644
index a9bb1c3c..00000000
--- a/vendor/github.com/fsnotify/fsnotify/backend_other.go
+++ /dev/null
@@ -1,66 +0,0 @@
-//go:build !darwin && !dragonfly && !freebsd && !openbsd && !linux && !netbsd && !solaris && !windows
-// +build !darwin,!dragonfly,!freebsd,!openbsd,!linux,!netbsd,!solaris,!windows
-
-package fsnotify
-
-import (
- "fmt"
- "runtime"
-)
-
-// Watcher watches a set of files, delivering events to a channel.
-type Watcher struct{}
-
-// NewWatcher creates a new Watcher.
-func NewWatcher() (*Watcher, error) {
- return nil, fmt.Errorf("fsnotify not supported on %s", runtime.GOOS)
-}
-
-// Close removes all watches and closes the events channel.
-func (w *Watcher) Close() error {
- return nil
-}
-
-// Add starts monitoring the path for changes.
-//
-// A path can only be watched once; attempting to watch it more than once will
-// return an error. Paths that do not yet exist on the filesystem cannot be
-// added. A watch will be automatically removed if the path is deleted.
-//
-// A path will remain watched if it gets renamed to somewhere else on the same
-// filesystem, but the monitor will get removed if the path gets deleted and
-// re-created, or if it's moved to a different filesystem.
-//
-// Notifications on network filesystems (NFS, SMB, FUSE, etc.) or special
-// filesystems (/proc, /sys, etc.) generally don't work.
-//
-// # Watching directories
-//
-// All files in a directory are monitored, including new files that are created
-// after the watcher is started. Subdirectories are not watched (i.e. it's
-// non-recursive).
-//
-// # Watching files
-//
-// Watching individual files (rather than directories) is generally not
-// recommended as many tools update files atomically. Instead of "just" writing
-// to the file a temporary file will be written to first, and if successful the
-// temporary file is moved to to destination removing the original, or some
-// variant thereof. The watcher on the original file is now lost, as it no
-// longer exists.
-//
-// Instead, watch the parent directory and use Event.Name to filter out files
-// you're not interested in. There is an example of this in [cmd/fsnotify/file.go].
-func (w *Watcher) Add(name string) error {
- return nil
-}
-
-// Remove stops monitoring the path for changes.
-//
-// Directories are always removed non-recursively. For example, if you added
-// /tmp/dir and /tmp/dir/subdir then you will need to remove both.
-//
-// Removing a path that has not yet been added returns [ErrNonExistentWatch].
-func (w *Watcher) Remove(name string) error {
- return nil
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/backend_windows.go b/vendor/github.com/fsnotify/fsnotify/backend_windows.go
deleted file mode 100644
index ae392867..00000000
--- a/vendor/github.com/fsnotify/fsnotify/backend_windows.go
+++ /dev/null
@@ -1,746 +0,0 @@
-//go:build windows
-// +build windows
-
-package fsnotify
-
-import (
- "errors"
- "fmt"
- "os"
- "path/filepath"
- "reflect"
- "runtime"
- "strings"
- "sync"
- "unsafe"
-
- "golang.org/x/sys/windows"
-)
-
-// Watcher watches a set of paths, delivering events on a channel.
-//
-// A watcher should not be copied (e.g. pass it by pointer, rather than by
-// value).
-//
-// # Linux notes
-//
-// When a file is removed a Remove event won't be emitted until all file
-// descriptors are closed, and deletes will always emit a Chmod. For example:
-//
-// fp := os.Open("file")
-// os.Remove("file") // Triggers Chmod
-// fp.Close() // Triggers Remove
-//
-// This is the event that inotify sends, so not much can be changed about this.
-//
-// The fs.inotify.max_user_watches sysctl variable specifies the upper limit
-// for the number of watches per user, and fs.inotify.max_user_instances
-// specifies the maximum number of inotify instances per user. Every Watcher you
-// create is an "instance", and every path you add is a "watch".
-//
-// These are also exposed in /proc as /proc/sys/fs/inotify/max_user_watches and
-// /proc/sys/fs/inotify/max_user_instances
-//
-// To increase them you can use sysctl or write the value to the /proc file:
-//
-// # Default values on Linux 5.18
-// sysctl fs.inotify.max_user_watches=124983
-// sysctl fs.inotify.max_user_instances=128
-//
-// To make the changes persist on reboot edit /etc/sysctl.conf or
-// /usr/lib/sysctl.d/50-default.conf (details differ per Linux distro; check
-// your distro's documentation):
-//
-// fs.inotify.max_user_watches=124983
-// fs.inotify.max_user_instances=128
-//
-// Reaching the limit will result in a "no space left on device" or "too many open
-// files" error.
-//
-// # kqueue notes (macOS, BSD)
-//
-// kqueue requires opening a file descriptor for every file that's being watched;
-// so if you're watching a directory with five files then that's six file
-// descriptors. You will run in to your system's "max open files" limit faster on
-// these platforms.
-//
-// The sysctl variables kern.maxfiles and kern.maxfilesperproc can be used to
-// control the maximum number of open files, as well as /etc/login.conf on BSD
-// systems.
-//
-// # macOS notes
-//
-// Spotlight indexing on macOS can result in multiple events (see [#15]). A
-// temporary workaround is to add your folder(s) to the "Spotlight Privacy
-// Settings" until we have a native FSEvents implementation (see [#11]).
-//
-// [#11]: https://github.com/fsnotify/fsnotify/issues/11
-// [#15]: https://github.com/fsnotify/fsnotify/issues/15
-type Watcher struct {
- // Events sends the filesystem change events.
- //
- // fsnotify can send the following events; a "path" here can refer to a
- // file, directory, symbolic link, or special file like a FIFO.
- //
- // fsnotify.Create A new path was created; this may be followed by one
- // or more Write events if data also gets written to a
- // file.
- //
- // fsnotify.Remove A path was removed.
- //
- // fsnotify.Rename A path was renamed. A rename is always sent with the
- // old path as Event.Name, and a Create event will be
- // sent with the new name. Renames are only sent for
- // paths that are currently watched; e.g. moving an
- // unmonitored file into a monitored directory will
- // show up as just a Create. Similarly, renaming a file
- // to outside a monitored directory will show up as
- // only a Rename.
- //
- // fsnotify.Write A file or named pipe was written to. A Truncate will
- // also trigger a Write. A single "write action"
- // initiated by the user may show up as one or multiple
- // writes, depending on when the system syncs things to
- // disk. For example when compiling a large Go program
- // you may get hundreds of Write events, so you
- // probably want to wait until you've stopped receiving
- // them (see the dedup example in cmd/fsnotify).
- //
- // fsnotify.Chmod Attributes were changed. On Linux this is also sent
- // when a file is removed (or more accurately, when a
- // link to an inode is removed). On kqueue it's sent
- // and on kqueue when a file is truncated. On Windows
- // it's never sent.
- Events chan Event
-
- // Errors sends any errors.
- Errors chan error
-
- port windows.Handle // Handle to completion port
- input chan *input // Inputs to the reader are sent on this channel
- quit chan chan<- error
-
- mu sync.Mutex // Protects access to watches, isClosed
- watches watchMap // Map of watches (key: i-number)
- isClosed bool // Set to true when Close() is first called
-}
-
-// NewWatcher creates a new Watcher.
-func NewWatcher() (*Watcher, error) {
- port, err := windows.CreateIoCompletionPort(windows.InvalidHandle, 0, 0, 0)
- if err != nil {
- return nil, os.NewSyscallError("CreateIoCompletionPort", err)
- }
- w := &Watcher{
- port: port,
- watches: make(watchMap),
- input: make(chan *input, 1),
- Events: make(chan Event, 50),
- Errors: make(chan error),
- quit: make(chan chan<- error, 1),
- }
- go w.readEvents()
- return w, nil
-}
-
-func (w *Watcher) sendEvent(name string, mask uint64) bool {
- if mask == 0 {
- return false
- }
-
- event := w.newEvent(name, uint32(mask))
- select {
- case ch := <-w.quit:
- w.quit <- ch
- case w.Events <- event:
- }
- return true
-}
-
-// Returns true if the error was sent, or false if watcher is closed.
-func (w *Watcher) sendError(err error) bool {
- select {
- case w.Errors <- err:
- return true
- case <-w.quit:
- }
- return false
-}
-
-// Close removes all watches and closes the events channel.
-func (w *Watcher) Close() error {
- w.mu.Lock()
- if w.isClosed {
- w.mu.Unlock()
- return nil
- }
- w.isClosed = true
- w.mu.Unlock()
-
- // Send "quit" message to the reader goroutine
- ch := make(chan error)
- w.quit <- ch
- if err := w.wakeupReader(); err != nil {
- return err
- }
- return <-ch
-}
-
-// Add starts monitoring the path for changes.
-//
-// A path can only be watched once; attempting to watch it more than once will
-// return an error. Paths that do not yet exist on the filesystem cannot be
-// added. A watch will be automatically removed if the path is deleted.
-//
-// A path will remain watched if it gets renamed to somewhere else on the same
-// filesystem, but the monitor will get removed if the path gets deleted and
-// re-created, or if it's moved to a different filesystem.
-//
-// Notifications on network filesystems (NFS, SMB, FUSE, etc.) or special
-// filesystems (/proc, /sys, etc.) generally don't work.
-//
-// # Watching directories
-//
-// All files in a directory are monitored, including new files that are created
-// after the watcher is started. Subdirectories are not watched (i.e. it's
-// non-recursive).
-//
-// # Watching files
-//
-// Watching individual files (rather than directories) is generally not
-// recommended as many tools update files atomically. Instead of "just" writing
-// to the file a temporary file will be written to first, and if successful the
-// temporary file is moved to to destination removing the original, or some
-// variant thereof. The watcher on the original file is now lost, as it no
-// longer exists.
-//
-// Instead, watch the parent directory and use Event.Name to filter out files
-// you're not interested in. There is an example of this in [cmd/fsnotify/file.go].
-func (w *Watcher) Add(name string) error {
- w.mu.Lock()
- if w.isClosed {
- w.mu.Unlock()
- return errors.New("watcher already closed")
- }
- w.mu.Unlock()
-
- in := &input{
- op: opAddWatch,
- path: filepath.Clean(name),
- flags: sysFSALLEVENTS,
- reply: make(chan error),
- }
- w.input <- in
- if err := w.wakeupReader(); err != nil {
- return err
- }
- return <-in.reply
-}
-
-// Remove stops monitoring the path for changes.
-//
-// Directories are always removed non-recursively. For example, if you added
-// /tmp/dir and /tmp/dir/subdir then you will need to remove both.
-//
-// Removing a path that has not yet been added returns [ErrNonExistentWatch].
-func (w *Watcher) Remove(name string) error {
- in := &input{
- op: opRemoveWatch,
- path: filepath.Clean(name),
- reply: make(chan error),
- }
- w.input <- in
- if err := w.wakeupReader(); err != nil {
- return err
- }
- return <-in.reply
-}
-
-// WatchList returns all paths added with [Add] (and are not yet removed).
-func (w *Watcher) WatchList() []string {
- w.mu.Lock()
- defer w.mu.Unlock()
-
- entries := make([]string, 0, len(w.watches))
- for _, entry := range w.watches {
- for _, watchEntry := range entry {
- entries = append(entries, watchEntry.path)
- }
- }
-
- return entries
-}
-
-// These options are from the old golang.org/x/exp/winfsnotify, where you could
-// add various options to the watch. This has long since been removed.
-//
-// The "sys" in the name is misleading as they're not part of any "system".
-//
-// This should all be removed at some point, and just use windows.FILE_NOTIFY_*
-const (
- sysFSALLEVENTS = 0xfff
- sysFSATTRIB = 0x4
- sysFSCREATE = 0x100
- sysFSDELETE = 0x200
- sysFSDELETESELF = 0x400
- sysFSMODIFY = 0x2
- sysFSMOVE = 0xc0
- sysFSMOVEDFROM = 0x40
- sysFSMOVEDTO = 0x80
- sysFSMOVESELF = 0x800
- sysFSIGNORED = 0x8000
-)
-
-func (w *Watcher) newEvent(name string, mask uint32) Event {
- e := Event{Name: name}
- if mask&sysFSCREATE == sysFSCREATE || mask&sysFSMOVEDTO == sysFSMOVEDTO {
- e.Op |= Create
- }
- if mask&sysFSDELETE == sysFSDELETE || mask&sysFSDELETESELF == sysFSDELETESELF {
- e.Op |= Remove
- }
- if mask&sysFSMODIFY == sysFSMODIFY {
- e.Op |= Write
- }
- if mask&sysFSMOVE == sysFSMOVE || mask&sysFSMOVESELF == sysFSMOVESELF || mask&sysFSMOVEDFROM == sysFSMOVEDFROM {
- e.Op |= Rename
- }
- if mask&sysFSATTRIB == sysFSATTRIB {
- e.Op |= Chmod
- }
- return e
-}
-
-const (
- opAddWatch = iota
- opRemoveWatch
-)
-
-const (
- provisional uint64 = 1 << (32 + iota)
-)
-
-type input struct {
- op int
- path string
- flags uint32
- reply chan error
-}
-
-type inode struct {
- handle windows.Handle
- volume uint32
- index uint64
-}
-
-type watch struct {
- ov windows.Overlapped
- ino *inode // i-number
- path string // Directory path
- mask uint64 // Directory itself is being watched with these notify flags
- names map[string]uint64 // Map of names being watched and their notify flags
- rename string // Remembers the old name while renaming a file
- buf [65536]byte // 64K buffer
-}
-
-type (
- indexMap map[uint64]*watch
- watchMap map[uint32]indexMap
-)
-
-func (w *Watcher) wakeupReader() error {
- err := windows.PostQueuedCompletionStatus(w.port, 0, 0, nil)
- if err != nil {
- return os.NewSyscallError("PostQueuedCompletionStatus", err)
- }
- return nil
-}
-
-func (w *Watcher) getDir(pathname string) (dir string, err error) {
- attr, err := windows.GetFileAttributes(windows.StringToUTF16Ptr(pathname))
- if err != nil {
- return "", os.NewSyscallError("GetFileAttributes", err)
- }
- if attr&windows.FILE_ATTRIBUTE_DIRECTORY != 0 {
- dir = pathname
- } else {
- dir, _ = filepath.Split(pathname)
- dir = filepath.Clean(dir)
- }
- return
-}
-
-func (w *Watcher) getIno(path string) (ino *inode, err error) {
- h, err := windows.CreateFile(windows.StringToUTF16Ptr(path),
- windows.FILE_LIST_DIRECTORY,
- windows.FILE_SHARE_READ|windows.FILE_SHARE_WRITE|windows.FILE_SHARE_DELETE,
- nil, windows.OPEN_EXISTING,
- windows.FILE_FLAG_BACKUP_SEMANTICS|windows.FILE_FLAG_OVERLAPPED, 0)
- if err != nil {
- return nil, os.NewSyscallError("CreateFile", err)
- }
-
- var fi windows.ByHandleFileInformation
- err = windows.GetFileInformationByHandle(h, &fi)
- if err != nil {
- windows.CloseHandle(h)
- return nil, os.NewSyscallError("GetFileInformationByHandle", err)
- }
- ino = &inode{
- handle: h,
- volume: fi.VolumeSerialNumber,
- index: uint64(fi.FileIndexHigh)<<32 | uint64(fi.FileIndexLow),
- }
- return ino, nil
-}
-
-// Must run within the I/O thread.
-func (m watchMap) get(ino *inode) *watch {
- if i := m[ino.volume]; i != nil {
- return i[ino.index]
- }
- return nil
-}
-
-// Must run within the I/O thread.
-func (m watchMap) set(ino *inode, watch *watch) {
- i := m[ino.volume]
- if i == nil {
- i = make(indexMap)
- m[ino.volume] = i
- }
- i[ino.index] = watch
-}
-
-// Must run within the I/O thread.
-func (w *Watcher) addWatch(pathname string, flags uint64) error {
- dir, err := w.getDir(pathname)
- if err != nil {
- return err
- }
-
- ino, err := w.getIno(dir)
- if err != nil {
- return err
- }
- w.mu.Lock()
- watchEntry := w.watches.get(ino)
- w.mu.Unlock()
- if watchEntry == nil {
- _, err := windows.CreateIoCompletionPort(ino.handle, w.port, 0, 0)
- if err != nil {
- windows.CloseHandle(ino.handle)
- return os.NewSyscallError("CreateIoCompletionPort", err)
- }
- watchEntry = &watch{
- ino: ino,
- path: dir,
- names: make(map[string]uint64),
- }
- w.mu.Lock()
- w.watches.set(ino, watchEntry)
- w.mu.Unlock()
- flags |= provisional
- } else {
- windows.CloseHandle(ino.handle)
- }
- if pathname == dir {
- watchEntry.mask |= flags
- } else {
- watchEntry.names[filepath.Base(pathname)] |= flags
- }
-
- err = w.startRead(watchEntry)
- if err != nil {
- return err
- }
-
- if pathname == dir {
- watchEntry.mask &= ^provisional
- } else {
- watchEntry.names[filepath.Base(pathname)] &= ^provisional
- }
- return nil
-}
-
-// Must run within the I/O thread.
-func (w *Watcher) remWatch(pathname string) error {
- dir, err := w.getDir(pathname)
- if err != nil {
- return err
- }
- ino, err := w.getIno(dir)
- if err != nil {
- return err
- }
-
- w.mu.Lock()
- watch := w.watches.get(ino)
- w.mu.Unlock()
-
- err = windows.CloseHandle(ino.handle)
- if err != nil {
- w.sendError(os.NewSyscallError("CloseHandle", err))
- }
- if watch == nil {
- return fmt.Errorf("%w: %s", ErrNonExistentWatch, pathname)
- }
- if pathname == dir {
- w.sendEvent(watch.path, watch.mask&sysFSIGNORED)
- watch.mask = 0
- } else {
- name := filepath.Base(pathname)
- w.sendEvent(filepath.Join(watch.path, name), watch.names[name]&sysFSIGNORED)
- delete(watch.names, name)
- }
-
- return w.startRead(watch)
-}
-
-// Must run within the I/O thread.
-func (w *Watcher) deleteWatch(watch *watch) {
- for name, mask := range watch.names {
- if mask&provisional == 0 {
- w.sendEvent(filepath.Join(watch.path, name), mask&sysFSIGNORED)
- }
- delete(watch.names, name)
- }
- if watch.mask != 0 {
- if watch.mask&provisional == 0 {
- w.sendEvent(watch.path, watch.mask&sysFSIGNORED)
- }
- watch.mask = 0
- }
-}
-
-// Must run within the I/O thread.
-func (w *Watcher) startRead(watch *watch) error {
- err := windows.CancelIo(watch.ino.handle)
- if err != nil {
- w.sendError(os.NewSyscallError("CancelIo", err))
- w.deleteWatch(watch)
- }
- mask := w.toWindowsFlags(watch.mask)
- for _, m := range watch.names {
- mask |= w.toWindowsFlags(m)
- }
- if mask == 0 {
- err := windows.CloseHandle(watch.ino.handle)
- if err != nil {
- w.sendError(os.NewSyscallError("CloseHandle", err))
- }
- w.mu.Lock()
- delete(w.watches[watch.ino.volume], watch.ino.index)
- w.mu.Unlock()
- return nil
- }
-
- rdErr := windows.ReadDirectoryChanges(watch.ino.handle, &watch.buf[0],
- uint32(unsafe.Sizeof(watch.buf)), false, mask, nil, &watch.ov, 0)
- if rdErr != nil {
- err := os.NewSyscallError("ReadDirectoryChanges", rdErr)
- if rdErr == windows.ERROR_ACCESS_DENIED && watch.mask&provisional == 0 {
- // Watched directory was probably removed
- w.sendEvent(watch.path, watch.mask&sysFSDELETESELF)
- err = nil
- }
- w.deleteWatch(watch)
- w.startRead(watch)
- return err
- }
- return nil
-}
-
-// readEvents reads from the I/O completion port, converts the
-// received events into Event objects and sends them via the Events channel.
-// Entry point to the I/O thread.
-func (w *Watcher) readEvents() {
- var (
- n uint32
- key uintptr
- ov *windows.Overlapped
- )
- runtime.LockOSThread()
-
- for {
- qErr := windows.GetQueuedCompletionStatus(w.port, &n, &key, &ov, windows.INFINITE)
- // This error is handled after the watch == nil check below. NOTE: this
- // seems odd, note sure if it's correct.
-
- watch := (*watch)(unsafe.Pointer(ov))
- if watch == nil {
- select {
- case ch := <-w.quit:
- w.mu.Lock()
- var indexes []indexMap
- for _, index := range w.watches {
- indexes = append(indexes, index)
- }
- w.mu.Unlock()
- for _, index := range indexes {
- for _, watch := range index {
- w.deleteWatch(watch)
- w.startRead(watch)
- }
- }
-
- err := windows.CloseHandle(w.port)
- if err != nil {
- err = os.NewSyscallError("CloseHandle", err)
- }
- close(w.Events)
- close(w.Errors)
- ch <- err
- return
- case in := <-w.input:
- switch in.op {
- case opAddWatch:
- in.reply <- w.addWatch(in.path, uint64(in.flags))
- case opRemoveWatch:
- in.reply <- w.remWatch(in.path)
- }
- default:
- }
- continue
- }
-
- switch qErr {
- case windows.ERROR_MORE_DATA:
- if watch == nil {
- w.sendError(errors.New("ERROR_MORE_DATA has unexpectedly null lpOverlapped buffer"))
- } else {
- // The i/o succeeded but the buffer is full.
- // In theory we should be building up a full packet.
- // In practice we can get away with just carrying on.
- n = uint32(unsafe.Sizeof(watch.buf))
- }
- case windows.ERROR_ACCESS_DENIED:
- // Watched directory was probably removed
- w.sendEvent(watch.path, watch.mask&sysFSDELETESELF)
- w.deleteWatch(watch)
- w.startRead(watch)
- continue
- case windows.ERROR_OPERATION_ABORTED:
- // CancelIo was called on this handle
- continue
- default:
- w.sendError(os.NewSyscallError("GetQueuedCompletionPort", qErr))
- continue
- case nil:
- }
-
- var offset uint32
- for {
- if n == 0 {
- w.sendError(errors.New("short read in readEvents()"))
- break
- }
-
- // Point "raw" to the event in the buffer
- raw := (*windows.FileNotifyInformation)(unsafe.Pointer(&watch.buf[offset]))
-
- // Create a buf that is the size of the path name
- size := int(raw.FileNameLength / 2)
- var buf []uint16
- // TODO: Use unsafe.Slice in Go 1.17; https://stackoverflow.com/questions/51187973
- sh := (*reflect.SliceHeader)(unsafe.Pointer(&buf))
- sh.Data = uintptr(unsafe.Pointer(&raw.FileName))
- sh.Len = size
- sh.Cap = size
- name := windows.UTF16ToString(buf)
- fullname := filepath.Join(watch.path, name)
-
- var mask uint64
- switch raw.Action {
- case windows.FILE_ACTION_REMOVED:
- mask = sysFSDELETESELF
- case windows.FILE_ACTION_MODIFIED:
- mask = sysFSMODIFY
- case windows.FILE_ACTION_RENAMED_OLD_NAME:
- watch.rename = name
- case windows.FILE_ACTION_RENAMED_NEW_NAME:
- // Update saved path of all sub-watches.
- old := filepath.Join(watch.path, watch.rename)
- w.mu.Lock()
- for _, watchMap := range w.watches {
- for _, ww := range watchMap {
- if strings.HasPrefix(ww.path, old) {
- ww.path = filepath.Join(fullname, strings.TrimPrefix(ww.path, old))
- }
- }
- }
- w.mu.Unlock()
-
- if watch.names[watch.rename] != 0 {
- watch.names[name] |= watch.names[watch.rename]
- delete(watch.names, watch.rename)
- mask = sysFSMOVESELF
- }
- }
-
- sendNameEvent := func() {
- w.sendEvent(fullname, watch.names[name]&mask)
- }
- if raw.Action != windows.FILE_ACTION_RENAMED_NEW_NAME {
- sendNameEvent()
- }
- if raw.Action == windows.FILE_ACTION_REMOVED {
- w.sendEvent(fullname, watch.names[name]&sysFSIGNORED)
- delete(watch.names, name)
- }
-
- w.sendEvent(fullname, watch.mask&w.toFSnotifyFlags(raw.Action))
- if raw.Action == windows.FILE_ACTION_RENAMED_NEW_NAME {
- fullname = filepath.Join(watch.path, watch.rename)
- sendNameEvent()
- }
-
- // Move to the next event in the buffer
- if raw.NextEntryOffset == 0 {
- break
- }
- offset += raw.NextEntryOffset
-
- // Error!
- if offset >= n {
- w.sendError(errors.New(
- "Windows system assumed buffer larger than it is, events have likely been missed."))
- break
- }
- }
-
- if err := w.startRead(watch); err != nil {
- w.sendError(err)
- }
- }
-}
-
-func (w *Watcher) toWindowsFlags(mask uint64) uint32 {
- var m uint32
- if mask&sysFSMODIFY != 0 {
- m |= windows.FILE_NOTIFY_CHANGE_LAST_WRITE
- }
- if mask&sysFSATTRIB != 0 {
- m |= windows.FILE_NOTIFY_CHANGE_ATTRIBUTES
- }
- if mask&(sysFSMOVE|sysFSCREATE|sysFSDELETE) != 0 {
- m |= windows.FILE_NOTIFY_CHANGE_FILE_NAME | windows.FILE_NOTIFY_CHANGE_DIR_NAME
- }
- return m
-}
-
-func (w *Watcher) toFSnotifyFlags(action uint32) uint64 {
- switch action {
- case windows.FILE_ACTION_ADDED:
- return sysFSCREATE
- case windows.FILE_ACTION_REMOVED:
- return sysFSDELETE
- case windows.FILE_ACTION_MODIFIED:
- return sysFSMODIFY
- case windows.FILE_ACTION_RENAMED_OLD_NAME:
- return sysFSMOVEDFROM
- case windows.FILE_ACTION_RENAMED_NEW_NAME:
- return sysFSMOVEDTO
- }
- return 0
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/fsnotify.go b/vendor/github.com/fsnotify/fsnotify/fsnotify.go
deleted file mode 100644
index 30a5bf0f..00000000
--- a/vendor/github.com/fsnotify/fsnotify/fsnotify.go
+++ /dev/null
@@ -1,81 +0,0 @@
-//go:build !plan9
-// +build !plan9
-
-// Package fsnotify provides a cross-platform interface for file system
-// notifications.
-package fsnotify
-
-import (
- "errors"
- "fmt"
- "strings"
-)
-
-// Event represents a file system notification.
-type Event struct {
- // Path to the file or directory.
- //
- // Paths are relative to the input; for example with Add("dir") the Name
- // will be set to "dir/file" if you create that file, but if you use
- // Add("/path/to/dir") it will be "/path/to/dir/file".
- Name string
-
- // File operation that triggered the event.
- //
- // This is a bitmask and some systems may send multiple operations at once.
- // Use the Event.Has() method instead of comparing with ==.
- Op Op
-}
-
-// Op describes a set of file operations.
-type Op uint32
-
-// The operations fsnotify can trigger; see the documentation on [Watcher] for a
-// full description, and check them with [Event.Has].
-const (
- Create Op = 1 << iota
- Write
- Remove
- Rename
- Chmod
-)
-
-// Common errors that can be reported by a watcher
-var (
- ErrNonExistentWatch = errors.New("can't remove non-existent watcher")
- ErrEventOverflow = errors.New("fsnotify queue overflow")
-)
-
-func (op Op) String() string {
- var b strings.Builder
- if op.Has(Create) {
- b.WriteString("|CREATE")
- }
- if op.Has(Remove) {
- b.WriteString("|REMOVE")
- }
- if op.Has(Write) {
- b.WriteString("|WRITE")
- }
- if op.Has(Rename) {
- b.WriteString("|RENAME")
- }
- if op.Has(Chmod) {
- b.WriteString("|CHMOD")
- }
- if b.Len() == 0 {
- return "[no events]"
- }
- return b.String()[1:]
-}
-
-// Has reports if this operation has the given operation.
-func (o Op) Has(h Op) bool { return o&h == h }
-
-// Has reports if this event has the given operation.
-func (e Event) Has(op Op) bool { return e.Op.Has(op) }
-
-// String returns a string representation of the event with their path.
-func (e Event) String() string {
- return fmt.Sprintf("%-13s %q", e.Op.String(), e.Name)
-}
diff --git a/vendor/github.com/fsnotify/fsnotify/mkdoc.zsh b/vendor/github.com/fsnotify/fsnotify/mkdoc.zsh
deleted file mode 100644
index b09ef768..00000000
--- a/vendor/github.com/fsnotify/fsnotify/mkdoc.zsh
+++ /dev/null
@@ -1,208 +0,0 @@
-#!/usr/bin/env zsh
-[ "${ZSH_VERSION:-}" = "" ] && echo >&2 "Only works with zsh" && exit 1
-setopt err_exit no_unset pipefail extended_glob
-
-# Simple script to update the godoc comments on all watchers. Probably took me
-# more time to write this than doing it manually, but ah well 🙃
-
-watcher=$(</tmp/x
- print -r -- $cmt >>/tmp/x
- tail -n+$(( end + 1 )) $file >>/tmp/x
- mv /tmp/x $file
- done
-}
-
-set-cmt '^type Watcher struct ' $watcher
-set-cmt '^func NewWatcher(' $new
-set-cmt '^func (w \*Watcher) Add(' $add
-set-cmt '^func (w \*Watcher) Remove(' $remove
-set-cmt '^func (w \*Watcher) Close(' $close
-set-cmt '^func (w \*Watcher) WatchList(' $watchlist
-set-cmt '^[[:space:]]*Events *chan Event$' $events
-set-cmt '^[[:space:]]*Errors *chan error$' $errors
diff --git a/vendor/github.com/fsnotify/fsnotify/system_bsd.go b/vendor/github.com/fsnotify/fsnotify/system_bsd.go
deleted file mode 100644
index 4322b0b8..00000000
--- a/vendor/github.com/fsnotify/fsnotify/system_bsd.go
+++ /dev/null
@@ -1,8 +0,0 @@
-//go:build freebsd || openbsd || netbsd || dragonfly
-// +build freebsd openbsd netbsd dragonfly
-
-package fsnotify
-
-import "golang.org/x/sys/unix"
-
-const openMode = unix.O_NONBLOCK | unix.O_RDONLY | unix.O_CLOEXEC
diff --git a/vendor/github.com/fsnotify/fsnotify/system_darwin.go b/vendor/github.com/fsnotify/fsnotify/system_darwin.go
deleted file mode 100644
index 5da5ffa7..00000000
--- a/vendor/github.com/fsnotify/fsnotify/system_darwin.go
+++ /dev/null
@@ -1,9 +0,0 @@
-//go:build darwin
-// +build darwin
-
-package fsnotify
-
-import "golang.org/x/sys/unix"
-
-// note: this constant is not defined on BSD
-const openMode = unix.O_EVTONLY | unix.O_CLOEXEC
diff --git a/vendor/github.com/getkin/kin-openapi/LICENSE b/vendor/github.com/getkin/kin-openapi/LICENSE
deleted file mode 100644
index 992b9831..00000000
--- a/vendor/github.com/getkin/kin-openapi/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2017-2018 the project authors.
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/callback.go b/vendor/github.com/getkin/kin-openapi/openapi3/callback.go
deleted file mode 100644
index 62cea72d..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/callback.go
+++ /dev/null
@@ -1,48 +0,0 @@
-package openapi3
-
-import (
- "context"
- "fmt"
- "sort"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type Callbacks map[string]*CallbackRef
-
-var _ jsonpointer.JSONPointable = (*Callbacks)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (c Callbacks) JSONLookup(token string) (interface{}, error) {
- ref, ok := c[token]
- if ref == nil || !ok {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// Callback is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#callback-object
-type Callback map[string]*PathItem
-
-// Validate returns an error if Callback does not comply with the OpenAPI spec.
-func (callback Callback) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- keys := make([]string, 0, len(callback))
- for key := range callback {
- keys = append(keys, key)
- }
- sort.Strings(keys)
- for _, key := range keys {
- v := callback[key]
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/components.go b/vendor/github.com/getkin/kin-openapi/openapi3/components.go
deleted file mode 100644
index 0981e8bf..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/components.go
+++ /dev/null
@@ -1,242 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "fmt"
- "regexp"
- "sort"
-)
-
-// Components is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#components-object
-type Components struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Schemas Schemas `json:"schemas,omitempty" yaml:"schemas,omitempty"`
- Parameters ParametersMap `json:"parameters,omitempty" yaml:"parameters,omitempty"`
- Headers Headers `json:"headers,omitempty" yaml:"headers,omitempty"`
- RequestBodies RequestBodies `json:"requestBodies,omitempty" yaml:"requestBodies,omitempty"`
- Responses Responses `json:"responses,omitempty" yaml:"responses,omitempty"`
- SecuritySchemes SecuritySchemes `json:"securitySchemes,omitempty" yaml:"securitySchemes,omitempty"`
- Examples Examples `json:"examples,omitempty" yaml:"examples,omitempty"`
- Links Links `json:"links,omitempty" yaml:"links,omitempty"`
- Callbacks Callbacks `json:"callbacks,omitempty" yaml:"callbacks,omitempty"`
-}
-
-func NewComponents() Components {
- return Components{}
-}
-
-// MarshalJSON returns the JSON encoding of Components.
-func (components Components) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 9+len(components.Extensions))
- for k, v := range components.Extensions {
- m[k] = v
- }
- if x := components.Schemas; len(x) != 0 {
- m["schemas"] = x
- }
- if x := components.Parameters; len(x) != 0 {
- m["parameters"] = x
- }
- if x := components.Headers; len(x) != 0 {
- m["headers"] = x
- }
- if x := components.RequestBodies; len(x) != 0 {
- m["requestBodies"] = x
- }
- if x := components.Responses; len(x) != 0 {
- m["responses"] = x
- }
- if x := components.SecuritySchemes; len(x) != 0 {
- m["securitySchemes"] = x
- }
- if x := components.Examples; len(x) != 0 {
- m["examples"] = x
- }
- if x := components.Links; len(x) != 0 {
- m["links"] = x
- }
- if x := components.Callbacks; len(x) != 0 {
- m["callbacks"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Components to a copy of data.
-func (components *Components) UnmarshalJSON(data []byte) error {
- type ComponentsBis Components
- var x ComponentsBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "schemas")
- delete(x.Extensions, "parameters")
- delete(x.Extensions, "headers")
- delete(x.Extensions, "requestBodies")
- delete(x.Extensions, "responses")
- delete(x.Extensions, "securitySchemes")
- delete(x.Extensions, "examples")
- delete(x.Extensions, "links")
- delete(x.Extensions, "callbacks")
- *components = Components(x)
- return nil
-}
-
-// Validate returns an error if Components does not comply with the OpenAPI spec.
-func (components *Components) Validate(ctx context.Context, opts ...ValidationOption) (err error) {
- ctx = WithValidationOptions(ctx, opts...)
-
- schemas := make([]string, 0, len(components.Schemas))
- for name := range components.Schemas {
- schemas = append(schemas, name)
- }
- sort.Strings(schemas)
- for _, k := range schemas {
- v := components.Schemas[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("schema %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("schema %q: %w", k, err)
- }
- }
-
- parameters := make([]string, 0, len(components.Parameters))
- for name := range components.Parameters {
- parameters = append(parameters, name)
- }
- sort.Strings(parameters)
- for _, k := range parameters {
- v := components.Parameters[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("parameter %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("parameter %q: %w", k, err)
- }
- }
-
- requestBodies := make([]string, 0, len(components.RequestBodies))
- for name := range components.RequestBodies {
- requestBodies = append(requestBodies, name)
- }
- sort.Strings(requestBodies)
- for _, k := range requestBodies {
- v := components.RequestBodies[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("request body %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("request body %q: %w", k, err)
- }
- }
-
- responses := make([]string, 0, len(components.Responses))
- for name := range components.Responses {
- responses = append(responses, name)
- }
- sort.Strings(responses)
- for _, k := range responses {
- v := components.Responses[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("response %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("response %q: %w", k, err)
- }
- }
-
- headers := make([]string, 0, len(components.Headers))
- for name := range components.Headers {
- headers = append(headers, name)
- }
- sort.Strings(headers)
- for _, k := range headers {
- v := components.Headers[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("header %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("header %q: %w", k, err)
- }
- }
-
- securitySchemes := make([]string, 0, len(components.SecuritySchemes))
- for name := range components.SecuritySchemes {
- securitySchemes = append(securitySchemes, name)
- }
- sort.Strings(securitySchemes)
- for _, k := range securitySchemes {
- v := components.SecuritySchemes[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("security scheme %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("security scheme %q: %w", k, err)
- }
- }
-
- examples := make([]string, 0, len(components.Examples))
- for name := range components.Examples {
- examples = append(examples, name)
- }
- sort.Strings(examples)
- for _, k := range examples {
- v := components.Examples[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("example %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("example %q: %w", k, err)
- }
- }
-
- links := make([]string, 0, len(components.Links))
- for name := range components.Links {
- links = append(links, name)
- }
- sort.Strings(links)
- for _, k := range links {
- v := components.Links[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("link %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("link %q: %w", k, err)
- }
- }
-
- callbacks := make([]string, 0, len(components.Callbacks))
- for name := range components.Callbacks {
- callbacks = append(callbacks, name)
- }
- sort.Strings(callbacks)
- for _, k := range callbacks {
- v := components.Callbacks[k]
- if err = ValidateIdentifier(k); err != nil {
- return fmt.Errorf("callback %q: %w", k, err)
- }
- if err = v.Validate(ctx); err != nil {
- return fmt.Errorf("callback %q: %w", k, err)
- }
- }
-
- return validateExtensions(ctx, components.Extensions)
-}
-
-const identifierPattern = `^[a-zA-Z0-9._-]+$`
-
-// IdentifierRegExp verifies whether Component object key matches 'identifierPattern' pattern, according to OapiAPI v3.x.0.
-// Hovever, to be able supporting legacy OpenAPI v2.x, there is a need to customize above pattern in orde not to fail
-// converted v2-v3 validation
-var IdentifierRegExp = regexp.MustCompile(identifierPattern)
-
-func ValidateIdentifier(value string) error {
- if IdentifierRegExp.MatchString(value) {
- return nil
- }
- return fmt.Errorf("identifier %q is not supported by OpenAPIv3 standard (regexp: %q)", value, identifierPattern)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/content.go b/vendor/github.com/getkin/kin-openapi/openapi3/content.go
deleted file mode 100644
index 81b070ee..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/content.go
+++ /dev/null
@@ -1,124 +0,0 @@
-package openapi3
-
-import (
- "context"
- "sort"
- "strings"
-)
-
-// Content is specified by OpenAPI/Swagger 3.0 standard.
-type Content map[string]*MediaType
-
-func NewContent() Content {
- return make(map[string]*MediaType)
-}
-
-func NewContentWithSchema(schema *Schema, consumes []string) Content {
- if len(consumes) == 0 {
- return Content{
- "*/*": NewMediaType().WithSchema(schema),
- }
- }
- content := make(map[string]*MediaType, len(consumes))
- for _, mediaType := range consumes {
- content[mediaType] = NewMediaType().WithSchema(schema)
- }
- return content
-}
-
-func NewContentWithSchemaRef(schema *SchemaRef, consumes []string) Content {
- if len(consumes) == 0 {
- return Content{
- "*/*": NewMediaType().WithSchemaRef(schema),
- }
- }
- content := make(map[string]*MediaType, len(consumes))
- for _, mediaType := range consumes {
- content[mediaType] = NewMediaType().WithSchemaRef(schema)
- }
- return content
-}
-
-func NewContentWithJSONSchema(schema *Schema) Content {
- return Content{
- "application/json": NewMediaType().WithSchema(schema),
- }
-}
-func NewContentWithJSONSchemaRef(schema *SchemaRef) Content {
- return Content{
- "application/json": NewMediaType().WithSchemaRef(schema),
- }
-}
-
-func NewContentWithFormDataSchema(schema *Schema) Content {
- return Content{
- "multipart/form-data": NewMediaType().WithSchema(schema),
- }
-}
-
-func NewContentWithFormDataSchemaRef(schema *SchemaRef) Content {
- return Content{
- "multipart/form-data": NewMediaType().WithSchemaRef(schema),
- }
-}
-
-func (content Content) Get(mime string) *MediaType {
- // If the mime is empty then short-circuit to the wildcard.
- // We do this here so that we catch only the specific case of
- // and empty mime rather than a present, but invalid, mime type.
- if mime == "" {
- return content["*/*"]
- }
- // Start by making the most specific match possible
- // by using the mime type in full.
- if v := content[mime]; v != nil {
- return v
- }
- // If an exact match is not found then we strip all
- // metadata from the mime type and only use the x/y
- // portion.
- i := strings.IndexByte(mime, ';')
- if i < 0 {
- // If there is no metadata then preserve the full mime type
- // string for later wildcard searches.
- i = len(mime)
- }
- mime = mime[:i]
- if v := content[mime]; v != nil {
- return v
- }
- // If the x/y pattern has no specific match then we
- // try the x/* pattern.
- i = strings.IndexByte(mime, '/')
- if i < 0 {
- // In the case that the given mime type is not valid because it is
- // missing the subtype we return nil so that this does not accidentally
- // resolve with the wildcard.
- return nil
- }
- mime = mime[:i] + "/*"
- if v := content[mime]; v != nil {
- return v
- }
- // Finally, the most generic match of */* is returned
- // as a catch-all.
- return content["*/*"]
-}
-
-// Validate returns an error if Content does not comply with the OpenAPI spec.
-func (content Content) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- keys := make([]string, 0, len(content))
- for key := range content {
- keys = append(keys, key)
- }
- sort.Strings(keys)
- for _, k := range keys {
- v := content[k]
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/discriminator.go b/vendor/github.com/getkin/kin-openapi/openapi3/discriminator.go
deleted file mode 100644
index 8b6b813f..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/discriminator.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
-)
-
-// Discriminator is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#discriminator-object
-type Discriminator struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- PropertyName string `json:"propertyName" yaml:"propertyName"` // required
- Mapping map[string]string `json:"mapping,omitempty" yaml:"mapping,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of Discriminator.
-func (discriminator Discriminator) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 2+len(discriminator.Extensions))
- for k, v := range discriminator.Extensions {
- m[k] = v
- }
- m["propertyName"] = discriminator.PropertyName
- if x := discriminator.Mapping; len(x) != 0 {
- m["mapping"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Discriminator to a copy of data.
-func (discriminator *Discriminator) UnmarshalJSON(data []byte) error {
- type DiscriminatorBis Discriminator
- var x DiscriminatorBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "propertyName")
- delete(x.Extensions, "mapping")
- *discriminator = Discriminator(x)
- return nil
-}
-
-// Validate returns an error if Discriminator does not comply with the OpenAPI spec.
-func (discriminator *Discriminator) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- return validateExtensions(ctx, discriminator.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/doc.go b/vendor/github.com/getkin/kin-openapi/openapi3/doc.go
deleted file mode 100644
index 41c9965c..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/doc.go
+++ /dev/null
@@ -1,4 +0,0 @@
-// Package openapi3 parses and writes OpenAPI 3 specification documents.
-//
-// See https://github.com/OAI/OpenAPI-Specification/blob/master/versions/3.0.3.md
-package openapi3
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/encoding.go b/vendor/github.com/getkin/kin-openapi/openapi3/encoding.go
deleted file mode 100644
index dc2e5443..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/encoding.go
+++ /dev/null
@@ -1,136 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "fmt"
- "sort"
-)
-
-// Encoding is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#encoding-object
-type Encoding struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- ContentType string `json:"contentType,omitempty" yaml:"contentType,omitempty"`
- Headers Headers `json:"headers,omitempty" yaml:"headers,omitempty"`
- Style string `json:"style,omitempty" yaml:"style,omitempty"`
- Explode *bool `json:"explode,omitempty" yaml:"explode,omitempty"`
- AllowReserved bool `json:"allowReserved,omitempty" yaml:"allowReserved,omitempty"`
-}
-
-func NewEncoding() *Encoding {
- return &Encoding{}
-}
-
-func (encoding *Encoding) WithHeader(name string, header *Header) *Encoding {
- return encoding.WithHeaderRef(name, &HeaderRef{
- Value: header,
- })
-}
-
-func (encoding *Encoding) WithHeaderRef(name string, ref *HeaderRef) *Encoding {
- headers := encoding.Headers
- if headers == nil {
- headers = make(map[string]*HeaderRef)
- encoding.Headers = headers
- }
- headers[name] = ref
- return encoding
-}
-
-// MarshalJSON returns the JSON encoding of Encoding.
-func (encoding Encoding) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 5+len(encoding.Extensions))
- for k, v := range encoding.Extensions {
- m[k] = v
- }
- if x := encoding.ContentType; x != "" {
- m["contentType"] = x
- }
- if x := encoding.Headers; len(x) != 0 {
- m["headers"] = x
- }
- if x := encoding.Style; x != "" {
- m["style"] = x
- }
- if x := encoding.Explode; x != nil {
- m["explode"] = x
- }
- if x := encoding.AllowReserved; x {
- m["allowReserved"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Encoding to a copy of data.
-func (encoding *Encoding) UnmarshalJSON(data []byte) error {
- type EncodingBis Encoding
- var x EncodingBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "contentType")
- delete(x.Extensions, "headers")
- delete(x.Extensions, "style")
- delete(x.Extensions, "explode")
- delete(x.Extensions, "allowReserved")
- *encoding = Encoding(x)
- return nil
-}
-
-// SerializationMethod returns a serialization method of request body.
-// When serialization method is not defined the method returns the default serialization method.
-func (encoding *Encoding) SerializationMethod() *SerializationMethod {
- sm := &SerializationMethod{Style: SerializationForm, Explode: true}
- if encoding != nil {
- if encoding.Style != "" {
- sm.Style = encoding.Style
- }
- if encoding.Explode != nil {
- sm.Explode = *encoding.Explode
- }
- }
- return sm
-}
-
-// Validate returns an error if Encoding does not comply with the OpenAPI spec.
-func (encoding *Encoding) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if encoding == nil {
- return nil
- }
-
- headers := make([]string, 0, len(encoding.Headers))
- for k := range encoding.Headers {
- headers = append(headers, k)
- }
- sort.Strings(headers)
- for _, k := range headers {
- v := encoding.Headers[k]
- if err := ValidateIdentifier(k); err != nil {
- return nil
- }
- if err := v.Validate(ctx); err != nil {
- return nil
- }
- }
-
- // Validate a media types's serialization method.
- sm := encoding.SerializationMethod()
- switch {
- case sm.Style == SerializationForm && sm.Explode,
- sm.Style == SerializationForm && !sm.Explode,
- sm.Style == SerializationSpaceDelimited && sm.Explode,
- sm.Style == SerializationSpaceDelimited && !sm.Explode,
- sm.Style == SerializationPipeDelimited && sm.Explode,
- sm.Style == SerializationPipeDelimited && !sm.Explode,
- sm.Style == SerializationDeepObject && sm.Explode:
- default:
- return fmt.Errorf("serialization method with style=%q and explode=%v is not supported by media type", sm.Style, sm.Explode)
- }
-
- return validateExtensions(ctx, encoding.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/errors.go b/vendor/github.com/getkin/kin-openapi/openapi3/errors.go
deleted file mode 100644
index 74baab9a..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/errors.go
+++ /dev/null
@@ -1,59 +0,0 @@
-package openapi3
-
-import (
- "bytes"
- "errors"
-)
-
-// MultiError is a collection of errors, intended for when
-// multiple issues need to be reported upstream
-type MultiError []error
-
-func (me MultiError) Error() string {
- return spliceErr(" | ", me)
-}
-
-func spliceErr(sep string, errs []error) string {
- buff := &bytes.Buffer{}
- for i, e := range errs {
- buff.WriteString(e.Error())
- if i != len(errs)-1 {
- buff.WriteString(sep)
- }
- }
- return buff.String()
-}
-
-// Is allows you to determine if a generic error is in fact a MultiError using `errors.Is()`
-// It will also return true if any of the contained errors match target
-func (me MultiError) Is(target error) bool {
- if _, ok := target.(MultiError); ok {
- return true
- }
- for _, e := range me {
- if errors.Is(e, target) {
- return true
- }
- }
- return false
-}
-
-// As allows you to use `errors.As()` to set target to the first error within the multi error that matches the target type
-func (me MultiError) As(target interface{}) bool {
- for _, e := range me {
- if errors.As(e, target) {
- return true
- }
- }
- return false
-}
-
-type multiErrorForOneOf MultiError
-
-func (meo multiErrorForOneOf) Error() string {
- return spliceErr(" Or ", meo)
-}
-
-func (meo multiErrorForOneOf) Unwrap() error {
- return MultiError(meo)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/example.go b/vendor/github.com/getkin/kin-openapi/openapi3/example.go
deleted file mode 100644
index 04338bee..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/example.go
+++ /dev/null
@@ -1,93 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type Examples map[string]*ExampleRef
-
-var _ jsonpointer.JSONPointable = (*Examples)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (e Examples) JSONLookup(token string) (interface{}, error) {
- ref, ok := e[token]
- if ref == nil || !ok {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// Example is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#example-object
-type Example struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Summary string `json:"summary,omitempty" yaml:"summary,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Value interface{} `json:"value,omitempty" yaml:"value,omitempty"`
- ExternalValue string `json:"externalValue,omitempty" yaml:"externalValue,omitempty"`
-}
-
-func NewExample(value interface{}) *Example {
- return &Example{Value: value}
-}
-
-// MarshalJSON returns the JSON encoding of Example.
-func (example Example) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(example.Extensions))
- for k, v := range example.Extensions {
- m[k] = v
- }
- if x := example.Summary; x != "" {
- m["summary"] = x
- }
- if x := example.Description; x != "" {
- m["description"] = x
- }
- if x := example.Value; x != nil {
- m["value"] = x
- }
- if x := example.ExternalValue; x != "" {
- m["externalValue"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Example to a copy of data.
-func (example *Example) UnmarshalJSON(data []byte) error {
- type ExampleBis Example
- var x ExampleBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "summary")
- delete(x.Extensions, "description")
- delete(x.Extensions, "value")
- delete(x.Extensions, "externalValue")
- *example = Example(x)
- return nil
-}
-
-// Validate returns an error if Example does not comply with the OpenAPI spec.
-func (example *Example) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if example.Value != nil && example.ExternalValue != "" {
- return errors.New("value and externalValue are mutually exclusive")
- }
- if example.Value == nil && example.ExternalValue == "" {
- return errors.New("no value or externalValue field")
- }
-
- return validateExtensions(ctx, example.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/example_validation.go b/vendor/github.com/getkin/kin-openapi/openapi3/example_validation.go
deleted file mode 100644
index fb7a1da1..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/example_validation.go
+++ /dev/null
@@ -1,16 +0,0 @@
-package openapi3
-
-import "context"
-
-func validateExampleValue(ctx context.Context, input interface{}, schema *Schema) error {
- opts := make([]SchemaValidationOption, 0, 2)
-
- if vo := getValidationOptions(ctx); vo.examplesValidationAsReq {
- opts = append(opts, VisitAsRequest())
- } else if vo.examplesValidationAsRes {
- opts = append(opts, VisitAsResponse())
- }
- opts = append(opts, MultiErrors())
-
- return schema.VisitJSON(input, opts...)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/extension.go b/vendor/github.com/getkin/kin-openapi/openapi3/extension.go
deleted file mode 100644
index c2995909..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/extension.go
+++ /dev/null
@@ -1,24 +0,0 @@
-package openapi3
-
-import (
- "context"
- "fmt"
- "sort"
- "strings"
-)
-
-func validateExtensions(ctx context.Context, extensions map[string]interface{}) error { // FIXME: newtype + Validate(...)
- var unknowns []string
- for k := range extensions {
- if !strings.HasPrefix(k, "x-") {
- unknowns = append(unknowns, k)
- }
- }
-
- if len(unknowns) != 0 {
- sort.Strings(unknowns)
- return fmt.Errorf("extra sibling fields: %+v", unknowns)
- }
-
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/external_docs.go b/vendor/github.com/getkin/kin-openapi/openapi3/external_docs.go
deleted file mode 100644
index 276a36cc..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/external_docs.go
+++ /dev/null
@@ -1,61 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "net/url"
-)
-
-// ExternalDocs is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#external-documentation-object
-type ExternalDocs struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- URL string `json:"url,omitempty" yaml:"url,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of ExternalDocs.
-func (e ExternalDocs) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 2+len(e.Extensions))
- for k, v := range e.Extensions {
- m[k] = v
- }
- if x := e.Description; x != "" {
- m["description"] = x
- }
- if x := e.URL; x != "" {
- m["url"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets ExternalDocs to a copy of data.
-func (e *ExternalDocs) UnmarshalJSON(data []byte) error {
- type ExternalDocsBis ExternalDocs
- var x ExternalDocsBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "description")
- delete(x.Extensions, "url")
- *e = ExternalDocs(x)
- return nil
-}
-
-// Validate returns an error if ExternalDocs does not comply with the OpenAPI spec.
-func (e *ExternalDocs) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if e.URL == "" {
- return errors.New("url is required")
- }
- if _, err := url.Parse(e.URL); err != nil {
- return fmt.Errorf("url is incorrect: %w", err)
- }
-
- return validateExtensions(ctx, e.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/header.go b/vendor/github.com/getkin/kin-openapi/openapi3/header.go
deleted file mode 100644
index 8bce69f2..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/header.go
+++ /dev/null
@@ -1,103 +0,0 @@
-package openapi3
-
-import (
- "context"
- "errors"
- "fmt"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type Headers map[string]*HeaderRef
-
-var _ jsonpointer.JSONPointable = (*Headers)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (h Headers) JSONLookup(token string) (interface{}, error) {
- ref, ok := h[token]
- if ref == nil || !ok {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// Header is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#header-object
-type Header struct {
- Parameter
-}
-
-var _ jsonpointer.JSONPointable = (*Header)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (header Header) JSONLookup(token string) (interface{}, error) {
- return header.Parameter.JSONLookup(token)
-}
-
-// MarshalJSON returns the JSON encoding of Header.
-func (header Header) MarshalJSON() ([]byte, error) {
- return header.Parameter.MarshalJSON()
-}
-
-// UnmarshalJSON sets Header to a copy of data.
-func (header *Header) UnmarshalJSON(data []byte) error {
- return header.Parameter.UnmarshalJSON(data)
-}
-
-// SerializationMethod returns a header's serialization method.
-func (header *Header) SerializationMethod() (*SerializationMethod, error) {
- style := header.Style
- if style == "" {
- style = SerializationSimple
- }
- explode := false
- if header.Explode != nil {
- explode = *header.Explode
- }
- return &SerializationMethod{Style: style, Explode: explode}, nil
-}
-
-// Validate returns an error if Header does not comply with the OpenAPI spec.
-func (header *Header) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if header.Name != "" {
- return errors.New("header 'name' MUST NOT be specified, it is given in the corresponding headers map")
- }
- if header.In != "" {
- return errors.New("header 'in' MUST NOT be specified, it is implicitly in header")
- }
-
- // Validate a parameter's serialization method.
- sm, err := header.SerializationMethod()
- if err != nil {
- return err
- }
- if smSupported := false ||
- sm.Style == SerializationSimple && !sm.Explode ||
- sm.Style == SerializationSimple && sm.Explode; !smSupported {
- e := fmt.Errorf("serialization method with style=%q and explode=%v is not supported by a header parameter", sm.Style, sm.Explode)
- return fmt.Errorf("header schema is invalid: %w", e)
- }
-
- if (header.Schema == nil) == (header.Content == nil) {
- e := fmt.Errorf("parameter must contain exactly one of content and schema: %v", header)
- return fmt.Errorf("header schema is invalid: %w", e)
- }
- if schema := header.Schema; schema != nil {
- if err := schema.Validate(ctx); err != nil {
- return fmt.Errorf("header schema is invalid: %w", err)
- }
- }
-
- if content := header.Content; content != nil {
- if err := content.Validate(ctx); err != nil {
- return fmt.Errorf("header content is invalid: %w", err)
- }
- }
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/info.go b/vendor/github.com/getkin/kin-openapi/openapi3/info.go
deleted file mode 100644
index 381047fc..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/info.go
+++ /dev/null
@@ -1,185 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
-)
-
-// Info is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#info-object
-type Info struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Title string `json:"title" yaml:"title"` // Required
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- TermsOfService string `json:"termsOfService,omitempty" yaml:"termsOfService,omitempty"`
- Contact *Contact `json:"contact,omitempty" yaml:"contact,omitempty"`
- License *License `json:"license,omitempty" yaml:"license,omitempty"`
- Version string `json:"version" yaml:"version"` // Required
-}
-
-// MarshalJSON returns the JSON encoding of Info.
-func (info Info) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 6+len(info.Extensions))
- for k, v := range info.Extensions {
- m[k] = v
- }
- m["title"] = info.Title
- if x := info.Description; x != "" {
- m["description"] = x
- }
- if x := info.TermsOfService; x != "" {
- m["termsOfService"] = x
- }
- if x := info.Contact; x != nil {
- m["contact"] = x
- }
- if x := info.License; x != nil {
- m["license"] = x
- }
- m["version"] = info.Version
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Info to a copy of data.
-func (info *Info) UnmarshalJSON(data []byte) error {
- type InfoBis Info
- var x InfoBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "title")
- delete(x.Extensions, "description")
- delete(x.Extensions, "termsOfService")
- delete(x.Extensions, "contact")
- delete(x.Extensions, "license")
- delete(x.Extensions, "version")
- *info = Info(x)
- return nil
-}
-
-// Validate returns an error if Info does not comply with the OpenAPI spec.
-func (info *Info) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if contact := info.Contact; contact != nil {
- if err := contact.Validate(ctx); err != nil {
- return err
- }
- }
-
- if license := info.License; license != nil {
- if err := license.Validate(ctx); err != nil {
- return err
- }
- }
-
- if info.Version == "" {
- return errors.New("value of version must be a non-empty string")
- }
-
- if info.Title == "" {
- return errors.New("value of title must be a non-empty string")
- }
-
- return validateExtensions(ctx, info.Extensions)
-}
-
-// Contact is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#contact-object
-type Contact struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Name string `json:"name,omitempty" yaml:"name,omitempty"`
- URL string `json:"url,omitempty" yaml:"url,omitempty"`
- Email string `json:"email,omitempty" yaml:"email,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of Contact.
-func (contact Contact) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 3+len(contact.Extensions))
- for k, v := range contact.Extensions {
- m[k] = v
- }
- if x := contact.Name; x != "" {
- m["name"] = x
- }
- if x := contact.URL; x != "" {
- m["url"] = x
- }
- if x := contact.Email; x != "" {
- m["email"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Contact to a copy of data.
-func (contact *Contact) UnmarshalJSON(data []byte) error {
- type ContactBis Contact
- var x ContactBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "name")
- delete(x.Extensions, "url")
- delete(x.Extensions, "email")
- *contact = Contact(x)
- return nil
-}
-
-// Validate returns an error if Contact does not comply with the OpenAPI spec.
-func (contact *Contact) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- return validateExtensions(ctx, contact.Extensions)
-}
-
-// License is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#license-object
-type License struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Name string `json:"name" yaml:"name"` // Required
- URL string `json:"url,omitempty" yaml:"url,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of License.
-func (license License) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 2+len(license.Extensions))
- for k, v := range license.Extensions {
- m[k] = v
- }
- m["name"] = license.Name
- if x := license.URL; x != "" {
- m["url"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets License to a copy of data.
-func (license *License) UnmarshalJSON(data []byte) error {
- type LicenseBis License
- var x LicenseBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "name")
- delete(x.Extensions, "url")
- *license = License(x)
- return nil
-}
-
-// Validate returns an error if License does not comply with the OpenAPI spec.
-func (license *License) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if license.Name == "" {
- return errors.New("value of license name must be a non-empty string")
- }
-
- return validateExtensions(ctx, license.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/internalize_refs.go b/vendor/github.com/getkin/kin-openapi/openapi3/internalize_refs.go
deleted file mode 100644
index b8506535..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/internalize_refs.go
+++ /dev/null
@@ -1,434 +0,0 @@
-package openapi3
-
-import (
- "context"
- "path/filepath"
- "strings"
-)
-
-type RefNameResolver func(string) string
-
-// DefaultRefResolver is a default implementation of refNameResolver for the
-// InternalizeRefs function.
-//
-// If a reference points to an element inside a document, it returns the last
-// element in the reference using filepath.Base. Otherwise if the reference points
-// to a file, it returns the file name trimmed of all extensions.
-func DefaultRefNameResolver(ref string) string {
- if ref == "" {
- return ""
- }
- split := strings.SplitN(ref, "#", 2)
- if len(split) == 2 {
- return filepath.Base(split[1])
- }
- ref = split[0]
- for ext := filepath.Ext(ref); len(ext) > 0; ext = filepath.Ext(ref) {
- ref = strings.TrimSuffix(ref, ext)
- }
- return filepath.Base(ref)
-}
-
-func schemaNames(s Schemas) []string {
- out := make([]string, 0, len(s))
- for i := range s {
- out = append(out, i)
- }
- return out
-}
-
-func parametersMapNames(s ParametersMap) []string {
- out := make([]string, 0, len(s))
- for i := range s {
- out = append(out, i)
- }
- return out
-}
-
-func isExternalRef(ref string, parentIsExternal bool) bool {
- return ref != "" && (!strings.HasPrefix(ref, "#/components/") || parentIsExternal)
-}
-
-func (doc *T) addSchemaToSpec(s *SchemaRef, refNameResolver RefNameResolver, parentIsExternal bool) bool {
- if s == nil || !isExternalRef(s.Ref, parentIsExternal) {
- return false
- }
-
- name := refNameResolver(s.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.Schemas[name]; ok {
- s.Ref = "#/components/schemas/" + name
- return true
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Schemas == nil {
- doc.Components.Schemas = make(Schemas)
- }
- doc.Components.Schemas[name] = s.Value.NewRef()
- s.Ref = "#/components/schemas/" + name
- return true
-}
-
-func (doc *T) addParameterToSpec(p *ParameterRef, refNameResolver RefNameResolver, parentIsExternal bool) bool {
- if p == nil || !isExternalRef(p.Ref, parentIsExternal) {
- return false
- }
- name := refNameResolver(p.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.Parameters[name]; ok {
- p.Ref = "#/components/parameters/" + name
- return true
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Parameters == nil {
- doc.Components.Parameters = make(ParametersMap)
- }
- doc.Components.Parameters[name] = &ParameterRef{Value: p.Value}
- p.Ref = "#/components/parameters/" + name
- return true
-}
-
-func (doc *T) addHeaderToSpec(h *HeaderRef, refNameResolver RefNameResolver, parentIsExternal bool) bool {
- if h == nil || !isExternalRef(h.Ref, parentIsExternal) {
- return false
- }
- name := refNameResolver(h.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.Headers[name]; ok {
- h.Ref = "#/components/headers/" + name
- return true
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Headers == nil {
- doc.Components.Headers = make(Headers)
- }
- doc.Components.Headers[name] = &HeaderRef{Value: h.Value}
- h.Ref = "#/components/headers/" + name
- return true
-}
-
-func (doc *T) addRequestBodyToSpec(r *RequestBodyRef, refNameResolver RefNameResolver, parentIsExternal bool) bool {
- if r == nil || !isExternalRef(r.Ref, parentIsExternal) {
- return false
- }
- name := refNameResolver(r.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.RequestBodies[name]; ok {
- r.Ref = "#/components/requestBodies/" + name
- return true
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.RequestBodies == nil {
- doc.Components.RequestBodies = make(RequestBodies)
- }
- doc.Components.RequestBodies[name] = &RequestBodyRef{Value: r.Value}
- r.Ref = "#/components/requestBodies/" + name
- return true
-}
-
-func (doc *T) addResponseToSpec(r *ResponseRef, refNameResolver RefNameResolver, parentIsExternal bool) bool {
- if r == nil || !isExternalRef(r.Ref, parentIsExternal) {
- return false
- }
- name := refNameResolver(r.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.Responses[name]; ok {
- r.Ref = "#/components/responses/" + name
- return true
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Responses == nil {
- doc.Components.Responses = make(Responses)
- }
- doc.Components.Responses[name] = &ResponseRef{Value: r.Value}
- r.Ref = "#/components/responses/" + name
- return true
-}
-
-func (doc *T) addSecuritySchemeToSpec(ss *SecuritySchemeRef, refNameResolver RefNameResolver, parentIsExternal bool) {
- if ss == nil || !isExternalRef(ss.Ref, parentIsExternal) {
- return
- }
- name := refNameResolver(ss.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.SecuritySchemes[name]; ok {
- ss.Ref = "#/components/securitySchemes/" + name
- return
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.SecuritySchemes == nil {
- doc.Components.SecuritySchemes = make(SecuritySchemes)
- }
- doc.Components.SecuritySchemes[name] = &SecuritySchemeRef{Value: ss.Value}
- ss.Ref = "#/components/securitySchemes/" + name
-
-}
-
-func (doc *T) addExampleToSpec(e *ExampleRef, refNameResolver RefNameResolver, parentIsExternal bool) {
- if e == nil || !isExternalRef(e.Ref, parentIsExternal) {
- return
- }
- name := refNameResolver(e.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.Examples[name]; ok {
- e.Ref = "#/components/examples/" + name
- return
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Examples == nil {
- doc.Components.Examples = make(Examples)
- }
- doc.Components.Examples[name] = &ExampleRef{Value: e.Value}
- e.Ref = "#/components/examples/" + name
-
-}
-
-func (doc *T) addLinkToSpec(l *LinkRef, refNameResolver RefNameResolver, parentIsExternal bool) {
- if l == nil || !isExternalRef(l.Ref, parentIsExternal) {
- return
- }
- name := refNameResolver(l.Ref)
- if doc.Components != nil {
- if _, ok := doc.Components.Links[name]; ok {
- l.Ref = "#/components/links/" + name
- return
- }
- }
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Links == nil {
- doc.Components.Links = make(Links)
- }
- doc.Components.Links[name] = &LinkRef{Value: l.Value}
- l.Ref = "#/components/links/" + name
-
-}
-
-func (doc *T) addCallbackToSpec(c *CallbackRef, refNameResolver RefNameResolver, parentIsExternal bool) bool {
- if c == nil || !isExternalRef(c.Ref, parentIsExternal) {
- return false
- }
- name := refNameResolver(c.Ref)
-
- if doc.Components == nil {
- doc.Components = &Components{}
- }
- if doc.Components.Callbacks == nil {
- doc.Components.Callbacks = make(Callbacks)
- }
- c.Ref = "#/components/callbacks/" + name
- doc.Components.Callbacks[name] = &CallbackRef{Value: c.Value}
- return true
-}
-
-func (doc *T) derefSchema(s *Schema, refNameResolver RefNameResolver, parentIsExternal bool) {
- if s == nil || doc.isVisitedSchema(s) {
- return
- }
-
- for _, list := range []SchemaRefs{s.AllOf, s.AnyOf, s.OneOf} {
- for _, s2 := range list {
- isExternal := doc.addSchemaToSpec(s2, refNameResolver, parentIsExternal)
- if s2 != nil {
- doc.derefSchema(s2.Value, refNameResolver, isExternal || parentIsExternal)
- }
- }
- }
- for _, s2 := range s.Properties {
- isExternal := doc.addSchemaToSpec(s2, refNameResolver, parentIsExternal)
- if s2 != nil {
- doc.derefSchema(s2.Value, refNameResolver, isExternal || parentIsExternal)
- }
- }
- for _, ref := range []*SchemaRef{s.Not, s.AdditionalProperties.Schema, s.Items} {
- isExternal := doc.addSchemaToSpec(ref, refNameResolver, parentIsExternal)
- if ref != nil {
- doc.derefSchema(ref.Value, refNameResolver, isExternal || parentIsExternal)
- }
- }
-}
-
-func (doc *T) derefHeaders(hs Headers, refNameResolver RefNameResolver, parentIsExternal bool) {
- for _, h := range hs {
- isExternal := doc.addHeaderToSpec(h, refNameResolver, parentIsExternal)
- if doc.isVisitedHeader(h.Value) {
- continue
- }
- doc.derefParameter(h.Value.Parameter, refNameResolver, parentIsExternal || isExternal)
- }
-}
-
-func (doc *T) derefExamples(es Examples, refNameResolver RefNameResolver, parentIsExternal bool) {
- for _, e := range es {
- doc.addExampleToSpec(e, refNameResolver, parentIsExternal)
- }
-}
-
-func (doc *T) derefContent(c Content, refNameResolver RefNameResolver, parentIsExternal bool) {
- for _, mediatype := range c {
- isExternal := doc.addSchemaToSpec(mediatype.Schema, refNameResolver, parentIsExternal)
- if mediatype.Schema != nil {
- doc.derefSchema(mediatype.Schema.Value, refNameResolver, isExternal || parentIsExternal)
- }
- doc.derefExamples(mediatype.Examples, refNameResolver, parentIsExternal)
- for _, e := range mediatype.Encoding {
- doc.derefHeaders(e.Headers, refNameResolver, parentIsExternal)
- }
- }
-}
-
-func (doc *T) derefLinks(ls Links, refNameResolver RefNameResolver, parentIsExternal bool) {
- for _, l := range ls {
- doc.addLinkToSpec(l, refNameResolver, parentIsExternal)
- }
-}
-
-func (doc *T) derefResponses(es Responses, refNameResolver RefNameResolver, parentIsExternal bool) {
- for _, e := range es {
- isExternal := doc.addResponseToSpec(e, refNameResolver, parentIsExternal)
- if e.Value != nil {
- doc.derefHeaders(e.Value.Headers, refNameResolver, isExternal || parentIsExternal)
- doc.derefContent(e.Value.Content, refNameResolver, isExternal || parentIsExternal)
- doc.derefLinks(e.Value.Links, refNameResolver, isExternal || parentIsExternal)
- }
- }
-}
-
-func (doc *T) derefParameter(p Parameter, refNameResolver RefNameResolver, parentIsExternal bool) {
- isExternal := doc.addSchemaToSpec(p.Schema, refNameResolver, parentIsExternal)
- doc.derefContent(p.Content, refNameResolver, parentIsExternal)
- if p.Schema != nil {
- doc.derefSchema(p.Schema.Value, refNameResolver, isExternal || parentIsExternal)
- }
-}
-
-func (doc *T) derefRequestBody(r RequestBody, refNameResolver RefNameResolver, parentIsExternal bool) {
- doc.derefContent(r.Content, refNameResolver, parentIsExternal)
-}
-
-func (doc *T) derefPaths(paths map[string]*PathItem, refNameResolver RefNameResolver, parentIsExternal bool) {
- for _, ops := range paths {
- if isExternalRef(ops.Ref, parentIsExternal) {
- parentIsExternal = true
- }
- // inline full operations
- ops.Ref = ""
-
- for _, param := range ops.Parameters {
- doc.addParameterToSpec(param, refNameResolver, parentIsExternal)
- }
-
- for _, op := range ops.Operations() {
- isExternal := doc.addRequestBodyToSpec(op.RequestBody, refNameResolver, parentIsExternal)
- if op.RequestBody != nil && op.RequestBody.Value != nil {
- doc.derefRequestBody(*op.RequestBody.Value, refNameResolver, parentIsExternal || isExternal)
- }
- for _, cb := range op.Callbacks {
- isExternal := doc.addCallbackToSpec(cb, refNameResolver, parentIsExternal)
- if cb.Value != nil {
- doc.derefPaths(*cb.Value, refNameResolver, parentIsExternal || isExternal)
- }
- }
- doc.derefResponses(op.Responses, refNameResolver, parentIsExternal)
- for _, param := range op.Parameters {
- isExternal := doc.addParameterToSpec(param, refNameResolver, parentIsExternal)
- if param.Value != nil {
- doc.derefParameter(*param.Value, refNameResolver, parentIsExternal || isExternal)
- }
- }
- }
- }
-}
-
-// InternalizeRefs removes all references to external files from the spec and moves them
-// to the components section.
-//
-// refNameResolver takes in references to returns a name to store the reference under locally.
-// It MUST return a unique name for each reference type.
-// A default implementation is provided that will suffice for most use cases. See the function
-// documention for more details.
-//
-// Example:
-//
-// doc.InternalizeRefs(context.Background(), nil)
-func (doc *T) InternalizeRefs(ctx context.Context, refNameResolver func(ref string) string) {
- doc.resetVisited()
-
- if refNameResolver == nil {
- refNameResolver = DefaultRefNameResolver
- }
-
- if components := doc.Components; components != nil {
- names := schemaNames(components.Schemas)
- for _, name := range names {
- schema := components.Schemas[name]
- isExternal := doc.addSchemaToSpec(schema, refNameResolver, false)
- if schema != nil {
- schema.Ref = "" // always dereference the top level
- doc.derefSchema(schema.Value, refNameResolver, isExternal)
- }
- }
- names = parametersMapNames(components.Parameters)
- for _, name := range names {
- p := components.Parameters[name]
- isExternal := doc.addParameterToSpec(p, refNameResolver, false)
- if p != nil && p.Value != nil {
- p.Ref = "" // always dereference the top level
- doc.derefParameter(*p.Value, refNameResolver, isExternal)
- }
- }
- doc.derefHeaders(components.Headers, refNameResolver, false)
- for _, req := range components.RequestBodies {
- isExternal := doc.addRequestBodyToSpec(req, refNameResolver, false)
- if req != nil && req.Value != nil {
- req.Ref = "" // always dereference the top level
- doc.derefRequestBody(*req.Value, refNameResolver, isExternal)
- }
- }
- doc.derefResponses(components.Responses, refNameResolver, false)
- for _, ss := range components.SecuritySchemes {
- doc.addSecuritySchemeToSpec(ss, refNameResolver, false)
- }
- doc.derefExamples(components.Examples, refNameResolver, false)
- doc.derefLinks(components.Links, refNameResolver, false)
- for _, cb := range components.Callbacks {
- isExternal := doc.addCallbackToSpec(cb, refNameResolver, false)
- if cb != nil && cb.Value != nil {
- cb.Ref = "" // always dereference the top level
- doc.derefPaths(*cb.Value, refNameResolver, isExternal)
- }
- }
- }
-
- doc.derefPaths(doc.Paths, refNameResolver, false)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/link.go b/vendor/github.com/getkin/kin-openapi/openapi3/link.go
deleted file mode 100644
index 08dfa8d6..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/link.go
+++ /dev/null
@@ -1,101 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type Links map[string]*LinkRef
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (links Links) JSONLookup(token string) (interface{}, error) {
- ref, ok := links[token]
- if ok == false {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref != nil && ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-var _ jsonpointer.JSONPointable = (*Links)(nil)
-
-// Link is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#link-object
-type Link struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- OperationRef string `json:"operationRef,omitempty" yaml:"operationRef,omitempty"`
- OperationID string `json:"operationId,omitempty" yaml:"operationId,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Parameters map[string]interface{} `json:"parameters,omitempty" yaml:"parameters,omitempty"`
- Server *Server `json:"server,omitempty" yaml:"server,omitempty"`
- RequestBody interface{} `json:"requestBody,omitempty" yaml:"requestBody,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of Link.
-func (link Link) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 6+len(link.Extensions))
- for k, v := range link.Extensions {
- m[k] = v
- }
-
- if x := link.OperationRef; x != "" {
- m["operationRef"] = x
- }
- if x := link.OperationID; x != "" {
- m["operationId"] = x
- }
- if x := link.Description; x != "" {
- m["description"] = x
- }
- if x := link.Parameters; len(x) != 0 {
- m["parameters"] = x
- }
- if x := link.Server; x != nil {
- m["server"] = x
- }
- if x := link.RequestBody; x != nil {
- m["requestBody"] = x
- }
-
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Link to a copy of data.
-func (link *Link) UnmarshalJSON(data []byte) error {
- type LinkBis Link
- var x LinkBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "operationRef")
- delete(x.Extensions, "operationId")
- delete(x.Extensions, "description")
- delete(x.Extensions, "parameters")
- delete(x.Extensions, "server")
- delete(x.Extensions, "requestBody")
- *link = Link(x)
- return nil
-}
-
-// Validate returns an error if Link does not comply with the OpenAPI spec.
-func (link *Link) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if link.OperationID == "" && link.OperationRef == "" {
- return errors.New("missing operationId or operationRef on link")
- }
- if link.OperationID != "" && link.OperationRef != "" {
- return fmt.Errorf("operationId %q and operationRef %q are mutually exclusive", link.OperationID, link.OperationRef)
- }
-
- return validateExtensions(ctx, link.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/loader.go b/vendor/github.com/getkin/kin-openapi/openapi3/loader.go
deleted file mode 100644
index 51020111..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/loader.go
+++ /dev/null
@@ -1,1051 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "net/url"
- "path"
- "path/filepath"
- "reflect"
- "sort"
- "strconv"
- "strings"
-
- "github.com/invopop/yaml"
-)
-
-var CircularReferenceError = "kin-openapi bug found: circular schema reference not handled"
-var CircularReferenceCounter = 3
-
-func foundUnresolvedRef(ref string) error {
- return fmt.Errorf("found unresolved ref: %q", ref)
-}
-
-func failedToResolveRefFragmentPart(value, what string) error {
- return fmt.Errorf("failed to resolve %q in fragment in URI: %q", what, value)
-}
-
-// Loader helps deserialize an OpenAPIv3 document
-type Loader struct {
- // IsExternalRefsAllowed enables visiting other files
- IsExternalRefsAllowed bool
-
- // ReadFromURIFunc allows overriding the any file/URL reading func
- ReadFromURIFunc ReadFromURIFunc
-
- Context context.Context
-
- rootDir string
- rootLocation string
-
- visitedPathItemRefs map[string]struct{}
-
- visitedDocuments map[string]*T
-
- visitedCallback map[*Callback]struct{}
- visitedExample map[*Example]struct{}
- visitedHeader map[*Header]struct{}
- visitedLink map[*Link]struct{}
- visitedParameter map[*Parameter]struct{}
- visitedRequestBody map[*RequestBody]struct{}
- visitedResponse map[*Response]struct{}
- visitedSchema map[*Schema]struct{}
- visitedSecurityScheme map[*SecurityScheme]struct{}
-}
-
-// NewLoader returns an empty Loader
-func NewLoader() *Loader {
- return &Loader{
- Context: context.Background(),
- }
-}
-
-func (loader *Loader) resetVisitedPathItemRefs() {
- loader.visitedPathItemRefs = make(map[string]struct{})
-}
-
-// LoadFromURI loads a spec from a remote URL
-func (loader *Loader) LoadFromURI(location *url.URL) (*T, error) {
- loader.resetVisitedPathItemRefs()
- return loader.loadFromURIInternal(location)
-}
-
-// LoadFromFile loads a spec from a local file path
-func (loader *Loader) LoadFromFile(location string) (*T, error) {
- loader.rootDir = path.Dir(location)
- return loader.LoadFromURI(&url.URL{Path: filepath.ToSlash(location)})
-}
-
-func (loader *Loader) loadFromURIInternal(location *url.URL) (*T, error) {
- data, err := loader.readURL(location)
- if err != nil {
- return nil, err
- }
- return loader.loadFromDataWithPathInternal(data, location)
-}
-
-func (loader *Loader) allowsExternalRefs(ref string) (err error) {
- if !loader.IsExternalRefsAllowed {
- err = fmt.Errorf("encountered disallowed external reference: %q", ref)
- }
- return
-}
-
-// loadSingleElementFromURI reads the data from ref and unmarshals to the passed element.
-func (loader *Loader) loadSingleElementFromURI(ref string, rootPath *url.URL, element interface{}) (*url.URL, error) {
- if err := loader.allowsExternalRefs(ref); err != nil {
- return nil, err
- }
-
- parsedURL, err := url.Parse(ref)
- if err != nil {
- return nil, err
- }
- if fragment := parsedURL.Fragment; fragment != "" {
- return nil, fmt.Errorf("unexpected ref fragment %q", fragment)
- }
-
- resolvedPath, err := resolvePath(rootPath, parsedURL)
- if err != nil {
- return nil, fmt.Errorf("could not resolve path: %w", err)
- }
-
- data, err := loader.readURL(resolvedPath)
- if err != nil {
- return nil, err
- }
- if err := unmarshal(data, element); err != nil {
- return nil, err
- }
-
- return resolvedPath, nil
-}
-
-func (loader *Loader) readURL(location *url.URL) ([]byte, error) {
- if f := loader.ReadFromURIFunc; f != nil {
- return f(loader, location)
- }
- return DefaultReadFromURI(loader, location)
-}
-
-// LoadFromData loads a spec from a byte array
-func (loader *Loader) LoadFromData(data []byte) (*T, error) {
- loader.resetVisitedPathItemRefs()
- doc := &T{}
- if err := unmarshal(data, doc); err != nil {
- return nil, err
- }
- if err := loader.ResolveRefsIn(doc, nil); err != nil {
- return nil, err
- }
- return doc, nil
-}
-
-// LoadFromDataWithPath takes the OpenAPI document data in bytes and a path where the resolver can find referred
-// elements and returns a *T with all resolved data or an error if unable to load data or resolve refs.
-func (loader *Loader) LoadFromDataWithPath(data []byte, location *url.URL) (*T, error) {
- loader.resetVisitedPathItemRefs()
- return loader.loadFromDataWithPathInternal(data, location)
-}
-
-func (loader *Loader) loadFromDataWithPathInternal(data []byte, location *url.URL) (*T, error) {
- if loader.visitedDocuments == nil {
- loader.visitedDocuments = make(map[string]*T)
- loader.rootLocation = location.Path
- }
- uri := location.String()
- if doc, ok := loader.visitedDocuments[uri]; ok {
- return doc, nil
- }
-
- doc := &T{}
- loader.visitedDocuments[uri] = doc
-
- if err := unmarshal(data, doc); err != nil {
- return nil, err
- }
- if err := loader.ResolveRefsIn(doc, location); err != nil {
- return nil, err
- }
-
- return doc, nil
-}
-
-func unmarshal(data []byte, v interface{}) error {
- // See https://github.com/getkin/kin-openapi/issues/680
- if err := json.Unmarshal(data, v); err != nil {
- // UnmarshalStrict(data, v) TODO: investigate how ymlv3 handles duplicate map keys
- return yaml.Unmarshal(data, v)
- }
- return nil
-}
-
-// ResolveRefsIn expands references if for instance spec was just unmarshalled
-func (loader *Loader) ResolveRefsIn(doc *T, location *url.URL) (err error) {
- if loader.Context == nil {
- loader.Context = context.Background()
- }
-
- if loader.visitedPathItemRefs == nil {
- loader.resetVisitedPathItemRefs()
- }
-
- if components := doc.Components; components != nil {
- for _, component := range components.Headers {
- if err = loader.resolveHeaderRef(doc, component, location); err != nil {
- return
- }
- }
- for _, component := range components.Parameters {
- if err = loader.resolveParameterRef(doc, component, location); err != nil {
- return
- }
- }
- for _, component := range components.RequestBodies {
- if err = loader.resolveRequestBodyRef(doc, component, location); err != nil {
- return
- }
- }
- for _, component := range components.Responses {
- if err = loader.resolveResponseRef(doc, component, location); err != nil {
- return
- }
- }
- for _, component := range components.Schemas {
- if err = loader.resolveSchemaRef(doc, component, location, []string{}); err != nil {
- return
- }
- }
- for _, component := range components.SecuritySchemes {
- if err = loader.resolveSecuritySchemeRef(doc, component, location); err != nil {
- return
- }
- }
-
- examples := make([]string, 0, len(components.Examples))
- for name := range components.Examples {
- examples = append(examples, name)
- }
- sort.Strings(examples)
- for _, name := range examples {
- component := components.Examples[name]
- if err = loader.resolveExampleRef(doc, component, location); err != nil {
- return
- }
- }
-
- for _, component := range components.Callbacks {
- if err = loader.resolveCallbackRef(doc, component, location); err != nil {
- return
- }
- }
- }
-
- // Visit all operations
- for _, pathItem := range doc.Paths {
- if pathItem == nil {
- continue
- }
- if err = loader.resolvePathItemRef(doc, pathItem, location); err != nil {
- return
- }
- }
-
- return
-}
-
-func join(basePath *url.URL, relativePath *url.URL) (*url.URL, error) {
- if basePath == nil {
- return relativePath, nil
- }
- newPath, err := url.Parse(basePath.String())
- if err != nil {
- return nil, fmt.Errorf("cannot copy path: %q", basePath.String())
- }
- newPath.Path = path.Join(path.Dir(newPath.Path), relativePath.Path)
- return newPath, nil
-}
-
-func resolvePath(basePath *url.URL, componentPath *url.URL) (*url.URL, error) {
- if componentPath.Scheme == "" && componentPath.Host == "" {
- // support absolute paths
- if componentPath.Path[0] == '/' {
- return componentPath, nil
- }
- return join(basePath, componentPath)
- }
- return componentPath, nil
-}
-
-func isSingleRefElement(ref string) bool {
- return !strings.Contains(ref, "#")
-}
-
-func (loader *Loader) resolveComponent(doc *T, ref string, path *url.URL, resolved interface{}) (
- componentDoc *T,
- componentPath *url.URL,
- err error,
-) {
- if componentDoc, ref, componentPath, err = loader.resolveRef(doc, ref, path); err != nil {
- return nil, nil, err
- }
-
- parsedURL, err := url.Parse(ref)
- if err != nil {
- return nil, nil, fmt.Errorf("cannot parse reference: %q: %v", ref, parsedURL)
- }
- fragment := parsedURL.Fragment
- if !strings.HasPrefix(fragment, "/") {
- return nil, nil, fmt.Errorf("expected fragment prefix '#/' in URI %q", ref)
- }
-
- drill := func(cursor interface{}) (interface{}, error) {
- for _, pathPart := range strings.Split(fragment[1:], "/") {
- pathPart = unescapeRefString(pathPart)
-
- if cursor, err = drillIntoField(cursor, pathPart); err != nil {
- e := failedToResolveRefFragmentPart(ref, pathPart)
- return nil, fmt.Errorf("%s: %w", e, err)
- }
- if cursor == nil {
- return nil, failedToResolveRefFragmentPart(ref, pathPart)
- }
- }
- return cursor, nil
- }
- var cursor interface{}
- if cursor, err = drill(componentDoc); err != nil {
- if path == nil {
- return nil, nil, err
- }
- var err2 error
- data, err2 := loader.readURL(path)
- if err2 != nil {
- return nil, nil, err
- }
- if err2 = unmarshal(data, &cursor); err2 != nil {
- return nil, nil, err
- }
- if cursor, err2 = drill(cursor); err2 != nil || cursor == nil {
- return nil, nil, err
- }
- err = nil
- }
-
- switch {
- case reflect.TypeOf(cursor) == reflect.TypeOf(resolved):
- reflect.ValueOf(resolved).Elem().Set(reflect.ValueOf(cursor).Elem())
- return componentDoc, componentPath, nil
-
- case reflect.TypeOf(cursor) == reflect.TypeOf(map[string]interface{}{}):
- codec := func(got, expect interface{}) error {
- enc, err := json.Marshal(got)
- if err != nil {
- return err
- }
- if err = json.Unmarshal(enc, expect); err != nil {
- return err
- }
- return nil
- }
- if err := codec(cursor, resolved); err != nil {
- return nil, nil, fmt.Errorf("bad data in %q (expecting %s)", ref, readableType(resolved))
- }
- return componentDoc, componentPath, nil
-
- default:
- return nil, nil, fmt.Errorf("bad data in %q (expecting %s)", ref, readableType(resolved))
- }
-}
-
-func readableType(x interface{}) string {
- switch x.(type) {
- case *Callback:
- return "callback object"
- case *CallbackRef:
- return "ref to callback object"
- case *ExampleRef:
- return "ref to example object"
- case *HeaderRef:
- return "ref to header object"
- case *LinkRef:
- return "ref to link object"
- case *ParameterRef:
- return "ref to parameter object"
- case *PathItem:
- return "pathItem object"
- case *RequestBodyRef:
- return "ref to requestBody object"
- case *ResponseRef:
- return "ref to response object"
- case *SchemaRef:
- return "ref to schema object"
- case *SecuritySchemeRef:
- return "ref to securityScheme object"
- default:
- panic(fmt.Sprintf("unreachable %T", x))
- }
-}
-
-func drillIntoField(cursor interface{}, fieldName string) (interface{}, error) {
- // Special case due to multijson
- if s, ok := cursor.(*SchemaRef); ok && fieldName == "additionalProperties" {
- if ap := s.Value.AdditionalProperties.Has; ap != nil {
- return *ap, nil
- }
- return s.Value.AdditionalProperties.Schema, nil
- }
-
- switch val := reflect.Indirect(reflect.ValueOf(cursor)); val.Kind() {
- case reflect.Map:
- elementValue := val.MapIndex(reflect.ValueOf(fieldName))
- if !elementValue.IsValid() {
- return nil, fmt.Errorf("map key %q not found", fieldName)
- }
- return elementValue.Interface(), nil
-
- case reflect.Slice:
- i, err := strconv.ParseUint(fieldName, 10, 32)
- if err != nil {
- return nil, err
- }
- index := int(i)
- if 0 > index || index >= val.Len() {
- return nil, errors.New("slice index out of bounds")
- }
- return val.Index(index).Interface(), nil
-
- case reflect.Struct:
- hasFields := false
- for i := 0; i < val.NumField(); i++ {
- hasFields = true
- if fieldName == strings.Split(val.Type().Field(i).Tag.Get("yaml"), ",")[0] {
- return val.Field(i).Interface(), nil
- }
- }
- // if cursor is a "ref wrapper" struct (e.g. RequestBodyRef),
- if _, ok := val.Type().FieldByName("Value"); ok {
- // try digging into its Value field
- return drillIntoField(val.FieldByName("Value").Interface(), fieldName)
- }
- if hasFields {
- if ff := val.Type().Field(0); ff.PkgPath == "" && ff.Name == "Extensions" {
- extensions := val.Field(0).Interface().(map[string]interface{})
- if enc, ok := extensions[fieldName]; ok {
- return enc, nil
- }
- }
- }
- return nil, fmt.Errorf("struct field %q not found", fieldName)
-
- default:
- return nil, errors.New("not a map, slice nor struct")
- }
-}
-
-func (loader *Loader) resolveRef(doc *T, ref string, path *url.URL) (*T, string, *url.URL, error) {
- if ref != "" && ref[0] == '#' {
- return doc, ref, path, nil
- }
-
- if err := loader.allowsExternalRefs(ref); err != nil {
- return nil, "", nil, err
- }
-
- parsedURL, err := url.Parse(ref)
- if err != nil {
- return nil, "", nil, fmt.Errorf("cannot parse reference: %q: %v", ref, parsedURL)
- }
- fragment := parsedURL.Fragment
- parsedURL.Fragment = ""
-
- var resolvedPath *url.URL
- if resolvedPath, err = resolvePath(path, parsedURL); err != nil {
- return nil, "", nil, fmt.Errorf("error resolving path: %w", err)
- }
-
- if doc, err = loader.loadFromURIInternal(resolvedPath); err != nil {
- return nil, "", nil, fmt.Errorf("error resolving reference %q: %w", ref, err)
- }
-
- return doc, "#" + fragment, resolvedPath, nil
-}
-
-func (loader *Loader) resolveHeaderRef(doc *T, component *HeaderRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedHeader == nil {
- loader.visitedHeader = make(map[*Header]struct{})
- }
- if _, ok := loader.visitedHeader[component.Value]; ok {
- return nil
- }
- loader.visitedHeader[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid header: value MUST be an object")
- }
- if ref := component.Ref; ref != "" {
- if isSingleRefElement(ref) {
- var header Header
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, &header); err != nil {
- return err
- }
- component.Value = &header
- } else {
- var resolved HeaderRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveHeaderRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- value := component.Value
- if value == nil {
- return nil
- }
-
- if schema := value.Schema; schema != nil {
- if err := loader.resolveSchemaRef(doc, schema, documentPath, []string{}); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveParameterRef(doc *T, component *ParameterRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedParameter == nil {
- loader.visitedParameter = make(map[*Parameter]struct{})
- }
- if _, ok := loader.visitedParameter[component.Value]; ok {
- return nil
- }
- loader.visitedParameter[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid parameter: value MUST be an object")
- }
- ref := component.Ref
- if ref != "" {
- if isSingleRefElement(ref) {
- var param Parameter
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, ¶m); err != nil {
- return err
- }
- component.Value = ¶m
- } else {
- var resolved ParameterRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveParameterRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- value := component.Value
- if value == nil {
- return nil
- }
-
- if value.Content != nil && value.Schema != nil {
- return errors.New("cannot contain both schema and content in a parameter")
- }
- for _, contentType := range value.Content {
- if schema := contentType.Schema; schema != nil {
- if err := loader.resolveSchemaRef(doc, schema, documentPath, []string{}); err != nil {
- return err
- }
- }
- }
- if schema := value.Schema; schema != nil {
- if err := loader.resolveSchemaRef(doc, schema, documentPath, []string{}); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveRequestBodyRef(doc *T, component *RequestBodyRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedRequestBody == nil {
- loader.visitedRequestBody = make(map[*RequestBody]struct{})
- }
- if _, ok := loader.visitedRequestBody[component.Value]; ok {
- return nil
- }
- loader.visitedRequestBody[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid requestBody: value MUST be an object")
- }
- if ref := component.Ref; ref != "" {
- if isSingleRefElement(ref) {
- var requestBody RequestBody
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, &requestBody); err != nil {
- return err
- }
- component.Value = &requestBody
- } else {
- var resolved RequestBodyRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err = loader.resolveRequestBodyRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- value := component.Value
- if value == nil {
- return nil
- }
-
- for _, contentType := range value.Content {
- examples := make([]string, 0, len(contentType.Examples))
- for name := range contentType.Examples {
- examples = append(examples, name)
- }
- sort.Strings(examples)
- for _, name := range examples {
- example := contentType.Examples[name]
- if err := loader.resolveExampleRef(doc, example, documentPath); err != nil {
- return err
- }
- contentType.Examples[name] = example
- }
- if schema := contentType.Schema; schema != nil {
- if err := loader.resolveSchemaRef(doc, schema, documentPath, []string{}); err != nil {
- return err
- }
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveResponseRef(doc *T, component *ResponseRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedResponse == nil {
- loader.visitedResponse = make(map[*Response]struct{})
- }
- if _, ok := loader.visitedResponse[component.Value]; ok {
- return nil
- }
- loader.visitedResponse[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid response: value MUST be an object")
- }
- ref := component.Ref
- if ref != "" {
- if isSingleRefElement(ref) {
- var resp Response
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, &resp); err != nil {
- return err
- }
- component.Value = &resp
- } else {
- var resolved ResponseRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveResponseRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- value := component.Value
- if value == nil {
- return nil
- }
-
- for _, header := range value.Headers {
- if err := loader.resolveHeaderRef(doc, header, documentPath); err != nil {
- return err
- }
- }
- for _, contentType := range value.Content {
- if contentType == nil {
- continue
- }
- examples := make([]string, 0, len(contentType.Examples))
- for name := range contentType.Examples {
- examples = append(examples, name)
- }
- sort.Strings(examples)
- for _, name := range examples {
- example := contentType.Examples[name]
- if err := loader.resolveExampleRef(doc, example, documentPath); err != nil {
- return err
- }
- contentType.Examples[name] = example
- }
- if schema := contentType.Schema; schema != nil {
- if err := loader.resolveSchemaRef(doc, schema, documentPath, []string{}); err != nil {
- return err
- }
- contentType.Schema = schema
- }
- }
- for _, link := range value.Links {
- if err := loader.resolveLinkRef(doc, link, documentPath); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveSchemaRef(doc *T, component *SchemaRef, documentPath *url.URL, visited []string) (err error) {
- if component == nil {
- return errors.New("invalid schema: value MUST be an object")
- }
-
- if component.Value != nil {
- if loader.visitedSchema == nil {
- loader.visitedSchema = make(map[*Schema]struct{})
- }
- if _, ok := loader.visitedSchema[component.Value]; ok {
- return nil
- }
- loader.visitedSchema[component.Value] = struct{}{}
- }
-
- ref := component.Ref
- if ref != "" {
- if isSingleRefElement(ref) {
- var schema Schema
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, &schema); err != nil {
- return err
- }
- component.Value = &schema
- } else {
- if visitedLimit(visited, ref) {
- visited = append(visited, ref)
- return fmt.Errorf("%s - %s", CircularReferenceError, strings.Join(visited, " -> "))
- }
- visited = append(visited, ref)
-
- var resolved SchemaRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveSchemaRef(doc, &resolved, componentPath, visited); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- if loader.visitedSchema == nil {
- loader.visitedSchema = make(map[*Schema]struct{})
- }
- loader.visitedSchema[component.Value] = struct{}{}
- }
- value := component.Value
- if value == nil {
- return nil
- }
-
- // ResolveRefs referred schemas
- if v := value.Items; v != nil {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- for _, v := range value.Properties {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- if v := value.AdditionalProperties.Schema; v != nil {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- if v := value.Not; v != nil {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- for _, v := range value.AllOf {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- for _, v := range value.AnyOf {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- for _, v := range value.OneOf {
- if err := loader.resolveSchemaRef(doc, v, documentPath, visited); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveSecuritySchemeRef(doc *T, component *SecuritySchemeRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedSecurityScheme == nil {
- loader.visitedSecurityScheme = make(map[*SecurityScheme]struct{})
- }
- if _, ok := loader.visitedSecurityScheme[component.Value]; ok {
- return nil
- }
- loader.visitedSecurityScheme[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid securityScheme: value MUST be an object")
- }
- if ref := component.Ref; ref != "" {
- if isSingleRefElement(ref) {
- var scheme SecurityScheme
- if _, err = loader.loadSingleElementFromURI(ref, documentPath, &scheme); err != nil {
- return err
- }
- component.Value = &scheme
- } else {
- var resolved SecuritySchemeRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveSecuritySchemeRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveExampleRef(doc *T, component *ExampleRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedExample == nil {
- loader.visitedExample = make(map[*Example]struct{})
- }
- if _, ok := loader.visitedExample[component.Value]; ok {
- return nil
- }
- loader.visitedExample[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid example: value MUST be an object")
- }
- if ref := component.Ref; ref != "" {
- if isSingleRefElement(ref) {
- var example Example
- if _, err = loader.loadSingleElementFromURI(ref, documentPath, &example); err != nil {
- return err
- }
- component.Value = &example
- } else {
- var resolved ExampleRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveExampleRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveCallbackRef(doc *T, component *CallbackRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedCallback == nil {
- loader.visitedCallback = make(map[*Callback]struct{})
- }
- if _, ok := loader.visitedCallback[component.Value]; ok {
- return nil
- }
- loader.visitedCallback[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid callback: value MUST be an object")
- }
- if ref := component.Ref; ref != "" {
- if isSingleRefElement(ref) {
- var resolved Callback
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, &resolved); err != nil {
- return err
- }
- component.Value = &resolved
- } else {
- var resolved CallbackRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err = loader.resolveCallbackRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- value := component.Value
- if value == nil {
- return nil
- }
-
- for _, pathItem := range *value {
- if err = loader.resolvePathItemRef(doc, pathItem, documentPath); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (loader *Loader) resolveLinkRef(doc *T, component *LinkRef, documentPath *url.URL) (err error) {
- if component != nil && component.Value != nil {
- if loader.visitedLink == nil {
- loader.visitedLink = make(map[*Link]struct{})
- }
- if _, ok := loader.visitedLink[component.Value]; ok {
- return nil
- }
- loader.visitedLink[component.Value] = struct{}{}
- }
-
- if component == nil {
- return errors.New("invalid link: value MUST be an object")
- }
- if ref := component.Ref; ref != "" {
- if isSingleRefElement(ref) {
- var link Link
- if _, err = loader.loadSingleElementFromURI(ref, documentPath, &link); err != nil {
- return err
- }
- component.Value = &link
- } else {
- var resolved LinkRef
- doc, componentPath, err := loader.resolveComponent(doc, ref, documentPath, &resolved)
- if err != nil {
- return err
- }
- if err := loader.resolveLinkRef(doc, &resolved, componentPath); err != nil {
- return err
- }
- component.Value = resolved.Value
- return nil
- }
- }
- return nil
-}
-
-func (loader *Loader) resolvePathItemRef(doc *T, pathItem *PathItem, documentPath *url.URL) (err error) {
- if pathItem == nil {
- return errors.New("invalid path item: value MUST be an object")
- }
- ref := pathItem.Ref
- if ref != "" {
- if pathItem.Summary != "" ||
- pathItem.Description != "" ||
- pathItem.Connect != nil ||
- pathItem.Delete != nil ||
- pathItem.Get != nil ||
- pathItem.Head != nil ||
- pathItem.Options != nil ||
- pathItem.Patch != nil ||
- pathItem.Post != nil ||
- pathItem.Put != nil ||
- pathItem.Trace != nil ||
- len(pathItem.Servers) != 0 ||
- len(pathItem.Parameters) != 0 {
- return nil
- }
- if isSingleRefElement(ref) {
- var p PathItem
- if documentPath, err = loader.loadSingleElementFromURI(ref, documentPath, &p); err != nil {
- return err
- }
- *pathItem = p
- } else {
- var resolved PathItem
- if doc, documentPath, err = loader.resolveComponent(doc, ref, documentPath, &resolved); err != nil {
- return err
- }
- *pathItem = resolved
- }
- pathItem.Ref = ref
- }
- return loader.resolvePathItemRefContinued(doc, pathItem, documentPath)
-}
-
-func (loader *Loader) resolvePathItemRefContinued(doc *T, pathItem *PathItem, documentPath *url.URL) (err error) {
- for _, parameter := range pathItem.Parameters {
- if err = loader.resolveParameterRef(doc, parameter, documentPath); err != nil {
- return
- }
- }
- for _, operation := range pathItem.Operations() {
- for _, parameter := range operation.Parameters {
- if err = loader.resolveParameterRef(doc, parameter, documentPath); err != nil {
- return
- }
- }
- if requestBody := operation.RequestBody; requestBody != nil {
- if err = loader.resolveRequestBodyRef(doc, requestBody, documentPath); err != nil {
- return
- }
- }
- for _, response := range operation.Responses {
- if err = loader.resolveResponseRef(doc, response, documentPath); err != nil {
- return
- }
- }
- for _, callback := range operation.Callbacks {
- if err = loader.resolveCallbackRef(doc, callback, documentPath); err != nil {
- return
- }
- }
- }
- return
-}
-
-func unescapeRefString(ref string) string {
- return strings.Replace(strings.Replace(ref, "~1", "/", -1), "~0", "~", -1)
-}
-
-func visitedLimit(visited []string, ref string) bool {
- visitedCount := 0
- for _, v := range visited {
- if v == ref {
- visitedCount++
- if visitedCount >= CircularReferenceCounter {
- return true
- }
- }
- }
- return false
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/loader_uri_reader.go b/vendor/github.com/getkin/kin-openapi/openapi3/loader_uri_reader.go
deleted file mode 100644
index 92ac043f..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/loader_uri_reader.go
+++ /dev/null
@@ -1,112 +0,0 @@
-package openapi3
-
-import (
- "errors"
- "fmt"
- "io/ioutil"
- "net/http"
- "net/url"
- "path/filepath"
- "sync"
-)
-
-// ReadFromURIFunc defines a function which reads the contents of a resource
-// located at a URI.
-type ReadFromURIFunc func(loader *Loader, url *url.URL) ([]byte, error)
-
-var uriMu = &sync.RWMutex{}
-
-// ErrURINotSupported indicates the ReadFromURIFunc does not know how to handle a
-// given URI.
-var ErrURINotSupported = errors.New("unsupported URI")
-
-// ReadFromURIs returns a ReadFromURIFunc which tries to read a URI using the
-// given reader functions, in the same order. If a reader function does not
-// support the URI and returns ErrURINotSupported, the next function is checked
-// until a match is found, or the URI is not supported by any.
-func ReadFromURIs(readers ...ReadFromURIFunc) ReadFromURIFunc {
- return func(loader *Loader, url *url.URL) ([]byte, error) {
- for i := range readers {
- buf, err := readers[i](loader, url)
- if err == ErrURINotSupported {
- continue
- } else if err != nil {
- return nil, err
- }
- return buf, nil
- }
- return nil, ErrURINotSupported
- }
-}
-
-// DefaultReadFromURI returns a caching ReadFromURIFunc which can read remote
-// HTTP URIs and local file URIs.
-var DefaultReadFromURI = URIMapCache(ReadFromURIs(ReadFromHTTP(http.DefaultClient), ReadFromFile))
-
-// ReadFromHTTP returns a ReadFromURIFunc which uses the given http.Client to
-// read the contents from a remote HTTP URI. This client may be customized to
-// implement timeouts, RFC 7234 caching, etc.
-func ReadFromHTTP(cl *http.Client) ReadFromURIFunc {
- return func(loader *Loader, location *url.URL) ([]byte, error) {
- if location.Scheme == "" || location.Host == "" {
- return nil, ErrURINotSupported
- }
- req, err := http.NewRequest("GET", location.String(), nil)
- if err != nil {
- return nil, err
- }
- resp, err := cl.Do(req)
- if err != nil {
- return nil, err
- }
- defer resp.Body.Close()
- if resp.StatusCode > 399 {
- return nil, fmt.Errorf("error loading %q: request returned status code %d", location.String(), resp.StatusCode)
- }
- return ioutil.ReadAll(resp.Body)
- }
-}
-
-// ReadFromFile is a ReadFromURIFunc which reads local file URIs.
-func ReadFromFile(loader *Loader, location *url.URL) ([]byte, error) {
- if location.Host != "" {
- return nil, ErrURINotSupported
- }
- if location.Scheme != "" && location.Scheme != "file" {
- return nil, ErrURINotSupported
- }
- return ioutil.ReadFile(location.Path)
-}
-
-// URIMapCache returns a ReadFromURIFunc that caches the contents read from URI
-// locations in a simple map. This cache implementation is suitable for
-// short-lived processes such as command-line tools which process OpenAPI
-// documents.
-func URIMapCache(reader ReadFromURIFunc) ReadFromURIFunc {
- cache := map[string][]byte{}
- return func(loader *Loader, location *url.URL) (buf []byte, err error) {
- if location.Scheme == "" || location.Scheme == "file" {
- if !filepath.IsAbs(location.Path) {
- // Do not cache relative file paths; this can cause trouble if
- // the current working directory changes when processing
- // multiple top-level documents.
- return reader(loader, location)
- }
- }
- uri := location.String()
- var ok bool
- uriMu.RLock()
- if buf, ok = cache[uri]; ok {
- uriMu.RUnlock()
- return
- }
- uriMu.RUnlock()
- if buf, err = reader(loader, location); err != nil {
- return
- }
- uriMu.Lock()
- defer uriMu.Unlock()
- cache[uri] = buf
- return
- }
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/media_type.go b/vendor/github.com/getkin/kin-openapi/openapi3/media_type.go
deleted file mode 100644
index 2a9b4721..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/media_type.go
+++ /dev/null
@@ -1,167 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "sort"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-// MediaType is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#media-type-object
-type MediaType struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Schema *SchemaRef `json:"schema,omitempty" yaml:"schema,omitempty"`
- Example interface{} `json:"example,omitempty" yaml:"example,omitempty"`
- Examples Examples `json:"examples,omitempty" yaml:"examples,omitempty"`
- Encoding map[string]*Encoding `json:"encoding,omitempty" yaml:"encoding,omitempty"`
-}
-
-var _ jsonpointer.JSONPointable = (*MediaType)(nil)
-
-func NewMediaType() *MediaType {
- return &MediaType{}
-}
-
-func (mediaType *MediaType) WithSchema(schema *Schema) *MediaType {
- if schema == nil {
- mediaType.Schema = nil
- } else {
- mediaType.Schema = &SchemaRef{Value: schema}
- }
- return mediaType
-}
-
-func (mediaType *MediaType) WithSchemaRef(schema *SchemaRef) *MediaType {
- mediaType.Schema = schema
- return mediaType
-}
-
-func (mediaType *MediaType) WithExample(name string, value interface{}) *MediaType {
- example := mediaType.Examples
- if example == nil {
- example = make(map[string]*ExampleRef)
- mediaType.Examples = example
- }
- example[name] = &ExampleRef{
- Value: NewExample(value),
- }
- return mediaType
-}
-
-func (mediaType *MediaType) WithEncoding(name string, enc *Encoding) *MediaType {
- encoding := mediaType.Encoding
- if encoding == nil {
- encoding = make(map[string]*Encoding)
- mediaType.Encoding = encoding
- }
- encoding[name] = enc
- return mediaType
-}
-
-// MarshalJSON returns the JSON encoding of MediaType.
-func (mediaType MediaType) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(mediaType.Extensions))
- for k, v := range mediaType.Extensions {
- m[k] = v
- }
- if x := mediaType.Schema; x != nil {
- m["schema"] = x
- }
- if x := mediaType.Example; x != nil {
- m["example"] = x
- }
- if x := mediaType.Examples; len(x) != 0 {
- m["examples"] = x
- }
- if x := mediaType.Encoding; len(x) != 0 {
- m["encoding"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets MediaType to a copy of data.
-func (mediaType *MediaType) UnmarshalJSON(data []byte) error {
- type MediaTypeBis MediaType
- var x MediaTypeBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "schema")
- delete(x.Extensions, "example")
- delete(x.Extensions, "examples")
- delete(x.Extensions, "encoding")
- *mediaType = MediaType(x)
- return nil
-}
-
-// Validate returns an error if MediaType does not comply with the OpenAPI spec.
-func (mediaType *MediaType) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if mediaType == nil {
- return nil
- }
- if schema := mediaType.Schema; schema != nil {
- if err := schema.Validate(ctx); err != nil {
- return err
- }
-
- if mediaType.Example != nil && mediaType.Examples != nil {
- return errors.New("example and examples are mutually exclusive")
- }
-
- if vo := getValidationOptions(ctx); !vo.examplesValidationDisabled {
- if example := mediaType.Example; example != nil {
- if err := validateExampleValue(ctx, example, schema.Value); err != nil {
- return fmt.Errorf("invalid example: %w", err)
- }
- }
-
- if examples := mediaType.Examples; examples != nil {
- names := make([]string, 0, len(examples))
- for name := range examples {
- names = append(names, name)
- }
- sort.Strings(names)
- for _, k := range names {
- v := examples[k]
- if err := v.Validate(ctx); err != nil {
- return fmt.Errorf("example %s: %w", k, err)
- }
- if err := validateExampleValue(ctx, v.Value.Value, schema.Value); err != nil {
- return fmt.Errorf("example %s: %w", k, err)
- }
- }
- }
- }
- }
-
- return validateExtensions(ctx, mediaType.Extensions)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (mediaType MediaType) JSONLookup(token string) (interface{}, error) {
- switch token {
- case "schema":
- if mediaType.Schema != nil {
- if mediaType.Schema.Ref != "" {
- return &Ref{Ref: mediaType.Schema.Ref}, nil
- }
- return mediaType.Schema.Value, nil
- }
- case "example":
- return mediaType.Example, nil
- case "examples":
- return mediaType.Examples, nil
- case "encoding":
- return mediaType.Encoding, nil
- }
- v, _, err := jsonpointer.GetForToken(mediaType.Extensions, token)
- return v, err
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/openapi3.go b/vendor/github.com/getkin/kin-openapi/openapi3/openapi3.go
deleted file mode 100644
index 8b8f71bb..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/openapi3.go
+++ /dev/null
@@ -1,156 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
-)
-
-// T is the root of an OpenAPI v3 document
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#openapi-object
-type T struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- OpenAPI string `json:"openapi" yaml:"openapi"` // Required
- Components *Components `json:"components,omitempty" yaml:"components,omitempty"`
- Info *Info `json:"info" yaml:"info"` // Required
- Paths Paths `json:"paths" yaml:"paths"` // Required
- Security SecurityRequirements `json:"security,omitempty" yaml:"security,omitempty"`
- Servers Servers `json:"servers,omitempty" yaml:"servers,omitempty"`
- Tags Tags `json:"tags,omitempty" yaml:"tags,omitempty"`
- ExternalDocs *ExternalDocs `json:"externalDocs,omitempty" yaml:"externalDocs,omitempty"`
-
- visited visitedComponent
-}
-
-// MarshalJSON returns the JSON encoding of T.
-func (doc T) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(doc.Extensions))
- for k, v := range doc.Extensions {
- m[k] = v
- }
- m["openapi"] = doc.OpenAPI
- if x := doc.Components; x != nil {
- m["components"] = x
- }
- m["info"] = doc.Info
- m["paths"] = doc.Paths
- if x := doc.Security; len(x) != 0 {
- m["security"] = x
- }
- if x := doc.Servers; len(x) != 0 {
- m["servers"] = x
- }
- if x := doc.Tags; len(x) != 0 {
- m["tags"] = x
- }
- if x := doc.ExternalDocs; x != nil {
- m["externalDocs"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets T to a copy of data.
-func (doc *T) UnmarshalJSON(data []byte) error {
- type TBis T
- var x TBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "openapi")
- delete(x.Extensions, "components")
- delete(x.Extensions, "info")
- delete(x.Extensions, "paths")
- delete(x.Extensions, "security")
- delete(x.Extensions, "servers")
- delete(x.Extensions, "tags")
- delete(x.Extensions, "externalDocs")
- *doc = T(x)
- return nil
-}
-
-func (doc *T) AddOperation(path string, method string, operation *Operation) {
- if doc.Paths == nil {
- doc.Paths = make(Paths)
- }
- pathItem := doc.Paths[path]
- if pathItem == nil {
- pathItem = &PathItem{}
- doc.Paths[path] = pathItem
- }
- pathItem.SetOperation(method, operation)
-}
-
-func (doc *T) AddServer(server *Server) {
- doc.Servers = append(doc.Servers, server)
-}
-
-// Validate returns an error if T does not comply with the OpenAPI spec.
-// Validations Options can be provided to modify the validation behavior.
-func (doc *T) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if doc.OpenAPI == "" {
- return errors.New("value of openapi must be a non-empty string")
- }
-
- var wrap func(error) error
- // NOTE: only mention info/components/paths/... key in this func's errors.
-
- wrap = func(e error) error { return fmt.Errorf("invalid components: %w", e) }
- if v := doc.Components; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- }
-
- wrap = func(e error) error { return fmt.Errorf("invalid info: %w", e) }
- if v := doc.Info; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- } else {
- return wrap(errors.New("must be an object"))
- }
-
- wrap = func(e error) error { return fmt.Errorf("invalid paths: %w", e) }
- if v := doc.Paths; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- } else {
- return wrap(errors.New("must be an object"))
- }
-
- wrap = func(e error) error { return fmt.Errorf("invalid security: %w", e) }
- if v := doc.Security; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- }
-
- wrap = func(e error) error { return fmt.Errorf("invalid servers: %w", e) }
- if v := doc.Servers; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- }
-
- wrap = func(e error) error { return fmt.Errorf("invalid tags: %w", e) }
- if v := doc.Tags; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- }
-
- wrap = func(e error) error { return fmt.Errorf("invalid external docs: %w", e) }
- if v := doc.ExternalDocs; v != nil {
- if err := v.Validate(ctx); err != nil {
- return wrap(err)
- }
- }
-
- return validateExtensions(ctx, doc.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/operation.go b/vendor/github.com/getkin/kin-openapi/openapi3/operation.go
deleted file mode 100644
index 645c0805..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/operation.go
+++ /dev/null
@@ -1,216 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "strconv"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-// Operation represents "operation" specified by" OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#operation-object
-type Operation struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- // Optional tags for documentation.
- Tags []string `json:"tags,omitempty" yaml:"tags,omitempty"`
-
- // Optional short summary.
- Summary string `json:"summary,omitempty" yaml:"summary,omitempty"`
-
- // Optional description. Should use CommonMark syntax.
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
-
- // Optional operation ID.
- OperationID string `json:"operationId,omitempty" yaml:"operationId,omitempty"`
-
- // Optional parameters.
- Parameters Parameters `json:"parameters,omitempty" yaml:"parameters,omitempty"`
-
- // Optional body parameter.
- RequestBody *RequestBodyRef `json:"requestBody,omitempty" yaml:"requestBody,omitempty"`
-
- // Responses.
- Responses Responses `json:"responses" yaml:"responses"` // Required
-
- // Optional callbacks
- Callbacks Callbacks `json:"callbacks,omitempty" yaml:"callbacks,omitempty"`
-
- Deprecated bool `json:"deprecated,omitempty" yaml:"deprecated,omitempty"`
-
- // Optional security requirements that overrides top-level security.
- Security *SecurityRequirements `json:"security,omitempty" yaml:"security,omitempty"`
-
- // Optional servers that overrides top-level servers.
- Servers *Servers `json:"servers,omitempty" yaml:"servers,omitempty"`
-
- ExternalDocs *ExternalDocs `json:"externalDocs,omitempty" yaml:"externalDocs,omitempty"`
-}
-
-var _ jsonpointer.JSONPointable = (*Operation)(nil)
-
-func NewOperation() *Operation {
- return &Operation{}
-}
-
-// MarshalJSON returns the JSON encoding of Operation.
-func (operation Operation) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 12+len(operation.Extensions))
- for k, v := range operation.Extensions {
- m[k] = v
- }
- if x := operation.Tags; len(x) != 0 {
- m["tags"] = x
- }
- if x := operation.Summary; x != "" {
- m["summary"] = x
- }
- if x := operation.Description; x != "" {
- m["description"] = x
- }
- if x := operation.OperationID; x != "" {
- m["operationId"] = x
- }
- if x := operation.Parameters; len(x) != 0 {
- m["parameters"] = x
- }
- if x := operation.RequestBody; x != nil {
- m["requestBody"] = x
- }
- m["responses"] = operation.Responses
- if x := operation.Callbacks; len(x) != 0 {
- m["callbacks"] = x
- }
- if x := operation.Deprecated; x {
- m["deprecated"] = x
- }
- if x := operation.Security; x != nil {
- m["security"] = x
- }
- if x := operation.Servers; x != nil {
- m["servers"] = x
- }
- if x := operation.ExternalDocs; x != nil {
- m["externalDocs"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Operation to a copy of data.
-func (operation *Operation) UnmarshalJSON(data []byte) error {
- type OperationBis Operation
- var x OperationBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "tags")
- delete(x.Extensions, "summary")
- delete(x.Extensions, "description")
- delete(x.Extensions, "operationId")
- delete(x.Extensions, "parameters")
- delete(x.Extensions, "requestBody")
- delete(x.Extensions, "responses")
- delete(x.Extensions, "callbacks")
- delete(x.Extensions, "deprecated")
- delete(x.Extensions, "security")
- delete(x.Extensions, "servers")
- delete(x.Extensions, "externalDocs")
- *operation = Operation(x)
- return nil
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (operation Operation) JSONLookup(token string) (interface{}, error) {
- switch token {
- case "requestBody":
- if operation.RequestBody != nil {
- if operation.RequestBody.Ref != "" {
- return &Ref{Ref: operation.RequestBody.Ref}, nil
- }
- return operation.RequestBody.Value, nil
- }
- case "tags":
- return operation.Tags, nil
- case "summary":
- return operation.Summary, nil
- case "description":
- return operation.Description, nil
- case "operationID":
- return operation.OperationID, nil
- case "parameters":
- return operation.Parameters, nil
- case "responses":
- return operation.Responses, nil
- case "callbacks":
- return operation.Callbacks, nil
- case "deprecated":
- return operation.Deprecated, nil
- case "security":
- return operation.Security, nil
- case "servers":
- return operation.Servers, nil
- case "externalDocs":
- return operation.ExternalDocs, nil
- }
-
- v, _, err := jsonpointer.GetForToken(operation.Extensions, token)
- return v, err
-}
-
-func (operation *Operation) AddParameter(p *Parameter) {
- operation.Parameters = append(operation.Parameters, &ParameterRef{
- Value: p,
- })
-}
-
-func (operation *Operation) AddResponse(status int, response *Response) {
- responses := operation.Responses
- if responses == nil {
- responses = NewResponses()
- operation.Responses = responses
- }
- code := "default"
- if status != 0 {
- code = strconv.FormatInt(int64(status), 10)
- }
- responses[code] = &ResponseRef{
- Value: response,
- }
-}
-
-// Validate returns an error if Operation does not comply with the OpenAPI spec.
-func (operation *Operation) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if v := operation.Parameters; v != nil {
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
-
- if v := operation.RequestBody; v != nil {
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
-
- if v := operation.Responses; v != nil {
- if err := v.Validate(ctx); err != nil {
- return err
- }
- } else {
- return errors.New("value of responses must be an object")
- }
-
- if v := operation.ExternalDocs; v != nil {
- if err := v.Validate(ctx); err != nil {
- return fmt.Errorf("invalid external docs: %w", err)
- }
- }
-
- return validateExtensions(ctx, operation.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/parameter.go b/vendor/github.com/getkin/kin-openapi/openapi3/parameter.go
deleted file mode 100644
index ec1893e9..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/parameter.go
+++ /dev/null
@@ -1,418 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "sort"
- "strconv"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type ParametersMap map[string]*ParameterRef
-
-var _ jsonpointer.JSONPointable = (*ParametersMap)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (p ParametersMap) JSONLookup(token string) (interface{}, error) {
- ref, ok := p[token]
- if ref == nil || ok == false {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// Parameters is specified by OpenAPI/Swagger 3.0 standard.
-type Parameters []*ParameterRef
-
-var _ jsonpointer.JSONPointable = (*Parameters)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (p Parameters) JSONLookup(token string) (interface{}, error) {
- index, err := strconv.Atoi(token)
- if err != nil {
- return nil, err
- }
-
- if index < 0 || index >= len(p) {
- return nil, fmt.Errorf("index %d out of bounds of array of length %d", index, len(p))
- }
-
- ref := p[index]
-
- if ref != nil && ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-func NewParameters() Parameters {
- return make(Parameters, 0, 4)
-}
-
-func (parameters Parameters) GetByInAndName(in string, name string) *Parameter {
- for _, item := range parameters {
- if v := item.Value; v != nil {
- if v.Name == name && v.In == in {
- return v
- }
- }
- }
- return nil
-}
-
-// Validate returns an error if Parameters does not comply with the OpenAPI spec.
-func (parameters Parameters) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- dupes := make(map[string]struct{})
- for _, parameterRef := range parameters {
- if v := parameterRef.Value; v != nil {
- key := v.In + ":" + v.Name
- if _, ok := dupes[key]; ok {
- return fmt.Errorf("more than one %q parameter has name %q", v.In, v.Name)
- }
- dupes[key] = struct{}{}
- }
-
- if err := parameterRef.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
-
-// Parameter is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#parameter-object
-type Parameter struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Name string `json:"name,omitempty" yaml:"name,omitempty"`
- In string `json:"in,omitempty" yaml:"in,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Style string `json:"style,omitempty" yaml:"style,omitempty"`
- Explode *bool `json:"explode,omitempty" yaml:"explode,omitempty"`
- AllowEmptyValue bool `json:"allowEmptyValue,omitempty" yaml:"allowEmptyValue,omitempty"`
- AllowReserved bool `json:"allowReserved,omitempty" yaml:"allowReserved,omitempty"`
- Deprecated bool `json:"deprecated,omitempty" yaml:"deprecated,omitempty"`
- Required bool `json:"required,omitempty" yaml:"required,omitempty"`
- Schema *SchemaRef `json:"schema,omitempty" yaml:"schema,omitempty"`
- Example interface{} `json:"example,omitempty" yaml:"example,omitempty"`
- Examples Examples `json:"examples,omitempty" yaml:"examples,omitempty"`
- Content Content `json:"content,omitempty" yaml:"content,omitempty"`
-}
-
-var _ jsonpointer.JSONPointable = (*Parameter)(nil)
-
-const (
- ParameterInPath = "path"
- ParameterInQuery = "query"
- ParameterInHeader = "header"
- ParameterInCookie = "cookie"
-)
-
-func NewPathParameter(name string) *Parameter {
- return &Parameter{
- Name: name,
- In: ParameterInPath,
- Required: true,
- }
-}
-
-func NewQueryParameter(name string) *Parameter {
- return &Parameter{
- Name: name,
- In: ParameterInQuery,
- }
-}
-
-func NewHeaderParameter(name string) *Parameter {
- return &Parameter{
- Name: name,
- In: ParameterInHeader,
- }
-}
-
-func NewCookieParameter(name string) *Parameter {
- return &Parameter{
- Name: name,
- In: ParameterInCookie,
- }
-}
-
-func (parameter *Parameter) WithDescription(value string) *Parameter {
- parameter.Description = value
- return parameter
-}
-
-func (parameter *Parameter) WithRequired(value bool) *Parameter {
- parameter.Required = value
- return parameter
-}
-
-func (parameter *Parameter) WithSchema(value *Schema) *Parameter {
- if value == nil {
- parameter.Schema = nil
- } else {
- parameter.Schema = &SchemaRef{
- Value: value,
- }
- }
- return parameter
-}
-
-// MarshalJSON returns the JSON encoding of Parameter.
-func (parameter Parameter) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 13+len(parameter.Extensions))
- for k, v := range parameter.Extensions {
- m[k] = v
- }
-
- if x := parameter.Name; x != "" {
- m["name"] = x
- }
- if x := parameter.In; x != "" {
- m["in"] = x
- }
- if x := parameter.Description; x != "" {
- m["description"] = x
- }
- if x := parameter.Style; x != "" {
- m["style"] = x
- }
- if x := parameter.Explode; x != nil {
- m["explode"] = x
- }
- if x := parameter.AllowEmptyValue; x {
- m["allowEmptyValue"] = x
- }
- if x := parameter.AllowReserved; x {
- m["allowReserved"] = x
- }
- if x := parameter.Deprecated; x {
- m["deprecated"] = x
- }
- if x := parameter.Required; x {
- m["required"] = x
- }
- if x := parameter.Schema; x != nil {
- m["schema"] = x
- }
- if x := parameter.Example; x != nil {
- m["example"] = x
- }
- if x := parameter.Examples; len(x) != 0 {
- m["examples"] = x
- }
- if x := parameter.Content; len(x) != 0 {
- m["content"] = x
- }
-
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Parameter to a copy of data.
-func (parameter *Parameter) UnmarshalJSON(data []byte) error {
- type ParameterBis Parameter
- var x ParameterBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
-
- delete(x.Extensions, "name")
- delete(x.Extensions, "in")
- delete(x.Extensions, "description")
- delete(x.Extensions, "style")
- delete(x.Extensions, "explode")
- delete(x.Extensions, "allowEmptyValue")
- delete(x.Extensions, "allowReserved")
- delete(x.Extensions, "deprecated")
- delete(x.Extensions, "required")
- delete(x.Extensions, "schema")
- delete(x.Extensions, "example")
- delete(x.Extensions, "examples")
- delete(x.Extensions, "content")
-
- *parameter = Parameter(x)
- return nil
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (parameter Parameter) JSONLookup(token string) (interface{}, error) {
- switch token {
- case "schema":
- if parameter.Schema != nil {
- if parameter.Schema.Ref != "" {
- return &Ref{Ref: parameter.Schema.Ref}, nil
- }
- return parameter.Schema.Value, nil
- }
- case "name":
- return parameter.Name, nil
- case "in":
- return parameter.In, nil
- case "description":
- return parameter.Description, nil
- case "style":
- return parameter.Style, nil
- case "explode":
- return parameter.Explode, nil
- case "allowEmptyValue":
- return parameter.AllowEmptyValue, nil
- case "allowReserved":
- return parameter.AllowReserved, nil
- case "deprecated":
- return parameter.Deprecated, nil
- case "required":
- return parameter.Required, nil
- case "example":
- return parameter.Example, nil
- case "examples":
- return parameter.Examples, nil
- case "content":
- return parameter.Content, nil
- }
-
- v, _, err := jsonpointer.GetForToken(parameter.Extensions, token)
- return v, err
-}
-
-// SerializationMethod returns a parameter's serialization method.
-// When a parameter's serialization method is not defined the method returns
-// the default serialization method corresponding to a parameter's location.
-func (parameter *Parameter) SerializationMethod() (*SerializationMethod, error) {
- switch parameter.In {
- case ParameterInPath, ParameterInHeader:
- style := parameter.Style
- if style == "" {
- style = SerializationSimple
- }
- explode := false
- if parameter.Explode != nil {
- explode = *parameter.Explode
- }
- return &SerializationMethod{Style: style, Explode: explode}, nil
- case ParameterInQuery, ParameterInCookie:
- style := parameter.Style
- if style == "" {
- style = SerializationForm
- }
- explode := true
- if parameter.Explode != nil {
- explode = *parameter.Explode
- }
- return &SerializationMethod{Style: style, Explode: explode}, nil
- default:
- return nil, fmt.Errorf("unexpected parameter's 'in': %q", parameter.In)
- }
-}
-
-// Validate returns an error if Parameter does not comply with the OpenAPI spec.
-func (parameter *Parameter) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if parameter.Name == "" {
- return errors.New("parameter name can't be blank")
- }
- in := parameter.In
- switch in {
- case
- ParameterInPath,
- ParameterInQuery,
- ParameterInHeader,
- ParameterInCookie:
- default:
- return fmt.Errorf("parameter can't have 'in' value %q", parameter.In)
- }
-
- if in == ParameterInPath && !parameter.Required {
- return fmt.Errorf("path parameter %q must be required", parameter.Name)
- }
-
- // Validate a parameter's serialization method.
- sm, err := parameter.SerializationMethod()
- if err != nil {
- return err
- }
- var smSupported bool
- switch {
- case parameter.In == ParameterInPath && sm.Style == SerializationSimple && !sm.Explode,
- parameter.In == ParameterInPath && sm.Style == SerializationSimple && sm.Explode,
- parameter.In == ParameterInPath && sm.Style == SerializationLabel && !sm.Explode,
- parameter.In == ParameterInPath && sm.Style == SerializationLabel && sm.Explode,
- parameter.In == ParameterInPath && sm.Style == SerializationMatrix && !sm.Explode,
- parameter.In == ParameterInPath && sm.Style == SerializationMatrix && sm.Explode,
-
- parameter.In == ParameterInQuery && sm.Style == SerializationForm && sm.Explode,
- parameter.In == ParameterInQuery && sm.Style == SerializationForm && !sm.Explode,
- parameter.In == ParameterInQuery && sm.Style == SerializationSpaceDelimited && sm.Explode,
- parameter.In == ParameterInQuery && sm.Style == SerializationSpaceDelimited && !sm.Explode,
- parameter.In == ParameterInQuery && sm.Style == SerializationPipeDelimited && sm.Explode,
- parameter.In == ParameterInQuery && sm.Style == SerializationPipeDelimited && !sm.Explode,
- parameter.In == ParameterInQuery && sm.Style == SerializationDeepObject && sm.Explode,
-
- parameter.In == ParameterInHeader && sm.Style == SerializationSimple && !sm.Explode,
- parameter.In == ParameterInHeader && sm.Style == SerializationSimple && sm.Explode,
-
- parameter.In == ParameterInCookie && sm.Style == SerializationForm && !sm.Explode,
- parameter.In == ParameterInCookie && sm.Style == SerializationForm && sm.Explode:
- smSupported = true
- }
- if !smSupported {
- e := fmt.Errorf("serialization method with style=%q and explode=%v is not supported by a %s parameter", sm.Style, sm.Explode, in)
- return fmt.Errorf("parameter %q schema is invalid: %w", parameter.Name, e)
- }
-
- if (parameter.Schema == nil) == (parameter.Content == nil) {
- e := errors.New("parameter must contain exactly one of content and schema")
- return fmt.Errorf("parameter %q schema is invalid: %w", parameter.Name, e)
- }
-
- if content := parameter.Content; content != nil {
- if err := content.Validate(ctx); err != nil {
- return fmt.Errorf("parameter %q content is invalid: %w", parameter.Name, err)
- }
- }
-
- if schema := parameter.Schema; schema != nil {
- if err := schema.Validate(ctx); err != nil {
- return fmt.Errorf("parameter %q schema is invalid: %w", parameter.Name, err)
- }
- if parameter.Example != nil && parameter.Examples != nil {
- return fmt.Errorf("parameter %q example and examples are mutually exclusive", parameter.Name)
- }
-
- if vo := getValidationOptions(ctx); vo.examplesValidationDisabled {
- return nil
- }
- if example := parameter.Example; example != nil {
- if err := validateExampleValue(ctx, example, schema.Value); err != nil {
- return fmt.Errorf("invalid example: %w", err)
- }
- } else if examples := parameter.Examples; examples != nil {
- names := make([]string, 0, len(examples))
- for name := range examples {
- names = append(names, name)
- }
- sort.Strings(names)
- for _, k := range names {
- v := examples[k]
- if err := v.Validate(ctx); err != nil {
- return fmt.Errorf("%s: %w", k, err)
- }
- if err := validateExampleValue(ctx, v.Value.Value, schema.Value); err != nil {
- return fmt.Errorf("%s: %w", k, err)
- }
- }
- }
- }
-
- return validateExtensions(ctx, parameter.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/path_item.go b/vendor/github.com/getkin/kin-openapi/openapi3/path_item.go
deleted file mode 100644
index fab75d93..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/path_item.go
+++ /dev/null
@@ -1,211 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "fmt"
- "net/http"
- "sort"
-)
-
-// PathItem is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#path-item-object
-type PathItem struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Ref string `json:"$ref,omitempty" yaml:"$ref,omitempty"`
- Summary string `json:"summary,omitempty" yaml:"summary,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Connect *Operation `json:"connect,omitempty" yaml:"connect,omitempty"`
- Delete *Operation `json:"delete,omitempty" yaml:"delete,omitempty"`
- Get *Operation `json:"get,omitempty" yaml:"get,omitempty"`
- Head *Operation `json:"head,omitempty" yaml:"head,omitempty"`
- Options *Operation `json:"options,omitempty" yaml:"options,omitempty"`
- Patch *Operation `json:"patch,omitempty" yaml:"patch,omitempty"`
- Post *Operation `json:"post,omitempty" yaml:"post,omitempty"`
- Put *Operation `json:"put,omitempty" yaml:"put,omitempty"`
- Trace *Operation `json:"trace,omitempty" yaml:"trace,omitempty"`
- Servers Servers `json:"servers,omitempty" yaml:"servers,omitempty"`
- Parameters Parameters `json:"parameters,omitempty" yaml:"parameters,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of PathItem.
-func (pathItem PathItem) MarshalJSON() ([]byte, error) {
- if ref := pathItem.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
-
- m := make(map[string]interface{}, 13+len(pathItem.Extensions))
- for k, v := range pathItem.Extensions {
- m[k] = v
- }
- if x := pathItem.Summary; x != "" {
- m["summary"] = x
- }
- if x := pathItem.Description; x != "" {
- m["description"] = x
- }
- if x := pathItem.Connect; x != nil {
- m["connect"] = x
- }
- if x := pathItem.Delete; x != nil {
- m["delete"] = x
- }
- if x := pathItem.Get; x != nil {
- m["get"] = x
- }
- if x := pathItem.Head; x != nil {
- m["head"] = x
- }
- if x := pathItem.Options; x != nil {
- m["options"] = x
- }
- if x := pathItem.Patch; x != nil {
- m["patch"] = x
- }
- if x := pathItem.Post; x != nil {
- m["post"] = x
- }
- if x := pathItem.Put; x != nil {
- m["put"] = x
- }
- if x := pathItem.Trace; x != nil {
- m["trace"] = x
- }
- if x := pathItem.Servers; len(x) != 0 {
- m["servers"] = x
- }
- if x := pathItem.Parameters; len(x) != 0 {
- m["parameters"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets PathItem to a copy of data.
-func (pathItem *PathItem) UnmarshalJSON(data []byte) error {
- type PathItemBis PathItem
- var x PathItemBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "$ref")
- delete(x.Extensions, "summary")
- delete(x.Extensions, "description")
- delete(x.Extensions, "connect")
- delete(x.Extensions, "delete")
- delete(x.Extensions, "get")
- delete(x.Extensions, "head")
- delete(x.Extensions, "options")
- delete(x.Extensions, "patch")
- delete(x.Extensions, "post")
- delete(x.Extensions, "put")
- delete(x.Extensions, "trace")
- delete(x.Extensions, "servers")
- delete(x.Extensions, "parameters")
- *pathItem = PathItem(x)
- return nil
-}
-
-func (pathItem *PathItem) Operations() map[string]*Operation {
- operations := make(map[string]*Operation)
- if v := pathItem.Connect; v != nil {
- operations[http.MethodConnect] = v
- }
- if v := pathItem.Delete; v != nil {
- operations[http.MethodDelete] = v
- }
- if v := pathItem.Get; v != nil {
- operations[http.MethodGet] = v
- }
- if v := pathItem.Head; v != nil {
- operations[http.MethodHead] = v
- }
- if v := pathItem.Options; v != nil {
- operations[http.MethodOptions] = v
- }
- if v := pathItem.Patch; v != nil {
- operations[http.MethodPatch] = v
- }
- if v := pathItem.Post; v != nil {
- operations[http.MethodPost] = v
- }
- if v := pathItem.Put; v != nil {
- operations[http.MethodPut] = v
- }
- if v := pathItem.Trace; v != nil {
- operations[http.MethodTrace] = v
- }
- return operations
-}
-
-func (pathItem *PathItem) GetOperation(method string) *Operation {
- switch method {
- case http.MethodConnect:
- return pathItem.Connect
- case http.MethodDelete:
- return pathItem.Delete
- case http.MethodGet:
- return pathItem.Get
- case http.MethodHead:
- return pathItem.Head
- case http.MethodOptions:
- return pathItem.Options
- case http.MethodPatch:
- return pathItem.Patch
- case http.MethodPost:
- return pathItem.Post
- case http.MethodPut:
- return pathItem.Put
- case http.MethodTrace:
- return pathItem.Trace
- default:
- panic(fmt.Errorf("unsupported HTTP method %q", method))
- }
-}
-
-func (pathItem *PathItem) SetOperation(method string, operation *Operation) {
- switch method {
- case http.MethodConnect:
- pathItem.Connect = operation
- case http.MethodDelete:
- pathItem.Delete = operation
- case http.MethodGet:
- pathItem.Get = operation
- case http.MethodHead:
- pathItem.Head = operation
- case http.MethodOptions:
- pathItem.Options = operation
- case http.MethodPatch:
- pathItem.Patch = operation
- case http.MethodPost:
- pathItem.Post = operation
- case http.MethodPut:
- pathItem.Put = operation
- case http.MethodTrace:
- pathItem.Trace = operation
- default:
- panic(fmt.Errorf("unsupported HTTP method %q", method))
- }
-}
-
-// Validate returns an error if PathItem does not comply with the OpenAPI spec.
-func (pathItem *PathItem) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- operations := pathItem.Operations()
-
- methods := make([]string, 0, len(operations))
- for method := range operations {
- methods = append(methods, method)
- }
- sort.Strings(methods)
- for _, method := range methods {
- operation := operations[method]
- if err := operation.Validate(ctx); err != nil {
- return fmt.Errorf("invalid operation %s: %v", method, err)
- }
- }
-
- return validateExtensions(ctx, pathItem.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/paths.go b/vendor/github.com/getkin/kin-openapi/openapi3/paths.go
deleted file mode 100644
index 0986b055..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/paths.go
+++ /dev/null
@@ -1,243 +0,0 @@
-package openapi3
-
-import (
- "context"
- "fmt"
- "sort"
- "strings"
-)
-
-// Paths is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#paths-object
-type Paths map[string]*PathItem
-
-// Validate returns an error if Paths does not comply with the OpenAPI spec.
-func (paths Paths) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- normalizedPaths := make(map[string]string, len(paths))
-
- keys := make([]string, 0, len(paths))
- for key := range paths {
- keys = append(keys, key)
- }
- sort.Strings(keys)
- for _, path := range keys {
- pathItem := paths[path]
- if path == "" || path[0] != '/' {
- return fmt.Errorf("path %q does not start with a forward slash (/)", path)
- }
-
- if pathItem == nil {
- pathItem = &PathItem{}
- paths[path] = pathItem
- }
-
- normalizedPath, _, varsInPath := normalizeTemplatedPath(path)
- if oldPath, ok := normalizedPaths[normalizedPath]; ok {
- return fmt.Errorf("conflicting paths %q and %q", path, oldPath)
- }
- normalizedPaths[path] = path
-
- var commonParams []string
- for _, parameterRef := range pathItem.Parameters {
- if parameterRef != nil {
- if parameter := parameterRef.Value; parameter != nil && parameter.In == ParameterInPath {
- commonParams = append(commonParams, parameter.Name)
- }
- }
- }
- operations := pathItem.Operations()
- methods := make([]string, 0, len(operations))
- for method := range operations {
- methods = append(methods, method)
- }
- sort.Strings(methods)
- for _, method := range methods {
- operation := operations[method]
- var setParams []string
- for _, parameterRef := range operation.Parameters {
- if parameterRef != nil {
- if parameter := parameterRef.Value; parameter != nil && parameter.In == ParameterInPath {
- setParams = append(setParams, parameter.Name)
- }
- }
- }
- if expected := len(setParams) + len(commonParams); expected != len(varsInPath) {
- expected -= len(varsInPath)
- if expected < 0 {
- expected *= -1
- }
- missing := make(map[string]struct{}, expected)
- definedParams := append(setParams, commonParams...)
- for _, name := range definedParams {
- if _, ok := varsInPath[name]; !ok {
- missing[name] = struct{}{}
- }
- }
- for name := range varsInPath {
- got := false
- for _, othername := range definedParams {
- if othername == name {
- got = true
- break
- }
- }
- if !got {
- missing[name] = struct{}{}
- }
- }
- if len(missing) != 0 {
- missings := make([]string, 0, len(missing))
- for name := range missing {
- missings = append(missings, name)
- }
- return fmt.Errorf("operation %s %s must define exactly all path parameters (missing: %v)", method, path, missings)
- }
- }
- }
-
- if err := pathItem.Validate(ctx); err != nil {
- return fmt.Errorf("invalid path %s: %v", path, err)
- }
- }
-
- if err := paths.validateUniqueOperationIDs(); err != nil {
- return err
- }
-
- return nil
-}
-
-// InMatchingOrder returns paths in the order they are matched against URLs.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#paths-object
-// When matching URLs, concrete (non-templated) paths would be matched
-// before their templated counterparts.
-func (paths Paths) InMatchingOrder() []string {
- // NOTE: sorting by number of variables ASC then by descending lexicographical
- // order seems to be a good heuristic.
- if paths == nil {
- return nil
- }
-
- vars := make(map[int][]string)
- max := 0
- for path := range paths {
- count := strings.Count(path, "}")
- vars[count] = append(vars[count], path)
- if count > max {
- max = count
- }
- }
-
- ordered := make([]string, 0, len(paths))
- for c := 0; c <= max; c++ {
- if ps, ok := vars[c]; ok {
- sort.Sort(sort.Reverse(sort.StringSlice(ps)))
- ordered = append(ordered, ps...)
- }
- }
- return ordered
-}
-
-// Find returns a path that matches the key.
-//
-// The method ignores differences in template variable names (except possible "*" suffix).
-//
-// For example:
-//
-// paths := openapi3.Paths {
-// "/person/{personName}": &openapi3.PathItem{},
-// }
-// pathItem := path.Find("/person/{name}")
-//
-// would return the correct path item.
-func (paths Paths) Find(key string) *PathItem {
- // Try directly access the map
- pathItem := paths[key]
- if pathItem != nil {
- return pathItem
- }
-
- normalizedPath, expected, _ := normalizeTemplatedPath(key)
- for path, pathItem := range paths {
- pathNormalized, got, _ := normalizeTemplatedPath(path)
- if got == expected && pathNormalized == normalizedPath {
- return pathItem
- }
- }
- return nil
-}
-
-func (paths Paths) validateUniqueOperationIDs() error {
- operationIDs := make(map[string]string)
- for urlPath, pathItem := range paths {
- if pathItem == nil {
- continue
- }
- for httpMethod, operation := range pathItem.Operations() {
- if operation == nil || operation.OperationID == "" {
- continue
- }
- endpoint := httpMethod + " " + urlPath
- if endpointDup, ok := operationIDs[operation.OperationID]; ok {
- if endpoint > endpointDup { // For make error message a bit more deterministic. May be useful for tests.
- endpoint, endpointDup = endpointDup, endpoint
- }
- return fmt.Errorf("operations %q and %q have the same operation id %q",
- endpoint, endpointDup, operation.OperationID)
- }
- operationIDs[operation.OperationID] = endpoint
- }
- }
- return nil
-}
-
-func normalizeTemplatedPath(path string) (string, uint, map[string]struct{}) {
- if strings.IndexByte(path, '{') < 0 {
- return path, 0, nil
- }
-
- var buffTpl strings.Builder
- buffTpl.Grow(len(path))
-
- var (
- cc rune
- count uint
- isVariable bool
- vars = make(map[string]struct{})
- buffVar strings.Builder
- )
- for i, c := range path {
- if isVariable {
- if c == '}' {
- // End path variable
- isVariable = false
-
- vars[buffVar.String()] = struct{}{}
- buffVar = strings.Builder{}
-
- // First append possible '*' before this character
- // The character '}' will be appended
- if i > 0 && cc == '*' {
- buffTpl.WriteRune(cc)
- }
- } else {
- buffVar.WriteRune(c)
- continue
- }
-
- } else if c == '{' {
- // Begin path variable
- isVariable = true
-
- // The character '{' will be appended
- count++
- }
-
- // Append the character
- buffTpl.WriteRune(c)
- cc = c
- }
- return buffTpl.String(), count, vars
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/ref.go b/vendor/github.com/getkin/kin-openapi/openapi3/ref.go
deleted file mode 100644
index a937de4a..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/ref.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package openapi3
-
-// Ref is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#reference-object
-type Ref struct {
- Ref string `json:"$ref" yaml:"$ref"`
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/refs.go b/vendor/github.com/getkin/kin-openapi/openapi3/refs.go
deleted file mode 100644
index cc9b41a4..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/refs.go
+++ /dev/null
@@ -1,704 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "fmt"
- "sort"
-
- "github.com/go-openapi/jsonpointer"
- "github.com/perimeterx/marshmallow"
-)
-
-// CallbackRef represents either a Callback or a $ref to a Callback.
-// When serializing and both fields are set, Ref is preferred over Value.
-type CallbackRef struct {
- Ref string
- Value *Callback
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*CallbackRef)(nil)
-
-// MarshalYAML returns the YAML encoding of CallbackRef.
-func (x CallbackRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of CallbackRef.
-func (x CallbackRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return json.Marshal(x.Value)
-}
-
-// UnmarshalJSON sets CallbackRef to a copy of data.
-func (x *CallbackRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if CallbackRef does not comply with the OpenAPI spec.
-func (x *CallbackRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *CallbackRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// ExampleRef represents either a Example or a $ref to a Example.
-// When serializing and both fields are set, Ref is preferred over Value.
-type ExampleRef struct {
- Ref string
- Value *Example
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*ExampleRef)(nil)
-
-// MarshalYAML returns the YAML encoding of ExampleRef.
-func (x ExampleRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of ExampleRef.
-func (x ExampleRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets ExampleRef to a copy of data.
-func (x *ExampleRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if ExampleRef does not comply with the OpenAPI spec.
-func (x *ExampleRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *ExampleRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// HeaderRef represents either a Header or a $ref to a Header.
-// When serializing and both fields are set, Ref is preferred over Value.
-type HeaderRef struct {
- Ref string
- Value *Header
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*HeaderRef)(nil)
-
-// MarshalYAML returns the YAML encoding of HeaderRef.
-func (x HeaderRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of HeaderRef.
-func (x HeaderRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets HeaderRef to a copy of data.
-func (x *HeaderRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if HeaderRef does not comply with the OpenAPI spec.
-func (x *HeaderRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *HeaderRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// LinkRef represents either a Link or a $ref to a Link.
-// When serializing and both fields are set, Ref is preferred over Value.
-type LinkRef struct {
- Ref string
- Value *Link
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*LinkRef)(nil)
-
-// MarshalYAML returns the YAML encoding of LinkRef.
-func (x LinkRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of LinkRef.
-func (x LinkRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets LinkRef to a copy of data.
-func (x *LinkRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if LinkRef does not comply with the OpenAPI spec.
-func (x *LinkRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *LinkRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// ParameterRef represents either a Parameter or a $ref to a Parameter.
-// When serializing and both fields are set, Ref is preferred over Value.
-type ParameterRef struct {
- Ref string
- Value *Parameter
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*ParameterRef)(nil)
-
-// MarshalYAML returns the YAML encoding of ParameterRef.
-func (x ParameterRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of ParameterRef.
-func (x ParameterRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets ParameterRef to a copy of data.
-func (x *ParameterRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if ParameterRef does not comply with the OpenAPI spec.
-func (x *ParameterRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *ParameterRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// RequestBodyRef represents either a RequestBody or a $ref to a RequestBody.
-// When serializing and both fields are set, Ref is preferred over Value.
-type RequestBodyRef struct {
- Ref string
- Value *RequestBody
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*RequestBodyRef)(nil)
-
-// MarshalYAML returns the YAML encoding of RequestBodyRef.
-func (x RequestBodyRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of RequestBodyRef.
-func (x RequestBodyRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets RequestBodyRef to a copy of data.
-func (x *RequestBodyRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if RequestBodyRef does not comply with the OpenAPI spec.
-func (x *RequestBodyRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *RequestBodyRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// ResponseRef represents either a Response or a $ref to a Response.
-// When serializing and both fields are set, Ref is preferred over Value.
-type ResponseRef struct {
- Ref string
- Value *Response
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*ResponseRef)(nil)
-
-// MarshalYAML returns the YAML encoding of ResponseRef.
-func (x ResponseRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of ResponseRef.
-func (x ResponseRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets ResponseRef to a copy of data.
-func (x *ResponseRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if ResponseRef does not comply with the OpenAPI spec.
-func (x *ResponseRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *ResponseRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// SchemaRef represents either a Schema or a $ref to a Schema.
-// When serializing and both fields are set, Ref is preferred over Value.
-type SchemaRef struct {
- Ref string
- Value *Schema
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*SchemaRef)(nil)
-
-// MarshalYAML returns the YAML encoding of SchemaRef.
-func (x SchemaRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of SchemaRef.
-func (x SchemaRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets SchemaRef to a copy of data.
-func (x *SchemaRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if SchemaRef does not comply with the OpenAPI spec.
-func (x *SchemaRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *SchemaRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
-
-// SecuritySchemeRef represents either a SecurityScheme or a $ref to a SecurityScheme.
-// When serializing and both fields are set, Ref is preferred over Value.
-type SecuritySchemeRef struct {
- Ref string
- Value *SecurityScheme
- extra []string
-}
-
-var _ jsonpointer.JSONPointable = (*SecuritySchemeRef)(nil)
-
-// MarshalYAML returns the YAML encoding of SecuritySchemeRef.
-func (x SecuritySchemeRef) MarshalYAML() (interface{}, error) {
- if ref := x.Ref; ref != "" {
- return &Ref{Ref: ref}, nil
- }
- return x.Value, nil
-}
-
-// MarshalJSON returns the JSON encoding of SecuritySchemeRef.
-func (x SecuritySchemeRef) MarshalJSON() ([]byte, error) {
- if ref := x.Ref; ref != "" {
- return json.Marshal(Ref{Ref: ref})
- }
- return x.Value.MarshalJSON()
-}
-
-// UnmarshalJSON sets SecuritySchemeRef to a copy of data.
-func (x *SecuritySchemeRef) UnmarshalJSON(data []byte) error {
- var refOnly Ref
- if extra, err := marshmallow.Unmarshal(data, &refOnly, marshmallow.WithExcludeKnownFieldsFromMap(true)); err == nil && refOnly.Ref != "" {
- x.Ref = refOnly.Ref
- if len(extra) != 0 {
- x.extra = make([]string, 0, len(extra))
- for key := range extra {
- x.extra = append(x.extra, key)
- }
- }
- return nil
- }
- return json.Unmarshal(data, &x.Value)
-}
-
-// Validate returns an error if SecuritySchemeRef does not comply with the OpenAPI spec.
-func (x *SecuritySchemeRef) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- if extra := x.extra; len(extra) != 0 {
- sort.Strings(extra)
-
- extras := make([]string, 0, len(extra))
- allowed := getValidationOptions(ctx).extraSiblingFieldsAllowed
- if allowed == nil {
- allowed = make(map[string]struct{}, 0)
- }
- for _, ex := range extra {
- if _, ok := allowed[ex]; !ok {
- extras = append(extras, ex)
- }
- }
- if len(extras) != 0 {
- return fmt.Errorf("extra sibling fields: %+v", extras)
- }
- }
- if v := x.Value; v != nil {
- return v.Validate(ctx)
- }
- return foundUnresolvedRef(x.Ref)
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (x *SecuritySchemeRef) JSONLookup(token string) (interface{}, error) {
- if token == "$ref" {
- return x.Ref, nil
- }
- ptr, _, err := jsonpointer.GetForToken(x.Value, token)
- return ptr, err
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/request_body.go b/vendor/github.com/getkin/kin-openapi/openapi3/request_body.go
deleted file mode 100644
index de8919f4..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/request_body.go
+++ /dev/null
@@ -1,146 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type RequestBodies map[string]*RequestBodyRef
-
-var _ jsonpointer.JSONPointable = (*RequestBodyRef)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (r RequestBodies) JSONLookup(token string) (interface{}, error) {
- ref, ok := r[token]
- if ok == false {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref != nil && ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// RequestBody is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#request-body-object
-type RequestBody struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Required bool `json:"required,omitempty" yaml:"required,omitempty"`
- Content Content `json:"content" yaml:"content"`
-}
-
-func NewRequestBody() *RequestBody {
- return &RequestBody{}
-}
-
-func (requestBody *RequestBody) WithDescription(value string) *RequestBody {
- requestBody.Description = value
- return requestBody
-}
-
-func (requestBody *RequestBody) WithRequired(value bool) *RequestBody {
- requestBody.Required = value
- return requestBody
-}
-
-func (requestBody *RequestBody) WithContent(content Content) *RequestBody {
- requestBody.Content = content
- return requestBody
-}
-
-func (requestBody *RequestBody) WithSchemaRef(value *SchemaRef, consumes []string) *RequestBody {
- requestBody.Content = NewContentWithSchemaRef(value, consumes)
- return requestBody
-}
-
-func (requestBody *RequestBody) WithSchema(value *Schema, consumes []string) *RequestBody {
- requestBody.Content = NewContentWithSchema(value, consumes)
- return requestBody
-}
-
-func (requestBody *RequestBody) WithJSONSchemaRef(value *SchemaRef) *RequestBody {
- requestBody.Content = NewContentWithJSONSchemaRef(value)
- return requestBody
-}
-
-func (requestBody *RequestBody) WithJSONSchema(value *Schema) *RequestBody {
- requestBody.Content = NewContentWithJSONSchema(value)
- return requestBody
-}
-
-func (requestBody *RequestBody) WithFormDataSchemaRef(value *SchemaRef) *RequestBody {
- requestBody.Content = NewContentWithFormDataSchemaRef(value)
- return requestBody
-}
-
-func (requestBody *RequestBody) WithFormDataSchema(value *Schema) *RequestBody {
- requestBody.Content = NewContentWithFormDataSchema(value)
- return requestBody
-}
-
-func (requestBody *RequestBody) GetMediaType(mediaType string) *MediaType {
- m := requestBody.Content
- if m == nil {
- return nil
- }
- return m[mediaType]
-}
-
-// MarshalJSON returns the JSON encoding of RequestBody.
-func (requestBody RequestBody) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 3+len(requestBody.Extensions))
- for k, v := range requestBody.Extensions {
- m[k] = v
- }
- if x := requestBody.Description; x != "" {
- m["description"] = requestBody.Description
- }
- if x := requestBody.Required; x {
- m["required"] = x
- }
- if x := requestBody.Content; true {
- m["content"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets RequestBody to a copy of data.
-func (requestBody *RequestBody) UnmarshalJSON(data []byte) error {
- type RequestBodyBis RequestBody
- var x RequestBodyBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "description")
- delete(x.Extensions, "required")
- delete(x.Extensions, "content")
- *requestBody = RequestBody(x)
- return nil
-}
-
-// Validate returns an error if RequestBody does not comply with the OpenAPI spec.
-func (requestBody *RequestBody) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if requestBody.Content == nil {
- return errors.New("content of the request body is required")
- }
-
- if vo := getValidationOptions(ctx); !vo.examplesValidationDisabled {
- vo.examplesValidationAsReq, vo.examplesValidationAsRes = true, false
- }
-
- if err := requestBody.Content.Validate(ctx); err != nil {
- return err
- }
-
- return validateExtensions(ctx, requestBody.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/response.go b/vendor/github.com/getkin/kin-openapi/openapi3/response.go
deleted file mode 100644
index b85c9145..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/response.go
+++ /dev/null
@@ -1,183 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "sort"
- "strconv"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-// Responses is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#responses-object
-type Responses map[string]*ResponseRef
-
-var _ jsonpointer.JSONPointable = (*Responses)(nil)
-
-func NewResponses() Responses {
- r := make(Responses)
- r["default"] = &ResponseRef{Value: NewResponse().WithDescription("")}
- return r
-}
-
-func (responses Responses) Default() *ResponseRef {
- return responses["default"]
-}
-
-func (responses Responses) Get(status int) *ResponseRef {
- return responses[strconv.FormatInt(int64(status), 10)]
-}
-
-// Validate returns an error if Responses does not comply with the OpenAPI spec.
-func (responses Responses) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if len(responses) == 0 {
- return errors.New("the responses object MUST contain at least one response code")
- }
-
- keys := make([]string, 0, len(responses))
- for key := range responses {
- keys = append(keys, key)
- }
- sort.Strings(keys)
- for _, key := range keys {
- v := responses[key]
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (responses Responses) JSONLookup(token string) (interface{}, error) {
- ref, ok := responses[token]
- if ok == false {
- return nil, fmt.Errorf("invalid token reference: %q", token)
- }
-
- if ref != nil && ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// Response is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#response-object
-type Response struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Description *string `json:"description,omitempty" yaml:"description,omitempty"`
- Headers Headers `json:"headers,omitempty" yaml:"headers,omitempty"`
- Content Content `json:"content,omitempty" yaml:"content,omitempty"`
- Links Links `json:"links,omitempty" yaml:"links,omitempty"`
-}
-
-func NewResponse() *Response {
- return &Response{}
-}
-
-func (response *Response) WithDescription(value string) *Response {
- response.Description = &value
- return response
-}
-
-func (response *Response) WithContent(content Content) *Response {
- response.Content = content
- return response
-}
-
-func (response *Response) WithJSONSchema(schema *Schema) *Response {
- response.Content = NewContentWithJSONSchema(schema)
- return response
-}
-
-func (response *Response) WithJSONSchemaRef(schema *SchemaRef) *Response {
- response.Content = NewContentWithJSONSchemaRef(schema)
- return response
-}
-
-// MarshalJSON returns the JSON encoding of Response.
-func (response Response) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(response.Extensions))
- for k, v := range response.Extensions {
- m[k] = v
- }
- if x := response.Description; x != nil {
- m["description"] = x
- }
- if x := response.Headers; len(x) != 0 {
- m["headers"] = x
- }
- if x := response.Content; len(x) != 0 {
- m["content"] = x
- }
- if x := response.Links; len(x) != 0 {
- m["links"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Response to a copy of data.
-func (response *Response) UnmarshalJSON(data []byte) error {
- type ResponseBis Response
- var x ResponseBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "description")
- delete(x.Extensions, "headers")
- delete(x.Extensions, "content")
- delete(x.Extensions, "links")
- *response = Response(x)
- return nil
-}
-
-// Validate returns an error if Response does not comply with the OpenAPI spec.
-func (response *Response) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if response.Description == nil {
- return errors.New("a short description of the response is required")
- }
- if vo := getValidationOptions(ctx); !vo.examplesValidationDisabled {
- vo.examplesValidationAsReq, vo.examplesValidationAsRes = false, true
- }
-
- if content := response.Content; content != nil {
- if err := content.Validate(ctx); err != nil {
- return err
- }
- }
-
- headers := make([]string, 0, len(response.Headers))
- for name := range response.Headers {
- headers = append(headers, name)
- }
- sort.Strings(headers)
- for _, name := range headers {
- header := response.Headers[name]
- if err := header.Validate(ctx); err != nil {
- return err
- }
- }
-
- links := make([]string, 0, len(response.Links))
- for name := range response.Links {
- links = append(links, name)
- }
- sort.Strings(links)
- for _, name := range links {
- link := response.Links[name]
- if err := link.Validate(ctx); err != nil {
- return err
- }
- }
-
- return validateExtensions(ctx, response.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/schema.go b/vendor/github.com/getkin/kin-openapi/openapi3/schema.go
deleted file mode 100644
index b6be8c1b..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/schema.go
+++ /dev/null
@@ -1,2123 +0,0 @@
-package openapi3
-
-import (
- "bytes"
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "math"
- "math/big"
- "reflect"
- "regexp"
- "sort"
- "strconv"
- "strings"
- "unicode/utf16"
-
- "github.com/go-openapi/jsonpointer"
- "github.com/mohae/deepcopy"
-)
-
-const (
- TypeArray = "array"
- TypeBoolean = "boolean"
- TypeInteger = "integer"
- TypeNumber = "number"
- TypeObject = "object"
- TypeString = "string"
-
- // constants for integer formats
- formatMinInt32 = float64(math.MinInt32)
- formatMaxInt32 = float64(math.MaxInt32)
- formatMinInt64 = float64(math.MinInt64)
- formatMaxInt64 = float64(math.MaxInt64)
-)
-
-var (
- // SchemaErrorDetailsDisabled disables printing of details about schema errors.
- SchemaErrorDetailsDisabled = false
-
- errSchema = errors.New("input does not match the schema")
-
- // ErrOneOfConflict is the SchemaError Origin when data matches more than one oneOf schema
- ErrOneOfConflict = errors.New("input matches more than one oneOf schemas")
-
- // ErrSchemaInputNaN may be returned when validating a number
- ErrSchemaInputNaN = errors.New("floating point NaN is not allowed")
- // ErrSchemaInputInf may be returned when validating a number
- ErrSchemaInputInf = errors.New("floating point Inf is not allowed")
-)
-
-// Float64Ptr is a helper for defining OpenAPI schemas.
-func Float64Ptr(value float64) *float64 {
- return &value
-}
-
-// BoolPtr is a helper for defining OpenAPI schemas.
-func BoolPtr(value bool) *bool {
- return &value
-}
-
-// Int64Ptr is a helper for defining OpenAPI schemas.
-func Int64Ptr(value int64) *int64 {
- return &value
-}
-
-// Uint64Ptr is a helper for defining OpenAPI schemas.
-func Uint64Ptr(value uint64) *uint64 {
- return &value
-}
-
-// NewSchemaRef simply builds a SchemaRef
-func NewSchemaRef(ref string, value *Schema) *SchemaRef {
- return &SchemaRef{
- Ref: ref,
- Value: value,
- }
-}
-
-type Schemas map[string]*SchemaRef
-
-var _ jsonpointer.JSONPointable = (*Schemas)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (s Schemas) JSONLookup(token string) (interface{}, error) {
- ref, ok := s[token]
- if ref == nil || ok == false {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-type SchemaRefs []*SchemaRef
-
-var _ jsonpointer.JSONPointable = (*SchemaRefs)(nil)
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (s SchemaRefs) JSONLookup(token string) (interface{}, error) {
- i, err := strconv.ParseUint(token, 10, 64)
- if err != nil {
- return nil, err
- }
-
- if i >= uint64(len(s)) {
- return nil, fmt.Errorf("index out of range: %d", i)
- }
-
- ref := s[i]
-
- if ref == nil || ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-// Schema is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#schema-object
-type Schema struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- OneOf SchemaRefs `json:"oneOf,omitempty" yaml:"oneOf,omitempty"`
- AnyOf SchemaRefs `json:"anyOf,omitempty" yaml:"anyOf,omitempty"`
- AllOf SchemaRefs `json:"allOf,omitempty" yaml:"allOf,omitempty"`
- Not *SchemaRef `json:"not,omitempty" yaml:"not,omitempty"`
- Type string `json:"type,omitempty" yaml:"type,omitempty"`
- Title string `json:"title,omitempty" yaml:"title,omitempty"`
- Format string `json:"format,omitempty" yaml:"format,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Enum []interface{} `json:"enum,omitempty" yaml:"enum,omitempty"`
- Default interface{} `json:"default,omitempty" yaml:"default,omitempty"`
- Example interface{} `json:"example,omitempty" yaml:"example,omitempty"`
- ExternalDocs *ExternalDocs `json:"externalDocs,omitempty" yaml:"externalDocs,omitempty"`
-
- // Array-related, here for struct compactness
- UniqueItems bool `json:"uniqueItems,omitempty" yaml:"uniqueItems,omitempty"`
- // Number-related, here for struct compactness
- ExclusiveMin bool `json:"exclusiveMinimum,omitempty" yaml:"exclusiveMinimum,omitempty"`
- ExclusiveMax bool `json:"exclusiveMaximum,omitempty" yaml:"exclusiveMaximum,omitempty"`
- // Properties
- Nullable bool `json:"nullable,omitempty" yaml:"nullable,omitempty"`
- ReadOnly bool `json:"readOnly,omitempty" yaml:"readOnly,omitempty"`
- WriteOnly bool `json:"writeOnly,omitempty" yaml:"writeOnly,omitempty"`
- AllowEmptyValue bool `json:"allowEmptyValue,omitempty" yaml:"allowEmptyValue,omitempty"`
- Deprecated bool `json:"deprecated,omitempty" yaml:"deprecated,omitempty"`
- XML *XML `json:"xml,omitempty" yaml:"xml,omitempty"`
-
- // Number
- Min *float64 `json:"minimum,omitempty" yaml:"minimum,omitempty"`
- Max *float64 `json:"maximum,omitempty" yaml:"maximum,omitempty"`
- MultipleOf *float64 `json:"multipleOf,omitempty" yaml:"multipleOf,omitempty"`
-
- // String
- MinLength uint64 `json:"minLength,omitempty" yaml:"minLength,omitempty"`
- MaxLength *uint64 `json:"maxLength,omitempty" yaml:"maxLength,omitempty"`
- Pattern string `json:"pattern,omitempty" yaml:"pattern,omitempty"`
- compiledPattern *regexp.Regexp
-
- // Array
- MinItems uint64 `json:"minItems,omitempty" yaml:"minItems,omitempty"`
- MaxItems *uint64 `json:"maxItems,omitempty" yaml:"maxItems,omitempty"`
- Items *SchemaRef `json:"items,omitempty" yaml:"items,omitempty"`
-
- // Object
- Required []string `json:"required,omitempty" yaml:"required,omitempty"`
- Properties Schemas `json:"properties,omitempty" yaml:"properties,omitempty"`
- MinProps uint64 `json:"minProperties,omitempty" yaml:"minProperties,omitempty"`
- MaxProps *uint64 `json:"maxProperties,omitempty" yaml:"maxProperties,omitempty"`
- AdditionalProperties AdditionalProperties `json:"additionalProperties,omitempty" yaml:"additionalProperties,omitempty"`
- Discriminator *Discriminator `json:"discriminator,omitempty" yaml:"discriminator,omitempty"`
-}
-
-type AdditionalProperties struct {
- Has *bool
- Schema *SchemaRef
-}
-
-// MarshalJSON returns the JSON encoding of AdditionalProperties.
-func (addProps AdditionalProperties) MarshalJSON() ([]byte, error) {
- if x := addProps.Has; x != nil {
- if *x {
- return []byte("true"), nil
- }
- return []byte("false"), nil
- }
- if x := addProps.Schema; x != nil {
- return json.Marshal(x)
- }
- return nil, nil
-}
-
-// UnmarshalJSON sets AdditionalProperties to a copy of data.
-func (addProps *AdditionalProperties) UnmarshalJSON(data []byte) error {
- var x interface{}
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- switch y := x.(type) {
- case nil:
- case bool:
- addProps.Has = &y
- case map[string]interface{}:
- if len(y) == 0 {
- addProps.Schema = &SchemaRef{Value: &Schema{}}
- } else {
- buf := new(bytes.Buffer)
- json.NewEncoder(buf).Encode(y)
- if err := json.NewDecoder(buf).Decode(&addProps.Schema); err != nil {
- return err
- }
- }
- default:
- return errors.New("cannot unmarshal additionalProperties: value must be either a schema object or a boolean")
- }
- return nil
-}
-
-var _ jsonpointer.JSONPointable = (*Schema)(nil)
-
-func NewSchema() *Schema {
- return &Schema{}
-}
-
-// MarshalJSON returns the JSON encoding of Schema.
-func (schema Schema) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 36+len(schema.Extensions))
- for k, v := range schema.Extensions {
- m[k] = v
- }
-
- if x := schema.OneOf; len(x) != 0 {
- m["oneOf"] = x
- }
- if x := schema.AnyOf; len(x) != 0 {
- m["anyOf"] = x
- }
- if x := schema.AllOf; len(x) != 0 {
- m["allOf"] = x
- }
- if x := schema.Not; x != nil {
- m["not"] = x
- }
- if x := schema.Type; len(x) != 0 {
- m["type"] = x
- }
- if x := schema.Title; len(x) != 0 {
- m["title"] = x
- }
- if x := schema.Format; len(x) != 0 {
- m["format"] = x
- }
- if x := schema.Description; len(x) != 0 {
- m["description"] = x
- }
- if x := schema.Enum; len(x) != 0 {
- m["enum"] = x
- }
- if x := schema.Default; x != nil {
- m["default"] = x
- }
- if x := schema.Example; x != nil {
- m["example"] = x
- }
- if x := schema.ExternalDocs; x != nil {
- m["externalDocs"] = x
- }
-
- // Array-related
- if x := schema.UniqueItems; x {
- m["uniqueItems"] = x
- }
- // Number-related
- if x := schema.ExclusiveMin; x {
- m["exclusiveMinimum"] = x
- }
- if x := schema.ExclusiveMax; x {
- m["exclusiveMaximum"] = x
- }
- // Properties
- if x := schema.Nullable; x {
- m["nullable"] = x
- }
- if x := schema.ReadOnly; x {
- m["readOnly"] = x
- }
- if x := schema.WriteOnly; x {
- m["writeOnly"] = x
- }
- if x := schema.AllowEmptyValue; x {
- m["allowEmptyValue"] = x
- }
- if x := schema.Deprecated; x {
- m["deprecated"] = x
- }
- if x := schema.XML; x != nil {
- m["xml"] = x
- }
-
- // Number
- if x := schema.Min; x != nil {
- m["minimum"] = x
- }
- if x := schema.Max; x != nil {
- m["maximum"] = x
- }
- if x := schema.MultipleOf; x != nil {
- m["multipleOf"] = x
- }
-
- // String
- if x := schema.MinLength; x != 0 {
- m["minLength"] = x
- }
- if x := schema.MaxLength; x != nil {
- m["maxLength"] = x
- }
- if x := schema.Pattern; x != "" {
- m["pattern"] = x
- }
-
- // Array
- if x := schema.MinItems; x != 0 {
- m["minItems"] = x
- }
- if x := schema.MaxItems; x != nil {
- m["maxItems"] = x
- }
- if x := schema.Items; x != nil {
- m["items"] = x
- }
-
- // Object
- if x := schema.Required; len(x) != 0 {
- m["required"] = x
- }
- if x := schema.Properties; len(x) != 0 {
- m["properties"] = x
- }
- if x := schema.MinProps; x != 0 {
- m["minProperties"] = x
- }
- if x := schema.MaxProps; x != nil {
- m["maxProperties"] = x
- }
- if x := schema.AdditionalProperties; x.Has != nil || x.Schema != nil {
- m["additionalProperties"] = &x
- }
- if x := schema.Discriminator; x != nil {
- m["discriminator"] = x
- }
-
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Schema to a copy of data.
-func (schema *Schema) UnmarshalJSON(data []byte) error {
- type SchemaBis Schema
- var x SchemaBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
-
- delete(x.Extensions, "oneOf")
- delete(x.Extensions, "anyOf")
- delete(x.Extensions, "allOf")
- delete(x.Extensions, "not")
- delete(x.Extensions, "type")
- delete(x.Extensions, "title")
- delete(x.Extensions, "format")
- delete(x.Extensions, "description")
- delete(x.Extensions, "enum")
- delete(x.Extensions, "default")
- delete(x.Extensions, "example")
- delete(x.Extensions, "externalDocs")
-
- // Array-related
- delete(x.Extensions, "uniqueItems")
- // Number-related
- delete(x.Extensions, "exclusiveMinimum")
- delete(x.Extensions, "exclusiveMaximum")
- // Properties
- delete(x.Extensions, "nullable")
- delete(x.Extensions, "readOnly")
- delete(x.Extensions, "writeOnly")
- delete(x.Extensions, "allowEmptyValue")
- delete(x.Extensions, "deprecated")
- delete(x.Extensions, "xml")
-
- // Number
- delete(x.Extensions, "minimum")
- delete(x.Extensions, "maximum")
- delete(x.Extensions, "multipleOf")
-
- // String
- delete(x.Extensions, "minLength")
- delete(x.Extensions, "maxLength")
- delete(x.Extensions, "pattern")
-
- // Array
- delete(x.Extensions, "minItems")
- delete(x.Extensions, "maxItems")
- delete(x.Extensions, "items")
-
- // Object
- delete(x.Extensions, "required")
- delete(x.Extensions, "properties")
- delete(x.Extensions, "minProperties")
- delete(x.Extensions, "maxProperties")
- delete(x.Extensions, "additionalProperties")
- delete(x.Extensions, "discriminator")
-
- *schema = Schema(x)
-
- if schema.Format == "date" {
- // This is a fix for: https://github.com/getkin/kin-openapi/issues/697
- if eg, ok := schema.Example.(string); ok {
- schema.Example = strings.TrimSuffix(eg, "T00:00:00Z")
- }
- }
- return nil
-}
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (schema Schema) JSONLookup(token string) (interface{}, error) {
- switch token {
- case "additionalProperties":
- if addProps := schema.AdditionalProperties.Has; addProps != nil {
- return *addProps, nil
- }
- if addProps := schema.AdditionalProperties.Schema; addProps != nil {
- if addProps.Ref != "" {
- return &Ref{Ref: addProps.Ref}, nil
- }
- return addProps.Value, nil
- }
- case "not":
- if schema.Not != nil {
- if schema.Not.Ref != "" {
- return &Ref{Ref: schema.Not.Ref}, nil
- }
- return schema.Not.Value, nil
- }
- case "items":
- if schema.Items != nil {
- if schema.Items.Ref != "" {
- return &Ref{Ref: schema.Items.Ref}, nil
- }
- return schema.Items.Value, nil
- }
- case "oneOf":
- return schema.OneOf, nil
- case "anyOf":
- return schema.AnyOf, nil
- case "allOf":
- return schema.AllOf, nil
- case "type":
- return schema.Type, nil
- case "title":
- return schema.Title, nil
- case "format":
- return schema.Format, nil
- case "description":
- return schema.Description, nil
- case "enum":
- return schema.Enum, nil
- case "default":
- return schema.Default, nil
- case "example":
- return schema.Example, nil
- case "externalDocs":
- return schema.ExternalDocs, nil
- case "uniqueItems":
- return schema.UniqueItems, nil
- case "exclusiveMin":
- return schema.ExclusiveMin, nil
- case "exclusiveMax":
- return schema.ExclusiveMax, nil
- case "nullable":
- return schema.Nullable, nil
- case "readOnly":
- return schema.ReadOnly, nil
- case "writeOnly":
- return schema.WriteOnly, nil
- case "allowEmptyValue":
- return schema.AllowEmptyValue, nil
- case "xml":
- return schema.XML, nil
- case "deprecated":
- return schema.Deprecated, nil
- case "min":
- return schema.Min, nil
- case "max":
- return schema.Max, nil
- case "multipleOf":
- return schema.MultipleOf, nil
- case "minLength":
- return schema.MinLength, nil
- case "maxLength":
- return schema.MaxLength, nil
- case "pattern":
- return schema.Pattern, nil
- case "minItems":
- return schema.MinItems, nil
- case "maxItems":
- return schema.MaxItems, nil
- case "required":
- return schema.Required, nil
- case "properties":
- return schema.Properties, nil
- case "minProps":
- return schema.MinProps, nil
- case "maxProps":
- return schema.MaxProps, nil
- case "discriminator":
- return schema.Discriminator, nil
- }
-
- v, _, err := jsonpointer.GetForToken(schema.Extensions, token)
- return v, err
-}
-
-func (schema *Schema) NewRef() *SchemaRef {
- return &SchemaRef{
- Value: schema,
- }
-}
-
-func NewOneOfSchema(schemas ...*Schema) *Schema {
- refs := make([]*SchemaRef, 0, len(schemas))
- for _, schema := range schemas {
- refs = append(refs, &SchemaRef{Value: schema})
- }
- return &Schema{
- OneOf: refs,
- }
-}
-
-func NewAnyOfSchema(schemas ...*Schema) *Schema {
- refs := make([]*SchemaRef, 0, len(schemas))
- for _, schema := range schemas {
- refs = append(refs, &SchemaRef{Value: schema})
- }
- return &Schema{
- AnyOf: refs,
- }
-}
-
-func NewAllOfSchema(schemas ...*Schema) *Schema {
- refs := make([]*SchemaRef, 0, len(schemas))
- for _, schema := range schemas {
- refs = append(refs, &SchemaRef{Value: schema})
- }
- return &Schema{
- AllOf: refs,
- }
-}
-
-func NewBoolSchema() *Schema {
- return &Schema{
- Type: TypeBoolean,
- }
-}
-
-func NewFloat64Schema() *Schema {
- return &Schema{
- Type: TypeNumber,
- }
-}
-
-func NewIntegerSchema() *Schema {
- return &Schema{
- Type: TypeInteger,
- }
-}
-
-func NewInt32Schema() *Schema {
- return &Schema{
- Type: TypeInteger,
- Format: "int32",
- }
-}
-
-func NewInt64Schema() *Schema {
- return &Schema{
- Type: TypeInteger,
- Format: "int64",
- }
-}
-
-func NewStringSchema() *Schema {
- return &Schema{
- Type: TypeString,
- }
-}
-
-func NewDateTimeSchema() *Schema {
- return &Schema{
- Type: TypeString,
- Format: "date-time",
- }
-}
-
-func NewUUIDSchema() *Schema {
- return &Schema{
- Type: TypeString,
- Format: "uuid",
- }
-}
-
-func NewBytesSchema() *Schema {
- return &Schema{
- Type: TypeString,
- Format: "byte",
- }
-}
-
-func NewArraySchema() *Schema {
- return &Schema{
- Type: TypeArray,
- }
-}
-
-func NewObjectSchema() *Schema {
- return &Schema{
- Type: TypeObject,
- Properties: make(Schemas),
- }
-}
-
-func (schema *Schema) WithNullable() *Schema {
- schema.Nullable = true
- return schema
-}
-
-func (schema *Schema) WithMin(value float64) *Schema {
- schema.Min = &value
- return schema
-}
-
-func (schema *Schema) WithMax(value float64) *Schema {
- schema.Max = &value
- return schema
-}
-
-func (schema *Schema) WithExclusiveMin(value bool) *Schema {
- schema.ExclusiveMin = value
- return schema
-}
-
-func (schema *Schema) WithExclusiveMax(value bool) *Schema {
- schema.ExclusiveMax = value
- return schema
-}
-
-func (schema *Schema) WithEnum(values ...interface{}) *Schema {
- schema.Enum = values
- return schema
-}
-
-func (schema *Schema) WithDefault(defaultValue interface{}) *Schema {
- schema.Default = defaultValue
- return schema
-}
-
-func (schema *Schema) WithFormat(value string) *Schema {
- schema.Format = value
- return schema
-}
-
-func (schema *Schema) WithLength(i int64) *Schema {
- n := uint64(i)
- schema.MinLength = n
- schema.MaxLength = &n
- return schema
-}
-
-func (schema *Schema) WithMinLength(i int64) *Schema {
- n := uint64(i)
- schema.MinLength = n
- return schema
-}
-
-func (schema *Schema) WithMaxLength(i int64) *Schema {
- n := uint64(i)
- schema.MaxLength = &n
- return schema
-}
-
-func (schema *Schema) WithLengthDecodedBase64(i int64) *Schema {
- n := uint64(i)
- v := (n*8 + 5) / 6
- schema.MinLength = v
- schema.MaxLength = &v
- return schema
-}
-
-func (schema *Schema) WithMinLengthDecodedBase64(i int64) *Schema {
- n := uint64(i)
- schema.MinLength = (n*8 + 5) / 6
- return schema
-}
-
-func (schema *Schema) WithMaxLengthDecodedBase64(i int64) *Schema {
- n := uint64(i)
- schema.MinLength = (n*8 + 5) / 6
- return schema
-}
-
-func (schema *Schema) WithPattern(pattern string) *Schema {
- schema.Pattern = pattern
- schema.compiledPattern = nil
- return schema
-}
-
-func (schema *Schema) WithItems(value *Schema) *Schema {
- schema.Items = &SchemaRef{
- Value: value,
- }
- return schema
-}
-
-func (schema *Schema) WithMinItems(i int64) *Schema {
- n := uint64(i)
- schema.MinItems = n
- return schema
-}
-
-func (schema *Schema) WithMaxItems(i int64) *Schema {
- n := uint64(i)
- schema.MaxItems = &n
- return schema
-}
-
-func (schema *Schema) WithUniqueItems(unique bool) *Schema {
- schema.UniqueItems = unique
- return schema
-}
-
-func (schema *Schema) WithProperty(name string, propertySchema *Schema) *Schema {
- return schema.WithPropertyRef(name, &SchemaRef{
- Value: propertySchema,
- })
-}
-
-func (schema *Schema) WithPropertyRef(name string, ref *SchemaRef) *Schema {
- properties := schema.Properties
- if properties == nil {
- properties = make(Schemas)
- schema.Properties = properties
- }
- properties[name] = ref
- return schema
-}
-
-func (schema *Schema) WithProperties(properties map[string]*Schema) *Schema {
- result := make(Schemas, len(properties))
- for k, v := range properties {
- result[k] = &SchemaRef{
- Value: v,
- }
- }
- schema.Properties = result
- return schema
-}
-
-func (schema *Schema) WithMinProperties(i int64) *Schema {
- n := uint64(i)
- schema.MinProps = n
- return schema
-}
-
-func (schema *Schema) WithMaxProperties(i int64) *Schema {
- n := uint64(i)
- schema.MaxProps = &n
- return schema
-}
-
-func (schema *Schema) WithAnyAdditionalProperties() *Schema {
- schema.AdditionalProperties = AdditionalProperties{Has: BoolPtr(true)}
- return schema
-}
-
-func (schema *Schema) WithoutAdditionalProperties() *Schema {
- schema.AdditionalProperties = AdditionalProperties{Has: BoolPtr(false)}
- return schema
-}
-
-func (schema *Schema) WithAdditionalProperties(v *Schema) *Schema {
- schema.AdditionalProperties = AdditionalProperties{}
- if v != nil {
- schema.AdditionalProperties.Schema = &SchemaRef{Value: v}
- }
- return schema
-}
-
-// IsEmpty tells whether schema is equivalent to the empty schema `{}`.
-func (schema *Schema) IsEmpty() bool {
- if schema.Type != "" || schema.Format != "" || len(schema.Enum) != 0 ||
- schema.UniqueItems || schema.ExclusiveMin || schema.ExclusiveMax ||
- schema.Nullable || schema.ReadOnly || schema.WriteOnly || schema.AllowEmptyValue ||
- schema.Min != nil || schema.Max != nil || schema.MultipleOf != nil ||
- schema.MinLength != 0 || schema.MaxLength != nil || schema.Pattern != "" ||
- schema.MinItems != 0 || schema.MaxItems != nil ||
- len(schema.Required) != 0 ||
- schema.MinProps != 0 || schema.MaxProps != nil {
- return false
- }
- if n := schema.Not; n != nil && !n.Value.IsEmpty() {
- return false
- }
- if ap := schema.AdditionalProperties.Schema; ap != nil && !ap.Value.IsEmpty() {
- return false
- }
- if apa := schema.AdditionalProperties.Has; apa != nil && !*apa {
- return false
- }
- if items := schema.Items; items != nil && !items.Value.IsEmpty() {
- return false
- }
- for _, s := range schema.Properties {
- if !s.Value.IsEmpty() {
- return false
- }
- }
- for _, s := range schema.OneOf {
- if !s.Value.IsEmpty() {
- return false
- }
- }
- for _, s := range schema.AnyOf {
- if !s.Value.IsEmpty() {
- return false
- }
- }
- for _, s := range schema.AllOf {
- if !s.Value.IsEmpty() {
- return false
- }
- }
- return true
-}
-
-// Validate returns an error if Schema does not comply with the OpenAPI spec.
-func (schema *Schema) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
- return schema.validate(ctx, []*Schema{})
-}
-
-func (schema *Schema) validate(ctx context.Context, stack []*Schema) error {
- validationOpts := getValidationOptions(ctx)
-
- for _, existing := range stack {
- if existing == schema {
- return nil
- }
- }
- stack = append(stack, schema)
-
- if schema.ReadOnly && schema.WriteOnly {
- return errors.New("a property MUST NOT be marked as both readOnly and writeOnly being true")
- }
-
- for _, item := range schema.OneOf {
- v := item.Value
- if v == nil {
- return foundUnresolvedRef(item.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- for _, item := range schema.AnyOf {
- v := item.Value
- if v == nil {
- return foundUnresolvedRef(item.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- for _, item := range schema.AllOf {
- v := item.Value
- if v == nil {
- return foundUnresolvedRef(item.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- if ref := schema.Not; ref != nil {
- v := ref.Value
- if v == nil {
- return foundUnresolvedRef(ref.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- schemaType := schema.Type
- switch schemaType {
- case "":
- case TypeBoolean:
- case TypeNumber:
- if format := schema.Format; len(format) > 0 {
- switch format {
- case "float", "double":
- default:
- if validationOpts.schemaFormatValidationEnabled {
- return unsupportedFormat(format)
- }
- }
- }
- case TypeInteger:
- if format := schema.Format; len(format) > 0 {
- switch format {
- case "int32", "int64":
- default:
- if validationOpts.schemaFormatValidationEnabled {
- return unsupportedFormat(format)
- }
- }
- }
- case TypeString:
- if format := schema.Format; len(format) > 0 {
- switch format {
- // Supported by OpenAPIv3.0.3:
- // https://spec.openapis.org/oas/v3.0.3
- case "byte", "binary", "date", "date-time", "password":
- // In JSON Draft-07 (not validated yet though):
- // https://json-schema.org/draft-07/json-schema-release-notes.html#formats
- case "iri", "iri-reference", "uri-template", "idn-email", "idn-hostname":
- case "json-pointer", "relative-json-pointer", "regex", "time":
- // In JSON Draft 2019-09 (not validated yet though):
- // https://json-schema.org/draft/2019-09/release-notes.html#format-vocabulary
- case "duration", "uuid":
- // Defined in some other specification
- case "email", "hostname", "ipv4", "ipv6", "uri", "uri-reference":
- default:
- // Try to check for custom defined formats
- if _, ok := SchemaStringFormats[format]; !ok && validationOpts.schemaFormatValidationEnabled {
- return unsupportedFormat(format)
- }
- }
- }
- if schema.Pattern != "" && !validationOpts.schemaPatternValidationDisabled {
- if err := schema.compilePattern(); err != nil {
- return err
- }
- }
- case TypeArray:
- if schema.Items == nil {
- return errors.New("when schema type is 'array', schema 'items' must be non-null")
- }
- case TypeObject:
- default:
- return fmt.Errorf("unsupported 'type' value %q", schemaType)
- }
-
- if ref := schema.Items; ref != nil {
- v := ref.Value
- if v == nil {
- return foundUnresolvedRef(ref.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- properties := make([]string, 0, len(schema.Properties))
- for name := range schema.Properties {
- properties = append(properties, name)
- }
- sort.Strings(properties)
- for _, name := range properties {
- ref := schema.Properties[name]
- v := ref.Value
- if v == nil {
- return foundUnresolvedRef(ref.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- if schema.AdditionalProperties.Has != nil && schema.AdditionalProperties.Schema != nil {
- return errors.New("additionalProperties are set to both boolean and schema")
- }
- if ref := schema.AdditionalProperties.Schema; ref != nil {
- v := ref.Value
- if v == nil {
- return foundUnresolvedRef(ref.Ref)
- }
- if err := v.validate(ctx, stack); err != nil {
- return err
- }
- }
-
- if v := schema.ExternalDocs; v != nil {
- if err := v.Validate(ctx); err != nil {
- return fmt.Errorf("invalid external docs: %w", err)
- }
- }
-
- if v := schema.Default; v != nil && !validationOpts.schemaDefaultsValidationDisabled {
- if err := schema.VisitJSON(v); err != nil {
- return fmt.Errorf("invalid default: %w", err)
- }
- }
-
- if x := schema.Example; x != nil && !validationOpts.examplesValidationDisabled {
- if err := validateExampleValue(ctx, x, schema); err != nil {
- return fmt.Errorf("invalid example: %w", err)
- }
- }
-
- return validateExtensions(ctx, schema.Extensions)
-}
-
-func (schema *Schema) IsMatching(value interface{}) bool {
- settings := newSchemaValidationSettings(FailFast())
- return schema.visitJSON(settings, value) == nil
-}
-
-func (schema *Schema) IsMatchingJSONBoolean(value bool) bool {
- settings := newSchemaValidationSettings(FailFast())
- return schema.visitJSON(settings, value) == nil
-}
-
-func (schema *Schema) IsMatchingJSONNumber(value float64) bool {
- settings := newSchemaValidationSettings(FailFast())
- return schema.visitJSON(settings, value) == nil
-}
-
-func (schema *Schema) IsMatchingJSONString(value string) bool {
- settings := newSchemaValidationSettings(FailFast())
- return schema.visitJSON(settings, value) == nil
-}
-
-func (schema *Schema) IsMatchingJSONArray(value []interface{}) bool {
- settings := newSchemaValidationSettings(FailFast())
- return schema.visitJSON(settings, value) == nil
-}
-
-func (schema *Schema) IsMatchingJSONObject(value map[string]interface{}) bool {
- settings := newSchemaValidationSettings(FailFast())
- return schema.visitJSON(settings, value) == nil
-}
-
-func (schema *Schema) VisitJSON(value interface{}, opts ...SchemaValidationOption) error {
- settings := newSchemaValidationSettings(opts...)
- return schema.visitJSON(settings, value)
-}
-
-func (schema *Schema) visitJSON(settings *schemaValidationSettings, value interface{}) (err error) {
- switch value := value.(type) {
- case nil:
- return schema.visitJSONNull(settings)
- case float64:
- if math.IsNaN(value) {
- return ErrSchemaInputNaN
- }
- if math.IsInf(value, 0) {
- return ErrSchemaInputInf
- }
- }
-
- if schema.IsEmpty() {
- return
- }
- if err = schema.visitSetOperations(settings, value); err != nil {
- return
- }
-
- switch value := value.(type) {
- case bool:
- return schema.visitJSONBoolean(settings, value)
- case json.Number:
- valueFloat64, err := value.Float64()
- if err != nil {
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "type",
- Reason: "cannot convert json.Number to float64",
- customizeMessageError: settings.customizeMessageError,
- Origin: err,
- }
- }
- return schema.visitJSONNumber(settings, valueFloat64)
- case int:
- return schema.visitJSONNumber(settings, float64(value))
- case int32:
- return schema.visitJSONNumber(settings, float64(value))
- case int64:
- return schema.visitJSONNumber(settings, float64(value))
- case float64:
- return schema.visitJSONNumber(settings, value)
- case string:
- return schema.visitJSONString(settings, value)
- case []interface{}:
- return schema.visitJSONArray(settings, value)
- case map[string]interface{}:
- return schema.visitJSONObject(settings, value)
- case map[interface{}]interface{}: // for YAML cf. issue #444
- values := make(map[string]interface{}, len(value))
- for key, v := range value {
- if k, ok := key.(string); ok {
- values[k] = v
- }
- }
- if len(value) == len(values) {
- return schema.visitJSONObject(settings, values)
- }
- }
-
- // Catch slice of non-empty interface type
- if reflect.TypeOf(value).Kind() == reflect.Slice {
- valueR := reflect.ValueOf(value)
- newValue := make([]interface{}, 0, valueR.Len())
- for i := 0; i < valueR.Len(); i++ {
- newValue = append(newValue, valueR.Index(i).Interface())
- }
- return schema.visitJSONArray(settings, newValue)
- }
-
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "type",
- Reason: fmt.Sprintf("unhandled value of type %T", value),
- customizeMessageError: settings.customizeMessageError,
- }
-}
-
-func (schema *Schema) visitSetOperations(settings *schemaValidationSettings, value interface{}) (err error) {
- if enum := schema.Enum; len(enum) != 0 {
- for _, v := range enum {
- switch c := value.(type) {
- case json.Number:
- var f float64
- if f, err = strconv.ParseFloat(c.String(), 64); err != nil {
- return err
- }
- if v == f {
- return
- }
- default:
- if reflect.DeepEqual(v, value) {
- return
- }
- }
- }
- if settings.failfast {
- return errSchema
- }
- allowedValues, _ := json.Marshal(enum)
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "enum",
- Reason: fmt.Sprintf("value is not one of the allowed values %s", string(allowedValues)),
- customizeMessageError: settings.customizeMessageError,
- }
- }
-
- if ref := schema.Not; ref != nil {
- v := ref.Value
- if v == nil {
- return foundUnresolvedRef(ref.Ref)
- }
- if err := v.visitJSON(settings, value); err == nil {
- if settings.failfast {
- return errSchema
- }
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "not",
- customizeMessageError: settings.customizeMessageError,
- }
- }
- }
-
- if v := schema.OneOf; len(v) > 0 {
- var discriminatorRef string
- if schema.Discriminator != nil {
- pn := schema.Discriminator.PropertyName
- if valuemap, okcheck := value.(map[string]interface{}); okcheck {
- discriminatorVal, okcheck := valuemap[pn]
- if !okcheck {
- return &SchemaError{
- Schema: schema,
- SchemaField: "discriminator",
- Reason: fmt.Sprintf("input does not contain the discriminator property %q", pn),
- }
- }
-
- discriminatorValString, okcheck := discriminatorVal.(string)
- if !okcheck {
- return &SchemaError{
- Value: discriminatorVal,
- Schema: schema,
- SchemaField: "discriminator",
- Reason: fmt.Sprintf("value of discriminator property %q is not a string", pn),
- }
- }
-
- if discriminatorRef, okcheck = schema.Discriminator.Mapping[discriminatorValString]; len(schema.Discriminator.Mapping) > 0 && !okcheck {
- return &SchemaError{
- Value: discriminatorVal,
- Schema: schema,
- SchemaField: "discriminator",
- Reason: fmt.Sprintf("discriminator property %q has invalid value", pn),
- }
- }
- }
- }
-
- var (
- ok = 0
- validationErrors = multiErrorForOneOf{}
- matchedOneOfIndices = make([]int, 0)
- tempValue = value
- )
- for idx, item := range v {
- v := item.Value
- if v == nil {
- return foundUnresolvedRef(item.Ref)
- }
-
- if discriminatorRef != "" && discriminatorRef != item.Ref {
- continue
- }
-
- // make a deep copy to protect origin value from being injected default value that defined in mismatched oneOf schema
- if settings.asreq || settings.asrep {
- tempValue = deepcopy.Copy(value)
- }
-
- if err := v.visitJSON(settings, tempValue); err != nil {
- validationErrors = append(validationErrors, err)
- continue
- }
-
- matchedOneOfIndices = append(matchedOneOfIndices, idx)
- ok++
- }
-
- if ok != 1 {
- if settings.failfast {
- return errSchema
- }
- e := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "oneOf",
- customizeMessageError: settings.customizeMessageError,
- }
- if ok > 1 {
- e.Origin = ErrOneOfConflict
- e.Reason = fmt.Sprintf(`value matches more than one schema from "oneOf" (matches schemas at indices %v)`, matchedOneOfIndices)
- } else {
- e.Origin = fmt.Errorf("doesn't match schema due to: %w", validationErrors)
- e.Reason = `value doesn't match any schema from "oneOf"`
- }
-
- return e
- }
-
- // run again to inject default value that defined in matched oneOf schema
- if settings.asreq || settings.asrep {
- _ = v[matchedOneOfIndices[0]].Value.visitJSON(settings, value)
- }
- }
-
- if v := schema.AnyOf; len(v) > 0 {
- var (
- ok = false
- matchedAnyOfIdx = 0
- tempValue = value
- )
- for idx, item := range v {
- v := item.Value
- if v == nil {
- return foundUnresolvedRef(item.Ref)
- }
- // make a deep copy to protect origin value from being injected default value that defined in mismatched anyOf schema
- if settings.asreq || settings.asrep {
- tempValue = deepcopy.Copy(value)
- }
- if err := v.visitJSON(settings, tempValue); err == nil {
- ok = true
- matchedAnyOfIdx = idx
- break
- }
- }
- if !ok {
- if settings.failfast {
- return errSchema
- }
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "anyOf",
- Reason: `doesn't match any schema from "anyOf"`,
- customizeMessageError: settings.customizeMessageError,
- }
- }
-
- _ = v[matchedAnyOfIdx].Value.visitJSON(settings, value)
- }
-
- for _, item := range schema.AllOf {
- v := item.Value
- if v == nil {
- return foundUnresolvedRef(item.Ref)
- }
- if err := v.visitJSON(settings, value); err != nil {
- if settings.failfast {
- return errSchema
- }
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "allOf",
- Reason: `doesn't match all schemas from "allOf"`,
- Origin: err,
- customizeMessageError: settings.customizeMessageError,
- }
- }
- }
- return
-}
-
-// The value is not considered in visitJSONNull because according to the spec
-// "null is not supported as a type" unless `nullable` is also set to true
-// https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#data-types
-// https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#schema-object
-func (schema *Schema) visitJSONNull(settings *schemaValidationSettings) (err error) {
- if schema.Nullable {
- return
- }
- if settings.failfast {
- return errSchema
- }
- return &SchemaError{
- Value: nil,
- Schema: schema,
- SchemaField: "nullable",
- Reason: "Value is not nullable",
- customizeMessageError: settings.customizeMessageError,
- }
-}
-
-func (schema *Schema) VisitJSONBoolean(value bool) error {
- settings := newSchemaValidationSettings()
- return schema.visitJSONBoolean(settings, value)
-}
-
-func (schema *Schema) visitJSONBoolean(settings *schemaValidationSettings, value bool) (err error) {
- if schemaType := schema.Type; schemaType != "" && schemaType != TypeBoolean {
- return schema.expectedType(settings, value)
- }
- return
-}
-
-func (schema *Schema) VisitJSONNumber(value float64) error {
- settings := newSchemaValidationSettings()
- return schema.visitJSONNumber(settings, value)
-}
-
-func (schema *Schema) visitJSONNumber(settings *schemaValidationSettings, value float64) error {
- var me MultiError
- schemaType := schema.Type
- if schemaType == TypeInteger {
- if bigFloat := big.NewFloat(value); !bigFloat.IsInt() {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "type",
- Reason: fmt.Sprintf("value must be an integer"),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- } else if schemaType != "" && schemaType != TypeNumber {
- return schema.expectedType(settings, value)
- }
-
- // formats
- if schemaType == TypeInteger && schema.Format != "" {
- formatMin := float64(0)
- formatMax := float64(0)
- switch schema.Format {
- case "int32":
- formatMin = formatMinInt32
- formatMax = formatMaxInt32
- case "int64":
- formatMin = formatMinInt64
- formatMax = formatMaxInt64
- default:
- if settings.formatValidationEnabled {
- return unsupportedFormat(schema.Format)
- }
- }
- if formatMin != 0 && formatMax != 0 && !(formatMin <= value && value <= formatMax) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "format",
- Reason: fmt.Sprintf("number must be an %s", schema.Format),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- }
-
- // "exclusiveMinimum"
- if v := schema.ExclusiveMin; v && !(*schema.Min < value) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "exclusiveMinimum",
- Reason: fmt.Sprintf("number must be more than %g", *schema.Min),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "exclusiveMaximum"
- if v := schema.ExclusiveMax; v && !(*schema.Max > value) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "exclusiveMaximum",
- Reason: fmt.Sprintf("number must be less than %g", *schema.Max),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "minimum"
- if v := schema.Min; v != nil && !(*v <= value) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "minimum",
- Reason: fmt.Sprintf("number must be at least %g", *v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "maximum"
- if v := schema.Max; v != nil && !(*v >= value) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "maximum",
- Reason: fmt.Sprintf("number must be at most %g", *v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "multipleOf"
- if v := schema.MultipleOf; v != nil {
- // "A numeric instance is valid only if division by this keyword's
- // value results in an integer."
- if bigFloat := big.NewFloat(value / *v); !bigFloat.IsInt() {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "multipleOf",
- Reason: fmt.Sprintf("number must be a multiple of %g", *v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- }
-
- if len(me) > 0 {
- return me
- }
-
- return nil
-}
-
-func (schema *Schema) VisitJSONString(value string) error {
- settings := newSchemaValidationSettings()
- return schema.visitJSONString(settings, value)
-}
-
-func (schema *Schema) visitJSONString(settings *schemaValidationSettings, value string) error {
- if schemaType := schema.Type; schemaType != "" && schemaType != TypeString {
- return schema.expectedType(settings, value)
- }
-
- var me MultiError
-
- // "minLength" and "maxLength"
- minLength := schema.MinLength
- maxLength := schema.MaxLength
- if minLength != 0 || maxLength != nil {
- // JSON schema string lengths are UTF-16, not UTF-8!
- length := int64(0)
- for _, r := range value {
- if utf16.IsSurrogate(r) {
- length += 2
- } else {
- length++
- }
- }
- if minLength != 0 && length < int64(minLength) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "minLength",
- Reason: fmt.Sprintf("minimum string length is %d", minLength),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- if maxLength != nil && length > int64(*maxLength) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "maxLength",
- Reason: fmt.Sprintf("maximum string length is %d", *maxLength),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- }
-
- // "pattern"
- if schema.Pattern != "" && schema.compiledPattern == nil && !settings.patternValidationDisabled {
- var err error
- if err = schema.compilePattern(); err != nil {
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- }
- if cp := schema.compiledPattern; cp != nil && !cp.MatchString(value) {
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "pattern",
- Reason: fmt.Sprintf(`string doesn't match the regular expression "%s"`, schema.Pattern),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "format"
- var formatStrErr string
- var formatErr error
- if format := schema.Format; format != "" {
- if f, ok := SchemaStringFormats[format]; ok {
- switch {
- case f.regexp != nil && f.callback == nil:
- if cp := f.regexp; !cp.MatchString(value) {
- formatStrErr = fmt.Sprintf(`string doesn't match the format %q (regular expression "%s")`, format, cp.String())
- }
- case f.regexp == nil && f.callback != nil:
- if err := f.callback(value); err != nil {
- var schemaErr = &SchemaError{}
- if errors.As(err, &schemaErr) {
- formatStrErr = fmt.Sprintf(`string doesn't match the format %q (%s)`, format, schemaErr.Reason)
- } else {
- formatStrErr = fmt.Sprintf(`string doesn't match the format %q (%v)`, format, err)
- }
- formatErr = err
- }
- default:
- formatStrErr = fmt.Sprintf("corrupted entry %q in SchemaStringFormats", format)
- }
- }
- }
- if formatStrErr != "" || formatErr != nil {
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "format",
- Reason: formatStrErr,
- Origin: formatErr,
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
-
- }
-
- if len(me) > 0 {
- return me
- }
-
- return nil
-}
-
-func (schema *Schema) VisitJSONArray(value []interface{}) error {
- settings := newSchemaValidationSettings()
- return schema.visitJSONArray(settings, value)
-}
-
-func (schema *Schema) visitJSONArray(settings *schemaValidationSettings, value []interface{}) error {
- if schemaType := schema.Type; schemaType != "" && schemaType != TypeArray {
- return schema.expectedType(settings, value)
- }
-
- var me MultiError
-
- lenValue := int64(len(value))
-
- // "minItems"
- if v := schema.MinItems; v != 0 && lenValue < int64(v) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "minItems",
- Reason: fmt.Sprintf("minimum number of items is %d", v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "maxItems"
- if v := schema.MaxItems; v != nil && lenValue > int64(*v) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "maxItems",
- Reason: fmt.Sprintf("maximum number of items is %d", *v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "uniqueItems"
- if sliceUniqueItemsChecker == nil {
- sliceUniqueItemsChecker = isSliceOfUniqueItems
- }
- if v := schema.UniqueItems; v && !sliceUniqueItemsChecker(value) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "uniqueItems",
- Reason: "duplicate items found",
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "items"
- if itemSchemaRef := schema.Items; itemSchemaRef != nil {
- itemSchema := itemSchemaRef.Value
- if itemSchema == nil {
- return foundUnresolvedRef(itemSchemaRef.Ref)
- }
- for i, item := range value {
- if err := itemSchema.visitJSON(settings, item); err != nil {
- err = markSchemaErrorIndex(err, i)
- if !settings.multiError {
- return err
- }
- if itemMe, ok := err.(MultiError); ok {
- me = append(me, itemMe...)
- } else {
- me = append(me, err)
- }
- }
- }
- }
-
- if len(me) > 0 {
- return me
- }
-
- return nil
-}
-
-func (schema *Schema) VisitJSONObject(value map[string]interface{}) error {
- settings := newSchemaValidationSettings()
- return schema.visitJSONObject(settings, value)
-}
-
-func (schema *Schema) visitJSONObject(settings *schemaValidationSettings, value map[string]interface{}) error {
- if schemaType := schema.Type; schemaType != "" && schemaType != TypeObject {
- return schema.expectedType(settings, value)
- }
-
- var me MultiError
-
- if settings.asreq || settings.asrep {
- properties := make([]string, 0, len(schema.Properties))
- for propName := range schema.Properties {
- properties = append(properties, propName)
- }
- sort.Strings(properties)
- for _, propName := range properties {
- propSchema := schema.Properties[propName]
- reqRO := settings.asreq && propSchema.Value.ReadOnly && !settings.readOnlyValidationDisabled
- repWO := settings.asrep && propSchema.Value.WriteOnly && !settings.writeOnlyValidationDisabled
-
- if value[propName] == nil {
- if dlft := propSchema.Value.Default; dlft != nil && !reqRO && !repWO {
- value[propName] = dlft
- if f := settings.defaultsSet; f != nil {
- settings.onceSettingDefaults.Do(f)
- }
- }
- }
-
- if value[propName] != nil {
- if reqRO {
- me = append(me, fmt.Errorf("readOnly property %q in request", propName))
- } else if repWO {
- me = append(me, fmt.Errorf("writeOnly property %q in response", propName))
- }
- }
- }
- }
-
- // "properties"
- properties := schema.Properties
- lenValue := int64(len(value))
-
- // "minProperties"
- if v := schema.MinProps; v != 0 && lenValue < int64(v) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "minProperties",
- Reason: fmt.Sprintf("there must be at least %d properties", v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "maxProperties"
- if v := schema.MaxProps; v != nil && lenValue > int64(*v) {
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "maxProperties",
- Reason: fmt.Sprintf("there must be at most %d properties", *v),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "additionalProperties"
- var additionalProperties *Schema
- if ref := schema.AdditionalProperties.Schema; ref != nil {
- additionalProperties = ref.Value
- }
- keys := make([]string, 0, len(value))
- for k := range value {
- keys = append(keys, k)
- }
- sort.Strings(keys)
- for _, k := range keys {
- v := value[k]
- if properties != nil {
- propertyRef := properties[k]
- if propertyRef != nil {
- p := propertyRef.Value
- if p == nil {
- return foundUnresolvedRef(propertyRef.Ref)
- }
- if err := p.visitJSON(settings, v); err != nil {
- if settings.failfast {
- return errSchema
- }
- err = markSchemaErrorKey(err, k)
- if !settings.multiError {
- return err
- }
- if v, ok := err.(MultiError); ok {
- me = append(me, v...)
- continue
- }
- me = append(me, err)
- }
- continue
- }
- }
- if allowed := schema.AdditionalProperties.Has; allowed == nil || *allowed {
- if additionalProperties != nil {
- if err := additionalProperties.visitJSON(settings, v); err != nil {
- if settings.failfast {
- return errSchema
- }
- err = markSchemaErrorKey(err, k)
- if !settings.multiError {
- return err
- }
- if v, ok := err.(MultiError); ok {
- me = append(me, v...)
- continue
- }
- me = append(me, err)
- }
- }
- continue
- }
- if settings.failfast {
- return errSchema
- }
- err := &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "properties",
- Reason: fmt.Sprintf("property %q is unsupported", k),
- customizeMessageError: settings.customizeMessageError,
- }
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
-
- // "required"
- for _, k := range schema.Required {
- if _, ok := value[k]; !ok {
- if s := schema.Properties[k]; s != nil && s.Value.ReadOnly && settings.asreq {
- continue
- }
- if s := schema.Properties[k]; s != nil && s.Value.WriteOnly && settings.asrep {
- continue
- }
- if settings.failfast {
- return errSchema
- }
- err := markSchemaErrorKey(&SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "required",
- Reason: fmt.Sprintf("property %q is missing", k),
- customizeMessageError: settings.customizeMessageError,
- }, k)
- if !settings.multiError {
- return err
- }
- me = append(me, err)
- }
- }
-
- if len(me) > 0 {
- return me
- }
-
- return nil
-}
-
-func (schema *Schema) expectedType(settings *schemaValidationSettings, value interface{}) error {
- if settings.failfast {
- return errSchema
- }
-
- a := "a"
- switch schema.Type {
- case TypeArray, TypeObject, TypeInteger:
- a = "an"
- }
- return &SchemaError{
- Value: value,
- Schema: schema,
- SchemaField: "type",
- Reason: fmt.Sprintf("value must be %s %s", a, schema.Type),
- customizeMessageError: settings.customizeMessageError,
- }
-}
-
-func (schema *Schema) compilePattern() (err error) {
- if schema.compiledPattern, err = regexp.Compile(schema.Pattern); err != nil {
- return &SchemaError{
- Schema: schema,
- SchemaField: "pattern",
- Origin: err,
- Reason: fmt.Sprintf("cannot compile pattern %q: %v", schema.Pattern, err),
- }
- }
- return nil
-}
-
-// SchemaError is an error that occurs during schema validation.
-type SchemaError struct {
- // Value is the value that failed validation.
- Value interface{}
- // reversePath is the path to the value that failed validation.
- reversePath []string
- // Schema is the schema that failed validation.
- Schema *Schema
- // SchemaField is the field of the schema that failed validation.
- SchemaField string
- // Reason is a human-readable message describing the error.
- // The message should never include the original value to prevent leakage of potentially sensitive inputs in error messages.
- Reason string
- // Origin is the original error that caused this error.
- Origin error
- // customizeMessageError is a function that can be used to customize the error message.
- customizeMessageError func(err *SchemaError) string
-}
-
-var _ interface{ Unwrap() error } = SchemaError{}
-
-func markSchemaErrorKey(err error, key string) error {
- var me multiErrorForOneOf
-
- if errors.As(err, &me) {
- err = me.Unwrap()
- }
-
- if v, ok := err.(*SchemaError); ok {
- v.reversePath = append(v.reversePath, key)
- return v
- }
- if v, ok := err.(MultiError); ok {
- for _, e := range v {
- _ = markSchemaErrorKey(e, key)
- }
- return v
- }
- return err
-}
-
-func markSchemaErrorIndex(err error, index int) error {
- return markSchemaErrorKey(err, strconv.FormatInt(int64(index), 10))
-}
-
-func (err *SchemaError) JSONPointer() []string {
- reversePath := err.reversePath
- path := append([]string(nil), reversePath...)
- for left, right := 0, len(path)-1; left < right; left, right = left+1, right-1 {
- path[left], path[right] = path[right], path[left]
- }
- return path
-}
-
-func (err *SchemaError) Error() string {
- if err.customizeMessageError != nil {
- if msg := err.customizeMessageError(err); msg != "" {
- return msg
- }
- }
-
- buf := bytes.NewBuffer(make([]byte, 0, 256))
-
- if len(err.reversePath) > 0 {
- buf.WriteString(`Error at "`)
- reversePath := err.reversePath
- for i := len(reversePath) - 1; i >= 0; i-- {
- buf.WriteByte('/')
- buf.WriteString(reversePath[i])
- }
- buf.WriteString(`": `)
- }
-
- if err.Origin != nil {
- buf.WriteString(err.Origin.Error())
-
- return buf.String()
- }
-
- reason := err.Reason
- if reason == "" {
- buf.WriteString(`Doesn't match schema "`)
- buf.WriteString(err.SchemaField)
- buf.WriteString(`"`)
- } else {
- buf.WriteString(reason)
- }
-
- if !SchemaErrorDetailsDisabled {
- buf.WriteString("\nSchema:\n ")
- encoder := json.NewEncoder(buf)
- encoder.SetIndent(" ", " ")
- if err := encoder.Encode(err.Schema); err != nil {
- panic(err)
- }
- buf.WriteString("\nValue:\n ")
- if err := encoder.Encode(err.Value); err != nil {
- panic(err)
- }
- }
-
- return buf.String()
-}
-
-func (err SchemaError) Unwrap() error {
- return err.Origin
-}
-
-func isSliceOfUniqueItems(xs []interface{}) bool {
- s := len(xs)
- m := make(map[string]struct{}, s)
- for _, x := range xs {
- // The input slice is coverted from a JSON string, there shall
- // have no error when covert it back.
- key, _ := json.Marshal(&x)
- m[string(key)] = struct{}{}
- }
- return s == len(m)
-}
-
-// SliceUniqueItemsChecker is an function used to check if an given slice
-// have unique items.
-type SliceUniqueItemsChecker func(items []interface{}) bool
-
-// By default using predefined func isSliceOfUniqueItems which make use of
-// json.Marshal to generate a key for map used to check if a given slice
-// have unique items.
-var sliceUniqueItemsChecker SliceUniqueItemsChecker = isSliceOfUniqueItems
-
-// RegisterArrayUniqueItemsChecker is used to register a customized function
-// used to check if JSON array have unique items.
-func RegisterArrayUniqueItemsChecker(fn SliceUniqueItemsChecker) {
- sliceUniqueItemsChecker = fn
-}
-
-func unsupportedFormat(format string) error {
- return fmt.Errorf("unsupported 'format' value %q", format)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/schema_formats.go b/vendor/github.com/getkin/kin-openapi/openapi3/schema_formats.go
deleted file mode 100644
index ecbc0ebf..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/schema_formats.go
+++ /dev/null
@@ -1,106 +0,0 @@
-package openapi3
-
-import (
- "fmt"
- "net"
- "regexp"
- "strings"
-)
-
-const (
- // FormatOfStringForUUIDOfRFC4122 is an optional predefined format for UUID v1-v5 as specified by RFC4122
- FormatOfStringForUUIDOfRFC4122 = `^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[1-5][0-9a-fA-F]{3}-[89abAB][0-9a-fA-F]{3}-[0-9a-fA-F]{12}$`
-
- // FormatOfStringForEmail pattern catches only some suspiciously wrong-looking email addresses.
- // Use DefineStringFormat(...) if you need something stricter.
- FormatOfStringForEmail = `^[^@]+@[^@<>",\s]+$`
-)
-
-// FormatCallback performs custom checks on exotic formats
-type FormatCallback func(value string) error
-
-// Format represents a format validator registered by either DefineStringFormat or DefineStringFormatCallback
-type Format struct {
- regexp *regexp.Regexp
- callback FormatCallback
-}
-
-// SchemaStringFormats allows for validating string formats
-var SchemaStringFormats = make(map[string]Format, 4)
-
-// DefineStringFormat defines a new regexp pattern for a given format
-func DefineStringFormat(name string, pattern string) {
- re, err := regexp.Compile(pattern)
- if err != nil {
- err := fmt.Errorf("format %q has invalid pattern %q: %w", name, pattern, err)
- panic(err)
- }
- SchemaStringFormats[name] = Format{regexp: re}
-}
-
-// DefineStringFormatCallback adds a validation function for a specific schema format entry
-func DefineStringFormatCallback(name string, callback FormatCallback) {
- SchemaStringFormats[name] = Format{callback: callback}
-}
-
-func validateIP(ip string) error {
- parsed := net.ParseIP(ip)
- if parsed == nil {
- return &SchemaError{
- Value: ip,
- Reason: "Not an IP address",
- }
- }
- return nil
-}
-
-func validateIPv4(ip string) error {
- if err := validateIP(ip); err != nil {
- return err
- }
-
- if !(strings.Count(ip, ":") < 2) {
- return &SchemaError{
- Value: ip,
- Reason: "Not an IPv4 address (it's IPv6)",
- }
- }
- return nil
-}
-
-func validateIPv6(ip string) error {
- if err := validateIP(ip); err != nil {
- return err
- }
-
- if !(strings.Count(ip, ":") >= 2) {
- return &SchemaError{
- Value: ip,
- Reason: "Not an IPv6 address (it's IPv4)",
- }
- }
- return nil
-}
-
-func init() {
- // Base64
- // The pattern supports base64 and b./ase64url. Padding ('=') is supported.
- DefineStringFormat("byte", `(^$|^[a-zA-Z0-9+/\-_]*=*$)`)
-
- // date
- DefineStringFormat("date", `^[0-9]{4}-(0[0-9]|10|11|12)-([0-2][0-9]|30|31)$`)
-
- // date-time
- DefineStringFormat("date-time", `^[0-9]{4}-(0[0-9]|10|11|12)-([0-2][0-9]|30|31)T[0-9]{2}:[0-9]{2}:[0-9]{2}(\.[0-9]+)?(Z|(\+|-)[0-9]{2}:[0-9]{2})?$`)
-
-}
-
-// DefineIPv4Format opts in ipv4 format validation on top of OAS 3 spec
-func DefineIPv4Format() {
- DefineStringFormatCallback("ipv4", validateIPv4)
-}
-
-// DefineIPv6Format opts in ipv6 format validation on top of OAS 3 spec
-func DefineIPv6Format() {
- DefineStringFormatCallback("ipv6", validateIPv6)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/schema_validation_settings.go b/vendor/github.com/getkin/kin-openapi/openapi3/schema_validation_settings.go
deleted file mode 100644
index 17aad2fa..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/schema_validation_settings.go
+++ /dev/null
@@ -1,79 +0,0 @@
-package openapi3
-
-import (
- "sync"
-)
-
-// SchemaValidationOption describes options a user has when validating request / response bodies.
-type SchemaValidationOption func(*schemaValidationSettings)
-
-type schemaValidationSettings struct {
- failfast bool
- multiError bool
- asreq, asrep bool // exclusive (XOR) fields
- formatValidationEnabled bool
- patternValidationDisabled bool
- readOnlyValidationDisabled bool
- writeOnlyValidationDisabled bool
-
- onceSettingDefaults sync.Once
- defaultsSet func()
-
- customizeMessageError func(err *SchemaError) string
-}
-
-// FailFast returns schema validation errors quicker.
-func FailFast() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.failfast = true }
-}
-
-func MultiErrors() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.multiError = true }
-}
-
-func VisitAsRequest() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.asreq, s.asrep = true, false }
-}
-
-func VisitAsResponse() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.asreq, s.asrep = false, true }
-}
-
-// EnableFormatValidation setting makes Validate not return an error when validating documents that mention schema formats that are not defined by the OpenAPIv3 specification.
-func EnableFormatValidation() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.formatValidationEnabled = true }
-}
-
-// DisablePatternValidation setting makes Validate not return an error when validating patterns that are not supported by the Go regexp engine.
-func DisablePatternValidation() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.patternValidationDisabled = true }
-}
-
-// DisableReadOnlyValidation setting makes Validate not return an error when validating properties marked as read-only
-func DisableReadOnlyValidation() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.readOnlyValidationDisabled = true }
-}
-
-// DisableWriteOnlyValidation setting makes Validate not return an error when validating properties marked as write-only
-func DisableWriteOnlyValidation() SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.writeOnlyValidationDisabled = true }
-}
-
-// DefaultsSet executes the given callback (once) IFF schema validation set default values.
-func DefaultsSet(f func()) SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.defaultsSet = f }
-}
-
-// SetSchemaErrorMessageCustomizer allows to override the schema error message.
-// If the passed function returns an empty string, it returns to the previous Error() implementation.
-func SetSchemaErrorMessageCustomizer(f func(err *SchemaError) string) SchemaValidationOption {
- return func(s *schemaValidationSettings) { s.customizeMessageError = f }
-}
-
-func newSchemaValidationSettings(opts ...SchemaValidationOption) *schemaValidationSettings {
- settings := &schemaValidationSettings{}
- for _, opt := range opts {
- opt(settings)
- }
- return settings
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/security_requirements.go b/vendor/github.com/getkin/kin-openapi/openapi3/security_requirements.go
deleted file mode 100644
index 87891c95..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/security_requirements.go
+++ /dev/null
@@ -1,51 +0,0 @@
-package openapi3
-
-import (
- "context"
-)
-
-type SecurityRequirements []SecurityRequirement
-
-func NewSecurityRequirements() *SecurityRequirements {
- return &SecurityRequirements{}
-}
-
-func (srs *SecurityRequirements) With(securityRequirement SecurityRequirement) *SecurityRequirements {
- *srs = append(*srs, securityRequirement)
- return srs
-}
-
-// Validate returns an error if SecurityRequirements does not comply with the OpenAPI spec.
-func (srs SecurityRequirements) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- for _, security := range srs {
- if err := security.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
-
-// SecurityRequirement is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#security-requirement-object
-type SecurityRequirement map[string][]string
-
-func NewSecurityRequirement() SecurityRequirement {
- return make(SecurityRequirement)
-}
-
-func (security SecurityRequirement) Authenticate(provider string, scopes ...string) SecurityRequirement {
- if len(scopes) == 0 {
- scopes = []string{} // Forces the variable to be encoded as an array instead of null
- }
- security[provider] = scopes
- return security
-}
-
-// Validate returns an error if SecurityRequirement does not comply with the OpenAPI spec.
-func (security *SecurityRequirement) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/security_scheme.go b/vendor/github.com/getkin/kin-openapi/openapi3/security_scheme.go
deleted file mode 100644
index 76cc21f3..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/security_scheme.go
+++ /dev/null
@@ -1,412 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "net/url"
-
- "github.com/go-openapi/jsonpointer"
-)
-
-type SecuritySchemes map[string]*SecuritySchemeRef
-
-// JSONLookup implements github.com/go-openapi/jsonpointer#JSONPointable
-func (s SecuritySchemes) JSONLookup(token string) (interface{}, error) {
- ref, ok := s[token]
- if ref == nil || ok == false {
- return nil, fmt.Errorf("object has no field %q", token)
- }
-
- if ref.Ref != "" {
- return &Ref{Ref: ref.Ref}, nil
- }
- return ref.Value, nil
-}
-
-var _ jsonpointer.JSONPointable = (*SecuritySchemes)(nil)
-
-// SecurityScheme is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#security-scheme-object
-type SecurityScheme struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Type string `json:"type,omitempty" yaml:"type,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Name string `json:"name,omitempty" yaml:"name,omitempty"`
- In string `json:"in,omitempty" yaml:"in,omitempty"`
- Scheme string `json:"scheme,omitempty" yaml:"scheme,omitempty"`
- BearerFormat string `json:"bearerFormat,omitempty" yaml:"bearerFormat,omitempty"`
- Flows *OAuthFlows `json:"flows,omitempty" yaml:"flows,omitempty"`
- OpenIdConnectUrl string `json:"openIdConnectUrl,omitempty" yaml:"openIdConnectUrl,omitempty"`
-}
-
-func NewSecurityScheme() *SecurityScheme {
- return &SecurityScheme{}
-}
-
-func NewCSRFSecurityScheme() *SecurityScheme {
- return &SecurityScheme{
- Type: "apiKey",
- In: "header",
- Name: "X-XSRF-TOKEN",
- }
-}
-
-func NewOIDCSecurityScheme(oidcUrl string) *SecurityScheme {
- return &SecurityScheme{
- Type: "openIdConnect",
- OpenIdConnectUrl: oidcUrl,
- }
-}
-
-func NewJWTSecurityScheme() *SecurityScheme {
- return &SecurityScheme{
- Type: "http",
- Scheme: "bearer",
- BearerFormat: "JWT",
- }
-}
-
-// MarshalJSON returns the JSON encoding of SecurityScheme.
-func (ss SecurityScheme) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 8+len(ss.Extensions))
- for k, v := range ss.Extensions {
- m[k] = v
- }
- if x := ss.Type; x != "" {
- m["type"] = x
- }
- if x := ss.Description; x != "" {
- m["description"] = x
- }
- if x := ss.Name; x != "" {
- m["name"] = x
- }
- if x := ss.In; x != "" {
- m["in"] = x
- }
- if x := ss.Scheme; x != "" {
- m["scheme"] = x
- }
- if x := ss.BearerFormat; x != "" {
- m["bearerFormat"] = x
- }
- if x := ss.Flows; x != nil {
- m["flows"] = x
- }
- if x := ss.OpenIdConnectUrl; x != "" {
- m["openIdConnectUrl"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets SecurityScheme to a copy of data.
-func (ss *SecurityScheme) UnmarshalJSON(data []byte) error {
- type SecuritySchemeBis SecurityScheme
- var x SecuritySchemeBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "type")
- delete(x.Extensions, "description")
- delete(x.Extensions, "name")
- delete(x.Extensions, "in")
- delete(x.Extensions, "scheme")
- delete(x.Extensions, "bearerFormat")
- delete(x.Extensions, "flows")
- delete(x.Extensions, "openIdConnectUrl")
- *ss = SecurityScheme(x)
- return nil
-}
-
-func (ss *SecurityScheme) WithType(value string) *SecurityScheme {
- ss.Type = value
- return ss
-}
-
-func (ss *SecurityScheme) WithDescription(value string) *SecurityScheme {
- ss.Description = value
- return ss
-}
-
-func (ss *SecurityScheme) WithName(value string) *SecurityScheme {
- ss.Name = value
- return ss
-}
-
-func (ss *SecurityScheme) WithIn(value string) *SecurityScheme {
- ss.In = value
- return ss
-}
-
-func (ss *SecurityScheme) WithScheme(value string) *SecurityScheme {
- ss.Scheme = value
- return ss
-}
-
-func (ss *SecurityScheme) WithBearerFormat(value string) *SecurityScheme {
- ss.BearerFormat = value
- return ss
-}
-
-// Validate returns an error if SecurityScheme does not comply with the OpenAPI spec.
-func (ss *SecurityScheme) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- hasIn := false
- hasBearerFormat := false
- hasFlow := false
- switch ss.Type {
- case "apiKey":
- hasIn = true
- case "http":
- scheme := ss.Scheme
- switch scheme {
- case "bearer":
- hasBearerFormat = true
- case "basic", "negotiate", "digest":
- default:
- return fmt.Errorf("security scheme of type 'http' has invalid 'scheme' value %q", scheme)
- }
- case "oauth2":
- hasFlow = true
- case "openIdConnect":
- if ss.OpenIdConnectUrl == "" {
- return fmt.Errorf("no OIDC URL found for openIdConnect security scheme %q", ss.Name)
- }
- default:
- return fmt.Errorf("security scheme 'type' can't be %q", ss.Type)
- }
-
- // Validate "in" and "name"
- if hasIn {
- switch ss.In {
- case "query", "header", "cookie":
- default:
- return fmt.Errorf("security scheme of type 'apiKey' should have 'in'. It can be 'query', 'header' or 'cookie', not %q", ss.In)
- }
- if ss.Name == "" {
- return errors.New("security scheme of type 'apiKey' should have 'name'")
- }
- } else if len(ss.In) > 0 {
- return fmt.Errorf("security scheme of type %q can't have 'in'", ss.Type)
- } else if len(ss.Name) > 0 {
- return fmt.Errorf("security scheme of type %q can't have 'name'", ss.Type)
- }
-
- // Validate "format"
- // "bearerFormat" is an arbitrary string so we only check if the scheme supports it
- if !hasBearerFormat && len(ss.BearerFormat) > 0 {
- return fmt.Errorf("security scheme of type %q can't have 'bearerFormat'", ss.Type)
- }
-
- // Validate "flow"
- if hasFlow {
- flow := ss.Flows
- if flow == nil {
- return fmt.Errorf("security scheme of type %q should have 'flows'", ss.Type)
- }
- if err := flow.Validate(ctx); err != nil {
- return fmt.Errorf("security scheme 'flow' is invalid: %w", err)
- }
- } else if ss.Flows != nil {
- return fmt.Errorf("security scheme of type %q can't have 'flows'", ss.Type)
- }
-
- return validateExtensions(ctx, ss.Extensions)
-}
-
-// OAuthFlows is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#oauth-flows-object
-type OAuthFlows struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Implicit *OAuthFlow `json:"implicit,omitempty" yaml:"implicit,omitempty"`
- Password *OAuthFlow `json:"password,omitempty" yaml:"password,omitempty"`
- ClientCredentials *OAuthFlow `json:"clientCredentials,omitempty" yaml:"clientCredentials,omitempty"`
- AuthorizationCode *OAuthFlow `json:"authorizationCode,omitempty" yaml:"authorizationCode,omitempty"`
-}
-
-type oAuthFlowType int
-
-const (
- oAuthFlowTypeImplicit oAuthFlowType = iota
- oAuthFlowTypePassword
- oAuthFlowTypeClientCredentials
- oAuthFlowAuthorizationCode
-)
-
-// MarshalJSON returns the JSON encoding of OAuthFlows.
-func (flows OAuthFlows) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(flows.Extensions))
- for k, v := range flows.Extensions {
- m[k] = v
- }
- if x := flows.Implicit; x != nil {
- m["implicit"] = x
- }
- if x := flows.Password; x != nil {
- m["password"] = x
- }
- if x := flows.ClientCredentials; x != nil {
- m["clientCredentials"] = x
- }
- if x := flows.AuthorizationCode; x != nil {
- m["authorizationCode"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets OAuthFlows to a copy of data.
-func (flows *OAuthFlows) UnmarshalJSON(data []byte) error {
- type OAuthFlowsBis OAuthFlows
- var x OAuthFlowsBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "implicit")
- delete(x.Extensions, "password")
- delete(x.Extensions, "clientCredentials")
- delete(x.Extensions, "authorizationCode")
- *flows = OAuthFlows(x)
- return nil
-}
-
-// Validate returns an error if OAuthFlows does not comply with the OpenAPI spec.
-func (flows *OAuthFlows) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if v := flows.Implicit; v != nil {
- if err := v.validate(ctx, oAuthFlowTypeImplicit, opts...); err != nil {
- return fmt.Errorf("the OAuth flow 'implicit' is invalid: %w", err)
- }
- }
-
- if v := flows.Password; v != nil {
- if err := v.validate(ctx, oAuthFlowTypePassword, opts...); err != nil {
- return fmt.Errorf("the OAuth flow 'password' is invalid: %w", err)
- }
- }
-
- if v := flows.ClientCredentials; v != nil {
- if err := v.validate(ctx, oAuthFlowTypeClientCredentials, opts...); err != nil {
- return fmt.Errorf("the OAuth flow 'clientCredentials' is invalid: %w", err)
- }
- }
-
- if v := flows.AuthorizationCode; v != nil {
- if err := v.validate(ctx, oAuthFlowAuthorizationCode, opts...); err != nil {
- return fmt.Errorf("the OAuth flow 'authorizationCode' is invalid: %w", err)
- }
- }
-
- return validateExtensions(ctx, flows.Extensions)
-}
-
-// OAuthFlow is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#oauth-flow-object
-type OAuthFlow struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- AuthorizationURL string `json:"authorizationUrl,omitempty" yaml:"authorizationUrl,omitempty"`
- TokenURL string `json:"tokenUrl,omitempty" yaml:"tokenUrl,omitempty"`
- RefreshURL string `json:"refreshUrl,omitempty" yaml:"refreshUrl,omitempty"`
- Scopes map[string]string `json:"scopes" yaml:"scopes"` // required
-}
-
-// MarshalJSON returns the JSON encoding of OAuthFlow.
-func (flow OAuthFlow) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(flow.Extensions))
- for k, v := range flow.Extensions {
- m[k] = v
- }
- if x := flow.AuthorizationURL; x != "" {
- m["authorizationUrl"] = x
- }
- if x := flow.TokenURL; x != "" {
- m["tokenUrl"] = x
- }
- if x := flow.RefreshURL; x != "" {
- m["refreshUrl"] = x
- }
- m["scopes"] = flow.Scopes
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets OAuthFlow to a copy of data.
-func (flow *OAuthFlow) UnmarshalJSON(data []byte) error {
- type OAuthFlowBis OAuthFlow
- var x OAuthFlowBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "authorizationUrl")
- delete(x.Extensions, "tokenUrl")
- delete(x.Extensions, "refreshUrl")
- delete(x.Extensions, "scopes")
- *flow = OAuthFlow(x)
- return nil
-}
-
-// Validate returns an error if OAuthFlows does not comply with the OpenAPI spec.
-func (flow *OAuthFlow) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if v := flow.RefreshURL; v != "" {
- if _, err := url.Parse(v); err != nil {
- return fmt.Errorf("field 'refreshUrl' is invalid: %w", err)
- }
- }
-
- if flow.Scopes == nil {
- return errors.New("field 'scopes' is missing")
- }
-
- return validateExtensions(ctx, flow.Extensions)
-}
-
-func (flow *OAuthFlow) validate(ctx context.Context, typ oAuthFlowType, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- typeIn := func(types ...oAuthFlowType) bool {
- for _, ty := range types {
- if ty == typ {
- return true
- }
- }
- return false
- }
-
- if in := typeIn(oAuthFlowTypeImplicit, oAuthFlowAuthorizationCode); true {
- switch {
- case flow.AuthorizationURL == "" && in:
- return errors.New("field 'authorizationUrl' is empty or missing")
- case flow.AuthorizationURL != "" && !in:
- return errors.New("field 'authorizationUrl' should not be set")
- case flow.AuthorizationURL != "":
- if _, err := url.Parse(flow.AuthorizationURL); err != nil {
- return fmt.Errorf("field 'authorizationUrl' is invalid: %w", err)
- }
- }
- }
-
- if in := typeIn(oAuthFlowTypePassword, oAuthFlowTypeClientCredentials, oAuthFlowAuthorizationCode); true {
- switch {
- case flow.TokenURL == "" && in:
- return errors.New("field 'tokenUrl' is empty or missing")
- case flow.TokenURL != "" && !in:
- return errors.New("field 'tokenUrl' should not be set")
- case flow.TokenURL != "":
- if _, err := url.Parse(flow.TokenURL); err != nil {
- return fmt.Errorf("field 'tokenUrl' is invalid: %w", err)
- }
- }
- }
-
- return flow.Validate(ctx, opts...)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/serialization_method.go b/vendor/github.com/getkin/kin-openapi/openapi3/serialization_method.go
deleted file mode 100644
index 2ec8bd2d..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/serialization_method.go
+++ /dev/null
@@ -1,17 +0,0 @@
-package openapi3
-
-const (
- SerializationSimple = "simple"
- SerializationLabel = "label"
- SerializationMatrix = "matrix"
- SerializationForm = "form"
- SerializationSpaceDelimited = "spaceDelimited"
- SerializationPipeDelimited = "pipeDelimited"
- SerializationDeepObject = "deepObject"
-)
-
-// SerializationMethod describes a serialization method of HTTP request's parameters and body.
-type SerializationMethod struct {
- Style string
- Explode bool
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/server.go b/vendor/github.com/getkin/kin-openapi/openapi3/server.go
deleted file mode 100644
index 9fc99f90..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/server.go
+++ /dev/null
@@ -1,278 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "errors"
- "fmt"
- "math"
- "net/url"
- "sort"
- "strings"
-)
-
-// Servers is specified by OpenAPI/Swagger standard version 3.
-type Servers []*Server
-
-// Validate returns an error if Servers does not comply with the OpenAPI spec.
-func (servers Servers) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- for _, v := range servers {
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
-
-// BasePath returns the base path of the first server in the list, or /.
-func (servers Servers) BasePath() (string, error) {
- for _, server := range servers {
- return server.BasePath()
- }
- return "/", nil
-}
-
-func (servers Servers) MatchURL(parsedURL *url.URL) (*Server, []string, string) {
- rawURL := parsedURL.String()
- if i := strings.IndexByte(rawURL, '?'); i >= 0 {
- rawURL = rawURL[:i]
- }
- for _, server := range servers {
- pathParams, remaining, ok := server.MatchRawURL(rawURL)
- if ok {
- return server, pathParams, remaining
- }
- }
- return nil, nil, ""
-}
-
-// Server is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#server-object
-type Server struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- URL string `json:"url" yaml:"url"` // Required
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- Variables map[string]*ServerVariable `json:"variables,omitempty" yaml:"variables,omitempty"`
-}
-
-// BasePath returns the base path extracted from the default values of variables, if any.
-// Assumes a valid struct (per Validate()).
-func (server *Server) BasePath() (string, error) {
- if server == nil {
- return "/", nil
- }
-
- uri := server.URL
- for name, svar := range server.Variables {
- uri = strings.ReplaceAll(uri, "{"+name+"}", svar.Default)
- }
-
- u, err := url.ParseRequestURI(uri)
- if err != nil {
- return "", err
- }
-
- if bp := u.Path; bp != "" {
- return bp, nil
- }
-
- return "/", nil
-}
-
-// MarshalJSON returns the JSON encoding of Server.
-func (server Server) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 3+len(server.Extensions))
- for k, v := range server.Extensions {
- m[k] = v
- }
- m["url"] = server.URL
- if x := server.Description; x != "" {
- m["description"] = x
- }
- if x := server.Variables; len(x) != 0 {
- m["variables"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Server to a copy of data.
-func (server *Server) UnmarshalJSON(data []byte) error {
- type ServerBis Server
- var x ServerBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "url")
- delete(x.Extensions, "description")
- delete(x.Extensions, "variables")
- *server = Server(x)
- return nil
-}
-
-func (server Server) ParameterNames() ([]string, error) {
- pattern := server.URL
- var params []string
- for len(pattern) > 0 {
- i := strings.IndexByte(pattern, '{')
- if i < 0 {
- break
- }
- pattern = pattern[i+1:]
- i = strings.IndexByte(pattern, '}')
- if i < 0 {
- return nil, errors.New("missing '}'")
- }
- params = append(params, strings.TrimSpace(pattern[:i]))
- pattern = pattern[i+1:]
- }
- return params, nil
-}
-
-func (server Server) MatchRawURL(input string) ([]string, string, bool) {
- pattern := server.URL
- var params []string
- for len(pattern) > 0 {
- c := pattern[0]
- if len(pattern) == 1 && c == '/' {
- break
- }
- if c == '{' {
- // Find end of pattern
- i := strings.IndexByte(pattern, '}')
- if i < 0 {
- return nil, "", false
- }
- pattern = pattern[i+1:]
-
- // Find next matching pattern character or next '/' whichever comes first
- np := -1
- if len(pattern) > 0 {
- np = strings.IndexByte(input, pattern[0])
- }
- ns := strings.IndexByte(input, '/')
-
- if np < 0 {
- i = ns
- } else if ns < 0 {
- i = np
- } else {
- i = int(math.Min(float64(np), float64(ns)))
- }
- if i < 0 {
- i = len(input)
- }
- params = append(params, input[:i])
- input = input[i:]
- continue
- }
- if len(input) == 0 || input[0] != c {
- return nil, "", false
- }
- pattern = pattern[1:]
- input = input[1:]
- }
- if input == "" {
- input = "/"
- }
- if input[0] != '/' {
- return nil, "", false
- }
- return params, input, true
-}
-
-// Validate returns an error if Server does not comply with the OpenAPI spec.
-func (server *Server) Validate(ctx context.Context, opts ...ValidationOption) (err error) {
- ctx = WithValidationOptions(ctx, opts...)
-
- if server.URL == "" {
- return errors.New("value of url must be a non-empty string")
- }
-
- opening, closing := strings.Count(server.URL, "{"), strings.Count(server.URL, "}")
- if opening != closing {
- return errors.New("server URL has mismatched { and }")
- }
-
- if opening != len(server.Variables) {
- return errors.New("server has undeclared variables")
- }
-
- variables := make([]string, 0, len(server.Variables))
- for name := range server.Variables {
- variables = append(variables, name)
- }
- sort.Strings(variables)
- for _, name := range variables {
- v := server.Variables[name]
- if !strings.Contains(server.URL, "{"+name+"}") {
- return errors.New("server has undeclared variables")
- }
- if err = v.Validate(ctx); err != nil {
- return
- }
- }
-
- return validateExtensions(ctx, server.Extensions)
-}
-
-// ServerVariable is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#server-variable-object
-type ServerVariable struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Enum []string `json:"enum,omitempty" yaml:"enum,omitempty"`
- Default string `json:"default,omitempty" yaml:"default,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of ServerVariable.
-func (serverVariable ServerVariable) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 4+len(serverVariable.Extensions))
- for k, v := range serverVariable.Extensions {
- m[k] = v
- }
- if x := serverVariable.Enum; len(x) != 0 {
- m["enum"] = x
- }
- if x := serverVariable.Default; x != "" {
- m["default"] = x
- }
- if x := serverVariable.Description; x != "" {
- m["description"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets ServerVariable to a copy of data.
-func (serverVariable *ServerVariable) UnmarshalJSON(data []byte) error {
- type ServerVariableBis ServerVariable
- var x ServerVariableBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "enum")
- delete(x.Extensions, "default")
- delete(x.Extensions, "description")
- *serverVariable = ServerVariable(x)
- return nil
-}
-
-// Validate returns an error if ServerVariable does not comply with the OpenAPI spec.
-func (serverVariable *ServerVariable) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if serverVariable.Default == "" {
- data, err := serverVariable.MarshalJSON()
- if err != nil {
- return err
- }
- return fmt.Errorf("field default is required in %s", data)
- }
-
- return validateExtensions(ctx, serverVariable.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/tag.go b/vendor/github.com/getkin/kin-openapi/openapi3/tag.go
deleted file mode 100644
index 93009a13..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/tag.go
+++ /dev/null
@@ -1,87 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
- "fmt"
-)
-
-// Tags is specified by OpenAPI/Swagger 3.0 standard.
-type Tags []*Tag
-
-func (tags Tags) Get(name string) *Tag {
- for _, tag := range tags {
- if tag.Name == name {
- return tag
- }
- }
- return nil
-}
-
-// Validate returns an error if Tags does not comply with the OpenAPI spec.
-func (tags Tags) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- for _, v := range tags {
- if err := v.Validate(ctx); err != nil {
- return err
- }
- }
- return nil
-}
-
-// Tag is specified by OpenAPI/Swagger 3.0 standard.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#tag-object
-type Tag struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Name string `json:"name,omitempty" yaml:"name,omitempty"`
- Description string `json:"description,omitempty" yaml:"description,omitempty"`
- ExternalDocs *ExternalDocs `json:"externalDocs,omitempty" yaml:"externalDocs,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of Tag.
-func (t Tag) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 3+len(t.Extensions))
- for k, v := range t.Extensions {
- m[k] = v
- }
- if x := t.Name; x != "" {
- m["name"] = x
- }
- if x := t.Description; x != "" {
- m["description"] = x
- }
- if x := t.ExternalDocs; x != nil {
- m["externalDocs"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets Tag to a copy of data.
-func (t *Tag) UnmarshalJSON(data []byte) error {
- type TagBis Tag
- var x TagBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "name")
- delete(x.Extensions, "description")
- delete(x.Extensions, "externalDocs")
- *t = Tag(x)
- return nil
-}
-
-// Validate returns an error if Tag does not comply with the OpenAPI spec.
-func (t *Tag) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- if v := t.ExternalDocs; v != nil {
- if err := v.Validate(ctx); err != nil {
- return fmt.Errorf("invalid external docs: %w", err)
- }
- }
-
- return validateExtensions(ctx, t.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/circularRef/base.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/circularRef/base.yml
deleted file mode 100644
index ff8240eb..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/circularRef/base.yml
+++ /dev/null
@@ -1,16 +0,0 @@
-openapi: "3.0.3"
-info:
- title: Recursive cyclic refs example
- version: "1.0"
-components:
- schemas:
- Foo:
- properties:
- foo2:
- $ref: "other.yml#/components/schemas/Foo2"
- bar:
- $ref: "#/components/schemas/Bar"
- Bar:
- properties:
- foo:
- $ref: "#/components/schemas/Foo"
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/circularRef/other.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/circularRef/other.yml
deleted file mode 100644
index 29b72d98..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/circularRef/other.yml
+++ /dev/null
@@ -1,10 +0,0 @@
-openapi: "3.0.3"
-info:
- title: Recursive cyclic refs example
- version: "1.0"
-components:
- schemas:
- Foo2:
- properties:
- id:
- type: string
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Bar.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Bar.yml
deleted file mode 100644
index cc59fc27..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Bar.yml
+++ /dev/null
@@ -1,2 +0,0 @@
-type: string
-example: bar
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Cat.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Cat.yml
deleted file mode 100644
index c476aa1a..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Cat.yml
+++ /dev/null
@@ -1,4 +0,0 @@
-type: object
-properties:
- cat:
- $ref: ../openapi.yml#/components/schemas/Cat
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Foo.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Foo.yml
deleted file mode 100644
index 53a23366..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Foo.yml
+++ /dev/null
@@ -1,4 +0,0 @@
-type: object
-properties:
- bar:
- $ref: ../openapi.yml#/components/schemas/Bar
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Foo/Foo2.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Foo/Foo2.yml
deleted file mode 100644
index aeac81f4..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/Foo/Foo2.yml
+++ /dev/null
@@ -1,4 +0,0 @@
-type: object
-properties:
- foo:
- $ref: ../../openapi.yml#/components/schemas/Foo
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/models/error.yaml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/models/error.yaml
deleted file mode 100644
index b4d40479..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/components/models/error.yaml
+++ /dev/null
@@ -1,2 +0,0 @@
-type: object
-title: ErrorDetails
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/issue615.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/issue615.yml
deleted file mode 100644
index d1370e32..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/issue615.yml
+++ /dev/null
@@ -1,60 +0,0 @@
-openapi: "3.0.3"
-info:
- title: Deep recursive cyclic refs example
- version: "1.0"
-paths:
- /foo:
- $ref: ./paths/foo.yml
-components:
- schemas:
- FilterColumnIncludes:
- type: object
- properties:
- $includes:
- $ref: '#/components/schemas/FilterPredicate'
- additionalProperties: false
- maxProperties: 1
- minProperties: 1
- FilterPredicate:
- oneOf:
- - $ref: '#/components/schemas/FilterValue'
- - type: array
- items:
- $ref: '#/components/schemas/FilterPredicate'
- minLength: 1
- - $ref: '#/components/schemas/FilterPredicateOp'
- - $ref: '#/components/schemas/FilterPredicateRangeOp'
- FilterPredicateOp:
- type: object
- properties:
- $any:
- oneOf:
- - type: array
- items:
- $ref: '#/components/schemas/FilterPredicate'
- $none:
- oneOf:
- - $ref: '#/components/schemas/FilterPredicate'
- - type: array
- items:
- $ref: '#/components/schemas/FilterPredicate'
- additionalProperties: false
- maxProperties: 1
- minProperties: 1
- FilterPredicateRangeOp:
- type: object
- properties:
- $lt:
- $ref: '#/components/schemas/FilterRangeValue'
- additionalProperties: false
- maxProperties: 2
- minProperties: 2
- FilterRangeValue:
- oneOf:
- - type: number
- - type: string
- FilterValue:
- oneOf:
- - type: number
- - type: string
- - type: boolean
\ No newline at end of file
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/openapi.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/openapi.yml
deleted file mode 100644
index 9f884c71..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/openapi.yml
+++ /dev/null
@@ -1,33 +0,0 @@
-openapi: "3.0.3"
-info:
- title: Recursive refs example
- version: "1.0"
-paths:
- /foo:
- $ref: ./paths/foo.yml
- /double-ref-foo:
- get:
- summary: Double ref response
- description: Reference response with double reference.
- responses:
- "400":
- $ref: "#/components/responses/400"
-components:
- schemas:
- Foo:
- $ref: ./components/Foo.yml
- Foo2:
- $ref: ./components/Foo/Foo2.yml
- Bar:
- $ref: ./components/Bar.yml
- Cat:
- $ref: ./components/Cat.yml
- Error:
- $ref: ./components/models/error.yaml
- responses:
- "400":
- description: 400 Bad Request
- content:
- application/json:
- schema:
- $ref: "#/components/schemas/Error"
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/openapi.yml.internalized.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/openapi.yml.internalized.yml
deleted file mode 100644
index 0d508527..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/openapi.yml.internalized.yml
+++ /dev/null
@@ -1,110 +0,0 @@
-{
- "components": {
- "parameters": {
- "number": {
- "in": "query",
- "name": "someNumber",
- "schema": {
- "type": "string"
- }
- }
- },
- "responses": {
- "400": {
- "content": {
- "application/json": {
- "schema": {
- "$ref": "#/components/schemas/Error"
- }
- }
- },
- "description": "400 Bad Request"
- }
- },
- "schemas": {
- "Bar": {
- "example": "bar",
- "type": "string"
- },
- "Error":{
- "title":"ErrorDetails",
- "type":"object"
- },
- "Foo": {
- "properties": {
- "bar": {
- "$ref": "#/components/schemas/Bar"
- }
- },
- "type": "object"
- },
- "Foo2": {
- "properties": {
- "foo": {
- "$ref": "#/components/schemas/Foo"
- }
- },
- "type": "object"
- },
- "error":{
- "title":"ErrorDetails",
- "type":"object"
- },
- "Cat": {
- "properties": {
- "cat": {
- "$ref": "#/components/schemas/Cat"
- }
- },
- "type": "object"
- }
- }
- },
- "info": {
- "title": "Recursive refs example",
- "version": "1.0"
- },
- "openapi": "3.0.3",
- "paths": {
- "/double-ref-foo": {
- "get": {
- "description": "Reference response with double reference.",
- "responses": {
- "400": {
- "$ref": "#/components/responses/400"
- }
- },
- "summary": "Double ref response"
- }
- },
- "/foo": {
- "get": {
- "responses": {
- "200": {
- "content": {
- "application/json": {
- "schema": {
- "properties": {
- "foo2": {
- "$ref": "#/components/schemas/Foo2"
- }
- },
- "type": "object"
- }
- }
- },
- "description": "OK"
- },
- "400": {
- "$ref": "#/components/responses/400"
- }
- }
- },
- "parameters": [
- {
- "$ref": "#/components/parameters/number"
- }
- ]
- }
- }
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/parameters/number.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/parameters/number.yml
deleted file mode 100644
index 29f0f264..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/parameters/number.yml
+++ /dev/null
@@ -1,4 +0,0 @@
-name: someNumber
-in: query
-schema:
- type: string
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/paths/foo.yml b/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/paths/foo.yml
deleted file mode 100644
index 4c845b53..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/testdata/recursiveRef/paths/foo.yml
+++ /dev/null
@@ -1,15 +0,0 @@
-parameters:
- - $ref: ../parameters/number.yml
-get:
- responses:
- "200":
- description: OK
- content:
- application/json:
- schema:
- type: object
- properties:
- foo2:
- $ref: ../openapi.yml#/components/schemas/Foo2
- "400":
- $ref: "../openapi.yml#/components/responses/400"
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/validation_options.go b/vendor/github.com/getkin/kin-openapi/openapi3/validation_options.go
deleted file mode 100644
index 0ca12e5a..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/validation_options.go
+++ /dev/null
@@ -1,112 +0,0 @@
-package openapi3
-
-import "context"
-
-// ValidationOption allows the modification of how the OpenAPI document is validated.
-type ValidationOption func(options *ValidationOptions)
-
-// ValidationOptions provides configuration for validating OpenAPI documents.
-type ValidationOptions struct {
- examplesValidationAsReq, examplesValidationAsRes bool
- examplesValidationDisabled bool
- schemaDefaultsValidationDisabled bool
- schemaFormatValidationEnabled bool
- schemaPatternValidationDisabled bool
- extraSiblingFieldsAllowed map[string]struct{}
-}
-
-type validationOptionsKey struct{}
-
-// AllowExtraSiblingFields called as AllowExtraSiblingFields("description") makes Validate not return an error when said field appears next to a $ref.
-func AllowExtraSiblingFields(fields ...string) ValidationOption {
- return func(options *ValidationOptions) {
- for _, field := range fields {
- if options.extraSiblingFieldsAllowed == nil {
- options.extraSiblingFieldsAllowed = make(map[string]struct{}, len(fields))
- }
- options.extraSiblingFieldsAllowed[field] = struct{}{}
- }
- }
-}
-
-// EnableSchemaFormatValidation makes Validate not return an error when validating documents that mention schema formats that are not defined by the OpenAPIv3 specification.
-// By default, schema format validation is disabled.
-func EnableSchemaFormatValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.schemaFormatValidationEnabled = true
- }
-}
-
-// DisableSchemaFormatValidation does the opposite of EnableSchemaFormatValidation.
-// By default, schema format validation is disabled.
-func DisableSchemaFormatValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.schemaFormatValidationEnabled = false
- }
-}
-
-// EnableSchemaPatternValidation does the opposite of DisableSchemaPatternValidation.
-// By default, schema pattern validation is enabled.
-func EnableSchemaPatternValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.schemaPatternValidationDisabled = false
- }
-}
-
-// DisableSchemaPatternValidation makes Validate not return an error when validating patterns that are not supported by the Go regexp engine.
-func DisableSchemaPatternValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.schemaPatternValidationDisabled = true
- }
-}
-
-// EnableSchemaDefaultsValidation does the opposite of DisableSchemaDefaultsValidation.
-// By default, schema default values are validated against their schema.
-func EnableSchemaDefaultsValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.schemaDefaultsValidationDisabled = false
- }
-}
-
-// DisableSchemaDefaultsValidation disables schemas' default field validation.
-// By default, schema default values are validated against their schema.
-func DisableSchemaDefaultsValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.schemaDefaultsValidationDisabled = true
- }
-}
-
-// EnableExamplesValidation does the opposite of DisableExamplesValidation.
-// By default, all schema examples are validated.
-func EnableExamplesValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.examplesValidationDisabled = false
- }
-}
-
-// DisableExamplesValidation disables all example schema validation.
-// By default, all schema examples are validated.
-func DisableExamplesValidation() ValidationOption {
- return func(options *ValidationOptions) {
- options.examplesValidationDisabled = true
- }
-}
-
-// WithValidationOptions allows adding validation options to a context object that can be used when validationg any OpenAPI type.
-func WithValidationOptions(ctx context.Context, opts ...ValidationOption) context.Context {
- if len(opts) == 0 {
- return ctx
- }
- options := &ValidationOptions{}
- for _, opt := range opts {
- opt(options)
- }
- return context.WithValue(ctx, validationOptionsKey{}, options)
-}
-
-func getValidationOptions(ctx context.Context) *ValidationOptions {
- if options, ok := ctx.Value(validationOptionsKey{}).(*ValidationOptions); ok {
- return options
- }
- return &ValidationOptions{}
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/visited.go b/vendor/github.com/getkin/kin-openapi/openapi3/visited.go
deleted file mode 100644
index 67f970e3..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/visited.go
+++ /dev/null
@@ -1,41 +0,0 @@
-package openapi3
-
-func newVisited() visitedComponent {
- return visitedComponent{
- header: make(map[*Header]struct{}),
- schema: make(map[*Schema]struct{}),
- }
-}
-
-type visitedComponent struct {
- header map[*Header]struct{}
- schema map[*Schema]struct{}
-}
-
-// resetVisited clears visitedComponent map
-// should be called before recursion over doc *T
-func (doc *T) resetVisited() {
- doc.visited = newVisited()
-}
-
-// isVisitedHeader returns `true` if the *Header pointer was already visited
-// otherwise it returns `false`
-func (doc *T) isVisitedHeader(h *Header) bool {
- if _, ok := doc.visited.header[h]; ok {
- return true
- }
-
- doc.visited.header[h] = struct{}{}
- return false
-}
-
-// isVisitedHeader returns `true` if the *Schema pointer was already visited
-// otherwise it returns `false`
-func (doc *T) isVisitedSchema(s *Schema) bool {
- if _, ok := doc.visited.schema[s]; ok {
- return true
- }
-
- doc.visited.schema[s] = struct{}{}
- return false
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3/xml.go b/vendor/github.com/getkin/kin-openapi/openapi3/xml.go
deleted file mode 100644
index 34ed3be3..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3/xml.go
+++ /dev/null
@@ -1,66 +0,0 @@
-package openapi3
-
-import (
- "context"
- "encoding/json"
-)
-
-// XML is specified by OpenAPI/Swagger standard version 3.
-// See https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#xml-object
-type XML struct {
- Extensions map[string]interface{} `json:"-" yaml:"-"`
-
- Name string `json:"name,omitempty" yaml:"name,omitempty"`
- Namespace string `json:"namespace,omitempty" yaml:"namespace,omitempty"`
- Prefix string `json:"prefix,omitempty" yaml:"prefix,omitempty"`
- Attribute bool `json:"attribute,omitempty" yaml:"attribute,omitempty"`
- Wrapped bool `json:"wrapped,omitempty" yaml:"wrapped,omitempty"`
-}
-
-// MarshalJSON returns the JSON encoding of XML.
-func (xml XML) MarshalJSON() ([]byte, error) {
- m := make(map[string]interface{}, 5+len(xml.Extensions))
- for k, v := range xml.Extensions {
- m[k] = v
- }
- if x := xml.Name; x != "" {
- m["name"] = x
- }
- if x := xml.Namespace; x != "" {
- m["namespace"] = x
- }
- if x := xml.Prefix; x != "" {
- m["prefix"] = x
- }
- if x := xml.Attribute; x {
- m["attribute"] = x
- }
- if x := xml.Wrapped; x {
- m["wrapped"] = x
- }
- return json.Marshal(m)
-}
-
-// UnmarshalJSON sets XML to a copy of data.
-func (xml *XML) UnmarshalJSON(data []byte) error {
- type XMLBis XML
- var x XMLBis
- if err := json.Unmarshal(data, &x); err != nil {
- return err
- }
- _ = json.Unmarshal(data, &x.Extensions)
- delete(x.Extensions, "name")
- delete(x.Extensions, "namespace")
- delete(x.Extensions, "prefix")
- delete(x.Extensions, "attribute")
- delete(x.Extensions, "wrapped")
- *xml = XML(x)
- return nil
-}
-
-// Validate returns an error if XML does not comply with the OpenAPI spec.
-func (xml *XML) Validate(ctx context.Context, opts ...ValidationOption) error {
- ctx = WithValidationOptions(ctx, opts...)
-
- return validateExtensions(ctx, xml.Extensions)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/authentication_input.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/authentication_input.go
deleted file mode 100644
index a53484b9..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/authentication_input.go
+++ /dev/null
@@ -1,29 +0,0 @@
-package openapi3filter
-
-import (
- "fmt"
-
- "github.com/getkin/kin-openapi/openapi3"
-)
-
-type AuthenticationInput struct {
- RequestValidationInput *RequestValidationInput
- SecuritySchemeName string
- SecurityScheme *openapi3.SecurityScheme
- Scopes []string
-}
-
-func (input *AuthenticationInput) NewError(err error) error {
- if err == nil {
- if len(input.Scopes) == 0 {
- err = fmt.Errorf("security requirement %q failed", input.SecuritySchemeName)
- } else {
- err = fmt.Errorf("security requirement %q (scopes: %+v) failed", input.SecuritySchemeName, input.Scopes)
- }
- }
- return &RequestError{
- Input: input.RequestValidationInput,
- Reason: "authorization failed",
- Err: err,
- }
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/errors.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/errors.go
deleted file mode 100644
index b5454a75..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/errors.go
+++ /dev/null
@@ -1,92 +0,0 @@
-package openapi3filter
-
-import (
- "bytes"
- "fmt"
-
- "github.com/getkin/kin-openapi/openapi3"
-)
-
-var _ error = &RequestError{}
-
-// RequestError is returned by ValidateRequest when request does not match OpenAPI spec
-type RequestError struct {
- Input *RequestValidationInput
- Parameter *openapi3.Parameter
- RequestBody *openapi3.RequestBody
- Reason string
- Err error
-}
-
-var _ interface{ Unwrap() error } = RequestError{}
-
-func (err *RequestError) Error() string {
- reason := err.Reason
- if e := err.Err; e != nil {
- if len(reason) == 0 || reason == e.Error() {
- reason = e.Error()
- } else {
- reason += ": " + e.Error()
- }
- }
- if v := err.Parameter; v != nil {
- return fmt.Sprintf("parameter %q in %s has an error: %s", v.Name, v.In, reason)
- } else if v := err.RequestBody; v != nil {
- return fmt.Sprintf("request body has an error: %s", reason)
- } else {
- return reason
- }
-}
-
-func (err RequestError) Unwrap() error {
- return err.Err
-}
-
-var _ error = &ResponseError{}
-
-// ResponseError is returned by ValidateResponse when response does not match OpenAPI spec
-type ResponseError struct {
- Input *ResponseValidationInput
- Reason string
- Err error
-}
-
-var _ interface{ Unwrap() error } = ResponseError{}
-
-func (err *ResponseError) Error() string {
- reason := err.Reason
- if e := err.Err; e != nil {
- if len(reason) == 0 {
- reason = e.Error()
- } else {
- reason += ": " + e.Error()
- }
- }
- return reason
-}
-
-func (err ResponseError) Unwrap() error {
- return err.Err
-}
-
-var _ error = &SecurityRequirementsError{}
-
-// SecurityRequirementsError is returned by ValidateSecurityRequirements
-// when no requirement is met.
-type SecurityRequirementsError struct {
- SecurityRequirements openapi3.SecurityRequirements
- Errors []error
-}
-
-func (err *SecurityRequirementsError) Error() string {
- buff := &bytes.Buffer{}
- buff.WriteString("security requirements failed: ")
- for i, e := range err.Errors {
- buff.WriteString(e.Error())
- if i != len(err.Errors)-1 {
- buff.WriteString(" | ")
- }
- }
-
- return buff.String()
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/internal.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/internal.go
deleted file mode 100644
index 5c6a8a6c..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/internal.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package openapi3filter
-
-import (
- "reflect"
- "strings"
-)
-
-func parseMediaType(contentType string) string {
- i := strings.IndexByte(contentType, ';')
- if i < 0 {
- return contentType
- }
- return contentType[:i]
-}
-
-func isNilValue(value interface{}) bool {
- if value == nil {
- return true
- }
- switch reflect.TypeOf(value).Kind() {
- case reflect.Ptr, reflect.Map, reflect.Array, reflect.Chan, reflect.Slice:
- return reflect.ValueOf(value).IsNil()
- }
- return false
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/middleware.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/middleware.go
deleted file mode 100644
index 3bcb9db4..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/middleware.go
+++ /dev/null
@@ -1,283 +0,0 @@
-package openapi3filter
-
-import (
- "bytes"
- "io"
- "io/ioutil"
- "log"
- "net/http"
-
- "github.com/getkin/kin-openapi/routers"
-)
-
-// Validator provides HTTP request and response validation middleware.
-type Validator struct {
- router routers.Router
- errFunc ErrFunc
- logFunc LogFunc
- strict bool
- options Options
-}
-
-// ErrFunc handles errors that may occur during validation.
-type ErrFunc func(w http.ResponseWriter, status int, code ErrCode, err error)
-
-// LogFunc handles log messages that may occur during validation.
-type LogFunc func(message string, err error)
-
-// ErrCode is used for classification of different types of errors that may
-// occur during validation. These may be used to write an appropriate response
-// in ErrFunc.
-type ErrCode int
-
-const (
- // ErrCodeOK indicates no error. It is also the default value.
- ErrCodeOK = 0
- // ErrCodeCannotFindRoute happens when the validator fails to resolve the
- // request to a defined OpenAPI route.
- ErrCodeCannotFindRoute = iota
- // ErrCodeRequestInvalid happens when the inbound request does not conform
- // to the OpenAPI 3 specification.
- ErrCodeRequestInvalid = iota
- // ErrCodeResponseInvalid happens when the wrapped handler response does
- // not conform to the OpenAPI 3 specification.
- ErrCodeResponseInvalid = iota
-)
-
-func (e ErrCode) responseText() string {
- switch e {
- case ErrCodeOK:
- return "OK"
- case ErrCodeCannotFindRoute:
- return "not found"
- case ErrCodeRequestInvalid:
- return "bad request"
- default:
- return "server error"
- }
-}
-
-// NewValidator returns a new response validation middlware, using the given
-// routes from an OpenAPI 3 specification.
-func NewValidator(router routers.Router, options ...ValidatorOption) *Validator {
- v := &Validator{
- router: router,
- errFunc: func(w http.ResponseWriter, status int, code ErrCode, _ error) {
- http.Error(w, code.responseText(), status)
- },
- logFunc: func(message string, err error) {
- log.Printf("%s: %v", message, err)
- },
- }
- for i := range options {
- options[i](v)
- }
- return v
-}
-
-// ValidatorOption defines an option that may be specified when creating a
-// Validator.
-type ValidatorOption func(*Validator)
-
-// OnErr provides a callback that handles writing an HTTP response on a
-// validation error. This allows customization of error responses without
-// prescribing a particular form. This callback is only called on response
-// validator errors in Strict mode.
-func OnErr(f ErrFunc) ValidatorOption {
- return func(v *Validator) {
- v.errFunc = f
- }
-}
-
-// OnLog provides a callback that handles logging in the Validator. This allows
-// the validator to integrate with a services' existing logging system without
-// prescribing a particular one.
-func OnLog(f LogFunc) ValidatorOption {
- return func(v *Validator) {
- v.logFunc = f
- }
-}
-
-// Strict, if set, causes an internal server error to be sent if the wrapped
-// handler response fails response validation. If not set, the response is sent
-// and the error is only logged.
-func Strict(strict bool) ValidatorOption {
- return func(v *Validator) {
- v.strict = strict
- }
-}
-
-// ValidationOptions sets request/response validation options on the validator.
-func ValidationOptions(options Options) ValidatorOption {
- return func(v *Validator) {
- v.options = options
- }
-}
-
-// Middleware returns an http.Handler which wraps the given handler with
-// request and response validation.
-func (v *Validator) Middleware(h http.Handler) http.Handler {
- return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
- route, pathParams, err := v.router.FindRoute(r)
- if err != nil {
- v.logFunc("validation error: failed to find route for "+r.URL.String(), err)
- v.errFunc(w, http.StatusNotFound, ErrCodeCannotFindRoute, err)
- return
- }
- requestValidationInput := &RequestValidationInput{
- Request: r,
- PathParams: pathParams,
- Route: route,
- Options: &v.options,
- }
- if err = ValidateRequest(r.Context(), requestValidationInput); err != nil {
- v.logFunc("invalid request", err)
- v.errFunc(w, http.StatusBadRequest, ErrCodeRequestInvalid, err)
- return
- }
-
- var wr responseWrapper
- if v.strict {
- wr = &strictResponseWrapper{w: w}
- } else {
- wr = newWarnResponseWrapper(w)
- }
-
- h.ServeHTTP(wr, r)
-
- if err = ValidateResponse(r.Context(), &ResponseValidationInput{
- RequestValidationInput: requestValidationInput,
- Status: wr.statusCode(),
- Header: wr.Header(),
- Body: ioutil.NopCloser(bytes.NewBuffer(wr.bodyContents())),
- Options: &v.options,
- }); err != nil {
- v.logFunc("invalid response", err)
- if v.strict {
- v.errFunc(w, http.StatusInternalServerError, ErrCodeResponseInvalid, err)
- }
- return
- }
-
- if err = wr.flushBodyContents(); err != nil {
- v.logFunc("failed to write response", err)
- }
- })
-}
-
-type responseWrapper interface {
- http.ResponseWriter
-
- // flushBodyContents writes the buffered response to the client, if it has
- // not yet been written.
- flushBodyContents() error
-
- // statusCode returns the response status code, 0 if not set yet.
- statusCode() int
-
- // bodyContents returns the buffered
- bodyContents() []byte
-}
-
-type warnResponseWrapper struct {
- w http.ResponseWriter
- headerWritten bool
- status int
- body bytes.Buffer
- tee io.Writer
-}
-
-func newWarnResponseWrapper(w http.ResponseWriter) *warnResponseWrapper {
- wr := &warnResponseWrapper{
- w: w,
- }
- wr.tee = io.MultiWriter(w, &wr.body)
- return wr
-}
-
-// Write implements http.ResponseWriter.
-func (wr *warnResponseWrapper) Write(b []byte) (int, error) {
- if !wr.headerWritten {
- wr.WriteHeader(http.StatusOK)
- }
- return wr.tee.Write(b)
-}
-
-// WriteHeader implements http.ResponseWriter.
-func (wr *warnResponseWrapper) WriteHeader(status int) {
- if !wr.headerWritten {
- // If the header hasn't been written, record the status for response
- // validation.
- wr.status = status
- wr.headerWritten = true
- }
- wr.w.WriteHeader(wr.status)
-}
-
-// Header implements http.ResponseWriter.
-func (wr *warnResponseWrapper) Header() http.Header {
- return wr.w.Header()
-}
-
-// Flush implements the optional http.Flusher interface.
-func (wr *warnResponseWrapper) Flush() {
- // If the wrapped http.ResponseWriter implements optional http.Flusher,
- // pass through.
- if fl, ok := wr.w.(http.Flusher); ok {
- fl.Flush()
- }
-}
-
-func (wr *warnResponseWrapper) flushBodyContents() error {
- return nil
-}
-
-func (wr *warnResponseWrapper) statusCode() int {
- return wr.status
-}
-
-func (wr *warnResponseWrapper) bodyContents() []byte {
- return wr.body.Bytes()
-}
-
-type strictResponseWrapper struct {
- w http.ResponseWriter
- headerWritten bool
- status int
- body bytes.Buffer
-}
-
-// Write implements http.ResponseWriter.
-func (wr *strictResponseWrapper) Write(b []byte) (int, error) {
- if !wr.headerWritten {
- wr.WriteHeader(http.StatusOK)
- }
- return wr.body.Write(b)
-}
-
-// WriteHeader implements http.ResponseWriter.
-func (wr *strictResponseWrapper) WriteHeader(status int) {
- if !wr.headerWritten {
- wr.status = status
- wr.headerWritten = true
- }
-}
-
-// Header implements http.ResponseWriter.
-func (wr *strictResponseWrapper) Header() http.Header {
- return wr.w.Header()
-}
-
-func (wr *strictResponseWrapper) flushBodyContents() error {
- wr.w.WriteHeader(wr.status)
- _, err := wr.w.Write(wr.body.Bytes())
- return err
-}
-
-func (wr *strictResponseWrapper) statusCode() int {
- return wr.status
-}
-
-func (wr *strictResponseWrapper) bodyContents() []byte {
- return wr.body.Bytes()
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/options.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/options.go
deleted file mode 100644
index 4ea9e990..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/options.go
+++ /dev/null
@@ -1,47 +0,0 @@
-package openapi3filter
-
-import "github.com/getkin/kin-openapi/openapi3"
-
-// DefaultOptions do not set an AuthenticationFunc.
-// A spec with security schemes defined will not pass validation
-// unless an AuthenticationFunc is defined.
-var DefaultOptions = &Options{}
-
-// Options used by ValidateRequest and ValidateResponse
-type Options struct {
- // Set ExcludeRequestBody so ValidateRequest skips request body validation
- ExcludeRequestBody bool
-
- // Set ExcludeResponseBody so ValidateResponse skips response body validation
- ExcludeResponseBody bool
-
- // Set ExcludeReadOnlyValidations so ValidateRequest skips read-only validations
- ExcludeReadOnlyValidations bool
-
- // Set ExcludeWriteOnlyValidations so ValidateResponse skips write-only validations
- ExcludeWriteOnlyValidations bool
-
- // Set IncludeResponseStatus so ValidateResponse fails on response
- // status not defined in OpenAPI spec
- IncludeResponseStatus bool
-
- MultiError bool
-
- // See NoopAuthenticationFunc
- AuthenticationFunc AuthenticationFunc
-
- // Indicates whether default values are set in the
- // request. If true, then they are not set
- SkipSettingDefaults bool
-
- customSchemaErrorFunc CustomSchemaErrorFunc
-}
-
-// CustomSchemaErrorFunc allows for custom the schema error message.
-type CustomSchemaErrorFunc func(err *openapi3.SchemaError) string
-
-// WithCustomSchemaErrorFunc sets a function to override the schema error message.
-// If the passed function returns an empty string, it returns to the previous Error() implementation.
-func (o *Options) WithCustomSchemaErrorFunc(f CustomSchemaErrorFunc) {
- o.customSchemaErrorFunc = f
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/req_resp_decoder.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/req_resp_decoder.go
deleted file mode 100644
index 384b5122..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/req_resp_decoder.go
+++ /dev/null
@@ -1,1297 +0,0 @@
-package openapi3filter
-
-import (
- "archive/zip"
- "bytes"
- "encoding/csv"
- "encoding/json"
- "errors"
- "fmt"
- "io"
- "io/ioutil"
- "mime"
- "mime/multipart"
- "net/http"
- "net/url"
- "regexp"
- "strconv"
- "strings"
-
- "gopkg.in/yaml.v3"
-
- "github.com/getkin/kin-openapi/openapi3"
-)
-
-// ParseErrorKind describes a kind of ParseError.
-// The type simplifies comparison of errors.
-type ParseErrorKind int
-
-const (
- // KindOther describes an untyped parsing error.
- KindOther ParseErrorKind = iota
- // KindUnsupportedFormat describes an error that happens when a value has an unsupported format.
- KindUnsupportedFormat
- // KindInvalidFormat describes an error that happens when a value does not conform a format
- // that is required by a serialization method.
- KindInvalidFormat
-)
-
-// ParseError describes errors which happens while parse operation's parameters, requestBody, or response.
-type ParseError struct {
- Kind ParseErrorKind
- Value interface{}
- Reason string
- Cause error
-
- path []interface{}
-}
-
-var _ interface{ Unwrap() error } = ParseError{}
-
-func (e *ParseError) Error() string {
- var msg []string
- if p := e.Path(); len(p) > 0 {
- var arr []string
- for _, v := range p {
- arr = append(arr, fmt.Sprintf("%v", v))
- }
- msg = append(msg, fmt.Sprintf("path %v", strings.Join(arr, ".")))
- }
- msg = append(msg, e.innerError())
- return strings.Join(msg, ": ")
-}
-
-func (e *ParseError) innerError() string {
- var msg []string
- if e.Value != nil {
- msg = append(msg, fmt.Sprintf("value %v", e.Value))
- }
- if e.Reason != "" {
- msg = append(msg, e.Reason)
- }
- if e.Cause != nil {
- if v, ok := e.Cause.(*ParseError); ok {
- msg = append(msg, v.innerError())
- } else {
- msg = append(msg, e.Cause.Error())
- }
- }
- return strings.Join(msg, ": ")
-}
-
-// RootCause returns a root cause of ParseError.
-func (e *ParseError) RootCause() error {
- if v, ok := e.Cause.(*ParseError); ok {
- return v.RootCause()
- }
- return e.Cause
-}
-
-func (e ParseError) Unwrap() error {
- return e.Cause
-}
-
-// Path returns a path to the root cause.
-func (e *ParseError) Path() []interface{} {
- var path []interface{}
- if v, ok := e.Cause.(*ParseError); ok {
- p := v.Path()
- if len(p) > 0 {
- path = append(path, p...)
- }
- }
- if len(e.path) > 0 {
- path = append(path, e.path...)
- }
- return path
-}
-
-func invalidSerializationMethodErr(sm *openapi3.SerializationMethod) error {
- return fmt.Errorf("invalid serialization method: style=%q, explode=%v", sm.Style, sm.Explode)
-}
-
-// Decodes a parameter defined via the content property as an object. It uses
-// the user specified decoder, or our build-in decoder for application/json
-func decodeContentParameter(param *openapi3.Parameter, input *RequestValidationInput) (
- value interface{},
- schema *openapi3.Schema,
- found bool,
- err error,
-) {
- var paramValues []string
- switch param.In {
- case openapi3.ParameterInPath:
- var paramValue string
- if paramValue, found = input.PathParams[param.Name]; found {
- paramValues = []string{paramValue}
- }
- case openapi3.ParameterInQuery:
- paramValues, found = input.GetQueryParams()[param.Name]
- case openapi3.ParameterInHeader:
- var headerValues []string
- if headerValues, found = input.Request.Header[http.CanonicalHeaderKey(param.Name)]; found {
- paramValues = headerValues
- }
- case openapi3.ParameterInCookie:
- var cookie *http.Cookie
- if cookie, err = input.Request.Cookie(param.Name); err == http.ErrNoCookie {
- found = false
- } else if err != nil {
- return
- } else {
- paramValues = []string{cookie.Value}
- found = true
- }
- default:
- err = fmt.Errorf("unsupported parameter.in: %q", param.In)
- return
- }
-
- if !found {
- if param.Required {
- err = fmt.Errorf("parameter %q is required, but missing", param.Name)
- }
- return
- }
-
- decoder := input.ParamDecoder
- if decoder == nil {
- decoder = defaultContentParameterDecoder
- }
-
- value, schema, err = decoder(param, paramValues)
- return
-}
-
-func defaultContentParameterDecoder(param *openapi3.Parameter, values []string) (
- outValue interface{},
- outSchema *openapi3.Schema,
- err error,
-) {
- // Only query parameters can have multiple values.
- if len(values) > 1 && param.In != openapi3.ParameterInQuery {
- err = fmt.Errorf("%s parameter %q cannot have multiple values", param.In, param.Name)
- return
- }
-
- content := param.Content
- if content == nil {
- err = fmt.Errorf("parameter %q expected to have content", param.Name)
- return
- }
- // We only know how to decode a parameter if it has one content, application/json
- if len(content) != 1 {
- err = fmt.Errorf("multiple content types for parameter %q", param.Name)
- return
- }
-
- mt := content.Get("application/json")
- if mt == nil {
- err = fmt.Errorf("parameter %q has no content schema", param.Name)
- return
- }
- outSchema = mt.Schema.Value
-
- unmarshal := func(encoded string, paramSchema *openapi3.SchemaRef) (decoded interface{}, err error) {
- if err = json.Unmarshal([]byte(encoded), &decoded); err != nil {
- if paramSchema != nil && paramSchema.Value.Type != "object" {
- decoded, err = encoded, nil
- }
- }
- return
- }
-
- if len(values) == 1 {
- if outValue, err = unmarshal(values[0], mt.Schema); err != nil {
- err = fmt.Errorf("error unmarshaling parameter %q", param.Name)
- return
- }
- } else {
- outArray := make([]interface{}, 0, len(values))
- for _, v := range values {
- var item interface{}
- if item, err = unmarshal(v, outSchema.Items); err != nil {
- err = fmt.Errorf("error unmarshaling parameter %q", param.Name)
- return
- }
- outArray = append(outArray, item)
- }
- outValue = outArray
- }
- return
-}
-
-type valueDecoder interface {
- DecodePrimitive(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error)
- DecodeArray(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) ([]interface{}, bool, error)
- DecodeObject(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (map[string]interface{}, bool, error)
-}
-
-// decodeStyledParameter returns a value of an operation's parameter from HTTP request for
-// parameters defined using the style format, and whether the parameter is supplied in the input.
-// The function returns ParseError when HTTP request contains an invalid value of a parameter.
-func decodeStyledParameter(param *openapi3.Parameter, input *RequestValidationInput) (interface{}, bool, error) {
- sm, err := param.SerializationMethod()
- if err != nil {
- return nil, false, err
- }
-
- var dec valueDecoder
- switch param.In {
- case openapi3.ParameterInPath:
- if len(input.PathParams) == 0 {
- return nil, false, nil
- }
- dec = &pathParamDecoder{pathParams: input.PathParams}
- case openapi3.ParameterInQuery:
- if len(input.GetQueryParams()) == 0 {
- return nil, false, nil
- }
- dec = &urlValuesDecoder{values: input.GetQueryParams()}
- case openapi3.ParameterInHeader:
- dec = &headerParamDecoder{header: input.Request.Header}
- case openapi3.ParameterInCookie:
- dec = &cookieParamDecoder{req: input.Request}
- default:
- return nil, false, fmt.Errorf("unsupported parameter's 'in': %s", param.In)
- }
-
- return decodeValue(dec, param.Name, sm, param.Schema, param.Required)
-}
-
-func decodeValue(dec valueDecoder, param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef, required bool) (interface{}, bool, error) {
- var found bool
-
- if len(schema.Value.AllOf) > 0 {
- var value interface{}
- var err error
- for _, sr := range schema.Value.AllOf {
- var f bool
- value, f, err = decodeValue(dec, param, sm, sr, required)
- found = found || f
- if value == nil || err != nil {
- break
- }
- }
- return value, found, err
- }
-
- if len(schema.Value.AnyOf) > 0 {
- for _, sr := range schema.Value.AnyOf {
- value, f, _ := decodeValue(dec, param, sm, sr, required)
- found = found || f
- if value != nil {
- return value, found, nil
- }
- }
- if required {
- return nil, found, fmt.Errorf("decoding anyOf for parameter %q failed", param)
- }
- return nil, found, nil
- }
-
- if len(schema.Value.OneOf) > 0 {
- isMatched := 0
- var value interface{}
- for _, sr := range schema.Value.OneOf {
- v, f, _ := decodeValue(dec, param, sm, sr, required)
- found = found || f
- if v != nil {
- value = v
- isMatched++
- }
- }
- if isMatched == 1 {
- return value, found, nil
- } else if isMatched > 1 {
- return nil, found, fmt.Errorf("decoding oneOf failed: %d schemas matched", isMatched)
- }
- if required {
- return nil, found, fmt.Errorf("decoding oneOf failed: %q is required", param)
- }
- return nil, found, nil
- }
-
- if schema.Value.Not != nil {
- // TODO(decode not): handle decoding "not" JSON Schema
- return nil, found, errors.New("not implemented: decoding 'not'")
- }
-
- if schema.Value.Type != "" {
- var decodeFn func(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error)
- switch schema.Value.Type {
- case "array":
- decodeFn = func(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error) {
- return dec.DecodeArray(param, sm, schema)
- }
- case "object":
- decodeFn = func(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error) {
- return dec.DecodeObject(param, sm, schema)
- }
- default:
- decodeFn = dec.DecodePrimitive
- }
- return decodeFn(param, sm, schema)
- }
- switch vDecoder := dec.(type) {
- case *pathParamDecoder:
- _, found = vDecoder.pathParams[param]
- case *urlValuesDecoder:
- if schema.Value.Pattern != "" {
- return dec.DecodePrimitive(param, sm, schema)
- }
- _, found = vDecoder.values[param]
- case *headerParamDecoder:
- _, found = vDecoder.header[http.CanonicalHeaderKey(param)]
- case *cookieParamDecoder:
- _, err := vDecoder.req.Cookie(param)
- found = err != http.ErrNoCookie
- default:
- return nil, found, errors.New("unsupported decoder")
- }
- return nil, found, nil
-}
-
-// pathParamDecoder decodes values of path parameters.
-type pathParamDecoder struct {
- pathParams map[string]string
-}
-
-func (d *pathParamDecoder) DecodePrimitive(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error) {
- var prefix string
- switch sm.Style {
- case "simple":
- // A prefix is empty for style "simple".
- case "label":
- prefix = "."
- case "matrix":
- prefix = ";" + param + "="
- default:
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- if d.pathParams == nil {
- // HTTP request does not contains a value of the target path parameter.
- return nil, false, nil
- }
- raw, ok := d.pathParams[param]
- if !ok || raw == "" {
- // HTTP request does not contains a value of the target path parameter.
- return nil, false, nil
- }
- src, err := cutPrefix(raw, prefix)
- if err != nil {
- return nil, ok, err
- }
- val, err := parsePrimitive(src, schema)
- return val, ok, err
-}
-
-func (d *pathParamDecoder) DecodeArray(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) ([]interface{}, bool, error) {
- var prefix, delim string
- switch {
- case sm.Style == "simple":
- delim = ","
- case sm.Style == "label" && !sm.Explode:
- prefix = "."
- delim = ","
- case sm.Style == "label" && sm.Explode:
- prefix = "."
- delim = "."
- case sm.Style == "matrix" && !sm.Explode:
- prefix = ";" + param + "="
- delim = ","
- case sm.Style == "matrix" && sm.Explode:
- prefix = ";" + param + "="
- delim = ";" + param + "="
- default:
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- if d.pathParams == nil {
- // HTTP request does not contains a value of the target path parameter.
- return nil, false, nil
- }
- raw, ok := d.pathParams[param]
- if !ok || raw == "" {
- // HTTP request does not contains a value of the target path parameter.
- return nil, false, nil
- }
- src, err := cutPrefix(raw, prefix)
- if err != nil {
- return nil, ok, err
- }
- val, err := parseArray(strings.Split(src, delim), schema)
- return val, ok, err
-}
-
-func (d *pathParamDecoder) DecodeObject(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (map[string]interface{}, bool, error) {
- var prefix, propsDelim, valueDelim string
- switch {
- case sm.Style == "simple" && !sm.Explode:
- propsDelim = ","
- valueDelim = ","
- case sm.Style == "simple" && sm.Explode:
- propsDelim = ","
- valueDelim = "="
- case sm.Style == "label" && !sm.Explode:
- prefix = "."
- propsDelim = ","
- valueDelim = ","
- case sm.Style == "label" && sm.Explode:
- prefix = "."
- propsDelim = "."
- valueDelim = "="
- case sm.Style == "matrix" && !sm.Explode:
- prefix = ";" + param + "="
- propsDelim = ","
- valueDelim = ","
- case sm.Style == "matrix" && sm.Explode:
- prefix = ";"
- propsDelim = ";"
- valueDelim = "="
- default:
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- if d.pathParams == nil {
- // HTTP request does not contains a value of the target path parameter.
- return nil, false, nil
- }
- raw, ok := d.pathParams[param]
- if !ok || raw == "" {
- // HTTP request does not contains a value of the target path parameter.
- return nil, false, nil
- }
- src, err := cutPrefix(raw, prefix)
- if err != nil {
- return nil, ok, err
- }
- props, err := propsFromString(src, propsDelim, valueDelim)
- if err != nil {
- return nil, ok, err
- }
- val, err := makeObject(props, schema)
- return val, ok, err
-}
-
-// cutPrefix validates that a raw value of a path parameter has the specified prefix,
-// and returns a raw value without the prefix.
-func cutPrefix(raw, prefix string) (string, error) {
- if prefix == "" {
- return raw, nil
- }
- if len(raw) < len(prefix) || raw[:len(prefix)] != prefix {
- return "", &ParseError{
- Kind: KindInvalidFormat,
- Value: raw,
- Reason: fmt.Sprintf("a value must be prefixed with %q", prefix),
- }
- }
- return raw[len(prefix):], nil
-}
-
-// urlValuesDecoder decodes values of query parameters.
-type urlValuesDecoder struct {
- values url.Values
-}
-
-func (d *urlValuesDecoder) DecodePrimitive(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error) {
- if sm.Style != "form" {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- values, ok := d.values[param]
- if len(values) == 0 {
- // HTTP request does not contain a value of the target query parameter.
- return nil, ok, nil
- }
-
- if schema.Value.Type == "" && schema.Value.Pattern != "" {
- return values[0], ok, nil
- }
- val, err := parsePrimitive(values[0], schema)
- return val, ok, err
-}
-
-func (d *urlValuesDecoder) DecodeArray(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) ([]interface{}, bool, error) {
- if sm.Style == "deepObject" {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- values, ok := d.values[param]
- if len(values) == 0 {
- // HTTP request does not contain a value of the target query parameter.
- return nil, ok, nil
- }
- if !sm.Explode {
- var delim string
- switch sm.Style {
- case "form":
- delim = ","
- case "spaceDelimited":
- delim = " "
- case "pipeDelimited":
- delim = "|"
- }
- values = strings.Split(values[0], delim)
- }
- val, err := d.parseArray(values, sm, schema)
- return val, ok, err
-}
-
-// parseArray returns an array that contains items from a raw array.
-// Every item is parsed as a primitive value.
-// The function returns an error when an error happened while parse array's items.
-func (d *urlValuesDecoder) parseArray(raw []string, sm *openapi3.SerializationMethod, schemaRef *openapi3.SchemaRef) ([]interface{}, error) {
- var value []interface{}
-
- for i, v := range raw {
- item, err := d.parseValue(v, schemaRef.Value.Items)
- if err != nil {
- if v, ok := err.(*ParseError); ok {
- return nil, &ParseError{path: []interface{}{i}, Cause: v}
- }
- return nil, fmt.Errorf("item %d: %w", i, err)
- }
-
- // If the items are nil, then the array is nil. There shouldn't be case where some values are actual primitive
- // values and some are nil values.
- if item == nil {
- return nil, nil
- }
- value = append(value, item)
- }
- return value, nil
-}
-
-func (d *urlValuesDecoder) parseValue(v string, schema *openapi3.SchemaRef) (interface{}, error) {
- if len(schema.Value.AllOf) > 0 {
- var value interface{}
- var err error
- for _, sr := range schema.Value.AllOf {
- value, err = d.parseValue(v, sr)
- if value == nil || err != nil {
- break
- }
- }
- return value, err
- }
-
- if len(schema.Value.AnyOf) > 0 {
- var value interface{}
- var err error
- for _, sr := range schema.Value.AnyOf {
- if value, err = d.parseValue(v, sr); err == nil {
- return value, nil
- }
- }
-
- return nil, err
- }
-
- if len(schema.Value.OneOf) > 0 {
- isMatched := 0
- var value interface{}
- var err error
- for _, sr := range schema.Value.OneOf {
- result, err := d.parseValue(v, sr)
- if err == nil {
- value = result
- isMatched++
- }
- }
- if isMatched == 1 {
- return value, nil
- } else if isMatched > 1 {
- return nil, fmt.Errorf("decoding oneOf failed: %d schemas matched", isMatched)
- } else if isMatched == 0 {
- return nil, fmt.Errorf("decoding oneOf failed: %d schemas matched", isMatched)
- }
-
- return nil, err
- }
-
- if schema.Value.Not != nil {
- // TODO(decode not): handle decoding "not" JSON Schema
- return nil, errors.New("not implemented: decoding 'not'")
- }
-
- return parsePrimitive(v, schema)
-
-}
-
-func (d *urlValuesDecoder) DecodeObject(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (map[string]interface{}, bool, error) {
- var propsFn func(url.Values) (map[string]string, error)
- switch sm.Style {
- case "form":
- propsFn = func(params url.Values) (map[string]string, error) {
- if len(params) == 0 {
- // HTTP request does not contain query parameters.
- return nil, nil
- }
- if sm.Explode {
- props := make(map[string]string)
- for key, values := range params {
- props[key] = values[0]
- }
- return props, nil
- }
- values := params[param]
- if len(values) == 0 {
- // HTTP request does not contain a value of the target query parameter.
- return nil, nil
- }
- return propsFromString(values[0], ",", ",")
- }
- case "deepObject":
- propsFn = func(params url.Values) (map[string]string, error) {
- props := make(map[string]string)
- for key, values := range params {
- groups := regexp.MustCompile(fmt.Sprintf("%s\\[(.+?)\\]", param)).FindAllStringSubmatch(key, -1)
- if len(groups) == 0 {
- // A query parameter's name does not match the required format, so skip it.
- continue
- }
- props[groups[0][1]] = values[0]
- }
- if len(props) == 0 {
- // HTTP request does not contain query parameters encoded by rules of style "deepObject".
- return nil, nil
- }
- return props, nil
- }
- default:
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- props, err := propsFn(d.values)
- if err != nil {
- return nil, false, err
- }
- if props == nil {
- return nil, false, nil
- }
-
- // check the props
- found := false
- for propName := range schema.Value.Properties {
- if _, ok := props[propName]; ok {
- found = true
- break
- }
- }
- val, err := makeObject(props, schema)
- return val, found, err
-}
-
-// headerParamDecoder decodes values of header parameters.
-type headerParamDecoder struct {
- header http.Header
-}
-
-func (d *headerParamDecoder) DecodePrimitive(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error) {
- if sm.Style != "simple" {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- raw, ok := d.header[http.CanonicalHeaderKey(param)]
- if !ok || len(raw) == 0 {
- // HTTP request does not contains a corresponding header or has the empty value
- return nil, ok, nil
- }
-
- val, err := parsePrimitive(raw[0], schema)
- return val, ok, err
-}
-
-func (d *headerParamDecoder) DecodeArray(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) ([]interface{}, bool, error) {
- if sm.Style != "simple" {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- raw, ok := d.header[http.CanonicalHeaderKey(param)]
- if !ok || len(raw) == 0 {
- // HTTP request does not contains a corresponding header
- return nil, ok, nil
- }
-
- val, err := parseArray(strings.Split(raw[0], ","), schema)
- return val, ok, err
-}
-
-func (d *headerParamDecoder) DecodeObject(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (map[string]interface{}, bool, error) {
- if sm.Style != "simple" {
- return nil, false, invalidSerializationMethodErr(sm)
- }
- valueDelim := ","
- if sm.Explode {
- valueDelim = "="
- }
-
- raw, ok := d.header[http.CanonicalHeaderKey(param)]
- if !ok || len(raw) == 0 {
- // HTTP request does not contain a corresponding header.
- return nil, ok, nil
- }
- props, err := propsFromString(raw[0], ",", valueDelim)
- if err != nil {
- return nil, ok, err
- }
- val, err := makeObject(props, schema)
- return val, ok, err
-}
-
-// cookieParamDecoder decodes values of cookie parameters.
-type cookieParamDecoder struct {
- req *http.Request
-}
-
-func (d *cookieParamDecoder) DecodePrimitive(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (interface{}, bool, error) {
- if sm.Style != "form" {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- cookie, err := d.req.Cookie(param)
- found := err != http.ErrNoCookie
- if !found {
- // HTTP request does not contain a corresponding cookie.
- return nil, found, nil
- }
- if err != nil {
- return nil, found, fmt.Errorf("decoding param %q: %w", param, err)
- }
-
- val, err := parsePrimitive(cookie.Value, schema)
- return val, found, err
-}
-
-func (d *cookieParamDecoder) DecodeArray(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) ([]interface{}, bool, error) {
- if sm.Style != "form" || sm.Explode {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- cookie, err := d.req.Cookie(param)
- found := err != http.ErrNoCookie
- if !found {
- // HTTP request does not contain a corresponding cookie.
- return nil, found, nil
- }
- if err != nil {
- return nil, found, fmt.Errorf("decoding param %q: %w", param, err)
- }
- val, err := parseArray(strings.Split(cookie.Value, ","), schema)
- return val, found, err
-}
-
-func (d *cookieParamDecoder) DecodeObject(param string, sm *openapi3.SerializationMethod, schema *openapi3.SchemaRef) (map[string]interface{}, bool, error) {
- if sm.Style != "form" || sm.Explode {
- return nil, false, invalidSerializationMethodErr(sm)
- }
-
- cookie, err := d.req.Cookie(param)
- found := err != http.ErrNoCookie
- if !found {
- // HTTP request does not contain a corresponding cookie.
- return nil, found, nil
- }
- if err != nil {
- return nil, found, fmt.Errorf("decoding param %q: %w", param, err)
- }
- props, err := propsFromString(cookie.Value, ",", ",")
- if err != nil {
- return nil, found, err
- }
- val, err := makeObject(props, schema)
- return val, found, err
-}
-
-// propsFromString returns a properties map that is created by splitting a source string by propDelim and valueDelim.
-// The source string must have a valid format: pairs separated by .
-// The function returns an error when the source string has an invalid format.
-func propsFromString(src, propDelim, valueDelim string) (map[string]string, error) {
- props := make(map[string]string)
- pairs := strings.Split(src, propDelim)
-
- // When propDelim and valueDelim is equal the source string follow the next rule:
- // every even item of pairs is a properies's name, and the subsequent odd item is a property's value.
- if propDelim == valueDelim {
- // Taking into account the rule above, a valid source string must be splitted by propDelim
- // to an array with an even number of items.
- if len(pairs)%2 != 0 {
- return nil, &ParseError{
- Kind: KindInvalidFormat,
- Value: src,
- Reason: fmt.Sprintf("a value must be a list of object's properties in format \"name%svalue\" separated by %s", valueDelim, propDelim),
- }
- }
- for i := 0; i < len(pairs)/2; i++ {
- props[pairs[i*2]] = pairs[i*2+1]
- }
- return props, nil
- }
-
- // When propDelim and valueDelim is not equal the source string follow the next rule:
- // every item of pairs is a string that follows format .
- for _, pair := range pairs {
- prop := strings.Split(pair, valueDelim)
- if len(prop) != 2 {
- return nil, &ParseError{
- Kind: KindInvalidFormat,
- Value: src,
- Reason: fmt.Sprintf("a value must be a list of object's properties in format \"name%svalue\" separated by %s", valueDelim, propDelim),
- }
- }
- props[prop[0]] = prop[1]
- }
- return props, nil
-}
-
-// makeObject returns an object that contains properties from props.
-// A value of every property is parsed as a primitive value.
-// The function returns an error when an error happened while parse object's properties.
-func makeObject(props map[string]string, schema *openapi3.SchemaRef) (map[string]interface{}, error) {
- obj := make(map[string]interface{})
- for propName, propSchema := range schema.Value.Properties {
- value, err := parsePrimitive(props[propName], propSchema)
- if err != nil {
- if v, ok := err.(*ParseError); ok {
- return nil, &ParseError{path: []interface{}{propName}, Cause: v}
- }
- return nil, fmt.Errorf("property %q: %w", propName, err)
- }
- obj[propName] = value
- }
- return obj, nil
-}
-
-// parseArray returns an array that contains items from a raw array.
-// Every item is parsed as a primitive value.
-// The function returns an error when an error happened while parse array's items.
-func parseArray(raw []string, schemaRef *openapi3.SchemaRef) ([]interface{}, error) {
- var value []interface{}
- for i, v := range raw {
- item, err := parsePrimitive(v, schemaRef.Value.Items)
- if err != nil {
- if v, ok := err.(*ParseError); ok {
- return nil, &ParseError{path: []interface{}{i}, Cause: v}
- }
- return nil, fmt.Errorf("item %d: %w", i, err)
- }
-
- // If the items are nil, then the array is nil. There shouldn't be case where some values are actual primitive
- // values and some are nil values.
- if item == nil {
- return nil, nil
- }
- value = append(value, item)
- }
- return value, nil
-}
-
-// parsePrimitive returns a value that is created by parsing a source string to a primitive type
-// that is specified by a schema. The function returns nil when the source string is empty.
-// The function panics when a schema has a non-primitive type.
-func parsePrimitive(raw string, schema *openapi3.SchemaRef) (interface{}, error) {
- if raw == "" {
- return nil, nil
- }
- switch schema.Value.Type {
- case "integer":
- if schema.Value.Format == "int32" {
- v, err := strconv.ParseInt(raw, 0, 32)
- if err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Value: raw, Reason: "an invalid " + schema.Value.Type, Cause: err.(*strconv.NumError).Err}
- }
- return int32(v), nil
- }
- v, err := strconv.ParseInt(raw, 0, 64)
- if err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Value: raw, Reason: "an invalid " + schema.Value.Type, Cause: err.(*strconv.NumError).Err}
- }
- return v, nil
- case "number":
- v, err := strconv.ParseFloat(raw, 64)
- if err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Value: raw, Reason: "an invalid " + schema.Value.Type, Cause: err.(*strconv.NumError).Err}
- }
- return v, nil
- case "boolean":
- v, err := strconv.ParseBool(raw)
- if err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Value: raw, Reason: "an invalid " + schema.Value.Type, Cause: err.(*strconv.NumError).Err}
- }
- return v, nil
- case "string":
- return raw, nil
- default:
- panic(fmt.Sprintf("schema has non primitive type %q", schema.Value.Type))
- }
-}
-
-// EncodingFn is a function that returns an encoding of a request body's part.
-type EncodingFn func(partName string) *openapi3.Encoding
-
-// BodyDecoder is an interface to decode a body of a request or response.
-// An implementation must return a value that is a primitive, []interface{}, or map[string]interface{}.
-type BodyDecoder func(io.Reader, http.Header, *openapi3.SchemaRef, EncodingFn) (interface{}, error)
-
-// bodyDecoders contains decoders for supported content types of a body.
-// By default, there is content type "application/json" is supported only.
-var bodyDecoders = make(map[string]BodyDecoder)
-
-// RegisteredBodyDecoder returns the registered body decoder for the given content type.
-//
-// If no decoder was registered for the given content type, nil is returned.
-// This call is not thread-safe: body decoders should not be created/destroyed by multiple goroutines.
-func RegisteredBodyDecoder(contentType string) BodyDecoder {
- return bodyDecoders[contentType]
-}
-
-// RegisterBodyDecoder registers a request body's decoder for a content type.
-//
-// If a decoder for the specified content type already exists, the function replaces
-// it with the specified decoder.
-// This call is not thread-safe: body decoders should not be created/destroyed by multiple goroutines.
-func RegisterBodyDecoder(contentType string, decoder BodyDecoder) {
- if contentType == "" {
- panic("contentType is empty")
- }
- if decoder == nil {
- panic("decoder is not defined")
- }
- bodyDecoders[contentType] = decoder
-}
-
-// UnregisterBodyDecoder dissociates a body decoder from a content type.
-//
-// Decoding this content type will result in an error.
-// This call is not thread-safe: body decoders should not be created/destroyed by multiple goroutines.
-func UnregisterBodyDecoder(contentType string) {
- if contentType == "" {
- panic("contentType is empty")
- }
- delete(bodyDecoders, contentType)
-}
-
-var headerCT = http.CanonicalHeaderKey("Content-Type")
-
-const prefixUnsupportedCT = "unsupported content type"
-
-// decodeBody returns a decoded body.
-// The function returns ParseError when a body is invalid.
-func decodeBody(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (
- string,
- interface{},
- error,
-) {
- contentType := header.Get(headerCT)
- if contentType == "" {
- if _, ok := body.(*multipart.Part); ok {
- contentType = "text/plain"
- }
- }
- mediaType := parseMediaType(contentType)
- decoder, ok := bodyDecoders[mediaType]
- if !ok {
- return "", nil, &ParseError{
- Kind: KindUnsupportedFormat,
- Reason: fmt.Sprintf("%s %q", prefixUnsupportedCT, mediaType),
- }
- }
- value, err := decoder(body, header, schema, encFn)
- if err != nil {
- return "", nil, err
- }
- return mediaType, value, nil
-}
-
-func init() {
- RegisterBodyDecoder("application/json", jsonBodyDecoder)
- RegisterBodyDecoder("application/json-patch+json", jsonBodyDecoder)
- RegisterBodyDecoder("application/octet-stream", FileBodyDecoder)
- RegisterBodyDecoder("application/problem+json", jsonBodyDecoder)
- RegisterBodyDecoder("application/x-www-form-urlencoded", urlencodedBodyDecoder)
- RegisterBodyDecoder("application/x-yaml", yamlBodyDecoder)
- RegisterBodyDecoder("application/yaml", yamlBodyDecoder)
- RegisterBodyDecoder("application/zip", zipFileBodyDecoder)
- RegisterBodyDecoder("multipart/form-data", multipartBodyDecoder)
- RegisterBodyDecoder("text/csv", csvBodyDecoder)
- RegisterBodyDecoder("text/plain", plainBodyDecoder)
-}
-
-func plainBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- data, err := ioutil.ReadAll(body)
- if err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Cause: err}
- }
- return string(data), nil
-}
-
-func jsonBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- var value interface{}
- dec := json.NewDecoder(body)
- dec.UseNumber()
- if err := dec.Decode(&value); err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Cause: err}
- }
- return value, nil
-}
-
-func yamlBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- var value interface{}
- if err := yaml.NewDecoder(body).Decode(&value); err != nil {
- return nil, &ParseError{Kind: KindInvalidFormat, Cause: err}
- }
- return value, nil
-}
-
-func urlencodedBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- // Validate schema of request body.
- // By the OpenAPI 3 specification request body's schema must have type "object".
- // Properties of the schema describes individual parts of request body.
- if schema.Value.Type != "object" {
- return nil, errors.New("unsupported schema of request body")
- }
- for propName, propSchema := range schema.Value.Properties {
- switch propSchema.Value.Type {
- case "object":
- return nil, fmt.Errorf("unsupported schema of request body's property %q", propName)
- case "array":
- items := propSchema.Value.Items.Value
- if items.Type != "string" && items.Type != "integer" && items.Type != "number" && items.Type != "boolean" {
- return nil, fmt.Errorf("unsupported schema of request body's property %q", propName)
- }
- }
- }
-
- // Parse form.
- b, err := ioutil.ReadAll(body)
- if err != nil {
- return nil, err
- }
- values, err := url.ParseQuery(string(b))
- if err != nil {
- return nil, err
- }
-
- // Make an object value from form values.
- obj := make(map[string]interface{})
- dec := &urlValuesDecoder{values: values}
- for name, prop := range schema.Value.Properties {
- var (
- value interface{}
- enc *openapi3.Encoding
- )
- if encFn != nil {
- enc = encFn(name)
- }
- sm := enc.SerializationMethod()
-
- if value, _, err = decodeValue(dec, name, sm, prop, false); err != nil {
- return nil, err
- }
- obj[name] = value
- }
-
- return obj, nil
-}
-
-func multipartBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- if schema.Value.Type != "object" {
- return nil, errors.New("unsupported schema of request body")
- }
-
- // Parse form.
- values := make(map[string][]interface{})
- contentType := header.Get(headerCT)
- _, params, err := mime.ParseMediaType(contentType)
- if err != nil {
- return nil, err
- }
- mr := multipart.NewReader(body, params["boundary"])
- for {
- var part *multipart.Part
- if part, err = mr.NextPart(); err == io.EOF {
- break
- }
- if err != nil {
- return nil, err
- }
-
- var (
- name = part.FormName()
- enc *openapi3.Encoding
- )
- if encFn != nil {
- enc = encFn(name)
- }
- subEncFn := func(string) *openapi3.Encoding { return enc }
-
- var valueSchema *openapi3.SchemaRef
- if len(schema.Value.AllOf) > 0 {
- var exists bool
- for _, sr := range schema.Value.AllOf {
- if valueSchema, exists = sr.Value.Properties[name]; exists {
- break
- }
- }
- if !exists {
- return nil, &ParseError{Kind: KindOther, Cause: fmt.Errorf("part %s: undefined", name)}
- }
- } else {
- // If the property's schema has type "array" it is means that the form contains a few parts with the same name.
- // Every such part has a type that is defined by an items schema in the property's schema.
- var exists bool
- if valueSchema, exists = schema.Value.Properties[name]; !exists {
- if anyProperties := schema.Value.AdditionalProperties.Has; anyProperties != nil {
- switch *anyProperties {
- case true:
- //additionalProperties: true
- continue
- default:
- //additionalProperties: false
- return nil, &ParseError{Kind: KindOther, Cause: fmt.Errorf("part %s: undefined", name)}
- }
- }
- if schema.Value.AdditionalProperties.Schema == nil {
- return nil, &ParseError{Kind: KindOther, Cause: fmt.Errorf("part %s: undefined", name)}
- }
- if valueSchema, exists = schema.Value.AdditionalProperties.Schema.Value.Properties[name]; !exists {
- return nil, &ParseError{Kind: KindOther, Cause: fmt.Errorf("part %s: undefined", name)}
- }
- }
- if valueSchema.Value.Type == "array" {
- valueSchema = valueSchema.Value.Items
- }
- }
-
- var value interface{}
- if _, value, err = decodeBody(part, http.Header(part.Header), valueSchema, subEncFn); err != nil {
- if v, ok := err.(*ParseError); ok {
- return nil, &ParseError{path: []interface{}{name}, Cause: v}
- }
- return nil, fmt.Errorf("part %s: %w", name, err)
- }
- values[name] = append(values[name], value)
- }
-
- allTheProperties := make(map[string]*openapi3.SchemaRef)
- if len(schema.Value.AllOf) > 0 {
- for _, sr := range schema.Value.AllOf {
- for k, v := range sr.Value.Properties {
- allTheProperties[k] = v
- }
- if addProps := sr.Value.AdditionalProperties.Schema; addProps != nil {
- for k, v := range addProps.Value.Properties {
- allTheProperties[k] = v
- }
- }
- }
- } else {
- for k, v := range schema.Value.Properties {
- allTheProperties[k] = v
- }
- if addProps := schema.Value.AdditionalProperties.Schema; addProps != nil {
- for k, v := range addProps.Value.Properties {
- allTheProperties[k] = v
- }
- }
- }
-
- // Make an object value from form values.
- obj := make(map[string]interface{})
- for name, prop := range allTheProperties {
- vv := values[name]
- if len(vv) == 0 {
- continue
- }
- if prop.Value.Type == "array" {
- obj[name] = vv
- } else {
- obj[name] = vv[0]
- }
- }
-
- return obj, nil
-}
-
-// FileBodyDecoder is a body decoder that decodes a file body to a string.
-func FileBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- data, err := ioutil.ReadAll(body)
- if err != nil {
- return nil, err
- }
- return string(data), nil
-}
-
-// zipFileBodyDecoder is a body decoder that decodes a zip file body to a string.
-func zipFileBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- buff := bytes.NewBuffer([]byte{})
- size, err := io.Copy(buff, body)
- if err != nil {
- return nil, err
- }
-
- zr, err := zip.NewReader(bytes.NewReader(buff.Bytes()), size)
- if err != nil {
- return nil, err
- }
-
- const bufferSize = 256
- content := make([]byte, 0, bufferSize*len(zr.File))
- buffer := make([]byte /*0,*/, bufferSize)
-
- for _, f := range zr.File {
- err := func() error {
- rc, err := f.Open()
- if err != nil {
- return err
- }
- defer func() {
- _ = rc.Close()
- }()
-
- for {
- n, err := rc.Read(buffer)
- if 0 < n {
- content = append(content, buffer...)
- }
- if err == io.EOF {
- break
- }
- if err != nil {
- return err
- }
- }
-
- return nil
- }()
-
- if err != nil {
- return nil, err
- }
- }
-
- return string(content), nil
-}
-
-// csvBodyDecoder is a body decoder that decodes a csv body to a string.
-func csvBodyDecoder(body io.Reader, header http.Header, schema *openapi3.SchemaRef, encFn EncodingFn) (interface{}, error) {
- r := csv.NewReader(body)
-
- var content string
- for {
- record, err := r.Read()
- if err == io.EOF {
- break
- }
- if err != nil {
- return nil, err
- }
-
- content += strings.Join(record, ",") + "\n"
- }
-
- return content, nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/req_resp_encoder.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/req_resp_encoder.go
deleted file mode 100644
index 36b7db6f..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/req_resp_encoder.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package openapi3filter
-
-import (
- "encoding/json"
- "fmt"
-)
-
-func encodeBody(body interface{}, mediaType string) ([]byte, error) {
- encoder, ok := bodyEncoders[mediaType]
- if !ok {
- return nil, &ParseError{
- Kind: KindUnsupportedFormat,
- Reason: fmt.Sprintf("%s %q", prefixUnsupportedCT, mediaType),
- }
- }
- return encoder(body)
-}
-
-type BodyEncoder func(body interface{}) ([]byte, error)
-
-var bodyEncoders = map[string]BodyEncoder{
- "application/json": json.Marshal,
-}
-
-func RegisterBodyEncoder(contentType string, encoder BodyEncoder) {
- if contentType == "" {
- panic("contentType is empty")
- }
- if encoder == nil {
- panic("encoder is not defined")
- }
- bodyEncoders[contentType] = encoder
-}
-
-// This call is not thread-safe: body encoders should not be created/destroyed by multiple goroutines.
-func UnregisterBodyEncoder(contentType string) {
- if contentType == "" {
- panic("contentType is empty")
- }
- delete(bodyEncoders, contentType)
-}
-
-// RegisteredBodyEncoder returns the registered body encoder for the given content type.
-//
-// If no encoder was registered for the given content type, nil is returned.
-// This call is not thread-safe: body encoders should not be created/destroyed by multiple goroutines.
-func RegisteredBodyEncoder(contentType string) BodyEncoder {
- return bodyEncoders[contentType]
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_request.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_request.go
deleted file mode 100644
index a8106a7c..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_request.go
+++ /dev/null
@@ -1,398 +0,0 @@
-package openapi3filter
-
-import (
- "bytes"
- "context"
- "errors"
- "fmt"
- "io"
- "io/ioutil"
- "net/http"
- "sort"
-
- "github.com/getkin/kin-openapi/openapi3"
-)
-
-// ErrAuthenticationServiceMissing is returned when no authentication service
-// is defined for the request validator
-var ErrAuthenticationServiceMissing = errors.New("missing AuthenticationFunc")
-
-// ErrInvalidRequired is returned when a required value of a parameter or request body is not defined.
-var ErrInvalidRequired = errors.New("value is required but missing")
-
-// ErrInvalidEmptyValue is returned when a value of a parameter or request body is empty while it's not allowed.
-var ErrInvalidEmptyValue = errors.New("empty value is not allowed")
-
-// ValidateRequest is used to validate the given input according to previous
-// loaded OpenAPIv3 spec. If the input does not match the OpenAPIv3 spec, a
-// non-nil error will be returned.
-//
-// Note: One can tune the behavior of uniqueItems: true verification
-// by registering a custom function with openapi3.RegisterArrayUniqueItemsChecker
-func ValidateRequest(ctx context.Context, input *RequestValidationInput) (err error) {
- var me openapi3.MultiError
-
- options := input.Options
- if options == nil {
- options = DefaultOptions
- }
- route := input.Route
- operation := route.Operation
- operationParameters := operation.Parameters
- pathItemParameters := route.PathItem.Parameters
-
- // Security
- security := operation.Security
- // If there aren't any security requirements for the operation
- if security == nil {
- // Use the global security requirements.
- security = &route.Spec.Security
- }
- if security != nil {
- if err = ValidateSecurityRequirements(ctx, input, *security); err != nil && !options.MultiError {
- return
- }
- if err != nil {
- me = append(me, err)
- }
- }
-
- // For each parameter of the PathItem
- for _, parameterRef := range pathItemParameters {
- parameter := parameterRef.Value
- if operationParameters != nil {
- if override := operationParameters.GetByInAndName(parameter.In, parameter.Name); override != nil {
- continue
- }
- }
-
- if err = ValidateParameter(ctx, input, parameter); err != nil && !options.MultiError {
- return
- }
- if err != nil {
- me = append(me, err)
- }
- }
-
- // For each parameter of the Operation
- for _, parameter := range operationParameters {
- if err = ValidateParameter(ctx, input, parameter.Value); err != nil && !options.MultiError {
- return
- }
- if err != nil {
- me = append(me, err)
- }
- }
-
- // RequestBody
- requestBody := operation.RequestBody
- if requestBody != nil && !options.ExcludeRequestBody {
- if err = ValidateRequestBody(ctx, input, requestBody.Value); err != nil && !options.MultiError {
- return
- }
- if err != nil {
- me = append(me, err)
- }
- }
-
- if len(me) > 0 {
- return me
- }
- return
-}
-
-// ValidateParameter validates a parameter's value by JSON schema.
-// The function returns RequestError with a ParseError cause when unable to parse a value.
-// The function returns RequestError with ErrInvalidRequired cause when a value of a required parameter is not defined.
-// The function returns RequestError with ErrInvalidEmptyValue cause when a value of a required parameter is not defined.
-// The function returns RequestError with a openapi3.SchemaError cause when a value is invalid by JSON schema.
-func ValidateParameter(ctx context.Context, input *RequestValidationInput, parameter *openapi3.Parameter) error {
- if parameter.Schema == nil && parameter.Content == nil {
- // We have no schema for the parameter. Assume that everything passes
- // a schema-less check, but this could also be an error. The OpenAPI
- // validation allows this to happen.
- return nil
- }
-
- options := input.Options
- if options == nil {
- options = DefaultOptions
- }
-
- var value interface{}
- var err error
- var found bool
- var schema *openapi3.Schema
-
- // Validation will ensure that we either have content or schema.
- if parameter.Content != nil {
- if value, schema, found, err = decodeContentParameter(parameter, input); err != nil {
- return &RequestError{Input: input, Parameter: parameter, Err: err}
- }
- } else {
- if value, found, err = decodeStyledParameter(parameter, input); err != nil {
- return &RequestError{Input: input, Parameter: parameter, Err: err}
- }
- schema = parameter.Schema.Value
- }
-
- // Set default value if needed
- if !options.SkipSettingDefaults && value == nil && schema != nil && schema.Default != nil {
- value = schema.Default
- req := input.Request
- switch parameter.In {
- case openapi3.ParameterInPath:
- // Path parameters are required.
- // Next check `parameter.Required && !found` will catch this.
- case openapi3.ParameterInQuery:
- q := req.URL.Query()
- q.Add(parameter.Name, fmt.Sprintf("%v", value))
- req.URL.RawQuery = q.Encode()
- case openapi3.ParameterInHeader:
- req.Header.Add(parameter.Name, fmt.Sprintf("%v", value))
- case openapi3.ParameterInCookie:
- req.AddCookie(&http.Cookie{
- Name: parameter.Name,
- Value: fmt.Sprintf("%v", value),
- })
- }
- }
-
- // Validate a parameter's value and presence.
- if parameter.Required && !found {
- return &RequestError{Input: input, Parameter: parameter, Reason: ErrInvalidRequired.Error(), Err: ErrInvalidRequired}
- }
-
- if isNilValue(value) {
- if !parameter.AllowEmptyValue && found {
- return &RequestError{Input: input, Parameter: parameter, Reason: ErrInvalidEmptyValue.Error(), Err: ErrInvalidEmptyValue}
- }
- return nil
- }
- if schema == nil {
- // A parameter's schema is not defined so skip validation of a parameter's value.
- return nil
- }
-
- var opts []openapi3.SchemaValidationOption
- if options.MultiError {
- opts = make([]openapi3.SchemaValidationOption, 0, 1)
- opts = append(opts, openapi3.MultiErrors())
- }
- if options.customSchemaErrorFunc != nil {
- opts = append(opts, openapi3.SetSchemaErrorMessageCustomizer(options.customSchemaErrorFunc))
- }
- if err = schema.VisitJSON(value, opts...); err != nil {
- return &RequestError{Input: input, Parameter: parameter, Err: err}
- }
- return nil
-}
-
-const prefixInvalidCT = "header Content-Type has unexpected value"
-
-// ValidateRequestBody validates data of a request's body.
-//
-// The function returns RequestError with ErrInvalidRequired cause when a value is required but not defined.
-// The function returns RequestError with a openapi3.SchemaError cause when a value is invalid by JSON schema.
-func ValidateRequestBody(ctx context.Context, input *RequestValidationInput, requestBody *openapi3.RequestBody) error {
- var (
- req = input.Request
- data []byte
- )
-
- options := input.Options
- if options == nil {
- options = DefaultOptions
- }
-
- if req.Body != http.NoBody && req.Body != nil {
- defer req.Body.Close()
- var err error
- if data, err = ioutil.ReadAll(req.Body); err != nil {
- return &RequestError{
- Input: input,
- RequestBody: requestBody,
- Reason: "reading failed",
- Err: err,
- }
- }
- // Put the data back into the input
- req.Body = nil
- if req.GetBody != nil {
- if req.Body, err = req.GetBody(); err != nil {
- req.Body = nil
- }
- }
- if req.Body == nil {
- req.ContentLength = int64(len(data))
- req.GetBody = func() (io.ReadCloser, error) {
- return io.NopCloser(bytes.NewReader(data)), nil
- }
- req.Body, _ = req.GetBody() // no error return
- }
- }
-
- if len(data) == 0 {
- if requestBody.Required {
- return &RequestError{Input: input, RequestBody: requestBody, Err: ErrInvalidRequired}
- }
- return nil
- }
-
- content := requestBody.Content
- if len(content) == 0 {
- // A request's body does not have declared content, so skip validation.
- return nil
- }
-
- inputMIME := req.Header.Get(headerCT)
- contentType := requestBody.Content.Get(inputMIME)
- if contentType == nil {
- return &RequestError{
- Input: input,
- RequestBody: requestBody,
- Reason: fmt.Sprintf("%s %q", prefixInvalidCT, inputMIME),
- }
- }
-
- if contentType.Schema == nil {
- // A JSON schema that describes the received data is not declared, so skip validation.
- return nil
- }
-
- encFn := func(name string) *openapi3.Encoding { return contentType.Encoding[name] }
- mediaType, value, err := decodeBody(bytes.NewReader(data), req.Header, contentType.Schema, encFn)
- if err != nil {
- return &RequestError{
- Input: input,
- RequestBody: requestBody,
- Reason: "failed to decode request body",
- Err: err,
- }
- }
-
- defaultsSet := false
- opts := make([]openapi3.SchemaValidationOption, 0, 4) // 4 potential opts here
- opts = append(opts, openapi3.VisitAsRequest())
- if !options.SkipSettingDefaults {
- opts = append(opts, openapi3.DefaultsSet(func() { defaultsSet = true }))
- }
- if options.MultiError {
- opts = append(opts, openapi3.MultiErrors())
- }
- if options.customSchemaErrorFunc != nil {
- opts = append(opts, openapi3.SetSchemaErrorMessageCustomizer(options.customSchemaErrorFunc))
- }
- if options.ExcludeReadOnlyValidations {
- opts = append(opts, openapi3.DisableReadOnlyValidation())
- }
-
- // Validate JSON with the schema
- if err := contentType.Schema.Value.VisitJSON(value, opts...); err != nil {
- schemaId := getSchemaIdentifier(contentType.Schema)
- schemaId = prependSpaceIfNeeded(schemaId)
- return &RequestError{
- Input: input,
- RequestBody: requestBody,
- Reason: fmt.Sprintf("doesn't match schema%s", schemaId),
- Err: err,
- }
- }
-
- if defaultsSet {
- var err error
- if data, err = encodeBody(value, mediaType); err != nil {
- return &RequestError{
- Input: input,
- RequestBody: requestBody,
- Reason: "rewriting failed",
- Err: err,
- }
- }
- // Put the data back into the input
- if req.Body != nil {
- req.Body.Close()
- }
- req.ContentLength = int64(len(data))
- req.GetBody = func() (io.ReadCloser, error) {
- return io.NopCloser(bytes.NewReader(data)), nil
- }
- req.Body, _ = req.GetBody() // no error return
- }
-
- return nil
-}
-
-// ValidateSecurityRequirements goes through multiple OpenAPI 3 security
-// requirements in order and returns nil on the first valid requirement.
-// If no requirement is met, errors are returned in order.
-func ValidateSecurityRequirements(ctx context.Context, input *RequestValidationInput, srs openapi3.SecurityRequirements) error {
- if len(srs) == 0 {
- return nil
- }
- var errs []error
- for _, sr := range srs {
- if err := validateSecurityRequirement(ctx, input, sr); err != nil {
- if len(errs) == 0 {
- errs = make([]error, 0, len(srs))
- }
- errs = append(errs, err)
- continue
- }
- return nil
- }
- return &SecurityRequirementsError{
- SecurityRequirements: srs,
- Errors: errs,
- }
-}
-
-// validateSecurityRequirement validates a single OpenAPI 3 security requirement
-func validateSecurityRequirement(ctx context.Context, input *RequestValidationInput, securityRequirement openapi3.SecurityRequirement) error {
- names := make([]string, 0, len(securityRequirement))
- for name := range securityRequirement {
- names = append(names, name)
- }
- sort.Strings(names)
-
- // Get authentication function
- options := input.Options
- if options == nil {
- options = DefaultOptions
- }
- f := options.AuthenticationFunc
- if f == nil {
- return ErrAuthenticationServiceMissing
- }
-
- var securitySchemes openapi3.SecuritySchemes
- if components := input.Route.Spec.Components; components != nil {
- securitySchemes = components.SecuritySchemes
- }
-
- // For each scheme for the requirement
- for _, name := range names {
- var securityScheme *openapi3.SecurityScheme
- if securitySchemes != nil {
- if ref := securitySchemes[name]; ref != nil {
- securityScheme = ref.Value
- }
- }
- if securityScheme == nil {
- return &RequestError{
- Input: input,
- Err: fmt.Errorf("security scheme %q is not declared", name),
- }
- }
- scopes := securityRequirement[name]
- if err := f(ctx, &AuthenticationInput{
- RequestValidationInput: input,
- SecuritySchemeName: name,
- SecurityScheme: securityScheme,
- Scopes: scopes,
- }); err != nil {
- return err
- }
- }
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_request_input.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_request_input.go
deleted file mode 100644
index 91dd102b..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_request_input.go
+++ /dev/null
@@ -1,38 +0,0 @@
-package openapi3filter
-
-import (
- "net/http"
- "net/url"
-
- "github.com/getkin/kin-openapi/openapi3"
- "github.com/getkin/kin-openapi/routers"
-)
-
-// A ContentParameterDecoder takes a parameter definition from the OpenAPI spec,
-// and the value which we received for it. It is expected to return the
-// value unmarshaled into an interface which can be traversed for
-// validation, it should also return the schema to be used for validating the
-// object, since there can be more than one in the content spec.
-//
-// If a query parameter appears multiple times, values[] will have more
-// than one value, but for all other parameter types it should have just
-// one.
-type ContentParameterDecoder func(param *openapi3.Parameter, values []string) (interface{}, *openapi3.Schema, error)
-
-type RequestValidationInput struct {
- Request *http.Request
- PathParams map[string]string
- QueryParams url.Values
- Route *routers.Route
- Options *Options
- ParamDecoder ContentParameterDecoder
-}
-
-func (input *RequestValidationInput) GetQueryParams() url.Values {
- q := input.QueryParams
- if q == nil {
- q = input.Request.URL.Query()
- input.QueryParams = q
- }
- return q
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_response.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_response.go
deleted file mode 100644
index 08ea4e19..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_response.go
+++ /dev/null
@@ -1,224 +0,0 @@
-// Package openapi3filter validates that requests and inputs request an OpenAPI 3 specification file.
-package openapi3filter
-
-import (
- "bytes"
- "context"
- "fmt"
- "io/ioutil"
- "net/http"
- "sort"
- "strings"
-
- "github.com/getkin/kin-openapi/openapi3"
-)
-
-// ValidateResponse is used to validate the given input according to previous
-// loaded OpenAPIv3 spec. If the input does not match the OpenAPIv3 spec, a
-// non-nil error will be returned.
-//
-// Note: One can tune the behavior of uniqueItems: true verification
-// by registering a custom function with openapi3.RegisterArrayUniqueItemsChecker
-func ValidateResponse(ctx context.Context, input *ResponseValidationInput) error {
- req := input.RequestValidationInput.Request
- switch req.Method {
- case "HEAD":
- return nil
- }
- status := input.Status
-
- // These status codes will never be validated.
- // TODO: The list is probably missing some.
- switch status {
- case http.StatusNotModified,
- http.StatusPermanentRedirect,
- http.StatusTemporaryRedirect,
- http.StatusMovedPermanently:
- return nil
- }
- route := input.RequestValidationInput.Route
- options := input.Options
- if options == nil {
- options = DefaultOptions
- }
-
- // Find input for the current status
- responses := route.Operation.Responses
- if len(responses) == 0 {
- return nil
- }
- responseRef := responses.Get(status) // Response
- if responseRef == nil {
- responseRef = responses.Default() // Default input
- }
- if responseRef == nil {
- // By default, status that is not documented is allowed.
- if !options.IncludeResponseStatus {
- return nil
- }
- return &ResponseError{Input: input, Reason: "status is not supported"}
- }
- response := responseRef.Value
- if response == nil {
- return &ResponseError{Input: input, Reason: "response has not been resolved"}
- }
-
- opts := make([]openapi3.SchemaValidationOption, 0, 3) // 3 potential options here
- if options.MultiError {
- opts = append(opts, openapi3.MultiErrors())
- }
- if options.customSchemaErrorFunc != nil {
- opts = append(opts, openapi3.SetSchemaErrorMessageCustomizer(options.customSchemaErrorFunc))
- }
- if options.ExcludeWriteOnlyValidations {
- opts = append(opts, openapi3.DisableWriteOnlyValidation())
- }
-
- headers := make([]string, 0, len(response.Headers))
- for k := range response.Headers {
- if k != headerCT {
- headers = append(headers, k)
- }
- }
- sort.Strings(headers)
- for _, headerName := range headers {
- headerRef := response.Headers[headerName]
- if err := validateResponseHeader(headerName, headerRef, input, opts); err != nil {
- return err
- }
- }
-
- if options.ExcludeResponseBody {
- // A user turned off validation of a response's body.
- return nil
- }
-
- content := response.Content
- if len(content) == 0 || options.ExcludeResponseBody {
- // An operation does not contains a validation schema for responses with this status code.
- return nil
- }
-
- inputMIME := input.Header.Get(headerCT)
- contentType := content.Get(inputMIME)
- if contentType == nil {
- return &ResponseError{
- Input: input,
- Reason: fmt.Sprintf("response header Content-Type has unexpected value: %q", inputMIME),
- }
- }
-
- if contentType.Schema == nil {
- // An operation does not contains a validation schema for responses with this status code.
- return nil
- }
-
- // Read response's body.
- body := input.Body
-
- // Response would contain partial or empty input body
- // after we begin reading.
- // Ensure that this doesn't happen.
- input.Body = nil
-
- // Ensure we close the reader
- defer body.Close()
-
- // Read all
- data, err := ioutil.ReadAll(body)
- if err != nil {
- return &ResponseError{
- Input: input,
- Reason: "failed to read response body",
- Err: err,
- }
- }
-
- // Put the data back into the response.
- input.SetBodyBytes(data)
-
- encFn := func(name string) *openapi3.Encoding { return contentType.Encoding[name] }
- _, value, err := decodeBody(bytes.NewBuffer(data), input.Header, contentType.Schema, encFn)
- if err != nil {
- return &ResponseError{
- Input: input,
- Reason: "failed to decode response body",
- Err: err,
- }
- }
-
- // Validate data with the schema.
- if err := contentType.Schema.Value.VisitJSON(value, append(opts, openapi3.VisitAsResponse())...); err != nil {
- schemaId := getSchemaIdentifier(contentType.Schema)
- schemaId = prependSpaceIfNeeded(schemaId)
- return &ResponseError{
- Input: input,
- Reason: fmt.Sprintf("response body doesn't match schema%s", schemaId),
- Err: err,
- }
- }
- return nil
-}
-
-func validateResponseHeader(headerName string, headerRef *openapi3.HeaderRef, input *ResponseValidationInput, opts []openapi3.SchemaValidationOption) error {
- var err error
- var decodedValue interface{}
- var found bool
- var sm *openapi3.SerializationMethod
- dec := &headerParamDecoder{header: input.Header}
-
- if sm, err = headerRef.Value.SerializationMethod(); err != nil {
- return &ResponseError{
- Input: input,
- Reason: fmt.Sprintf("unable to get header %q serialization method", headerName),
- Err: err,
- }
- }
-
- if decodedValue, found, err = decodeValue(dec, headerName, sm, headerRef.Value.Schema, headerRef.Value.Required); err != nil {
- return &ResponseError{
- Input: input,
- Reason: fmt.Sprintf("unable to decode header %q value", headerName),
- Err: err,
- }
- }
-
- if found {
- if err = headerRef.Value.Schema.Value.VisitJSON(decodedValue, opts...); err != nil {
- return &ResponseError{
- Input: input,
- Reason: fmt.Sprintf("response header %q doesn't match schema", headerName),
- Err: err,
- }
- }
- } else if headerRef.Value.Required {
- return &ResponseError{
- Input: input,
- Reason: fmt.Sprintf("response header %q missing", headerName),
- }
- }
- return nil
-}
-
-// getSchemaIdentifier gets something by which a schema could be identified.
-// A schema by itself doesn't have a true identity field. This function makes
-// a best effort to get a value that can fill that void.
-func getSchemaIdentifier(schema *openapi3.SchemaRef) string {
- var id string
-
- if schema != nil {
- id = strings.TrimSpace(schema.Ref)
- }
- if id == "" && schema.Value != nil {
- id = strings.TrimSpace(schema.Value.Title)
- }
-
- return id
-}
-
-func prependSpaceIfNeeded(value string) string {
- if len(value) > 0 {
- value = " " + value
- }
- return value
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_response_input.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_response_input.go
deleted file mode 100644
index edf38730..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validate_response_input.go
+++ /dev/null
@@ -1,42 +0,0 @@
-package openapi3filter
-
-import (
- "bytes"
- "io"
- "io/ioutil"
- "net/http"
-)
-
-type ResponseValidationInput struct {
- RequestValidationInput *RequestValidationInput
- Status int
- Header http.Header
- Body io.ReadCloser
- Options *Options
-}
-
-func (input *ResponseValidationInput) SetBodyBytes(value []byte) *ResponseValidationInput {
- input.Body = ioutil.NopCloser(bytes.NewReader(value))
- return input
-}
-
-var JSONPrefixes = []string{
- ")]}',\n",
-}
-
-// TrimJSONPrefix trims one of the possible prefixes
-func TrimJSONPrefix(data []byte) []byte {
-search:
- for _, prefix := range JSONPrefixes {
- if len(data) < len(prefix) {
- continue
- }
- for i, b := range data[:len(prefix)] {
- if b != prefix[i] {
- continue search
- }
- }
- return data[len(prefix):]
- }
- return data
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_error.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_error.go
deleted file mode 100644
index 7e685cde..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_error.go
+++ /dev/null
@@ -1,85 +0,0 @@
-package openapi3filter
-
-import (
- "bytes"
- "strconv"
-)
-
-// ValidationError struct provides granular error information
-// useful for communicating issues back to end user and developer.
-// Based on https://jsonapi.org/format/#error-objects
-type ValidationError struct {
- // A unique identifier for this particular occurrence of the problem.
- Id string `json:"id,omitempty" yaml:"id,omitempty"`
- // The HTTP status code applicable to this problem.
- Status int `json:"status,omitempty" yaml:"status,omitempty"`
- // An application-specific error code, expressed as a string value.
- Code string `json:"code,omitempty" yaml:"code,omitempty"`
- // A short, human-readable summary of the problem. It **SHOULD NOT** change from occurrence to occurrence of the problem, except for purposes of localization.
- Title string `json:"title,omitempty" yaml:"title,omitempty"`
- // A human-readable explanation specific to this occurrence of the problem.
- Detail string `json:"detail,omitempty" yaml:"detail,omitempty"`
- // An object containing references to the source of the error
- Source *ValidationErrorSource `json:"source,omitempty" yaml:"source,omitempty"`
-}
-
-// ValidationErrorSource struct
-type ValidationErrorSource struct {
- // A JSON Pointer [RFC6901] to the associated entity in the request document [e.g. \"/data\" for a primary data object, or \"/data/attributes/title\" for a specific attribute].
- Pointer string `json:"pointer,omitempty" yaml:"pointer,omitempty"`
- // A string indicating which query parameter caused the error.
- Parameter string `json:"parameter,omitempty" yaml:"parameter,omitempty"`
-}
-
-var _ error = &ValidationError{}
-
-// Error implements the error interface.
-func (e *ValidationError) Error() string {
- b := new(bytes.Buffer)
- b.WriteString("[")
- if e.Status != 0 {
- b.WriteString(strconv.Itoa(e.Status))
- }
- b.WriteString("]")
- b.WriteString("[")
- if e.Code != "" {
- b.WriteString(e.Code)
- }
- b.WriteString("]")
- b.WriteString("[")
- if e.Id != "" {
- b.WriteString(e.Id)
- }
- b.WriteString("]")
- b.WriteString(" ")
- if e.Title != "" {
- b.WriteString(e.Title)
- b.WriteString(" ")
- }
- if e.Detail != "" {
- b.WriteString("| ")
- b.WriteString(e.Detail)
- b.WriteString(" ")
- }
- if e.Source != nil {
- b.WriteString("[source ")
- if e.Source.Parameter != "" {
- b.WriteString("parameter=")
- b.WriteString(e.Source.Parameter)
- } else if e.Source.Pointer != "" {
- b.WriteString("pointer=")
- b.WriteString(e.Source.Pointer)
- }
- b.WriteString("]")
- }
-
- if b.Len() == 0 {
- return "no error"
- }
- return b.String()
-}
-
-// StatusCode implements the StatusCoder interface for DefaultErrorEncoder
-func (e *ValidationError) StatusCode() int {
- return e.Status
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_error_encoder.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_error_encoder.go
deleted file mode 100644
index 779887db..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_error_encoder.go
+++ /dev/null
@@ -1,185 +0,0 @@
-package openapi3filter
-
-import (
- "context"
- "fmt"
- "net/http"
- "strings"
-
- "github.com/getkin/kin-openapi/openapi3"
- "github.com/getkin/kin-openapi/routers"
-)
-
-// ValidationErrorEncoder wraps a base ErrorEncoder to handle ValidationErrors
-type ValidationErrorEncoder struct {
- Encoder ErrorEncoder
-}
-
-// Encode implements the ErrorEncoder interface for encoding ValidationErrors
-func (enc *ValidationErrorEncoder) Encode(ctx context.Context, err error, w http.ResponseWriter) {
- if e, ok := err.(*routers.RouteError); ok {
- cErr := convertRouteError(e)
- enc.Encoder(ctx, cErr, w)
- return
- }
-
- e, ok := err.(*RequestError)
- if !ok {
- enc.Encoder(ctx, err, w)
- return
- }
-
- var cErr *ValidationError
- if e.Err == nil {
- cErr = convertBasicRequestError(e)
- } else if e.Err == ErrInvalidRequired {
- cErr = convertErrInvalidRequired(e)
- } else if e.Err == ErrInvalidEmptyValue {
- cErr = convertErrInvalidEmptyValue(e)
- } else if innerErr, ok := e.Err.(*ParseError); ok {
- cErr = convertParseError(e, innerErr)
- } else if innerErr, ok := e.Err.(*openapi3.SchemaError); ok {
- cErr = convertSchemaError(e, innerErr)
- }
-
- if cErr != nil {
- enc.Encoder(ctx, cErr, w)
- return
- }
- enc.Encoder(ctx, err, w)
-}
-
-func convertRouteError(e *routers.RouteError) *ValidationError {
- status := http.StatusNotFound
- if e.Error() == routers.ErrMethodNotAllowed.Error() {
- status = http.StatusMethodNotAllowed
- }
- return &ValidationError{Status: status, Title: e.Error()}
-}
-
-func convertBasicRequestError(e *RequestError) *ValidationError {
- if strings.HasPrefix(e.Reason, prefixInvalidCT) {
- if strings.HasSuffix(e.Reason, `""`) {
- return &ValidationError{
- Status: http.StatusUnsupportedMediaType,
- Title: "header Content-Type is required",
- }
- }
- return &ValidationError{
- Status: http.StatusUnsupportedMediaType,
- Title: prefixUnsupportedCT + strings.TrimPrefix(e.Reason, prefixInvalidCT),
- }
- }
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: e.Error(),
- }
-}
-
-func convertErrInvalidRequired(e *RequestError) *ValidationError {
- if e.Err == ErrInvalidRequired && e.Parameter != nil {
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: fmt.Sprintf("parameter %q in %s is required", e.Parameter.Name, e.Parameter.In),
- }
- }
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: e.Error(),
- }
-}
-
-func convertErrInvalidEmptyValue(e *RequestError) *ValidationError {
- if e.Err == ErrInvalidEmptyValue && e.Parameter != nil {
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: fmt.Sprintf("parameter %q in %s is not allowed to be empty", e.Parameter.Name, e.Parameter.In),
- }
- }
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: e.Error(),
- }
-}
-
-func convertParseError(e *RequestError, innerErr *ParseError) *ValidationError {
- // We treat path params of the wrong type like a 404 instead of a 400
- if innerErr.Kind == KindInvalidFormat && e.Parameter != nil && e.Parameter.In == "path" {
- return &ValidationError{
- Status: http.StatusNotFound,
- Title: fmt.Sprintf("resource not found with %q value: %v", e.Parameter.Name, innerErr.Value),
- }
- } else if strings.HasPrefix(innerErr.Reason, prefixUnsupportedCT) {
- return &ValidationError{
- Status: http.StatusUnsupportedMediaType,
- Title: innerErr.Reason,
- }
- } else if innerErr.RootCause() != nil {
- if rootErr, ok := innerErr.Cause.(*ParseError); ok &&
- rootErr.Kind == KindInvalidFormat && e.Parameter.In == "query" {
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: fmt.Sprintf("parameter %q in %s is invalid: %v is %s",
- e.Parameter.Name, e.Parameter.In, rootErr.Value, rootErr.Reason),
- }
- }
- return &ValidationError{
- Status: http.StatusBadRequest,
- Title: innerErr.Reason,
- }
- }
- return nil
-}
-
-func convertSchemaError(e *RequestError, innerErr *openapi3.SchemaError) *ValidationError {
- cErr := &ValidationError{Title: innerErr.Reason}
-
- // Handle "Origin" error
- if originErr, ok := innerErr.Origin.(*openapi3.SchemaError); ok {
- cErr = convertSchemaError(e, originErr)
- }
-
- // Add http status code
- if e.Parameter != nil {
- cErr.Status = http.StatusBadRequest
- } else if e.RequestBody != nil {
- cErr.Status = http.StatusUnprocessableEntity
- }
-
- // Add error source
- if e.Parameter != nil {
- // We have a JSONPointer in the query param too so need to
- // make sure 'Parameter' check takes priority over 'Pointer'
- cErr.Source = &ValidationErrorSource{Parameter: e.Parameter.Name}
- } else if ptr := innerErr.JSONPointer(); ptr != nil {
- cErr.Source = &ValidationErrorSource{Pointer: toJSONPointer(ptr)}
- }
-
- // Add details on allowed values for enums
- if innerErr.SchemaField == "enum" {
- enums := make([]string, 0, len(innerErr.Schema.Enum))
- for _, enum := range innerErr.Schema.Enum {
- enums = append(enums, fmt.Sprintf("%v", enum))
- }
- cErr.Detail = fmt.Sprintf("value %v at %s must be one of: %s",
- innerErr.Value,
- toJSONPointer(innerErr.JSONPointer()),
- strings.Join(enums, ", "))
- value := fmt.Sprintf("%v", innerErr.Value)
- if e.Parameter != nil &&
- (e.Parameter.Explode == nil || *e.Parameter.Explode) &&
- (e.Parameter.Style == "" || e.Parameter.Style == "form") &&
- strings.Contains(value, ",") {
- parts := strings.Split(value, ",")
- cErr.Detail = fmt.Sprintf("%s; perhaps you intended '?%s=%s'",
- cErr.Detail,
- e.Parameter.Name,
- strings.Join(parts, "&"+e.Parameter.Name+"="))
- }
- }
- return cErr
-}
-
-func toJSONPointer(reversePath []string) string {
- return "/" + strings.Join(reversePath, "/")
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_handler.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_handler.go
deleted file mode 100644
index d4bb1efa..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_handler.go
+++ /dev/null
@@ -1,107 +0,0 @@
-package openapi3filter
-
-import (
- "context"
- "net/http"
-
- "github.com/getkin/kin-openapi/openapi3"
- "github.com/getkin/kin-openapi/routers"
- legacyrouter "github.com/getkin/kin-openapi/routers/legacy"
-)
-
-// AuthenticationFunc allows for custom security requirement validation.
-// A non-nil error fails authentication according to https://spec.openapis.org/oas/v3.1.0#security-requirement-object
-// See ValidateSecurityRequirements
-type AuthenticationFunc func(context.Context, *AuthenticationInput) error
-
-// NoopAuthenticationFunc is an AuthenticationFunc
-func NoopAuthenticationFunc(context.Context, *AuthenticationInput) error { return nil }
-
-var _ AuthenticationFunc = NoopAuthenticationFunc
-
-type ValidationHandler struct {
- Handler http.Handler
- AuthenticationFunc AuthenticationFunc
- File string
- ErrorEncoder ErrorEncoder
- router routers.Router
-}
-
-func (h *ValidationHandler) Load() error {
- loader := openapi3.NewLoader()
- doc, err := loader.LoadFromFile(h.File)
- if err != nil {
- return err
- }
- if err := doc.Validate(loader.Context); err != nil {
- return err
- }
- if h.router, err = legacyrouter.NewRouter(doc); err != nil {
- return err
- }
-
- // set defaults
- if h.Handler == nil {
- h.Handler = http.DefaultServeMux
- }
- if h.AuthenticationFunc == nil {
- h.AuthenticationFunc = NoopAuthenticationFunc
- }
- if h.ErrorEncoder == nil {
- h.ErrorEncoder = DefaultErrorEncoder
- }
-
- return nil
-}
-
-func (h *ValidationHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
- if handled := h.before(w, r); handled {
- return
- }
- // TODO: validateResponse
- h.Handler.ServeHTTP(w, r)
-}
-
-// Middleware implements gorilla/mux MiddlewareFunc
-func (h *ValidationHandler) Middleware(next http.Handler) http.Handler {
- return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
- if handled := h.before(w, r); handled {
- return
- }
- // TODO: validateResponse
- next.ServeHTTP(w, r)
- })
-}
-
-func (h *ValidationHandler) before(w http.ResponseWriter, r *http.Request) (handled bool) {
- if err := h.validateRequest(r); err != nil {
- h.ErrorEncoder(r.Context(), err, w)
- return true
- }
- return false
-}
-
-func (h *ValidationHandler) validateRequest(r *http.Request) error {
- // Find route
- route, pathParams, err := h.router.FindRoute(r)
- if err != nil {
- return err
- }
-
- options := &Options{
- AuthenticationFunc: h.AuthenticationFunc,
- }
-
- // Validate request
- requestValidationInput := &RequestValidationInput{
- Request: r,
- PathParams: pathParams,
- Route: route,
- Options: options,
- }
- if err = ValidateRequest(r.Context(), requestValidationInput); err != nil {
- return err
- }
-
- return nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_kit.go b/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_kit.go
deleted file mode 100644
index 9e11e4fc..00000000
--- a/vendor/github.com/getkin/kin-openapi/openapi3filter/validation_kit.go
+++ /dev/null
@@ -1,85 +0,0 @@
-package openapi3filter
-
-import (
- "context"
- "encoding/json"
- "net/http"
-)
-
-///////////////////////////////////////////////////////////////////////////////////
-// We didn't want to tie kin-openapi too tightly with go-kit.
-// This file contains the ErrorEncoder and DefaultErrorEncoder function
-// borrowed from this project.
-//
-// The MIT License (MIT)
-//
-// Copyright (c) 2015 Peter Bourgon
-//
-// Permission is hereby granted, free of charge, to any person obtaining a copy
-// of this software and associated documentation files (the "Software"), to deal
-// in the Software without restriction, including without limitation the rights
-// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-// copies of the Software, and to permit persons to whom the Software is
-// furnished to do so, subject to the following conditions:
-//
-// The above copyright notice and this permission notice shall be included in all
-// copies or substantial portions of the Software.
-//
-// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-// SOFTWARE.
-///////////////////////////////////////////////////////////////////////////////////
-
-// ErrorEncoder is responsible for encoding an error to the ResponseWriter.
-// Users are encouraged to use custom ErrorEncoders to encode HTTP errors to
-// their clients, and will likely want to pass and check for their own error
-// types. See the example shipping/handling service.
-type ErrorEncoder func(ctx context.Context, err error, w http.ResponseWriter)
-
-// StatusCoder is checked by DefaultErrorEncoder. If an error value implements
-// StatusCoder, the StatusCode will be used when encoding the error. By default,
-// StatusInternalServerError (500) is used.
-type StatusCoder interface {
- StatusCode() int
-}
-
-// Headerer is checked by DefaultErrorEncoder. If an error value implements
-// Headerer, the provided headers will be applied to the response writer, after
-// the Content-Type is set.
-type Headerer interface {
- Headers() http.Header
-}
-
-// DefaultErrorEncoder writes the error to the ResponseWriter, by default a
-// content type of text/plain, a body of the plain text of the error, and a
-// status code of 500. If the error implements Headerer, the provided headers
-// will be applied to the response. If the error implements json.Marshaler, and
-// the marshaling succeeds, a content type of application/json and the JSON
-// encoded form of the error will be used. If the error implements StatusCoder,
-// the provided StatusCode will be used instead of 500.
-func DefaultErrorEncoder(_ context.Context, err error, w http.ResponseWriter) {
- contentType, body := "text/plain; charset=utf-8", []byte(err.Error())
- if marshaler, ok := err.(json.Marshaler); ok {
- if jsonBody, marshalErr := marshaler.MarshalJSON(); marshalErr == nil {
- contentType, body = "application/json; charset=utf-8", jsonBody
- }
- }
- w.Header().Set("Content-Type", contentType)
- if headerer, ok := err.(Headerer); ok {
- for k, values := range headerer.Headers() {
- for _, v := range values {
- w.Header().Add(k, v)
- }
- }
- }
- code := http.StatusInternalServerError
- if sc, ok := err.(StatusCoder); ok {
- code = sc.StatusCode()
- }
- w.WriteHeader(code)
- w.Write(body)
-}
diff --git a/vendor/github.com/getkin/kin-openapi/routers/legacy/pathpattern/node.go b/vendor/github.com/getkin/kin-openapi/routers/legacy/pathpattern/node.go
deleted file mode 100644
index 011dda35..00000000
--- a/vendor/github.com/getkin/kin-openapi/routers/legacy/pathpattern/node.go
+++ /dev/null
@@ -1,328 +0,0 @@
-// Package pathpattern implements path matching.
-//
-// Examples of supported patterns:
-// - "/"
-// - "/abc""
-// - "/abc/{variable}" (matches until next '/' or end-of-string)
-// - "/abc/{variable*}" (matches everything, including "/abc" if "/abc" has noot)
-// - "/abc/{ variable | prefix_(.*}_suffix }" (matches regular expressions)
-package pathpattern
-
-import (
- "bytes"
- "fmt"
- "regexp"
- "sort"
- "strings"
-)
-
-var DefaultOptions = &Options{
- SupportWildcard: true,
-}
-
-type Options struct {
- SupportWildcard bool
- SupportRegExp bool
-}
-
-// PathFromHost converts a host pattern to a path pattern.
-//
-// Examples:
-// - PathFromHost("some-subdomain.domain.com", false) -> "com/./domain/./some-subdomain"
-// - PathFromHost("some-subdomain.domain.com", true) -> "com/./domain/./subdomain/-/some"
-func PathFromHost(host string, specialDashes bool) string {
- buf := make([]byte, 0, len(host))
- end := len(host)
-
- // Go from end to start
- for start := end - 1; start >= 0; start-- {
- switch host[start] {
- case '.':
- buf = append(buf, host[start+1:end]...)
- buf = append(buf, '/', '.', '/')
- end = start
- case '-':
- if specialDashes {
- buf = append(buf, host[start+1:end]...)
- buf = append(buf, '/', '-', '/')
- end = start
- }
- }
- }
- buf = append(buf, host[:end]...)
- return string(buf)
-}
-
-type Node struct {
- VariableNames []string
- Value interface{}
- Suffixes SuffixList
-}
-
-func (currentNode *Node) String() string {
- buf := bytes.NewBuffer(make([]byte, 0, 255))
- currentNode.toBuffer(buf, "")
- return buf.String()
-}
-
-func (currentNode *Node) toBuffer(buf *bytes.Buffer, linePrefix string) {
- if value := currentNode.Value; value != nil {
- buf.WriteString(linePrefix)
- buf.WriteString("VALUE: ")
- fmt.Fprint(buf, value)
- buf.WriteString("\n")
- }
- suffixes := currentNode.Suffixes
- if len(suffixes) > 0 {
- newLinePrefix := linePrefix + " "
- for _, suffix := range suffixes {
- buf.WriteString(linePrefix)
- buf.WriteString("PATTERN: ")
- buf.WriteString(suffix.String())
- buf.WriteString("\n")
- suffix.Node.toBuffer(buf, newLinePrefix)
- }
- }
-}
-
-type SuffixKind int
-
-// Note that order is important!
-const (
- // SuffixKindConstant matches a constant string
- SuffixKindConstant = SuffixKind(iota)
-
- // SuffixKindRegExp matches a regular expression
- SuffixKindRegExp
-
- // SuffixKindVariable matches everything until '/'
- SuffixKindVariable
-
- // SuffixKindEverything matches everything (until end-of-string)
- SuffixKindEverything
-)
-
-// Suffix describes condition that
-type Suffix struct {
- Kind SuffixKind
- Pattern string
-
- // compiled regular expression
- regExp *regexp.Regexp
-
- // Next node
- Node *Node
-}
-
-func EqualSuffix(a, b Suffix) bool {
- return a.Kind == b.Kind && a.Pattern == b.Pattern
-}
-
-func (suffix Suffix) String() string {
- switch suffix.Kind {
- case SuffixKindConstant:
- return suffix.Pattern
- case SuffixKindVariable:
- return "{_}"
- case SuffixKindEverything:
- return "{_*}"
- default:
- return "{_|" + suffix.Pattern + "}"
- }
-}
-
-type SuffixList []Suffix
-
-func (list SuffixList) Less(i, j int) bool {
- a, b := list[i], list[j]
- ak, bk := a.Kind, b.Kind
- if ak < bk {
- return true
- } else if bk < ak {
- return false
- }
- return a.Pattern > b.Pattern
-}
-
-func (list SuffixList) Len() int {
- return len(list)
-}
-
-func (list SuffixList) Swap(i, j int) {
- a, b := list[i], list[j]
- list[i], list[j] = b, a
-}
-
-func (currentNode *Node) MustAdd(path string, value interface{}, options *Options) {
- node, err := currentNode.CreateNode(path, options)
- if err != nil {
- panic(err)
- }
- node.Value = value
-}
-
-func (currentNode *Node) Add(path string, value interface{}, options *Options) error {
- node, err := currentNode.CreateNode(path, options)
- if err != nil {
- return err
- }
- node.Value = value
- return nil
-}
-
-func (currentNode *Node) CreateNode(path string, options *Options) (*Node, error) {
- if options == nil {
- options = DefaultOptions
- }
- for strings.HasSuffix(path, "/") {
- path = path[:len(path)-1]
- }
- remaining := path
- var variableNames []string
-loop:
- for {
- //remaining = strings.TrimPrefix(remaining, "/")
- if len(remaining) == 0 {
- // This node is the right one
- // Check whether another route already leads to this node
- currentNode.VariableNames = variableNames
- return currentNode, nil
- }
-
- suffix := Suffix{}
- var i int
- if strings.HasPrefix(remaining, "/") {
- remaining = remaining[1:]
- suffix.Kind = SuffixKindConstant
- suffix.Pattern = "/"
- } else {
- i = strings.IndexAny(remaining, "/{")
- if i < 0 {
- i = len(remaining)
- }
- if i > 0 {
- // Constant string pattern
- suffix.Kind = SuffixKindConstant
- suffix.Pattern = remaining[:i]
- remaining = remaining[i:]
- } else if remaining[0] == '{' {
- // This is probably a variable
- suffix.Kind = SuffixKindVariable
-
- // Find variable name
- i := strings.IndexByte(remaining, '}')
- if i < 0 {
- return nil, fmt.Errorf("missing '}' in: %s", path)
- }
- variableName := strings.TrimSpace(remaining[1:i])
- remaining = remaining[i+1:]
-
- if options.SupportRegExp {
- // See if it has regular expression
- i = strings.IndexByte(variableName, '|')
- if i >= 0 {
- suffix.Kind = SuffixKindRegExp
- suffix.Pattern = strings.TrimSpace(variableName[i+1:])
- variableName = strings.TrimSpace(variableName[:i])
- }
- }
- if suffix.Kind == SuffixKindVariable && options.SupportWildcard {
- if strings.HasSuffix(variableName, "*") {
- suffix.Kind = SuffixKindEverything
- }
- }
- variableNames = append(variableNames, variableName)
- }
- }
-
- // Find existing matcher
- for _, existing := range currentNode.Suffixes {
- if EqualSuffix(existing, suffix) {
- currentNode = existing.Node
- continue loop
- }
- }
-
- // Compile regular expression
- if suffix.Kind == SuffixKindRegExp {
- regExp, err := regexp.Compile(suffix.Pattern)
- if err != nil {
- return nil, fmt.Errorf("invalid regular expression in: %s", path)
- }
- suffix.regExp = regExp
- }
-
- // Create new node
- newNode := &Node{}
- suffix.Node = newNode
- currentNode.Suffixes = append(currentNode.Suffixes, suffix)
- sort.Sort(currentNode.Suffixes)
- currentNode = newNode
- continue loop
- }
-}
-
-func (currentNode *Node) Match(path string) (*Node, []string) {
- for strings.HasSuffix(path, "/") {
- path = path[:len(path)-1]
- }
- variableValues := make([]string, 0, 8)
- return currentNode.matchRemaining(path, variableValues)
-}
-
-func (currentNode *Node) matchRemaining(remaining string, paramValues []string) (*Node, []string) {
- // Check if this node matches
- if len(remaining) == 0 && currentNode.Value != nil {
- return currentNode, paramValues
- }
-
- // See if any suffix matches
- for _, suffix := range currentNode.Suffixes {
- var resultNode *Node
- var resultValues []string
- switch suffix.Kind {
- case SuffixKindConstant:
- pattern := suffix.Pattern
- if strings.HasPrefix(remaining, pattern) {
- newRemaining := remaining[len(pattern):]
- resultNode, resultValues = suffix.Node.matchRemaining(newRemaining, paramValues)
- } else if len(remaining) == 0 && pattern == "/" {
- resultNode, resultValues = suffix.Node.matchRemaining(remaining, paramValues)
- }
- case SuffixKindVariable:
- i := strings.IndexByte(remaining, '/')
- if i < 0 {
- i = len(remaining)
- }
- newParamValues := append(paramValues, remaining[:i])
- newRemaining := remaining[i:]
- resultNode, resultValues = suffix.Node.matchRemaining(newRemaining, newParamValues)
- case SuffixKindEverything:
- newParamValues := append(paramValues, remaining)
- resultNode, resultValues = suffix.Node, newParamValues
- case SuffixKindRegExp:
- i := strings.IndexByte(remaining, '/')
- if i < 0 {
- i = len(remaining)
- }
- paramValue := remaining[:i]
- regExp := suffix.regExp
- if regExp.MatchString(paramValue) {
- matches := regExp.FindStringSubmatch(paramValue)
- if len(matches) > 1 {
- paramValue = matches[1]
- }
- newParamValues := append(paramValues, paramValue)
- newRemaining := remaining[i:]
- resultNode, resultValues = suffix.Node.matchRemaining(newRemaining, newParamValues)
- }
- }
- if resultNode != nil && resultNode.Value != nil {
- // This suffix matched
- return resultNode, resultValues
- }
- }
-
- // No suffix matched
- return nil, nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/routers/legacy/router.go b/vendor/github.com/getkin/kin-openapi/routers/legacy/router.go
deleted file mode 100644
index 911422b8..00000000
--- a/vendor/github.com/getkin/kin-openapi/routers/legacy/router.go
+++ /dev/null
@@ -1,167 +0,0 @@
-// Package legacy implements a router.
-//
-// It differs from the gorilla/mux router:
-// * it provides granular errors: "path not found", "method not allowed", "variable missing from path"
-// * it does not handle matching routes with extensions (e.g. /books/{id}.json)
-// * it handles path patterns with a different syntax (e.g. /params/{x}/{y}/{z.*})
-package legacy
-
-import (
- "context"
- "errors"
- "fmt"
- "net/http"
- "strings"
-
- "github.com/getkin/kin-openapi/openapi3"
- "github.com/getkin/kin-openapi/routers"
- "github.com/getkin/kin-openapi/routers/legacy/pathpattern"
-)
-
-// Routers maps a HTTP request to a Router.
-type Routers []*Router
-
-// FindRoute extracts the route and parameters of an http.Request
-func (rs Routers) FindRoute(req *http.Request) (routers.Router, *routers.Route, map[string]string, error) {
- for _, router := range rs {
- // Skip routers that have DO NOT have servers
- if len(router.doc.Servers) == 0 {
- continue
- }
- route, pathParams, err := router.FindRoute(req)
- if err == nil {
- return router, route, pathParams, nil
- }
- }
- for _, router := range rs {
- // Skip routers that DO have servers
- if len(router.doc.Servers) > 0 {
- continue
- }
- route, pathParams, err := router.FindRoute(req)
- if err == nil {
- return router, route, pathParams, nil
- }
- }
- return nil, nil, nil, &routers.RouteError{
- Reason: "none of the routers match",
- }
-}
-
-// Router maps a HTTP request to an OpenAPI operation.
-type Router struct {
- doc *openapi3.T
- pathNode *pathpattern.Node
-}
-
-// NewRouter creates a new router.
-//
-// If the given OpenAPIv3 document has servers, router will use them.
-// All operations of the document will be added to the router.
-func NewRouter(doc *openapi3.T, opts ...openapi3.ValidationOption) (routers.Router, error) {
- if err := doc.Validate(context.Background(), opts...); err != nil {
- return nil, fmt.Errorf("validating OpenAPI failed: %w", err)
- }
- router := &Router{doc: doc}
- root := router.node()
- for path, pathItem := range doc.Paths {
- for method, operation := range pathItem.Operations() {
- method = strings.ToUpper(method)
- if err := root.Add(method+" "+path, &routers.Route{
- Spec: doc,
- Path: path,
- PathItem: pathItem,
- Method: method,
- Operation: operation,
- }, nil); err != nil {
- return nil, err
- }
- }
- }
- return router, nil
-}
-
-// AddRoute adds a route in the router.
-func (router *Router) AddRoute(route *routers.Route) error {
- method := route.Method
- if method == "" {
- return errors.New("route is missing method")
- }
- method = strings.ToUpper(method)
- path := route.Path
- if path == "" {
- return errors.New("route is missing path")
- }
- return router.node().Add(method+" "+path, router, nil)
-}
-
-func (router *Router) node() *pathpattern.Node {
- root := router.pathNode
- if root == nil {
- root = &pathpattern.Node{}
- router.pathNode = root
- }
- return root
-}
-
-// FindRoute extracts the route and parameters of an http.Request
-func (router *Router) FindRoute(req *http.Request) (*routers.Route, map[string]string, error) {
- method, url := req.Method, req.URL
- doc := router.doc
-
- // Get server
- servers := doc.Servers
- var server *openapi3.Server
- var remainingPath string
- var pathParams map[string]string
- if len(servers) == 0 {
- remainingPath = url.Path
- } else {
- var paramValues []string
- server, paramValues, remainingPath = servers.MatchURL(url)
- if server == nil {
- return nil, nil, &routers.RouteError{
- Reason: routers.ErrPathNotFound.Error(),
- }
- }
- pathParams = make(map[string]string)
- paramNames, err := server.ParameterNames()
- if err != nil {
- return nil, nil, err
- }
- for i, value := range paramValues {
- name := paramNames[i]
- pathParams[name] = value
- }
- }
-
- // Get PathItem
- root := router.node()
- var route *routers.Route
- node, paramValues := root.Match(method + " " + remainingPath)
- if node != nil {
- route, _ = node.Value.(*routers.Route)
- }
- if route == nil {
- pathItem := doc.Paths[remainingPath]
- if pathItem == nil {
- return nil, nil, &routers.RouteError{Reason: routers.ErrPathNotFound.Error()}
- }
- if pathItem.GetOperation(method) == nil {
- return nil, nil, &routers.RouteError{Reason: routers.ErrMethodNotAllowed.Error()}
- }
- }
-
- if pathParams == nil {
- pathParams = make(map[string]string, len(paramValues))
- }
- paramKeys := node.VariableNames
- for i, value := range paramValues {
- key := paramKeys[i]
- if strings.HasSuffix(key, "*") {
- key = key[:len(key)-1]
- }
- pathParams[key] = value
- }
- return route, pathParams, nil
-}
diff --git a/vendor/github.com/getkin/kin-openapi/routers/types.go b/vendor/github.com/getkin/kin-openapi/routers/types.go
deleted file mode 100644
index 93746cfe..00000000
--- a/vendor/github.com/getkin/kin-openapi/routers/types.go
+++ /dev/null
@@ -1,42 +0,0 @@
-package routers
-
-import (
- "net/http"
-
- "github.com/getkin/kin-openapi/openapi3"
-)
-
-// Router helps link http.Request.s and an OpenAPIv3 spec
-type Router interface {
- // FindRoute matches an HTTP request with the operation it resolves to.
- // Hosts are matched from the OpenAPIv3 servers key.
- //
- // If you experience ErrPathNotFound and have localhost hosts specified as your servers,
- // turning these server URLs as relative (leaving only the path) should resolve this.
- //
- // See openapi3filter for example uses with request and response validation.
- FindRoute(req *http.Request) (route *Route, pathParams map[string]string, err error)
-}
-
-// Route describes the operation an http.Request can match
-type Route struct {
- Spec *openapi3.T
- Server *openapi3.Server
- Path string
- PathItem *openapi3.PathItem
- Method string
- Operation *openapi3.Operation
-}
-
-// ErrPathNotFound is returned when no route match is found
-var ErrPathNotFound error = &RouteError{"no matching operation was found"}
-
-// ErrMethodNotAllowed is returned when no method of the matched route matches
-var ErrMethodNotAllowed error = &RouteError{"method not allowed"}
-
-// RouteError describes Router errors
-type RouteError struct {
- Reason string
-}
-
-func (e *RouteError) Error() string { return e.Reason }
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/LICENSE b/vendor/github.com/go-echarts/go-echarts/v2/LICENSE
deleted file mode 100644
index 17f9843e..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2019~now chenjiandongx
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/actions/global.go b/vendor/github.com/go-echarts/go-echarts/v2/actions/global.go
deleted file mode 100644
index 5bf5a564..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/actions/global.go
+++ /dev/null
@@ -1,29 +0,0 @@
-package actions
-
-import (
- "math/rand"
- "time"
-)
-
-func init() {
- rand.Seed(time.Now().UnixNano())
-}
-
-// Type kind of dispatch action
-type Type string
-
-// Areas means select-boxes. Multi-boxes can be specified.
-// If Areas is empty, all of the select-boxes will be deleted.
-// The first area.
-type Areas struct {
-
- //BrushType Optional: 'polygon', 'rect', 'lineX', 'lineY'
- BrushType string `json:"brushType,omitempty"`
-
- // CoordRange Only for "coordinate system area", define the area with the
- // coordinates.
- CoordRange []string `json:"coordRange,omitempty"`
-
- // XAxisIndex Assigns which of the xAxisIndex can use Area selecting.
- XAxisIndex interface{} `json:"xAxisIndex,omitempty"`
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/bar.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/bar.go
deleted file mode 100644
index d8e8dbbd..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/bar.go
+++ /dev/null
@@ -1,63 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Bar represents a bar chart.
-type Bar struct {
- RectChart
-
- isXYReversal bool
-}
-
-// Type returns the chart type.
-func (Bar) Type() string { return types.ChartBar }
-
-// NewBar creates a new bar chart instance.
-func NewBar() *Bar {
- c := &Bar{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// EnablePolarType enables the polar bar.
-func (c *Bar) EnablePolarType() *Bar {
- c.hasXYAxis = false
- c.hasPolar = true
- return c
-}
-
-// SetXAxis sets the X axis.
-func (c *Bar) SetXAxis(x interface{}) *Bar {
- c.xAxisData = x
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Bar) AddSeries(name string, data []opts.BarData, options ...SeriesOpts) *Bar {
- series := SingleSeries{Name: name, Type: types.ChartBar, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// XYReversal checks if X axis and Y axis are reversed.
-func (c *Bar) XYReversal() *Bar {
- c.isXYReversal = true
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Bar) Validate() {
- c.XAxisList[0].Data = c.xAxisData
- if c.isXYReversal {
- c.YAxisList[0].Data = c.xAxisData
- c.XAxisList[0].Data = nil
- }
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/bar3d.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/bar3d.go
deleted file mode 100644
index 579b972d..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/bar3d.go
+++ /dev/null
@@ -1,30 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Bar3D represents a 3D bar chart.
-type Bar3D struct {
- Chart3D
-}
-
-// Type returns the chart type.
-func (Bar3D) Type() string { return types.ChartBar3D }
-
-// NewBar3D creates a new 3D bar chart.
-func NewBar3D() *Bar3D {
- c := &Bar3D{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.initChart3D()
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Bar3D) AddSeries(name string, data []opts.Chart3DData, options ...SeriesOpts) *Bar3D {
- c.addSeries(types.ChartBar3D, name, data, options...)
- return c
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/base.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/base.go
deleted file mode 100644
index 30dd7722..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/base.go
+++ /dev/null
@@ -1,399 +0,0 @@
-package charts
-
-import (
- "bytes"
- "encoding/json"
- "html/template"
-
- "github.com/go-echarts/go-echarts/v2/actions"
- "github.com/go-echarts/go-echarts/v2/datasets"
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
-)
-
-// GlobalOpts sets the Global options for charts.
-type GlobalOpts func(bc *BaseConfiguration)
-
-// GlobalActions sets the Global actions for charts
-type GlobalActions func(ba *BaseActions)
-
-// BaseConfiguration represents an option set needed by all chart types.
-type BaseConfiguration struct {
- opts.Legend `json:"legend"`
- opts.Tooltip `json:"tooltip"`
- opts.Toolbox `json:"toolbox"`
- opts.Title `json:"title"`
- opts.Dataset `json:"dataset"`
- opts.Polar `json:"polar"`
- opts.AngleAxis `json:"angleAxis"`
- opts.RadiusAxis `json:"radiusAxis"`
- opts.Brush `json:"brush"`
- *opts.AxisPointer `json:"axisPointer"`
-
- render.Renderer `json:"-"`
- opts.Initialization `json:"-"`
- opts.Assets `json:"-"`
- opts.RadarComponent `json:"-"`
- opts.GeoComponent `json:"-"`
- opts.ParallelComponent `json:"-"`
- opts.JSFunctions `json:"-"`
- opts.SingleAxis `json:"-"`
-
- MultiSeries
- XYAxis
-
- opts.XAxis3D
- opts.YAxis3D
- opts.ZAxis3D
- opts.Grid3D
- opts.Grid
-
- legends []string
- // Colors is the color list of palette.
- // If no color is set in series, the colors would be adopted sequentially and circularly
- // from this list as the colors of series.
- Colors []string
- appendColor []string // append customize color to the Colors(reverse order)
-
- DataZoomList []opts.DataZoom `json:"datazoom,omitempty"`
- VisualMapList []opts.VisualMap `json:"visualmap,omitempty"`
-
- // ParallelAxisList represents the component list which is the coordinate axis for parallel coordinate.
- ParallelAxisList []opts.ParallelAxis
-
- has3DAxis bool
- hasXYAxis bool
- hasGeo bool
- hasRadar bool
- hasParallel bool
- hasSingleAxis bool
- hasPolar bool
- hasBrush bool
-
- GridList []opts.Grid `json:"grid,omitempty"`
-}
-
-// BaseActions represents a dispatchAction set needed by all chart types.
-type BaseActions struct {
- actions.Type `json:"type,omitempty"`
- actions.Areas `json:"areas,omitempty"`
-}
-
-// JSON wraps all the options to a map so that it could be used in the base template
-//
-// Get data in bytes
-// bs, _ : = json.Marshal(bar.JSON())
-func (bc *BaseConfiguration) JSON() map[string]interface{} {
- return bc.json()
-}
-
-// JSONNotEscaped works like method JSON, but it returns a marshaled object whose characters will not be escaped in the template
-func (bc *BaseConfiguration) JSONNotEscaped() template.HTML {
- obj := bc.json()
- buff := bytes.NewBufferString("")
- enc := json.NewEncoder(buff)
- enc.SetEscapeHTML(false)
- enc.Encode(obj)
-
- return template.HTML(buff.String())
-}
-
-// JSONNotEscapedAction works like method JSON, but it returns a marshaled object whose characters will not be escaped in the template
-func (ba *BaseActions) JSONNotEscapedAction() template.HTML {
- obj := ba.json()
- buff := bytes.NewBufferString("")
- enc := json.NewEncoder(buff)
- enc.SetEscapeHTML(false)
- enc.Encode(obj)
-
- return template.HTML(buff.String())
-}
-
-func (bc *BaseConfiguration) json() map[string]interface{} {
- obj := map[string]interface{}{
- "title": bc.Title,
- "legend": bc.Legend,
- "tooltip": bc.Tooltip,
- "series": bc.MultiSeries,
- "dataset": bc.Dataset,
- }
- if bc.AxisPointer != nil {
- obj["axisPointer"] = bc.AxisPointer
- }
- if bc.hasPolar {
- obj["polar"] = bc.Polar
- obj["angleAxis"] = bc.AngleAxis
- obj["radiusAxis"] = bc.RadiusAxis
- }
-
- if bc.hasGeo {
- obj["geo"] = bc.GeoComponent
- }
-
- if bc.hasRadar {
- obj["radar"] = bc.RadarComponent
- }
-
- if bc.hasParallel {
- obj["parallel"] = bc.ParallelComponent
- obj["parallelAxis"] = bc.ParallelAxisList
- }
-
- if bc.hasSingleAxis {
- obj["singleAxis"] = bc.SingleAxis
- }
-
- if bc.Toolbox.Show {
- obj["toolbox"] = bc.Toolbox
- }
-
- if len(bc.DataZoomList) > 0 {
- obj["dataZoom"] = bc.DataZoomList
- }
-
- if len(bc.VisualMapList) > 0 {
- obj["visualMap"] = bc.VisualMapList
- }
-
- if bc.hasXYAxis {
- obj["xAxis"] = bc.XAxisList
- obj["yAxis"] = bc.YAxisList
- }
-
- if bc.has3DAxis {
- obj["xAxis3D"] = bc.XAxis3D
- obj["yAxis3D"] = bc.YAxis3D
- obj["zAxis3D"] = bc.ZAxis3D
- obj["grid3D"] = bc.Grid3D
- }
-
- if bc.Theme == "white" {
- obj["color"] = bc.Colors
- }
-
- if bc.BackgroundColor != "" {
- obj["backgroundColor"] = bc.BackgroundColor
- }
-
- if len(bc.GridList) > 0 {
- obj["grid"] = bc.GridList
- }
-
- if bc.hasBrush {
- obj["brush"] = bc.Brush
- }
-
- return obj
-}
-
-// GetAssets returns the Assets options.
-func (bc *BaseConfiguration) GetAssets() opts.Assets {
- return bc.Assets
-}
-
-func (bc *BaseConfiguration) initBaseConfiguration() {
- bc.initSeriesColors()
- bc.InitAssets()
- bc.initXYAxis()
- bc.Initialization.Validate()
-}
-
-func (bc *BaseConfiguration) initSeriesColors() {
- bc.Colors = []string{
- "#5470c6", "#91cc75", "#fac858", "#ee6666", "#73c0de",
- "#3ba272", "#fc8452", "#9a60b4", "#ea7ccc",
- }
-}
-
-func (bc *BaseConfiguration) insertSeriesColors(colors []string) {
- reversed := reverseSlice(colors)
- for i := 0; i < len(reversed); i++ {
- bc.Colors = append(bc.Colors, "")
- copy(bc.Colors[1:], bc.Colors[0:])
- bc.Colors[0] = reversed[i]
- }
-}
-
-func (bc *BaseConfiguration) setBaseGlobalOptions(opts ...GlobalOpts) {
- for _, opt := range opts {
- opt(bc)
- }
-}
-
-func (bc *BaseActions) setBaseGlobalActions(opts ...GlobalActions) {
- for _, opt := range opts {
- opt(bc)
- }
-}
-
-func (ba *BaseActions) json() map[string]interface{} {
- obj := map[string]interface{}{
- "type": ba.Type,
- "areas": ba.Areas,
- }
- return obj
-}
-
-// WithAreas sets the areas of the action
-func WithAreas(act actions.Areas) GlobalActions {
- return func(ba *BaseActions) {
- ba.Areas = act
- }
-}
-
-// WithType sets the type of the action
-func WithType(act actions.Type) GlobalActions {
- return func(ba *BaseActions) {
- ba.Type = act
- }
-}
-
-// WithAngleAxisOps sets the angle of the axis.
-func WithAngleAxisOps(opt opts.AngleAxis) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.AngleAxis = opt
- }
-}
-
-// WithRadiusAxisOps sets the radius of the axis.
-func WithRadiusAxisOps(opt opts.RadiusAxis) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.RadiusAxis = opt
- }
-}
-
-// WithBrush sets the Brush.
-func WithBrush(opt opts.Brush) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.hasBrush = true
- bc.Brush = opt
- }
-}
-
-// WithPolarOps sets the polar.
-func WithPolarOps(opt opts.Polar) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Polar = opt
- }
-}
-
-// WithTitleOpts sets the title.
-func WithTitleOpts(opt opts.Title) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Title = opt
- }
-}
-
-// WithToolboxOpts sets the toolbox.
-func WithToolboxOpts(opt opts.Toolbox) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Toolbox = opt
- }
-}
-
-// WithSingleAxisOpts sets the single axis.
-func WithSingleAxisOpts(opt opts.SingleAxis) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.SingleAxis = opt
- }
-}
-
-// WithTooltipOpts sets the tooltip.
-func WithTooltipOpts(opt opts.Tooltip) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Tooltip = opt
- }
-}
-
-// WithLegendOpts sets the legend.
-func WithLegendOpts(opt opts.Legend) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Legend = opt
- }
-}
-
-// WithInitializationOpts sets the initialization.
-func WithInitializationOpts(opt opts.Initialization) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Initialization = opt
- if bc.Initialization.Theme != "" &&
- bc.Initialization.Theme != "white" &&
- bc.Initialization.Theme != "dark" {
- bc.JSAssets.Add("themes/" + opt.Theme + ".js")
- }
- bc.Initialization.Validate()
- }
-}
-
-// WithDataZoomOpts sets the list of the zoom data.
-func WithDataZoomOpts(opt ...opts.DataZoom) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.DataZoomList = append(bc.DataZoomList, opt...)
- }
-}
-
-// WithVisualMapOpts sets the List of the visual map.
-func WithVisualMapOpts(opt ...opts.VisualMap) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.VisualMapList = append(bc.VisualMapList, opt...)
- }
-}
-
-// WithRadarComponentOpts sets the component of the radar.
-func WithRadarComponentOpts(opt opts.RadarComponent) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.RadarComponent = opt
- }
-}
-
-// WithGeoComponentOpts sets the geo component.
-func WithGeoComponentOpts(opt opts.GeoComponent) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.GeoComponent = opt
- bc.JSAssets.Add("maps/" + datasets.MapFileNames[opt.Map] + ".js")
- }
-
-}
-
-// WithParallelComponentOpts sets the parallel component.
-func WithParallelComponentOpts(opt opts.ParallelComponent) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.ParallelComponent = opt
- }
-}
-
-// WithParallelAxisList sets the list of the parallel axis.
-func WithParallelAxisList(opt []opts.ParallelAxis) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.ParallelAxisList = opt
- }
-}
-
-// WithColorsOpts sets the color.
-func WithColorsOpts(opt opts.Colors) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.insertSeriesColors(opt)
- }
-}
-
-// reverseSlice reverses the string slice.
-func reverseSlice(s []string) []string {
- for i, j := 0, len(s)-1; i < j; i, j = i+1, j-1 {
- s[i], s[j] = s[j], s[i]
- }
- return s
-}
-
-// WithGridOpts sets the List of the grid.
-func WithGridOpts(opt ...opts.Grid) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.GridList = append(bc.GridList, opt...)
- }
-}
-
-// WithAxisPointerOpts sets the axis pointer.
-func WithAxisPointerOpts(opt *opts.AxisPointer) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.AxisPointer = opt
- }
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/boxplot.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/boxplot.go
deleted file mode 100644
index 1b2b090a..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/boxplot.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// BoxPlot represents a boxplot chart.
-type BoxPlot struct {
- RectChart
-}
-
-// Type returns the chart type.
-func (BoxPlot) Type() string { return types.ChartBoxPlot }
-
-// NewBoxPlot creates a new boxplot chart.
-func NewBoxPlot() *BoxPlot {
- c := &BoxPlot{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// SetXAxis adds the X axis.
-func (c *BoxPlot) SetXAxis(x interface{}) *BoxPlot {
- c.xAxisData = x
- return c
-}
-
-// AddSeries adds the new series.
-func (c *BoxPlot) AddSeries(name string, data []opts.BoxPlotData, options ...SeriesOpts) *BoxPlot {
- series := SingleSeries{Name: name, Type: types.ChartBoxPlot, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *BoxPlot) Validate() {
- c.XAxisList[0].Data = c.xAxisData
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/chart3d.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/chart3d.go
deleted file mode 100644
index abb35852..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/chart3d.go
+++ /dev/null
@@ -1,66 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Chart3D is a chart in 3D coordinates.
-type Chart3D struct {
- BaseConfiguration
-}
-
-// WithXAxis3DOpts sets the X axis of the Chart3D instance.
-func WithXAxis3DOpts(opt opts.XAxis3D) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.XAxis3D = opt
- }
-}
-
-// WithYAxis3DOpts sets the Y axis of the Chart3D instance.
-func WithYAxis3DOpts(opt opts.YAxis3D) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.YAxis3D = opt
- }
-}
-
-// WithZAxis3DOpts sets the Z axis of the Chart3D instance.
-func WithZAxis3DOpts(opt opts.ZAxis3D) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.ZAxis3D = opt
- }
-}
-
-// WithGrid3DOpts sets the grid of the Chart3D instance.
-func WithGrid3DOpts(opt opts.Grid3D) GlobalOpts {
- return func(bc *BaseConfiguration) {
- bc.Grid3D = opt
- }
-}
-
-func (c *Chart3D) initChart3D() {
- c.JSAssets.Add("echarts-gl.min.js")
- c.has3DAxis = true
-}
-
-func (c *Chart3D) addSeries(chartType, name string, data []opts.Chart3DData, options ...SeriesOpts) {
- series := SingleSeries{
- Name: name,
- Type: chartType,
- Data: data,
- CoordSystem: types.ChartCartesian3D,
- }
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
-}
-
-// SetGlobalOptions sets options for the Chart3D instance.
-func (c *Chart3D) SetGlobalOptions(options ...GlobalOpts) *Chart3D {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Chart3D) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/effectscatter.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/effectscatter.go
deleted file mode 100644
index 75419dba..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/effectscatter.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// EffectScatter represents an effect scatter chart.
-type EffectScatter struct {
- RectChart
-}
-
-// Type returns the chart type.
-func (EffectScatter) Type() string { return types.ChartEffectScatter }
-
-// NewEffectScatter creates a new effect scatter chart.
-func NewEffectScatter() *EffectScatter {
- c := &EffectScatter{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// SetXAxis adds the X axis.
-func (c *EffectScatter) SetXAxis(x interface{}) *EffectScatter {
- c.xAxisData = x
- return c
-}
-
-// AddSeries adds the Y axis.
-func (c *EffectScatter) AddSeries(name string, data []opts.EffectScatterData, options ...SeriesOpts) *EffectScatter {
- series := SingleSeries{Name: name, Type: types.ChartEffectScatter, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *EffectScatter) Validate() {
- c.XAxisList[0].Data = c.xAxisData
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/funnel.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/funnel.go
deleted file mode 100644
index a4c22748..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/funnel.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Funnel represents a funnel chart.
-type Funnel struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Funnel) Type() string { return types.ChartFunnel }
-
-// NewFunnel creates a new funnel chart.
-func NewFunnel() *Funnel {
- c := &Funnel{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Funnel) AddSeries(name string, data []opts.FunnelData, options ...SeriesOpts) *Funnel {
- series := SingleSeries{Name: name, Type: types.ChartFunnel, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Funnel instance.
-func (c *Funnel) SetGlobalOptions(options ...GlobalOpts) *Funnel {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Gauge instance.
-func (c *Funnel) SetDispatchActions(actions ...GlobalActions) *Funnel {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Funnel) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/gauge.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/gauge.go
deleted file mode 100644
index 4325fb86..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/gauge.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Gauge represents a gauge chart.
-type Gauge struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Gauge) Type() string { return types.ChartGauge }
-
-// NewGauge creates a new gauge chart.
-func NewGauge() *Gauge {
- c := &Gauge{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Gauge) AddSeries(name string, data []opts.GaugeData, options ...SeriesOpts) *Gauge {
- series := SingleSeries{Name: name, Type: types.ChartGauge, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Gauge instance.
-func (c *Gauge) SetGlobalOptions(options ...GlobalOpts) *Gauge {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Gauge instance.
-func (c *Gauge) SetDispatchActions(actions ...GlobalActions) *Gauge {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Gauge) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/geo.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/geo.go
deleted file mode 100644
index 8bfd768f..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/geo.go
+++ /dev/null
@@ -1,75 +0,0 @@
-package charts
-
-import (
- "log"
-
- "github.com/go-echarts/go-echarts/v2/datasets"
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Geo represents a geo chart.
-type Geo struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Geo) Type() string { return types.ChartGeo }
-
-var geoFormatter = `function (params) {
- return params.name + ' : ' + params.value[2];
-}`
-
-// NewGeo creates a new geo chart.
-func NewGeo() *Geo {
- c := &Geo{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasGeo = true
- return c
-}
-
-// AddSeries adds new data sets.
-// geoType options:
-// * types.ChartScatter
-// * types.ChartEffectScatter
-// * types.ChartHeatMap
-func (c *Geo) AddSeries(name, geoType string, data []opts.GeoData, options ...SeriesOpts) *Geo {
- series := SingleSeries{Name: name, Type: geoType, Data: data, CoordSystem: types.ChartGeo}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-func (c *Geo) extendValue(region string, v float32) []float32 {
- res := make([]float32, 0)
- tv := datasets.Coordinates[region]
- if tv == [2]float32{0, 0} {
- log.Printf("goecharts: No coordinate is specified for %s\n", region)
- } else {
- res = append(tv[:], v)
- }
- return res
-}
-
-// SetGlobalOptions sets options for the Geo instance.
-func (c *Geo) SetGlobalOptions(options ...GlobalOpts) *Geo {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Geo instance.
-func (c *Geo) SetDispatchActions(actions ...GlobalActions) *Geo {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Geo) Validate() {
- if c.Tooltip.Formatter == "" {
- c.Tooltip.Formatter = opts.FuncOpts(geoFormatter)
- }
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/graph.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/graph.go
deleted file mode 100644
index 52bca617..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/graph.go
+++ /dev/null
@@ -1,55 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Graph represents a graph chart.
-type Graph struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Graph) Type() string { return types.ChartGraph }
-
-// NewGraph creates a new graph chart.
-func NewGraph() *Graph {
- chart := new(Graph)
- chart.initBaseConfiguration()
- chart.Renderer = render.NewChartRender(chart, chart.Validate)
- return chart
-}
-
-// AddSeries adds the new series.
-func (c *Graph) AddSeries(name string, nodes []opts.GraphNode, links []opts.GraphLink, options ...SeriesOpts) *Graph {
- series := SingleSeries{Name: name, Type: types.ChartGraph, Links: links, Data: nodes}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Graph instance.
-func (c *Graph) SetGlobalOptions(options ...GlobalOpts) *Graph {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Graph instance.
-func (c *Graph) SetDispatchActions(actions ...GlobalActions) *Graph {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Graph) Validate() {
- // If there is no layout setting, default layout is set to "force".
- for i := 0; i < len(c.MultiSeries); i++ {
- if c.MultiSeries[i].Layout == "" {
- c.MultiSeries[i].Layout = "force"
- }
- }
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/heatmap.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/heatmap.go
deleted file mode 100644
index 25374e1f..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/heatmap.go
+++ /dev/null
@@ -1,43 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// HeatMap represents a heatmap chart.
-type HeatMap struct {
- RectChart
-}
-
-// Type returns the chart type.
-func (HeatMap) Type() string { return types.ChartHeatMap }
-
-// NewHeatMap creates a new heatmap chart.
-func NewHeatMap() *HeatMap {
- c := &HeatMap{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// SetXAxis adds the X axis.
-func (c *HeatMap) SetXAxis(x interface{}) *HeatMap {
- c.xAxisData = x
- return c
-}
-
-// AddSeries adds the new series.
-func (c *HeatMap) AddSeries(name string, data []opts.HeatMapData, options ...SeriesOpts) *HeatMap {
- series := SingleSeries{Name: name, Type: types.ChartHeatMap, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *HeatMap) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/kline.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/kline.go
deleted file mode 100644
index 8f7bf92f..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/kline.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Kline represents a kline chart.
-type Kline struct {
- RectChart
-}
-
-// Type returns the chart type.
-func (Kline) Type() string { return types.ChartKline }
-
-// NewKLine creates a new kline chart.
-func NewKLine() *Kline {
- c := &Kline{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// SetXAxis adds the X axis.
-func (c *Kline) SetXAxis(xAxis interface{}) *Kline {
- c.xAxisData = xAxis
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Kline) AddSeries(name string, data []opts.KlineData, options ...SeriesOpts) *Kline {
- series := SingleSeries{Name: name, Type: types.ChartKline, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Kline) Validate() {
- c.XAxisList[0].Data = c.xAxisData
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/line.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/line.go
deleted file mode 100644
index 2348bb5b..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/line.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Line represents a line chart.
-type Line struct {
- RectChart
-}
-
-// Type returns the chart type.
-func (Line) Type() string { return types.ChartLine }
-
-// NewLine creates a new line chart.
-func NewLine() *Line {
- c := &Line{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// SetXAxis adds the X axis.
-func (c *Line) SetXAxis(x interface{}) *Line {
- c.xAxisData = x
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Line) AddSeries(name string, data []opts.LineData, options ...SeriesOpts) *Line {
- series := SingleSeries{Name: name, Type: types.ChartLine, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Line) Validate() {
- c.XAxisList[0].Data = c.xAxisData
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/line3d.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/line3d.go
deleted file mode 100644
index 17750458..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/line3d.go
+++ /dev/null
@@ -1,30 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Line3D represents a 3D line chart.
-type Line3D struct {
- Chart3D
-}
-
-// Type returns the chart type.
-func (Line3D) Type() string { return types.ChartLine3D }
-
-// NewLine3D creates a new 3D line chart.
-func NewLine3D() *Line3D {
- c := &Line3D{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.initChart3D()
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Line3D) AddSeries(name string, data []opts.Chart3DData, options ...SeriesOpts) *Line3D {
- c.addSeries(types.ChartLine3D, name, data, options...)
- return c
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/liquid.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/liquid.go
deleted file mode 100644
index b39b58da..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/liquid.go
+++ /dev/null
@@ -1,50 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Liquid represents a liquid chart.
-type Liquid struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Liquid) Type() string { return types.ChartLiquid }
-
-// NewLiquid creates a new liquid chart.
-func NewLiquid() *Liquid {
- c := &Liquid{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.JSAssets.Add("echarts-liquidfill.min.js")
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Liquid) AddSeries(name string, data []opts.LiquidData, options ...SeriesOpts) *Liquid {
- series := SingleSeries{Name: name, Type: types.ChartLiquid, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Liquid instance.
-func (c *Liquid) SetGlobalOptions(options ...GlobalOpts) *Liquid {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Liquid instance.
-func (c *Liquid) SetDispatchActions(actions ...GlobalActions) *Liquid {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Liquid) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/map.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/map.go
deleted file mode 100644
index f4a46b8e..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/map.go
+++ /dev/null
@@ -1,58 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/datasets"
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Map represents a map chart.
-type Map struct {
- BaseConfiguration
- BaseActions
-
- mapType string
-}
-
-// Type returns the chart type.
-func (Map) Type() string { return types.ChartMap }
-
-// NewMap creates a new map chart.
-func NewMap() *Map {
- c := &Map{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// RegisterMapType registers the given mapType.
-func (c *Map) RegisterMapType(mapType string) {
- c.mapType = mapType
- c.JSAssets.Add("maps/" + datasets.MapFileNames[mapType] + ".js")
-}
-
-// AddSeries adds new data sets.
-func (c *Map) AddSeries(name string, data []opts.MapData, options ...SeriesOpts) *Map {
- series := SingleSeries{Name: name, Type: types.ChartMap, MapType: c.mapType, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Map instance.
-func (c *Map) SetGlobalOptions(options ...GlobalOpts) *Map {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Radar instance.
-func (c *Map) SetDispatchActions(actions ...GlobalActions) *Map {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Map) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/parallel.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/parallel.go
deleted file mode 100644
index f76112c4..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/parallel.go
+++ /dev/null
@@ -1,50 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Parallel represents a parallel axis.
-type Parallel struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Parallel) Type() string { return types.ChartParallel }
-
-// NewParallel creates a new parallel instance.
-func NewParallel() *Parallel {
- c := &Parallel{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasParallel = true
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Parallel) AddSeries(name string, data []opts.ParallelData, options ...SeriesOpts) *Parallel {
- series := SingleSeries{Name: name, Type: types.ChartParallel, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Parallel instance.
-func (c *Parallel) SetGlobalOptions(options ...GlobalOpts) *Parallel {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Radar instance.
-func (c *Parallel) SetDispatchActions(actions ...GlobalActions) *Parallel {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Parallel) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/pie.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/pie.go
deleted file mode 100644
index 885e3000..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/pie.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Pie represents a pie chart.
-type Pie struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Pie) Type() string { return types.ChartPie }
-
-// NewPie creates a new pie chart.
-func NewPie() *Pie {
- c := &Pie{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Pie) AddSeries(name string, data []opts.PieData, options ...SeriesOpts) *Pie {
- series := SingleSeries{Name: name, Type: types.ChartPie, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Pie instance.
-func (c *Pie) SetGlobalOptions(options ...GlobalOpts) *Pie {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Pie instance.
-func (c *Pie) SetDispatchActions(actions ...GlobalActions) *Pie {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Pie) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/radar.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/radar.go
deleted file mode 100644
index a0335769..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/radar.go
+++ /dev/null
@@ -1,52 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Radar represents a radar chart.
-type Radar struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Radar) Type() string { return types.ChartRadar }
-
-// NewRadar creates a new radar chart.
-func NewRadar() *Radar {
- c := &Radar{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasRadar = true
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Radar) AddSeries(name string, data []opts.RadarData, options ...SeriesOpts) *Radar {
- series := SingleSeries{Name: name, Type: types.ChartRadar, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- c.legends = append(c.legends, name)
- return c
-}
-
-// SetGlobalOptions sets options for the Radar instance.
-func (c *Radar) SetGlobalOptions(options ...GlobalOpts) *Radar {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Radar instance.
-func (c *Radar) SetDispatchActions(actions ...GlobalActions) *Radar {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Radar) Validate() {
- c.Legend.Data = c.legends
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/rectangle.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/rectangle.go
deleted file mode 100644
index 25f90a9f..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/rectangle.go
+++ /dev/null
@@ -1,111 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
-)
-
-type Overlaper interface {
- overlap() MultiSeries
-}
-
-// XYAxis represent the X and Y axis in the rectangular coordinates.
-type XYAxis struct {
- XAxisList []opts.XAxis `json:"xaxis"`
- YAxisList []opts.YAxis `json:"yaxis"`
-}
-
-func (xy *XYAxis) initXYAxis() {
- xy.XAxisList = append(xy.XAxisList, opts.XAxis{})
- xy.YAxisList = append(xy.YAxisList, opts.YAxis{})
-}
-
-// ExtendXAxis adds new X axes.
-func (xy *XYAxis) ExtendXAxis(xAxis ...opts.XAxis) {
- xy.XAxisList = append(xy.XAxisList, xAxis...)
-}
-
-// ExtendYAxis adds new Y axes.
-func (xy *XYAxis) ExtendYAxis(yAxis ...opts.YAxis) {
- xy.YAxisList = append(xy.YAxisList, yAxis...)
-}
-
-// WithXAxisOpts sets the X axis.
-func WithXAxisOpts(opt opts.XAxis, index ...int) GlobalOpts {
- return func(bc *BaseConfiguration) {
- if len(index) == 0 {
- index = []int{0}
- }
- for i := 0; i < len(index); i++ {
- bc.XYAxis.XAxisList[index[i]] = opt
- }
- }
-}
-
-// WithYAxisOpts sets the Y axis.
-func WithYAxisOpts(opt opts.YAxis, index ...int) GlobalOpts {
- return func(bc *BaseConfiguration) {
- if len(index) == 0 {
- index = []int{0}
- }
- for i := 0; i < len(index); i++ {
- bc.XYAxis.YAxisList[index[i]] = opt
- }
- }
-}
-
-// RectConfiguration contains options for the rectangular coordinates.
-type RectConfiguration struct {
- BaseConfiguration
- BaseActions
-}
-
-func (rect *RectConfiguration) setRectGlobalOptions(options ...GlobalOpts) {
- rect.BaseConfiguration.setBaseGlobalOptions(options...)
-}
-
-func (rect *RectConfiguration) setRectGlobalActions(options ...GlobalActions) {
- rect.BaseActions.setBaseGlobalActions(options...)
-}
-
-// RectChart is a chart in RectChart coordinate.
-type RectChart struct {
- RectConfiguration
-
- xAxisData interface{}
-}
-
-func (rc *RectChart) overlap() MultiSeries {
- return rc.MultiSeries
-}
-
-// SetGlobalOptions sets options for the RectChart instance.
-func (rc *RectChart) SetGlobalOptions(options ...GlobalOpts) *RectChart {
- rc.RectConfiguration.setRectGlobalOptions(options...)
- return rc
-}
-
-//SetDispatchActions sets actions for the RectChart instance.
-func (rc *RectChart) SetDispatchActions(options ...GlobalActions) *RectChart {
- rc.RectConfiguration.setRectGlobalActions(options...)
- return rc
-}
-
-// Overlap composes multiple charts into one single canvas.
-// It is only suited for some of the charts which are in rectangular coordinate.
-// Supported charts: Bar/BoxPlot/Line/Scatter/EffectScatter/Kline/HeatMap
-func (rc *RectChart) Overlap(a ...Overlaper) {
- for i := 0; i < len(a); i++ {
- rc.MultiSeries = append(rc.MultiSeries, a[i].overlap()...)
- }
-}
-
-// Validate validates the given configuration.
-func (rc *RectChart) Validate() {
- // Make sure that the data of X axis won't be cleaned for XAxisOpts
- rc.XAxisList[0].Data = rc.xAxisData
- // Make sure that the labels of Y axis show correctly
- for i := 0; i < len(rc.YAxisList); i++ {
- rc.YAxisList[i].AxisLabel.Show = true
- }
- rc.Assets.Validate(rc.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/sankey.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/sankey.go
deleted file mode 100644
index b9da64e4..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/sankey.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Sankey represents a sankey chart.
-type Sankey struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Sankey) Type() string { return types.ChartSankey }
-
-// NewSankey creates a new sankey chart.
-func NewSankey() *Sankey {
- c := &Sankey{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Sankey) AddSeries(name string, nodes []opts.SankeyNode, links []opts.SankeyLink, options ...SeriesOpts) *Sankey {
- series := SingleSeries{Name: name, Type: types.ChartSankey, Data: nodes, Links: links}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Sankey instance.
-func (c *Sankey) SetGlobalOptions(options ...GlobalOpts) *Sankey {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Sankey instance.
-func (c *Sankey) SetDispatchActions(actions ...GlobalActions) *Sankey {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Sankey) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/scatter.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/scatter.go
deleted file mode 100644
index 3ea80327..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/scatter.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Scatter represents a scatter chart.
-type Scatter struct {
- RectChart
-}
-
-// Type returns the chart type.
-func (Scatter) Type() string { return types.ChartScatter }
-
-// NewScatter creates a new scatter chart.
-func NewScatter() *Scatter {
- c := &Scatter{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasXYAxis = true
- return c
-}
-
-// SetXAxis adds the X axis.
-func (c *Scatter) SetXAxis(x interface{}) *Scatter {
- c.xAxisData = x
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Scatter) AddSeries(name string, data []opts.ScatterData, options ...SeriesOpts) *Scatter {
- series := SingleSeries{Name: name, Type: types.ChartScatter, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Scatter) Validate() {
- c.XAxisList[0].Data = c.xAxisData
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/scatter3d.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/scatter3d.go
deleted file mode 100644
index f71e3c11..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/scatter3d.go
+++ /dev/null
@@ -1,30 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Scatter3D represents a 3D scatter chart.
-type Scatter3D struct {
- Chart3D
-}
-
-// Type returns the chart type.
-func (Scatter3D) Type() string { return types.ChartScatter3D }
-
-// NewScatter3D creates a new 3D scatter chart.
-func NewScatter3D() *Scatter3D {
- c := &Scatter3D{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.initChart3D()
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Scatter3D) AddSeries(name string, data []opts.Chart3DData, options ...SeriesOpts) *Scatter3D {
- c.addSeries(types.ChartScatter3D, name, data, options...)
- return c
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/series.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/series.go
deleted file mode 100644
index c9ec534d..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/series.go
+++ /dev/null
@@ -1,496 +0,0 @@
-package charts
-
-import "github.com/go-echarts/go-echarts/v2/opts"
-
-type SingleSeries struct {
- Name string `json:"name,omitempty"`
- Type string `json:"type,omitempty"`
-
- // Rectangular charts
- Stack string `json:"stack,omitempty"`
- XAxisIndex int `json:"xAxisIndex,omitempty"`
- YAxisIndex int `json:"yAxisIndex,omitempty"`
-
- // Bar
- BarGap string `json:"barGap,omitempty"`
- BarCategoryGap string `json:"barCategoryGap,omitempty"`
- ShowBackground bool `json:"showBackground,omitempty"`
- RoundCap bool `json:"roundCap,omitempty"`
-
- // Bar3D
- Shading string `json:"shading,omitempty"`
-
- // Graph
- Links interface{} `json:"links,omitempty"`
- Layout string `json:"layout,omitempty"`
- Force interface{} `json:"force,omitempty"`
- Categories interface{} `json:"categories,omitempty"`
- Roam bool `json:"roam,omitempty"`
- EdgeSymbol interface{} `json:"edgeSymbol,omitempty"`
- EdgeSymbolSize interface{} `json:"edgeSymbolSize,omitempty"`
- EdgeLabel interface{} `json:"edgeLabel,omitempty"`
- Draggable bool `json:"draggable,omitempty"`
- FocusNodeAdjacency bool `json:"focusNodeAdjacency,omitempty"`
-
- // Line
- Step interface{} `json:"step,omitempty"`
- Smooth bool `json:"smooth"`
- ConnectNulls bool `json:"connectNulls"`
- ShowSymbol bool `json:"showSymbol"`
-
- // Liquid
- IsLiquidOutline bool `json:"outline,omitempty"`
- IsWaveAnimation bool `json:"waveAnimation"`
-
- // Map
- MapType string `json:"map,omitempty"`
- CoordSystem string `json:"coordinateSystem,omitempty"`
-
- // Pie
- RoseType interface{} `json:"roseType,omitempty"`
- Center interface{} `json:"center,omitempty"`
- Radius interface{} `json:"radius,omitempty"`
-
- // Scatter
- SymbolSize float32 `json:"symbolSize,omitempty"`
-
- // Tree
- Orient string `json:"orient,omitempty"`
- ExpandAndCollapse bool `json:"expandAndCollapse,omitempty"`
- InitialTreeDepth int `json:"initialTreeDepth,omitempty"`
- Leaves interface{} `json:"leaves,omitempty"`
- Left string `json:"left,omitempty"`
- Right string `json:"right,omitempty"`
- Top string `json:"top,omitempty"`
- Bottom string `json:"bottom,omitempty"`
-
- // TreeMap
- LeafDepth int `json:"leafDepth,omitempty"`
- Levels interface{} `json:"levels,omitempty"`
- UpperLabel interface{} `json:"upperLabel,omitempty"`
-
- // WordCloud
- Shape string `json:"shape,omitempty"`
- SizeRange []float32 `json:"sizeRange,omitempty"`
- RotationRange []float32 `json:"rotationRange,omitempty"`
-
- // Sunburst
- NodeClick string `json:"nodeClick,omitempty"`
- Sort string `json:"sort,omitempty"`
- RenderLabelForZeroData bool `json:"renderLabelForZeroData"`
- SelectedMode bool `json:"selectedMode"`
- Animation bool `json:"animation"`
- AnimationThreshold int `json:"animationThreshold,omitempty"`
- AnimationDuration int `json:"animationDuration,omitempty"`
- AnimationEasing string `json:"animationEasing,omitempty"`
- AnimationDelay int `json:"animationDelay,omitempty"`
- AnimationDurationUpdate int `json:"animationDurationUpdate,omitempty"`
- AnimationEasingUpdate string `json:"animationEasingUpdate,omitempty"`
- AnimationDelayUpdate int `json:"animationDelayUpdate,omitempty"`
-
- // series data
- Data interface{} `json:"data"`
-
- // series options
- *opts.Encode `json:"encode,omitempty"`
- *opts.ItemStyle `json:"itemStyle,omitempty"`
- *opts.Label `json:"label,omitempty"`
- *opts.LabelLine `json:"labelLine,omitempty"`
- *opts.Emphasis `json:"emphasis,omitempty"`
- *opts.MarkLines `json:"markLine,omitempty"`
- *opts.MarkAreas `json:"markArea,omitempty"`
- *opts.MarkPoints `json:"markPoint,omitempty"`
- *opts.RippleEffect `json:"rippleEffect,omitempty"`
- *opts.LineStyle `json:"lineStyle,omitempty"`
- *opts.AreaStyle `json:"areaStyle,omitempty"`
- *opts.TextStyle `json:"textStyle,omitempty"`
- *opts.CircularStyle `json:"circular,omitempty"`
-}
-
-type SeriesOpts func(s *SingleSeries)
-
-// WithLabelOpts sets the label.
-func WithLabelOpts(opt opts.Label) SeriesOpts {
- return func(s *SingleSeries) {
- s.Label = &opt
- }
-}
-
-// WithEmphasisOpts sets the emphasis.
-func WithEmphasisOpts(opt opts.Emphasis) SeriesOpts {
- return func(s *SingleSeries) {
- s.Emphasis = &opt
- }
-}
-
-// WithAreaStyleOpts sets the area style.
-func WithAreaStyleOpts(opt opts.AreaStyle) SeriesOpts {
- return func(s *SingleSeries) {
- s.AreaStyle = &opt
- }
-}
-
-// WithItemStyleOpts sets the item style.
-func WithItemStyleOpts(opt opts.ItemStyle) SeriesOpts {
- return func(s *SingleSeries) {
- s.ItemStyle = &opt
- }
-}
-
-// WithRippleEffectOpts sets the ripple effect.
-func WithRippleEffectOpts(opt opts.RippleEffect) SeriesOpts {
- return func(s *SingleSeries) {
- s.RippleEffect = &opt
- }
-}
-
-// WithLineStyleOpts sets the line style.
-func WithLineStyleOpts(opt opts.LineStyle) SeriesOpts {
- return func(s *SingleSeries) {
- s.LineStyle = &opt
- }
-}
-
-// With CircularStyle Opts
-func WithCircularStyleOpts(opt opts.CircularStyle) SeriesOpts {
- return func(s *SingleSeries) {
- s.CircularStyle = &opt
- }
-}
-
-/* Chart Options */
-
-// WithBarChartOpts sets the BarChart option.
-func WithBarChartOpts(opt opts.BarChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Stack = opt.Stack
- s.BarGap = opt.BarGap
- s.BarCategoryGap = opt.BarCategoryGap
- s.XAxisIndex = opt.XAxisIndex
- s.YAxisIndex = opt.YAxisIndex
- s.ShowBackground = opt.ShowBackground
- s.RoundCap = opt.RoundCap
- s.CoordSystem = opt.CoordSystem
- }
-}
-
-// WithSunburstOpts sets the SunburstChart option.
-func WithSunburstOpts(opt opts.SunburstChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.NodeClick = opt.NodeClick
- s.Sort = opt.Sort
- s.RenderLabelForZeroData = opt.RenderLabelForZeroData
- s.SelectedMode = opt.SelectedMode
- s.Animation = opt.Animation
- s.AnimationThreshold = opt.AnimationThreshold
- s.AnimationDuration = opt.AnimationDuration
- s.AnimationEasing = opt.AnimationEasing
- s.AnimationDelay = opt.AnimationDelay
- s.AnimationDurationUpdate = opt.AnimationDurationUpdate
- s.AnimationEasingUpdate = opt.AnimationEasingUpdate
- s.AnimationDelayUpdate = opt.AnimationDelayUpdate
- }
-}
-
-// WithGraphChartOpts sets the GraphChart option.
-func WithGraphChartOpts(opt opts.GraphChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Layout = opt.Layout
- s.Force = opt.Force
- s.Roam = opt.Roam
- s.EdgeSymbol = opt.EdgeSymbol
- s.EdgeSymbolSize = opt.EdgeSymbolSize
- s.Draggable = opt.Draggable
- s.FocusNodeAdjacency = opt.FocusNodeAdjacency
- s.Categories = opt.Categories
- s.EdgeLabel = opt.EdgeLabel
- }
-}
-
-// WithHeatMapChartOpts sets the HeatMapChart option.
-func WithHeatMapChartOpts(opt opts.HeatMapChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.XAxisIndex = opt.XAxisIndex
- s.YAxisIndex = opt.YAxisIndex
- }
-}
-
-// WithLineChartOpts sets the LineChart option.
-func WithLineChartOpts(opt opts.LineChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.YAxisIndex = opt.YAxisIndex
- s.Stack = opt.Stack
- s.Smooth = opt.Smooth
- s.ShowSymbol = opt.ShowSymbol
- s.Step = opt.Step
- s.XAxisIndex = opt.XAxisIndex
- s.YAxisIndex = opt.YAxisIndex
- s.ConnectNulls = opt.ConnectNulls
- }
-}
-
-// WithPieChartOpts sets the PieChart option.
-func WithPieChartOpts(opt opts.PieChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.RoseType = opt.RoseType
- s.Center = opt.Center
- s.Radius = opt.Radius
- }
-}
-
-// WithScatterChartOpts sets the ScatterChart option.
-func WithScatterChartOpts(opt opts.ScatterChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.XAxisIndex = opt.XAxisIndex
- s.YAxisIndex = opt.YAxisIndex
- }
-}
-
-// WithLiquidChartOpts sets the LiquidChart option.
-func WithLiquidChartOpts(opt opts.LiquidChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Shape = opt.Shape
- s.IsLiquidOutline = opt.IsShowOutline
- s.IsWaveAnimation = opt.IsWaveAnimation
- }
-}
-
-// WithBar3DChartOpts sets the Bar3DChart option.
-func WithBar3DChartOpts(opt opts.Bar3DChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Shading = opt.Shading
- }
-}
-
-// WithTreeOpts sets the TreeChart option.
-func WithTreeOpts(opt opts.TreeChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Layout = opt.Layout
- s.Orient = opt.Orient
- s.ExpandAndCollapse = opt.ExpandAndCollapse
- s.InitialTreeDepth = opt.InitialTreeDepth
- s.Roam = opt.Roam
- s.Label = opt.Label
- s.Leaves = opt.Leaves
- s.Right = opt.Right
- s.Left = opt.Left
- s.Top = opt.Top
- s.Bottom = opt.Bottom
- }
-}
-
-// WithTreeMapOpts sets the TreeMapChart options.
-func WithTreeMapOpts(opt opts.TreeMapChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Animation = opt.Animation
- s.LeafDepth = opt.LeafDepth
- s.Roam = opt.Roam
- s.Levels = opt.Levels
- s.UpperLabel = opt.UpperLabel
- s.Right = opt.Right
- s.Left = opt.Left
- s.Top = opt.Top
- s.Bottom = opt.Bottom
- }
-}
-
-// WithWorldCloudChartOpts sets the WorldCloudChart option.
-func WithWorldCloudChartOpts(opt opts.WordCloudChart) SeriesOpts {
- return func(s *SingleSeries) {
- s.Shape = opt.Shape
- s.SizeRange = opt.SizeRange
- s.RotationRange = opt.RotationRange
- }
-}
-
-// WithMarkLineNameTypeItemOpts sets the type of the MarkLine.
-func WithMarkLineNameTypeItemOpts(opt ...opts.MarkLineNameTypeItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkLines == nil {
- s.MarkLines = &opts.MarkLines{}
- }
- for _, o := range opt {
- s.MarkLines.Data = append(s.MarkLines.Data, o)
- }
- }
-}
-
-// WithMarkLineStyleOpts sets the style of the MarkLine.
-func WithMarkLineStyleOpts(opt opts.MarkLineStyle) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkLines == nil {
- s.MarkLines = &opts.MarkLines{}
- }
-
- s.MarkLines.MarkLineStyle = opt
- }
-}
-
-// WithMarkLineNameCoordItemOpts sets the coordinates of the MarkLine.
-func WithMarkLineNameCoordItemOpts(opt ...opts.MarkLineNameCoordItem) SeriesOpts {
- type MLNameCoord struct {
- Name string `json:"name,omitempty"`
- Coord []interface{} `json:"coord"`
- }
- return func(s *SingleSeries) {
- if s.MarkLines == nil {
- s.MarkLines = &opts.MarkLines{}
- }
- for _, o := range opt {
- s.MarkLines.Data = append(s.MarkLines.Data, []MLNameCoord{{Name: o.Name, Coord: o.Coordinate0}, {Coord: o.Coordinate1}})
- }
- }
-}
-
-// WithMarkLineNameXAxisItemOpts sets the X axis of the MarkLine.
-func WithMarkLineNameXAxisItemOpts(opt ...opts.MarkLineNameXAxisItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkLines == nil {
- s.MarkLines = &opts.MarkLines{}
- }
- for _, o := range opt {
- s.MarkLines.Data = append(s.MarkLines.Data, o)
- }
- }
-}
-
-// WithMarkLineNameYAxisItemOpts sets the Y axis of the MarkLine.
-func WithMarkLineNameYAxisItemOpts(opt ...opts.MarkLineNameYAxisItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkLines == nil {
- s.MarkLines = &opts.MarkLines{}
- }
- for _, o := range opt {
- s.MarkLines.Data = append(s.MarkLines.Data, o)
- }
- }
-}
-
-// WithMarkAreaNameTypeItemOpts sets the type of the MarkArea.
-func WithMarkAreaNameTypeItemOpts(opt ...opts.MarkAreaNameTypeItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkAreas == nil {
- s.MarkAreas = &opts.MarkAreas{}
- }
- for _, o := range opt {
- s.MarkAreas.Data = append(s.MarkAreas.Data, o)
- }
- }
-}
-
-// WithMarkAreaStyleOpts sets the style of the MarkArea.
-func WithMarkAreaStyleOpts(opt opts.MarkAreaStyle) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkAreas == nil {
- s.MarkAreas = &opts.MarkAreas{}
- }
-
- s.MarkAreas.MarkAreaStyle = opt
- }
-}
-
-// WithMarkAreaNameCoordItemOpts sets the coordinates of the MarkLine.
-func WithMarkAreaNameCoordItemOpts(opt ...opts.MarkAreaNameCoordItem) SeriesOpts {
- type MANameCoord struct {
- Name string `json:"name,omitempty"`
- ItemStyle *opts.ItemStyle `json:"itemStyle"`
- Coord []interface{} `json:"coord"`
- }
- return func(s *SingleSeries) {
- if s.MarkAreas == nil {
- s.MarkAreas = &opts.MarkAreas{}
- }
- for _, o := range opt {
- s.MarkAreas.Data = append(s.MarkAreas.Data, []MANameCoord{{Name: o.Name, ItemStyle: o.ItemStyle, Coord: o.Coordinate0}, {Coord: o.Coordinate1}})
- }
- }
-}
-
-// WithMarkAreaNameXAxisItemOpts sets the X axis of the MarkLine.
-func WithMarkAreaNameXAxisItemOpts(opt ...opts.MarkAreaNameXAxisItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkAreas == nil {
- s.MarkAreas = &opts.MarkAreas{}
- }
- for _, o := range opt {
- s.MarkAreas.Data = append(s.MarkAreas.Data, o)
- }
- }
-}
-
-// WithMarkAreaNameYAxisItemOpts sets the Y axis of the MarkLine.
-func WithMarkAreaNameYAxisItemOpts(opt ...opts.MarkAreaNameYAxisItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkAreas == nil {
- s.MarkAreas = &opts.MarkAreas{}
- }
- for _, o := range opt {
- s.MarkAreas.Data = append(s.MarkAreas.Data, o)
- }
- }
-}
-
-// WithMarkPointNameTypeItemOpts sets the type of the MarkPoint.
-func WithMarkPointNameTypeItemOpts(opt ...opts.MarkPointNameTypeItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkPoints == nil {
- s.MarkPoints = &opts.MarkPoints{}
- }
- for _, o := range opt {
- s.MarkPoints.Data = append(s.MarkPoints.Data, o)
- }
- }
-}
-
-// WithMarkPointStyleOpts sets the style of the MarkPoint.
-func WithMarkPointStyleOpts(opt opts.MarkPointStyle) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkPoints == nil {
- s.MarkPoints = &opts.MarkPoints{}
- }
-
- s.MarkPoints.MarkPointStyle = opt
- }
-}
-
-// WithMarkPointNameCoordItemOpts sets the coordinated of the MarkPoint.
-func WithMarkPointNameCoordItemOpts(opt ...opts.MarkPointNameCoordItem) SeriesOpts {
- return func(s *SingleSeries) {
- if s.MarkPoints == nil {
- s.MarkPoints = &opts.MarkPoints{}
- }
- for _, o := range opt {
- s.MarkPoints.Data = append(s.MarkPoints.Data, o)
- }
- }
-}
-
-func (s *SingleSeries) ConfigureSeriesOpts(options ...SeriesOpts) {
- for _, opt := range options {
- opt(s)
- }
-}
-
-// MultiSeries represents multiple series.
-type MultiSeries []SingleSeries
-
-// SetSeriesOptions sets options for all the series.
-// Previous options will be overwrote every time hence setting them on the `AddSeries` if you want
-// to customize each series individually
-//
-// here -> ↓ <-
-//
-// func (c *Bar) AddSeries(name string, data []opts.BarData, options ...SeriesOpts)
-func (ms *MultiSeries) SetSeriesOptions(opts ...SeriesOpts) {
- s := *ms
- for i := 0; i < len(s); i++ {
- s[i].ConfigureSeriesOpts(opts...)
- }
-}
-
-// WithEncodeOpts Set encodes for dataSets
-func WithEncodeOpts(opt opts.Encode) SeriesOpts {
- return func(s *SingleSeries) {
- s.Encode = &opt
- }
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/sunburst.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/sunburst.go
deleted file mode 100644
index 5b5e73fa..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/sunburst.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Sunburst represents a sunburst chart.
-type Sunburst struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Sunburst) Type() string { return types.ChartSunburst }
-
-// NewSunburst creates a new sunburst chart instance.
-func NewSunburst() *Sunburst {
- c := &Sunburst{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Sunburst) AddSeries(name string, data []opts.SunBurstData, options ...SeriesOpts) *Sunburst {
- series := SingleSeries{Name: name, Type: types.ChartSunburst, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Pie instance.
-func (c *Sunburst) SetGlobalOptions(options ...GlobalOpts) *Sunburst {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Sunburst instance.
-func (c *Sunburst) SetDispatchActions(actions ...GlobalActions) *Sunburst {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Sunburst) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/surface3d.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/surface3d.go
deleted file mode 100644
index 972c9ff0..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/surface3d.go
+++ /dev/null
@@ -1,30 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Surface3D represents a 3D surface chart.
-type Surface3D struct {
- Chart3D
-}
-
-// Type returns the chart type.
-func (Surface3D) Type() string { return types.ChartSurface3D }
-
-// NewSurface3D creates a new 3d surface chart.
-func NewSurface3D() *Surface3D {
- c := &Surface3D{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.initChart3D()
- return c
-}
-
-// AddSeries adds the new series.
-func (c *Surface3D) AddSeries(name string, data []opts.Chart3DData, options ...SeriesOpts) *Surface3D {
- c.addSeries(types.ChartScatter3D, name, data, options...)
- return c
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/themeriver.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/themeriver.go
deleted file mode 100644
index a2936f40..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/themeriver.go
+++ /dev/null
@@ -1,54 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// ThemeRiver represents a theme river chart.
-type ThemeRiver struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (ThemeRiver) Type() string { return types.ChartThemeRiver }
-
-// NewThemeRiver creates a new theme river chart.
-func NewThemeRiver() *ThemeRiver {
- c := &ThemeRiver{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.hasSingleAxis = true
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *ThemeRiver) AddSeries(name string, data []opts.ThemeRiverData, options ...SeriesOpts) *ThemeRiver {
- cd := make([][3]interface{}, len(data))
- for i := 0; i < len(data); i++ {
- cd[i] = data[i].ToList()
- }
- series := SingleSeries{Name: name, Type: types.ChartThemeRiver, Data: cd}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the ThemeRiver instance.
-func (c *ThemeRiver) SetGlobalOptions(options ...GlobalOpts) *ThemeRiver {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the ThemeRiver instance.
-func (c *ThemeRiver) SetDispatchActions(actions ...GlobalActions) *ThemeRiver {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *ThemeRiver) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/tree.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/tree.go
deleted file mode 100644
index 5b1d8863..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/tree.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// Tree represents a Tree chart.
-type Tree struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (Tree) Type() string { return types.ChartTree }
-
-// NewTree creates a new Tree chart instance.
-func NewTree() *Tree {
- c := &Tree{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *Tree) AddSeries(name string, data []opts.TreeData, options ...SeriesOpts) *Tree {
- series := SingleSeries{Name: name, Type: types.ChartTree, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the Tree instance.
-func (c *Tree) SetGlobalOptions(options ...GlobalOpts) *Tree {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the Tree instance.
-func (c *Tree) SetDispatchActions(actions ...GlobalActions) *Tree {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *Tree) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/treemap.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/treemap.go
deleted file mode 100644
index 684264b6..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/treemap.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// TreeMap represents a TreeMap chart.
-type TreeMap struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (TreeMap) Type() string { return types.ChartTreeMap }
-
-// NewTreeMap creates a new TreeMap chart instance.
-func NewTreeMap() *TreeMap {
- c := &TreeMap{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *TreeMap) AddSeries(name string, data []opts.TreeMapNode, options ...SeriesOpts) *TreeMap {
- series := SingleSeries{Name: name, Type: types.ChartTreeMap, Data: data}
- series.ConfigureSeriesOpts(options...)
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the TreeMap instance.
-func (c *TreeMap) SetGlobalOptions(options ...GlobalOpts) *TreeMap {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the TreeMap instance.
-func (c *TreeMap) SetDispatchActions(actions ...GlobalActions) *TreeMap {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *TreeMap) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/charts/wordcloud.go b/vendor/github.com/go-echarts/go-echarts/v2/charts/wordcloud.go
deleted file mode 100644
index 51361752..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/charts/wordcloud.go
+++ /dev/null
@@ -1,66 +0,0 @@
-package charts
-
-import (
- "github.com/go-echarts/go-echarts/v2/opts"
- "github.com/go-echarts/go-echarts/v2/render"
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-// WordCloud represents a word cloud chart.
-type WordCloud struct {
- BaseConfiguration
- BaseActions
-}
-
-// Type returns the chart type.
-func (WordCloud) Type() string { return types.ChartWordCloud }
-
-var wcTextColor = `function () {
- return 'rgb(' + [
- Math.round(Math.random() * 160),
- Math.round(Math.random() * 160),
- Math.round(Math.random() * 160)].join(',') + ')';
-}`
-
-// NewWordCloud creates a new word cloud chart.
-func NewWordCloud() *WordCloud {
- c := &WordCloud{}
- c.initBaseConfiguration()
- c.Renderer = render.NewChartRender(c, c.Validate)
- c.JSAssets.Add("echarts-wordcloud.min.js")
- return c
-}
-
-// AddSeries adds new data sets.
-func (c *WordCloud) AddSeries(name string, data []opts.WordCloudData, options ...SeriesOpts) *WordCloud {
- series := SingleSeries{Name: name, Type: types.ChartWordCloud, Data: data}
- series.ConfigureSeriesOpts(options...)
-
- // set default random color for WordCloud chart
- if series.TextStyle == nil {
- series.TextStyle = &opts.TextStyle{Normal: &opts.TextStyle{}}
- }
- if series.TextStyle.Normal.Color == "" {
- series.TextStyle.Normal.Color = opts.FuncOpts(wcTextColor)
- }
-
- c.MultiSeries = append(c.MultiSeries, series)
- return c
-}
-
-// SetGlobalOptions sets options for the WordCloud instance.
-func (c *WordCloud) SetGlobalOptions(options ...GlobalOpts) *WordCloud {
- c.BaseConfiguration.setBaseGlobalOptions(options...)
- return c
-}
-
-// SetDispatchActions sets actions for the WordCloud instance.
-func (c *WordCloud) SetDispatchActions(actions ...GlobalActions) *WordCloud {
- c.BaseActions.setBaseGlobalActions(actions...)
- return c
-}
-
-// Validate validates the given configuration.
-func (c *WordCloud) Validate() {
- c.Assets.Validate(c.AssetsHost)
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/datasets/coordinates.go b/vendor/github.com/go-echarts/go-echarts/v2/datasets/coordinates.go
deleted file mode 100644
index 47f0ff60..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/datasets/coordinates.go
+++ /dev/null
@@ -1,3755 +0,0 @@
-package datasets
-
-var Coordinates = map[string][2]float32{
- "阿城": {126.58, 45.32},
- "阿克è‹": {80.19, 41.09},
- "阿勒泰": {88.12, 47.5},
- "阿图什": {76.08, 39.42},
- "安达": {125.18, 46.24},
- "安国": {115.2, 38.24},
- "安康": {109.01, 32.41},
- "安陆": {113.41, 31.15},
- "安庆": {117.02, 30.31},
- "安丘": {119.12, 36.25},
- "安顺": {105.55, 26.14},
- "安阳": {114.35, 36.1},
- "éžå±±": {122.85, 41.12},
- "å·´ä¸": {106.43, 31.51},
- "霸州": {116.24, 39.06},
- "白城": {122.5, 45.38},
- "白山": {126.26, 41.56},
- "白银": {104.12, 36.33},
- "百色": {106.36, 23.54},
- "èšŒåŸ ": {117.21, 32.56},
- "包头": {110, 40.58},
- "å®é¸¡": {107.15, 34.38},
- "ä¿å®š": {115.48, 38.85},
- "ä¿å±±": {99.1, 25.08},
- "北海": {109.12, 21.49},
- "北æµ": {110.21, 22.42},
- "北票": {120.47, 41.48},
- "本溪": {123.73, 41.3},
- "毕节": {105.18, 27.18},
- "滨州": {118.03, 37.36},
- "亳州": {115.47, 33.52},
- "åšä¹": {82.08, 44.57},
- "æ²§å·ž": {116.83, 38.33},
- "昌å‰": {87.18, 44.02},
- "昌邑": {119.24, 39.52},
- "常德": {111.69, 29.05},
- "常熟": {120.74, 31.64},
- "常州": {119.95, 31.79},
- "巢湖": {117.52, 31.36},
- "æœé˜³": {120.27, 41.34},
- "潮阳": {116.36, 23.16},
- "潮州": {116.63, 23.68},
- "郴州": {113.02, 25.46},
- "æˆéƒ½": {104.06, 30.67},
- "承德": {117.93, 40.97},
- "澄海": {116.46, 23.28},
- "赤峰": {118.87, 42.28},
- "赤水": {105.42, 28.34},
- "崇州": {103.4, 30.39},
- "æ»å·ž": {118.18, 32.18},
- "楚雄": {101.32, 25.01},
- "慈溪": {121.15, 30.11},
- "从化": {113.33, 23.33},
- "è¾¾å·": {107.29, 31.14},
- "大安": {124.18, 45.3},
- "大ç†": {100.13, 25.34},
- "大连": {121.62, 38.92},
- "大庆": {125.03, 46.58},
- "大石桥": {122.31, 40.37},
- "大åŒ": {113.3, 40.12},
- "大冶": {114.58, 30.06},
- "丹东": {124.37, 40.13},
- "丹江å£": {108.3, 32.33},
- "丹阳": {119.32, 32},
- "å„‹å·ž": {109.34, 19.31},
- "当阳": {111.47, 30.5},
- "å¾·æƒ ": {125.42, 44.32},
- "德令哈": {97.23, 37.22},
- "å¾·å…´": {117.35, 28.57},
- "德阳": {104.37, 31.13},
- "å¾·å·ž": {116.29, 37.45},
- "ç™»å°": {113.02, 34.27},
- "é‚“å·ž": {112.05, 32.42},
- "定州": {115, 38.3},
- "东å·": {103.12, 26.06},
- "东港": {124.08, 39.53},
- "东莞": {113.75, 23.04},
- "东胜": {109.59, 39.48},
- "东å°": {120.19, 32.51},
- "东阳": {120.14, 29.16},
- "东è¥": {118.49, 37.46},
- "éƒ½æ±Ÿå °": {103.37, 31.01},
- "都匀": {107.31, 26.15},
- "敦化": {128.13, 43.22},
- "敦煌": {94.41, 40.08},
- "峨眉山": {103.29, 29.36},
- "é¢å°”å¤çº³": {120.11, 50.13},
- "鄂尔多斯": {109.781327, 39.608266},
- "é„‚å·ž": {114.52, 30.23},
- "æ©å¹³": {112.19, 22.12},
- "æ©æ–½": {109.29, 30.16},
- "二连浩特": {111.58, 43.38},
- "番禺": {113.22, 22.57},
- "防城港": {108.2, 21.37},
- "肥城": {116.46, 36.14},
- "丰城": {115.48, 28.12},
- "丰å—": {118.06, 39.34},
- "丰镇": {113.09, 40.27},
- "凤城": {124.02, 40.28},
- "奉化": {121.24, 29.39},
- "佛山": {113.11, 23.05},
- "涪陵": {107.22, 29.42},
- "ç¦å®‰": {119.39, 27.06},
- "ç¦æ¸…": {119.23, 25.42},
- "ç¦å·ž": {119.3, 26.08},
- "抚顺": {123.97, 41.97},
- "阜康": {87.58, 44.09},
- "阜新": {121.39, 42.01},
- "阜阳": {115.48, 32.54},
- "富锦": {132.02, 47.15},
- "富阳": {119.95, 30.07},
- "ç›–å·ž": {122.21, 40.24},
- "赣州": {114.56, 28.52},
- "高安": {115.22, 28.25},
- "高碑店": {115.51, 39.2},
- "高密": {119.44, 36.22},
- "高明": {112.5, 22.53},
- "高平": {112.55, 35.48},
- "高è¦": {112.26, 23.02},
- "高邮": {119.27, 32.47},
- "高州": {110.5, 21.54},
- "æ ¼å°”æœ¨": {94.55, 36.26},
- "个旧": {103.09, 23.21},
- "æ ¹æ²³": {121.29, 50.48},
- "公主å²": {124.49, 43.31},
- "巩义": {112.58, 34.46},
- "å¤äº¤": {112.09, 37.54},
- "广汉": {104.15, 30.58},
- "广水": {113.48, 31.37},
- "广元": {105.51, 32.28},
- "广州": {113.23, 23.16},
- "è´µæ± ": {117.28, 30.39},
- "贵港": {109.36, 23.06},
- "贵阳": {106.71, 26.57},
- "æ¡‚æž—": {110.28, 25.29},
- "æ¡‚å¹³": {110.04, 23.22},
- "哈尔滨": {126.63, 45.75},
- "哈密": {93.28, 42.5},
- "海城": {122.43, 40.51},
- "æµ·å£": {110.35, 20.02},
- "海拉尔": {119.39, 49.12},
- "æµ·æž—": {129.21, 44.35},
- "海伦": {126.57, 47.28},
- "æµ·é—¨": {121.15, 31.89},
- "æµ·å®": {120.42, 30.32},
- "邯郸": {114.47, 36.6},
- "韩城": {110.27, 35.28},
- "汉ä¸": {107.01, 33.04},
- "æå·ž": {120.19, 30.26},
- "蒿城": {114.5, 38.02},
- "åˆå·": {106.15, 30.02},
- "åˆè‚¥": {117.27, 31.86},
- "åˆå±±": {108.52, 23.47},
- "和龙": {129, 42.32},
- "和田": {79.55, 37.09},
- "æ²³æ± ": {108.03, 24.42},
- "河间": {116.05, 38.26},
- "河津": {110.41, 35.35},
- "æ²³æº": {114.68, 23.73},
- "èæ³½": {115.480656, 35.23375},
- "鹤å£": {114.11, 35.54},
- "鹤岗": {130.16, 47.2},
- "鹤山": {112.57, 22.46},
- "黑河": {127.29, 50.14},
- "è¡¡æ°´": {115.72, 37.72},
- "衡阳": {112.37, 26.53},
- "洪湖": {113.27, 29.48},
- "洪江": {109.59, 27.07},
- "侯马": {111.21, 35.37},
- "呼和浩特": {111.65, 40.82},
- "æ¹–å·ž": {120.1, 30.86},
- "葫芦岛": {120.836932, 40.711052},
- "花都": {113.12, 23.23},
- "åŽé˜´": {110.05, 34.34},
- "åŽè“¥": {106.44, 30.26},
- "化州": {110.37, 21.39},
- "桦甸": {126.44, 42.58},
- "怀化": {109.58, 27.33},
- "淮安": {119.15, 33.5},
- "淮北": {116.47, 33.57},
- "æ·®å—": {116.58, 32.37},
- "淮阴": {119.02, 33.36},
- "黄骅": {117.21, 38.21},
- "黄山": {118.18, 29.43},
- "黄石": {115.06, 30.12},
- "黄州": {114.52, 30.27},
- "ç²æ˜¥": {130.22, 42.52},
- "辉县": {113.47, 35.27},
- "æƒ é˜³": {114.28, 22.48},
- "æƒ å·ž": {114.4, 23.09},
- "éœæž—éƒå‹’": {119.38, 45.32},
- "éœå·ž": {111.42, 36.34},
- "鸡西": {130.57, 45.17},
- "å‰å®‰": {114.58, 27.07},
- "å‰é¦–": {109.43, 28.18},
- "å³å¢¨": {120.45, 36.38},
- "集安": {126.11, 41.08},
- "集å®": {113.06, 41.02},
- "济å—": {117, 36.65},
- "济å®": {116.59, 35.38},
- "济æº": {112.35, 35.04},
- "冀州": {115.33, 37.34},
- "佳木斯": {130.22, 46.47},
- "嘉兴": {120.76, 30.77},
- "嘉峪关": {98.289152, 39.77313},
- "简阳": {104.32, 30.24},
- "建德": {119.16, 29.29},
- "建瓯": {118.2, 27.03},
- "建阳": {118.07, 27.21},
- "江都": {119.32, 32.26},
- "江津": {106.16, 29.18},
- "江门": {113.06, 22.61},
- "江山": {118.37, 28.45},
- "江阴": {120.26, 31.91},
- "江油": {104.42, 31.48},
- "å§œå °": {120.08, 32.34},
- "胶å—": {119.97, 35.88},
- "胶州": {120.03336, 36.264622},
- "焦作": {113.21, 35.24},
- "蛟河": {127.21, 43.42},
- "æé˜³": {116.35, 23.55},
- "介休": {111.55, 37.02},
- "界首": {115.21, 33.15},
- "金昌": {102.188043, 38.520089},
- "金åŽ": {119.64, 29.12},
- "金å›": {119.56, 31.74},
- "津市": {111.52, 29.38},
- "锦州": {121.15, 41.13},
- "晋城": {112.51, 35.3},
- "晋江": {118.35, 24.49},
- "晋州": {115.02, 38.02},
- "è†é—¨": {112.12, 31.02},
- "è†æ²™": {112.16, 30.18},
- "è†å·ž": {112.239741, 30.335165},
- "井冈山": {114.1, 26.34},
- "景德镇": {117.13, 29.17},
- "景洪": {100.48, 22.01},
- "é–æ±Ÿ": {120.17, 32.02},
- "乿±Ÿ": {115.97, 29.71},
- "ä¹å°": {125.51, 44.09},
- "酒泉": {98.31, 39.44},
- "å¥å®¹": {119.16, 31.95},
- "喀什": {75.59, 39.3},
- "å¼€å°": {114.35, 34.79},
- "开平": {112.4, 22.22},
- "开原": {124.02, 42.32},
- "开远": {103.13, 23.43},
- "凯里": {107.58, 26.35},
- "克拉玛ä¾": {84.77, 45.59},
- "库尔勒": {86.06, 41.68},
- "奎屯": {84.56, 44.27},
- "昆明": {102.73, 25.04},
- "昆山": {120.95, 31.39},
- "廓åŠ": {116.42, 39.31},
- "拉è¨": {91.11, 29.97},
- "莱芜": {117.67, 36.19},
- "莱西": {120.53, 36.86},
- "莱阳": {120.42, 36.58},
- "莱州": {119.942327, 37.177017},
- "兰溪": {119.28, 29.12},
- "å…°å·ž": {103.73, 36.03},
- "阆ä¸": {105.58, 31.36},
- "廊åŠ": {116.7, 39.53},
- "è€æ²³å£": {111.4, 32.23},
- "乿˜Œ": {113.21, 25.09},
- "ä¹é™µ": {117.12, 37.44},
- "ä¹å¹³": {117.08, 28.58},
- "乿¸…": {120.58, 28.08},
- "ä¹å±±": {103.44, 29.36},
- "é›·å·ž": {110.04, 20.54},
- "耒阳": {112.51, 26.24},
- "冷水江": {111.26, 27.42},
- "冷水滩": {111.35, 26.26},
- "醴陵": {113.3, 27.4},
- "丽水": {119.92, 28.45},
- "利å·": {108.56, 30.18},
- "溧阳": {119.48, 31.43},
- "连云港": {119.16, 34.59},
- "连州": {112.23, 24.48},
- "æ¶Ÿæº": {111.41, 27.41},
- "廉江": {110.17, 21.37},
- "辽阳": {123.12, 41.16},
- "è¾½æº": {125.09, 42.54},
- "èŠåŸŽ": {115.97, 36.45},
- "æž—å·ž": {113.49, 36.03},
- "临安": {119.72, 30.23},
- "临å·": {116.21, 27.59},
- "临汾": {111.5, 36.08},
- "临海": {121.08, 28.51},
- "临河": {107.22, 40.46},
- "临江": {126.53, 41.49},
- "临清": {115.42, 36.51},
- "临å¤": {103.12, 35.37},
- "临湘": {113.27, 29.29},
- "临沂": {118.35, 35.05},
- "èµç¥¥": {106.44, 22.07},
- "çµå®": {110.52, 34.31},
- "凌海": {121.21, 41.1},
- "凌æº": {119.22, 41.14},
- "æµé˜³": {113.37, 28.09},
- "柳州": {109.4, 24.33},
- "å…安": {116.28, 31.44},
- "å…盘水": {104.5, 26.35},
- "龙海": {117.48, 24.26},
- "龙井": {129.26, 42.46},
- "é¾™å£": {120.21, 37.39},
- "龙泉": {119.08, 28.04},
- "龙岩": {117.01, 25.06},
- "娄底": {111.59, 27.44},
- "泸州": {105.39, 28.91},
- "鹿泉": {114.19, 38.04},
- "潞城": {113.14, 36.21},
- "罗定": {111.33, 22.46},
- "洛阳": {112.44, 34.7},
- "漯河": {114.02, 33.33},
- "麻城": {115.01, 31.1},
- "马éžå±±": {118.48, 31.56},
- "满洲里": {117.23, 49.35},
- "茂å": {110.88, 21.68},
- "梅河å£": {125.4, 42.32},
- "梅州": {116.1, 24.55},
- "汨罗": {113.03, 28.49},
- "密山": {131.5, 45.32},
- "绵阳": {104.73, 31.48},
- "明光": {117.58, 32.47},
- "牡丹江": {129.58, 44.6},
- "å—安": {118.23, 24.57},
- "å—æ˜Œ": {115.89, 28.68},
- "å—å……": {106.110698, 30.837793},
- "å—å·": {107.05, 29.1},
- "å—宫": {115.23, 37.22},
- "å—æµ·": {113.09, 23.01},
- "å—京": {118.78, 32.04},
- "å—å®": {108.33, 22.84},
- "å—å¹³": {118.1, 26.38},
- "å—通": {121.05, 32.08},
- "å—阳": {112.32, 33},
- "è®·æ²³": {124.51, 48.29},
- "内江": {105.02, 29.36},
- "å®å®‰": {129.28, 44.21},
- "宿³¢": {121.56, 29.86},
- "å®å¾·": {119.31, 26.39},
- "攀æžèб": {101.718637, 26.582347},
- "盘锦": {122.070714, 41.119997},
- "å½å·ž": {103.57, 30.59},
- "蓬莱": {120.75, 37.8},
- "邳州": {117.59, 34.19},
- "平顶山": {113.29, 33.75},
- "平度": {119.97, 36.77},
- "平湖": {121.01, 30.42},
- "平凉": {106.4, 35.32},
- "è乡": {113.5, 27.37},
- "泊头": {116.34, 38.04},
- "莆田": {119.01, 24.26},
- "濮阳": {115.01, 35.44},
- "浦圻": {113.51, 29.42},
- "普兰店": {121.58, 39.23},
- "æ™®å®": {116.1, 23.18},
- "ä¸ƒå°æ²³": {130.49, 45.48},
- "é½é½å“ˆå°”": {123.97, 47.33},
- "å¯ä¹": {121.39, 31.48},
- "潜江": {112.53, 30.26},
- "钦州": {108.37, 21.57},
- "秦皇岛": {119.57, 39.95},
- "æ²é˜³": {112.57, 35.05},
- "é’å²›": {120.33, 36.07},
- "é’铜峡": {105.59, 37.56},
- "é’å·ž": {118.28, 36.42},
- "清远": {113.01, 23.7},
- "清镇": {106.27, 26.33},
- "邛崃": {103.28, 30.26},
- "ç¼æµ·": {110.28, 19.14},
- "ç¼å±±": {110.21, 19.59},
- "曲阜": {116.58, 35.36},
- "曲é–": {103.79, 25.51},
- "衢州": {118.88, 28.97},
- "泉州": {118.58, 24.93},
- "任丘": {116.07, 38.42},
- "日喀则": {88.51, 29.16},
- "日照": {119.46, 35.42},
- "è£æˆ": {122.41, 37.16},
- "如皋": {120.33, 32.23},
- "æ±å·ž": {112.5, 34.09},
- "乳山": {121.52, 36.89},
- "瑞安": {120.38, 27.48},
- "瑞昌": {115.38, 29.4},
- "瑞金": {116.01, 25.53},
- "瑞丽": {97.5, 24},
- "三河": {117.04, 39.58},
- "三门峡": {111.19, 34.76},
- "三明": {117.36, 26.13},
- "三水": {112.52, 23.1},
- "三亚": {109.511909, 18.252847},
- "沙河": {114.3, 36.51},
- "厦门": {118.1, 24.46},
- "汕头": {116.69, 23.39},
- "汕尾": {115.375279, 22.786211},
- "商丘": {115.38, 34.26},
- "商州": {109.57, 33.52},
- "上饶": {117.58, 25.27},
- "上虞": {120.52, 30.01},
- "å°šå¿—": {127.55, 45.14},
- "韶关": {113.62, 24.84},
- "韶山": {112.29, 27.54},
- "邵æ¦": {117.29, 27.2},
- "邵阳": {111.28, 27.14},
- "ç»å…´": {120.58, 30.01},
- "深圳": {114.07, 22.62},
- "深州": {115.32, 38.01},
- "沈阳": {123.38, 41.8},
- "åå °": {110.47, 32.4},
- "石河å": {86, 44.18},
- "石家庄": {114.48, 38.03},
- "石狮": {118.38, 24.44},
- "石首": {112.24, 29.43},
- "石嘴山": {106.39, 39.04},
- "寿光": {118.73, 36.86},
- "舒兰": {126.57, 44.24},
- "åŒåŸŽ": {126.15, 45.22},
- "åŒé¸å±±": {131.11, 46.38},
- "顺德": {113.15, 22.5},
- "朔州": {112.26, 39.19},
- "æ€èŒ…": {100.58, 22.48},
- "四会": {112.41, 23.21},
- "四平": {124.22, 43.1},
- "æ¾åŽŸ": {124.49, 45.11},
- "è‹å·ž": {120.62, 31.32},
- "宿è¿": {118.3, 33.96},
- "宿州": {116.58, 33.38},
- "绥芬河": {131.11, 44.25},
- "绥化": {126.59, 46.38},
- "éšå·ž": {113.22, 31.42},
- "é‚å®": {105.33, 30.31},
- "塔城": {82.59, 46.46},
- "å°åŒ—": {121.3, 25.03},
- "å°å±±": {112.48, 22.15},
- "å°å·ž": {121.420757, 28.656386},
- "太仓": {121.1, 31.45},
- "太原": {112.53, 37.87},
- "泰安": {117.13, 36.18},
- "æ³°å…´": {120.01, 32.1},
- "æ³°å·ž": {119.9, 32.49},
- "å”å±±": {118.02, 39.63},
- "æ´®å—": {122.47, 45.2},
- "滕州": {117.09, 35.06},
- "天门": {113.1, 30.39},
- "天水": {105.42, 34.37},
- "天长": {118.59, 32.41},
- "铿³•": {123.32, 42.28},
- "é“力": {128.01, 46.59},
- "é“å²": {123.51, 42.18},
- "通化": {125.56, 41.43},
- "通辽": {122.16, 43.37},
- "通什": {109.31, 18.46},
- "通州": {121.03, 32.05},
- "åŒæ±Ÿ": {132.3, 47.39},
- "æ¡ä¹¡": {120.32, 30.38},
- "铜å·": {109.11, 35.09},
- "铜陵": {117.48, 30.56},
- "铜ä»": {109.12, 27.43},
- "图们": {129.51, 42.57},
- "åé²ç•ª": {89.11, 42.54},
- "瓦房店": {121.979603, 39.627114},
- "畹町": {98.04, 24.06},
- "万县": {108.21, 30.5},
- "万æº": {108.03, 32.03},
- "卿µ·": {122.1, 37.5},
- "æ½åŠ": {119.1, 36.62},
- "å«è¾‰": {114.03, 35.24},
- "æ¸å—": {109.5, 34.52},
- "温å²": {121.21, 28.22},
- "温州": {120.65, 28.01},
- "文登": {122.05, 37.2},
- "乌海": {106.48, 39.4},
- "乌兰浩特": {122.03, 46.03},
- "ä¹Œé²æœ¨é½": {87.68, 43.77},
- "æ— é”¡": {120.29, 31.59},
- "å´å·": {110.47, 21.26},
- "å´æ±Ÿ": {120.63, 31.16},
- "å´å¿ ": {106.11, 37.59},
- "芜湖": {118.38, 31.33},
- "梧州": {111.2, 23.29},
- "五常": {127.11, 44.55},
- "äº”å¤§è¿žæ± ": {126.07, 48.38},
- "æ¦å®‰": {114.11, 36.42},
- "æ¦å†ˆ": {110.37, 26.43},
- "æ¦æ±‰": {114.31, 30.52},
- "æ¦å¨": {102.39, 37.56},
- "æ¦ç©´": {115.33, 29.51},
- "æ¦å¤·å±±": {118.02, 27.46},
- "舞钢": {113.3, 33.17},
- "西安": {108.95, 34.27},
- "西昌": {102.16, 27.54},
- "西峰": {107.4, 35.45},
- "西å®": {101.74, 36.56},
- "锡林浩特": {116.03, 43.57},
- "仙桃": {113.27, 30.22},
- "å’¸å®": {114.17, 29.53},
- "咸阳": {108.72, 34.36},
- "湘æ½": {112.91, 27.87},
- "湘乡": {112.31, 27.44},
- "襄樊": {112.08, 32.02},
- "项城": {114.54, 33.26},
- "è§å±±": {120.16, 30.09},
- "åæ„Ÿ": {113.54, 30.56},
- "å义": {111.48, 37.08},
- "忻州": {112.43, 38.24},
- "辛集": {115.12, 37.54},
- "新会": {113.01, 22.32},
- "æ–°ä¹": {114.41, 38.2},
- "新密": {113.22, 34.31},
- "æ–°æ°‘": {122.49, 41.59},
- "æ–°æ³°": {117.45, 35.54},
- "新乡": {113.52, 35.18},
- "新沂": {118.2, 34.22},
- "æ–°ä½™": {114.56, 27.48},
- "新郑": {113.43, 34.24},
- "信阳": {114.04, 32.07},
- "é‚¢å°": {114.48, 37.05},
- "è¥é˜³": {113.21, 34.46},
- "兴城": {120.41, 40.37},
- "兴化": {119.5, 32.56},
- "å…´å®": {115.43, 24.09},
- "å…´å¹³": {108.29, 34.18},
- "兴义": {104.53, 25.05},
- "å¾å·ž": {117.2, 34.26},
- "许昌": {113.49, 34.01},
- "宣å¨": {104.06, 26.13},
- "宣州": {118.44, 30.57},
- "牙克石": {120.4, 49.17},
- "雅安": {102.59, 29.59},
- "烟å°": {121.39, 37.52},
- "延安": {109.47, 36.6},
- "å»¶å‰": {129.3, 42.54},
- "ç›åŸŽ": {120.13, 33.38},
- "ç›åœ¨": {120.08, 33.22},
- "å…–å·ž": {116.49, 35.32},
- "åƒå¸ˆ": {112.47, 34.43},
- "扬ä¸": {119.49, 32.14},
- "扬州": {119.42, 32.39},
- "阳春": {111.48, 22.1},
- "阳江": {111.95, 21.85},
- "阳泉": {113.57, 37.85},
- "伊春": {128.56, 47.42},
- "伊å®": {81.2, 43.55},
- "仪å¾": {119.1, 32.16},
- "宜宾": {104.56, 29.77},
- "宜昌": {111.3, 30.7},
- "宜城": {112.15, 31.42},
- "宜春": {114.23, 27.47},
- "宜兴": {119.82, 31.36},
- "宜州": {108.4, 24.28},
- "义马": {111.55, 34.43},
- "义乌": {120.06, 29.32},
- "益阳": {112.2, 28.36},
- "é“¶å·": {106.27, 38.47},
- "应城": {113.33, 30.57},
- "英德": {113.22, 24.1},
- "é¹°æ½": {117.03, 28.14},
- "è¥å£": {122.18, 40.65},
- "永安": {117.23, 25.58},
- "æ°¸å·": {105.53, 29.23},
- "永济": {110.27, 34.52},
- "永康": {120.01, 29.54},
- "永州": {111.37, 26.13},
- "ä½™æ": {120.18, 30.26},
- "余姚": {121.1, 30.02},
- "æ„‰æ ‘": {126.32, 44.49},
- "榆次": {112.43, 37.41},
- "榆林": {109.47, 38.18},
- "禹城": {116.39, 36.56},
- "禹州": {113.28, 34.09},
- "玉林": {110.09, 22.38},
- "玉门": {97.35, 39.49},
- "玉溪": {102.52, 24.35},
- "沅江": {112.22, 28.5},
- "原平": {112.42, 38.43},
- "岳阳": {113.09, 29.37},
- "云浮": {112.02, 22.93},
- "è¿åŸŽ": {110.59, 35.02},
- "枣阳": {112.44, 32.07},
- "枣庄": {117.57, 34.86},
- "增城": {113.49, 23.18},
- "扎兰屯": {122.47, 48},
- "湛江": {110.359377, 21.270708},
- "å¼ å®¶æ¸¯": {120.555821, 31.875428},
- "å¼ å®¶ç•Œ": {110.479191, 29.117096},
- "å¼ å®¶å£": {114.87, 40.82},
- "å¼ æŽ–": {100.26, 38.56},
- "ç« ä¸˜": {117.53, 36.72},
- "漳平": {117.24, 25.17},
- "漳州": {117.39, 24.31},
- "æ¨Ÿæ ‘": {115.32, 28.03},
- "长春": {125.35, 43.88},
- "é•¿è‘›": {113.47, 34.12},
- "é•¿ä¹": {119.31, 25.58},
- "é•¿æ²™": {113, 28.21},
- "é•¿æ²»": {113.08, 36.18},
- "招远": {120.38, 37.35},
- "æ˜é€š": {103.42, 27.2},
- "肇东": {125.58, 46.04},
- "肇庆": {112.44, 23.05},
- "镇江": {119.44, 32.2},
- "郑州": {113.65, 34.76},
- "æžåŸŽ": {111.27, 30.23},
- "ä¸å±±": {113.38, 22.52},
- "钟祥": {112.34, 31.1},
- "舟山": {122.207216, 29.985295},
- "周å£": {114.38, 33.37},
- "æ ªæ´²": {113.16, 27.83},
- "ç æµ·": {113.52, 22.3},
- "诸城": {119.24, 35.59},
- "诸暨": {120.23, 29.71},
- "驻马店": {114.01, 32.58},
- "庄河": {122.58, 39.41},
- "æ¶¿å·ž": {115.59, 39.29},
- "资兴": {113.13, 25.58},
- "资阳": {104.38, 30.09},
- "æ·„åš": {118.05, 36.78},
- "自贡": {104.778442, 29.33903},
- "邹城": {116.58, 35.24},
- "éµåŒ–": {117.58, 40.11},
- "éµä¹‰": {106.9, 27.7},
- "晋ä¸": {112.75, 37.68},
- "啿¢": {111.13, 37.52},
- "呼伦è´å°”": {119.77, 49.22},
- "巴彦淖尔": {107.42, 40.75},
- "抚州": {116.35, 28},
- "襄阳": {112.2, 32.08},
- "黄冈": {114.87, 30.45},
- "红河": {103.4, 23.37},
- "文山": {104.25, 23.37},
- "商洛": {109.93, 33.87},
- "定西": {104.62, 35.58},
- "陇å—": {104.92, 33.4},
- "北京市": {116.4, 39.9},
- "天安门": {116.38, 39.9},
- "东城区": {116.42, 39.93},
- "西城区": {116.37, 39.92},
- "崇文区": {116.43, 39.88},
- "宣æ¦åŒº": {116.35, 39.87},
- "丰å°åŒº": {116.28, 39.85},
- "石景山区": {116.22, 39.9},
- "海淀区": {116.3, 39.95},
- "门头沟区": {116.1, 39.93},
- "房山区": {116.13, 39.75},
- "通州区": {116.65, 39.92},
- "顺义区": {116.65, 40.13},
- "昌平区": {116.23, 40.22},
- "大兴区": {116.33, 39.73},
- "怀柔区": {116.63, 40.32},
- "平谷区": {117.12, 40.13},
- "密云县": {116.83, 40.37},
- "延庆县": {115.97, 40.45},
- "天津市": {117.2, 39.12},
- "河西区": {117.22, 39.12},
- "å—开区": {117.15, 39.13},
- "河北区": {117.18, 39.15},
- "红桥区": {117.15, 39.17},
- "塘沽区": {117.65, 39.02},
- "汉沽区": {117.8, 39.25},
- "大港区": {117.45, 38.83},
- "东丽区": {117.3, 39.08},
- "西é’区": {117, 39.13},
- "æ´¥å—区": {117.38, 38.98},
- "北辰区": {117.13, 39.22},
- "æ¦æ¸…区": {117.03, 39.38},
- "å®å»åŒº": {117.3, 39.72},
- "滨海新区": {117.68, 39.03},
- "宿²³åŽ¿": {117.82, 39.33},
- "陿µ·åŽ¿": {116.92, 38.93},
- "蓟县": {117.4, 40.05},
- "石家庄市": {114.52, 38.05},
- "井陉矿区": {114.05, 38.08},
- "裕åŽåŒº": {114.52, 38.02},
- "井陉县": {114.13, 38.03},
- "æ£å®šåŽ¿": {114.57, 38.15},
- "æ ¾åŸŽåŽ¿": {114.65, 37.88},
- "行å”县": {114.55, 38.43},
- "çµå¯¿åŽ¿": {114.37, 38.3},
- "高邑县": {114.6, 37.6},
- "深泽县": {115.2, 38.18},
- "赞皇县": {114.38, 37.67},
- "æ— æžåŽ¿": {114.97, 38.18},
- "平山县": {114.2, 38.25},
- "å…ƒæ°åŽ¿": {114.52, 37.75},
- "赵县": {114.77, 37.75},
- "辛集市": {115.22, 37.92},
- "è—城市": {114.83, 38.03},
- "晋州市": {115.03, 38.03},
- "æ–°ä¹å¸‚": {114.68, 38.35},
- "鹿泉市": {114.3, 38.08},
- "å”山市": {118.2, 39.63},
- "è·¯å—区": {118.17, 39.63},
- "路北区": {118.22, 39.63},
- "å¤å†¶åŒº": {118.42, 39.73},
- "开平区": {118.27, 39.68},
- "丰å—区": {118.1, 39.57},
- "丰润区": {118.17, 39.83},
- "滦县": {118.7, 39.75},
- "滦å—县": {118.68, 39.5},
- "ä¹äºåŽ¿": {118.9, 39.42},
- "è¿è¥¿åŽ¿": {118.32, 40.15},
- "玉田县": {117.73, 39.88},
- "唿µ·åŽ¿": {118.45, 39.27},
- "éµåŒ–市": {117.95, 40.18},
- "è¿å®‰å¸‚": {118.7, 40.02},
- "秦皇岛市": {119.6, 39.93},
- "海港区": {119.6, 39.93},
- "山海关区": {119.77, 40},
- "北戴河区": {119.48, 39.83},
- "é’龙满æ—自治县": {118.95, 40.4},
- "昌黎县": {119.17, 39.7},
- "抚å®åŽ¿": {119.23, 39.88},
- "å¢é¾™åŽ¿": {118.87, 39.88},
- "邯郸市": {114.48, 36.62},
- "邯山区": {114.48, 36.6},
- "丛å°åŒº": {114.48, 36.63},
- "å¤å…´åŒº": {114.45, 36.63},
- "峰峰矿区": {114.2, 36.42},
- "邯郸县": {114.53, 36.6},
- "临漳县": {114.62, 36.35},
- "æˆå®‰åŽ¿": {114.68, 36.43},
- "大å县": {115.15, 36.28},
- "涉县": {113.67, 36.57},
- "ç£åŽ¿": {114.37, 36.35},
- "肥乡县": {114.8, 36.55},
- "永年县": {114.48, 36.78},
- "邱县": {115.17, 36.82},
- "鸡泽县": {114.87, 36.92},
- "广平县": {114.93, 36.48},
- "馆陶县": {115.3, 36.53},
- "é县": {114.93, 36.37},
- "曲周县": {114.95, 36.78},
- "æ¦å®‰å¸‚": {114.2, 36.7},
- "é‚¢å°å¸‚": {114.48, 37.07},
- "é‚¢å°åŽ¿": {114.5, 37.08},
- "临城县": {114.5, 37.43},
- "内丘县": {114.52, 37.3},
- "æŸä¹¡åŽ¿": {114.68, 37.5},
- "隆尧县": {114.77, 37.35},
- "任县": {114.68, 37.13},
- "å—和县": {114.68, 37},
- "宿™‹åŽ¿": {114.92, 37.62},
- "巨鹿县": {115.03, 37.22},
- "新河县": {115.25, 37.53},
- "广宗县": {115.15, 37.07},
- "平乡县": {115.03, 37.07},
- "å¨åŽ¿": {115.25, 36.98},
- "清河县": {115.67, 37.07},
- "临西县": {115.5, 36.85},
- "å—宫市": {115.38, 37.35},
- "沙河市": {114.5, 36.85},
- "ä¿å®šå¸‚": {115.47, 38.87},
- "北市区": {115.48, 38.87},
- "å—市区": {115.5, 38.85},
- "满城县": {115.32, 38.95},
- "清苑县": {115.48, 38.77},
- "涞水县": {115.72, 39.4},
- "阜平县": {114.18, 38.85},
- "徿°´åŽ¿": {115.65, 39.02},
- "定兴县": {115.77, 39.27},
- "å”县": {114.98, 38.75},
- "高阳县": {115.78, 38.68},
- "容城县": {115.87, 39.05},
- "æ¶žæºåŽ¿": {114.68, 39.35},
- "望都县": {115.15, 38.72},
- "安新县": {115.93, 38.92},
- "易县": {115.5, 39.35},
- "曲阳县": {114.7, 38.62},
- "è ¡åŽ¿": {115.57, 38.48},
- "顺平县": {115.13, 38.83},
- "åšé‡ŽåŽ¿": {115.47, 38.45},
- "雄县": {116.1, 38.98},
- "涿州市": {115.97, 39.48},
- "定州市": {114.97, 38.52},
- "安国市": {115.32, 38.42},
- "高碑店市": {115.85, 39.33},
- "å¼ å®¶å£å¸‚": {114.88, 40.82},
- "宣化区": {115.05, 40.6},
- "下花å›åŒº": {115.27, 40.48},
- "宣化县": {115.02, 40.55},
- "å¼ åŒ—åŽ¿": {114.7, 41.15},
- "康ä¿åŽ¿": {114.62, 41.85},
- "æ²½æºåŽ¿": {115.7, 41.67},
- "尚义县": {113.97, 41.08},
- "蔚县": {114.57, 39.85},
- "阳原县": {114.17, 40.12},
- "怀安县": {114.42, 40.67},
- "万全县": {114.72, 40.75},
- "怀æ¥åŽ¿": {115.52, 40.4},
- "涿鹿县": {115.22, 40.38},
- "赤城县": {115.83, 40.92},
- "崇礼县": {115.27, 40.97},
- "承德市": {117.93, 40.97},
- "åŒæ»¦åŒº": {117.78, 40.95},
- "鹰手è¥å矿区": {117.65, 40.55},
- "承德县": {118.17, 40.77},
- "兴隆县": {117.52, 40.43},
- "平泉县": {118.68, 41},
- "滦平县": {117.33, 40.93},
- "隆化县": {117.72, 41.32},
- "䏰宿»¡æ—自治县": {116.65, 41.2},
- "宽城满æ—自治县": {118.48, 40.6},
- "围场满æ—è’™å¤æ—自治县": {117.75, 41.93},
- "沧州市": {116.83, 38.3},
- "è¿æ²³åŒº": {116.85, 38.32},
- "沧县": {116.87, 38.3},
- "é’县": {116.82, 38.58},
- "东光县": {116.53, 37.88},
- "海兴县": {117.48, 38.13},
- "ç›å±±åŽ¿": {117.22, 38.05},
- "肃å®åŽ¿": {115.83, 38.43},
- "å—皮县": {116.7, 38.03},
- "å´æ¡¥åŽ¿": {116.38, 37.62},
- "献县": {116.12, 38.18},
- "åŸæ‘回æ—自治县": {117.1, 38.07},
- "泊头市": {116.57, 38.07},
- "任丘市": {116.1, 38.72},
- "黄骅市": {117.35, 38.37},
- "河间市": {116.08, 38.43},
- "廊åŠå¸‚": {116.7, 39.52},
- "安次区": {116.68, 39.52},
- "广阳区": {116.72, 39.53},
- "固安县": {116.3, 39.43},
- "永清县": {116.5, 39.32},
- "香河县": {117, 39.77},
- "大城县": {116.63, 38.7},
- "文安县": {116.47, 38.87},
- "大厂回æ—自治县": {116.98, 39.88},
- "霸州市": {116.4, 39.1},
- "三河市": {117.07, 39.98},
- "衡水市": {115.68, 37.73},
- "桃城区": {115.68, 37.73},
- "枣强县": {115.72, 37.52},
- "æ¦é‚‘县": {115.88, 37.82},
- "æ¦å¼ºåŽ¿": {115.98, 38.03},
- "饶阳县": {115.73, 38.23},
- "安平县": {115.52, 38.23},
- "故城县": {115.97, 37.35},
- "景县": {116.27, 37.7},
- "阜城县": {116.15, 37.87},
- "冀州市": {115.57, 37.57},
- "深州市": {115.55, 38.02},
- "太原市": {112.55, 37.87},
- "å°åº—区": {112.57, 37.73},
- "迎泽区": {112.57, 37.87},
- "æèбå²åŒº": {112.57, 37.88},
- "å°–è‰åªåŒº": {112.48, 37.93},
- "ä¸‡æŸæž—区": {112.52, 37.87},
- "晋æºåŒº": {112.48, 37.73},
- "清å¾åŽ¿": {112.35, 37.6},
- "阳曲县": {112.67, 38.07},
- "娄烦县": {111.78, 38.07},
- "å¤äº¤å¸‚": {112.17, 37.92},
- "大åŒå¸‚": {113.3, 40.08},
- "å—郊区": {113.13, 40},
- "æ–°è£åŒº": {113.15, 40.27},
- "阳高县": {113.75, 40.37},
- "天镇县": {114.08, 40.42},
- "广çµåŽ¿": {114.28, 39.77},
- "çµä¸˜åŽ¿": {114.23, 39.43},
- "浑æºåŽ¿": {113.68, 39.7},
- "左云县": {112.7, 40},
- "大åŒåŽ¿": {113.6, 40.03},
- "阳泉市": {113.57, 37.85},
- "平定县": {113.62, 37.8},
- "盂县": {113.4, 38.08},
- "长治市": {113.12, 36.2},
- "长治县": {113.03, 36.05},
- "襄垣县": {113.05, 36.53},
- "屯留县": {112.88, 36.32},
- "平顺县": {113.43, 36.2},
- "黎城县": {113.38, 36.5},
- "壶关县": {113.2, 36.12},
- "é•¿å县": {112.87, 36.12},
- "æ¦ä¹¡åŽ¿": {112.85, 36.83},
- "æ²åŽ¿": {112.7, 36.75},
- "æ²æºåŽ¿": {112.33, 36.5},
- "潞城市": {113.22, 36.33},
- "晋城市": {112.83, 35.5},
- "æ²æ°´åŽ¿": {112.18, 35.68},
- "阳城县": {112.42, 35.48},
- "陵å·åŽ¿": {113.27, 35.78},
- "泽州县": {112.83, 35.5},
- "高平市": {112.92, 35.8},
- "朔州市": {112.43, 39.33},
- "朔城区": {112.43, 39.33},
- "山阴县": {112.82, 39.52},
- "应县": {113.18, 39.55},
- "å³çŽ‰åŽ¿": {112.47, 39.98},
- "怀ä»åŽ¿": {113.08, 39.83},
- "晋ä¸å¸‚": {112.75, 37.68},
- "榆次区": {112.75, 37.68},
- "榆社县": {112.97, 37.07},
- "å·¦æƒåŽ¿": {113.37, 37.07},
- "和顺县": {113.57, 37.33},
- "昔阳县": {113.7, 37.62},
- "寿阳县": {113.18, 37.88},
- "太谷县": {112.55, 37.42},
- "ç¥åŽ¿": {112.33, 37.35},
- "å¹³é¥åŽ¿": {112.17, 37.18},
- "çµçŸ³åŽ¿": {111.77, 36.85},
- "介休市": {111.92, 37.03},
- "è¿åŸŽå¸‚": {110.98, 35.02},
- "ç›æ¹–区": {110.98, 35.02},
- "临猗县": {110.77, 35.15},
- "万è£åŽ¿": {110.83, 35.42},
- "闻喜县": {111.22, 35.35},
- "稷山县": {110.97, 35.6},
- "新绛县": {111.22, 35.62},
- "绛县": {111.57, 35.48},
- "垣曲县": {111.67, 35.3},
- "å¤åŽ¿": {111.22, 35.15},
- "平陆县": {111.22, 34.83},
- "芮城县": {110.68, 34.7},
- "河津市": {110.7, 35.6},
- "忻州市": {112.73, 38.42},
- "忻府区": {112.73, 38.42},
- "定襄县": {112.95, 38.48},
- "五å°åŽ¿": {113.25, 38.73},
- "代县": {112.95, 39.07},
- "ç¹å³™åŽ¿": {113.25, 39.18},
- "宿¦åŽ¿": {112.3, 39},
- "é™ä¹åŽ¿": {111.93, 38.37},
- "ç¥žæ± åŽ¿": {112.2, 39.08},
- "五寨县": {111.85, 38.9},
- "岢岚县": {111.57, 38.7},
- "河曲县": {111.13, 39.38},
- "å关县": {111.5, 39.43},
- "原平市": {112.7, 38.73},
- "临汾市": {111.52, 36.08},
- "尧都区": {111.52, 36.08},
- "曲沃县": {111.47, 35.63},
- "翼城县": {111.72, 35.73},
- "襄汾县": {111.43, 35.88},
- "洪洞县": {111.67, 36.25},
- "å¤åŽ¿": {111.92, 36.27},
- "安泽县": {112.25, 36.15},
- "浮山县": {111.83, 35.97},
- "å‰åŽ¿": {110.68, 36.1},
- "乡å®åŽ¿": {110.83, 35.97},
- "大å®åŽ¿": {110.75, 36.47},
- "隰县": {110.93, 36.7},
- "永和县": {110.63, 36.77},
- "蒲县": {111.08, 36.42},
- "汾西县": {111.57, 36.65},
- "侯马市": {111.35, 35.62},
- "éœå·žå¸‚": {111.72, 36.57},
- "啿¢å¸‚": {111.13, 37.52},
- "离石区": {111.13, 37.52},
- "文水县": {112.02, 37.43},
- "交城县": {112.15, 37.55},
- "兴县": {111.12, 38.47},
- "临县": {110.98, 37.95},
- "柳林县": {110.9, 37.43},
- "石楼县": {110.83, 37},
- "岚县": {111.67, 38.28},
- "方山县": {111.23, 37.88},
- "ä¸é˜³åŽ¿": {111.18, 37.33},
- "交å£åŽ¿": {111.2, 36.97},
- "å义市": {111.77, 37.15},
- "汾阳市": {111.78, 37.27},
- "呼和浩特市": {111.73, 40.83},
- "回民区": {111.6, 40.8},
- "玉泉区": {111.67, 40.75},
- "赛罕区": {111.68, 40.8},
- "土默特左旗": {111.13, 40.72},
- "托克托县": {111.18, 40.27},
- "å’Œæž—æ ¼å°”åŽ¿": {111.82, 40.38},
- "清水河县": {111.68, 39.92},
- "æ¦å·åŽ¿": {111.45, 41.08},
- "包头市": {109.83, 40.65},
- "东河区": {110.02, 40.58},
- "昆都仑区": {109.83, 40.63},
- "石æ‹åŒº": {110.27, 40.68},
- "ä¹åŽŸåŒº": {109.97, 40.6},
- "åœŸé»˜ç‰¹å³æ——": {110.52, 40.57},
- "固阳县": {110.05, 41.03},
- "达尔罕茂明安è”åˆæ——": {110.43, 41.7},
- "乌海市": {106.82, 39.67},
- "海勃湾区": {106.83, 39.7},
- "æµ·å—区": {106.88, 39.43},
- "乌达区": {106.7, 39.5},
- "赤峰市": {118.92, 42.27},
- "红山区": {118.97, 42.28},
- "å…ƒå®å±±åŒº": {119.28, 42.03},
- "æ¾å±±åŒº": {118.92, 42.28},
- "阿é²ç§‘å°”æ²æ——": {120.08, 43.88},
- "巴林左旗": {119.38, 43.98},
- "å·´æž—å³æ——": {118.67, 43.52},
- "林西县": {118.05, 43.6},
- "克什克腾旗": {117.53, 43.25},
- "ç¿ç‰›ç‰¹æ——": {119.02, 42.93},
- "å–€å–‡æ²æ——": {118.7, 41.93},
- "å®åŸŽåŽ¿": {119.33, 41.6},
- "敖汉旗": {119.9, 42.28},
- "通辽市": {122.27, 43.62},
- "ç§‘å°”æ²åŒº": {122.27, 43.62},
- "ç§‘å°”æ²å·¦ç¿¼ä¸æ——": {123.32, 44.13},
- "ç§‘å°”æ²å·¦ç¿¼åŽæ——": {122.35, 42.95},
- "å¼€é²åŽ¿": {121.3, 43.6},
- "库伦旗": {121.77, 42.73},
- "奈曼旗": {120.65, 42.85},
- "扎é²ç‰¹æ——": {120.92, 44.55},
- "éœæž—éƒå‹’市": {119.65, 45.53},
- "鄂尔多斯市": {109.8, 39.62},
- "东胜区": {110, 39.82},
- "达拉特旗": {110.03, 40.4},
- "å‡†æ ¼å°”æ——": {111.23, 39.87},
- "é„‚æ‰˜å…‹å‰æ——": {107.48, 38.18},
- "鄂托克旗": {107.98, 39.1},
- "æé”¦æ——": {108.72, 39.83},
- "乌审旗": {108.85, 38.6},
- "ä¼Šé‡‘éœæ´›æ——": {109.73, 39.57},
- "呼伦è´å°”市": {119.77, 49.22},
- "海拉尔区": {119.77, 49.22},
- "é˜¿è£æ——": {123.47, 48.13},
- "鄂伦春自治旗": {123.72, 50.58},
- "鄂温克æ—自治旗": {119.75, 49.13},
- "陈巴尔虎旗": {119.43, 49.32},
- "新巴尔虎左旗": {118.27, 48.22},
- "æ–°å·´å°”è™Žå³æ——": {116.82, 48.67},
- "满洲里市": {117.45, 49.58},
- "牙克石市": {120.73, 49.28},
- "扎兰屯市": {122.75, 47.98},
- "é¢å°”å¤çº³å¸‚": {120.18, 50.23},
- "æ ¹æ²³å¸‚": {121.52, 50.78},
- "巴彦淖尔市": {107.42, 40.75},
- "临河区": {107.4, 40.75},
- "五原县": {108.27, 41.1},
- "磴å£åŽ¿": {107.02, 40.33},
- "ä¹Œæ‹‰ç‰¹å‰æ——": {108.65, 40.72},
- "ä¹Œæ‹‰ç‰¹ä¸æ——": {108.52, 41.57},
- "ä¹Œæ‹‰ç‰¹åŽæ——": {107.07, 41.1},
- "æé”¦åŽæ——": {107.15, 40.88},
- "乌兰察布市": {113.12, 40.98},
- "集å®åŒº": {113.1, 41.03},
- "å“资县": {112.57, 40.9},
- "化德县": {114, 41.9},
- "商都县": {113.53, 41.55},
- "兴和县": {113.88, 40.88},
- "凉城县": {112.48, 40.53},
- "察哈尔å³ç¿¼å‰æ——": {113.22, 40.78},
- "察哈尔å³ç¿¼ä¸æ——": {112.63, 41.27},
- "察哈尔å³ç¿¼åŽæ——": {113.18, 41.45},
- "å››å王旗": {111.7, 41.52},
- "丰镇市": {113.15, 40.43},
- "兴安盟": {122.05, 46.08},
- "乌兰浩特市": {122.05, 46.08},
- "阿尔山市": {119.93, 47.18},
- "ç§‘å°”æ²å³ç¿¼å‰æ——": {121.92, 46.07},
- "ç§‘å°”æ²å³ç¿¼ä¸æ——": {121.47, 45.05},
- "扎赉特旗": {122.9, 46.73},
- "çªæ³‰åŽ¿": {121.57, 45.38},
- "锡林éƒå‹’盟": {116.07, 43.95},
- "二连浩特市": {111.98, 43.65},
- "锡林浩特市": {116.07, 43.93},
- "阿巴嘎旗": {114.97, 44.02},
- "è‹å°¼ç‰¹å·¦æ——": {113.63, 43.85},
- "è‹å°¼ç‰¹å³æ——": {112.65, 42.75},
- "东乌ç ç©†æ²æ——": {116.97, 45.52},
- "西乌ç ç©†æ²æ——": {117.6, 44.58},
- "太仆寺旗": {115.28, 41.9},
- "镶黄旗": {113.83, 42.23},
- "æ£é•¶ç™½æ——": {115, 42.3},
- "æ£è“æ——": {116, 42.25},
- "多伦县": {116.47, 42.18},
- "阿拉善盟": {105.67, 38.83},
- "阿拉善左旗": {105.67, 38.83},
- "é˜¿æ‹‰å–„å³æ——": {101.68, 39.2},
- "颿µŽçº³æ——": {101.07, 41.97},
- "沈阳市": {123.43, 41.8},
- "和平区": {123.4, 41.78},
- "沈河区": {123.45, 41.8},
- "大东区": {123.47, 41.8},
- "皇姑区": {123.42, 41.82},
- "é“西区": {123.35, 41.8},
- "è‹å®¶å±¯åŒº": {123.33, 41.67},
- "东陵区": {123.47, 41.77},
- "新城å区": {123.52, 42.05},
- "于洪区": {123.3, 41.78},
- "è¾½ä¸åŽ¿": {122.72, 41.52},
- "康平县": {123.35, 42.75},
- "法库县": {123.4, 42.5},
- "新民市": {122.82, 42},
- "大连市": {121.62, 38.92},
- "ä¸å±±åŒº": {121.63, 38.92},
- "西岗区": {121.6, 38.92},
- "沙河å£åŒº": {121.58, 38.9},
- "甘井å区": {121.57, 38.95},
- "旅顺å£åŒº": {121.27, 38.82},
- "金州区": {121.7, 39.1},
- "长海县": {122.58, 39.27},
- "瓦房店市": {122, 39.62},
- "普兰店市": {121.95, 39.4},
- "庄河市": {122.98, 39.7},
- "éžå±±å¸‚": {122.98, 41.1},
- "立山区": {123, 41.15},
- "åƒå±±åŒº": {122.97, 41.07},
- "å°å®‰åŽ¿": {122.42, 41.38},
- "岫岩满æ—自治县": {123.28, 40.28},
- "海城市": {122.7, 40.88},
- "抚顺市": {123.98, 41.88},
- "新抚区": {123.88, 41.87},
- "东洲区": {124.02, 41.85},
- "望花区": {123.78, 41.85},
- "顺城区": {123.93, 41.88},
- "抚顺县": {123.9, 41.88},
- "新宾满æ—自治县": {125.03, 41.73},
- "清原满æ—自治县": {124.92, 42.1},
- "本溪市": {123.77, 41.3},
- "平山区": {123.77, 41.3},
- "溪湖区": {123.77, 41.33},
- "明山区": {123.82, 41.3},
- "å—芬区": {123.73, 41.1},
- "本溪满æ—自治县": {124.12, 41.3},
- "æ¡“ä»æ»¡æ—自治县": {125.35, 41.27},
- "丹东市": {124.38, 40.13},
- "å…ƒå®åŒº": {124.38, 40.13},
- "振兴区": {124.35, 40.08},
- "振安区": {124.42, 40.17},
- "宽甸满æ—自治县": {124.78, 40.73},
- "东港市": {124.15, 39.87},
- "凤城市": {124.07, 40.45},
- "锦州市": {121.13, 41.1},
- "å¤å¡”区": {121.12, 41.13},
- "凌河区": {121.15, 41.12},
- "太和区": {121.1, 41.1},
- "黑山县": {122.12, 41.7},
- "义县": {121.23, 41.53},
- "凌海市": {121.35, 41.17},
- "è¥å£å¸‚": {122.23, 40.67},
- "ç«™å‰åŒº": {122.27, 40.68},
- "西市区": {122.22, 40.67},
- "鲅鱼圈区": {122.12, 40.27},
- "è€è¾¹åŒº": {122.37, 40.67},
- "盖州市": {122.35, 40.4},
- "大石桥市": {122.5, 40.65},
- "阜新市": {121.67, 42.02},
- "海州区": {121.65, 42.02},
- "太平区": {121.67, 42.02},
- "清河门区": {121.42, 41.75},
- "细河区": {121.68, 42.03},
- "é˜œæ–°è’™å¤æ—自治县": {121.75, 42.07},
- "å½°æ¦åŽ¿": {122.53, 42.38},
- "辽阳市": {123.17, 41.27},
- "白塔区": {123.17, 41.27},
- "文圣区": {123.18, 41.27},
- "å®ä¼ŸåŒº": {123.2, 41.2},
- "弓长å²åŒº": {123.45, 41.13},
- "å¤ªåæ²³åŒº": {123.18, 41.25},
- "辽阳县": {123.07, 41.22},
- "ç¯å¡”市": {123.33, 41.42},
- "盘锦市": {122.07, 41.12},
- "åŒå°å区": {122.05, 41.2},
- "兴隆å°åŒº": {122.07, 41.12},
- "大洼县": {122.07, 40.98},
- "盘山县": {122.02, 41.25},
- "é“å²å¸‚": {123.83, 42.28},
- "银州区": {123.85, 42.28},
- "é“å²åŽ¿": {123.83, 42.3},
- "西丰县": {124.72, 42.73},
- "昌图县": {124.1, 42.78},
- "调兵山市": {123.55, 42.47},
- "开原市": {124.03, 42.55},
- "æœé˜³å¸‚": {120.45, 41.57},
- "åŒå¡”区": {120.45, 41.57},
- "龙城区": {120.43, 41.6},
- "æœé˜³åŽ¿": {120.47, 41.58},
- "建平县": {119.63, 41.4},
- "北票市": {120.77, 41.8},
- "凌æºå¸‚": {119.4, 41.25},
- "葫芦岛市": {120.83, 40.72},
- "连山区": {120.87, 40.77},
- "龙港区": {120.93, 40.72},
- "å—票区": {120.75, 41.1},
- "绥ä¸åŽ¿": {120.33, 40.32},
- "建昌县": {119.8, 40.82},
- "兴城市": {120.72, 40.62},
- "长春市": {125.32, 43.9},
- "å—关区": {125.33, 43.87},
- "宽城区": {125.32, 43.92},
- "æœé˜³åŒº": {125.28, 43.83},
- "二é“区": {125.37, 43.87},
- "绿å›åŒº": {125.25, 43.88},
- "åŒé˜³åŒº": {125.67, 43.52},
- "农安县": {125.18, 44.43},
- "ä¹å°å¸‚": {125.83, 44.15},
- "æ¦†æ ‘å¸‚": {126.55, 44.82},
- "å¾·æƒ å¸‚": {125.7, 44.53},
- "剿ž—市": {126.55, 43.83},
- "昌邑区": {126.57, 43.88},
- "é¾™æ½åŒº": {126.57, 43.92},
- "船è¥åŒº": {126.53, 43.83},
- "丰满区": {126.57, 43.82},
- "æ°¸å‰åŽ¿": {126.5, 43.67},
- "蛟河市": {127.33, 43.72},
- "桦甸市": {126.73, 42.97},
- "舒兰市": {126.95, 44.42},
- "ç£çŸ³å¸‚": {126.05, 42.95},
- "四平市": {124.35, 43.17},
- "é“东区": {124.38, 43.17},
- "æ¢¨æ ‘åŽ¿": {124.33, 43.32},
- "伊通满æ—自治县": {125.3, 43.35},
- "公主å²å¸‚": {124.82, 43.5},
- "åŒè¾½å¸‚": {123.5, 43.52},
- "è¾½æºå¸‚": {125.13, 42.88},
- "龙山区": {125.12, 42.9},
- "西安区": {125.15, 42.92},
- "东丰县": {125.53, 42.68},
- "东辽县": {125, 42.92},
- "通化市": {125.93, 41.73},
- "东昌区": {125.95, 41.73},
- "äºŒé“æ±ŸåŒº": {126.03, 41.77},
- "通化县": {125.75, 41.68},
- "辉å—县": {126.03, 42.68},
- "柳河县": {125.73, 42.28},
- "梅河å£å¸‚": {125.68, 42.53},
- "集安市": {126.18, 41.12},
- "白山市": {126.42, 41.93},
- "八铿±ŸåŒº": {126.4, 41.93},
- "抚æ¾åŽ¿": {127.28, 42.33},
- "é–宇县": {126.8, 42.4},
- "长白æœé²œæ—自治县": {128.2, 41.42},
- "临江市": {126.9, 41.8},
- "æ¾åŽŸå¸‚": {124.82, 45.13},
- "宿±ŸåŒº": {124.8, 45.17},
- "é•¿å²åŽ¿": {123.98, 44.28},
- "乾安县": {124.02, 45.02},
- "扶余县": {126.02, 44.98},
- "白城市": {122.83, 45.62},
- "洮北区": {122.85, 45.62},
- "镇赉县": {123.2, 45.85},
- "通榆县": {123.08, 44.82},
- "æ´®å—市": {122.78, 45.33},
- "大安市": {124.28, 45.5},
- "å»¶è¾¹æœé²œæ—自治州": {129.5, 42.88},
- "å»¶å‰å¸‚": {129.5, 42.88},
- "图们市": {129.83, 42.97},
- "敦化市": {128.23, 43.37},
- "ç²æ˜¥å¸‚": {130.37, 42.87},
- "龙井市": {129.42, 42.77},
- "和龙市": {129, 42.53},
- "汪清县": {129.75, 43.32},
- "安图县": {128.9, 43.12},
- "哈尔滨市": {126.53, 45.8},
- "é“里区": {126.62, 45.77},
- "å—岗区": {126.68, 45.77},
- "é“外区": {126.65, 45.78},
- "香åŠåŒº": {126.68, 45.72},
- "平房区": {126.62, 45.62},
- "æ¾åŒ—区": {126.55, 45.8},
- "呼兰区": {126.58, 45.9},
- "ä¾å…°åŽ¿": {129.55, 46.32},
- "æ–¹æ£åŽ¿": {128.83, 45.83},
- "宾县": {127.48, 45.75},
- "巴彦县": {127.4, 46.08},
- "木兰县": {128.03, 45.95},
- "通河县": {128.75, 45.97},
- "延寿县": {128.33, 45.45},
- "åŒåŸŽå¸‚": {126.32, 45.37},
- "尚志市": {127.95, 45.22},
- "五常市": {127.15, 44.92},
- "é½é½å“ˆå°”市": {123.95, 47.33},
- "龙沙区": {123.95, 47.32},
- "建åŽåŒº": {123.95, 47.35},
- "é“锋区": {123.98, 47.35},
- "昂昂溪区": {123.8, 47.15},
- "富拉尔基区": {123.62, 47.2},
- "龙江县": {123.18, 47.33},
- "ä¾å®‰åŽ¿": {125.3, 47.88},
- "æ³°æ¥åŽ¿": {123.42, 46.4},
- "甘å—县": {123.5, 47.92},
- "富裕县": {124.47, 47.82},
- "克山县": {125.87, 48.03},
- "克东县": {126.25, 48.03},
- "拜泉县": {126.08, 47.6},
- "讷河市": {124.87, 48.48},
- "鸡西市": {130.97, 45.3},
- "é¸¡å† åŒº": {130.97, 45.3},
- "æ’山区": {130.93, 45.2},
- "æ»´é“区": {130.78, 45.37},
- "æ¢¨æ ‘åŒº": {130.68, 45.08},
- "åŸŽåæ²³åŒº": {131, 45.33},
- "麻山区": {130.52, 45.2},
- "鸡东县": {131.13, 45.25},
- "虎林市": {132.98, 45.77},
- "密山市": {131.87, 45.55},
- "鹤岗市": {130.27, 47.33},
- "å‘阳区": {130.28, 47.33},
- "工农区": {130.25, 47.32},
- "兴安区": {130.22, 47.27},
- "东山区": {130.32, 47.33},
- "兴山区": {130.3, 47.37},
- "è北县": {130.83, 47.58},
- "绥滨县": {131.85, 47.28},
- "åŒé¸å±±å¸‚": {131.15, 46.63},
- "尖山区": {131.17, 46.63},
- "å²ä¸œåŒº": {131.13, 46.57},
- "四方å°åŒº": {131.33, 46.58},
- "集贤县": {131.13, 46.72},
- "å‹è°ŠåŽ¿": {131.8, 46.78},
- "宿¸…县": {132.2, 46.32},
- "饶河县": {134.02, 46.8},
- "大庆市": {125.03, 46.58},
- "è¨å°”图区": {125.02, 46.6},
- "龙凤区": {125.1, 46.53},
- "让胡路区": {124.85, 46.65},
- "红岗区": {124.88, 46.4},
- "大åŒåŒº": {124.82, 46.03},
- "肇州县": {125.27, 45.7},
- "肇æºåŽ¿": {125.08, 45.52},
- "林甸县": {124.87, 47.18},
- "æœå°”ä¼¯ç‰¹è’™å¤æ—自治县": {124.45, 46.87},
- "伊春市": {128.9, 47.73},
- "å—岔区": {129.28, 47.13},
- "å‹å¥½åŒº": {128.82, 47.85},
- "西林区": {129.28, 47.48},
- "ç¿ å³¦åŒº": {128.65, 47.72},
- "æ–°é’区": {129.53, 48.28},
- "美溪区": {129.13, 47.63},
- "金山屯区": {129.43, 47.42},
- "五è¥åŒº": {129.25, 48.12},
- "乌马河区": {128.78, 47.72},
- "汤旺河区": {129.57, 48.45},
- "带å²åŒº": {129.02, 47.02},
- "乌伊å²åŒº": {129.42, 48.6},
- "红星区": {129.38, 48.23},
- "上甘å²åŒº": {129.02, 47.97},
- "嘉è«åŽ¿": {130.38, 48.88},
- "é“力市": {128.02, 46.98},
- "佳木斯市": {130.37, 46.82},
- "å‰è¿›åŒº": {130.37, 46.82},
- "东风区": {130.4, 46.82},
- "桦å—县": {130.57, 46.23},
- "桦å·åŽ¿": {130.72, 47.02},
- "汤原县": {129.9, 46.73},
- "抚远县": {134.28, 48.37},
- "åŒæ±Ÿå¸‚": {132.52, 47.65},
- "富锦市": {132.03, 47.25},
- "ä¸ƒå°æ²³å¸‚": {130.95, 45.78},
- "新兴区": {130.83, 45.8},
- "桃山区": {130.97, 45.77},
- "èŒ„åæ²³åŒº": {131.07, 45.77},
- "勃利县": {130.57, 45.75},
- "牡丹江市": {129.6, 44.58},
- "东安区": {129.62, 44.58},
- "阳明区": {129.63, 44.6},
- "爱民区": {129.58, 44.58},
- "东å®åŽ¿": {131.12, 44.07},
- "æž—å£åŽ¿": {130.27, 45.3},
- "绥芬河市": {131.15, 44.42},
- "海林市": {129.38, 44.57},
- "å®å®‰å¸‚": {129.47, 44.35},
- "穆棱市": {130.52, 44.92},
- "黑河市": {127.48, 50.25},
- "爱辉区": {127.48, 50.25},
- "逊克县": {128.47, 49.58},
- "å™å´åŽ¿": {127.32, 49.42},
- "北安市": {126.52, 48.23},
- "äº”å¤§è¿žæ± å¸‚": {126.2, 48.52},
- "绥化市": {126.98, 46.63},
- "北林区": {126.98, 46.63},
- "望奎县": {126.48, 46.83},
- "兰西县": {126.28, 46.27},
- "é’冈县": {126.1, 46.68},
- "庆安县": {127.52, 46.88},
- "明水县": {125.9, 47.18},
- "绥棱县": {127.1, 47.25},
- "安达市": {125.33, 46.4},
- "肇东市": {125.98, 46.07},
- "海伦市": {126.97, 47.47},
- "大兴安å²åœ°åŒº": {124.12, 50.42},
- "呼玛县": {126.65, 51.73},
- "塔河县": {124.7, 52.32},
- "æ¼ æ²³åŽ¿": {122.53, 52.97},
- "上海市": {121.47, 31.23},
- "黄浦区": {121.48, 31.23},
- "墿¹¾åŒº": {121.47, 31.22},
- "徿±‡åŒº": {121.43, 31.18},
- "é•¿å®åŒº": {121.42, 31.22},
- "é™å®‰åŒº": {121.45, 31.23},
- "闸北区": {121.45, 31.25},
- "虹å£åŒº": {121.5, 31.27},
- "æ¨æµ¦åŒº": {121.52, 31.27},
- "闵行区": {121.38, 31.12},
- "å®å±±åŒº": {121.48, 31.4},
- "嘉定区": {121.27, 31.38},
- "浦东新区": {121.53, 31.22},
- "金山区": {121.33, 30.75},
- "æ¾æ±ŸåŒº": {121.22, 31.03},
- "é’æµ¦åŒº": {121.12, 31.15},
- "å—æ±‡åŒº": {121.75, 31.05},
- "奉贤区": {121.47, 30.92},
- "崇明县": {121.4, 31.62},
- "å—京市": {118.78, 32.07},
- "玄æ¦åŒº": {118.8, 32.05},
- "白下区": {118.78, 32.03},
- "秦淮区": {118.8, 32.02},
- "建邺区": {118.75, 32.03},
- "下关区": {118.73, 32.08},
- "浦å£åŒº": {118.62, 32.05},
- "æ –éœžåŒº": {118.88, 32.12},
- "雨花å°åŒº": {118.77, 32},
- "江å®åŒº": {118.85, 31.95},
- "å…åˆåŒº": {118.83, 32.35},
- "溧水县": {119.02, 31.65},
- "高淳县": {118.88, 31.33},
- "æ— é”¡å¸‚": {120.3, 31.57},
- "崇安区": {120.3, 31.58},
- "å—长区": {120.3, 31.57},
- "北塘区": {120.28, 31.58},
- "锡山区": {120.35, 31.6},
- "æƒ å±±åŒº": {120.28, 31.68},
- "滨湖区": {120.27, 31.57},
- "江阴市": {120.27, 31.9},
- "宜兴市": {119.82, 31.35},
- "å¾å·žå¸‚": {117.18, 34.27},
- "云龙区": {117.22, 34.25},
- "ä¹é‡ŒåŒº": {117.13, 34.3},
- "贾汪区": {117.45, 34.45},
- "泉山区": {117.18, 34.25},
- "丰县": {116.6, 34.7},
- "沛县": {116.93, 34.73},
- "铜山县": {117.17, 34.18},
- "ç¢å®åŽ¿": {117.95, 33.9},
- "新沂市": {118.35, 34.38},
- "邳州市": {117.95, 34.32},
- "常州市": {119.95, 31.78},
- "天å®åŒº": {119.93, 31.75},
- "钟楼区": {119.93, 31.78},
- "æˆšå¢…å °åŒº": {120.05, 31.73},
- "新北区": {119.97, 31.83},
- "æ¦è¿›åŒº": {119.93, 31.72},
- "溧阳市": {119.48, 31.42},
- "金å›å¸‚": {119.57, 31.75},
- "è‹å·žå¸‚": {120.58, 31.3},
- "沧浪区": {120.63, 31.3},
- "平江区": {120.63, 31.32},
- "金阊区": {120.6, 31.32},
- "虎丘区": {120.57, 31.3},
- "å´ä¸åŒº": {120.63, 31.27},
- "相城区": {120.63, 31.37},
- "常熟市": {120.75, 31.65},
- "å¼ å®¶æ¸¯å¸‚": {120.55, 31.87},
- "昆山市": {120.98, 31.38},
- "å´æ±Ÿå¸‚": {120.63, 31.17},
- "太仓市": {121.1, 31.45},
- "å—通市": {120.88, 31.98},
- "å´‡å·åŒº": {120.85, 32},
- "港闸区": {120.8, 32.03},
- "海安县": {120.45, 32.55},
- "如东县": {121.18, 32.32},
- "å¯ä¸œå¸‚": {121.65, 31.82},
- "如皋市": {120.57, 32.4},
- "通州市": {121.07, 32.08},
- "海门市": {121.17, 31.9},
- "连云港市": {119.22, 34.6},
- "连云区": {119.37, 34.75},
- "新浦区": {119.17, 34.6},
- "赣榆县": {119.12, 34.83},
- "东海县": {118.77, 34.53},
- "çŒäº‘县": {119.25, 34.3},
- "çŒå—县": {119.35, 34.08},
- "淮安市": {119.02, 33.62},
- "清河区": {119.02, 33.6},
- "楚州区": {119.13, 33.5},
- "淮阴区": {119.03, 33.63},
- "清浦区": {119.03, 33.58},
- "涟水县": {119.27, 33.78},
- "洪泽县": {118.83, 33.3},
- "盱眙县": {118.48, 33},
- "金湖县": {119.02, 33.02},
- "ç›åŸŽå¸‚": {120.15, 33.35},
- "äºæ¹–区": {120.13, 33.4},
- "ç›éƒ½åŒº": {120.15, 33.33},
- "哿°´åŽ¿": {119.57, 34.2},
- "滨海县": {119.83, 33.98},
- "阜å®åŽ¿": {119.8, 33.78},
- "射阳县": {120.25, 33.78},
- "建湖县": {119.8, 33.47},
- "东å°å¸‚": {120.3, 32.85},
- "大丰市": {120.47, 33.2},
- "扬州市": {119.4, 32.4},
- "广陵区": {119.43, 32.38},
- "邗江区": {119.4, 32.38},
- "维扬区": {119.4, 32.42},
- "å®åº”县": {119.3, 33.23},
- "仪å¾å¸‚": {119.18, 32.27},
- "高邮市": {119.43, 32.78},
- "江都市": {119.55, 32.43},
- "镇江市": {119.45, 32.2},
- "京å£åŒº": {119.47, 32.2},
- "润州区": {119.4, 32.2},
- "丹徒区": {119.45, 32.13},
- "丹阳市": {119.57, 32},
- "扬ä¸å¸‚": {119.82, 32.23},
- "å¥å®¹å¸‚": {119.17, 31.95},
- "泰州市": {119.92, 32.45},
- "兴化市": {119.85, 32.92},
- "é–æ±Ÿå¸‚": {120.27, 32.02},
- "泰兴市": {120.02, 32.17},
- "å§œå °å¸‚": {120.15, 32.52},
- "宿è¿å¸‚": {118.28, 33.97},
- "宿城区": {118.25, 33.97},
- "宿豫区": {118.32, 33.95},
- "æ²é˜³åŽ¿": {118.77, 34.13},
- "泗阳县": {118.68, 33.72},
- "泗洪县": {118.22, 33.47},
- "æå·žå¸‚": {120.15, 30.28},
- "上城区": {120.17, 30.25},
- "下城区": {120.17, 30.28},
- "江干区": {120.2, 30.27},
- "拱墅区": {120.13, 30.32},
- "滨江区": {120.2, 30.2},
- "è§å±±åŒº": {120.27, 30.17},
- "ä½™æåŒº": {120.3, 30.42},
- "æ¡åºåŽ¿": {119.67, 29.8},
- "淳安县": {119.03, 29.6},
- "建德市": {119.28, 29.48},
- "富阳市": {119.95, 30.05},
- "临安市": {119.72, 30.23},
- "宿³¢å¸‚": {121.55, 29.88},
- "海曙区": {121.55, 29.87},
- "江东区": {121.57, 29.87},
- "北仑区": {121.85, 29.93},
- "镇海区": {121.72, 29.95},
- "鄞州区": {121.53, 29.83},
- "象山县": {121.87, 29.48},
- "宿µ·åŽ¿": {121.43, 29.28},
- "余姚市": {121.15, 30.03},
- "慈溪市": {121.23, 30.17},
- "奉化市": {121.4, 29.65},
- "温州市": {120.7, 28},
- "鹿城区": {120.65, 28.02},
- "龙湾区": {120.82, 27.93},
- "洞头县": {121.15, 27.83},
- "永嘉县": {120.68, 28.15},
- "平阳县": {120.57, 27.67},
- "è‹å—县": {120.4, 27.5},
- "æ–‡æˆåŽ¿": {120.08, 27.78},
- "泰顺县": {119.72, 27.57},
- "瑞安市": {120.63, 27.78},
- "乿¸…市": {120.95, 28.13},
- "嘉兴市": {120.75, 30.75},
- "秀洲区": {120.7, 30.77},
- "嘉善县": {120.92, 30.85},
- "æµ·ç›åŽ¿": {120.95, 30.53},
- "æµ·å®å¸‚": {120.68, 30.53},
- "平湖市": {121.02, 30.7},
- "æ¡ä¹¡å¸‚": {120.57, 30.63},
- "湖州市": {120.08, 30.9},
- "å´å…´åŒº": {120.12, 30.87},
- "å—æµ”区": {120.43, 30.88},
- "德清县": {119.97, 30.53},
- "长兴县": {119.9, 31.02},
- "安å‰åŽ¿": {119.68, 30.63},
- "ç»å…´å¸‚": {120.57, 30},
- "越城区": {120.57, 30},
- "ç»å…´åŽ¿": {120.47, 30.08},
- "新昌县": {120.9, 29.5},
- "诸暨市": {120.23, 29.72},
- "上虞市": {120.87, 30.03},
- "嵊州市": {120.82, 29.58},
- "金åŽå¸‚": {119.65, 29.08},
- "婺城区": {119.65, 29.08},
- "金东区": {119.7, 29.08},
- "æ¦ä¹‰åŽ¿": {119.82, 28.9},
- "浦江县": {119.88, 29.45},
- "ç£å®‰åŽ¿": {120.43, 29.05},
- "兰溪市": {119.45, 29.22},
- "义乌市": {120.07, 29.3},
- "东阳市": {120.23, 29.28},
- "永康市": {120.03, 28.9},
- "衢州市": {118.87, 28.93},
- "柯城区": {118.87, 28.93},
- "衢江区": {118.93, 28.98},
- "常山县": {118.52, 28.9},
- "开化县": {118.42, 29.13},
- "龙游县": {119.17, 29.03},
- "江山市": {118.62, 28.75},
- "舟山市": {122.2, 30},
- "定海区": {122.1, 30.02},
- "普陀区": {122.3, 29.95},
- "岱山县": {122.2, 30.25},
- "嵊泗县": {122.45, 30.73},
- "å°å·žå¸‚": {121.43, 28.68},
- "椒江区": {121.43, 28.68},
- "黄岩区": {121.27, 28.65},
- "路桥区": {121.38, 28.58},
- "玉环县": {121.23, 28.13},
- "三门县": {121.38, 29.12},
- "天å°åŽ¿": {121.03, 29.13},
- "仙居县": {120.73, 28.87},
- "温å²å¸‚": {121.37, 28.37},
- "临海市": {121.12, 28.85},
- "丽水市": {119.92, 28.45},
- "莲都区": {119.92, 28.45},
- "é’田县": {120.28, 28.15},
- "缙云县": {120.07, 28.65},
- "邿˜ŒåŽ¿": {119.27, 28.6},
- "æ¾é˜³åŽ¿": {119.48, 28.45},
- "云和县": {119.57, 28.12},
- "庆元县": {119.05, 27.62},
- "景å®ç•²æ—自治县": {119.63, 27.98},
- "龙泉市": {119.13, 28.08},
- "åˆè‚¥å¸‚": {117.25, 31.83},
- "瑶海区": {117.3, 31.87},
- "åºé˜³åŒº": {117.25, 31.88},
- "蜀山区": {117.27, 31.85},
- "包河区": {117.3, 31.8},
- "长丰县": {117.17, 32.48},
- "肥东县": {117.47, 31.88},
- "肥西县": {117.17, 31.72},
- "芜湖市": {118.38, 31.33},
- "镜湖区": {118.37, 31.35},
- "é¸ æ±ŸåŒº": {118.38, 31.37},
- "芜湖县": {118.57, 31.15},
- "ç¹æ˜ŒåŽ¿": {118.2, 31.08},
- "å—陵县": {118.33, 30.92},
- "èšŒåŸ å¸‚": {117.38, 32.92},
- "龙忹–区": {117.38, 32.95},
- "蚌山区": {117.35, 32.95},
- "禹会区": {117.33, 32.93},
- "淮上区": {117.35, 32.97},
- "怀远县": {117.18, 32.97},
- "五河县": {117.88, 33.15},
- "固镇县": {117.32, 33.32},
- "æ·®å—市": {117, 32.63},
- "大通区": {117.05, 32.63},
- "田家庵区": {117, 32.67},
- "谢家集区": {116.85, 32.6},
- "八公山区": {116.83, 32.63},
- "潘集区": {116.82, 32.78},
- "凤å°åŽ¿": {116.72, 32.7},
- "马éžå±±å¸‚": {118.5, 31.7},
- "金家庄区": {118.48, 31.73},
- "花山区": {118.5, 31.72},
- "雨山区": {118.48, 31.68},
- "当涂县": {118.48, 31.55},
- "淮北市": {116.8, 33.95},
- "æœé›†åŒº": {116.82, 34},
- "相山区": {116.8, 33.95},
- "烈山区": {116.8, 33.9},
- "濉溪县": {116.77, 33.92},
- "铜陵市": {117.82, 30.93},
- "铜官山区": {117.82, 30.93},
- "ç‹®å山区": {117.85, 30.95},
- "铜陵县": {117.78, 30.95},
- "安庆市": {117.05, 30.53},
- "迎江区": {117.05, 30.5},
- "大观区": {117.03, 30.52},
- "怀å®åŽ¿": {116.83, 30.72},
- "枞阳县": {117.2, 30.7},
- "潜山县": {116.57, 30.63},
- "太湖县": {116.27, 30.43},
- "宿æ¾åŽ¿": {116.12, 30.15},
- "望江县": {116.68, 30.13},
- "岳西县": {116.35, 30.85},
- "æ¡åŸŽå¸‚": {116.95, 31.05},
- "黄山市": {118.33, 29.72},
- "屯溪区": {118.33, 29.72},
- "黄山区": {118.13, 30.3},
- "徽州区": {118.33, 29.82},
- "æ™åŽ¿": {118.43, 29.87},
- "休å®åŽ¿": {118.18, 29.78},
- "黟县": {117.93, 29.93},
- "ç¥é—¨åŽ¿": {117.72, 29.87},
- "æ»å·žå¸‚": {118.32, 32.3},
- "ç…çŠåŒº": {118.3, 32.3},
- "å—谯区": {118.3, 32.32},
- "æ¥å®‰åŽ¿": {118.43, 32.45},
- "全椒县": {118.27, 32.1},
- "定远县": {117.67, 32.53},
- "凤阳县": {117.57, 32.87},
- "天长市": {119, 32.7},
- "明光市": {117.98, 32.78},
- "阜阳市": {115.82, 32.9},
- "é¢å·žåŒº": {115.8, 32.88},
- "é¢ä¸œåŒº": {115.85, 32.92},
- "颿³‰åŒº": {115.8, 32.93},
- "临泉县": {115.25, 33.07},
- "太和县": {115.62, 33.17},
- "阜å—县": {115.58, 32.63},
- "é¢ä¸ŠåŽ¿": {116.27, 32.63},
- "宿州市": {116.98, 33.63},
- "埇桥区": {116.97, 33.63},
- "ç €å±±åŽ¿": {116.35, 34.42},
- "è§åŽ¿": {116.93, 34.18},
- "çµç’§åŽ¿": {117.55, 33.55},
- "泗县": {117.88, 33.48},
- "巢湖市": {117.87, 31.6},
- "居巢区": {117.85, 31.6},
- "åºæ±ŸåŽ¿": {117.28, 31.25},
- "æ— ä¸ºåŽ¿": {117.92, 31.3},
- "å«å±±åŽ¿": {118.1, 31.72},
- "和县": {118.37, 31.72},
- "å…安市": {116.5, 31.77},
- "金安区": {116.5, 31.77},
- "裕安区": {116.48, 31.77},
- "寿县": {116.78, 32.58},
- "éœé‚±åŽ¿": {116.27, 32.33},
- "舒城县": {116.93, 31.47},
- "金寨县": {115.92, 31.72},
- "éœå±±åŽ¿": {116.33, 31.4},
- "亳州市": {115.78, 33.85},
- "谯城区": {115.77, 33.88},
- "涡阳县": {116.22, 33.52},
- "蒙城县": {116.57, 33.27},
- "利辛县": {116.2, 33.15},
- "æ± å·žå¸‚": {117.48, 30.67},
- "è´µæ± åŒº": {117.48, 30.65},
- "东至县": {117.02, 30.1},
- "石å°åŽ¿": {117.48, 30.22},
- "é’阳县": {117.85, 30.65},
- "宣城市": {118.75, 30.95},
- "宣州区": {118.75, 30.95},
- "郎溪县": {119.17, 31.13},
- "广德县": {119.42, 30.9},
- "泾县": {118.4, 30.7},
- "绩溪县": {118.6, 30.07},
- "旌德县": {118.53, 30.28},
- "å®å›½å¸‚": {118.98, 30.63},
- "ç¦å·žå¸‚": {119.3, 26.08},
- "鼓楼区": {119.3, 26.08},
- "å°æ±ŸåŒº": {119.3, 26.07},
- "仓山区": {119.32, 26.05},
- "马尾区": {119.45, 26},
- "晋安区": {119.32, 26.08},
- "闽侯县": {119.13, 26.15},
- "连江县": {119.53, 26.2},
- "ç½—æºåŽ¿": {119.55, 26.48},
- "闽清县": {118.85, 26.22},
- "永泰县": {118.93, 25.87},
- "å¹³æ½åŽ¿": {119.78, 25.52},
- "ç¦æ¸…市": {119.38, 25.72},
- "é•¿ä¹å¸‚": {119.52, 25.97},
- "厦门市": {118.08, 24.48},
- "æ€æ˜ŽåŒº": {118.08, 24.45},
- "海沧区": {117.98, 24.47},
- "湖里区": {118.08, 24.52},
- "集美区": {118.1, 24.57},
- "åŒå®‰åŒº": {118.15, 24.73},
- "翔安区": {118.23, 24.62},
- "莆田市": {119, 25.43},
- "城厢区": {119, 25.43},
- "涵江区": {119.1, 25.45},
- "è”城区": {119.02, 25.43},
- "秀屿区": {119.08, 25.32},
- "仙游县": {118.68, 25.37},
- "三明市": {117.62, 26.27},
- "梅列区": {117.63, 26.27},
- "三元区": {117.6, 26.23},
- "明溪县": {117.2, 26.37},
- "清æµåŽ¿": {116.82, 26.18},
- "å®åŒ–县": {116.65, 26.27},
- "大田县": {117.85, 25.7},
- "尤溪县": {118.18, 26.17},
- "沙县": {117.78, 26.4},
- "å°†ä¹åŽ¿": {117.47, 26.73},
- "æ³°å®åŽ¿": {117.17, 26.9},
- "建å®åŽ¿": {116.83, 26.83},
- "永安市": {117.37, 25.98},
- "泉州市": {118.67, 24.88},
- "鲤城区": {118.6, 24.92},
- "丰泽区": {118.6, 24.92},
- "洛江区": {118.67, 24.95},
- "泉港区": {118.88, 25.12},
- "æƒ å®‰åŽ¿": {118.8, 25.03},
- "安溪县": {118.18, 25.07},
- "永春县": {118.3, 25.32},
- "德化县": {118.23, 25.5},
- "金门县": {118.32, 24.43},
- "石狮市": {118.65, 24.73},
- "晋江市": {118.58, 24.82},
- "å—安市": {118.38, 24.97},
- "漳州市": {117.65, 24.52},
- "芗城区": {117.65, 24.52},
- "龙文区": {117.72, 24.52},
- "云霄县": {117.33, 23.95},
- "漳浦县": {117.62, 24.13},
- "è¯å®‰åŽ¿": {117.18, 23.72},
- "长泰县": {117.75, 24.62},
- "东山县": {117.43, 23.7},
- "å—é–县": {117.37, 24.52},
- "平和县": {117.3, 24.37},
- "åŽå®‰åŽ¿": {117.53, 25.02},
- "龙海市": {117.82, 24.45},
- "å—平市": {118.17, 26.65},
- "延平区": {118.17, 26.65},
- "顺昌县": {117.8, 26.8},
- "浦城县": {118.53, 27.92},
- "光泽县": {117.33, 27.55},
- "æ¾æºªåŽ¿": {118.78, 27.53},
- "政和县": {118.85, 27.37},
- "邵æ¦å¸‚": {117.48, 27.37},
- "æ¦å¤·å±±å¸‚": {118.03, 27.77},
- "建瓯市": {118.32, 27.03},
- "建阳市": {118.12, 27.33},
- "龙岩市": {117.03, 25.1},
- "新罗区": {117.03, 25.1},
- "长汀县": {116.35, 25.83},
- "永定县": {116.73, 24.72},
- "上æåŽ¿": {116.42, 25.05},
- "æ¦å¹³åŽ¿": {116.1, 25.1},
- "连城县": {116.75, 25.72},
- "漳平市": {117.42, 25.3},
- "å®å¾·å¸‚": {119.52, 26.67},
- "蕉城区": {119.52, 26.67},
- "霞浦县": {120, 26.88},
- "å¤ç”°åŽ¿": {118.75, 26.58},
- "å±å—县": {118.98, 26.92},
- "寿å®åŽ¿": {119.5, 27.47},
- "周å®åŽ¿": {119.33, 27.12},
- "柘è£åŽ¿": {119.9, 27.23},
- "ç¦å®‰å¸‚": {119.65, 27.08},
- "ç¦é¼Žå¸‚": {120.22, 27.33},
- "å—æ˜Œå¸‚": {115.85, 28.68},
- "东湖区": {115.9, 28.68},
- "西湖区": {115.87, 28.67},
- "é’云谱区": {115.92, 28.63},
- "湾里区": {115.73, 28.72},
- "é’山湖区": {115.95, 28.68},
- "å—æ˜ŒåŽ¿": {115.93, 28.55},
- "新建县": {115.82, 28.7},
- "安义县": {115.55, 28.85},
- "进贤县": {116.27, 28.37},
- "景德镇市": {117.17, 29.27},
- "昌江区": {117.17, 29.27},
- "ç 山区": {117.2, 29.3},
- "æµ®æ¢åŽ¿": {117.25, 29.37},
- "ä¹å¹³å¸‚": {117.12, 28.97},
- "è乡市": {113.85, 27.63},
- "安æºåŒº": {113.87, 27.65},
- "湘东区": {113.73, 27.65},
- "莲花县": {113.95, 27.13},
- "ä¸Šæ —åŽ¿": {113.8, 27.88},
- "芦溪县": {114.03, 27.63},
- "乿±Ÿå¸‚": {116, 29.7},
- "åºå±±åŒº": {115.98, 29.68},
- "浔阳区": {115.98, 29.73},
- "乿±ŸåŽ¿": {115.88, 29.62},
- "æ¦å®åŽ¿": {115.1, 29.27},
- "修水县": {114.57, 29.03},
- "永修县": {115.8, 29.03},
- "德安县": {115.77, 29.33},
- "星å县": {116.03, 29.45},
- "都昌县": {116.18, 29.27},
- "æ¹–å£åŽ¿": {116.22, 29.73},
- "彿³½åŽ¿": {116.55, 29.9},
- "瑞昌市": {115.67, 29.68},
- "新余市": {114.92, 27.82},
- "æ¸æ°´åŒº": {114.93, 27.8},
- "分宜县": {114.67, 27.82},
- "é¹°æ½å¸‚": {117.07, 28.27},
- "月湖区": {117.05, 28.23},
- "余江县": {116.82, 28.2},
- "贵溪市": {117.22, 28.28},
- "赣州市": {114.93, 25.83},
- "ç« è´¡åŒº": {114.93, 25.87},
- "赣县": {115, 25.87},
- "信丰县": {114.93, 25.38},
- "大余县": {114.35, 25.4},
- "上犹县": {114.53, 25.8},
- "崇义县": {114.3, 25.7},
- "安远县": {115.38, 25.13},
- "é¾™å—县": {114.78, 24.92},
- "定å—县": {115.03, 24.78},
- "å…¨å—县": {114.52, 24.75},
- "å®éƒ½åŽ¿": {116.02, 26.48},
- "于都县": {115.42, 25.95},
- "兴国县": {115.35, 26.33},
- "会昌县": {115.78, 25.6},
- "寻乌县": {115.65, 24.95},
- "石城县": {116.33, 26.33},
- "瑞金市": {116.03, 25.88},
- "å—康市": {114.75, 25.65},
- "å‰å®‰å¸‚": {114.98, 27.12},
- "å‰å·žåŒº": {114.98, 27.12},
- "é’原区": {115, 27.1},
- "å‰å®‰åŽ¿": {114.9, 27.05},
- "剿°´åŽ¿": {115.13, 27.22},
- "峡江县": {115.33, 27.62},
- "新干县": {115.4, 27.77},
- "永丰县": {115.43, 27.32},
- "泰和县": {114.88, 26.8},
- "é‚å·åŽ¿": {114.52, 26.33},
- "万安县": {114.78, 26.47},
- "安ç¦åŽ¿": {114.62, 27.38},
- "永新县": {114.23, 26.95},
- "井冈山市": {114.27, 26.72},
- "宜春市": {114.38, 27.8},
- "è¢å·žåŒº": {114.38, 27.8},
- "奉新县": {115.38, 28.7},
- "万载县": {114.43, 28.12},
- "上高县": {114.92, 28.23},
- "宜丰县": {114.78, 28.38},
- "é–安县": {115.35, 28.87},
- "铜鼓县": {114.37, 28.53},
- "丰城市": {115.78, 28.2},
- "æ¨Ÿæ ‘å¸‚": {115.53, 28.07},
- "高安市": {115.37, 28.42},
- "抚州市": {116.35, 28},
- "临å·åŒº": {116.35, 27.98},
- "å—城县": {116.63, 27.55},
- "黎å·åŽ¿": {116.92, 27.3},
- "å—丰县": {116.53, 27.22},
- "å´‡ä»åŽ¿": {116.05, 27.77},
- "ä¹å®‰åŽ¿": {115.83, 27.43},
- "宜黄县": {116.22, 27.55},
- "金溪县": {116.77, 27.92},
- "资溪县": {117.07, 27.7},
- "东乡县": {116.62, 28.23},
- "广昌县": {116.32, 26.83},
- "上饶市": {117.97, 28.45},
- "信州区": {117.95, 28.43},
- "上饶县": {117.92, 28.43},
- "广丰县": {118.18, 28.43},
- "玉山县": {118.25, 28.68},
- "铅山县": {117.7, 28.32},
- "横峰县": {117.6, 28.42},
- "弋阳县": {117.43, 28.4},
- "余干县": {116.68, 28.7},
- "鄱阳县": {116.67, 29},
- "万年县": {117.07, 28.7},
- "婺æºåŽ¿": {117.85, 29.25},
- "德兴市": {117.57, 28.95},
- "济å—市": {116.98, 36.67},
- "历下区": {117.08, 36.67},
- "市ä¸åŒº": {117.57, 34.87},
- "æ§è«åŒº": {116.93, 36.65},
- "天桥区": {116.98, 36.68},
- "历城区": {117.07, 36.68},
- "长清区": {116.73, 36.55},
- "平阴县": {116.45, 36.28},
- "济阳县": {117.22, 36.98},
- "商河县": {117.15, 37.32},
- "ç« ä¸˜å¸‚": {117.53, 36.72},
- "é’岛市": {120.38, 36.07},
- "市å—区": {120.38, 36.07},
- "市北区": {120.38, 36.08},
- "四方区": {120.35, 36.1},
- "黄岛区": {120.18, 35.97},
- "崂山区": {120.47, 36.1},
- "æŽæ²§åŒº": {120.43, 36.15},
- "城阳区": {120.37, 36.3},
- "胶州市": {120.03, 36.27},
- "å³å¢¨å¸‚": {120.45, 36.38},
- "平度市": {119.95, 36.78},
- "胶å—市": {120.03, 35.87},
- "莱西市": {120.5, 36.87},
- "æ·„åšå¸‚": {118.05, 36.82},
- "å¼ åº—åŒº": {118.03, 36.82},
- "åšå±±åŒº": {117.85, 36.5},
- "临淄区": {118.3, 36.82},
- "周æ‘区": {117.87, 36.8},
- "æ¡“å°åŽ¿": {118.08, 36.97},
- "高é’县": {117.82, 37.17},
- "沂æºåŽ¿": {118.17, 36.18},
- "枣庄市": {117.32, 34.82},
- "薛城区": {117.25, 34.8},
- "峄城区": {117.58, 34.77},
- "å°å„¿åº„区": {117.73, 34.57},
- "å±±äºåŒº": {117.45, 35.08},
- "滕州市": {117.15, 35.08},
- "东è¥å¸‚": {118.67, 37.43},
- "东è¥åŒº": {118.5, 37.47},
- "æ²³å£åŒº": {118.53, 37.88},
- "垦利县": {118.55, 37.58},
- "利津县": {118.25, 37.48},
- "广饶县": {118.4, 37.07},
- "烟å°å¸‚": {121.43, 37.45},
- "èŠç½˜åŒº": {121.38, 37.53},
- "ç¦å±±åŒº": {121.25, 37.5},
- "牟平区": {121.6, 37.38},
- "莱山区": {121.43, 37.5},
- "长岛县": {120.73, 37.92},
- "é¾™å£å¸‚": {120.52, 37.65},
- "莱阳市": {120.7, 36.98},
- "莱州市": {119.93, 37.18},
- "蓬莱市": {120.75, 37.82},
- "招远市": {120.4, 37.37},
- "æ –éœžå¸‚": {120.83, 37.3},
- "海阳市": {121.15, 36.78},
- "æ½åŠå¸‚": {119.15, 36.7},
- "æ½åŸŽåŒº": {119.1, 36.72},
- "寒äºåŒº": {119.22, 36.77},
- "åŠå区": {119.17, 36.67},
- "奎文区": {119.12, 36.72},
- "临æœåŽ¿": {118.53, 36.52},
- "昌ä¹åŽ¿": {118.82, 36.7},
- "é’州市": {118.47, 36.68},
- "诸城市": {119.4, 36},
- "寿光市": {118.73, 36.88},
- "安丘市": {119.2, 36.43},
- "高密市": {119.75, 36.38},
- "昌邑市": {119.4, 36.87},
- "济å®å¸‚": {116.58, 35.42},
- "任城区": {116.58, 35.42},
- "微山县": {117.13, 34.82},
- "é±¼å°åŽ¿": {116.65, 35},
- "金乡县": {116.3, 35.07},
- "嘉祥县": {116.33, 35.42},
- "汶上县": {116.48, 35.73},
- "泗水县": {117.27, 35.67},
- "æ¢å±±åŽ¿": {116.08, 35.8},
- "曲阜市": {116.98, 35.58},
- "兖州市": {116.83, 35.55},
- "邹城市": {116.97, 35.4},
- "泰安市": {117.08, 36.2},
- "泰山区": {117.13, 36.18},
- "岱岳区": {117, 36.18},
- "å®é˜³åŽ¿": {116.8, 35.77},
- "东平县": {116.47, 35.93},
- "新泰市": {117.77, 35.92},
- "肥城市": {116.77, 36.18},
- "卿µ·å¸‚": {122.12, 37.52},
- "çŽ¯ç¿ åŒº": {122.12, 37.5},
- "文登市": {122.05, 37.2},
- "è£æˆå¸‚": {122.42, 37.17},
- "乳山市": {121.53, 36.92},
- "日照市": {119.52, 35.42},
- "东港区": {119.45, 35.42},
- "岚山区": {119.33, 35.1},
- "五莲县": {119.2, 35.75},
- "莒县": {118.83, 35.58},
- "莱芜市": {117.67, 36.22},
- "莱城区": {117.65, 36.2},
- "钢城区": {117.8, 36.07},
- "临沂市": {118.35, 35.05},
- "兰山区": {118.33, 35.07},
- "罗庄区": {118.28, 34.98},
- "河东区": {118.4, 35.08},
- "沂å—县": {118.47, 35.55},
- "郯城县": {118.35, 34.62},
- "沂水县": {118.62, 35.78},
- "è‹å±±åŽ¿": {118.05, 34.85},
- "费县": {117.97, 35.27},
- "平邑县": {117.63, 35.5},
- "莒å—县": {118.83, 35.18},
- "蒙阴县": {117.93, 35.72},
- "临æ²åŽ¿": {118.65, 34.92},
- "德州市": {116.3, 37.45},
- "德城区": {116.3, 37.45},
- "陵县": {116.57, 37.33},
- "宿´¥åŽ¿": {116.78, 37.65},
- "庆云县": {117.38, 37.78},
- "临邑县": {116.87, 37.18},
- "齿²³åŽ¿": {116.75, 36.8},
- "平原县": {116.43, 37.17},
- "夿´¥åŽ¿": {116, 36.95},
- "æ¦åŸŽåŽ¿": {116.07, 37.22},
- "ä¹é™µå¸‚": {117.23, 37.73},
- "禹城市": {116.63, 36.93},
- "èŠåŸŽå¸‚": {115.98, 36.45},
- "东昌府区": {115.98, 36.45},
- "阳谷县": {115.78, 36.12},
- "莘县": {115.67, 36.23},
- "茌平县": {116.25, 36.58},
- "东阿县": {116.25, 36.33},
- "å† åŽ¿": {115.43, 36.48},
- "高å”县": {116.23, 36.87},
- "临清市": {115.7, 36.85},
- "滨州市": {117.97, 37.38},
- "滨城区": {118, 37.38},
- "æƒ æ°‘åŽ¿": {117.5, 37.48},
- "阳信县": {117.58, 37.63},
- "æ— æ££åŽ¿": {117.6, 37.73},
- "沾化县": {118.13, 37.7},
- "åšå…´åŽ¿": {118.13, 37.15},
- "邹平县": {117.73, 36.88},
- "牡丹区": {115.43, 35.25},
- "曹县": {115.53, 34.83},
- "å•县": {116.08, 34.8},
- "æˆæ¦åŽ¿": {115.88, 34.95},
- "巨野县": {116.08, 35.4},
- "郓城县": {115.93, 35.6},
- "鄄城县": {115.5, 35.57},
- "定陶县": {115.57, 35.07},
- "东明县": {115.08, 35.28},
- "郑州市": {113.62, 34.75},
- "ä¸åŽŸåŒº": {113.6, 34.75},
- "二七区": {113.65, 34.73},
- "管城回æ—区": {113.67, 34.75},
- "金水区": {113.65, 34.78},
- "上街区": {113.28, 34.82},
- "æƒ æµŽåŒº": {113.6, 34.87},
- "ä¸ç‰ŸåŽ¿": {113.97, 34.72},
- "巩义市": {112.98, 34.77},
- "è¥é˜³å¸‚": {113.4, 34.78},
- "新密市": {113.38, 34.53},
- "新郑市": {113.73, 34.4},
- "ç™»å°å¸‚": {113.03, 34.47},
- "å¼€å°å¸‚": {114.3, 34.8},
- "é¾™äºåŒº": {114.35, 34.8},
- "顺河回æ—区": {114.35, 34.8},
- "æžåŽ¿": {114.78, 34.55},
- "通许县": {114.47, 34.48},
- "å°‰æ°åŽ¿": {114.18, 34.42},
- "å¼€å°åŽ¿": {114.43, 34.77},
- "兰考县": {114.82, 34.82},
- "洛阳市": {112.45, 34.62},
- "è€åŸŽåŒº": {112.47, 34.68},
- "西工区": {112.43, 34.67},
- "涧西区": {112.4, 34.67},
- "å‰åˆ©åŒº": {112.58, 34.9},
- "洛龙区": {112.45, 34.62},
- "åŸæ´¥åŽ¿": {112.43, 34.83},
- "新安县": {112.15, 34.72},
- "æ ¾å·åŽ¿": {111.62, 33.78},
- "嵩县": {112.1, 34.15},
- "æ±é˜³åŽ¿": {112.47, 34.15},
- "宜阳县": {112.17, 34.52},
- "æ´›å®åŽ¿": {111.65, 34.38},
- "伊å·åŽ¿": {112.42, 34.42},
- "åƒå¸ˆå¸‚": {112.78, 34.73},
- "平顶山市": {113.18, 33.77},
- "æ–°åŽåŒº": {113.3, 33.73},
- "å«ä¸œåŒº": {113.33, 33.73},
- "石龙区": {112.88, 33.9},
- "湛河区": {113.28, 33.73},
- "å®ä¸°åŽ¿": {113.07, 33.88},
- "å¶åŽ¿": {113.35, 33.62},
- "é²å±±åŽ¿": {112.9, 33.73},
- "éƒåŽ¿": {113.22, 33.97},
- "舞钢市": {113.52, 33.3},
- "æ±å·žå¸‚": {112.83, 34.17},
- "安阳市": {114.38, 36.1},
- "文峰区": {114.35, 36.08},
- "北关区": {114.35, 36.12},
- "殷都区": {114.3, 36.12},
- "龙安区": {114.32, 36.1},
- "安阳县": {114.35, 36.1},
- "汤阴县": {114.35, 35.92},
- "滑县": {114.52, 35.58},
- "内黄县": {114.9, 35.95},
- "林州市": {113.82, 36.07},
- "鹤å£å¸‚": {114.28, 35.75},
- "鹤山区": {114.15, 35.95},
- "山城区": {114.18, 35.9},
- "淇滨区": {114.3, 35.73},
- "浚县": {114.55, 35.67},
- "淇县": {114.2, 35.6},
- "新乡市": {113.9, 35.3},
- "红旗区": {113.87, 35.3},
- "嫿»¨åŒº": {113.85, 35.3},
- "凤泉区": {113.92, 35.38},
- "牧野区": {113.9, 35.32},
- "新乡县": {113.8, 35.2},
- "获嘉县": {113.65, 35.27},
- "原阳县": {113.97, 35.05},
- "延津县": {114.2, 35.15},
- "å°ä¸˜åŽ¿": {114.42, 35.05},
- "长垣县": {114.68, 35.2},
- "å«è¾‰å¸‚": {114.07, 35.4},
- "辉县市": {113.8, 35.47},
- "焦作市": {113.25, 35.22},
- "解放区": {113.22, 35.25},
- "ä¸ç«™åŒº": {113.17, 35.23},
- "马æ‘区": {113.32, 35.27},
- "山阳区": {113.25, 35.22},
- "ä¿®æ¦åŽ¿": {113.43, 35.23},
- "åšçˆ±åŽ¿": {113.07, 35.17},
- "æ¦é™ŸåŽ¿": {113.38, 35.1},
- "温县": {113.08, 34.93},
- "济æºå¸‚": {112.58, 35.07},
- "æ²é˜³å¸‚": {112.93, 35.08},
- "åŸå·žå¸‚": {112.78, 34.9},
- "濮阳市": {115.03, 35.77},
- "åŽé¾™åŒº": {115.07, 35.78},
- "清丰县": {115.12, 35.9},
- "å—ä¹åŽ¿": {115.2, 36.08},
- "范县": {115.5, 35.87},
- "å°å‰åŽ¿": {115.85, 36},
- "濮阳县": {115.02, 35.7},
- "许昌市": {113.85, 34.03},
- "é都区": {113.82, 34.03},
- "许昌县": {113.83, 34},
- "鄢陵县": {114.2, 34.1},
- "襄城县": {113.48, 33.85},
- "禹州市": {113.47, 34.17},
- "长葛市": {113.77, 34.22},
- "漯河市": {114.02, 33.58},
- "郾城区": {114, 33.58},
- "å¬é™µåŒº": {114.07, 33.57},
- "舞阳县": {113.6, 33.43},
- "临é¢åŽ¿": {113.93, 33.82},
- "三门峡市": {111.2, 34.78},
- "湖滨区": {111.2, 34.78},
- "æ¸‘æ± åŽ¿": {111.75, 34.77},
- "陕县": {111.08, 34.7},
- "墿°åŽ¿": {111.05, 34.05},
- "义马市": {111.87, 34.75},
- "çµå®å¸‚": {110.87, 34.52},
- "å—阳市": {112.52, 33},
- "宛城区": {112.55, 33.02},
- "å§é¾™åŒº": {112.53, 32.98},
- "å—å¬åŽ¿": {112.43, 33.5},
- "方城县": {113, 33.27},
- "西峡县": {111.48, 33.28},
- "镇平县": {112.23, 33.03},
- "内乡县": {111.85, 33.05},
- "æ·…å·åŽ¿": {111.48, 33.13},
- "社旗县": {112.93, 33.05},
- "唿²³åŽ¿": {112.83, 32.7},
- "新野县": {112.35, 32.52},
- "æ¡æŸåŽ¿": {113.4, 32.37},
- "邓州市": {112.08, 32.68},
- "商丘市": {115.65, 34.45},
- "æ¢å›åŒº": {115.63, 34.45},
- "ç¢é˜³åŒº": {115.63, 34.38},
- "æ°‘æƒåŽ¿": {115.13, 34.65},
- "ç¢åŽ¿": {115.07, 34.45},
- "å®é™µåŽ¿": {115.32, 34.45},
- "柘城县": {115.3, 34.07},
- "虞城县": {115.85, 34.4},
- "å¤é‚‘县": {116.13, 34.23},
- "永城市": {116.43, 33.92},
- "信阳市": {114.07, 32.13},
- "浉河区": {114.05, 32.12},
- "平桥区": {114.12, 32.1},
- "罗山县": {114.53, 32.2},
- "光山县": {114.9, 32.02},
- "新县": {114.87, 31.63},
- "商城县": {115.4, 31.8},
- "固始县": {115.68, 32.18},
- "æ½¢å·åŽ¿": {115.03, 32.13},
- "淮滨县": {115.4, 32.43},
- "æ¯åŽ¿": {114.73, 32.35},
- "周å£å¸‚": {114.65, 33.62},
- "扶沟县": {114.38, 34.07},
- "西åŽåŽ¿": {114.53, 33.8},
- "商水县": {114.6, 33.53},
- "沈丘县": {115.07, 33.4},
- "郸城县": {115.2, 33.65},
- "淮阳县": {114.88, 33.73},
- "太康县": {114.85, 34.07},
- "鹿邑县": {115.48, 33.87},
- "项城市": {114.9, 33.45},
- "驻马店市": {114.02, 32.98},
- "驿城区": {114.05, 32.97},
- "西平县": {114.02, 33.38},
- "上蔡县": {114.27, 33.27},
- "平舆县": {114.63, 32.97},
- "æ£é˜³åŽ¿": {114.38, 32.6},
- "确山县": {114.02, 32.8},
- "泌阳县": {113.32, 32.72},
- "æ±å—县": {114.35, 33},
- "é‚平县": {114, 33.15},
- "新蔡县": {114.98, 32.75},
- "æ¦æ±‰å¸‚": {114.3, 30.6},
- "江岸区": {114.3, 30.6},
- "江汉区": {114.27, 30.6},
- "硚å£åŒº": {114.27, 30.57},
- "汉阳区": {114.27, 30.55},
- "æ¦æ˜ŒåŒº": {114.3, 30.57},
- "é’山区": {114.38, 30.63},
- "洪山区": {114.33, 30.5},
- "东西湖区": {114.13, 30.62},
- "汉å—区": {114.08, 30.32},
- "蔡甸区": {114.03, 30.58},
- "江å¤åŒº": {114.32, 30.35},
- "黄陂区": {114.37, 30.87},
- "新洲区": {114.8, 30.85},
- "黄石市": {115.03, 30.2},
- "黄石港区": {115.07, 30.23},
- "西塞山区": {115.12, 30.2},
- "下陆区": {114.97, 30.18},
- "é“山区": {114.9, 30.2},
- "阳新县": {115.2, 29.85},
- "大冶市": {114.97, 30.1},
- "åå °å¸‚": {110.78, 32.65},
- "茅ç®åŒº": {110.82, 32.6},
- "å¼ æ¹¾åŒº": {110.78, 32.65},
- "郧县": {110.82, 32.83},
- "郧西县": {110.42, 33},
- "竹山县": {110.23, 32.23},
- "竹溪县": {109.72, 32.32},
- "房县": {110.73, 32.07},
- "丹江å£å¸‚": {111.52, 32.55},
- "宜昌市": {111.28, 30.7},
- "西陵区": {111.27, 30.7},
- "ä¼å®¶å²—区": {111.35, 30.65},
- "点军区": {111.27, 30.7},
- "猇äºåŒº": {111.42, 30.53},
- "夷陵区": {111.32, 30.77},
- "远安县": {111.63, 31.07},
- "兴山县": {110.75, 31.35},
- "ç§å½’县": {110.98, 30.83},
- "长阳土家æ—自治县": {111.18, 30.47},
- "五峰土家æ—自治县": {110.67, 30.2},
- "宜都市": {111.45, 30.4},
- "当阳市": {111.78, 30.82},
- "æžæ±Ÿå¸‚": {111.77, 30.43},
- "襄樊市": {112.15, 32.02},
- "襄城区": {112.15, 32.02},
- "樊城区": {112.13, 32.03},
- "襄阳区": {112.2, 32.08},
- "å—æ¼³åŽ¿": {111.83, 31.78},
- "谷城县": {111.65, 32.27},
- "ä¿åº·åŽ¿": {111.25, 31.88},
- "è€æ²³å£å¸‚": {111.67, 32.38},
- "枣阳市": {112.75, 32.13},
- "宜城市": {112.25, 31.72},
- "鄂州市": {114.88, 30.4},
- "æ¢å湖区": {114.67, 30.08},
- "åŽå®¹åŒº": {114.73, 30.53},
- "鄂城区": {114.88, 30.4},
- "è†é—¨å¸‚": {112.2, 31.03},
- "东å®åŒº": {112.2, 31.05},
- "掇刀区": {112.2, 30.98},
- "京山县": {113.1, 31.02},
- "沙洋县": {112.58, 30.7},
- "钟祥市": {112.58, 31.17},
- "åæ„Ÿå¸‚": {113.92, 30.93},
- "åå—区": {113.92, 30.92},
- "åæ˜ŒåŽ¿": {113.97, 31.25},
- "大悟县": {114.12, 31.57},
- "云梦县": {113.75, 31.02},
- "应城市": {113.57, 30.95},
- "安陆市": {113.68, 31.27},
- "汉å·å¸‚": {113.83, 30.65},
- "è†å·žå¸‚": {112.23, 30.33},
- "沙市区": {112.25, 30.32},
- "è†å·žåŒº": {112.18, 30.35},
- "公安县": {112.23, 30.07},
- "监利县": {112.88, 29.82},
- "江陵县": {112.42, 30.03},
- "石首市": {112.4, 29.73},
- "洪湖市": {113.45, 29.8},
- "æ¾æ»‹å¸‚": {111.77, 30.18},
- "黄冈市": {114.87, 30.45},
- "黄州区": {114.88, 30.43},
- "团风县": {114.87, 30.63},
- "红安县": {114.62, 31.28},
- "罗田县": {115.4, 30.78},
- "英山县": {115.67, 30.75},
- "æµ æ°´åŽ¿": {115.27, 30.45},
- "蕲春县": {115.43, 30.23},
- "黄梅县": {115.93, 30.08},
- "麻城市": {115.03, 31.18},
- "æ¦ç©´å¸‚": {115.55, 29.85},
- "å’¸å®å¸‚": {114.32, 29.85},
- "咸安区": {114.3, 29.87},
- "嘉鱼县": {113.9, 29.98},
- "通城县": {113.82, 29.25},
- "崇阳县": {114.03, 29.55},
- "通山县": {114.52, 29.6},
- "赤å£å¸‚": {113.88, 29.72},
- "éšå·žå¸‚": {113.37, 31.72},
- "曾都区": {113.37, 31.72},
- "广水市": {113.82, 31.62},
- "æ©æ–½åœŸå®¶æ—è‹—æ—自治州": {109.47, 30.3},
- "æ©æ–½å¸‚": {109.47, 30.3},
- "利å·å¸‚": {108.93, 30.3},
- "建始县": {109.73, 30.6},
- "巴东县": {110.33, 31.05},
- "宣æ©åŽ¿": {109.48, 29.98},
- "咸丰县": {109.15, 29.68},
- "æ¥å‡¤åŽ¿": {109.4, 29.52},
- "鹤峰县": {110.03, 29.9},
- "仙桃市": {113.45, 30.37},
- "潜江市": {112.88, 30.42},
- "天门市": {113.17, 30.67},
- "神农架林区": {110.67, 31.75},
- "长沙市": {112.93, 28.23},
- "芙蓉区": {113.03, 28.18},
- "天心区": {112.98, 28.12},
- "岳麓区": {112.93, 28.23},
- "å¼€ç¦åŒº": {112.98, 28.25},
- "雨花区": {113.03, 28.13},
- "长沙县": {113.07, 28.25},
- "望城县": {112.82, 28.37},
- "å®ä¹¡åŽ¿": {112.55, 28.25},
- "æµé˜³å¸‚": {113.63, 28.15},
- "æ ªæ´²å¸‚": {113.13, 27.83},
- "è·å¡˜åŒº": {113.17, 27.87},
- "芦淞区": {113.15, 27.83},
- "石峰区": {113.1, 27.87},
- "天元区": {113.12, 27.83},
- "æ ªæ´²åŽ¿": {113.13, 27.72},
- "攸县": {113.33, 27},
- "茶陵县": {113.53, 26.8},
- "炎陵县": {113.77, 26.48},
- "醴陵市": {113.48, 27.67},
- "湘æ½å¸‚": {112.93, 27.83},
- "雨湖区": {112.9, 27.87},
- "岳塘区": {112.95, 27.87},
- "湘æ½åŽ¿": {112.95, 27.78},
- "湘乡市": {112.53, 27.73},
- "韶山市": {112.52, 27.93},
- "衡阳市": {112.57, 26.9},
- "ç æ™–区": {112.62, 26.9},
- "é›å³°åŒº": {112.6, 26.88},
- "石鼓区": {112.6, 26.9},
- "蒸湘区": {112.6, 26.9},
- "å—岳区": {112.73, 27.25},
- "衡阳县": {112.37, 26.97},
- "è¡¡å—县": {112.67, 26.73},
- "衡山县": {112.87, 27.23},
- "衡东县": {112.95, 27.08},
- "ç¥ä¸œåŽ¿": {112.12, 26.78},
- "耒阳市": {112.85, 26.42},
- "常å®å¸‚": {112.38, 26.42},
- "邵阳市": {111.47, 27.25},
- "åŒæ¸…区": {111.47, 27.23},
- "大祥区": {111.45, 27.23},
- "北塔区": {111.45, 27.25},
- "邵东县": {111.75, 27.25},
- "新邵县": {111.45, 27.32},
- "邵阳县": {111.27, 27},
- "隆回县": {111.03, 27.12},
- "æ´žå£åŽ¿": {110.57, 27.05},
- "绥å®åŽ¿": {110.15, 26.58},
- "æ–°å®åŽ¿": {110.85, 26.43},
- "城æ¥è‹—æ—自治县": {110.32, 26.37},
- "æ¦å†ˆå¸‚": {110.63, 26.73},
- "岳阳市": {113.12, 29.37},
- "岳阳楼区": {113.1, 29.37},
- "云溪区": {113.3, 29.47},
- "å›å±±åŒº": {113, 29.43},
- "岳阳县": {113.12, 29.15},
- "åŽå®¹åŽ¿": {112.57, 29.52},
- "湘阴县": {112.88, 28.68},
- "平江县": {113.58, 28.72},
- "汨罗市": {113.08, 28.8},
- "临湘市": {113.47, 29.48},
- "常德市": {111.68, 29.05},
- "æ¦é™µåŒº": {111.68, 29.03},
- "鼎城区": {111.68, 29.02},
- "安乡县": {112.17, 29.42},
- "汉寿县": {111.97, 28.9},
- "澧县": {111.75, 29.63},
- "临澧县": {111.65, 29.45},
- "桃æºåŽ¿": {111.48, 28.9},
- "石门县": {111.38, 29.58},
- "津市市": {111.88, 29.62},
- "å¼ å®¶ç•Œå¸‚": {110.47, 29.13},
- "永定区": {110.48, 29.13},
- "æ¦é™µæºåŒº": {110.53, 29.35},
- "慈利县": {111.12, 29.42},
- "æ¡‘æ¤åŽ¿": {110.15, 29.4},
- "益阳市": {112.32, 28.6},
- "资阳区": {112.32, 28.6},
- "赫山区": {112.37, 28.6},
- "å—县": {112.4, 29.38},
- "桃江县": {112.12, 28.53},
- "安化县": {111.22, 28.38},
- "沅江市": {112.38, 28.85},
- "郴州市": {113.02, 25.78},
- "北湖区": {113.02, 25.8},
- "è‹ä»™åŒº": {113.03, 25.8},
- "桂阳县": {112.73, 25.73},
- "å®œç« åŽ¿": {112.95, 25.4},
- "永兴县": {113.1, 26.13},
- "嘉禾县": {112.37, 25.58},
- "临æ¦åŽ¿": {112.55, 25.28},
- "æ±åŸŽåŽ¿": {113.68, 25.55},
- "桂东县": {113.93, 26.08},
- "安ä»åŽ¿": {113.27, 26.7},
- "资兴市": {113.23, 25.98},
- "永州市": {111.62, 26.43},
- "冷水滩区": {111.6, 26.43},
- "ç¥é˜³åŽ¿": {111.85, 26.58},
- "东安县": {111.28, 26.4},
- "åŒç‰ŒåŽ¿": {111.65, 25.97},
- "é“县": {111.58, 25.53},
- "江永县": {111.33, 25.28},
- "å®è¿œåŽ¿": {111.93, 25.6},
- "è“山县": {112.18, 25.37},
- "新田县": {112.22, 25.92},
- "江åŽç‘¶æ—自治县": {111.58, 25.18},
- "怀化市": {110, 27.57},
- "鹤城区": {109.95, 27.55},
- "䏿–¹åŽ¿": {109.93, 27.4},
- "沅陵县": {110.38, 28.47},
- "辰溪县": {110.18, 28},
- "溆浦县": {110.58, 27.92},
- "会åŒåŽ¿": {109.72, 26.87},
- "麻阳苗æ—自治县": {109.8, 27.87},
- "新晃侗æ—自治县": {109.17, 27.37},
- "芷江侗æ—自治县": {109.68, 27.45},
- "é–å·žè‹—æ—ä¾—æ—自治县": {109.68, 26.58},
- "通é“ä¾—æ—自治县": {109.78, 26.17},
- "洪江市": {109.82, 27.2},
- "娄底市": {112, 27.73},
- "娄星区": {112, 27.73},
- "åŒå³°åŽ¿": {112.2, 27.45},
- "新化县": {111.3, 27.75},
- "冷水江市": {111.43, 27.68},
- "æ¶Ÿæºå¸‚": {111.67, 27.7},
- "湘西土家æ—è‹—æ—自治州": {109.73, 28.32},
- "å‰é¦–市": {109.73, 28.32},
- "泸溪县": {110.22, 28.22},
- "凤凰县": {109.6, 27.95},
- "花垣县": {109.48, 28.58},
- "ä¿é–县": {109.65, 28.72},
- "å¤ä¸ˆåŽ¿": {109.95, 28.62},
- "永顺县": {109.85, 29},
- "龙山县": {109.43, 29.47},
- "广州市": {113.27, 23.13},
- "è”æ¹¾åŒº": {113.23, 23.13},
- "越秀区": {113.27, 23.13},
- "æµ·ç 区": {113.25, 23.1},
- "天河区": {113.35, 23.12},
- "黄埔区": {113.45, 23.1},
- "番禺区": {113.35, 22.95},
- "花都区": {113.22, 23.4},
- "增城市": {113.83, 23.3},
- "从化市": {113.58, 23.55},
- "韶关市": {113.6, 24.82},
- "æ¦æ±ŸåŒº": {113.57, 24.8},
- "浈江区": {113.6, 24.8},
- "曲江区": {113.6, 24.68},
- "始兴县": {114.07, 24.95},
- "ä»åŒ–县": {113.75, 25.08},
- "ç¿æºåŽ¿": {114.13, 24.35},
- "ä¹³æºç‘¶æ—自治县": {113.27, 24.78},
- "新丰县": {114.2, 24.07},
- "乿˜Œå¸‚": {113.35, 25.13},
- "å—雄市": {114.3, 25.12},
- "深圳市": {114.05, 22.55},
- "罗湖区": {114.12, 22.55},
- "ç¦ç”°åŒº": {114.05, 22.53},
- "å—山区": {113.92, 22.52},
- "å®å®‰åŒº": {113.9, 22.57},
- "龙岗区": {114.27, 22.73},
- "ç›ç”°åŒº": {114.22, 22.55},
- "ç æµ·å¸‚": {113.57, 22.27},
- "香洲区": {113.55, 22.27},
- "斗门区": {113.28, 22.22},
- "金湾区": {113.4, 22.07},
- "汕头市": {116.68, 23.35},
- "龙湖区": {116.72, 23.37},
- "金平区": {116.7, 23.37},
- "潮阳区": {116.6, 23.27},
- "æ½®å—区": {116.43, 23.25},
- "澄海区": {116.77, 23.48},
- "å—æ¾³åŽ¿": {117.02, 23.42},
- "佛山市": {113.12, 23.02},
- "å—æµ·åŒº": {113.15, 23.03},
- "顺德区": {113.3, 22.8},
- "三水区": {112.87, 23.17},
- "高明区": {112.88, 22.9},
- "江门市": {113.08, 22.58},
- "新会区": {113.03, 22.47},
- "å°å±±å¸‚": {112.78, 22.25},
- "开平市": {112.67, 22.38},
- "鹤山市": {112.97, 22.77},
- "æ©å¹³å¸‚": {112.3, 22.18},
- "湛江市": {110.35, 21.27},
- "赤åŽåŒº": {110.37, 21.27},
- "霞山区": {110.4, 21.2},
- "å¡å¤´åŒº": {110.47, 21.23},
- "éº»ç« åŒº": {110.32, 21.27},
- "邿ºªåŽ¿": {110.25, 21.38},
- "å¾é—»åŽ¿": {110.17, 20.33},
- "廉江市": {110.27, 21.62},
- "雷州市": {110.08, 20.92},
- "å´å·å¸‚": {110.77, 21.43},
- "茂å市": {110.92, 21.67},
- "茂å—区": {110.92, 21.63},
- "茂港区": {111.02, 21.47},
- "电白县": {111, 21.5},
- "高州市": {110.85, 21.92},
- "化州市": {110.63, 21.67},
- "信宜市": {110.95, 22.35},
- "肇庆市": {112.47, 23.05},
- "端州区": {112.48, 23.05},
- "鼎湖区": {112.57, 23.17},
- "广å®åŽ¿": {112.43, 23.63},
- "怀集县": {112.18, 23.92},
- "å°å¼€åŽ¿": {111.5, 23.43},
- "德庆县": {111.77, 23.15},
- "高è¦å¸‚": {112.45, 23.03},
- "四会市": {112.68, 23.33},
- "æƒ å·žå¸‚": {114.42, 23.12},
- "æƒ åŸŽåŒº": {114.4, 23.08},
- "æƒ é˜³åŒº": {114.47, 22.8},
- "åšç½—县": {114.28, 23.18},
- "æƒ ä¸œåŽ¿": {114.72, 22.98},
- "龙门县": {114.25, 23.73},
- "梅州市": {116.12, 24.28},
- "梅江区": {116.12, 24.32},
- "梅县": {116.05, 24.28},
- "大埔县": {116.7, 24.35},
- "丰顺县": {116.18, 23.77},
- "五åŽåŽ¿": {115.77, 23.93},
- "平远县": {115.88, 24.57},
- "蕉å²åŽ¿": {116.17, 24.67},
- "å…´å®å¸‚": {115.73, 24.15},
- "汕尾市": {115.37, 22.78},
- "海丰县": {115.33, 22.97},
- "陆河县": {115.65, 23.3},
- "陆丰市": {115.65, 22.95},
- "æ²³æºå¸‚": {114.7, 23.73},
- "æºåŸŽåŒº": {114.7, 23.73},
- "紫金县": {115.18, 23.63},
- "é¾™å·åŽ¿": {115.25, 24.1},
- "连平县": {114.48, 24.37},
- "和平县": {114.93, 24.45},
- "东æºåŽ¿": {114.77, 23.82},
- "阳江市": {111.98, 21.87},
- "江城区": {111.95, 21.87},
- "阳西县": {111.62, 21.75},
- "阳东县": {112.02, 21.88},
- "阳春市": {111.78, 22.18},
- "清远市": {113.03, 23.7},
- "清城区": {113.02, 23.7},
- "佛冈县": {113.53, 23.88},
- "阳山县": {112.63, 24.48},
- "连山壮æ—ç‘¶æ—自治县": {112.08, 24.57},
- "连å—ç‘¶æ—自治县": {112.28, 24.72},
- "清新县": {112.98, 23.73},
- "英德市": {113.4, 24.18},
- "连州市": {112.38, 24.78},
- "东莞市": {113.75, 23.05},
- "ä¸å±±å¸‚": {113.38, 22.52},
- "潮州市": {116.62, 23.67},
- "湘桥区": {116.63, 23.68},
- "潮安县": {116.68, 23.45},
- "饶平县": {117, 23.67},
- "æé˜³å¸‚": {116.37, 23.55},
- "æä¸œåŽ¿": {116.42, 23.57},
- "æè¥¿åŽ¿": {115.83, 23.43},
- "æƒ æ¥åŽ¿": {116.28, 23.03},
- "æ™®å®å¸‚": {116.18, 23.3},
- "云浮市": {112.03, 22.92},
- "云城区": {112.03, 22.93},
- "新兴县": {112.23, 22.7},
- "éƒå—县": {111.53, 23.23},
- "云安县": {112, 23.08},
- "罗定市": {111.57, 22.77},
- "å—å®å¸‚": {108.37, 22.82},
- "å…´å®åŒº": {108.38, 22.87},
- "江å—区": {108.28, 22.78},
- "西乡塘区": {108.3, 22.83},
- "良庆区": {108.32, 22.77},
- "é‚•å®åŒº": {108.48, 22.75},
- "æ¦é¸£åŽ¿": {108.27, 23.17},
- "隆安县": {107.68, 23.18},
- "马山县": {108.17, 23.72},
- "上林县": {108.6, 23.43},
- "宾阳县": {108.8, 23.22},
- "横县": {109.27, 22.68},
- "柳州市": {109.42, 24.33},
- "柳å—区": {109.38, 24.35},
- "柳江县": {109.33, 24.27},
- "柳城县": {109.23, 24.65},
- "鹿寨县": {109.73, 24.48},
- "èžå®‰åŽ¿": {109.4, 25.23},
- "èžæ°´è‹—æ—自治县": {109.25, 25.07},
- "三江侗æ—自治县": {109.6, 25.78},
- "桂林市": {110.28, 25.28},
- "阳朔县": {110.48, 24.78},
- "临桂县": {110.2, 25.23},
- "çµå·åŽ¿": {110.32, 25.42},
- "全州县": {111.07, 25.93},
- "兴安县": {110.67, 25.62},
- "æ°¸ç¦åŽ¿": {109.98, 24.98},
- "çŒé˜³åŽ¿": {111.15, 25.48},
- "é¾™èƒœå„æ—自治县": {110, 25.8},
- "资æºåŽ¿": {110.63, 26.03},
- "å¹³ä¹åŽ¿": {110.63, 24.63},
- "æåŸŽç‘¶æ—自治县": {110.83, 24.83},
- "梧州市": {111.27, 23.48},
- "è‹æ¢§åŽ¿": {111.23, 23.42},
- "藤县": {110.92, 23.38},
- "蒙山县": {110.52, 24.2},
- "岑溪市": {110.98, 22.92},
- "北海市": {109.12, 21.48},
- "é“山港区": {109.43, 21.53},
- "åˆæµ¦åŽ¿": {109.2, 21.67},
- "防城港市": {108.35, 21.7},
- "港å£åŒº": {108.37, 21.65},
- "防城区": {108.35, 21.77},
- "上æ€åŽ¿": {107.98, 22.15},
- "东兴市": {107.97, 21.53},
- "钦州市": {108.62, 21.95},
- "钦北区": {108.63, 21.98},
- "çµå±±åŽ¿": {109.3, 22.43},
- "浦北县": {109.55, 22.27},
- "贵港市": {109.6, 23.1},
- "覃塘区": {109.42, 23.13},
- "å¹³å—县": {110.38, 23.55},
- "桂平市": {110.08, 23.4},
- "玉林市": {110.17, 22.63},
- "容县": {110.55, 22.87},
- "陆å·åŽ¿": {110.27, 22.33},
- "åšç™½åŽ¿": {109.97, 22.28},
- "兴业县": {109.87, 22.75},
- "北æµå¸‚": {110.35, 22.72},
- "百色市": {106.62, 23.9},
- "田阳县": {106.92, 23.73},
- "田东县": {107.12, 23.6},
- "平果县": {107.58, 23.32},
- "å¾·ä¿åŽ¿": {106.62, 23.33},
- "é–西县": {106.42, 23.13},
- "é‚£å¡åŽ¿": {105.83, 23.42},
- "凌云县": {106.57, 24.35},
- "ä¹ä¸šåŽ¿": {106.55, 24.78},
- "田林县": {106.23, 24.3},
- "西林县": {105.1, 24.5},
- "éš†æž—å„æ—自治县": {105.33, 24.77},
- "贺州市": {111.55, 24.42},
- "æ˜å¹³åŽ¿": {110.8, 24.17},
- "钟山县": {111.3, 24.53},
- "富å·ç‘¶æ—自治县": {111.27, 24.83},
- "æ²³æ± å¸‚": {108.07, 24.7},
- "金城江区": {108.05, 24.7},
- "å—丹县": {107.53, 24.98},
- "天峨县": {107.17, 25},
- "凤山县": {107.05, 24.55},
- "东兰县": {107.37, 24.52},
- "罗城仫佬æ—自治县": {108.9, 24.78},
- "çŽ¯æ±Ÿæ¯›å—æ—自治县": {108.25, 24.83},
- "巴马瑶æ—自治县": {107.25, 24.15},
- "都安瑶æ—自治县": {108.1, 23.93},
- "大化瑶æ—自治县": {107.98, 23.73},
- "宜州市": {108.67, 24.5},
- "æ¥å®¾å¸‚": {109.23, 23.73},
- "忻城县": {108.67, 24.07},
- "象州县": {109.68, 23.97},
- "æ¦å®£åŽ¿": {109.67, 23.6},
- "金秀瑶æ—自治县": {110.18, 24.13},
- "åˆå±±å¸‚": {108.87, 23.82},
- "崇左市": {107.37, 22.4},
- "扶绥县": {107.9, 22.63},
- "宿˜ŽåŽ¿": {107.07, 22.13},
- "龙州县": {106.85, 22.35},
- "大新县": {107.2, 22.83},
- "天ç‰åŽ¿": {107.13, 23.08},
- "å‡ç¥¥å¸‚": {106.75, 22.12},
- "æµ·å£å¸‚": {110.32, 20.03},
- "秀英区": {110.28, 20.02},
- "é¾™åŽåŒº": {110.3, 20.03},
- "ç¼å±±åŒº": {110.35, 20},
- "美兰区": {110.37, 20.03},
- "三亚市": {109.5, 18.25},
- "五指山市": {109.52, 18.78},
- "ç¼æµ·å¸‚": {110.47, 19.25},
- "儋州市": {109.57, 19.52},
- "文昌市": {110.8, 19.55},
- "万å®å¸‚": {110.4, 18.8},
- "东方市": {108.63, 19.1},
- "定安县": {110.32, 19.7},
- "屯昌县": {110.1, 19.37},
- "澄迈县": {110, 19.73},
- "临高县": {109.68, 19.92},
- "白沙黎æ—自治县": {109.45, 19.23},
- "昌江黎æ—自治县": {109.05, 19.25},
- "ä¹ä¸œé»Žæ—自治县": {109.17, 18.75},
- "陵水黎æ—自治县": {110.03, 18.5},
- "ä¿äºé»Žæ—è‹—æ—自治县": {109.7, 18.63},
- "ç¼ä¸é»Žæ—è‹—æ—自治县": {109.83, 19.03},
- "é‡åº†å¸‚": {106.55, 29.57},
- "万州区": {108.4, 30.82},
- "涪陵区": {107.4, 29.72},
- "æ¸ä¸åŒº": {106.57, 29.55},
- "大渡å£åŒº": {106.48, 29.48},
- "江北区": {106.57, 29.6},
- "æ²™åªå区": {106.45, 29.53},
- "ä¹é¾™å¡åŒº": {106.5, 29.5},
- "å—岸区": {106.57, 29.52},
- "北碚区": {106.4, 29.8},
- "万盛区": {106.92, 28.97},
- "åŒæ¡¥åŒº": {105.78, 29.48},
- "æ¸åŒ—区": {106.63, 29.72},
- "å·´å—区": {106.52, 29.38},
- "黔江区": {108.77, 29.53},
- "长寿区": {107.08, 29.87},
- "綦江县": {106.65, 29.03},
- "æ½¼å—县": {105.83, 30.18},
- "铜æ¢åŽ¿": {106.05, 29.85},
- "大足县": {105.72, 29.7},
- "è£æ˜ŒåŽ¿": {105.58, 29.4},
- "璧山县": {106.22, 29.6},
- "æ¢å¹³åŽ¿": {107.8, 30.68},
- "城å£åŽ¿": {108.67, 31.95},
- "丰都县": {107.73, 29.87},
- "垫江县": {107.35, 30.33},
- "æ¦éš†åŽ¿": {107.75, 29.33},
- "å¿ åŽ¿": {108.02, 30.3},
- "开县": {108.42, 31.18},
- "云阳县": {108.67, 30.95},
- "奉节县": {109.47, 31.02},
- "巫山县": {109.88, 31.08},
- "巫溪县": {109.63, 31.4},
- "石柱土家æ—自治县": {108.12, 30},
- "秀山土家æ—è‹—æ—自治县": {108.98, 28.45},
- "酉阳土家æ—è‹—æ—自治县": {108.77, 28.85},
- "彿°´è‹—æ—土家æ—自治县": {108.17, 29.3},
- "æˆéƒ½å¸‚": {104.07, 30.67},
- "锦江区": {104.08, 30.67},
- "é’羊区": {104.05, 30.68},
- "金牛区": {104.05, 30.7},
- "æ¦ä¾¯åŒº": {104.05, 30.65},
- "æˆåŽåŒº": {104.1, 30.67},
- "龙泉驿区": {104.27, 30.57},
- "é’白江区": {104.23, 30.88},
- "新都区": {104.15, 30.83},
- "温江区": {103.83, 30.7},
- "é‡‘å ‚åŽ¿": {104.43, 30.85},
- "åŒæµåŽ¿": {103.92, 30.58},
- "郫县": {103.88, 30.82},
- "大邑县": {103.52, 30.58},
- "蒲江县": {103.5, 30.2},
- "新津县": {103.82, 30.42},
- "éƒ½æ±Ÿå °å¸‚": {103.62, 31},
- "å½å·žå¸‚": {103.93, 30.98},
- "邛崃市": {103.47, 30.42},
- "崇州市": {103.67, 30.63},
- "自贡市": {104.78, 29.35},
- "自æµäº•区": {104.77, 29.35},
- "贡井区": {104.72, 29.35},
- "大安区": {104.77, 29.37},
- "沿滩区": {104.87, 29.27},
- "è£åŽ¿": {104.42, 29.47},
- "富顺县": {104.98, 29.18},
- "攀æžèб参": {101.72, 26.58},
- "东区": {101.7, 26.55},
- "西区": {101.6, 26.6},
- "ä»å’ŒåŒº": {101.73, 26.5},
- "米易县": {102.12, 26.88},
- "ç›è¾¹åŽ¿": {101.85, 26.7},
- "泸州市": {105.43, 28.87},
- "江阳区": {105.45, 28.88},
- "纳溪区": {105.37, 28.77},
- "龙马æ½åŒº": {105.43, 28.9},
- "泸县": {105.38, 29.15},
- "åˆæ±ŸåŽ¿": {105.83, 28.82},
- "噿°¸åŽ¿": {105.43, 28.17},
- "å¤è”ºåŽ¿": {105.82, 28.05},
- "德阳市": {104.38, 31.13},
- "旌阳区": {104.38, 31.13},
- "䏿±ŸåŽ¿": {104.68, 31.03},
- "罗江县": {104.5, 31.32},
- "广汉市": {104.28, 30.98},
- "什邡市": {104.17, 31.13},
- "绵竹市": {104.2, 31.35},
- "绵阳市": {104.73, 31.47},
- "涪城区": {104.73, 31.47},
- "游仙区": {104.75, 31.47},
- "三å°åŽ¿": {105.08, 31.1},
- "ç›äºåŽ¿": {105.38, 31.22},
- "安县": {104.57, 31.53},
- "梓潼县": {105.17, 31.63},
- "北å·ç¾Œæ—自治县": {104.45, 31.82},
- "å¹³æ¦åŽ¿": {104.53, 32.42},
- "江油市": {104.75, 31.78},
- "广元市": {105.83, 32.43},
- "å…ƒå区": {105.97, 32.32},
- "æœå¤©åŒº": {105.88, 32.65},
- "æ—ºè‹åŽ¿": {106.28, 32.23},
- "é’å·åŽ¿": {105.23, 32.58},
- "剑é˜åŽ¿": {105.52, 32.28},
- "è‹æºªåŽ¿": {105.93, 31.73},
- "é‚å®å¸‚": {105.57, 30.52},
- "船山区": {105.57, 30.52},
- "安居区": {105.45, 30.35},
- "蓬溪县": {105.72, 30.78},
- "射洪县": {105.38, 30.87},
- "大英县": {105.25, 30.58},
- "内江市": {105.05, 29.58},
- "东兴区": {105.07, 29.6},
- "å¨è¿œåŽ¿": {104.67, 29.53},
- "资ä¸åŽ¿": {104.85, 29.78},
- "隆??县": {105.28, 29.35},
- "ä¹å±±å¸‚": {103.77, 29.57},
- "沙湾区": {103.55, 29.42},
- "五通桥区": {103.82, 29.4},
- "金壿²³åŒº": {103.08, 29.25},
- "çŠä¸ºåŽ¿": {103.95, 29.22},
- "äº•ç ”åŽ¿": {104.07, 29.65},
- "夹江县": {103.57, 29.73},
- "æ²å·åŽ¿": {103.9, 28.97},
- "å³¨è¾¹å½æ—自治县": {103.27, 29.23},
- "é©¬è¾¹å½æ—自治县": {103.55, 28.83},
- "峨眉山市": {103.48, 29.6},
- "å—充市": {106.08, 30.78},
- "顺庆区": {106.08, 30.78},
- "高åªåŒº": {106.1, 30.77},
- "嘉陵区": {106.05, 30.77},
- "å—部县": {106.07, 31.35},
- "è¥å±±åŽ¿": {106.57, 31.08},
- "蓬安县": {106.42, 31.03},
- "仪陇县": {106.28, 31.27},
- "西充县": {105.88, 31},
- "阆ä¸å¸‚": {106, 31.55},
- "眉山市": {103.83, 30.05},
- "东å¡åŒº": {103.83, 30.05},
- "ä»å¯¿åŽ¿": {104.15, 30},
- "å½å±±åŽ¿": {103.87, 30.2},
- "洪雅县": {103.37, 29.92},
- "丹棱县": {103.52, 30.02},
- "é’神县": {103.85, 29.83},
- "宜宾市": {104.62, 28.77},
- "ç¿ å±åŒº": {104.62, 28.77},
- "宜宾县": {104.55, 28.7},
- "å—æºªåŽ¿": {104.98, 28.85},
- "江安县": {105.07, 28.73},
- "é•¿å®åŽ¿": {104.92, 28.58},
- "高县": {104.52, 28.43},
- "ç™åŽ¿": {104.72, 28.45},
- "ç 连县": {104.52, 28.17},
- "兴文县": {105.23, 28.3},
- "å±å±±åŽ¿": {104.33, 28.83},
- "广安市": {106.63, 30.47},
- "å²³æ± åŽ¿": {106.43, 30.55},
- "æ¦èƒœåŽ¿": {106.28, 30.35},
- "邻水县": {106.93, 30.33},
- "åŽè“¥å¸‚": {106.77, 30.38},
- "达州市": {107.5, 31.22},
- "通å·åŒº": {107.48, 31.22},
- "达县": {107.5, 31.2},
- "宣汉县": {107.72, 31.35},
- "开江县": {107.87, 31.08},
- "大竹县": {107.2, 30.73},
- "æ¸ åŽ¿": {106.97, 30.83},
- "万æºå¸‚": {108.03, 32.07},
- "雅安市": {103, 29.98},
- "雨城区": {103, 29.98},
- "å山县": {103.12, 30.08},
- "è¥ç»åŽ¿": {102.85, 29.8},
- "汉æºåŽ¿": {102.65, 29.35},
- "石棉县": {102.37, 29.23},
- "天全县": {102.75, 30.07},
- "芦山县": {102.92, 30.15},
- "å®å…´åŽ¿": {102.82, 30.37},
- "å·´ä¸å¸‚": {106.77, 31.85},
- "巴州区": {106.77, 31.85},
- "通江县": {107.23, 31.92},
- "å—æ±ŸåŽ¿": {106.83, 32.35},
- "平昌县": {107.1, 31.57},
- "资阳市": {104.65, 30.12},
- "雿±ŸåŒº": {104.65, 30.12},
- "安岳县": {105.33, 30.1},
- "ä¹è‡³åŽ¿": {105.02, 30.28},
- "简阳市": {104.55, 30.4},
- "阿åè—æ—羌æ—自治州": {102.22, 31.9},
- "æ±¶å·åŽ¿": {103.58, 31.48},
- "ç†åŽ¿": {103.17, 31.43},
- "茂县": {103.85, 31.68},
- "æ¾æ½˜åŽ¿": {103.6, 32.63},
- "ä¹å¯¨æ²ŸåŽ¿": {104.23, 33.27},
- "金å·åŽ¿": {102.07, 31.48},
- "å°é‡‘县": {102.37, 31},
- "黑水县": {102.98, 32.07},
- "马尔康县": {102.22, 31.9},
- "壤塘县": {100.98, 32.27},
- "阿å县": {101.7, 32.9},
- "若尔盖县": {102.95, 33.58},
- "红原县": {102.55, 32.8},
- "甘åœè—æ—自治州": {101.97, 30.05},
- "康定县": {101.97, 30.05},
- "泸定县": {102.23, 29.92},
- "丹巴县": {101.88, 30.88},
- "ä¹é¾™åŽ¿": {101.5, 29},
- "雅江县": {101.02, 30.03},
- "é“åšåŽ¿": {101.12, 30.98},
- "炉éœåŽ¿": {100.68, 31.4},
- "甘åœåŽ¿": {99.98, 31.62},
- "新龙县": {100.32, 30.95},
- "å¾·æ ¼åŽ¿": {98.58, 31.82},
- "白玉县": {98.83, 31.22},
- "çŸ³æ¸ åŽ¿": {98.1, 32.98},
- "色达县": {100.33, 32.27},
- "ç†å¡˜åŽ¿": {100.27, 30},
- "巴塘县": {99.1, 30},
- "乡城县": {99.8, 28.93},
- "稻城县": {100.3, 29.03},
- "å¾—è£åŽ¿": {99.28, 28.72},
- "凉山彿—自治州": {102.27, 27.9},
- "西昌市": {102.27, 27.9},
- "æœ¨é‡Œè—æ—自治县": {101.28, 27.93},
- "ç›æºåŽ¿": {101.5, 27.43},
- "德昌县": {102.18, 27.4},
- "会ç†åŽ¿": {102.25, 26.67},
- "会东县": {102.58, 26.63},
- "å®å—县": {102.77, 27.07},
- "æ™®æ ¼åŽ¿": {102.53, 27.38},
- "布拖县": {102.82, 27.72},
- "金阳县": {103.25, 27.7},
- "æ˜è§‰åŽ¿": {102.85, 28.02},
- "喜德县": {102.42, 28.32},
- "冕å®åŽ¿": {102.17, 28.55},
- "越西县": {102.52, 28.65},
- "甘洛县": {102.77, 28.97},
- "美姑县": {103.13, 28.33},
- "雷波县": {103.57, 28.27},
- "贵阳市": {106.63, 26.65},
- "å—æ˜ŽåŒº": {106.72, 26.57},
- "云岩区": {106.72, 26.62},
- "乌当区": {106.75, 26.63},
- "白云区": {106.65, 26.68},
- "å°æ²³åŒº": {106.7, 26.53},
- "开阳县": {106.97, 27.07},
- "æ¯çƒ½åŽ¿": {106.73, 27.1},
- "修文县": {106.58, 26.83},
- "清镇市": {106.47, 26.55},
- "å…盘水市": {104.83, 26.6},
- "钟山区": {104.83, 26.6},
- "å…æžç‰¹åŒº": {105.48, 26.22},
- "水城县": {104.95, 26.55},
- "盘县": {104.47, 25.72},
- "éµä¹‰å¸‚": {106.92, 27.73},
- "红花岗区": {106.92, 27.65},
- "汇å·åŒº": {106.92, 27.73},
- "éµä¹‰åŽ¿": {106.83, 27.53},
- "æ¡æ¢“县": {106.82, 28.13},
- "绥阳县": {107.18, 27.95},
- "æ£å®‰åŽ¿": {107.43, 28.55},
- "é“真仡佬æ—è‹—æ—自治县": {107.6, 28.88},
- "务å·ä»¡ä½¬æ—è‹—æ—自治县": {107.88, 28.53},
- "凤冈县": {107.72, 27.97},
- "湄æ½åŽ¿": {107.48, 27.77},
- "余庆县": {107.88, 27.22},
- "ä¹ æ°´åŽ¿": {106.22, 28.32},
- "赤水市": {105.7, 28.58},
- "仿€€å¸‚": {106.42, 27.82},
- "安顺市": {105.95, 26.25},
- "西秀区": {105.92, 26.25},
- "å¹³å县": {106.25, 26.42},
- "普定县": {105.75, 26.32},
- "镇å®å¸ƒä¾æ—è‹—æ—自治县": {105.77, 26.07},
- "å…³å²å¸ƒä¾æ—è‹—æ—自治县": {105.62, 25.95},
- "紫云苗æ—å¸ƒä¾æ—自治县": {106.08, 25.75},
- "铜ä»åœ°åŒº": {109.18, 27.72},
- "铜ä»å¸‚": {109.18, 27.72},
- "江å£åŽ¿": {108.85, 27.7},
- "玉å±ä¾—æ—自治县": {108.92, 27.23},
- "石阡县": {108.23, 27.52},
- "æ€å—县": {108.25, 27.93},
- "å°æ±ŸåœŸå®¶æ—è‹—æ—自治县": {108.4, 28},
- "德江县": {108.12, 28.27},
- "沿河土家æ—自治县": {108.5, 28.57},
- "æ¾æ¡ƒè‹—æ—自治县": {109.2, 28.17},
- "万山特区": {109.2, 27.52},
- "兴义市": {104.9, 25.08},
- "å…´ä»åŽ¿": {105.18, 25.43},
- "普安县": {104.95, 25.78},
- "晴隆县": {105.22, 25.83},
- "贞丰县": {105.65, 25.38},
- "望谟县": {106.1, 25.17},
- "册亨县": {105.82, 24.98},
- "安龙县": {105.47, 25.12},
- "毕节地区": {105.28, 27.3},
- "毕节市": {105.28, 27.3},
- "大方县": {105.6, 27.15},
- "黔西县": {106.03, 27.03},
- "金沙县": {106.22, 27.47},
- "织金县": {105.77, 26.67},
- "纳é›åŽ¿": {105.38, 26.78},
- "èµ«ç« åŽ¿": {104.72, 27.13},
- "黔东å—è‹—æ—ä¾—æ—自治州": {107.97, 26.58},
- "凯里市": {107.97, 26.58},
- "黄平县": {107.9, 26.9},
- "施秉县": {108.12, 27.03},
- "三穗县": {108.68, 26.97},
- "镇远县": {108.42, 27.05},
- "岑巩县": {108.82, 27.18},
- "天柱县": {109.2, 26.92},
- "锦å±åŽ¿": {109.2, 26.68},
- "剑河县": {108.45, 26.73},
- "å°æ±ŸåŽ¿": {108.32, 26.67},
- "黎平县": {109.13, 26.23},
- "榕江县": {108.52, 25.93},
- "从江县": {108.9, 25.75},
- "雷山县": {108.07, 26.38},
- "麻江县": {107.58, 26.5},
- "丹寨县": {107.8, 26.2},
- "é»”å—å¸ƒä¾æ—è‹—æ—自治州": {107.52, 26.27},
- "都匀市": {107.52, 26.27},
- "ç¦æ³‰å¸‚": {107.5, 26.7},
- "è”æ³¢åŽ¿": {107.88, 25.42},
- "贵定县": {107.23, 26.58},
- "瓮安县": {107.47, 27.07},
- "独山县": {107.53, 25.83},
- "平塘县": {107.32, 25.83},
- "罗甸县": {106.75, 25.43},
- "长顺县": {106.45, 26.03},
- "龙里县": {106.97, 26.45},
- "æƒ æ°´åŽ¿": {106.65, 26.13},
- "三都水æ—自治县": {107.87, 25.98},
- "昆明市": {102.72, 25.05},
- "五åŽåŒº": {102.7, 25.05},
- "盘龙区": {102.72, 25.03},
- "官渡区": {102.75, 25.02},
- "西山区": {102.67, 25.03},
- "东å·åŒº": {103.18, 26.08},
- "呈贡县": {102.8, 24.88},
- "晋å®åŽ¿": {102.6, 24.67},
- "富民县": {102.5, 25.22},
- "宜良县": {103.15, 24.92},
- "çŸ³æž—å½æ—自治县": {103.27, 24.77},
- "嵩明县": {103.03, 25.35},
- "禄åŠå½æ—è‹—æ—自治县": {102.47, 25.55},
- "寻甸回æ—彿—自治县": {103.25, 25.57},
- "安å®å¸‚": {102.48, 24.92},
- "曲é–市": {103.8, 25.5},
- "麒麟区": {103.8, 25.5},
- "马龙县": {103.58, 25.43},
- "陆良县": {103.67, 25.03},
- "师宗县": {103.98, 24.83},
- "罗平县": {104.3, 24.88},
- "富æºåŽ¿": {104.25, 25.67},
- "会泽县": {103.3, 26.42},
- "沾益县": {103.82, 25.62},
- "宣å¨å¸‚": {104.1, 26.22},
- "玉溪市": {102.55, 24.35},
- "江å·åŽ¿": {102.75, 24.28},
- "澄江县": {102.92, 24.67},
- "通海县": {102.75, 24.12},
- "åŽå®åŽ¿": {102.93, 24.2},
- "易门县": {102.17, 24.67},
- "峍山彿—自治县": {102.4, 24.18},
- "æ–°å¹³å½æ—å‚£æ—自治县": {101.98, 24.07},
- "ä¿å±±å¸‚": {99.17, 25.12},
- "隆阳区": {99.17, 25.12},
- "施甸县": {99.18, 24.73},
- "腾冲县": {98.5, 25.03},
- "龙陵县": {98.68, 24.58},
- "昌å®åŽ¿": {99.6, 24.83},
- "æ˜é€šå¸‚": {103.72, 27.33},
- "æ˜é˜³åŒº": {103.72, 27.33},
- "é²ç”¸åŽ¿": {103.55, 27.2},
- "巧家县": {102.92, 26.92},
- "ç›æ´¥åŽ¿": {104.23, 28.12},
- "大关县": {103.88, 27.75},
- "永善县": {103.63, 28.23},
- "绥江县": {103.95, 28.6},
- "镇雄县": {104.87, 27.45},
- "å½è‰¯åŽ¿": {104.05, 27.63},
- "å¨ä¿¡åŽ¿": {105.05, 27.85},
- "水富县": {104.4, 28.63},
- "丽江市": {100.23, 26.88},
- "å¤åŸŽåŒº": {100.23, 26.88},
- "玉龙纳西æ—自治县": {100.23, 26.82},
- "永胜县": {100.75, 26.68},
- "åŽåªåŽ¿": {101.27, 26.63},
- "å®è’—彿—自治县": {100.85, 27.28},
- "墨江哈尼æ—自治县": {101.68, 23.43},
- "æ™¯ä¸œå½æ—自治县": {100.83, 24.45},
- "景谷傣æ—彿—自治县": {100.7, 23.5},
- "江城哈尼æ—彿—自治县": {101.85, 22.58},
- "澜沧拉祜æ—自治县": {99.93, 22.55},
- "西盟佤æ—自治县": {99.62, 22.63},
- "临沧市": {100.08, 23.88},
- "临翔区": {100.08, 23.88},
- "凤庆县": {99.92, 24.6},
- "云县": {100.13, 24.45},
- "永德县": {99.25, 24.03},
- "镇康县": {98.83, 23.78},
- "耿马傣æ—佤æ—自治县": {99.4, 23.55},
- "æ²§æºä½¤æ—自治县": {99.25, 23.15},
- "æ¥šé›„å½æ—自治州": {101.55, 25.03},
- "楚雄市": {101.55, 25.03},
- "åŒæŸåŽ¿": {101.63, 24.7},
- "牟定县": {101.53, 25.32},
- "å—åŽåŽ¿": {101.27, 25.2},
- "姚安县": {101.23, 25.5},
- "大姚县": {101.32, 25.73},
- "æ°¸ä»åŽ¿": {101.67, 26.07},
- "元谋县": {101.88, 25.7},
- "æ¦å®šåŽ¿": {102.4, 25.53},
- "禄丰县": {102.08, 25.15},
- "红河哈尼æ—彿—自治州": {103.4, 23.37},
- "个旧市": {103.15, 23.37},
- "开远市": {103.27, 23.72},
- "蒙自县": {103.4, 23.37},
- "å±è¾¹è‹—æ—自治县": {103.68, 22.98},
- "建水县": {102.83, 23.62},
- "石å±åŽ¿": {102.5, 23.72},
- "弥勒县": {103.43, 24.4},
- "泸西县": {103.77, 24.53},
- "元阳县": {102.83, 23.23},
- "红河县": {102.42, 23.37},
- "绿春县": {102.4, 23},
- "æ²³å£ç‘¶æ—自治县": {103.97, 22.52},
- "文山壮æ—è‹—æ—自治州": {104.25, 23.37},
- "文山县": {104.25, 23.37},
- "ç šå±±åŽ¿": {104.33, 23.62},
- "西畴县": {104.67, 23.45},
- "éº»æ —å¡åŽ¿": {104.7, 23.12},
- "马关县": {104.4, 23.02},
- "丘北县": {104.18, 24.05},
- "广å—县": {105.07, 24.05},
- "富å®åŽ¿": {105.62, 23.63},
- "西åŒç‰ˆçº³å‚£æ—自治州": {100.8, 22.02},
- "景洪市": {100.8, 22.02},
- "勿µ·åŽ¿": {100.45, 21.97},
- "å‹è…ŠåŽ¿": {101.57, 21.48},
- "大ç†ç™½æ—自治州": {100.23, 25.6},
- "大ç†å¸‚": {100.23, 25.6},
- "æ¼¾æ¿žå½æ—自治县": {99.95, 25.67},
- "祥云县": {100.55, 25.48},
- "宾å·åŽ¿": {100.58, 25.83},
- "弥渡县": {100.48, 25.35},
- "å—æ¶§å½æ—自治县": {100.52, 25.05},
- "å·å±±å½æ—回æ—自治县": {100.3, 25.23},
- "永平县": {99.53, 25.47},
- "云龙县": {99.37, 25.88},
- "æ´±æºåŽ¿": {99.95, 26.12},
- "剑å·åŽ¿": {99.9, 26.53},
- "鹤庆县": {100.18, 26.57},
- "å¾·å®å‚£æ—景颇æ—自治州": {98.58, 24.43},
- "瑞丽市": {97.85, 24.02},
- "潞西市": {98.58, 24.43},
- "æ¢æ²³åŽ¿": {98.3, 24.82},
- "盈江县": {97.93, 24.72},
- "陇å·åŽ¿": {97.8, 24.2},
- "怒江傈僳æ—自治州": {98.85, 25.85},
- "泸水县": {98.85, 25.85},
- "ç¦è´¡åŽ¿": {98.87, 26.9},
- "è´¡å±±ç‹¬é¾™æ—æ€’æ—自治县": {98.67, 27.73},
- "å…°åªç™½æ—普米æ—自治县": {99.42, 26.45},
- "è¿ªåº†è—æ—自治州": {99.7, 27.83},
- "é¦™æ ¼é‡Œæ‹‰åŽ¿": {99.7, 27.83},
- "德钦县": {98.92, 28.48},
- "维西傈僳æ—自治县": {99.28, 27.18},
- "拉è¨å¸‚": {91.13, 29.65},
- "林周县": {91.25, 29.9},
- "当雄县": {91.1, 30.48},
- "尼木县": {90.15, 29.45},
- "曲水县": {90.73, 29.37},
- "å †é¾™å¾·åº†åŽ¿": {91, 29.65},
- "è¾¾åœåŽ¿": {91.35, 29.68},
- "墨竹工å¡åŽ¿": {91.73, 29.83},
- "昌都地区": {97.18, 31.13},
- "昌都县": {97.18, 31.13},
- "江达县": {98.22, 31.5},
- "贡觉县": {98.27, 30.87},
- "类乌é½åŽ¿": {96.6, 31.22},
- "ä¸é’县": {95.6, 31.42},
- "察雅县": {97.57, 30.65},
- "八宿县": {96.92, 30.05},
- "左贡县": {97.85, 29.67},
- "芒康县": {98.6, 29.68},
- "洛隆县": {95.83, 30.75},
- "è¾¹å县": {94.7, 30.93},
- "å±±å—地区": {91.77, 29.23},
- "乃东县": {91.77, 29.23},
- "扎囊县": {91.33, 29.25},
- "贡嘎县": {90.98, 29.3},
- "桑日县": {92.02, 29.27},
- "ç¼ç»“县": {91.68, 29.03},
- "曲æ¾åŽ¿": {92.2, 29.07},
- "措美县": {91.43, 28.43},
- "洛扎县": {90.87, 28.38},
- "åŠ æŸ¥åŽ¿": {92.58, 29.15},
- "隆å县": {92.47, 28.42},
- "错那县": {91.95, 28},
- "浪å¡å县": {90.4, 28.97},
- "日喀则地区": {88.88, 29.27},
- "日喀则市": {88.88, 29.27},
- "å—æœ¨æž—县": {89.1, 29.68},
- "江åœåŽ¿": {89.6, 28.92},
- "定日县": {87.12, 28.67},
- "è¨è¿¦åŽ¿": {88.02, 28.9},
- "拉åœåŽ¿": {87.63, 29.08},
- "昂ä»åŽ¿": {87.23, 29.3},
- "谢通门县": {88.27, 29.43},
- "白朗县": {89.27, 29.12},
- "ä»å¸ƒåŽ¿": {89.83, 29.23},
- "康马县": {89.68, 28.57},
- "定结县": {87.77, 28.37},
- "仲巴县": {84.03, 29.77},
- "亚东县": {88.9, 27.48},
- "å‰éš†åŽ¿": {85.3, 28.85},
- "è‚æ‹‰æœ¨åŽ¿": {85.98, 28.17},
- "è¨å˜ŽåŽ¿": {85.23, 29.33},
- "岗巴县": {88.52, 28.28},
- "那曲地区": {92.07, 31.48},
- "那曲县": {92.07, 31.48},
- "嘉黎县": {93.25, 30.65},
- "比如县": {93.68, 31.48},
- "è‚è£åŽ¿": {92.3, 32.12},
- "安多县": {91.68, 32.27},
- "申扎县": {88.7, 30.93},
- "索县": {93.78, 31.88},
- "çæˆˆåŽ¿": {90.02, 31.37},
- "å·´é’县": {94.03, 31.93},
- "尼玛县": {87.23, 31.78},
- "阿里地区": {80.1, 32.5},
- "普兰县": {81.17, 30.3},
- "æœè¾¾åŽ¿": {79.8, 31.48},
- "噶尔县": {80.1, 32.5},
- "日土县": {79.72, 33.38},
- "é©å‰åŽ¿": {81.12, 32.4},
- "改则县": {84.07, 32.3},
- "措勤县": {85.17, 31.02},
- "æž—èŠåœ°åŒº": {94.37, 29.68},
- "æž—èŠåŽ¿": {94.37, 29.68},
- "工布江达县": {93.25, 29.88},
- "米林县": {94.22, 29.22},
- "墨脱县": {95.33, 29.33},
- "波密县": {95.77, 29.87},
- "察隅县": {97.47, 28.67},
- "朗县": {93.07, 29.05},
- "西安市": {108.93, 34.27},
- "新城区": {108.95, 34.27},
- "碑林区": {108.93, 34.23},
- "莲湖区": {108.93, 34.27},
- "çžæ¡¥åŒº": {109.07, 34.27},
- "未央区": {108.93, 34.28},
- "é›å¡”区": {108.95, 34.22},
- "阎良区": {109.23, 34.65},
- "临潼区": {109.22, 34.37},
- "长安区": {108.93, 34.17},
- "è“田县": {109.32, 34.15},
- "周至县": {108.2, 34.17},
- "户县": {108.6, 34.1},
- "高陵县": {109.08, 34.53},
- "铜å·å¸‚": {108.93, 34.9},
- "王益区": {109.07, 35.07},
- "å°å°åŒº": {109.1, 35.1},
- "耀州区": {108.98, 34.92},
- "宜å›åŽ¿": {109.12, 35.4},
- "å®é¸¡å¸‚": {107.13, 34.37},
- "æ¸æ»¨åŒº": {107.15, 34.37},
- "金å°åŒº": {107.13, 34.38},
- "陈仓区": {107.37, 34.37},
- "凤翔县": {107.38, 34.52},
- "å²å±±åŽ¿": {107.62, 34.45},
- "扶风县": {107.87, 34.37},
- "眉县": {107.75, 34.28},
- "陇县": {106.85, 34.9},
- "åƒé˜³åŽ¿": {107.13, 34.65},
- "麟游县": {107.78, 34.68},
- "凤县": {106.52, 33.92},
- "太白县": {107.32, 34.07},
- "咸阳市": {108.7, 34.33},
- "秦都区": {108.72, 34.35},
- "æ¨å‡ŒåŒº": {108.07, 34.28},
- "æ¸åŸŽåŒº": {108.73, 34.33},
- "三原县": {108.93, 34.62},
- "泾阳县": {108.83, 34.53},
- "乾县": {108.23, 34.53},
- "礼泉县": {108.42, 34.48},
- "永寿县": {108.13, 34.7},
- "彬县": {108.08, 35.03},
- "é•¿æ¦åŽ¿": {107.78, 35.2},
- "旬邑县": {108.33, 35.12},
- "淳化县": {108.58, 34.78},
- "æ¦åŠŸåŽ¿": {108.2, 34.27},
- "兴平市": {108.48, 34.3},
- "æ¸å—市": {109.5, 34.5},
- "临æ¸åŒº": {109.48, 34.5},
- "åŽåŽ¿": {109.77, 34.52},
- "潼关县": {110.23, 34.55},
- "大è”县": {109.93, 34.8},
- "åˆé˜³åŽ¿": {110.15, 35.23},
- "澄城县": {109.93, 35.18},
- "蒲城县": {109.58, 34.95},
- "白水县": {109.58, 35.18},
- "富平县": {109.18, 34.75},
- "韩城市": {110.43, 35.48},
- "åŽé˜´å¸‚": {110.08, 34.57},
- "延安市": {109.48, 36.6},
- "å®å¡”区": {109.48, 36.6},
- "延长县": {110, 36.58},
- "å»¶å·åŽ¿": {110.18, 36.88},
- "å长县": {109.67, 37.13},
- "安塞县": {109.32, 36.87},
- "志丹县": {108.77, 36.82},
- "甘泉县": {109.35, 36.28},
- "富县": {109.37, 35.98},
- "æ´›å·åŽ¿": {109.43, 35.77},
- "宜å·åŽ¿": {110.17, 36.05},
- "黄龙县": {109.83, 35.58},
- "黄陵县": {109.25, 35.58},
- "汉ä¸å¸‚": {107.02, 33.07},
- "汉å°åŒº": {107.03, 33.07},
- "å—郑县": {106.93, 33},
- "城固县": {107.33, 33.15},
- "洋县": {107.55, 33.22},
- "西乡县": {107.77, 32.98},
- "勉县": {106.67, 33.15},
- "å®å¼ºåŽ¿": {106.25, 32.83},
- "略阳县": {106.15, 33.33},
- "镇巴县": {107.9, 32.53},
- "ç•™å县": {106.92, 33.62},
- "ä½›åªåŽ¿": {107.98, 33.53},
- "榆林市": {109.73, 38.28},
- "榆阳区": {109.75, 38.28},
- "神木县": {110.5, 38.83},
- "府谷县": {111.07, 39.03},
- "横山县": {109.28, 37.95},
- "é–边县": {108.8, 37.6},
- "定边县": {107.6, 37.58},
- "绥德县": {110.25, 37.5},
- "米脂县": {110.18, 37.75},
- "佳县": {110.48, 38.02},
- "å´å ¡åŽ¿": {110.73, 37.45},
- "清涧县": {110.12, 37.08},
- "åæ´²åŽ¿": {110.03, 37.62},
- "安康市": {109.02, 32.68},
- "汉滨区": {109.02, 32.68},
- "汉阴县": {108.5, 32.9},
- "石泉县": {108.25, 33.05},
- "å®é™•县": {108.32, 33.32},
- "紫阳县": {108.53, 32.52},
- "岚皋县": {108.9, 32.32},
- "平利县": {109.35, 32.4},
- "镇åªåŽ¿": {109.52, 31.88},
- "旬阳县": {109.38, 32.83},
- "白河县": {110.1, 32.82},
- "商洛市": {109.93, 33.87},
- "商州区": {109.93, 33.87},
- "æ´›å—县": {110.13, 34.08},
- "丹凤县": {110.33, 33.7},
- "商å—县": {110.88, 33.53},
- "山阳县": {109.88, 33.53},
- "镇安县": {109.15, 33.43},
- "柞水县": {109.1, 33.68},
- "兰州市": {103.82, 36.07},
- "城关区": {103.83, 36.05},
- "西固区": {103.62, 36.1},
- "红å¤åŒº": {102.87, 36.33},
- "永登县": {103.27, 36.73},
- "皋兰县": {103.95, 36.33},
- "榆ä¸åŽ¿": {104.12, 35.85},
- "嘉峪关市": {98.27, 39.8},
- "金昌市": {102.18, 38.5},
- "金å·åŒº": {102.18, 38.5},
- "永昌县": {101.97, 38.25},
- "白银市": {104.18, 36.55},
- "白银区": {104.18, 36.55},
- "å¹³å·åŒº": {104.83, 36.73},
- "é–远县": {104.68, 36.57},
- "会å®åŽ¿": {105.05, 35.7},
- "景泰县": {104.07, 37.15},
- "天水市": {105.72, 34.58},
- "清水县": {106.13, 34.75},
- "秦安县": {105.67, 34.87},
- "甘谷县": {105.33, 34.73},
- "æ¦å±±åŽ¿": {104.88, 34.72},
- "å¼ å®¶å·å›žæ—自治县": {106.22, 35},
- "æ¦å¨å¸‚": {102.63, 37.93},
- "凉州区": {102.63, 37.93},
- "民勤县": {103.08, 38.62},
- "夿µªåŽ¿": {102.88, 37.47},
- "天ç¥è—æ—自治县": {103.13, 36.98},
- "å¼ æŽ–å¸‚": {100.45, 38.93},
- "甘州区": {100.45, 38.93},
- "肃å—裕固æ—自治县": {99.62, 38.83},
- "æ°‘ä¹åŽ¿": {100.82, 38.43},
- "临泽县": {100.17, 39.13},
- "高å°åŽ¿": {99.82, 39.38},
- "山丹县": {101.08, 38.78},
- "平凉市": {106.67, 35.55},
- "崆峒区": {106.67, 35.55},
- "æ³¾å·åŽ¿": {107.37, 35.33},
- "çµå°åŽ¿": {107.62, 35.07},
- "崇信县": {107.03, 35.3},
- "åŽäºåŽ¿": {106.65, 35.22},
- "庄浪县": {106.05, 35.2},
- "é™å®åŽ¿": {105.72, 35.52},
- "酒泉市": {98.52, 39.75},
- "肃州区": {98.52, 39.75},
- "金塔县": {98.9, 39.98},
- "è‚ƒåŒ—è’™å¤æ—自治县": {94.88, 39.52},
- "阿克塞哈è¨å…‹æ—自治县": {94.33, 39.63},
- "玉门市": {97.05, 40.28},
- "敦煌市": {94.67, 40.13},
- "庆阳市": {107.63, 35.73},
- "西峰区": {107.63, 35.73},
- "庆城县": {107.88, 36},
- "环县": {107.3, 36.58},
- "åŽæ± 县": {107.98, 36.47},
- "åˆæ°´åŽ¿": {108.02, 35.82},
- "æ£å®åŽ¿": {108.37, 35.5},
- "å®åŽ¿": {107.92, 35.5},
- "镇原县": {107.2, 35.68},
- "定西市": {104.62, 35.58},
- "安定区": {104.62, 35.58},
- "通æ¸åŽ¿": {105.25, 35.2},
- "陇西县": {104.63, 35},
- "æ¸æºåŽ¿": {104.22, 35.13},
- "临洮县": {103.87, 35.38},
- "漳县": {104.47, 34.85},
- "岷县": {104.03, 34.43},
- "陇å—市": {104.92, 33.4},
- "æ¦éƒ½åŒº": {104.92, 33.4},
- "æˆåŽ¿": {105.72, 33.73},
- "文县": {104.68, 32.95},
- "宕昌县": {104.38, 34.05},
- "康县": {105.6, 33.33},
- "西和县": {105.3, 34.02},
- "礼县": {105.17, 34.18},
- "徽县": {106.08, 33.77},
- "两当县": {106.3, 33.92},
- "临å¤å›žæ—自治州": {103.22, 35.6},
- "临å¤å¸‚": {103.22, 35.6},
- "临å¤åŽ¿": {103, 35.5},
- "康ä¹åŽ¿": {103.72, 35.37},
- "æ°¸é–县": {103.32, 35.93},
- "广河县": {103.58, 35.48},
- "和政县": {103.35, 35.43},
- "东乡æ—自治县": {103.4, 35.67},
- "甘å—è—æ—自治州": {102.92, 34.98},
- "åˆä½œå¸‚": {102.92, 34.98},
- "临æ½åŽ¿": {103.35, 34.7},
- "å“尼县": {103.5, 34.58},
- "舟曲县": {104.37, 33.78},
- "è¿éƒ¨åŽ¿": {103.22, 34.05},
- "玛曲县": {102.07, 34},
- "碌曲县": {102.48, 34.58},
- "夿²³åŽ¿": {102.52, 35.2},
- "西å®å¸‚": {101.78, 36.62},
- "城东区": {101.8, 36.62},
- "城ä¸åŒº": {101.78, 36.62},
- "城西区": {101.77, 36.62},
- "城北区": {101.77, 36.67},
- "大通回æ—土æ—自治县": {101.68, 36.93},
- "湟ä¸åŽ¿": {101.57, 36.5},
- "湟æºåŽ¿": {101.27, 36.68},
- "海东地区": {102.12, 36.5},
- "平安县": {102.12, 36.5},
- "民和回æ—土æ—自治县": {102.8, 36.33},
- "ä¹éƒ½åŽ¿": {102.4, 36.48},
- "互助土æ—自治县": {101.95, 36.83},
- "化隆回æ—自治县": {102.27, 36.1},
- "循化撒拉æ—自治县": {102.48, 35.85},
- "æµ·åŒ—è—æ—自治州": {100.9, 36.97},
- "é—¨æºå›žæ—自治县": {101.62, 37.38},
- "ç¥è¿žåŽ¿": {100.25, 38.18},
- "æµ·æ™åŽ¿": {100.98, 36.9},
- "刚察县": {100.13, 37.33},
- "黄å—è—æ—自治州": {102.02, 35.52},
- "åŒä»åŽ¿": {102.02, 35.52},
- "尖扎县": {102.03, 35.93},
- "泽库县": {101.47, 35.03},
- "æ²³å—è’™å¤æ—自治县": {101.6, 34.73},
- "æµ·å—è—æ—自治州": {100.62, 36.28},
- "共和县": {100.62, 36.28},
- "åŒå¾·åŽ¿": {100.57, 35.25},
- "贵德县": {101.43, 36.05},
- "兴海县": {99.98, 35.58},
- "è´µå—县": {100.75, 35.58},
- "æžœæ´›è—æ—自治州": {100.23, 34.48},
- "玛æ²åŽ¿": {100.23, 34.48},
- "ç玛县": {100.73, 32.93},
- "甘德县": {99.9, 33.97},
- "达日县": {99.65, 33.75},
- "久治县": {101.48, 33.43},
- "玛多县": {98.18, 34.92},
- "çŽ‰æ ‘è—æ—自治州": {97.02, 33},
- "çŽ‰æ ‘åŽ¿": {97.02, 33},
- "æ‚多县": {95.3, 32.9},
- "称多县": {97.1, 33.37},
- "治多县": {95.62, 33.85},
- "囊谦县": {96.48, 32.2},
- "曲麻莱县": {95.8, 34.13},
- "æµ·è¥¿è’™å¤æ—è—æ—自治州": {97.37, 37.37},
- "æ ¼å°”æœ¨å¸‚": {94.9, 36.42},
- "德令哈市": {97.37, 37.37},
- "乌兰县": {98.48, 36.93},
- "都兰县": {98.08, 36.3},
- "天峻县": {99.02, 37.3},
- "é“¶å·å¸‚": {106.28, 38.47},
- "兴庆区": {106.28, 38.48},
- "西å¤åŒº": {106.18, 38.48},
- "金凤区": {106.25, 38.47},
- "æ°¸å®åŽ¿": {106.25, 38.28},
- "贺兰县": {106.35, 38.55},
- "çµæ¦å¸‚": {106.33, 38.1},
- "石嘴山市": {106.38, 39.02},
- "大æ¦å£åŒº": {106.38, 39.02},
- "æƒ å†œåŒº": {106.78, 39.25},
- "平罗县": {106.53, 38.9},
- "å´å¿ 市": {106.2, 37.98},
- "利通区": {106.2, 37.98},
- "ç›æ± 县": {107.4, 37.78},
- "åŒå¿ƒåŽ¿": {105.92, 36.98},
- "é’铜峡市": {106.07, 38.02},
- "固原市": {106.28, 36},
- "原州区": {106.28, 36},
- "西å‰åŽ¿": {105.73, 35.97},
- "隆德县": {106.12, 35.62},
- "æ³¾æºåŽ¿": {106.33, 35.48},
- "å½é˜³åŽ¿": {106.63, 35.85},
- "ä¸å«å¸‚": {105.18, 37.52},
- "æ²™å¡å¤´åŒº": {105.18, 37.52},
- "ä¸å®åŽ¿": {105.67, 37.48},
- "海原县": {105.65, 36.57},
- "ä¹Œé²æœ¨é½å¸‚": {87.62, 43.82},
- "天山区": {87.65, 43.78},
- "æ²™ä¾å·´å…‹åŒº": {87.6, 43.78},
- "新市区": {87.6, 43.85},
- "水磨沟区": {87.63, 43.83},
- "头屯河区": {87.42, 43.87},
- "è¾¾å‚城区": {88.3, 43.35},
- "ä¹Œé²æœ¨é½åŽ¿": {87.6, 43.8},
- "克拉玛ä¾å¸‚": {84.87, 45.6},
- "独山å区": {84.85, 44.32},
- "克拉玛ä¾åŒº": {84.87, 45.6},
- "白碱滩区": {85.13, 45.7},
- "乌尔禾区": {85.68, 46.08},
- "åé²ç•ªåœ°åŒº": {89.17, 42.95},
- "åé²ç•ªå¸‚": {89.17, 42.95},
- "鄯善县": {90.22, 42.87},
- "托克逊县": {88.65, 42.78},
- "哈密地区": {93.52, 42.83},
- "哈密市": {93.52, 42.83},
- "伊å¾åŽ¿": {94.7, 43.25},
- "昌å‰å›žæ—自治州": {87.3, 44.02},
- "昌å‰å¸‚": {87.3, 44.02},
- "阜康市": {87.98, 44.15},
- "米泉市": {87.65, 43.97},
- "呼图å£åŽ¿": {86.9, 44.18},
- "玛纳斯县": {86.22, 44.3},
- "奇å°åŽ¿": {89.58, 44.02},
- "剿œ¨è¨å°”县": {89.18, 44},
- "木垒哈è¨å…‹è‡ªæ²»åŽ¿": {90.28, 43.83},
- "åšå°”塔拉蒙å¤è‡ªæ²»å·ž": {82.07, 44.9},
- "åšä¹å¸‚": {82.07, 44.9},
- "精河县": {82.88, 44.6},
- "温泉县": {81.03, 44.97},
- "å·´éŸ³éƒæ¥žè’™å¤è‡ªæ²»å·ž": {86.15, 41.77},
- "库尔勒市": {86.15, 41.77},
- "è½®å°åŽ¿": {84.27, 41.78},
- "å°‰çŠåŽ¿": {86.25, 41.33},
- "若羌县": {88.17, 39.02},
- "且末县": {85.53, 38.13},
- "焉耆回æ—自治县": {86.57, 42.07},
- "å’Œé™åŽ¿": {86.4, 42.32},
- "和硕县": {86.87, 42.27},
- "åšæ¹–县": {86.63, 41.98},
- "阿克è‹åœ°åŒº": {80.27, 41.17},
- "阿克è‹å¸‚": {80.27, 41.17},
- "温宿县": {80.23, 41.28},
- "库车县": {82.97, 41.72},
- "沙雅县": {82.78, 41.22},
- "新和县": {82.6, 41.55},
- "拜城县": {81.87, 41.8},
- "乌什县": {79.23, 41.22},
- "阿瓦æåŽ¿": {80.38, 40.63},
- "柯åªåŽ¿": {79.05, 40.5},
- "阿图什市": {76.17, 39.72},
- "阿克陶县": {75.95, 39.15},
- "阿åˆå¥‡åŽ¿": {78.45, 40.93},
- "乌æ°åŽ¿": {75.25, 39.72},
- "喀什地区": {75.98, 39.47},
- "喀什市": {75.98, 39.47},
- "ç–附县": {75.85, 39.38},
- "ç–勒县": {76.05, 39.4},
- "è‹±å‰æ²™åŽ¿": {76.17, 38.93},
- "泽普县": {77.27, 38.18},
- "莎车县": {77.23, 38.42},
- "å¶åŸŽåŽ¿": {77.42, 37.88},
- "麦盖æåŽ¿": {77.65, 38.9},
- "岳普湖县": {76.77, 39.23},
- "伽师县": {76.73, 39.5},
- "巴楚县": {78.55, 39.78},
- "和田地区": {79.92, 37.12},
- "和田市": {79.92, 37.12},
- "和田县": {79.93, 37.1},
- "墨玉县": {79.73, 37.27},
- "皮山县": {78.28, 37.62},
- "洛浦县": {80.18, 37.07},
- "ç–勒县": {80.8, 37},
- "于田县": {81.67, 36.85},
- "民丰县": {82.68, 37.07},
- "伊çŠå“ˆè¨å…‹è‡ªæ²»å·ž": {81.32, 43.92},
- "伊å®å¸‚": {81.32, 43.92},
- "奎屯市": {84.9, 44.42},
- "伊å®åŽ¿": {81.52, 43.98},
- "察布查尔锡伯自治县": {81.15, 43.83},
- "éœåŸŽåŽ¿": {80.88, 44.05},
- "巩留县": {82.23, 43.48},
- "æ–°æºåŽ¿": {83.25, 43.43},
- "æ˜è‹åŽ¿": {81.13, 43.15},
- "特克斯县": {81.83, 43.22},
- "尼勒克县": {82.5, 43.78},
- "塔城地区": {82.98, 46.75},
- "塔城市": {82.98, 46.75},
- "乌è‹å¸‚": {84.68, 44.43},
- "颿•县": {83.63, 46.53},
- "沙湾县": {85.62, 44.33},
- "托里县": {83.6, 45.93},
- "裕民县": {82.98, 46.2},
- "和布克赛尔蒙å¤è‡ªæ²»åŽ¿": {85.72, 46.8},
- "阿勒泰地区": {88.13, 47.85},
- "阿勒泰市": {88.13, 47.85},
- "布尔津县": {86.85, 47.7},
- "富蕴县": {89.52, 47},
- "ç¦æµ·åŽ¿": {87.5, 47.12},
- "哈巴河县": {86.42, 48.07},
- "é’æ²³åŽ¿": {90.38, 46.67},
- "剿œ¨ä¹ƒåŽ¿": {85.88, 47.43},
- "石河å市": {86.03, 44.3},
- "阿拉尔市": {81.28, 40.55},
- "图木舒克市": {79.13, 39.85},
- "äº”å®¶æ¸ å¸‚": {87.53, 44.17},
- "å°åŒ—市": {121.5, 25.03},
- "高雄市": {120.28, 22.62},
- "基隆市": {121.73, 25.13},
- "å°ä¸å¸‚": {120.67, 24.15},
- "å°å—市": {120.2, 23},
- "新竹市": {120.95, 24.82},
- "嘉义市": {120.43, 23.48},
- "å°åŒ—县": {121.47, 25.02},
- "宜兰县": {121.75, 24.77},
- "桃å›åŽ¿": {121.3, 24.97},
- "è‹—æ —åŽ¿": {120.8, 24.53},
- "å°ä¸åŽ¿": {120.72, 24.25},
- "彰化县": {120.53, 24.08},
- "å—æŠ•åŽ¿": {120.67, 23.92},
- "云林县": {120.53, 23.72},
- "å°å—县": {120.32, 23.32},
- "高雄县": {120.37, 22.63},
- "å±ä¸œåŽ¿": {120.48, 22.67},
- "å°ä¸œåŽ¿": {121.15, 22.75},
- "花莲县": {121.6, 23.98},
- "澎湖县": {119.58, 23.58},
- "北京": {116.407526, 39.90403},
- "天津": {117.200983, 39.084158},
- "河北": {114.468664, 38.037057},
- "山西": {112.562398, 37.873531},
- "内蒙å¤": {111.765617, 40.817498},
- "è¾½å®": {123.42944, 41.835441},
- "剿ž—": {125.32599, 43.896536},
- "黑龙江": {126.661669, 45.742347},
- "上海": {121.473701, 31.230416},
- "江è‹": {118.763232, 32.061707},
- "浙江": {120.152791, 30.267446},
- "安徽": {117.284922, 31.861184},
- "ç¦å»º": {119.295144, 26.100779},
- "江西": {115.909228, 28.675696},
- "山东": {117.020359, 36.66853},
- "æ²³å—": {113.753602, 34.765515},
- "湖北": {114.341861, 30.546498},
- "æ¹–å—": {112.98381, 28.112444},
- "广东": {113.26653, 23.132191},
- "广西": {108.327546, 22.815478},
- "æµ·å—": {110.349228, 20.017377},
- "é‡åº†": {106.551556, 29.563009},
- "å››å·": {104.075931, 30.651651},
- "贵州": {106.70741, 26.598194},
- "云å—": {102.710002, 25.045806},
- "西è—": {91.117212, 29.646922},
- "陕西": {108.954239, 34.265472},
- "甘肃": {103.826308, 36.059421},
- "é’æµ·": {101.780199, 36.620901},
- "å®å¤": {106.258754, 38.471317},
- "æ–°ç–†": {87.627704, 43.793026},
- "香港": {114.173355, 22.320048},
- "澳门": {113.54909, 22.198951},
- "å°æ¹¾": {121.509062, 25.044332},
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/datasets/mapfiles.go b/vendor/github.com/go-echarts/go-echarts/v2/datasets/mapfiles.go
deleted file mode 100644
index 64d8a3c5..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/datasets/mapfiles.go
+++ /dev/null
@@ -1,404 +0,0 @@
-package datasets
-
-var MapFileNames = map[string]string{
- "china": "china",
- "world": "world",
- "广东": "guangdong",
- "安徽": "anhui",
- "ç¦å»º": "fujian",
- "甘肃": "gansu",
- "广西": "guangxi",
- "贵州": "guizhou",
- "æµ·å—": "hainan",
- "河北": "hebei",
- "黑龙江": "heilongjiang",
- "æ²³å—": "henan",
- "湖北": "hubei",
- "æ¹–å—": "hunan",
- "江è‹": "jiangsu",
- "江西": "jiangxi",
- "剿ž—": "jilin",
- "è¾½å®": "liaoning",
- "内蒙å¤": "neimenggu",
- "å®å¤": "ningxia",
- "é’æµ·": "qinghai",
- "山东": "shandong",
- "山西": "shanxi",
- "陕西": "shanxi1",
- "å››å·": "sichuan",
- "å°æ¹¾": "taiwan",
- "æ–°ç–†": "xinjiang",
- "西è—": "xizang",
- "云å—": "yunnan",
- "浙江": "zhejiang",
- "ä¸ƒå°æ²³": "hei1_long2_jiang1_qi1_tai2_he2",
- "万å®": "hai3_nan2_wan4_ning2",
- "三亚": "hai3_nan2_san1_ya4",
- "三明": "fu2_jian4_san1_ming2",
- "三沙": "hai3_nan2_san1_sha1",
- "三门峡": "he2_nan2_san1_men2_xia2",
- "上海": "shanghai",
- "上饶": "jiang1_xi1_shang4_rao2",
- "东方": "hai3_nan2_dong1_fang1",
- "东沙群岛": "guang3_dong1_dong1_sha1_qun2_dao3",
- "东莞": "guang3_dong1_dong1_guan1",
- "东è¥": "shan1_dong1_dong1_ying2",
- "ä¸å«": "ning2_xia4_zhong1_wei4",
- "ä¸å±±": "guang3_dong1_zhong1_shan1",
- "临å¤å›žæ—自治州": "gan1_su4_lin2_xia4_hui2_zu2_zi4_zhi4_zhou1",
- "临汾": "shan1_xi1_lin2_fen2",
- "临沂": "shan1_dong1_lin2_yi2",
- "临沧": "yun2_nan2_lin2_cang1",
- "临高县": "hai3_nan2_lin2_gao1_xian4",
- "丹东": "liao2_ning2_dan1_dong1",
- "丽水": "zhe4_jiang1_li4_shui3",
- "丽江": "yun2_nan2_li4_jiang1",
- "乌兰察布": "nei4_meng2_gu3_wu1_lan2_cha2_bu4",
- "乌海": "nei4_meng2_gu3_wu1_hai3",
- "ä¹Œé²æœ¨é½": "xin1_jiang1_wu1_lu3_mu4_qi2",
- "ä¹ä¸œé»Žæ—自治县": "hai3_nan2_le4_dong1_li2_zu2_zi4_zhi4_xian4",
- "ä¹å±±": "si4_chuan1_le4_shan1",
- "乿±Ÿ": "jiang1_xi1_jiu3_jiang1",
- "云浮": "guang3_dong1_yun2_fu2",
- "äº”å®¶æ¸ ": "xin1_jiang1_wu3_jia1_qu2",
- "五指山": "hai3_nan2_wu3_zhi3_shan1",
- "亳州": "an1_hui1_bo2_zhou1",
- "仙桃": "hu2_bei3_xian1_tao2",
- "伊春": "hei1_long2_jiang1_yi1_chun1",
- "伊çŠå“ˆè¨å…‹è‡ªæ²»å·ž": "xin1_jiang1_yi1_li2_ha1_sa4_ke4_zi4_zhi4_zhou1",
- "佛山": "guang3_dong1_fo2_shan1",
- "佳木斯": "hei1_long2_jiang1_jia1_mu4_si1",
- "ä¿äºé»Žæ—è‹—æ—自治县": "hai3_nan2_bao3_ting2_li2_zu2_miao2_zu2_zi4_zhi4_xian4",
- "ä¿å®š": "he2_bei3_bao3_ding4",
- "ä¿å±±": "yun2_nan2_bao3_shan1",
- "信阳": "he2_nan2_xin4_yang2",
- "å„‹å·ž": "hai3_nan2_dan1_zhou1",
- "å…‹åœå‹’è‹æŸ¯å°”å…‹åœè‡ªæ²»å·ž": "xin1_jiang1_ke4_zi1_le4_su1_ke1_er3_ke4_zi1_zi4_zhi4_zhou1",
- "克拉玛ä¾": "xin1_jiang1_ke4_la1_ma3_yi1",
- "å…安": "an1_hui1_liu4_an1",
- "å…盘水": "gui4_zhou1_liu4_pan2_shui3",
- "å…°å·ž": "gan1_su4_lan2_zhou1",
- "兴安盟": "nei4_meng2_gu3_xing1_an1_meng2",
- "内江": "si4_chuan1_nei4_jiang1",
- "凉山彿—自治州": "si4_chuan1_liang2_shan1_yi2_zu2_zi4_zhi4_zhou1",
- "包头": "nei4_meng2_gu3_bao1_tou2",
- "北京": "beijing",
- "北屯": "xin1_jiang1_bei3_tun2",
- "北海": "guang3_xi1_bei3_hai3",
- "åå °": "hu2_bei3_shi2_yan4",
- "å—京": "jiang1_su1_nan2_jing1",
- "å—å……": "si4_chuan1_nan2_chong1",
- "å—å®": "guang3_xi1_nan2_ning2",
- "å—å¹³": "fu2_jian4_nan2_ping2",
- "å—æ˜Œ": "jiang1_xi1_nan2_chang1",
- "å—通": "jiang1_su1_nan2_tong1",
- "å—阳": "he2_nan2_nan2_yang2",
- "åšå°”塔拉蒙å¤è‡ªæ²»å·ž": "xin1_jiang1_bo2_er3_ta3_la1_meng2_gu3_zi4_zhi4_zhou1",
- "厦门": "fu2_jian4_sha4_men2",
- "åŒæ²³": "xin1_jiang1_shuang1_he2",
- "åŒé¸å±±": "hei1_long2_jiang1_shuang1_ya1_shan1",
- "å¯å…‹è¾¾æ‹‰": "xin1_jiang1_ke3_ke4_da2_la1",
- "å°å·ž": "zhe4_jiang1_tai2_zhou1",
- "åˆè‚¥": "an1_hui1_he2_fei2",
- "å‰å®‰": "jiang1_xi1_ji2_an1",
- "剿ž—市": "ji2_lin2_ji2_lin2",
- "åé²ç•ª": "xin1_jiang1_tu3_lu3_fan1",
- "啿¢": "shan1_xi1_lv3_liang2",
- "å´å¿ ": "ning2_xia4_wu2_zhong1",
- "周å£": "he2_nan2_zhou1_kou3",
- "呼伦è´å°”": "nei4_meng2_gu3_hu1_lun2_bei4_er3",
- "呼和浩特": "nei4_meng2_gu3_hu1_he2_hao4_te4",
- "和田地区": "xin1_jiang1_he2_tian2_di4_qu1",
- "å’¸å®": "hu2_bei3_xian2_ning2",
- "咸阳": "shan3_xi1_xian2_yang2",
- "哈密": "xin1_jiang1_ha1_mi4",
- "哈尔滨": "hei1_long2_jiang1_ha1_er3_bin1",
- "å”å±±": "he2_bei3_tang2_shan1",
- "商丘": "he2_nan2_shang1_qiu1",
- "商洛": "shan3_xi1_shang1_luo4",
- "喀什地区": "xin1_jiang1_ka1_shi2_di4_qu1",
- "嘉兴": "zhe4_jiang1_jia1_xing1",
- "嘉峪关": "gan1_su4_jia1_yu4_guan1",
- "四平": "ji2_lin2_si4_ping2",
- "固原": "ning2_xia4_gu4_yuan2",
- "图木舒克": "xin1_jiang1_tu2_mu4_shu1_ke4",
- "塔城地区": "xin1_jiang1_ta3_cheng2_di4_qu1",
- "大兴安å²åœ°åŒº": "hei1_long2_jiang1_da4_xing1_an1_ling2_di4_qu1",
- "大åŒ": "shan1_xi1_da4_tong2",
- "大庆": "hei1_long2_jiang1_da4_qing4",
- "大ç†ç™½æ—自治州": "yun2_nan2_da4_li3_bai2_zu2_zi4_zhi4_zhou1",
- "大连": "liao2_ning2_da4_lian2",
- "天水": "gan1_su4_tian1_shui3",
- "天津": "tianjin",
- "天门": "hu2_bei3_tian1_men2",
- "太原": "shan1_xi1_tai4_yuan2",
- "卿µ·": "shan1_dong1_wei1_hai3",
- "娄底": "hu2_nan2_lou2_di3",
- "åæ„Ÿ": "hu2_bei3_xiao4_gan3",
- "å®å¾·": "fu2_jian4_ning2_de2",
- "宿³¢": "zhe4_jiang1_ning2_bo1",
- "安庆": "an1_hui1_an1_qing4",
- "安康": "shan3_xi1_an1_kang1",
- "安阳": "he2_nan2_an1_yang2",
- "安顺": "gui4_zhou1_an1_shun4",
- "定安县": "hai3_nan2_ding4_an1_xian4",
- "定西": "gan1_su4_ding4_xi1",
- "宜宾": "si4_chuan1_yi2_bin1",
- "宜昌": "hu2_bei3_yi2_chang1",
- "宜春": "jiang1_xi1_yi2_chun1",
- "å®é¸¡": "shan3_xi1_bao3_ji1",
- "宣城": "an1_hui1_xuan1_cheng2",
- "宿州": "an1_hui1_su4_zhou1",
- "宿è¿": "jiang1_su1_su4_qian1",
- "屯昌县": "hai3_nan2_tun2_chang1_xian4",
- "å±±å—": "xi1_cang2_shan1_nan2",
- "岳阳": "hu2_nan2_yue4_yang2",
- "崇左": "guang3_xi1_chong2_zuo3",
- "å·´ä¸": "si4_chuan1_ba1_zhong1",
- "巴彦淖尔": "nei4_meng2_gu3_ba1_yan4_nao4_er3",
- "å·´éŸ³éƒæ¥žè’™å¤è‡ªæ²»å·ž": "xin1_jiang1_ba1_yin1_guo1_leng2_meng2_gu3_zi4_zhi4_zhou1",
- "常州": "jiang1_su1_chang2_zhou1",
- "常德": "hu2_nan2_chang2_de2",
- "平凉": "gan1_su4_ping2_liang2",
- "平顶山": "he2_nan2_ping2_ding3_shan1",
- "广元": "si4_chuan1_guang3_yuan2",
- "广安": "si4_chuan1_guang3_an1",
- "广州": "guang3_dong1_guang3_zhou1",
- "庆阳": "gan1_su4_qing4_yang2",
- "廊åŠ": "he2_bei3_lang2_fang1",
- "延安": "shan3_xi1_yan2_an1",
- "å»¶è¾¹æœé²œæ—自治州": "ji2_lin2_yan2_bian1_zhao1_xian1_zu2_zi4_zhi4_zhou1",
- "å¼€å°": "he2_nan2_kai1_feng1",
- "å¼ å®¶å£": "he2_bei3_zhang1_jia1_kou3",
- "å¼ å®¶ç•Œ": "hu2_nan2_zhang1_jia1_jie4",
- "å¼ æŽ–": "gan1_su4_zhang1_ye4",
- "å¾å·ž": "jiang1_su1_xu2_zhou1",
- "å¾·å®å‚£æ—景颇æ—自治州": "yun2_nan2_de2_hong2_dai3_zu2_jing3_po3_zu2_zi4_zhi4_zhou1",
- "å¾·å·ž": "shan1_dong1_de2_zhou1",
- "德阳": "si4_chuan1_de2_yang2",
- "忻州": "shan1_xi1_xin1_zhou1",
- "怀化": "hu2_nan2_huai2_hua4",
- "怒江傈僳æ—自治州": "yun2_nan2_nu4_jiang1_li4_su4_zu2_zi4_zhi4_zhou1",
- "æ©æ–½åœŸå®¶æ—è‹—æ—自治州": "hu2_bei3_en1_shi1_tu3_jia1_zu2_miao2_zu2_zi4_zhi4_zhou1",
- "æƒ å·ž": "guang3_dong1_hui4_zhou1",
- "æˆéƒ½": "si4_chuan1_cheng2_du1",
- "扬州": "jiang1_su1_yang2_zhou1",
- "承德": "he2_bei3_cheng2_de2",
- "抚州": "jiang1_xi1_fu3_zhou1",
- "抚顺": "liao2_ning2_fu3_shun4",
- "拉è¨": "xi1_cang2_la1_sa4",
- "æé˜³": "guang3_dong1_jie1_yang2",
- "攀æžèб": "si4_chuan1_pan1_zhi1_hua1",
- "文山壮æ—è‹—æ—自治州": "yun2_nan2_wen2_shan1_zhuang4_zu2_miao2_zu2_zi4_zhi4_zhou1",
- "文昌": "hai3_nan2_wen2_chang1",
- "新乡": "he2_nan2_xin1_xiang1",
- "æ–°ä½™": "jiang1_xi1_xin1_yu2",
- "æ— é”¡": "jiang1_su1_wu2_xi2",
- "日喀则": "xi1_cang2_ri4_ka1_ze2",
- "日照": "shan1_dong1_ri4_zhao4",
- "昆明": "yun2_nan2_kun1_ming2",
- "昆玉": "xin1_jiang1_kun1_yu4",
- "昌å‰å›žæ—自治州": "xin1_jiang1_chang1_ji2_hui2_zu2_zi4_zhi4_zhou1",
- "昌江黎æ—自治县": "hai3_nan2_chang1_jiang1_li2_zu2_zi4_zhi4_xian4",
- "昌都": "xi1_cang2_chang1_du1",
- "æ˜é€š": "yun2_nan2_zhao1_tong1",
- "晋ä¸": "shan1_xi1_jin4_zhong1",
- "晋城": "shan1_xi1_jin4_cheng2",
- "普洱": "yun2_nan2_pu3_er3",
- "景德镇": "jiang1_xi1_jing3_de2_zhen4",
- "曲é–": "yun2_nan2_qu1_jing4",
- "朔州": "shan1_xi1_shuo4_zhou1",
- "æœé˜³": "liao2_ning2_zhao1_yang2",
- "本溪": "liao2_ning2_ben3_xi1",
- "æ¥å®¾": "guang3_xi1_lai2_bin1",
- "æå·ž": "zhe4_jiang1_hang2_zhou1",
- "æ¾åŽŸ": "ji2_lin2_song1_yuan2",
- "æž—èŠ": "xi1_cang2_lin2_zhi1",
- "æžœæ´›è—æ—自治州": "qing1_hai3_guo3_luo4_cang2_zu2_zi4_zhi4_zhou1",
- "枣庄": "shan1_dong1_zao3_zhuang1",
- "柳州": "guang3_xi1_liu3_zhou1",
- "æ ªæ´²": "hu2_nan2_zhu1_zhou1",
- "æ¡‚æž—": "guang3_xi1_gui4_lin2",
- "梅州": "guang3_dong1_mei2_zhou1",
- "梧州": "guang3_xi1_wu2_zhou1",
- "æ¥šé›„å½æ—自治州": "yun2_nan2_chu3_xiong2_yi2_zu2_zi4_zhi4_zhou1",
- "榆林": "shan3_xi1_yu2_lin2",
- "æ¦å¨": "gan1_su4_wu3_wei1",
- "æ¦æ±‰": "hu2_bei3_wu3_han4",
- "毕节": "gui4_zhou1_bi4_jie2",
- "永州": "hu2_nan2_yong3_zhou1",
- "汉ä¸": "shan3_xi1_han4_zhong1",
- "汕头": "guang3_dong1_shan4_tou2",
- "汕尾": "guang3_dong1_shan4_wei3",
- "江门": "guang3_dong1_jiang1_men2",
- "æ± å·ž": "an1_hui1_chi2_zhou1",
- "沈阳": "liao2_ning2_shen3_yang2",
- "æ²§å·ž": "he2_nan2_cang1_zhou1",
- "æ²³æ± ": "guang3_xi1_he2_chi2",
- "æ²³æº": "guang3_dong1_he2_yuan2",
- "泉州": "fu2_jian4_quan2_zhou1",
- "泰安": "shan1_dong1_tai4_an1",
- "æ³°å·ž": "jiang1_su1_tai4_zhou1",
- "泸州": "si4_chuan1_lu2_zhou1",
- "洛阳": "he2_nan2_luo4_yang2",
- "济å—": "shan1_dong1_ji4_nan2",
- "济å®": "shan1_dong1_ji4_ning2",
- "济æº": "he2_nan2_ji4_yuan2",
- "海东": "qing1_hai3_hai3_dong1",
- "æµ·åŒ—è—æ—自治州": "qing1_hai3_hai3_bei3_cang2_zu2_zi4_zhi4_zhou1",
- "æµ·å—è—æ—自治州": "qing1_hai3_hai3_nan2_cang2_zu2_zi4_zhi4_zhou1",
- "æµ·å£": "hai3_nan2_hai3_kou3",
- "æµ·è¥¿è’™å¤æ—è—æ—自治州": "qing1_hai3_hai3_xi1_meng2_gu3_zu2_cang2_zu2_zi4_zhi4_zhou1",
- "æ·„åš": "shan1_dong1_zi1_bo2",
- "淮北": "an1_hui1_huai2_bei3",
- "æ·®å—": "an1_hui1_huai2_nan2",
- "淮安": "jiang1_su1_huai2_an1",
- "深圳": "guang3_dong1_shen1_zhen4",
- "清远": "guang3_dong1_qing1_yuan3",
- "温州": "zhe4_jiang1_wen1_zhou1",
- "æ¸å—": "shan3_xi1_wei4_nan2",
- "æ¹–å·ž": "zhe4_jiang1_hu2_zhou1",
- "湘æ½": "hu2_nan2_xiang1_tan2",
- "湘西土家æ—è‹—æ—自治州": "hu2_nan2_xiang1_xi1_tu3_jia1_zu2_miao2_zu2_zi4_zhi4_zhou1",
- "湛江": "guang3_dong1_zhan4_jiang1",
- "æ»å·ž": "an1_hui1_chu2_zhou1",
- "滨州": "shan1_dong1_bin1_zhou1",
- "漯河": "he2_nan2_ta4_he2",
- "漳州": "fu2_jian4_zhang1_zhou1",
- "æ½åŠ": "shan1_dong1_wei2_fang1",
- "潜江": "hu2_bei3_qian2_jiang1",
- "潮州": "guang3_dong1_chao2_zhou1",
- "澄迈县": "hai3_nan2_cheng2_mai4_xian4",
- "澳门": "aomen",
- "濮阳": "he2_nan2_pu2_yang2",
- "烟å°": "shan1_dong1_yan1_tai2",
- "焦作": "he2_nan2_jiao1_zuo4",
- "牡丹江": "hei1_long2_jiang1_mu3_dan1_jiang1",
- "玉林": "guang3_xi1_yu4_lin2",
- "çŽ‰æ ‘è—æ—自治州": "qing1_hai3_yu4_shu4_cang2_zu2_zi4_zhi4_zhou1",
- "玉溪": "yun2_nan2_yu4_xi1",
- "ç æµ·": "guang3_dong1_zhu1_hai3",
- "ç¼ä¸é»Žæ—è‹—æ—自治县": "hai3_nan2_qiong2_zhong1_li2_zu2_miao2_zu2_zi4_zhi4_xian4",
- "ç¼æµ·": "hai3_nan2_qiong2_hai3",
- "甘å—è—æ—自治州": "gan1_su4_gan1_nan2_cang2_zu2_zi4_zhi4_zhou1",
- "甘åœè—æ—自治州": "si4_chuan1_gan1_zi1_cang2_zu2_zi4_zhi4_zhou1",
- "白城": "ji2_lin2_bai2_cheng2",
- "白山": "ji2_lin2_bai2_shan1",
- "白沙黎æ—自治县": "hai3_nan2_bai2_sha1_li2_zu2_zi4_zhi4_xian4",
- "白银": "gan1_su4_bai2_yin2",
- "百色": "guang3_xi1_bai3_se4",
- "益阳": "hu2_nan2_yi4_yang2",
- "ç›åŸŽ": "jiang1_su1_yan2_cheng2",
- "盘锦": "liao2_ning2_pan2_jin3",
- "眉山": "si4_chuan1_mei2_shan1",
- "石嘴山": "ning2_xia4_shi2_zui3_shan1",
- "石家庄": "he2_bei3_shi2_jia1_zhuang1",
- "石河å": "xin1_jiang1_shi2_he2_zi3",
- "神农架林区": "hu2_bei3_shen2_nong2_jia4_lin2_qu1",
- "ç¦å·ž": "fu2_jian4_fu2_zhou1",
- "秦皇岛": "he2_bei3_qin2_huang2_dao3",
- "红河哈尼æ—彿—自治州": "yun2_nan2_hong2_he2_ha1_ni2_zu2_yi2_zu2_zi4_zhi4_zhou1",
- "ç»å…´": "zhe4_jiang1_shao4_xing1",
- "绥化": "hei1_long2_jiang1_sui1_hua4",
- "绵阳": "si4_chuan1_mian2_yang2",
- "èŠåŸŽ": "shan1_dong1_liao2_cheng2",
- "肇庆": "guang3_dong1_zhao4_qing4",
- "自贡": "si4_chuan1_zi4_gong4",
- "舟山": "zhe4_jiang1_zhou1_shan1",
- "芜湖": "an1_hui1_wu2_hu2",
- "è‹å·ž": "jiang1_su1_su1_zhou1",
- "茂å": "guang3_dong1_mao4_ming2",
- "è†å·ž": "hu2_bei3_jing1_zhou1",
- "è†é—¨": "hu2_bei3_jing1_men2",
- "莆田": "fu2_jian4_fu3_tian2",
- "莱芜": "shan1_dong1_lai2_wu2",
- "èæ³½": "shan1_dong1_he2_ze2",
- "è乡": "jiang1_xi1_ping2_xiang1",
- "è¥å£": "liao2_ning2_ying2_kou3",
- "葫芦岛": "liao2_ning2_hu2_lu2_dao3",
- "èšŒåŸ ": "an1_hui1_bang4_bu4",
- "è¡¡æ°´": "he2_bei3_heng2_shui3",
- "衡阳": "hu2_nan2_heng2_yang2",
- "衢州": "zhe4_jiang1_qu2_zhou1",
- "襄阳": "hu2_bei3_xiang1_yang2",
- "西åŒç‰ˆçº³å‚£æ—自治州": "yun2_nan2_xi1_shuang1_ban3_na4_dai3_zu2_zi4_zhi4_zhou1",
- "西å®": "qing1_hai3_xi1_ning2",
- "西安": "shan3_xi1_xi1_an1",
- "许昌": "he2_nan2_xu3_chang1",
- "贵港": "guang3_xi1_gui4_gang3",
- "贵阳": "gui4_zhou1_gui4_yang2",
- "贺州": "guang3_xi1_he4_zhou1",
- "资阳": "si4_chuan1_zi1_yang2",
- "赣州": "jiang1_xi1_gan4_zhou1",
- "赤峰": "nei4_meng2_gu3_chi4_feng1",
- "è¾½æº": "ji2_lin2_liao2_yuan2",
- "辽阳": "liao2_ning2_liao2_yang2",
- "达州": "si4_chuan1_da2_zhou1",
- "è¿åŸŽ": "shan1_xi1_yun4_cheng2",
- "连云港": "jiang1_su1_lian2_yun2_gang3",
- "è¿ªåº†è—æ—自治州": "yun2_nan2_di2_qing4_cang2_zu2_zi4_zhi4_zhou1",
- "通化": "ji2_lin2_tong1_hua4",
- "通辽": "nei4_meng2_gu3_tong1_liao2",
- "é‚å®": "si4_chuan1_sui4_ning2",
- "éµä¹‰": "gui4_zhou1_zun1_yi4",
- "é‚¢å°": "he2_bei3_xing2_tai2",
- "那曲地区": "xi1_cang2_na4_qu1_di4_qu1",
- "邯郸": "he2_bei3_han2_dan1",
- "邵阳": "hu2_nan2_shao4_yang2",
- "郑州": "he2_nan2_zheng4_zhou1",
- "郴州": "hu2_nan2_chen1_zhou1",
- "鄂尔多斯": "nei4_meng2_gu3_e4_er3_duo1_si1",
- "é„‚å·ž": "hu2_bei3_e4_zhou1",
- "酒泉": "gan1_su4_jiu3_quan2",
- "é‡åº†": "chongqing",
- "金åŽ": "zhe4_jiang1_jin1_hua2",
- "金昌": "gan1_su4_jin1_chang1",
- "钦州": "guang3_xi1_qin1_zhou1",
- "é“å²": "liao2_ning2_tie3_ling2",
- "é“门关": "xin1_jiang1_tie3_men2_guan1",
- "铜ä»": "gui4_zhou1_tong2_ren2",
- "铜å·": "shan3_xi1_tong2_chuan1",
- "铜陵": "an1_hui1_tong2_ling2",
- "é“¶å·": "ning2_xia4_yin2_chuan1",
- "锡林éƒå‹’盟": "nei4_meng2_gu3_xi2_lin2_guo1_le4_meng2",
- "锦州": "liao2_ning2_jin3_zhou1",
- "镇江": "jiang1_su1_zhen4_jiang1",
- "长春": "ji2_lin2_chang2_chun1",
- "é•¿æ²™": "hu2_nan2_chang2_sha1",
- "é•¿æ²»": "shan1_xi1_chang2_zhi4",
- "阜新": "liao2_ning2_fu4_xin1",
- "阜阳": "an1_hui1_fu4_yang2",
- "防城港": "guang3_xi1_fang2_cheng2_gang3",
- "阳江": "guang3_dong1_yang2_jiang1",
- "阳泉": "shan1_xi1_yang2_quan2",
- "阿克è‹åœ°åŒº": "xin1_jiang1_a1_ke4_su1_di4_qu1",
- "阿勒泰地区": "xin1_jiang1_a1_le4_tai4_di4_qu1",
- "阿åè—æ—羌æ—自治州": "si4_chuan1_a1_ba4_cang2_zu2_qiang1_zu2_zi4_zhi4_zhou1",
- "阿拉善盟": "nei4_meng2_gu3_a1_la1_shan4_meng2",
- "阿拉尔": "xin1_jiang1_a1_la1_er3",
- "阿里地区": "xi1_cang2_a1_li3_di4_qu1",
- "陇å—": "gan1_su4_long3_nan2",
- "陵水黎æ—自治县": "hai3_nan2_ling2_shui3_li2_zu2_zi4_zhi4_xian4",
- "éšå·ž": "hu2_bei3_sui2_zhou1",
- "雅安": "si4_chuan1_ya3_an1",
- "é’å²›": "shan1_dong1_qing1_dao3",
- "éžå±±": "liao2_ning2_an1_shan1",
- "韶关": "guang3_dong1_shao2_guan1",
- "香港": "xianggang",
- "马éžå±±": "an1_hui1_ma3_an1_shan1",
- "驻马店": "he2_nan2_zhu4_ma3_dian4",
- "鸡西": "hei1_long2_jiang1_ji1_xi1",
- "鹤å£": "he2_nan2_he4_bi4",
- "鹤岗": "hei1_long2_jiang1_he4_gang3",
- "é¹°æ½": "jiang1_xi1_ying1_tan2",
- "黄冈": "hu2_bei3_huang2_gang1",
- "黄å—è—æ—自治州": "qing1_hai3_huang2_nan2_cang2_zu2_zi4_zhi4_zhou1",
- "黄山": "an1_hui1_huang2_shan1",
- "黄石": "hu2_bei3_huang2_shi2",
- "黑河": "hei1_long2_jiang1_hei1_he2",
- "黔东å—è‹—æ—ä¾—æ—自治州": "gui4_zhou1_qian2_dong1_nan2_miao2_zu2_tong1_zu2_zi4_zhi4_zhou1",
- "é»”å—å¸ƒä¾æ—è‹—æ—自治州": "gui4_zhou1_qian2_nan2_bu4_yi1_zu2_miao2_zu2_zi4_zhi4_zhou1",
- "黔西å—å¸ƒä¾æ—è‹—æ—自治州": "gui4_zhou1_qian2_xi1_nan2_bu4_yi1_zu2_miao2_zu2_zi4_zhi4_zhou1",
- "é½é½å“ˆå°”": "hei1_long2_jiang1_qi2_qi2_ha1_er3",
- "龙岩": "fu2_jian4_long2_yan2",
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/opts/charts.go b/vendor/github.com/go-echarts/go-echarts/v2/opts/charts.go
deleted file mode 100644
index 5e9b06e5..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/opts/charts.go
+++ /dev/null
@@ -1,698 +0,0 @@
-package opts
-
-// BarChart
-// https://echarts.apache.org/en/option.html#series-bar
-type BarChart struct {
- Type string
- // Name of stack. On the same category axis, the series with the
- // same stack name would be put on top of each other.
- Stack string
-
- // The gap between bars between different series, is a percent value like '30%',
- // which means 30% of the bar width.
- // Set barGap as '-100%' can overlap bars that belong to different series,
- // which is useful when putting a series of bar as background.
- // In a single coordinate system, this attribute is shared by multiple 'bar' series.
- // This attribute should be set on the last 'bar' series in the coordinate system,
- // then it will be adopted by all 'bar' series in the coordinate system.
- BarGap string
-
- // The bar gap of a single series, defaults to be 20% of the category gap,
- // can be set as a fixed value.
- // In a single coordinate system, this attribute is shared by multiple 'bar' series.
- // This attribute should be set on the last 'bar' series in the coordinate system,
- // then it will be adopted by all 'bar' series in the coordinate system.
- BarCategoryGap string
-
- // Index of x axis to combine with, which is useful for multiple x axes in one chart.
- XAxisIndex int
-
- // Index of y axis to combine with, which is useful for multiple y axes in one chart.
- YAxisIndex int
-
- ShowBackground bool
- RoundCap bool
- CoordSystem string
-}
-
-// SunburstChart
-// https://echarts.apache.org/en/option.html#series-sunburst
-type SunburstChart struct {
- // The action of clicking a sector
- NodeClick string `json:"nodeClick,omitempty"`
- // Sorting method that sectors use based on value
- Sort string `json:"sort,omitempty"`
- // If there is no name, whether need to render it.
- RenderLabelForZeroData bool `json:"renderLabelForZeroData"`
- // Selected mode
- SelectedMode bool `json:"selectedMode"`
- // Whether to enable animation.
- Animation bool `json:"animation"`
- // Whether to set graphic number threshold to animation
- AnimationThreshold int `json:"animationThreshold,omitempty"`
- // Duration of the first animation
- AnimationDuration int `json:"animationDuration,omitempty"`
- // Easing method used for the first animation
- AnimationEasing string `json:"animationEasing,omitempty"`
- // Delay before updating the first animation
- AnimationDelay int `json:"animationDelay,omitempty"`
- // Time for animation to complete
- AnimationDurationUpdate int `json:"animationDurationUpdate,omitempty"`
- // Easing method used for animation.
- AnimationEasingUpdate string `json:"animationEasingUpdate,omitempty"`
- // Delay before updating animation
- AnimationDelayUpdate int `json:"animationDelayUpdate,omitempty"`
-}
-
-// BarData
-// https://echarts.apache.org/en/option.html#series-bar.data
-type BarData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-
- // The style setting of the text label in a single bar.
- Label *Label `json:"label,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // Tooltip settings in this series data.
- Tooltip *Tooltip `json:"tooltip,omitempty"`
-}
-
-// Bar3DChart is the option set for a 3D bar chart.
-type Bar3DChart struct {
- // Shading is the coloring effect of 3D graphics in 3D Bar.
- // The following three coloring methods are supported in echarts-gl:
- // Options:
- //
- // * "color": Only display colors, not affected by other factors such as lighting.
- // * "lambert": Through the classic [lambert] coloring, can express the light and dark that the light shows.
- // * "realistic": Realistic rendering, combined with light.ambientCubemap and postEffect,
- // can improve the quality and texture of the display.
- // [Physical Based Rendering (PBR)] (https://www.marmoset.co/posts/physically-based-rendering-and-you-can-too/)
- // is used in ECharts GL to represent realistic materials.
- Shading string
-}
-
-// BoxPlotData
-// https://echarts.apache.org/en/option.html#series-boxplot.data
-type BoxPlotData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-
- // The style setting of the text label in a single bar.
- Label *Label `json:"label,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // Emphasis settings in this series data.
- Emphasis *Emphasis `json:"emphasis,omitempty"`
-
- // Tooltip settings in this series data.
- Tooltip *Tooltip `json:"tooltip,omitempty"`
-}
-
-// EffectScatterData
-// https://echarts.apache.org/en/option.html#series-effectScatter.data
-type EffectScatterData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// FunnelData
-// https://echarts.apache.org/en/option.html#series-funnel.data
-type FunnelData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// GeoData
-type GeoData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// GaugeData
-// https://echarts.apache.org/en/option.html#series-gauge.data
-type GaugeData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// GraphChart is the option set for graph chart.
-// https://echarts.apache.org/en/option.html#series-graph
-type GraphChart struct {
- // Graph layout.
- // * 'none' No layout, use x, y provided in node as the position of node.
- // * 'circular' Adopt circular layout, see the example Les Miserables.
- // * 'force' Adopt force-directed layout, see the example Force, the
- // detail about layout configurations are in graph.force
- Layout string
-
- // Force is the option set for graph force layout.
- Force *GraphForce
-
- // Whether to enable mouse zooming and translating. false by default.
- // If either zooming or translating is wanted, it can be set to 'scale' or 'move'.
- // Otherwise, set it to be true to enable both.
- Roam bool
-
- // EdgeSymbol is the symbols of two ends of edge line.
- // * 'circle'
- // * 'arrow'
- // * 'none'
- // example: ["circle", "arrow"] or "circle"
- EdgeSymbol interface{}
-
- // EdgeSymbolSize is size of symbol of two ends of edge line. Can be an array or a single number
- // example: [5,10] or 5
- EdgeSymbolSize interface{}
-
- // Draggable allows you to move the nodes with the mouse if they are not fixed.
- Draggable bool
-
- // Whether to focus/highlight the hover node and it's adjacencies.
- FocusNodeAdjacency bool
-
- // The categories of node, which is optional. If there is a classification of nodes,
- // the category of each node can be assigned through data[i].category.
- // And the style of category will also be applied to the style of nodes. categories can also be used in legend.
- Categories []*GraphCategory
-
- // EdgeLabel is the properties of an label of edge.
- EdgeLabel *EdgeLabel `json:"edgeLabel"`
-}
-
-// GraphNode represents a data node in graph chart.
-// https://echarts.apache.org/en/option.html#series-graph.data
-type GraphNode struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // x value of node position.
- X float32 `json:"x,omitempty"`
-
- // y value of node position.
- Y float32 `json:"y,omitempty"`
-
- // Value of data item.
- Value float32 `json:"value,omitempty"`
-
- // If node are fixed when doing force directed layout.
- Fixed bool `json:"fixed,omitempty"`
-
- // Index of category which the data item belongs to.
- Category interface{} `json:"category,omitempty"`
-
- // Symbol of node of this category.
- // Icon types provided by ECharts includes
- // 'circle', 'rect', 'roundRect', 'triangle', 'diamond', 'pin', 'arrow', 'none'
- // It can be set to an image with 'image://url' , in which URL is the link to an image, or dataURI of an image.
- Symbol string `json:"symbol,omitempty"`
-
- // node of this category symbol size. It can be set to single numbers like 10,
- // or use an array to represent width and height. For example, [20, 10] means symbol width is 20, and height is10.
- SymbolSize interface{} `json:"symbolSize,omitempty"`
-
- // The style of this node.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// GraphLink represents relationship between two data nodes.
-// https://echarts.apache.org/en/option.html#series-graph.links
-type GraphLink struct {
- // A string representing the name of source node on edge. Can also be a number representing the node index.
- Source interface{} `json:"source,omitempty"`
-
- // A string representing the name of target node on edge. Can also be a number representing node index.
- Target interface{} `json:"target,omitempty"`
-
- // value of edge, can be mapped to edge length in force graph.
- Value float32 `json:"value,omitempty"`
-
- // Label for this link.
- Label *EdgeLabel `json:"label,omitempty"`
-}
-
-// GraphCategory represents a category for data nodes.
-// The categories of node, which is optional. If there is a classification of nodes,
-// the category of each node can be assigned through data[i].category.
-// And the style of category will also be applied to the style of nodes. categories can also be used in legend.
-// https://echarts.apache.org/en/option.html#series-graph.categories
-type GraphCategory struct {
- // Name of category, which is used to correspond with legend and the content of tooltip.
- Name string `json:"name"`
-
- // The label style of node in this category.
- Label *Label `json:"label,omitempty"`
-}
-
-// HeatMapChart is the option set for a heatmap chart.
-// https://echarts.apache.org/en/option.html#series-heatmap
-type HeatMapChart struct {
- // Index of x axis to combine with, which is useful for multiple x axes in one chart.
- XAxisIndex int
-
- // Index of y axis to combine with, which is useful for multiple y axes in one chart.
- YAxisIndex int
-}
-
-// HeatMapData
-// https://echarts.apache.org/en/option.html#series-heatmap.data
-type HeatMapData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// KlineData
-// https://echarts.apache.org/en/option.html#series-candlestick.data
-type KlineData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// LineChart is the options set for a line chart.
-// https://echarts.apache.org/en/option.html#series-line
-type LineChart struct {
- // If stack the value. On the same category axis, the series with the same stack name would be put on top of each other.
- // The effect of the below example could be seen through stack switching of toolbox on the top right corner:
- Stack string
-
- // Whether to show as smooth curve.
- // If is typed in boolean, then it means whether to enable smoothing. If is
- // typed in number, valued from 0 to 1, then it means smoothness. A smaller value makes it less smooth.
- Smooth bool
-
- // Whether to show as a step line. It can be true, false. Or 'start', 'middle', 'end'.
- // Which will configure the turn point of step line.
- Step interface{}
-
- // Index of x axis to combine with, which is useful for multiple x axes in one chart.
- XAxisIndex int
-
- // Index of y axis to combine with, which is useful for multiple y axes in one chart.
- YAxisIndex int
-
- // Whether to connect the line across null points.
- ConnectNulls bool
-
- // Whether to show symbol. It would be shown during tooltip hover.
- ShowSymbol bool
-}
-
-// LineData
-// https://echarts.apache.org/en/option.html#series-line.data
-type LineData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-
- // Symbol of single data.
- // Icon types provided by ECharts includes 'circle', 'rect', 'roundRect', 'triangle', 'diamond', 'pin', 'arrow', 'none'
- // It can be set to an image with 'image://url' , in which URL is the link to an image, or dataURI of an image.
- Symbol string `json:"symbol,omitempty"`
-
- // single data symbol size. It can be set to single numbers like 10, or
- // use an array to represent width and height. For example, [20, 10] means symbol width is 20, and height is10
- SymbolSize int `json:"symbolSize,omitempty"`
-
- // Index of x axis to combine with, which is useful for multiple x axes in one chart.
- XAxisIndex int
-
- // Index of y axis to combine with, which is useful for multiple y axes in one chart.
- YAxisIndex int
-}
-
-// LiquidChart
-// reference https://github.com/ecomfe/echarts-liquidfill
-type LiquidChart struct {
- // Shape of single data.
- // Icon types provided by ECharts includes 'circle', 'rect', 'roundRect', 'triangle', 'diamond', 'pin', 'arrow', 'none'
- // It can be set to an image with 'image://url' , in which URL is the link to an image, or dataURI of an image.
- Shape string
-
- // Whether to show outline
- IsShowOutline bool
-
- // Whether to stop animation
- IsWaveAnimation bool
-}
-
-// LiquidData
-// reference https://github.com/ecomfe/echarts-liquidfill
-type LiquidData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// MapData
-// https://echarts.apache.org/en/option.html#series-map.data
-type MapData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// ParallelData
-// https://echarts.apache.org/en/option.html#series-parallel.data
-type ParallelData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// PieChart is the option set for a pie chart.
-// https://echarts.apache.org/en/option.html#series-pie
-type PieChart struct {
- // Whether to show as Nightingale chart, which distinguishes data through radius. There are 2 optional modes:
- // * 'radius' Use central angle to show the percentage of data, radius to show data size.
- // * 'area' All the sectors will share the same central angle, the data size is shown only through radiuses.
- RoseType string
-
- // Center position of Pie chart, the first of which is the horizontal position, and the second is the vertical position.
- // Percentage is supported. When set in percentage, the item is relative to the container width,
- // and the second item to the height.
- //
- // Example:
- //
- // Set to absolute pixel values ->> center: [400, 300]
- // Set to relative percent ->> center: ['50%', '50%']
- Center interface{}
-
- // Radius of Pie chart. Value can be:
- // * number: Specify outside radius directly.
- // * string: For example, '20%', means that the outside radius is 20% of the viewport
- // size (the little one between width and height of the chart container).
- //
- // Array.: The first item specifies the inside radius, and the
- // second item specifies the outside radius. Each item follows the definitions above.
- Radius interface{}
-}
-
-// PieData
-// https://echarts.apache.org/en/option.html#series-pie.data
-type PieData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-
- // Whether the data item is selected.
- Selected bool `json:"selected,omitempty"`
-
- // The label configuration of a single sector.
- Label *Label `json:"label,omitempty"`
-
- // Graphic style of , emphasis is the style when it is highlighted, like being hovered by mouse, or highlighted via legend connect.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // tooltip settings in this series data.
- Tooltip *Tooltip `json:"tooltip,omitempty"`
-}
-
-// RadarData
-// https://echarts.apache.org/en/option.html#series-radar
-type RadarData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-// SankeyLink represents relationship between two data nodes.
-// https://echarts.apache.org/en/option.html#series-sankey.links
-type SankeyLink struct {
- // The name of source node of edge
- Source interface{} `json:"source,omitempty"`
-
- // The name of target node of edge
- Target interface{} `json:"target,omitempty"`
-
- // The value of edge, which decides the width of edge.
- Value float32 `json:"value,omitempty"`
-}
-
-// SankeyNode represents a data node.
-// https://echarts.apache.org/en/option.html#series-sankey.nodes
-type SankeyNode struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value string `json:"value,omitempty"`
-
- // Depth of the node within the chart
- Depth *int `json:"depth,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// ScatterChart is the option set for a scatter chart.
-// https://echarts.apache.org/en/option.html#series-scatter
-type ScatterChart struct {
- // Index of x axis to combine with, which is useful for multiple x axes in one chart.
- XAxisIndex int
-
- // Index of x axis to combine with, which is useful for multiple y axes in one chart.
- YAxisIndex int
-}
-
-// ScatterData
-// https://echarts.apache.org/en/option.html#series-scatter.data
-type ScatterData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-
- // Symbol
- Symbol string `json:"symbol,omitempty"`
-
- // SymbolSize
- SymbolSize int `json:"symbolSize,omitempty"`
-
- // SymbolRotate
- SymbolRotate int `json:"symbolRotate,omitempty"`
-
- // Index of x axis to combine with, which is useful for multiple x axes in one chart.
- XAxisIndex int `json:"xAxisIndex,omitempty"`
-
- // Index of y axis to combine with, which is useful for multiple y axes in one chart.
- YAxisIndex int `json:"yAxisIndex,omitempty"`
-}
-
-// ThemeRiverData
-// https://echarts.apache.org/en/option.html#series-themeRiver
-type ThemeRiverData struct {
- // the time attribute of time and theme.
- Date string `json:"date,omitempty"`
-
- // the value of an event or theme at a time point.
- Value float64 `json:"value,omitempty"`
-
- // the name of an event or theme.
- Name string `json:"name,omitempty"`
-}
-
-// ToList converts the themeriver data to a list
-func (trd ThemeRiverData) ToList() [3]interface{} {
- return [3]interface{}{trd.Date, trd.Value, trd.Name}
-}
-
-// WordCloudChart is the option set for a word cloud chart.
-type WordCloudChart struct {
- // Shape of WordCloud
- // Optional: "circle", "rect", "roundRect", "triangle", "diamond", "pin", "arrow"
- Shape string
-
- // range of font size
- SizeRange []float32
-
- // range of font rotation angle
- RotationRange []float32
-}
-
-// WordCloudData
-type WordCloudData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
-
- // Value of a single data item.
- Value interface{} `json:"value,omitempty"`
-}
-
-type Chart3DData struct {
- // Name of the data item.
- Name string `json:"name,omitempty"`
-
- // Value of the data item.
- // []interface{}{1, 2, 3}
- Value []interface{} `json:"value,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // The style setting of the text label in a single bar.
- Label *Label `json:"label,omitempty"`
-}
-
-type TreeChart struct {
- // The layout of the tree, which can be orthogonal and radial.
- // * 'orthogonal' refer to the horizontal and vertical direction.
- // * 'radial' refers to the view that the root node as the center and each layer of nodes as the ring.
- Layout string
-
- // The direction of the orthogonal layout in the tree diagram.
- // * 'from left to right' or 'LR'
- // * 'from right to left' or 'RL'
- // * 'from top to bottom' or 'TB'
- // * 'from bottom to top' or 'BT'
- Orient string `json:"orient,omitempty"`
-
- // Whether to enable mouse zooming and translating. false by default.
- // If either zooming or translating is wanted, it can be set to 'scale' or 'move'.
- // Otherwise, set it to be true to enable both.
- Roam bool `json:"roam"`
-
- // Subtree collapses and expands interaction, default true.
- ExpandAndCollapse bool `json:"expandAndCollapse,omitempty"`
-
- // The initial level (depth) of the tree. The root node is the 0th layer, then the first layer, the second layer, ... , until the leaf node.
- // This configuration item is primarily used in conjunction with collapsing and expansion interactions.
- // The purpose is to prevent the nodes from obscuring each other. If set as -1 or null or undefined, all nodes are expanded.
- InitialTreeDepth int `json:"initialTreeDepth,omitempty"`
-
- // The style setting of the text label in a single bar.
- Label *Label `json:"label,omitempty"`
-
- // Leaf node special configuration, the leaf node and non-leaf node label location is different.
- Leaves *TreeLeaves `json:"leaves,omitempty"`
-
- // Distance between tree component and the sides of the container.
- // value can be instant pixel value like 20;
- // It can also be a percentage value relative to container width like '20%';
- Left string `json:"left,omitempty"`
- Right string `json:"right,omitempty"`
- Top string `json:"top,omitempty"`
- Bottom string `json:"bottom,omitempty"`
-}
-
-type TreeData struct {
- // Name of the data item.
- Name string `json:"name,omitempty"`
-
- // Value of the data item.
- Value int `json:"value,omitempty"`
-
- Children []*TreeData `json:"children,omitempty"`
-
- // Symbol of node of this category.
- // Icon types provided by ECharts includes
- // 'circle', 'rect', 'roundRect', 'triangle', 'diamond', 'pin', 'arrow', 'none'
- // It can be set to an image with 'image://url' , in which URL is the link to an image, or dataURI of an image.
- Symbol string `json:"symbol,omitempty"`
-
- // node of this category symbol size. It can be set to single numbers like 10,
- // or use an array to represent width and height. For example, [20, 10] means symbol width is 20, and height is10.
- SymbolSize interface{} `json:"symbolSize,omitempty"`
-
- // If set as `true`, the node is collapsed in the initialization.
- Collapsed bool `json:"collapsed,omitempty"`
-
- // LineStyle settings in this series data.
- LineStyle *LineStyle `json:"lineStyle,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-type TreeMapChart struct {
- // Whether to enable animation.
- Animation bool `json:"animation"`
-
- // leafDepth represents how many levels are shown at most. For example, when leafDepth is set to 1, only one level will be shown.
- // leafDepth is null/undefined by default, which means that "drill down" is disabled.
- LeafDepth int `json:"leafDeapth,omitempty"`
-
- // Roam describes whether to enable mouse zooming and translating. false by default.
- Roam bool `json:"roam"`
-
- // Label decribes the style of the label in each node.
- Label *Label `json:"label,omitempty"`
-
- // UpperLabel is used to specify whether show label when the treemap node has children.
- UpperLabel *UpperLabel `json:"upperLabel,omitempty"`
-
- // ColorMappingBy specifies the rule according to which each node obtain color from color list.
- ColorMappingBy string `json:"colorMappingBy,omitempty"`
-
- // Levels provide configration for each node level
- Levels *[]TreeMapLevel `json:"levels,omitempty"`
-
- // Distance between treemap component and the sides of the container.
- // value can be instant pixel value like 20;
- // It can also be a percentage value relative to container width like '20%';
- Left string `json:"left,omitempty"`
- Right string `json:"right,omitempty"`
- Top string `json:"top,omitempty"`
- Bottom string `json:"bottom,omitempty"`
-}
-
-type TreeMapNode struct {
- // Name of the tree node item.
- Name string `json:"name"`
-
- // Value of the tree node item.
- Value int `json:"value,omitempty"`
-
- Children []TreeMapNode `json:"children,omitempty"`
-}
-
-// SunBurstData data
-type SunBurstData struct {
- // Name of data item.
- Name string `json:"name,omitempty"`
- // Value of data item.
- Value float64 `json:"value,omitempty"`
- // sub item of data item
- Children []*SunBurstData `json:"children,omitempty"`
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/opts/global.go b/vendor/github.com/go-echarts/go-echarts/v2/opts/global.go
deleted file mode 100644
index aafb5ee6..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/opts/global.go
+++ /dev/null
@@ -1,1429 +0,0 @@
-package opts
-
-import (
- "fmt"
- "math/rand"
- "reflect"
- "regexp"
- "strconv"
- "strings"
- "time"
-
- "github.com/go-echarts/go-echarts/v2/types"
-)
-
-func init() {
- rand.Seed(time.Now().UnixNano())
-}
-
-// Initialization contains options for the canvas.
-type Initialization struct {
- // HTML title
- PageTitle string `default:"Awesome go-echarts"`
-
- // Width of canvas
- Width string `default:"900px"`
-
- // Height of canvas
- Height string `default:"500px"`
-
- // BackgroundColor of canvas
- BackgroundColor string
-
- // Chart unique ID
- ChartID string
-
- // Assets host
- AssetsHost string `default:"https://go-echarts.github.io/go-echarts-assets/assets/"`
-
- // Theme of chart
- Theme string `default:"white"`
-}
-
-// Validate validates the initialization configurations.
-func (opt *Initialization) Validate() {
- setDefaultValue(opt)
- if opt.ChartID == "" {
- opt.ChartID = generateUniqueID()
- }
-}
-
-// set default values for the struct field.
-// origin from: https://github.com/mcuadros/go-defaults
-func setDefaultValue(ptr interface{}) {
- elem := reflect.ValueOf(ptr).Elem()
- t := elem.Type()
-
- for i := 0; i < t.NumField(); i++ {
- // handle `default` tag only
- if defaultVal := t.Field(i).Tag.Get("default"); defaultVal != "" {
- setField(elem.Field(i), defaultVal)
- }
- }
-}
-
-// setField handles String/Bool types only.
-func setField(field reflect.Value, defaultVal string) {
- switch field.Kind() {
- case reflect.String:
- if field.String() == "" {
- field.Set(reflect.ValueOf(defaultVal).Convert(field.Type()))
- }
- case reflect.Bool:
- if val, err := strconv.ParseBool(defaultVal); err == nil {
- field.Set(reflect.ValueOf(val).Convert(field.Type()))
- }
- }
-}
-
-const (
- chartIDSize = 12
-)
-
-// generate the unique ID for each chart.
-func generateUniqueID() string {
- var b [chartIDSize]byte
- for i := range b {
- b[i] = randByte()
- }
- return string(b[:])
-}
-
-func randByte() byte {
- c := 65 // A
- if rand.Intn(10) > 5 {
- c = 97 // a
- }
- return byte(c + rand.Intn(26))
-}
-
-// Title is the option set for a title component.
-// https://echarts.apache.org/en/option.html#title
-type Title struct {
- // The main title text, supporting \n for newlines.
- Title string `json:"text,omitempty"`
-
- // TextStyle of the main title.
- TitleStyle *TextStyle `json:"textStyle,omitempty"`
-
- // The hyper link of main title text.
- Link string `json:"link,omitempty"`
-
- // Subtitle text, supporting \n for newlines.
- Subtitle string `json:"subtext,omitempty"`
-
- // TextStyle of the sub title.
- SubtitleStyle *TextStyle `json:"subtextStyle,omitempty"`
-
- // The hyper link of sub title text.
- SubLink string `json:"sublink,omitempty"`
-
- // Open the hyper link of main title in specified tab.
- // options:
- // 'self' opening it in current tab
- // 'blank' opening it in a new tab
- Target string `json:"target,omitempty"`
-
- // Distance between title component and the top side of the container.
- // top value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'top', 'middle', or 'bottom'.
- // If the left value is set to be 'top', 'middle', or 'bottom',
- // then the component will be aligned automatically based on position.
- Top string `json:"top,omitempty"`
-
- // Distance between title component and the bottom side of the container.
- // bottom value can be instant pixel value like 20;
- // it can also be a percentage value relative to container width like '20%'.
- // Adaptive by default.
- Bottom string `json:"bottom,omitempty"`
-
- // Distance between title component and the left side of the container.
- // left value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'left', 'center', or 'right'.
- // If the left value is set to be 'left', 'center', or 'right',
- // then the component will be aligned automatically based on position.
- Left string `json:"left,omitempty"`
-
- // Distance between title component and the right side of the container.
- // right value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- // Adaptive by default.
- Right string `json:"right,omitempty"`
-}
-
-// Legend is the option set for a legend component.
-// Legend component shows symbol, color and name of different series. You can click legends to toggle displaying series in the chart.
-// https://echarts.apache.org/en/option.html#legend
-type Legend struct {
- // Whether to show the Legend, default true.
- Show bool `json:"show"`
-
- // Type of legend. Optional values:
- // "plain": Simple legend. (default)
- // "scroll": Scrollable legend. It helps when too many legend items needed to be shown.
- Type string `json:"type"`
-
- // Distance between legend component and the left side of the container.
- // left value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'left', 'center', or 'right'.
- // If the left value is set to be 'left', 'center', or 'right', then the component
- // will be aligned automatically based on position.
- Left string `json:"left,omitempty"`
-
- // Distance between legend component and the top side of the container.
- // top value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'top', 'middle', or 'bottom'.
- // If the left value is set to be 'top', 'middle', or 'bottom', then the component
- // will be aligned automatically based on position.
- Top string `json:"top,omitempty"`
-
- // Distance between legend component and the right side of the container.
- // right value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- // Adaptive by default.
- Right string `json:"right,omitempty"`
-
- // Distance between legend component and the bottom side of the container.
- // bottom value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- // Adaptive by default.
- Bottom string `json:"bottom,omitempty"`
-
- // Data array of legend. An array item is usually a name representing string.
- // set Data as []string{} if you wants to hide the legend.
- Data interface{} `json:"data,omitempty"`
-
- // The layout orientation of legend.
- // Options: 'horizontal', 'vertical'
- Orient string `json:"orient,omitempty"`
-
- // Legend color when not selected.
- InactiveColor string `json:"inactiveColor,omitempty"`
-
- // State table of selected legend.
- // example:
- // var selected = map[string]bool{}
- // selected["series1"] = true
- // selected["series2"] = false
- Selected map[string]bool `json:"selected,omitempty"`
-
- // Selected mode of legend, which controls whether series can be toggled displaying by clicking legends.
- // It is enabled by default, and you may set it to be false to disabled it.
- // Besides, it can be set to 'single' or 'multiple', for single selection and multiple selection.
- SelectedMode string `json:"selectedMode,omitempty"`
-
- // Legend space around content. The unit is px.
- // Default values for each position are 5.
- // And they can be set to different values with left, right, top, and bottom.
- // Examples:
- // 1. Set padding to be 5
- // padding: 5
- // 2. Set the top and bottom paddings to be 5, and left and right paddings to be 10
- // padding: [5, 10]
- // 3. Set each of the four paddings separately
- // padding: [
- // 5, // up
- // 10, // right
- // 5, // down
- // 10, // left
- // ]
- Padding interface{} `json:"padding,omitempty"`
-
- // Image width of legend symbol.
- ItemWidth int `json:"itemWidth,omitempty"`
-
- // Image height of legend symbol.
- ItemHeight int `json:"itemHeight,omitempty"`
-
- // Legend X position, right/left/center
- X string `json:"x,omitempty"`
-
- // Legend Y position, right/left/center
- Y string `json:"y,omitempty"`
-
- // Width of legend component. Adaptive by default.
- Width string `json:"width,omitempty"`
-
- // Height of legend component. Adaptive by default.
- Height string `json:"height,omitempty"`
-
- // Legend marker and text aligning.
- // By default, it automatically calculates from component location and orientation.
- // When left value of this component is 'right' and orient is 'vertical', it would be aligned to 'right'.
- // Options: auto/left/right
- Align string `json:"align,omitempty"`
-
- // Legend text style.
- TextStyle *TextStyle `json:"textStyle,omitempty"`
-}
-
-// Tooltip is the option set for a tooltip component.
-// https://echarts.apache.org/en/option.html#tooltip
-type Tooltip struct {
- // Whether to show the tooltip component, including tooltip floating layer and axisPointer.
- Show bool `json:"show"`
-
- // Type of triggering.
- // Options:
- // * 'item': Triggered by data item, which is mainly used for charts that
- // don't have a category axis like scatter charts or pie charts.
- // * 'axis': Triggered by axes, which is mainly used for charts that have category axes,
- // like bar charts or line charts.
- // * 'none': Trigger nothing.
- Trigger string `json:"trigger,omitempty"`
-
- // Conditions to trigger tooltip. Options:
- // * 'mousemove': Trigger when mouse moves.
- // * 'click': Trigger when mouse clicks.
- // * 'mousemove|click': Trigger when mouse clicks and moves.
- // * 'none': Do not triggered by 'mousemove' and 'click'. Tooltip can be triggered and hidden
- // manually by calling action.tooltip.showTip and action.tooltip.hideTip.
- // It can also be triggered by axisPointer.handle in this case.
- TriggerOn string `json:"triggerOn,omitempty"`
-
- // Whether mouse is allowed to enter the floating layer of tooltip, whose default value is false.
- // If you need to interact in the tooltip like with links or buttons, it can be set as true.
- Enterable bool `json:"enterable,omitempty"`
-
- // The content formatter of tooltip's floating layer which supports string template and callback function.
- //
- // 1. String template
- // The template variables are {a}, {b}, {c}, {d} and {e}, which stands for series name,
- // data name and data value and ect. When trigger is set to be 'axis', there may be data from multiple series.
- // In this time, series index can be refereed as {a0}, {a1}, or {a2}.
- // {a}, {b}, {c}, {d} have different meanings for different series types:
- //
- // * Line (area) charts, bar (column) charts, K charts: {a} for series name,
- // {b} for category name, {c} for data value, {d} for none;
- // * Scatter (bubble) charts: {a} for series name, {b} for data name, {c} for data value, {d} for none;
- // * Map: {a} for series name, {b} for area name, {c} for merging data, {d} for none;
- // * Pie charts, gauge charts, funnel charts: {a} for series name, {b} for data item name,
- // {c} for data value, {d} for percentage.
- //
- // 2. Callback function
- // The format of callback function:
- // (params: Object|Array, ticket: string, callback: (ticket: string, html: string)) => string
- // The first parameter params is the data that the formatter needs. Its format is shown as follows:
- // {
- // componentType: 'series',
- // // Series type
- // seriesType: string,
- // // Series index in option.series
- // seriesIndex: number,
- // // Series name
- // seriesName: string,
- // // Data name, or category name
- // name: string,
- // // Data index in input data array
- // dataIndex: number,
- // // Original data as input
- // data: Object,
- // // Value of data. In most series it is the same as data.
- // // But in some series it is some part of the data (e.g., in map, radar)
- // value: number|Array|Object,
- // // encoding info of coordinate system
- // // Key: coord, like ('x' 'y' 'radius' 'angle')
- // // value: Must be an array, not null/undefined. Contain dimension indices, like:
- // // {
- // // x: [2] // values on dimension index 2 are mapped to x axis.
- // // y: [0] // values on dimension index 0 are mapped to y axis.
- // // }
- // encode: Object,
- // // dimension names list
- // dimensionNames: Array,
- // // data dimension index, for example 0 or 1 or 2 ...
- // // Only work in `radar` series.
- // dimensionIndex: number,
- // // Color of data
- // color: string,
- //
- // // the percentage of pie chart
- // percent: number,
- // }
- Formatter string `json:"formatter,omitempty"`
-
- // Configuration item for axisPointer
- AxisPointer *AxisPointer `json:"axisPointer,omitempty"`
-}
-
-// AxisPointer is the option set for an axisPointer component
-// https://echarts.apache.org/en/option.html#axisPointer
-type AxisPointer struct {
-
- // Indicator type.
- // Options:
- // - 'line' line indicator.
- // - 'shadow' shadow crosshair indicator.
- // - 'none' no indicator displayed.
- // - 'cross' crosshair indicator, which is actually the shortcut of enable two axisPointers of two orthometric axes.
- Type string `json:"type,omitempty"`
-
- // Whether snap to point automatically. The default value is auto determined.
- // This feature usually makes sense in value axis and time axis, where tiny points can be seeked automatically.
- Snap bool `json:"snap,omitempty"`
-
- Link []AxisPointerLink `json:"link,omitempty"`
-
- Axis string `json:"axis,omitempty"`
-}
-
-type AxisPointerLink struct {
- XAxisIndex []int `json:"xAxisIndex,omitempty"`
- YAxisIndex []int `json:"yAxisIndex,omitempty"`
- XAxisName string `json:"xAxisName,omitempty"`
- YAxisName string `json:"yAxisName,omitempty"`
-}
-
-//Brush is an area-selecting component, with which user can select part of data from a chart to display in detail, or do calculations with them.
-//https://echarts.apache.org/en/option.html#brush
-type Brush struct {
-
- //XAxisIndex Assigns which of the xAxisIndex can use brush selecting.
- XAxisIndex interface{} `json:"xAxisIndex,omitempty"`
-
- //Brushlink is a mapping of dataIndex. So data of every series with brushLink should be guaranteed to correspond to the other.
- Brushlink interface{} `json:"brushlink,omitempty"`
-
- //OutOfBrush Defines visual effects of items out of selection
- OutOfBrush *BrushOutOfBrush `json:"outOfBrush,omitempty"`
-}
-
-//BrushOutOfBrush
-//https://echarts.apache.org/en/option.html#brush.outOfBrush
-type BrushOutOfBrush struct {
- ColorAlpha float32 `json:"colorAlpha,omitempty"`
-}
-
-// Toolbox is the option set for a toolbox component.
-// https://echarts.apache.org/en/option.html#toolbox
-type Toolbox struct {
- // Whether to show toolbox component.
- Show bool `json:"show"`
-
- // The layout orientation of toolbox's icon.
- // Options: 'horizontal','vertical'
- Orient string `json:"orient,omitempty"`
-
- // Distance between toolbox component and the left side of the container.
- // left value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'left', 'center', or 'right'.
- // If the left value is set to be 'left', 'center', or 'right', then the component
- // will be aligned automatically based on position.
- Left string `json:"left,omitempty"`
-
- // Distance between toolbox component and the top side of the container.
- // top value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'top', 'middle', or 'bottom'.
- // If the left value is set to be 'top', 'middle', or 'bottom', then the component
- // will be aligned automatically based on position.
- Top string `json:"top,omitempty"`
-
- // Distance between toolbox component and the right side of the container.
- // right value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- // Adaptive by default.
- Right string `json:"right,omitempty"`
-
- // Distance between toolbox component and the bottom side of the container.
- // bottom value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- // Adaptive by default.
- Bottom string `json:"bottom,omitempty"`
-
- // The configuration item for each tool.
- // Besides the tools we provide, user-defined toolbox is also supported.
- Feature *ToolBoxFeature `json:"feature,omitempty"`
-}
-
-// ToolBoxFeature is a feature component under toolbox.
-// https://echarts.apache.org/en/option.html#toolbox
-type ToolBoxFeature struct {
- // Save as image tool
- SaveAsImage *ToolBoxFeatureSaveAsImage `json:"saveAsImage,omitempty"`
-
- // Data brush
- Brush *ToolBoxFeatureBrush `json:"brush"`
-
- // Data area zooming, which only supports rectangular coordinate by now.
- DataZoom *ToolBoxFeatureDataZoom `json:"dataZoom,omitempty"`
-
- // Data view tool, which could display data in current chart and updates chart after being edited.
- DataView *ToolBoxFeatureDataView `json:"dataView,omitempty"`
-
- // Restore configuration item.
- Restore *ToolBoxFeatureRestore `json:"restore,omitempty"`
-}
-
-// ToolBoxFeatureSaveAsImage is the option for saving chart as image.
-// https://echarts.apache.org/en/option.html#toolbox.feature.saveAsImage
-type ToolBoxFeatureSaveAsImage struct {
- // Whether to show the tool.
- Show bool `json:"show"`
-
- // toolbox.feature.saveAsImage. type = 'png'
- // File suffix of the image saved.
- // If the renderer is set to be 'canvas' when chart initialized (default), t
- // hen 'png' (default) and 'jpeg' are supported.
- // If the renderer is set to be 'svg' when when chart initialized, then only 'svg' is supported
- // for type ('svg' type is supported since v4.8.0).
- Type string `json:"png,omitempty"`
-
- // Name to save the image, whose default value is title.text.
- Name string `json:"name,omitempty"`
-
- // Title for the tool.
- Title string `json:"title,omitempty"`
-}
-
-//ToolBoxFeatureBrush brush-selecting icon.
-//https://echarts.apache.org/en/option.html#toolbox.feature.brush
-type ToolBoxFeatureBrush struct {
-
- //Icons used, whose values are:
- // 'rect': Enabling selecting with rectangle area.
- // 'polygon': Enabling selecting with any shape.
- // 'lineX': Enabling horizontal selecting.
- // 'lineY': Enabling vertical selecting.
- // 'keep': Switching between single selecting and multiple selecting. The latter one can select multiple areas, while the former one cancels previous selection.
- // 'clear': Clearing all selection.
- Type []string `json:"type,omitempty"`
-}
-
-// ToolBoxFeatureDataZoom
-// https://echarts.apache.org/en/option.html#toolbox.feature.dataZoom
-type ToolBoxFeatureDataZoom struct {
- // Whether to show the tool.
- Show bool `json:"show"`
-
- //Defines which yAxis should be controlled. By default, it controls all y axes.
- //If it is set to be false, then no y axis is controlled.
- //If it is set to be then it controls axis with axisIndex of 3.
- //If it is set to be [0, 3], it controls the x-axes with axisIndex of 0 and 3.
- YAxisIndex interface{} `json:"yAxisIndex,omitempty"`
-
- // Restored and zoomed title text.
- // m["zoom"] = "area zooming"
- // m["back"] = "restore area zooming"
- Title map[string]string `json:"title"`
-}
-
-// ToolBoxFeatureDataView
-// https://echarts.apache.org/en/option.html#toolbox.feature.dataView
-type ToolBoxFeatureDataView struct {
- // Whether to show the tool.
- Show bool `json:"show"`
-
- // title for the tool.
- Title string `json:"title,omitempty"`
-
- // There are 3 names in data view
- // you could set them like this: []string["data view", "turn off", "refresh"]
- Lang []string `json:"lang"`
-
- // Background color of the floating layer in data view.
- BackgroundColor string `json:"backgroundColor"`
-}
-
-// ToolBoxFeatureRestore
-// https://echarts.apache.org/en/option.html#toolbox.feature.restore
-type ToolBoxFeatureRestore struct {
- // Whether to show the tool.
- Show bool `json:"show"`
-
- // title for the tool.
- Title string `json:"title,omitempty"`
-}
-
-// AxisLabel settings related to axis label.
-// https://echarts.apache.org/en/option.html#xAxis.axisLabel
-type AxisLabel struct {
- // Set this to false to prevent the axis label from appearing.
- Show bool `json:"show"`
-
- // Interval of Axis label, which is available in category axis.
- // It uses a strategy that labels do not overlap by default.
- // You may set it to be 0 to display all labels compulsively.
- // If it is set to be 1, it means that labels are shown once after one label.
- // And if it is set to be 2, it means labels are shown once after two labels, and so on.
- Interval string `json:"interval,omitempty"`
-
- // Set this to true so the axis labels face the inside direction.
- Inside bool `json:"inside,omitempty"`
-
- // Rotation degree of axis label, which is especially useful when there is no enough space for category axis.
- // Rotation degree is from -90 to 90.
- Rotate float64 `json:"rotate,omitempty"`
-
- // The margin between the axis label and the axis line.
- Margin float64 `json:"margin,omitempty"`
-
- // Formatter of axis label, which supports string template and callback function.
- //
- // Example:
- //
- // Use string template; template variable is the default label of axis {value}
- // formatter: '{value} kg'
- //
- // Use callback function; function parameters are axis index
- //
- //
- // formatter: function (value, index) {
- // // Formatted to be month/day; display year only in the first label
- // var date = new Date(value);
- // var texts = [(date.getMonth() + 1), date.getDate()];
- // if (idx === 0) {
- // texts.unshift(date.getYear());
- // }
- // return texts.join('/');
- // }
- Formatter string `json:"formatter,omitempty"`
-
- ShowMinLabel bool `json:"showMinLabel"`
- ShowMaxLabel bool `json:"showMaxLabel"`
-
- // Color of axis label is set to be axisLine.lineStyle.color by default. Callback function is supported,
- // in the following format:
- //
- // (val: string) => Color
- // Parameter is the text of label, and return value is the color. See the following example:
- //
- // textStyle: {
- // color: function (value, index) {
- // return value >= 0 ? 'green' : 'red';
- // }
- // }
- Color string `json:"color,omitempty"`
-
- // axis label font style
- FontStyle string `json:"fontStyle,omitempty"`
- // axis label font weight
- FontWeight string `json:"fontWeight,omitempty"`
- // axis label font family
- FontFamily string `json:"fontFamily,omitempty"`
- // axis label font size
- FontSize string `json:"fontSize,omitempty"`
- // Horizontal alignment of axis label
- Align string `json:"align,omitempty"`
- // Vertical alignment of axis label
- VerticalAlign string `json:"verticalAlign,omitempty"`
- // Line height of the axis label
- LineHeight string `json:"lineHeight,omitempty"`
-}
-
-type AxisTick struct {
- // Set this to false to prevent the axis tick from showing.
- Show bool `json:"show"`
-
- // interval of axisTick, which is available in category axis. is set to be the same as axisLabel.interval by default.
- // It uses a strategy that labels do not overlap by default.
- // You may set it to be 0 to display all labels compulsively.
- // If it is set to be 1, it means that labels are shown once after one label. And if it is set to be 2, it means labels are shown once after two labels, and so on.
- // On the other hand, you can control by callback function, whose format is shown below:
- // (index:number, value: string) => boolean
- // The first parameter is index of category, and the second parameter is the name of category. The return values decides whether to display label.
- Interval string `json:"interval,omitempty"`
-
- // Align axis tick with label, which is available only when boundaryGap is set to be true in category axis.
- AlignWithLabel bool `json:"alignWithLabel,omitempty"`
-}
-
-// AxisLine controls settings related to axis line.
-// https://echarts.apache.org/en/option.html#yAxis.axisLine
-type AxisLine struct {
- // Set this to false to prevent the axis line from showing.
- Show bool `json:"show"`
-
- // Specifies whether X or Y axis lies on the other's origin position, where value is 0 on axis.
- // Valid only if the other axis is of value type, and contains 0 value.
- OnZero bool `json:"onZero,omitempty"`
-
- // When multiple axes exists, this option can be used to specify which axis can be "onZero" to.
- OnZeroAxisIndex int `json:"onZeroAxisIndex,omitempty"`
-
- // Symbol of the two ends of the axis. It could be a string, representing the same symbol for two ends; or an array
- // with two string elements, representing the two ends separately. It's set to be 'none' by default, meaning no
- //arrow for either end. If it is set to be 'arrow', there shall be two arrows. If there should only one arrow
- //at the end, it should set to be ['none', 'arrow'].
- Symbol string `json:"symbol,omitempty"`
-
- // Size of the arrows at two ends. The first is the width perpendicular to the axis, the next is the width parallel to the axis.
- SymbolSize []float64 `json:"symbolSize,omitempty"`
-
- // Arrow offset of axis. If is array, the first number is the offset of the arrow at the beginning, and the second
- // number is the offset of the arrow at the end. If is number, it means the arrows have the same offset.
- SymbolOffset []float64 `json:"symbolOffset,omitempty"`
-
- LineStyle *LineStyle `json:"lineStyle,omitempty"`
-}
-
-// XAxis is the option set for X axis.
-// https://echarts.apache.org/en/option.html#xAxis
-type XAxis struct {
- // Name of axis.
- Name string `json:"name,omitempty"`
-
- // Type of axis.
- // Option:
- // * 'value': Numerical axis, suitable for continuous data.
- // * 'category': Category axis, suitable for discrete category data.
- // Category data can be auto retrieved from series.data or dataset.source,
- // or can be specified via xAxis.data.
- // * 'time' Time axis, suitable for continuous time series data. As compared to value axis,
- // it has a better formatting for time and a different tick calculation method. For example,
- // it decides to use month, week, day or hour for tick based on the range of span.
- // * 'log' Log axis, suitable for log data.
- Type string `json:"type,omitempty"`
-
- // Set this to false to prevent the axis from showing.
- Show bool `json:"show,omitempty"`
-
- // Category data, available in type: 'category' axis.
- Data interface{} `json:"data,omitempty"`
-
- // Number of segments that the axis is split into. Note that this number serves only as a
- // recommendation, and the true segments may be adjusted based on readability.
- // This is unavailable for category axis.
- SplitNumber int `json:"splitNumber,omitempty"`
-
- // It is available only in numerical axis, i.e., type: 'value'.
- // It specifies whether not to contain zero position of axis compulsively.
- // When it is set to be true, the axis may not contain zero position,
- // which is useful in the scatter chart for both value axes.
- // This configuration item is unavailable when the min and max are set.
- Scale bool `json:"scale,omitempty"`
-
- // The minimum value of axis.
- // It can be set to a special value 'dataMin' so that the minimum value on this axis is set to be the minimum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- Min interface{} `json:"min,omitempty"`
-
- // The maximum value of axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- Max interface{} `json:"max,omitempty"`
-
- // Minimum gap between split lines. For 'time' axis, MinInterval is in unit of milliseconds.
- MinInterval float64 `json:"minInterval,omitempty"`
-
- // Maximum gap between split lines. For 'time' axis, MaxInterval is in unit of milliseconds.
- MaxInterval float64 `json:"maxInterval,omitempty"`
-
- // The index of grid which the x axis belongs to. Defaults to be in the first grid.
- // default 0
- GridIndex int `json:"gridIndex,omitempty"`
-
- // Split area of X axis in grid area.
- SplitArea *SplitArea `json:"splitArea,omitempty"`
-
- // Split line of X axis in grid area.
- SplitLine *SplitLine `json:"splitLine,omitempty"`
-
- // Settings related to axis label.
- AxisLabel *AxisLabel `json:"axisLabel,omitempty"`
-
- // Settings related to axis tick.
- AxisTick *AxisTick `json:"axisTick,omitempty"`
-}
-
-// YAxis is the option set for Y axis.
-// https://echarts.apache.org/en/option.html#yAxis
-type YAxis struct {
- // Name of axis.
- Name string `json:"name,omitempty"`
-
- // Type of axis.
- // Option:
- // * 'value': Numerical axis, suitable for continuous data.
- // * 'category': Category axis, suitable for discrete category data.
- // Category data can be auto retrieved from series.data or dataset.source,
- // or can be specified via xAxis.data.
- // * 'time' Time axis, suitable for continuous time series data. As compared to value axis,
- // it has a better formatting for time and a different tick calculation method. For example,
- // it decides to use month, week, day or hour for tick based on the range of span.
- // * 'log' Log axis, suitable for log data.
- Type string `json:"type,omitempty"`
-
- // Set this to false to prevent the axis from showing.
- Show bool `json:"show,omitempty"`
-
- // Category data, available in type: 'category' axis.
- Data interface{} `json:"data,omitempty"`
-
- // Number of segments that the axis is split into. Note that this number serves only as a
- // recommendation, and the true segments may be adjusted based on readability.
- // This is unavailable for category axis.
- SplitNumber int `json:"splitNumber,omitempty"`
-
- // It is available only in numerical axis, i.e., type: 'value'.
- // It specifies whether not to contain zero position of axis compulsively.
- // When it is set to be true, the axis may not contain zero position,
- // which is useful in the scatter chart for both value axes.
- // This configuration item is unavailable when the min and max are set.
- Scale bool `json:"scale,omitempty"`
-
- // The minimum value of axis.
- // It can be set to a special value 'dataMin' so that the minimum value on this axis is set to be the minimum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- Min interface{} `json:"min,omitempty"`
-
- // The maximum value of axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- Max interface{} `json:"max,omitempty"`
-
- // The index of grid which the Y axis belongs to. Defaults to be in the first grid.
- // default 0
- GridIndex int `json:"gridIndex,omitempty"`
-
- // Split area of Y axis in grid area.
- SplitArea *SplitArea `json:"splitArea,omitempty"`
-
- // Split line of Y axis in grid area.
- SplitLine *SplitLine `json:"splitLine,omitempty"`
-
- // Settings related to axis label.
- AxisLabel *AxisLabel `json:"axisLabel,omitempty"`
-
- // Settings related to axis line.
- AxisLine *AxisLine `json:"axisLine,omitempty"`
-}
-
-// TextStyle is the option set for a text style component.
-type TextStyle struct {
- // Font color
- Color string `json:"color,omitempty"`
-
- // Font style
- // Options: 'normal', 'italic', 'oblique'
- FontStyle string `json:"fontStyle,omitempty"`
-
- // Font size
- FontSize int `json:"fontSize,omitempty"`
-
- // Font family the main title font family.
- // Options: "sans-serif", 'serif' , 'monospace', 'Arial', 'Courier New', 'Microsoft YaHei', ...
- FontFamily string `json:"fontFamily,omitempty"`
-
- // Padding title space around content.
- // The unit is px. Default values for each position are 5.
- // And they can be set to different values with left, right, top, and bottom.
- Padding interface{} `json:"padding,omitempty"`
-
- // compatible for WordCloud
- Normal *TextStyle `json:"normal,omitempty"`
-}
-
-// SplitArea is the option set for a split area.
-type SplitArea struct {
- // Set this to true to show the splitArea.
- Show bool `json:"show"`
-
- // Split area style.
- AreaStyle *AreaStyle `json:"areaStyle,omitempty"`
-}
-
-// SplitLine is the option set for a split line.
-type SplitLine struct {
- // Set this to true to show the splitLine.
- Show bool `json:"show"`
-
- // Split line style.
- LineStyle *LineStyle `json:"lineStyle,omitempty"`
-
- // Align split line with label, which is available only when boundaryGap is set to be true in category axis.
- AlignWithLabel bool `json:"alignWithLabel,omitempty"`
-}
-
-// VisualMap is a type of component for visual encoding, which maps the data to visual channels.
-// https://echarts.apache.org/en/option.html#visualMap
-type VisualMap struct {
- // Mapping type.
- // Options: "continuous", "piecewise"
- Type string `json:"type,omitempty" default:"continuous"`
-
- // Whether show handles, which can be dragged to adjust "selected range".
- Calculable bool `json:"calculable"`
-
- // Specify the min dataValue for the visualMap component.
- // [visualMap.min, visualMax.max] make up the domain of visual mapping.
- Min float32 `json:"min,omitempty"`
-
- // Specify the max dataValue for the visualMap component.
- // [visualMap.min, visualMax.max] make up the domain of visual mapping.
- Max float32 `json:"max,omitempty"`
-
- // Specify selected range, that is, the dataValue corresponding to the two handles.
- Range []float32 `json:"range,omitempty"`
-
- // The label text on both ends, such as ['High', 'Low'].
- Text []string `json:"text,omitempty"`
-
- // Define visual channels that will mapped from dataValues that are in selected range.
- InRange *VisualMapInRange `json:"inRange,omitempty"`
-
- // Whether to show visualMap-piecewise component. If set as false,
- // visualMap-piecewise component will not show,
- // but it can still perform visual mapping from dataValue to visual channel in chart.
- Show bool `json:"show"`
-
- // Distance between visualMap component and the left side of the container.
- // left value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'left', 'center', or 'right'.
- // If the left value is set to be 'left', 'center', or 'right',
- // then the component will be aligned automatically based on position.
- Left string `json:"left,omitempty"`
-
- // Distance between visualMap component and the right side of the container.
- // right value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- Right string `json:"right,omitempty"`
-
- // Distance between visualMap component and the top side of the container.
- // top value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'top', 'middle', or 'bottom'.
- // If the left value is set to be 'top', 'middle', or 'bottom',
- // then the component will be aligned automatically based on position.
- Top string `json:"top,omitempty"`
-
- // Distance between visualMap component and the bottom side of the container.
- // bottom value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- Bottom string `json:"bottom,omitempty"`
-}
-
-// VisualMapInRange is a visual map instance in a range.
-type VisualMapInRange struct {
- // Color
- Color []string `json:"color,omitempty"`
-
- // Symbol type at the two ends of the mark line. It can be an array for two ends, or assigned separately.
- // Options: "circle", "rect", "roundRect", "triangle", "diamond", "pin", "arrow", "none"
- Symbol string `json:"symbol,omitempty"`
-
- // Symbol size.
- SymbolSize float32 `json:"symbolSize,omitempty"`
-}
-
-// DataZoom is the option set for a zoom component.
-// dataZoom component is used for zooming a specific area, which enables user to
-// investigate data in detail, or get an overview of the data, or get rid of outlier points.
-// https://echarts.apache.org/en/option.html#dataZoom
-type DataZoom struct {
- // Data zoom component of inside type, Options: "inside", "slider"
- Type string `json:"type" default:"inside"`
-
- // The start percentage of the window out of the data extent, in the range of 0 ~ 100.
- // default 0
- Start float32 `json:"start,omitempty"`
-
- // The end percentage of the window out of the data extent, in the range of 0 ~ 100.
- // default 100
- End float32 `json:"end,omitempty"`
-
- // Specify the frame rate of views refreshing, with unit millisecond (ms).
- // If animation set as true and animationDurationUpdate set as bigger than 0,
- // you can keep throttle as the default value 100 (or set it as a value bigger than 0),
- // otherwise it might be not smooth when dragging.
- // If animation set as false or animationDurationUpdate set as 0, and data size is not very large,
- // and it seems to be not smooth when dragging, you can set throttle as 0 to improve that.
- Throttle float32 `json:"throttle,omitempty"`
-
- // Specify which xAxis is/are controlled by the dataZoom-inside when Cartesian coordinate system is used.
- // By default the first xAxis that parallel to dataZoom are controlled when dataZoom-inside.
- // Orient is set as 'horizontal'. But it is recommended to specify it explicitly but not use default value.
- // If it is set as a single number, one axis is controlled, while if it is set as an Array ,
- // multiple axes are controlled.
- XAxisIndex interface{} `json:"xAxisIndex,omitempty"`
-
- // Specify which yAxis is/are controlled by the dataZoom-inside when Cartesian coordinate system is used.
- // By default the first yAxis that parallel to dataZoom are controlled when dataZoom-inside.
- // Orient is set as 'vertical'. But it is recommended to specify it explicitly but not use default value.
- // If it is set as a single number, one axis is controlled, while if it is set as an Array ,
- // multiple axes are controlled.
- YAxisIndex interface{} `json:"yAxisIndex,omitempty"`
-}
-
-// SingleAxis is the option set for single axis.
-// https://echarts.apache.org/en/option.html#singleAxis
-type SingleAxis struct {
- // The minimum value of axis.
- // It can be set to a special value 'dataMin' so that the minimum value on this axis is set to be the minimum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- Min interface{} `json:"min,omitempty"`
-
- // The maximum value of axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- Max interface{} `json:"max,omitempty"`
-
- // Type of axis.
- // Option:
- // * 'value': Numerical axis, suitable for continuous data.
- // * 'category': Category axis, suitable for discrete category data.
- // Category data can be auto retrieved from series.data or dataset.source,
- // or can be specified via xAxis.data.
- // * 'time' Time axis, suitable for continuous time series data. As compared to value axis,
- // it has a better formatting for time and a different tick calculation method. For example,
- // it decides to use month, week, day or hour for tick based on the range of span.
- // * 'log' Log axis, suitable for log data.
- Type string `json:"type,omitempty"`
-
- // Distance between grid component and the left side of the container.
- // left value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'left', 'center', or 'right'.
- // If the left value is set to be 'left', 'center', or 'right',
- // then the component will be aligned automatically based on position.
- Left string `json:"left,omitempty"`
-
- // Distance between grid component and the right side of the container.
- // right value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- Right string `json:"right,omitempty"`
-
- // Distance between grid component and the top side of the container.
- // top value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'; and it can also be 'top', 'middle', or 'bottom'.
- // If the left value is set to be 'top', 'middle', or 'bottom',
- // then the component will be aligned automatically based on position.
- Top string `json:"top,omitempty"`
-
- // Distance between grid component and the bottom side of the container.
- // bottom value can be instant pixel value like 20; it can also be a percentage
- // value relative to container width like '20%'.
- Bottom string `json:"bottom,omitempty"`
-}
-
-// Indicator is the option set for a radar chart.
-type Indicator struct {
- // Indicator name
- Name string `json:"name,omitempty"`
-
- // The maximum value of indicator. It is an optional configuration, but we recommend to set it manually.
- Max float32 `json:"max,omitempty"`
-
- // The minimum value of indicator. It it an optional configuration, with default value of 0.
- Min float32 `json:"min,omitempty"`
-
- // Specify a color the the indicator.
- Color string `json:"color,omitempty"`
-}
-
-// RadarComponent is the option set for a radar component.
-// https://echarts.apache.org/en/option.html#radar
-type RadarComponent struct {
- // Indicator of radar chart, which is used to assign multiple variables(dimensions) in radar chart.
- Indicator []*Indicator `json:"indicator,omitempty"`
-
- // Radar render type, in which 'polygon' and 'circle' are supported.
- Shape string `json:"shape,omitempty"`
-
- // Segments of indicator axis.
- // default 5
- SplitNumber int `json:"splitNumber,omitempty"`
-
- // Center position of , the first of which is the horizontal position, and the second is the vertical position.
- // Percentage is supported. When set in percentage, the item is relative to the container width and height.
- Center interface{} `json:"center,omitempty"`
-
- // Split area of axis in grid area.
- SplitArea *SplitArea `json:"splitArea,omitempty"`
-
- // Split line of axis in grid area.
- SplitLine *SplitLine `json:"splitLine,omitempty"`
-}
-
-// GeoComponent is the option set for geo component.
-// https://echarts.apache.org/en/option.html#geo
-type GeoComponent struct {
- // Map charts.
- Map string `json:"map,omitempty"`
-
- // Graphic style of Map Area Border, emphasis is the style when it is highlighted,
- // like being hovered by mouse, or highlighted via legend connect.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // Set this to true, to prevent interaction with the axis.
- Silent bool `json:"silent,omitempty"`
-}
-
-// ParallelComponent is the option set for parallel component.
-type ParallelComponent struct {
- // Distance between parallel component and the left side of the container.
- // Left value can be instant pixel value like 20.
- // It can also be a percentage value relative to container width like '20%';
- // and it can also be 'left', 'center', or 'right'.
- // If the left value is set to be 'left', 'center', or 'right',
- // then the component will be aligned automatically based on position.
- Left string `json:"left,omitempty"`
-
- // Distance between parallel component and the top side of the container.
- // Top value can be instant pixel value like 20.
- // It can also be a percentage value relative to container width like '20%'.
- // and it can also be 'top', 'middle', or 'bottom'.
- // If the left value is set to be 'top', 'middle', or 'bottom',
- // then the component will be aligned automatically based on position.
- Top string `json:"top,omitempty"`
-
- // Distance between parallel component and the right side of the container.
- // Right value can be instant pixel value like 20.
- // It can also be a percentage value relative to container width like '20%'.
- Right string `json:"right,omitempty"`
-
- // Distance between parallel component and the bottom side of the container.
- // Bottom value can be instant pixel value like 20.
- // It can also be a percentage value relative to container width like '20%'.
- Bottom string `json:"bottom,omitempty"`
-}
-
-// ParallelAxis is the option set for a parallel axis.
-type ParallelAxis struct {
- // Dimension index of coordinate axis.
- Dim int `json:"dim,omitempty"`
-
- // Name of axis.
- Name string `json:"name,omitempty"`
-
- // The maximum value of axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure axis tick is equally distributed when not set.
- // In category axis, it can also be set as the ordinal number.
- Max interface{} `json:"max,omitempty"`
-
- // Compulsively set segmentation interval for axis.
- Inverse bool `json:"inverse,omitempty"`
-
- // Location of axis name. Options: "start", "middle", "center", "end"
- // default "end"
- NameLocation string `json:"nameLocation,omitempty"`
-
- // Type of axis.
- // Options:
- // "value":Numerical axis, suitable for continuous data.
- // "category" Category axis, suitable for discrete category data. Category data can be auto retrieved from series.
- // "log" Log axis, suitable for log data.
- Type string `json:"type,omitempty"`
-
- // Category data,works on (type: "category").
- Data interface{} `json:"data,omitempty"`
-}
-
-// Polar Bar options
-type Polar struct {
- ID string `json:"id,omitempty"`
- Zlevel int `json:"zlevel,omitempty"`
- Z int `json:"z,omitempty"`
- Center [2]string `json:"center,omitempty"`
- Radius [2]string `json:"radius,omitempty"`
- Tooltip Tooltip `json:"tooltip,omitempty"`
-}
-
-type PolarAxisBase struct {
- ID string `json:"id,omitempty"`
- PolarIndex int `json:"polarIndex,omitempty"`
- StartAngle float64 `json:"startAngle,omitempty"`
- Type string `json:"type,omitempty"`
- BoundaryGap bool `json:"boundaryGap,omitempty"`
- Min float64 `json:"min,omitempty"`
- Max float64 `json:"max,omitempty"`
- Scale bool `json:"scale,omitempty"`
- SplitNumber int `json:"splitNumber,omitempty"`
- MinInterval float64 `json:"minInterval,omitempty"`
- MaxInterval float64 `json:"maxInterval,omitempty"`
- Interval float64 `json:"interval,omitempty"`
- LogBase float64 `json:"logBase,omitempty"`
- Silent bool `json:"silent,omitempty"`
- TriggerEvent bool `json:"triggerEvent,omitempty"`
-}
-
-type AngleAxis struct {
- PolarAxisBase
- Clockwise bool `json:"clockwise,omitempty"`
-}
-
-type RadiusAxis struct {
- PolarAxisBase
- Name string `json:"name,omitempty"`
- NameLocation string `json:"nameLocation,omitempty"`
- NameTextStyle TextStyle `json:"nameTextStyle,omitempty"`
- NameGap float64 `json:"nameGap,omitempty"`
- NameRadius float64 `json:"nameRotate,omitempty"`
- Inverse bool `json:"inverse,omitempty"`
-}
-
-var funcPat = regexp.MustCompile(`\n|\t`)
-
-const funcMarker = "__f__"
-
-type JSFunctions struct {
- Fns []string
-}
-
-// AddJSFuncs adds a new JS function.
-func (f *JSFunctions) AddJSFuncs(fn ...string) {
- for i := 0; i < len(fn); i++ {
- f.Fns = append(f.Fns, funcPat.ReplaceAllString(fn[i], ""))
- }
-}
-
-// FuncOpts is the option set for handling function type.
-func FuncOpts(fn string) string {
- return replaceJsFuncs(fn)
-}
-
-// replace and clear up js functions string
-func replaceJsFuncs(fn string) string {
- return fmt.Sprintf("%s%s%s", funcMarker, funcPat.ReplaceAllString(fn, ""), funcMarker)
-}
-
-type Colors []string
-
-// Assets contains options for static assets.
-type Assets struct {
- JSAssets types.OrderedSet
- CSSAssets types.OrderedSet
-
- CustomizedJSAssets types.OrderedSet
- CustomizedCSSAssets types.OrderedSet
-}
-
-// InitAssets inits the static assets storage.
-func (opt *Assets) InitAssets() {
- opt.JSAssets.Init("echarts.min.js")
- opt.CSSAssets.Init()
-
- opt.CustomizedJSAssets.Init()
- opt.CustomizedCSSAssets.Init()
-}
-
-// AddCustomizedJSAssets adds the customized javascript assets which will not be added the `host` prefix.
-func (opt *Assets) AddCustomizedJSAssets(assets ...string) {
- for i := 0; i < len(assets); i++ {
- opt.CustomizedJSAssets.Add(assets[i])
- }
-}
-
-// AddCustomizedCSSAssets adds the customized css assets which will not be added the `host` prefix.
-func (opt *Assets) AddCustomizedCSSAssets(assets ...string) {
- for i := 0; i < len(assets); i++ {
- opt.CustomizedCSSAssets.Add(assets[i])
- }
-}
-
-// Validate validates the static assets configurations
-func (opt *Assets) Validate(host string) {
- for i := 0; i < len(opt.JSAssets.Values); i++ {
- if !strings.HasPrefix(opt.JSAssets.Values[i], host) {
- opt.JSAssets.Values[i] = host + opt.JSAssets.Values[i]
- }
- }
-
- for i := 0; i < len(opt.CSSAssets.Values); i++ {
- if !strings.HasPrefix(opt.CSSAssets.Values[i], host) {
- opt.CSSAssets.Values[i] = host + opt.CSSAssets.Values[i]
- }
- }
-}
-
-// XAxis3D contains options for X axis in the 3D coordinate.
-type XAxis3D struct {
- // Whether to display the x-axis.
- Show bool `json:"show,omitempty"`
-
- // The name of the axis.
- Name string `json:"name,omitempty"`
-
- // The index of the grid3D component used by the axis. The default is to use the first grid3D component.
- Grid3DIndex int `json:"grid3DIndex,omitempty"`
-
- // The type of the axis.
- // Optional:
- // * 'value' The value axis. Suitable for continuous data.
- // * 'category' The category axis. Suitable for the discrete category data.
- // For this type, the category data must be set through data.
- // * 'time' The timeline. Suitable for the continuous timing data. The time axis has a
- // time format compared to the value axis, and the scale calculation is also different.
- // For example, the scale of the month, week, day, and hour ranges can be determined according to the range of the span.
- // * 'log' Logarithmic axis. Suitable for the logarithmic data.
- Type string `json:"type,omitempty"`
-
- // The minimum value of axis.
- // It can be set to a special value 'dataMin' so that the minimum value on this axis is set to be the minimum label.
- // It will be automatically computed to make sure the axis tick is equally distributed when not set.
- // In the category axis, it can also be set as the ordinal number. For example,
- // if a category axis has data: ['categoryA', 'categoryB', 'categoryC'],
- // and the ordinal 2 represents 'categoryC'. Moreover, it can be set as a negative number, like -3.
- Min interface{} `json:"min,omitempty"`
-
- // The maximum value of the axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure the axis tick is equally distributed when not set.
- // In the category axis, it can also be set as the ordinal number. For example, if a category axis
- // has data: ['categoryA', 'categoryB', 'categoryC'], and the ordinal 2 represents 'categoryC'.
- // Moreover, it can be set as a negative number, like -3.
- Max interface{} `json:"max,omitempty"`
-
- // Category data, available in type: 'category' axis.
- // If type is specified as 'category', but axis.data is not specified, axis.data will be auto
- // collected from series.data. It brings convenience, but we should notice that axis.data provides
- // then value range of the 'category' axis. If it is auto collected from series.data,
- // Only the values appearing in series.data can be collected. For example,
- // if series.data is empty, nothing will be collected.
- Data interface{} `json:"data,omitempty"`
-}
-
-// YAxis3D contains options for Y axis in the 3D coordinate.
-type YAxis3D struct {
- // Whether to display the y-axis.
- Show bool `json:"show,omitempty"`
-
- // The name of the axis.
- Name string `json:"name,omitempty"`
-
- // The index of the grid3D component used by the axis. The default is to use the first grid3D component.
- Grid3DIndex int `json:"grid3DIndex,omitempty"`
-
- // The type of the axis.
- // Optional:
- // * 'value' The value axis. Suitable for continuous data.
- // * 'category' The category axis. Suitable for the discrete category data.
- // For this type, the category data must be set through data.
- // * 'time' The timeline. Suitable for the continuous timing data. The time axis has a
- // time format compared to the value axis, and the scale calculation is also different.
- // For example, the scale of the month, week, day, and hour ranges can be determined according to the range of the span.
- // * 'log' Logarithmic axis. Suitable for the logarithmic data.
- Type string `json:"type,omitempty"`
-
- // The minimum value of axis.
- // It can be set to a special value 'dataMin' so that the minimum value on this axis is set to be the minimum label.
- // It will be automatically computed to make sure the axis tick is equally distributed when not set.
- // In the category axis, it can also be set as the ordinal number. For example,
- // if a category axis has data: ['categoryA', 'categoryB', 'categoryC'],
- // and the ordinal 2 represents 'categoryC'. Moreover, it can be set as a negative number, like -3.
- Min interface{} `json:"min,omitempty"`
-
- // The maximum value of the axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure the axis tick is equally distributed when not set.
- // In the category axis, it can also be set as the ordinal number. For example, if a category axis
- // has data: ['categoryA', 'categoryB', 'categoryC'], and the ordinal 2 represents 'categoryC'.
- // Moreover, it can be set as a negative number, like -3.
- Max interface{} `json:"max,omitempty"`
-
- // Category data, available in type: 'category' axis.
- // If type is specified as 'category', but axis.data is not specified, axis.data will be auto
- // collected from series.data. It brings convenience, but we should notice that axis.data provides
- // then value range of the 'category' axis. If it is auto collected from series.data,
- // Only the values appearing in series.data can be collected. For example,
- // if series.data is empty, nothing will be collected.
- Data interface{} `json:"data,omitempty"`
-}
-
-// ZAxis3D contains options for Z axis in the 3D coordinate.
-type ZAxis3D struct {
- // Whether to display the z-axis.
- Show bool `json:"show,omitempty"`
-
- // The name of the axis.
- Name string `json:"name,omitempty"`
-
- // The index of the grid3D component used by the axis. The default is to use the first grid3D component.
- Grid3DIndex int `json:"grid3DIndex,omitempty"`
-
- // The type of the axis.
- // Optional:
- // * 'value' The value axis. Suitable for continuous data.
- // * 'category' The category axis. Suitable for the discrete category data.
- // For this type, the category data must be set through data.
- // * 'time' The timeline. Suitable for the continuous timing data. The time axis has a
- // time format compared to the value axis, and the scale calculation is also different.
- // For example, the scale of the month, week, day, and hour ranges can be determined according to the range of the span.
- // * 'log' Logarithmic axis. Suitable for the logarithmic data.
- Type string `json:"type,omitempty"`
-
- // The minimum value of axis.
- // It can be set to a special value 'dataMin' so that the minimum value on this axis is set to be the minimum label.
- // It will be automatically computed to make sure the axis tick is equally distributed when not set.
- // In the category axis, it can also be set as the ordinal number. For example,
- // if a category axis has data: ['categoryA', 'categoryB', 'categoryC'],
- // and the ordinal 2 represents 'categoryC'. Moreover, it can be set as a negative number, like -3.
- Min interface{} `json:"min,omitempty"`
-
- // The maximum value of the axis.
- // It can be set to a special value 'dataMax' so that the minimum value on this axis is set to be the maximum label.
- // It will be automatically computed to make sure the axis tick is equally distributed when not set.
- // In the category axis, it can also be set as the ordinal number. For example, if a category axis
- // has data: ['categoryA', 'categoryB', 'categoryC'], and the ordinal 2 represents 'categoryC'.
- // Moreover, it can be set as a negative number, like -3.
- Max interface{} `json:"max,omitempty"`
-
- // Category data, available in type: 'category' axis.
- // If type is specified as 'category', but axis.data is not specified, axis.data will be auto
- // collected from series.data. It brings convenience, but we should notice that axis.data provides
- // then value range of the 'category' axis. If it is auto collected from series.data,
- // Only the values appearing in series.data can be collected. For example,
- // if series.data is empty, nothing will be collected.
- Data interface{} `json:"data,omitempty"`
-}
-
-// Grid3D contains options for the 3D coordinate.
-type Grid3D struct {
- // Whether to show the coordinate.
- Show bool `json:"show,omitempty"`
-
- // 3D Cartesian coordinates width
- // default 100
- BoxWidth float32 `json:"boxWidth,omitempty"`
-
- // 3D Cartesian coordinates height
- // default 100
- BoxHeight float32 `json:"boxHeight,omitempty"`
-
- // 3D Cartesian coordinates depth
- // default 100
- BoxDepth float32 `json:"boxDepth,omitempty"`
-
- // Rotate or scale fellows the mouse
- ViewControl *ViewControl `json:"viewControl,omitempty"`
-}
-
-// ViewControl contains options for view controlling.
-type ViewControl struct {
- // Whether to rotate automatically.
- AutoRotate bool `json:"autoRotate,omitempty"`
-
- // Rotate Speed, (angle/s).
- // default 10
- AutoRotateSpeed float32 `json:"autoRotateSpeed,omitempty"`
-}
-
-// Grid Drawing grid in rectangular coordinate.
-// https://echarts.apache.org/en/option.html#grid
-type Grid struct {
- // Distance between grid component and the left side of the container.
- Left string `json:"left,omitempty"`
-
- // Distance between grid component and the right side of the container.
- Right string `json:"right,omitempty"`
-
- // Distance between grid component and the top side of the container.
- Top string `json:"top,omitempty"`
-
- // Distance between grid component and the bottom side of the container.
- Bottom string `json:"bottom,omitempty"`
-
- // Width of grid component.
- Width string `json:"width,omitempty"`
-
- ContainLabel bool `json:"containLabel,omitempty"`
-
- // Height of grid component. Adaptive by default.
- Height string `json:"height,omitempty"`
-}
-
-//Dataset brings convenience in data management separated with styles and enables data reuse by different series.
-//More importantly, it enables data encoding from data to visual, which brings convenience in some scenarios.
-//https://echarts.apache.org/en/option.html#dataset.id
-type Dataset struct {
- //source
- Source interface{} `json:"source"`
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/opts/series.go b/vendor/github.com/go-echarts/go-echarts/v2/opts/series.go
deleted file mode 100644
index defe10c0..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/opts/series.go
+++ /dev/null
@@ -1,690 +0,0 @@
-package opts
-
-import (
- "fmt"
-)
-
-// Label contains options for a label text.
-// https://echarts.apache.org/en/option.html#series-line.label
-type Label struct {
- // Whether to show label.
- Show bool `json:"show"`
-
- // Color is the text color.
- // If set as "auto", the color will assigned as visual color, such as series color.
- Color string `json:"color,omitempty"`
-
- // font style.
- // Options are: 'normal', 'italic', 'oblique'
- FontStyle string `json:"fontStyle,omitempty"`
-
- // font thick weight.
- // Options are: 'normal', 'bold', 'bolder', 'lighter', 100 | 200 | 300 | 400...
- FontWeight string `json:"fontWeight,omitempty"`
-
- // font family.
- // Can also be 'serif' , 'monospace', ...
- FontFamily string `json:"fontFamily,omitempty"`
-
- // font size.
- FontSize float32 `json:"fontSize,omitempty"`
-
- // Horizontal alignment of text, automatic by default.
- // Options are: 'left', 'center', 'right'
- Align string `json:"align,omitempty"`
-
- // Vertical alignment of text, automatic by default.
- // Options are: 'top', 'middle', 'bottom'
- VerticalAlign string `json:"verticalAlign,omitempty"`
-
- // Line height of the text fragment.
- LineHeight float32 `json:"lineHeight,omitempty"`
-
- // Background color of the text fragment.
- BackgroundColor string `json:"backgroundColor,omitempty"`
-
- // Border color of the text fragment.
- BorderColor string `json:"borderColor,omitempty"`
-
- // Border width of the text fragment.
- BorderWidth float32 `json:"borderWidth,omitempty"`
-
- // the text fragment border type.
- // Possible values are: 'solid', 'dashed', 'dotted'
- BorderType string `json:"borderType,omitempty"`
-
- // To set the line dash offset. With borderType , we can make the line style more flexible.
- BorderDashOffset float32 `json:"borderDashOffset,omitempty"`
-
- // Border radius of the text fragment.
- BorderRadius float32 `json:"borderRadius,omitempty"`
-
- // Padding of the text fragment, for example:
- // padding: [3, 4, 5, 6]: represents padding of [top, right, bottom, left].
- // padding: 4: represents padding: [4, 4, 4, 4].
- // padding: [3, 4]: represents padding: [3, 4, 3, 4].
- // Notice, width and height specifies the width and height of the content, without padding.
- Padding string `json:"padding,omitempty"`
-
- // Label position. Followings are the options:
- //
- // [x, y]
- // Use relative percentage, or absolute pixel values to represent position of label
- // relative to top-left corner of bounding box. For example:
- //
- // Absolute pixel values: position: [10, 10],
- // Relative percentage: position: ["50%", "50%"]
- //
- // "top"
- // "left"
- // "right"
- // "bottom"
- // "inside"
- // "insideLeft"
- // "insideRight"
- // "insideTop"
- // "insideBottom"
- // "insideTopLeft"
- // "insideBottomLeft"
- // "insideTopRight"
- // "insideBottomRight"
- Position string `json:"position,omitempty"`
-
- // Data label formatter, which supports string template and callback function.
- // In either form, \n is supported to represent a new line.
- // String template, Model variation includes:
- //
- // {a}: series name.
- // {b}: the name of a data item.
- // {c}: the value of a data item.
- // {@xxx}: the value of a dimension named"xxx", for example,{@product}refers the value of"product"` dimension.
- // {@[n]}: the value of a dimension at the index ofn, for example,{@[3]}` refers the value at dimensions[3].
- Formatter string `json:"formatter,omitempty"`
-}
-
-// LabelLine Configuration of label guide line.
-type LabelLine struct {
- // Whether to show the label guide line.
- Show bool `json:"show"`
- // Whether to show the label guide line above the corresponding element.
- ShowAbove bool `json:"showAbove"`
- // The length of the second segment of guide line.
- Length2 float64 `json:"length2,omitempty"`
- // smoothness of guide line.
- Smooth bool `json:"smooth"`
- // Minimum turn angle between two segments of guide line
- MinTurnAngle float64 `json:"minTurnAngle,omitempty"`
- // The style of label line
- LineStyle *LineStyle `json:"lineStyle,omitempty"`
-}
-
-// Emphasis is the style when it is highlighted, like being hovered by mouse, or highlighted via legend connect.
-type Emphasis struct {
- // the emphasis style of label
- Label *Label `json:"label,omitempty"`
-
- // the emphasis style of item
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// ItemStyle represents a style of an item.
-type ItemStyle struct {
- // Color of chart
- // Kline Up candle color
- Color string `json:"color,omitempty"`
-
- // Kline Down candle color
- Color0 string `json:"color0,omitempty"`
-
- // BorderColor is the hart border color
- // Kline Up candle border color
- BorderColor string `json:"borderColor,omitempty"`
-
- // Kline Down candle border color
- BorderColor0 string `json:"borderColor0,omitempty"`
-
- // Color saturation of a border or gap.
- BorderColorSaturation float32 `json:"borderColorSaturation,omitempty"`
-
- // Border width of a node
- BorderWidth float32 `json:"borderWidth,omitempty"`
-
- // Gaps between child nodes.
- GapWidth float32 `json:"gapWidth,omitempty"`
-
- // Opacity of the component. Supports value from 0 to 1, and the component will not be drawn when set to 0.
- Opacity float32 `json:"opacity,omitempty"`
-}
-
-// MarkLines represents a series of marklines.
-type MarkLines struct {
- Data []interface{} `json:"data,omitempty"`
- MarkLineStyle
-}
-
-// MarkLineStyle contains styling options for a MarkLine.
-type MarkLineStyle struct {
- // Symbol type at the two ends of the mark line. It can be an array for two ends, or assigned separately.
- // Options: "circle", "rect", "roundRect", "triangle", "diamond", "pin", "arrow", "none"
- Symbol []string `json:"symbol,omitempty"`
-
- // Symbol size.
- SymbolSize float32 `json:"symbolSize,omitempty"`
-
- // Mark line text options.
- Label *Label `json:"label,omitempty"`
-}
-
-// CircularStyle contains styling options for circular layout.
-type CircularStyle struct {
- RotateLabel bool `json:"rotateLabel,omitempty"`
-}
-
-// MarkLineNameTypeItem represents type for a MarkLine.
-type MarkLineNameTypeItem struct {
- // Mark line name.
- Name string `json:"name,omitempty"`
-
- // Mark line type, options: "average", "min", "max".
- Type string `json:"type,omitempty"`
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-}
-
-// MarkLineNameYAxisItem defines a MarkLine on a Y axis.
-type MarkLineNameYAxisItem struct {
- // Mark line name
- Name string `json:"name,omitempty"`
-
- // Y axis data
- YAxis interface{} `json:"yAxis,omitempty"`
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-}
-
-// MarkLineNameXAxisItem defines a MarkLine on a X axis.
-type MarkLineNameXAxisItem struct {
- // Mark line name
- Name string `json:"name,omitempty"`
-
- // X axis data
- XAxis interface{} `json:"xAxis,omitempty"`
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-}
-
-// MarkLineNameCoordItem represents coordinates for a MarkLine.
-type MarkLineNameCoordItem struct {
- // Mark line name
- Name string `json:"name,omitempty"`
-
- // Mark line start coordinate
- Coordinate0 []interface{}
-
- // Mark line end coordinate
- Coordinate1 []interface{}
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-}
-
-// MarkAreas represents a series of markareas.
-type MarkAreas struct {
- Data []interface{} `json:"data,omitempty"`
- MarkAreaStyle
-}
-
-// MarkAreaStyle contains styling options for a MarkArea.
-type MarkAreaStyle struct {
- // Mark area text options.
- Label *Label `json:"label,omitempty"`
-
- // ItemStyle settings
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// MarkAreaNameTypeItem represents type for a MarkArea.
-type MarkAreaNameTypeItem struct {
- // Mark area name.
- Name string `json:"name,omitempty"`
-
- // Mark area type, options: "average", "min", "max".
- Type string `json:"type,omitempty"`
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-
- // ItemStyle settings
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// MarkAreaNameYAxisItem defines a MarkArea on a Y axis.
-type MarkAreaNameYAxisItem struct {
- // Mark area name
- Name string `json:"name,omitempty"`
-
- // Y axis data
- YAxis interface{} `json:"yAxis,omitempty"`
-}
-
-// MarkAreaNameXAxisItem defines a MarkArea on a X axis.
-type MarkAreaNameXAxisItem struct {
- // Mark area name
- Name string `json:"name,omitempty"`
-
- // X axis data
- XAxis interface{} `json:"xAxis,omitempty"`
-}
-
-// MarkAreaNameCoordItem represents coordinates for a MarkArea.
-type MarkAreaNameCoordItem struct {
- // Mark area name
- Name string `json:"name,omitempty"`
-
- // Mark area start coordinate
- Coordinate0 []interface{}
-
- // Mark area end coordinate
- Coordinate1 []interface{}
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-
- // Mark point text options.
- Label *Label `json:"label,omitempty"`
-
- // ItemStyle settings
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// MarkPoints represents a series of markpoints.
-type MarkPoints struct {
- Data []interface{} `json:"data,omitempty"`
- MarkPointStyle
-}
-
-// MarkPointStyle contains styling options for a MarkPoint.
-type MarkPointStyle struct {
- // Symbol type at the two ends of the mark line. It can be an array for two ends, or assigned separately.
- // Options: "circle", "rect", "roundRect", "triangle", "diamond", "pin", "arrow", "none"
- Symbol []string `json:"symbol,omitempty"`
-
- // Symbol size.
- SymbolSize float32 `json:"symbolSize,omitempty"`
-
- // Symbol rotate.
- SymbolRotate float32 `json:"symbolRotate,omitempty"`
-
- // Mark point text options.
- Label *Label `json:"label,omitempty"`
-}
-
-// MarkPointNameTypeItem represents type for a MarkPoint.
-type MarkPointNameTypeItem struct {
- // Name of markpoint
- Name string `json:"name,omitempty"`
-
- // Mark point type, options: "average", "min", "max".
- Type string `json:"type,omitempty"`
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-
- // ItemStyle settings
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-}
-
-// MarkPointNameCoordItem represents coordinates for a MarkPoint.
-type MarkPointNameCoordItem struct {
- // Name of markpoint
- Name string `json:"name,omitempty"`
-
- // Mark point coordinate
- Coordinate []interface{} `json:"coord,omitempty"`
-
- // Value in mark point
- Value string `json:"value,omitempty"`
-
- // Works only when type is assigned.
- // It is used to state the dimension used to calculate maximum value or minimum value.
- // It may be the direct name of a dimension, like x,
- // or angle for line charts, or open, or close for candlestick charts.
- ValueDim string `json:"valueDim,omitempty"`
-
- // Mark point text options.
- Label *Label `json:"label,omitempty"`
-
- // ItemStyle settings
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // Symbol type
- // Options: "circle", "rect", "roundRect", "triangle", "diamond", "pin", "arrow", "none"
- Symbol string `json:"symbol,omitempty"`
-
- // Symbol size.
- SymbolSize float32 `json:"symbolSize,omitempty"`
-
- // Symbol rotate.
- SymbolRotate float32 `json:"symbolRotate,omitempty"`
-}
-
-// RippleEffect is the option set for the ripple effect.
-type RippleEffect struct {
- // The period duration of animation, in seconds.
- // default 4(s)
- Period float32 `json:"period,omitempty"`
-
- // The maximum zooming scale of ripples in animation.
- // default 2.5
- Scale float32 `json:"scale,omitempty"`
-
- // The brush type for ripples. options: "stroke" and "fill".
- // default "fill"
- BrushType string `json:"brushType,omitempty"`
-}
-
-// LineStyle is the option set for a link style component.
-type LineStyle struct {
- // Line color
- Color string `json:"color,omitempty"`
-
- // Width of line. default 1
- Width float32 `json:"width,omitempty"`
-
- // Type of line,options: "solid", "dashed", "dotted". default "solid"
- Type string `json:"type,omitempty"`
-
- // Opacity of the component. Supports value from 0 to 1, and the component will not be drawn when set to 0.
- Opacity float32 `json:"opacity,omitempty"`
-
- // Curveness of edge. The values from 0 to 1 could be set.
- // it would be larger as the the value becomes larger. default 0
- Curveness float32 `json:"curveness,omitempty"`
-}
-
-// AreaStyle is the option set for an area style component.
-type AreaStyle struct {
- // Fill area color.
- Color string `json:"color,omitempty"`
-
- // Opacity of the component. Supports value from 0 to 1, and the component will not be drawn when set to 0.
- Opacity float32 `json:"opacity,omitempty"`
-}
-
-// Configuration items about force-directed layout. Force-directed layout simulates
-// spring/charge model, which will add a repulsion between 2 nodes and add a attraction
-// between 2 nodes of each edge. In each iteration nodes will move under the effect
-// of repulsion and attraction. After several iterations, the nodes will be static in a
-// balanced position. As a result, the energy local minimum of this whole model will be realized.
-// The result of force-directed layout has a good symmetries and clustering, which is also aesthetically pleasing.
-type GraphForce struct {
- // The initial layout before force-directed layout, which will influence on the result of force-directed layout.
- // It defaults not to do any layout and use x, y provided in node as the position of node.
- // If it doesn't exist, the position will be generated randomly.
- // You can also use circular layout "circular".
- InitLayout string `json:"initLayout,omitempty"`
-
- // The repulsion factor between nodes. The repulsion will be stronger and the distance
- // between 2 nodes becomes further as this value becomes larger.
- // It can be an array to represent the range of repulsion. In this case larger value have larger
- // repulsion and smaller value will have smaller repulsion.
- Repulsion float32 `json:"repulsion,omitempty"`
-
- // The gravity factor enforcing nodes approach to the center. The nodes will be
- // closer to the center as the value becomes larger. default 0.1
- Gravity float32 `json:"gravity,omitempty"`
-
- // The distance between 2 nodes on edge. This distance is also affected by repulsion.
- // It can be an array to represent the range of edge length. In this case edge with larger
- // value will be shorter, which means two nodes are closer. And edge with smaller value will be longer.
- // default 30
- EdgeLength float32 `json:"edgeLength,omitempty"`
-}
-
-// Leaf node special configuration, the leaf node and non-leaf node label location is different.
-type TreeLeaves struct {
- // The style setting of the text label in a single bar.
- Label *Label `json:"label,omitempty"`
-
- // LineStyle settings in this series data.
- LineStyle *LineStyle `json:"lineStyle,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // Emphasis settings in this series data.
- Emphasis *Emphasis `json:"emphasis,omitempty"`
-}
-
-// TreeMapLevel is level specific configuration.
-type TreeMapLevel struct {
- // Color defines a list for a node level, if empty, retreived from global color list.
- Color []string `json:"color,omitempty"`
-
- // ColorAlpha indicates the range of tranparent rate (color alpha) for nodes in a level.
- ColorAlpha []float32 `json:"colorAlpha,omitempty"`
-
- // ColorSaturation indicates the range of saturation (color alpha) for nodes in a level.
- ColorSaturation []float32 `json:"colorSaturation,omitempty"`
-
- // ColorMappingBy specifies the rule according to which each node obtain color from color list.
- ColorMappingBy string `json:"colorMappingBy,omitempty"`
-
- // UpperLabel is used to specify whether show label when the treemap node has children.
- UpperLabel *UpperLabel `json:"upperLabel,omitempty"`
-
- // ItemStyle settings in this series data.
- ItemStyle *ItemStyle `json:"itemStyle,omitempty"`
-
- // Emphasis settings in this series data.
- Emphasis *Emphasis `json:"emphasis,omitempty"`
-}
-
-// UpperLabel is used to specify whether show label when the treemap node has children.
-// https://echarts.apache.org/en/option.html#series-treemap.upperLabel
-type UpperLabel struct {
- // Show is true to show upper label.
- Show bool `json:"show,omitempty"`
-
- // Position is the label's position.
- // * top
- // * left
- // * right
- // * bottom
- // * inside
- // * insideLeft
- // * insideRight
- // * insideTop
- // * insideBottom
- // * insideTopLeft
- // * insideBottomLeft
- // * insideTopRight
- // * insideBottomRight
- Position string `json:"position,omitempty"`
-
- // Distance to the host graphic element.
- // It is valid only when position is string value (like 'top', 'insideRight').
- Distance float32 `json:"distance,omitempty"`
-
- // Rotate label, from -90 degree to 90, positive value represents rotate anti-clockwise.
- Rotate float32 `json:"rotate,omitempty"`
-
- // Whether to move text slightly. For example: [30, 40] means move 30 horizontally and move 40 vertically.
- Offset []float32 `json:"offset,omitempty"`
-
- // Color is the text color
- Color string `json:"color,omitempty"`
-
- // FontStyle
- // * "normal"
- // * "italic"
- // * "oblique"
- FontStyle string `json:"fontStyle,omitempty"`
-
- // FontWeight can be the string or a number
- // * "normal"
- // * "bold"
- // * "bolder"
- // * "lighter"
- // 100 | 200 | 300| 400 ...
- FontWeight interface{} `json:"fontWeight,omitempty"`
-
- // FontSize
- FontSize float32 `json:"fontSize,omitempty"`
-
- // Align is a horizontal alignment of text, automatic by default.
- // * "left"
- // * "center"
- // * "right"
- Align string `json:"align,omitempty"`
-
- // Align is a horizontal alignment of text, automatic by default.
- // * "top"
- // * "middle"
- // * "bottom"
- VerticalAlign string `json:"verticalAlign,omitempty"`
-
- // Padding of the text fragment, for example:
- // Padding: [3, 4, 5, 6]: represents padding of [top, right, bottom, left].
- // Padding: 4: represents padding: [4, 4, 4, 4].
- // Padding: [3, 4]: represents padding: [3, 4, 3, 4].
- Padding interface{} `json:"padding,omitempty"`
-
- // Width of text block
- Width float32 `json:"width,omitempty"`
-
- // Height of text block
- Height float32 `json:"height,omitempty"`
-
- // Upper label formatter, which supports string template and callback function.
- // In either form, \n is supported to represent a new line.
- // String template, Model variation includes:
- //
- // {a}: series name.
- // {b}: the name of a data item.
- // {c}: the value of a data item.
- // {@xxx}: the value of a dimension named"xxx", for example,{@product}refers the value of"product"` dimension.
- // {@[n]}: the value of a dimension at the index ofn, for example,{@[3]}` refers the value at dimensions[3].
- Formatter string `json:"formatter,omitempty"`
-}
-
-// RGBColor returns the color with RGB format
-func RGBColor(r, g, b uint16) string {
- return fmt.Sprintf("rgb(%d,%d,%d)", r, g, b)
-}
-
-// RGBAColor returns the color with RGBA format
-func RGBAColor(r, g, b uint16, a float32) string {
- return fmt.Sprintf("rgba(%d,%d,%d,%f)", r, g, b, a)
-}
-
-// HSLColor returns the color with HSL format
-func HSLColor(h, s, l float32) string {
- return fmt.Sprintf("hsl(%f,%f%%,%f%%)", h, s, l)
-}
-
-// HSLAColor returns the color with HSLA format
-func HSLAColor(h, s, l, a float32) string {
- return fmt.Sprintf("hsla(%f,%f%%,%f%%,%f)", h, s, l, a)
-}
-
-// EdgeLabel is the properties of an label of edge.
-// https://echarts.apache.org/en/option.html#series-graph.edgeLabel
-type EdgeLabel struct {
-
- // Show is true to show label on edge.
- Show bool `json:"show,omitempty"`
-
- // Position is the label's position in line of edge.
- // * "start"
- // * "middle"
- // * "end"
- Position string `json:"position,omitempty"`
-
- // Color is the text color
- Color string `json:"color,omitempty"`
-
- // FontStyle
- // * "normal"
- // * "italic"
- // * "oblique"
- FontStyle string `json:"fontStyle,omitempty"`
-
- // FontWeight can be the string or a number
- // * "normal"
- // * "bold"
- // * "bolder"
- // * "lighter"
- // 100 | 200 | 300| 400 ...
- FontWeight interface{} `json:"fontWeight,omitempty"`
-
- // FontSize
- FontSize float32 `json:"fontSize,omitempty"`
-
- // Align is a horizontal alignment of text, automatic by default.
- // * "left"
- // * "center"
- // * "right"
- Align string `json:"align,omitempty"`
-
- // Align is a horizontal alignment of text, automatic by default.
- // * "top"
- // * "middle"
- // * "bottom"
- VerticalAlign string `json:"verticalAlign,omitempty"`
-
- // Padding of the text fragment, for example:
- // Padding: [3, 4, 5, 6]: represents padding of [top, right, bottom, left].
- // Padding: 4: represents padding: [4, 4, 4, 4].
- // Padding: [3, 4]: represents padding: [3, 4, 3, 4].
- Padding interface{} `json:"padding,omitempty"`
-
- // Width of text block
- Width float32 `json:"width,omitempty"`
-
- // Height of text block
- Height float32 `json:"height,omitempty"`
-
- // Edge label formatter, which supports string template and callback function.
- // In either form, \n is supported to represent a new line.
- // String template, Model variation includes:
- //
- // {a}: series name.
- // {b}: the name of a data item.
- // {c}: the value of a data item.
- // {@xxx}: the value of a dimension named"xxx", for example,{@product}refers the value of"product"` dimension.
- // {@[n]}: the value of a dimension at the index ofn, for example,{@[3]}` refers the value at dimensions[3].
- Formatter string `json:"formatter,omitempty"`
-}
-
-// Define what is encoded to for each dimension of data
-// https://echarts.apache.org/en/option.html#series-candlestick.encode
-type Encode struct {
- X interface{} `json:"x"`
-
- Y interface{} `json:"y"`
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/render/engine.go b/vendor/github.com/go-echarts/go-echarts/v2/render/engine.go
deleted file mode 100644
index fb01a6d4..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/render/engine.go
+++ /dev/null
@@ -1,101 +0,0 @@
-package render
-
-import (
- "bytes"
- "fmt"
- "html/template"
- "io"
- "regexp"
-
- tpls "github.com/go-echarts/go-echarts/v2/templates"
-)
-
-// Renderer
-// Any kinds of charts have their render implementation and
-// you can define your own render logic easily.
-type Renderer interface {
- Render(w io.Writer) error
-}
-
-const (
- ModChart = "chart"
- ModPage = "page"
-)
-
-var (
- pat = regexp.MustCompile(`(__f__")|("__f__)|(__f__)`)
-)
-
-type pageRender struct {
- c interface{}
- before []func()
-}
-
-// NewPageRender returns a render implementation for Page.
-func NewPageRender(c interface{}, before ...func()) Renderer {
- return &pageRender{c: c, before: before}
-}
-
-// Render renders the page into the given io.Writer.
-func (r *pageRender) Render(w io.Writer) error {
- for _, fn := range r.before {
- fn()
- }
-
- contents := []string{tpls.HeaderTpl, tpls.BaseTpl, tpls.PageTpl}
- tpl := MustTemplate(ModPage, contents)
-
- var buf bytes.Buffer
- if err := tpl.ExecuteTemplate(&buf, ModPage, r.c); err != nil {
- return err
- }
-
- content := pat.ReplaceAll(buf.Bytes(), []byte(""))
-
- _, err := w.Write(content)
- return err
-}
-
-type chartRender struct {
- c interface{}
- before []func()
-}
-
-// NewChartRender returns a render implementation for Chart.
-func NewChartRender(c interface{}, before ...func()) Renderer {
- return &chartRender{c: c, before: before}
-}
-
-// Render renders the chart into the given io.Writer.
-func (r *chartRender) Render(w io.Writer) error {
- for _, fn := range r.before {
- fn()
- }
-
- contents := []string{tpls.HeaderTpl, tpls.BaseTpl, tpls.ChartTpl}
- tpl := MustTemplate(ModChart, contents)
-
- var buf bytes.Buffer
- if err := tpl.ExecuteTemplate(&buf, ModChart, r.c); err != nil {
- return err
- }
-
- content := pat.ReplaceAll(buf.Bytes(), []byte(""))
-
- _, err := w.Write(content)
- return err
-}
-
-// MustTemplate creates a new template with the given name and parsed contents.
-func MustTemplate(name string, contents []string) *template.Template {
- tpl := template.Must(template.New(name).Parse(contents[0])).Funcs(template.FuncMap{
- "safeJS": func(s interface{}) template.JS {
- return template.JS(fmt.Sprint(s))
- },
- })
-
- for _, cont := range contents[1:] {
- tpl = template.Must(tpl.Parse(cont))
- }
- return tpl
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/templates/base.go b/vendor/github.com/go-echarts/go-echarts/v2/templates/base.go
deleted file mode 100644
index f89a1087..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/templates/base.go
+++ /dev/null
@@ -1,22 +0,0 @@
-package templates
-
-var BaseTpl = `
-{{- define "base" }}
-
-
-
-{{ end }}
-`
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/templates/chart.go b/vendor/github.com/go-echarts/go-echarts/v2/templates/chart.go
deleted file mode 100644
index bcaa55d1..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/templates/chart.go
+++ /dev/null
@@ -1,17 +0,0 @@
-package templates
-
-var ChartTpl = `
-{{- define "chart" }}
-
-
- {{- template "header" . }}
-
- {{- template "base" . }}
-
-
-
-{{ end }}
-`
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/templates/header.go b/vendor/github.com/go-echarts/go-echarts/v2/templates/header.go
deleted file mode 100644
index e6dfc44e..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/templates/header.go
+++ /dev/null
@@ -1,22 +0,0 @@
-package templates
-
-var HeaderTpl = `
-{{ define "header" }}
-
-
- {{ .PageTitle }}
-{{- range .JSAssets.Values }}
-
-{{- end }}
-{{- range .CustomizedJSAssets.Values }}
-
-{{- end }}
-{{- range .CSSAssets.Values }}
-
-{{- end }}
-{{- range .CustomizedCSSAssets.Values }}
-
-{{- end }}
-
-{{ end }}
-`
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/templates/page.go b/vendor/github.com/go-echarts/go-echarts/v2/templates/page.go
deleted file mode 100644
index 139ba902..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/templates/page.go
+++ /dev/null
@@ -1,25 +0,0 @@
-package templates
-
-var PageTpl = `
-{{- define "page" }}
-
-
- {{- template "header" . }}
-
-{{ if eq .Layout "none" }}
- {{- range .Charts }} {{ template "base" . }} {{- end }}
-{{ end }}
-
-{{ if eq .Layout "center" }}
-
- {{- range .Charts }} {{ template "base" . }} {{- end }}
-{{ end }}
-
-{{ if eq .Layout "flex" }}
-
- {{- range .Charts }} {{ template "base" . }} {{- end }}
-{{ end }}
-
-
-{{ end }}
-`
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/types/charts.go b/vendor/github.com/go-echarts/go-echarts/v2/types/charts.go
deleted file mode 100644
index 5e0deeb8..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/types/charts.go
+++ /dev/null
@@ -1,32 +0,0 @@
-package types
-
-// Chart Type contains string representations of chart types.
-const (
- ChartBar = "bar"
- ChartBar3D = "bar3D"
- ChartBoxPlot = "boxplot"
- ChartCartesian3D = "cartesian3D"
- ChartEffectScatter = "effectScatter"
- ChartFunnel = "funnel"
- ChartGauge = "gauge"
- ChartGeo = "geo"
- ChartGraph = "graph"
- ChartHeatMap = "heatmap"
- ChartKline = "candlestick"
- ChartLine = "line"
- ChartLine3D = "line3D"
- ChartLiquid = "liquidFill"
- ChartMap = "map"
- ChartParallel = "parallel"
- ChartPie = "pie"
- ChartRadar = "radar"
- ChartSankey = "sankey"
- ChartScatter = "scatter"
- ChartScatter3D = "scatter3D"
- ChartSurface3D = "surface"
- ChartThemeRiver = "themeRiver"
- ChartWordCloud = "wordCloud"
- ChartTree = "tree"
- ChartTreeMap = "treemap"
- ChartSunburst = "sunburst"
-)
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/types/orderedset.go b/vendor/github.com/go-echarts/go-echarts/v2/types/orderedset.go
deleted file mode 100644
index 6ffc54fc..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/types/orderedset.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package types
-
-// OrderedSet represents an ordered set.
-type OrderedSet struct {
- filter map[string]bool
- Values []string
-}
-
-// Init creates a new OrderedSet instance, and adds any given items into this set.
-func (o *OrderedSet) Init(items ...string) {
- o.filter = make(map[string]bool)
- for _, item := range items {
- o.Add(item)
- }
-}
-
-// Add adds a new item into the ordered set.
-func (o *OrderedSet) Add(item string) {
- if !o.filter[item] {
- o.filter[item] = true
- o.Values = append(o.Values, item)
- }
-}
diff --git a/vendor/github.com/go-echarts/go-echarts/v2/types/themes.go b/vendor/github.com/go-echarts/go-echarts/v2/types/themes.go
deleted file mode 100644
index 28f5f735..00000000
--- a/vendor/github.com/go-echarts/go-echarts/v2/types/themes.go
+++ /dev/null
@@ -1,17 +0,0 @@
-package types
-
-// Theme Type contains string representations of theme types.
-const (
- ThemeChalk = "chalk"
- ThemeEssos = "essos"
- ThemeInfographic = "infographic"
- ThemeMacarons = "macarons"
- ThemePurplePassion = "purple-passion"
- ThemeRoma = "roma"
- ThemeRomantic = "romantic"
- ThemeShine = "shine"
- ThemeVintage = "vintage"
- ThemeWalden = "walden"
- ThemeWesteros = "westeros"
- ThemeWonderland = "wonderland"
-)
diff --git a/vendor/github.com/go-openapi/jsonpointer/.editorconfig b/vendor/github.com/go-openapi/jsonpointer/.editorconfig
deleted file mode 100644
index 3152da69..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/.editorconfig
+++ /dev/null
@@ -1,26 +0,0 @@
-# top-most EditorConfig file
-root = true
-
-# Unix-style newlines with a newline ending every file
-[*]
-end_of_line = lf
-insert_final_newline = true
-indent_style = space
-indent_size = 2
-trim_trailing_whitespace = true
-
-# Set default charset
-[*.{js,py,go,scala,rb,java,html,css,less,sass,md}]
-charset = utf-8
-
-# Tab indentation (no size specified)
-[*.go]
-indent_style = tab
-
-[*.md]
-trim_trailing_whitespace = false
-
-# Matches the exact files either package.json or .travis.yml
-[{package.json,.travis.yml}]
-indent_style = space
-indent_size = 2
diff --git a/vendor/github.com/go-openapi/jsonpointer/.gitignore b/vendor/github.com/go-openapi/jsonpointer/.gitignore
deleted file mode 100644
index 769c2440..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/.gitignore
+++ /dev/null
@@ -1 +0,0 @@
-secrets.yml
diff --git a/vendor/github.com/go-openapi/jsonpointer/.travis.yml b/vendor/github.com/go-openapi/jsonpointer/.travis.yml
deleted file mode 100644
index 03a22fe0..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/.travis.yml
+++ /dev/null
@@ -1,15 +0,0 @@
-after_success:
-- bash <(curl -s https://codecov.io/bash)
-go:
-- 1.14.x
-- 1.15.x
-install:
-- GO111MODULE=off go get -u gotest.tools/gotestsum
-env:
-- GO111MODULE=on
-language: go
-notifications:
- slack:
- secure: a5VgoiwB1G/AZqzmephPZIhEB9avMlsWSlVnM1dSAtYAwdrQHGTQxAmpOxYIoSPDhWNN5bfZmjd29++UlTwLcHSR+e0kJhH6IfDlsHj/HplNCJ9tyI0zYc7XchtdKgeMxMzBKCzgwFXGSbQGydXTliDNBo0HOzmY3cou/daMFTP60K+offcjS+3LRAYb1EroSRXZqrk1nuF/xDL3792DZUdPMiFR/L/Df6y74D6/QP4sTkTDFQitz4Wy/7jbsfj8dG6qK2zivgV6/l+w4OVjFkxVpPXogDWY10vVXNVynqxfJ7to2d1I9lNCHE2ilBCkWMIPdyJF7hjF8pKW+82yP4EzRh0vu8Xn0HT5MZpQxdRY/YMxNrWaG7SxsoEaO4q5uhgdzAqLYY3TRa7MjIK+7Ur+aqOeTXn6OKwVi0CjvZ6mIU3WUKSwiwkFZMbjRAkSb5CYwMEfGFO/z964xz83qGt6WAtBXNotqCQpTIiKtDHQeLOMfksHImCg6JLhQcWBVxamVgu0G3Pdh8Y6DyPnxraXY95+QDavbjqv7TeYT9T/FNnrkXaTTK0s4iWE5H4ACU0Qvz0wUYgfQrZv0/Hp7V17+rabUwnzYySHCy9SWX/7OV9Cfh31iMp9ZIffr76xmmThtOEqs8TrTtU6BWI3rWwvA9cXQipZTVtL0oswrGw=
-script:
-- gotestsum -f short-verbose -- -race -coverprofile=coverage.txt -covermode=atomic ./...
diff --git a/vendor/github.com/go-openapi/jsonpointer/CODE_OF_CONDUCT.md b/vendor/github.com/go-openapi/jsonpointer/CODE_OF_CONDUCT.md
deleted file mode 100644
index 9322b065..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/CODE_OF_CONDUCT.md
+++ /dev/null
@@ -1,74 +0,0 @@
-# Contributor Covenant Code of Conduct
-
-## Our Pledge
-
-In the interest of fostering an open and welcoming environment, we as
-contributors and maintainers pledge to making participation in our project and
-our community a harassment-free experience for everyone, regardless of age, body
-size, disability, ethnicity, gender identity and expression, level of experience,
-nationality, personal appearance, race, religion, or sexual identity and
-orientation.
-
-## Our Standards
-
-Examples of behavior that contributes to creating a positive environment
-include:
-
-* Using welcoming and inclusive language
-* Being respectful of differing viewpoints and experiences
-* Gracefully accepting constructive criticism
-* Focusing on what is best for the community
-* Showing empathy towards other community members
-
-Examples of unacceptable behavior by participants include:
-
-* The use of sexualized language or imagery and unwelcome sexual attention or
-advances
-* Trolling, insulting/derogatory comments, and personal or political attacks
-* Public or private harassment
-* Publishing others' private information, such as a physical or electronic
- address, without explicit permission
-* Other conduct which could reasonably be considered inappropriate in a
- professional setting
-
-## Our Responsibilities
-
-Project maintainers are responsible for clarifying the standards of acceptable
-behavior and are expected to take appropriate and fair corrective action in
-response to any instances of unacceptable behavior.
-
-Project maintainers have the right and responsibility to remove, edit, or
-reject comments, commits, code, wiki edits, issues, and other contributions
-that are not aligned to this Code of Conduct, or to ban temporarily or
-permanently any contributor for other behaviors that they deem inappropriate,
-threatening, offensive, or harmful.
-
-## Scope
-
-This Code of Conduct applies both within project spaces and in public spaces
-when an individual is representing the project or its community. Examples of
-representing a project or community include using an official project e-mail
-address, posting via an official social media account, or acting as an appointed
-representative at an online or offline event. Representation of a project may be
-further defined and clarified by project maintainers.
-
-## Enforcement
-
-Instances of abusive, harassing, or otherwise unacceptable behavior may be
-reported by contacting the project team at ivan+abuse@flanders.co.nz. All
-complaints will be reviewed and investigated and will result in a response that
-is deemed necessary and appropriate to the circumstances. The project team is
-obligated to maintain confidentiality with regard to the reporter of an incident.
-Further details of specific enforcement policies may be posted separately.
-
-Project maintainers who do not follow or enforce the Code of Conduct in good
-faith may face temporary or permanent repercussions as determined by other
-members of the project's leadership.
-
-## Attribution
-
-This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
-available at [http://contributor-covenant.org/version/1/4][version]
-
-[homepage]: http://contributor-covenant.org
-[version]: http://contributor-covenant.org/version/1/4/
diff --git a/vendor/github.com/go-openapi/jsonpointer/LICENSE b/vendor/github.com/go-openapi/jsonpointer/LICENSE
deleted file mode 100644
index d6456956..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/LICENSE
+++ /dev/null
@@ -1,202 +0,0 @@
-
- Apache License
- Version 2.0, January 2004
- http://www.apache.org/licenses/
-
- TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
-
- 1. Definitions.
-
- "License" shall mean the terms and conditions for use, reproduction,
- and distribution as defined by Sections 1 through 9 of this document.
-
- "Licensor" shall mean the copyright owner or entity authorized by
- the copyright owner that is granting the License.
-
- "Legal Entity" shall mean the union of the acting entity and all
- other entities that control, are controlled by, or are under common
- control with that entity. For the purposes of this definition,
- "control" means (i) the power, direct or indirect, to cause the
- direction or management of such entity, whether by contract or
- otherwise, or (ii) ownership of fifty percent (50%) or more of the
- outstanding shares, or (iii) beneficial ownership of such entity.
-
- "You" (or "Your") shall mean an individual or Legal Entity
- exercising permissions granted by this License.
-
- "Source" form shall mean the preferred form for making modifications,
- including but not limited to software source code, documentation
- source, and configuration files.
-
- "Object" form shall mean any form resulting from mechanical
- transformation or translation of a Source form, including but
- not limited to compiled object code, generated documentation,
- and conversions to other media types.
-
- "Work" shall mean the work of authorship, whether in Source or
- Object form, made available under the License, as indicated by a
- copyright notice that is included in or attached to the work
- (an example is provided in the Appendix below).
-
- "Derivative Works" shall mean any work, whether in Source or Object
- form, that is based on (or derived from) the Work and for which the
- editorial revisions, annotations, elaborations, or other modifications
- represent, as a whole, an original work of authorship. For the purposes
- of this License, Derivative Works shall not include works that remain
- separable from, or merely link (or bind by name) to the interfaces of,
- the Work and Derivative Works thereof.
-
- "Contribution" shall mean any work of authorship, including
- the original version of the Work and any modifications or additions
- to that Work or Derivative Works thereof, that is intentionally
- submitted to Licensor for inclusion in the Work by the copyright owner
- or by an individual or Legal Entity authorized to submit on behalf of
- the copyright owner. For the purposes of this definition, "submitted"
- means any form of electronic, verbal, or written communication sent
- to the Licensor or its representatives, including but not limited to
- communication on electronic mailing lists, source code control systems,
- and issue tracking systems that are managed by, or on behalf of, the
- Licensor for the purpose of discussing and improving the Work, but
- excluding communication that is conspicuously marked or otherwise
- designated in writing by the copyright owner as "Not a Contribution."
-
- "Contributor" shall mean Licensor and any individual or Legal Entity
- on behalf of whom a Contribution has been received by Licensor and
- subsequently incorporated within the Work.
-
- 2. Grant of Copyright License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- copyright license to reproduce, prepare Derivative Works of,
- publicly display, publicly perform, sublicense, and distribute the
- Work and such Derivative Works in Source or Object form.
-
- 3. Grant of Patent License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- (except as stated in this section) patent license to make, have made,
- use, offer to sell, sell, import, and otherwise transfer the Work,
- where such license applies only to those patent claims licensable
- by such Contributor that are necessarily infringed by their
- Contribution(s) alone or by combination of their Contribution(s)
- with the Work to which such Contribution(s) was submitted. If You
- institute patent litigation against any entity (including a
- cross-claim or counterclaim in a lawsuit) alleging that the Work
- or a Contribution incorporated within the Work constitutes direct
- or contributory patent infringement, then any patent licenses
- granted to You under this License for that Work shall terminate
- as of the date such litigation is filed.
-
- 4. Redistribution. You may reproduce and distribute copies of the
- Work or Derivative Works thereof in any medium, with or without
- modifications, and in Source or Object form, provided that You
- meet the following conditions:
-
- (a) You must give any other recipients of the Work or
- Derivative Works a copy of this License; and
-
- (b) You must cause any modified files to carry prominent notices
- stating that You changed the files; and
-
- (c) You must retain, in the Source form of any Derivative Works
- that You distribute, all copyright, patent, trademark, and
- attribution notices from the Source form of the Work,
- excluding those notices that do not pertain to any part of
- the Derivative Works; and
-
- (d) If the Work includes a "NOTICE" text file as part of its
- distribution, then any Derivative Works that You distribute must
- include a readable copy of the attribution notices contained
- within such NOTICE file, excluding those notices that do not
- pertain to any part of the Derivative Works, in at least one
- of the following places: within a NOTICE text file distributed
- as part of the Derivative Works; within the Source form or
- documentation, if provided along with the Derivative Works; or,
- within a display generated by the Derivative Works, if and
- wherever such third-party notices normally appear. The contents
- of the NOTICE file are for informational purposes only and
- do not modify the License. You may add Your own attribution
- notices within Derivative Works that You distribute, alongside
- or as an addendum to the NOTICE text from the Work, provided
- that such additional attribution notices cannot be construed
- as modifying the License.
-
- You may add Your own copyright statement to Your modifications and
- may provide additional or different license terms and conditions
- for use, reproduction, or distribution of Your modifications, or
- for any such Derivative Works as a whole, provided Your use,
- reproduction, and distribution of the Work otherwise complies with
- the conditions stated in this License.
-
- 5. Submission of Contributions. Unless You explicitly state otherwise,
- any Contribution intentionally submitted for inclusion in the Work
- by You to the Licensor shall be under the terms and conditions of
- this License, without any additional terms or conditions.
- Notwithstanding the above, nothing herein shall supersede or modify
- the terms of any separate license agreement you may have executed
- with Licensor regarding such Contributions.
-
- 6. Trademarks. This License does not grant permission to use the trade
- names, trademarks, service marks, or product names of the Licensor,
- except as required for reasonable and customary use in describing the
- origin of the Work and reproducing the content of the NOTICE file.
-
- 7. Disclaimer of Warranty. Unless required by applicable law or
- agreed to in writing, Licensor provides the Work (and each
- Contributor provides its Contributions) on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
- implied, including, without limitation, any warranties or conditions
- of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
- PARTICULAR PURPOSE. You are solely responsible for determining the
- appropriateness of using or redistributing the Work and assume any
- risks associated with Your exercise of permissions under this License.
-
- 8. Limitation of Liability. In no event and under no legal theory,
- whether in tort (including negligence), contract, or otherwise,
- unless required by applicable law (such as deliberate and grossly
- negligent acts) or agreed to in writing, shall any Contributor be
- liable to You for damages, including any direct, indirect, special,
- incidental, or consequential damages of any character arising as a
- result of this License or out of the use or inability to use the
- Work (including but not limited to damages for loss of goodwill,
- work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses), even if such Contributor
- has been advised of the possibility of such damages.
-
- 9. Accepting Warranty or Additional Liability. While redistributing
- the Work or Derivative Works thereof, You may choose to offer,
- and charge a fee for, acceptance of support, warranty, indemnity,
- or other liability obligations and/or rights consistent with this
- License. However, in accepting such obligations, You may act only
- on Your own behalf and on Your sole responsibility, not on behalf
- of any other Contributor, and only if You agree to indemnify,
- defend, and hold each Contributor harmless for any liability
- incurred by, or claims asserted against, such Contributor by reason
- of your accepting any such warranty or additional liability.
-
- END OF TERMS AND CONDITIONS
-
- APPENDIX: How to apply the Apache License to your work.
-
- To apply the Apache License to your work, attach the following
- boilerplate notice, with the fields enclosed by brackets "[]"
- replaced with your own identifying information. (Don't include
- the brackets!) The text should be enclosed in the appropriate
- comment syntax for the file format. We also recommend that a
- file or class name and description of purpose be included on the
- same "printed page" as the copyright notice for easier
- identification within third-party archives.
-
- Copyright [yyyy] [name of copyright owner]
-
- Licensed under the Apache License, Version 2.0 (the "License");
- you may not use this file except in compliance with the License.
- You may obtain a copy of the License at
-
- http://www.apache.org/licenses/LICENSE-2.0
-
- Unless required by applicable law or agreed to in writing, software
- distributed under the License is distributed on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- See the License for the specific language governing permissions and
- limitations under the License.
diff --git a/vendor/github.com/go-openapi/jsonpointer/README.md b/vendor/github.com/go-openapi/jsonpointer/README.md
deleted file mode 100644
index 813788af..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/README.md
+++ /dev/null
@@ -1,15 +0,0 @@
-# gojsonpointer [](https://travis-ci.org/go-openapi/jsonpointer) [](https://codecov.io/gh/go-openapi/jsonpointer) [](https://slackin.goswagger.io)
-
-[](https://raw.githubusercontent.com/go-openapi/jsonpointer/master/LICENSE) [](http://godoc.org/github.com/go-openapi/jsonpointer)
-An implementation of JSON Pointer - Go language
-
-## Status
-Completed YES
-
-Tested YES
-
-## References
-http://tools.ietf.org/html/draft-ietf-appsawg-json-pointer-07
-
-### Note
-The 4.Evaluation part of the previous reference, starting with 'If the currently referenced value is a JSON array, the reference token MUST contain either...' is not implemented.
diff --git a/vendor/github.com/go-openapi/jsonpointer/pointer.go b/vendor/github.com/go-openapi/jsonpointer/pointer.go
deleted file mode 100644
index 7df9853d..00000000
--- a/vendor/github.com/go-openapi/jsonpointer/pointer.go
+++ /dev/null
@@ -1,390 +0,0 @@
-// Copyright 2013 sigu-399 ( https://github.com/sigu-399 )
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-// author sigu-399
-// author-github https://github.com/sigu-399
-// author-mail sigu.399@gmail.com
-//
-// repository-name jsonpointer
-// repository-desc An implementation of JSON Pointer - Go language
-//
-// description Main and unique file.
-//
-// created 25-02-2013
-
-package jsonpointer
-
-import (
- "errors"
- "fmt"
- "reflect"
- "strconv"
- "strings"
-
- "github.com/go-openapi/swag"
-)
-
-const (
- emptyPointer = ``
- pointerSeparator = `/`
-
- invalidStart = `JSON pointer must be empty or start with a "` + pointerSeparator
-)
-
-var jsonPointableType = reflect.TypeOf(new(JSONPointable)).Elem()
-var jsonSetableType = reflect.TypeOf(new(JSONSetable)).Elem()
-
-// JSONPointable is an interface for structs to implement when they need to customize the
-// json pointer process
-type JSONPointable interface {
- JSONLookup(string) (interface{}, error)
-}
-
-// JSONSetable is an interface for structs to implement when they need to customize the
-// json pointer process
-type JSONSetable interface {
- JSONSet(string, interface{}) error
-}
-
-// New creates a new json pointer for the given string
-func New(jsonPointerString string) (Pointer, error) {
-
- var p Pointer
- err := p.parse(jsonPointerString)
- return p, err
-
-}
-
-// Pointer the json pointer reprsentation
-type Pointer struct {
- referenceTokens []string
-}
-
-// "Constructor", parses the given string JSON pointer
-func (p *Pointer) parse(jsonPointerString string) error {
-
- var err error
-
- if jsonPointerString != emptyPointer {
- if !strings.HasPrefix(jsonPointerString, pointerSeparator) {
- err = errors.New(invalidStart)
- } else {
- referenceTokens := strings.Split(jsonPointerString, pointerSeparator)
- for _, referenceToken := range referenceTokens[1:] {
- p.referenceTokens = append(p.referenceTokens, referenceToken)
- }
- }
- }
-
- return err
-}
-
-// Get uses the pointer to retrieve a value from a JSON document
-func (p *Pointer) Get(document interface{}) (interface{}, reflect.Kind, error) {
- return p.get(document, swag.DefaultJSONNameProvider)
-}
-
-// Set uses the pointer to set a value from a JSON document
-func (p *Pointer) Set(document interface{}, value interface{}) (interface{}, error) {
- return document, p.set(document, value, swag.DefaultJSONNameProvider)
-}
-
-// GetForToken gets a value for a json pointer token 1 level deep
-func GetForToken(document interface{}, decodedToken string) (interface{}, reflect.Kind, error) {
- return getSingleImpl(document, decodedToken, swag.DefaultJSONNameProvider)
-}
-
-// SetForToken gets a value for a json pointer token 1 level deep
-func SetForToken(document interface{}, decodedToken string, value interface{}) (interface{}, error) {
- return document, setSingleImpl(document, value, decodedToken, swag.DefaultJSONNameProvider)
-}
-
-func getSingleImpl(node interface{}, decodedToken string, nameProvider *swag.NameProvider) (interface{}, reflect.Kind, error) {
- rValue := reflect.Indirect(reflect.ValueOf(node))
- kind := rValue.Kind()
-
- if rValue.Type().Implements(jsonPointableType) {
- r, err := node.(JSONPointable).JSONLookup(decodedToken)
- if err != nil {
- return nil, kind, err
- }
- return r, kind, nil
- }
-
- switch kind {
- case reflect.Struct:
- nm, ok := nameProvider.GetGoNameForType(rValue.Type(), decodedToken)
- if !ok {
- return nil, kind, fmt.Errorf("object has no field %q", decodedToken)
- }
- fld := rValue.FieldByName(nm)
- return fld.Interface(), kind, nil
-
- case reflect.Map:
- kv := reflect.ValueOf(decodedToken)
- mv := rValue.MapIndex(kv)
-
- if mv.IsValid() {
- return mv.Interface(), kind, nil
- }
- return nil, kind, fmt.Errorf("object has no key %q", decodedToken)
-
- case reflect.Slice:
- tokenIndex, err := strconv.Atoi(decodedToken)
- if err != nil {
- return nil, kind, err
- }
- sLength := rValue.Len()
- if tokenIndex < 0 || tokenIndex >= sLength {
- return nil, kind, fmt.Errorf("index out of bounds array[0,%d] index '%d'", sLength-1, tokenIndex)
- }
-
- elem := rValue.Index(tokenIndex)
- return elem.Interface(), kind, nil
-
- default:
- return nil, kind, fmt.Errorf("invalid token reference %q", decodedToken)
- }
-
-}
-
-func setSingleImpl(node, data interface{}, decodedToken string, nameProvider *swag.NameProvider) error {
- rValue := reflect.Indirect(reflect.ValueOf(node))
-
- if ns, ok := node.(JSONSetable); ok { // pointer impl
- return ns.JSONSet(decodedToken, data)
- }
-
- if rValue.Type().Implements(jsonSetableType) {
- return node.(JSONSetable).JSONSet(decodedToken, data)
- }
-
- switch rValue.Kind() {
- case reflect.Struct:
- nm, ok := nameProvider.GetGoNameForType(rValue.Type(), decodedToken)
- if !ok {
- return fmt.Errorf("object has no field %q", decodedToken)
- }
- fld := rValue.FieldByName(nm)
- if fld.IsValid() {
- fld.Set(reflect.ValueOf(data))
- }
- return nil
-
- case reflect.Map:
- kv := reflect.ValueOf(decodedToken)
- rValue.SetMapIndex(kv, reflect.ValueOf(data))
- return nil
-
- case reflect.Slice:
- tokenIndex, err := strconv.Atoi(decodedToken)
- if err != nil {
- return err
- }
- sLength := rValue.Len()
- if tokenIndex < 0 || tokenIndex >= sLength {
- return fmt.Errorf("index out of bounds array[0,%d] index '%d'", sLength, tokenIndex)
- }
-
- elem := rValue.Index(tokenIndex)
- if !elem.CanSet() {
- return fmt.Errorf("can't set slice index %s to %v", decodedToken, data)
- }
- elem.Set(reflect.ValueOf(data))
- return nil
-
- default:
- return fmt.Errorf("invalid token reference %q", decodedToken)
- }
-
-}
-
-func (p *Pointer) get(node interface{}, nameProvider *swag.NameProvider) (interface{}, reflect.Kind, error) {
-
- if nameProvider == nil {
- nameProvider = swag.DefaultJSONNameProvider
- }
-
- kind := reflect.Invalid
-
- // Full document when empty
- if len(p.referenceTokens) == 0 {
- return node, kind, nil
- }
-
- for _, token := range p.referenceTokens {
-
- decodedToken := Unescape(token)
-
- r, knd, err := getSingleImpl(node, decodedToken, nameProvider)
- if err != nil {
- return nil, knd, err
- }
- node, kind = r, knd
-
- }
-
- rValue := reflect.ValueOf(node)
- kind = rValue.Kind()
-
- return node, kind, nil
-}
-
-func (p *Pointer) set(node, data interface{}, nameProvider *swag.NameProvider) error {
- knd := reflect.ValueOf(node).Kind()
-
- if knd != reflect.Ptr && knd != reflect.Struct && knd != reflect.Map && knd != reflect.Slice && knd != reflect.Array {
- return fmt.Errorf("only structs, pointers, maps and slices are supported for setting values")
- }
-
- if nameProvider == nil {
- nameProvider = swag.DefaultJSONNameProvider
- }
-
- // Full document when empty
- if len(p.referenceTokens) == 0 {
- return nil
- }
-
- lastI := len(p.referenceTokens) - 1
- for i, token := range p.referenceTokens {
- isLastToken := i == lastI
- decodedToken := Unescape(token)
-
- if isLastToken {
-
- return setSingleImpl(node, data, decodedToken, nameProvider)
- }
-
- rValue := reflect.Indirect(reflect.ValueOf(node))
- kind := rValue.Kind()
-
- if rValue.Type().Implements(jsonPointableType) {
- r, err := node.(JSONPointable).JSONLookup(decodedToken)
- if err != nil {
- return err
- }
- fld := reflect.ValueOf(r)
- if fld.CanAddr() && fld.Kind() != reflect.Interface && fld.Kind() != reflect.Map && fld.Kind() != reflect.Slice && fld.Kind() != reflect.Ptr {
- node = fld.Addr().Interface()
- continue
- }
- node = r
- continue
- }
-
- switch kind {
- case reflect.Struct:
- nm, ok := nameProvider.GetGoNameForType(rValue.Type(), decodedToken)
- if !ok {
- return fmt.Errorf("object has no field %q", decodedToken)
- }
- fld := rValue.FieldByName(nm)
- if fld.CanAddr() && fld.Kind() != reflect.Interface && fld.Kind() != reflect.Map && fld.Kind() != reflect.Slice && fld.Kind() != reflect.Ptr {
- node = fld.Addr().Interface()
- continue
- }
- node = fld.Interface()
-
- case reflect.Map:
- kv := reflect.ValueOf(decodedToken)
- mv := rValue.MapIndex(kv)
-
- if !mv.IsValid() {
- return fmt.Errorf("object has no key %q", decodedToken)
- }
- if mv.CanAddr() && mv.Kind() != reflect.Interface && mv.Kind() != reflect.Map && mv.Kind() != reflect.Slice && mv.Kind() != reflect.Ptr {
- node = mv.Addr().Interface()
- continue
- }
- node = mv.Interface()
-
- case reflect.Slice:
- tokenIndex, err := strconv.Atoi(decodedToken)
- if err != nil {
- return err
- }
- sLength := rValue.Len()
- if tokenIndex < 0 || tokenIndex >= sLength {
- return fmt.Errorf("index out of bounds array[0,%d] index '%d'", sLength, tokenIndex)
- }
-
- elem := rValue.Index(tokenIndex)
- if elem.CanAddr() && elem.Kind() != reflect.Interface && elem.Kind() != reflect.Map && elem.Kind() != reflect.Slice && elem.Kind() != reflect.Ptr {
- node = elem.Addr().Interface()
- continue
- }
- node = elem.Interface()
-
- default:
- return fmt.Errorf("invalid token reference %q", decodedToken)
- }
-
- }
-
- return nil
-}
-
-// DecodedTokens returns the decoded tokens
-func (p *Pointer) DecodedTokens() []string {
- result := make([]string, 0, len(p.referenceTokens))
- for _, t := range p.referenceTokens {
- result = append(result, Unescape(t))
- }
- return result
-}
-
-// IsEmpty returns true if this is an empty json pointer
-// this indicates that it points to the root document
-func (p *Pointer) IsEmpty() bool {
- return len(p.referenceTokens) == 0
-}
-
-// Pointer to string representation function
-func (p *Pointer) String() string {
-
- if len(p.referenceTokens) == 0 {
- return emptyPointer
- }
-
- pointerString := pointerSeparator + strings.Join(p.referenceTokens, pointerSeparator)
-
- return pointerString
-}
-
-// Specific JSON pointer encoding here
-// ~0 => ~
-// ~1 => /
-// ... and vice versa
-
-const (
- encRefTok0 = `~0`
- encRefTok1 = `~1`
- decRefTok0 = `~`
- decRefTok1 = `/`
-)
-
-// Unescape unescapes a json pointer reference token string to the original representation
-func Unescape(token string) string {
- step1 := strings.Replace(token, encRefTok1, decRefTok1, -1)
- step2 := strings.Replace(step1, encRefTok0, decRefTok0, -1)
- return step2
-}
-
-// Escape escapes a pointer reference token string
-func Escape(token string) string {
- step1 := strings.Replace(token, decRefTok0, encRefTok0, -1)
- step2 := strings.Replace(step1, decRefTok1, encRefTok1, -1)
- return step2
-}
diff --git a/vendor/github.com/go-openapi/swag/.editorconfig b/vendor/github.com/go-openapi/swag/.editorconfig
deleted file mode 100644
index 3152da69..00000000
--- a/vendor/github.com/go-openapi/swag/.editorconfig
+++ /dev/null
@@ -1,26 +0,0 @@
-# top-most EditorConfig file
-root = true
-
-# Unix-style newlines with a newline ending every file
-[*]
-end_of_line = lf
-insert_final_newline = true
-indent_style = space
-indent_size = 2
-trim_trailing_whitespace = true
-
-# Set default charset
-[*.{js,py,go,scala,rb,java,html,css,less,sass,md}]
-charset = utf-8
-
-# Tab indentation (no size specified)
-[*.go]
-indent_style = tab
-
-[*.md]
-trim_trailing_whitespace = false
-
-# Matches the exact files either package.json or .travis.yml
-[{package.json,.travis.yml}]
-indent_style = space
-indent_size = 2
diff --git a/vendor/github.com/go-openapi/swag/.gitignore b/vendor/github.com/go-openapi/swag/.gitignore
deleted file mode 100644
index d69b53ac..00000000
--- a/vendor/github.com/go-openapi/swag/.gitignore
+++ /dev/null
@@ -1,4 +0,0 @@
-secrets.yml
-vendor
-Godeps
-.idea
diff --git a/vendor/github.com/go-openapi/swag/.golangci.yml b/vendor/github.com/go-openapi/swag/.golangci.yml
deleted file mode 100644
index 625c3d6a..00000000
--- a/vendor/github.com/go-openapi/swag/.golangci.yml
+++ /dev/null
@@ -1,22 +0,0 @@
-linters-settings:
- govet:
- check-shadowing: true
- golint:
- min-confidence: 0
- gocyclo:
- min-complexity: 25
- maligned:
- suggest-new: true
- dupl:
- threshold: 100
- goconst:
- min-len: 3
- min-occurrences: 2
-
-linters:
- enable-all: true
- disable:
- - maligned
- - lll
- - gochecknoinits
- - gochecknoglobals
diff --git a/vendor/github.com/go-openapi/swag/.travis.yml b/vendor/github.com/go-openapi/swag/.travis.yml
deleted file mode 100644
index aa26d876..00000000
--- a/vendor/github.com/go-openapi/swag/.travis.yml
+++ /dev/null
@@ -1,15 +0,0 @@
-after_success:
-- bash <(curl -s https://codecov.io/bash)
-go:
-- 1.11.x
-- 1.12.x
-install:
-- GO111MODULE=off go get -u gotest.tools/gotestsum
-env:
-- GO111MODULE=on
-language: go
-notifications:
- slack:
- secure: QUWvCkBBK09GF7YtEvHHVt70JOkdlNBG0nIKu/5qc4/nW5HP8I2w0SEf/XR2je0eED1Qe3L/AfMCWwrEj+IUZc3l4v+ju8X8R3Lomhme0Eb0jd1MTMCuPcBT47YCj0M7RON7vXtbFfm1hFJ/jLe5+9FXz0hpXsR24PJc5ZIi/ogNwkaPqG4BmndzecpSh0vc2FJPZUD9LT0I09REY/vXR0oQAalLkW0asGD5taHZTUZq/kBpsNxaAFrLM23i4mUcf33M5fjLpvx5LRICrX/57XpBrDh2TooBU6Qj3CgoY0uPRYUmSNxbVx1czNzl2JtEpb5yjoxfVPQeg0BvQM00G8LJINISR+ohrjhkZmAqchDupAX+yFrxTtORa78CtnIL6z/aTNlgwwVD8kvL/1pFA/JWYmKDmz93mV/+6wubGzNSQCstzjkFA4/iZEKewKUoRIAi/fxyscP6L/rCpmY/4llZZvrnyTqVbt6URWpopUpH4rwYqreXAtJxJsfBJIeSmUIiDIOMGkCTvyTEW3fWGmGoqWtSHLoaWDyAIGb7azb+KvfpWtEcoPFWfSWU+LGee0A/YsUhBl7ADB9A0CJEuR8q4BPpKpfLwPKSiKSAXL7zDkyjExyhtgqbSl2jS+rKIHOZNL8JkCcTP2MKMVd563C5rC5FMKqu3S9m2b6380E=
-script:
-- gotestsum -f short-verbose -- -race -coverprofile=coverage.txt -covermode=atomic ./...
diff --git a/vendor/github.com/go-openapi/swag/CODE_OF_CONDUCT.md b/vendor/github.com/go-openapi/swag/CODE_OF_CONDUCT.md
deleted file mode 100644
index 9322b065..00000000
--- a/vendor/github.com/go-openapi/swag/CODE_OF_CONDUCT.md
+++ /dev/null
@@ -1,74 +0,0 @@
-# Contributor Covenant Code of Conduct
-
-## Our Pledge
-
-In the interest of fostering an open and welcoming environment, we as
-contributors and maintainers pledge to making participation in our project and
-our community a harassment-free experience for everyone, regardless of age, body
-size, disability, ethnicity, gender identity and expression, level of experience,
-nationality, personal appearance, race, religion, or sexual identity and
-orientation.
-
-## Our Standards
-
-Examples of behavior that contributes to creating a positive environment
-include:
-
-* Using welcoming and inclusive language
-* Being respectful of differing viewpoints and experiences
-* Gracefully accepting constructive criticism
-* Focusing on what is best for the community
-* Showing empathy towards other community members
-
-Examples of unacceptable behavior by participants include:
-
-* The use of sexualized language or imagery and unwelcome sexual attention or
-advances
-* Trolling, insulting/derogatory comments, and personal or political attacks
-* Public or private harassment
-* Publishing others' private information, such as a physical or electronic
- address, without explicit permission
-* Other conduct which could reasonably be considered inappropriate in a
- professional setting
-
-## Our Responsibilities
-
-Project maintainers are responsible for clarifying the standards of acceptable
-behavior and are expected to take appropriate and fair corrective action in
-response to any instances of unacceptable behavior.
-
-Project maintainers have the right and responsibility to remove, edit, or
-reject comments, commits, code, wiki edits, issues, and other contributions
-that are not aligned to this Code of Conduct, or to ban temporarily or
-permanently any contributor for other behaviors that they deem inappropriate,
-threatening, offensive, or harmful.
-
-## Scope
-
-This Code of Conduct applies both within project spaces and in public spaces
-when an individual is representing the project or its community. Examples of
-representing a project or community include using an official project e-mail
-address, posting via an official social media account, or acting as an appointed
-representative at an online or offline event. Representation of a project may be
-further defined and clarified by project maintainers.
-
-## Enforcement
-
-Instances of abusive, harassing, or otherwise unacceptable behavior may be
-reported by contacting the project team at ivan+abuse@flanders.co.nz. All
-complaints will be reviewed and investigated and will result in a response that
-is deemed necessary and appropriate to the circumstances. The project team is
-obligated to maintain confidentiality with regard to the reporter of an incident.
-Further details of specific enforcement policies may be posted separately.
-
-Project maintainers who do not follow or enforce the Code of Conduct in good
-faith may face temporary or permanent repercussions as determined by other
-members of the project's leadership.
-
-## Attribution
-
-This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
-available at [http://contributor-covenant.org/version/1/4][version]
-
-[homepage]: http://contributor-covenant.org
-[version]: http://contributor-covenant.org/version/1/4/
diff --git a/vendor/github.com/go-openapi/swag/LICENSE b/vendor/github.com/go-openapi/swag/LICENSE
deleted file mode 100644
index d6456956..00000000
--- a/vendor/github.com/go-openapi/swag/LICENSE
+++ /dev/null
@@ -1,202 +0,0 @@
-
- Apache License
- Version 2.0, January 2004
- http://www.apache.org/licenses/
-
- TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
-
- 1. Definitions.
-
- "License" shall mean the terms and conditions for use, reproduction,
- and distribution as defined by Sections 1 through 9 of this document.
-
- "Licensor" shall mean the copyright owner or entity authorized by
- the copyright owner that is granting the License.
-
- "Legal Entity" shall mean the union of the acting entity and all
- other entities that control, are controlled by, or are under common
- control with that entity. For the purposes of this definition,
- "control" means (i) the power, direct or indirect, to cause the
- direction or management of such entity, whether by contract or
- otherwise, or (ii) ownership of fifty percent (50%) or more of the
- outstanding shares, or (iii) beneficial ownership of such entity.
-
- "You" (or "Your") shall mean an individual or Legal Entity
- exercising permissions granted by this License.
-
- "Source" form shall mean the preferred form for making modifications,
- including but not limited to software source code, documentation
- source, and configuration files.
-
- "Object" form shall mean any form resulting from mechanical
- transformation or translation of a Source form, including but
- not limited to compiled object code, generated documentation,
- and conversions to other media types.
-
- "Work" shall mean the work of authorship, whether in Source or
- Object form, made available under the License, as indicated by a
- copyright notice that is included in or attached to the work
- (an example is provided in the Appendix below).
-
- "Derivative Works" shall mean any work, whether in Source or Object
- form, that is based on (or derived from) the Work and for which the
- editorial revisions, annotations, elaborations, or other modifications
- represent, as a whole, an original work of authorship. For the purposes
- of this License, Derivative Works shall not include works that remain
- separable from, or merely link (or bind by name) to the interfaces of,
- the Work and Derivative Works thereof.
-
- "Contribution" shall mean any work of authorship, including
- the original version of the Work and any modifications or additions
- to that Work or Derivative Works thereof, that is intentionally
- submitted to Licensor for inclusion in the Work by the copyright owner
- or by an individual or Legal Entity authorized to submit on behalf of
- the copyright owner. For the purposes of this definition, "submitted"
- means any form of electronic, verbal, or written communication sent
- to the Licensor or its representatives, including but not limited to
- communication on electronic mailing lists, source code control systems,
- and issue tracking systems that are managed by, or on behalf of, the
- Licensor for the purpose of discussing and improving the Work, but
- excluding communication that is conspicuously marked or otherwise
- designated in writing by the copyright owner as "Not a Contribution."
-
- "Contributor" shall mean Licensor and any individual or Legal Entity
- on behalf of whom a Contribution has been received by Licensor and
- subsequently incorporated within the Work.
-
- 2. Grant of Copyright License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- copyright license to reproduce, prepare Derivative Works of,
- publicly display, publicly perform, sublicense, and distribute the
- Work and such Derivative Works in Source or Object form.
-
- 3. Grant of Patent License. Subject to the terms and conditions of
- this License, each Contributor hereby grants to You a perpetual,
- worldwide, non-exclusive, no-charge, royalty-free, irrevocable
- (except as stated in this section) patent license to make, have made,
- use, offer to sell, sell, import, and otherwise transfer the Work,
- where such license applies only to those patent claims licensable
- by such Contributor that are necessarily infringed by their
- Contribution(s) alone or by combination of their Contribution(s)
- with the Work to which such Contribution(s) was submitted. If You
- institute patent litigation against any entity (including a
- cross-claim or counterclaim in a lawsuit) alleging that the Work
- or a Contribution incorporated within the Work constitutes direct
- or contributory patent infringement, then any patent licenses
- granted to You under this License for that Work shall terminate
- as of the date such litigation is filed.
-
- 4. Redistribution. You may reproduce and distribute copies of the
- Work or Derivative Works thereof in any medium, with or without
- modifications, and in Source or Object form, provided that You
- meet the following conditions:
-
- (a) You must give any other recipients of the Work or
- Derivative Works a copy of this License; and
-
- (b) You must cause any modified files to carry prominent notices
- stating that You changed the files; and
-
- (c) You must retain, in the Source form of any Derivative Works
- that You distribute, all copyright, patent, trademark, and
- attribution notices from the Source form of the Work,
- excluding those notices that do not pertain to any part of
- the Derivative Works; and
-
- (d) If the Work includes a "NOTICE" text file as part of its
- distribution, then any Derivative Works that You distribute must
- include a readable copy of the attribution notices contained
- within such NOTICE file, excluding those notices that do not
- pertain to any part of the Derivative Works, in at least one
- of the following places: within a NOTICE text file distributed
- as part of the Derivative Works; within the Source form or
- documentation, if provided along with the Derivative Works; or,
- within a display generated by the Derivative Works, if and
- wherever such third-party notices normally appear. The contents
- of the NOTICE file are for informational purposes only and
- do not modify the License. You may add Your own attribution
- notices within Derivative Works that You distribute, alongside
- or as an addendum to the NOTICE text from the Work, provided
- that such additional attribution notices cannot be construed
- as modifying the License.
-
- You may add Your own copyright statement to Your modifications and
- may provide additional or different license terms and conditions
- for use, reproduction, or distribution of Your modifications, or
- for any such Derivative Works as a whole, provided Your use,
- reproduction, and distribution of the Work otherwise complies with
- the conditions stated in this License.
-
- 5. Submission of Contributions. Unless You explicitly state otherwise,
- any Contribution intentionally submitted for inclusion in the Work
- by You to the Licensor shall be under the terms and conditions of
- this License, without any additional terms or conditions.
- Notwithstanding the above, nothing herein shall supersede or modify
- the terms of any separate license agreement you may have executed
- with Licensor regarding such Contributions.
-
- 6. Trademarks. This License does not grant permission to use the trade
- names, trademarks, service marks, or product names of the Licensor,
- except as required for reasonable and customary use in describing the
- origin of the Work and reproducing the content of the NOTICE file.
-
- 7. Disclaimer of Warranty. Unless required by applicable law or
- agreed to in writing, Licensor provides the Work (and each
- Contributor provides its Contributions) on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
- implied, including, without limitation, any warranties or conditions
- of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
- PARTICULAR PURPOSE. You are solely responsible for determining the
- appropriateness of using or redistributing the Work and assume any
- risks associated with Your exercise of permissions under this License.
-
- 8. Limitation of Liability. In no event and under no legal theory,
- whether in tort (including negligence), contract, or otherwise,
- unless required by applicable law (such as deliberate and grossly
- negligent acts) or agreed to in writing, shall any Contributor be
- liable to You for damages, including any direct, indirect, special,
- incidental, or consequential damages of any character arising as a
- result of this License or out of the use or inability to use the
- Work (including but not limited to damages for loss of goodwill,
- work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses), even if such Contributor
- has been advised of the possibility of such damages.
-
- 9. Accepting Warranty or Additional Liability. While redistributing
- the Work or Derivative Works thereof, You may choose to offer,
- and charge a fee for, acceptance of support, warranty, indemnity,
- or other liability obligations and/or rights consistent with this
- License. However, in accepting such obligations, You may act only
- on Your own behalf and on Your sole responsibility, not on behalf
- of any other Contributor, and only if You agree to indemnify,
- defend, and hold each Contributor harmless for any liability
- incurred by, or claims asserted against, such Contributor by reason
- of your accepting any such warranty or additional liability.
-
- END OF TERMS AND CONDITIONS
-
- APPENDIX: How to apply the Apache License to your work.
-
- To apply the Apache License to your work, attach the following
- boilerplate notice, with the fields enclosed by brackets "[]"
- replaced with your own identifying information. (Don't include
- the brackets!) The text should be enclosed in the appropriate
- comment syntax for the file format. We also recommend that a
- file or class name and description of purpose be included on the
- same "printed page" as the copyright notice for easier
- identification within third-party archives.
-
- Copyright [yyyy] [name of copyright owner]
-
- Licensed under the Apache License, Version 2.0 (the "License");
- you may not use this file except in compliance with the License.
- You may obtain a copy of the License at
-
- http://www.apache.org/licenses/LICENSE-2.0
-
- Unless required by applicable law or agreed to in writing, software
- distributed under the License is distributed on an "AS IS" BASIS,
- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
- See the License for the specific language governing permissions and
- limitations under the License.
diff --git a/vendor/github.com/go-openapi/swag/README.md b/vendor/github.com/go-openapi/swag/README.md
deleted file mode 100644
index eb60ae80..00000000
--- a/vendor/github.com/go-openapi/swag/README.md
+++ /dev/null
@@ -1,22 +0,0 @@
-# Swag [](https://travis-ci.org/go-openapi/swag) [](https://codecov.io/gh/go-openapi/swag) [](https://slackin.goswagger.io)
-
-[](https://raw.githubusercontent.com/go-openapi/swag/master/LICENSE)
-[](http://godoc.org/github.com/go-openapi/swag)
-[](https://golangci.com)
-[](https://goreportcard.com/report/github.com/go-openapi/swag)
-
-Contains a bunch of helper functions for go-openapi and go-swagger projects.
-
-You may also use it standalone for your projects.
-
-* convert between value and pointers for builtin types
-* convert from string to builtin types (wraps strconv)
-* fast json concatenation
-* search in path
-* load from file or http
-* name mangling
-
-
-This repo has only few dependencies outside of the standard library:
-
-* YAML utilities depend on gopkg.in/yaml.v2
diff --git a/vendor/github.com/go-openapi/swag/convert.go b/vendor/github.com/go-openapi/swag/convert.go
deleted file mode 100644
index 7da35c31..00000000
--- a/vendor/github.com/go-openapi/swag/convert.go
+++ /dev/null
@@ -1,208 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "math"
- "strconv"
- "strings"
-)
-
-// same as ECMA Number.MAX_SAFE_INTEGER and Number.MIN_SAFE_INTEGER
-const (
- maxJSONFloat = float64(1<<53 - 1) // 9007199254740991.0 2^53 - 1
- minJSONFloat = -float64(1<<53 - 1) //-9007199254740991.0 -2^53 - 1
- epsilon float64 = 1e-9
-)
-
-// IsFloat64AJSONInteger allow for integers [-2^53, 2^53-1] inclusive
-func IsFloat64AJSONInteger(f float64) bool {
- if math.IsNaN(f) || math.IsInf(f, 0) || f < minJSONFloat || f > maxJSONFloat {
- return false
- }
- fa := math.Abs(f)
- g := float64(uint64(f))
- ga := math.Abs(g)
-
- diff := math.Abs(f - g)
-
- // more info: https://floating-point-gui.de/errors/comparison/#look-out-for-edge-cases
- switch {
- case f == g: // best case
- return true
- case f == float64(int64(f)) || f == float64(uint64(f)): // optimistic case
- return true
- case f == 0 || g == 0 || diff < math.SmallestNonzeroFloat64: // very close to 0 values
- return diff < (epsilon * math.SmallestNonzeroFloat64)
- }
- // check the relative error
- return diff/math.Min(fa+ga, math.MaxFloat64) < epsilon
-}
-
-var evaluatesAsTrue map[string]struct{}
-
-func init() {
- evaluatesAsTrue = map[string]struct{}{
- "true": {},
- "1": {},
- "yes": {},
- "ok": {},
- "y": {},
- "on": {},
- "selected": {},
- "checked": {},
- "t": {},
- "enabled": {},
- }
-}
-
-// ConvertBool turn a string into a boolean
-func ConvertBool(str string) (bool, error) {
- _, ok := evaluatesAsTrue[strings.ToLower(str)]
- return ok, nil
-}
-
-// ConvertFloat32 turn a string into a float32
-func ConvertFloat32(str string) (float32, error) {
- f, err := strconv.ParseFloat(str, 32)
- if err != nil {
- return 0, err
- }
- return float32(f), nil
-}
-
-// ConvertFloat64 turn a string into a float64
-func ConvertFloat64(str string) (float64, error) {
- return strconv.ParseFloat(str, 64)
-}
-
-// ConvertInt8 turn a string into int8 boolean
-func ConvertInt8(str string) (int8, error) {
- i, err := strconv.ParseInt(str, 10, 8)
- if err != nil {
- return 0, err
- }
- return int8(i), nil
-}
-
-// ConvertInt16 turn a string into a int16
-func ConvertInt16(str string) (int16, error) {
- i, err := strconv.ParseInt(str, 10, 16)
- if err != nil {
- return 0, err
- }
- return int16(i), nil
-}
-
-// ConvertInt32 turn a string into a int32
-func ConvertInt32(str string) (int32, error) {
- i, err := strconv.ParseInt(str, 10, 32)
- if err != nil {
- return 0, err
- }
- return int32(i), nil
-}
-
-// ConvertInt64 turn a string into a int64
-func ConvertInt64(str string) (int64, error) {
- return strconv.ParseInt(str, 10, 64)
-}
-
-// ConvertUint8 turn a string into a uint8
-func ConvertUint8(str string) (uint8, error) {
- i, err := strconv.ParseUint(str, 10, 8)
- if err != nil {
- return 0, err
- }
- return uint8(i), nil
-}
-
-// ConvertUint16 turn a string into a uint16
-func ConvertUint16(str string) (uint16, error) {
- i, err := strconv.ParseUint(str, 10, 16)
- if err != nil {
- return 0, err
- }
- return uint16(i), nil
-}
-
-// ConvertUint32 turn a string into a uint32
-func ConvertUint32(str string) (uint32, error) {
- i, err := strconv.ParseUint(str, 10, 32)
- if err != nil {
- return 0, err
- }
- return uint32(i), nil
-}
-
-// ConvertUint64 turn a string into a uint64
-func ConvertUint64(str string) (uint64, error) {
- return strconv.ParseUint(str, 10, 64)
-}
-
-// FormatBool turns a boolean into a string
-func FormatBool(value bool) string {
- return strconv.FormatBool(value)
-}
-
-// FormatFloat32 turns a float32 into a string
-func FormatFloat32(value float32) string {
- return strconv.FormatFloat(float64(value), 'f', -1, 32)
-}
-
-// FormatFloat64 turns a float64 into a string
-func FormatFloat64(value float64) string {
- return strconv.FormatFloat(value, 'f', -1, 64)
-}
-
-// FormatInt8 turns an int8 into a string
-func FormatInt8(value int8) string {
- return strconv.FormatInt(int64(value), 10)
-}
-
-// FormatInt16 turns an int16 into a string
-func FormatInt16(value int16) string {
- return strconv.FormatInt(int64(value), 10)
-}
-
-// FormatInt32 turns an int32 into a string
-func FormatInt32(value int32) string {
- return strconv.Itoa(int(value))
-}
-
-// FormatInt64 turns an int64 into a string
-func FormatInt64(value int64) string {
- return strconv.FormatInt(value, 10)
-}
-
-// FormatUint8 turns an uint8 into a string
-func FormatUint8(value uint8) string {
- return strconv.FormatUint(uint64(value), 10)
-}
-
-// FormatUint16 turns an uint16 into a string
-func FormatUint16(value uint16) string {
- return strconv.FormatUint(uint64(value), 10)
-}
-
-// FormatUint32 turns an uint32 into a string
-func FormatUint32(value uint32) string {
- return strconv.FormatUint(uint64(value), 10)
-}
-
-// FormatUint64 turns an uint64 into a string
-func FormatUint64(value uint64) string {
- return strconv.FormatUint(value, 10)
-}
diff --git a/vendor/github.com/go-openapi/swag/convert_types.go b/vendor/github.com/go-openapi/swag/convert_types.go
deleted file mode 100644
index c95e4e78..00000000
--- a/vendor/github.com/go-openapi/swag/convert_types.go
+++ /dev/null
@@ -1,595 +0,0 @@
-package swag
-
-import "time"
-
-// This file was taken from the aws go sdk
-
-// String returns a pointer to of the string value passed in.
-func String(v string) *string {
- return &v
-}
-
-// StringValue returns the value of the string pointer passed in or
-// "" if the pointer is nil.
-func StringValue(v *string) string {
- if v != nil {
- return *v
- }
- return ""
-}
-
-// StringSlice converts a slice of string values into a slice of
-// string pointers
-func StringSlice(src []string) []*string {
- dst := make([]*string, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// StringValueSlice converts a slice of string pointers into a slice of
-// string values
-func StringValueSlice(src []*string) []string {
- dst := make([]string, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// StringMap converts a string map of string values into a string
-// map of string pointers
-func StringMap(src map[string]string) map[string]*string {
- dst := make(map[string]*string)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// StringValueMap converts a string map of string pointers into a string
-// map of string values
-func StringValueMap(src map[string]*string) map[string]string {
- dst := make(map[string]string)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Bool returns a pointer to of the bool value passed in.
-func Bool(v bool) *bool {
- return &v
-}
-
-// BoolValue returns the value of the bool pointer passed in or
-// false if the pointer is nil.
-func BoolValue(v *bool) bool {
- if v != nil {
- return *v
- }
- return false
-}
-
-// BoolSlice converts a slice of bool values into a slice of
-// bool pointers
-func BoolSlice(src []bool) []*bool {
- dst := make([]*bool, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// BoolValueSlice converts a slice of bool pointers into a slice of
-// bool values
-func BoolValueSlice(src []*bool) []bool {
- dst := make([]bool, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// BoolMap converts a string map of bool values into a string
-// map of bool pointers
-func BoolMap(src map[string]bool) map[string]*bool {
- dst := make(map[string]*bool)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// BoolValueMap converts a string map of bool pointers into a string
-// map of bool values
-func BoolValueMap(src map[string]*bool) map[string]bool {
- dst := make(map[string]bool)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Int returns a pointer to of the int value passed in.
-func Int(v int) *int {
- return &v
-}
-
-// IntValue returns the value of the int pointer passed in or
-// 0 if the pointer is nil.
-func IntValue(v *int) int {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// IntSlice converts a slice of int values into a slice of
-// int pointers
-func IntSlice(src []int) []*int {
- dst := make([]*int, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// IntValueSlice converts a slice of int pointers into a slice of
-// int values
-func IntValueSlice(src []*int) []int {
- dst := make([]int, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// IntMap converts a string map of int values into a string
-// map of int pointers
-func IntMap(src map[string]int) map[string]*int {
- dst := make(map[string]*int)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// IntValueMap converts a string map of int pointers into a string
-// map of int values
-func IntValueMap(src map[string]*int) map[string]int {
- dst := make(map[string]int)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Int32 returns a pointer to of the int64 value passed in.
-func Int32(v int32) *int32 {
- return &v
-}
-
-// Int32Value returns the value of the int64 pointer passed in or
-// 0 if the pointer is nil.
-func Int32Value(v *int32) int32 {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// Int32Slice converts a slice of int64 values into a slice of
-// int32 pointers
-func Int32Slice(src []int32) []*int32 {
- dst := make([]*int32, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// Int32ValueSlice converts a slice of int32 pointers into a slice of
-// int32 values
-func Int32ValueSlice(src []*int32) []int32 {
- dst := make([]int32, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// Int32Map converts a string map of int32 values into a string
-// map of int32 pointers
-func Int32Map(src map[string]int32) map[string]*int32 {
- dst := make(map[string]*int32)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// Int32ValueMap converts a string map of int32 pointers into a string
-// map of int32 values
-func Int32ValueMap(src map[string]*int32) map[string]int32 {
- dst := make(map[string]int32)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Int64 returns a pointer to of the int64 value passed in.
-func Int64(v int64) *int64 {
- return &v
-}
-
-// Int64Value returns the value of the int64 pointer passed in or
-// 0 if the pointer is nil.
-func Int64Value(v *int64) int64 {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// Int64Slice converts a slice of int64 values into a slice of
-// int64 pointers
-func Int64Slice(src []int64) []*int64 {
- dst := make([]*int64, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// Int64ValueSlice converts a slice of int64 pointers into a slice of
-// int64 values
-func Int64ValueSlice(src []*int64) []int64 {
- dst := make([]int64, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// Int64Map converts a string map of int64 values into a string
-// map of int64 pointers
-func Int64Map(src map[string]int64) map[string]*int64 {
- dst := make(map[string]*int64)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// Int64ValueMap converts a string map of int64 pointers into a string
-// map of int64 values
-func Int64ValueMap(src map[string]*int64) map[string]int64 {
- dst := make(map[string]int64)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Uint returns a pouinter to of the uint value passed in.
-func Uint(v uint) *uint {
- return &v
-}
-
-// UintValue returns the value of the uint pouinter passed in or
-// 0 if the pouinter is nil.
-func UintValue(v *uint) uint {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// UintSlice converts a slice of uint values uinto a slice of
-// uint pouinters
-func UintSlice(src []uint) []*uint {
- dst := make([]*uint, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// UintValueSlice converts a slice of uint pouinters uinto a slice of
-// uint values
-func UintValueSlice(src []*uint) []uint {
- dst := make([]uint, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// UintMap converts a string map of uint values uinto a string
-// map of uint pouinters
-func UintMap(src map[string]uint) map[string]*uint {
- dst := make(map[string]*uint)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// UintValueMap converts a string map of uint pouinters uinto a string
-// map of uint values
-func UintValueMap(src map[string]*uint) map[string]uint {
- dst := make(map[string]uint)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Uint32 returns a pouinter to of the uint64 value passed in.
-func Uint32(v uint32) *uint32 {
- return &v
-}
-
-// Uint32Value returns the value of the uint64 pouinter passed in or
-// 0 if the pouinter is nil.
-func Uint32Value(v *uint32) uint32 {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// Uint32Slice converts a slice of uint64 values uinto a slice of
-// uint32 pouinters
-func Uint32Slice(src []uint32) []*uint32 {
- dst := make([]*uint32, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// Uint32ValueSlice converts a slice of uint32 pouinters uinto a slice of
-// uint32 values
-func Uint32ValueSlice(src []*uint32) []uint32 {
- dst := make([]uint32, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// Uint32Map converts a string map of uint32 values uinto a string
-// map of uint32 pouinters
-func Uint32Map(src map[string]uint32) map[string]*uint32 {
- dst := make(map[string]*uint32)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// Uint32ValueMap converts a string map of uint32 pouinters uinto a string
-// map of uint32 values
-func Uint32ValueMap(src map[string]*uint32) map[string]uint32 {
- dst := make(map[string]uint32)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Uint64 returns a pouinter to of the uint64 value passed in.
-func Uint64(v uint64) *uint64 {
- return &v
-}
-
-// Uint64Value returns the value of the uint64 pouinter passed in or
-// 0 if the pouinter is nil.
-func Uint64Value(v *uint64) uint64 {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// Uint64Slice converts a slice of uint64 values uinto a slice of
-// uint64 pouinters
-func Uint64Slice(src []uint64) []*uint64 {
- dst := make([]*uint64, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// Uint64ValueSlice converts a slice of uint64 pouinters uinto a slice of
-// uint64 values
-func Uint64ValueSlice(src []*uint64) []uint64 {
- dst := make([]uint64, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// Uint64Map converts a string map of uint64 values uinto a string
-// map of uint64 pouinters
-func Uint64Map(src map[string]uint64) map[string]*uint64 {
- dst := make(map[string]*uint64)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// Uint64ValueMap converts a string map of uint64 pouinters uinto a string
-// map of uint64 values
-func Uint64ValueMap(src map[string]*uint64) map[string]uint64 {
- dst := make(map[string]uint64)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Float64 returns a pointer to of the float64 value passed in.
-func Float64(v float64) *float64 {
- return &v
-}
-
-// Float64Value returns the value of the float64 pointer passed in or
-// 0 if the pointer is nil.
-func Float64Value(v *float64) float64 {
- if v != nil {
- return *v
- }
- return 0
-}
-
-// Float64Slice converts a slice of float64 values into a slice of
-// float64 pointers
-func Float64Slice(src []float64) []*float64 {
- dst := make([]*float64, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// Float64ValueSlice converts a slice of float64 pointers into a slice of
-// float64 values
-func Float64ValueSlice(src []*float64) []float64 {
- dst := make([]float64, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// Float64Map converts a string map of float64 values into a string
-// map of float64 pointers
-func Float64Map(src map[string]float64) map[string]*float64 {
- dst := make(map[string]*float64)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// Float64ValueMap converts a string map of float64 pointers into a string
-// map of float64 values
-func Float64ValueMap(src map[string]*float64) map[string]float64 {
- dst := make(map[string]float64)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
-
-// Time returns a pointer to of the time.Time value passed in.
-func Time(v time.Time) *time.Time {
- return &v
-}
-
-// TimeValue returns the value of the time.Time pointer passed in or
-// time.Time{} if the pointer is nil.
-func TimeValue(v *time.Time) time.Time {
- if v != nil {
- return *v
- }
- return time.Time{}
-}
-
-// TimeSlice converts a slice of time.Time values into a slice of
-// time.Time pointers
-func TimeSlice(src []time.Time) []*time.Time {
- dst := make([]*time.Time, len(src))
- for i := 0; i < len(src); i++ {
- dst[i] = &(src[i])
- }
- return dst
-}
-
-// TimeValueSlice converts a slice of time.Time pointers into a slice of
-// time.Time values
-func TimeValueSlice(src []*time.Time) []time.Time {
- dst := make([]time.Time, len(src))
- for i := 0; i < len(src); i++ {
- if src[i] != nil {
- dst[i] = *(src[i])
- }
- }
- return dst
-}
-
-// TimeMap converts a string map of time.Time values into a string
-// map of time.Time pointers
-func TimeMap(src map[string]time.Time) map[string]*time.Time {
- dst := make(map[string]*time.Time)
- for k, val := range src {
- v := val
- dst[k] = &v
- }
- return dst
-}
-
-// TimeValueMap converts a string map of time.Time pointers into a string
-// map of time.Time values
-func TimeValueMap(src map[string]*time.Time) map[string]time.Time {
- dst := make(map[string]time.Time)
- for k, val := range src {
- if val != nil {
- dst[k] = *val
- }
- }
- return dst
-}
diff --git a/vendor/github.com/go-openapi/swag/doc.go b/vendor/github.com/go-openapi/swag/doc.go
deleted file mode 100644
index 8d2c8c50..00000000
--- a/vendor/github.com/go-openapi/swag/doc.go
+++ /dev/null
@@ -1,32 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-/*
-Package swag contains a bunch of helper functions for go-openapi and go-swagger projects.
-
-You may also use it standalone for your projects.
-
- * convert between value and pointers for builtin types
- * convert from string to builtin types (wraps strconv)
- * fast json concatenation
- * search in path
- * load from file or http
- * name mangling
-
-
-This repo has only few dependencies outside of the standard library:
-
- * YAML utilities depend on gopkg.in/yaml.v2
-*/
-package swag
diff --git a/vendor/github.com/go-openapi/swag/json.go b/vendor/github.com/go-openapi/swag/json.go
deleted file mode 100644
index edf93d84..00000000
--- a/vendor/github.com/go-openapi/swag/json.go
+++ /dev/null
@@ -1,312 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "bytes"
- "encoding/json"
- "log"
- "reflect"
- "strings"
- "sync"
-
- "github.com/mailru/easyjson/jlexer"
- "github.com/mailru/easyjson/jwriter"
-)
-
-// nullJSON represents a JSON object with null type
-var nullJSON = []byte("null")
-
-// DefaultJSONNameProvider the default cache for types
-var DefaultJSONNameProvider = NewNameProvider()
-
-const comma = byte(',')
-
-var closers map[byte]byte
-
-func init() {
- closers = map[byte]byte{
- '{': '}',
- '[': ']',
- }
-}
-
-type ejMarshaler interface {
- MarshalEasyJSON(w *jwriter.Writer)
-}
-
-type ejUnmarshaler interface {
- UnmarshalEasyJSON(w *jlexer.Lexer)
-}
-
-// WriteJSON writes json data, prefers finding an appropriate interface to short-circuit the marshaller
-// so it takes the fastest option available.
-func WriteJSON(data interface{}) ([]byte, error) {
- if d, ok := data.(ejMarshaler); ok {
- jw := new(jwriter.Writer)
- d.MarshalEasyJSON(jw)
- return jw.BuildBytes()
- }
- if d, ok := data.(json.Marshaler); ok {
- return d.MarshalJSON()
- }
- return json.Marshal(data)
-}
-
-// ReadJSON reads json data, prefers finding an appropriate interface to short-circuit the unmarshaller
-// so it takes the fastes option available
-func ReadJSON(data []byte, value interface{}) error {
- trimmedData := bytes.Trim(data, "\x00")
- if d, ok := value.(ejUnmarshaler); ok {
- jl := &jlexer.Lexer{Data: trimmedData}
- d.UnmarshalEasyJSON(jl)
- return jl.Error()
- }
- if d, ok := value.(json.Unmarshaler); ok {
- return d.UnmarshalJSON(trimmedData)
- }
- return json.Unmarshal(trimmedData, value)
-}
-
-// DynamicJSONToStruct converts an untyped json structure into a struct
-func DynamicJSONToStruct(data interface{}, target interface{}) error {
- // TODO: convert straight to a json typed map (mergo + iterate?)
- b, err := WriteJSON(data)
- if err != nil {
- return err
- }
- return ReadJSON(b, target)
-}
-
-// ConcatJSON concatenates multiple json objects efficiently
-func ConcatJSON(blobs ...[]byte) []byte {
- if len(blobs) == 0 {
- return nil
- }
-
- last := len(blobs) - 1
- for blobs[last] == nil || bytes.Equal(blobs[last], nullJSON) {
- // strips trailing null objects
- last--
- if last < 0 {
- // there was nothing but "null"s or nil...
- return nil
- }
- }
- if last == 0 {
- return blobs[0]
- }
-
- var opening, closing byte
- var idx, a int
- buf := bytes.NewBuffer(nil)
-
- for i, b := range blobs[:last+1] {
- if b == nil || bytes.Equal(b, nullJSON) {
- // a null object is in the list: skip it
- continue
- }
- if len(b) > 0 && opening == 0 { // is this an array or an object?
- opening, closing = b[0], closers[b[0]]
- }
-
- if opening != '{' && opening != '[' {
- continue // don't know how to concatenate non container objects
- }
-
- if len(b) < 3 { // yep empty but also the last one, so closing this thing
- if i == last && a > 0 {
- if err := buf.WriteByte(closing); err != nil {
- log.Println(err)
- }
- }
- continue
- }
-
- idx = 0
- if a > 0 { // we need to join with a comma for everything beyond the first non-empty item
- if err := buf.WriteByte(comma); err != nil {
- log.Println(err)
- }
- idx = 1 // this is not the first or the last so we want to drop the leading bracket
- }
-
- if i != last { // not the last one, strip brackets
- if _, err := buf.Write(b[idx : len(b)-1]); err != nil {
- log.Println(err)
- }
- } else { // last one, strip only the leading bracket
- if _, err := buf.Write(b[idx:]); err != nil {
- log.Println(err)
- }
- }
- a++
- }
- // somehow it ended up being empty, so provide a default value
- if buf.Len() == 0 {
- if err := buf.WriteByte(opening); err != nil {
- log.Println(err)
- }
- if err := buf.WriteByte(closing); err != nil {
- log.Println(err)
- }
- }
- return buf.Bytes()
-}
-
-// ToDynamicJSON turns an object into a properly JSON typed structure
-func ToDynamicJSON(data interface{}) interface{} {
- // TODO: convert straight to a json typed map (mergo + iterate?)
- b, err := json.Marshal(data)
- if err != nil {
- log.Println(err)
- }
- var res interface{}
- if err := json.Unmarshal(b, &res); err != nil {
- log.Println(err)
- }
- return res
-}
-
-// FromDynamicJSON turns an object into a properly JSON typed structure
-func FromDynamicJSON(data, target interface{}) error {
- b, err := json.Marshal(data)
- if err != nil {
- log.Println(err)
- }
- return json.Unmarshal(b, target)
-}
-
-// NameProvider represents an object capabale of translating from go property names
-// to json property names
-// This type is thread-safe.
-type NameProvider struct {
- lock *sync.Mutex
- index map[reflect.Type]nameIndex
-}
-
-type nameIndex struct {
- jsonNames map[string]string
- goNames map[string]string
-}
-
-// NewNameProvider creates a new name provider
-func NewNameProvider() *NameProvider {
- return &NameProvider{
- lock: &sync.Mutex{},
- index: make(map[reflect.Type]nameIndex),
- }
-}
-
-func buildnameIndex(tpe reflect.Type, idx, reverseIdx map[string]string) {
- for i := 0; i < tpe.NumField(); i++ {
- targetDes := tpe.Field(i)
-
- if targetDes.PkgPath != "" { // unexported
- continue
- }
-
- if targetDes.Anonymous { // walk embedded structures tree down first
- buildnameIndex(targetDes.Type, idx, reverseIdx)
- continue
- }
-
- if tag := targetDes.Tag.Get("json"); tag != "" {
-
- parts := strings.Split(tag, ",")
- if len(parts) == 0 {
- continue
- }
-
- nm := parts[0]
- if nm == "-" {
- continue
- }
- if nm == "" { // empty string means we want to use the Go name
- nm = targetDes.Name
- }
-
- idx[nm] = targetDes.Name
- reverseIdx[targetDes.Name] = nm
- }
- }
-}
-
-func newNameIndex(tpe reflect.Type) nameIndex {
- var idx = make(map[string]string, tpe.NumField())
- var reverseIdx = make(map[string]string, tpe.NumField())
-
- buildnameIndex(tpe, idx, reverseIdx)
- return nameIndex{jsonNames: idx, goNames: reverseIdx}
-}
-
-// GetJSONNames gets all the json property names for a type
-func (n *NameProvider) GetJSONNames(subject interface{}) []string {
- n.lock.Lock()
- defer n.lock.Unlock()
- tpe := reflect.Indirect(reflect.ValueOf(subject)).Type()
- names, ok := n.index[tpe]
- if !ok {
- names = n.makeNameIndex(tpe)
- }
-
- res := make([]string, 0, len(names.jsonNames))
- for k := range names.jsonNames {
- res = append(res, k)
- }
- return res
-}
-
-// GetJSONName gets the json name for a go property name
-func (n *NameProvider) GetJSONName(subject interface{}, name string) (string, bool) {
- tpe := reflect.Indirect(reflect.ValueOf(subject)).Type()
- return n.GetJSONNameForType(tpe, name)
-}
-
-// GetJSONNameForType gets the json name for a go property name on a given type
-func (n *NameProvider) GetJSONNameForType(tpe reflect.Type, name string) (string, bool) {
- n.lock.Lock()
- defer n.lock.Unlock()
- names, ok := n.index[tpe]
- if !ok {
- names = n.makeNameIndex(tpe)
- }
- nme, ok := names.goNames[name]
- return nme, ok
-}
-
-func (n *NameProvider) makeNameIndex(tpe reflect.Type) nameIndex {
- names := newNameIndex(tpe)
- n.index[tpe] = names
- return names
-}
-
-// GetGoName gets the go name for a json property name
-func (n *NameProvider) GetGoName(subject interface{}, name string) (string, bool) {
- tpe := reflect.Indirect(reflect.ValueOf(subject)).Type()
- return n.GetGoNameForType(tpe, name)
-}
-
-// GetGoNameForType gets the go name for a given type for a json property name
-func (n *NameProvider) GetGoNameForType(tpe reflect.Type, name string) (string, bool) {
- n.lock.Lock()
- defer n.lock.Unlock()
- names, ok := n.index[tpe]
- if !ok {
- names = n.makeNameIndex(tpe)
- }
- nme, ok := names.jsonNames[name]
- return nme, ok
-}
diff --git a/vendor/github.com/go-openapi/swag/loading.go b/vendor/github.com/go-openapi/swag/loading.go
deleted file mode 100644
index 70f4fb36..00000000
--- a/vendor/github.com/go-openapi/swag/loading.go
+++ /dev/null
@@ -1,80 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "fmt"
- "io/ioutil"
- "log"
- "net/http"
- "path/filepath"
- "strings"
- "time"
-)
-
-// LoadHTTPTimeout the default timeout for load requests
-var LoadHTTPTimeout = 30 * time.Second
-
-// LoadFromFileOrHTTP loads the bytes from a file or a remote http server based on the path passed in
-func LoadFromFileOrHTTP(path string) ([]byte, error) {
- return LoadStrategy(path, ioutil.ReadFile, loadHTTPBytes(LoadHTTPTimeout))(path)
-}
-
-// LoadFromFileOrHTTPWithTimeout loads the bytes from a file or a remote http server based on the path passed in
-// timeout arg allows for per request overriding of the request timeout
-func LoadFromFileOrHTTPWithTimeout(path string, timeout time.Duration) ([]byte, error) {
- return LoadStrategy(path, ioutil.ReadFile, loadHTTPBytes(timeout))(path)
-}
-
-// LoadStrategy returns a loader function for a given path or uri
-func LoadStrategy(path string, local, remote func(string) ([]byte, error)) func(string) ([]byte, error) {
- if strings.HasPrefix(path, "http") {
- return remote
- }
- return func(pth string) ([]byte, error) {
- upth, err := pathUnescape(pth)
- if err != nil {
- return nil, err
- }
- return local(filepath.FromSlash(upth))
- }
-}
-
-func loadHTTPBytes(timeout time.Duration) func(path string) ([]byte, error) {
- return func(path string) ([]byte, error) {
- client := &http.Client{Timeout: timeout}
- req, err := http.NewRequest("GET", path, nil)
- if err != nil {
- return nil, err
- }
- resp, err := client.Do(req)
- defer func() {
- if resp != nil {
- if e := resp.Body.Close(); e != nil {
- log.Println(e)
- }
- }
- }()
- if err != nil {
- return nil, err
- }
-
- if resp.StatusCode != http.StatusOK {
- return nil, fmt.Errorf("could not access document at %q [%s] ", path, resp.Status)
- }
-
- return ioutil.ReadAll(resp.Body)
- }
-}
diff --git a/vendor/github.com/go-openapi/swag/name_lexem.go b/vendor/github.com/go-openapi/swag/name_lexem.go
deleted file mode 100644
index aa7f6a9b..00000000
--- a/vendor/github.com/go-openapi/swag/name_lexem.go
+++ /dev/null
@@ -1,87 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import "unicode"
-
-type (
- nameLexem interface {
- GetUnsafeGoName() string
- GetOriginal() string
- IsInitialism() bool
- }
-
- initialismNameLexem struct {
- original string
- matchedInitialism string
- }
-
- casualNameLexem struct {
- original string
- }
-)
-
-func newInitialismNameLexem(original, matchedInitialism string) *initialismNameLexem {
- return &initialismNameLexem{
- original: original,
- matchedInitialism: matchedInitialism,
- }
-}
-
-func newCasualNameLexem(original string) *casualNameLexem {
- return &casualNameLexem{
- original: original,
- }
-}
-
-func (l *initialismNameLexem) GetUnsafeGoName() string {
- return l.matchedInitialism
-}
-
-func (l *casualNameLexem) GetUnsafeGoName() string {
- var first rune
- var rest string
- for i, orig := range l.original {
- if i == 0 {
- first = orig
- continue
- }
- if i > 0 {
- rest = l.original[i:]
- break
- }
- }
- if len(l.original) > 1 {
- return string(unicode.ToUpper(first)) + lower(rest)
- }
-
- return l.original
-}
-
-func (l *initialismNameLexem) GetOriginal() string {
- return l.original
-}
-
-func (l *casualNameLexem) GetOriginal() string {
- return l.original
-}
-
-func (l *initialismNameLexem) IsInitialism() bool {
- return true
-}
-
-func (l *casualNameLexem) IsInitialism() bool {
- return false
-}
diff --git a/vendor/github.com/go-openapi/swag/net.go b/vendor/github.com/go-openapi/swag/net.go
deleted file mode 100644
index 821235f8..00000000
--- a/vendor/github.com/go-openapi/swag/net.go
+++ /dev/null
@@ -1,38 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "net"
- "strconv"
-)
-
-// SplitHostPort splits a network address into a host and a port.
-// The port is -1 when there is no port to be found
-func SplitHostPort(addr string) (host string, port int, err error) {
- h, p, err := net.SplitHostPort(addr)
- if err != nil {
- return "", -1, err
- }
- if p == "" {
- return "", -1, &net.AddrError{Err: "missing port in address", Addr: addr}
- }
-
- pi, err := strconv.Atoi(p)
- if err != nil {
- return "", -1, err
- }
- return h, pi, nil
-}
diff --git a/vendor/github.com/go-openapi/swag/path.go b/vendor/github.com/go-openapi/swag/path.go
deleted file mode 100644
index 941bd017..00000000
--- a/vendor/github.com/go-openapi/swag/path.go
+++ /dev/null
@@ -1,59 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "os"
- "path/filepath"
- "runtime"
- "strings"
-)
-
-const (
- // GOPATHKey represents the env key for gopath
- GOPATHKey = "GOPATH"
-)
-
-// FindInSearchPath finds a package in a provided lists of paths
-func FindInSearchPath(searchPath, pkg string) string {
- pathsList := filepath.SplitList(searchPath)
- for _, path := range pathsList {
- if evaluatedPath, err := filepath.EvalSymlinks(filepath.Join(path, "src", pkg)); err == nil {
- if _, err := os.Stat(evaluatedPath); err == nil {
- return evaluatedPath
- }
- }
- }
- return ""
-}
-
-// FindInGoSearchPath finds a package in the $GOPATH:$GOROOT
-func FindInGoSearchPath(pkg string) string {
- return FindInSearchPath(FullGoSearchPath(), pkg)
-}
-
-// FullGoSearchPath gets the search paths for finding packages
-func FullGoSearchPath() string {
- allPaths := os.Getenv(GOPATHKey)
- if allPaths == "" {
- allPaths = filepath.Join(os.Getenv("HOME"), "go")
- }
- if allPaths != "" {
- allPaths = strings.Join([]string{allPaths, runtime.GOROOT()}, ":")
- } else {
- allPaths = runtime.GOROOT()
- }
- return allPaths
-}
diff --git a/vendor/github.com/go-openapi/swag/post_go18.go b/vendor/github.com/go-openapi/swag/post_go18.go
deleted file mode 100644
index c2e686d3..00000000
--- a/vendor/github.com/go-openapi/swag/post_go18.go
+++ /dev/null
@@ -1,23 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-// +build go1.8
-
-package swag
-
-import "net/url"
-
-func pathUnescape(path string) (string, error) {
- return url.PathUnescape(path)
-}
diff --git a/vendor/github.com/go-openapi/swag/post_go19.go b/vendor/github.com/go-openapi/swag/post_go19.go
deleted file mode 100644
index eb2f2d8b..00000000
--- a/vendor/github.com/go-openapi/swag/post_go19.go
+++ /dev/null
@@ -1,67 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-// +build go1.9
-
-package swag
-
-import (
- "sort"
- "sync"
-)
-
-// indexOfInitialisms is a thread-safe implementation of the sorted index of initialisms.
-// Since go1.9, this may be implemented with sync.Map.
-type indexOfInitialisms struct {
- sortMutex *sync.Mutex
- index *sync.Map
-}
-
-func newIndexOfInitialisms() *indexOfInitialisms {
- return &indexOfInitialisms{
- sortMutex: new(sync.Mutex),
- index: new(sync.Map),
- }
-}
-
-func (m *indexOfInitialisms) load(initial map[string]bool) *indexOfInitialisms {
- m.sortMutex.Lock()
- defer m.sortMutex.Unlock()
- for k, v := range initial {
- m.index.Store(k, v)
- }
- return m
-}
-
-func (m *indexOfInitialisms) isInitialism(key string) bool {
- _, ok := m.index.Load(key)
- return ok
-}
-
-func (m *indexOfInitialisms) add(key string) *indexOfInitialisms {
- m.index.Store(key, true)
- return m
-}
-
-func (m *indexOfInitialisms) sorted() (result []string) {
- m.sortMutex.Lock()
- defer m.sortMutex.Unlock()
- m.index.Range(func(key, value interface{}) bool {
- k := key.(string)
- result = append(result, k)
- return true
- })
- sort.Sort(sort.Reverse(byInitialism(result)))
- return
-}
diff --git a/vendor/github.com/go-openapi/swag/pre_go18.go b/vendor/github.com/go-openapi/swag/pre_go18.go
deleted file mode 100644
index 6607f339..00000000
--- a/vendor/github.com/go-openapi/swag/pre_go18.go
+++ /dev/null
@@ -1,23 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-// +build !go1.8
-
-package swag
-
-import "net/url"
-
-func pathUnescape(path string) (string, error) {
- return url.QueryUnescape(path)
-}
diff --git a/vendor/github.com/go-openapi/swag/pre_go19.go b/vendor/github.com/go-openapi/swag/pre_go19.go
deleted file mode 100644
index 4bae187d..00000000
--- a/vendor/github.com/go-openapi/swag/pre_go19.go
+++ /dev/null
@@ -1,69 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-// +build !go1.9
-
-package swag
-
-import (
- "sort"
- "sync"
-)
-
-// indexOfInitialisms is a thread-safe implementation of the sorted index of initialisms.
-// Before go1.9, this may be implemented with a mutex on the map.
-type indexOfInitialisms struct {
- getMutex *sync.Mutex
- index map[string]bool
-}
-
-func newIndexOfInitialisms() *indexOfInitialisms {
- return &indexOfInitialisms{
- getMutex: new(sync.Mutex),
- index: make(map[string]bool, 50),
- }
-}
-
-func (m *indexOfInitialisms) load(initial map[string]bool) *indexOfInitialisms {
- m.getMutex.Lock()
- defer m.getMutex.Unlock()
- for k, v := range initial {
- m.index[k] = v
- }
- return m
-}
-
-func (m *indexOfInitialisms) isInitialism(key string) bool {
- m.getMutex.Lock()
- defer m.getMutex.Unlock()
- _, ok := m.index[key]
- return ok
-}
-
-func (m *indexOfInitialisms) add(key string) *indexOfInitialisms {
- m.getMutex.Lock()
- defer m.getMutex.Unlock()
- m.index[key] = true
- return m
-}
-
-func (m *indexOfInitialisms) sorted() (result []string) {
- m.getMutex.Lock()
- defer m.getMutex.Unlock()
- for k := range m.index {
- result = append(result, k)
- }
- sort.Sort(sort.Reverse(byInitialism(result)))
- return
-}
diff --git a/vendor/github.com/go-openapi/swag/split.go b/vendor/github.com/go-openapi/swag/split.go
deleted file mode 100644
index a1825fb7..00000000
--- a/vendor/github.com/go-openapi/swag/split.go
+++ /dev/null
@@ -1,262 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "unicode"
-)
-
-var nameReplaceTable = map[rune]string{
- '@': "At ",
- '&': "And ",
- '|': "Pipe ",
- '$': "Dollar ",
- '!': "Bang ",
- '-': "",
- '_': "",
-}
-
-type (
- splitter struct {
- postSplitInitialismCheck bool
- initialisms []string
- }
-
- splitterOption func(*splitter) *splitter
-)
-
-// split calls the splitter; splitter provides more control and post options
-func split(str string) []string {
- lexems := newSplitter().split(str)
- result := make([]string, 0, len(lexems))
-
- for _, lexem := range lexems {
- result = append(result, lexem.GetOriginal())
- }
-
- return result
-
-}
-
-func (s *splitter) split(str string) []nameLexem {
- return s.toNameLexems(str)
-}
-
-func newSplitter(options ...splitterOption) *splitter {
- splitter := &splitter{
- postSplitInitialismCheck: false,
- initialisms: initialisms,
- }
-
- for _, option := range options {
- splitter = option(splitter)
- }
-
- return splitter
-}
-
-// withPostSplitInitialismCheck allows to catch initialisms after main split process
-func withPostSplitInitialismCheck(s *splitter) *splitter {
- s.postSplitInitialismCheck = true
- return s
-}
-
-type (
- initialismMatch struct {
- start, end int
- body []rune
- complete bool
- }
- initialismMatches []*initialismMatch
-)
-
-func (s *splitter) toNameLexems(name string) []nameLexem {
- nameRunes := []rune(name)
- matches := s.gatherInitialismMatches(nameRunes)
- return s.mapMatchesToNameLexems(nameRunes, matches)
-}
-
-func (s *splitter) gatherInitialismMatches(nameRunes []rune) initialismMatches {
- matches := make(initialismMatches, 0)
-
- for currentRunePosition, currentRune := range nameRunes {
- newMatches := make(initialismMatches, 0, len(matches))
-
- // check current initialism matches
- for _, match := range matches {
- if keepCompleteMatch := match.complete; keepCompleteMatch {
- newMatches = append(newMatches, match)
- continue
- }
-
- // drop failed match
- currentMatchRune := match.body[currentRunePosition-match.start]
- if !s.initialismRuneEqual(currentMatchRune, currentRune) {
- continue
- }
-
- // try to complete ongoing match
- if currentRunePosition-match.start == len(match.body)-1 {
- // we are close; the next step is to check the symbol ahead
- // if it is a small letter, then it is not the end of match
- // but beginning of the next word
-
- if currentRunePosition < len(nameRunes)-1 {
- nextRune := nameRunes[currentRunePosition+1]
- if newWord := unicode.IsLower(nextRune); newWord {
- // oh ok, it was the start of a new word
- continue
- }
- }
-
- match.complete = true
- match.end = currentRunePosition
- }
-
- newMatches = append(newMatches, match)
- }
-
- // check for new initialism matches
- for _, initialism := range s.initialisms {
- initialismRunes := []rune(initialism)
- if s.initialismRuneEqual(initialismRunes[0], currentRune) {
- newMatches = append(newMatches, &initialismMatch{
- start: currentRunePosition,
- body: initialismRunes,
- complete: false,
- })
- }
- }
-
- matches = newMatches
- }
-
- return matches
-}
-
-func (s *splitter) mapMatchesToNameLexems(nameRunes []rune, matches initialismMatches) []nameLexem {
- nameLexems := make([]nameLexem, 0)
-
- var lastAcceptedMatch *initialismMatch
- for _, match := range matches {
- if !match.complete {
- continue
- }
-
- if firstMatch := lastAcceptedMatch == nil; firstMatch {
- nameLexems = append(nameLexems, s.breakCasualString(nameRunes[:match.start])...)
- nameLexems = append(nameLexems, s.breakInitialism(string(match.body)))
-
- lastAcceptedMatch = match
-
- continue
- }
-
- if overlappedMatch := match.start <= lastAcceptedMatch.end; overlappedMatch {
- continue
- }
-
- middle := nameRunes[lastAcceptedMatch.end+1 : match.start]
- nameLexems = append(nameLexems, s.breakCasualString(middle)...)
- nameLexems = append(nameLexems, s.breakInitialism(string(match.body)))
-
- lastAcceptedMatch = match
- }
-
- // we have not found any accepted matches
- if lastAcceptedMatch == nil {
- return s.breakCasualString(nameRunes)
- }
-
- if lastAcceptedMatch.end+1 != len(nameRunes) {
- rest := nameRunes[lastAcceptedMatch.end+1:]
- nameLexems = append(nameLexems, s.breakCasualString(rest)...)
- }
-
- return nameLexems
-}
-
-func (s *splitter) initialismRuneEqual(a, b rune) bool {
- return a == b
-}
-
-func (s *splitter) breakInitialism(original string) nameLexem {
- return newInitialismNameLexem(original, original)
-}
-
-func (s *splitter) breakCasualString(str []rune) []nameLexem {
- segments := make([]nameLexem, 0)
- currentSegment := ""
-
- addCasualNameLexem := func(original string) {
- segments = append(segments, newCasualNameLexem(original))
- }
-
- addInitialismNameLexem := func(original, match string) {
- segments = append(segments, newInitialismNameLexem(original, match))
- }
-
- addNameLexem := func(original string) {
- if s.postSplitInitialismCheck {
- for _, initialism := range s.initialisms {
- if upper(initialism) == upper(original) {
- addInitialismNameLexem(original, initialism)
- return
- }
- }
- }
-
- addCasualNameLexem(original)
- }
-
- for _, rn := range string(str) {
- if replace, found := nameReplaceTable[rn]; found {
- if currentSegment != "" {
- addNameLexem(currentSegment)
- currentSegment = ""
- }
-
- if replace != "" {
- addNameLexem(replace)
- }
-
- continue
- }
-
- if !unicode.In(rn, unicode.L, unicode.M, unicode.N, unicode.Pc) {
- if currentSegment != "" {
- addNameLexem(currentSegment)
- currentSegment = ""
- }
-
- continue
- }
-
- if unicode.IsUpper(rn) {
- if currentSegment != "" {
- addNameLexem(currentSegment)
- }
- currentSegment = ""
- }
-
- currentSegment += string(rn)
- }
-
- if currentSegment != "" {
- addNameLexem(currentSegment)
- }
-
- return segments
-}
diff --git a/vendor/github.com/go-openapi/swag/util.go b/vendor/github.com/go-openapi/swag/util.go
deleted file mode 100644
index 9eac16af..00000000
--- a/vendor/github.com/go-openapi/swag/util.go
+++ /dev/null
@@ -1,385 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "reflect"
- "strings"
- "unicode"
-)
-
-// commonInitialisms are common acronyms that are kept as whole uppercased words.
-var commonInitialisms *indexOfInitialisms
-
-// initialisms is a slice of sorted initialisms
-var initialisms []string
-
-var isInitialism func(string) bool
-
-// GoNamePrefixFunc sets an optional rule to prefix go names
-// which do not start with a letter.
-//
-// e.g. to help converting "123" into "{prefix}123"
-//
-// The default is to prefix with "X"
-var GoNamePrefixFunc func(string) string
-
-func init() {
- // Taken from https://github.com/golang/lint/blob/3390df4df2787994aea98de825b964ac7944b817/lint.go#L732-L769
- var configuredInitialisms = map[string]bool{
- "ACL": true,
- "API": true,
- "ASCII": true,
- "CPU": true,
- "CSS": true,
- "DNS": true,
- "EOF": true,
- "GUID": true,
- "HTML": true,
- "HTTPS": true,
- "HTTP": true,
- "ID": true,
- "IP": true,
- "IPv4": true,
- "IPv6": true,
- "JSON": true,
- "LHS": true,
- "OAI": true,
- "QPS": true,
- "RAM": true,
- "RHS": true,
- "RPC": true,
- "SLA": true,
- "SMTP": true,
- "SQL": true,
- "SSH": true,
- "TCP": true,
- "TLS": true,
- "TTL": true,
- "UDP": true,
- "UI": true,
- "UID": true,
- "UUID": true,
- "URI": true,
- "URL": true,
- "UTF8": true,
- "VM": true,
- "XML": true,
- "XMPP": true,
- "XSRF": true,
- "XSS": true,
- }
-
- // a thread-safe index of initialisms
- commonInitialisms = newIndexOfInitialisms().load(configuredInitialisms)
- initialisms = commonInitialisms.sorted()
-
- // a test function
- isInitialism = commonInitialisms.isInitialism
-}
-
-const (
- //collectionFormatComma = "csv"
- collectionFormatSpace = "ssv"
- collectionFormatTab = "tsv"
- collectionFormatPipe = "pipes"
- collectionFormatMulti = "multi"
-)
-
-// JoinByFormat joins a string array by a known format (e.g. swagger's collectionFormat attribute):
-// ssv: space separated value
-// tsv: tab separated value
-// pipes: pipe (|) separated value
-// csv: comma separated value (default)
-func JoinByFormat(data []string, format string) []string {
- if len(data) == 0 {
- return data
- }
- var sep string
- switch format {
- case collectionFormatSpace:
- sep = " "
- case collectionFormatTab:
- sep = "\t"
- case collectionFormatPipe:
- sep = "|"
- case collectionFormatMulti:
- return data
- default:
- sep = ","
- }
- return []string{strings.Join(data, sep)}
-}
-
-// SplitByFormat splits a string by a known format:
-// ssv: space separated value
-// tsv: tab separated value
-// pipes: pipe (|) separated value
-// csv: comma separated value (default)
-//
-func SplitByFormat(data, format string) []string {
- if data == "" {
- return nil
- }
- var sep string
- switch format {
- case collectionFormatSpace:
- sep = " "
- case collectionFormatTab:
- sep = "\t"
- case collectionFormatPipe:
- sep = "|"
- case collectionFormatMulti:
- return nil
- default:
- sep = ","
- }
- var result []string
- for _, s := range strings.Split(data, sep) {
- if ts := strings.TrimSpace(s); ts != "" {
- result = append(result, ts)
- }
- }
- return result
-}
-
-type byInitialism []string
-
-func (s byInitialism) Len() int {
- return len(s)
-}
-func (s byInitialism) Swap(i, j int) {
- s[i], s[j] = s[j], s[i]
-}
-func (s byInitialism) Less(i, j int) bool {
- if len(s[i]) != len(s[j]) {
- return len(s[i]) < len(s[j])
- }
-
- return strings.Compare(s[i], s[j]) > 0
-}
-
-// Removes leading whitespaces
-func trim(str string) string {
- return strings.Trim(str, " ")
-}
-
-// Shortcut to strings.ToUpper()
-func upper(str string) string {
- return strings.ToUpper(trim(str))
-}
-
-// Shortcut to strings.ToLower()
-func lower(str string) string {
- return strings.ToLower(trim(str))
-}
-
-// Camelize an uppercased word
-func Camelize(word string) (camelized string) {
- for pos, ru := range []rune(word) {
- if pos > 0 {
- camelized += string(unicode.ToLower(ru))
- } else {
- camelized += string(unicode.ToUpper(ru))
- }
- }
- return
-}
-
-// ToFileName lowercases and underscores a go type name
-func ToFileName(name string) string {
- in := split(name)
- out := make([]string, 0, len(in))
-
- for _, w := range in {
- out = append(out, lower(w))
- }
-
- return strings.Join(out, "_")
-}
-
-// ToCommandName lowercases and underscores a go type name
-func ToCommandName(name string) string {
- in := split(name)
- out := make([]string, 0, len(in))
-
- for _, w := range in {
- out = append(out, lower(w))
- }
- return strings.Join(out, "-")
-}
-
-// ToHumanNameLower represents a code name as a human series of words
-func ToHumanNameLower(name string) string {
- in := newSplitter(withPostSplitInitialismCheck).split(name)
- out := make([]string, 0, len(in))
-
- for _, w := range in {
- if !w.IsInitialism() {
- out = append(out, lower(w.GetOriginal()))
- } else {
- out = append(out, w.GetOriginal())
- }
- }
-
- return strings.Join(out, " ")
-}
-
-// ToHumanNameTitle represents a code name as a human series of words with the first letters titleized
-func ToHumanNameTitle(name string) string {
- in := newSplitter(withPostSplitInitialismCheck).split(name)
-
- out := make([]string, 0, len(in))
- for _, w := range in {
- original := w.GetOriginal()
- if !w.IsInitialism() {
- out = append(out, Camelize(original))
- } else {
- out = append(out, original)
- }
- }
- return strings.Join(out, " ")
-}
-
-// ToJSONName camelcases a name which can be underscored or pascal cased
-func ToJSONName(name string) string {
- in := split(name)
- out := make([]string, 0, len(in))
-
- for i, w := range in {
- if i == 0 {
- out = append(out, lower(w))
- continue
- }
- out = append(out, Camelize(w))
- }
- return strings.Join(out, "")
-}
-
-// ToVarName camelcases a name which can be underscored or pascal cased
-func ToVarName(name string) string {
- res := ToGoName(name)
- if isInitialism(res) {
- return lower(res)
- }
- if len(res) <= 1 {
- return lower(res)
- }
- return lower(res[:1]) + res[1:]
-}
-
-// ToGoName translates a swagger name which can be underscored or camel cased to a name that golint likes
-func ToGoName(name string) string {
- lexems := newSplitter(withPostSplitInitialismCheck).split(name)
-
- result := ""
- for _, lexem := range lexems {
- goName := lexem.GetUnsafeGoName()
-
- // to support old behavior
- if lexem.IsInitialism() {
- goName = upper(goName)
- }
- result += goName
- }
-
- if len(result) > 0 {
- // Only prefix with X when the first character isn't an ascii letter
- first := []rune(result)[0]
- if !unicode.IsLetter(first) || (first > unicode.MaxASCII && !unicode.IsUpper(first)) {
- if GoNamePrefixFunc == nil {
- return "X" + result
- }
- result = GoNamePrefixFunc(name) + result
- }
- first = []rune(result)[0]
- if unicode.IsLetter(first) && !unicode.IsUpper(first) {
- result = string(append([]rune{unicode.ToUpper(first)}, []rune(result)[1:]...))
- }
- }
-
- return result
-}
-
-// ContainsStrings searches a slice of strings for a case-sensitive match
-func ContainsStrings(coll []string, item string) bool {
- for _, a := range coll {
- if a == item {
- return true
- }
- }
- return false
-}
-
-// ContainsStringsCI searches a slice of strings for a case-insensitive match
-func ContainsStringsCI(coll []string, item string) bool {
- for _, a := range coll {
- if strings.EqualFold(a, item) {
- return true
- }
- }
- return false
-}
-
-type zeroable interface {
- IsZero() bool
-}
-
-// IsZero returns true when the value passed into the function is a zero value.
-// This allows for safer checking of interface values.
-func IsZero(data interface{}) bool {
- // check for things that have an IsZero method instead
- if vv, ok := data.(zeroable); ok {
- return vv.IsZero()
- }
- // continue with slightly more complex reflection
- v := reflect.ValueOf(data)
- switch v.Kind() {
- case reflect.String:
- return v.Len() == 0
- case reflect.Bool:
- return !v.Bool()
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return v.Int() == 0
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return v.Uint() == 0
- case reflect.Float32, reflect.Float64:
- return v.Float() == 0
- case reflect.Interface, reflect.Map, reflect.Ptr, reflect.Slice:
- return v.IsNil()
- case reflect.Struct, reflect.Array:
- return reflect.DeepEqual(data, reflect.Zero(v.Type()).Interface())
- case reflect.Invalid:
- return true
- }
- return false
-}
-
-// AddInitialisms add additional initialisms
-func AddInitialisms(words ...string) {
- for _, word := range words {
- //commonInitialisms[upper(word)] = true
- commonInitialisms.add(upper(word))
- }
- // sort again
- initialisms = commonInitialisms.sorted()
-}
-
-// CommandLineOptionsGroup represents a group of user-defined command line options
-type CommandLineOptionsGroup struct {
- ShortDescription string
- LongDescription string
- Options interface{}
-}
diff --git a/vendor/github.com/go-openapi/swag/yaml.go b/vendor/github.com/go-openapi/swag/yaml.go
deleted file mode 100644
index ec969144..00000000
--- a/vendor/github.com/go-openapi/swag/yaml.go
+++ /dev/null
@@ -1,246 +0,0 @@
-// Copyright 2015 go-swagger maintainers
-//
-// Licensed under the Apache License, Version 2.0 (the "License");
-// you may not use this file except in compliance with the License.
-// You may obtain a copy of the License at
-//
-// http://www.apache.org/licenses/LICENSE-2.0
-//
-// Unless required by applicable law or agreed to in writing, software
-// distributed under the License is distributed on an "AS IS" BASIS,
-// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-// See the License for the specific language governing permissions and
-// limitations under the License.
-
-package swag
-
-import (
- "encoding/json"
- "fmt"
- "path/filepath"
- "strconv"
-
- "github.com/mailru/easyjson/jlexer"
- "github.com/mailru/easyjson/jwriter"
- yaml "gopkg.in/yaml.v2"
-)
-
-// YAMLMatcher matches yaml
-func YAMLMatcher(path string) bool {
- ext := filepath.Ext(path)
- return ext == ".yaml" || ext == ".yml"
-}
-
-// YAMLToJSON converts YAML unmarshaled data into json compatible data
-func YAMLToJSON(data interface{}) (json.RawMessage, error) {
- jm, err := transformData(data)
- if err != nil {
- return nil, err
- }
- b, err := WriteJSON(jm)
- return json.RawMessage(b), err
-}
-
-// BytesToYAMLDoc converts a byte slice into a YAML document
-func BytesToYAMLDoc(data []byte) (interface{}, error) {
- var canary map[interface{}]interface{} // validate this is an object and not a different type
- if err := yaml.Unmarshal(data, &canary); err != nil {
- return nil, err
- }
-
- var document yaml.MapSlice // preserve order that is present in the document
- if err := yaml.Unmarshal(data, &document); err != nil {
- return nil, err
- }
- return document, nil
-}
-
-// JSONMapSlice represent a JSON object, with the order of keys maintained
-type JSONMapSlice []JSONMapItem
-
-// MarshalJSON renders a JSONMapSlice as JSON
-func (s JSONMapSlice) MarshalJSON() ([]byte, error) {
- w := &jwriter.Writer{Flags: jwriter.NilMapAsEmpty | jwriter.NilSliceAsEmpty}
- s.MarshalEasyJSON(w)
- return w.BuildBytes()
-}
-
-// MarshalEasyJSON renders a JSONMapSlice as JSON, using easyJSON
-func (s JSONMapSlice) MarshalEasyJSON(w *jwriter.Writer) {
- w.RawByte('{')
-
- ln := len(s)
- last := ln - 1
- for i := 0; i < ln; i++ {
- s[i].MarshalEasyJSON(w)
- if i != last { // last item
- w.RawByte(',')
- }
- }
-
- w.RawByte('}')
-}
-
-// UnmarshalJSON makes a JSONMapSlice from JSON
-func (s *JSONMapSlice) UnmarshalJSON(data []byte) error {
- l := jlexer.Lexer{Data: data}
- s.UnmarshalEasyJSON(&l)
- return l.Error()
-}
-
-// UnmarshalEasyJSON makes a JSONMapSlice from JSON, using easyJSON
-func (s *JSONMapSlice) UnmarshalEasyJSON(in *jlexer.Lexer) {
- if in.IsNull() {
- in.Skip()
- return
- }
-
- var result JSONMapSlice
- in.Delim('{')
- for !in.IsDelim('}') {
- var mi JSONMapItem
- mi.UnmarshalEasyJSON(in)
- result = append(result, mi)
- }
- *s = result
-}
-
-// JSONMapItem represents the value of a key in a JSON object held by JSONMapSlice
-type JSONMapItem struct {
- Key string
- Value interface{}
-}
-
-// MarshalJSON renders a JSONMapItem as JSON
-func (s JSONMapItem) MarshalJSON() ([]byte, error) {
- w := &jwriter.Writer{Flags: jwriter.NilMapAsEmpty | jwriter.NilSliceAsEmpty}
- s.MarshalEasyJSON(w)
- return w.BuildBytes()
-}
-
-// MarshalEasyJSON renders a JSONMapItem as JSON, using easyJSON
-func (s JSONMapItem) MarshalEasyJSON(w *jwriter.Writer) {
- w.String(s.Key)
- w.RawByte(':')
- w.Raw(WriteJSON(s.Value))
-}
-
-// UnmarshalJSON makes a JSONMapItem from JSON
-func (s *JSONMapItem) UnmarshalJSON(data []byte) error {
- l := jlexer.Lexer{Data: data}
- s.UnmarshalEasyJSON(&l)
- return l.Error()
-}
-
-// UnmarshalEasyJSON makes a JSONMapItem from JSON, using easyJSON
-func (s *JSONMapItem) UnmarshalEasyJSON(in *jlexer.Lexer) {
- key := in.UnsafeString()
- in.WantColon()
- value := in.Interface()
- in.WantComma()
- s.Key = key
- s.Value = value
-}
-
-func transformData(input interface{}) (out interface{}, err error) {
- format := func(t interface{}) (string, error) {
- switch k := t.(type) {
- case string:
- return k, nil
- case uint:
- return strconv.FormatUint(uint64(k), 10), nil
- case uint8:
- return strconv.FormatUint(uint64(k), 10), nil
- case uint16:
- return strconv.FormatUint(uint64(k), 10), nil
- case uint32:
- return strconv.FormatUint(uint64(k), 10), nil
- case uint64:
- return strconv.FormatUint(k, 10), nil
- case int:
- return strconv.Itoa(k), nil
- case int8:
- return strconv.FormatInt(int64(k), 10), nil
- case int16:
- return strconv.FormatInt(int64(k), 10), nil
- case int32:
- return strconv.FormatInt(int64(k), 10), nil
- case int64:
- return strconv.FormatInt(k, 10), nil
- default:
- return "", fmt.Errorf("unexpected map key type, got: %T", k)
- }
- }
-
- switch in := input.(type) {
- case yaml.MapSlice:
-
- o := make(JSONMapSlice, len(in))
- for i, mi := range in {
- var nmi JSONMapItem
- if nmi.Key, err = format(mi.Key); err != nil {
- return nil, err
- }
-
- v, ert := transformData(mi.Value)
- if ert != nil {
- return nil, ert
- }
- nmi.Value = v
- o[i] = nmi
- }
- return o, nil
- case map[interface{}]interface{}:
- o := make(JSONMapSlice, 0, len(in))
- for ke, va := range in {
- var nmi JSONMapItem
- if nmi.Key, err = format(ke); err != nil {
- return nil, err
- }
-
- v, ert := transformData(va)
- if ert != nil {
- return nil, ert
- }
- nmi.Value = v
- o = append(o, nmi)
- }
- return o, nil
- case []interface{}:
- len1 := len(in)
- o := make([]interface{}, len1)
- for i := 0; i < len1; i++ {
- o[i], err = transformData(in[i])
- if err != nil {
- return nil, err
- }
- }
- return o, nil
- }
- return input, nil
-}
-
-// YAMLDoc loads a yaml document from either http or a file and converts it to json
-func YAMLDoc(path string) (json.RawMessage, error) {
- yamlDoc, err := YAMLData(path)
- if err != nil {
- return nil, err
- }
-
- data, err := YAMLToJSON(yamlDoc)
- if err != nil {
- return nil, err
- }
-
- return data, nil
-}
-
-// YAMLData loads a yaml document from either http or a file
-func YAMLData(path string) (interface{}, error) {
- data, err := LoadFromFileOrHTTP(path)
- if err != nil {
- return nil, err
- }
-
- return BytesToYAMLDoc(data)
-}
diff --git a/vendor/github.com/go-playground/locales/.gitignore b/vendor/github.com/go-playground/locales/.gitignore
deleted file mode 100644
index daf913b1..00000000
--- a/vendor/github.com/go-playground/locales/.gitignore
+++ /dev/null
@@ -1,24 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
diff --git a/vendor/github.com/go-playground/locales/.travis.yml b/vendor/github.com/go-playground/locales/.travis.yml
deleted file mode 100644
index d50237a6..00000000
--- a/vendor/github.com/go-playground/locales/.travis.yml
+++ /dev/null
@@ -1,26 +0,0 @@
-language: go
-go:
- - 1.13.1
- - tip
-matrix:
- allow_failures:
- - go: tip
-
-notifications:
- email:
- recipients: dean.karn@gmail.com
- on_success: change
- on_failure: always
-
-before_install:
- - go install github.com/mattn/goveralls
-
-# Only clone the most recent commit.
-git:
- depth: 1
-
-script:
- - go test -v -race -covermode=atomic -coverprofile=coverage.coverprofile ./...
-
-after_success: |
- goveralls -coverprofile=coverage.coverprofile -service travis-ci -repotoken $COVERALLS_TOKEN
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/locales/LICENSE b/vendor/github.com/go-playground/locales/LICENSE
deleted file mode 100644
index 75854ac4..00000000
--- a/vendor/github.com/go-playground/locales/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2016 Go Playground
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/locales/README.md b/vendor/github.com/go-playground/locales/README.md
deleted file mode 100644
index 7b6be2c6..00000000
--- a/vendor/github.com/go-playground/locales/README.md
+++ /dev/null
@@ -1,170 +0,0 @@
-## locales
-

-[](https://travis-ci.org/go-playground/locales)
-[](https://godoc.org/github.com/go-playground/locales)
-
-
-Locales is a set of locales generated from the [Unicode CLDR Project](http://cldr.unicode.org/) which can be used independently or within
-an i18n package; these were built for use with, but not exclusive to, [Universal Translator](https://github.com/go-playground/universal-translator).
-
-Features
---------
-- [x] Rules generated from the latest [CLDR](http://cldr.unicode.org/index/downloads) data, v36.0.1
-- [x] Contains Cardinal, Ordinal and Range Plural Rules
-- [x] Contains Month, Weekday and Timezone translations built in
-- [x] Contains Date & Time formatting functions
-- [x] Contains Number, Currency, Accounting and Percent formatting functions
-- [x] Supports the "Gregorian" calendar only ( my time isn't unlimited, had to draw the line somewhere )
-
-Full Tests
---------------------
-I could sure use your help adding tests for every locale, it is a huge undertaking and I just don't have the free time to do it all at the moment;
-any help would be **greatly appreciated!!!!** please see [issue](https://github.com/go-playground/locales/issues/1) for details.
-
-Installation
------------
-
-Use go get
-
-```shell
-go get github.com/go-playground/locales
-```
-
-NOTES
---------
-You'll notice most return types are []byte, this is because most of the time the results will be concatenated with a larger body
-of text and can avoid some allocations if already appending to a byte array, otherwise just cast as string.
-
-Usage
--------
-```go
-package main
-
-import (
- "fmt"
- "time"
-
- "github.com/go-playground/locales/currency"
- "github.com/go-playground/locales/en_CA"
-)
-
-func main() {
-
- loc, _ := time.LoadLocation("America/Toronto")
- datetime := time.Date(2016, 02, 03, 9, 0, 1, 0, loc)
-
- l := en_CA.New()
-
- // Dates
- fmt.Println(l.FmtDateFull(datetime))
- fmt.Println(l.FmtDateLong(datetime))
- fmt.Println(l.FmtDateMedium(datetime))
- fmt.Println(l.FmtDateShort(datetime))
-
- // Times
- fmt.Println(l.FmtTimeFull(datetime))
- fmt.Println(l.FmtTimeLong(datetime))
- fmt.Println(l.FmtTimeMedium(datetime))
- fmt.Println(l.FmtTimeShort(datetime))
-
- // Months Wide
- fmt.Println(l.MonthWide(time.January))
- fmt.Println(l.MonthWide(time.February))
- fmt.Println(l.MonthWide(time.March))
- // ...
-
- // Months Abbreviated
- fmt.Println(l.MonthAbbreviated(time.January))
- fmt.Println(l.MonthAbbreviated(time.February))
- fmt.Println(l.MonthAbbreviated(time.March))
- // ...
-
- // Months Narrow
- fmt.Println(l.MonthNarrow(time.January))
- fmt.Println(l.MonthNarrow(time.February))
- fmt.Println(l.MonthNarrow(time.March))
- // ...
-
- // Weekdays Wide
- fmt.Println(l.WeekdayWide(time.Sunday))
- fmt.Println(l.WeekdayWide(time.Monday))
- fmt.Println(l.WeekdayWide(time.Tuesday))
- // ...
-
- // Weekdays Abbreviated
- fmt.Println(l.WeekdayAbbreviated(time.Sunday))
- fmt.Println(l.WeekdayAbbreviated(time.Monday))
- fmt.Println(l.WeekdayAbbreviated(time.Tuesday))
- // ...
-
- // Weekdays Short
- fmt.Println(l.WeekdayShort(time.Sunday))
- fmt.Println(l.WeekdayShort(time.Monday))
- fmt.Println(l.WeekdayShort(time.Tuesday))
- // ...
-
- // Weekdays Narrow
- fmt.Println(l.WeekdayNarrow(time.Sunday))
- fmt.Println(l.WeekdayNarrow(time.Monday))
- fmt.Println(l.WeekdayNarrow(time.Tuesday))
- // ...
-
- var f64 float64
-
- f64 = -10356.4523
-
- // Number
- fmt.Println(l.FmtNumber(f64, 2))
-
- // Currency
- fmt.Println(l.FmtCurrency(f64, 2, currency.CAD))
- fmt.Println(l.FmtCurrency(f64, 2, currency.USD))
-
- // Accounting
- fmt.Println(l.FmtAccounting(f64, 2, currency.CAD))
- fmt.Println(l.FmtAccounting(f64, 2, currency.USD))
-
- f64 = 78.12
-
- // Percent
- fmt.Println(l.FmtPercent(f64, 0))
-
- // Plural Rules for locale, so you know what rules you must cover
- fmt.Println(l.PluralsCardinal())
- fmt.Println(l.PluralsOrdinal())
-
- // Cardinal Plural Rules
- fmt.Println(l.CardinalPluralRule(1, 0))
- fmt.Println(l.CardinalPluralRule(1.0, 0))
- fmt.Println(l.CardinalPluralRule(1.0, 1))
- fmt.Println(l.CardinalPluralRule(3, 0))
-
- // Ordinal Plural Rules
- fmt.Println(l.OrdinalPluralRule(21, 0)) // 21st
- fmt.Println(l.OrdinalPluralRule(22, 0)) // 22nd
- fmt.Println(l.OrdinalPluralRule(33, 0)) // 33rd
- fmt.Println(l.OrdinalPluralRule(34, 0)) // 34th
-
- // Range Plural Rules
- fmt.Println(l.RangePluralRule(1, 0, 1, 0)) // 1-1
- fmt.Println(l.RangePluralRule(1, 0, 2, 0)) // 1-2
- fmt.Println(l.RangePluralRule(5, 0, 8, 0)) // 5-8
-}
-```
-
-NOTES:
--------
-These rules were generated from the [Unicode CLDR Project](http://cldr.unicode.org/), if you encounter any issues
-I strongly encourage contributing to the CLDR project to get the locale information corrected and the next time
-these locales are regenerated the fix will come with.
-
-I do however realize that time constraints are often important and so there are two options:
-
-1. Create your own locale, copy, paste and modify, and ensure it complies with the `Translator` interface.
-2. Add an exception in the locale generation code directly and once regenerated, fix will be in place.
-
-Please to not make fixes inside the locale files, they WILL get overwritten when the locales are regenerated.
-
-License
-------
-Distributed under MIT License, please see license file in code for more details.
diff --git a/vendor/github.com/go-playground/locales/currency/currency.go b/vendor/github.com/go-playground/locales/currency/currency.go
deleted file mode 100644
index b5a95fb0..00000000
--- a/vendor/github.com/go-playground/locales/currency/currency.go
+++ /dev/null
@@ -1,311 +0,0 @@
-package currency
-
-// Type is the currency type associated with the locales currency enum
-type Type int
-
-// locale currencies
-const (
- ADP Type = iota
- AED
- AFA
- AFN
- ALK
- ALL
- AMD
- ANG
- AOA
- AOK
- AON
- AOR
- ARA
- ARL
- ARM
- ARP
- ARS
- ATS
- AUD
- AWG
- AZM
- AZN
- BAD
- BAM
- BAN
- BBD
- BDT
- BEC
- BEF
- BEL
- BGL
- BGM
- BGN
- BGO
- BHD
- BIF
- BMD
- BND
- BOB
- BOL
- BOP
- BOV
- BRB
- BRC
- BRE
- BRL
- BRN
- BRR
- BRZ
- BSD
- BTN
- BUK
- BWP
- BYB
- BYN
- BYR
- BZD
- CAD
- CDF
- CHE
- CHF
- CHW
- CLE
- CLF
- CLP
- CNH
- CNX
- CNY
- COP
- COU
- CRC
- CSD
- CSK
- CUC
- CUP
- CVE
- CYP
- CZK
- DDM
- DEM
- DJF
- DKK
- DOP
- DZD
- ECS
- ECV
- EEK
- EGP
- ERN
- ESA
- ESB
- ESP
- ETB
- EUR
- FIM
- FJD
- FKP
- FRF
- GBP
- GEK
- GEL
- GHC
- GHS
- GIP
- GMD
- GNF
- GNS
- GQE
- GRD
- GTQ
- GWE
- GWP
- GYD
- HKD
- HNL
- HRD
- HRK
- HTG
- HUF
- IDR
- IEP
- ILP
- ILR
- ILS
- INR
- IQD
- IRR
- ISJ
- ISK
- ITL
- JMD
- JOD
- JPY
- KES
- KGS
- KHR
- KMF
- KPW
- KRH
- KRO
- KRW
- KWD
- KYD
- KZT
- LAK
- LBP
- LKR
- LRD
- LSL
- LTL
- LTT
- LUC
- LUF
- LUL
- LVL
- LVR
- LYD
- MAD
- MAF
- MCF
- MDC
- MDL
- MGA
- MGF
- MKD
- MKN
- MLF
- MMK
- MNT
- MOP
- MRO
- MRU
- MTL
- MTP
- MUR
- MVP
- MVR
- MWK
- MXN
- MXP
- MXV
- MYR
- MZE
- MZM
- MZN
- NAD
- NGN
- NIC
- NIO
- NLG
- NOK
- NPR
- NZD
- OMR
- PAB
- PEI
- PEN
- PES
- PGK
- PHP
- PKR
- PLN
- PLZ
- PTE
- PYG
- QAR
- RHD
- ROL
- RON
- RSD
- RUB
- RUR
- RWF
- SAR
- SBD
- SCR
- SDD
- SDG
- SDP
- SEK
- SGD
- SHP
- SIT
- SKK
- SLL
- SOS
- SRD
- SRG
- SSP
- STD
- STN
- SUR
- SVC
- SYP
- SZL
- THB
- TJR
- TJS
- TMM
- TMT
- TND
- TOP
- TPE
- TRL
- TRY
- TTD
- TWD
- TZS
- UAH
- UAK
- UGS
- UGX
- USD
- USN
- USS
- UYI
- UYP
- UYU
- UYW
- UZS
- VEB
- VEF
- VES
- VND
- VNN
- VUV
- WST
- XAF
- XAG
- XAU
- XBA
- XBB
- XBC
- XBD
- XCD
- XDR
- XEU
- XFO
- XFU
- XOF
- XPD
- XPF
- XPT
- XRE
- XSU
- XTS
- XUA
- XXX
- YDD
- YER
- YUD
- YUM
- YUN
- YUR
- ZAL
- ZAR
- ZMK
- ZMW
- ZRN
- ZRZ
- ZWD
- ZWL
- ZWR
-)
diff --git a/vendor/github.com/go-playground/locales/logo.png b/vendor/github.com/go-playground/locales/logo.png
deleted file mode 100644
index 3038276e..00000000
Binary files a/vendor/github.com/go-playground/locales/logo.png and /dev/null differ
diff --git a/vendor/github.com/go-playground/locales/rules.go b/vendor/github.com/go-playground/locales/rules.go
deleted file mode 100644
index 92029001..00000000
--- a/vendor/github.com/go-playground/locales/rules.go
+++ /dev/null
@@ -1,293 +0,0 @@
-package locales
-
-import (
- "strconv"
- "time"
-
- "github.com/go-playground/locales/currency"
-)
-
-// // ErrBadNumberValue is returned when the number passed for
-// // plural rule determination cannot be parsed
-// type ErrBadNumberValue struct {
-// NumberValue string
-// InnerError error
-// }
-
-// // Error returns ErrBadNumberValue error string
-// func (e *ErrBadNumberValue) Error() string {
-// return fmt.Sprintf("Invalid Number Value '%s' %s", e.NumberValue, e.InnerError)
-// }
-
-// var _ error = new(ErrBadNumberValue)
-
-// PluralRule denotes the type of plural rules
-type PluralRule int
-
-// PluralRule's
-const (
- PluralRuleUnknown PluralRule = iota
- PluralRuleZero // zero
- PluralRuleOne // one - singular
- PluralRuleTwo // two - dual
- PluralRuleFew // few - paucal
- PluralRuleMany // many - also used for fractions if they have a separate class
- PluralRuleOther // other - required—general plural form—also used if the language only has a single form
-)
-
-const (
- pluralsString = "UnknownZeroOneTwoFewManyOther"
-)
-
-// Translator encapsulates an instance of a locale
-// NOTE: some values are returned as a []byte just in case the caller
-// wishes to add more and can help avoid allocations; otherwise just cast as string
-type Translator interface {
-
- // The following Functions are for overriding, debugging or developing
- // with a Translator Locale
-
- // Locale returns the string value of the translator
- Locale() string
-
- // returns an array of cardinal plural rules associated
- // with this translator
- PluralsCardinal() []PluralRule
-
- // returns an array of ordinal plural rules associated
- // with this translator
- PluralsOrdinal() []PluralRule
-
- // returns an array of range plural rules associated
- // with this translator
- PluralsRange() []PluralRule
-
- // returns the cardinal PluralRule given 'num' and digits/precision of 'v' for locale
- CardinalPluralRule(num float64, v uint64) PluralRule
-
- // returns the ordinal PluralRule given 'num' and digits/precision of 'v' for locale
- OrdinalPluralRule(num float64, v uint64) PluralRule
-
- // returns the ordinal PluralRule given 'num1', 'num2' and digits/precision of 'v1' and 'v2' for locale
- RangePluralRule(num1 float64, v1 uint64, num2 float64, v2 uint64) PluralRule
-
- // returns the locales abbreviated month given the 'month' provided
- MonthAbbreviated(month time.Month) string
-
- // returns the locales abbreviated months
- MonthsAbbreviated() []string
-
- // returns the locales narrow month given the 'month' provided
- MonthNarrow(month time.Month) string
-
- // returns the locales narrow months
- MonthsNarrow() []string
-
- // returns the locales wide month given the 'month' provided
- MonthWide(month time.Month) string
-
- // returns the locales wide months
- MonthsWide() []string
-
- // returns the locales abbreviated weekday given the 'weekday' provided
- WeekdayAbbreviated(weekday time.Weekday) string
-
- // returns the locales abbreviated weekdays
- WeekdaysAbbreviated() []string
-
- // returns the locales narrow weekday given the 'weekday' provided
- WeekdayNarrow(weekday time.Weekday) string
-
- // WeekdaysNarrowreturns the locales narrow weekdays
- WeekdaysNarrow() []string
-
- // returns the locales short weekday given the 'weekday' provided
- WeekdayShort(weekday time.Weekday) string
-
- // returns the locales short weekdays
- WeekdaysShort() []string
-
- // returns the locales wide weekday given the 'weekday' provided
- WeekdayWide(weekday time.Weekday) string
-
- // returns the locales wide weekdays
- WeekdaysWide() []string
-
- // The following Functions are common Formatting functionsfor the Translator's Locale
-
- // returns 'num' with digits/precision of 'v' for locale and handles both Whole and Real numbers based on 'v'
- FmtNumber(num float64, v uint64) string
-
- // returns 'num' with digits/precision of 'v' for locale and handles both Whole and Real numbers based on 'v'
- // NOTE: 'num' passed into FmtPercent is assumed to be in percent already
- FmtPercent(num float64, v uint64) string
-
- // returns the currency representation of 'num' with digits/precision of 'v' for locale
- FmtCurrency(num float64, v uint64, currency currency.Type) string
-
- // returns the currency representation of 'num' with digits/precision of 'v' for locale
- // in accounting notation.
- FmtAccounting(num float64, v uint64, currency currency.Type) string
-
- // returns the short date representation of 't' for locale
- FmtDateShort(t time.Time) string
-
- // returns the medium date representation of 't' for locale
- FmtDateMedium(t time.Time) string
-
- // returns the long date representation of 't' for locale
- FmtDateLong(t time.Time) string
-
- // returns the full date representation of 't' for locale
- FmtDateFull(t time.Time) string
-
- // returns the short time representation of 't' for locale
- FmtTimeShort(t time.Time) string
-
- // returns the medium time representation of 't' for locale
- FmtTimeMedium(t time.Time) string
-
- // returns the long time representation of 't' for locale
- FmtTimeLong(t time.Time) string
-
- // returns the full time representation of 't' for locale
- FmtTimeFull(t time.Time) string
-}
-
-// String returns the string value of PluralRule
-func (p PluralRule) String() string {
-
- switch p {
- case PluralRuleZero:
- return pluralsString[7:11]
- case PluralRuleOne:
- return pluralsString[11:14]
- case PluralRuleTwo:
- return pluralsString[14:17]
- case PluralRuleFew:
- return pluralsString[17:20]
- case PluralRuleMany:
- return pluralsString[20:24]
- case PluralRuleOther:
- return pluralsString[24:]
- default:
- return pluralsString[:7]
- }
-}
-
-//
-// Precision Notes:
-//
-// must specify a precision >= 0, and here is why https://play.golang.org/p/LyL90U0Vyh
-//
-// v := float64(3.141)
-// i := float64(int64(v))
-//
-// fmt.Println(v - i)
-//
-// or
-//
-// s := strconv.FormatFloat(v-i, 'f', -1, 64)
-// fmt.Println(s)
-//
-// these will not print what you'd expect: 0.14100000000000001
-// and so this library requires a precision to be specified, or
-// inaccurate plural rules could be applied.
-//
-//
-//
-// n - absolute value of the source number (integer and decimals).
-// i - integer digits of n.
-// v - number of visible fraction digits in n, with trailing zeros.
-// w - number of visible fraction digits in n, without trailing zeros.
-// f - visible fractional digits in n, with trailing zeros.
-// t - visible fractional digits in n, without trailing zeros.
-//
-//
-// Func(num float64, v uint64) // v = digits/precision and prevents -1 as a special case as this can lead to very unexpected behaviour, see precision note's above.
-//
-// n := math.Abs(num)
-// i := int64(n)
-// v := v
-//
-//
-// w := strconv.FormatFloat(num-float64(i), 'f', int(v), 64) // then parse backwards on string until no more zero's....
-// f := strconv.FormatFloat(n, 'f', int(v), 64) // then turn everything after decimal into an int64
-// t := strconv.FormatFloat(n, 'f', int(v), 64) // then parse backwards on string until no more zero's....
-//
-//
-//
-// General Inclusion Rules
-// - v will always be available inherently
-// - all require n
-// - w requires i
-//
-
-// W returns the number of visible fraction digits in N, without trailing zeros.
-func W(n float64, v uint64) (w int64) {
-
- s := strconv.FormatFloat(n-float64(int64(n)), 'f', int(v), 64)
-
- // with either be '0' or '0.xxxx', so if 1 then w will be zero
- // otherwise need to parse
- if len(s) != 1 {
-
- s = s[2:]
- end := len(s) + 1
-
- for i := end; i >= 0; i-- {
- if s[i] != '0' {
- end = i + 1
- break
- }
- }
-
- w = int64(len(s[:end]))
- }
-
- return
-}
-
-// F returns the visible fractional digits in N, with trailing zeros.
-func F(n float64, v uint64) (f int64) {
-
- s := strconv.FormatFloat(n-float64(int64(n)), 'f', int(v), 64)
-
- // with either be '0' or '0.xxxx', so if 1 then f will be zero
- // otherwise need to parse
- if len(s) != 1 {
-
- // ignoring error, because it can't fail as we generated
- // the string internally from a real number
- f, _ = strconv.ParseInt(s[2:], 10, 64)
- }
-
- return
-}
-
-// T returns the visible fractional digits in N, without trailing zeros.
-func T(n float64, v uint64) (t int64) {
-
- s := strconv.FormatFloat(n-float64(int64(n)), 'f', int(v), 64)
-
- // with either be '0' or '0.xxxx', so if 1 then t will be zero
- // otherwise need to parse
- if len(s) != 1 {
-
- s = s[2:]
- end := len(s) + 1
-
- for i := end; i >= 0; i-- {
- if s[i] != '0' {
- end = i + 1
- break
- }
- }
-
- // ignoring error, because it can't fail as we generated
- // the string internally from a real number
- t, _ = strconv.ParseInt(s[:end], 10, 64)
- }
-
- return
-}
diff --git a/vendor/github.com/go-playground/universal-translator/.gitignore b/vendor/github.com/go-playground/universal-translator/.gitignore
deleted file mode 100644
index bc4e07f3..00000000
--- a/vendor/github.com/go-playground/universal-translator/.gitignore
+++ /dev/null
@@ -1,25 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
-*.coverprofile
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/universal-translator/.travis.yml b/vendor/github.com/go-playground/universal-translator/.travis.yml
deleted file mode 100644
index 39b8b923..00000000
--- a/vendor/github.com/go-playground/universal-translator/.travis.yml
+++ /dev/null
@@ -1,27 +0,0 @@
-language: go
-go:
- - 1.13.4
- - tip
-matrix:
- allow_failures:
- - go: tip
-
-notifications:
- email:
- recipients: dean.karn@gmail.com
- on_success: change
- on_failure: always
-
-before_install:
- - go install github.com/mattn/goveralls
-
-# Only clone the most recent commit.
-git:
- depth: 1
-
-script:
- - go test -v -race -covermode=atomic -coverprofile=coverage.coverprofile ./...
-
-after_success: |
- [ $TRAVIS_GO_VERSION = 1.13.4 ] &&
- goveralls -coverprofile=coverage.coverprofile -service travis-ci -repotoken $COVERALLS_TOKEN
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/universal-translator/LICENSE b/vendor/github.com/go-playground/universal-translator/LICENSE
deleted file mode 100644
index 8d8aba15..00000000
--- a/vendor/github.com/go-playground/universal-translator/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2016 Go Playground
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/go-playground/universal-translator/Makefile b/vendor/github.com/go-playground/universal-translator/Makefile
deleted file mode 100644
index ec3455bd..00000000
--- a/vendor/github.com/go-playground/universal-translator/Makefile
+++ /dev/null
@@ -1,18 +0,0 @@
-GOCMD=GO111MODULE=on go
-
-linters-install:
- @golangci-lint --version >/dev/null 2>&1 || { \
- echo "installing linting tools..."; \
- curl -sfL https://raw.githubusercontent.com/golangci/golangci-lint/master/install.sh| sh -s v1.41.1; \
- }
-
-lint: linters-install
- golangci-lint run
-
-test:
- $(GOCMD) test -cover -race ./...
-
-bench:
- $(GOCMD) test -bench=. -benchmem ./...
-
-.PHONY: test lint linters-install
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/universal-translator/README.md b/vendor/github.com/go-playground/universal-translator/README.md
deleted file mode 100644
index d9b66547..00000000
--- a/vendor/github.com/go-playground/universal-translator/README.md
+++ /dev/null
@@ -1,87 +0,0 @@
-## universal-translator
-

-[](https://coveralls.io/github/go-playground/universal-translator)
-[](https://goreportcard.com/report/github.com/go-playground/universal-translator)
-[](https://godoc.org/github.com/go-playground/universal-translator)
-
-
-Universal Translator is an i18n Translator for Go/Golang using CLDR data + pluralization rules
-
-Why another i18n library?
---------------------------
-Because none of the plural rules seem to be correct out there, including the previous implementation of this package,
-so I took it upon myself to create [locales](https://github.com/go-playground/locales) for everyone to use; this package
-is a thin wrapper around [locales](https://github.com/go-playground/locales) in order to store and translate text for
-use in your applications.
-
-Features
---------
-- [x] Rules generated from the [CLDR](http://cldr.unicode.org/index/downloads) data, v36.0.1
-- [x] Contains Cardinal, Ordinal and Range Plural Rules
-- [x] Contains Month, Weekday and Timezone translations built in
-- [x] Contains Date & Time formatting functions
-- [x] Contains Number, Currency, Accounting and Percent formatting functions
-- [x] Supports the "Gregorian" calendar only ( my time isn't unlimited, had to draw the line somewhere )
-- [x] Support loading translations from files
-- [x] Exporting translations to file(s), mainly for getting them professionally translated
-- [ ] Code Generation for translation files -> Go code.. i.e. after it has been professionally translated
-- [ ] Tests for all languages, I need help with this, please see [here](https://github.com/go-playground/locales/issues/1)
-
-Installation
------------
-
-Use go get
-
-```shell
-go get github.com/go-playground/universal-translator
-```
-
-Usage & Documentation
--------
-
-Please see https://godoc.org/github.com/go-playground/universal-translator for usage docs
-
-##### Examples:
-
-- [Basic](https://github.com/go-playground/universal-translator/tree/master/_examples/basic)
-- [Full - no files](https://github.com/go-playground/universal-translator/tree/master/_examples/full-no-files)
-- [Full - with files](https://github.com/go-playground/universal-translator/tree/master/_examples/full-with-files)
-
-File formatting
---------------
-All types, Plain substitution, Cardinal, Ordinal and Range translations can all be contained within the same file(s);
-they are only separated for easy viewing.
-
-##### Examples:
-
-- [Formats](https://github.com/go-playground/universal-translator/tree/master/_examples/file-formats)
-
-##### Basic Makeup
-NOTE: not all fields are needed for all translation types, see [examples](https://github.com/go-playground/universal-translator/tree/master/_examples/file-formats)
-```json
-{
- "locale": "en",
- "key": "days-left",
- "trans": "You have {0} day left.",
- "type": "Cardinal",
- "rule": "One",
- "override": false
-}
-```
-|Field|Description|
-|---|---|
-|locale|The locale for which the translation is for.|
-|key|The translation key that will be used to store and lookup each translation; normally it is a string or integer.|
-|trans|The actual translation text.|
-|type|The type of translation Cardinal, Ordinal, Range or "" for a plain substitution(not required to be defined if plain used)|
-|rule|The plural rule for which the translation is for eg. One, Two, Few, Many or Other.(not required to be defined if plain used)|
-|override|If you wish to override an existing translation that has already been registered, set this to 'true'. 99% of the time there is no need to define it.|
-
-Help With Tests
----------------
-To anyone interesting in helping or contributing, I sure could use some help creating tests for each language.
-Please see issue [here](https://github.com/go-playground/locales/issues/1) for details.
-
-License
-------
-Distributed under MIT License, please see license file in code for more details.
diff --git a/vendor/github.com/go-playground/universal-translator/errors.go b/vendor/github.com/go-playground/universal-translator/errors.go
deleted file mode 100644
index 38b163b6..00000000
--- a/vendor/github.com/go-playground/universal-translator/errors.go
+++ /dev/null
@@ -1,148 +0,0 @@
-package ut
-
-import (
- "errors"
- "fmt"
-
- "github.com/go-playground/locales"
-)
-
-var (
- // ErrUnknowTranslation indicates the translation could not be found
- ErrUnknowTranslation = errors.New("Unknown Translation")
-)
-
-var _ error = new(ErrConflictingTranslation)
-var _ error = new(ErrRangeTranslation)
-var _ error = new(ErrOrdinalTranslation)
-var _ error = new(ErrCardinalTranslation)
-var _ error = new(ErrMissingPluralTranslation)
-var _ error = new(ErrExistingTranslator)
-
-// ErrExistingTranslator is the error representing a conflicting translator
-type ErrExistingTranslator struct {
- locale string
-}
-
-// Error returns ErrExistingTranslator's internal error text
-func (e *ErrExistingTranslator) Error() string {
- return fmt.Sprintf("error: conflicting translator for locale '%s'", e.locale)
-}
-
-// ErrConflictingTranslation is the error representing a conflicting translation
-type ErrConflictingTranslation struct {
- locale string
- key interface{}
- rule locales.PluralRule
- text string
-}
-
-// Error returns ErrConflictingTranslation's internal error text
-func (e *ErrConflictingTranslation) Error() string {
-
- if _, ok := e.key.(string); !ok {
- return fmt.Sprintf("error: conflicting key '%#v' rule '%s' with text '%s' for locale '%s', value being ignored", e.key, e.rule, e.text, e.locale)
- }
-
- return fmt.Sprintf("error: conflicting key '%s' rule '%s' with text '%s' for locale '%s', value being ignored", e.key, e.rule, e.text, e.locale)
-}
-
-// ErrRangeTranslation is the error representing a range translation error
-type ErrRangeTranslation struct {
- text string
-}
-
-// Error returns ErrRangeTranslation's internal error text
-func (e *ErrRangeTranslation) Error() string {
- return e.text
-}
-
-// ErrOrdinalTranslation is the error representing an ordinal translation error
-type ErrOrdinalTranslation struct {
- text string
-}
-
-// Error returns ErrOrdinalTranslation's internal error text
-func (e *ErrOrdinalTranslation) Error() string {
- return e.text
-}
-
-// ErrCardinalTranslation is the error representing a cardinal translation error
-type ErrCardinalTranslation struct {
- text string
-}
-
-// Error returns ErrCardinalTranslation's internal error text
-func (e *ErrCardinalTranslation) Error() string {
- return e.text
-}
-
-// ErrMissingPluralTranslation is the error signifying a missing translation given
-// the locales plural rules.
-type ErrMissingPluralTranslation struct {
- locale string
- key interface{}
- rule locales.PluralRule
- translationType string
-}
-
-// Error returns ErrMissingPluralTranslation's internal error text
-func (e *ErrMissingPluralTranslation) Error() string {
-
- if _, ok := e.key.(string); !ok {
- return fmt.Sprintf("error: missing '%s' plural rule '%s' for translation with key '%#v' and locale '%s'", e.translationType, e.rule, e.key, e.locale)
- }
-
- return fmt.Sprintf("error: missing '%s' plural rule '%s' for translation with key '%s' and locale '%s'", e.translationType, e.rule, e.key, e.locale)
-}
-
-// ErrMissingBracket is the error representing a missing bracket in a translation
-// eg. This is a {0 <-- missing ending '}'
-type ErrMissingBracket struct {
- locale string
- key interface{}
- text string
-}
-
-// Error returns ErrMissingBracket error message
-func (e *ErrMissingBracket) Error() string {
- return fmt.Sprintf("error: missing bracket '{}', in translation. locale: '%s' key: '%v' text: '%s'", e.locale, e.key, e.text)
-}
-
-// ErrBadParamSyntax is the error representing a bad parameter definition in a translation
-// eg. This is a {must-be-int}
-type ErrBadParamSyntax struct {
- locale string
- param string
- key interface{}
- text string
-}
-
-// Error returns ErrBadParamSyntax error message
-func (e *ErrBadParamSyntax) Error() string {
- return fmt.Sprintf("error: bad parameter syntax, missing parameter '%s' in translation. locale: '%s' key: '%v' text: '%s'", e.param, e.locale, e.key, e.text)
-}
-
-// import/export errors
-
-// ErrMissingLocale is the error representing an expected locale that could
-// not be found aka locale not registered with the UniversalTranslator Instance
-type ErrMissingLocale struct {
- locale string
-}
-
-// Error returns ErrMissingLocale's internal error text
-func (e *ErrMissingLocale) Error() string {
- return fmt.Sprintf("error: locale '%s' not registered.", e.locale)
-}
-
-// ErrBadPluralDefinition is the error representing an incorrect plural definition
-// usually found within translations defined within files during the import process.
-type ErrBadPluralDefinition struct {
- tl translation
-}
-
-// Error returns ErrBadPluralDefinition's internal error text
-func (e *ErrBadPluralDefinition) Error() string {
- return fmt.Sprintf("error: bad plural definition '%#v'", e.tl)
-}
diff --git a/vendor/github.com/go-playground/universal-translator/import_export.go b/vendor/github.com/go-playground/universal-translator/import_export.go
deleted file mode 100644
index 87a1b465..00000000
--- a/vendor/github.com/go-playground/universal-translator/import_export.go
+++ /dev/null
@@ -1,274 +0,0 @@
-package ut
-
-import (
- "encoding/json"
- "fmt"
- "os"
- "path/filepath"
-
- "io"
-
- "github.com/go-playground/locales"
-)
-
-type translation struct {
- Locale string `json:"locale"`
- Key interface{} `json:"key"` // either string or integer
- Translation string `json:"trans"`
- PluralType string `json:"type,omitempty"`
- PluralRule string `json:"rule,omitempty"`
- OverrideExisting bool `json:"override,omitempty"`
-}
-
-const (
- cardinalType = "Cardinal"
- ordinalType = "Ordinal"
- rangeType = "Range"
-)
-
-// ImportExportFormat is the format of the file import or export
-type ImportExportFormat uint8
-
-// supported Export Formats
-const (
- FormatJSON ImportExportFormat = iota
-)
-
-// Export writes the translations out to a file on disk.
-//
-// NOTE: this currently only works with string or int translations keys.
-func (t *UniversalTranslator) Export(format ImportExportFormat, dirname string) error {
-
- _, err := os.Stat(dirname)
- if err != nil {
-
- if !os.IsNotExist(err) {
- return err
- }
-
- if err = os.MkdirAll(dirname, 0744); err != nil {
- return err
- }
- }
-
- // build up translations
- var trans []translation
- var b []byte
- var ext string
-
- for _, locale := range t.translators {
-
- for k, v := range locale.(*translator).translations {
- trans = append(trans, translation{
- Locale: locale.Locale(),
- Key: k,
- Translation: v.text,
- })
- }
-
- for k, pluralTrans := range locale.(*translator).cardinalTanslations {
-
- for i, plural := range pluralTrans {
-
- // leave enough for all plural rules
- // but not all are set for all languages.
- if plural == nil {
- continue
- }
-
- trans = append(trans, translation{
- Locale: locale.Locale(),
- Key: k.(string),
- Translation: plural.text,
- PluralType: cardinalType,
- PluralRule: locales.PluralRule(i).String(),
- })
- }
- }
-
- for k, pluralTrans := range locale.(*translator).ordinalTanslations {
-
- for i, plural := range pluralTrans {
-
- // leave enough for all plural rules
- // but not all are set for all languages.
- if plural == nil {
- continue
- }
-
- trans = append(trans, translation{
- Locale: locale.Locale(),
- Key: k.(string),
- Translation: plural.text,
- PluralType: ordinalType,
- PluralRule: locales.PluralRule(i).String(),
- })
- }
- }
-
- for k, pluralTrans := range locale.(*translator).rangeTanslations {
-
- for i, plural := range pluralTrans {
-
- // leave enough for all plural rules
- // but not all are set for all languages.
- if plural == nil {
- continue
- }
-
- trans = append(trans, translation{
- Locale: locale.Locale(),
- Key: k.(string),
- Translation: plural.text,
- PluralType: rangeType,
- PluralRule: locales.PluralRule(i).String(),
- })
- }
- }
-
- switch format {
- case FormatJSON:
- b, err = json.MarshalIndent(trans, "", " ")
- ext = ".json"
- }
-
- if err != nil {
- return err
- }
-
- err = os.WriteFile(filepath.Join(dirname, fmt.Sprintf("%s%s", locale.Locale(), ext)), b, 0644)
- if err != nil {
- return err
- }
-
- trans = trans[0:0]
- }
-
- return nil
-}
-
-// Import reads the translations out of a file or directory on disk.
-//
-// NOTE: this currently only works with string or int translations keys.
-func (t *UniversalTranslator) Import(format ImportExportFormat, dirnameOrFilename string) error {
-
- fi, err := os.Stat(dirnameOrFilename)
- if err != nil {
- return err
- }
-
- processFn := func(filename string) error {
-
- f, err := os.Open(filename)
- if err != nil {
- return err
- }
- defer f.Close()
-
- return t.ImportByReader(format, f)
- }
-
- if !fi.IsDir() {
- return processFn(dirnameOrFilename)
- }
-
- // recursively go through directory
- walker := func(path string, info os.FileInfo, err error) error {
-
- if info.IsDir() {
- return nil
- }
-
- switch format {
- case FormatJSON:
- // skip non JSON files
- if filepath.Ext(info.Name()) != ".json" {
- return nil
- }
- }
-
- return processFn(path)
- }
-
- return filepath.Walk(dirnameOrFilename, walker)
-}
-
-// ImportByReader imports the the translations found within the contents read from the supplied reader.
-//
-// NOTE: generally used when assets have been embedded into the binary and are already in memory.
-func (t *UniversalTranslator) ImportByReader(format ImportExportFormat, reader io.Reader) error {
-
- b, err := io.ReadAll(reader)
- if err != nil {
- return err
- }
-
- var trans []translation
-
- switch format {
- case FormatJSON:
- err = json.Unmarshal(b, &trans)
- }
-
- if err != nil {
- return err
- }
-
- for _, tl := range trans {
-
- locale, found := t.FindTranslator(tl.Locale)
- if !found {
- return &ErrMissingLocale{locale: tl.Locale}
- }
-
- pr := stringToPR(tl.PluralRule)
-
- if pr == locales.PluralRuleUnknown {
-
- err = locale.Add(tl.Key, tl.Translation, tl.OverrideExisting)
- if err != nil {
- return err
- }
-
- continue
- }
-
- switch tl.PluralType {
- case cardinalType:
- err = locale.AddCardinal(tl.Key, tl.Translation, pr, tl.OverrideExisting)
- case ordinalType:
- err = locale.AddOrdinal(tl.Key, tl.Translation, pr, tl.OverrideExisting)
- case rangeType:
- err = locale.AddRange(tl.Key, tl.Translation, pr, tl.OverrideExisting)
- default:
- return &ErrBadPluralDefinition{tl: tl}
- }
-
- if err != nil {
- return err
- }
- }
-
- return nil
-}
-
-func stringToPR(s string) locales.PluralRule {
-
- switch s {
- case "Zero":
- return locales.PluralRuleZero
- case "One":
- return locales.PluralRuleOne
- case "Two":
- return locales.PluralRuleTwo
- case "Few":
- return locales.PluralRuleFew
- case "Many":
- return locales.PluralRuleMany
- case "Other":
- return locales.PluralRuleOther
- default:
- return locales.PluralRuleUnknown
- }
-
-}
diff --git a/vendor/github.com/go-playground/universal-translator/logo.png b/vendor/github.com/go-playground/universal-translator/logo.png
deleted file mode 100644
index a37aa8c0..00000000
Binary files a/vendor/github.com/go-playground/universal-translator/logo.png and /dev/null differ
diff --git a/vendor/github.com/go-playground/universal-translator/translator.go b/vendor/github.com/go-playground/universal-translator/translator.go
deleted file mode 100644
index 24b18db9..00000000
--- a/vendor/github.com/go-playground/universal-translator/translator.go
+++ /dev/null
@@ -1,420 +0,0 @@
-package ut
-
-import (
- "fmt"
- "strconv"
- "strings"
-
- "github.com/go-playground/locales"
-)
-
-const (
- paramZero = "{0}"
- paramOne = "{1}"
- unknownTranslation = ""
-)
-
-// Translator is universal translators
-// translator instance which is a thin wrapper
-// around locales.Translator instance providing
-// some extra functionality
-type Translator interface {
- locales.Translator
-
- // adds a normal translation for a particular language/locale
- // {#} is the only replacement type accepted and are ad infinitum
- // eg. one: '{0} day left' other: '{0} days left'
- Add(key interface{}, text string, override bool) error
-
- // adds a cardinal plural translation for a particular language/locale
- // {0} is the only replacement type accepted and only one variable is accepted as
- // multiple cannot be used for a plural rule determination, unless it is a range;
- // see AddRange below.
- // eg. in locale 'en' one: '{0} day left' other: '{0} days left'
- AddCardinal(key interface{}, text string, rule locales.PluralRule, override bool) error
-
- // adds an ordinal plural translation for a particular language/locale
- // {0} is the only replacement type accepted and only one variable is accepted as
- // multiple cannot be used for a plural rule determination, unless it is a range;
- // see AddRange below.
- // eg. in locale 'en' one: '{0}st day of spring' other: '{0}nd day of spring'
- // - 1st, 2nd, 3rd...
- AddOrdinal(key interface{}, text string, rule locales.PluralRule, override bool) error
-
- // adds a range plural translation for a particular language/locale
- // {0} and {1} are the only replacement types accepted and only these are accepted.
- // eg. in locale 'nl' one: '{0}-{1} day left' other: '{0}-{1} days left'
- AddRange(key interface{}, text string, rule locales.PluralRule, override bool) error
-
- // creates the translation for the locale given the 'key' and params passed in
- T(key interface{}, params ...string) (string, error)
-
- // creates the cardinal translation for the locale given the 'key', 'num' and 'digit' arguments
- // and param passed in
- C(key interface{}, num float64, digits uint64, param string) (string, error)
-
- // creates the ordinal translation for the locale given the 'key', 'num' and 'digit' arguments
- // and param passed in
- O(key interface{}, num float64, digits uint64, param string) (string, error)
-
- // creates the range translation for the locale given the 'key', 'num1', 'digit1', 'num2' and
- // 'digit2' arguments and 'param1' and 'param2' passed in
- R(key interface{}, num1 float64, digits1 uint64, num2 float64, digits2 uint64, param1, param2 string) (string, error)
-
- // VerifyTranslations checks to ensures that no plural rules have been
- // missed within the translations.
- VerifyTranslations() error
-}
-
-var _ Translator = new(translator)
-var _ locales.Translator = new(translator)
-
-type translator struct {
- locales.Translator
- translations map[interface{}]*transText
- cardinalTanslations map[interface{}][]*transText // array index is mapped to locales.PluralRule index + the locales.PluralRuleUnknown
- ordinalTanslations map[interface{}][]*transText
- rangeTanslations map[interface{}][]*transText
-}
-
-type transText struct {
- text string
- indexes []int
-}
-
-func newTranslator(trans locales.Translator) Translator {
- return &translator{
- Translator: trans,
- translations: make(map[interface{}]*transText), // translation text broken up by byte index
- cardinalTanslations: make(map[interface{}][]*transText),
- ordinalTanslations: make(map[interface{}][]*transText),
- rangeTanslations: make(map[interface{}][]*transText),
- }
-}
-
-// Add adds a normal translation for a particular language/locale
-// {#} is the only replacement type accepted and are ad infinitum
-// eg. one: '{0} day left' other: '{0} days left'
-func (t *translator) Add(key interface{}, text string, override bool) error {
-
- if _, ok := t.translations[key]; ok && !override {
- return &ErrConflictingTranslation{locale: t.Locale(), key: key, text: text}
- }
-
- lb := strings.Count(text, "{")
- rb := strings.Count(text, "}")
-
- if lb != rb {
- return &ErrMissingBracket{locale: t.Locale(), key: key, text: text}
- }
-
- trans := &transText{
- text: text,
- }
-
- var idx int
-
- for i := 0; i < lb; i++ {
- s := "{" + strconv.Itoa(i) + "}"
- idx = strings.Index(text, s)
- if idx == -1 {
- return &ErrBadParamSyntax{locale: t.Locale(), param: s, key: key, text: text}
- }
-
- trans.indexes = append(trans.indexes, idx)
- trans.indexes = append(trans.indexes, idx+len(s))
- }
-
- t.translations[key] = trans
-
- return nil
-}
-
-// AddCardinal adds a cardinal plural translation for a particular language/locale
-// {0} is the only replacement type accepted and only one variable is accepted as
-// multiple cannot be used for a plural rule determination, unless it is a range;
-// see AddRange below.
-// eg. in locale 'en' one: '{0} day left' other: '{0} days left'
-func (t *translator) AddCardinal(key interface{}, text string, rule locales.PluralRule, override bool) error {
-
- var verified bool
-
- // verify plural rule exists for locale
- for _, pr := range t.PluralsCardinal() {
- if pr == rule {
- verified = true
- break
- }
- }
-
- if !verified {
- return &ErrCardinalTranslation{text: fmt.Sprintf("error: cardinal plural rule '%s' does not exist for locale '%s' key: '%v' text: '%s'", rule, t.Locale(), key, text)}
- }
-
- tarr, ok := t.cardinalTanslations[key]
- if ok {
- // verify not adding a conflicting record
- if len(tarr) > 0 && tarr[rule] != nil && !override {
- return &ErrConflictingTranslation{locale: t.Locale(), key: key, rule: rule, text: text}
- }
-
- } else {
- tarr = make([]*transText, 7)
- t.cardinalTanslations[key] = tarr
- }
-
- trans := &transText{
- text: text,
- indexes: make([]int, 2),
- }
-
- tarr[rule] = trans
-
- idx := strings.Index(text, paramZero)
- if idx == -1 {
- tarr[rule] = nil
- return &ErrCardinalTranslation{text: fmt.Sprintf("error: parameter '%s' not found, may want to use 'Add' instead of 'AddCardinal'. locale: '%s' key: '%v' text: '%s'", paramZero, t.Locale(), key, text)}
- }
-
- trans.indexes[0] = idx
- trans.indexes[1] = idx + len(paramZero)
-
- return nil
-}
-
-// AddOrdinal adds an ordinal plural translation for a particular language/locale
-// {0} is the only replacement type accepted and only one variable is accepted as
-// multiple cannot be used for a plural rule determination, unless it is a range;
-// see AddRange below.
-// eg. in locale 'en' one: '{0}st day of spring' other: '{0}nd day of spring' - 1st, 2nd, 3rd...
-func (t *translator) AddOrdinal(key interface{}, text string, rule locales.PluralRule, override bool) error {
-
- var verified bool
-
- // verify plural rule exists for locale
- for _, pr := range t.PluralsOrdinal() {
- if pr == rule {
- verified = true
- break
- }
- }
-
- if !verified {
- return &ErrOrdinalTranslation{text: fmt.Sprintf("error: ordinal plural rule '%s' does not exist for locale '%s' key: '%v' text: '%s'", rule, t.Locale(), key, text)}
- }
-
- tarr, ok := t.ordinalTanslations[key]
- if ok {
- // verify not adding a conflicting record
- if len(tarr) > 0 && tarr[rule] != nil && !override {
- return &ErrConflictingTranslation{locale: t.Locale(), key: key, rule: rule, text: text}
- }
-
- } else {
- tarr = make([]*transText, 7)
- t.ordinalTanslations[key] = tarr
- }
-
- trans := &transText{
- text: text,
- indexes: make([]int, 2),
- }
-
- tarr[rule] = trans
-
- idx := strings.Index(text, paramZero)
- if idx == -1 {
- tarr[rule] = nil
- return &ErrOrdinalTranslation{text: fmt.Sprintf("error: parameter '%s' not found, may want to use 'Add' instead of 'AddOrdinal'. locale: '%s' key: '%v' text: '%s'", paramZero, t.Locale(), key, text)}
- }
-
- trans.indexes[0] = idx
- trans.indexes[1] = idx + len(paramZero)
-
- return nil
-}
-
-// AddRange adds a range plural translation for a particular language/locale
-// {0} and {1} are the only replacement types accepted and only these are accepted.
-// eg. in locale 'nl' one: '{0}-{1} day left' other: '{0}-{1} days left'
-func (t *translator) AddRange(key interface{}, text string, rule locales.PluralRule, override bool) error {
-
- var verified bool
-
- // verify plural rule exists for locale
- for _, pr := range t.PluralsRange() {
- if pr == rule {
- verified = true
- break
- }
- }
-
- if !verified {
- return &ErrRangeTranslation{text: fmt.Sprintf("error: range plural rule '%s' does not exist for locale '%s' key: '%v' text: '%s'", rule, t.Locale(), key, text)}
- }
-
- tarr, ok := t.rangeTanslations[key]
- if ok {
- // verify not adding a conflicting record
- if len(tarr) > 0 && tarr[rule] != nil && !override {
- return &ErrConflictingTranslation{locale: t.Locale(), key: key, rule: rule, text: text}
- }
-
- } else {
- tarr = make([]*transText, 7)
- t.rangeTanslations[key] = tarr
- }
-
- trans := &transText{
- text: text,
- indexes: make([]int, 4),
- }
-
- tarr[rule] = trans
-
- idx := strings.Index(text, paramZero)
- if idx == -1 {
- tarr[rule] = nil
- return &ErrRangeTranslation{text: fmt.Sprintf("error: parameter '%s' not found, are you sure you're adding a Range Translation? locale: '%s' key: '%v' text: '%s'", paramZero, t.Locale(), key, text)}
- }
-
- trans.indexes[0] = idx
- trans.indexes[1] = idx + len(paramZero)
-
- idx = strings.Index(text, paramOne)
- if idx == -1 {
- tarr[rule] = nil
- return &ErrRangeTranslation{text: fmt.Sprintf("error: parameter '%s' not found, a Range Translation requires two parameters. locale: '%s' key: '%v' text: '%s'", paramOne, t.Locale(), key, text)}
- }
-
- trans.indexes[2] = idx
- trans.indexes[3] = idx + len(paramOne)
-
- return nil
-}
-
-// T creates the translation for the locale given the 'key' and params passed in
-func (t *translator) T(key interface{}, params ...string) (string, error) {
-
- trans, ok := t.translations[key]
- if !ok {
- return unknownTranslation, ErrUnknowTranslation
- }
-
- b := make([]byte, 0, 64)
-
- var start, end, count int
-
- for i := 0; i < len(trans.indexes); i++ {
- end = trans.indexes[i]
- b = append(b, trans.text[start:end]...)
- b = append(b, params[count]...)
- i++
- start = trans.indexes[i]
- count++
- }
-
- b = append(b, trans.text[start:]...)
-
- return string(b), nil
-}
-
-// C creates the cardinal translation for the locale given the 'key', 'num' and 'digit' arguments and param passed in
-func (t *translator) C(key interface{}, num float64, digits uint64, param string) (string, error) {
-
- tarr, ok := t.cardinalTanslations[key]
- if !ok {
- return unknownTranslation, ErrUnknowTranslation
- }
-
- rule := t.CardinalPluralRule(num, digits)
-
- trans := tarr[rule]
-
- b := make([]byte, 0, 64)
- b = append(b, trans.text[:trans.indexes[0]]...)
- b = append(b, param...)
- b = append(b, trans.text[trans.indexes[1]:]...)
-
- return string(b), nil
-}
-
-// O creates the ordinal translation for the locale given the 'key', 'num' and 'digit' arguments and param passed in
-func (t *translator) O(key interface{}, num float64, digits uint64, param string) (string, error) {
-
- tarr, ok := t.ordinalTanslations[key]
- if !ok {
- return unknownTranslation, ErrUnknowTranslation
- }
-
- rule := t.OrdinalPluralRule(num, digits)
-
- trans := tarr[rule]
-
- b := make([]byte, 0, 64)
- b = append(b, trans.text[:trans.indexes[0]]...)
- b = append(b, param...)
- b = append(b, trans.text[trans.indexes[1]:]...)
-
- return string(b), nil
-}
-
-// R creates the range translation for the locale given the 'key', 'num1', 'digit1', 'num2' and 'digit2' arguments
-// and 'param1' and 'param2' passed in
-func (t *translator) R(key interface{}, num1 float64, digits1 uint64, num2 float64, digits2 uint64, param1, param2 string) (string, error) {
-
- tarr, ok := t.rangeTanslations[key]
- if !ok {
- return unknownTranslation, ErrUnknowTranslation
- }
-
- rule := t.RangePluralRule(num1, digits1, num2, digits2)
-
- trans := tarr[rule]
-
- b := make([]byte, 0, 64)
- b = append(b, trans.text[:trans.indexes[0]]...)
- b = append(b, param1...)
- b = append(b, trans.text[trans.indexes[1]:trans.indexes[2]]...)
- b = append(b, param2...)
- b = append(b, trans.text[trans.indexes[3]:]...)
-
- return string(b), nil
-}
-
-// VerifyTranslations checks to ensures that no plural rules have been
-// missed within the translations.
-func (t *translator) VerifyTranslations() error {
-
- for k, v := range t.cardinalTanslations {
-
- for _, rule := range t.PluralsCardinal() {
-
- if v[rule] == nil {
- return &ErrMissingPluralTranslation{locale: t.Locale(), translationType: "plural", rule: rule, key: k}
- }
- }
- }
-
- for k, v := range t.ordinalTanslations {
-
- for _, rule := range t.PluralsOrdinal() {
-
- if v[rule] == nil {
- return &ErrMissingPluralTranslation{locale: t.Locale(), translationType: "ordinal", rule: rule, key: k}
- }
- }
- }
-
- for k, v := range t.rangeTanslations {
-
- for _, rule := range t.PluralsRange() {
-
- if v[rule] == nil {
- return &ErrMissingPluralTranslation{locale: t.Locale(), translationType: "range", rule: rule, key: k}
- }
- }
- }
-
- return nil
-}
diff --git a/vendor/github.com/go-playground/universal-translator/universal_translator.go b/vendor/github.com/go-playground/universal-translator/universal_translator.go
deleted file mode 100644
index dbf707f5..00000000
--- a/vendor/github.com/go-playground/universal-translator/universal_translator.go
+++ /dev/null
@@ -1,113 +0,0 @@
-package ut
-
-import (
- "strings"
-
- "github.com/go-playground/locales"
-)
-
-// UniversalTranslator holds all locale & translation data
-type UniversalTranslator struct {
- translators map[string]Translator
- fallback Translator
-}
-
-// New returns a new UniversalTranslator instance set with
-// the fallback locale and locales it should support
-func New(fallback locales.Translator, supportedLocales ...locales.Translator) *UniversalTranslator {
-
- t := &UniversalTranslator{
- translators: make(map[string]Translator),
- }
-
- for _, v := range supportedLocales {
-
- trans := newTranslator(v)
- t.translators[strings.ToLower(trans.Locale())] = trans
-
- if fallback.Locale() == v.Locale() {
- t.fallback = trans
- }
- }
-
- if t.fallback == nil && fallback != nil {
- t.fallback = newTranslator(fallback)
- }
-
- return t
-}
-
-// FindTranslator trys to find a Translator based on an array of locales
-// and returns the first one it can find, otherwise returns the
-// fallback translator.
-func (t *UniversalTranslator) FindTranslator(locales ...string) (trans Translator, found bool) {
-
- for _, locale := range locales {
-
- if trans, found = t.translators[strings.ToLower(locale)]; found {
- return
- }
- }
-
- return t.fallback, false
-}
-
-// GetTranslator returns the specified translator for the given locale,
-// or fallback if not found
-func (t *UniversalTranslator) GetTranslator(locale string) (trans Translator, found bool) {
-
- if trans, found = t.translators[strings.ToLower(locale)]; found {
- return
- }
-
- return t.fallback, false
-}
-
-// GetFallback returns the fallback locale
-func (t *UniversalTranslator) GetFallback() Translator {
- return t.fallback
-}
-
-// AddTranslator adds the supplied translator, if it already exists the override param
-// will be checked and if false an error will be returned, otherwise the translator will be
-// overridden; if the fallback matches the supplied translator it will be overridden as well
-// NOTE: this is normally only used when translator is embedded within a library
-func (t *UniversalTranslator) AddTranslator(translator locales.Translator, override bool) error {
-
- lc := strings.ToLower(translator.Locale())
- _, ok := t.translators[lc]
- if ok && !override {
- return &ErrExistingTranslator{locale: translator.Locale()}
- }
-
- trans := newTranslator(translator)
-
- if t.fallback.Locale() == translator.Locale() {
-
- // because it's optional to have a fallback, I don't impose that limitation
- // don't know why you wouldn't but...
- if !override {
- return &ErrExistingTranslator{locale: translator.Locale()}
- }
-
- t.fallback = trans
- }
-
- t.translators[lc] = trans
-
- return nil
-}
-
-// VerifyTranslations runs through all locales and identifies any issues
-// eg. missing plural rules for a locale
-func (t *UniversalTranslator) VerifyTranslations() (err error) {
-
- for _, trans := range t.translators {
- err = trans.VerifyTranslations()
- if err != nil {
- return
- }
- }
-
- return
-}
diff --git a/vendor/github.com/go-playground/validator/v10/.gitignore b/vendor/github.com/go-playground/validator/v10/.gitignore
deleted file mode 100644
index 2410a91b..00000000
--- a/vendor/github.com/go-playground/validator/v10/.gitignore
+++ /dev/null
@@ -1,31 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-bin
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
-*.test
-*.out
-*.txt
-cover.html
-README.html
-.idea
diff --git a/vendor/github.com/go-playground/validator/v10/LICENSE b/vendor/github.com/go-playground/validator/v10/LICENSE
deleted file mode 100644
index 6a2ae9aa..00000000
--- a/vendor/github.com/go-playground/validator/v10/LICENSE
+++ /dev/null
@@ -1,22 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2015 Dean Karn
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
-
diff --git a/vendor/github.com/go-playground/validator/v10/MAINTAINERS.md b/vendor/github.com/go-playground/validator/v10/MAINTAINERS.md
deleted file mode 100644
index b809c4ce..00000000
--- a/vendor/github.com/go-playground/validator/v10/MAINTAINERS.md
+++ /dev/null
@@ -1,16 +0,0 @@
-## Maintainers Guide
-
-### Semantic Versioning
-Semantic versioning as defined [here](https://semver.org) must be strictly adhered to.
-
-### External Dependencies
-Any new external dependencies MUST:
-- Have a compatible LICENSE present.
-- Be actively maintained.
-- Be approved by @go-playground/admins
-
-### PR Merge Requirements
-- Up-to-date branch.
-- Passing tests and linting.
-- CODEOWNERS approval.
-- Tests that cover both the Happy and Unhappy paths.
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/validator/v10/Makefile b/vendor/github.com/go-playground/validator/v10/Makefile
deleted file mode 100644
index ec3455bd..00000000
--- a/vendor/github.com/go-playground/validator/v10/Makefile
+++ /dev/null
@@ -1,18 +0,0 @@
-GOCMD=GO111MODULE=on go
-
-linters-install:
- @golangci-lint --version >/dev/null 2>&1 || { \
- echo "installing linting tools..."; \
- curl -sfL https://raw.githubusercontent.com/golangci/golangci-lint/master/install.sh| sh -s v1.41.1; \
- }
-
-lint: linters-install
- golangci-lint run
-
-test:
- $(GOCMD) test -cover -race ./...
-
-bench:
- $(GOCMD) test -bench=. -benchmem ./...
-
-.PHONY: test lint linters-install
\ No newline at end of file
diff --git a/vendor/github.com/go-playground/validator/v10/README.md b/vendor/github.com/go-playground/validator/v10/README.md
deleted file mode 100644
index 5f8878d2..00000000
--- a/vendor/github.com/go-playground/validator/v10/README.md
+++ /dev/null
@@ -1,338 +0,0 @@
-Package validator
-=================
-
[](https://gitter.im/go-playground/validator?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
-
-[](https://travis-ci.org/go-playground/validator)
-[](https://coveralls.io/github/go-playground/validator?branch=master)
-[](https://goreportcard.com/report/github.com/go-playground/validator)
-[](https://pkg.go.dev/github.com/go-playground/validator/v10)
-
-
-Package validator implements value validations for structs and individual fields based on tags.
-
-It has the following **unique** features:
-
-- Cross Field and Cross Struct validations by using validation tags or custom validators.
-- Slice, Array and Map diving, which allows any or all levels of a multidimensional field to be validated.
-- Ability to dive into both map keys and values for validation
-- Handles type interface by determining it's underlying type prior to validation.
-- Handles custom field types such as sql driver Valuer see [Valuer](https://golang.org/src/database/sql/driver/types.go?s=1210:1293#L29)
-- Alias validation tags, which allows for mapping of several validations to a single tag for easier defining of validations on structs
-- Extraction of custom defined Field Name e.g. can specify to extract the JSON name while validating and have it available in the resulting FieldError
-- Customizable i18n aware error messages.
-- Default validator for the [gin](https://github.com/gin-gonic/gin) web framework; upgrading from v8 to v9 in gin see [here](https://github.com/go-playground/validator/tree/master/_examples/gin-upgrading-overriding)
-
-Installation
-------------
-
-Use go get.
-
- go get github.com/go-playground/validator/v10
-
-Then import the validator package into your own code.
-
- import "github.com/go-playground/validator/v10"
-
-Error Return Value
--------
-
-Validation functions return type error
-
-They return type error to avoid the issue discussed in the following, where err is always != nil:
-
-* http://stackoverflow.com/a/29138676/3158232
-* https://github.com/go-playground/validator/issues/134
-
-Validator returns only InvalidValidationError for bad validation input, nil or ValidationErrors as type error; so, in your code all you need to do is check if the error returned is not nil, and if it's not check if error is InvalidValidationError ( if necessary, most of the time it isn't ) type cast it to type ValidationErrors like so:
-
-```go
-err := validate.Struct(mystruct)
-validationErrors := err.(validator.ValidationErrors)
- ```
-
-Usage and documentation
-------
-
-Please see https://pkg.go.dev/github.com/go-playground/validator/v10 for detailed usage docs.
-
-##### Examples:
-
-- [Simple](https://github.com/go-playground/validator/blob/master/_examples/simple/main.go)
-- [Custom Field Types](https://github.com/go-playground/validator/blob/master/_examples/custom/main.go)
-- [Struct Level](https://github.com/go-playground/validator/blob/master/_examples/struct-level/main.go)
-- [Translations & Custom Errors](https://github.com/go-playground/validator/blob/master/_examples/translations/main.go)
-- [Gin upgrade and/or override validator](https://github.com/go-playground/validator/tree/v9/_examples/gin-upgrading-overriding)
-- [wash - an example application putting it all together](https://github.com/bluesuncorp/wash)
-
-Baked-in Validations
-------
-
-### Fields:
-
-| Tag | Description |
-| - | - |
-| eqcsfield | Field Equals Another Field (relative)|
-| eqfield | Field Equals Another Field |
-| fieldcontains | NOT DOCUMENTED IN doc.go |
-| fieldexcludes | NOT DOCUMENTED IN doc.go |
-| gtcsfield | Field Greater Than Another Relative Field |
-| gtecsfield | Field Greater Than or Equal To Another Relative Field |
-| gtefield | Field Greater Than or Equal To Another Field |
-| gtfield | Field Greater Than Another Field |
-| ltcsfield | Less Than Another Relative Field |
-| ltecsfield | Less Than or Equal To Another Relative Field |
-| ltefield | Less Than or Equal To Another Field |
-| ltfield | Less Than Another Field |
-| necsfield | Field Does Not Equal Another Field (relative) |
-| nefield | Field Does Not Equal Another Field |
-
-### Network:
-
-| Tag | Description |
-| - | - |
-| cidr | Classless Inter-Domain Routing CIDR |
-| cidrv4 | Classless Inter-Domain Routing CIDRv4 |
-| cidrv6 | Classless Inter-Domain Routing CIDRv6 |
-| datauri | Data URL |
-| fqdn | Full Qualified Domain Name (FQDN) |
-| hostname | Hostname RFC 952 |
-| hostname_port | HostPort |
-| hostname_rfc1123 | Hostname RFC 1123 |
-| ip | Internet Protocol Address IP |
-| ip4_addr | Internet Protocol Address IPv4 |
-| ip6_addr | Internet Protocol Address IPv6 |
-| ip_addr | Internet Protocol Address IP |
-| ipv4 | Internet Protocol Address IPv4 |
-| ipv6 | Internet Protocol Address IPv6 |
-| mac | Media Access Control Address MAC |
-| tcp4_addr | Transmission Control Protocol Address TCPv4 |
-| tcp6_addr | Transmission Control Protocol Address TCPv6 |
-| tcp_addr | Transmission Control Protocol Address TCP |
-| udp4_addr | User Datagram Protocol Address UDPv4 |
-| udp6_addr | User Datagram Protocol Address UDPv6 |
-| udp_addr | User Datagram Protocol Address UDP |
-| unix_addr | Unix domain socket end point Address |
-| uri | URI String |
-| url | URL String |
-| url_encoded | URL Encoded |
-| urn_rfc2141 | Urn RFC 2141 String |
-
-### Strings:
-
-| Tag | Description |
-| - | - |
-| alpha | Alpha Only |
-| alphanum | Alphanumeric |
-| alphanumunicode | Alphanumeric Unicode |
-| alphaunicode | Alpha Unicode |
-| ascii | ASCII |
-| boolean | Boolean |
-| contains | Contains |
-| containsany | Contains Any |
-| containsrune | Contains Rune |
-| endsnotwith | Ends Not With |
-| endswith | Ends With |
-| excludes | Excludes |
-| excludesall | Excludes All |
-| excludesrune | Excludes Rune |
-| lowercase | Lowercase |
-| multibyte | Multi-Byte Characters |
-| number | NOT DOCUMENTED IN doc.go |
-| numeric | Numeric |
-| printascii | Printable ASCII |
-| startsnotwith | Starts Not With |
-| startswith | Starts With |
-| uppercase | Uppercase |
-
-### Format:
-| Tag | Description |
-| - | - |
-| base64 | Base64 String |
-| base64url | Base64URL String |
-| bic | Business Identifier Code (ISO 9362) |
-| bcp47_language_tag | Language tag (BCP 47) |
-| btc_addr | Bitcoin Address |
-| btc_addr_bech32 | Bitcoin Bech32 Address (segwit) |
-| credit_card | Credit Card Number |
-| datetime | Datetime |
-| e164 | e164 formatted phone number |
-| email | E-mail String
-| eth_addr | Ethereum Address |
-| hexadecimal | Hexadecimal String |
-| hexcolor | Hexcolor String |
-| hsl | HSL String |
-| hsla | HSLA String |
-| html | HTML Tags |
-| html_encoded | HTML Encoded |
-| isbn | International Standard Book Number |
-| isbn10 | International Standard Book Number 10 |
-| isbn13 | International Standard Book Number 13 |
-| iso3166_1_alpha2 | Two-letter country code (ISO 3166-1 alpha-2) |
-| iso3166_1_alpha3 | Three-letter country code (ISO 3166-1 alpha-3) |
-| iso3166_1_alpha_numeric | Numeric country code (ISO 3166-1 numeric) |
-| iso3166_2 | Country subdivision code (ISO 3166-2) |
-| iso4217 | Currency code (ISO 4217) |
-| json | JSON |
-| jwt | JSON Web Token (JWT) |
-| latitude | Latitude |
-| longitude | Longitude |
-| postcode_iso3166_alpha2 | Postcode |
-| postcode_iso3166_alpha2_field | Postcode |
-| rgb | RGB String |
-| rgba | RGBA String |
-| ssn | Social Security Number SSN |
-| timezone | Timezone |
-| uuid | Universally Unique Identifier UUID |
-| uuid3 | Universally Unique Identifier UUID v3 |
-| uuid3_rfc4122 | Universally Unique Identifier UUID v3 RFC4122 |
-| uuid4 | Universally Unique Identifier UUID v4 |
-| uuid4_rfc4122 | Universally Unique Identifier UUID v4 RFC4122 |
-| uuid5 | Universally Unique Identifier UUID v5 |
-| uuid5_rfc4122 | Universally Unique Identifier UUID v5 RFC4122 |
-| uuid_rfc4122 | Universally Unique Identifier UUID RFC4122 |
-| md4 | MD4 hash |
-| md5 | MD5 hash |
-| sha256 | SHA256 hash |
-| sha384 | SHA384 hash |
-| sha512 | SHA512 hash |
-| ripemd128 | RIPEMD-128 hash |
-| ripemd128 | RIPEMD-160 hash |
-| tiger128 | TIGER128 hash |
-| tiger160 | TIGER160 hash |
-| tiger192 | TIGER192 hash |
-| semver | Semantic Versioning 2.0.0 |
-| ulid | Universally Unique Lexicographically Sortable Identifier ULID |
-
-### Comparisons:
-| Tag | Description |
-| - | - |
-| eq | Equals |
-| gt | Greater than|
-| gte | Greater than or equal |
-| lt | Less Than |
-| lte | Less Than or Equal |
-| ne | Not Equal |
-
-### Other:
-| Tag | Description |
-| - | - |
-| dir | Directory |
-| file | File path |
-| isdefault | Is Default |
-| len | Length |
-| max | Maximum |
-| min | Minimum |
-| oneof | One Of |
-| required | Required |
-| required_if | Required If |
-| required_unless | Required Unless |
-| required_with | Required With |
-| required_with_all | Required With All |
-| required_without | Required Without |
-| required_without_all | Required Without All |
-| excluded_if | Excluded If |
-| excluded_unless | Excluded Unless |
-| excluded_with | Excluded With |
-| excluded_with_all | Excluded With All |
-| excluded_without | Excluded Without |
-| excluded_without_all | Excluded Without All |
-| unique | Unique |
-
-#### Aliases:
-| Tag | Description |
-| - | - |
-| iscolor | hexcolor\|rgb\|rgba\|hsl\|hsla |
-| country_code | iso3166_1_alpha2\|iso3166_1_alpha3\|iso3166_1_alpha_numeric |
-
-Benchmarks
-------
-###### Run on MacBook Pro (15-inch, 2017) go version go1.10.2 darwin/amd64
-```go
-goos: darwin
-goarch: amd64
-pkg: github.com/go-playground/validator
-BenchmarkFieldSuccess-8 20000000 83.6 ns/op 0 B/op 0 allocs/op
-BenchmarkFieldSuccessParallel-8 50000000 26.8 ns/op 0 B/op 0 allocs/op
-BenchmarkFieldFailure-8 5000000 291 ns/op 208 B/op 4 allocs/op
-BenchmarkFieldFailureParallel-8 20000000 107 ns/op 208 B/op 4 allocs/op
-BenchmarkFieldArrayDiveSuccess-8 2000000 623 ns/op 201 B/op 11 allocs/op
-BenchmarkFieldArrayDiveSuccessParallel-8 10000000 237 ns/op 201 B/op 11 allocs/op
-BenchmarkFieldArrayDiveFailure-8 2000000 859 ns/op 412 B/op 16 allocs/op
-BenchmarkFieldArrayDiveFailureParallel-8 5000000 335 ns/op 413 B/op 16 allocs/op
-BenchmarkFieldMapDiveSuccess-8 1000000 1292 ns/op 432 B/op 18 allocs/op
-BenchmarkFieldMapDiveSuccessParallel-8 3000000 467 ns/op 432 B/op 18 allocs/op
-BenchmarkFieldMapDiveFailure-8 1000000 1082 ns/op 512 B/op 16 allocs/op
-BenchmarkFieldMapDiveFailureParallel-8 5000000 425 ns/op 512 B/op 16 allocs/op
-BenchmarkFieldMapDiveWithKeysSuccess-8 1000000 1539 ns/op 480 B/op 21 allocs/op
-BenchmarkFieldMapDiveWithKeysSuccessParallel-8 3000000 613 ns/op 480 B/op 21 allocs/op
-BenchmarkFieldMapDiveWithKeysFailure-8 1000000 1413 ns/op 721 B/op 21 allocs/op
-BenchmarkFieldMapDiveWithKeysFailureParallel-8 3000000 575 ns/op 721 B/op 21 allocs/op
-BenchmarkFieldCustomTypeSuccess-8 10000000 216 ns/op 32 B/op 2 allocs/op
-BenchmarkFieldCustomTypeSuccessParallel-8 20000000 82.2 ns/op 32 B/op 2 allocs/op
-BenchmarkFieldCustomTypeFailure-8 5000000 274 ns/op 208 B/op 4 allocs/op
-BenchmarkFieldCustomTypeFailureParallel-8 20000000 116 ns/op 208 B/op 4 allocs/op
-BenchmarkFieldOrTagSuccess-8 2000000 740 ns/op 16 B/op 1 allocs/op
-BenchmarkFieldOrTagSuccessParallel-8 3000000 474 ns/op 16 B/op 1 allocs/op
-BenchmarkFieldOrTagFailure-8 3000000 471 ns/op 224 B/op 5 allocs/op
-BenchmarkFieldOrTagFailureParallel-8 3000000 414 ns/op 224 B/op 5 allocs/op
-BenchmarkStructLevelValidationSuccess-8 10000000 213 ns/op 32 B/op 2 allocs/op
-BenchmarkStructLevelValidationSuccessParallel-8 20000000 91.8 ns/op 32 B/op 2 allocs/op
-BenchmarkStructLevelValidationFailure-8 3000000 473 ns/op 304 B/op 8 allocs/op
-BenchmarkStructLevelValidationFailureParallel-8 10000000 234 ns/op 304 B/op 8 allocs/op
-BenchmarkStructSimpleCustomTypeSuccess-8 5000000 385 ns/op 32 B/op 2 allocs/op
-BenchmarkStructSimpleCustomTypeSuccessParallel-8 10000000 161 ns/op 32 B/op 2 allocs/op
-BenchmarkStructSimpleCustomTypeFailure-8 2000000 640 ns/op 424 B/op 9 allocs/op
-BenchmarkStructSimpleCustomTypeFailureParallel-8 5000000 318 ns/op 440 B/op 10 allocs/op
-BenchmarkStructFilteredSuccess-8 2000000 597 ns/op 288 B/op 9 allocs/op
-BenchmarkStructFilteredSuccessParallel-8 10000000 266 ns/op 288 B/op 9 allocs/op
-BenchmarkStructFilteredFailure-8 3000000 454 ns/op 256 B/op 7 allocs/op
-BenchmarkStructFilteredFailureParallel-8 10000000 214 ns/op 256 B/op 7 allocs/op
-BenchmarkStructPartialSuccess-8 3000000 502 ns/op 256 B/op 6 allocs/op
-BenchmarkStructPartialSuccessParallel-8 10000000 225 ns/op 256 B/op 6 allocs/op
-BenchmarkStructPartialFailure-8 2000000 702 ns/op 480 B/op 11 allocs/op
-BenchmarkStructPartialFailureParallel-8 5000000 329 ns/op 480 B/op 11 allocs/op
-BenchmarkStructExceptSuccess-8 2000000 793 ns/op 496 B/op 12 allocs/op
-BenchmarkStructExceptSuccessParallel-8 10000000 193 ns/op 240 B/op 5 allocs/op
-BenchmarkStructExceptFailure-8 2000000 639 ns/op 464 B/op 10 allocs/op
-BenchmarkStructExceptFailureParallel-8 5000000 300 ns/op 464 B/op 10 allocs/op
-BenchmarkStructSimpleCrossFieldSuccess-8 3000000 417 ns/op 72 B/op 3 allocs/op
-BenchmarkStructSimpleCrossFieldSuccessParallel-8 10000000 163 ns/op 72 B/op 3 allocs/op
-BenchmarkStructSimpleCrossFieldFailure-8 2000000 645 ns/op 304 B/op 8 allocs/op
-BenchmarkStructSimpleCrossFieldFailureParallel-8 5000000 285 ns/op 304 B/op 8 allocs/op
-BenchmarkStructSimpleCrossStructCrossFieldSuccess-8 3000000 588 ns/op 80 B/op 4 allocs/op
-BenchmarkStructSimpleCrossStructCrossFieldSuccessParallel-8 10000000 221 ns/op 80 B/op 4 allocs/op
-BenchmarkStructSimpleCrossStructCrossFieldFailure-8 2000000 868 ns/op 320 B/op 9 allocs/op
-BenchmarkStructSimpleCrossStructCrossFieldFailureParallel-8 5000000 337 ns/op 320 B/op 9 allocs/op
-BenchmarkStructSimpleSuccess-8 5000000 260 ns/op 0 B/op 0 allocs/op
-BenchmarkStructSimpleSuccessParallel-8 20000000 90.6 ns/op 0 B/op 0 allocs/op
-BenchmarkStructSimpleFailure-8 2000000 619 ns/op 424 B/op 9 allocs/op
-BenchmarkStructSimpleFailureParallel-8 5000000 296 ns/op 424 B/op 9 allocs/op
-BenchmarkStructComplexSuccess-8 1000000 1454 ns/op 128 B/op 8 allocs/op
-BenchmarkStructComplexSuccessParallel-8 3000000 579 ns/op 128 B/op 8 allocs/op
-BenchmarkStructComplexFailure-8 300000 4140 ns/op 3041 B/op 53 allocs/op
-BenchmarkStructComplexFailureParallel-8 1000000 2127 ns/op 3041 B/op 53 allocs/op
-BenchmarkOneof-8 10000000 140 ns/op 0 B/op 0 allocs/op
-BenchmarkOneofParallel-8 20000000 70.1 ns/op 0 B/op 0 allocs/op
-```
-
-Complementary Software
-----------------------
-
-Here is a list of software that complements using this library either pre or post validation.
-
-* [form](https://github.com/go-playground/form) - Decodes url.Values into Go value(s) and Encodes Go value(s) into url.Values. Dual Array and Full map support.
-* [mold](https://github.com/go-playground/mold) - A general library to help modify or set data within data structures and other objects
-
-How to Contribute
-------
-
-Make a pull request...
-
-License
--------
-Distributed under MIT License, please see license file within the code for more details.
-
-Maintainers
------------
-This project has grown large enough that more than one person is required to properly support the community.
-If you are interested in becoming a maintainer please reach out to me https://github.com/deankarn
diff --git a/vendor/github.com/go-playground/validator/v10/baked_in.go b/vendor/github.com/go-playground/validator/v10/baked_in.go
deleted file mode 100644
index c9b1db40..00000000
--- a/vendor/github.com/go-playground/validator/v10/baked_in.go
+++ /dev/null
@@ -1,2526 +0,0 @@
-package validator
-
-import (
- "bytes"
- "context"
- "crypto/sha256"
- "encoding/hex"
- "encoding/json"
- "fmt"
- "net"
- "net/url"
- "os"
- "reflect"
- "strconv"
- "strings"
- "sync"
- "time"
- "unicode/utf8"
-
- "golang.org/x/crypto/sha3"
- "golang.org/x/text/language"
-
- urn "github.com/leodido/go-urn"
-)
-
-// Func accepts a FieldLevel interface for all validation needs. The return
-// value should be true when validation succeeds.
-type Func func(fl FieldLevel) bool
-
-// FuncCtx accepts a context.Context and FieldLevel interface for all
-// validation needs. The return value should be true when validation succeeds.
-type FuncCtx func(ctx context.Context, fl FieldLevel) bool
-
-// wrapFunc wraps noramal Func makes it compatible with FuncCtx
-func wrapFunc(fn Func) FuncCtx {
- if fn == nil {
- return nil // be sure not to wrap a bad function.
- }
- return func(ctx context.Context, fl FieldLevel) bool {
- return fn(fl)
- }
-}
-
-var (
- restrictedTags = map[string]struct{}{
- diveTag: {},
- keysTag: {},
- endKeysTag: {},
- structOnlyTag: {},
- omitempty: {},
- skipValidationTag: {},
- utf8HexComma: {},
- utf8Pipe: {},
- noStructLevelTag: {},
- requiredTag: {},
- isdefault: {},
- }
-
- // bakedInAliases is a default mapping of a single validation tag that
- // defines a common or complex set of validation(s) to simplify
- // adding validation to structs.
- bakedInAliases = map[string]string{
- "iscolor": "hexcolor|rgb|rgba|hsl|hsla",
- "country_code": "iso3166_1_alpha2|iso3166_1_alpha3|iso3166_1_alpha_numeric",
- }
-
- // bakedInValidators is the default map of ValidationFunc
- // you can add, remove or even replace items to suite your needs,
- // or even disregard and use your own map if so desired.
- bakedInValidators = map[string]Func{
- "required": hasValue,
- "required_if": requiredIf,
- "required_unless": requiredUnless,
- "required_with": requiredWith,
- "required_with_all": requiredWithAll,
- "required_without": requiredWithout,
- "required_without_all": requiredWithoutAll,
- "excluded_if": excludedIf,
- "excluded_unless": excludedUnless,
- "excluded_with": excludedWith,
- "excluded_with_all": excludedWithAll,
- "excluded_without": excludedWithout,
- "excluded_without_all": excludedWithoutAll,
- "isdefault": isDefault,
- "len": hasLengthOf,
- "min": hasMinOf,
- "max": hasMaxOf,
- "eq": isEq,
- "ne": isNe,
- "lt": isLt,
- "lte": isLte,
- "gt": isGt,
- "gte": isGte,
- "eqfield": isEqField,
- "eqcsfield": isEqCrossStructField,
- "necsfield": isNeCrossStructField,
- "gtcsfield": isGtCrossStructField,
- "gtecsfield": isGteCrossStructField,
- "ltcsfield": isLtCrossStructField,
- "ltecsfield": isLteCrossStructField,
- "nefield": isNeField,
- "gtefield": isGteField,
- "gtfield": isGtField,
- "ltefield": isLteField,
- "ltfield": isLtField,
- "fieldcontains": fieldContains,
- "fieldexcludes": fieldExcludes,
- "alpha": isAlpha,
- "alphanum": isAlphanum,
- "alphaunicode": isAlphaUnicode,
- "alphanumunicode": isAlphanumUnicode,
- "boolean": isBoolean,
- "numeric": isNumeric,
- "number": isNumber,
- "hexadecimal": isHexadecimal,
- "hexcolor": isHEXColor,
- "rgb": isRGB,
- "rgba": isRGBA,
- "hsl": isHSL,
- "hsla": isHSLA,
- "e164": isE164,
- "email": isEmail,
- "url": isURL,
- "uri": isURI,
- "urn_rfc2141": isUrnRFC2141, // RFC 2141
- "file": isFile,
- "base64": isBase64,
- "base64url": isBase64URL,
- "contains": contains,
- "containsany": containsAny,
- "containsrune": containsRune,
- "excludes": excludes,
- "excludesall": excludesAll,
- "excludesrune": excludesRune,
- "startswith": startsWith,
- "endswith": endsWith,
- "startsnotwith": startsNotWith,
- "endsnotwith": endsNotWith,
- "isbn": isISBN,
- "isbn10": isISBN10,
- "isbn13": isISBN13,
- "eth_addr": isEthereumAddress,
- "btc_addr": isBitcoinAddress,
- "btc_addr_bech32": isBitcoinBech32Address,
- "uuid": isUUID,
- "uuid3": isUUID3,
- "uuid4": isUUID4,
- "uuid5": isUUID5,
- "uuid_rfc4122": isUUIDRFC4122,
- "uuid3_rfc4122": isUUID3RFC4122,
- "uuid4_rfc4122": isUUID4RFC4122,
- "uuid5_rfc4122": isUUID5RFC4122,
- "ulid": isULID,
- "md4": isMD4,
- "md5": isMD5,
- "sha256": isSHA256,
- "sha384": isSHA384,
- "sha512": isSHA512,
- "ripemd128": isRIPEMD128,
- "ripemd160": isRIPEMD160,
- "tiger128": isTIGER128,
- "tiger160": isTIGER160,
- "tiger192": isTIGER192,
- "ascii": isASCII,
- "printascii": isPrintableASCII,
- "multibyte": hasMultiByteCharacter,
- "datauri": isDataURI,
- "latitude": isLatitude,
- "longitude": isLongitude,
- "ssn": isSSN,
- "ipv4": isIPv4,
- "ipv6": isIPv6,
- "ip": isIP,
- "cidrv4": isCIDRv4,
- "cidrv6": isCIDRv6,
- "cidr": isCIDR,
- "tcp4_addr": isTCP4AddrResolvable,
- "tcp6_addr": isTCP6AddrResolvable,
- "tcp_addr": isTCPAddrResolvable,
- "udp4_addr": isUDP4AddrResolvable,
- "udp6_addr": isUDP6AddrResolvable,
- "udp_addr": isUDPAddrResolvable,
- "ip4_addr": isIP4AddrResolvable,
- "ip6_addr": isIP6AddrResolvable,
- "ip_addr": isIPAddrResolvable,
- "unix_addr": isUnixAddrResolvable,
- "mac": isMAC,
- "hostname": isHostnameRFC952, // RFC 952
- "hostname_rfc1123": isHostnameRFC1123, // RFC 1123
- "fqdn": isFQDN,
- "unique": isUnique,
- "oneof": isOneOf,
- "html": isHTML,
- "html_encoded": isHTMLEncoded,
- "url_encoded": isURLEncoded,
- "dir": isDir,
- "json": isJSON,
- "jwt": isJWT,
- "hostname_port": isHostnamePort,
- "lowercase": isLowercase,
- "uppercase": isUppercase,
- "datetime": isDatetime,
- "timezone": isTimeZone,
- "iso3166_1_alpha2": isIso3166Alpha2,
- "iso3166_1_alpha3": isIso3166Alpha3,
- "iso3166_1_alpha_numeric": isIso3166AlphaNumeric,
- "iso3166_2": isIso31662,
- "iso4217": isIso4217,
- "iso4217_numeric": isIso4217Numeric,
- "bcp47_language_tag": isBCP47LanguageTag,
- "postcode_iso3166_alpha2": isPostcodeByIso3166Alpha2,
- "postcode_iso3166_alpha2_field": isPostcodeByIso3166Alpha2Field,
- "bic": isIsoBicFormat,
- "semver": isSemverFormat,
- "dns_rfc1035_label": isDnsRFC1035LabelFormat,
- "credit_card": isCreditCard,
- }
-)
-
-var (
- oneofValsCache = map[string][]string{}
- oneofValsCacheRWLock = sync.RWMutex{}
-)
-
-func parseOneOfParam2(s string) []string {
- oneofValsCacheRWLock.RLock()
- vals, ok := oneofValsCache[s]
- oneofValsCacheRWLock.RUnlock()
- if !ok {
- oneofValsCacheRWLock.Lock()
- vals = splitParamsRegex.FindAllString(s, -1)
- for i := 0; i < len(vals); i++ {
- vals[i] = strings.Replace(vals[i], "'", "", -1)
- }
- oneofValsCache[s] = vals
- oneofValsCacheRWLock.Unlock()
- }
- return vals
-}
-
-func isURLEncoded(fl FieldLevel) bool {
- return uRLEncodedRegex.MatchString(fl.Field().String())
-}
-
-func isHTMLEncoded(fl FieldLevel) bool {
- return hTMLEncodedRegex.MatchString(fl.Field().String())
-}
-
-func isHTML(fl FieldLevel) bool {
- return hTMLRegex.MatchString(fl.Field().String())
-}
-
-func isOneOf(fl FieldLevel) bool {
- vals := parseOneOfParam2(fl.Param())
-
- field := fl.Field()
-
- var v string
- switch field.Kind() {
- case reflect.String:
- v = field.String()
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- v = strconv.FormatInt(field.Int(), 10)
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64:
- v = strconv.FormatUint(field.Uint(), 10)
- default:
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
- }
- for i := 0; i < len(vals); i++ {
- if vals[i] == v {
- return true
- }
- }
- return false
-}
-
-// isUnique is the validation function for validating if each array|slice|map value is unique
-func isUnique(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
- v := reflect.ValueOf(struct{}{})
-
- switch field.Kind() {
- case reflect.Slice, reflect.Array:
- elem := field.Type().Elem()
- if elem.Kind() == reflect.Ptr {
- elem = elem.Elem()
- }
-
- if param == "" {
- m := reflect.MakeMap(reflect.MapOf(elem, v.Type()))
-
- for i := 0; i < field.Len(); i++ {
- m.SetMapIndex(reflect.Indirect(field.Index(i)), v)
- }
- return field.Len() == m.Len()
- }
-
- sf, ok := elem.FieldByName(param)
- if !ok {
- panic(fmt.Sprintf("Bad field name %s", param))
- }
-
- sfTyp := sf.Type
- if sfTyp.Kind() == reflect.Ptr {
- sfTyp = sfTyp.Elem()
- }
-
- m := reflect.MakeMap(reflect.MapOf(sfTyp, v.Type()))
- for i := 0; i < field.Len(); i++ {
- m.SetMapIndex(reflect.Indirect(reflect.Indirect(field.Index(i)).FieldByName(param)), v)
- }
- return field.Len() == m.Len()
- case reflect.Map:
- m := reflect.MakeMap(reflect.MapOf(field.Type().Elem(), v.Type()))
-
- for _, k := range field.MapKeys() {
- m.SetMapIndex(field.MapIndex(k), v)
- }
- return field.Len() == m.Len()
- default:
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
- }
-}
-
-// isMAC is the validation function for validating if the field's value is a valid MAC address.
-func isMAC(fl FieldLevel) bool {
- _, err := net.ParseMAC(fl.Field().String())
-
- return err == nil
-}
-
-// isCIDRv4 is the validation function for validating if the field's value is a valid v4 CIDR address.
-func isCIDRv4(fl FieldLevel) bool {
- ip, _, err := net.ParseCIDR(fl.Field().String())
-
- return err == nil && ip.To4() != nil
-}
-
-// isCIDRv6 is the validation function for validating if the field's value is a valid v6 CIDR address.
-func isCIDRv6(fl FieldLevel) bool {
- ip, _, err := net.ParseCIDR(fl.Field().String())
-
- return err == nil && ip.To4() == nil
-}
-
-// isCIDR is the validation function for validating if the field's value is a valid v4 or v6 CIDR address.
-func isCIDR(fl FieldLevel) bool {
- _, _, err := net.ParseCIDR(fl.Field().String())
-
- return err == nil
-}
-
-// isIPv4 is the validation function for validating if a value is a valid v4 IP address.
-func isIPv4(fl FieldLevel) bool {
- ip := net.ParseIP(fl.Field().String())
-
- return ip != nil && ip.To4() != nil
-}
-
-// isIPv6 is the validation function for validating if the field's value is a valid v6 IP address.
-func isIPv6(fl FieldLevel) bool {
- ip := net.ParseIP(fl.Field().String())
-
- return ip != nil && ip.To4() == nil
-}
-
-// isIP is the validation function for validating if the field's value is a valid v4 or v6 IP address.
-func isIP(fl FieldLevel) bool {
- ip := net.ParseIP(fl.Field().String())
-
- return ip != nil
-}
-
-// isSSN is the validation function for validating if the field's value is a valid SSN.
-func isSSN(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Len() != 11 {
- return false
- }
-
- return sSNRegex.MatchString(field.String())
-}
-
-// isLongitude is the validation function for validating if the field's value is a valid longitude coordinate.
-func isLongitude(fl FieldLevel) bool {
- field := fl.Field()
-
- var v string
- switch field.Kind() {
- case reflect.String:
- v = field.String()
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- v = strconv.FormatInt(field.Int(), 10)
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64:
- v = strconv.FormatUint(field.Uint(), 10)
- case reflect.Float32:
- v = strconv.FormatFloat(field.Float(), 'f', -1, 32)
- case reflect.Float64:
- v = strconv.FormatFloat(field.Float(), 'f', -1, 64)
- default:
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
- }
-
- return longitudeRegex.MatchString(v)
-}
-
-// isLatitude is the validation function for validating if the field's value is a valid latitude coordinate.
-func isLatitude(fl FieldLevel) bool {
- field := fl.Field()
-
- var v string
- switch field.Kind() {
- case reflect.String:
- v = field.String()
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- v = strconv.FormatInt(field.Int(), 10)
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64:
- v = strconv.FormatUint(field.Uint(), 10)
- case reflect.Float32:
- v = strconv.FormatFloat(field.Float(), 'f', -1, 32)
- case reflect.Float64:
- v = strconv.FormatFloat(field.Float(), 'f', -1, 64)
- default:
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
- }
-
- return latitudeRegex.MatchString(v)
-}
-
-// isDataURI is the validation function for validating if the field's value is a valid data URI.
-func isDataURI(fl FieldLevel) bool {
- uri := strings.SplitN(fl.Field().String(), ",", 2)
-
- if len(uri) != 2 {
- return false
- }
-
- if !dataURIRegex.MatchString(uri[0]) {
- return false
- }
-
- return base64Regex.MatchString(uri[1])
-}
-
-// hasMultiByteCharacter is the validation function for validating if the field's value has a multi byte character.
-func hasMultiByteCharacter(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Len() == 0 {
- return true
- }
-
- return multibyteRegex.MatchString(field.String())
-}
-
-// isPrintableASCII is the validation function for validating if the field's value is a valid printable ASCII character.
-func isPrintableASCII(fl FieldLevel) bool {
- return printableASCIIRegex.MatchString(fl.Field().String())
-}
-
-// isASCII is the validation function for validating if the field's value is a valid ASCII character.
-func isASCII(fl FieldLevel) bool {
- return aSCIIRegex.MatchString(fl.Field().String())
-}
-
-// isUUID5 is the validation function for validating if the field's value is a valid v5 UUID.
-func isUUID5(fl FieldLevel) bool {
- return uUID5Regex.MatchString(fl.Field().String())
-}
-
-// isUUID4 is the validation function for validating if the field's value is a valid v4 UUID.
-func isUUID4(fl FieldLevel) bool {
- return uUID4Regex.MatchString(fl.Field().String())
-}
-
-// isUUID3 is the validation function for validating if the field's value is a valid v3 UUID.
-func isUUID3(fl FieldLevel) bool {
- return uUID3Regex.MatchString(fl.Field().String())
-}
-
-// isUUID is the validation function for validating if the field's value is a valid UUID of any version.
-func isUUID(fl FieldLevel) bool {
- return uUIDRegex.MatchString(fl.Field().String())
-}
-
-// isUUID5RFC4122 is the validation function for validating if the field's value is a valid RFC4122 v5 UUID.
-func isUUID5RFC4122(fl FieldLevel) bool {
- return uUID5RFC4122Regex.MatchString(fl.Field().String())
-}
-
-// isUUID4RFC4122 is the validation function for validating if the field's value is a valid RFC4122 v4 UUID.
-func isUUID4RFC4122(fl FieldLevel) bool {
- return uUID4RFC4122Regex.MatchString(fl.Field().String())
-}
-
-// isUUID3RFC4122 is the validation function for validating if the field's value is a valid RFC4122 v3 UUID.
-func isUUID3RFC4122(fl FieldLevel) bool {
- return uUID3RFC4122Regex.MatchString(fl.Field().String())
-}
-
-// isUUIDRFC4122 is the validation function for validating if the field's value is a valid RFC4122 UUID of any version.
-func isUUIDRFC4122(fl FieldLevel) bool {
- return uUIDRFC4122Regex.MatchString(fl.Field().String())
-}
-
-// isULID is the validation function for validating if the field's value is a valid ULID.
-func isULID(fl FieldLevel) bool {
- return uLIDRegex.MatchString(fl.Field().String())
-}
-
-// isMD4 is the validation function for validating if the field's value is a valid MD4.
-func isMD4(fl FieldLevel) bool {
- return md4Regex.MatchString(fl.Field().String())
-}
-
-// isMD5 is the validation function for validating if the field's value is a valid MD5.
-func isMD5(fl FieldLevel) bool {
- return md5Regex.MatchString(fl.Field().String())
-}
-
-// isSHA256 is the validation function for validating if the field's value is a valid SHA256.
-func isSHA256(fl FieldLevel) bool {
- return sha256Regex.MatchString(fl.Field().String())
-}
-
-// isSHA384 is the validation function for validating if the field's value is a valid SHA384.
-func isSHA384(fl FieldLevel) bool {
- return sha384Regex.MatchString(fl.Field().String())
-}
-
-// isSHA512 is the validation function for validating if the field's value is a valid SHA512.
-func isSHA512(fl FieldLevel) bool {
- return sha512Regex.MatchString(fl.Field().String())
-}
-
-// isRIPEMD128 is the validation function for validating if the field's value is a valid PIPEMD128.
-func isRIPEMD128(fl FieldLevel) bool {
- return ripemd128Regex.MatchString(fl.Field().String())
-}
-
-// isRIPEMD160 is the validation function for validating if the field's value is a valid PIPEMD160.
-func isRIPEMD160(fl FieldLevel) bool {
- return ripemd160Regex.MatchString(fl.Field().String())
-}
-
-// isTIGER128 is the validation function for validating if the field's value is a valid TIGER128.
-func isTIGER128(fl FieldLevel) bool {
- return tiger128Regex.MatchString(fl.Field().String())
-}
-
-// isTIGER160 is the validation function for validating if the field's value is a valid TIGER160.
-func isTIGER160(fl FieldLevel) bool {
- return tiger160Regex.MatchString(fl.Field().String())
-}
-
-// isTIGER192 is the validation function for validating if the field's value is a valid isTIGER192.
-func isTIGER192(fl FieldLevel) bool {
- return tiger192Regex.MatchString(fl.Field().String())
-}
-
-// isISBN is the validation function for validating if the field's value is a valid v10 or v13 ISBN.
-func isISBN(fl FieldLevel) bool {
- return isISBN10(fl) || isISBN13(fl)
-}
-
-// isISBN13 is the validation function for validating if the field's value is a valid v13 ISBN.
-func isISBN13(fl FieldLevel) bool {
- s := strings.Replace(strings.Replace(fl.Field().String(), "-", "", 4), " ", "", 4)
-
- if !iSBN13Regex.MatchString(s) {
- return false
- }
-
- var checksum int32
- var i int32
-
- factor := []int32{1, 3}
-
- for i = 0; i < 12; i++ {
- checksum += factor[i%2] * int32(s[i]-'0')
- }
-
- return (int32(s[12]-'0'))-((10-(checksum%10))%10) == 0
-}
-
-// isISBN10 is the validation function for validating if the field's value is a valid v10 ISBN.
-func isISBN10(fl FieldLevel) bool {
- s := strings.Replace(strings.Replace(fl.Field().String(), "-", "", 3), " ", "", 3)
-
- if !iSBN10Regex.MatchString(s) {
- return false
- }
-
- var checksum int32
- var i int32
-
- for i = 0; i < 9; i++ {
- checksum += (i + 1) * int32(s[i]-'0')
- }
-
- if s[9] == 'X' {
- checksum += 10 * 10
- } else {
- checksum += 10 * int32(s[9]-'0')
- }
-
- return checksum%11 == 0
-}
-
-// isEthereumAddress is the validation function for validating if the field's value is a valid Ethereum address.
-func isEthereumAddress(fl FieldLevel) bool {
- address := fl.Field().String()
-
- if !ethAddressRegex.MatchString(address) {
- return false
- }
-
- if ethAddressRegexUpper.MatchString(address) || ethAddressRegexLower.MatchString(address) {
- return true
- }
-
- // Checksum validation. Reference: https://github.com/ethereum/EIPs/blob/master/EIPS/eip-55.md
- address = address[2:] // Skip "0x" prefix.
- h := sha3.NewLegacyKeccak256()
- // hash.Hash's io.Writer implementation says it never returns an error. https://golang.org/pkg/hash/#Hash
- _, _ = h.Write([]byte(strings.ToLower(address)))
- hash := hex.EncodeToString(h.Sum(nil))
-
- for i := 0; i < len(address); i++ {
- if address[i] <= '9' { // Skip 0-9 digits: they don't have upper/lower-case.
- continue
- }
- if hash[i] > '7' && address[i] >= 'a' || hash[i] <= '7' && address[i] <= 'F' {
- return false
- }
- }
-
- return true
-}
-
-// isBitcoinAddress is the validation function for validating if the field's value is a valid btc address
-func isBitcoinAddress(fl FieldLevel) bool {
- address := fl.Field().String()
-
- if !btcAddressRegex.MatchString(address) {
- return false
- }
-
- alphabet := []byte("123456789ABCDEFGHJKLMNPQRSTUVWXYZabcdefghijkmnopqrstuvwxyz")
-
- decode := [25]byte{}
-
- for _, n := range []byte(address) {
- d := bytes.IndexByte(alphabet, n)
-
- for i := 24; i >= 0; i-- {
- d += 58 * int(decode[i])
- decode[i] = byte(d % 256)
- d /= 256
- }
- }
-
- h := sha256.New()
- _, _ = h.Write(decode[:21])
- d := h.Sum([]byte{})
- h = sha256.New()
- _, _ = h.Write(d)
-
- validchecksum := [4]byte{}
- computedchecksum := [4]byte{}
-
- copy(computedchecksum[:], h.Sum(d[:0]))
- copy(validchecksum[:], decode[21:])
-
- return validchecksum == computedchecksum
-}
-
-// isBitcoinBech32Address is the validation function for validating if the field's value is a valid bech32 btc address
-func isBitcoinBech32Address(fl FieldLevel) bool {
- address := fl.Field().String()
-
- if !btcLowerAddressRegexBech32.MatchString(address) && !btcUpperAddressRegexBech32.MatchString(address) {
- return false
- }
-
- am := len(address) % 8
-
- if am == 0 || am == 3 || am == 5 {
- return false
- }
-
- address = strings.ToLower(address)
-
- alphabet := "qpzry9x8gf2tvdw0s3jn54khce6mua7l"
-
- hr := []int{3, 3, 0, 2, 3} // the human readable part will always be bc
- addr := address[3:]
- dp := make([]int, 0, len(addr))
-
- for _, c := range addr {
- dp = append(dp, strings.IndexRune(alphabet, c))
- }
-
- ver := dp[0]
-
- if ver < 0 || ver > 16 {
- return false
- }
-
- if ver == 0 {
- if len(address) != 42 && len(address) != 62 {
- return false
- }
- }
-
- values := append(hr, dp...)
-
- GEN := []int{0x3b6a57b2, 0x26508e6d, 0x1ea119fa, 0x3d4233dd, 0x2a1462b3}
-
- p := 1
-
- for _, v := range values {
- b := p >> 25
- p = (p&0x1ffffff)<<5 ^ v
-
- for i := 0; i < 5; i++ {
- if (b>>uint(i))&1 == 1 {
- p ^= GEN[i]
- }
- }
- }
-
- if p != 1 {
- return false
- }
-
- b := uint(0)
- acc := 0
- mv := (1 << 5) - 1
- var sw []int
-
- for _, v := range dp[1 : len(dp)-6] {
- acc = (acc << 5) | v
- b += 5
- for b >= 8 {
- b -= 8
- sw = append(sw, (acc>>b)&mv)
- }
- }
-
- if len(sw) < 2 || len(sw) > 40 {
- return false
- }
-
- return true
-}
-
-// excludesRune is the validation function for validating that the field's value does not contain the rune specified within the param.
-func excludesRune(fl FieldLevel) bool {
- return !containsRune(fl)
-}
-
-// excludesAll is the validation function for validating that the field's value does not contain any of the characters specified within the param.
-func excludesAll(fl FieldLevel) bool {
- return !containsAny(fl)
-}
-
-// excludes is the validation function for validating that the field's value does not contain the text specified within the param.
-func excludes(fl FieldLevel) bool {
- return !contains(fl)
-}
-
-// containsRune is the validation function for validating that the field's value contains the rune specified within the param.
-func containsRune(fl FieldLevel) bool {
- r, _ := utf8.DecodeRuneInString(fl.Param())
-
- return strings.ContainsRune(fl.Field().String(), r)
-}
-
-// containsAny is the validation function for validating that the field's value contains any of the characters specified within the param.
-func containsAny(fl FieldLevel) bool {
- return strings.ContainsAny(fl.Field().String(), fl.Param())
-}
-
-// contains is the validation function for validating that the field's value contains the text specified within the param.
-func contains(fl FieldLevel) bool {
- return strings.Contains(fl.Field().String(), fl.Param())
-}
-
-// startsWith is the validation function for validating that the field's value starts with the text specified within the param.
-func startsWith(fl FieldLevel) bool {
- return strings.HasPrefix(fl.Field().String(), fl.Param())
-}
-
-// endsWith is the validation function for validating that the field's value ends with the text specified within the param.
-func endsWith(fl FieldLevel) bool {
- return strings.HasSuffix(fl.Field().String(), fl.Param())
-}
-
-// startsNotWith is the validation function for validating that the field's value does not start with the text specified within the param.
-func startsNotWith(fl FieldLevel) bool {
- return !startsWith(fl)
-}
-
-// endsNotWith is the validation function for validating that the field's value does not end with the text specified within the param.
-func endsNotWith(fl FieldLevel) bool {
- return !endsWith(fl)
-}
-
-// fieldContains is the validation function for validating if the current field's value contains the field specified by the param's value.
-func fieldContains(fl FieldLevel) bool {
- field := fl.Field()
-
- currentField, _, ok := fl.GetStructFieldOK()
-
- if !ok {
- return false
- }
-
- return strings.Contains(field.String(), currentField.String())
-}
-
-// fieldExcludes is the validation function for validating if the current field's value excludes the field specified by the param's value.
-func fieldExcludes(fl FieldLevel) bool {
- field := fl.Field()
-
- currentField, _, ok := fl.GetStructFieldOK()
- if !ok {
- return true
- }
-
- return !strings.Contains(field.String(), currentField.String())
-}
-
-// isNeField is the validation function for validating if the current field's value is not equal to the field specified by the param's value.
-func isNeField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- currentField, currentKind, ok := fl.GetStructFieldOK()
-
- if !ok || currentKind != kind {
- return true
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() != currentField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() != currentField.Uint()
-
- case reflect.Float32, reflect.Float64:
- return field.Float() != currentField.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) != int64(currentField.Len())
-
- case reflect.Bool:
- return field.Bool() != currentField.Bool()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && currentField.Type().ConvertibleTo(timeType) {
-
- t := currentField.Interface().(time.Time)
- fieldTime := field.Interface().(time.Time)
-
- return !fieldTime.Equal(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != currentField.Type() {
- return true
- }
- }
-
- // default reflect.String:
- return field.String() != currentField.String()
-}
-
-// isNe is the validation function for validating that the field's value does not equal the provided param value.
-func isNe(fl FieldLevel) bool {
- return !isEq(fl)
-}
-
-// isLteCrossStructField is the validation function for validating if the current field's value is less than or equal to the field, within a separate struct, specified by the param's value.
-func isLteCrossStructField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- topField, topKind, ok := fl.GetStructFieldOK()
- if !ok || topKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() <= topField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() <= topField.Uint()
-
- case reflect.Float32, reflect.Float64:
- return field.Float() <= topField.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) <= int64(topField.Len())
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && topField.Type().ConvertibleTo(timeType) {
-
- fieldTime := field.Convert(timeType).Interface().(time.Time)
- topTime := topField.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.Before(topTime) || fieldTime.Equal(topTime)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != topField.Type() {
- return false
- }
- }
-
- // default reflect.String:
- return field.String() <= topField.String()
-}
-
-// isLtCrossStructField is the validation function for validating if the current field's value is less than the field, within a separate struct, specified by the param's value.
-// NOTE: This is exposed for use within your own custom functions and not intended to be called directly.
-func isLtCrossStructField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- topField, topKind, ok := fl.GetStructFieldOK()
- if !ok || topKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() < topField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() < topField.Uint()
-
- case reflect.Float32, reflect.Float64:
- return field.Float() < topField.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) < int64(topField.Len())
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && topField.Type().ConvertibleTo(timeType) {
-
- fieldTime := field.Convert(timeType).Interface().(time.Time)
- topTime := topField.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.Before(topTime)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != topField.Type() {
- return false
- }
- }
-
- // default reflect.String:
- return field.String() < topField.String()
-}
-
-// isGteCrossStructField is the validation function for validating if the current field's value is greater than or equal to the field, within a separate struct, specified by the param's value.
-func isGteCrossStructField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- topField, topKind, ok := fl.GetStructFieldOK()
- if !ok || topKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() >= topField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() >= topField.Uint()
-
- case reflect.Float32, reflect.Float64:
- return field.Float() >= topField.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) >= int64(topField.Len())
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && topField.Type().ConvertibleTo(timeType) {
-
- fieldTime := field.Convert(timeType).Interface().(time.Time)
- topTime := topField.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.After(topTime) || fieldTime.Equal(topTime)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != topField.Type() {
- return false
- }
- }
-
- // default reflect.String:
- return field.String() >= topField.String()
-}
-
-// isGtCrossStructField is the validation function for validating if the current field's value is greater than the field, within a separate struct, specified by the param's value.
-func isGtCrossStructField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- topField, topKind, ok := fl.GetStructFieldOK()
- if !ok || topKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() > topField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() > topField.Uint()
-
- case reflect.Float32, reflect.Float64:
- return field.Float() > topField.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) > int64(topField.Len())
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && topField.Type().ConvertibleTo(timeType) {
-
- fieldTime := field.Convert(timeType).Interface().(time.Time)
- topTime := topField.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.After(topTime)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != topField.Type() {
- return false
- }
- }
-
- // default reflect.String:
- return field.String() > topField.String()
-}
-
-// isNeCrossStructField is the validation function for validating that the current field's value is not equal to the field, within a separate struct, specified by the param's value.
-func isNeCrossStructField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- topField, currentKind, ok := fl.GetStructFieldOK()
- if !ok || currentKind != kind {
- return true
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return topField.Int() != field.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return topField.Uint() != field.Uint()
-
- case reflect.Float32, reflect.Float64:
- return topField.Float() != field.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(topField.Len()) != int64(field.Len())
-
- case reflect.Bool:
- return topField.Bool() != field.Bool()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && topField.Type().ConvertibleTo(timeType) {
-
- t := field.Convert(timeType).Interface().(time.Time)
- fieldTime := topField.Convert(timeType).Interface().(time.Time)
-
- return !fieldTime.Equal(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != topField.Type() {
- return true
- }
- }
-
- // default reflect.String:
- return topField.String() != field.String()
-}
-
-// isEqCrossStructField is the validation function for validating that the current field's value is equal to the field, within a separate struct, specified by the param's value.
-func isEqCrossStructField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- topField, topKind, ok := fl.GetStructFieldOK()
- if !ok || topKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return topField.Int() == field.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return topField.Uint() == field.Uint()
-
- case reflect.Float32, reflect.Float64:
- return topField.Float() == field.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(topField.Len()) == int64(field.Len())
-
- case reflect.Bool:
- return topField.Bool() == field.Bool()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && topField.Type().ConvertibleTo(timeType) {
-
- t := field.Convert(timeType).Interface().(time.Time)
- fieldTime := topField.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.Equal(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != topField.Type() {
- return false
- }
- }
-
- // default reflect.String:
- return topField.String() == field.String()
-}
-
-// isEqField is the validation function for validating if the current field's value is equal to the field specified by the param's value.
-func isEqField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- currentField, currentKind, ok := fl.GetStructFieldOK()
- if !ok || currentKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() == currentField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() == currentField.Uint()
-
- case reflect.Float32, reflect.Float64:
- return field.Float() == currentField.Float()
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) == int64(currentField.Len())
-
- case reflect.Bool:
- return field.Bool() == currentField.Bool()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && currentField.Type().ConvertibleTo(timeType) {
-
- t := currentField.Convert(timeType).Interface().(time.Time)
- fieldTime := field.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.Equal(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != currentField.Type() {
- return false
- }
- }
-
- // default reflect.String:
- return field.String() == currentField.String()
-}
-
-// isEq is the validation function for validating if the current field's value is equal to the param's value.
-func isEq(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- switch field.Kind() {
-
- case reflect.String:
- return field.String() == param
-
- case reflect.Slice, reflect.Map, reflect.Array:
- p := asInt(param)
-
- return int64(field.Len()) == p
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- p := asIntFromType(field.Type(), param)
-
- return field.Int() == p
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- p := asUint(param)
-
- return field.Uint() == p
-
- case reflect.Float32, reflect.Float64:
- p := asFloat(param)
-
- return field.Float() == p
-
- case reflect.Bool:
- p := asBool(param)
-
- return field.Bool() == p
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isPostcodeByIso3166Alpha2 validates by value which is country code in iso 3166 alpha 2
-// example: `postcode_iso3166_alpha2=US`
-func isPostcodeByIso3166Alpha2(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- reg, found := postCodeRegexDict[param]
- if !found {
- return false
- }
-
- return reg.MatchString(field.String())
-}
-
-// isPostcodeByIso3166Alpha2Field validates by field which represents for a value of country code in iso 3166 alpha 2
-// example: `postcode_iso3166_alpha2_field=CountryCode`
-func isPostcodeByIso3166Alpha2Field(fl FieldLevel) bool {
- field := fl.Field()
- params := parseOneOfParam2(fl.Param())
-
- if len(params) != 1 {
- return false
- }
-
- currentField, kind, _, found := fl.GetStructFieldOKAdvanced2(fl.Parent(), params[0])
- if !found {
- return false
- }
-
- if kind != reflect.String {
- panic(fmt.Sprintf("Bad field type %T", currentField.Interface()))
- }
-
- reg, found := postCodeRegexDict[currentField.String()]
- if !found {
- return false
- }
-
- return reg.MatchString(field.String())
-}
-
-// isBase64 is the validation function for validating if the current field's value is a valid base 64.
-func isBase64(fl FieldLevel) bool {
- return base64Regex.MatchString(fl.Field().String())
-}
-
-// isBase64URL is the validation function for validating if the current field's value is a valid base64 URL safe string.
-func isBase64URL(fl FieldLevel) bool {
- return base64URLRegex.MatchString(fl.Field().String())
-}
-
-// isURI is the validation function for validating if the current field's value is a valid URI.
-func isURI(fl FieldLevel) bool {
- field := fl.Field()
-
- switch field.Kind() {
- case reflect.String:
-
- s := field.String()
-
- // checks needed as of Go 1.6 because of change https://github.com/golang/go/commit/617c93ce740c3c3cc28cdd1a0d712be183d0b328#diff-6c2d018290e298803c0c9419d8739885L195
- // emulate browser and strip the '#' suffix prior to validation. see issue-#237
- if i := strings.Index(s, "#"); i > -1 {
- s = s[:i]
- }
-
- if len(s) == 0 {
- return false
- }
-
- _, err := url.ParseRequestURI(s)
-
- return err == nil
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isURL is the validation function for validating if the current field's value is a valid URL.
-func isURL(fl FieldLevel) bool {
- field := fl.Field()
-
- switch field.Kind() {
- case reflect.String:
-
- var i int
- s := field.String()
-
- // checks needed as of Go 1.6 because of change https://github.com/golang/go/commit/617c93ce740c3c3cc28cdd1a0d712be183d0b328#diff-6c2d018290e298803c0c9419d8739885L195
- // emulate browser and strip the '#' suffix prior to validation. see issue-#237
- if i = strings.Index(s, "#"); i > -1 {
- s = s[:i]
- }
-
- if len(s) == 0 {
- return false
- }
-
- url, err := url.ParseRequestURI(s)
-
- if err != nil || url.Scheme == "" {
- return false
- }
-
- return true
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isUrnRFC2141 is the validation function for validating if the current field's value is a valid URN as per RFC 2141.
-func isUrnRFC2141(fl FieldLevel) bool {
- field := fl.Field()
-
- switch field.Kind() {
- case reflect.String:
-
- str := field.String()
-
- _, match := urn.Parse([]byte(str))
-
- return match
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isFile is the validation function for validating if the current field's value is a valid file path.
-func isFile(fl FieldLevel) bool {
- field := fl.Field()
-
- switch field.Kind() {
- case reflect.String:
- fileInfo, err := os.Stat(field.String())
- if err != nil {
- return false
- }
-
- return !fileInfo.IsDir()
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isE164 is the validation function for validating if the current field's value is a valid e.164 formatted phone number.
-func isE164(fl FieldLevel) bool {
- return e164Regex.MatchString(fl.Field().String())
-}
-
-// isEmail is the validation function for validating if the current field's value is a valid email address.
-func isEmail(fl FieldLevel) bool {
- return emailRegex.MatchString(fl.Field().String())
-}
-
-// isHSLA is the validation function for validating if the current field's value is a valid HSLA color.
-func isHSLA(fl FieldLevel) bool {
- return hslaRegex.MatchString(fl.Field().String())
-}
-
-// isHSL is the validation function for validating if the current field's value is a valid HSL color.
-func isHSL(fl FieldLevel) bool {
- return hslRegex.MatchString(fl.Field().String())
-}
-
-// isRGBA is the validation function for validating if the current field's value is a valid RGBA color.
-func isRGBA(fl FieldLevel) bool {
- return rgbaRegex.MatchString(fl.Field().String())
-}
-
-// isRGB is the validation function for validating if the current field's value is a valid RGB color.
-func isRGB(fl FieldLevel) bool {
- return rgbRegex.MatchString(fl.Field().String())
-}
-
-// isHEXColor is the validation function for validating if the current field's value is a valid HEX color.
-func isHEXColor(fl FieldLevel) bool {
- return hexColorRegex.MatchString(fl.Field().String())
-}
-
-// isHexadecimal is the validation function for validating if the current field's value is a valid hexadecimal.
-func isHexadecimal(fl FieldLevel) bool {
- return hexadecimalRegex.MatchString(fl.Field().String())
-}
-
-// isNumber is the validation function for validating if the current field's value is a valid number.
-func isNumber(fl FieldLevel) bool {
- switch fl.Field().Kind() {
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr, reflect.Float32, reflect.Float64:
- return true
- default:
- return numberRegex.MatchString(fl.Field().String())
- }
-}
-
-// isNumeric is the validation function for validating if the current field's value is a valid numeric value.
-func isNumeric(fl FieldLevel) bool {
- switch fl.Field().Kind() {
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr, reflect.Float32, reflect.Float64:
- return true
- default:
- return numericRegex.MatchString(fl.Field().String())
- }
-}
-
-// isAlphanum is the validation function for validating if the current field's value is a valid alphanumeric value.
-func isAlphanum(fl FieldLevel) bool {
- return alphaNumericRegex.MatchString(fl.Field().String())
-}
-
-// isAlpha is the validation function for validating if the current field's value is a valid alpha value.
-func isAlpha(fl FieldLevel) bool {
- return alphaRegex.MatchString(fl.Field().String())
-}
-
-// isAlphanumUnicode is the validation function for validating if the current field's value is a valid alphanumeric unicode value.
-func isAlphanumUnicode(fl FieldLevel) bool {
- return alphaUnicodeNumericRegex.MatchString(fl.Field().String())
-}
-
-// isAlphaUnicode is the validation function for validating if the current field's value is a valid alpha unicode value.
-func isAlphaUnicode(fl FieldLevel) bool {
- return alphaUnicodeRegex.MatchString(fl.Field().String())
-}
-
-// isBoolean is the validation function for validating if the current field's value is a valid boolean value or can be safely converted to a boolean value.
-func isBoolean(fl FieldLevel) bool {
- switch fl.Field().Kind() {
- case reflect.Bool:
- return true
- default:
- _, err := strconv.ParseBool(fl.Field().String())
- return err == nil
- }
-}
-
-// isDefault is the opposite of required aka hasValue
-func isDefault(fl FieldLevel) bool {
- return !hasValue(fl)
-}
-
-// hasValue is the validation function for validating if the current field's value is not the default static value.
-func hasValue(fl FieldLevel) bool {
- field := fl.Field()
- switch field.Kind() {
- case reflect.Slice, reflect.Map, reflect.Ptr, reflect.Interface, reflect.Chan, reflect.Func:
- return !field.IsNil()
- default:
- if fl.(*validate).fldIsPointer && field.Interface() != nil {
- return true
- }
- return field.IsValid() && field.Interface() != reflect.Zero(field.Type()).Interface()
- }
-}
-
-// requireCheckField is a func for check field kind
-func requireCheckFieldKind(fl FieldLevel, param string, defaultNotFoundValue bool) bool {
- field := fl.Field()
- kind := field.Kind()
- var nullable, found bool
- if len(param) > 0 {
- field, kind, nullable, found = fl.GetStructFieldOKAdvanced2(fl.Parent(), param)
- if !found {
- return defaultNotFoundValue
- }
- }
- switch kind {
- case reflect.Invalid:
- return defaultNotFoundValue
- case reflect.Slice, reflect.Map, reflect.Ptr, reflect.Interface, reflect.Chan, reflect.Func:
- return field.IsNil()
- default:
- if nullable && field.Interface() != nil {
- return false
- }
- return field.IsValid() && field.Interface() == reflect.Zero(field.Type()).Interface()
- }
-}
-
-// requireCheckFieldValue is a func for check field value
-func requireCheckFieldValue(fl FieldLevel, param string, value string, defaultNotFoundValue bool) bool {
- field, kind, _, found := fl.GetStructFieldOKAdvanced2(fl.Parent(), param)
- if !found {
- return defaultNotFoundValue
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return field.Int() == asInt(value)
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return field.Uint() == asUint(value)
-
- case reflect.Float32, reflect.Float64:
- return field.Float() == asFloat(value)
-
- case reflect.Slice, reflect.Map, reflect.Array:
- return int64(field.Len()) == asInt(value)
-
- case reflect.Bool:
- return field.Bool() == asBool(value)
- }
-
- // default reflect.String:
- return field.String() == value
-}
-
-// requiredIf is the validation function
-// The field under validation must be present and not empty only if all the other specified fields are equal to the value following with the specified field.
-func requiredIf(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- if len(params)%2 != 0 {
- panic(fmt.Sprintf("Bad param number for required_if %s", fl.FieldName()))
- }
- for i := 0; i < len(params); i += 2 {
- if !requireCheckFieldValue(fl, params[i], params[i+1], false) {
- return true
- }
- }
- return hasValue(fl)
-}
-
-// excludedIf is the validation function
-// The field under validation must not be present or is empty only if all the other specified fields are equal to the value following with the specified field.
-func excludedIf(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- if len(params)%2 != 0 {
- panic(fmt.Sprintf("Bad param number for excluded_if %s", fl.FieldName()))
- }
-
- for i := 0; i < len(params); i += 2 {
- if !requireCheckFieldValue(fl, params[i], params[i+1], false) {
- return false
- }
- }
- return true
-}
-
-// requiredUnless is the validation function
-// The field under validation must be present and not empty only unless all the other specified fields are equal to the value following with the specified field.
-func requiredUnless(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- if len(params)%2 != 0 {
- panic(fmt.Sprintf("Bad param number for required_unless %s", fl.FieldName()))
- }
-
- for i := 0; i < len(params); i += 2 {
- if requireCheckFieldValue(fl, params[i], params[i+1], false) {
- return true
- }
- }
- return hasValue(fl)
-}
-
-// excludedUnless is the validation function
-// The field under validation must not be present or is empty unless all the other specified fields are equal to the value following with the specified field.
-func excludedUnless(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- if len(params)%2 != 0 {
- panic(fmt.Sprintf("Bad param number for excluded_unless %s", fl.FieldName()))
- }
- for i := 0; i < len(params); i += 2 {
- if !requireCheckFieldValue(fl, params[i], params[i+1], false) {
- return true
- }
- }
- return !hasValue(fl)
-}
-
-// excludedWith is the validation function
-// The field under validation must not be present or is empty if any of the other specified fields are present.
-func excludedWith(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- for _, param := range params {
- if !requireCheckFieldKind(fl, param, true) {
- return !hasValue(fl)
- }
- }
- return true
-}
-
-// requiredWith is the validation function
-// The field under validation must be present and not empty only if any of the other specified fields are present.
-func requiredWith(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- for _, param := range params {
- if !requireCheckFieldKind(fl, param, true) {
- return hasValue(fl)
- }
- }
- return true
-}
-
-// excludedWithAll is the validation function
-// The field under validation must not be present or is empty if all of the other specified fields are present.
-func excludedWithAll(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- for _, param := range params {
- if requireCheckFieldKind(fl, param, true) {
- return true
- }
- }
- return !hasValue(fl)
-}
-
-// requiredWithAll is the validation function
-// The field under validation must be present and not empty only if all of the other specified fields are present.
-func requiredWithAll(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- for _, param := range params {
- if requireCheckFieldKind(fl, param, true) {
- return true
- }
- }
- return hasValue(fl)
-}
-
-// excludedWithout is the validation function
-// The field under validation must not be present or is empty when any of the other specified fields are not present.
-func excludedWithout(fl FieldLevel) bool {
- if requireCheckFieldKind(fl, strings.TrimSpace(fl.Param()), true) {
- return !hasValue(fl)
- }
- return true
-}
-
-// requiredWithout is the validation function
-// The field under validation must be present and not empty only when any of the other specified fields are not present.
-func requiredWithout(fl FieldLevel) bool {
- if requireCheckFieldKind(fl, strings.TrimSpace(fl.Param()), true) {
- return hasValue(fl)
- }
- return true
-}
-
-// excludedWithoutAll is the validation function
-// The field under validation must not be present or is empty when all of the other specified fields are not present.
-func excludedWithoutAll(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- for _, param := range params {
- if !requireCheckFieldKind(fl, param, true) {
- return true
- }
- }
- return !hasValue(fl)
-}
-
-// requiredWithoutAll is the validation function
-// The field under validation must be present and not empty only when all of the other specified fields are not present.
-func requiredWithoutAll(fl FieldLevel) bool {
- params := parseOneOfParam2(fl.Param())
- for _, param := range params {
- if !requireCheckFieldKind(fl, param, true) {
- return true
- }
- }
- return hasValue(fl)
-}
-
-// isGteField is the validation function for validating if the current field's value is greater than or equal to the field specified by the param's value.
-func isGteField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- currentField, currentKind, ok := fl.GetStructFieldOK()
- if !ok || currentKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
-
- return field.Int() >= currentField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
-
- return field.Uint() >= currentField.Uint()
-
- case reflect.Float32, reflect.Float64:
-
- return field.Float() >= currentField.Float()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && currentField.Type().ConvertibleTo(timeType) {
-
- t := currentField.Convert(timeType).Interface().(time.Time)
- fieldTime := field.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.After(t) || fieldTime.Equal(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != currentField.Type() {
- return false
- }
- }
-
- // default reflect.String
- return len(field.String()) >= len(currentField.String())
-}
-
-// isGtField is the validation function for validating if the current field's value is greater than the field specified by the param's value.
-func isGtField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- currentField, currentKind, ok := fl.GetStructFieldOK()
- if !ok || currentKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
-
- return field.Int() > currentField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
-
- return field.Uint() > currentField.Uint()
-
- case reflect.Float32, reflect.Float64:
-
- return field.Float() > currentField.Float()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && currentField.Type().ConvertibleTo(timeType) {
-
- t := currentField.Convert(timeType).Interface().(time.Time)
- fieldTime := field.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.After(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != currentField.Type() {
- return false
- }
- }
-
- // default reflect.String
- return len(field.String()) > len(currentField.String())
-}
-
-// isGte is the validation function for validating if the current field's value is greater than or equal to the param's value.
-func isGte(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- switch field.Kind() {
-
- case reflect.String:
- p := asInt(param)
-
- return int64(utf8.RuneCountInString(field.String())) >= p
-
- case reflect.Slice, reflect.Map, reflect.Array:
- p := asInt(param)
-
- return int64(field.Len()) >= p
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- p := asIntFromType(field.Type(), param)
-
- return field.Int() >= p
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- p := asUint(param)
-
- return field.Uint() >= p
-
- case reflect.Float32, reflect.Float64:
- p := asFloat(param)
-
- return field.Float() >= p
-
- case reflect.Struct:
-
- if field.Type().ConvertibleTo(timeType) {
-
- now := time.Now().UTC()
- t := field.Convert(timeType).Interface().(time.Time)
-
- return t.After(now) || t.Equal(now)
- }
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isGt is the validation function for validating if the current field's value is greater than the param's value.
-func isGt(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- switch field.Kind() {
-
- case reflect.String:
- p := asInt(param)
-
- return int64(utf8.RuneCountInString(field.String())) > p
-
- case reflect.Slice, reflect.Map, reflect.Array:
- p := asInt(param)
-
- return int64(field.Len()) > p
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- p := asIntFromType(field.Type(), param)
-
- return field.Int() > p
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- p := asUint(param)
-
- return field.Uint() > p
-
- case reflect.Float32, reflect.Float64:
- p := asFloat(param)
-
- return field.Float() > p
- case reflect.Struct:
-
- if field.Type().ConvertibleTo(timeType) {
-
- return field.Convert(timeType).Interface().(time.Time).After(time.Now().UTC())
- }
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// hasLengthOf is the validation function for validating if the current field's value is equal to the param's value.
-func hasLengthOf(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- switch field.Kind() {
-
- case reflect.String:
- p := asInt(param)
-
- return int64(utf8.RuneCountInString(field.String())) == p
-
- case reflect.Slice, reflect.Map, reflect.Array:
- p := asInt(param)
-
- return int64(field.Len()) == p
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- p := asIntFromType(field.Type(), param)
-
- return field.Int() == p
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- p := asUint(param)
-
- return field.Uint() == p
-
- case reflect.Float32, reflect.Float64:
- p := asFloat(param)
-
- return field.Float() == p
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// hasMinOf is the validation function for validating if the current field's value is greater than or equal to the param's value.
-func hasMinOf(fl FieldLevel) bool {
- return isGte(fl)
-}
-
-// isLteField is the validation function for validating if the current field's value is less than or equal to the field specified by the param's value.
-func isLteField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- currentField, currentKind, ok := fl.GetStructFieldOK()
- if !ok || currentKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
-
- return field.Int() <= currentField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
-
- return field.Uint() <= currentField.Uint()
-
- case reflect.Float32, reflect.Float64:
-
- return field.Float() <= currentField.Float()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && currentField.Type().ConvertibleTo(timeType) {
-
- t := currentField.Convert(timeType).Interface().(time.Time)
- fieldTime := field.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.Before(t) || fieldTime.Equal(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != currentField.Type() {
- return false
- }
- }
-
- // default reflect.String
- return len(field.String()) <= len(currentField.String())
-}
-
-// isLtField is the validation function for validating if the current field's value is less than the field specified by the param's value.
-func isLtField(fl FieldLevel) bool {
- field := fl.Field()
- kind := field.Kind()
-
- currentField, currentKind, ok := fl.GetStructFieldOK()
- if !ok || currentKind != kind {
- return false
- }
-
- switch kind {
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
-
- return field.Int() < currentField.Int()
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
-
- return field.Uint() < currentField.Uint()
-
- case reflect.Float32, reflect.Float64:
-
- return field.Float() < currentField.Float()
-
- case reflect.Struct:
-
- fieldType := field.Type()
-
- if fieldType.ConvertibleTo(timeType) && currentField.Type().ConvertibleTo(timeType) {
-
- t := currentField.Convert(timeType).Interface().(time.Time)
- fieldTime := field.Convert(timeType).Interface().(time.Time)
-
- return fieldTime.Before(t)
- }
-
- // Not Same underlying type i.e. struct and time
- if fieldType != currentField.Type() {
- return false
- }
- }
-
- // default reflect.String
- return len(field.String()) < len(currentField.String())
-}
-
-// isLte is the validation function for validating if the current field's value is less than or equal to the param's value.
-func isLte(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- switch field.Kind() {
-
- case reflect.String:
- p := asInt(param)
-
- return int64(utf8.RuneCountInString(field.String())) <= p
-
- case reflect.Slice, reflect.Map, reflect.Array:
- p := asInt(param)
-
- return int64(field.Len()) <= p
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- p := asIntFromType(field.Type(), param)
-
- return field.Int() <= p
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- p := asUint(param)
-
- return field.Uint() <= p
-
- case reflect.Float32, reflect.Float64:
- p := asFloat(param)
-
- return field.Float() <= p
-
- case reflect.Struct:
-
- if field.Type().ConvertibleTo(timeType) {
-
- now := time.Now().UTC()
- t := field.Convert(timeType).Interface().(time.Time)
-
- return t.Before(now) || t.Equal(now)
- }
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isLt is the validation function for validating if the current field's value is less than the param's value.
-func isLt(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- switch field.Kind() {
-
- case reflect.String:
- p := asInt(param)
-
- return int64(utf8.RuneCountInString(field.String())) < p
-
- case reflect.Slice, reflect.Map, reflect.Array:
- p := asInt(param)
-
- return int64(field.Len()) < p
-
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- p := asIntFromType(field.Type(), param)
-
- return field.Int() < p
-
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- p := asUint(param)
-
- return field.Uint() < p
-
- case reflect.Float32, reflect.Float64:
- p := asFloat(param)
-
- return field.Float() < p
-
- case reflect.Struct:
-
- if field.Type().ConvertibleTo(timeType) {
-
- return field.Convert(timeType).Interface().(time.Time).Before(time.Now().UTC())
- }
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// hasMaxOf is the validation function for validating if the current field's value is less than or equal to the param's value.
-func hasMaxOf(fl FieldLevel) bool {
- return isLte(fl)
-}
-
-// isTCP4AddrResolvable is the validation function for validating if the field's value is a resolvable tcp4 address.
-func isTCP4AddrResolvable(fl FieldLevel) bool {
- if !isIP4Addr(fl) {
- return false
- }
-
- _, err := net.ResolveTCPAddr("tcp4", fl.Field().String())
- return err == nil
-}
-
-// isTCP6AddrResolvable is the validation function for validating if the field's value is a resolvable tcp6 address.
-func isTCP6AddrResolvable(fl FieldLevel) bool {
- if !isIP6Addr(fl) {
- return false
- }
-
- _, err := net.ResolveTCPAddr("tcp6", fl.Field().String())
-
- return err == nil
-}
-
-// isTCPAddrResolvable is the validation function for validating if the field's value is a resolvable tcp address.
-func isTCPAddrResolvable(fl FieldLevel) bool {
- if !isIP4Addr(fl) && !isIP6Addr(fl) {
- return false
- }
-
- _, err := net.ResolveTCPAddr("tcp", fl.Field().String())
-
- return err == nil
-}
-
-// isUDP4AddrResolvable is the validation function for validating if the field's value is a resolvable udp4 address.
-func isUDP4AddrResolvable(fl FieldLevel) bool {
- if !isIP4Addr(fl) {
- return false
- }
-
- _, err := net.ResolveUDPAddr("udp4", fl.Field().String())
-
- return err == nil
-}
-
-// isUDP6AddrResolvable is the validation function for validating if the field's value is a resolvable udp6 address.
-func isUDP6AddrResolvable(fl FieldLevel) bool {
- if !isIP6Addr(fl) {
- return false
- }
-
- _, err := net.ResolveUDPAddr("udp6", fl.Field().String())
-
- return err == nil
-}
-
-// isUDPAddrResolvable is the validation function for validating if the field's value is a resolvable udp address.
-func isUDPAddrResolvable(fl FieldLevel) bool {
- if !isIP4Addr(fl) && !isIP6Addr(fl) {
- return false
- }
-
- _, err := net.ResolveUDPAddr("udp", fl.Field().String())
-
- return err == nil
-}
-
-// isIP4AddrResolvable is the validation function for validating if the field's value is a resolvable ip4 address.
-func isIP4AddrResolvable(fl FieldLevel) bool {
- if !isIPv4(fl) {
- return false
- }
-
- _, err := net.ResolveIPAddr("ip4", fl.Field().String())
-
- return err == nil
-}
-
-// isIP6AddrResolvable is the validation function for validating if the field's value is a resolvable ip6 address.
-func isIP6AddrResolvable(fl FieldLevel) bool {
- if !isIPv6(fl) {
- return false
- }
-
- _, err := net.ResolveIPAddr("ip6", fl.Field().String())
-
- return err == nil
-}
-
-// isIPAddrResolvable is the validation function for validating if the field's value is a resolvable ip address.
-func isIPAddrResolvable(fl FieldLevel) bool {
- if !isIP(fl) {
- return false
- }
-
- _, err := net.ResolveIPAddr("ip", fl.Field().String())
-
- return err == nil
-}
-
-// isUnixAddrResolvable is the validation function for validating if the field's value is a resolvable unix address.
-func isUnixAddrResolvable(fl FieldLevel) bool {
- _, err := net.ResolveUnixAddr("unix", fl.Field().String())
-
- return err == nil
-}
-
-func isIP4Addr(fl FieldLevel) bool {
- val := fl.Field().String()
-
- if idx := strings.LastIndex(val, ":"); idx != -1 {
- val = val[0:idx]
- }
-
- ip := net.ParseIP(val)
-
- return ip != nil && ip.To4() != nil
-}
-
-func isIP6Addr(fl FieldLevel) bool {
- val := fl.Field().String()
-
- if idx := strings.LastIndex(val, ":"); idx != -1 {
- if idx != 0 && val[idx-1:idx] == "]" {
- val = val[1 : idx-1]
- }
- }
-
- ip := net.ParseIP(val)
-
- return ip != nil && ip.To4() == nil
-}
-
-func isHostnameRFC952(fl FieldLevel) bool {
- return hostnameRegexRFC952.MatchString(fl.Field().String())
-}
-
-func isHostnameRFC1123(fl FieldLevel) bool {
- return hostnameRegexRFC1123.MatchString(fl.Field().String())
-}
-
-func isFQDN(fl FieldLevel) bool {
- val := fl.Field().String()
-
- if val == "" {
- return false
- }
-
- return fqdnRegexRFC1123.MatchString(val)
-}
-
-// isDir is the validation function for validating if the current field's value is a valid directory.
-func isDir(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Kind() == reflect.String {
- fileInfo, err := os.Stat(field.String())
- if err != nil {
- return false
- }
-
- return fileInfo.IsDir()
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isJSON is the validation function for validating if the current field's value is a valid json string.
-func isJSON(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Kind() == reflect.String {
- val := field.String()
- return json.Valid([]byte(val))
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isJWT is the validation function for validating if the current field's value is a valid JWT string.
-func isJWT(fl FieldLevel) bool {
- return jWTRegex.MatchString(fl.Field().String())
-}
-
-// isHostnamePort validates a : combination for fields typically used for socket address.
-func isHostnamePort(fl FieldLevel) bool {
- val := fl.Field().String()
- host, port, err := net.SplitHostPort(val)
- if err != nil {
- return false
- }
- // Port must be a iny <= 65535.
- if portNum, err := strconv.ParseInt(port, 10, 32); err != nil || portNum > 65535 || portNum < 1 {
- return false
- }
-
- // If host is specified, it should match a DNS name
- if host != "" {
- return hostnameRegexRFC1123.MatchString(host)
- }
- return true
-}
-
-// isLowercase is the validation function for validating if the current field's value is a lowercase string.
-func isLowercase(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Kind() == reflect.String {
- if field.String() == "" {
- return false
- }
- return field.String() == strings.ToLower(field.String())
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isUppercase is the validation function for validating if the current field's value is an uppercase string.
-func isUppercase(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Kind() == reflect.String {
- if field.String() == "" {
- return false
- }
- return field.String() == strings.ToUpper(field.String())
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isDatetime is the validation function for validating if the current field's value is a valid datetime string.
-func isDatetime(fl FieldLevel) bool {
- field := fl.Field()
- param := fl.Param()
-
- if field.Kind() == reflect.String {
- _, err := time.Parse(param, field.String())
-
- return err == nil
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isTimeZone is the validation function for validating if the current field's value is a valid time zone string.
-func isTimeZone(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Kind() == reflect.String {
- // empty value is converted to UTC by time.LoadLocation but disallow it as it is not a valid time zone name
- if field.String() == "" {
- return false
- }
-
- // Local value is converted to the current system time zone by time.LoadLocation but disallow it as it is not a valid time zone name
- if strings.ToLower(field.String()) == "local" {
- return false
- }
-
- _, err := time.LoadLocation(field.String())
- return err == nil
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isIso3166Alpha2 is the validation function for validating if the current field's value is a valid iso3166-1 alpha-2 country code.
-func isIso3166Alpha2(fl FieldLevel) bool {
- val := fl.Field().String()
- return iso3166_1_alpha2[val]
-}
-
-// isIso3166Alpha2 is the validation function for validating if the current field's value is a valid iso3166-1 alpha-3 country code.
-func isIso3166Alpha3(fl FieldLevel) bool {
- val := fl.Field().String()
- return iso3166_1_alpha3[val]
-}
-
-// isIso3166Alpha2 is the validation function for validating if the current field's value is a valid iso3166-1 alpha-numeric country code.
-func isIso3166AlphaNumeric(fl FieldLevel) bool {
- field := fl.Field()
-
- var code int
- switch field.Kind() {
- case reflect.String:
- i, err := strconv.Atoi(field.String())
- if err != nil {
- return false
- }
- code = i % 1000
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- code = int(field.Int() % 1000)
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64:
- code = int(field.Uint() % 1000)
- default:
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
- }
- return iso3166_1_alpha_numeric[code]
-}
-
-// isIso31662 is the validation function for validating if the current field's value is a valid iso3166-2 code.
-func isIso31662(fl FieldLevel) bool {
- val := fl.Field().String()
- return iso3166_2[val]
-}
-
-// isIso4217 is the validation function for validating if the current field's value is a valid iso4217 currency code.
-func isIso4217(fl FieldLevel) bool {
- val := fl.Field().String()
- return iso4217[val]
-}
-
-// isIso4217Numeric is the validation function for validating if the current field's value is a valid iso4217 numeric currency code.
-func isIso4217Numeric(fl FieldLevel) bool {
- field := fl.Field()
-
- var code int
- switch field.Kind() {
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- code = int(field.Int())
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64:
- code = int(field.Uint())
- default:
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
- }
- return iso4217_numeric[code]
-}
-
-// isBCP47LanguageTag is the validation function for validating if the current field's value is a valid BCP 47 language tag, as parsed by language.Parse
-func isBCP47LanguageTag(fl FieldLevel) bool {
- field := fl.Field()
-
- if field.Kind() == reflect.String {
- _, err := language.Parse(field.String())
- return err == nil
- }
-
- panic(fmt.Sprintf("Bad field type %T", field.Interface()))
-}
-
-// isIsoBicFormat is the validation function for validating if the current field's value is a valid Business Identifier Code (SWIFT code), defined in ISO 9362
-func isIsoBicFormat(fl FieldLevel) bool {
- bicString := fl.Field().String()
-
- return bicRegex.MatchString(bicString)
-}
-
-// isSemverFormat is the validation function for validating if the current field's value is a valid semver version, defined in Semantic Versioning 2.0.0
-func isSemverFormat(fl FieldLevel) bool {
- semverString := fl.Field().String()
-
- return semverRegex.MatchString(semverString)
-}
-
-// isDnsRFC1035LabelFormat is the validation function
-// for validating if the current field's value is
-// a valid dns RFC 1035 label, defined in RFC 1035.
-func isDnsRFC1035LabelFormat(fl FieldLevel) bool {
- val := fl.Field().String()
- return dnsRegexRFC1035Label.MatchString(val)
-}
-
-// isCreditCard is the validation function for validating if the current field's value is a valid credit card number
-func isCreditCard(fl FieldLevel) bool {
- val := fl.Field().String()
- var creditCard bytes.Buffer
- segments := strings.Split(val, " ")
- for _, segment := range segments {
- if len(segment) < 3 {
- return false
- }
- creditCard.WriteString(segment)
- }
-
- ccDigits := strings.Split(creditCard.String(), "")
- size := len(ccDigits)
- if size < 12 || size > 19 {
- return false
- }
-
- sum := 0
- for i, digit := range ccDigits {
- value, err := strconv.Atoi(digit)
- if err != nil {
- return false
- }
- if size%2 == 0 && i%2 == 0 || size%2 == 1 && i%2 == 1 {
- v := value * 2
- if v >= 10 {
- sum += 1 + (v % 10)
- } else {
- sum += v
- }
- } else {
- sum += value
- }
- }
- return (sum % 10) == 0
-}
diff --git a/vendor/github.com/go-playground/validator/v10/cache.go b/vendor/github.com/go-playground/validator/v10/cache.go
deleted file mode 100644
index 7b84c91f..00000000
--- a/vendor/github.com/go-playground/validator/v10/cache.go
+++ /dev/null
@@ -1,327 +0,0 @@
-package validator
-
-import (
- "fmt"
- "reflect"
- "strings"
- "sync"
- "sync/atomic"
-)
-
-type tagType uint8
-
-const (
- typeDefault tagType = iota
- typeOmitEmpty
- typeIsDefault
- typeNoStructLevel
- typeStructOnly
- typeDive
- typeOr
- typeKeys
- typeEndKeys
-)
-
-const (
- invalidValidation = "Invalid validation tag on field '%s'"
- undefinedValidation = "Undefined validation function '%s' on field '%s'"
- keysTagNotDefined = "'" + endKeysTag + "' tag encountered without a corresponding '" + keysTag + "' tag"
-)
-
-type structCache struct {
- lock sync.Mutex
- m atomic.Value // map[reflect.Type]*cStruct
-}
-
-func (sc *structCache) Get(key reflect.Type) (c *cStruct, found bool) {
- c, found = sc.m.Load().(map[reflect.Type]*cStruct)[key]
- return
-}
-
-func (sc *structCache) Set(key reflect.Type, value *cStruct) {
- m := sc.m.Load().(map[reflect.Type]*cStruct)
- nm := make(map[reflect.Type]*cStruct, len(m)+1)
- for k, v := range m {
- nm[k] = v
- }
- nm[key] = value
- sc.m.Store(nm)
-}
-
-type tagCache struct {
- lock sync.Mutex
- m atomic.Value // map[string]*cTag
-}
-
-func (tc *tagCache) Get(key string) (c *cTag, found bool) {
- c, found = tc.m.Load().(map[string]*cTag)[key]
- return
-}
-
-func (tc *tagCache) Set(key string, value *cTag) {
- m := tc.m.Load().(map[string]*cTag)
- nm := make(map[string]*cTag, len(m)+1)
- for k, v := range m {
- nm[k] = v
- }
- nm[key] = value
- tc.m.Store(nm)
-}
-
-type cStruct struct {
- name string
- fields []*cField
- fn StructLevelFuncCtx
-}
-
-type cField struct {
- idx int
- name string
- altName string
- namesEqual bool
- cTags *cTag
-}
-
-type cTag struct {
- tag string
- aliasTag string
- actualAliasTag string
- param string
- keys *cTag // only populated when using tag's 'keys' and 'endkeys' for map key validation
- next *cTag
- fn FuncCtx
- typeof tagType
- hasTag bool
- hasAlias bool
- hasParam bool // true if parameter used eg. eq= where the equal sign has been set
- isBlockEnd bool // indicates the current tag represents the last validation in the block
- runValidationWhenNil bool
-}
-
-func (v *Validate) extractStructCache(current reflect.Value, sName string) *cStruct {
- v.structCache.lock.Lock()
- defer v.structCache.lock.Unlock() // leave as defer! because if inner panics, it will never get unlocked otherwise!
-
- typ := current.Type()
-
- // could have been multiple trying to access, but once first is done this ensures struct
- // isn't parsed again.
- cs, ok := v.structCache.Get(typ)
- if ok {
- return cs
- }
-
- cs = &cStruct{name: sName, fields: make([]*cField, 0), fn: v.structLevelFuncs[typ]}
-
- numFields := current.NumField()
- rules := v.rules[typ]
-
- var ctag *cTag
- var fld reflect.StructField
- var tag string
- var customName string
-
- for i := 0; i < numFields; i++ {
-
- fld = typ.Field(i)
-
- if !fld.Anonymous && len(fld.PkgPath) > 0 {
- continue
- }
-
- if rtag, ok := rules[fld.Name]; ok {
- tag = rtag
- } else {
- tag = fld.Tag.Get(v.tagName)
- }
-
- if tag == skipValidationTag {
- continue
- }
-
- customName = fld.Name
-
- if v.hasTagNameFunc {
- name := v.tagNameFunc(fld)
- if len(name) > 0 {
- customName = name
- }
- }
-
- // NOTE: cannot use shared tag cache, because tags may be equal, but things like alias may be different
- // and so only struct level caching can be used instead of combined with Field tag caching
-
- if len(tag) > 0 {
- ctag, _ = v.parseFieldTagsRecursive(tag, fld.Name, "", false)
- } else {
- // even if field doesn't have validations need cTag for traversing to potential inner/nested
- // elements of the field.
- ctag = new(cTag)
- }
-
- cs.fields = append(cs.fields, &cField{
- idx: i,
- name: fld.Name,
- altName: customName,
- cTags: ctag,
- namesEqual: fld.Name == customName,
- })
- }
- v.structCache.Set(typ, cs)
- return cs
-}
-
-func (v *Validate) parseFieldTagsRecursive(tag string, fieldName string, alias string, hasAlias bool) (firstCtag *cTag, current *cTag) {
- var t string
- noAlias := len(alias) == 0
- tags := strings.Split(tag, tagSeparator)
-
- for i := 0; i < len(tags); i++ {
- t = tags[i]
- if noAlias {
- alias = t
- }
-
- // check map for alias and process new tags, otherwise process as usual
- if tagsVal, found := v.aliases[t]; found {
- if i == 0 {
- firstCtag, current = v.parseFieldTagsRecursive(tagsVal, fieldName, t, true)
- } else {
- next, curr := v.parseFieldTagsRecursive(tagsVal, fieldName, t, true)
- current.next, current = next, curr
-
- }
- continue
- }
-
- var prevTag tagType
-
- if i == 0 {
- current = &cTag{aliasTag: alias, hasAlias: hasAlias, hasTag: true, typeof: typeDefault}
- firstCtag = current
- } else {
- prevTag = current.typeof
- current.next = &cTag{aliasTag: alias, hasAlias: hasAlias, hasTag: true}
- current = current.next
- }
-
- switch t {
- case diveTag:
- current.typeof = typeDive
- continue
-
- case keysTag:
- current.typeof = typeKeys
-
- if i == 0 || prevTag != typeDive {
- panic(fmt.Sprintf("'%s' tag must be immediately preceded by the '%s' tag", keysTag, diveTag))
- }
-
- current.typeof = typeKeys
-
- // need to pass along only keys tag
- // need to increment i to skip over the keys tags
- b := make([]byte, 0, 64)
-
- i++
-
- for ; i < len(tags); i++ {
-
- b = append(b, tags[i]...)
- b = append(b, ',')
-
- if tags[i] == endKeysTag {
- break
- }
- }
-
- current.keys, _ = v.parseFieldTagsRecursive(string(b[:len(b)-1]), fieldName, "", false)
- continue
-
- case endKeysTag:
- current.typeof = typeEndKeys
-
- // if there are more in tags then there was no keysTag defined
- // and an error should be thrown
- if i != len(tags)-1 {
- panic(keysTagNotDefined)
- }
- return
-
- case omitempty:
- current.typeof = typeOmitEmpty
- continue
-
- case structOnlyTag:
- current.typeof = typeStructOnly
- continue
-
- case noStructLevelTag:
- current.typeof = typeNoStructLevel
- continue
-
- default:
- if t == isdefault {
- current.typeof = typeIsDefault
- }
- // if a pipe character is needed within the param you must use the utf8Pipe representation "0x7C"
- orVals := strings.Split(t, orSeparator)
-
- for j := 0; j < len(orVals); j++ {
- vals := strings.SplitN(orVals[j], tagKeySeparator, 2)
- if noAlias {
- alias = vals[0]
- current.aliasTag = alias
- } else {
- current.actualAliasTag = t
- }
-
- if j > 0 {
- current.next = &cTag{aliasTag: alias, actualAliasTag: current.actualAliasTag, hasAlias: hasAlias, hasTag: true}
- current = current.next
- }
- current.hasParam = len(vals) > 1
-
- current.tag = vals[0]
- if len(current.tag) == 0 {
- panic(strings.TrimSpace(fmt.Sprintf(invalidValidation, fieldName)))
- }
-
- if wrapper, ok := v.validations[current.tag]; ok {
- current.fn = wrapper.fn
- current.runValidationWhenNil = wrapper.runValidatinOnNil
- } else {
- panic(strings.TrimSpace(fmt.Sprintf(undefinedValidation, current.tag, fieldName)))
- }
-
- if len(orVals) > 1 {
- current.typeof = typeOr
- }
-
- if len(vals) > 1 {
- current.param = strings.Replace(strings.Replace(vals[1], utf8HexComma, ",", -1), utf8Pipe, "|", -1)
- }
- }
- current.isBlockEnd = true
- }
- }
- return
-}
-
-func (v *Validate) fetchCacheTag(tag string) *cTag {
- // find cached tag
- ctag, found := v.tagCache.Get(tag)
- if !found {
- v.tagCache.lock.Lock()
- defer v.tagCache.lock.Unlock()
-
- // could have been multiple trying to access, but once first is done this ensures tag
- // isn't parsed again.
- ctag, found = v.tagCache.Get(tag)
- if !found {
- ctag, _ = v.parseFieldTagsRecursive(tag, "", "", false)
- v.tagCache.Set(tag, ctag)
- }
- }
- return ctag
-}
diff --git a/vendor/github.com/go-playground/validator/v10/country_codes.go b/vendor/github.com/go-playground/validator/v10/country_codes.go
deleted file mode 100644
index 0d9eda03..00000000
--- a/vendor/github.com/go-playground/validator/v10/country_codes.go
+++ /dev/null
@@ -1,1132 +0,0 @@
-package validator
-
-var iso3166_1_alpha2 = map[string]bool{
- // see: https://www.iso.org/iso-3166-country-codes.html
- "AF": true, "AX": true, "AL": true, "DZ": true, "AS": true,
- "AD": true, "AO": true, "AI": true, "AQ": true, "AG": true,
- "AR": true, "AM": true, "AW": true, "AU": true, "AT": true,
- "AZ": true, "BS": true, "BH": true, "BD": true, "BB": true,
- "BY": true, "BE": true, "BZ": true, "BJ": true, "BM": true,
- "BT": true, "BO": true, "BQ": true, "BA": true, "BW": true,
- "BV": true, "BR": true, "IO": true, "BN": true, "BG": true,
- "BF": true, "BI": true, "KH": true, "CM": true, "CA": true,
- "CV": true, "KY": true, "CF": true, "TD": true, "CL": true,
- "CN": true, "CX": true, "CC": true, "CO": true, "KM": true,
- "CG": true, "CD": true, "CK": true, "CR": true, "CI": true,
- "HR": true, "CU": true, "CW": true, "CY": true, "CZ": true,
- "DK": true, "DJ": true, "DM": true, "DO": true, "EC": true,
- "EG": true, "SV": true, "GQ": true, "ER": true, "EE": true,
- "ET": true, "FK": true, "FO": true, "FJ": true, "FI": true,
- "FR": true, "GF": true, "PF": true, "TF": true, "GA": true,
- "GM": true, "GE": true, "DE": true, "GH": true, "GI": true,
- "GR": true, "GL": true, "GD": true, "GP": true, "GU": true,
- "GT": true, "GG": true, "GN": true, "GW": true, "GY": true,
- "HT": true, "HM": true, "VA": true, "HN": true, "HK": true,
- "HU": true, "IS": true, "IN": true, "ID": true, "IR": true,
- "IQ": true, "IE": true, "IM": true, "IL": true, "IT": true,
- "JM": true, "JP": true, "JE": true, "JO": true, "KZ": true,
- "KE": true, "KI": true, "KP": true, "KR": true, "KW": true,
- "KG": true, "LA": true, "LV": true, "LB": true, "LS": true,
- "LR": true, "LY": true, "LI": true, "LT": true, "LU": true,
- "MO": true, "MK": true, "MG": true, "MW": true, "MY": true,
- "MV": true, "ML": true, "MT": true, "MH": true, "MQ": true,
- "MR": true, "MU": true, "YT": true, "MX": true, "FM": true,
- "MD": true, "MC": true, "MN": true, "ME": true, "MS": true,
- "MA": true, "MZ": true, "MM": true, "NA": true, "NR": true,
- "NP": true, "NL": true, "NC": true, "NZ": true, "NI": true,
- "NE": true, "NG": true, "NU": true, "NF": true, "MP": true,
- "NO": true, "OM": true, "PK": true, "PW": true, "PS": true,
- "PA": true, "PG": true, "PY": true, "PE": true, "PH": true,
- "PN": true, "PL": true, "PT": true, "PR": true, "QA": true,
- "RE": true, "RO": true, "RU": true, "RW": true, "BL": true,
- "SH": true, "KN": true, "LC": true, "MF": true, "PM": true,
- "VC": true, "WS": true, "SM": true, "ST": true, "SA": true,
- "SN": true, "RS": true, "SC": true, "SL": true, "SG": true,
- "SX": true, "SK": true, "SI": true, "SB": true, "SO": true,
- "ZA": true, "GS": true, "SS": true, "ES": true, "LK": true,
- "SD": true, "SR": true, "SJ": true, "SZ": true, "SE": true,
- "CH": true, "SY": true, "TW": true, "TJ": true, "TZ": true,
- "TH": true, "TL": true, "TG": true, "TK": true, "TO": true,
- "TT": true, "TN": true, "TR": true, "TM": true, "TC": true,
- "TV": true, "UG": true, "UA": true, "AE": true, "GB": true,
- "US": true, "UM": true, "UY": true, "UZ": true, "VU": true,
- "VE": true, "VN": true, "VG": true, "VI": true, "WF": true,
- "EH": true, "YE": true, "ZM": true, "ZW": true,
-}
-
-var iso3166_1_alpha3 = map[string]bool{
- // see: https://www.iso.org/iso-3166-country-codes.html
- "AFG": true, "ALB": true, "DZA": true, "ASM": true, "AND": true,
- "AGO": true, "AIA": true, "ATA": true, "ATG": true, "ARG": true,
- "ARM": true, "ABW": true, "AUS": true, "AUT": true, "AZE": true,
- "BHS": true, "BHR": true, "BGD": true, "BRB": true, "BLR": true,
- "BEL": true, "BLZ": true, "BEN": true, "BMU": true, "BTN": true,
- "BOL": true, "BES": true, "BIH": true, "BWA": true, "BVT": true,
- "BRA": true, "IOT": true, "BRN": true, "BGR": true, "BFA": true,
- "BDI": true, "CPV": true, "KHM": true, "CMR": true, "CAN": true,
- "CYM": true, "CAF": true, "TCD": true, "CHL": true, "CHN": true,
- "CXR": true, "CCK": true, "COL": true, "COM": true, "COD": true,
- "COG": true, "COK": true, "CRI": true, "HRV": true, "CUB": true,
- "CUW": true, "CYP": true, "CZE": true, "CIV": true, "DNK": true,
- "DJI": true, "DMA": true, "DOM": true, "ECU": true, "EGY": true,
- "SLV": true, "GNQ": true, "ERI": true, "EST": true, "SWZ": true,
- "ETH": true, "FLK": true, "FRO": true, "FJI": true, "FIN": true,
- "FRA": true, "GUF": true, "PYF": true, "ATF": true, "GAB": true,
- "GMB": true, "GEO": true, "DEU": true, "GHA": true, "GIB": true,
- "GRC": true, "GRL": true, "GRD": true, "GLP": true, "GUM": true,
- "GTM": true, "GGY": true, "GIN": true, "GNB": true, "GUY": true,
- "HTI": true, "HMD": true, "VAT": true, "HND": true, "HKG": true,
- "HUN": true, "ISL": true, "IND": true, "IDN": true, "IRN": true,
- "IRQ": true, "IRL": true, "IMN": true, "ISR": true, "ITA": true,
- "JAM": true, "JPN": true, "JEY": true, "JOR": true, "KAZ": true,
- "KEN": true, "KIR": true, "PRK": true, "KOR": true, "KWT": true,
- "KGZ": true, "LAO": true, "LVA": true, "LBN": true, "LSO": true,
- "LBR": true, "LBY": true, "LIE": true, "LTU": true, "LUX": true,
- "MAC": true, "MDG": true, "MWI": true, "MYS": true, "MDV": true,
- "MLI": true, "MLT": true, "MHL": true, "MTQ": true, "MRT": true,
- "MUS": true, "MYT": true, "MEX": true, "FSM": true, "MDA": true,
- "MCO": true, "MNG": true, "MNE": true, "MSR": true, "MAR": true,
- "MOZ": true, "MMR": true, "NAM": true, "NRU": true, "NPL": true,
- "NLD": true, "NCL": true, "NZL": true, "NIC": true, "NER": true,
- "NGA": true, "NIU": true, "NFK": true, "MKD": true, "MNP": true,
- "NOR": true, "OMN": true, "PAK": true, "PLW": true, "PSE": true,
- "PAN": true, "PNG": true, "PRY": true, "PER": true, "PHL": true,
- "PCN": true, "POL": true, "PRT": true, "PRI": true, "QAT": true,
- "ROU": true, "RUS": true, "RWA": true, "REU": true, "BLM": true,
- "SHN": true, "KNA": true, "LCA": true, "MAF": true, "SPM": true,
- "VCT": true, "WSM": true, "SMR": true, "STP": true, "SAU": true,
- "SEN": true, "SRB": true, "SYC": true, "SLE": true, "SGP": true,
- "SXM": true, "SVK": true, "SVN": true, "SLB": true, "SOM": true,
- "ZAF": true, "SGS": true, "SSD": true, "ESP": true, "LKA": true,
- "SDN": true, "SUR": true, "SJM": true, "SWE": true, "CHE": true,
- "SYR": true, "TWN": true, "TJK": true, "TZA": true, "THA": true,
- "TLS": true, "TGO": true, "TKL": true, "TON": true, "TTO": true,
- "TUN": true, "TUR": true, "TKM": true, "TCA": true, "TUV": true,
- "UGA": true, "UKR": true, "ARE": true, "GBR": true, "UMI": true,
- "USA": true, "URY": true, "UZB": true, "VUT": true, "VEN": true,
- "VNM": true, "VGB": true, "VIR": true, "WLF": true, "ESH": true,
- "YEM": true, "ZMB": true, "ZWE": true, "ALA": true,
-}
-var iso3166_1_alpha_numeric = map[int]bool{
- // see: https://www.iso.org/iso-3166-country-codes.html
- 4: true, 8: true, 12: true, 16: true, 20: true,
- 24: true, 660: true, 10: true, 28: true, 32: true,
- 51: true, 533: true, 36: true, 40: true, 31: true,
- 44: true, 48: true, 50: true, 52: true, 112: true,
- 56: true, 84: true, 204: true, 60: true, 64: true,
- 68: true, 535: true, 70: true, 72: true, 74: true,
- 76: true, 86: true, 96: true, 100: true, 854: true,
- 108: true, 132: true, 116: true, 120: true, 124: true,
- 136: true, 140: true, 148: true, 152: true, 156: true,
- 162: true, 166: true, 170: true, 174: true, 180: true,
- 178: true, 184: true, 188: true, 191: true, 192: true,
- 531: true, 196: true, 203: true, 384: true, 208: true,
- 262: true, 212: true, 214: true, 218: true, 818: true,
- 222: true, 226: true, 232: true, 233: true, 748: true,
- 231: true, 238: true, 234: true, 242: true, 246: true,
- 250: true, 254: true, 258: true, 260: true, 266: true,
- 270: true, 268: true, 276: true, 288: true, 292: true,
- 300: true, 304: true, 308: true, 312: true, 316: true,
- 320: true, 831: true, 324: true, 624: true, 328: true,
- 332: true, 334: true, 336: true, 340: true, 344: true,
- 348: true, 352: true, 356: true, 360: true, 364: true,
- 368: true, 372: true, 833: true, 376: true, 380: true,
- 388: true, 392: true, 832: true, 400: true, 398: true,
- 404: true, 296: true, 408: true, 410: true, 414: true,
- 417: true, 418: true, 428: true, 422: true, 426: true,
- 430: true, 434: true, 438: true, 440: true, 442: true,
- 446: true, 450: true, 454: true, 458: true, 462: true,
- 466: true, 470: true, 584: true, 474: true, 478: true,
- 480: true, 175: true, 484: true, 583: true, 498: true,
- 492: true, 496: true, 499: true, 500: true, 504: true,
- 508: true, 104: true, 516: true, 520: true, 524: true,
- 528: true, 540: true, 554: true, 558: true, 562: true,
- 566: true, 570: true, 574: true, 807: true, 580: true,
- 578: true, 512: true, 586: true, 585: true, 275: true,
- 591: true, 598: true, 600: true, 604: true, 608: true,
- 612: true, 616: true, 620: true, 630: true, 634: true,
- 642: true, 643: true, 646: true, 638: true, 652: true,
- 654: true, 659: true, 662: true, 663: true, 666: true,
- 670: true, 882: true, 674: true, 678: true, 682: true,
- 686: true, 688: true, 690: true, 694: true, 702: true,
- 534: true, 703: true, 705: true, 90: true, 706: true,
- 710: true, 239: true, 728: true, 724: true, 144: true,
- 729: true, 740: true, 744: true, 752: true, 756: true,
- 760: true, 158: true, 762: true, 834: true, 764: true,
- 626: true, 768: true, 772: true, 776: true, 780: true,
- 788: true, 792: true, 795: true, 796: true, 798: true,
- 800: true, 804: true, 784: true, 826: true, 581: true,
- 840: true, 858: true, 860: true, 548: true, 862: true,
- 704: true, 92: true, 850: true, 876: true, 732: true,
- 887: true, 894: true, 716: true, 248: true,
-}
-
-var iso3166_2 = map[string]bool{
- "AD-02" : true, "AD-03" : true, "AD-04" : true, "AD-05" : true, "AD-06" : true,
- "AD-07" : true, "AD-08" : true, "AE-AJ" : true, "AE-AZ" : true, "AE-DU" : true,
- "AE-FU" : true, "AE-RK" : true, "AE-SH" : true, "AE-UQ" : true, "AF-BAL" : true,
- "AF-BAM" : true, "AF-BDG" : true, "AF-BDS" : true, "AF-BGL" : true, "AF-DAY" : true,
- "AF-FRA" : true, "AF-FYB" : true, "AF-GHA" : true, "AF-GHO" : true, "AF-HEL" : true,
- "AF-HER" : true, "AF-JOW" : true, "AF-KAB" : true, "AF-KAN" : true, "AF-KAP" : true,
- "AF-KDZ" : true, "AF-KHO" : true, "AF-KNR" : true, "AF-LAG" : true, "AF-LOG" : true,
- "AF-NAN" : true, "AF-NIM" : true, "AF-NUR" : true, "AF-PAN" : true, "AF-PAR" : true,
- "AF-PIA" : true, "AF-PKA" : true, "AF-SAM" : true, "AF-SAR" : true, "AF-TAK" : true,
- "AF-URU" : true, "AF-WAR" : true, "AF-ZAB" : true, "AG-03" : true, "AG-04" : true,
- "AG-05" : true, "AG-06" : true, "AG-07" : true, "AG-08" : true, "AG-10" : true,
- "AG-11" : true, "AL-01" : true, "AL-02" : true, "AL-03" : true, "AL-04" : true,
- "AL-05" : true, "AL-06" : true, "AL-07" : true, "AL-08" : true, "AL-09" : true,
- "AL-10" : true, "AL-11" : true, "AL-12" : true, "AL-BR" : true, "AL-BU" : true,
- "AL-DI" : true, "AL-DL" : true, "AL-DR" : true, "AL-DV" : true, "AL-EL" : true,
- "AL-ER" : true, "AL-FR" : true, "AL-GJ" : true, "AL-GR" : true, "AL-HA" : true,
- "AL-KA" : true, "AL-KB" : true, "AL-KC" : true, "AL-KO" : true, "AL-KR" : true,
- "AL-KU" : true, "AL-LB" : true, "AL-LE" : true, "AL-LU" : true, "AL-MK" : true,
- "AL-MM" : true, "AL-MR" : true, "AL-MT" : true, "AL-PG" : true, "AL-PQ" : true,
- "AL-PR" : true, "AL-PU" : true, "AL-SH" : true, "AL-SK" : true, "AL-SR" : true,
- "AL-TE" : true, "AL-TP" : true, "AL-TR" : true, "AL-VL" : true, "AM-AG" : true,
- "AM-AR" : true, "AM-AV" : true, "AM-ER" : true, "AM-GR" : true, "AM-KT" : true,
- "AM-LO" : true, "AM-SH" : true, "AM-SU" : true, "AM-TV" : true, "AM-VD" : true,
- "AO-BGO" : true, "AO-BGU" : true, "AO-BIE" : true, "AO-CAB" : true, "AO-CCU" : true,
- "AO-CNN" : true, "AO-CNO" : true, "AO-CUS" : true, "AO-HUA" : true, "AO-HUI" : true,
- "AO-LNO" : true, "AO-LSU" : true, "AO-LUA" : true, "AO-MAL" : true, "AO-MOX" : true,
- "AO-NAM" : true, "AO-UIG" : true, "AO-ZAI" : true, "AR-A" : true, "AR-B" : true,
- "AR-C" : true, "AR-D" : true, "AR-E" : true, "AR-G" : true, "AR-H" : true,
- "AR-J" : true, "AR-K" : true, "AR-L" : true, "AR-M" : true, "AR-N" : true,
- "AR-P" : true, "AR-Q" : true, "AR-R" : true, "AR-S" : true, "AR-T" : true,
- "AR-U" : true, "AR-V" : true, "AR-W" : true, "AR-X" : true, "AR-Y" : true,
- "AR-Z" : true, "AT-1" : true, "AT-2" : true, "AT-3" : true, "AT-4" : true,
- "AT-5" : true, "AT-6" : true, "AT-7" : true, "AT-8" : true, "AT-9" : true,
- "AU-ACT" : true, "AU-NSW" : true, "AU-NT" : true, "AU-QLD" : true, "AU-SA" : true,
- "AU-TAS" : true, "AU-VIC" : true, "AU-WA" : true, "AZ-ABS" : true, "AZ-AGA" : true,
- "AZ-AGC" : true, "AZ-AGM" : true, "AZ-AGS" : true, "AZ-AGU" : true, "AZ-AST" : true,
- "AZ-BA" : true, "AZ-BAB" : true, "AZ-BAL" : true, "AZ-BAR" : true, "AZ-BEY" : true,
- "AZ-BIL" : true, "AZ-CAB" : true, "AZ-CAL" : true, "AZ-CUL" : true, "AZ-DAS" : true,
- "AZ-FUZ" : true, "AZ-GA" : true, "AZ-GAD" : true, "AZ-GOR" : true, "AZ-GOY" : true,
- "AZ-GYG" : true, "AZ-HAC" : true, "AZ-IMI" : true, "AZ-ISM" : true, "AZ-KAL" : true,
- "AZ-KAN" : true, "AZ-KUR" : true, "AZ-LA" : true, "AZ-LAC" : true, "AZ-LAN" : true,
- "AZ-LER" : true, "AZ-MAS" : true, "AZ-MI" : true, "AZ-NA" : true, "AZ-NEF" : true,
- "AZ-NV" : true, "AZ-NX" : true, "AZ-OGU" : true, "AZ-ORD" : true, "AZ-QAB" : true,
- "AZ-QAX" : true, "AZ-QAZ" : true, "AZ-QBA" : true, "AZ-QBI" : true, "AZ-QOB" : true,
- "AZ-QUS" : true, "AZ-SA" : true, "AZ-SAB" : true, "AZ-SAD" : true, "AZ-SAH" : true,
- "AZ-SAK" : true, "AZ-SAL" : true, "AZ-SAR" : true, "AZ-SAT" : true, "AZ-SBN" : true,
- "AZ-SIY" : true, "AZ-SKR" : true, "AZ-SM" : true, "AZ-SMI" : true, "AZ-SMX" : true,
- "AZ-SR" : true, "AZ-SUS" : true, "AZ-TAR" : true, "AZ-TOV" : true, "AZ-UCA" : true,
- "AZ-XA" : true, "AZ-XAC" : true, "AZ-XCI" : true, "AZ-XIZ" : true, "AZ-XVD" : true,
- "AZ-YAR" : true, "AZ-YE" : true, "AZ-YEV" : true, "AZ-ZAN" : true, "AZ-ZAQ" : true,
- "AZ-ZAR" : true, "BA-01" : true, "BA-02" : true, "BA-03" : true, "BA-04" : true,
- "BA-05" : true, "BA-06" : true, "BA-07" : true, "BA-08" : true, "BA-09" : true,
- "BA-10" : true, "BA-BIH" : true, "BA-BRC" : true, "BA-SRP" : true, "BB-01" : true,
- "BB-02" : true, "BB-03" : true, "BB-04" : true, "BB-05" : true, "BB-06" : true,
- "BB-07" : true, "BB-08" : true, "BB-09" : true, "BB-10" : true, "BB-11" : true,
- "BD-01" : true, "BD-02" : true, "BD-03" : true, "BD-04" : true, "BD-05" : true,
- "BD-06" : true, "BD-07" : true, "BD-08" : true, "BD-09" : true, "BD-10" : true,
- "BD-11" : true, "BD-12" : true, "BD-13" : true, "BD-14" : true, "BD-15" : true,
- "BD-16" : true, "BD-17" : true, "BD-18" : true, "BD-19" : true, "BD-20" : true,
- "BD-21" : true, "BD-22" : true, "BD-23" : true, "BD-24" : true, "BD-25" : true,
- "BD-26" : true, "BD-27" : true, "BD-28" : true, "BD-29" : true, "BD-30" : true,
- "BD-31" : true, "BD-32" : true, "BD-33" : true, "BD-34" : true, "BD-35" : true,
- "BD-36" : true, "BD-37" : true, "BD-38" : true, "BD-39" : true, "BD-40" : true,
- "BD-41" : true, "BD-42" : true, "BD-43" : true, "BD-44" : true, "BD-45" : true,
- "BD-46" : true, "BD-47" : true, "BD-48" : true, "BD-49" : true, "BD-50" : true,
- "BD-51" : true, "BD-52" : true, "BD-53" : true, "BD-54" : true, "BD-55" : true,
- "BD-56" : true, "BD-57" : true, "BD-58" : true, "BD-59" : true, "BD-60" : true,
- "BD-61" : true, "BD-62" : true, "BD-63" : true, "BD-64" : true, "BD-A" : true,
- "BD-B" : true, "BD-C" : true, "BD-D" : true, "BD-E" : true, "BD-F" : true,
- "BD-G" : true, "BE-BRU" : true, "BE-VAN" : true, "BE-VBR" : true, "BE-VLG" : true,
- "BE-VLI" : true, "BE-VOV" : true, "BE-VWV" : true, "BE-WAL" : true, "BE-WBR" : true,
- "BE-WHT" : true, "BE-WLG" : true, "BE-WLX" : true, "BE-WNA" : true, "BF-01" : true,
- "BF-02" : true, "BF-03" : true, "BF-04" : true, "BF-05" : true, "BF-06" : true,
- "BF-07" : true, "BF-08" : true, "BF-09" : true, "BF-10" : true, "BF-11" : true,
- "BF-12" : true, "BF-13" : true, "BF-BAL" : true, "BF-BAM" : true, "BF-BAN" : true,
- "BF-BAZ" : true, "BF-BGR" : true, "BF-BLG" : true, "BF-BLK" : true, "BF-COM" : true,
- "BF-GAN" : true, "BF-GNA" : true, "BF-GOU" : true, "BF-HOU" : true, "BF-IOB" : true,
- "BF-KAD" : true, "BF-KEN" : true, "BF-KMD" : true, "BF-KMP" : true, "BF-KOP" : true,
- "BF-KOS" : true, "BF-KOT" : true, "BF-KOW" : true, "BF-LER" : true, "BF-LOR" : true,
- "BF-MOU" : true, "BF-NAM" : true, "BF-NAO" : true, "BF-NAY" : true, "BF-NOU" : true,
- "BF-OUB" : true, "BF-OUD" : true, "BF-PAS" : true, "BF-PON" : true, "BF-SEN" : true,
- "BF-SIS" : true, "BF-SMT" : true, "BF-SNG" : true, "BF-SOM" : true, "BF-SOR" : true,
- "BF-TAP" : true, "BF-TUI" : true, "BF-YAG" : true, "BF-YAT" : true, "BF-ZIR" : true,
- "BF-ZON" : true, "BF-ZOU" : true, "BG-01" : true, "BG-02" : true, "BG-03" : true,
- "BG-04" : true, "BG-05" : true, "BG-06" : true, "BG-07" : true, "BG-08" : true,
- "BG-09" : true, "BG-10" : true, "BG-11" : true, "BG-12" : true, "BG-13" : true,
- "BG-14" : true, "BG-15" : true, "BG-16" : true, "BG-17" : true, "BG-18" : true,
- "BG-19" : true, "BG-20" : true, "BG-21" : true, "BG-22" : true, "BG-23" : true,
- "BG-24" : true, "BG-25" : true, "BG-26" : true, "BG-27" : true, "BG-28" : true,
- "BH-13" : true, "BH-14" : true, "BH-15" : true, "BH-16" : true, "BH-17" : true,
- "BI-BB" : true, "BI-BL" : true, "BI-BM" : true, "BI-BR" : true, "BI-CA" : true,
- "BI-CI" : true, "BI-GI" : true, "BI-KI" : true, "BI-KR" : true, "BI-KY" : true,
- "BI-MA" : true, "BI-MU" : true, "BI-MW" : true, "BI-NG" : true, "BI-RT" : true,
- "BI-RY" : true, "BJ-AK" : true, "BJ-AL" : true, "BJ-AQ" : true, "BJ-BO" : true,
- "BJ-CO" : true, "BJ-DO" : true, "BJ-KO" : true, "BJ-LI" : true, "BJ-MO" : true,
- "BJ-OU" : true, "BJ-PL" : true, "BJ-ZO" : true, "BN-BE" : true, "BN-BM" : true,
- "BN-TE" : true, "BN-TU" : true, "BO-B" : true, "BO-C" : true, "BO-H" : true,
- "BO-L" : true, "BO-N" : true, "BO-O" : true, "BO-P" : true, "BO-S" : true,
- "BO-T" : true, "BQ-BO" : true, "BQ-SA" : true, "BQ-SE" : true, "BR-AC" : true,
- "BR-AL" : true, "BR-AM" : true, "BR-AP" : true, "BR-BA" : true, "BR-CE" : true,
- "BR-DF" : true, "BR-ES" : true, "BR-FN" : true, "BR-GO" : true, "BR-MA" : true,
- "BR-MG" : true, "BR-MS" : true, "BR-MT" : true, "BR-PA" : true, "BR-PB" : true,
- "BR-PE" : true, "BR-PI" : true, "BR-PR" : true, "BR-RJ" : true, "BR-RN" : true,
- "BR-RO" : true, "BR-RR" : true, "BR-RS" : true, "BR-SC" : true, "BR-SE" : true,
- "BR-SP" : true, "BR-TO" : true, "BS-AK" : true, "BS-BI" : true, "BS-BP" : true,
- "BS-BY" : true, "BS-CE" : true, "BS-CI" : true, "BS-CK" : true, "BS-CO" : true,
- "BS-CS" : true, "BS-EG" : true, "BS-EX" : true, "BS-FP" : true, "BS-GC" : true,
- "BS-HI" : true, "BS-HT" : true, "BS-IN" : true, "BS-LI" : true, "BS-MC" : true,
- "BS-MG" : true, "BS-MI" : true, "BS-NE" : true, "BS-NO" : true, "BS-NS" : true,
- "BS-RC" : true, "BS-RI" : true, "BS-SA" : true, "BS-SE" : true, "BS-SO" : true,
- "BS-SS" : true, "BS-SW" : true, "BS-WG" : true, "BT-11" : true, "BT-12" : true,
- "BT-13" : true, "BT-14" : true, "BT-15" : true, "BT-21" : true, "BT-22" : true,
- "BT-23" : true, "BT-24" : true, "BT-31" : true, "BT-32" : true, "BT-33" : true,
- "BT-34" : true, "BT-41" : true, "BT-42" : true, "BT-43" : true, "BT-44" : true,
- "BT-45" : true, "BT-GA" : true, "BT-TY" : true, "BW-CE" : true, "BW-GH" : true,
- "BW-KG" : true, "BW-KL" : true, "BW-KW" : true, "BW-NE" : true, "BW-NW" : true,
- "BW-SE" : true, "BW-SO" : true, "BY-BR" : true, "BY-HM" : true, "BY-HO" : true,
- "BY-HR" : true, "BY-MA" : true, "BY-MI" : true, "BY-VI" : true, "BZ-BZ" : true,
- "BZ-CY" : true, "BZ-CZL" : true, "BZ-OW" : true, "BZ-SC" : true, "BZ-TOL" : true,
- "CA-AB" : true, "CA-BC" : true, "CA-MB" : true, "CA-NB" : true, "CA-NL" : true,
- "CA-NS" : true, "CA-NT" : true, "CA-NU" : true, "CA-ON" : true, "CA-PE" : true,
- "CA-QC" : true, "CA-SK" : true, "CA-YT" : true, "CD-BC" : true, "CD-BN" : true,
- "CD-EQ" : true, "CD-KA" : true, "CD-KE" : true, "CD-KN" : true, "CD-KW" : true,
- "CD-MA" : true, "CD-NK" : true, "CD-OR" : true, "CD-SK" : true, "CF-AC" : true,
- "CF-BB" : true, "CF-BGF" : true, "CF-BK" : true, "CF-HK" : true, "CF-HM" : true,
- "CF-HS" : true, "CF-KB" : true, "CF-KG" : true, "CF-LB" : true, "CF-MB" : true,
- "CF-MP" : true, "CF-NM" : true, "CF-OP" : true, "CF-SE" : true, "CF-UK" : true,
- "CF-VK" : true, "CG-11" : true, "CG-12" : true, "CG-13" : true, "CG-14" : true,
- "CG-15" : true, "CG-2" : true, "CG-5" : true, "CG-7" : true, "CG-8" : true,
- "CG-9" : true, "CG-BZV" : true, "CH-AG" : true, "CH-AI" : true, "CH-AR" : true,
- "CH-BE" : true, "CH-BL" : true, "CH-BS" : true, "CH-FR" : true, "CH-GE" : true,
- "CH-GL" : true, "CH-GR" : true, "CH-JU" : true, "CH-LU" : true, "CH-NE" : true,
- "CH-NW" : true, "CH-OW" : true, "CH-SG" : true, "CH-SH" : true, "CH-SO" : true,
- "CH-SZ" : true, "CH-TG" : true, "CH-TI" : true, "CH-UR" : true, "CH-VD" : true,
- "CH-VS" : true, "CH-ZG" : true, "CH-ZH" : true, "CI-01" : true, "CI-02" : true,
- "CI-03" : true, "CI-04" : true, "CI-05" : true, "CI-06" : true, "CI-07" : true,
- "CI-08" : true, "CI-09" : true, "CI-10" : true, "CI-11" : true, "CI-12" : true,
- "CI-13" : true, "CI-14" : true, "CI-15" : true, "CI-16" : true, "CI-17" : true,
- "CI-18" : true, "CI-19" : true, "CL-AI" : true, "CL-AN" : true, "CL-AP" : true,
- "CL-AR" : true, "CL-AT" : true, "CL-BI" : true, "CL-CO" : true, "CL-LI" : true,
- "CL-LL" : true, "CL-LR" : true, "CL-MA" : true, "CL-ML" : true, "CL-RM" : true,
- "CL-TA" : true, "CL-VS" : true, "CM-AD" : true, "CM-CE" : true, "CM-EN" : true,
- "CM-ES" : true, "CM-LT" : true, "CM-NO" : true, "CM-NW" : true, "CM-OU" : true,
- "CM-SU" : true, "CM-SW" : true, "CN-11" : true, "CN-12" : true, "CN-13" : true,
- "CN-14" : true, "CN-15" : true, "CN-21" : true, "CN-22" : true, "CN-23" : true,
- "CN-31" : true, "CN-32" : true, "CN-33" : true, "CN-34" : true, "CN-35" : true,
- "CN-36" : true, "CN-37" : true, "CN-41" : true, "CN-42" : true, "CN-43" : true,
- "CN-44" : true, "CN-45" : true, "CN-46" : true, "CN-50" : true, "CN-51" : true,
- "CN-52" : true, "CN-53" : true, "CN-54" : true, "CN-61" : true, "CN-62" : true,
- "CN-63" : true, "CN-64" : true, "CN-65" : true, "CN-71" : true, "CN-91" : true,
- "CN-92" : true, "CO-AMA" : true, "CO-ANT" : true, "CO-ARA" : true, "CO-ATL" : true,
- "CO-BOL" : true, "CO-BOY" : true, "CO-CAL" : true, "CO-CAQ" : true, "CO-CAS" : true,
- "CO-CAU" : true, "CO-CES" : true, "CO-CHO" : true, "CO-COR" : true, "CO-CUN" : true,
- "CO-DC" : true, "CO-GUA" : true, "CO-GUV" : true, "CO-HUI" : true, "CO-LAG" : true,
- "CO-MAG" : true, "CO-MET" : true, "CO-NAR" : true, "CO-NSA" : true, "CO-PUT" : true,
- "CO-QUI" : true, "CO-RIS" : true, "CO-SAN" : true, "CO-SAP" : true, "CO-SUC" : true,
- "CO-TOL" : true, "CO-VAC" : true, "CO-VAU" : true, "CO-VID" : true, "CR-A" : true,
- "CR-C" : true, "CR-G" : true, "CR-H" : true, "CR-L" : true, "CR-P" : true,
- "CR-SJ" : true, "CU-01" : true, "CU-02" : true, "CU-03" : true, "CU-04" : true,
- "CU-05" : true, "CU-06" : true, "CU-07" : true, "CU-08" : true, "CU-09" : true,
- "CU-10" : true, "CU-11" : true, "CU-12" : true, "CU-13" : true, "CU-14" : true,
- "CU-99" : true, "CV-B" : true, "CV-BR" : true, "CV-BV" : true, "CV-CA" : true,
- "CV-CF" : true, "CV-CR" : true, "CV-MA" : true, "CV-MO" : true, "CV-PA" : true,
- "CV-PN" : true, "CV-PR" : true, "CV-RB" : true, "CV-RG" : true, "CV-RS" : true,
- "CV-S" : true, "CV-SD" : true, "CV-SF" : true, "CV-SL" : true, "CV-SM" : true,
- "CV-SO" : true, "CV-SS" : true, "CV-SV" : true, "CV-TA" : true, "CV-TS" : true,
- "CY-01" : true, "CY-02" : true, "CY-03" : true, "CY-04" : true, "CY-05" : true,
- "CY-06" : true, "CZ-10" : true, "CZ-101" : true, "CZ-102" : true, "CZ-103" : true,
- "CZ-104" : true, "CZ-105" : true, "CZ-106" : true, "CZ-107" : true, "CZ-108" : true,
- "CZ-109" : true, "CZ-110" : true, "CZ-111" : true, "CZ-112" : true, "CZ-113" : true,
- "CZ-114" : true, "CZ-115" : true, "CZ-116" : true, "CZ-117" : true, "CZ-118" : true,
- "CZ-119" : true, "CZ-120" : true, "CZ-121" : true, "CZ-122" : true, "CZ-20" : true,
- "CZ-201" : true, "CZ-202" : true, "CZ-203" : true, "CZ-204" : true, "CZ-205" : true,
- "CZ-206" : true, "CZ-207" : true, "CZ-208" : true, "CZ-209" : true, "CZ-20A" : true,
- "CZ-20B" : true, "CZ-20C" : true, "CZ-31" : true, "CZ-311" : true, "CZ-312" : true,
- "CZ-313" : true, "CZ-314" : true, "CZ-315" : true, "CZ-316" : true, "CZ-317" : true,
- "CZ-32" : true, "CZ-321" : true, "CZ-322" : true, "CZ-323" : true, "CZ-324" : true,
- "CZ-325" : true, "CZ-326" : true, "CZ-327" : true, "CZ-41" : true, "CZ-411" : true,
- "CZ-412" : true, "CZ-413" : true, "CZ-42" : true, "CZ-421" : true, "CZ-422" : true,
- "CZ-423" : true, "CZ-424" : true, "CZ-425" : true, "CZ-426" : true, "CZ-427" : true,
- "CZ-51" : true, "CZ-511" : true, "CZ-512" : true, "CZ-513" : true, "CZ-514" : true,
- "CZ-52" : true, "CZ-521" : true, "CZ-522" : true, "CZ-523" : true, "CZ-524" : true,
- "CZ-525" : true, "CZ-53" : true, "CZ-531" : true, "CZ-532" : true, "CZ-533" : true,
- "CZ-534" : true, "CZ-63" : true, "CZ-631" : true, "CZ-632" : true, "CZ-633" : true,
- "CZ-634" : true, "CZ-635" : true, "CZ-64" : true, "CZ-641" : true, "CZ-642" : true,
- "CZ-643" : true, "CZ-644" : true, "CZ-645" : true, "CZ-646" : true, "CZ-647" : true,
- "CZ-71" : true, "CZ-711" : true, "CZ-712" : true, "CZ-713" : true, "CZ-714" : true,
- "CZ-715" : true, "CZ-72" : true, "CZ-721" : true, "CZ-722" : true, "CZ-723" : true,
- "CZ-724" : true, "CZ-80" : true, "CZ-801" : true, "CZ-802" : true, "CZ-803" : true,
- "CZ-804" : true, "CZ-805" : true, "CZ-806" : true, "DE-BB" : true, "DE-BE" : true,
- "DE-BW" : true, "DE-BY" : true, "DE-HB" : true, "DE-HE" : true, "DE-HH" : true,
- "DE-MV" : true, "DE-NI" : true, "DE-NW" : true, "DE-RP" : true, "DE-SH" : true,
- "DE-SL" : true, "DE-SN" : true, "DE-ST" : true, "DE-TH" : true, "DJ-AR" : true,
- "DJ-AS" : true, "DJ-DI" : true, "DJ-DJ" : true, "DJ-OB" : true, "DJ-TA" : true,
- "DK-81" : true, "DK-82" : true, "DK-83" : true, "DK-84" : true, "DK-85" : true,
- "DM-01" : true, "DM-02" : true, "DM-03" : true, "DM-04" : true, "DM-05" : true,
- "DM-06" : true, "DM-07" : true, "DM-08" : true, "DM-09" : true, "DM-10" : true,
- "DO-01" : true, "DO-02" : true, "DO-03" : true, "DO-04" : true, "DO-05" : true,
- "DO-06" : true, "DO-07" : true, "DO-08" : true, "DO-09" : true, "DO-10" : true,
- "DO-11" : true, "DO-12" : true, "DO-13" : true, "DO-14" : true, "DO-15" : true,
- "DO-16" : true, "DO-17" : true, "DO-18" : true, "DO-19" : true, "DO-20" : true,
- "DO-21" : true, "DO-22" : true, "DO-23" : true, "DO-24" : true, "DO-25" : true,
- "DO-26" : true, "DO-27" : true, "DO-28" : true, "DO-29" : true, "DO-30" : true,
- "DZ-01" : true, "DZ-02" : true, "DZ-03" : true, "DZ-04" : true, "DZ-05" : true,
- "DZ-06" : true, "DZ-07" : true, "DZ-08" : true, "DZ-09" : true, "DZ-10" : true,
- "DZ-11" : true, "DZ-12" : true, "DZ-13" : true, "DZ-14" : true, "DZ-15" : true,
- "DZ-16" : true, "DZ-17" : true, "DZ-18" : true, "DZ-19" : true, "DZ-20" : true,
- "DZ-21" : true, "DZ-22" : true, "DZ-23" : true, "DZ-24" : true, "DZ-25" : true,
- "DZ-26" : true, "DZ-27" : true, "DZ-28" : true, "DZ-29" : true, "DZ-30" : true,
- "DZ-31" : true, "DZ-32" : true, "DZ-33" : true, "DZ-34" : true, "DZ-35" : true,
- "DZ-36" : true, "DZ-37" : true, "DZ-38" : true, "DZ-39" : true, "DZ-40" : true,
- "DZ-41" : true, "DZ-42" : true, "DZ-43" : true, "DZ-44" : true, "DZ-45" : true,
- "DZ-46" : true, "DZ-47" : true, "DZ-48" : true, "EC-A" : true, "EC-B" : true,
- "EC-C" : true, "EC-D" : true, "EC-E" : true, "EC-F" : true, "EC-G" : true,
- "EC-H" : true, "EC-I" : true, "EC-L" : true, "EC-M" : true, "EC-N" : true,
- "EC-O" : true, "EC-P" : true, "EC-R" : true, "EC-S" : true, "EC-SD" : true,
- "EC-SE" : true, "EC-T" : true, "EC-U" : true, "EC-W" : true, "EC-X" : true,
- "EC-Y" : true, "EC-Z" : true, "EE-37" : true, "EE-39" : true, "EE-44" : true,
- "EE-49" : true, "EE-51" : true, "EE-57" : true, "EE-59" : true, "EE-65" : true,
- "EE-67" : true, "EE-70" : true, "EE-74" : true, "EE-78" : true, "EE-82" : true,
- "EE-84" : true, "EE-86" : true, "EG-ALX" : true, "EG-ASN" : true, "EG-AST" : true,
- "EG-BA" : true, "EG-BH" : true, "EG-BNS" : true, "EG-C" : true, "EG-DK" : true,
- "EG-DT" : true, "EG-FYM" : true, "EG-GH" : true, "EG-GZ" : true, "EG-HU" : true,
- "EG-IS" : true, "EG-JS" : true, "EG-KB" : true, "EG-KFS" : true, "EG-KN" : true,
- "EG-MN" : true, "EG-MNF" : true, "EG-MT" : true, "EG-PTS" : true, "EG-SHG" : true,
- "EG-SHR" : true, "EG-SIN" : true, "EG-SU" : true, "EG-SUZ" : true, "EG-WAD" : true,
- "ER-AN" : true, "ER-DK" : true, "ER-DU" : true, "ER-GB" : true, "ER-MA" : true,
- "ER-SK" : true, "ES-A" : true, "ES-AB" : true, "ES-AL" : true, "ES-AN" : true,
- "ES-AR" : true, "ES-AS" : true, "ES-AV" : true, "ES-B" : true, "ES-BA" : true,
- "ES-BI" : true, "ES-BU" : true, "ES-C" : true, "ES-CA" : true, "ES-CB" : true,
- "ES-CC" : true, "ES-CE" : true, "ES-CL" : true, "ES-CM" : true, "ES-CN" : true,
- "ES-CO" : true, "ES-CR" : true, "ES-CS" : true, "ES-CT" : true, "ES-CU" : true,
- "ES-EX" : true, "ES-GA" : true, "ES-GC" : true, "ES-GI" : true, "ES-GR" : true,
- "ES-GU" : true, "ES-H" : true, "ES-HU" : true, "ES-IB" : true, "ES-J" : true,
- "ES-L" : true, "ES-LE" : true, "ES-LO" : true, "ES-LU" : true, "ES-M" : true,
- "ES-MA" : true, "ES-MC" : true, "ES-MD" : true, "ES-ML" : true, "ES-MU" : true,
- "ES-NA" : true, "ES-NC" : true, "ES-O" : true, "ES-OR" : true, "ES-P" : true,
- "ES-PM" : true, "ES-PO" : true, "ES-PV" : true, "ES-RI" : true, "ES-S" : true,
- "ES-SA" : true, "ES-SE" : true, "ES-SG" : true, "ES-SO" : true, "ES-SS" : true,
- "ES-T" : true, "ES-TE" : true, "ES-TF" : true, "ES-TO" : true, "ES-V" : true,
- "ES-VA" : true, "ES-VC" : true, "ES-VI" : true, "ES-Z" : true, "ES-ZA" : true,
- "ET-AA" : true, "ET-AF" : true, "ET-AM" : true, "ET-BE" : true, "ET-DD" : true,
- "ET-GA" : true, "ET-HA" : true, "ET-OR" : true, "ET-SN" : true, "ET-SO" : true,
- "ET-TI" : true, "FI-01" : true, "FI-02" : true, "FI-03" : true, "FI-04" : true,
- "FI-05" : true, "FI-06" : true, "FI-07" : true, "FI-08" : true, "FI-09" : true,
- "FI-10" : true, "FI-11" : true, "FI-12" : true, "FI-13" : true, "FI-14" : true,
- "FI-15" : true, "FI-16" : true, "FI-17" : true, "FI-18" : true, "FI-19" : true,
- "FJ-C" : true, "FJ-E" : true, "FJ-N" : true, "FJ-R" : true, "FJ-W" : true,
- "FM-KSA" : true, "FM-PNI" : true, "FM-TRK" : true, "FM-YAP" : true, "FR-01" : true,
- "FR-02" : true, "FR-03" : true, "FR-04" : true, "FR-05" : true, "FR-06" : true,
- "FR-07" : true, "FR-08" : true, "FR-09" : true, "FR-10" : true, "FR-11" : true,
- "FR-12" : true, "FR-13" : true, "FR-14" : true, "FR-15" : true, "FR-16" : true,
- "FR-17" : true, "FR-18" : true, "FR-19" : true, "FR-21" : true, "FR-22" : true,
- "FR-23" : true, "FR-24" : true, "FR-25" : true, "FR-26" : true, "FR-27" : true,
- "FR-28" : true, "FR-29" : true, "FR-2A" : true, "FR-2B" : true, "FR-30" : true,
- "FR-31" : true, "FR-32" : true, "FR-33" : true, "FR-34" : true, "FR-35" : true,
- "FR-36" : true, "FR-37" : true, "FR-38" : true, "FR-39" : true, "FR-40" : true,
- "FR-41" : true, "FR-42" : true, "FR-43" : true, "FR-44" : true, "FR-45" : true,
- "FR-46" : true, "FR-47" : true, "FR-48" : true, "FR-49" : true, "FR-50" : true,
- "FR-51" : true, "FR-52" : true, "FR-53" : true, "FR-54" : true, "FR-55" : true,
- "FR-56" : true, "FR-57" : true, "FR-58" : true, "FR-59" : true, "FR-60" : true,
- "FR-61" : true, "FR-62" : true, "FR-63" : true, "FR-64" : true, "FR-65" : true,
- "FR-66" : true, "FR-67" : true, "FR-68" : true, "FR-69" : true, "FR-70" : true,
- "FR-71" : true, "FR-72" : true, "FR-73" : true, "FR-74" : true, "FR-75" : true,
- "FR-76" : true, "FR-77" : true, "FR-78" : true, "FR-79" : true, "FR-80" : true,
- "FR-81" : true, "FR-82" : true, "FR-83" : true, "FR-84" : true, "FR-85" : true,
- "FR-86" : true, "FR-87" : true, "FR-88" : true, "FR-89" : true, "FR-90" : true,
- "FR-91" : true, "FR-92" : true, "FR-93" : true, "FR-94" : true, "FR-95" : true,
- "FR-ARA" : true, "FR-BFC" : true, "FR-BL" : true, "FR-BRE" : true, "FR-COR" : true,
- "FR-CP" : true, "FR-CVL" : true, "FR-GES" : true, "FR-GF" : true, "FR-GP" : true,
- "FR-GUA" : true, "FR-HDF" : true, "FR-IDF" : true, "FR-LRE" : true, "FR-MAY" : true,
- "FR-MF" : true, "FR-MQ" : true, "FR-NAQ" : true, "FR-NC" : true, "FR-NOR" : true,
- "FR-OCC" : true, "FR-PAC" : true, "FR-PDL" : true, "FR-PF" : true, "FR-PM" : true,
- "FR-RE" : true, "FR-TF" : true, "FR-WF" : true, "FR-YT" : true, "GA-1" : true,
- "GA-2" : true, "GA-3" : true, "GA-4" : true, "GA-5" : true, "GA-6" : true,
- "GA-7" : true, "GA-8" : true, "GA-9" : true, "GB-ABC" : true, "GB-ABD" : true,
- "GB-ABE" : true, "GB-AGB" : true, "GB-AGY" : true, "GB-AND" : true, "GB-ANN" : true,
- "GB-ANS" : true, "GB-BAS" : true, "GB-BBD" : true, "GB-BDF" : true, "GB-BDG" : true,
- "GB-BEN" : true, "GB-BEX" : true, "GB-BFS" : true, "GB-BGE" : true, "GB-BGW" : true,
- "GB-BIR" : true, "GB-BKM" : true, "GB-BMH" : true, "GB-BNE" : true, "GB-BNH" : true,
- "GB-BNS" : true, "GB-BOL" : true, "GB-BPL" : true, "GB-BRC" : true, "GB-BRD" : true,
- "GB-BRY" : true, "GB-BST" : true, "GB-BUR" : true, "GB-CAM" : true, "GB-CAY" : true,
- "GB-CBF" : true, "GB-CCG" : true, "GB-CGN" : true, "GB-CHE" : true, "GB-CHW" : true,
- "GB-CLD" : true, "GB-CLK" : true, "GB-CMA" : true, "GB-CMD" : true, "GB-CMN" : true,
- "GB-CON" : true, "GB-COV" : true, "GB-CRF" : true, "GB-CRY" : true, "GB-CWY" : true,
- "GB-DAL" : true, "GB-DBY" : true, "GB-DEN" : true, "GB-DER" : true, "GB-DEV" : true,
- "GB-DGY" : true, "GB-DNC" : true, "GB-DND" : true, "GB-DOR" : true, "GB-DRS" : true,
- "GB-DUD" : true, "GB-DUR" : true, "GB-EAL" : true, "GB-EAW" : true, "GB-EAY" : true,
- "GB-EDH" : true, "GB-EDU" : true, "GB-ELN" : true, "GB-ELS" : true, "GB-ENF" : true,
- "GB-ENG" : true, "GB-ERW" : true, "GB-ERY" : true, "GB-ESS" : true, "GB-ESX" : true,
- "GB-FAL" : true, "GB-FIF" : true, "GB-FLN" : true, "GB-FMO" : true, "GB-GAT" : true,
- "GB-GBN" : true, "GB-GLG" : true, "GB-GLS" : true, "GB-GRE" : true, "GB-GWN" : true,
- "GB-HAL" : true, "GB-HAM" : true, "GB-HAV" : true, "GB-HCK" : true, "GB-HEF" : true,
- "GB-HIL" : true, "GB-HLD" : true, "GB-HMF" : true, "GB-HNS" : true, "GB-HPL" : true,
- "GB-HRT" : true, "GB-HRW" : true, "GB-HRY" : true, "GB-IOS" : true, "GB-IOW" : true,
- "GB-ISL" : true, "GB-IVC" : true, "GB-KEC" : true, "GB-KEN" : true, "GB-KHL" : true,
- "GB-KIR" : true, "GB-KTT" : true, "GB-KWL" : true, "GB-LAN" : true, "GB-LBC" : true,
- "GB-LBH" : true, "GB-LCE" : true, "GB-LDS" : true, "GB-LEC" : true, "GB-LEW" : true,
- "GB-LIN" : true, "GB-LIV" : true, "GB-LND" : true, "GB-LUT" : true, "GB-MAN" : true,
- "GB-MDB" : true, "GB-MDW" : true, "GB-MEA" : true, "GB-MIK" : true, "GD-01" : true,
- "GB-MLN" : true, "GB-MON" : true, "GB-MRT" : true, "GB-MRY" : true, "GB-MTY" : true,
- "GB-MUL" : true, "GB-NAY" : true, "GB-NBL" : true, "GB-NEL" : true, "GB-NET" : true,
- "GB-NFK" : true, "GB-NGM" : true, "GB-NIR" : true, "GB-NLK" : true, "GB-NLN" : true,
- "GB-NMD" : true, "GB-NSM" : true, "GB-NTH" : true, "GB-NTL" : true, "GB-NTT" : true,
- "GB-NTY" : true, "GB-NWM" : true, "GB-NWP" : true, "GB-NYK" : true, "GB-OLD" : true,
- "GB-ORK" : true, "GB-OXF" : true, "GB-PEM" : true, "GB-PKN" : true, "GB-PLY" : true,
- "GB-POL" : true, "GB-POR" : true, "GB-POW" : true, "GB-PTE" : true, "GB-RCC" : true,
- "GB-RCH" : true, "GB-RCT" : true, "GB-RDB" : true, "GB-RDG" : true, "GB-RFW" : true,
- "GB-RIC" : true, "GB-ROT" : true, "GB-RUT" : true, "GB-SAW" : true, "GB-SAY" : true,
- "GB-SCB" : true, "GB-SCT" : true, "GB-SFK" : true, "GB-SFT" : true, "GB-SGC" : true,
- "GB-SHF" : true, "GB-SHN" : true, "GB-SHR" : true, "GB-SKP" : true, "GB-SLF" : true,
- "GB-SLG" : true, "GB-SLK" : true, "GB-SND" : true, "GB-SOL" : true, "GB-SOM" : true,
- "GB-SOS" : true, "GB-SRY" : true, "GB-STE" : true, "GB-STG" : true, "GB-STH" : true,
- "GB-STN" : true, "GB-STS" : true, "GB-STT" : true, "GB-STY" : true, "GB-SWA" : true,
- "GB-SWD" : true, "GB-SWK" : true, "GB-TAM" : true, "GB-TFW" : true, "GB-THR" : true,
- "GB-TOB" : true, "GB-TOF" : true, "GB-TRF" : true, "GB-TWH" : true, "GB-UKM" : true,
- "GB-VGL" : true, "GB-WAR" : true, "GB-WBK" : true, "GB-WDU" : true, "GB-WFT" : true,
- "GB-WGN" : true, "GB-WIL" : true, "GB-WKF" : true, "GB-WLL" : true, "GB-WLN" : true,
- "GB-WLS" : true, "GB-WLV" : true, "GB-WND" : true, "GB-WNM" : true, "GB-WOK" : true,
- "GB-WOR" : true, "GB-WRL" : true, "GB-WRT" : true, "GB-WRX" : true, "GB-WSM" : true,
- "GB-WSX" : true, "GB-YOR" : true, "GB-ZET" : true, "GD-02" : true, "GD-03" : true,
- "GD-04" : true, "GD-05" : true, "GD-06" : true, "GD-10" : true, "GE-AB" : true,
- "GE-AJ" : true, "GE-GU" : true, "GE-IM" : true, "GE-KA" : true, "GE-KK" : true,
- "GE-MM" : true, "GE-RL" : true, "GE-SJ" : true, "GE-SK" : true, "GE-SZ" : true,
- "GE-TB" : true, "GH-AA" : true, "GH-AH" : true, "GH-BA" : true, "GH-CP" : true,
- "GH-EP" : true, "GH-NP" : true, "GH-TV" : true, "GH-UE" : true, "GH-UW" : true,
- "GH-WP" : true, "GL-KU" : true, "GL-QA" : true, "GL-QE" : true, "GL-SM" : true,
- "GM-B" : true, "GM-L" : true, "GM-M" : true, "GM-N" : true, "GM-U" : true,
- "GM-W" : true, "GN-B" : true, "GN-BE" : true, "GN-BF" : true, "GN-BK" : true,
- "GN-C" : true, "GN-CO" : true, "GN-D" : true, "GN-DB" : true, "GN-DI" : true,
- "GN-DL" : true, "GN-DU" : true, "GN-F" : true, "GN-FA" : true, "GN-FO" : true,
- "GN-FR" : true, "GN-GA" : true, "GN-GU" : true, "GN-K" : true, "GN-KA" : true,
- "GN-KB" : true, "GN-KD" : true, "GN-KE" : true, "GN-KN" : true, "GN-KO" : true,
- "GN-KS" : true, "GN-L" : true, "GN-LA" : true, "GN-LE" : true, "GN-LO" : true,
- "GN-M" : true, "GN-MC" : true, "GN-MD" : true, "GN-ML" : true, "GN-MM" : true,
- "GN-N" : true, "GN-NZ" : true, "GN-PI" : true, "GN-SI" : true, "GN-TE" : true,
- "GN-TO" : true, "GN-YO" : true, "GQ-AN" : true, "GQ-BN" : true, "GQ-BS" : true,
- "GQ-C" : true, "GQ-CS" : true, "GQ-I" : true, "GQ-KN" : true, "GQ-LI" : true,
- "GQ-WN" : true, "GR-01" : true, "GR-03" : true, "GR-04" : true, "GR-05" : true,
- "GR-06" : true, "GR-07" : true, "GR-11" : true, "GR-12" : true, "GR-13" : true,
- "GR-14" : true, "GR-15" : true, "GR-16" : true, "GR-17" : true, "GR-21" : true,
- "GR-22" : true, "GR-23" : true, "GR-24" : true, "GR-31" : true, "GR-32" : true,
- "GR-33" : true, "GR-34" : true, "GR-41" : true, "GR-42" : true, "GR-43" : true,
- "GR-44" : true, "GR-51" : true, "GR-52" : true, "GR-53" : true, "GR-54" : true,
- "GR-55" : true, "GR-56" : true, "GR-57" : true, "GR-58" : true, "GR-59" : true,
- "GR-61" : true, "GR-62" : true, "GR-63" : true, "GR-64" : true, "GR-69" : true,
- "GR-71" : true, "GR-72" : true, "GR-73" : true, "GR-81" : true, "GR-82" : true,
- "GR-83" : true, "GR-84" : true, "GR-85" : true, "GR-91" : true, "GR-92" : true,
- "GR-93" : true, "GR-94" : true, "GR-A" : true, "GR-A1" : true, "GR-B" : true,
- "GR-C" : true, "GR-D" : true, "GR-E" : true, "GR-F" : true, "GR-G" : true,
- "GR-H" : true, "GR-I" : true, "GR-J" : true, "GR-K" : true, "GR-L" : true,
- "GR-M" : true, "GT-AV" : true, "GT-BV" : true, "GT-CM" : true, "GT-CQ" : true,
- "GT-ES" : true, "GT-GU" : true, "GT-HU" : true, "GT-IZ" : true, "GT-JA" : true,
- "GT-JU" : true, "GT-PE" : true, "GT-PR" : true, "GT-QC" : true, "GT-QZ" : true,
- "GT-RE" : true, "GT-SA" : true, "GT-SM" : true, "GT-SO" : true, "GT-SR" : true,
- "GT-SU" : true, "GT-TO" : true, "GT-ZA" : true, "GW-BA" : true, "GW-BL" : true,
- "GW-BM" : true, "GW-BS" : true, "GW-CA" : true, "GW-GA" : true, "GW-L" : true,
- "GW-N" : true, "GW-OI" : true, "GW-QU" : true, "GW-S" : true, "GW-TO" : true,
- "GY-BA" : true, "GY-CU" : true, "GY-DE" : true, "GY-EB" : true, "GY-ES" : true,
- "GY-MA" : true, "GY-PM" : true, "GY-PT" : true, "GY-UD" : true, "GY-UT" : true,
- "HN-AT" : true, "HN-CH" : true, "HN-CL" : true, "HN-CM" : true, "HN-CP" : true,
- "HN-CR" : true, "HN-EP" : true, "HN-FM" : true, "HN-GD" : true, "HN-IB" : true,
- "HN-IN" : true, "HN-LE" : true, "HN-LP" : true, "HN-OC" : true, "HN-OL" : true,
- "HN-SB" : true, "HN-VA" : true, "HN-YO" : true, "HR-01" : true, "HR-02" : true,
- "HR-03" : true, "HR-04" : true, "HR-05" : true, "HR-06" : true, "HR-07" : true,
- "HR-08" : true, "HR-09" : true, "HR-10" : true, "HR-11" : true, "HR-12" : true,
- "HR-13" : true, "HR-14" : true, "HR-15" : true, "HR-16" : true, "HR-17" : true,
- "HR-18" : true, "HR-19" : true, "HR-20" : true, "HR-21" : true, "HT-AR" : true,
- "HT-CE" : true, "HT-GA" : true, "HT-ND" : true, "HT-NE" : true, "HT-NO" : true,
- "HT-OU" : true, "HT-SD" : true, "HT-SE" : true, "HU-BA" : true, "HU-BC" : true,
- "HU-BE" : true, "HU-BK" : true, "HU-BU" : true, "HU-BZ" : true, "HU-CS" : true,
- "HU-DE" : true, "HU-DU" : true, "HU-EG" : true, "HU-ER" : true, "HU-FE" : true,
- "HU-GS" : true, "HU-GY" : true, "HU-HB" : true, "HU-HE" : true, "HU-HV" : true,
- "HU-JN" : true, "HU-KE" : true, "HU-KM" : true, "HU-KV" : true, "HU-MI" : true,
- "HU-NK" : true, "HU-NO" : true, "HU-NY" : true, "HU-PE" : true, "HU-PS" : true,
- "HU-SD" : true, "HU-SF" : true, "HU-SH" : true, "HU-SK" : true, "HU-SN" : true,
- "HU-SO" : true, "HU-SS" : true, "HU-ST" : true, "HU-SZ" : true, "HU-TB" : true,
- "HU-TO" : true, "HU-VA" : true, "HU-VE" : true, "HU-VM" : true, "HU-ZA" : true,
- "HU-ZE" : true, "ID-AC" : true, "ID-BA" : true, "ID-BB" : true, "ID-BE" : true,
- "ID-BT" : true, "ID-GO" : true, "ID-IJ" : true, "ID-JA" : true, "ID-JB" : true,
- "ID-JI" : true, "ID-JK" : true, "ID-JT" : true, "ID-JW" : true, "ID-KA" : true,
- "ID-KB" : true, "ID-KI" : true, "ID-KR" : true, "ID-KS" : true, "ID-KT" : true,
- "ID-LA" : true, "ID-MA" : true, "ID-ML" : true, "ID-MU" : true, "ID-NB" : true,
- "ID-NT" : true, "ID-NU" : true, "ID-PA" : true, "ID-PB" : true, "ID-RI" : true,
- "ID-SA" : true, "ID-SB" : true, "ID-SG" : true, "ID-SL" : true, "ID-SM" : true,
- "ID-SN" : true, "ID-SR" : true, "ID-SS" : true, "ID-ST" : true, "ID-SU" : true,
- "ID-YO" : true, "IE-C" : true, "IE-CE" : true, "IE-CN" : true, "IE-CO" : true,
- "IE-CW" : true, "IE-D" : true, "IE-DL" : true, "IE-G" : true, "IE-KE" : true,
- "IE-KK" : true, "IE-KY" : true, "IE-L" : true, "IE-LD" : true, "IE-LH" : true,
- "IE-LK" : true, "IE-LM" : true, "IE-LS" : true, "IE-M" : true, "IE-MH" : true,
- "IE-MN" : true, "IE-MO" : true, "IE-OY" : true, "IE-RN" : true, "IE-SO" : true,
- "IE-TA" : true, "IE-U" : true, "IE-WD" : true, "IE-WH" : true, "IE-WW" : true,
- "IE-WX" : true, "IL-D" : true, "IL-HA" : true, "IL-JM" : true, "IL-M" : true,
- "IL-TA" : true, "IL-Z" : true, "IN-AN" : true, "IN-AP" : true, "IN-AR" : true,
- "IN-AS" : true, "IN-BR" : true, "IN-CH" : true, "IN-CT" : true, "IN-DD" : true,
- "IN-DL" : true, "IN-DN" : true, "IN-GA" : true, "IN-GJ" : true, "IN-HP" : true,
- "IN-HR" : true, "IN-JH" : true, "IN-JK" : true, "IN-KA" : true, "IN-KL" : true,
- "IN-LD" : true, "IN-MH" : true, "IN-ML" : true, "IN-MN" : true, "IN-MP" : true,
- "IN-MZ" : true, "IN-NL" : true, "IN-OR" : true, "IN-PB" : true, "IN-PY" : true,
- "IN-RJ" : true, "IN-SK" : true, "IN-TN" : true, "IN-TR" : true, "IN-UP" : true,
- "IN-UT" : true, "IN-WB" : true, "IQ-AN" : true, "IQ-AR" : true, "IQ-BA" : true,
- "IQ-BB" : true, "IQ-BG" : true, "IQ-DA" : true, "IQ-DI" : true, "IQ-DQ" : true,
- "IQ-KA" : true, "IQ-MA" : true, "IQ-MU" : true, "IQ-NA" : true, "IQ-NI" : true,
- "IQ-QA" : true, "IQ-SD" : true, "IQ-SW" : true, "IQ-TS" : true, "IQ-WA" : true,
- "IR-01" : true, "IR-02" : true, "IR-03" : true, "IR-04" : true, "IR-05" : true,
- "IR-06" : true, "IR-07" : true, "IR-08" : true, "IR-10" : true, "IR-11" : true,
- "IR-12" : true, "IR-13" : true, "IR-14" : true, "IR-15" : true, "IR-16" : true,
- "IR-17" : true, "IR-18" : true, "IR-19" : true, "IR-20" : true, "IR-21" : true,
- "IR-22" : true, "IR-23" : true, "IR-24" : true, "IR-25" : true, "IR-26" : true,
- "IR-27" : true, "IR-28" : true, "IR-29" : true, "IR-30" : true, "IR-31" : true,
- "IS-0" : true, "IS-1" : true, "IS-2" : true, "IS-3" : true, "IS-4" : true,
- "IS-5" : true, "IS-6" : true, "IS-7" : true, "IS-8" : true, "IT-21" : true,
- "IT-23" : true, "IT-25" : true, "IT-32" : true, "IT-34" : true, "IT-36" : true,
- "IT-42" : true, "IT-45" : true, "IT-52" : true, "IT-55" : true, "IT-57" : true,
- "IT-62" : true, "IT-65" : true, "IT-67" : true, "IT-72" : true, "IT-75" : true,
- "IT-77" : true, "IT-78" : true, "IT-82" : true, "IT-88" : true, "IT-AG" : true,
- "IT-AL" : true, "IT-AN" : true, "IT-AO" : true, "IT-AP" : true, "IT-AQ" : true,
- "IT-AR" : true, "IT-AT" : true, "IT-AV" : true, "IT-BA" : true, "IT-BG" : true,
- "IT-BI" : true, "IT-BL" : true, "IT-BN" : true, "IT-BO" : true, "IT-BR" : true,
- "IT-BS" : true, "IT-BT" : true, "IT-BZ" : true, "IT-CA" : true, "IT-CB" : true,
- "IT-CE" : true, "IT-CH" : true, "IT-CI" : true, "IT-CL" : true, "IT-CN" : true,
- "IT-CO" : true, "IT-CR" : true, "IT-CS" : true, "IT-CT" : true, "IT-CZ" : true,
- "IT-EN" : true, "IT-FC" : true, "IT-FE" : true, "IT-FG" : true, "IT-FI" : true,
- "IT-FM" : true, "IT-FR" : true, "IT-GE" : true, "IT-GO" : true, "IT-GR" : true,
- "IT-IM" : true, "IT-IS" : true, "IT-KR" : true, "IT-LC" : true, "IT-LE" : true,
- "IT-LI" : true, "IT-LO" : true, "IT-LT" : true, "IT-LU" : true, "IT-MB" : true,
- "IT-MC" : true, "IT-ME" : true, "IT-MI" : true, "IT-MN" : true, "IT-MO" : true,
- "IT-MS" : true, "IT-MT" : true, "IT-NA" : true, "IT-NO" : true, "IT-NU" : true,
- "IT-OG" : true, "IT-OR" : true, "IT-OT" : true, "IT-PA" : true, "IT-PC" : true,
- "IT-PD" : true, "IT-PE" : true, "IT-PG" : true, "IT-PI" : true, "IT-PN" : true,
- "IT-PO" : true, "IT-PR" : true, "IT-PT" : true, "IT-PU" : true, "IT-PV" : true,
- "IT-PZ" : true, "IT-RA" : true, "IT-RC" : true, "IT-RE" : true, "IT-RG" : true,
- "IT-RI" : true, "IT-RM" : true, "IT-RN" : true, "IT-RO" : true, "IT-SA" : true,
- "IT-SI" : true, "IT-SO" : true, "IT-SP" : true, "IT-SR" : true, "IT-SS" : true,
- "IT-SV" : true, "IT-TA" : true, "IT-TE" : true, "IT-TN" : true, "IT-TO" : true,
- "IT-TP" : true, "IT-TR" : true, "IT-TS" : true, "IT-TV" : true, "IT-UD" : true,
- "IT-VA" : true, "IT-VB" : true, "IT-VC" : true, "IT-VE" : true, "IT-VI" : true,
- "IT-VR" : true, "IT-VS" : true, "IT-VT" : true, "IT-VV" : true, "JM-01" : true,
- "JM-02" : true, "JM-03" : true, "JM-04" : true, "JM-05" : true, "JM-06" : true,
- "JM-07" : true, "JM-08" : true, "JM-09" : true, "JM-10" : true, "JM-11" : true,
- "JM-12" : true, "JM-13" : true, "JM-14" : true, "JO-AJ" : true, "JO-AM" : true,
- "JO-AQ" : true, "JO-AT" : true, "JO-AZ" : true, "JO-BA" : true, "JO-IR" : true,
- "JO-JA" : true, "JO-KA" : true, "JO-MA" : true, "JO-MD" : true, "JO-MN" : true,
- "JP-01" : true, "JP-02" : true, "JP-03" : true, "JP-04" : true, "JP-05" : true,
- "JP-06" : true, "JP-07" : true, "JP-08" : true, "JP-09" : true, "JP-10" : true,
- "JP-11" : true, "JP-12" : true, "JP-13" : true, "JP-14" : true, "JP-15" : true,
- "JP-16" : true, "JP-17" : true, "JP-18" : true, "JP-19" : true, "JP-20" : true,
- "JP-21" : true, "JP-22" : true, "JP-23" : true, "JP-24" : true, "JP-25" : true,
- "JP-26" : true, "JP-27" : true, "JP-28" : true, "JP-29" : true, "JP-30" : true,
- "JP-31" : true, "JP-32" : true, "JP-33" : true, "JP-34" : true, "JP-35" : true,
- "JP-36" : true, "JP-37" : true, "JP-38" : true, "JP-39" : true, "JP-40" : true,
- "JP-41" : true, "JP-42" : true, "JP-43" : true, "JP-44" : true, "JP-45" : true,
- "JP-46" : true, "JP-47" : true, "KE-110" : true, "KE-200" : true, "KE-300" : true,
- "KE-400" : true, "KE-500" : true, "KE-700" : true, "KE-800" : true, "KG-B" : true,
- "KG-C" : true, "KG-GB" : true, "KG-J" : true, "KG-N" : true, "KG-O" : true,
- "KG-T" : true, "KG-Y" : true, "KH-1" : true, "KH-10" : true, "KH-11" : true,
- "KH-12" : true, "KH-13" : true, "KH-14" : true, "KH-15" : true, "KH-16" : true,
- "KH-17" : true, "KH-18" : true, "KH-19" : true, "KH-2" : true, "KH-20" : true,
- "KH-21" : true, "KH-22" : true, "KH-23" : true, "KH-24" : true, "KH-3" : true,
- "KH-4" : true, "KH-5" : true, "KH-6" : true, "KH-7" : true, "KH-8" : true,
- "KH-9" : true, "KI-G" : true, "KI-L" : true, "KI-P" : true, "KM-A" : true,
- "KM-G" : true, "KM-M" : true, "KN-01" : true, "KN-02" : true, "KN-03" : true,
- "KN-04" : true, "KN-05" : true, "KN-06" : true, "KN-07" : true, "KN-08" : true,
- "KN-09" : true, "KN-10" : true, "KN-11" : true, "KN-12" : true, "KN-13" : true,
- "KN-15" : true, "KN-K" : true, "KN-N" : true, "KP-01" : true, "KP-02" : true,
- "KP-03" : true, "KP-04" : true, "KP-05" : true, "KP-06" : true, "KP-07" : true,
- "KP-08" : true, "KP-09" : true, "KP-10" : true, "KP-13" : true, "KR-11" : true,
- "KR-26" : true, "KR-27" : true, "KR-28" : true, "KR-29" : true, "KR-30" : true,
- "KR-31" : true, "KR-41" : true, "KR-42" : true, "KR-43" : true, "KR-44" : true,
- "KR-45" : true, "KR-46" : true, "KR-47" : true, "KR-48" : true, "KR-49" : true,
- "KW-AH" : true, "KW-FA" : true, "KW-HA" : true, "KW-JA" : true, "KW-KU" : true,
- "KW-MU" : true, "KZ-AKM" : true, "KZ-AKT" : true, "KZ-ALA" : true, "KZ-ALM" : true,
- "KZ-AST" : true, "KZ-ATY" : true, "KZ-KAR" : true, "KZ-KUS" : true, "KZ-KZY" : true,
- "KZ-MAN" : true, "KZ-PAV" : true, "KZ-SEV" : true, "KZ-VOS" : true, "KZ-YUZ" : true,
- "KZ-ZAP" : true, "KZ-ZHA" : true, "LA-AT" : true, "LA-BK" : true, "LA-BL" : true,
- "LA-CH" : true, "LA-HO" : true, "LA-KH" : true, "LA-LM" : true, "LA-LP" : true,
- "LA-OU" : true, "LA-PH" : true, "LA-SL" : true, "LA-SV" : true, "LA-VI" : true,
- "LA-VT" : true, "LA-XA" : true, "LA-XE" : true, "LA-XI" : true, "LA-XS" : true,
- "LB-AK" : true, "LB-AS" : true, "LB-BA" : true, "LB-BH" : true, "LB-BI" : true,
- "LB-JA" : true, "LB-JL" : true, "LB-NA" : true, "LI-01" : true, "LI-02" : true,
- "LI-03" : true, "LI-04" : true, "LI-05" : true, "LI-06" : true, "LI-07" : true,
- "LI-08" : true, "LI-09" : true, "LI-10" : true, "LI-11" : true, "LK-1" : true,
- "LK-11" : true, "LK-12" : true, "LK-13" : true, "LK-2" : true, "LK-21" : true,
- "LK-22" : true, "LK-23" : true, "LK-3" : true, "LK-31" : true, "LK-32" : true,
- "LK-33" : true, "LK-4" : true, "LK-41" : true, "LK-42" : true, "LK-43" : true,
- "LK-44" : true, "LK-45" : true, "LK-5" : true, "LK-51" : true, "LK-52" : true,
- "LK-53" : true, "LK-6" : true, "LK-61" : true, "LK-62" : true, "LK-7" : true,
- "LK-71" : true, "LK-72" : true, "LK-8" : true, "LK-81" : true, "LK-82" : true,
- "LK-9" : true, "LK-91" : true, "LK-92" : true, "LR-BG" : true, "LR-BM" : true,
- "LR-CM" : true, "LR-GB" : true, "LR-GG" : true, "LR-GK" : true, "LR-LO" : true,
- "LR-MG" : true, "LR-MO" : true, "LR-MY" : true, "LR-NI" : true, "LR-RI" : true,
- "LR-SI" : true, "LS-A" : true, "LS-B" : true, "LS-C" : true, "LS-D" : true,
- "LS-E" : true, "LS-F" : true, "LS-G" : true, "LS-H" : true, "LS-J" : true,
- "LS-K" : true, "LT-AL" : true, "LT-KL" : true, "LT-KU" : true, "LT-MR" : true,
- "LT-PN" : true, "LT-SA" : true, "LT-TA" : true, "LT-TE" : true, "LT-UT" : true,
- "LT-VL" : true, "LU-D" : true, "LU-G" : true, "LU-L" : true, "LV-001" : true,
- "LV-002" : true, "LV-003" : true, "LV-004" : true, "LV-005" : true, "LV-006" : true,
- "LV-007" : true, "LV-008" : true, "LV-009" : true, "LV-010" : true, "LV-011" : true,
- "LV-012" : true, "LV-013" : true, "LV-014" : true, "LV-015" : true, "LV-016" : true,
- "LV-017" : true, "LV-018" : true, "LV-019" : true, "LV-020" : true, "LV-021" : true,
- "LV-022" : true, "LV-023" : true, "LV-024" : true, "LV-025" : true, "LV-026" : true,
- "LV-027" : true, "LV-028" : true, "LV-029" : true, "LV-030" : true, "LV-031" : true,
- "LV-032" : true, "LV-033" : true, "LV-034" : true, "LV-035" : true, "LV-036" : true,
- "LV-037" : true, "LV-038" : true, "LV-039" : true, "LV-040" : true, "LV-041" : true,
- "LV-042" : true, "LV-043" : true, "LV-044" : true, "LV-045" : true, "LV-046" : true,
- "LV-047" : true, "LV-048" : true, "LV-049" : true, "LV-050" : true, "LV-051" : true,
- "LV-052" : true, "LV-053" : true, "LV-054" : true, "LV-055" : true, "LV-056" : true,
- "LV-057" : true, "LV-058" : true, "LV-059" : true, "LV-060" : true, "LV-061" : true,
- "LV-062" : true, "LV-063" : true, "LV-064" : true, "LV-065" : true, "LV-066" : true,
- "LV-067" : true, "LV-068" : true, "LV-069" : true, "LV-070" : true, "LV-071" : true,
- "LV-072" : true, "LV-073" : true, "LV-074" : true, "LV-075" : true, "LV-076" : true,
- "LV-077" : true, "LV-078" : true, "LV-079" : true, "LV-080" : true, "LV-081" : true,
- "LV-082" : true, "LV-083" : true, "LV-084" : true, "LV-085" : true, "LV-086" : true,
- "LV-087" : true, "LV-088" : true, "LV-089" : true, "LV-090" : true, "LV-091" : true,
- "LV-092" : true, "LV-093" : true, "LV-094" : true, "LV-095" : true, "LV-096" : true,
- "LV-097" : true, "LV-098" : true, "LV-099" : true, "LV-100" : true, "LV-101" : true,
- "LV-102" : true, "LV-103" : true, "LV-104" : true, "LV-105" : true, "LV-106" : true,
- "LV-107" : true, "LV-108" : true, "LV-109" : true, "LV-110" : true, "LV-DGV" : true,
- "LV-JEL" : true, "LV-JKB" : true, "LV-JUR" : true, "LV-LPX" : true, "LV-REZ" : true,
- "LV-RIX" : true, "LV-VEN" : true, "LV-VMR" : true, "LY-BA" : true, "LY-BU" : true,
- "LY-DR" : true, "LY-GT" : true, "LY-JA" : true, "LY-JB" : true, "LY-JG" : true,
- "LY-JI" : true, "LY-JU" : true, "LY-KF" : true, "LY-MB" : true, "LY-MI" : true,
- "LY-MJ" : true, "LY-MQ" : true, "LY-NL" : true, "LY-NQ" : true, "LY-SB" : true,
- "LY-SR" : true, "LY-TB" : true, "LY-WA" : true, "LY-WD" : true, "LY-WS" : true,
- "LY-ZA" : true, "MA-01" : true, "MA-02" : true, "MA-03" : true, "MA-04" : true,
- "MA-05" : true, "MA-06" : true, "MA-07" : true, "MA-08" : true, "MA-09" : true,
- "MA-10" : true, "MA-11" : true, "MA-12" : true, "MA-13" : true, "MA-14" : true,
- "MA-15" : true, "MA-16" : true, "MA-AGD" : true, "MA-AOU" : true, "MA-ASZ" : true,
- "MA-AZI" : true, "MA-BEM" : true, "MA-BER" : true, "MA-BES" : true, "MA-BOD" : true,
- "MA-BOM" : true, "MA-CAS" : true, "MA-CHE" : true, "MA-CHI" : true, "MA-CHT" : true,
- "MA-ERR" : true, "MA-ESI" : true, "MA-ESM" : true, "MA-FAH" : true, "MA-FES" : true,
- "MA-FIG" : true, "MA-GUE" : true, "MA-HAJ" : true, "MA-HAO" : true, "MA-HOC" : true,
- "MA-IFR" : true, "MA-INE" : true, "MA-JDI" : true, "MA-JRA" : true, "MA-KEN" : true,
- "MA-KES" : true, "MA-KHE" : true, "MA-KHN" : true, "MA-KHO" : true, "MA-LAA" : true,
- "MA-LAR" : true, "MA-MED" : true, "MA-MEK" : true, "MA-MMD" : true, "MA-MMN" : true,
- "MA-MOH" : true, "MA-MOU" : true, "MA-NAD" : true, "MA-NOU" : true, "MA-OUA" : true,
- "MA-OUD" : true, "MA-OUJ" : true, "MA-RAB" : true, "MA-SAF" : true, "MA-SAL" : true,
- "MA-SEF" : true, "MA-SET" : true, "MA-SIK" : true, "MA-SKH" : true, "MA-SYB" : true,
- "MA-TAI" : true, "MA-TAO" : true, "MA-TAR" : true, "MA-TAT" : true, "MA-TAZ" : true,
- "MA-TET" : true, "MA-TIZ" : true, "MA-TNG" : true, "MA-TNT" : true, "MA-ZAG" : true,
- "MC-CL" : true, "MC-CO" : true, "MC-FO" : true, "MC-GA" : true, "MC-JE" : true,
- "MC-LA" : true, "MC-MA" : true, "MC-MC" : true, "MC-MG" : true, "MC-MO" : true,
- "MC-MU" : true, "MC-PH" : true, "MC-SD" : true, "MC-SO" : true, "MC-SP" : true,
- "MC-SR" : true, "MC-VR" : true, "MD-AN" : true, "MD-BA" : true, "MD-BD" : true,
- "MD-BR" : true, "MD-BS" : true, "MD-CA" : true, "MD-CL" : true, "MD-CM" : true,
- "MD-CR" : true, "MD-CS" : true, "MD-CT" : true, "MD-CU" : true, "MD-DO" : true,
- "MD-DR" : true, "MD-DU" : true, "MD-ED" : true, "MD-FA" : true, "MD-FL" : true,
- "MD-GA" : true, "MD-GL" : true, "MD-HI" : true, "MD-IA" : true, "MD-LE" : true,
- "MD-NI" : true, "MD-OC" : true, "MD-OR" : true, "MD-RE" : true, "MD-RI" : true,
- "MD-SD" : true, "MD-SI" : true, "MD-SN" : true, "MD-SO" : true, "MD-ST" : true,
- "MD-SV" : true, "MD-TA" : true, "MD-TE" : true, "MD-UN" : true, "ME-01" : true,
- "ME-02" : true, "ME-03" : true, "ME-04" : true, "ME-05" : true, "ME-06" : true,
- "ME-07" : true, "ME-08" : true, "ME-09" : true, "ME-10" : true, "ME-11" : true,
- "ME-12" : true, "ME-13" : true, "ME-14" : true, "ME-15" : true, "ME-16" : true,
- "ME-17" : true, "ME-18" : true, "ME-19" : true, "ME-20" : true, "ME-21" : true,
- "MG-A" : true, "MG-D" : true, "MG-F" : true, "MG-M" : true, "MG-T" : true,
- "MG-U" : true, "MH-ALK" : true, "MH-ALL" : true, "MH-ARN" : true, "MH-AUR" : true,
- "MH-EBO" : true, "MH-ENI" : true, "MH-JAB" : true, "MH-JAL" : true, "MH-KIL" : true,
- "MH-KWA" : true, "MH-L" : true, "MH-LAE" : true, "MH-LIB" : true, "MH-LIK" : true,
- "MH-MAJ" : true, "MH-MAL" : true, "MH-MEJ" : true, "MH-MIL" : true, "MH-NMK" : true,
- "MH-NMU" : true, "MH-RON" : true, "MH-T" : true, "MH-UJA" : true, "MH-UTI" : true,
- "MH-WTJ" : true, "MH-WTN" : true, "MK-01" : true, "MK-02" : true, "MK-03" : true,
- "MK-04" : true, "MK-05" : true, "MK-06" : true, "MK-07" : true, "MK-08" : true,
- "MK-09" : true, "MK-10" : true, "MK-11" : true, "MK-12" : true, "MK-13" : true,
- "MK-14" : true, "MK-15" : true, "MK-16" : true, "MK-17" : true, "MK-18" : true,
- "MK-19" : true, "MK-20" : true, "MK-21" : true, "MK-22" : true, "MK-23" : true,
- "MK-24" : true, "MK-25" : true, "MK-26" : true, "MK-27" : true, "MK-28" : true,
- "MK-29" : true, "MK-30" : true, "MK-31" : true, "MK-32" : true, "MK-33" : true,
- "MK-34" : true, "MK-35" : true, "MK-36" : true, "MK-37" : true, "MK-38" : true,
- "MK-39" : true, "MK-40" : true, "MK-41" : true, "MK-42" : true, "MK-43" : true,
- "MK-44" : true, "MK-45" : true, "MK-46" : true, "MK-47" : true, "MK-48" : true,
- "MK-49" : true, "MK-50" : true, "MK-51" : true, "MK-52" : true, "MK-53" : true,
- "MK-54" : true, "MK-55" : true, "MK-56" : true, "MK-57" : true, "MK-58" : true,
- "MK-59" : true, "MK-60" : true, "MK-61" : true, "MK-62" : true, "MK-63" : true,
- "MK-64" : true, "MK-65" : true, "MK-66" : true, "MK-67" : true, "MK-68" : true,
- "MK-69" : true, "MK-70" : true, "MK-71" : true, "MK-72" : true, "MK-73" : true,
- "MK-74" : true, "MK-75" : true, "MK-76" : true, "MK-77" : true, "MK-78" : true,
- "MK-79" : true, "MK-80" : true, "MK-81" : true, "MK-82" : true, "MK-83" : true,
- "MK-84" : true, "ML-1" : true, "ML-2" : true, "ML-3" : true, "ML-4" : true,
- "ML-5" : true, "ML-6" : true, "ML-7" : true, "ML-8" : true, "ML-BK0" : true,
- "MM-01" : true, "MM-02" : true, "MM-03" : true, "MM-04" : true, "MM-05" : true,
- "MM-06" : true, "MM-07" : true, "MM-11" : true, "MM-12" : true, "MM-13" : true,
- "MM-14" : true, "MM-15" : true, "MM-16" : true, "MM-17" : true, "MN-035" : true,
- "MN-037" : true, "MN-039" : true, "MN-041" : true, "MN-043" : true, "MN-046" : true,
- "MN-047" : true, "MN-049" : true, "MN-051" : true, "MN-053" : true, "MN-055" : true,
- "MN-057" : true, "MN-059" : true, "MN-061" : true, "MN-063" : true, "MN-064" : true,
- "MN-065" : true, "MN-067" : true, "MN-069" : true, "MN-071" : true, "MN-073" : true,
- "MN-1" : true, "MR-01" : true, "MR-02" : true, "MR-03" : true, "MR-04" : true,
- "MR-05" : true, "MR-06" : true, "MR-07" : true, "MR-08" : true, "MR-09" : true,
- "MR-10" : true, "MR-11" : true, "MR-12" : true, "MR-NKC" : true, "MT-01" : true,
- "MT-02" : true, "MT-03" : true, "MT-04" : true, "MT-05" : true, "MT-06" : true,
- "MT-07" : true, "MT-08" : true, "MT-09" : true, "MT-10" : true, "MT-11" : true,
- "MT-12" : true, "MT-13" : true, "MT-14" : true, "MT-15" : true, "MT-16" : true,
- "MT-17" : true, "MT-18" : true, "MT-19" : true, "MT-20" : true, "MT-21" : true,
- "MT-22" : true, "MT-23" : true, "MT-24" : true, "MT-25" : true, "MT-26" : true,
- "MT-27" : true, "MT-28" : true, "MT-29" : true, "MT-30" : true, "MT-31" : true,
- "MT-32" : true, "MT-33" : true, "MT-34" : true, "MT-35" : true, "MT-36" : true,
- "MT-37" : true, "MT-38" : true, "MT-39" : true, "MT-40" : true, "MT-41" : true,
- "MT-42" : true, "MT-43" : true, "MT-44" : true, "MT-45" : true, "MT-46" : true,
- "MT-47" : true, "MT-48" : true, "MT-49" : true, "MT-50" : true, "MT-51" : true,
- "MT-52" : true, "MT-53" : true, "MT-54" : true, "MT-55" : true, "MT-56" : true,
- "MT-57" : true, "MT-58" : true, "MT-59" : true, "MT-60" : true, "MT-61" : true,
- "MT-62" : true, "MT-63" : true, "MT-64" : true, "MT-65" : true, "MT-66" : true,
- "MT-67" : true, "MT-68" : true, "MU-AG" : true, "MU-BL" : true, "MU-BR" : true,
- "MU-CC" : true, "MU-CU" : true, "MU-FL" : true, "MU-GP" : true, "MU-MO" : true,
- "MU-PA" : true, "MU-PL" : true, "MU-PU" : true, "MU-PW" : true, "MU-QB" : true,
- "MU-RO" : true, "MU-RP" : true, "MU-SA" : true, "MU-VP" : true, "MV-00" : true,
- "MV-01" : true, "MV-02" : true, "MV-03" : true, "MV-04" : true, "MV-05" : true,
- "MV-07" : true, "MV-08" : true, "MV-12" : true, "MV-13" : true, "MV-14" : true,
- "MV-17" : true, "MV-20" : true, "MV-23" : true, "MV-24" : true, "MV-25" : true,
- "MV-26" : true, "MV-27" : true, "MV-28" : true, "MV-29" : true, "MV-CE" : true,
- "MV-MLE" : true, "MV-NC" : true, "MV-NO" : true, "MV-SC" : true, "MV-SU" : true,
- "MV-UN" : true, "MV-US" : true, "MW-BA" : true, "MW-BL" : true, "MW-C" : true,
- "MW-CK" : true, "MW-CR" : true, "MW-CT" : true, "MW-DE" : true, "MW-DO" : true,
- "MW-KR" : true, "MW-KS" : true, "MW-LI" : true, "MW-LK" : true, "MW-MC" : true,
- "MW-MG" : true, "MW-MH" : true, "MW-MU" : true, "MW-MW" : true, "MW-MZ" : true,
- "MW-N" : true, "MW-NB" : true, "MW-NE" : true, "MW-NI" : true, "MW-NK" : true,
- "MW-NS" : true, "MW-NU" : true, "MW-PH" : true, "MW-RU" : true, "MW-S" : true,
- "MW-SA" : true, "MW-TH" : true, "MW-ZO" : true, "MX-AGU" : true, "MX-BCN" : true,
- "MX-BCS" : true, "MX-CAM" : true, "MX-CHH" : true, "MX-CHP" : true, "MX-COA" : true,
- "MX-COL" : true, "MX-DIF" : true, "MX-DUR" : true, "MX-GRO" : true, "MX-GUA" : true,
- "MX-HID" : true, "MX-JAL" : true, "MX-MEX" : true, "MX-MIC" : true, "MX-MOR" : true,
- "MX-NAY" : true, "MX-NLE" : true, "MX-OAX" : true, "MX-PUE" : true, "MX-QUE" : true,
- "MX-ROO" : true, "MX-SIN" : true, "MX-SLP" : true, "MX-SON" : true, "MX-TAB" : true,
- "MX-TAM" : true, "MX-TLA" : true, "MX-VER" : true, "MX-YUC" : true, "MX-ZAC" : true,
- "MY-01" : true, "MY-02" : true, "MY-03" : true, "MY-04" : true, "MY-05" : true,
- "MY-06" : true, "MY-07" : true, "MY-08" : true, "MY-09" : true, "MY-10" : true,
- "MY-11" : true, "MY-12" : true, "MY-13" : true, "MY-14" : true, "MY-15" : true,
- "MY-16" : true, "MZ-A" : true, "MZ-B" : true, "MZ-G" : true, "MZ-I" : true,
- "MZ-L" : true, "MZ-MPM" : true, "MZ-N" : true, "MZ-P" : true, "MZ-Q" : true,
- "MZ-S" : true, "MZ-T" : true, "NA-CA" : true, "NA-ER" : true, "NA-HA" : true,
- "NA-KA" : true, "NA-KH" : true, "NA-KU" : true, "NA-OD" : true, "NA-OH" : true,
- "NA-OK" : true, "NA-ON" : true, "NA-OS" : true, "NA-OT" : true, "NA-OW" : true,
- "NE-1" : true, "NE-2" : true, "NE-3" : true, "NE-4" : true, "NE-5" : true,
- "NE-6" : true, "NE-7" : true, "NE-8" : true, "NG-AB" : true, "NG-AD" : true,
- "NG-AK" : true, "NG-AN" : true, "NG-BA" : true, "NG-BE" : true, "NG-BO" : true,
- "NG-BY" : true, "NG-CR" : true, "NG-DE" : true, "NG-EB" : true, "NG-ED" : true,
- "NG-EK" : true, "NG-EN" : true, "NG-FC" : true, "NG-GO" : true, "NG-IM" : true,
- "NG-JI" : true, "NG-KD" : true, "NG-KE" : true, "NG-KN" : true, "NG-KO" : true,
- "NG-KT" : true, "NG-KW" : true, "NG-LA" : true, "NG-NA" : true, "NG-NI" : true,
- "NG-OG" : true, "NG-ON" : true, "NG-OS" : true, "NG-OY" : true, "NG-PL" : true,
- "NG-RI" : true, "NG-SO" : true, "NG-TA" : true, "NG-YO" : true, "NG-ZA" : true,
- "NI-AN" : true, "NI-AS" : true, "NI-BO" : true, "NI-CA" : true, "NI-CI" : true,
- "NI-CO" : true, "NI-ES" : true, "NI-GR" : true, "NI-JI" : true, "NI-LE" : true,
- "NI-MD" : true, "NI-MN" : true, "NI-MS" : true, "NI-MT" : true, "NI-NS" : true,
- "NI-RI" : true, "NI-SJ" : true, "NL-AW" : true, "NL-BQ1" : true, "NL-BQ2" : true,
- "NL-BQ3" : true, "NL-CW" : true, "NL-DR" : true, "NL-FL" : true, "NL-FR" : true,
- "NL-GE" : true, "NL-GR" : true, "NL-LI" : true, "NL-NB" : true, "NL-NH" : true,
- "NL-OV" : true, "NL-SX" : true, "NL-UT" : true, "NL-ZE" : true, "NL-ZH" : true,
- "NO-01" : true, "NO-02" : true, "NO-03" : true, "NO-04" : true, "NO-05" : true,
- "NO-06" : true, "NO-07" : true, "NO-08" : true, "NO-09" : true, "NO-10" : true,
- "NO-11" : true, "NO-12" : true, "NO-14" : true, "NO-15" : true, "NO-16" : true,
- "NO-17" : true, "NO-18" : true, "NO-19" : true, "NO-20" : true, "NO-21" : true,
- "NO-22" : true, "NP-1" : true, "NP-2" : true, "NP-3" : true, "NP-4" : true,
- "NP-5" : true, "NP-BA" : true, "NP-BH" : true, "NP-DH" : true, "NP-GA" : true,
- "NP-JA" : true, "NP-KA" : true, "NP-KO" : true, "NP-LU" : true, "NP-MA" : true,
- "NP-ME" : true, "NP-NA" : true, "NP-RA" : true, "NP-SA" : true, "NP-SE" : true,
- "NR-01" : true, "NR-02" : true, "NR-03" : true, "NR-04" : true, "NR-05" : true,
- "NR-06" : true, "NR-07" : true, "NR-08" : true, "NR-09" : true, "NR-10" : true,
- "NR-11" : true, "NR-12" : true, "NR-13" : true, "NR-14" : true, "NZ-AUK" : true,
- "NZ-BOP" : true, "NZ-CAN" : true, "NZ-CIT" : true, "NZ-GIS" : true, "NZ-HKB" : true,
- "NZ-MBH" : true, "NZ-MWT" : true, "NZ-N" : true, "NZ-NSN" : true, "NZ-NTL" : true,
- "NZ-OTA" : true, "NZ-S" : true, "NZ-STL" : true, "NZ-TAS" : true, "NZ-TKI" : true,
- "NZ-WGN" : true, "NZ-WKO" : true, "NZ-WTC" : true, "OM-BA" : true, "OM-BU" : true,
- "OM-DA" : true, "OM-MA" : true, "OM-MU" : true, "OM-SH" : true, "OM-WU" : true,
- "OM-ZA" : true, "OM-ZU" : true, "PA-1" : true, "PA-2" : true, "PA-3" : true,
- "PA-4" : true, "PA-5" : true, "PA-6" : true, "PA-7" : true, "PA-8" : true,
- "PA-9" : true, "PA-EM" : true, "PA-KY" : true, "PA-NB" : true, "PE-AMA" : true,
- "PE-ANC" : true, "PE-APU" : true, "PE-ARE" : true, "PE-AYA" : true, "PE-CAJ" : true,
- "PE-CAL" : true, "PE-CUS" : true, "PE-HUC" : true, "PE-HUV" : true, "PE-ICA" : true,
- "PE-JUN" : true, "PE-LAL" : true, "PE-LAM" : true, "PE-LIM" : true, "PE-LMA" : true,
- "PE-LOR" : true, "PE-MDD" : true, "PE-MOQ" : true, "PE-PAS" : true, "PE-PIU" : true,
- "PE-PUN" : true, "PE-SAM" : true, "PE-TAC" : true, "PE-TUM" : true, "PE-UCA" : true,
- "PG-CPK" : true, "PG-CPM" : true, "PG-EBR" : true, "PG-EHG" : true, "PG-EPW" : true,
- "PG-ESW" : true, "PG-GPK" : true, "PG-MBA" : true, "PG-MPL" : true, "PG-MPM" : true,
- "PG-MRL" : true, "PG-NCD" : true, "PG-NIK" : true, "PG-NPP" : true, "PG-NSB" : true,
- "PG-SAN" : true, "PG-SHM" : true, "PG-WBK" : true, "PG-WHM" : true, "PG-WPD" : true,
- "PH-00" : true, "PH-01" : true, "PH-02" : true, "PH-03" : true, "PH-05" : true,
- "PH-06" : true, "PH-07" : true, "PH-08" : true, "PH-09" : true, "PH-10" : true,
- "PH-11" : true, "PH-12" : true, "PH-13" : true, "PH-14" : true, "PH-15" : true,
- "PH-40" : true, "PH-41" : true, "PH-ABR" : true, "PH-AGN" : true, "PH-AGS" : true,
- "PH-AKL" : true, "PH-ALB" : true, "PH-ANT" : true, "PH-APA" : true, "PH-AUR" : true,
- "PH-BAN" : true, "PH-BAS" : true, "PH-BEN" : true, "PH-BIL" : true, "PH-BOH" : true,
- "PH-BTG" : true, "PH-BTN" : true, "PH-BUK" : true, "PH-BUL" : true, "PH-CAG" : true,
- "PH-CAM" : true, "PH-CAN" : true, "PH-CAP" : true, "PH-CAS" : true, "PH-CAT" : true,
- "PH-CAV" : true, "PH-CEB" : true, "PH-COM" : true, "PH-DAO" : true, "PH-DAS" : true,
- "PH-DAV" : true, "PH-DIN" : true, "PH-EAS" : true, "PH-GUI" : true, "PH-IFU" : true,
- "PH-ILI" : true, "PH-ILN" : true, "PH-ILS" : true, "PH-ISA" : true, "PH-KAL" : true,
- "PH-LAG" : true, "PH-LAN" : true, "PH-LAS" : true, "PH-LEY" : true, "PH-LUN" : true,
- "PH-MAD" : true, "PH-MAG" : true, "PH-MAS" : true, "PH-MDC" : true, "PH-MDR" : true,
- "PH-MOU" : true, "PH-MSC" : true, "PH-MSR" : true, "PH-NCO" : true, "PH-NEC" : true,
- "PH-NER" : true, "PH-NSA" : true, "PH-NUE" : true, "PH-NUV" : true, "PH-PAM" : true,
- "PH-PAN" : true, "PH-PLW" : true, "PH-QUE" : true, "PH-QUI" : true, "PH-RIZ" : true,
- "PH-ROM" : true, "PH-SAR" : true, "PH-SCO" : true, "PH-SIG" : true, "PH-SLE" : true,
- "PH-SLU" : true, "PH-SOR" : true, "PH-SUK" : true, "PH-SUN" : true, "PH-SUR" : true,
- "PH-TAR" : true, "PH-TAW" : true, "PH-WSA" : true, "PH-ZAN" : true, "PH-ZAS" : true,
- "PH-ZMB" : true, "PH-ZSI" : true, "PK-BA" : true, "PK-GB" : true, "PK-IS" : true,
- "PK-JK" : true, "PK-KP" : true, "PK-PB" : true, "PK-SD" : true, "PK-TA" : true,
- "PL-DS" : true, "PL-KP" : true, "PL-LB" : true, "PL-LD" : true, "PL-LU" : true,
- "PL-MA" : true, "PL-MZ" : true, "PL-OP" : true, "PL-PD" : true, "PL-PK" : true,
- "PL-PM" : true, "PL-SK" : true, "PL-SL" : true, "PL-WN" : true, "PL-WP" : true,
- "PL-ZP" : true, "PS-BTH" : true, "PS-DEB" : true, "PS-GZA" : true, "PS-HBN" : true,
- "PS-JEM" : true, "PS-JEN" : true, "PS-JRH" : true, "PS-KYS" : true, "PS-NBS" : true,
- "PS-NGZ" : true, "PS-QQA" : true, "PS-RBH" : true, "PS-RFH" : true, "PS-SLT" : true,
- "PS-TBS" : true, "PS-TKM" : true, "PT-01" : true, "PT-02" : true, "PT-03" : true,
- "PT-04" : true, "PT-05" : true, "PT-06" : true, "PT-07" : true, "PT-08" : true,
- "PT-09" : true, "PT-10" : true, "PT-11" : true, "PT-12" : true, "PT-13" : true,
- "PT-14" : true, "PT-15" : true, "PT-16" : true, "PT-17" : true, "PT-18" : true,
- "PT-20" : true, "PT-30" : true, "PW-002" : true, "PW-004" : true, "PW-010" : true,
- "PW-050" : true, "PW-100" : true, "PW-150" : true, "PW-212" : true, "PW-214" : true,
- "PW-218" : true, "PW-222" : true, "PW-224" : true, "PW-226" : true, "PW-227" : true,
- "PW-228" : true, "PW-350" : true, "PW-370" : true, "PY-1" : true, "PY-10" : true,
- "PY-11" : true, "PY-12" : true, "PY-13" : true, "PY-14" : true, "PY-15" : true,
- "PY-16" : true, "PY-19" : true, "PY-2" : true, "PY-3" : true, "PY-4" : true,
- "PY-5" : true, "PY-6" : true, "PY-7" : true, "PY-8" : true, "PY-9" : true,
- "PY-ASU" : true, "QA-DA" : true, "QA-KH" : true, "QA-MS" : true, "QA-RA" : true,
- "QA-US" : true, "QA-WA" : true, "QA-ZA" : true, "RO-AB" : true, "RO-AG" : true,
- "RO-AR" : true, "RO-B" : true, "RO-BC" : true, "RO-BH" : true, "RO-BN" : true,
- "RO-BR" : true, "RO-BT" : true, "RO-BV" : true, "RO-BZ" : true, "RO-CJ" : true,
- "RO-CL" : true, "RO-CS" : true, "RO-CT" : true, "RO-CV" : true, "RO-DB" : true,
- "RO-DJ" : true, "RO-GJ" : true, "RO-GL" : true, "RO-GR" : true, "RO-HD" : true,
- "RO-HR" : true, "RO-IF" : true, "RO-IL" : true, "RO-IS" : true, "RO-MH" : true,
- "RO-MM" : true, "RO-MS" : true, "RO-NT" : true, "RO-OT" : true, "RO-PH" : true,
- "RO-SB" : true, "RO-SJ" : true, "RO-SM" : true, "RO-SV" : true, "RO-TL" : true,
- "RO-TM" : true, "RO-TR" : true, "RO-VL" : true, "RO-VN" : true, "RO-VS" : true,
- "RS-00" : true, "RS-01" : true, "RS-02" : true, "RS-03" : true, "RS-04" : true,
- "RS-05" : true, "RS-06" : true, "RS-07" : true, "RS-08" : true, "RS-09" : true,
- "RS-10" : true, "RS-11" : true, "RS-12" : true, "RS-13" : true, "RS-14" : true,
- "RS-15" : true, "RS-16" : true, "RS-17" : true, "RS-18" : true, "RS-19" : true,
- "RS-20" : true, "RS-21" : true, "RS-22" : true, "RS-23" : true, "RS-24" : true,
- "RS-25" : true, "RS-26" : true, "RS-27" : true, "RS-28" : true, "RS-29" : true,
- "RS-KM" : true, "RS-VO" : true, "RU-AD" : true, "RU-AL" : true, "RU-ALT" : true,
- "RU-AMU" : true, "RU-ARK" : true, "RU-AST" : true, "RU-BA" : true, "RU-BEL" : true,
- "RU-BRY" : true, "RU-BU" : true, "RU-CE" : true, "RU-CHE" : true, "RU-CHU" : true,
- "RU-CU" : true, "RU-DA" : true, "RU-IN" : true, "RU-IRK" : true, "RU-IVA" : true,
- "RU-KAM" : true, "RU-KB" : true, "RU-KC" : true, "RU-KDA" : true, "RU-KEM" : true,
- "RU-KGD" : true, "RU-KGN" : true, "RU-KHA" : true, "RU-KHM" : true, "RU-KIR" : true,
- "RU-KK" : true, "RU-KL" : true, "RU-KLU" : true, "RU-KO" : true, "RU-KOS" : true,
- "RU-KR" : true, "RU-KRS" : true, "RU-KYA" : true, "RU-LEN" : true, "RU-LIP" : true,
- "RU-MAG" : true, "RU-ME" : true, "RU-MO" : true, "RU-MOS" : true, "RU-MOW" : true,
- "RU-MUR" : true, "RU-NEN" : true, "RU-NGR" : true, "RU-NIZ" : true, "RU-NVS" : true,
- "RU-OMS" : true, "RU-ORE" : true, "RU-ORL" : true, "RU-PER" : true, "RU-PNZ" : true,
- "RU-PRI" : true, "RU-PSK" : true, "RU-ROS" : true, "RU-RYA" : true, "RU-SA" : true,
- "RU-SAK" : true, "RU-SAM" : true, "RU-SAR" : true, "RU-SE" : true, "RU-SMO" : true,
- "RU-SPE" : true, "RU-STA" : true, "RU-SVE" : true, "RU-TA" : true, "RU-TAM" : true,
- "RU-TOM" : true, "RU-TUL" : true, "RU-TVE" : true, "RU-TY" : true, "RU-TYU" : true,
- "RU-UD" : true, "RU-ULY" : true, "RU-VGG" : true, "RU-VLA" : true, "RU-VLG" : true,
- "RU-VOR" : true, "RU-YAN" : true, "RU-YAR" : true, "RU-YEV" : true, "RU-ZAB" : true,
- "RW-01" : true, "RW-02" : true, "RW-03" : true, "RW-04" : true, "RW-05" : true,
- "SA-01" : true, "SA-02" : true, "SA-03" : true, "SA-04" : true, "SA-05" : true,
- "SA-06" : true, "SA-07" : true, "SA-08" : true, "SA-09" : true, "SA-10" : true,
- "SA-11" : true, "SA-12" : true, "SA-14" : true, "SB-CE" : true, "SB-CH" : true,
- "SB-CT" : true, "SB-GU" : true, "SB-IS" : true, "SB-MK" : true, "SB-ML" : true,
- "SB-RB" : true, "SB-TE" : true, "SB-WE" : true, "SC-01" : true, "SC-02" : true,
- "SC-03" : true, "SC-04" : true, "SC-05" : true, "SC-06" : true, "SC-07" : true,
- "SC-08" : true, "SC-09" : true, "SC-10" : true, "SC-11" : true, "SC-12" : true,
- "SC-13" : true, "SC-14" : true, "SC-15" : true, "SC-16" : true, "SC-17" : true,
- "SC-18" : true, "SC-19" : true, "SC-20" : true, "SC-21" : true, "SC-22" : true,
- "SC-23" : true, "SC-24" : true, "SC-25" : true, "SD-DC" : true, "SD-DE" : true,
- "SD-DN" : true, "SD-DS" : true, "SD-DW" : true, "SD-GD" : true, "SD-GZ" : true,
- "SD-KA" : true, "SD-KH" : true, "SD-KN" : true, "SD-KS" : true, "SD-NB" : true,
- "SD-NO" : true, "SD-NR" : true, "SD-NW" : true, "SD-RS" : true, "SD-SI" : true,
- "SE-AB" : true, "SE-AC" : true, "SE-BD" : true, "SE-C" : true, "SE-D" : true,
- "SE-E" : true, "SE-F" : true, "SE-G" : true, "SE-H" : true, "SE-I" : true,
- "SE-K" : true, "SE-M" : true, "SE-N" : true, "SE-O" : true, "SE-S" : true,
- "SE-T" : true, "SE-U" : true, "SE-W" : true, "SE-X" : true, "SE-Y" : true,
- "SE-Z" : true, "SG-01" : true, "SG-02" : true, "SG-03" : true, "SG-04" : true,
- "SG-05" : true, "SH-AC" : true, "SH-HL" : true, "SH-TA" : true, "SI-001" : true,
- "SI-002" : true, "SI-003" : true, "SI-004" : true, "SI-005" : true, "SI-006" : true,
- "SI-007" : true, "SI-008" : true, "SI-009" : true, "SI-010" : true, "SI-011" : true,
- "SI-012" : true, "SI-013" : true, "SI-014" : true, "SI-015" : true, "SI-016" : true,
- "SI-017" : true, "SI-018" : true, "SI-019" : true, "SI-020" : true, "SI-021" : true,
- "SI-022" : true, "SI-023" : true, "SI-024" : true, "SI-025" : true, "SI-026" : true,
- "SI-027" : true, "SI-028" : true, "SI-029" : true, "SI-030" : true, "SI-031" : true,
- "SI-032" : true, "SI-033" : true, "SI-034" : true, "SI-035" : true, "SI-036" : true,
- "SI-037" : true, "SI-038" : true, "SI-039" : true, "SI-040" : true, "SI-041" : true,
- "SI-042" : true, "SI-043" : true, "SI-044" : true, "SI-045" : true, "SI-046" : true,
- "SI-047" : true, "SI-048" : true, "SI-049" : true, "SI-050" : true, "SI-051" : true,
- "SI-052" : true, "SI-053" : true, "SI-054" : true, "SI-055" : true, "SI-056" : true,
- "SI-057" : true, "SI-058" : true, "SI-059" : true, "SI-060" : true, "SI-061" : true,
- "SI-062" : true, "SI-063" : true, "SI-064" : true, "SI-065" : true, "SI-066" : true,
- "SI-067" : true, "SI-068" : true, "SI-069" : true, "SI-070" : true, "SI-071" : true,
- "SI-072" : true, "SI-073" : true, "SI-074" : true, "SI-075" : true, "SI-076" : true,
- "SI-077" : true, "SI-078" : true, "SI-079" : true, "SI-080" : true, "SI-081" : true,
- "SI-082" : true, "SI-083" : true, "SI-084" : true, "SI-085" : true, "SI-086" : true,
- "SI-087" : true, "SI-088" : true, "SI-089" : true, "SI-090" : true, "SI-091" : true,
- "SI-092" : true, "SI-093" : true, "SI-094" : true, "SI-095" : true, "SI-096" : true,
- "SI-097" : true, "SI-098" : true, "SI-099" : true, "SI-100" : true, "SI-101" : true,
- "SI-102" : true, "SI-103" : true, "SI-104" : true, "SI-105" : true, "SI-106" : true,
- "SI-107" : true, "SI-108" : true, "SI-109" : true, "SI-110" : true, "SI-111" : true,
- "SI-112" : true, "SI-113" : true, "SI-114" : true, "SI-115" : true, "SI-116" : true,
- "SI-117" : true, "SI-118" : true, "SI-119" : true, "SI-120" : true, "SI-121" : true,
- "SI-122" : true, "SI-123" : true, "SI-124" : true, "SI-125" : true, "SI-126" : true,
- "SI-127" : true, "SI-128" : true, "SI-129" : true, "SI-130" : true, "SI-131" : true,
- "SI-132" : true, "SI-133" : true, "SI-134" : true, "SI-135" : true, "SI-136" : true,
- "SI-137" : true, "SI-138" : true, "SI-139" : true, "SI-140" : true, "SI-141" : true,
- "SI-142" : true, "SI-143" : true, "SI-144" : true, "SI-146" : true, "SI-147" : true,
- "SI-148" : true, "SI-149" : true, "SI-150" : true, "SI-151" : true, "SI-152" : true,
- "SI-153" : true, "SI-154" : true, "SI-155" : true, "SI-156" : true, "SI-157" : true,
- "SI-158" : true, "SI-159" : true, "SI-160" : true, "SI-161" : true, "SI-162" : true,
- "SI-163" : true, "SI-164" : true, "SI-165" : true, "SI-166" : true, "SI-167" : true,
- "SI-168" : true, "SI-169" : true, "SI-170" : true, "SI-171" : true, "SI-172" : true,
- "SI-173" : true, "SI-174" : true, "SI-175" : true, "SI-176" : true, "SI-177" : true,
- "SI-178" : true, "SI-179" : true, "SI-180" : true, "SI-181" : true, "SI-182" : true,
- "SI-183" : true, "SI-184" : true, "SI-185" : true, "SI-186" : true, "SI-187" : true,
- "SI-188" : true, "SI-189" : true, "SI-190" : true, "SI-191" : true, "SI-192" : true,
- "SI-193" : true, "SI-194" : true, "SI-195" : true, "SI-196" : true, "SI-197" : true,
- "SI-198" : true, "SI-199" : true, "SI-200" : true, "SI-201" : true, "SI-202" : true,
- "SI-203" : true, "SI-204" : true, "SI-205" : true, "SI-206" : true, "SI-207" : true,
- "SI-208" : true, "SI-209" : true, "SI-210" : true, "SI-211" : true, "SK-BC" : true,
- "SK-BL" : true, "SK-KI" : true, "SK-NI" : true, "SK-PV" : true, "SK-TA" : true,
- "SK-TC" : true, "SK-ZI" : true, "SL-E" : true, "SL-N" : true, "SL-S" : true,
- "SL-W" : true, "SM-01" : true, "SM-02" : true, "SM-03" : true, "SM-04" : true,
- "SM-05" : true, "SM-06" : true, "SM-07" : true, "SM-08" : true, "SM-09" : true,
- "SN-DB" : true, "SN-DK" : true, "SN-FK" : true, "SN-KA" : true, "SN-KD" : true,
- "SN-KE" : true, "SN-KL" : true, "SN-LG" : true, "SN-MT" : true, "SN-SE" : true,
- "SN-SL" : true, "SN-TC" : true, "SN-TH" : true, "SN-ZG" : true, "SO-AW" : true,
- "SO-BK" : true, "SO-BN" : true, "SO-BR" : true, "SO-BY" : true, "SO-GA" : true,
- "SO-GE" : true, "SO-HI" : true, "SO-JD" : true, "SO-JH" : true, "SO-MU" : true,
- "SO-NU" : true, "SO-SA" : true, "SO-SD" : true, "SO-SH" : true, "SO-SO" : true,
- "SO-TO" : true, "SO-WO" : true, "SR-BR" : true, "SR-CM" : true, "SR-CR" : true,
- "SR-MA" : true, "SR-NI" : true, "SR-PM" : true, "SR-PR" : true, "SR-SA" : true,
- "SR-SI" : true, "SR-WA" : true, "SS-BN" : true, "SS-BW" : true, "SS-EC" : true,
- "SS-EE8" : true, "SS-EW" : true, "SS-JG" : true, "SS-LK" : true, "SS-NU" : true,
- "SS-UY" : true, "SS-WR" : true, "ST-P" : true, "ST-S" : true, "SV-AH" : true,
- "SV-CA" : true, "SV-CH" : true, "SV-CU" : true, "SV-LI" : true, "SV-MO" : true,
- "SV-PA" : true, "SV-SA" : true, "SV-SM" : true, "SV-SO" : true, "SV-SS" : true,
- "SV-SV" : true, "SV-UN" : true, "SV-US" : true, "SY-DI" : true, "SY-DR" : true,
- "SY-DY" : true, "SY-HA" : true, "SY-HI" : true, "SY-HL" : true, "SY-HM" : true,
- "SY-ID" : true, "SY-LA" : true, "SY-QU" : true, "SY-RA" : true, "SY-RD" : true,
- "SY-SU" : true, "SY-TA" : true, "SZ-HH" : true, "SZ-LU" : true, "SZ-MA" : true,
- "SZ-SH" : true, "TD-BA" : true, "TD-BG" : true, "TD-BO" : true, "TD-CB" : true,
- "TD-EN" : true, "TD-GR" : true, "TD-HL" : true, "TD-KA" : true, "TD-LC" : true,
- "TD-LO" : true, "TD-LR" : true, "TD-MA" : true, "TD-MC" : true, "TD-ME" : true,
- "TD-MO" : true, "TD-ND" : true, "TD-OD" : true, "TD-SA" : true, "TD-SI" : true,
- "TD-TA" : true, "TD-TI" : true, "TD-WF" : true, "TG-C" : true, "TG-K" : true,
- "TG-M" : true, "TG-P" : true, "TG-S" : true, "TH-10" : true, "TH-11" : true,
- "TH-12" : true, "TH-13" : true, "TH-14" : true, "TH-15" : true, "TH-16" : true,
- "TH-17" : true, "TH-18" : true, "TH-19" : true, "TH-20" : true, "TH-21" : true,
- "TH-22" : true, "TH-23" : true, "TH-24" : true, "TH-25" : true, "TH-26" : true,
- "TH-27" : true, "TH-30" : true, "TH-31" : true, "TH-32" : true, "TH-33" : true,
- "TH-34" : true, "TH-35" : true, "TH-36" : true, "TH-37" : true, "TH-39" : true,
- "TH-40" : true, "TH-41" : true, "TH-42" : true, "TH-43" : true, "TH-44" : true,
- "TH-45" : true, "TH-46" : true, "TH-47" : true, "TH-48" : true, "TH-49" : true,
- "TH-50" : true, "TH-51" : true, "TH-52" : true, "TH-53" : true, "TH-54" : true,
- "TH-55" : true, "TH-56" : true, "TH-57" : true, "TH-58" : true, "TH-60" : true,
- "TH-61" : true, "TH-62" : true, "TH-63" : true, "TH-64" : true, "TH-65" : true,
- "TH-66" : true, "TH-67" : true, "TH-70" : true, "TH-71" : true, "TH-72" : true,
- "TH-73" : true, "TH-74" : true, "TH-75" : true, "TH-76" : true, "TH-77" : true,
- "TH-80" : true, "TH-81" : true, "TH-82" : true, "TH-83" : true, "TH-84" : true,
- "TH-85" : true, "TH-86" : true, "TH-90" : true, "TH-91" : true, "TH-92" : true,
- "TH-93" : true, "TH-94" : true, "TH-95" : true, "TH-96" : true, "TH-S" : true,
- "TJ-GB" : true, "TJ-KT" : true, "TJ-SU" : true, "TL-AL" : true, "TL-AN" : true,
- "TL-BA" : true, "TL-BO" : true, "TL-CO" : true, "TL-DI" : true, "TL-ER" : true,
- "TL-LA" : true, "TL-LI" : true, "TL-MF" : true, "TL-MT" : true, "TL-OE" : true,
- "TL-VI" : true, "TM-A" : true, "TM-B" : true, "TM-D" : true, "TM-L" : true,
- "TM-M" : true, "TM-S" : true, "TN-11" : true, "TN-12" : true, "TN-13" : true,
- "TN-14" : true, "TN-21" : true, "TN-22" : true, "TN-23" : true, "TN-31" : true,
- "TN-32" : true, "TN-33" : true, "TN-34" : true, "TN-41" : true, "TN-42" : true,
- "TN-43" : true, "TN-51" : true, "TN-52" : true, "TN-53" : true, "TN-61" : true,
- "TN-71" : true, "TN-72" : true, "TN-73" : true, "TN-81" : true, "TN-82" : true,
- "TN-83" : true, "TO-01" : true, "TO-02" : true, "TO-03" : true, "TO-04" : true,
- "TO-05" : true, "TR-01" : true, "TR-02" : true, "TR-03" : true, "TR-04" : true,
- "TR-05" : true, "TR-06" : true, "TR-07" : true, "TR-08" : true, "TR-09" : true,
- "TR-10" : true, "TR-11" : true, "TR-12" : true, "TR-13" : true, "TR-14" : true,
- "TR-15" : true, "TR-16" : true, "TR-17" : true, "TR-18" : true, "TR-19" : true,
- "TR-20" : true, "TR-21" : true, "TR-22" : true, "TR-23" : true, "TR-24" : true,
- "TR-25" : true, "TR-26" : true, "TR-27" : true, "TR-28" : true, "TR-29" : true,
- "TR-30" : true, "TR-31" : true, "TR-32" : true, "TR-33" : true, "TR-34" : true,
- "TR-35" : true, "TR-36" : true, "TR-37" : true, "TR-38" : true, "TR-39" : true,
- "TR-40" : true, "TR-41" : true, "TR-42" : true, "TR-43" : true, "TR-44" : true,
- "TR-45" : true, "TR-46" : true, "TR-47" : true, "TR-48" : true, "TR-49" : true,
- "TR-50" : true, "TR-51" : true, "TR-52" : true, "TR-53" : true, "TR-54" : true,
- "TR-55" : true, "TR-56" : true, "TR-57" : true, "TR-58" : true, "TR-59" : true,
- "TR-60" : true, "TR-61" : true, "TR-62" : true, "TR-63" : true, "TR-64" : true,
- "TR-65" : true, "TR-66" : true, "TR-67" : true, "TR-68" : true, "TR-69" : true,
- "TR-70" : true, "TR-71" : true, "TR-72" : true, "TR-73" : true, "TR-74" : true,
- "TR-75" : true, "TR-76" : true, "TR-77" : true, "TR-78" : true, "TR-79" : true,
- "TR-80" : true, "TR-81" : true, "TT-ARI" : true, "TT-CHA" : true, "TT-CTT" : true,
- "TT-DMN" : true, "TT-ETO" : true, "TT-PED" : true, "TT-POS" : true, "TT-PRT" : true,
- "TT-PTF" : true, "TT-RCM" : true, "TT-SFO" : true, "TT-SGE" : true, "TT-SIP" : true,
- "TT-SJL" : true, "TT-TUP" : true, "TT-WTO" : true, "TV-FUN" : true, "TV-NIT" : true,
- "TV-NKF" : true, "TV-NKL" : true, "TV-NMA" : true, "TV-NMG" : true, "TV-NUI" : true,
- "TV-VAI" : true, "TW-CHA" : true, "TW-CYI" : true, "TW-CYQ" : true, "TW-HSQ" : true,
- "TW-HSZ" : true, "TW-HUA" : true, "TW-ILA" : true, "TW-KEE" : true, "TW-KHH" : true,
- "TW-KHQ" : true, "TW-MIA" : true, "TW-NAN" : true, "TW-PEN" : true, "TW-PIF" : true,
- "TW-TAO" : true, "TW-TNN" : true, "TW-TNQ" : true, "TW-TPE" : true, "TW-TPQ" : true,
- "TW-TTT" : true, "TW-TXG" : true, "TW-TXQ" : true, "TW-YUN" : true, "TZ-01" : true,
- "TZ-02" : true, "TZ-03" : true, "TZ-04" : true, "TZ-05" : true, "TZ-06" : true,
- "TZ-07" : true, "TZ-08" : true, "TZ-09" : true, "TZ-10" : true, "TZ-11" : true,
- "TZ-12" : true, "TZ-13" : true, "TZ-14" : true, "TZ-15" : true, "TZ-16" : true,
- "TZ-17" : true, "TZ-18" : true, "TZ-19" : true, "TZ-20" : true, "TZ-21" : true,
- "TZ-22" : true, "TZ-23" : true, "TZ-24" : true, "TZ-25" : true, "TZ-26" : true,
- "UA-05" : true, "UA-07" : true, "UA-09" : true, "UA-12" : true, "UA-14" : true,
- "UA-18" : true, "UA-21" : true, "UA-23" : true, "UA-26" : true, "UA-30" : true,
- "UA-32" : true, "UA-35" : true, "UA-40" : true, "UA-43" : true, "UA-46" : true,
- "UA-48" : true, "UA-51" : true, "UA-53" : true, "UA-56" : true, "UA-59" : true,
- "UA-61" : true, "UA-63" : true, "UA-65" : true, "UA-68" : true, "UA-71" : true,
- "UA-74" : true, "UA-77" : true, "UG-101" : true, "UG-102" : true, "UG-103" : true,
- "UG-104" : true, "UG-105" : true, "UG-106" : true, "UG-107" : true, "UG-108" : true,
- "UG-109" : true, "UG-110" : true, "UG-111" : true, "UG-112" : true, "UG-113" : true,
- "UG-114" : true, "UG-115" : true, "UG-116" : true, "UG-201" : true, "UG-202" : true,
- "UG-203" : true, "UG-204" : true, "UG-205" : true, "UG-206" : true, "UG-207" : true,
- "UG-208" : true, "UG-209" : true, "UG-210" : true, "UG-211" : true, "UG-212" : true,
- "UG-213" : true, "UG-214" : true, "UG-215" : true, "UG-216" : true, "UG-217" : true,
- "UG-218" : true, "UG-219" : true, "UG-220" : true, "UG-221" : true, "UG-222" : true,
- "UG-223" : true, "UG-224" : true, "UG-301" : true, "UG-302" : true, "UG-303" : true,
- "UG-304" : true, "UG-305" : true, "UG-306" : true, "UG-307" : true, "UG-308" : true,
- "UG-309" : true, "UG-310" : true, "UG-311" : true, "UG-312" : true, "UG-313" : true,
- "UG-314" : true, "UG-315" : true, "UG-316" : true, "UG-317" : true, "UG-318" : true,
- "UG-319" : true, "UG-320" : true, "UG-321" : true, "UG-401" : true, "UG-402" : true,
- "UG-403" : true, "UG-404" : true, "UG-405" : true, "UG-406" : true, "UG-407" : true,
- "UG-408" : true, "UG-409" : true, "UG-410" : true, "UG-411" : true, "UG-412" : true,
- "UG-413" : true, "UG-414" : true, "UG-415" : true, "UG-416" : true, "UG-417" : true,
- "UG-418" : true, "UG-419" : true, "UG-C" : true, "UG-E" : true, "UG-N" : true,
- "UG-W" : true, "UM-67" : true, "UM-71" : true, "UM-76" : true, "UM-79" : true,
- "UM-81" : true, "UM-84" : true, "UM-86" : true, "UM-89" : true, "UM-95" : true,
- "US-AK" : true, "US-AL" : true, "US-AR" : true, "US-AS" : true, "US-AZ" : true,
- "US-CA" : true, "US-CO" : true, "US-CT" : true, "US-DC" : true, "US-DE" : true,
- "US-FL" : true, "US-GA" : true, "US-GU" : true, "US-HI" : true, "US-IA" : true,
- "US-ID" : true, "US-IL" : true, "US-IN" : true, "US-KS" : true, "US-KY" : true,
- "US-LA" : true, "US-MA" : true, "US-MD" : true, "US-ME" : true, "US-MI" : true,
- "US-MN" : true, "US-MO" : true, "US-MP" : true, "US-MS" : true, "US-MT" : true,
- "US-NC" : true, "US-ND" : true, "US-NE" : true, "US-NH" : true, "US-NJ" : true,
- "US-NM" : true, "US-NV" : true, "US-NY" : true, "US-OH" : true, "US-OK" : true,
- "US-OR" : true, "US-PA" : true, "US-PR" : true, "US-RI" : true, "US-SC" : true,
- "US-SD" : true, "US-TN" : true, "US-TX" : true, "US-UM" : true, "US-UT" : true,
- "US-VA" : true, "US-VI" : true, "US-VT" : true, "US-WA" : true, "US-WI" : true,
- "US-WV" : true, "US-WY" : true, "UY-AR" : true, "UY-CA" : true, "UY-CL" : true,
- "UY-CO" : true, "UY-DU" : true, "UY-FD" : true, "UY-FS" : true, "UY-LA" : true,
- "UY-MA" : true, "UY-MO" : true, "UY-PA" : true, "UY-RN" : true, "UY-RO" : true,
- "UY-RV" : true, "UY-SA" : true, "UY-SJ" : true, "UY-SO" : true, "UY-TA" : true,
- "UY-TT" : true, "UZ-AN" : true, "UZ-BU" : true, "UZ-FA" : true, "UZ-JI" : true,
- "UZ-NG" : true, "UZ-NW" : true, "UZ-QA" : true, "UZ-QR" : true, "UZ-SA" : true,
- "UZ-SI" : true, "UZ-SU" : true, "UZ-TK" : true, "UZ-TO" : true, "UZ-XO" : true,
- "VC-01" : true, "VC-02" : true, "VC-03" : true, "VC-04" : true, "VC-05" : true,
- "VC-06" : true, "VE-A" : true, "VE-B" : true, "VE-C" : true, "VE-D" : true,
- "VE-E" : true, "VE-F" : true, "VE-G" : true, "VE-H" : true, "VE-I" : true,
- "VE-J" : true, "VE-K" : true, "VE-L" : true, "VE-M" : true, "VE-N" : true,
- "VE-O" : true, "VE-P" : true, "VE-R" : true, "VE-S" : true, "VE-T" : true,
- "VE-U" : true, "VE-V" : true, "VE-W" : true, "VE-X" : true, "VE-Y" : true,
- "VE-Z" : true, "VN-01" : true, "VN-02" : true, "VN-03" : true, "VN-04" : true,
- "VN-05" : true, "VN-06" : true, "VN-07" : true, "VN-09" : true, "VN-13" : true,
- "VN-14" : true, "VN-15" : true, "VN-18" : true, "VN-20" : true, "VN-21" : true,
- "VN-22" : true, "VN-23" : true, "VN-24" : true, "VN-25" : true, "VN-26" : true,
- "VN-27" : true, "VN-28" : true, "VN-29" : true, "VN-30" : true, "VN-31" : true,
- "VN-32" : true, "VN-33" : true, "VN-34" : true, "VN-35" : true, "VN-36" : true,
- "VN-37" : true, "VN-39" : true, "VN-40" : true, "VN-41" : true, "VN-43" : true,
- "VN-44" : true, "VN-45" : true, "VN-46" : true, "VN-47" : true, "VN-49" : true,
- "VN-50" : true, "VN-51" : true, "VN-52" : true, "VN-53" : true, "VN-54" : true,
- "VN-55" : true, "VN-56" : true, "VN-57" : true, "VN-58" : true, "VN-59" : true,
- "VN-61" : true, "VN-63" : true, "VN-66" : true, "VN-67" : true, "VN-68" : true,
- "VN-69" : true, "VN-70" : true, "VN-71" : true, "VN-72" : true, "VN-73" : true,
- "VN-CT" : true, "VN-DN" : true, "VN-HN" : true, "VN-HP" : true, "VN-SG" : true,
- "VU-MAP" : true, "VU-PAM" : true, "VU-SAM" : true, "VU-SEE" : true, "VU-TAE" : true,
- "VU-TOB" : true, "WS-AA" : true, "WS-AL" : true, "WS-AT" : true, "WS-FA" : true,
- "WS-GE" : true, "WS-GI" : true, "WS-PA" : true, "WS-SA" : true, "WS-TU" : true,
- "WS-VF" : true, "WS-VS" : true, "YE-AB" : true, "YE-AD" : true, "YE-AM" : true,
- "YE-BA" : true, "YE-DA" : true, "YE-DH" : true, "YE-HD" : true, "YE-HJ" : true,
- "YE-IB" : true, "YE-JA" : true, "YE-LA" : true, "YE-MA" : true, "YE-MR" : true,
- "YE-MU" : true, "YE-MW" : true, "YE-RA" : true, "YE-SD" : true, "YE-SH" : true,
- "YE-SN" : true, "YE-TA" : true, "ZA-EC" : true, "ZA-FS" : true, "ZA-GP" : true,
- "ZA-LP" : true, "ZA-MP" : true, "ZA-NC" : true, "ZA-NW" : true, "ZA-WC" : true,
- "ZA-ZN" : true, "ZM-01" : true, "ZM-02" : true, "ZM-03" : true, "ZM-04" : true,
- "ZM-05" : true, "ZM-06" : true, "ZM-07" : true, "ZM-08" : true, "ZM-09" : true,
- "ZW-BU" : true, "ZW-HA" : true, "ZW-MA" : true, "ZW-MC" : true, "ZW-ME" : true,
- "ZW-MI" : true, "ZW-MN" : true, "ZW-MS" : true, "ZW-MV" : true, "ZW-MW" : true,
-}
diff --git a/vendor/github.com/go-playground/validator/v10/currency_codes.go b/vendor/github.com/go-playground/validator/v10/currency_codes.go
deleted file mode 100644
index a5cd9b18..00000000
--- a/vendor/github.com/go-playground/validator/v10/currency_codes.go
+++ /dev/null
@@ -1,79 +0,0 @@
-package validator
-
-var iso4217 = map[string]bool{
- "AFN": true, "EUR": true, "ALL": true, "DZD": true, "USD": true,
- "AOA": true, "XCD": true, "ARS": true, "AMD": true, "AWG": true,
- "AUD": true, "AZN": true, "BSD": true, "BHD": true, "BDT": true,
- "BBD": true, "BYN": true, "BZD": true, "XOF": true, "BMD": true,
- "INR": true, "BTN": true, "BOB": true, "BOV": true, "BAM": true,
- "BWP": true, "NOK": true, "BRL": true, "BND": true, "BGN": true,
- "BIF": true, "CVE": true, "KHR": true, "XAF": true, "CAD": true,
- "KYD": true, "CLP": true, "CLF": true, "CNY": true, "COP": true,
- "COU": true, "KMF": true, "CDF": true, "NZD": true, "CRC": true,
- "HRK": true, "CUP": true, "CUC": true, "ANG": true, "CZK": true,
- "DKK": true, "DJF": true, "DOP": true, "EGP": true, "SVC": true,
- "ERN": true, "SZL": true, "ETB": true, "FKP": true, "FJD": true,
- "XPF": true, "GMD": true, "GEL": true, "GHS": true, "GIP": true,
- "GTQ": true, "GBP": true, "GNF": true, "GYD": true, "HTG": true,
- "HNL": true, "HKD": true, "HUF": true, "ISK": true, "IDR": true,
- "XDR": true, "IRR": true, "IQD": true, "ILS": true, "JMD": true,
- "JPY": true, "JOD": true, "KZT": true, "KES": true, "KPW": true,
- "KRW": true, "KWD": true, "KGS": true, "LAK": true, "LBP": true,
- "LSL": true, "ZAR": true, "LRD": true, "LYD": true, "CHF": true,
- "MOP": true, "MKD": true, "MGA": true, "MWK": true, "MYR": true,
- "MVR": true, "MRU": true, "MUR": true, "XUA": true, "MXN": true,
- "MXV": true, "MDL": true, "MNT": true, "MAD": true, "MZN": true,
- "MMK": true, "NAD": true, "NPR": true, "NIO": true, "NGN": true,
- "OMR": true, "PKR": true, "PAB": true, "PGK": true, "PYG": true,
- "PEN": true, "PHP": true, "PLN": true, "QAR": true, "RON": true,
- "RUB": true, "RWF": true, "SHP": true, "WST": true, "STN": true,
- "SAR": true, "RSD": true, "SCR": true, "SLL": true, "SGD": true,
- "XSU": true, "SBD": true, "SOS": true, "SSP": true, "LKR": true,
- "SDG": true, "SRD": true, "SEK": true, "CHE": true, "CHW": true,
- "SYP": true, "TWD": true, "TJS": true, "TZS": true, "THB": true,
- "TOP": true, "TTD": true, "TND": true, "TRY": true, "TMT": true,
- "UGX": true, "UAH": true, "AED": true, "USN": true, "UYU": true,
- "UYI": true, "UYW": true, "UZS": true, "VUV": true, "VES": true,
- "VND": true, "YER": true, "ZMW": true, "ZWL": true, "XBA": true,
- "XBB": true, "XBC": true, "XBD": true, "XTS": true, "XXX": true,
- "XAU": true, "XPD": true, "XPT": true, "XAG": true,
-}
-
-var iso4217_numeric = map[int]bool{
- 8: true, 12: true, 32: true, 36: true, 44: true,
- 48: true, 50: true, 51: true, 52: true, 60: true,
- 64: true, 68: true, 72: true, 84: true, 90: true,
- 96: true, 104: true, 108: true, 116: true, 124: true,
- 132: true, 136: true, 144: true, 152: true, 156: true,
- 170: true, 174: true, 188: true, 191: true, 192: true,
- 203: true, 208: true, 214: true, 222: true, 230: true,
- 232: true, 238: true, 242: true, 262: true, 270: true,
- 292: true, 320: true, 324: true, 328: true, 332: true,
- 340: true, 344: true, 348: true, 352: true, 356: true,
- 360: true, 364: true, 368: true, 376: true, 388: true,
- 392: true, 398: true, 400: true, 404: true, 408: true,
- 410: true, 414: true, 417: true, 418: true, 422: true,
- 426: true, 430: true, 434: true, 446: true, 454: true,
- 458: true, 462: true, 480: true, 484: true, 496: true,
- 498: true, 504: true, 512: true, 516: true, 524: true,
- 532: true, 533: true, 548: true, 554: true, 558: true,
- 566: true, 578: true, 586: true, 590: true, 598: true,
- 600: true, 604: true, 608: true, 634: true, 643: true,
- 646: true, 654: true, 682: true, 690: true, 694: true,
- 702: true, 704: true, 706: true, 710: true, 728: true,
- 748: true, 752: true, 756: true, 760: true, 764: true,
- 776: true, 780: true, 784: true, 788: true, 800: true,
- 807: true, 818: true, 826: true, 834: true, 840: true,
- 858: true, 860: true, 882: true, 886: true, 901: true,
- 927: true, 928: true, 929: true, 930: true, 931: true,
- 932: true, 933: true, 934: true, 936: true, 938: true,
- 940: true, 941: true, 943: true, 944: true, 946: true,
- 947: true, 948: true, 949: true, 950: true, 951: true,
- 952: true, 953: true, 955: true, 956: true, 957: true,
- 958: true, 959: true, 960: true, 961: true, 962: true,
- 963: true, 964: true, 965: true, 967: true, 968: true,
- 969: true, 970: true, 971: true, 972: true, 973: true,
- 975: true, 976: true, 977: true, 978: true, 979: true,
- 980: true, 981: true, 984: true, 985: true, 986: true,
- 990: true, 994: true, 997: true, 999: true,
-}
diff --git a/vendor/github.com/go-playground/validator/v10/doc.go b/vendor/github.com/go-playground/validator/v10/doc.go
deleted file mode 100644
index 7341c67d..00000000
--- a/vendor/github.com/go-playground/validator/v10/doc.go
+++ /dev/null
@@ -1,1401 +0,0 @@
-/*
-Package validator implements value validations for structs and individual fields
-based on tags.
-
-It can also handle Cross-Field and Cross-Struct validation for nested structs
-and has the ability to dive into arrays and maps of any type.
-
-see more examples https://github.com/go-playground/validator/tree/master/_examples
-
-Singleton
-
-Validator is designed to be thread-safe and used as a singleton instance.
-It caches information about your struct and validations,
-in essence only parsing your validation tags once per struct type.
-Using multiple instances neglects the benefit of caching.
-The not thread-safe functions are explicitly marked as such in the documentation.
-
-Validation Functions Return Type error
-
-Doing things this way is actually the way the standard library does, see the
-file.Open method here:
-
- https://golang.org/pkg/os/#Open.
-
-The authors return type "error" to avoid the issue discussed in the following,
-where err is always != nil:
-
- http://stackoverflow.com/a/29138676/3158232
- https://github.com/go-playground/validator/issues/134
-
-Validator only InvalidValidationError for bad validation input, nil or
-ValidationErrors as type error; so, in your code all you need to do is check
-if the error returned is not nil, and if it's not check if error is
-InvalidValidationError ( if necessary, most of the time it isn't ) type cast
-it to type ValidationErrors like so err.(validator.ValidationErrors).
-
-Custom Validation Functions
-
-Custom Validation functions can be added. Example:
-
- // Structure
- func customFunc(fl validator.FieldLevel) bool {
-
- if fl.Field().String() == "invalid" {
- return false
- }
-
- return true
- }
-
- validate.RegisterValidation("custom tag name", customFunc)
- // NOTES: using the same tag name as an existing function
- // will overwrite the existing one
-
-Cross-Field Validation
-
-Cross-Field Validation can be done via the following tags:
- - eqfield
- - nefield
- - gtfield
- - gtefield
- - ltfield
- - ltefield
- - eqcsfield
- - necsfield
- - gtcsfield
- - gtecsfield
- - ltcsfield
- - ltecsfield
-
-If, however, some custom cross-field validation is required, it can be done
-using a custom validation.
-
-Why not just have cross-fields validation tags (i.e. only eqcsfield and not
-eqfield)?
-
-The reason is efficiency. If you want to check a field within the same struct
-"eqfield" only has to find the field on the same struct (1 level). But, if we
-used "eqcsfield" it could be multiple levels down. Example:
-
- type Inner struct {
- StartDate time.Time
- }
-
- type Outer struct {
- InnerStructField *Inner
- CreatedAt time.Time `validate:"ltecsfield=InnerStructField.StartDate"`
- }
-
- now := time.Now()
-
- inner := &Inner{
- StartDate: now,
- }
-
- outer := &Outer{
- InnerStructField: inner,
- CreatedAt: now,
- }
-
- errs := validate.Struct(outer)
-
- // NOTE: when calling validate.Struct(val) topStruct will be the top level struct passed
- // into the function
- // when calling validate.VarWithValue(val, field, tag) val will be
- // whatever you pass, struct, field...
- // when calling validate.Field(field, tag) val will be nil
-
-Multiple Validators
-
-Multiple validators on a field will process in the order defined. Example:
-
- type Test struct {
- Field `validate:"max=10,min=1"`
- }
-
- // max will be checked then min
-
-Bad Validator definitions are not handled by the library. Example:
-
- type Test struct {
- Field `validate:"min=10,max=0"`
- }
-
- // this definition of min max will never succeed
-
-Using Validator Tags
-
-Baked In Cross-Field validation only compares fields on the same struct.
-If Cross-Field + Cross-Struct validation is needed you should implement your
-own custom validator.
-
-Comma (",") is the default separator of validation tags. If you wish to
-have a comma included within the parameter (i.e. excludesall=,) you will need to
-use the UTF-8 hex representation 0x2C, which is replaced in the code as a comma,
-so the above will become excludesall=0x2C.
-
- type Test struct {
- Field `validate:"excludesall=,"` // BAD! Do not include a comma.
- Field `validate:"excludesall=0x2C"` // GOOD! Use the UTF-8 hex representation.
- }
-
-Pipe ("|") is the 'or' validation tags deparator. If you wish to
-have a pipe included within the parameter i.e. excludesall=| you will need to
-use the UTF-8 hex representation 0x7C, which is replaced in the code as a pipe,
-so the above will become excludesall=0x7C
-
- type Test struct {
- Field `validate:"excludesall=|"` // BAD! Do not include a a pipe!
- Field `validate:"excludesall=0x7C"` // GOOD! Use the UTF-8 hex representation.
- }
-
-
-Baked In Validators and Tags
-
-Here is a list of the current built in validators:
-
-
-Skip Field
-
-Tells the validation to skip this struct field; this is particularly
-handy in ignoring embedded structs from being validated. (Usage: -)
- Usage: -
-
-
-Or Operator
-
-This is the 'or' operator allowing multiple validators to be used and
-accepted. (Usage: rgb|rgba) <-- this would allow either rgb or rgba
-colors to be accepted. This can also be combined with 'and' for example
-( Usage: omitempty,rgb|rgba)
-
- Usage: |
-
-StructOnly
-
-When a field that is a nested struct is encountered, and contains this flag
-any validation on the nested struct will be run, but none of the nested
-struct fields will be validated. This is useful if inside of your program
-you know the struct will be valid, but need to verify it has been assigned.
-NOTE: only "required" and "omitempty" can be used on a struct itself.
-
- Usage: structonly
-
-NoStructLevel
-
-Same as structonly tag except that any struct level validations will not run.
-
- Usage: nostructlevel
-
-Omit Empty
-
-Allows conditional validation, for example if a field is not set with
-a value (Determined by the "required" validator) then other validation
-such as min or max won't run, but if a value is set validation will run.
-
- Usage: omitempty
-
-Dive
-
-This tells the validator to dive into a slice, array or map and validate that
-level of the slice, array or map with the validation tags that follow.
-Multidimensional nesting is also supported, each level you wish to dive will
-require another dive tag. dive has some sub-tags, 'keys' & 'endkeys', please see
-the Keys & EndKeys section just below.
-
- Usage: dive
-
-Example #1
-
- [][]string with validation tag "gt=0,dive,len=1,dive,required"
- // gt=0 will be applied to []
- // len=1 will be applied to []string
- // required will be applied to string
-
-Example #2
-
- [][]string with validation tag "gt=0,dive,dive,required"
- // gt=0 will be applied to []
- // []string will be spared validation
- // required will be applied to string
-
-Keys & EndKeys
-
-These are to be used together directly after the dive tag and tells the validator
-that anything between 'keys' and 'endkeys' applies to the keys of a map and not the
-values; think of it like the 'dive' tag, but for map keys instead of values.
-Multidimensional nesting is also supported, each level you wish to validate will
-require another 'keys' and 'endkeys' tag. These tags are only valid for maps.
-
- Usage: dive,keys,othertagvalidation(s),endkeys,valuevalidationtags
-
-Example #1
-
- map[string]string with validation tag "gt=0,dive,keys,eg=1|eq=2,endkeys,required"
- // gt=0 will be applied to the map itself
- // eg=1|eq=2 will be applied to the map keys
- // required will be applied to map values
-
-Example #2
-
- map[[2]string]string with validation tag "gt=0,dive,keys,dive,eq=1|eq=2,endkeys,required"
- // gt=0 will be applied to the map itself
- // eg=1|eq=2 will be applied to each array element in the the map keys
- // required will be applied to map values
-
-Required
-
-This validates that the value is not the data types default zero value.
-For numbers ensures value is not zero. For strings ensures value is
-not "". For slices, maps, pointers, interfaces, channels and functions
-ensures the value is not nil.
-
- Usage: required
-
-Required If
-
-The field under validation must be present and not empty only if all
-the other specified fields are equal to the value following the specified
-field. For strings ensures value is not "". For slices, maps, pointers,
-interfaces, channels and functions ensures the value is not nil.
-
- Usage: required_if
-
-Examples:
-
- // require the field if the Field1 is equal to the parameter given:
- Usage: required_if=Field1 foobar
-
- // require the field if the Field1 and Field2 is equal to the value respectively:
- Usage: required_if=Field1 foo Field2 bar
-
-Required Unless
-
-The field under validation must be present and not empty unless all
-the other specified fields are equal to the value following the specified
-field. For strings ensures value is not "". For slices, maps, pointers,
-interfaces, channels and functions ensures the value is not nil.
-
- Usage: required_unless
-
-Examples:
-
- // require the field unless the Field1 is equal to the parameter given:
- Usage: required_unless=Field1 foobar
-
- // require the field unless the Field1 and Field2 is equal to the value respectively:
- Usage: required_unless=Field1 foo Field2 bar
-
-Required With
-
-The field under validation must be present and not empty only if any
-of the other specified fields are present. For strings ensures value is
-not "". For slices, maps, pointers, interfaces, channels and functions
-ensures the value is not nil.
-
- Usage: required_with
-
-Examples:
-
- // require the field if the Field1 is present:
- Usage: required_with=Field1
-
- // require the field if the Field1 or Field2 is present:
- Usage: required_with=Field1 Field2
-
-Required With All
-
-The field under validation must be present and not empty only if all
-of the other specified fields are present. For strings ensures value is
-not "". For slices, maps, pointers, interfaces, channels and functions
-ensures the value is not nil.
-
- Usage: required_with_all
-
-Example:
-
- // require the field if the Field1 and Field2 is present:
- Usage: required_with_all=Field1 Field2
-
-Required Without
-
-The field under validation must be present and not empty only when any
-of the other specified fields are not present. For strings ensures value is
-not "". For slices, maps, pointers, interfaces, channels and functions
-ensures the value is not nil.
-
- Usage: required_without
-
-Examples:
-
- // require the field if the Field1 is not present:
- Usage: required_without=Field1
-
- // require the field if the Field1 or Field2 is not present:
- Usage: required_without=Field1 Field2
-
-Required Without All
-
-The field under validation must be present and not empty only when all
-of the other specified fields are not present. For strings ensures value is
-not "". For slices, maps, pointers, interfaces, channels and functions
-ensures the value is not nil.
-
- Usage: required_without_all
-
-Example:
-
- // require the field if the Field1 and Field2 is not present:
- Usage: required_without_all=Field1 Field2
-
-Excluded If
-
-The field under validation must not be present or not empty only if all
-the other specified fields are equal to the value following the specified
-field. For strings ensures value is not "". For slices, maps, pointers,
-interfaces, channels and functions ensures the value is not nil.
-
- Usage: excluded_if
-
-Examples:
-
- // exclude the field if the Field1 is equal to the parameter given:
- Usage: excluded_if=Field1 foobar
-
- // exclude the field if the Field1 and Field2 is equal to the value respectively:
- Usage: excluded_if=Field1 foo Field2 bar
-
-Excluded Unless
-
-The field under validation must not be present or empty unless all
-the other specified fields are equal to the value following the specified
-field. For strings ensures value is not "". For slices, maps, pointers,
-interfaces, channels and functions ensures the value is not nil.
-
- Usage: excluded_unless
-
-Examples:
-
- // exclude the field unless the Field1 is equal to the parameter given:
- Usage: excluded_unless=Field1 foobar
-
- // exclude the field unless the Field1 and Field2 is equal to the value respectively:
- Usage: excluded_unless=Field1 foo Field2 bar
-
-Is Default
-
-This validates that the value is the default value and is almost the
-opposite of required.
-
- Usage: isdefault
-
-Length
-
-For numbers, length will ensure that the value is
-equal to the parameter given. For strings, it checks that
-the string length is exactly that number of characters. For slices,
-arrays, and maps, validates the number of items.
-
-Example #1
-
- Usage: len=10
-
-Example #2 (time.Duration)
-
-For time.Duration, len will ensure that the value is equal to the duration given
-in the parameter.
-
- Usage: len=1h30m
-
-Maximum
-
-For numbers, max will ensure that the value is
-less than or equal to the parameter given. For strings, it checks
-that the string length is at most that number of characters. For
-slices, arrays, and maps, validates the number of items.
-
-Example #1
-
- Usage: max=10
-
-Example #2 (time.Duration)
-
-For time.Duration, max will ensure that the value is less than or equal to the
-duration given in the parameter.
-
- Usage: max=1h30m
-
-Minimum
-
-For numbers, min will ensure that the value is
-greater or equal to the parameter given. For strings, it checks that
-the string length is at least that number of characters. For slices,
-arrays, and maps, validates the number of items.
-
-Example #1
-
- Usage: min=10
-
-Example #2 (time.Duration)
-
-For time.Duration, min will ensure that the value is greater than or equal to
-the duration given in the parameter.
-
- Usage: min=1h30m
-
-Equals
-
-For strings & numbers, eq will ensure that the value is
-equal to the parameter given. For slices, arrays, and maps,
-validates the number of items.
-
-Example #1
-
- Usage: eq=10
-
-Example #2 (time.Duration)
-
-For time.Duration, eq will ensure that the value is equal to the duration given
-in the parameter.
-
- Usage: eq=1h30m
-
-Not Equal
-
-For strings & numbers, ne will ensure that the value is not
-equal to the parameter given. For slices, arrays, and maps,
-validates the number of items.
-
-Example #1
-
- Usage: ne=10
-
-Example #2 (time.Duration)
-
-For time.Duration, ne will ensure that the value is not equal to the duration
-given in the parameter.
-
- Usage: ne=1h30m
-
-One Of
-
-For strings, ints, and uints, oneof will ensure that the value
-is one of the values in the parameter. The parameter should be
-a list of values separated by whitespace. Values may be
-strings or numbers. To match strings with spaces in them, include
-the target string between single quotes.
-
- Usage: oneof=red green
- oneof='red green' 'blue yellow'
- oneof=5 7 9
-
-Greater Than
-
-For numbers, this will ensure that the value is greater than the
-parameter given. For strings, it checks that the string length
-is greater than that number of characters. For slices, arrays
-and maps it validates the number of items.
-
-Example #1
-
- Usage: gt=10
-
-Example #2 (time.Time)
-
-For time.Time ensures the time value is greater than time.Now.UTC().
-
- Usage: gt
-
-Example #3 (time.Duration)
-
-For time.Duration, gt will ensure that the value is greater than the duration
-given in the parameter.
-
- Usage: gt=1h30m
-
-Greater Than or Equal
-
-Same as 'min' above. Kept both to make terminology with 'len' easier.
-
-Example #1
-
- Usage: gte=10
-
-Example #2 (time.Time)
-
-For time.Time ensures the time value is greater than or equal to time.Now.UTC().
-
- Usage: gte
-
-Example #3 (time.Duration)
-
-For time.Duration, gte will ensure that the value is greater than or equal to
-the duration given in the parameter.
-
- Usage: gte=1h30m
-
-Less Than
-
-For numbers, this will ensure that the value is less than the parameter given.
-For strings, it checks that the string length is less than that number of
-characters. For slices, arrays, and maps it validates the number of items.
-
-Example #1
-
- Usage: lt=10
-
-Example #2 (time.Time)
-
-For time.Time ensures the time value is less than time.Now.UTC().
-
- Usage: lt
-
-Example #3 (time.Duration)
-
-For time.Duration, lt will ensure that the value is less than the duration given
-in the parameter.
-
- Usage: lt=1h30m
-
-Less Than or Equal
-
-Same as 'max' above. Kept both to make terminology with 'len' easier.
-
-Example #1
-
- Usage: lte=10
-
-Example #2 (time.Time)
-
-For time.Time ensures the time value is less than or equal to time.Now.UTC().
-
- Usage: lte
-
-Example #3 (time.Duration)
-
-For time.Duration, lte will ensure that the value is less than or equal to the
-duration given in the parameter.
-
- Usage: lte=1h30m
-
-Field Equals Another Field
-
-This will validate the field value against another fields value either within
-a struct or passed in field.
-
-Example #1:
-
- // Validation on Password field using:
- Usage: eqfield=ConfirmPassword
-
-Example #2:
-
- // Validating by field:
- validate.VarWithValue(password, confirmpassword, "eqfield")
-
-Field Equals Another Field (relative)
-
-This does the same as eqfield except that it validates the field provided relative
-to the top level struct.
-
- Usage: eqcsfield=InnerStructField.Field)
-
-Field Does Not Equal Another Field
-
-This will validate the field value against another fields value either within
-a struct or passed in field.
-
-Examples:
-
- // Confirm two colors are not the same:
- //
- // Validation on Color field:
- Usage: nefield=Color2
-
- // Validating by field:
- validate.VarWithValue(color1, color2, "nefield")
-
-Field Does Not Equal Another Field (relative)
-
-This does the same as nefield except that it validates the field provided
-relative to the top level struct.
-
- Usage: necsfield=InnerStructField.Field
-
-Field Greater Than Another Field
-
-Only valid for Numbers, time.Duration and time.Time types, this will validate
-the field value against another fields value either within a struct or passed in
-field. usage examples are for validation of a Start and End date:
-
-Example #1:
-
- // Validation on End field using:
- validate.Struct Usage(gtfield=Start)
-
-Example #2:
-
- // Validating by field:
- validate.VarWithValue(start, end, "gtfield")
-
-Field Greater Than Another Relative Field
-
-This does the same as gtfield except that it validates the field provided
-relative to the top level struct.
-
- Usage: gtcsfield=InnerStructField.Field
-
-Field Greater Than or Equal To Another Field
-
-Only valid for Numbers, time.Duration and time.Time types, this will validate
-the field value against another fields value either within a struct or passed in
-field. usage examples are for validation of a Start and End date:
-
-Example #1:
-
- // Validation on End field using:
- validate.Struct Usage(gtefield=Start)
-
-Example #2:
-
- // Validating by field:
- validate.VarWithValue(start, end, "gtefield")
-
-Field Greater Than or Equal To Another Relative Field
-
-This does the same as gtefield except that it validates the field provided relative
-to the top level struct.
-
- Usage: gtecsfield=InnerStructField.Field
-
-Less Than Another Field
-
-Only valid for Numbers, time.Duration and time.Time types, this will validate
-the field value against another fields value either within a struct or passed in
-field. usage examples are for validation of a Start and End date:
-
-Example #1:
-
- // Validation on End field using:
- validate.Struct Usage(ltfield=Start)
-
-Example #2:
-
- // Validating by field:
- validate.VarWithValue(start, end, "ltfield")
-
-Less Than Another Relative Field
-
-This does the same as ltfield except that it validates the field provided relative
-to the top level struct.
-
- Usage: ltcsfield=InnerStructField.Field
-
-Less Than or Equal To Another Field
-
-Only valid for Numbers, time.Duration and time.Time types, this will validate
-the field value against another fields value either within a struct or passed in
-field. usage examples are for validation of a Start and End date:
-
-Example #1:
-
- // Validation on End field using:
- validate.Struct Usage(ltefield=Start)
-
-Example #2:
-
- // Validating by field:
- validate.VarWithValue(start, end, "ltefield")
-
-Less Than or Equal To Another Relative Field
-
-This does the same as ltefield except that it validates the field provided relative
-to the top level struct.
-
- Usage: ltecsfield=InnerStructField.Field
-
-Field Contains Another Field
-
-This does the same as contains except for struct fields. It should only be used
-with string types. See the behavior of reflect.Value.String() for behavior on
-other types.
-
- Usage: containsfield=InnerStructField.Field
-
-Field Excludes Another Field
-
-This does the same as excludes except for struct fields. It should only be used
-with string types. See the behavior of reflect.Value.String() for behavior on
-other types.
-
- Usage: excludesfield=InnerStructField.Field
-
-Unique
-
-For arrays & slices, unique will ensure that there are no duplicates.
-For maps, unique will ensure that there are no duplicate values.
-For slices of struct, unique will ensure that there are no duplicate values
-in a field of the struct specified via a parameter.
-
- // For arrays, slices, and maps:
- Usage: unique
-
- // For slices of struct:
- Usage: unique=field
-
-Alpha Only
-
-This validates that a string value contains ASCII alpha characters only
-
- Usage: alpha
-
-Alphanumeric
-
-This validates that a string value contains ASCII alphanumeric characters only
-
- Usage: alphanum
-
-Alpha Unicode
-
-This validates that a string value contains unicode alpha characters only
-
- Usage: alphaunicode
-
-Alphanumeric Unicode
-
-This validates that a string value contains unicode alphanumeric characters only
-
- Usage: alphanumunicode
-
-Boolean
-
-This validates that a string value can successfully be parsed into a boolean with strconv.ParseBool
-
- Usage: boolean
-
-Number
-
-This validates that a string value contains number values only.
-For integers or float it returns true.
-
- Usage: number
-
-Numeric
-
-This validates that a string value contains a basic numeric value.
-basic excludes exponents etc...
-for integers or float it returns true.
-
- Usage: numeric
-
-Hexadecimal String
-
-This validates that a string value contains a valid hexadecimal.
-
- Usage: hexadecimal
-
-Hexcolor String
-
-This validates that a string value contains a valid hex color including
-hashtag (#)
-
- Usage: hexcolor
-
-Lowercase String
-
-This validates that a string value contains only lowercase characters. An empty string is not a valid lowercase string.
-
- Usage: lowercase
-
-Uppercase String
-
-This validates that a string value contains only uppercase characters. An empty string is not a valid uppercase string.
-
- Usage: uppercase
-
-RGB String
-
-This validates that a string value contains a valid rgb color
-
- Usage: rgb
-
-RGBA String
-
-This validates that a string value contains a valid rgba color
-
- Usage: rgba
-
-HSL String
-
-This validates that a string value contains a valid hsl color
-
- Usage: hsl
-
-HSLA String
-
-This validates that a string value contains a valid hsla color
-
- Usage: hsla
-
-E.164 Phone Number String
-
-This validates that a string value contains a valid E.164 Phone number
-https://en.wikipedia.org/wiki/E.164 (ex. +1123456789)
-
- Usage: e164
-
-E-mail String
-
-This validates that a string value contains a valid email
-This may not conform to all possibilities of any rfc standard, but neither
-does any email provider accept all possibilities.
-
- Usage: email
-
-JSON String
-
-This validates that a string value is valid JSON
-
- Usage: json
-
-JWT String
-
-This validates that a string value is a valid JWT
-
- Usage: jwt
-
-File path
-
-This validates that a string value contains a valid file path and that
-the file exists on the machine.
-This is done using os.Stat, which is a platform independent function.
-
- Usage: file
-
-URL String
-
-This validates that a string value contains a valid url
-This will accept any url the golang request uri accepts but must contain
-a schema for example http:// or rtmp://
-
- Usage: url
-
-URI String
-
-This validates that a string value contains a valid uri
-This will accept any uri the golang request uri accepts
-
- Usage: uri
-
-Urn RFC 2141 String
-
-This validataes that a string value contains a valid URN
-according to the RFC 2141 spec.
-
- Usage: urn_rfc2141
-
-Base64 String
-
-This validates that a string value contains a valid base64 value.
-Although an empty string is valid base64 this will report an empty string
-as an error, if you wish to accept an empty string as valid you can use
-this with the omitempty tag.
-
- Usage: base64
-
-Base64URL String
-
-This validates that a string value contains a valid base64 URL safe value
-according the the RFC4648 spec.
-Although an empty string is a valid base64 URL safe value, this will report
-an empty string as an error, if you wish to accept an empty string as valid
-you can use this with the omitempty tag.
-
- Usage: base64url
-
-Bitcoin Address
-
-This validates that a string value contains a valid bitcoin address.
-The format of the string is checked to ensure it matches one of the three formats
-P2PKH, P2SH and performs checksum validation.
-
- Usage: btc_addr
-
-Bitcoin Bech32 Address (segwit)
-
-This validates that a string value contains a valid bitcoin Bech32 address as defined
-by bip-0173 (https://github.com/bitcoin/bips/blob/master/bip-0173.mediawiki)
-Special thanks to Pieter Wuille for providng reference implementations.
-
- Usage: btc_addr_bech32
-
-Ethereum Address
-
-This validates that a string value contains a valid ethereum address.
-The format of the string is checked to ensure it matches the standard Ethereum address format.
-
- Usage: eth_addr
-
-Contains
-
-This validates that a string value contains the substring value.
-
- Usage: contains=@
-
-Contains Any
-
-This validates that a string value contains any Unicode code points
-in the substring value.
-
- Usage: containsany=!@#?
-
-Contains Rune
-
-This validates that a string value contains the supplied rune value.
-
- Usage: containsrune=@
-
-Excludes
-
-This validates that a string value does not contain the substring value.
-
- Usage: excludes=@
-
-Excludes All
-
-This validates that a string value does not contain any Unicode code
-points in the substring value.
-
- Usage: excludesall=!@#?
-
-Excludes Rune
-
-This validates that a string value does not contain the supplied rune value.
-
- Usage: excludesrune=@
-
-Starts With
-
-This validates that a string value starts with the supplied string value
-
- Usage: startswith=hello
-
-Ends With
-
-This validates that a string value ends with the supplied string value
-
- Usage: endswith=goodbye
-
-Does Not Start With
-
-This validates that a string value does not start with the supplied string value
-
- Usage: startsnotwith=hello
-
-Does Not End With
-
-This validates that a string value does not end with the supplied string value
-
- Usage: endsnotwith=goodbye
-
-International Standard Book Number
-
-This validates that a string value contains a valid isbn10 or isbn13 value.
-
- Usage: isbn
-
-International Standard Book Number 10
-
-This validates that a string value contains a valid isbn10 value.
-
- Usage: isbn10
-
-International Standard Book Number 13
-
-This validates that a string value contains a valid isbn13 value.
-
- Usage: isbn13
-
-Universally Unique Identifier UUID
-
-This validates that a string value contains a valid UUID. Uppercase UUID values will not pass - use `uuid_rfc4122` instead.
-
- Usage: uuid
-
-Universally Unique Identifier UUID v3
-
-This validates that a string value contains a valid version 3 UUID. Uppercase UUID values will not pass - use `uuid3_rfc4122` instead.
-
- Usage: uuid3
-
-Universally Unique Identifier UUID v4
-
-This validates that a string value contains a valid version 4 UUID. Uppercase UUID values will not pass - use `uuid4_rfc4122` instead.
-
- Usage: uuid4
-
-Universally Unique Identifier UUID v5
-
-This validates that a string value contains a valid version 5 UUID. Uppercase UUID values will not pass - use `uuid5_rfc4122` instead.
-
- Usage: uuid5
-
-Universally Unique Lexicographically Sortable Identifier ULID
-
-This validates that a string value contains a valid ULID value.
-
- Usage: ulid
-
-ASCII
-
-This validates that a string value contains only ASCII characters.
-NOTE: if the string is blank, this validates as true.
-
- Usage: ascii
-
-Printable ASCII
-
-This validates that a string value contains only printable ASCII characters.
-NOTE: if the string is blank, this validates as true.
-
- Usage: printascii
-
-Multi-Byte Characters
-
-This validates that a string value contains one or more multibyte characters.
-NOTE: if the string is blank, this validates as true.
-
- Usage: multibyte
-
-Data URL
-
-This validates that a string value contains a valid DataURI.
-NOTE: this will also validate that the data portion is valid base64
-
- Usage: datauri
-
-Latitude
-
-This validates that a string value contains a valid latitude.
-
- Usage: latitude
-
-Longitude
-
-This validates that a string value contains a valid longitude.
-
- Usage: longitude
-
-Social Security Number SSN
-
-This validates that a string value contains a valid U.S. Social Security Number.
-
- Usage: ssn
-
-Internet Protocol Address IP
-
-This validates that a string value contains a valid IP Address.
-
- Usage: ip
-
-Internet Protocol Address IPv4
-
-This validates that a string value contains a valid v4 IP Address.
-
- Usage: ipv4
-
-Internet Protocol Address IPv6
-
-This validates that a string value contains a valid v6 IP Address.
-
- Usage: ipv6
-
-Classless Inter-Domain Routing CIDR
-
-This validates that a string value contains a valid CIDR Address.
-
- Usage: cidr
-
-Classless Inter-Domain Routing CIDRv4
-
-This validates that a string value contains a valid v4 CIDR Address.
-
- Usage: cidrv4
-
-Classless Inter-Domain Routing CIDRv6
-
-This validates that a string value contains a valid v6 CIDR Address.
-
- Usage: cidrv6
-
-Transmission Control Protocol Address TCP
-
-This validates that a string value contains a valid resolvable TCP Address.
-
- Usage: tcp_addr
-
-Transmission Control Protocol Address TCPv4
-
-This validates that a string value contains a valid resolvable v4 TCP Address.
-
- Usage: tcp4_addr
-
-Transmission Control Protocol Address TCPv6
-
-This validates that a string value contains a valid resolvable v6 TCP Address.
-
- Usage: tcp6_addr
-
-User Datagram Protocol Address UDP
-
-This validates that a string value contains a valid resolvable UDP Address.
-
- Usage: udp_addr
-
-User Datagram Protocol Address UDPv4
-
-This validates that a string value contains a valid resolvable v4 UDP Address.
-
- Usage: udp4_addr
-
-User Datagram Protocol Address UDPv6
-
-This validates that a string value contains a valid resolvable v6 UDP Address.
-
- Usage: udp6_addr
-
-Internet Protocol Address IP
-
-This validates that a string value contains a valid resolvable IP Address.
-
- Usage: ip_addr
-
-Internet Protocol Address IPv4
-
-This validates that a string value contains a valid resolvable v4 IP Address.
-
- Usage: ip4_addr
-
-Internet Protocol Address IPv6
-
-This validates that a string value contains a valid resolvable v6 IP Address.
-
- Usage: ip6_addr
-
-Unix domain socket end point Address
-
-This validates that a string value contains a valid Unix Address.
-
- Usage: unix_addr
-
-Media Access Control Address MAC
-
-This validates that a string value contains a valid MAC Address.
-
- Usage: mac
-
-Note: See Go's ParseMAC for accepted formats and types:
-
- http://golang.org/src/net/mac.go?s=866:918#L29
-
-Hostname RFC 952
-
-This validates that a string value is a valid Hostname according to RFC 952 https://tools.ietf.org/html/rfc952
-
- Usage: hostname
-
-Hostname RFC 1123
-
-This validates that a string value is a valid Hostname according to RFC 1123 https://tools.ietf.org/html/rfc1123
-
- Usage: hostname_rfc1123 or if you want to continue to use 'hostname' in your tags, create an alias.
-
-Full Qualified Domain Name (FQDN)
-
-This validates that a string value contains a valid FQDN.
-
- Usage: fqdn
-
-HTML Tags
-
-This validates that a string value appears to be an HTML element tag
-including those described at https://developer.mozilla.org/en-US/docs/Web/HTML/Element
-
- Usage: html
-
-HTML Encoded
-
-This validates that a string value is a proper character reference in decimal
-or hexadecimal format
-
- Usage: html_encoded
-
-URL Encoded
-
-This validates that a string value is percent-encoded (URL encoded) according
-to https://tools.ietf.org/html/rfc3986#section-2.1
-
- Usage: url_encoded
-
-Directory
-
-This validates that a string value contains a valid directory and that
-it exists on the machine.
-This is done using os.Stat, which is a platform independent function.
-
- Usage: dir
-
-HostPort
-
-This validates that a string value contains a valid DNS hostname and port that
-can be used to valiate fields typically passed to sockets and connections.
-
- Usage: hostname_port
-
-Datetime
-
-This validates that a string value is a valid datetime based on the supplied datetime format.
-Supplied format must match the official Go time format layout as documented in https://golang.org/pkg/time/
-
- Usage: datetime=2006-01-02
-
-Iso3166-1 alpha-2
-
-This validates that a string value is a valid country code based on iso3166-1 alpha-2 standard.
-see: https://www.iso.org/iso-3166-country-codes.html
-
- Usage: iso3166_1_alpha2
-
-Iso3166-1 alpha-3
-
-This validates that a string value is a valid country code based on iso3166-1 alpha-3 standard.
-see: https://www.iso.org/iso-3166-country-codes.html
-
- Usage: iso3166_1_alpha3
-
-Iso3166-1 alpha-numeric
-
-This validates that a string value is a valid country code based on iso3166-1 alpha-numeric standard.
-see: https://www.iso.org/iso-3166-country-codes.html
-
- Usage: iso3166_1_alpha3
-
-BCP 47 Language Tag
-
-This validates that a string value is a valid BCP 47 language tag, as parsed by language.Parse.
-More information on https://pkg.go.dev/golang.org/x/text/language
-
- Usage: bcp47_language_tag
-
-BIC (SWIFT code)
-
-This validates that a string value is a valid Business Identifier Code (SWIFT code), defined in ISO 9362.
-More information on https://www.iso.org/standard/60390.html
-
- Usage: bic
-
-RFC 1035 label
-
-This validates that a string value is a valid dns RFC 1035 label, defined in RFC 1035.
-More information on https://datatracker.ietf.org/doc/html/rfc1035
-
- Usage: dns_rfc1035_label
-
-TimeZone
-
-This validates that a string value is a valid time zone based on the time zone database present on the system.
-Although empty value and Local value are allowed by time.LoadLocation golang function, they are not allowed by this validator.
-More information on https://golang.org/pkg/time/#LoadLocation
-
- Usage: timezone
-
-Semantic Version
-
-This validates that a string value is a valid semver version, defined in Semantic Versioning 2.0.0.
-More information on https://semver.org/
-
- Usage: semver
-
-Credit Card
-
-This validates that a string value contains a valid credit card number using Luhn algoritm.
-
- Usage: credit_card
-
-Alias Validators and Tags
-
-NOTE: When returning an error, the tag returned in "FieldError" will be
-the alias tag unless the dive tag is part of the alias. Everything after the
-dive tag is not reported as the alias tag. Also, the "ActualTag" in the before
-case will be the actual tag within the alias that failed.
-
-Here is a list of the current built in alias tags:
-
- "iscolor"
- alias is "hexcolor|rgb|rgba|hsl|hsla" (Usage: iscolor)
- "country_code"
- alias is "iso3166_1_alpha2|iso3166_1_alpha3|iso3166_1_alpha_numeric" (Usage: country_code)
-
-Validator notes:
-
- regex
- a regex validator won't be added because commas and = signs can be part
- of a regex which conflict with the validation definitions. Although
- workarounds can be made, they take away from using pure regex's.
- Furthermore it's quick and dirty but the regex's become harder to
- maintain and are not reusable, so it's as much a programming philosophy
- as anything.
-
- In place of this new validator functions should be created; a regex can
- be used within the validator function and even be precompiled for better
- efficiency within regexes.go.
-
- And the best reason, you can submit a pull request and we can keep on
- adding to the validation library of this package!
-
-Non standard validators
-
-A collection of validation rules that are frequently needed but are more
-complex than the ones found in the baked in validators.
-A non standard validator must be registered manually like you would
-with your own custom validation functions.
-
-Example of registration and use:
-
- type Test struct {
- TestField string `validate:"yourtag"`
- }
-
- t := &Test{
- TestField: "Test"
- }
-
- validate := validator.New()
- validate.RegisterValidation("yourtag", validators.NotBlank)
-
-Here is a list of the current non standard validators:
-
- NotBlank
- This validates that the value is not blank or with length zero.
- For strings ensures they do not contain only spaces. For channels, maps, slices and arrays
- ensures they don't have zero length. For others, a non empty value is required.
-
- Usage: notblank
-
-Panics
-
-This package panics when bad input is provided, this is by design, bad code like
-that should not make it to production.
-
- type Test struct {
- TestField string `validate:"nonexistantfunction=1"`
- }
-
- t := &Test{
- TestField: "Test"
- }
-
- validate.Struct(t) // this will panic
-*/
-package validator
diff --git a/vendor/github.com/go-playground/validator/v10/errors.go b/vendor/github.com/go-playground/validator/v10/errors.go
deleted file mode 100644
index 9a1b1abe..00000000
--- a/vendor/github.com/go-playground/validator/v10/errors.go
+++ /dev/null
@@ -1,275 +0,0 @@
-package validator
-
-import (
- "bytes"
- "fmt"
- "reflect"
- "strings"
-
- ut "github.com/go-playground/universal-translator"
-)
-
-const (
- fieldErrMsg = "Key: '%s' Error:Field validation for '%s' failed on the '%s' tag"
-)
-
-// ValidationErrorsTranslations is the translation return type
-type ValidationErrorsTranslations map[string]string
-
-// InvalidValidationError describes an invalid argument passed to
-// `Struct`, `StructExcept`, StructPartial` or `Field`
-type InvalidValidationError struct {
- Type reflect.Type
-}
-
-// Error returns InvalidValidationError message
-func (e *InvalidValidationError) Error() string {
-
- if e.Type == nil {
- return "validator: (nil)"
- }
-
- return "validator: (nil " + e.Type.String() + ")"
-}
-
-// ValidationErrors is an array of FieldError's
-// for use in custom error messages post validation.
-type ValidationErrors []FieldError
-
-// Error is intended for use in development + debugging and not intended to be a production error message.
-// It allows ValidationErrors to subscribe to the Error interface.
-// All information to create an error message specific to your application is contained within
-// the FieldError found within the ValidationErrors array
-func (ve ValidationErrors) Error() string {
-
- buff := bytes.NewBufferString("")
-
- var fe *fieldError
-
- for i := 0; i < len(ve); i++ {
-
- fe = ve[i].(*fieldError)
- buff.WriteString(fe.Error())
- buff.WriteString("\n")
- }
-
- return strings.TrimSpace(buff.String())
-}
-
-// Translate translates all of the ValidationErrors
-func (ve ValidationErrors) Translate(ut ut.Translator) ValidationErrorsTranslations {
-
- trans := make(ValidationErrorsTranslations)
-
- var fe *fieldError
-
- for i := 0; i < len(ve); i++ {
- fe = ve[i].(*fieldError)
-
- // // in case an Anonymous struct was used, ensure that the key
- // // would be 'Username' instead of ".Username"
- // if len(fe.ns) > 0 && fe.ns[:1] == "." {
- // trans[fe.ns[1:]] = fe.Translate(ut)
- // continue
- // }
-
- trans[fe.ns] = fe.Translate(ut)
- }
-
- return trans
-}
-
-// FieldError contains all functions to get error details
-type FieldError interface {
-
- // Tag returns the validation tag that failed. if the
- // validation was an alias, this will return the
- // alias name and not the underlying tag that failed.
- //
- // eg. alias "iscolor": "hexcolor|rgb|rgba|hsl|hsla"
- // will return "iscolor"
- Tag() string
-
- // ActualTag returns the validation tag that failed, even if an
- // alias the actual tag within the alias will be returned.
- // If an 'or' validation fails the entire or will be returned.
- //
- // eg. alias "iscolor": "hexcolor|rgb|rgba|hsl|hsla"
- // will return "hexcolor|rgb|rgba|hsl|hsla"
- ActualTag() string
-
- // Namespace returns the namespace for the field error, with the tag
- // name taking precedence over the field's actual name.
- //
- // eg. JSON name "User.fname"
- //
- // See StructNamespace() for a version that returns actual names.
- //
- // NOTE: this field can be blank when validating a single primitive field
- // using validate.Field(...) as there is no way to extract it's name
- Namespace() string
-
- // StructNamespace returns the namespace for the field error, with the field's
- // actual name.
- //
- // eq. "User.FirstName" see Namespace for comparison
- //
- // NOTE: this field can be blank when validating a single primitive field
- // using validate.Field(...) as there is no way to extract its name
- StructNamespace() string
-
- // Field returns the fields name with the tag name taking precedence over the
- // field's actual name.
- //
- // eq. JSON name "fname"
- // see StructField for comparison
- Field() string
-
- // StructField returns the field's actual name from the struct, when able to determine.
- //
- // eq. "FirstName"
- // see Field for comparison
- StructField() string
-
- // Value returns the actual field's value in case needed for creating the error
- // message
- Value() interface{}
-
- // Param returns the param value, in string form for comparison; this will also
- // help with generating an error message
- Param() string
-
- // Kind returns the Field's reflect Kind
- //
- // eg. time.Time's kind is a struct
- Kind() reflect.Kind
-
- // Type returns the Field's reflect Type
- //
- // eg. time.Time's type is time.Time
- Type() reflect.Type
-
- // Translate returns the FieldError's translated error
- // from the provided 'ut.Translator' and registered 'TranslationFunc'
- //
- // NOTE: if no registered translator can be found it returns the same as
- // calling fe.Error()
- Translate(ut ut.Translator) string
-
- // Error returns the FieldError's message
- Error() string
-}
-
-// compile time interface checks
-var _ FieldError = new(fieldError)
-var _ error = new(fieldError)
-
-// fieldError contains a single field's validation error along
-// with other properties that may be needed for error message creation
-// it complies with the FieldError interface
-type fieldError struct {
- v *Validate
- tag string
- actualTag string
- ns string
- structNs string
- fieldLen uint8
- structfieldLen uint8
- value interface{}
- param string
- kind reflect.Kind
- typ reflect.Type
-}
-
-// Tag returns the validation tag that failed.
-func (fe *fieldError) Tag() string {
- return fe.tag
-}
-
-// ActualTag returns the validation tag that failed, even if an
-// alias the actual tag within the alias will be returned.
-func (fe *fieldError) ActualTag() string {
- return fe.actualTag
-}
-
-// Namespace returns the namespace for the field error, with the tag
-// name taking precedence over the field's actual name.
-func (fe *fieldError) Namespace() string {
- return fe.ns
-}
-
-// StructNamespace returns the namespace for the field error, with the field's
-// actual name.
-func (fe *fieldError) StructNamespace() string {
- return fe.structNs
-}
-
-// Field returns the field's name with the tag name taking precedence over the
-// field's actual name.
-func (fe *fieldError) Field() string {
-
- return fe.ns[len(fe.ns)-int(fe.fieldLen):]
- // // return fe.field
- // fld := fe.ns[len(fe.ns)-int(fe.fieldLen):]
-
- // log.Println("FLD:", fld)
-
- // if len(fld) > 0 && fld[:1] == "." {
- // return fld[1:]
- // }
-
- // return fld
-}
-
-// StructField returns the field's actual name from the struct, when able to determine.
-func (fe *fieldError) StructField() string {
- // return fe.structField
- return fe.structNs[len(fe.structNs)-int(fe.structfieldLen):]
-}
-
-// Value returns the actual field's value in case needed for creating the error
-// message
-func (fe *fieldError) Value() interface{} {
- return fe.value
-}
-
-// Param returns the param value, in string form for comparison; this will
-// also help with generating an error message
-func (fe *fieldError) Param() string {
- return fe.param
-}
-
-// Kind returns the Field's reflect Kind
-func (fe *fieldError) Kind() reflect.Kind {
- return fe.kind
-}
-
-// Type returns the Field's reflect Type
-func (fe *fieldError) Type() reflect.Type {
- return fe.typ
-}
-
-// Error returns the fieldError's error message
-func (fe *fieldError) Error() string {
- return fmt.Sprintf(fieldErrMsg, fe.ns, fe.Field(), fe.tag)
-}
-
-// Translate returns the FieldError's translated error
-// from the provided 'ut.Translator' and registered 'TranslationFunc'
-//
-// NOTE: if no registered translation can be found, it returns the original
-// untranslated error message.
-func (fe *fieldError) Translate(ut ut.Translator) string {
-
- m, ok := fe.v.transTagFunc[ut]
- if !ok {
- return fe.Error()
- }
-
- fn, ok := m[fe.tag]
- if !ok {
- return fe.Error()
- }
-
- return fn(ut, fe)
-}
diff --git a/vendor/github.com/go-playground/validator/v10/field_level.go b/vendor/github.com/go-playground/validator/v10/field_level.go
deleted file mode 100644
index ef35826e..00000000
--- a/vendor/github.com/go-playground/validator/v10/field_level.go
+++ /dev/null
@@ -1,120 +0,0 @@
-package validator
-
-import "reflect"
-
-// FieldLevel contains all the information and helper functions
-// to validate a field
-type FieldLevel interface {
-
- // Top returns the top level struct, if any
- Top() reflect.Value
-
- // Parent returns the current fields parent struct, if any or
- // the comparison value if called 'VarWithValue'
- Parent() reflect.Value
-
- // Field returns current field for validation
- Field() reflect.Value
-
- // FieldName returns the field's name with the tag
- // name taking precedence over the fields actual name.
- FieldName() string
-
- // StructFieldName returns the struct field's name
- StructFieldName() string
-
- // Param returns param for validation against current field
- Param() string
-
- // GetTag returns the current validations tag name
- GetTag() string
-
- // ExtractType gets the actual underlying type of field value.
- // It will dive into pointers, customTypes and return you the
- // underlying value and it's kind.
- ExtractType(field reflect.Value) (value reflect.Value, kind reflect.Kind, nullable bool)
-
- // GetStructFieldOK traverses the parent struct to retrieve a specific field denoted by the provided namespace
- // in the param and returns the field, field kind and whether is was successful in retrieving
- // the field at all.
- //
- // NOTE: when not successful ok will be false, this can happen when a nested struct is nil and so the field
- // could not be retrieved because it didn't exist.
- //
- // Deprecated: Use GetStructFieldOK2() instead which also return if the value is nullable.
- GetStructFieldOK() (reflect.Value, reflect.Kind, bool)
-
- // GetStructFieldOKAdvanced is the same as GetStructFieldOK except that it accepts the parent struct to start looking for
- // the field and namespace allowing more extensibility for validators.
- //
- // Deprecated: Use GetStructFieldOKAdvanced2() instead which also return if the value is nullable.
- GetStructFieldOKAdvanced(val reflect.Value, namespace string) (reflect.Value, reflect.Kind, bool)
-
- // GetStructFieldOK2 traverses the parent struct to retrieve a specific field denoted by the provided namespace
- // in the param and returns the field, field kind, if it's a nullable type and whether is was successful in retrieving
- // the field at all.
- //
- // NOTE: when not successful ok will be false, this can happen when a nested struct is nil and so the field
- // could not be retrieved because it didn't exist.
- GetStructFieldOK2() (reflect.Value, reflect.Kind, bool, bool)
-
- // GetStructFieldOKAdvanced2 is the same as GetStructFieldOK except that it accepts the parent struct to start looking for
- // the field and namespace allowing more extensibility for validators.
- GetStructFieldOKAdvanced2(val reflect.Value, namespace string) (reflect.Value, reflect.Kind, bool, bool)
-}
-
-var _ FieldLevel = new(validate)
-
-// Field returns current field for validation
-func (v *validate) Field() reflect.Value {
- return v.flField
-}
-
-// FieldName returns the field's name with the tag
-// name taking precedence over the fields actual name.
-func (v *validate) FieldName() string {
- return v.cf.altName
-}
-
-// GetTag returns the current validations tag name
-func (v *validate) GetTag() string {
- return v.ct.tag
-}
-
-// StructFieldName returns the struct field's name
-func (v *validate) StructFieldName() string {
- return v.cf.name
-}
-
-// Param returns param for validation against current field
-func (v *validate) Param() string {
- return v.ct.param
-}
-
-// GetStructFieldOK returns Param returns param for validation against current field
-//
-// Deprecated: Use GetStructFieldOK2() instead which also return if the value is nullable.
-func (v *validate) GetStructFieldOK() (reflect.Value, reflect.Kind, bool) {
- current, kind, _, found := v.getStructFieldOKInternal(v.slflParent, v.ct.param)
- return current, kind, found
-}
-
-// GetStructFieldOKAdvanced is the same as GetStructFieldOK except that it accepts the parent struct to start looking for
-// the field and namespace allowing more extensibility for validators.
-//
-// Deprecated: Use GetStructFieldOKAdvanced2() instead which also return if the value is nullable.
-func (v *validate) GetStructFieldOKAdvanced(val reflect.Value, namespace string) (reflect.Value, reflect.Kind, bool) {
- current, kind, _, found := v.GetStructFieldOKAdvanced2(val, namespace)
- return current, kind, found
-}
-
-// GetStructFieldOK2 returns Param returns param for validation against current field
-func (v *validate) GetStructFieldOK2() (reflect.Value, reflect.Kind, bool, bool) {
- return v.getStructFieldOKInternal(v.slflParent, v.ct.param)
-}
-
-// GetStructFieldOKAdvanced2 is the same as GetStructFieldOK except that it accepts the parent struct to start looking for
-// the field and namespace allowing more extensibility for validators.
-func (v *validate) GetStructFieldOKAdvanced2(val reflect.Value, namespace string) (reflect.Value, reflect.Kind, bool, bool) {
- return v.getStructFieldOKInternal(val, namespace)
-}
diff --git a/vendor/github.com/go-playground/validator/v10/logo.png b/vendor/github.com/go-playground/validator/v10/logo.png
deleted file mode 100644
index 355000f5..00000000
Binary files a/vendor/github.com/go-playground/validator/v10/logo.png and /dev/null differ
diff --git a/vendor/github.com/go-playground/validator/v10/postcode_regexes.go b/vendor/github.com/go-playground/validator/v10/postcode_regexes.go
deleted file mode 100644
index e7e7b687..00000000
--- a/vendor/github.com/go-playground/validator/v10/postcode_regexes.go
+++ /dev/null
@@ -1,173 +0,0 @@
-package validator
-
-import "regexp"
-
-var postCodePatternDict = map[string]string{
- "GB": `^GIR[ ]?0AA|((AB|AL|B|BA|BB|BD|BH|BL|BN|BR|BS|BT|CA|CB|CF|CH|CM|CO|CR|CT|CV|CW|DA|DD|DE|DG|DH|DL|DN|DT|DY|E|EC|EH|EN|EX|FK|FY|G|GL|GY|GU|HA|HD|HG|HP|HR|HS|HU|HX|IG|IM|IP|IV|JE|KA|KT|KW|KY|L|LA|LD|LE|LL|LN|LS|LU|M|ME|MK|ML|N|NE|NG|NN|NP|NR|NW|OL|OX|PA|PE|PH|PL|PO|PR|RG|RH|RM|S|SA|SE|SG|SK|SL|SM|SN|SO|SP|SR|SS|ST|SW|SY|TA|TD|TF|TN|TQ|TR|TS|TW|UB|W|WA|WC|WD|WF|WN|WR|WS|WV|YO|ZE)(\d[\dA-Z]?[ ]?\d[ABD-HJLN-UW-Z]{2}))|BFPO[ ]?\d{1,4}$`,
- "JE": `^JE\d[\dA-Z]?[ ]?\d[ABD-HJLN-UW-Z]{2}$`,
- "GG": `^GY\d[\dA-Z]?[ ]?\d[ABD-HJLN-UW-Z]{2}$`,
- "IM": `^IM\d[\dA-Z]?[ ]?\d[ABD-HJLN-UW-Z]{2}$`,
- "US": `^\d{5}([ \-]\d{4})?$`,
- "CA": `^[ABCEGHJKLMNPRSTVXY]\d[ABCEGHJ-NPRSTV-Z][ ]?\d[ABCEGHJ-NPRSTV-Z]\d$`,
- "DE": `^\d{5}$`,
- "JP": `^\d{3}-\d{4}$`,
- "FR": `^\d{2}[ ]?\d{3}$`,
- "AU": `^\d{4}$`,
- "IT": `^\d{5}$`,
- "CH": `^\d{4}$`,
- "AT": `^\d{4}$`,
- "ES": `^\d{5}$`,
- "NL": `^\d{4}[ ]?[A-Z]{2}$`,
- "BE": `^\d{4}$`,
- "DK": `^\d{4}$`,
- "SE": `^\d{3}[ ]?\d{2}$`,
- "NO": `^\d{4}$`,
- "BR": `^\d{5}[\-]?\d{3}$`,
- "PT": `^\d{4}([\-]\d{3})?$`,
- "FI": `^\d{5}$`,
- "AX": `^22\d{3}$`,
- "KR": `^\d{3}[\-]\d{3}$`,
- "CN": `^\d{6}$`,
- "TW": `^\d{3}(\d{2})?$`,
- "SG": `^\d{6}$`,
- "DZ": `^\d{5}$`,
- "AD": `^AD\d{3}$`,
- "AR": `^([A-HJ-NP-Z])?\d{4}([A-Z]{3})?$`,
- "AM": `^(37)?\d{4}$`,
- "AZ": `^\d{4}$`,
- "BH": `^((1[0-2]|[2-9])\d{2})?$`,
- "BD": `^\d{4}$`,
- "BB": `^(BB\d{5})?$`,
- "BY": `^\d{6}$`,
- "BM": `^[A-Z]{2}[ ]?[A-Z0-9]{2}$`,
- "BA": `^\d{5}$`,
- "IO": `^BBND 1ZZ$`,
- "BN": `^[A-Z]{2}[ ]?\d{4}$`,
- "BG": `^\d{4}$`,
- "KH": `^\d{5}$`,
- "CV": `^\d{4}$`,
- "CL": `^\d{7}$`,
- "CR": `^\d{4,5}|\d{3}-\d{4}$`,
- "HR": `^\d{5}$`,
- "CY": `^\d{4}$`,
- "CZ": `^\d{3}[ ]?\d{2}$`,
- "DO": `^\d{5}$`,
- "EC": `^([A-Z]\d{4}[A-Z]|(?:[A-Z]{2})?\d{6})?$`,
- "EG": `^\d{5}$`,
- "EE": `^\d{5}$`,
- "FO": `^\d{3}$`,
- "GE": `^\d{4}$`,
- "GR": `^\d{3}[ ]?\d{2}$`,
- "GL": `^39\d{2}$`,
- "GT": `^\d{5}$`,
- "HT": `^\d{4}$`,
- "HN": `^(?:\d{5})?$`,
- "HU": `^\d{4}$`,
- "IS": `^\d{3}$`,
- "IN": `^\d{6}$`,
- "ID": `^\d{5}$`,
- "IL": `^\d{5}$`,
- "JO": `^\d{5}$`,
- "KZ": `^\d{6}$`,
- "KE": `^\d{5}$`,
- "KW": `^\d{5}$`,
- "LA": `^\d{5}$`,
- "LV": `^\d{4}$`,
- "LB": `^(\d{4}([ ]?\d{4})?)?$`,
- "LI": `^(948[5-9])|(949[0-7])$`,
- "LT": `^\d{5}$`,
- "LU": `^\d{4}$`,
- "MK": `^\d{4}$`,
- "MY": `^\d{5}$`,
- "MV": `^\d{5}$`,
- "MT": `^[A-Z]{3}[ ]?\d{2,4}$`,
- "MU": `^(\d{3}[A-Z]{2}\d{3})?$`,
- "MX": `^\d{5}$`,
- "MD": `^\d{4}$`,
- "MC": `^980\d{2}$`,
- "MA": `^\d{5}$`,
- "NP": `^\d{5}$`,
- "NZ": `^\d{4}$`,
- "NI": `^((\d{4}-)?\d{3}-\d{3}(-\d{1})?)?$`,
- "NG": `^(\d{6})?$`,
- "OM": `^(PC )?\d{3}$`,
- "PK": `^\d{5}$`,
- "PY": `^\d{4}$`,
- "PH": `^\d{4}$`,
- "PL": `^\d{2}-\d{3}$`,
- "PR": `^00[679]\d{2}([ \-]\d{4})?$`,
- "RO": `^\d{6}$`,
- "RU": `^\d{6}$`,
- "SM": `^4789\d$`,
- "SA": `^\d{5}$`,
- "SN": `^\d{5}$`,
- "SK": `^\d{3}[ ]?\d{2}$`,
- "SI": `^\d{4}$`,
- "ZA": `^\d{4}$`,
- "LK": `^\d{5}$`,
- "TJ": `^\d{6}$`,
- "TH": `^\d{5}$`,
- "TN": `^\d{4}$`,
- "TR": `^\d{5}$`,
- "TM": `^\d{6}$`,
- "UA": `^\d{5}$`,
- "UY": `^\d{5}$`,
- "UZ": `^\d{6}$`,
- "VA": `^00120$`,
- "VE": `^\d{4}$`,
- "ZM": `^\d{5}$`,
- "AS": `^96799$`,
- "CC": `^6799$`,
- "CK": `^\d{4}$`,
- "RS": `^\d{6}$`,
- "ME": `^8\d{4}$`,
- "CS": `^\d{5}$`,
- "YU": `^\d{5}$`,
- "CX": `^6798$`,
- "ET": `^\d{4}$`,
- "FK": `^FIQQ 1ZZ$`,
- "NF": `^2899$`,
- "FM": `^(9694[1-4])([ \-]\d{4})?$`,
- "GF": `^9[78]3\d{2}$`,
- "GN": `^\d{3}$`,
- "GP": `^9[78][01]\d{2}$`,
- "GS": `^SIQQ 1ZZ$`,
- "GU": `^969[123]\d([ \-]\d{4})?$`,
- "GW": `^\d{4}$`,
- "HM": `^\d{4}$`,
- "IQ": `^\d{5}$`,
- "KG": `^\d{6}$`,
- "LR": `^\d{4}$`,
- "LS": `^\d{3}$`,
- "MG": `^\d{3}$`,
- "MH": `^969[67]\d([ \-]\d{4})?$`,
- "MN": `^\d{6}$`,
- "MP": `^9695[012]([ \-]\d{4})?$`,
- "MQ": `^9[78]2\d{2}$`,
- "NC": `^988\d{2}$`,
- "NE": `^\d{4}$`,
- "VI": `^008(([0-4]\d)|(5[01]))([ \-]\d{4})?$`,
- "VN": `^[0-9]{1,6}$`,
- "PF": `^987\d{2}$`,
- "PG": `^\d{3}$`,
- "PM": `^9[78]5\d{2}$`,
- "PN": `^PCRN 1ZZ$`,
- "PW": `^96940$`,
- "RE": `^9[78]4\d{2}$`,
- "SH": `^(ASCN|STHL) 1ZZ$`,
- "SJ": `^\d{4}$`,
- "SO": `^\d{5}$`,
- "SZ": `^[HLMS]\d{3}$`,
- "TC": `^TKCA 1ZZ$`,
- "WF": `^986\d{2}$`,
- "XK": `^\d{5}$`,
- "YT": `^976\d{2}$`,
-}
-
-var postCodeRegexDict = map[string]*regexp.Regexp{}
-
-func init() {
- for countryCode, pattern := range postCodePatternDict {
- postCodeRegexDict[countryCode] = regexp.MustCompile(pattern)
- }
-}
diff --git a/vendor/github.com/go-playground/validator/v10/regexes.go b/vendor/github.com/go-playground/validator/v10/regexes.go
deleted file mode 100644
index 9c1c6342..00000000
--- a/vendor/github.com/go-playground/validator/v10/regexes.go
+++ /dev/null
@@ -1,131 +0,0 @@
-package validator
-
-import "regexp"
-
-const (
- alphaRegexString = "^[a-zA-Z]+$"
- alphaNumericRegexString = "^[a-zA-Z0-9]+$"
- alphaUnicodeRegexString = "^[\\p{L}]+$"
- alphaUnicodeNumericRegexString = "^[\\p{L}\\p{N}]+$"
- numericRegexString = "^[-+]?[0-9]+(?:\\.[0-9]+)?$"
- numberRegexString = "^[0-9]+$"
- hexadecimalRegexString = "^(0[xX])?[0-9a-fA-F]+$"
- hexColorRegexString = "^#(?:[0-9a-fA-F]{3}|[0-9a-fA-F]{4}|[0-9a-fA-F]{6}|[0-9a-fA-F]{8})$"
- rgbRegexString = "^rgb\\(\\s*(?:(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])|(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])%\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])%\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])%)\\s*\\)$"
- rgbaRegexString = "^rgba\\(\\s*(?:(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])|(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])%\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])%\\s*,\\s*(?:0|[1-9]\\d?|1\\d\\d?|2[0-4]\\d|25[0-5])%)\\s*,\\s*(?:(?:0.[1-9]*)|[01])\\s*\\)$"
- hslRegexString = "^hsl\\(\\s*(?:0|[1-9]\\d?|[12]\\d\\d|3[0-5]\\d|360)\\s*,\\s*(?:(?:0|[1-9]\\d?|100)%)\\s*,\\s*(?:(?:0|[1-9]\\d?|100)%)\\s*\\)$"
- hslaRegexString = "^hsla\\(\\s*(?:0|[1-9]\\d?|[12]\\d\\d|3[0-5]\\d|360)\\s*,\\s*(?:(?:0|[1-9]\\d?|100)%)\\s*,\\s*(?:(?:0|[1-9]\\d?|100)%)\\s*,\\s*(?:(?:0.[1-9]*)|[01])\\s*\\)$"
- emailRegexString = "^(?:(?:(?:(?:[a-zA-Z]|\\d|[!#\\$%&'\\*\\+\\-\\/=\\?\\^_`{\\|}~]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])+(?:\\.([a-zA-Z]|\\d|[!#\\$%&'\\*\\+\\-\\/=\\?\\^_`{\\|}~]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])+)*)|(?:(?:\\x22)(?:(?:(?:(?:\\x20|\\x09)*(?:\\x0d\\x0a))?(?:\\x20|\\x09)+)?(?:(?:[\\x01-\\x08\\x0b\\x0c\\x0e-\\x1f\\x7f]|\\x21|[\\x23-\\x5b]|[\\x5d-\\x7e]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])|(?:(?:[\\x01-\\x09\\x0b\\x0c\\x0d-\\x7f]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}]))))*(?:(?:(?:\\x20|\\x09)*(?:\\x0d\\x0a))?(\\x20|\\x09)+)?(?:\\x22))))@(?:(?:(?:[a-zA-Z]|\\d|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])|(?:(?:[a-zA-Z]|\\d|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])(?:[a-zA-Z]|\\d|-|\\.|~|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])*(?:[a-zA-Z]|\\d|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])))\\.)+(?:(?:[a-zA-Z]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])|(?:(?:[a-zA-Z]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])(?:[a-zA-Z]|\\d|-|\\.|~|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])*(?:[a-zA-Z]|[\\x{00A0}-\\x{D7FF}\\x{F900}-\\x{FDCF}\\x{FDF0}-\\x{FFEF}])))\\.?$"
- e164RegexString = "^\\+[1-9]?[0-9]{7,14}$"
- base64RegexString = "^(?:[A-Za-z0-9+\\/]{4})*(?:[A-Za-z0-9+\\/]{2}==|[A-Za-z0-9+\\/]{3}=|[A-Za-z0-9+\\/]{4})$"
- base64URLRegexString = "^(?:[A-Za-z0-9-_]{4})*(?:[A-Za-z0-9-_]{2}==|[A-Za-z0-9-_]{3}=|[A-Za-z0-9-_]{4})$"
- iSBN10RegexString = "^(?:[0-9]{9}X|[0-9]{10})$"
- iSBN13RegexString = "^(?:(?:97(?:8|9))[0-9]{10})$"
- uUID3RegexString = "^[0-9a-f]{8}-[0-9a-f]{4}-3[0-9a-f]{3}-[0-9a-f]{4}-[0-9a-f]{12}$"
- uUID4RegexString = "^[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}$"
- uUID5RegexString = "^[0-9a-f]{8}-[0-9a-f]{4}-5[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}$"
- uUIDRegexString = "^[0-9a-f]{8}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{12}$"
- uUID3RFC4122RegexString = "^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-3[0-9a-fA-F]{3}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$"
- uUID4RFC4122RegexString = "^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-4[0-9a-fA-F]{3}-[89abAB][0-9a-fA-F]{3}-[0-9a-fA-F]{12}$"
- uUID5RFC4122RegexString = "^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-5[0-9a-fA-F]{3}-[89abAB][0-9a-fA-F]{3}-[0-9a-fA-F]{12}$"
- uUIDRFC4122RegexString = "^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$"
- uLIDRegexString = "^[A-HJKMNP-TV-Z0-9]{26}$"
- md4RegexString = "^[0-9a-f]{32}$"
- md5RegexString = "^[0-9a-f]{32}$"
- sha256RegexString = "^[0-9a-f]{64}$"
- sha384RegexString = "^[0-9a-f]{96}$"
- sha512RegexString = "^[0-9a-f]{128}$"
- ripemd128RegexString = "^[0-9a-f]{32}$"
- ripemd160RegexString = "^[0-9a-f]{40}$"
- tiger128RegexString = "^[0-9a-f]{32}$"
- tiger160RegexString = "^[0-9a-f]{40}$"
- tiger192RegexString = "^[0-9a-f]{48}$"
- aSCIIRegexString = "^[\x00-\x7F]*$"
- printableASCIIRegexString = "^[\x20-\x7E]*$"
- multibyteRegexString = "[^\x00-\x7F]"
- dataURIRegexString = `^data:((?:\w+\/(?:([^;]|;[^;]).)+)?)`
- latitudeRegexString = "^[-+]?([1-8]?\\d(\\.\\d+)?|90(\\.0+)?)$"
- longitudeRegexString = "^[-+]?(180(\\.0+)?|((1[0-7]\\d)|([1-9]?\\d))(\\.\\d+)?)$"
- sSNRegexString = `^[0-9]{3}[ -]?(0[1-9]|[1-9][0-9])[ -]?([1-9][0-9]{3}|[0-9][1-9][0-9]{2}|[0-9]{2}[1-9][0-9]|[0-9]{3}[1-9])$`
- hostnameRegexStringRFC952 = `^[a-zA-Z]([a-zA-Z0-9\-]+[\.]?)*[a-zA-Z0-9]$` // https://tools.ietf.org/html/rfc952
- hostnameRegexStringRFC1123 = `^([a-zA-Z0-9]{1}[a-zA-Z0-9-]{0,62}){1}(\.[a-zA-Z0-9]{1}[a-zA-Z0-9-]{0,62})*?$` // accepts hostname starting with a digit https://tools.ietf.org/html/rfc1123
- fqdnRegexStringRFC1123 = `^([a-zA-Z0-9]{1}[a-zA-Z0-9-]{0,62})(\.[a-zA-Z0-9]{1}[a-zA-Z0-9-]{0,62})*?(\.[a-zA-Z]{1}[a-zA-Z0-9]{0,62})\.?$` // same as hostnameRegexStringRFC1123 but must contain a non numerical TLD (possibly ending with '.')
- btcAddressRegexString = `^[13][a-km-zA-HJ-NP-Z1-9]{25,34}$` // bitcoin address
- btcAddressUpperRegexStringBech32 = `^BC1[02-9AC-HJ-NP-Z]{7,76}$` // bitcoin bech32 address https://en.bitcoin.it/wiki/Bech32
- btcAddressLowerRegexStringBech32 = `^bc1[02-9ac-hj-np-z]{7,76}$` // bitcoin bech32 address https://en.bitcoin.it/wiki/Bech32
- ethAddressRegexString = `^0x[0-9a-fA-F]{40}$`
- ethAddressUpperRegexString = `^0x[0-9A-F]{40}$`
- ethAddressLowerRegexString = `^0x[0-9a-f]{40}$`
- uRLEncodedRegexString = `^(?:[^%]|%[0-9A-Fa-f]{2})*$`
- hTMLEncodedRegexString = `[x]?([0-9a-fA-F]{2})|(>)|(<)|(")|(&)+[;]?`
- hTMLRegexString = `<[/]?([a-zA-Z]+).*?>`
- jWTRegexString = "^[A-Za-z0-9-_]+\\.[A-Za-z0-9-_]+\\.[A-Za-z0-9-_]*$"
- splitParamsRegexString = `'[^']*'|\S+`
- bicRegexString = `^[A-Za-z]{6}[A-Za-z0-9]{2}([A-Za-z0-9]{3})?$`
- semverRegexString = `^(0|[1-9]\d*)\.(0|[1-9]\d*)\.(0|[1-9]\d*)(?:-((?:0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*)(?:\.(?:0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*))*))?(?:\+([0-9a-zA-Z-]+(?:\.[0-9a-zA-Z-]+)*))?$` // numbered capture groups https://semver.org/
- dnsRegexStringRFC1035Label = "^[a-z]([-a-z0-9]*[a-z0-9]){0,62}$"
-)
-
-var (
- alphaRegex = regexp.MustCompile(alphaRegexString)
- alphaNumericRegex = regexp.MustCompile(alphaNumericRegexString)
- alphaUnicodeRegex = regexp.MustCompile(alphaUnicodeRegexString)
- alphaUnicodeNumericRegex = regexp.MustCompile(alphaUnicodeNumericRegexString)
- numericRegex = regexp.MustCompile(numericRegexString)
- numberRegex = regexp.MustCompile(numberRegexString)
- hexadecimalRegex = regexp.MustCompile(hexadecimalRegexString)
- hexColorRegex = regexp.MustCompile(hexColorRegexString)
- rgbRegex = regexp.MustCompile(rgbRegexString)
- rgbaRegex = regexp.MustCompile(rgbaRegexString)
- hslRegex = regexp.MustCompile(hslRegexString)
- hslaRegex = regexp.MustCompile(hslaRegexString)
- e164Regex = regexp.MustCompile(e164RegexString)
- emailRegex = regexp.MustCompile(emailRegexString)
- base64Regex = regexp.MustCompile(base64RegexString)
- base64URLRegex = regexp.MustCompile(base64URLRegexString)
- iSBN10Regex = regexp.MustCompile(iSBN10RegexString)
- iSBN13Regex = regexp.MustCompile(iSBN13RegexString)
- uUID3Regex = regexp.MustCompile(uUID3RegexString)
- uUID4Regex = regexp.MustCompile(uUID4RegexString)
- uUID5Regex = regexp.MustCompile(uUID5RegexString)
- uUIDRegex = regexp.MustCompile(uUIDRegexString)
- uUID3RFC4122Regex = regexp.MustCompile(uUID3RFC4122RegexString)
- uUID4RFC4122Regex = regexp.MustCompile(uUID4RFC4122RegexString)
- uUID5RFC4122Regex = regexp.MustCompile(uUID5RFC4122RegexString)
- uUIDRFC4122Regex = regexp.MustCompile(uUIDRFC4122RegexString)
- uLIDRegex = regexp.MustCompile(uLIDRegexString)
- md4Regex = regexp.MustCompile(md4RegexString)
- md5Regex = regexp.MustCompile(md5RegexString)
- sha256Regex = regexp.MustCompile(sha256RegexString)
- sha384Regex = regexp.MustCompile(sha384RegexString)
- sha512Regex = regexp.MustCompile(sha512RegexString)
- ripemd128Regex = regexp.MustCompile(ripemd128RegexString)
- ripemd160Regex = regexp.MustCompile(ripemd160RegexString)
- tiger128Regex = regexp.MustCompile(tiger128RegexString)
- tiger160Regex = regexp.MustCompile(tiger160RegexString)
- tiger192Regex = regexp.MustCompile(tiger192RegexString)
- aSCIIRegex = regexp.MustCompile(aSCIIRegexString)
- printableASCIIRegex = regexp.MustCompile(printableASCIIRegexString)
- multibyteRegex = regexp.MustCompile(multibyteRegexString)
- dataURIRegex = regexp.MustCompile(dataURIRegexString)
- latitudeRegex = regexp.MustCompile(latitudeRegexString)
- longitudeRegex = regexp.MustCompile(longitudeRegexString)
- sSNRegex = regexp.MustCompile(sSNRegexString)
- hostnameRegexRFC952 = regexp.MustCompile(hostnameRegexStringRFC952)
- hostnameRegexRFC1123 = regexp.MustCompile(hostnameRegexStringRFC1123)
- fqdnRegexRFC1123 = regexp.MustCompile(fqdnRegexStringRFC1123)
- btcAddressRegex = regexp.MustCompile(btcAddressRegexString)
- btcUpperAddressRegexBech32 = regexp.MustCompile(btcAddressUpperRegexStringBech32)
- btcLowerAddressRegexBech32 = regexp.MustCompile(btcAddressLowerRegexStringBech32)
- ethAddressRegex = regexp.MustCompile(ethAddressRegexString)
- ethAddressRegexUpper = regexp.MustCompile(ethAddressUpperRegexString)
- ethAddressRegexLower = regexp.MustCompile(ethAddressLowerRegexString)
- uRLEncodedRegex = regexp.MustCompile(uRLEncodedRegexString)
- hTMLEncodedRegex = regexp.MustCompile(hTMLEncodedRegexString)
- hTMLRegex = regexp.MustCompile(hTMLRegexString)
- jWTRegex = regexp.MustCompile(jWTRegexString)
- splitParamsRegex = regexp.MustCompile(splitParamsRegexString)
- bicRegex = regexp.MustCompile(bicRegexString)
- semverRegex = regexp.MustCompile(semverRegexString)
- dnsRegexRFC1035Label = regexp.MustCompile(dnsRegexStringRFC1035Label)
-)
diff --git a/vendor/github.com/go-playground/validator/v10/struct_level.go b/vendor/github.com/go-playground/validator/v10/struct_level.go
deleted file mode 100644
index c0d89cfb..00000000
--- a/vendor/github.com/go-playground/validator/v10/struct_level.go
+++ /dev/null
@@ -1,175 +0,0 @@
-package validator
-
-import (
- "context"
- "reflect"
-)
-
-// StructLevelFunc accepts all values needed for struct level validation
-type StructLevelFunc func(sl StructLevel)
-
-// StructLevelFuncCtx accepts all values needed for struct level validation
-// but also allows passing of contextual validation information via context.Context.
-type StructLevelFuncCtx func(ctx context.Context, sl StructLevel)
-
-// wrapStructLevelFunc wraps normal StructLevelFunc makes it compatible with StructLevelFuncCtx
-func wrapStructLevelFunc(fn StructLevelFunc) StructLevelFuncCtx {
- return func(ctx context.Context, sl StructLevel) {
- fn(sl)
- }
-}
-
-// StructLevel contains all the information and helper functions
-// to validate a struct
-type StructLevel interface {
-
- // Validator returns the main validation object, in case one wants to call validations internally.
- // this is so you don't have to use anonymous functions to get access to the validate
- // instance.
- Validator() *Validate
-
- // Top returns the top level struct, if any
- Top() reflect.Value
-
- // Parent returns the current fields parent struct, if any
- Parent() reflect.Value
-
- // Current returns the current struct.
- Current() reflect.Value
-
- // ExtractType gets the actual underlying type of field value.
- // It will dive into pointers, customTypes and return you the
- // underlying value and its kind.
- ExtractType(field reflect.Value) (value reflect.Value, kind reflect.Kind, nullable bool)
-
- // ReportError reports an error just by passing the field and tag information
- //
- // NOTES:
- //
- // fieldName and altName get appended to the existing namespace that
- // validator is on. e.g. pass 'FirstName' or 'Names[0]' depending
- // on the nesting
- //
- // tag can be an existing validation tag or just something you make up
- // and process on the flip side it's up to you.
- ReportError(field interface{}, fieldName, structFieldName string, tag, param string)
-
- // ReportValidationErrors reports an error just by passing ValidationErrors
- //
- // NOTES:
- //
- // relativeNamespace and relativeActualNamespace get appended to the
- // existing namespace that validator is on.
- // e.g. pass 'User.FirstName' or 'Users[0].FirstName' depending
- // on the nesting. most of the time they will be blank, unless you validate
- // at a level lower the the current field depth
- ReportValidationErrors(relativeNamespace, relativeActualNamespace string, errs ValidationErrors)
-}
-
-var _ StructLevel = new(validate)
-
-// Top returns the top level struct
-//
-// NOTE: this can be the same as the current struct being validated
-// if not is a nested struct.
-//
-// this is only called when within Struct and Field Level validation and
-// should not be relied upon for an acurate value otherwise.
-func (v *validate) Top() reflect.Value {
- return v.top
-}
-
-// Parent returns the current structs parent
-//
-// NOTE: this can be the same as the current struct being validated
-// if not is a nested struct.
-//
-// this is only called when within Struct and Field Level validation and
-// should not be relied upon for an acurate value otherwise.
-func (v *validate) Parent() reflect.Value {
- return v.slflParent
-}
-
-// Current returns the current struct.
-func (v *validate) Current() reflect.Value {
- return v.slCurrent
-}
-
-// Validator returns the main validation object, in case one want to call validations internally.
-func (v *validate) Validator() *Validate {
- return v.v
-}
-
-// ExtractType gets the actual underlying type of field value.
-func (v *validate) ExtractType(field reflect.Value) (reflect.Value, reflect.Kind, bool) {
- return v.extractTypeInternal(field, false)
-}
-
-// ReportError reports an error just by passing the field and tag information
-func (v *validate) ReportError(field interface{}, fieldName, structFieldName, tag, param string) {
-
- fv, kind, _ := v.extractTypeInternal(reflect.ValueOf(field), false)
-
- if len(structFieldName) == 0 {
- structFieldName = fieldName
- }
-
- v.str1 = string(append(v.ns, fieldName...))
-
- if v.v.hasTagNameFunc || fieldName != structFieldName {
- v.str2 = string(append(v.actualNs, structFieldName...))
- } else {
- v.str2 = v.str1
- }
-
- if kind == reflect.Invalid {
-
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: tag,
- actualTag: tag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(fieldName)),
- structfieldLen: uint8(len(structFieldName)),
- param: param,
- kind: kind,
- },
- )
- return
- }
-
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: tag,
- actualTag: tag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(fieldName)),
- structfieldLen: uint8(len(structFieldName)),
- value: fv.Interface(),
- param: param,
- kind: kind,
- typ: fv.Type(),
- },
- )
-}
-
-// ReportValidationErrors reports ValidationErrors obtained from running validations within the Struct Level validation.
-//
-// NOTE: this function prepends the current namespace to the relative ones.
-func (v *validate) ReportValidationErrors(relativeNamespace, relativeStructNamespace string, errs ValidationErrors) {
-
- var err *fieldError
-
- for i := 0; i < len(errs); i++ {
-
- err = errs[i].(*fieldError)
- err.ns = string(append(append(v.ns, relativeNamespace...), err.ns...))
- err.structNs = string(append(append(v.actualNs, relativeStructNamespace...), err.structNs...))
-
- v.errs = append(v.errs, err)
- }
-}
diff --git a/vendor/github.com/go-playground/validator/v10/translations.go b/vendor/github.com/go-playground/validator/v10/translations.go
deleted file mode 100644
index 4d9d75c1..00000000
--- a/vendor/github.com/go-playground/validator/v10/translations.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package validator
-
-import ut "github.com/go-playground/universal-translator"
-
-// TranslationFunc is the function type used to register or override
-// custom translations
-type TranslationFunc func(ut ut.Translator, fe FieldError) string
-
-// RegisterTranslationsFunc allows for registering of translations
-// for a 'ut.Translator' for use within the 'TranslationFunc'
-type RegisterTranslationsFunc func(ut ut.Translator) error
diff --git a/vendor/github.com/go-playground/validator/v10/util.go b/vendor/github.com/go-playground/validator/v10/util.go
deleted file mode 100644
index 36da8551..00000000
--- a/vendor/github.com/go-playground/validator/v10/util.go
+++ /dev/null
@@ -1,288 +0,0 @@
-package validator
-
-import (
- "reflect"
- "strconv"
- "strings"
- "time"
-)
-
-// extractTypeInternal gets the actual underlying type of field value.
-// It will dive into pointers, customTypes and return you the
-// underlying value and it's kind.
-func (v *validate) extractTypeInternal(current reflect.Value, nullable bool) (reflect.Value, reflect.Kind, bool) {
-
-BEGIN:
- switch current.Kind() {
- case reflect.Ptr:
-
- nullable = true
-
- if current.IsNil() {
- return current, reflect.Ptr, nullable
- }
-
- current = current.Elem()
- goto BEGIN
-
- case reflect.Interface:
-
- nullable = true
-
- if current.IsNil() {
- return current, reflect.Interface, nullable
- }
-
- current = current.Elem()
- goto BEGIN
-
- case reflect.Invalid:
- return current, reflect.Invalid, nullable
-
- default:
-
- if v.v.hasCustomFuncs {
-
- if fn, ok := v.v.customFuncs[current.Type()]; ok {
- current = reflect.ValueOf(fn(current))
- goto BEGIN
- }
- }
-
- return current, current.Kind(), nullable
- }
-}
-
-// getStructFieldOKInternal traverses a struct to retrieve a specific field denoted by the provided namespace and
-// returns the field, field kind and whether is was successful in retrieving the field at all.
-//
-// NOTE: when not successful ok will be false, this can happen when a nested struct is nil and so the field
-// could not be retrieved because it didn't exist.
-func (v *validate) getStructFieldOKInternal(val reflect.Value, namespace string) (current reflect.Value, kind reflect.Kind, nullable bool, found bool) {
-
-BEGIN:
- current, kind, nullable = v.ExtractType(val)
- if kind == reflect.Invalid {
- return
- }
-
- if namespace == "" {
- found = true
- return
- }
-
- switch kind {
-
- case reflect.Ptr, reflect.Interface:
- return
-
- case reflect.Struct:
-
- typ := current.Type()
- fld := namespace
- var ns string
-
- if !typ.ConvertibleTo(timeType) {
-
- idx := strings.Index(namespace, namespaceSeparator)
-
- if idx != -1 {
- fld = namespace[:idx]
- ns = namespace[idx+1:]
- } else {
- ns = ""
- }
-
- bracketIdx := strings.Index(fld, leftBracket)
- if bracketIdx != -1 {
- fld = fld[:bracketIdx]
-
- ns = namespace[bracketIdx:]
- }
-
- val = current.FieldByName(fld)
- namespace = ns
- goto BEGIN
- }
-
- case reflect.Array, reflect.Slice:
- idx := strings.Index(namespace, leftBracket)
- idx2 := strings.Index(namespace, rightBracket)
-
- arrIdx, _ := strconv.Atoi(namespace[idx+1 : idx2])
-
- if arrIdx >= current.Len() {
- return
- }
-
- startIdx := idx2 + 1
-
- if startIdx < len(namespace) {
- if namespace[startIdx:startIdx+1] == namespaceSeparator {
- startIdx++
- }
- }
-
- val = current.Index(arrIdx)
- namespace = namespace[startIdx:]
- goto BEGIN
-
- case reflect.Map:
- idx := strings.Index(namespace, leftBracket) + 1
- idx2 := strings.Index(namespace, rightBracket)
-
- endIdx := idx2
-
- if endIdx+1 < len(namespace) {
- if namespace[endIdx+1:endIdx+2] == namespaceSeparator {
- endIdx++
- }
- }
-
- key := namespace[idx:idx2]
-
- switch current.Type().Key().Kind() {
- case reflect.Int:
- i, _ := strconv.Atoi(key)
- val = current.MapIndex(reflect.ValueOf(i))
- namespace = namespace[endIdx+1:]
-
- case reflect.Int8:
- i, _ := strconv.ParseInt(key, 10, 8)
- val = current.MapIndex(reflect.ValueOf(int8(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Int16:
- i, _ := strconv.ParseInt(key, 10, 16)
- val = current.MapIndex(reflect.ValueOf(int16(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Int32:
- i, _ := strconv.ParseInt(key, 10, 32)
- val = current.MapIndex(reflect.ValueOf(int32(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Int64:
- i, _ := strconv.ParseInt(key, 10, 64)
- val = current.MapIndex(reflect.ValueOf(i))
- namespace = namespace[endIdx+1:]
-
- case reflect.Uint:
- i, _ := strconv.ParseUint(key, 10, 0)
- val = current.MapIndex(reflect.ValueOf(uint(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Uint8:
- i, _ := strconv.ParseUint(key, 10, 8)
- val = current.MapIndex(reflect.ValueOf(uint8(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Uint16:
- i, _ := strconv.ParseUint(key, 10, 16)
- val = current.MapIndex(reflect.ValueOf(uint16(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Uint32:
- i, _ := strconv.ParseUint(key, 10, 32)
- val = current.MapIndex(reflect.ValueOf(uint32(i)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Uint64:
- i, _ := strconv.ParseUint(key, 10, 64)
- val = current.MapIndex(reflect.ValueOf(i))
- namespace = namespace[endIdx+1:]
-
- case reflect.Float32:
- f, _ := strconv.ParseFloat(key, 32)
- val = current.MapIndex(reflect.ValueOf(float32(f)))
- namespace = namespace[endIdx+1:]
-
- case reflect.Float64:
- f, _ := strconv.ParseFloat(key, 64)
- val = current.MapIndex(reflect.ValueOf(f))
- namespace = namespace[endIdx+1:]
-
- case reflect.Bool:
- b, _ := strconv.ParseBool(key)
- val = current.MapIndex(reflect.ValueOf(b))
- namespace = namespace[endIdx+1:]
-
- // reflect.Type = string
- default:
- val = current.MapIndex(reflect.ValueOf(key))
- namespace = namespace[endIdx+1:]
- }
-
- goto BEGIN
- }
-
- // if got here there was more namespace, cannot go any deeper
- panic("Invalid field namespace")
-}
-
-// asInt returns the parameter as a int64
-// or panics if it can't convert
-func asInt(param string) int64 {
- i, err := strconv.ParseInt(param, 0, 64)
- panicIf(err)
-
- return i
-}
-
-// asIntFromTimeDuration parses param as time.Duration and returns it as int64
-// or panics on error.
-func asIntFromTimeDuration(param string) int64 {
- d, err := time.ParseDuration(param)
- if err != nil {
- // attempt parsing as an an integer assuming nanosecond precision
- return asInt(param)
- }
- return int64(d)
-}
-
-// asIntFromType calls the proper function to parse param as int64,
-// given a field's Type t.
-func asIntFromType(t reflect.Type, param string) int64 {
- switch t {
- case timeDurationType:
- return asIntFromTimeDuration(param)
- default:
- return asInt(param)
- }
-}
-
-// asUint returns the parameter as a uint64
-// or panics if it can't convert
-func asUint(param string) uint64 {
-
- i, err := strconv.ParseUint(param, 0, 64)
- panicIf(err)
-
- return i
-}
-
-// asFloat returns the parameter as a float64
-// or panics if it can't convert
-func asFloat(param string) float64 {
-
- i, err := strconv.ParseFloat(param, 64)
- panicIf(err)
-
- return i
-}
-
-// asBool returns the parameter as a bool
-// or panics if it can't convert
-func asBool(param string) bool {
-
- i, err := strconv.ParseBool(param)
- panicIf(err)
-
- return i
-}
-
-func panicIf(err error) {
- if err != nil {
- panic(err.Error())
- }
-}
diff --git a/vendor/github.com/go-playground/validator/v10/validator.go b/vendor/github.com/go-playground/validator/v10/validator.go
deleted file mode 100644
index 80da095a..00000000
--- a/vendor/github.com/go-playground/validator/v10/validator.go
+++ /dev/null
@@ -1,486 +0,0 @@
-package validator
-
-import (
- "context"
- "fmt"
- "reflect"
- "strconv"
-)
-
-// per validate construct
-type validate struct {
- v *Validate
- top reflect.Value
- ns []byte
- actualNs []byte
- errs ValidationErrors
- includeExclude map[string]struct{} // reset only if StructPartial or StructExcept are called, no need otherwise
- ffn FilterFunc
- slflParent reflect.Value // StructLevel & FieldLevel
- slCurrent reflect.Value // StructLevel & FieldLevel
- flField reflect.Value // StructLevel & FieldLevel
- cf *cField // StructLevel & FieldLevel
- ct *cTag // StructLevel & FieldLevel
- misc []byte // misc reusable
- str1 string // misc reusable
- str2 string // misc reusable
- fldIsPointer bool // StructLevel & FieldLevel
- isPartial bool
- hasExcludes bool
-}
-
-// parent and current will be the same the first run of validateStruct
-func (v *validate) validateStruct(ctx context.Context, parent reflect.Value, current reflect.Value, typ reflect.Type, ns []byte, structNs []byte, ct *cTag) {
-
- cs, ok := v.v.structCache.Get(typ)
- if !ok {
- cs = v.v.extractStructCache(current, typ.Name())
- }
-
- if len(ns) == 0 && len(cs.name) != 0 {
-
- ns = append(ns, cs.name...)
- ns = append(ns, '.')
-
- structNs = append(structNs, cs.name...)
- structNs = append(structNs, '.')
- }
-
- // ct is nil on top level struct, and structs as fields that have no tag info
- // so if nil or if not nil and the structonly tag isn't present
- if ct == nil || ct.typeof != typeStructOnly {
-
- var f *cField
-
- for i := 0; i < len(cs.fields); i++ {
-
- f = cs.fields[i]
-
- if v.isPartial {
-
- if v.ffn != nil {
- // used with StructFiltered
- if v.ffn(append(structNs, f.name...)) {
- continue
- }
-
- } else {
- // used with StructPartial & StructExcept
- _, ok = v.includeExclude[string(append(structNs, f.name...))]
-
- if (ok && v.hasExcludes) || (!ok && !v.hasExcludes) {
- continue
- }
- }
- }
-
- v.traverseField(ctx, current, current.Field(f.idx), ns, structNs, f, f.cTags)
- }
- }
-
- // check if any struct level validations, after all field validations already checked.
- // first iteration will have no info about nostructlevel tag, and is checked prior to
- // calling the next iteration of validateStruct called from traverseField.
- if cs.fn != nil {
-
- v.slflParent = parent
- v.slCurrent = current
- v.ns = ns
- v.actualNs = structNs
-
- cs.fn(ctx, v)
- }
-}
-
-// traverseField validates any field, be it a struct or single field, ensures it's validity and passes it along to be validated via it's tag options
-func (v *validate) traverseField(ctx context.Context, parent reflect.Value, current reflect.Value, ns []byte, structNs []byte, cf *cField, ct *cTag) {
- var typ reflect.Type
- var kind reflect.Kind
-
- current, kind, v.fldIsPointer = v.extractTypeInternal(current, false)
-
- switch kind {
- case reflect.Ptr, reflect.Interface, reflect.Invalid:
-
- if ct == nil {
- return
- }
-
- if ct.typeof == typeOmitEmpty || ct.typeof == typeIsDefault {
- return
- }
-
- if ct.hasTag {
- if kind == reflect.Invalid {
- v.str1 = string(append(ns, cf.altName...))
- if v.v.hasTagNameFunc {
- v.str2 = string(append(structNs, cf.name...))
- } else {
- v.str2 = v.str1
- }
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: ct.aliasTag,
- actualTag: ct.tag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(cf.altName)),
- structfieldLen: uint8(len(cf.name)),
- param: ct.param,
- kind: kind,
- },
- )
- return
- }
-
- v.str1 = string(append(ns, cf.altName...))
- if v.v.hasTagNameFunc {
- v.str2 = string(append(structNs, cf.name...))
- } else {
- v.str2 = v.str1
- }
- if !ct.runValidationWhenNil {
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: ct.aliasTag,
- actualTag: ct.tag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(cf.altName)),
- structfieldLen: uint8(len(cf.name)),
- value: current.Interface(),
- param: ct.param,
- kind: kind,
- typ: current.Type(),
- },
- )
- return
- }
- }
-
- case reflect.Struct:
-
- typ = current.Type()
-
- if !typ.ConvertibleTo(timeType) {
-
- if ct != nil {
-
- if ct.typeof == typeStructOnly {
- goto CONTINUE
- } else if ct.typeof == typeIsDefault {
- // set Field Level fields
- v.slflParent = parent
- v.flField = current
- v.cf = cf
- v.ct = ct
-
- if !ct.fn(ctx, v) {
- v.str1 = string(append(ns, cf.altName...))
-
- if v.v.hasTagNameFunc {
- v.str2 = string(append(structNs, cf.name...))
- } else {
- v.str2 = v.str1
- }
-
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: ct.aliasTag,
- actualTag: ct.tag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(cf.altName)),
- structfieldLen: uint8(len(cf.name)),
- value: current.Interface(),
- param: ct.param,
- kind: kind,
- typ: typ,
- },
- )
- return
- }
- }
-
- ct = ct.next
- }
-
- if ct != nil && ct.typeof == typeNoStructLevel {
- return
- }
-
- CONTINUE:
- // if len == 0 then validating using 'Var' or 'VarWithValue'
- // Var - doesn't make much sense to do it that way, should call 'Struct', but no harm...
- // VarWithField - this allows for validating against each field within the struct against a specific value
- // pretty handy in certain situations
- if len(cf.name) > 0 {
- ns = append(append(ns, cf.altName...), '.')
- structNs = append(append(structNs, cf.name...), '.')
- }
-
- v.validateStruct(ctx, parent, current, typ, ns, structNs, ct)
- return
- }
- }
-
- if ct == nil || !ct.hasTag {
- return
- }
-
- typ = current.Type()
-
-OUTER:
- for {
- if ct == nil {
- return
- }
-
- switch ct.typeof {
-
- case typeOmitEmpty:
-
- // set Field Level fields
- v.slflParent = parent
- v.flField = current
- v.cf = cf
- v.ct = ct
-
- if !hasValue(v) {
- return
- }
-
- ct = ct.next
- continue
-
- case typeEndKeys:
- return
-
- case typeDive:
-
- ct = ct.next
-
- // traverse slice or map here
- // or panic ;)
- switch kind {
- case reflect.Slice, reflect.Array:
-
- var i64 int64
- reusableCF := &cField{}
-
- for i := 0; i < current.Len(); i++ {
-
- i64 = int64(i)
-
- v.misc = append(v.misc[0:0], cf.name...)
- v.misc = append(v.misc, '[')
- v.misc = strconv.AppendInt(v.misc, i64, 10)
- v.misc = append(v.misc, ']')
-
- reusableCF.name = string(v.misc)
-
- if cf.namesEqual {
- reusableCF.altName = reusableCF.name
- } else {
-
- v.misc = append(v.misc[0:0], cf.altName...)
- v.misc = append(v.misc, '[')
- v.misc = strconv.AppendInt(v.misc, i64, 10)
- v.misc = append(v.misc, ']')
-
- reusableCF.altName = string(v.misc)
- }
- v.traverseField(ctx, parent, current.Index(i), ns, structNs, reusableCF, ct)
- }
-
- case reflect.Map:
-
- var pv string
- reusableCF := &cField{}
-
- for _, key := range current.MapKeys() {
-
- pv = fmt.Sprintf("%v", key.Interface())
-
- v.misc = append(v.misc[0:0], cf.name...)
- v.misc = append(v.misc, '[')
- v.misc = append(v.misc, pv...)
- v.misc = append(v.misc, ']')
-
- reusableCF.name = string(v.misc)
-
- if cf.namesEqual {
- reusableCF.altName = reusableCF.name
- } else {
- v.misc = append(v.misc[0:0], cf.altName...)
- v.misc = append(v.misc, '[')
- v.misc = append(v.misc, pv...)
- v.misc = append(v.misc, ']')
-
- reusableCF.altName = string(v.misc)
- }
-
- if ct != nil && ct.typeof == typeKeys && ct.keys != nil {
- v.traverseField(ctx, parent, key, ns, structNs, reusableCF, ct.keys)
- // can be nil when just keys being validated
- if ct.next != nil {
- v.traverseField(ctx, parent, current.MapIndex(key), ns, structNs, reusableCF, ct.next)
- }
- } else {
- v.traverseField(ctx, parent, current.MapIndex(key), ns, structNs, reusableCF, ct)
- }
- }
-
- default:
- // throw error, if not a slice or map then should not have gotten here
- // bad dive tag
- panic("dive error! can't dive on a non slice or map")
- }
-
- return
-
- case typeOr:
-
- v.misc = v.misc[0:0]
-
- for {
-
- // set Field Level fields
- v.slflParent = parent
- v.flField = current
- v.cf = cf
- v.ct = ct
-
- if ct.fn(ctx, v) {
- if ct.isBlockEnd {
- ct = ct.next
- continue OUTER
- }
-
- // drain rest of the 'or' values, then continue or leave
- for {
-
- ct = ct.next
-
- if ct == nil {
- return
- }
-
- if ct.typeof != typeOr {
- continue OUTER
- }
-
- if ct.isBlockEnd {
- ct = ct.next
- continue OUTER
- }
- }
- }
-
- v.misc = append(v.misc, '|')
- v.misc = append(v.misc, ct.tag...)
-
- if ct.hasParam {
- v.misc = append(v.misc, '=')
- v.misc = append(v.misc, ct.param...)
- }
-
- if ct.isBlockEnd || ct.next == nil {
- // if we get here, no valid 'or' value and no more tags
- v.str1 = string(append(ns, cf.altName...))
-
- if v.v.hasTagNameFunc {
- v.str2 = string(append(structNs, cf.name...))
- } else {
- v.str2 = v.str1
- }
-
- if ct.hasAlias {
-
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: ct.aliasTag,
- actualTag: ct.actualAliasTag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(cf.altName)),
- structfieldLen: uint8(len(cf.name)),
- value: current.Interface(),
- param: ct.param,
- kind: kind,
- typ: typ,
- },
- )
-
- } else {
-
- tVal := string(v.misc)[1:]
-
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: tVal,
- actualTag: tVal,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(cf.altName)),
- structfieldLen: uint8(len(cf.name)),
- value: current.Interface(),
- param: ct.param,
- kind: kind,
- typ: typ,
- },
- )
- }
-
- return
- }
-
- ct = ct.next
- }
-
- default:
-
- // set Field Level fields
- v.slflParent = parent
- v.flField = current
- v.cf = cf
- v.ct = ct
-
- if !ct.fn(ctx, v) {
-
- v.str1 = string(append(ns, cf.altName...))
-
- if v.v.hasTagNameFunc {
- v.str2 = string(append(structNs, cf.name...))
- } else {
- v.str2 = v.str1
- }
-
- v.errs = append(v.errs,
- &fieldError{
- v: v.v,
- tag: ct.aliasTag,
- actualTag: ct.tag,
- ns: v.str1,
- structNs: v.str2,
- fieldLen: uint8(len(cf.altName)),
- structfieldLen: uint8(len(cf.name)),
- value: current.Interface(),
- param: ct.param,
- kind: kind,
- typ: typ,
- },
- )
-
- return
- }
- ct = ct.next
- }
- }
-
-}
diff --git a/vendor/github.com/go-playground/validator/v10/validator_instance.go b/vendor/github.com/go-playground/validator/v10/validator_instance.go
deleted file mode 100644
index 9493da49..00000000
--- a/vendor/github.com/go-playground/validator/v10/validator_instance.go
+++ /dev/null
@@ -1,699 +0,0 @@
-package validator
-
-import (
- "context"
- "errors"
- "fmt"
- "reflect"
- "strings"
- "sync"
- "time"
-
- ut "github.com/go-playground/universal-translator"
-)
-
-const (
- defaultTagName = "validate"
- utf8HexComma = "0x2C"
- utf8Pipe = "0x7C"
- tagSeparator = ","
- orSeparator = "|"
- tagKeySeparator = "="
- structOnlyTag = "structonly"
- noStructLevelTag = "nostructlevel"
- omitempty = "omitempty"
- isdefault = "isdefault"
- requiredWithoutAllTag = "required_without_all"
- requiredWithoutTag = "required_without"
- requiredWithTag = "required_with"
- requiredWithAllTag = "required_with_all"
- requiredIfTag = "required_if"
- requiredUnlessTag = "required_unless"
- excludedWithoutAllTag = "excluded_without_all"
- excludedWithoutTag = "excluded_without"
- excludedWithTag = "excluded_with"
- excludedWithAllTag = "excluded_with_all"
- excludedIfTag = "excluded_if"
- excludedUnlessTag = "excluded_unless"
- skipValidationTag = "-"
- diveTag = "dive"
- keysTag = "keys"
- endKeysTag = "endkeys"
- requiredTag = "required"
- namespaceSeparator = "."
- leftBracket = "["
- rightBracket = "]"
- restrictedTagChars = ".[],|=+()`~!@#$%^&*\\\"/?<>{}"
- restrictedAliasErr = "Alias '%s' either contains restricted characters or is the same as a restricted tag needed for normal operation"
- restrictedTagErr = "Tag '%s' either contains restricted characters or is the same as a restricted tag needed for normal operation"
-)
-
-var (
- timeDurationType = reflect.TypeOf(time.Duration(0))
- timeType = reflect.TypeOf(time.Time{})
-
- defaultCField = &cField{namesEqual: true}
-)
-
-// FilterFunc is the type used to filter fields using
-// StructFiltered(...) function.
-// returning true results in the field being filtered/skiped from
-// validation
-type FilterFunc func(ns []byte) bool
-
-// CustomTypeFunc allows for overriding or adding custom field type handler functions
-// field = field value of the type to return a value to be validated
-// example Valuer from sql drive see https://golang.org/src/database/sql/driver/types.go?s=1210:1293#L29
-type CustomTypeFunc func(field reflect.Value) interface{}
-
-// TagNameFunc allows for adding of a custom tag name parser
-type TagNameFunc func(field reflect.StructField) string
-
-type internalValidationFuncWrapper struct {
- fn FuncCtx
- runValidatinOnNil bool
-}
-
-// Validate contains the validator settings and cache
-type Validate struct {
- tagName string
- pool *sync.Pool
- hasCustomFuncs bool
- hasTagNameFunc bool
- tagNameFunc TagNameFunc
- structLevelFuncs map[reflect.Type]StructLevelFuncCtx
- customFuncs map[reflect.Type]CustomTypeFunc
- aliases map[string]string
- validations map[string]internalValidationFuncWrapper
- transTagFunc map[ut.Translator]map[string]TranslationFunc // map[]map[]TranslationFunc
- rules map[reflect.Type]map[string]string
- tagCache *tagCache
- structCache *structCache
-}
-
-// New returns a new instance of 'validate' with sane defaults.
-// Validate is designed to be thread-safe and used as a singleton instance.
-// It caches information about your struct and validations,
-// in essence only parsing your validation tags once per struct type.
-// Using multiple instances neglects the benefit of caching.
-func New() *Validate {
-
- tc := new(tagCache)
- tc.m.Store(make(map[string]*cTag))
-
- sc := new(structCache)
- sc.m.Store(make(map[reflect.Type]*cStruct))
-
- v := &Validate{
- tagName: defaultTagName,
- aliases: make(map[string]string, len(bakedInAliases)),
- validations: make(map[string]internalValidationFuncWrapper, len(bakedInValidators)),
- tagCache: tc,
- structCache: sc,
- }
-
- // must copy alias validators for separate validations to be used in each validator instance
- for k, val := range bakedInAliases {
- v.RegisterAlias(k, val)
- }
-
- // must copy validators for separate validations to be used in each instance
- for k, val := range bakedInValidators {
-
- switch k {
- // these require that even if the value is nil that the validation should run, omitempty still overrides this behaviour
- case requiredIfTag, requiredUnlessTag, requiredWithTag, requiredWithAllTag, requiredWithoutTag, requiredWithoutAllTag,
- excludedIfTag, excludedUnlessTag, excludedWithTag, excludedWithAllTag, excludedWithoutTag, excludedWithoutAllTag:
- _ = v.registerValidation(k, wrapFunc(val), true, true)
- default:
- // no need to error check here, baked in will always be valid
- _ = v.registerValidation(k, wrapFunc(val), true, false)
- }
- }
-
- v.pool = &sync.Pool{
- New: func() interface{} {
- return &validate{
- v: v,
- ns: make([]byte, 0, 64),
- actualNs: make([]byte, 0, 64),
- misc: make([]byte, 32),
- }
- },
- }
-
- return v
-}
-
-// SetTagName allows for changing of the default tag name of 'validate'
-func (v *Validate) SetTagName(name string) {
- v.tagName = name
-}
-
-// ValidateMapCtx validates a map using a map of validation rules and allows passing of contextual
-// validation validation information via context.Context.
-func (v Validate) ValidateMapCtx(ctx context.Context, data map[string]interface{}, rules map[string]interface{}) map[string]interface{} {
- errs := make(map[string]interface{})
- for field, rule := range rules {
- if ruleObj, ok := rule.(map[string]interface{}); ok {
- if dataObj, ok := data[field].(map[string]interface{}); ok {
- err := v.ValidateMapCtx(ctx, dataObj, ruleObj)
- if len(err) > 0 {
- errs[field] = err
- }
- } else if dataObjs, ok := data[field].([]map[string]interface{}); ok {
- for _, obj := range dataObjs {
- err := v.ValidateMapCtx(ctx, obj, ruleObj)
- if len(err) > 0 {
- errs[field] = err
- }
- }
- } else {
- errs[field] = errors.New("The field: '" + field + "' is not a map to dive")
- }
- } else if ruleStr, ok := rule.(string); ok {
- err := v.VarCtx(ctx, data[field], ruleStr)
- if err != nil {
- errs[field] = err
- }
- }
- }
- return errs
-}
-
-// ValidateMap validates map data from a map of tags
-func (v *Validate) ValidateMap(data map[string]interface{}, rules map[string]interface{}) map[string]interface{} {
- return v.ValidateMapCtx(context.Background(), data, rules)
-}
-
-// RegisterTagNameFunc registers a function to get alternate names for StructFields.
-//
-// eg. to use the names which have been specified for JSON representations of structs, rather than normal Go field names:
-//
-// validate.RegisterTagNameFunc(func(fld reflect.StructField) string {
-// name := strings.SplitN(fld.Tag.Get("json"), ",", 2)[0]
-// // skip if tag key says it should be ignored
-// if name == "-" {
-// return ""
-// }
-// return name
-// })
-func (v *Validate) RegisterTagNameFunc(fn TagNameFunc) {
- v.tagNameFunc = fn
- v.hasTagNameFunc = true
-}
-
-// RegisterValidation adds a validation with the given tag
-//
-// NOTES:
-// - if the key already exists, the previous validation function will be replaced.
-// - this method is not thread-safe it is intended that these all be registered prior to any validation
-func (v *Validate) RegisterValidation(tag string, fn Func, callValidationEvenIfNull ...bool) error {
- return v.RegisterValidationCtx(tag, wrapFunc(fn), callValidationEvenIfNull...)
-}
-
-// RegisterValidationCtx does the same as RegisterValidation on accepts a FuncCtx validation
-// allowing context.Context validation support.
-func (v *Validate) RegisterValidationCtx(tag string, fn FuncCtx, callValidationEvenIfNull ...bool) error {
- var nilCheckable bool
- if len(callValidationEvenIfNull) > 0 {
- nilCheckable = callValidationEvenIfNull[0]
- }
- return v.registerValidation(tag, fn, false, nilCheckable)
-}
-
-func (v *Validate) registerValidation(tag string, fn FuncCtx, bakedIn bool, nilCheckable bool) error {
- if len(tag) == 0 {
- return errors.New("function Key cannot be empty")
- }
-
- if fn == nil {
- return errors.New("function cannot be empty")
- }
-
- _, ok := restrictedTags[tag]
- if !bakedIn && (ok || strings.ContainsAny(tag, restrictedTagChars)) {
- panic(fmt.Sprintf(restrictedTagErr, tag))
- }
- v.validations[tag] = internalValidationFuncWrapper{fn: fn, runValidatinOnNil: nilCheckable}
- return nil
-}
-
-// RegisterAlias registers a mapping of a single validation tag that
-// defines a common or complex set of validation(s) to simplify adding validation
-// to structs.
-//
-// NOTE: this function is not thread-safe it is intended that these all be registered prior to any validation
-func (v *Validate) RegisterAlias(alias, tags string) {
-
- _, ok := restrictedTags[alias]
-
- if ok || strings.ContainsAny(alias, restrictedTagChars) {
- panic(fmt.Sprintf(restrictedAliasErr, alias))
- }
-
- v.aliases[alias] = tags
-}
-
-// RegisterStructValidation registers a StructLevelFunc against a number of types.
-//
-// NOTE:
-// - this method is not thread-safe it is intended that these all be registered prior to any validation
-func (v *Validate) RegisterStructValidation(fn StructLevelFunc, types ...interface{}) {
- v.RegisterStructValidationCtx(wrapStructLevelFunc(fn), types...)
-}
-
-// RegisterStructValidationCtx registers a StructLevelFuncCtx against a number of types and allows passing
-// of contextual validation information via context.Context.
-//
-// NOTE:
-// - this method is not thread-safe it is intended that these all be registered prior to any validation
-func (v *Validate) RegisterStructValidationCtx(fn StructLevelFuncCtx, types ...interface{}) {
-
- if v.structLevelFuncs == nil {
- v.structLevelFuncs = make(map[reflect.Type]StructLevelFuncCtx)
- }
-
- for _, t := range types {
- tv := reflect.ValueOf(t)
- if tv.Kind() == reflect.Ptr {
- t = reflect.Indirect(tv).Interface()
- }
-
- v.structLevelFuncs[reflect.TypeOf(t)] = fn
- }
-}
-
-// RegisterStructValidationMapRules registers validate map rules.
-// Be aware that map validation rules supersede those defined on a/the struct if present.
-//
-// NOTE: this method is not thread-safe it is intended that these all be registered prior to any validation
-func (v *Validate) RegisterStructValidationMapRules(rules map[string]string, types ...interface{}) {
- if v.rules == nil {
- v.rules = make(map[reflect.Type]map[string]string)
- }
-
- deepCopyRules := make(map[string]string)
- for i, rule := range rules {
- deepCopyRules[i] = rule
- }
-
- for _, t := range types {
- typ := reflect.TypeOf(t)
-
- if typ.Kind() == reflect.Ptr {
- typ = typ.Elem()
- }
-
- if typ.Kind() != reflect.Struct {
- continue
- }
- v.rules[typ] = deepCopyRules
- }
-}
-
-// RegisterCustomTypeFunc registers a CustomTypeFunc against a number of types
-//
-// NOTE: this method is not thread-safe it is intended that these all be registered prior to any validation
-func (v *Validate) RegisterCustomTypeFunc(fn CustomTypeFunc, types ...interface{}) {
-
- if v.customFuncs == nil {
- v.customFuncs = make(map[reflect.Type]CustomTypeFunc)
- }
-
- for _, t := range types {
- v.customFuncs[reflect.TypeOf(t)] = fn
- }
-
- v.hasCustomFuncs = true
-}
-
-// RegisterTranslation registers translations against the provided tag.
-func (v *Validate) RegisterTranslation(tag string, trans ut.Translator, registerFn RegisterTranslationsFunc, translationFn TranslationFunc) (err error) {
-
- if v.transTagFunc == nil {
- v.transTagFunc = make(map[ut.Translator]map[string]TranslationFunc)
- }
-
- if err = registerFn(trans); err != nil {
- return
- }
-
- m, ok := v.transTagFunc[trans]
- if !ok {
- m = make(map[string]TranslationFunc)
- v.transTagFunc[trans] = m
- }
-
- m[tag] = translationFn
-
- return
-}
-
-// Struct validates a structs exposed fields, and automatically validates nested structs, unless otherwise specified.
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) Struct(s interface{}) error {
- return v.StructCtx(context.Background(), s)
-}
-
-// StructCtx validates a structs exposed fields, and automatically validates nested structs, unless otherwise specified
-// and also allows passing of context.Context for contextual validation information.
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructCtx(ctx context.Context, s interface{}) (err error) {
-
- val := reflect.ValueOf(s)
- top := val
-
- if val.Kind() == reflect.Ptr && !val.IsNil() {
- val = val.Elem()
- }
-
- if val.Kind() != reflect.Struct || val.Type().ConvertibleTo(timeType) {
- return &InvalidValidationError{Type: reflect.TypeOf(s)}
- }
-
- // good to validate
- vd := v.pool.Get().(*validate)
- vd.top = top
- vd.isPartial = false
- // vd.hasExcludes = false // only need to reset in StructPartial and StructExcept
-
- vd.validateStruct(ctx, top, val, val.Type(), vd.ns[0:0], vd.actualNs[0:0], nil)
-
- if len(vd.errs) > 0 {
- err = vd.errs
- vd.errs = nil
- }
-
- v.pool.Put(vd)
-
- return
-}
-
-// StructFiltered validates a structs exposed fields, that pass the FilterFunc check and automatically validates
-// nested structs, unless otherwise specified.
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructFiltered(s interface{}, fn FilterFunc) error {
- return v.StructFilteredCtx(context.Background(), s, fn)
-}
-
-// StructFilteredCtx validates a structs exposed fields, that pass the FilterFunc check and automatically validates
-// nested structs, unless otherwise specified and also allows passing of contextual validation information via
-// context.Context
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructFilteredCtx(ctx context.Context, s interface{}, fn FilterFunc) (err error) {
- val := reflect.ValueOf(s)
- top := val
-
- if val.Kind() == reflect.Ptr && !val.IsNil() {
- val = val.Elem()
- }
-
- if val.Kind() != reflect.Struct || val.Type().ConvertibleTo(timeType) {
- return &InvalidValidationError{Type: reflect.TypeOf(s)}
- }
-
- // good to validate
- vd := v.pool.Get().(*validate)
- vd.top = top
- vd.isPartial = true
- vd.ffn = fn
- // vd.hasExcludes = false // only need to reset in StructPartial and StructExcept
-
- vd.validateStruct(ctx, top, val, val.Type(), vd.ns[0:0], vd.actualNs[0:0], nil)
-
- if len(vd.errs) > 0 {
- err = vd.errs
- vd.errs = nil
- }
-
- v.pool.Put(vd)
-
- return
-}
-
-// StructPartial validates the fields passed in only, ignoring all others.
-// Fields may be provided in a namespaced fashion relative to the struct provided
-// eg. NestedStruct.Field or NestedArrayField[0].Struct.Name
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructPartial(s interface{}, fields ...string) error {
- return v.StructPartialCtx(context.Background(), s, fields...)
-}
-
-// StructPartialCtx validates the fields passed in only, ignoring all others and allows passing of contextual
-// validation validation information via context.Context
-// Fields may be provided in a namespaced fashion relative to the struct provided
-// eg. NestedStruct.Field or NestedArrayField[0].Struct.Name
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructPartialCtx(ctx context.Context, s interface{}, fields ...string) (err error) {
- val := reflect.ValueOf(s)
- top := val
-
- if val.Kind() == reflect.Ptr && !val.IsNil() {
- val = val.Elem()
- }
-
- if val.Kind() != reflect.Struct || val.Type().ConvertibleTo(timeType) {
- return &InvalidValidationError{Type: reflect.TypeOf(s)}
- }
-
- // good to validate
- vd := v.pool.Get().(*validate)
- vd.top = top
- vd.isPartial = true
- vd.ffn = nil
- vd.hasExcludes = false
- vd.includeExclude = make(map[string]struct{})
-
- typ := val.Type()
- name := typ.Name()
-
- for _, k := range fields {
-
- flds := strings.Split(k, namespaceSeparator)
- if len(flds) > 0 {
-
- vd.misc = append(vd.misc[0:0], name...)
- // Don't append empty name for unnamed structs
- if len(vd.misc) != 0 {
- vd.misc = append(vd.misc, '.')
- }
-
- for _, s := range flds {
-
- idx := strings.Index(s, leftBracket)
-
- if idx != -1 {
- for idx != -1 {
- vd.misc = append(vd.misc, s[:idx]...)
- vd.includeExclude[string(vd.misc)] = struct{}{}
-
- idx2 := strings.Index(s, rightBracket)
- idx2++
- vd.misc = append(vd.misc, s[idx:idx2]...)
- vd.includeExclude[string(vd.misc)] = struct{}{}
- s = s[idx2:]
- idx = strings.Index(s, leftBracket)
- }
- } else {
-
- vd.misc = append(vd.misc, s...)
- vd.includeExclude[string(vd.misc)] = struct{}{}
- }
-
- vd.misc = append(vd.misc, '.')
- }
- }
- }
-
- vd.validateStruct(ctx, top, val, typ, vd.ns[0:0], vd.actualNs[0:0], nil)
-
- if len(vd.errs) > 0 {
- err = vd.errs
- vd.errs = nil
- }
-
- v.pool.Put(vd)
-
- return
-}
-
-// StructExcept validates all fields except the ones passed in.
-// Fields may be provided in a namespaced fashion relative to the struct provided
-// i.e. NestedStruct.Field or NestedArrayField[0].Struct.Name
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructExcept(s interface{}, fields ...string) error {
- return v.StructExceptCtx(context.Background(), s, fields...)
-}
-
-// StructExceptCtx validates all fields except the ones passed in and allows passing of contextual
-// validation validation information via context.Context
-// Fields may be provided in a namespaced fashion relative to the struct provided
-// i.e. NestedStruct.Field or NestedArrayField[0].Struct.Name
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-func (v *Validate) StructExceptCtx(ctx context.Context, s interface{}, fields ...string) (err error) {
- val := reflect.ValueOf(s)
- top := val
-
- if val.Kind() == reflect.Ptr && !val.IsNil() {
- val = val.Elem()
- }
-
- if val.Kind() != reflect.Struct || val.Type().ConvertibleTo(timeType) {
- return &InvalidValidationError{Type: reflect.TypeOf(s)}
- }
-
- // good to validate
- vd := v.pool.Get().(*validate)
- vd.top = top
- vd.isPartial = true
- vd.ffn = nil
- vd.hasExcludes = true
- vd.includeExclude = make(map[string]struct{})
-
- typ := val.Type()
- name := typ.Name()
-
- for _, key := range fields {
-
- vd.misc = vd.misc[0:0]
-
- if len(name) > 0 {
- vd.misc = append(vd.misc, name...)
- vd.misc = append(vd.misc, '.')
- }
-
- vd.misc = append(vd.misc, key...)
- vd.includeExclude[string(vd.misc)] = struct{}{}
- }
-
- vd.validateStruct(ctx, top, val, typ, vd.ns[0:0], vd.actualNs[0:0], nil)
-
- if len(vd.errs) > 0 {
- err = vd.errs
- vd.errs = nil
- }
-
- v.pool.Put(vd)
-
- return
-}
-
-// Var validates a single variable using tag style validation.
-// eg.
-// var i int
-// validate.Var(i, "gt=1,lt=10")
-//
-// WARNING: a struct can be passed for validation eg. time.Time is a struct or
-// if you have a custom type and have registered a custom type handler, so must
-// allow it; however unforeseen validations will occur if trying to validate a
-// struct that is meant to be passed to 'validate.Struct'
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-// validate Array, Slice and maps fields which may contain more than one error
-func (v *Validate) Var(field interface{}, tag string) error {
- return v.VarCtx(context.Background(), field, tag)
-}
-
-// VarCtx validates a single variable using tag style validation and allows passing of contextual
-// validation validation information via context.Context.
-// eg.
-// var i int
-// validate.Var(i, "gt=1,lt=10")
-//
-// WARNING: a struct can be passed for validation eg. time.Time is a struct or
-// if you have a custom type and have registered a custom type handler, so must
-// allow it; however unforeseen validations will occur if trying to validate a
-// struct that is meant to be passed to 'validate.Struct'
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-// validate Array, Slice and maps fields which may contain more than one error
-func (v *Validate) VarCtx(ctx context.Context, field interface{}, tag string) (err error) {
- if len(tag) == 0 || tag == skipValidationTag {
- return nil
- }
-
- ctag := v.fetchCacheTag(tag)
- val := reflect.ValueOf(field)
- vd := v.pool.Get().(*validate)
- vd.top = val
- vd.isPartial = false
- vd.traverseField(ctx, val, val, vd.ns[0:0], vd.actualNs[0:0], defaultCField, ctag)
-
- if len(vd.errs) > 0 {
- err = vd.errs
- vd.errs = nil
- }
- v.pool.Put(vd)
- return
-}
-
-// VarWithValue validates a single variable, against another variable/field's value using tag style validation
-// eg.
-// s1 := "abcd"
-// s2 := "abcd"
-// validate.VarWithValue(s1, s2, "eqcsfield") // returns true
-//
-// WARNING: a struct can be passed for validation eg. time.Time is a struct or
-// if you have a custom type and have registered a custom type handler, so must
-// allow it; however unforeseen validations will occur if trying to validate a
-// struct that is meant to be passed to 'validate.Struct'
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-// validate Array, Slice and maps fields which may contain more than one error
-func (v *Validate) VarWithValue(field interface{}, other interface{}, tag string) error {
- return v.VarWithValueCtx(context.Background(), field, other, tag)
-}
-
-// VarWithValueCtx validates a single variable, against another variable/field's value using tag style validation and
-// allows passing of contextual validation validation information via context.Context.
-// eg.
-// s1 := "abcd"
-// s2 := "abcd"
-// validate.VarWithValue(s1, s2, "eqcsfield") // returns true
-//
-// WARNING: a struct can be passed for validation eg. time.Time is a struct or
-// if you have a custom type and have registered a custom type handler, so must
-// allow it; however unforeseen validations will occur if trying to validate a
-// struct that is meant to be passed to 'validate.Struct'
-//
-// It returns InvalidValidationError for bad values passed in and nil or ValidationErrors as error otherwise.
-// You will need to assert the error if it's not nil eg. err.(validator.ValidationErrors) to access the array of errors.
-// validate Array, Slice and maps fields which may contain more than one error
-func (v *Validate) VarWithValueCtx(ctx context.Context, field interface{}, other interface{}, tag string) (err error) {
- if len(tag) == 0 || tag == skipValidationTag {
- return nil
- }
- ctag := v.fetchCacheTag(tag)
- otherVal := reflect.ValueOf(other)
- vd := v.pool.Get().(*validate)
- vd.top = otherVal
- vd.isPartial = false
- vd.traverseField(ctx, otherVal, reflect.ValueOf(field), vd.ns[0:0], vd.actualNs[0:0], defaultCField, ctag)
-
- if len(vd.errs) > 0 {
- err = vd.errs
- vd.errs = nil
- }
- v.pool.Put(vd)
- return
-}
diff --git a/vendor/github.com/golang/protobuf/AUTHORS b/vendor/github.com/golang/protobuf/AUTHORS
deleted file mode 100644
index 15167cd7..00000000
--- a/vendor/github.com/golang/protobuf/AUTHORS
+++ /dev/null
@@ -1,3 +0,0 @@
-# This source code refers to The Go Authors for copyright purposes.
-# The master list of authors is in the main Go distribution,
-# visible at http://tip.golang.org/AUTHORS.
diff --git a/vendor/github.com/golang/protobuf/CONTRIBUTORS b/vendor/github.com/golang/protobuf/CONTRIBUTORS
deleted file mode 100644
index 1c4577e9..00000000
--- a/vendor/github.com/golang/protobuf/CONTRIBUTORS
+++ /dev/null
@@ -1,3 +0,0 @@
-# This source code was written by the Go contributors.
-# The master list of contributors is in the main Go distribution,
-# visible at http://tip.golang.org/CONTRIBUTORS.
diff --git a/vendor/github.com/golang/protobuf/LICENSE b/vendor/github.com/golang/protobuf/LICENSE
deleted file mode 100644
index 0f646931..00000000
--- a/vendor/github.com/golang/protobuf/LICENSE
+++ /dev/null
@@ -1,28 +0,0 @@
-Copyright 2010 The Go Authors. All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are
-met:
-
- * Redistributions of source code must retain the above copyright
-notice, this list of conditions and the following disclaimer.
- * Redistributions in binary form must reproduce the above
-copyright notice, this list of conditions and the following disclaimer
-in the documentation and/or other materials provided with the
-distribution.
- * Neither the name of Google Inc. nor the names of its
-contributors may be used to endorse or promote products derived from
-this software without specific prior written permission.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
-"AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
-LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
-A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
-OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
-SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
-LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
-DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
-THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
-
diff --git a/vendor/github.com/golang/protobuf/jsonpb/decode.go b/vendor/github.com/golang/protobuf/jsonpb/decode.go
deleted file mode 100644
index 6c16c255..00000000
--- a/vendor/github.com/golang/protobuf/jsonpb/decode.go
+++ /dev/null
@@ -1,530 +0,0 @@
-// Copyright 2015 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package jsonpb
-
-import (
- "encoding/json"
- "errors"
- "fmt"
- "io"
- "math"
- "reflect"
- "strconv"
- "strings"
- "time"
-
- "github.com/golang/protobuf/proto"
- "google.golang.org/protobuf/encoding/protojson"
- protoV2 "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
-)
-
-const wrapJSONUnmarshalV2 = false
-
-// UnmarshalNext unmarshals the next JSON object from d into m.
-func UnmarshalNext(d *json.Decoder, m proto.Message) error {
- return new(Unmarshaler).UnmarshalNext(d, m)
-}
-
-// Unmarshal unmarshals a JSON object from r into m.
-func Unmarshal(r io.Reader, m proto.Message) error {
- return new(Unmarshaler).Unmarshal(r, m)
-}
-
-// UnmarshalString unmarshals a JSON object from s into m.
-func UnmarshalString(s string, m proto.Message) error {
- return new(Unmarshaler).Unmarshal(strings.NewReader(s), m)
-}
-
-// Unmarshaler is a configurable object for converting from a JSON
-// representation to a protocol buffer object.
-type Unmarshaler struct {
- // AllowUnknownFields specifies whether to allow messages to contain
- // unknown JSON fields, as opposed to failing to unmarshal.
- AllowUnknownFields bool
-
- // AnyResolver is used to resolve the google.protobuf.Any well-known type.
- // If unset, the global registry is used by default.
- AnyResolver AnyResolver
-}
-
-// JSONPBUnmarshaler is implemented by protobuf messages that customize the way
-// they are unmarshaled from JSON. Messages that implement this should also
-// implement JSONPBMarshaler so that the custom format can be produced.
-//
-// The JSON unmarshaling must follow the JSON to proto specification:
-// https://developers.google.com/protocol-buffers/docs/proto3#json
-//
-// Deprecated: Custom types should implement protobuf reflection instead.
-type JSONPBUnmarshaler interface {
- UnmarshalJSONPB(*Unmarshaler, []byte) error
-}
-
-// Unmarshal unmarshals a JSON object from r into m.
-func (u *Unmarshaler) Unmarshal(r io.Reader, m proto.Message) error {
- return u.UnmarshalNext(json.NewDecoder(r), m)
-}
-
-// UnmarshalNext unmarshals the next JSON object from d into m.
-func (u *Unmarshaler) UnmarshalNext(d *json.Decoder, m proto.Message) error {
- if m == nil {
- return errors.New("invalid nil message")
- }
-
- // Parse the next JSON object from the stream.
- raw := json.RawMessage{}
- if err := d.Decode(&raw); err != nil {
- return err
- }
-
- // Check for custom unmarshalers first since they may not properly
- // implement protobuf reflection that the logic below relies on.
- if jsu, ok := m.(JSONPBUnmarshaler); ok {
- return jsu.UnmarshalJSONPB(u, raw)
- }
-
- mr := proto.MessageReflect(m)
-
- // NOTE: For historical reasons, a top-level null is treated as a noop.
- // This is incorrect, but kept for compatibility.
- if string(raw) == "null" && mr.Descriptor().FullName() != "google.protobuf.Value" {
- return nil
- }
-
- if wrapJSONUnmarshalV2 {
- // NOTE: If input message is non-empty, we need to preserve merge semantics
- // of the old jsonpb implementation. These semantics are not supported by
- // the protobuf JSON specification.
- isEmpty := true
- mr.Range(func(protoreflect.FieldDescriptor, protoreflect.Value) bool {
- isEmpty = false // at least one iteration implies non-empty
- return false
- })
- if !isEmpty {
- // Perform unmarshaling into a newly allocated, empty message.
- mr = mr.New()
-
- // Use a defer to copy all unmarshaled fields into the original message.
- dst := proto.MessageReflect(m)
- defer mr.Range(func(fd protoreflect.FieldDescriptor, v protoreflect.Value) bool {
- dst.Set(fd, v)
- return true
- })
- }
-
- // Unmarshal using the v2 JSON unmarshaler.
- opts := protojson.UnmarshalOptions{
- DiscardUnknown: u.AllowUnknownFields,
- }
- if u.AnyResolver != nil {
- opts.Resolver = anyResolver{u.AnyResolver}
- }
- return opts.Unmarshal(raw, mr.Interface())
- } else {
- if err := u.unmarshalMessage(mr, raw); err != nil {
- return err
- }
- return protoV2.CheckInitialized(mr.Interface())
- }
-}
-
-func (u *Unmarshaler) unmarshalMessage(m protoreflect.Message, in []byte) error {
- md := m.Descriptor()
- fds := md.Fields()
-
- if jsu, ok := proto.MessageV1(m.Interface()).(JSONPBUnmarshaler); ok {
- return jsu.UnmarshalJSONPB(u, in)
- }
-
- if string(in) == "null" && md.FullName() != "google.protobuf.Value" {
- return nil
- }
-
- switch wellKnownType(md.FullName()) {
- case "Any":
- var jsonObject map[string]json.RawMessage
- if err := json.Unmarshal(in, &jsonObject); err != nil {
- return err
- }
-
- rawTypeURL, ok := jsonObject["@type"]
- if !ok {
- return errors.New("Any JSON doesn't have '@type'")
- }
- typeURL, err := unquoteString(string(rawTypeURL))
- if err != nil {
- return fmt.Errorf("can't unmarshal Any's '@type': %q", rawTypeURL)
- }
- m.Set(fds.ByNumber(1), protoreflect.ValueOfString(typeURL))
-
- var m2 protoreflect.Message
- if u.AnyResolver != nil {
- mi, err := u.AnyResolver.Resolve(typeURL)
- if err != nil {
- return err
- }
- m2 = proto.MessageReflect(mi)
- } else {
- mt, err := protoregistry.GlobalTypes.FindMessageByURL(typeURL)
- if err != nil {
- if err == protoregistry.NotFound {
- return fmt.Errorf("could not resolve Any message type: %v", typeURL)
- }
- return err
- }
- m2 = mt.New()
- }
-
- if wellKnownType(m2.Descriptor().FullName()) != "" {
- rawValue, ok := jsonObject["value"]
- if !ok {
- return errors.New("Any JSON doesn't have 'value'")
- }
- if err := u.unmarshalMessage(m2, rawValue); err != nil {
- return fmt.Errorf("can't unmarshal Any nested proto %v: %v", typeURL, err)
- }
- } else {
- delete(jsonObject, "@type")
- rawJSON, err := json.Marshal(jsonObject)
- if err != nil {
- return fmt.Errorf("can't generate JSON for Any's nested proto to be unmarshaled: %v", err)
- }
- if err = u.unmarshalMessage(m2, rawJSON); err != nil {
- return fmt.Errorf("can't unmarshal Any nested proto %v: %v", typeURL, err)
- }
- }
-
- rawWire, err := protoV2.Marshal(m2.Interface())
- if err != nil {
- return fmt.Errorf("can't marshal proto %v into Any.Value: %v", typeURL, err)
- }
- m.Set(fds.ByNumber(2), protoreflect.ValueOfBytes(rawWire))
- return nil
- case "BoolValue", "BytesValue", "StringValue",
- "Int32Value", "UInt32Value", "FloatValue",
- "Int64Value", "UInt64Value", "DoubleValue":
- fd := fds.ByNumber(1)
- v, err := u.unmarshalValue(m.NewField(fd), in, fd)
- if err != nil {
- return err
- }
- m.Set(fd, v)
- return nil
- case "Duration":
- v, err := unquoteString(string(in))
- if err != nil {
- return err
- }
- d, err := time.ParseDuration(v)
- if err != nil {
- return fmt.Errorf("bad Duration: %v", err)
- }
-
- sec := d.Nanoseconds() / 1e9
- nsec := d.Nanoseconds() % 1e9
- m.Set(fds.ByNumber(1), protoreflect.ValueOfInt64(int64(sec)))
- m.Set(fds.ByNumber(2), protoreflect.ValueOfInt32(int32(nsec)))
- return nil
- case "Timestamp":
- v, err := unquoteString(string(in))
- if err != nil {
- return err
- }
- t, err := time.Parse(time.RFC3339Nano, v)
- if err != nil {
- return fmt.Errorf("bad Timestamp: %v", err)
- }
-
- sec := t.Unix()
- nsec := t.Nanosecond()
- m.Set(fds.ByNumber(1), protoreflect.ValueOfInt64(int64(sec)))
- m.Set(fds.ByNumber(2), protoreflect.ValueOfInt32(int32(nsec)))
- return nil
- case "Value":
- switch {
- case string(in) == "null":
- m.Set(fds.ByNumber(1), protoreflect.ValueOfEnum(0))
- case string(in) == "true":
- m.Set(fds.ByNumber(4), protoreflect.ValueOfBool(true))
- case string(in) == "false":
- m.Set(fds.ByNumber(4), protoreflect.ValueOfBool(false))
- case hasPrefixAndSuffix('"', in, '"'):
- s, err := unquoteString(string(in))
- if err != nil {
- return fmt.Errorf("unrecognized type for Value %q", in)
- }
- m.Set(fds.ByNumber(3), protoreflect.ValueOfString(s))
- case hasPrefixAndSuffix('[', in, ']'):
- v := m.Mutable(fds.ByNumber(6))
- return u.unmarshalMessage(v.Message(), in)
- case hasPrefixAndSuffix('{', in, '}'):
- v := m.Mutable(fds.ByNumber(5))
- return u.unmarshalMessage(v.Message(), in)
- default:
- f, err := strconv.ParseFloat(string(in), 0)
- if err != nil {
- return fmt.Errorf("unrecognized type for Value %q", in)
- }
- m.Set(fds.ByNumber(2), protoreflect.ValueOfFloat64(f))
- }
- return nil
- case "ListValue":
- var jsonArray []json.RawMessage
- if err := json.Unmarshal(in, &jsonArray); err != nil {
- return fmt.Errorf("bad ListValue: %v", err)
- }
-
- lv := m.Mutable(fds.ByNumber(1)).List()
- for _, raw := range jsonArray {
- ve := lv.NewElement()
- if err := u.unmarshalMessage(ve.Message(), raw); err != nil {
- return err
- }
- lv.Append(ve)
- }
- return nil
- case "Struct":
- var jsonObject map[string]json.RawMessage
- if err := json.Unmarshal(in, &jsonObject); err != nil {
- return fmt.Errorf("bad StructValue: %v", err)
- }
-
- mv := m.Mutable(fds.ByNumber(1)).Map()
- for key, raw := range jsonObject {
- kv := protoreflect.ValueOf(key).MapKey()
- vv := mv.NewValue()
- if err := u.unmarshalMessage(vv.Message(), raw); err != nil {
- return fmt.Errorf("bad value in StructValue for key %q: %v", key, err)
- }
- mv.Set(kv, vv)
- }
- return nil
- }
-
- var jsonObject map[string]json.RawMessage
- if err := json.Unmarshal(in, &jsonObject); err != nil {
- return err
- }
-
- // Handle known fields.
- for i := 0; i < fds.Len(); i++ {
- fd := fds.Get(i)
- if fd.IsWeak() && fd.Message().IsPlaceholder() {
- continue // weak reference is not linked in
- }
-
- // Search for any raw JSON value associated with this field.
- var raw json.RawMessage
- name := string(fd.Name())
- if fd.Kind() == protoreflect.GroupKind {
- name = string(fd.Message().Name())
- }
- if v, ok := jsonObject[name]; ok {
- delete(jsonObject, name)
- raw = v
- }
- name = string(fd.JSONName())
- if v, ok := jsonObject[name]; ok {
- delete(jsonObject, name)
- raw = v
- }
-
- field := m.NewField(fd)
- // Unmarshal the field value.
- if raw == nil || (string(raw) == "null" && !isSingularWellKnownValue(fd) && !isSingularJSONPBUnmarshaler(field, fd)) {
- continue
- }
- v, err := u.unmarshalValue(field, raw, fd)
- if err != nil {
- return err
- }
- m.Set(fd, v)
- }
-
- // Handle extension fields.
- for name, raw := range jsonObject {
- if !strings.HasPrefix(name, "[") || !strings.HasSuffix(name, "]") {
- continue
- }
-
- // Resolve the extension field by name.
- xname := protoreflect.FullName(name[len("[") : len(name)-len("]")])
- xt, _ := protoregistry.GlobalTypes.FindExtensionByName(xname)
- if xt == nil && isMessageSet(md) {
- xt, _ = protoregistry.GlobalTypes.FindExtensionByName(xname.Append("message_set_extension"))
- }
- if xt == nil {
- continue
- }
- delete(jsonObject, name)
- fd := xt.TypeDescriptor()
- if fd.ContainingMessage().FullName() != m.Descriptor().FullName() {
- return fmt.Errorf("extension field %q does not extend message %q", xname, m.Descriptor().FullName())
- }
-
- field := m.NewField(fd)
- // Unmarshal the field value.
- if raw == nil || (string(raw) == "null" && !isSingularWellKnownValue(fd) && !isSingularJSONPBUnmarshaler(field, fd)) {
- continue
- }
- v, err := u.unmarshalValue(field, raw, fd)
- if err != nil {
- return err
- }
- m.Set(fd, v)
- }
-
- if !u.AllowUnknownFields && len(jsonObject) > 0 {
- for name := range jsonObject {
- return fmt.Errorf("unknown field %q in %v", name, md.FullName())
- }
- }
- return nil
-}
-
-func isSingularWellKnownValue(fd protoreflect.FieldDescriptor) bool {
- if fd.Cardinality() == protoreflect.Repeated {
- return false
- }
- if md := fd.Message(); md != nil {
- return md.FullName() == "google.protobuf.Value"
- }
- if ed := fd.Enum(); ed != nil {
- return ed.FullName() == "google.protobuf.NullValue"
- }
- return false
-}
-
-func isSingularJSONPBUnmarshaler(v protoreflect.Value, fd protoreflect.FieldDescriptor) bool {
- if fd.Message() != nil && fd.Cardinality() != protoreflect.Repeated {
- _, ok := proto.MessageV1(v.Interface()).(JSONPBUnmarshaler)
- return ok
- }
- return false
-}
-
-func (u *Unmarshaler) unmarshalValue(v protoreflect.Value, in []byte, fd protoreflect.FieldDescriptor) (protoreflect.Value, error) {
- switch {
- case fd.IsList():
- var jsonArray []json.RawMessage
- if err := json.Unmarshal(in, &jsonArray); err != nil {
- return v, err
- }
- lv := v.List()
- for _, raw := range jsonArray {
- ve, err := u.unmarshalSingularValue(lv.NewElement(), raw, fd)
- if err != nil {
- return v, err
- }
- lv.Append(ve)
- }
- return v, nil
- case fd.IsMap():
- var jsonObject map[string]json.RawMessage
- if err := json.Unmarshal(in, &jsonObject); err != nil {
- return v, err
- }
- kfd := fd.MapKey()
- vfd := fd.MapValue()
- mv := v.Map()
- for key, raw := range jsonObject {
- var kv protoreflect.MapKey
- if kfd.Kind() == protoreflect.StringKind {
- kv = protoreflect.ValueOf(key).MapKey()
- } else {
- v, err := u.unmarshalSingularValue(kfd.Default(), []byte(key), kfd)
- if err != nil {
- return v, err
- }
- kv = v.MapKey()
- }
-
- vv, err := u.unmarshalSingularValue(mv.NewValue(), raw, vfd)
- if err != nil {
- return v, err
- }
- mv.Set(kv, vv)
- }
- return v, nil
- default:
- return u.unmarshalSingularValue(v, in, fd)
- }
-}
-
-var nonFinite = map[string]float64{
- `"NaN"`: math.NaN(),
- `"Infinity"`: math.Inf(+1),
- `"-Infinity"`: math.Inf(-1),
-}
-
-func (u *Unmarshaler) unmarshalSingularValue(v protoreflect.Value, in []byte, fd protoreflect.FieldDescriptor) (protoreflect.Value, error) {
- switch fd.Kind() {
- case protoreflect.BoolKind:
- return unmarshalValue(in, new(bool))
- case protoreflect.Int32Kind, protoreflect.Sint32Kind, protoreflect.Sfixed32Kind:
- return unmarshalValue(trimQuote(in), new(int32))
- case protoreflect.Int64Kind, protoreflect.Sint64Kind, protoreflect.Sfixed64Kind:
- return unmarshalValue(trimQuote(in), new(int64))
- case protoreflect.Uint32Kind, protoreflect.Fixed32Kind:
- return unmarshalValue(trimQuote(in), new(uint32))
- case protoreflect.Uint64Kind, protoreflect.Fixed64Kind:
- return unmarshalValue(trimQuote(in), new(uint64))
- case protoreflect.FloatKind:
- if f, ok := nonFinite[string(in)]; ok {
- return protoreflect.ValueOfFloat32(float32(f)), nil
- }
- return unmarshalValue(trimQuote(in), new(float32))
- case protoreflect.DoubleKind:
- if f, ok := nonFinite[string(in)]; ok {
- return protoreflect.ValueOfFloat64(float64(f)), nil
- }
- return unmarshalValue(trimQuote(in), new(float64))
- case protoreflect.StringKind:
- return unmarshalValue(in, new(string))
- case protoreflect.BytesKind:
- return unmarshalValue(in, new([]byte))
- case protoreflect.EnumKind:
- if hasPrefixAndSuffix('"', in, '"') {
- vd := fd.Enum().Values().ByName(protoreflect.Name(trimQuote(in)))
- if vd == nil {
- return v, fmt.Errorf("unknown value %q for enum %s", in, fd.Enum().FullName())
- }
- return protoreflect.ValueOfEnum(vd.Number()), nil
- }
- return unmarshalValue(in, new(protoreflect.EnumNumber))
- case protoreflect.MessageKind, protoreflect.GroupKind:
- err := u.unmarshalMessage(v.Message(), in)
- return v, err
- default:
- panic(fmt.Sprintf("invalid kind %v", fd.Kind()))
- }
-}
-
-func unmarshalValue(in []byte, v interface{}) (protoreflect.Value, error) {
- err := json.Unmarshal(in, v)
- return protoreflect.ValueOf(reflect.ValueOf(v).Elem().Interface()), err
-}
-
-func unquoteString(in string) (out string, err error) {
- err = json.Unmarshal([]byte(in), &out)
- return out, err
-}
-
-func hasPrefixAndSuffix(prefix byte, in []byte, suffix byte) bool {
- if len(in) >= 2 && in[0] == prefix && in[len(in)-1] == suffix {
- return true
- }
- return false
-}
-
-// trimQuote is like unquoteString but simply strips surrounding quotes.
-// This is incorrect, but is behavior done by the legacy implementation.
-func trimQuote(in []byte) []byte {
- if len(in) >= 2 && in[0] == '"' && in[len(in)-1] == '"' {
- in = in[1 : len(in)-1]
- }
- return in
-}
diff --git a/vendor/github.com/golang/protobuf/jsonpb/encode.go b/vendor/github.com/golang/protobuf/jsonpb/encode.go
deleted file mode 100644
index 685c80a6..00000000
--- a/vendor/github.com/golang/protobuf/jsonpb/encode.go
+++ /dev/null
@@ -1,559 +0,0 @@
-// Copyright 2015 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package jsonpb
-
-import (
- "encoding/json"
- "errors"
- "fmt"
- "io"
- "math"
- "reflect"
- "sort"
- "strconv"
- "strings"
- "time"
-
- "github.com/golang/protobuf/proto"
- "google.golang.org/protobuf/encoding/protojson"
- protoV2 "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
-)
-
-const wrapJSONMarshalV2 = false
-
-// Marshaler is a configurable object for marshaling protocol buffer messages
-// to the specified JSON representation.
-type Marshaler struct {
- // OrigName specifies whether to use the original protobuf name for fields.
- OrigName bool
-
- // EnumsAsInts specifies whether to render enum values as integers,
- // as opposed to string values.
- EnumsAsInts bool
-
- // EmitDefaults specifies whether to render fields with zero values.
- EmitDefaults bool
-
- // Indent controls whether the output is compact or not.
- // If empty, the output is compact JSON. Otherwise, every JSON object
- // entry and JSON array value will be on its own line.
- // Each line will be preceded by repeated copies of Indent, where the
- // number of copies is the current indentation depth.
- Indent string
-
- // AnyResolver is used to resolve the google.protobuf.Any well-known type.
- // If unset, the global registry is used by default.
- AnyResolver AnyResolver
-}
-
-// JSONPBMarshaler is implemented by protobuf messages that customize the
-// way they are marshaled to JSON. Messages that implement this should also
-// implement JSONPBUnmarshaler so that the custom format can be parsed.
-//
-// The JSON marshaling must follow the proto to JSON specification:
-// https://developers.google.com/protocol-buffers/docs/proto3#json
-//
-// Deprecated: Custom types should implement protobuf reflection instead.
-type JSONPBMarshaler interface {
- MarshalJSONPB(*Marshaler) ([]byte, error)
-}
-
-// Marshal serializes a protobuf message as JSON into w.
-func (jm *Marshaler) Marshal(w io.Writer, m proto.Message) error {
- b, err := jm.marshal(m)
- if len(b) > 0 {
- if _, err := w.Write(b); err != nil {
- return err
- }
- }
- return err
-}
-
-// MarshalToString serializes a protobuf message as JSON in string form.
-func (jm *Marshaler) MarshalToString(m proto.Message) (string, error) {
- b, err := jm.marshal(m)
- if err != nil {
- return "", err
- }
- return string(b), nil
-}
-
-func (jm *Marshaler) marshal(m proto.Message) ([]byte, error) {
- v := reflect.ValueOf(m)
- if m == nil || (v.Kind() == reflect.Ptr && v.IsNil()) {
- return nil, errors.New("Marshal called with nil")
- }
-
- // Check for custom marshalers first since they may not properly
- // implement protobuf reflection that the logic below relies on.
- if jsm, ok := m.(JSONPBMarshaler); ok {
- return jsm.MarshalJSONPB(jm)
- }
-
- if wrapJSONMarshalV2 {
- opts := protojson.MarshalOptions{
- UseProtoNames: jm.OrigName,
- UseEnumNumbers: jm.EnumsAsInts,
- EmitUnpopulated: jm.EmitDefaults,
- Indent: jm.Indent,
- }
- if jm.AnyResolver != nil {
- opts.Resolver = anyResolver{jm.AnyResolver}
- }
- return opts.Marshal(proto.MessageReflect(m).Interface())
- } else {
- // Check for unpopulated required fields first.
- m2 := proto.MessageReflect(m)
- if err := protoV2.CheckInitialized(m2.Interface()); err != nil {
- return nil, err
- }
-
- w := jsonWriter{Marshaler: jm}
- err := w.marshalMessage(m2, "", "")
- return w.buf, err
- }
-}
-
-type jsonWriter struct {
- *Marshaler
- buf []byte
-}
-
-func (w *jsonWriter) write(s string) {
- w.buf = append(w.buf, s...)
-}
-
-func (w *jsonWriter) marshalMessage(m protoreflect.Message, indent, typeURL string) error {
- if jsm, ok := proto.MessageV1(m.Interface()).(JSONPBMarshaler); ok {
- b, err := jsm.MarshalJSONPB(w.Marshaler)
- if err != nil {
- return err
- }
- if typeURL != "" {
- // we are marshaling this object to an Any type
- var js map[string]*json.RawMessage
- if err = json.Unmarshal(b, &js); err != nil {
- return fmt.Errorf("type %T produced invalid JSON: %v", m.Interface(), err)
- }
- turl, err := json.Marshal(typeURL)
- if err != nil {
- return fmt.Errorf("failed to marshal type URL %q to JSON: %v", typeURL, err)
- }
- js["@type"] = (*json.RawMessage)(&turl)
- if b, err = json.Marshal(js); err != nil {
- return err
- }
- }
- w.write(string(b))
- return nil
- }
-
- md := m.Descriptor()
- fds := md.Fields()
-
- // Handle well-known types.
- const secondInNanos = int64(time.Second / time.Nanosecond)
- switch wellKnownType(md.FullName()) {
- case "Any":
- return w.marshalAny(m, indent)
- case "BoolValue", "BytesValue", "StringValue",
- "Int32Value", "UInt32Value", "FloatValue",
- "Int64Value", "UInt64Value", "DoubleValue":
- fd := fds.ByNumber(1)
- return w.marshalValue(fd, m.Get(fd), indent)
- case "Duration":
- const maxSecondsInDuration = 315576000000
- // "Generated output always contains 0, 3, 6, or 9 fractional digits,
- // depending on required precision."
- s := m.Get(fds.ByNumber(1)).Int()
- ns := m.Get(fds.ByNumber(2)).Int()
- if s < -maxSecondsInDuration || s > maxSecondsInDuration {
- return fmt.Errorf("seconds out of range %v", s)
- }
- if ns <= -secondInNanos || ns >= secondInNanos {
- return fmt.Errorf("ns out of range (%v, %v)", -secondInNanos, secondInNanos)
- }
- if (s > 0 && ns < 0) || (s < 0 && ns > 0) {
- return errors.New("signs of seconds and nanos do not match")
- }
- var sign string
- if s < 0 || ns < 0 {
- sign, s, ns = "-", -1*s, -1*ns
- }
- x := fmt.Sprintf("%s%d.%09d", sign, s, ns)
- x = strings.TrimSuffix(x, "000")
- x = strings.TrimSuffix(x, "000")
- x = strings.TrimSuffix(x, ".000")
- w.write(fmt.Sprintf(`"%vs"`, x))
- return nil
- case "Timestamp":
- // "RFC 3339, where generated output will always be Z-normalized
- // and uses 0, 3, 6 or 9 fractional digits."
- s := m.Get(fds.ByNumber(1)).Int()
- ns := m.Get(fds.ByNumber(2)).Int()
- if ns < 0 || ns >= secondInNanos {
- return fmt.Errorf("ns out of range [0, %v)", secondInNanos)
- }
- t := time.Unix(s, ns).UTC()
- // time.RFC3339Nano isn't exactly right (we need to get 3/6/9 fractional digits).
- x := t.Format("2006-01-02T15:04:05.000000000")
- x = strings.TrimSuffix(x, "000")
- x = strings.TrimSuffix(x, "000")
- x = strings.TrimSuffix(x, ".000")
- w.write(fmt.Sprintf(`"%vZ"`, x))
- return nil
- case "Value":
- // JSON value; which is a null, number, string, bool, object, or array.
- od := md.Oneofs().Get(0)
- fd := m.WhichOneof(od)
- if fd == nil {
- return errors.New("nil Value")
- }
- return w.marshalValue(fd, m.Get(fd), indent)
- case "Struct", "ListValue":
- // JSON object or array.
- fd := fds.ByNumber(1)
- return w.marshalValue(fd, m.Get(fd), indent)
- }
-
- w.write("{")
- if w.Indent != "" {
- w.write("\n")
- }
-
- firstField := true
- if typeURL != "" {
- if err := w.marshalTypeURL(indent, typeURL); err != nil {
- return err
- }
- firstField = false
- }
-
- for i := 0; i < fds.Len(); {
- fd := fds.Get(i)
- if od := fd.ContainingOneof(); od != nil {
- fd = m.WhichOneof(od)
- i += od.Fields().Len()
- if fd == nil {
- continue
- }
- } else {
- i++
- }
-
- v := m.Get(fd)
-
- if !m.Has(fd) {
- if !w.EmitDefaults || fd.ContainingOneof() != nil {
- continue
- }
- if fd.Cardinality() != protoreflect.Repeated && (fd.Message() != nil || fd.Syntax() == protoreflect.Proto2) {
- v = protoreflect.Value{} // use "null" for singular messages or proto2 scalars
- }
- }
-
- if !firstField {
- w.writeComma()
- }
- if err := w.marshalField(fd, v, indent); err != nil {
- return err
- }
- firstField = false
- }
-
- // Handle proto2 extensions.
- if md.ExtensionRanges().Len() > 0 {
- // Collect a sorted list of all extension descriptor and values.
- type ext struct {
- desc protoreflect.FieldDescriptor
- val protoreflect.Value
- }
- var exts []ext
- m.Range(func(fd protoreflect.FieldDescriptor, v protoreflect.Value) bool {
- if fd.IsExtension() {
- exts = append(exts, ext{fd, v})
- }
- return true
- })
- sort.Slice(exts, func(i, j int) bool {
- return exts[i].desc.Number() < exts[j].desc.Number()
- })
-
- for _, ext := range exts {
- if !firstField {
- w.writeComma()
- }
- if err := w.marshalField(ext.desc, ext.val, indent); err != nil {
- return err
- }
- firstField = false
- }
- }
-
- if w.Indent != "" {
- w.write("\n")
- w.write(indent)
- }
- w.write("}")
- return nil
-}
-
-func (w *jsonWriter) writeComma() {
- if w.Indent != "" {
- w.write(",\n")
- } else {
- w.write(",")
- }
-}
-
-func (w *jsonWriter) marshalAny(m protoreflect.Message, indent string) error {
- // "If the Any contains a value that has a special JSON mapping,
- // it will be converted as follows: {"@type": xxx, "value": yyy}.
- // Otherwise, the value will be converted into a JSON object,
- // and the "@type" field will be inserted to indicate the actual data type."
- md := m.Descriptor()
- typeURL := m.Get(md.Fields().ByNumber(1)).String()
- rawVal := m.Get(md.Fields().ByNumber(2)).Bytes()
-
- var m2 protoreflect.Message
- if w.AnyResolver != nil {
- mi, err := w.AnyResolver.Resolve(typeURL)
- if err != nil {
- return err
- }
- m2 = proto.MessageReflect(mi)
- } else {
- mt, err := protoregistry.GlobalTypes.FindMessageByURL(typeURL)
- if err != nil {
- return err
- }
- m2 = mt.New()
- }
-
- if err := protoV2.Unmarshal(rawVal, m2.Interface()); err != nil {
- return err
- }
-
- if wellKnownType(m2.Descriptor().FullName()) == "" {
- return w.marshalMessage(m2, indent, typeURL)
- }
-
- w.write("{")
- if w.Indent != "" {
- w.write("\n")
- }
- if err := w.marshalTypeURL(indent, typeURL); err != nil {
- return err
- }
- w.writeComma()
- if w.Indent != "" {
- w.write(indent)
- w.write(w.Indent)
- w.write(`"value": `)
- } else {
- w.write(`"value":`)
- }
- if err := w.marshalMessage(m2, indent+w.Indent, ""); err != nil {
- return err
- }
- if w.Indent != "" {
- w.write("\n")
- w.write(indent)
- }
- w.write("}")
- return nil
-}
-
-func (w *jsonWriter) marshalTypeURL(indent, typeURL string) error {
- if w.Indent != "" {
- w.write(indent)
- w.write(w.Indent)
- }
- w.write(`"@type":`)
- if w.Indent != "" {
- w.write(" ")
- }
- b, err := json.Marshal(typeURL)
- if err != nil {
- return err
- }
- w.write(string(b))
- return nil
-}
-
-// marshalField writes field description and value to the Writer.
-func (w *jsonWriter) marshalField(fd protoreflect.FieldDescriptor, v protoreflect.Value, indent string) error {
- if w.Indent != "" {
- w.write(indent)
- w.write(w.Indent)
- }
- w.write(`"`)
- switch {
- case fd.IsExtension():
- // For message set, use the fname of the message as the extension name.
- name := string(fd.FullName())
- if isMessageSet(fd.ContainingMessage()) {
- name = strings.TrimSuffix(name, ".message_set_extension")
- }
-
- w.write("[" + name + "]")
- case w.OrigName:
- name := string(fd.Name())
- if fd.Kind() == protoreflect.GroupKind {
- name = string(fd.Message().Name())
- }
- w.write(name)
- default:
- w.write(string(fd.JSONName()))
- }
- w.write(`":`)
- if w.Indent != "" {
- w.write(" ")
- }
- return w.marshalValue(fd, v, indent)
-}
-
-func (w *jsonWriter) marshalValue(fd protoreflect.FieldDescriptor, v protoreflect.Value, indent string) error {
- switch {
- case fd.IsList():
- w.write("[")
- comma := ""
- lv := v.List()
- for i := 0; i < lv.Len(); i++ {
- w.write(comma)
- if w.Indent != "" {
- w.write("\n")
- w.write(indent)
- w.write(w.Indent)
- w.write(w.Indent)
- }
- if err := w.marshalSingularValue(fd, lv.Get(i), indent+w.Indent); err != nil {
- return err
- }
- comma = ","
- }
- if w.Indent != "" {
- w.write("\n")
- w.write(indent)
- w.write(w.Indent)
- }
- w.write("]")
- return nil
- case fd.IsMap():
- kfd := fd.MapKey()
- vfd := fd.MapValue()
- mv := v.Map()
-
- // Collect a sorted list of all map keys and values.
- type entry struct{ key, val protoreflect.Value }
- var entries []entry
- mv.Range(func(k protoreflect.MapKey, v protoreflect.Value) bool {
- entries = append(entries, entry{k.Value(), v})
- return true
- })
- sort.Slice(entries, func(i, j int) bool {
- switch kfd.Kind() {
- case protoreflect.BoolKind:
- return !entries[i].key.Bool() && entries[j].key.Bool()
- case protoreflect.Int32Kind, protoreflect.Sint32Kind, protoreflect.Sfixed32Kind, protoreflect.Int64Kind, protoreflect.Sint64Kind, protoreflect.Sfixed64Kind:
- return entries[i].key.Int() < entries[j].key.Int()
- case protoreflect.Uint32Kind, protoreflect.Fixed32Kind, protoreflect.Uint64Kind, protoreflect.Fixed64Kind:
- return entries[i].key.Uint() < entries[j].key.Uint()
- case protoreflect.StringKind:
- return entries[i].key.String() < entries[j].key.String()
- default:
- panic("invalid kind")
- }
- })
-
- w.write(`{`)
- comma := ""
- for _, entry := range entries {
- w.write(comma)
- if w.Indent != "" {
- w.write("\n")
- w.write(indent)
- w.write(w.Indent)
- w.write(w.Indent)
- }
-
- s := fmt.Sprint(entry.key.Interface())
- b, err := json.Marshal(s)
- if err != nil {
- return err
- }
- w.write(string(b))
-
- w.write(`:`)
- if w.Indent != "" {
- w.write(` `)
- }
-
- if err := w.marshalSingularValue(vfd, entry.val, indent+w.Indent); err != nil {
- return err
- }
- comma = ","
- }
- if w.Indent != "" {
- w.write("\n")
- w.write(indent)
- w.write(w.Indent)
- }
- w.write(`}`)
- return nil
- default:
- return w.marshalSingularValue(fd, v, indent)
- }
-}
-
-func (w *jsonWriter) marshalSingularValue(fd protoreflect.FieldDescriptor, v protoreflect.Value, indent string) error {
- switch {
- case !v.IsValid():
- w.write("null")
- return nil
- case fd.Message() != nil:
- return w.marshalMessage(v.Message(), indent+w.Indent, "")
- case fd.Enum() != nil:
- if fd.Enum().FullName() == "google.protobuf.NullValue" {
- w.write("null")
- return nil
- }
-
- vd := fd.Enum().Values().ByNumber(v.Enum())
- if vd == nil || w.EnumsAsInts {
- w.write(strconv.Itoa(int(v.Enum())))
- } else {
- w.write(`"` + string(vd.Name()) + `"`)
- }
- return nil
- default:
- switch v.Interface().(type) {
- case float32, float64:
- switch {
- case math.IsInf(v.Float(), +1):
- w.write(`"Infinity"`)
- return nil
- case math.IsInf(v.Float(), -1):
- w.write(`"-Infinity"`)
- return nil
- case math.IsNaN(v.Float()):
- w.write(`"NaN"`)
- return nil
- }
- case int64, uint64:
- w.write(fmt.Sprintf(`"%d"`, v.Interface()))
- return nil
- }
-
- b, err := json.Marshal(v.Interface())
- if err != nil {
- return err
- }
- w.write(string(b))
- return nil
- }
-}
diff --git a/vendor/github.com/golang/protobuf/jsonpb/json.go b/vendor/github.com/golang/protobuf/jsonpb/json.go
deleted file mode 100644
index 480e2448..00000000
--- a/vendor/github.com/golang/protobuf/jsonpb/json.go
+++ /dev/null
@@ -1,69 +0,0 @@
-// Copyright 2015 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package jsonpb provides functionality to marshal and unmarshal between a
-// protocol buffer message and JSON. It follows the specification at
-// https://developers.google.com/protocol-buffers/docs/proto3#json.
-//
-// Do not rely on the default behavior of the standard encoding/json package
-// when called on generated message types as it does not operate correctly.
-//
-// Deprecated: Use the "google.golang.org/protobuf/encoding/protojson"
-// package instead.
-package jsonpb
-
-import (
- "github.com/golang/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
- "google.golang.org/protobuf/runtime/protoimpl"
-)
-
-// AnyResolver takes a type URL, present in an Any message,
-// and resolves it into an instance of the associated message.
-type AnyResolver interface {
- Resolve(typeURL string) (proto.Message, error)
-}
-
-type anyResolver struct{ AnyResolver }
-
-func (r anyResolver) FindMessageByName(message protoreflect.FullName) (protoreflect.MessageType, error) {
- return r.FindMessageByURL(string(message))
-}
-
-func (r anyResolver) FindMessageByURL(url string) (protoreflect.MessageType, error) {
- m, err := r.Resolve(url)
- if err != nil {
- return nil, err
- }
- return protoimpl.X.MessageTypeOf(m), nil
-}
-
-func (r anyResolver) FindExtensionByName(field protoreflect.FullName) (protoreflect.ExtensionType, error) {
- return protoregistry.GlobalTypes.FindExtensionByName(field)
-}
-
-func (r anyResolver) FindExtensionByNumber(message protoreflect.FullName, field protoreflect.FieldNumber) (protoreflect.ExtensionType, error) {
- return protoregistry.GlobalTypes.FindExtensionByNumber(message, field)
-}
-
-func wellKnownType(s protoreflect.FullName) string {
- if s.Parent() == "google.protobuf" {
- switch s.Name() {
- case "Empty", "Any",
- "BoolValue", "BytesValue", "StringValue",
- "Int32Value", "UInt32Value", "FloatValue",
- "Int64Value", "UInt64Value", "DoubleValue",
- "Duration", "Timestamp",
- "NullValue", "Struct", "Value", "ListValue":
- return string(s.Name())
- }
- }
- return ""
-}
-
-func isMessageSet(md protoreflect.MessageDescriptor) bool {
- ms, ok := md.(interface{ IsMessageSet() bool })
- return ok && ms.IsMessageSet()
-}
diff --git a/vendor/github.com/golang/protobuf/proto/buffer.go b/vendor/github.com/golang/protobuf/proto/buffer.go
deleted file mode 100644
index e810e6fe..00000000
--- a/vendor/github.com/golang/protobuf/proto/buffer.go
+++ /dev/null
@@ -1,324 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "errors"
- "fmt"
-
- "google.golang.org/protobuf/encoding/prototext"
- "google.golang.org/protobuf/encoding/protowire"
- "google.golang.org/protobuf/runtime/protoimpl"
-)
-
-const (
- WireVarint = 0
- WireFixed32 = 5
- WireFixed64 = 1
- WireBytes = 2
- WireStartGroup = 3
- WireEndGroup = 4
-)
-
-// EncodeVarint returns the varint encoded bytes of v.
-func EncodeVarint(v uint64) []byte {
- return protowire.AppendVarint(nil, v)
-}
-
-// SizeVarint returns the length of the varint encoded bytes of v.
-// This is equal to len(EncodeVarint(v)).
-func SizeVarint(v uint64) int {
- return protowire.SizeVarint(v)
-}
-
-// DecodeVarint parses a varint encoded integer from b,
-// returning the integer value and the length of the varint.
-// It returns (0, 0) if there is a parse error.
-func DecodeVarint(b []byte) (uint64, int) {
- v, n := protowire.ConsumeVarint(b)
- if n < 0 {
- return 0, 0
- }
- return v, n
-}
-
-// Buffer is a buffer for encoding and decoding the protobuf wire format.
-// It may be reused between invocations to reduce memory usage.
-type Buffer struct {
- buf []byte
- idx int
- deterministic bool
-}
-
-// NewBuffer allocates a new Buffer initialized with buf,
-// where the contents of buf are considered the unread portion of the buffer.
-func NewBuffer(buf []byte) *Buffer {
- return &Buffer{buf: buf}
-}
-
-// SetDeterministic specifies whether to use deterministic serialization.
-//
-// Deterministic serialization guarantees that for a given binary, equal
-// messages will always be serialized to the same bytes. This implies:
-//
-// - Repeated serialization of a message will return the same bytes.
-// - Different processes of the same binary (which may be executing on
-// different machines) will serialize equal messages to the same bytes.
-//
-// Note that the deterministic serialization is NOT canonical across
-// languages. It is not guaranteed to remain stable over time. It is unstable
-// across different builds with schema changes due to unknown fields.
-// Users who need canonical serialization (e.g., persistent storage in a
-// canonical form, fingerprinting, etc.) should define their own
-// canonicalization specification and implement their own serializer rather
-// than relying on this API.
-//
-// If deterministic serialization is requested, map entries will be sorted
-// by keys in lexographical order. This is an implementation detail and
-// subject to change.
-func (b *Buffer) SetDeterministic(deterministic bool) {
- b.deterministic = deterministic
-}
-
-// SetBuf sets buf as the internal buffer,
-// where the contents of buf are considered the unread portion of the buffer.
-func (b *Buffer) SetBuf(buf []byte) {
- b.buf = buf
- b.idx = 0
-}
-
-// Reset clears the internal buffer of all written and unread data.
-func (b *Buffer) Reset() {
- b.buf = b.buf[:0]
- b.idx = 0
-}
-
-// Bytes returns the internal buffer.
-func (b *Buffer) Bytes() []byte {
- return b.buf
-}
-
-// Unread returns the unread portion of the buffer.
-func (b *Buffer) Unread() []byte {
- return b.buf[b.idx:]
-}
-
-// Marshal appends the wire-format encoding of m to the buffer.
-func (b *Buffer) Marshal(m Message) error {
- var err error
- b.buf, err = marshalAppend(b.buf, m, b.deterministic)
- return err
-}
-
-// Unmarshal parses the wire-format message in the buffer and
-// places the decoded results in m.
-// It does not reset m before unmarshaling.
-func (b *Buffer) Unmarshal(m Message) error {
- err := UnmarshalMerge(b.Unread(), m)
- b.idx = len(b.buf)
- return err
-}
-
-type unknownFields struct{ XXX_unrecognized protoimpl.UnknownFields }
-
-func (m *unknownFields) String() string { panic("not implemented") }
-func (m *unknownFields) Reset() { panic("not implemented") }
-func (m *unknownFields) ProtoMessage() { panic("not implemented") }
-
-// DebugPrint dumps the encoded bytes of b with a header and footer including s
-// to stdout. This is only intended for debugging.
-func (*Buffer) DebugPrint(s string, b []byte) {
- m := MessageReflect(new(unknownFields))
- m.SetUnknown(b)
- b, _ = prototext.MarshalOptions{AllowPartial: true, Indent: "\t"}.Marshal(m.Interface())
- fmt.Printf("==== %s ====\n%s==== %s ====\n", s, b, s)
-}
-
-// EncodeVarint appends an unsigned varint encoding to the buffer.
-func (b *Buffer) EncodeVarint(v uint64) error {
- b.buf = protowire.AppendVarint(b.buf, v)
- return nil
-}
-
-// EncodeZigzag32 appends a 32-bit zig-zag varint encoding to the buffer.
-func (b *Buffer) EncodeZigzag32(v uint64) error {
- return b.EncodeVarint(uint64((uint32(v) << 1) ^ uint32((int32(v) >> 31))))
-}
-
-// EncodeZigzag64 appends a 64-bit zig-zag varint encoding to the buffer.
-func (b *Buffer) EncodeZigzag64(v uint64) error {
- return b.EncodeVarint(uint64((uint64(v) << 1) ^ uint64((int64(v) >> 63))))
-}
-
-// EncodeFixed32 appends a 32-bit little-endian integer to the buffer.
-func (b *Buffer) EncodeFixed32(v uint64) error {
- b.buf = protowire.AppendFixed32(b.buf, uint32(v))
- return nil
-}
-
-// EncodeFixed64 appends a 64-bit little-endian integer to the buffer.
-func (b *Buffer) EncodeFixed64(v uint64) error {
- b.buf = protowire.AppendFixed64(b.buf, uint64(v))
- return nil
-}
-
-// EncodeRawBytes appends a length-prefixed raw bytes to the buffer.
-func (b *Buffer) EncodeRawBytes(v []byte) error {
- b.buf = protowire.AppendBytes(b.buf, v)
- return nil
-}
-
-// EncodeStringBytes appends a length-prefixed raw bytes to the buffer.
-// It does not validate whether v contains valid UTF-8.
-func (b *Buffer) EncodeStringBytes(v string) error {
- b.buf = protowire.AppendString(b.buf, v)
- return nil
-}
-
-// EncodeMessage appends a length-prefixed encoded message to the buffer.
-func (b *Buffer) EncodeMessage(m Message) error {
- var err error
- b.buf = protowire.AppendVarint(b.buf, uint64(Size(m)))
- b.buf, err = marshalAppend(b.buf, m, b.deterministic)
- return err
-}
-
-// DecodeVarint consumes an encoded unsigned varint from the buffer.
-func (b *Buffer) DecodeVarint() (uint64, error) {
- v, n := protowire.ConsumeVarint(b.buf[b.idx:])
- if n < 0 {
- return 0, protowire.ParseError(n)
- }
- b.idx += n
- return uint64(v), nil
-}
-
-// DecodeZigzag32 consumes an encoded 32-bit zig-zag varint from the buffer.
-func (b *Buffer) DecodeZigzag32() (uint64, error) {
- v, err := b.DecodeVarint()
- if err != nil {
- return 0, err
- }
- return uint64((uint32(v) >> 1) ^ uint32((int32(v&1)<<31)>>31)), nil
-}
-
-// DecodeZigzag64 consumes an encoded 64-bit zig-zag varint from the buffer.
-func (b *Buffer) DecodeZigzag64() (uint64, error) {
- v, err := b.DecodeVarint()
- if err != nil {
- return 0, err
- }
- return uint64((uint64(v) >> 1) ^ uint64((int64(v&1)<<63)>>63)), nil
-}
-
-// DecodeFixed32 consumes a 32-bit little-endian integer from the buffer.
-func (b *Buffer) DecodeFixed32() (uint64, error) {
- v, n := protowire.ConsumeFixed32(b.buf[b.idx:])
- if n < 0 {
- return 0, protowire.ParseError(n)
- }
- b.idx += n
- return uint64(v), nil
-}
-
-// DecodeFixed64 consumes a 64-bit little-endian integer from the buffer.
-func (b *Buffer) DecodeFixed64() (uint64, error) {
- v, n := protowire.ConsumeFixed64(b.buf[b.idx:])
- if n < 0 {
- return 0, protowire.ParseError(n)
- }
- b.idx += n
- return uint64(v), nil
-}
-
-// DecodeRawBytes consumes a length-prefixed raw bytes from the buffer.
-// If alloc is specified, it returns a copy the raw bytes
-// rather than a sub-slice of the buffer.
-func (b *Buffer) DecodeRawBytes(alloc bool) ([]byte, error) {
- v, n := protowire.ConsumeBytes(b.buf[b.idx:])
- if n < 0 {
- return nil, protowire.ParseError(n)
- }
- b.idx += n
- if alloc {
- v = append([]byte(nil), v...)
- }
- return v, nil
-}
-
-// DecodeStringBytes consumes a length-prefixed raw bytes from the buffer.
-// It does not validate whether the raw bytes contain valid UTF-8.
-func (b *Buffer) DecodeStringBytes() (string, error) {
- v, n := protowire.ConsumeString(b.buf[b.idx:])
- if n < 0 {
- return "", protowire.ParseError(n)
- }
- b.idx += n
- return v, nil
-}
-
-// DecodeMessage consumes a length-prefixed message from the buffer.
-// It does not reset m before unmarshaling.
-func (b *Buffer) DecodeMessage(m Message) error {
- v, err := b.DecodeRawBytes(false)
- if err != nil {
- return err
- }
- return UnmarshalMerge(v, m)
-}
-
-// DecodeGroup consumes a message group from the buffer.
-// It assumes that the start group marker has already been consumed and
-// consumes all bytes until (and including the end group marker).
-// It does not reset m before unmarshaling.
-func (b *Buffer) DecodeGroup(m Message) error {
- v, n, err := consumeGroup(b.buf[b.idx:])
- if err != nil {
- return err
- }
- b.idx += n
- return UnmarshalMerge(v, m)
-}
-
-// consumeGroup parses b until it finds an end group marker, returning
-// the raw bytes of the message (excluding the end group marker) and the
-// the total length of the message (including the end group marker).
-func consumeGroup(b []byte) ([]byte, int, error) {
- b0 := b
- depth := 1 // assume this follows a start group marker
- for {
- _, wtyp, tagLen := protowire.ConsumeTag(b)
- if tagLen < 0 {
- return nil, 0, protowire.ParseError(tagLen)
- }
- b = b[tagLen:]
-
- var valLen int
- switch wtyp {
- case protowire.VarintType:
- _, valLen = protowire.ConsumeVarint(b)
- case protowire.Fixed32Type:
- _, valLen = protowire.ConsumeFixed32(b)
- case protowire.Fixed64Type:
- _, valLen = protowire.ConsumeFixed64(b)
- case protowire.BytesType:
- _, valLen = protowire.ConsumeBytes(b)
- case protowire.StartGroupType:
- depth++
- case protowire.EndGroupType:
- depth--
- default:
- return nil, 0, errors.New("proto: cannot parse reserved wire type")
- }
- if valLen < 0 {
- return nil, 0, protowire.ParseError(valLen)
- }
- b = b[valLen:]
-
- if depth == 0 {
- return b0[:len(b0)-len(b)-tagLen], len(b0) - len(b), nil
- }
- }
-}
diff --git a/vendor/github.com/golang/protobuf/proto/defaults.go b/vendor/github.com/golang/protobuf/proto/defaults.go
deleted file mode 100644
index d399bf06..00000000
--- a/vendor/github.com/golang/protobuf/proto/defaults.go
+++ /dev/null
@@ -1,63 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "google.golang.org/protobuf/reflect/protoreflect"
-)
-
-// SetDefaults sets unpopulated scalar fields to their default values.
-// Fields within a oneof are not set even if they have a default value.
-// SetDefaults is recursively called upon any populated message fields.
-func SetDefaults(m Message) {
- if m != nil {
- setDefaults(MessageReflect(m))
- }
-}
-
-func setDefaults(m protoreflect.Message) {
- fds := m.Descriptor().Fields()
- for i := 0; i < fds.Len(); i++ {
- fd := fds.Get(i)
- if !m.Has(fd) {
- if fd.HasDefault() && fd.ContainingOneof() == nil {
- v := fd.Default()
- if fd.Kind() == protoreflect.BytesKind {
- v = protoreflect.ValueOf(append([]byte(nil), v.Bytes()...)) // copy the default bytes
- }
- m.Set(fd, v)
- }
- continue
- }
- }
-
- m.Range(func(fd protoreflect.FieldDescriptor, v protoreflect.Value) bool {
- switch {
- // Handle singular message.
- case fd.Cardinality() != protoreflect.Repeated:
- if fd.Message() != nil {
- setDefaults(m.Get(fd).Message())
- }
- // Handle list of messages.
- case fd.IsList():
- if fd.Message() != nil {
- ls := m.Get(fd).List()
- for i := 0; i < ls.Len(); i++ {
- setDefaults(ls.Get(i).Message())
- }
- }
- // Handle map of messages.
- case fd.IsMap():
- if fd.MapValue().Message() != nil {
- ms := m.Get(fd).Map()
- ms.Range(func(_ protoreflect.MapKey, v protoreflect.Value) bool {
- setDefaults(v.Message())
- return true
- })
- }
- }
- return true
- })
-}
diff --git a/vendor/github.com/golang/protobuf/proto/deprecated.go b/vendor/github.com/golang/protobuf/proto/deprecated.go
deleted file mode 100644
index e8db57e0..00000000
--- a/vendor/github.com/golang/protobuf/proto/deprecated.go
+++ /dev/null
@@ -1,113 +0,0 @@
-// Copyright 2018 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "encoding/json"
- "errors"
- "fmt"
- "strconv"
-
- protoV2 "google.golang.org/protobuf/proto"
-)
-
-var (
- // Deprecated: No longer returned.
- ErrNil = errors.New("proto: Marshal called with nil")
-
- // Deprecated: No longer returned.
- ErrTooLarge = errors.New("proto: message encodes to over 2 GB")
-
- // Deprecated: No longer returned.
- ErrInternalBadWireType = errors.New("proto: internal error: bad wiretype for oneof")
-)
-
-// Deprecated: Do not use.
-type Stats struct{ Emalloc, Dmalloc, Encode, Decode, Chit, Cmiss, Size uint64 }
-
-// Deprecated: Do not use.
-func GetStats() Stats { return Stats{} }
-
-// Deprecated: Do not use.
-func MarshalMessageSet(interface{}) ([]byte, error) {
- return nil, errors.New("proto: not implemented")
-}
-
-// Deprecated: Do not use.
-func UnmarshalMessageSet([]byte, interface{}) error {
- return errors.New("proto: not implemented")
-}
-
-// Deprecated: Do not use.
-func MarshalMessageSetJSON(interface{}) ([]byte, error) {
- return nil, errors.New("proto: not implemented")
-}
-
-// Deprecated: Do not use.
-func UnmarshalMessageSetJSON([]byte, interface{}) error {
- return errors.New("proto: not implemented")
-}
-
-// Deprecated: Do not use.
-func RegisterMessageSetType(Message, int32, string) {}
-
-// Deprecated: Do not use.
-func EnumName(m map[int32]string, v int32) string {
- s, ok := m[v]
- if ok {
- return s
- }
- return strconv.Itoa(int(v))
-}
-
-// Deprecated: Do not use.
-func UnmarshalJSONEnum(m map[string]int32, data []byte, enumName string) (int32, error) {
- if data[0] == '"' {
- // New style: enums are strings.
- var repr string
- if err := json.Unmarshal(data, &repr); err != nil {
- return -1, err
- }
- val, ok := m[repr]
- if !ok {
- return 0, fmt.Errorf("unrecognized enum %s value %q", enumName, repr)
- }
- return val, nil
- }
- // Old style: enums are ints.
- var val int32
- if err := json.Unmarshal(data, &val); err != nil {
- return 0, fmt.Errorf("cannot unmarshal %#q into enum %s", data, enumName)
- }
- return val, nil
-}
-
-// Deprecated: Do not use; this type existed for intenal-use only.
-type InternalMessageInfo struct{}
-
-// Deprecated: Do not use; this method existed for intenal-use only.
-func (*InternalMessageInfo) DiscardUnknown(m Message) {
- DiscardUnknown(m)
-}
-
-// Deprecated: Do not use; this method existed for intenal-use only.
-func (*InternalMessageInfo) Marshal(b []byte, m Message, deterministic bool) ([]byte, error) {
- return protoV2.MarshalOptions{Deterministic: deterministic}.MarshalAppend(b, MessageV2(m))
-}
-
-// Deprecated: Do not use; this method existed for intenal-use only.
-func (*InternalMessageInfo) Merge(dst, src Message) {
- protoV2.Merge(MessageV2(dst), MessageV2(src))
-}
-
-// Deprecated: Do not use; this method existed for intenal-use only.
-func (*InternalMessageInfo) Size(m Message) int {
- return protoV2.Size(MessageV2(m))
-}
-
-// Deprecated: Do not use; this method existed for intenal-use only.
-func (*InternalMessageInfo) Unmarshal(m Message, b []byte) error {
- return protoV2.UnmarshalOptions{Merge: true}.Unmarshal(b, MessageV2(m))
-}
diff --git a/vendor/github.com/golang/protobuf/proto/discard.go b/vendor/github.com/golang/protobuf/proto/discard.go
deleted file mode 100644
index 2187e877..00000000
--- a/vendor/github.com/golang/protobuf/proto/discard.go
+++ /dev/null
@@ -1,58 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "google.golang.org/protobuf/reflect/protoreflect"
-)
-
-// DiscardUnknown recursively discards all unknown fields from this message
-// and all embedded messages.
-//
-// When unmarshaling a message with unrecognized fields, the tags and values
-// of such fields are preserved in the Message. This allows a later call to
-// marshal to be able to produce a message that continues to have those
-// unrecognized fields. To avoid this, DiscardUnknown is used to
-// explicitly clear the unknown fields after unmarshaling.
-func DiscardUnknown(m Message) {
- if m != nil {
- discardUnknown(MessageReflect(m))
- }
-}
-
-func discardUnknown(m protoreflect.Message) {
- m.Range(func(fd protoreflect.FieldDescriptor, val protoreflect.Value) bool {
- switch {
- // Handle singular message.
- case fd.Cardinality() != protoreflect.Repeated:
- if fd.Message() != nil {
- discardUnknown(m.Get(fd).Message())
- }
- // Handle list of messages.
- case fd.IsList():
- if fd.Message() != nil {
- ls := m.Get(fd).List()
- for i := 0; i < ls.Len(); i++ {
- discardUnknown(ls.Get(i).Message())
- }
- }
- // Handle map of messages.
- case fd.IsMap():
- if fd.MapValue().Message() != nil {
- ms := m.Get(fd).Map()
- ms.Range(func(_ protoreflect.MapKey, v protoreflect.Value) bool {
- discardUnknown(v.Message())
- return true
- })
- }
- }
- return true
- })
-
- // Discard unknown fields.
- if len(m.GetUnknown()) > 0 {
- m.SetUnknown(nil)
- }
-}
diff --git a/vendor/github.com/golang/protobuf/proto/extensions.go b/vendor/github.com/golang/protobuf/proto/extensions.go
deleted file mode 100644
index 42fc120c..00000000
--- a/vendor/github.com/golang/protobuf/proto/extensions.go
+++ /dev/null
@@ -1,356 +0,0 @@
-// Copyright 2010 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "errors"
- "fmt"
- "reflect"
-
- "google.golang.org/protobuf/encoding/protowire"
- "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
- "google.golang.org/protobuf/runtime/protoiface"
- "google.golang.org/protobuf/runtime/protoimpl"
-)
-
-type (
- // ExtensionDesc represents an extension descriptor and
- // is used to interact with an extension field in a message.
- //
- // Variables of this type are generated in code by protoc-gen-go.
- ExtensionDesc = protoimpl.ExtensionInfo
-
- // ExtensionRange represents a range of message extensions.
- // Used in code generated by protoc-gen-go.
- ExtensionRange = protoiface.ExtensionRangeV1
-
- // Deprecated: Do not use; this is an internal type.
- Extension = protoimpl.ExtensionFieldV1
-
- // Deprecated: Do not use; this is an internal type.
- XXX_InternalExtensions = protoimpl.ExtensionFields
-)
-
-// ErrMissingExtension reports whether the extension was not present.
-var ErrMissingExtension = errors.New("proto: missing extension")
-
-var errNotExtendable = errors.New("proto: not an extendable proto.Message")
-
-// HasExtension reports whether the extension field is present in m
-// either as an explicitly populated field or as an unknown field.
-func HasExtension(m Message, xt *ExtensionDesc) (has bool) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() {
- return false
- }
-
- // Check whether any populated known field matches the field number.
- xtd := xt.TypeDescriptor()
- if isValidExtension(mr.Descriptor(), xtd) {
- has = mr.Has(xtd)
- } else {
- mr.Range(func(fd protoreflect.FieldDescriptor, _ protoreflect.Value) bool {
- has = int32(fd.Number()) == xt.Field
- return !has
- })
- }
-
- // Check whether any unknown field matches the field number.
- for b := mr.GetUnknown(); !has && len(b) > 0; {
- num, _, n := protowire.ConsumeField(b)
- has = int32(num) == xt.Field
- b = b[n:]
- }
- return has
-}
-
-// ClearExtension removes the extension field from m
-// either as an explicitly populated field or as an unknown field.
-func ClearExtension(m Message, xt *ExtensionDesc) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() {
- return
- }
-
- xtd := xt.TypeDescriptor()
- if isValidExtension(mr.Descriptor(), xtd) {
- mr.Clear(xtd)
- } else {
- mr.Range(func(fd protoreflect.FieldDescriptor, _ protoreflect.Value) bool {
- if int32(fd.Number()) == xt.Field {
- mr.Clear(fd)
- return false
- }
- return true
- })
- }
- clearUnknown(mr, fieldNum(xt.Field))
-}
-
-// ClearAllExtensions clears all extensions from m.
-// This includes populated fields and unknown fields in the extension range.
-func ClearAllExtensions(m Message) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() {
- return
- }
-
- mr.Range(func(fd protoreflect.FieldDescriptor, _ protoreflect.Value) bool {
- if fd.IsExtension() {
- mr.Clear(fd)
- }
- return true
- })
- clearUnknown(mr, mr.Descriptor().ExtensionRanges())
-}
-
-// GetExtension retrieves a proto2 extended field from m.
-//
-// If the descriptor is type complete (i.e., ExtensionDesc.ExtensionType is non-nil),
-// then GetExtension parses the encoded field and returns a Go value of the specified type.
-// If the field is not present, then the default value is returned (if one is specified),
-// otherwise ErrMissingExtension is reported.
-//
-// If the descriptor is type incomplete (i.e., ExtensionDesc.ExtensionType is nil),
-// then GetExtension returns the raw encoded bytes for the extension field.
-func GetExtension(m Message, xt *ExtensionDesc) (interface{}, error) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() || mr.Descriptor().ExtensionRanges().Len() == 0 {
- return nil, errNotExtendable
- }
-
- // Retrieve the unknown fields for this extension field.
- var bo protoreflect.RawFields
- for bi := mr.GetUnknown(); len(bi) > 0; {
- num, _, n := protowire.ConsumeField(bi)
- if int32(num) == xt.Field {
- bo = append(bo, bi[:n]...)
- }
- bi = bi[n:]
- }
-
- // For type incomplete descriptors, only retrieve the unknown fields.
- if xt.ExtensionType == nil {
- return []byte(bo), nil
- }
-
- // If the extension field only exists as unknown fields, unmarshal it.
- // This is rarely done since proto.Unmarshal eagerly unmarshals extensions.
- xtd := xt.TypeDescriptor()
- if !isValidExtension(mr.Descriptor(), xtd) {
- return nil, fmt.Errorf("proto: bad extended type; %T does not extend %T", xt.ExtendedType, m)
- }
- if !mr.Has(xtd) && len(bo) > 0 {
- m2 := mr.New()
- if err := (proto.UnmarshalOptions{
- Resolver: extensionResolver{xt},
- }.Unmarshal(bo, m2.Interface())); err != nil {
- return nil, err
- }
- if m2.Has(xtd) {
- mr.Set(xtd, m2.Get(xtd))
- clearUnknown(mr, fieldNum(xt.Field))
- }
- }
-
- // Check whether the message has the extension field set or a default.
- var pv protoreflect.Value
- switch {
- case mr.Has(xtd):
- pv = mr.Get(xtd)
- case xtd.HasDefault():
- pv = xtd.Default()
- default:
- return nil, ErrMissingExtension
- }
-
- v := xt.InterfaceOf(pv)
- rv := reflect.ValueOf(v)
- if isScalarKind(rv.Kind()) {
- rv2 := reflect.New(rv.Type())
- rv2.Elem().Set(rv)
- v = rv2.Interface()
- }
- return v, nil
-}
-
-// extensionResolver is a custom extension resolver that stores a single
-// extension type that takes precedence over the global registry.
-type extensionResolver struct{ xt protoreflect.ExtensionType }
-
-func (r extensionResolver) FindExtensionByName(field protoreflect.FullName) (protoreflect.ExtensionType, error) {
- if xtd := r.xt.TypeDescriptor(); xtd.FullName() == field {
- return r.xt, nil
- }
- return protoregistry.GlobalTypes.FindExtensionByName(field)
-}
-
-func (r extensionResolver) FindExtensionByNumber(message protoreflect.FullName, field protoreflect.FieldNumber) (protoreflect.ExtensionType, error) {
- if xtd := r.xt.TypeDescriptor(); xtd.ContainingMessage().FullName() == message && xtd.Number() == field {
- return r.xt, nil
- }
- return protoregistry.GlobalTypes.FindExtensionByNumber(message, field)
-}
-
-// GetExtensions returns a list of the extensions values present in m,
-// corresponding with the provided list of extension descriptors, xts.
-// If an extension is missing in m, the corresponding value is nil.
-func GetExtensions(m Message, xts []*ExtensionDesc) ([]interface{}, error) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() {
- return nil, errNotExtendable
- }
-
- vs := make([]interface{}, len(xts))
- for i, xt := range xts {
- v, err := GetExtension(m, xt)
- if err != nil {
- if err == ErrMissingExtension {
- continue
- }
- return vs, err
- }
- vs[i] = v
- }
- return vs, nil
-}
-
-// SetExtension sets an extension field in m to the provided value.
-func SetExtension(m Message, xt *ExtensionDesc, v interface{}) error {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() || mr.Descriptor().ExtensionRanges().Len() == 0 {
- return errNotExtendable
- }
-
- rv := reflect.ValueOf(v)
- if reflect.TypeOf(v) != reflect.TypeOf(xt.ExtensionType) {
- return fmt.Errorf("proto: bad extension value type. got: %T, want: %T", v, xt.ExtensionType)
- }
- if rv.Kind() == reflect.Ptr {
- if rv.IsNil() {
- return fmt.Errorf("proto: SetExtension called with nil value of type %T", v)
- }
- if isScalarKind(rv.Elem().Kind()) {
- v = rv.Elem().Interface()
- }
- }
-
- xtd := xt.TypeDescriptor()
- if !isValidExtension(mr.Descriptor(), xtd) {
- return fmt.Errorf("proto: bad extended type; %T does not extend %T", xt.ExtendedType, m)
- }
- mr.Set(xtd, xt.ValueOf(v))
- clearUnknown(mr, fieldNum(xt.Field))
- return nil
-}
-
-// SetRawExtension inserts b into the unknown fields of m.
-//
-// Deprecated: Use Message.ProtoReflect.SetUnknown instead.
-func SetRawExtension(m Message, fnum int32, b []byte) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() {
- return
- }
-
- // Verify that the raw field is valid.
- for b0 := b; len(b0) > 0; {
- num, _, n := protowire.ConsumeField(b0)
- if int32(num) != fnum {
- panic(fmt.Sprintf("mismatching field number: got %d, want %d", num, fnum))
- }
- b0 = b0[n:]
- }
-
- ClearExtension(m, &ExtensionDesc{Field: fnum})
- mr.SetUnknown(append(mr.GetUnknown(), b...))
-}
-
-// ExtensionDescs returns a list of extension descriptors found in m,
-// containing descriptors for both populated extension fields in m and
-// also unknown fields of m that are in the extension range.
-// For the later case, an type incomplete descriptor is provided where only
-// the ExtensionDesc.Field field is populated.
-// The order of the extension descriptors is undefined.
-func ExtensionDescs(m Message) ([]*ExtensionDesc, error) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() || mr.Descriptor().ExtensionRanges().Len() == 0 {
- return nil, errNotExtendable
- }
-
- // Collect a set of known extension descriptors.
- extDescs := make(map[protoreflect.FieldNumber]*ExtensionDesc)
- mr.Range(func(fd protoreflect.FieldDescriptor, v protoreflect.Value) bool {
- if fd.IsExtension() {
- xt := fd.(protoreflect.ExtensionTypeDescriptor)
- if xd, ok := xt.Type().(*ExtensionDesc); ok {
- extDescs[fd.Number()] = xd
- }
- }
- return true
- })
-
- // Collect a set of unknown extension descriptors.
- extRanges := mr.Descriptor().ExtensionRanges()
- for b := mr.GetUnknown(); len(b) > 0; {
- num, _, n := protowire.ConsumeField(b)
- if extRanges.Has(num) && extDescs[num] == nil {
- extDescs[num] = nil
- }
- b = b[n:]
- }
-
- // Transpose the set of descriptors into a list.
- var xts []*ExtensionDesc
- for num, xt := range extDescs {
- if xt == nil {
- xt = &ExtensionDesc{Field: int32(num)}
- }
- xts = append(xts, xt)
- }
- return xts, nil
-}
-
-// isValidExtension reports whether xtd is a valid extension descriptor for md.
-func isValidExtension(md protoreflect.MessageDescriptor, xtd protoreflect.ExtensionTypeDescriptor) bool {
- return xtd.ContainingMessage() == md && md.ExtensionRanges().Has(xtd.Number())
-}
-
-// isScalarKind reports whether k is a protobuf scalar kind (except bytes).
-// This function exists for historical reasons since the representation of
-// scalars differs between v1 and v2, where v1 uses *T and v2 uses T.
-func isScalarKind(k reflect.Kind) bool {
- switch k {
- case reflect.Bool, reflect.Int32, reflect.Int64, reflect.Uint32, reflect.Uint64, reflect.Float32, reflect.Float64, reflect.String:
- return true
- default:
- return false
- }
-}
-
-// clearUnknown removes unknown fields from m where remover.Has reports true.
-func clearUnknown(m protoreflect.Message, remover interface {
- Has(protoreflect.FieldNumber) bool
-}) {
- var bo protoreflect.RawFields
- for bi := m.GetUnknown(); len(bi) > 0; {
- num, _, n := protowire.ConsumeField(bi)
- if !remover.Has(num) {
- bo = append(bo, bi[:n]...)
- }
- bi = bi[n:]
- }
- if bi := m.GetUnknown(); len(bi) != len(bo) {
- m.SetUnknown(bo)
- }
-}
-
-type fieldNum protoreflect.FieldNumber
-
-func (n1 fieldNum) Has(n2 protoreflect.FieldNumber) bool {
- return protoreflect.FieldNumber(n1) == n2
-}
diff --git a/vendor/github.com/golang/protobuf/proto/properties.go b/vendor/github.com/golang/protobuf/proto/properties.go
deleted file mode 100644
index dcdc2202..00000000
--- a/vendor/github.com/golang/protobuf/proto/properties.go
+++ /dev/null
@@ -1,306 +0,0 @@
-// Copyright 2010 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "fmt"
- "reflect"
- "strconv"
- "strings"
- "sync"
-
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/runtime/protoimpl"
-)
-
-// StructProperties represents protocol buffer type information for a
-// generated protobuf message in the open-struct API.
-//
-// Deprecated: Do not use.
-type StructProperties struct {
- // Prop are the properties for each field.
- //
- // Fields belonging to a oneof are stored in OneofTypes instead, with a
- // single Properties representing the parent oneof held here.
- //
- // The order of Prop matches the order of fields in the Go struct.
- // Struct fields that are not related to protobufs have a "XXX_" prefix
- // in the Properties.Name and must be ignored by the user.
- Prop []*Properties
-
- // OneofTypes contains information about the oneof fields in this message.
- // It is keyed by the protobuf field name.
- OneofTypes map[string]*OneofProperties
-}
-
-// Properties represents the type information for a protobuf message field.
-//
-// Deprecated: Do not use.
-type Properties struct {
- // Name is a placeholder name with little meaningful semantic value.
- // If the name has an "XXX_" prefix, the entire Properties must be ignored.
- Name string
- // OrigName is the protobuf field name or oneof name.
- OrigName string
- // JSONName is the JSON name for the protobuf field.
- JSONName string
- // Enum is a placeholder name for enums.
- // For historical reasons, this is neither the Go name for the enum,
- // nor the protobuf name for the enum.
- Enum string // Deprecated: Do not use.
- // Weak contains the full name of the weakly referenced message.
- Weak string
- // Wire is a string representation of the wire type.
- Wire string
- // WireType is the protobuf wire type for the field.
- WireType int
- // Tag is the protobuf field number.
- Tag int
- // Required reports whether this is a required field.
- Required bool
- // Optional reports whether this is a optional field.
- Optional bool
- // Repeated reports whether this is a repeated field.
- Repeated bool
- // Packed reports whether this is a packed repeated field of scalars.
- Packed bool
- // Proto3 reports whether this field operates under the proto3 syntax.
- Proto3 bool
- // Oneof reports whether this field belongs within a oneof.
- Oneof bool
-
- // Default is the default value in string form.
- Default string
- // HasDefault reports whether the field has a default value.
- HasDefault bool
-
- // MapKeyProp is the properties for the key field for a map field.
- MapKeyProp *Properties
- // MapValProp is the properties for the value field for a map field.
- MapValProp *Properties
-}
-
-// OneofProperties represents the type information for a protobuf oneof.
-//
-// Deprecated: Do not use.
-type OneofProperties struct {
- // Type is a pointer to the generated wrapper type for the field value.
- // This is nil for messages that are not in the open-struct API.
- Type reflect.Type
- // Field is the index into StructProperties.Prop for the containing oneof.
- Field int
- // Prop is the properties for the field.
- Prop *Properties
-}
-
-// String formats the properties in the protobuf struct field tag style.
-func (p *Properties) String() string {
- s := p.Wire
- s += "," + strconv.Itoa(p.Tag)
- if p.Required {
- s += ",req"
- }
- if p.Optional {
- s += ",opt"
- }
- if p.Repeated {
- s += ",rep"
- }
- if p.Packed {
- s += ",packed"
- }
- s += ",name=" + p.OrigName
- if p.JSONName != "" {
- s += ",json=" + p.JSONName
- }
- if len(p.Enum) > 0 {
- s += ",enum=" + p.Enum
- }
- if len(p.Weak) > 0 {
- s += ",weak=" + p.Weak
- }
- if p.Proto3 {
- s += ",proto3"
- }
- if p.Oneof {
- s += ",oneof"
- }
- if p.HasDefault {
- s += ",def=" + p.Default
- }
- return s
-}
-
-// Parse populates p by parsing a string in the protobuf struct field tag style.
-func (p *Properties) Parse(tag string) {
- // For example: "bytes,49,opt,name=foo,def=hello!"
- for len(tag) > 0 {
- i := strings.IndexByte(tag, ',')
- if i < 0 {
- i = len(tag)
- }
- switch s := tag[:i]; {
- case strings.HasPrefix(s, "name="):
- p.OrigName = s[len("name="):]
- case strings.HasPrefix(s, "json="):
- p.JSONName = s[len("json="):]
- case strings.HasPrefix(s, "enum="):
- p.Enum = s[len("enum="):]
- case strings.HasPrefix(s, "weak="):
- p.Weak = s[len("weak="):]
- case strings.Trim(s, "0123456789") == "":
- n, _ := strconv.ParseUint(s, 10, 32)
- p.Tag = int(n)
- case s == "opt":
- p.Optional = true
- case s == "req":
- p.Required = true
- case s == "rep":
- p.Repeated = true
- case s == "varint" || s == "zigzag32" || s == "zigzag64":
- p.Wire = s
- p.WireType = WireVarint
- case s == "fixed32":
- p.Wire = s
- p.WireType = WireFixed32
- case s == "fixed64":
- p.Wire = s
- p.WireType = WireFixed64
- case s == "bytes":
- p.Wire = s
- p.WireType = WireBytes
- case s == "group":
- p.Wire = s
- p.WireType = WireStartGroup
- case s == "packed":
- p.Packed = true
- case s == "proto3":
- p.Proto3 = true
- case s == "oneof":
- p.Oneof = true
- case strings.HasPrefix(s, "def="):
- // The default tag is special in that everything afterwards is the
- // default regardless of the presence of commas.
- p.HasDefault = true
- p.Default, i = tag[len("def="):], len(tag)
- }
- tag = strings.TrimPrefix(tag[i:], ",")
- }
-}
-
-// Init populates the properties from a protocol buffer struct tag.
-//
-// Deprecated: Do not use.
-func (p *Properties) Init(typ reflect.Type, name, tag string, f *reflect.StructField) {
- p.Name = name
- p.OrigName = name
- if tag == "" {
- return
- }
- p.Parse(tag)
-
- if typ != nil && typ.Kind() == reflect.Map {
- p.MapKeyProp = new(Properties)
- p.MapKeyProp.Init(nil, "Key", f.Tag.Get("protobuf_key"), nil)
- p.MapValProp = new(Properties)
- p.MapValProp.Init(nil, "Value", f.Tag.Get("protobuf_val"), nil)
- }
-}
-
-var propertiesCache sync.Map // map[reflect.Type]*StructProperties
-
-// GetProperties returns the list of properties for the type represented by t,
-// which must be a generated protocol buffer message in the open-struct API,
-// where protobuf message fields are represented by exported Go struct fields.
-//
-// Deprecated: Use protobuf reflection instead.
-func GetProperties(t reflect.Type) *StructProperties {
- if p, ok := propertiesCache.Load(t); ok {
- return p.(*StructProperties)
- }
- p, _ := propertiesCache.LoadOrStore(t, newProperties(t))
- return p.(*StructProperties)
-}
-
-func newProperties(t reflect.Type) *StructProperties {
- if t.Kind() != reflect.Struct {
- panic(fmt.Sprintf("%v is not a generated message in the open-struct API", t))
- }
-
- var hasOneof bool
- prop := new(StructProperties)
-
- // Construct a list of properties for each field in the struct.
- for i := 0; i < t.NumField(); i++ {
- p := new(Properties)
- f := t.Field(i)
- tagField := f.Tag.Get("protobuf")
- p.Init(f.Type, f.Name, tagField, &f)
-
- tagOneof := f.Tag.Get("protobuf_oneof")
- if tagOneof != "" {
- hasOneof = true
- p.OrigName = tagOneof
- }
-
- // Rename unrelated struct fields with the "XXX_" prefix since so much
- // user code simply checks for this to exclude special fields.
- if tagField == "" && tagOneof == "" && !strings.HasPrefix(p.Name, "XXX_") {
- p.Name = "XXX_" + p.Name
- p.OrigName = "XXX_" + p.OrigName
- } else if p.Weak != "" {
- p.Name = p.OrigName // avoid possible "XXX_" prefix on weak field
- }
-
- prop.Prop = append(prop.Prop, p)
- }
-
- // Construct a mapping of oneof field names to properties.
- if hasOneof {
- var oneofWrappers []interface{}
- if fn, ok := reflect.PtrTo(t).MethodByName("XXX_OneofFuncs"); ok {
- oneofWrappers = fn.Func.Call([]reflect.Value{reflect.Zero(fn.Type.In(0))})[3].Interface().([]interface{})
- }
- if fn, ok := reflect.PtrTo(t).MethodByName("XXX_OneofWrappers"); ok {
- oneofWrappers = fn.Func.Call([]reflect.Value{reflect.Zero(fn.Type.In(0))})[0].Interface().([]interface{})
- }
- if m, ok := reflect.Zero(reflect.PtrTo(t)).Interface().(protoreflect.ProtoMessage); ok {
- if m, ok := m.ProtoReflect().(interface{ ProtoMessageInfo() *protoimpl.MessageInfo }); ok {
- oneofWrappers = m.ProtoMessageInfo().OneofWrappers
- }
- }
-
- prop.OneofTypes = make(map[string]*OneofProperties)
- for _, wrapper := range oneofWrappers {
- p := &OneofProperties{
- Type: reflect.ValueOf(wrapper).Type(), // *T
- Prop: new(Properties),
- }
- f := p.Type.Elem().Field(0)
- p.Prop.Name = f.Name
- p.Prop.Parse(f.Tag.Get("protobuf"))
-
- // Determine the struct field that contains this oneof.
- // Each wrapper is assignable to exactly one parent field.
- var foundOneof bool
- for i := 0; i < t.NumField() && !foundOneof; i++ {
- if p.Type.AssignableTo(t.Field(i).Type) {
- p.Field = i
- foundOneof = true
- }
- }
- if !foundOneof {
- panic(fmt.Sprintf("%v is not a generated message in the open-struct API", t))
- }
- prop.OneofTypes[p.Prop.OrigName] = p
- }
- }
-
- return prop
-}
-
-func (sp *StructProperties) Len() int { return len(sp.Prop) }
-func (sp *StructProperties) Less(i, j int) bool { return false }
-func (sp *StructProperties) Swap(i, j int) { return }
diff --git a/vendor/github.com/golang/protobuf/proto/proto.go b/vendor/github.com/golang/protobuf/proto/proto.go
deleted file mode 100644
index 5aee89c3..00000000
--- a/vendor/github.com/golang/protobuf/proto/proto.go
+++ /dev/null
@@ -1,167 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package proto provides functionality for handling protocol buffer messages.
-// In particular, it provides marshaling and unmarshaling between a protobuf
-// message and the binary wire format.
-//
-// See https://developers.google.com/protocol-buffers/docs/gotutorial for
-// more information.
-//
-// Deprecated: Use the "google.golang.org/protobuf/proto" package instead.
-package proto
-
-import (
- protoV2 "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/runtime/protoiface"
- "google.golang.org/protobuf/runtime/protoimpl"
-)
-
-const (
- ProtoPackageIsVersion1 = true
- ProtoPackageIsVersion2 = true
- ProtoPackageIsVersion3 = true
- ProtoPackageIsVersion4 = true
-)
-
-// GeneratedEnum is any enum type generated by protoc-gen-go
-// which is a named int32 kind.
-// This type exists for documentation purposes.
-type GeneratedEnum interface{}
-
-// GeneratedMessage is any message type generated by protoc-gen-go
-// which is a pointer to a named struct kind.
-// This type exists for documentation purposes.
-type GeneratedMessage interface{}
-
-// Message is a protocol buffer message.
-//
-// This is the v1 version of the message interface and is marginally better
-// than an empty interface as it lacks any method to programatically interact
-// with the contents of the message.
-//
-// A v2 message is declared in "google.golang.org/protobuf/proto".Message and
-// exposes protobuf reflection as a first-class feature of the interface.
-//
-// To convert a v1 message to a v2 message, use the MessageV2 function.
-// To convert a v2 message to a v1 message, use the MessageV1 function.
-type Message = protoiface.MessageV1
-
-// MessageV1 converts either a v1 or v2 message to a v1 message.
-// It returns nil if m is nil.
-func MessageV1(m GeneratedMessage) protoiface.MessageV1 {
- return protoimpl.X.ProtoMessageV1Of(m)
-}
-
-// MessageV2 converts either a v1 or v2 message to a v2 message.
-// It returns nil if m is nil.
-func MessageV2(m GeneratedMessage) protoV2.Message {
- return protoimpl.X.ProtoMessageV2Of(m)
-}
-
-// MessageReflect returns a reflective view for a message.
-// It returns nil if m is nil.
-func MessageReflect(m Message) protoreflect.Message {
- return protoimpl.X.MessageOf(m)
-}
-
-// Marshaler is implemented by messages that can marshal themselves.
-// This interface is used by the following functions: Size, Marshal,
-// Buffer.Marshal, and Buffer.EncodeMessage.
-//
-// Deprecated: Do not implement.
-type Marshaler interface {
- // Marshal formats the encoded bytes of the message.
- // It should be deterministic and emit valid protobuf wire data.
- // The caller takes ownership of the returned buffer.
- Marshal() ([]byte, error)
-}
-
-// Unmarshaler is implemented by messages that can unmarshal themselves.
-// This interface is used by the following functions: Unmarshal, UnmarshalMerge,
-// Buffer.Unmarshal, Buffer.DecodeMessage, and Buffer.DecodeGroup.
-//
-// Deprecated: Do not implement.
-type Unmarshaler interface {
- // Unmarshal parses the encoded bytes of the protobuf wire input.
- // The provided buffer is only valid for during method call.
- // It should not reset the receiver message.
- Unmarshal([]byte) error
-}
-
-// Merger is implemented by messages that can merge themselves.
-// This interface is used by the following functions: Clone and Merge.
-//
-// Deprecated: Do not implement.
-type Merger interface {
- // Merge merges the contents of src into the receiver message.
- // It clones all data structures in src such that it aliases no mutable
- // memory referenced by src.
- Merge(src Message)
-}
-
-// RequiredNotSetError is an error type returned when
-// marshaling or unmarshaling a message with missing required fields.
-type RequiredNotSetError struct {
- err error
-}
-
-func (e *RequiredNotSetError) Error() string {
- if e.err != nil {
- return e.err.Error()
- }
- return "proto: required field not set"
-}
-func (e *RequiredNotSetError) RequiredNotSet() bool {
- return true
-}
-
-func checkRequiredNotSet(m protoV2.Message) error {
- if err := protoV2.CheckInitialized(m); err != nil {
- return &RequiredNotSetError{err: err}
- }
- return nil
-}
-
-// Clone returns a deep copy of src.
-func Clone(src Message) Message {
- return MessageV1(protoV2.Clone(MessageV2(src)))
-}
-
-// Merge merges src into dst, which must be messages of the same type.
-//
-// Populated scalar fields in src are copied to dst, while populated
-// singular messages in src are merged into dst by recursively calling Merge.
-// The elements of every list field in src is appended to the corresponded
-// list fields in dst. The entries of every map field in src is copied into
-// the corresponding map field in dst, possibly replacing existing entries.
-// The unknown fields of src are appended to the unknown fields of dst.
-func Merge(dst, src Message) {
- protoV2.Merge(MessageV2(dst), MessageV2(src))
-}
-
-// Equal reports whether two messages are equal.
-// If two messages marshal to the same bytes under deterministic serialization,
-// then Equal is guaranteed to report true.
-//
-// Two messages are equal if they are the same protobuf message type,
-// have the same set of populated known and extension field values,
-// and the same set of unknown fields values.
-//
-// Scalar values are compared with the equivalent of the == operator in Go,
-// except bytes values which are compared using bytes.Equal and
-// floating point values which specially treat NaNs as equal.
-// Message values are compared by recursively calling Equal.
-// Lists are equal if each element value is also equal.
-// Maps are equal if they have the same set of keys, where the pair of values
-// for each key is also equal.
-func Equal(x, y Message) bool {
- return protoV2.Equal(MessageV2(x), MessageV2(y))
-}
-
-func isMessageSet(md protoreflect.MessageDescriptor) bool {
- ms, ok := md.(interface{ IsMessageSet() bool })
- return ok && ms.IsMessageSet()
-}
diff --git a/vendor/github.com/golang/protobuf/proto/registry.go b/vendor/github.com/golang/protobuf/proto/registry.go
deleted file mode 100644
index 066b4323..00000000
--- a/vendor/github.com/golang/protobuf/proto/registry.go
+++ /dev/null
@@ -1,317 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "bytes"
- "compress/gzip"
- "fmt"
- "io/ioutil"
- "reflect"
- "strings"
- "sync"
-
- "google.golang.org/protobuf/reflect/protodesc"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
- "google.golang.org/protobuf/runtime/protoimpl"
-)
-
-// filePath is the path to the proto source file.
-type filePath = string // e.g., "google/protobuf/descriptor.proto"
-
-// fileDescGZIP is the compressed contents of the encoded FileDescriptorProto.
-type fileDescGZIP = []byte
-
-var fileCache sync.Map // map[filePath]fileDescGZIP
-
-// RegisterFile is called from generated code to register the compressed
-// FileDescriptorProto with the file path for a proto source file.
-//
-// Deprecated: Use protoregistry.GlobalFiles.RegisterFile instead.
-func RegisterFile(s filePath, d fileDescGZIP) {
- // Decompress the descriptor.
- zr, err := gzip.NewReader(bytes.NewReader(d))
- if err != nil {
- panic(fmt.Sprintf("proto: invalid compressed file descriptor: %v", err))
- }
- b, err := ioutil.ReadAll(zr)
- if err != nil {
- panic(fmt.Sprintf("proto: invalid compressed file descriptor: %v", err))
- }
-
- // Construct a protoreflect.FileDescriptor from the raw descriptor.
- // Note that DescBuilder.Build automatically registers the constructed
- // file descriptor with the v2 registry.
- protoimpl.DescBuilder{RawDescriptor: b}.Build()
-
- // Locally cache the raw descriptor form for the file.
- fileCache.Store(s, d)
-}
-
-// FileDescriptor returns the compressed FileDescriptorProto given the file path
-// for a proto source file. It returns nil if not found.
-//
-// Deprecated: Use protoregistry.GlobalFiles.FindFileByPath instead.
-func FileDescriptor(s filePath) fileDescGZIP {
- if v, ok := fileCache.Load(s); ok {
- return v.(fileDescGZIP)
- }
-
- // Find the descriptor in the v2 registry.
- var b []byte
- if fd, _ := protoregistry.GlobalFiles.FindFileByPath(s); fd != nil {
- b, _ = Marshal(protodesc.ToFileDescriptorProto(fd))
- }
-
- // Locally cache the raw descriptor form for the file.
- if len(b) > 0 {
- v, _ := fileCache.LoadOrStore(s, protoimpl.X.CompressGZIP(b))
- return v.(fileDescGZIP)
- }
- return nil
-}
-
-// enumName is the name of an enum. For historical reasons, the enum name is
-// neither the full Go name nor the full protobuf name of the enum.
-// The name is the dot-separated combination of just the proto package that the
-// enum is declared within followed by the Go type name of the generated enum.
-type enumName = string // e.g., "my.proto.package.GoMessage_GoEnum"
-
-// enumsByName maps enum values by name to their numeric counterpart.
-type enumsByName = map[string]int32
-
-// enumsByNumber maps enum values by number to their name counterpart.
-type enumsByNumber = map[int32]string
-
-var enumCache sync.Map // map[enumName]enumsByName
-var numFilesCache sync.Map // map[protoreflect.FullName]int
-
-// RegisterEnum is called from the generated code to register the mapping of
-// enum value names to enum numbers for the enum identified by s.
-//
-// Deprecated: Use protoregistry.GlobalTypes.RegisterEnum instead.
-func RegisterEnum(s enumName, _ enumsByNumber, m enumsByName) {
- if _, ok := enumCache.Load(s); ok {
- panic("proto: duplicate enum registered: " + s)
- }
- enumCache.Store(s, m)
-
- // This does not forward registration to the v2 registry since this API
- // lacks sufficient information to construct a complete v2 enum descriptor.
-}
-
-// EnumValueMap returns the mapping from enum value names to enum numbers for
-// the enum of the given name. It returns nil if not found.
-//
-// Deprecated: Use protoregistry.GlobalTypes.FindEnumByName instead.
-func EnumValueMap(s enumName) enumsByName {
- if v, ok := enumCache.Load(s); ok {
- return v.(enumsByName)
- }
-
- // Check whether the cache is stale. If the number of files in the current
- // package differs, then it means that some enums may have been recently
- // registered upstream that we do not know about.
- var protoPkg protoreflect.FullName
- if i := strings.LastIndexByte(s, '.'); i >= 0 {
- protoPkg = protoreflect.FullName(s[:i])
- }
- v, _ := numFilesCache.Load(protoPkg)
- numFiles, _ := v.(int)
- if protoregistry.GlobalFiles.NumFilesByPackage(protoPkg) == numFiles {
- return nil // cache is up-to-date; was not found earlier
- }
-
- // Update the enum cache for all enums declared in the given proto package.
- numFiles = 0
- protoregistry.GlobalFiles.RangeFilesByPackage(protoPkg, func(fd protoreflect.FileDescriptor) bool {
- walkEnums(fd, func(ed protoreflect.EnumDescriptor) {
- name := protoimpl.X.LegacyEnumName(ed)
- if _, ok := enumCache.Load(name); !ok {
- m := make(enumsByName)
- evs := ed.Values()
- for i := evs.Len() - 1; i >= 0; i-- {
- ev := evs.Get(i)
- m[string(ev.Name())] = int32(ev.Number())
- }
- enumCache.LoadOrStore(name, m)
- }
- })
- numFiles++
- return true
- })
- numFilesCache.Store(protoPkg, numFiles)
-
- // Check cache again for enum map.
- if v, ok := enumCache.Load(s); ok {
- return v.(enumsByName)
- }
- return nil
-}
-
-// walkEnums recursively walks all enums declared in d.
-func walkEnums(d interface {
- Enums() protoreflect.EnumDescriptors
- Messages() protoreflect.MessageDescriptors
-}, f func(protoreflect.EnumDescriptor)) {
- eds := d.Enums()
- for i := eds.Len() - 1; i >= 0; i-- {
- f(eds.Get(i))
- }
- mds := d.Messages()
- for i := mds.Len() - 1; i >= 0; i-- {
- walkEnums(mds.Get(i), f)
- }
-}
-
-// messageName is the full name of protobuf message.
-type messageName = string
-
-var messageTypeCache sync.Map // map[messageName]reflect.Type
-
-// RegisterType is called from generated code to register the message Go type
-// for a message of the given name.
-//
-// Deprecated: Use protoregistry.GlobalTypes.RegisterMessage instead.
-func RegisterType(m Message, s messageName) {
- mt := protoimpl.X.LegacyMessageTypeOf(m, protoreflect.FullName(s))
- if err := protoregistry.GlobalTypes.RegisterMessage(mt); err != nil {
- panic(err)
- }
- messageTypeCache.Store(s, reflect.TypeOf(m))
-}
-
-// RegisterMapType is called from generated code to register the Go map type
-// for a protobuf message representing a map entry.
-//
-// Deprecated: Do not use.
-func RegisterMapType(m interface{}, s messageName) {
- t := reflect.TypeOf(m)
- if t.Kind() != reflect.Map {
- panic(fmt.Sprintf("invalid map kind: %v", t))
- }
- if _, ok := messageTypeCache.Load(s); ok {
- panic(fmt.Errorf("proto: duplicate proto message registered: %s", s))
- }
- messageTypeCache.Store(s, t)
-}
-
-// MessageType returns the message type for a named message.
-// It returns nil if not found.
-//
-// Deprecated: Use protoregistry.GlobalTypes.FindMessageByName instead.
-func MessageType(s messageName) reflect.Type {
- if v, ok := messageTypeCache.Load(s); ok {
- return v.(reflect.Type)
- }
-
- // Derive the message type from the v2 registry.
- var t reflect.Type
- if mt, _ := protoregistry.GlobalTypes.FindMessageByName(protoreflect.FullName(s)); mt != nil {
- t = messageGoType(mt)
- }
-
- // If we could not get a concrete type, it is possible that it is a
- // pseudo-message for a map entry.
- if t == nil {
- d, _ := protoregistry.GlobalFiles.FindDescriptorByName(protoreflect.FullName(s))
- if md, _ := d.(protoreflect.MessageDescriptor); md != nil && md.IsMapEntry() {
- kt := goTypeForField(md.Fields().ByNumber(1))
- vt := goTypeForField(md.Fields().ByNumber(2))
- t = reflect.MapOf(kt, vt)
- }
- }
-
- // Locally cache the message type for the given name.
- if t != nil {
- v, _ := messageTypeCache.LoadOrStore(s, t)
- return v.(reflect.Type)
- }
- return nil
-}
-
-func goTypeForField(fd protoreflect.FieldDescriptor) reflect.Type {
- switch k := fd.Kind(); k {
- case protoreflect.EnumKind:
- if et, _ := protoregistry.GlobalTypes.FindEnumByName(fd.Enum().FullName()); et != nil {
- return enumGoType(et)
- }
- return reflect.TypeOf(protoreflect.EnumNumber(0))
- case protoreflect.MessageKind, protoreflect.GroupKind:
- if mt, _ := protoregistry.GlobalTypes.FindMessageByName(fd.Message().FullName()); mt != nil {
- return messageGoType(mt)
- }
- return reflect.TypeOf((*protoreflect.Message)(nil)).Elem()
- default:
- return reflect.TypeOf(fd.Default().Interface())
- }
-}
-
-func enumGoType(et protoreflect.EnumType) reflect.Type {
- return reflect.TypeOf(et.New(0))
-}
-
-func messageGoType(mt protoreflect.MessageType) reflect.Type {
- return reflect.TypeOf(MessageV1(mt.Zero().Interface()))
-}
-
-// MessageName returns the full protobuf name for the given message type.
-//
-// Deprecated: Use protoreflect.MessageDescriptor.FullName instead.
-func MessageName(m Message) messageName {
- if m == nil {
- return ""
- }
- if m, ok := m.(interface{ XXX_MessageName() messageName }); ok {
- return m.XXX_MessageName()
- }
- return messageName(protoimpl.X.MessageDescriptorOf(m).FullName())
-}
-
-// RegisterExtension is called from the generated code to register
-// the extension descriptor.
-//
-// Deprecated: Use protoregistry.GlobalTypes.RegisterExtension instead.
-func RegisterExtension(d *ExtensionDesc) {
- if err := protoregistry.GlobalTypes.RegisterExtension(d); err != nil {
- panic(err)
- }
-}
-
-type extensionsByNumber = map[int32]*ExtensionDesc
-
-var extensionCache sync.Map // map[messageName]extensionsByNumber
-
-// RegisteredExtensions returns a map of the registered extensions for the
-// provided protobuf message, indexed by the extension field number.
-//
-// Deprecated: Use protoregistry.GlobalTypes.RangeExtensionsByMessage instead.
-func RegisteredExtensions(m Message) extensionsByNumber {
- // Check whether the cache is stale. If the number of extensions for
- // the given message differs, then it means that some extensions were
- // recently registered upstream that we do not know about.
- s := MessageName(m)
- v, _ := extensionCache.Load(s)
- xs, _ := v.(extensionsByNumber)
- if protoregistry.GlobalTypes.NumExtensionsByMessage(protoreflect.FullName(s)) == len(xs) {
- return xs // cache is up-to-date
- }
-
- // Cache is stale, re-compute the extensions map.
- xs = make(extensionsByNumber)
- protoregistry.GlobalTypes.RangeExtensionsByMessage(protoreflect.FullName(s), func(xt protoreflect.ExtensionType) bool {
- if xd, ok := xt.(*ExtensionDesc); ok {
- xs[int32(xt.TypeDescriptor().Number())] = xd
- } else {
- // TODO: This implies that the protoreflect.ExtensionType is a
- // custom type not generated by protoc-gen-go. We could try and
- // convert the type to an ExtensionDesc.
- }
- return true
- })
- extensionCache.Store(s, xs)
- return xs
-}
diff --git a/vendor/github.com/golang/protobuf/proto/text_decode.go b/vendor/github.com/golang/protobuf/proto/text_decode.go
deleted file mode 100644
index 47eb3e44..00000000
--- a/vendor/github.com/golang/protobuf/proto/text_decode.go
+++ /dev/null
@@ -1,801 +0,0 @@
-// Copyright 2010 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "encoding"
- "errors"
- "fmt"
- "reflect"
- "strconv"
- "strings"
- "unicode/utf8"
-
- "google.golang.org/protobuf/encoding/prototext"
- protoV2 "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
-)
-
-const wrapTextUnmarshalV2 = false
-
-// ParseError is returned by UnmarshalText.
-type ParseError struct {
- Message string
-
- // Deprecated: Do not use.
- Line, Offset int
-}
-
-func (e *ParseError) Error() string {
- if wrapTextUnmarshalV2 {
- return e.Message
- }
- if e.Line == 1 {
- return fmt.Sprintf("line 1.%d: %v", e.Offset, e.Message)
- }
- return fmt.Sprintf("line %d: %v", e.Line, e.Message)
-}
-
-// UnmarshalText parses a proto text formatted string into m.
-func UnmarshalText(s string, m Message) error {
- if u, ok := m.(encoding.TextUnmarshaler); ok {
- return u.UnmarshalText([]byte(s))
- }
-
- m.Reset()
- mi := MessageV2(m)
-
- if wrapTextUnmarshalV2 {
- err := prototext.UnmarshalOptions{
- AllowPartial: true,
- }.Unmarshal([]byte(s), mi)
- if err != nil {
- return &ParseError{Message: err.Error()}
- }
- return checkRequiredNotSet(mi)
- } else {
- if err := newTextParser(s).unmarshalMessage(mi.ProtoReflect(), ""); err != nil {
- return err
- }
- return checkRequiredNotSet(mi)
- }
-}
-
-type textParser struct {
- s string // remaining input
- done bool // whether the parsing is finished (success or error)
- backed bool // whether back() was called
- offset, line int
- cur token
-}
-
-type token struct {
- value string
- err *ParseError
- line int // line number
- offset int // byte number from start of input, not start of line
- unquoted string // the unquoted version of value, if it was a quoted string
-}
-
-func newTextParser(s string) *textParser {
- p := new(textParser)
- p.s = s
- p.line = 1
- p.cur.line = 1
- return p
-}
-
-func (p *textParser) unmarshalMessage(m protoreflect.Message, terminator string) (err error) {
- md := m.Descriptor()
- fds := md.Fields()
-
- // A struct is a sequence of "name: value", terminated by one of
- // '>' or '}', or the end of the input. A name may also be
- // "[extension]" or "[type/url]".
- //
- // The whole struct can also be an expanded Any message, like:
- // [type/url] < ... struct contents ... >
- seen := make(map[protoreflect.FieldNumber]bool)
- for {
- tok := p.next()
- if tok.err != nil {
- return tok.err
- }
- if tok.value == terminator {
- break
- }
- if tok.value == "[" {
- if err := p.unmarshalExtensionOrAny(m, seen); err != nil {
- return err
- }
- continue
- }
-
- // This is a normal, non-extension field.
- name := protoreflect.Name(tok.value)
- fd := fds.ByName(name)
- switch {
- case fd == nil:
- gd := fds.ByName(protoreflect.Name(strings.ToLower(string(name))))
- if gd != nil && gd.Kind() == protoreflect.GroupKind && gd.Message().Name() == name {
- fd = gd
- }
- case fd.Kind() == protoreflect.GroupKind && fd.Message().Name() != name:
- fd = nil
- case fd.IsWeak() && fd.Message().IsPlaceholder():
- fd = nil
- }
- if fd == nil {
- typeName := string(md.FullName())
- if m, ok := m.Interface().(Message); ok {
- t := reflect.TypeOf(m)
- if t.Kind() == reflect.Ptr {
- typeName = t.Elem().String()
- }
- }
- return p.errorf("unknown field name %q in %v", name, typeName)
- }
- if od := fd.ContainingOneof(); od != nil && m.WhichOneof(od) != nil {
- return p.errorf("field '%s' would overwrite already parsed oneof '%s'", name, od.Name())
- }
- if fd.Cardinality() != protoreflect.Repeated && seen[fd.Number()] {
- return p.errorf("non-repeated field %q was repeated", fd.Name())
- }
- seen[fd.Number()] = true
-
- // Consume any colon.
- if err := p.checkForColon(fd); err != nil {
- return err
- }
-
- // Parse into the field.
- v := m.Get(fd)
- if !m.Has(fd) && (fd.IsList() || fd.IsMap() || fd.Message() != nil) {
- v = m.Mutable(fd)
- }
- if v, err = p.unmarshalValue(v, fd); err != nil {
- return err
- }
- m.Set(fd, v)
-
- if err := p.consumeOptionalSeparator(); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (p *textParser) unmarshalExtensionOrAny(m protoreflect.Message, seen map[protoreflect.FieldNumber]bool) error {
- name, err := p.consumeExtensionOrAnyName()
- if err != nil {
- return err
- }
-
- // If it contains a slash, it's an Any type URL.
- if slashIdx := strings.LastIndex(name, "/"); slashIdx >= 0 {
- tok := p.next()
- if tok.err != nil {
- return tok.err
- }
- // consume an optional colon
- if tok.value == ":" {
- tok = p.next()
- if tok.err != nil {
- return tok.err
- }
- }
-
- var terminator string
- switch tok.value {
- case "<":
- terminator = ">"
- case "{":
- terminator = "}"
- default:
- return p.errorf("expected '{' or '<', found %q", tok.value)
- }
-
- mt, err := protoregistry.GlobalTypes.FindMessageByURL(name)
- if err != nil {
- return p.errorf("unrecognized message %q in google.protobuf.Any", name[slashIdx+len("/"):])
- }
- m2 := mt.New()
- if err := p.unmarshalMessage(m2, terminator); err != nil {
- return err
- }
- b, err := protoV2.Marshal(m2.Interface())
- if err != nil {
- return p.errorf("failed to marshal message of type %q: %v", name[slashIdx+len("/"):], err)
- }
-
- urlFD := m.Descriptor().Fields().ByName("type_url")
- valFD := m.Descriptor().Fields().ByName("value")
- if seen[urlFD.Number()] {
- return p.errorf("Any message unpacked multiple times, or %q already set", urlFD.Name())
- }
- if seen[valFD.Number()] {
- return p.errorf("Any message unpacked multiple times, or %q already set", valFD.Name())
- }
- m.Set(urlFD, protoreflect.ValueOfString(name))
- m.Set(valFD, protoreflect.ValueOfBytes(b))
- seen[urlFD.Number()] = true
- seen[valFD.Number()] = true
- return nil
- }
-
- xname := protoreflect.FullName(name)
- xt, _ := protoregistry.GlobalTypes.FindExtensionByName(xname)
- if xt == nil && isMessageSet(m.Descriptor()) {
- xt, _ = protoregistry.GlobalTypes.FindExtensionByName(xname.Append("message_set_extension"))
- }
- if xt == nil {
- return p.errorf("unrecognized extension %q", name)
- }
- fd := xt.TypeDescriptor()
- if fd.ContainingMessage().FullName() != m.Descriptor().FullName() {
- return p.errorf("extension field %q does not extend message %q", name, m.Descriptor().FullName())
- }
-
- if err := p.checkForColon(fd); err != nil {
- return err
- }
-
- v := m.Get(fd)
- if !m.Has(fd) && (fd.IsList() || fd.IsMap() || fd.Message() != nil) {
- v = m.Mutable(fd)
- }
- v, err = p.unmarshalValue(v, fd)
- if err != nil {
- return err
- }
- m.Set(fd, v)
- return p.consumeOptionalSeparator()
-}
-
-func (p *textParser) unmarshalValue(v protoreflect.Value, fd protoreflect.FieldDescriptor) (protoreflect.Value, error) {
- tok := p.next()
- if tok.err != nil {
- return v, tok.err
- }
- if tok.value == "" {
- return v, p.errorf("unexpected EOF")
- }
-
- switch {
- case fd.IsList():
- lv := v.List()
- var err error
- if tok.value == "[" {
- // Repeated field with list notation, like [1,2,3].
- for {
- vv := lv.NewElement()
- vv, err = p.unmarshalSingularValue(vv, fd)
- if err != nil {
- return v, err
- }
- lv.Append(vv)
-
- tok := p.next()
- if tok.err != nil {
- return v, tok.err
- }
- if tok.value == "]" {
- break
- }
- if tok.value != "," {
- return v, p.errorf("Expected ']' or ',' found %q", tok.value)
- }
- }
- return v, nil
- }
-
- // One value of the repeated field.
- p.back()
- vv := lv.NewElement()
- vv, err = p.unmarshalSingularValue(vv, fd)
- if err != nil {
- return v, err
- }
- lv.Append(vv)
- return v, nil
- case fd.IsMap():
- // The map entry should be this sequence of tokens:
- // < key : KEY value : VALUE >
- // However, implementations may omit key or value, and technically
- // we should support them in any order.
- var terminator string
- switch tok.value {
- case "<":
- terminator = ">"
- case "{":
- terminator = "}"
- default:
- return v, p.errorf("expected '{' or '<', found %q", tok.value)
- }
-
- keyFD := fd.MapKey()
- valFD := fd.MapValue()
-
- mv := v.Map()
- kv := keyFD.Default()
- vv := mv.NewValue()
- for {
- tok := p.next()
- if tok.err != nil {
- return v, tok.err
- }
- if tok.value == terminator {
- break
- }
- var err error
- switch tok.value {
- case "key":
- if err := p.consumeToken(":"); err != nil {
- return v, err
- }
- if kv, err = p.unmarshalSingularValue(kv, keyFD); err != nil {
- return v, err
- }
- if err := p.consumeOptionalSeparator(); err != nil {
- return v, err
- }
- case "value":
- if err := p.checkForColon(valFD); err != nil {
- return v, err
- }
- if vv, err = p.unmarshalSingularValue(vv, valFD); err != nil {
- return v, err
- }
- if err := p.consumeOptionalSeparator(); err != nil {
- return v, err
- }
- default:
- p.back()
- return v, p.errorf(`expected "key", "value", or %q, found %q`, terminator, tok.value)
- }
- }
- mv.Set(kv.MapKey(), vv)
- return v, nil
- default:
- p.back()
- return p.unmarshalSingularValue(v, fd)
- }
-}
-
-func (p *textParser) unmarshalSingularValue(v protoreflect.Value, fd protoreflect.FieldDescriptor) (protoreflect.Value, error) {
- tok := p.next()
- if tok.err != nil {
- return v, tok.err
- }
- if tok.value == "" {
- return v, p.errorf("unexpected EOF")
- }
-
- switch fd.Kind() {
- case protoreflect.BoolKind:
- switch tok.value {
- case "true", "1", "t", "True":
- return protoreflect.ValueOfBool(true), nil
- case "false", "0", "f", "False":
- return protoreflect.ValueOfBool(false), nil
- }
- case protoreflect.Int32Kind, protoreflect.Sint32Kind, protoreflect.Sfixed32Kind:
- if x, err := strconv.ParseInt(tok.value, 0, 32); err == nil {
- return protoreflect.ValueOfInt32(int32(x)), nil
- }
-
- // The C++ parser accepts large positive hex numbers that uses
- // two's complement arithmetic to represent negative numbers.
- // This feature is here for backwards compatibility with C++.
- if strings.HasPrefix(tok.value, "0x") {
- if x, err := strconv.ParseUint(tok.value, 0, 32); err == nil {
- return protoreflect.ValueOfInt32(int32(-(int64(^x) + 1))), nil
- }
- }
- case protoreflect.Int64Kind, protoreflect.Sint64Kind, protoreflect.Sfixed64Kind:
- if x, err := strconv.ParseInt(tok.value, 0, 64); err == nil {
- return protoreflect.ValueOfInt64(int64(x)), nil
- }
-
- // The C++ parser accepts large positive hex numbers that uses
- // two's complement arithmetic to represent negative numbers.
- // This feature is here for backwards compatibility with C++.
- if strings.HasPrefix(tok.value, "0x") {
- if x, err := strconv.ParseUint(tok.value, 0, 64); err == nil {
- return protoreflect.ValueOfInt64(int64(-(int64(^x) + 1))), nil
- }
- }
- case protoreflect.Uint32Kind, protoreflect.Fixed32Kind:
- if x, err := strconv.ParseUint(tok.value, 0, 32); err == nil {
- return protoreflect.ValueOfUint32(uint32(x)), nil
- }
- case protoreflect.Uint64Kind, protoreflect.Fixed64Kind:
- if x, err := strconv.ParseUint(tok.value, 0, 64); err == nil {
- return protoreflect.ValueOfUint64(uint64(x)), nil
- }
- case protoreflect.FloatKind:
- // Ignore 'f' for compatibility with output generated by C++,
- // but don't remove 'f' when the value is "-inf" or "inf".
- v := tok.value
- if strings.HasSuffix(v, "f") && v != "-inf" && v != "inf" {
- v = v[:len(v)-len("f")]
- }
- if x, err := strconv.ParseFloat(v, 32); err == nil {
- return protoreflect.ValueOfFloat32(float32(x)), nil
- }
- case protoreflect.DoubleKind:
- // Ignore 'f' for compatibility with output generated by C++,
- // but don't remove 'f' when the value is "-inf" or "inf".
- v := tok.value
- if strings.HasSuffix(v, "f") && v != "-inf" && v != "inf" {
- v = v[:len(v)-len("f")]
- }
- if x, err := strconv.ParseFloat(v, 64); err == nil {
- return protoreflect.ValueOfFloat64(float64(x)), nil
- }
- case protoreflect.StringKind:
- if isQuote(tok.value[0]) {
- return protoreflect.ValueOfString(tok.unquoted), nil
- }
- case protoreflect.BytesKind:
- if isQuote(tok.value[0]) {
- return protoreflect.ValueOfBytes([]byte(tok.unquoted)), nil
- }
- case protoreflect.EnumKind:
- if x, err := strconv.ParseInt(tok.value, 0, 32); err == nil {
- return protoreflect.ValueOfEnum(protoreflect.EnumNumber(x)), nil
- }
- vd := fd.Enum().Values().ByName(protoreflect.Name(tok.value))
- if vd != nil {
- return protoreflect.ValueOfEnum(vd.Number()), nil
- }
- case protoreflect.MessageKind, protoreflect.GroupKind:
- var terminator string
- switch tok.value {
- case "{":
- terminator = "}"
- case "<":
- terminator = ">"
- default:
- return v, p.errorf("expected '{' or '<', found %q", tok.value)
- }
- err := p.unmarshalMessage(v.Message(), terminator)
- return v, err
- default:
- panic(fmt.Sprintf("invalid kind %v", fd.Kind()))
- }
- return v, p.errorf("invalid %v: %v", fd.Kind(), tok.value)
-}
-
-// Consume a ':' from the input stream (if the next token is a colon),
-// returning an error if a colon is needed but not present.
-func (p *textParser) checkForColon(fd protoreflect.FieldDescriptor) *ParseError {
- tok := p.next()
- if tok.err != nil {
- return tok.err
- }
- if tok.value != ":" {
- if fd.Message() == nil {
- return p.errorf("expected ':', found %q", tok.value)
- }
- p.back()
- }
- return nil
-}
-
-// consumeExtensionOrAnyName consumes an extension name or an Any type URL and
-// the following ']'. It returns the name or URL consumed.
-func (p *textParser) consumeExtensionOrAnyName() (string, error) {
- tok := p.next()
- if tok.err != nil {
- return "", tok.err
- }
-
- // If extension name or type url is quoted, it's a single token.
- if len(tok.value) > 2 && isQuote(tok.value[0]) && tok.value[len(tok.value)-1] == tok.value[0] {
- name, err := unquoteC(tok.value[1:len(tok.value)-1], rune(tok.value[0]))
- if err != nil {
- return "", err
- }
- return name, p.consumeToken("]")
- }
-
- // Consume everything up to "]"
- var parts []string
- for tok.value != "]" {
- parts = append(parts, tok.value)
- tok = p.next()
- if tok.err != nil {
- return "", p.errorf("unrecognized type_url or extension name: %s", tok.err)
- }
- if p.done && tok.value != "]" {
- return "", p.errorf("unclosed type_url or extension name")
- }
- }
- return strings.Join(parts, ""), nil
-}
-
-// consumeOptionalSeparator consumes an optional semicolon or comma.
-// It is used in unmarshalMessage to provide backward compatibility.
-func (p *textParser) consumeOptionalSeparator() error {
- tok := p.next()
- if tok.err != nil {
- return tok.err
- }
- if tok.value != ";" && tok.value != "," {
- p.back()
- }
- return nil
-}
-
-func (p *textParser) errorf(format string, a ...interface{}) *ParseError {
- pe := &ParseError{fmt.Sprintf(format, a...), p.cur.line, p.cur.offset}
- p.cur.err = pe
- p.done = true
- return pe
-}
-
-func (p *textParser) skipWhitespace() {
- i := 0
- for i < len(p.s) && (isWhitespace(p.s[i]) || p.s[i] == '#') {
- if p.s[i] == '#' {
- // comment; skip to end of line or input
- for i < len(p.s) && p.s[i] != '\n' {
- i++
- }
- if i == len(p.s) {
- break
- }
- }
- if p.s[i] == '\n' {
- p.line++
- }
- i++
- }
- p.offset += i
- p.s = p.s[i:len(p.s)]
- if len(p.s) == 0 {
- p.done = true
- }
-}
-
-func (p *textParser) advance() {
- // Skip whitespace
- p.skipWhitespace()
- if p.done {
- return
- }
-
- // Start of non-whitespace
- p.cur.err = nil
- p.cur.offset, p.cur.line = p.offset, p.line
- p.cur.unquoted = ""
- switch p.s[0] {
- case '<', '>', '{', '}', ':', '[', ']', ';', ',', '/':
- // Single symbol
- p.cur.value, p.s = p.s[0:1], p.s[1:len(p.s)]
- case '"', '\'':
- // Quoted string
- i := 1
- for i < len(p.s) && p.s[i] != p.s[0] && p.s[i] != '\n' {
- if p.s[i] == '\\' && i+1 < len(p.s) {
- // skip escaped char
- i++
- }
- i++
- }
- if i >= len(p.s) || p.s[i] != p.s[0] {
- p.errorf("unmatched quote")
- return
- }
- unq, err := unquoteC(p.s[1:i], rune(p.s[0]))
- if err != nil {
- p.errorf("invalid quoted string %s: %v", p.s[0:i+1], err)
- return
- }
- p.cur.value, p.s = p.s[0:i+1], p.s[i+1:len(p.s)]
- p.cur.unquoted = unq
- default:
- i := 0
- for i < len(p.s) && isIdentOrNumberChar(p.s[i]) {
- i++
- }
- if i == 0 {
- p.errorf("unexpected byte %#x", p.s[0])
- return
- }
- p.cur.value, p.s = p.s[0:i], p.s[i:len(p.s)]
- }
- p.offset += len(p.cur.value)
-}
-
-// Back off the parser by one token. Can only be done between calls to next().
-// It makes the next advance() a no-op.
-func (p *textParser) back() { p.backed = true }
-
-// Advances the parser and returns the new current token.
-func (p *textParser) next() *token {
- if p.backed || p.done {
- p.backed = false
- return &p.cur
- }
- p.advance()
- if p.done {
- p.cur.value = ""
- } else if len(p.cur.value) > 0 && isQuote(p.cur.value[0]) {
- // Look for multiple quoted strings separated by whitespace,
- // and concatenate them.
- cat := p.cur
- for {
- p.skipWhitespace()
- if p.done || !isQuote(p.s[0]) {
- break
- }
- p.advance()
- if p.cur.err != nil {
- return &p.cur
- }
- cat.value += " " + p.cur.value
- cat.unquoted += p.cur.unquoted
- }
- p.done = false // parser may have seen EOF, but we want to return cat
- p.cur = cat
- }
- return &p.cur
-}
-
-func (p *textParser) consumeToken(s string) error {
- tok := p.next()
- if tok.err != nil {
- return tok.err
- }
- if tok.value != s {
- p.back()
- return p.errorf("expected %q, found %q", s, tok.value)
- }
- return nil
-}
-
-var errBadUTF8 = errors.New("proto: bad UTF-8")
-
-func unquoteC(s string, quote rune) (string, error) {
- // This is based on C++'s tokenizer.cc.
- // Despite its name, this is *not* parsing C syntax.
- // For instance, "\0" is an invalid quoted string.
-
- // Avoid allocation in trivial cases.
- simple := true
- for _, r := range s {
- if r == '\\' || r == quote {
- simple = false
- break
- }
- }
- if simple {
- return s, nil
- }
-
- buf := make([]byte, 0, 3*len(s)/2)
- for len(s) > 0 {
- r, n := utf8.DecodeRuneInString(s)
- if r == utf8.RuneError && n == 1 {
- return "", errBadUTF8
- }
- s = s[n:]
- if r != '\\' {
- if r < utf8.RuneSelf {
- buf = append(buf, byte(r))
- } else {
- buf = append(buf, string(r)...)
- }
- continue
- }
-
- ch, tail, err := unescape(s)
- if err != nil {
- return "", err
- }
- buf = append(buf, ch...)
- s = tail
- }
- return string(buf), nil
-}
-
-func unescape(s string) (ch string, tail string, err error) {
- r, n := utf8.DecodeRuneInString(s)
- if r == utf8.RuneError && n == 1 {
- return "", "", errBadUTF8
- }
- s = s[n:]
- switch r {
- case 'a':
- return "\a", s, nil
- case 'b':
- return "\b", s, nil
- case 'f':
- return "\f", s, nil
- case 'n':
- return "\n", s, nil
- case 'r':
- return "\r", s, nil
- case 't':
- return "\t", s, nil
- case 'v':
- return "\v", s, nil
- case '?':
- return "?", s, nil // trigraph workaround
- case '\'', '"', '\\':
- return string(r), s, nil
- case '0', '1', '2', '3', '4', '5', '6', '7':
- if len(s) < 2 {
- return "", "", fmt.Errorf(`\%c requires 2 following digits`, r)
- }
- ss := string(r) + s[:2]
- s = s[2:]
- i, err := strconv.ParseUint(ss, 8, 8)
- if err != nil {
- return "", "", fmt.Errorf(`\%s contains non-octal digits`, ss)
- }
- return string([]byte{byte(i)}), s, nil
- case 'x', 'X', 'u', 'U':
- var n int
- switch r {
- case 'x', 'X':
- n = 2
- case 'u':
- n = 4
- case 'U':
- n = 8
- }
- if len(s) < n {
- return "", "", fmt.Errorf(`\%c requires %d following digits`, r, n)
- }
- ss := s[:n]
- s = s[n:]
- i, err := strconv.ParseUint(ss, 16, 64)
- if err != nil {
- return "", "", fmt.Errorf(`\%c%s contains non-hexadecimal digits`, r, ss)
- }
- if r == 'x' || r == 'X' {
- return string([]byte{byte(i)}), s, nil
- }
- if i > utf8.MaxRune {
- return "", "", fmt.Errorf(`\%c%s is not a valid Unicode code point`, r, ss)
- }
- return string(rune(i)), s, nil
- }
- return "", "", fmt.Errorf(`unknown escape \%c`, r)
-}
-
-func isIdentOrNumberChar(c byte) bool {
- switch {
- case 'A' <= c && c <= 'Z', 'a' <= c && c <= 'z':
- return true
- case '0' <= c && c <= '9':
- return true
- }
- switch c {
- case '-', '+', '.', '_':
- return true
- }
- return false
-}
-
-func isWhitespace(c byte) bool {
- switch c {
- case ' ', '\t', '\n', '\r':
- return true
- }
- return false
-}
-
-func isQuote(c byte) bool {
- switch c {
- case '"', '\'':
- return true
- }
- return false
-}
diff --git a/vendor/github.com/golang/protobuf/proto/text_encode.go b/vendor/github.com/golang/protobuf/proto/text_encode.go
deleted file mode 100644
index a31134ee..00000000
--- a/vendor/github.com/golang/protobuf/proto/text_encode.go
+++ /dev/null
@@ -1,560 +0,0 @@
-// Copyright 2010 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- "bytes"
- "encoding"
- "fmt"
- "io"
- "math"
- "sort"
- "strings"
-
- "google.golang.org/protobuf/encoding/prototext"
- "google.golang.org/protobuf/encoding/protowire"
- "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
-)
-
-const wrapTextMarshalV2 = false
-
-// TextMarshaler is a configurable text format marshaler.
-type TextMarshaler struct {
- Compact bool // use compact text format (one line)
- ExpandAny bool // expand google.protobuf.Any messages of known types
-}
-
-// Marshal writes the proto text format of m to w.
-func (tm *TextMarshaler) Marshal(w io.Writer, m Message) error {
- b, err := tm.marshal(m)
- if len(b) > 0 {
- if _, err := w.Write(b); err != nil {
- return err
- }
- }
- return err
-}
-
-// Text returns a proto text formatted string of m.
-func (tm *TextMarshaler) Text(m Message) string {
- b, _ := tm.marshal(m)
- return string(b)
-}
-
-func (tm *TextMarshaler) marshal(m Message) ([]byte, error) {
- mr := MessageReflect(m)
- if mr == nil || !mr.IsValid() {
- return []byte(""), nil
- }
-
- if wrapTextMarshalV2 {
- if m, ok := m.(encoding.TextMarshaler); ok {
- return m.MarshalText()
- }
-
- opts := prototext.MarshalOptions{
- AllowPartial: true,
- EmitUnknown: true,
- }
- if !tm.Compact {
- opts.Indent = " "
- }
- if !tm.ExpandAny {
- opts.Resolver = (*protoregistry.Types)(nil)
- }
- return opts.Marshal(mr.Interface())
- } else {
- w := &textWriter{
- compact: tm.Compact,
- expandAny: tm.ExpandAny,
- complete: true,
- }
-
- if m, ok := m.(encoding.TextMarshaler); ok {
- b, err := m.MarshalText()
- if err != nil {
- return nil, err
- }
- w.Write(b)
- return w.buf, nil
- }
-
- err := w.writeMessage(mr)
- return w.buf, err
- }
-}
-
-var (
- defaultTextMarshaler = TextMarshaler{}
- compactTextMarshaler = TextMarshaler{Compact: true}
-)
-
-// MarshalText writes the proto text format of m to w.
-func MarshalText(w io.Writer, m Message) error { return defaultTextMarshaler.Marshal(w, m) }
-
-// MarshalTextString returns a proto text formatted string of m.
-func MarshalTextString(m Message) string { return defaultTextMarshaler.Text(m) }
-
-// CompactText writes the compact proto text format of m to w.
-func CompactText(w io.Writer, m Message) error { return compactTextMarshaler.Marshal(w, m) }
-
-// CompactTextString returns a compact proto text formatted string of m.
-func CompactTextString(m Message) string { return compactTextMarshaler.Text(m) }
-
-var (
- newline = []byte("\n")
- endBraceNewline = []byte("}\n")
- posInf = []byte("inf")
- negInf = []byte("-inf")
- nan = []byte("nan")
-)
-
-// textWriter is an io.Writer that tracks its indentation level.
-type textWriter struct {
- compact bool // same as TextMarshaler.Compact
- expandAny bool // same as TextMarshaler.ExpandAny
- complete bool // whether the current position is a complete line
- indent int // indentation level; never negative
- buf []byte
-}
-
-func (w *textWriter) Write(p []byte) (n int, _ error) {
- newlines := bytes.Count(p, newline)
- if newlines == 0 {
- if !w.compact && w.complete {
- w.writeIndent()
- }
- w.buf = append(w.buf, p...)
- w.complete = false
- return len(p), nil
- }
-
- frags := bytes.SplitN(p, newline, newlines+1)
- if w.compact {
- for i, frag := range frags {
- if i > 0 {
- w.buf = append(w.buf, ' ')
- n++
- }
- w.buf = append(w.buf, frag...)
- n += len(frag)
- }
- return n, nil
- }
-
- for i, frag := range frags {
- if w.complete {
- w.writeIndent()
- }
- w.buf = append(w.buf, frag...)
- n += len(frag)
- if i+1 < len(frags) {
- w.buf = append(w.buf, '\n')
- n++
- }
- }
- w.complete = len(frags[len(frags)-1]) == 0
- return n, nil
-}
-
-func (w *textWriter) WriteByte(c byte) error {
- if w.compact && c == '\n' {
- c = ' '
- }
- if !w.compact && w.complete {
- w.writeIndent()
- }
- w.buf = append(w.buf, c)
- w.complete = c == '\n'
- return nil
-}
-
-func (w *textWriter) writeName(fd protoreflect.FieldDescriptor) {
- if !w.compact && w.complete {
- w.writeIndent()
- }
- w.complete = false
-
- if fd.Kind() != protoreflect.GroupKind {
- w.buf = append(w.buf, fd.Name()...)
- w.WriteByte(':')
- } else {
- // Use message type name for group field name.
- w.buf = append(w.buf, fd.Message().Name()...)
- }
-
- if !w.compact {
- w.WriteByte(' ')
- }
-}
-
-func requiresQuotes(u string) bool {
- // When type URL contains any characters except [0-9A-Za-z./\-]*, it must be quoted.
- for _, ch := range u {
- switch {
- case ch == '.' || ch == '/' || ch == '_':
- continue
- case '0' <= ch && ch <= '9':
- continue
- case 'A' <= ch && ch <= 'Z':
- continue
- case 'a' <= ch && ch <= 'z':
- continue
- default:
- return true
- }
- }
- return false
-}
-
-// writeProto3Any writes an expanded google.protobuf.Any message.
-//
-// It returns (false, nil) if sv value can't be unmarshaled (e.g. because
-// required messages are not linked in).
-//
-// It returns (true, error) when sv was written in expanded format or an error
-// was encountered.
-func (w *textWriter) writeProto3Any(m protoreflect.Message) (bool, error) {
- md := m.Descriptor()
- fdURL := md.Fields().ByName("type_url")
- fdVal := md.Fields().ByName("value")
-
- url := m.Get(fdURL).String()
- mt, err := protoregistry.GlobalTypes.FindMessageByURL(url)
- if err != nil {
- return false, nil
- }
-
- b := m.Get(fdVal).Bytes()
- m2 := mt.New()
- if err := proto.Unmarshal(b, m2.Interface()); err != nil {
- return false, nil
- }
- w.Write([]byte("["))
- if requiresQuotes(url) {
- w.writeQuotedString(url)
- } else {
- w.Write([]byte(url))
- }
- if w.compact {
- w.Write([]byte("]:<"))
- } else {
- w.Write([]byte("]: <\n"))
- w.indent++
- }
- if err := w.writeMessage(m2); err != nil {
- return true, err
- }
- if w.compact {
- w.Write([]byte("> "))
- } else {
- w.indent--
- w.Write([]byte(">\n"))
- }
- return true, nil
-}
-
-func (w *textWriter) writeMessage(m protoreflect.Message) error {
- md := m.Descriptor()
- if w.expandAny && md.FullName() == "google.protobuf.Any" {
- if canExpand, err := w.writeProto3Any(m); canExpand {
- return err
- }
- }
-
- fds := md.Fields()
- for i := 0; i < fds.Len(); {
- fd := fds.Get(i)
- if od := fd.ContainingOneof(); od != nil {
- fd = m.WhichOneof(od)
- i += od.Fields().Len()
- } else {
- i++
- }
- if fd == nil || !m.Has(fd) {
- continue
- }
-
- switch {
- case fd.IsList():
- lv := m.Get(fd).List()
- for j := 0; j < lv.Len(); j++ {
- w.writeName(fd)
- v := lv.Get(j)
- if err := w.writeSingularValue(v, fd); err != nil {
- return err
- }
- w.WriteByte('\n')
- }
- case fd.IsMap():
- kfd := fd.MapKey()
- vfd := fd.MapValue()
- mv := m.Get(fd).Map()
-
- type entry struct{ key, val protoreflect.Value }
- var entries []entry
- mv.Range(func(k protoreflect.MapKey, v protoreflect.Value) bool {
- entries = append(entries, entry{k.Value(), v})
- return true
- })
- sort.Slice(entries, func(i, j int) bool {
- switch kfd.Kind() {
- case protoreflect.BoolKind:
- return !entries[i].key.Bool() && entries[j].key.Bool()
- case protoreflect.Int32Kind, protoreflect.Sint32Kind, protoreflect.Sfixed32Kind, protoreflect.Int64Kind, protoreflect.Sint64Kind, protoreflect.Sfixed64Kind:
- return entries[i].key.Int() < entries[j].key.Int()
- case protoreflect.Uint32Kind, protoreflect.Fixed32Kind, protoreflect.Uint64Kind, protoreflect.Fixed64Kind:
- return entries[i].key.Uint() < entries[j].key.Uint()
- case protoreflect.StringKind:
- return entries[i].key.String() < entries[j].key.String()
- default:
- panic("invalid kind")
- }
- })
- for _, entry := range entries {
- w.writeName(fd)
- w.WriteByte('<')
- if !w.compact {
- w.WriteByte('\n')
- }
- w.indent++
- w.writeName(kfd)
- if err := w.writeSingularValue(entry.key, kfd); err != nil {
- return err
- }
- w.WriteByte('\n')
- w.writeName(vfd)
- if err := w.writeSingularValue(entry.val, vfd); err != nil {
- return err
- }
- w.WriteByte('\n')
- w.indent--
- w.WriteByte('>')
- w.WriteByte('\n')
- }
- default:
- w.writeName(fd)
- if err := w.writeSingularValue(m.Get(fd), fd); err != nil {
- return err
- }
- w.WriteByte('\n')
- }
- }
-
- if b := m.GetUnknown(); len(b) > 0 {
- w.writeUnknownFields(b)
- }
- return w.writeExtensions(m)
-}
-
-func (w *textWriter) writeSingularValue(v protoreflect.Value, fd protoreflect.FieldDescriptor) error {
- switch fd.Kind() {
- case protoreflect.FloatKind, protoreflect.DoubleKind:
- switch vf := v.Float(); {
- case math.IsInf(vf, +1):
- w.Write(posInf)
- case math.IsInf(vf, -1):
- w.Write(negInf)
- case math.IsNaN(vf):
- w.Write(nan)
- default:
- fmt.Fprint(w, v.Interface())
- }
- case protoreflect.StringKind:
- // NOTE: This does not validate UTF-8 for historical reasons.
- w.writeQuotedString(string(v.String()))
- case protoreflect.BytesKind:
- w.writeQuotedString(string(v.Bytes()))
- case protoreflect.MessageKind, protoreflect.GroupKind:
- var bra, ket byte = '<', '>'
- if fd.Kind() == protoreflect.GroupKind {
- bra, ket = '{', '}'
- }
- w.WriteByte(bra)
- if !w.compact {
- w.WriteByte('\n')
- }
- w.indent++
- m := v.Message()
- if m2, ok := m.Interface().(encoding.TextMarshaler); ok {
- b, err := m2.MarshalText()
- if err != nil {
- return err
- }
- w.Write(b)
- } else {
- w.writeMessage(m)
- }
- w.indent--
- w.WriteByte(ket)
- case protoreflect.EnumKind:
- if ev := fd.Enum().Values().ByNumber(v.Enum()); ev != nil {
- fmt.Fprint(w, ev.Name())
- } else {
- fmt.Fprint(w, v.Enum())
- }
- default:
- fmt.Fprint(w, v.Interface())
- }
- return nil
-}
-
-// writeQuotedString writes a quoted string in the protocol buffer text format.
-func (w *textWriter) writeQuotedString(s string) {
- w.WriteByte('"')
- for i := 0; i < len(s); i++ {
- switch c := s[i]; c {
- case '\n':
- w.buf = append(w.buf, `\n`...)
- case '\r':
- w.buf = append(w.buf, `\r`...)
- case '\t':
- w.buf = append(w.buf, `\t`...)
- case '"':
- w.buf = append(w.buf, `\"`...)
- case '\\':
- w.buf = append(w.buf, `\\`...)
- default:
- if isPrint := c >= 0x20 && c < 0x7f; isPrint {
- w.buf = append(w.buf, c)
- } else {
- w.buf = append(w.buf, fmt.Sprintf(`\%03o`, c)...)
- }
- }
- }
- w.WriteByte('"')
-}
-
-func (w *textWriter) writeUnknownFields(b []byte) {
- if !w.compact {
- fmt.Fprintf(w, "/* %d unknown bytes */\n", len(b))
- }
-
- for len(b) > 0 {
- num, wtyp, n := protowire.ConsumeTag(b)
- if n < 0 {
- return
- }
- b = b[n:]
-
- if wtyp == protowire.EndGroupType {
- w.indent--
- w.Write(endBraceNewline)
- continue
- }
- fmt.Fprint(w, num)
- if wtyp != protowire.StartGroupType {
- w.WriteByte(':')
- }
- if !w.compact || wtyp == protowire.StartGroupType {
- w.WriteByte(' ')
- }
- switch wtyp {
- case protowire.VarintType:
- v, n := protowire.ConsumeVarint(b)
- if n < 0 {
- return
- }
- b = b[n:]
- fmt.Fprint(w, v)
- case protowire.Fixed32Type:
- v, n := protowire.ConsumeFixed32(b)
- if n < 0 {
- return
- }
- b = b[n:]
- fmt.Fprint(w, v)
- case protowire.Fixed64Type:
- v, n := protowire.ConsumeFixed64(b)
- if n < 0 {
- return
- }
- b = b[n:]
- fmt.Fprint(w, v)
- case protowire.BytesType:
- v, n := protowire.ConsumeBytes(b)
- if n < 0 {
- return
- }
- b = b[n:]
- fmt.Fprintf(w, "%q", v)
- case protowire.StartGroupType:
- w.WriteByte('{')
- w.indent++
- default:
- fmt.Fprintf(w, "/* unknown wire type %d */", wtyp)
- }
- w.WriteByte('\n')
- }
-}
-
-// writeExtensions writes all the extensions in m.
-func (w *textWriter) writeExtensions(m protoreflect.Message) error {
- md := m.Descriptor()
- if md.ExtensionRanges().Len() == 0 {
- return nil
- }
-
- type ext struct {
- desc protoreflect.FieldDescriptor
- val protoreflect.Value
- }
- var exts []ext
- m.Range(func(fd protoreflect.FieldDescriptor, v protoreflect.Value) bool {
- if fd.IsExtension() {
- exts = append(exts, ext{fd, v})
- }
- return true
- })
- sort.Slice(exts, func(i, j int) bool {
- return exts[i].desc.Number() < exts[j].desc.Number()
- })
-
- for _, ext := range exts {
- // For message set, use the name of the message as the extension name.
- name := string(ext.desc.FullName())
- if isMessageSet(ext.desc.ContainingMessage()) {
- name = strings.TrimSuffix(name, ".message_set_extension")
- }
-
- if !ext.desc.IsList() {
- if err := w.writeSingularExtension(name, ext.val, ext.desc); err != nil {
- return err
- }
- } else {
- lv := ext.val.List()
- for i := 0; i < lv.Len(); i++ {
- if err := w.writeSingularExtension(name, lv.Get(i), ext.desc); err != nil {
- return err
- }
- }
- }
- }
- return nil
-}
-
-func (w *textWriter) writeSingularExtension(name string, v protoreflect.Value, fd protoreflect.FieldDescriptor) error {
- fmt.Fprintf(w, "[%s]:", name)
- if !w.compact {
- w.WriteByte(' ')
- }
- if err := w.writeSingularValue(v, fd); err != nil {
- return err
- }
- w.WriteByte('\n')
- return nil
-}
-
-func (w *textWriter) writeIndent() {
- if !w.complete {
- return
- }
- for i := 0; i < w.indent*2; i++ {
- w.buf = append(w.buf, ' ')
- }
- w.complete = false
-}
diff --git a/vendor/github.com/golang/protobuf/proto/wire.go b/vendor/github.com/golang/protobuf/proto/wire.go
deleted file mode 100644
index d7c28da5..00000000
--- a/vendor/github.com/golang/protobuf/proto/wire.go
+++ /dev/null
@@ -1,78 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-import (
- protoV2 "google.golang.org/protobuf/proto"
- "google.golang.org/protobuf/runtime/protoiface"
-)
-
-// Size returns the size in bytes of the wire-format encoding of m.
-func Size(m Message) int {
- if m == nil {
- return 0
- }
- mi := MessageV2(m)
- return protoV2.Size(mi)
-}
-
-// Marshal returns the wire-format encoding of m.
-func Marshal(m Message) ([]byte, error) {
- b, err := marshalAppend(nil, m, false)
- if b == nil {
- b = zeroBytes
- }
- return b, err
-}
-
-var zeroBytes = make([]byte, 0, 0)
-
-func marshalAppend(buf []byte, m Message, deterministic bool) ([]byte, error) {
- if m == nil {
- return nil, ErrNil
- }
- mi := MessageV2(m)
- nbuf, err := protoV2.MarshalOptions{
- Deterministic: deterministic,
- AllowPartial: true,
- }.MarshalAppend(buf, mi)
- if err != nil {
- return buf, err
- }
- if len(buf) == len(nbuf) {
- if !mi.ProtoReflect().IsValid() {
- return buf, ErrNil
- }
- }
- return nbuf, checkRequiredNotSet(mi)
-}
-
-// Unmarshal parses a wire-format message in b and places the decoded results in m.
-//
-// Unmarshal resets m before starting to unmarshal, so any existing data in m is always
-// removed. Use UnmarshalMerge to preserve and append to existing data.
-func Unmarshal(b []byte, m Message) error {
- m.Reset()
- return UnmarshalMerge(b, m)
-}
-
-// UnmarshalMerge parses a wire-format message in b and places the decoded results in m.
-func UnmarshalMerge(b []byte, m Message) error {
- mi := MessageV2(m)
- out, err := protoV2.UnmarshalOptions{
- AllowPartial: true,
- Merge: true,
- }.UnmarshalState(protoiface.UnmarshalInput{
- Buf: b,
- Message: mi.ProtoReflect(),
- })
- if err != nil {
- return err
- }
- if out.Flags&protoiface.UnmarshalInitialized > 0 {
- return nil
- }
- return checkRequiredNotSet(mi)
-}
diff --git a/vendor/github.com/golang/protobuf/proto/wrappers.go b/vendor/github.com/golang/protobuf/proto/wrappers.go
deleted file mode 100644
index 398e3485..00000000
--- a/vendor/github.com/golang/protobuf/proto/wrappers.go
+++ /dev/null
@@ -1,34 +0,0 @@
-// Copyright 2019 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package proto
-
-// Bool stores v in a new bool value and returns a pointer to it.
-func Bool(v bool) *bool { return &v }
-
-// Int stores v in a new int32 value and returns a pointer to it.
-//
-// Deprecated: Use Int32 instead.
-func Int(v int) *int32 { return Int32(int32(v)) }
-
-// Int32 stores v in a new int32 value and returns a pointer to it.
-func Int32(v int32) *int32 { return &v }
-
-// Int64 stores v in a new int64 value and returns a pointer to it.
-func Int64(v int64) *int64 { return &v }
-
-// Uint32 stores v in a new uint32 value and returns a pointer to it.
-func Uint32(v uint32) *uint32 { return &v }
-
-// Uint64 stores v in a new uint64 value and returns a pointer to it.
-func Uint64(v uint64) *uint64 { return &v }
-
-// Float32 stores v in a new float32 value and returns a pointer to it.
-func Float32(v float32) *float32 { return &v }
-
-// Float64 stores v in a new float64 value and returns a pointer to it.
-func Float64(v float64) *float64 { return &v }
-
-// String stores v in a new string value and returns a pointer to it.
-func String(v string) *string { return &v }
diff --git a/vendor/github.com/golang/protobuf/ptypes/any.go b/vendor/github.com/golang/protobuf/ptypes/any.go
deleted file mode 100644
index 85f9f573..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/any.go
+++ /dev/null
@@ -1,179 +0,0 @@
-// Copyright 2016 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package ptypes
-
-import (
- "fmt"
- "strings"
-
- "github.com/golang/protobuf/proto"
- "google.golang.org/protobuf/reflect/protoreflect"
- "google.golang.org/protobuf/reflect/protoregistry"
-
- anypb "github.com/golang/protobuf/ptypes/any"
-)
-
-const urlPrefix = "type.googleapis.com/"
-
-// AnyMessageName returns the message name contained in an anypb.Any message.
-// Most type assertions should use the Is function instead.
-//
-// Deprecated: Call the any.MessageName method instead.
-func AnyMessageName(any *anypb.Any) (string, error) {
- name, err := anyMessageName(any)
- return string(name), err
-}
-func anyMessageName(any *anypb.Any) (protoreflect.FullName, error) {
- if any == nil {
- return "", fmt.Errorf("message is nil")
- }
- name := protoreflect.FullName(any.TypeUrl)
- if i := strings.LastIndex(any.TypeUrl, "/"); i >= 0 {
- name = name[i+len("/"):]
- }
- if !name.IsValid() {
- return "", fmt.Errorf("message type url %q is invalid", any.TypeUrl)
- }
- return name, nil
-}
-
-// MarshalAny marshals the given message m into an anypb.Any message.
-//
-// Deprecated: Call the anypb.New function instead.
-func MarshalAny(m proto.Message) (*anypb.Any, error) {
- switch dm := m.(type) {
- case DynamicAny:
- m = dm.Message
- case *DynamicAny:
- if dm == nil {
- return nil, proto.ErrNil
- }
- m = dm.Message
- }
- b, err := proto.Marshal(m)
- if err != nil {
- return nil, err
- }
- return &anypb.Any{TypeUrl: urlPrefix + proto.MessageName(m), Value: b}, nil
-}
-
-// Empty returns a new message of the type specified in an anypb.Any message.
-// It returns protoregistry.NotFound if the corresponding message type could not
-// be resolved in the global registry.
-//
-// Deprecated: Use protoregistry.GlobalTypes.FindMessageByName instead
-// to resolve the message name and create a new instance of it.
-func Empty(any *anypb.Any) (proto.Message, error) {
- name, err := anyMessageName(any)
- if err != nil {
- return nil, err
- }
- mt, err := protoregistry.GlobalTypes.FindMessageByName(name)
- if err != nil {
- return nil, err
- }
- return proto.MessageV1(mt.New().Interface()), nil
-}
-
-// UnmarshalAny unmarshals the encoded value contained in the anypb.Any message
-// into the provided message m. It returns an error if the target message
-// does not match the type in the Any message or if an unmarshal error occurs.
-//
-// The target message m may be a *DynamicAny message. If the underlying message
-// type could not be resolved, then this returns protoregistry.NotFound.
-//
-// Deprecated: Call the any.UnmarshalTo method instead.
-func UnmarshalAny(any *anypb.Any, m proto.Message) error {
- if dm, ok := m.(*DynamicAny); ok {
- if dm.Message == nil {
- var err error
- dm.Message, err = Empty(any)
- if err != nil {
- return err
- }
- }
- m = dm.Message
- }
-
- anyName, err := AnyMessageName(any)
- if err != nil {
- return err
- }
- msgName := proto.MessageName(m)
- if anyName != msgName {
- return fmt.Errorf("mismatched message type: got %q want %q", anyName, msgName)
- }
- return proto.Unmarshal(any.Value, m)
-}
-
-// Is reports whether the Any message contains a message of the specified type.
-//
-// Deprecated: Call the any.MessageIs method instead.
-func Is(any *anypb.Any, m proto.Message) bool {
- if any == nil || m == nil {
- return false
- }
- name := proto.MessageName(m)
- if !strings.HasSuffix(any.TypeUrl, name) {
- return false
- }
- return len(any.TypeUrl) == len(name) || any.TypeUrl[len(any.TypeUrl)-len(name)-1] == '/'
-}
-
-// DynamicAny is a value that can be passed to UnmarshalAny to automatically
-// allocate a proto.Message for the type specified in an anypb.Any message.
-// The allocated message is stored in the embedded proto.Message.
-//
-// Example:
-// var x ptypes.DynamicAny
-// if err := ptypes.UnmarshalAny(a, &x); err != nil { ... }
-// fmt.Printf("unmarshaled message: %v", x.Message)
-//
-// Deprecated: Use the any.UnmarshalNew method instead to unmarshal
-// the any message contents into a new instance of the underlying message.
-type DynamicAny struct{ proto.Message }
-
-func (m DynamicAny) String() string {
- if m.Message == nil {
- return ""
- }
- return m.Message.String()
-}
-func (m DynamicAny) Reset() {
- if m.Message == nil {
- return
- }
- m.Message.Reset()
-}
-func (m DynamicAny) ProtoMessage() {
- return
-}
-func (m DynamicAny) ProtoReflect() protoreflect.Message {
- if m.Message == nil {
- return nil
- }
- return dynamicAny{proto.MessageReflect(m.Message)}
-}
-
-type dynamicAny struct{ protoreflect.Message }
-
-func (m dynamicAny) Type() protoreflect.MessageType {
- return dynamicAnyType{m.Message.Type()}
-}
-func (m dynamicAny) New() protoreflect.Message {
- return dynamicAnyType{m.Message.Type()}.New()
-}
-func (m dynamicAny) Interface() protoreflect.ProtoMessage {
- return DynamicAny{proto.MessageV1(m.Message.Interface())}
-}
-
-type dynamicAnyType struct{ protoreflect.MessageType }
-
-func (t dynamicAnyType) New() protoreflect.Message {
- return dynamicAny{t.MessageType.New()}
-}
-func (t dynamicAnyType) Zero() protoreflect.Message {
- return dynamicAny{t.MessageType.Zero()}
-}
diff --git a/vendor/github.com/golang/protobuf/ptypes/any/any.pb.go b/vendor/github.com/golang/protobuf/ptypes/any/any.pb.go
deleted file mode 100644
index 0ef27d33..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/any/any.pb.go
+++ /dev/null
@@ -1,62 +0,0 @@
-// Code generated by protoc-gen-go. DO NOT EDIT.
-// source: github.com/golang/protobuf/ptypes/any/any.proto
-
-package any
-
-import (
- protoreflect "google.golang.org/protobuf/reflect/protoreflect"
- protoimpl "google.golang.org/protobuf/runtime/protoimpl"
- anypb "google.golang.org/protobuf/types/known/anypb"
- reflect "reflect"
-)
-
-// Symbols defined in public import of google/protobuf/any.proto.
-
-type Any = anypb.Any
-
-var File_github_com_golang_protobuf_ptypes_any_any_proto protoreflect.FileDescriptor
-
-var file_github_com_golang_protobuf_ptypes_any_any_proto_rawDesc = []byte{
- 0x0a, 0x2f, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x67, 0x6f, 0x6c,
- 0x61, 0x6e, 0x67, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x70, 0x74, 0x79,
- 0x70, 0x65, 0x73, 0x2f, 0x61, 0x6e, 0x79, 0x2f, 0x61, 0x6e, 0x79, 0x2e, 0x70, 0x72, 0x6f, 0x74,
- 0x6f, 0x1a, 0x19, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
- 0x75, 0x66, 0x2f, 0x61, 0x6e, 0x79, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x42, 0x2b, 0x5a, 0x29,
- 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x67, 0x6f, 0x6c, 0x61, 0x6e,
- 0x67, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x70, 0x74, 0x79, 0x70, 0x65,
- 0x73, 0x2f, 0x61, 0x6e, 0x79, 0x3b, 0x61, 0x6e, 0x79, 0x50, 0x00, 0x62, 0x06, 0x70, 0x72, 0x6f,
- 0x74, 0x6f, 0x33,
-}
-
-var file_github_com_golang_protobuf_ptypes_any_any_proto_goTypes = []interface{}{}
-var file_github_com_golang_protobuf_ptypes_any_any_proto_depIdxs = []int32{
- 0, // [0:0] is the sub-list for method output_type
- 0, // [0:0] is the sub-list for method input_type
- 0, // [0:0] is the sub-list for extension type_name
- 0, // [0:0] is the sub-list for extension extendee
- 0, // [0:0] is the sub-list for field type_name
-}
-
-func init() { file_github_com_golang_protobuf_ptypes_any_any_proto_init() }
-func file_github_com_golang_protobuf_ptypes_any_any_proto_init() {
- if File_github_com_golang_protobuf_ptypes_any_any_proto != nil {
- return
- }
- type x struct{}
- out := protoimpl.TypeBuilder{
- File: protoimpl.DescBuilder{
- GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
- RawDescriptor: file_github_com_golang_protobuf_ptypes_any_any_proto_rawDesc,
- NumEnums: 0,
- NumMessages: 0,
- NumExtensions: 0,
- NumServices: 0,
- },
- GoTypes: file_github_com_golang_protobuf_ptypes_any_any_proto_goTypes,
- DependencyIndexes: file_github_com_golang_protobuf_ptypes_any_any_proto_depIdxs,
- }.Build()
- File_github_com_golang_protobuf_ptypes_any_any_proto = out.File
- file_github_com_golang_protobuf_ptypes_any_any_proto_rawDesc = nil
- file_github_com_golang_protobuf_ptypes_any_any_proto_goTypes = nil
- file_github_com_golang_protobuf_ptypes_any_any_proto_depIdxs = nil
-}
diff --git a/vendor/github.com/golang/protobuf/ptypes/doc.go b/vendor/github.com/golang/protobuf/ptypes/doc.go
deleted file mode 100644
index d3c33259..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/doc.go
+++ /dev/null
@@ -1,10 +0,0 @@
-// Copyright 2016 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package ptypes provides functionality for interacting with well-known types.
-//
-// Deprecated: Well-known types have specialized functionality directly
-// injected into the generated packages for each message type.
-// See the deprecation notice for each function for the suggested alternative.
-package ptypes
diff --git a/vendor/github.com/golang/protobuf/ptypes/duration.go b/vendor/github.com/golang/protobuf/ptypes/duration.go
deleted file mode 100644
index b2b55dd8..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/duration.go
+++ /dev/null
@@ -1,76 +0,0 @@
-// Copyright 2016 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package ptypes
-
-import (
- "errors"
- "fmt"
- "time"
-
- durationpb "github.com/golang/protobuf/ptypes/duration"
-)
-
-// Range of google.protobuf.Duration as specified in duration.proto.
-// This is about 10,000 years in seconds.
-const (
- maxSeconds = int64(10000 * 365.25 * 24 * 60 * 60)
- minSeconds = -maxSeconds
-)
-
-// Duration converts a durationpb.Duration to a time.Duration.
-// Duration returns an error if dur is invalid or overflows a time.Duration.
-//
-// Deprecated: Call the dur.AsDuration and dur.CheckValid methods instead.
-func Duration(dur *durationpb.Duration) (time.Duration, error) {
- if err := validateDuration(dur); err != nil {
- return 0, err
- }
- d := time.Duration(dur.Seconds) * time.Second
- if int64(d/time.Second) != dur.Seconds {
- return 0, fmt.Errorf("duration: %v is out of range for time.Duration", dur)
- }
- if dur.Nanos != 0 {
- d += time.Duration(dur.Nanos) * time.Nanosecond
- if (d < 0) != (dur.Nanos < 0) {
- return 0, fmt.Errorf("duration: %v is out of range for time.Duration", dur)
- }
- }
- return d, nil
-}
-
-// DurationProto converts a time.Duration to a durationpb.Duration.
-//
-// Deprecated: Call the durationpb.New function instead.
-func DurationProto(d time.Duration) *durationpb.Duration {
- nanos := d.Nanoseconds()
- secs := nanos / 1e9
- nanos -= secs * 1e9
- return &durationpb.Duration{
- Seconds: int64(secs),
- Nanos: int32(nanos),
- }
-}
-
-// validateDuration determines whether the durationpb.Duration is valid
-// according to the definition in google/protobuf/duration.proto.
-// A valid durpb.Duration may still be too large to fit into a time.Duration
-// Note that the range of durationpb.Duration is about 10,000 years,
-// while the range of time.Duration is about 290 years.
-func validateDuration(dur *durationpb.Duration) error {
- if dur == nil {
- return errors.New("duration: nil Duration")
- }
- if dur.Seconds < minSeconds || dur.Seconds > maxSeconds {
- return fmt.Errorf("duration: %v: seconds out of range", dur)
- }
- if dur.Nanos <= -1e9 || dur.Nanos >= 1e9 {
- return fmt.Errorf("duration: %v: nanos out of range", dur)
- }
- // Seconds and Nanos must have the same sign, unless d.Nanos is zero.
- if (dur.Seconds < 0 && dur.Nanos > 0) || (dur.Seconds > 0 && dur.Nanos < 0) {
- return fmt.Errorf("duration: %v: seconds and nanos have different signs", dur)
- }
- return nil
-}
diff --git a/vendor/github.com/golang/protobuf/ptypes/duration/duration.pb.go b/vendor/github.com/golang/protobuf/ptypes/duration/duration.pb.go
deleted file mode 100644
index d0079ee3..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/duration/duration.pb.go
+++ /dev/null
@@ -1,63 +0,0 @@
-// Code generated by protoc-gen-go. DO NOT EDIT.
-// source: github.com/golang/protobuf/ptypes/duration/duration.proto
-
-package duration
-
-import (
- protoreflect "google.golang.org/protobuf/reflect/protoreflect"
- protoimpl "google.golang.org/protobuf/runtime/protoimpl"
- durationpb "google.golang.org/protobuf/types/known/durationpb"
- reflect "reflect"
-)
-
-// Symbols defined in public import of google/protobuf/duration.proto.
-
-type Duration = durationpb.Duration
-
-var File_github_com_golang_protobuf_ptypes_duration_duration_proto protoreflect.FileDescriptor
-
-var file_github_com_golang_protobuf_ptypes_duration_duration_proto_rawDesc = []byte{
- 0x0a, 0x39, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x67, 0x6f, 0x6c,
- 0x61, 0x6e, 0x67, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x70, 0x74, 0x79,
- 0x70, 0x65, 0x73, 0x2f, 0x64, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x2f, 0x64, 0x75, 0x72,
- 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x1e, 0x67, 0x6f, 0x6f,
- 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x64, 0x75, 0x72,
- 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x42, 0x35, 0x5a, 0x33, 0x67,
- 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x67, 0x6f, 0x6c, 0x61, 0x6e, 0x67,
- 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x70, 0x74, 0x79, 0x70, 0x65, 0x73,
- 0x2f, 0x64, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x3b, 0x64, 0x75, 0x72, 0x61, 0x74, 0x69,
- 0x6f, 0x6e, 0x50, 0x00, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
-}
-
-var file_github_com_golang_protobuf_ptypes_duration_duration_proto_goTypes = []interface{}{}
-var file_github_com_golang_protobuf_ptypes_duration_duration_proto_depIdxs = []int32{
- 0, // [0:0] is the sub-list for method output_type
- 0, // [0:0] is the sub-list for method input_type
- 0, // [0:0] is the sub-list for extension type_name
- 0, // [0:0] is the sub-list for extension extendee
- 0, // [0:0] is the sub-list for field type_name
-}
-
-func init() { file_github_com_golang_protobuf_ptypes_duration_duration_proto_init() }
-func file_github_com_golang_protobuf_ptypes_duration_duration_proto_init() {
- if File_github_com_golang_protobuf_ptypes_duration_duration_proto != nil {
- return
- }
- type x struct{}
- out := protoimpl.TypeBuilder{
- File: protoimpl.DescBuilder{
- GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
- RawDescriptor: file_github_com_golang_protobuf_ptypes_duration_duration_proto_rawDesc,
- NumEnums: 0,
- NumMessages: 0,
- NumExtensions: 0,
- NumServices: 0,
- },
- GoTypes: file_github_com_golang_protobuf_ptypes_duration_duration_proto_goTypes,
- DependencyIndexes: file_github_com_golang_protobuf_ptypes_duration_duration_proto_depIdxs,
- }.Build()
- File_github_com_golang_protobuf_ptypes_duration_duration_proto = out.File
- file_github_com_golang_protobuf_ptypes_duration_duration_proto_rawDesc = nil
- file_github_com_golang_protobuf_ptypes_duration_duration_proto_goTypes = nil
- file_github_com_golang_protobuf_ptypes_duration_duration_proto_depIdxs = nil
-}
diff --git a/vendor/github.com/golang/protobuf/ptypes/timestamp.go b/vendor/github.com/golang/protobuf/ptypes/timestamp.go
deleted file mode 100644
index 8368a3f7..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/timestamp.go
+++ /dev/null
@@ -1,112 +0,0 @@
-// Copyright 2016 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package ptypes
-
-import (
- "errors"
- "fmt"
- "time"
-
- timestamppb "github.com/golang/protobuf/ptypes/timestamp"
-)
-
-// Range of google.protobuf.Duration as specified in timestamp.proto.
-const (
- // Seconds field of the earliest valid Timestamp.
- // This is time.Date(1, 1, 1, 0, 0, 0, 0, time.UTC).Unix().
- minValidSeconds = -62135596800
- // Seconds field just after the latest valid Timestamp.
- // This is time.Date(10000, 1, 1, 0, 0, 0, 0, time.UTC).Unix().
- maxValidSeconds = 253402300800
-)
-
-// Timestamp converts a timestamppb.Timestamp to a time.Time.
-// It returns an error if the argument is invalid.
-//
-// Unlike most Go functions, if Timestamp returns an error, the first return
-// value is not the zero time.Time. Instead, it is the value obtained from the
-// time.Unix function when passed the contents of the Timestamp, in the UTC
-// locale. This may or may not be a meaningful time; many invalid Timestamps
-// do map to valid time.Times.
-//
-// A nil Timestamp returns an error. The first return value in that case is
-// undefined.
-//
-// Deprecated: Call the ts.AsTime and ts.CheckValid methods instead.
-func Timestamp(ts *timestamppb.Timestamp) (time.Time, error) {
- // Don't return the zero value on error, because corresponds to a valid
- // timestamp. Instead return whatever time.Unix gives us.
- var t time.Time
- if ts == nil {
- t = time.Unix(0, 0).UTC() // treat nil like the empty Timestamp
- } else {
- t = time.Unix(ts.Seconds, int64(ts.Nanos)).UTC()
- }
- return t, validateTimestamp(ts)
-}
-
-// TimestampNow returns a google.protobuf.Timestamp for the current time.
-//
-// Deprecated: Call the timestamppb.Now function instead.
-func TimestampNow() *timestamppb.Timestamp {
- ts, err := TimestampProto(time.Now())
- if err != nil {
- panic("ptypes: time.Now() out of Timestamp range")
- }
- return ts
-}
-
-// TimestampProto converts the time.Time to a google.protobuf.Timestamp proto.
-// It returns an error if the resulting Timestamp is invalid.
-//
-// Deprecated: Call the timestamppb.New function instead.
-func TimestampProto(t time.Time) (*timestamppb.Timestamp, error) {
- ts := ×tamppb.Timestamp{
- Seconds: t.Unix(),
- Nanos: int32(t.Nanosecond()),
- }
- if err := validateTimestamp(ts); err != nil {
- return nil, err
- }
- return ts, nil
-}
-
-// TimestampString returns the RFC 3339 string for valid Timestamps.
-// For invalid Timestamps, it returns an error message in parentheses.
-//
-// Deprecated: Call the ts.AsTime method instead,
-// followed by a call to the Format method on the time.Time value.
-func TimestampString(ts *timestamppb.Timestamp) string {
- t, err := Timestamp(ts)
- if err != nil {
- return fmt.Sprintf("(%v)", err)
- }
- return t.Format(time.RFC3339Nano)
-}
-
-// validateTimestamp determines whether a Timestamp is valid.
-// A valid timestamp represents a time in the range [0001-01-01, 10000-01-01)
-// and has a Nanos field in the range [0, 1e9).
-//
-// If the Timestamp is valid, validateTimestamp returns nil.
-// Otherwise, it returns an error that describes the problem.
-//
-// Every valid Timestamp can be represented by a time.Time,
-// but the converse is not true.
-func validateTimestamp(ts *timestamppb.Timestamp) error {
- if ts == nil {
- return errors.New("timestamp: nil Timestamp")
- }
- if ts.Seconds < minValidSeconds {
- return fmt.Errorf("timestamp: %v before 0001-01-01", ts)
- }
- if ts.Seconds >= maxValidSeconds {
- return fmt.Errorf("timestamp: %v after 10000-01-01", ts)
- }
- if ts.Nanos < 0 || ts.Nanos >= 1e9 {
- return fmt.Errorf("timestamp: %v: nanos not in range [0, 1e9)", ts)
- }
- return nil
-}
diff --git a/vendor/github.com/golang/protobuf/ptypes/timestamp/timestamp.pb.go b/vendor/github.com/golang/protobuf/ptypes/timestamp/timestamp.pb.go
deleted file mode 100644
index a76f8076..00000000
--- a/vendor/github.com/golang/protobuf/ptypes/timestamp/timestamp.pb.go
+++ /dev/null
@@ -1,64 +0,0 @@
-// Code generated by protoc-gen-go. DO NOT EDIT.
-// source: github.com/golang/protobuf/ptypes/timestamp/timestamp.proto
-
-package timestamp
-
-import (
- protoreflect "google.golang.org/protobuf/reflect/protoreflect"
- protoimpl "google.golang.org/protobuf/runtime/protoimpl"
- timestamppb "google.golang.org/protobuf/types/known/timestamppb"
- reflect "reflect"
-)
-
-// Symbols defined in public import of google/protobuf/timestamp.proto.
-
-type Timestamp = timestamppb.Timestamp
-
-var File_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto protoreflect.FileDescriptor
-
-var file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_rawDesc = []byte{
- 0x0a, 0x3b, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x67, 0x6f, 0x6c,
- 0x61, 0x6e, 0x67, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x70, 0x74, 0x79,
- 0x70, 0x65, 0x73, 0x2f, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x2f, 0x74, 0x69,
- 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x1f, 0x67,
- 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x74,
- 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x42, 0x37,
- 0x5a, 0x35, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x67, 0x6f, 0x6c,
- 0x61, 0x6e, 0x67, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x70, 0x74, 0x79,
- 0x70, 0x65, 0x73, 0x2f, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x3b, 0x74, 0x69,
- 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x50, 0x00, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f,
- 0x33,
-}
-
-var file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_goTypes = []interface{}{}
-var file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_depIdxs = []int32{
- 0, // [0:0] is the sub-list for method output_type
- 0, // [0:0] is the sub-list for method input_type
- 0, // [0:0] is the sub-list for extension type_name
- 0, // [0:0] is the sub-list for extension extendee
- 0, // [0:0] is the sub-list for field type_name
-}
-
-func init() { file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_init() }
-func file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_init() {
- if File_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto != nil {
- return
- }
- type x struct{}
- out := protoimpl.TypeBuilder{
- File: protoimpl.DescBuilder{
- GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
- RawDescriptor: file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_rawDesc,
- NumEnums: 0,
- NumMessages: 0,
- NumExtensions: 0,
- NumServices: 0,
- },
- GoTypes: file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_goTypes,
- DependencyIndexes: file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_depIdxs,
- }.Build()
- File_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto = out.File
- file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_rawDesc = nil
- file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_goTypes = nil
- file_github_com_golang_protobuf_ptypes_timestamp_timestamp_proto_depIdxs = nil
-}
diff --git a/vendor/github.com/gorilla/websocket/.gitignore b/vendor/github.com/gorilla/websocket/.gitignore
deleted file mode 100644
index cd3fcd1e..00000000
--- a/vendor/github.com/gorilla/websocket/.gitignore
+++ /dev/null
@@ -1,25 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-
-.idea/
-*.iml
diff --git a/vendor/github.com/gorilla/websocket/AUTHORS b/vendor/github.com/gorilla/websocket/AUTHORS
deleted file mode 100644
index 1931f400..00000000
--- a/vendor/github.com/gorilla/websocket/AUTHORS
+++ /dev/null
@@ -1,9 +0,0 @@
-# This is the official list of Gorilla WebSocket authors for copyright
-# purposes.
-#
-# Please keep the list sorted.
-
-Gary Burd
-Google LLC (https://opensource.google.com/)
-Joachim Bauch
-
diff --git a/vendor/github.com/gorilla/websocket/LICENSE b/vendor/github.com/gorilla/websocket/LICENSE
deleted file mode 100644
index 9171c972..00000000
--- a/vendor/github.com/gorilla/websocket/LICENSE
+++ /dev/null
@@ -1,22 +0,0 @@
-Copyright (c) 2013 The Gorilla WebSocket Authors. All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are met:
-
- Redistributions of source code must retain the above copyright notice, this
- list of conditions and the following disclaimer.
-
- Redistributions in binary form must reproduce the above copyright notice,
- this list of conditions and the following disclaimer in the documentation
- and/or other materials provided with the distribution.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
-ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
-WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/gorilla/websocket/README.md b/vendor/github.com/gorilla/websocket/README.md
deleted file mode 100644
index 2517a287..00000000
--- a/vendor/github.com/gorilla/websocket/README.md
+++ /dev/null
@@ -1,39 +0,0 @@
-# Gorilla WebSocket
-
-[](https://godoc.org/github.com/gorilla/websocket)
-[](https://circleci.com/gh/gorilla/websocket)
-
-Gorilla WebSocket is a [Go](http://golang.org/) implementation of the
-[WebSocket](http://www.rfc-editor.org/rfc/rfc6455.txt) protocol.
-
-
----
-
-âš ï¸ **[The Gorilla WebSocket Package is looking for a new maintainer](https://github.com/gorilla/websocket/issues/370)**
-
----
-
-### Documentation
-
-* [API Reference](https://pkg.go.dev/github.com/gorilla/websocket?tab=doc)
-* [Chat example](https://github.com/gorilla/websocket/tree/master/examples/chat)
-* [Command example](https://github.com/gorilla/websocket/tree/master/examples/command)
-* [Client and server example](https://github.com/gorilla/websocket/tree/master/examples/echo)
-* [File watch example](https://github.com/gorilla/websocket/tree/master/examples/filewatch)
-
-### Status
-
-The Gorilla WebSocket package provides a complete and tested implementation of
-the [WebSocket](http://www.rfc-editor.org/rfc/rfc6455.txt) protocol. The
-package API is stable.
-
-### Installation
-
- go get github.com/gorilla/websocket
-
-### Protocol Compliance
-
-The Gorilla WebSocket package passes the server tests in the [Autobahn Test
-Suite](https://github.com/crossbario/autobahn-testsuite) using the application in the [examples/autobahn
-subdirectory](https://github.com/gorilla/websocket/tree/master/examples/autobahn).
-
diff --git a/vendor/github.com/gorilla/websocket/client.go b/vendor/github.com/gorilla/websocket/client.go
deleted file mode 100644
index 2efd8355..00000000
--- a/vendor/github.com/gorilla/websocket/client.go
+++ /dev/null
@@ -1,422 +0,0 @@
-// Copyright 2013 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "bytes"
- "context"
- "crypto/tls"
- "errors"
- "io"
- "io/ioutil"
- "net"
- "net/http"
- "net/http/httptrace"
- "net/url"
- "strings"
- "time"
-)
-
-// ErrBadHandshake is returned when the server response to opening handshake is
-// invalid.
-var ErrBadHandshake = errors.New("websocket: bad handshake")
-
-var errInvalidCompression = errors.New("websocket: invalid compression negotiation")
-
-// NewClient creates a new client connection using the given net connection.
-// The URL u specifies the host and request URI. Use requestHeader to specify
-// the origin (Origin), subprotocols (Sec-WebSocket-Protocol) and cookies
-// (Cookie). Use the response.Header to get the selected subprotocol
-// (Sec-WebSocket-Protocol) and cookies (Set-Cookie).
-//
-// If the WebSocket handshake fails, ErrBadHandshake is returned along with a
-// non-nil *http.Response so that callers can handle redirects, authentication,
-// etc.
-//
-// Deprecated: Use Dialer instead.
-func NewClient(netConn net.Conn, u *url.URL, requestHeader http.Header, readBufSize, writeBufSize int) (c *Conn, response *http.Response, err error) {
- d := Dialer{
- ReadBufferSize: readBufSize,
- WriteBufferSize: writeBufSize,
- NetDial: func(net, addr string) (net.Conn, error) {
- return netConn, nil
- },
- }
- return d.Dial(u.String(), requestHeader)
-}
-
-// A Dialer contains options for connecting to WebSocket server.
-//
-// It is safe to call Dialer's methods concurrently.
-type Dialer struct {
- // NetDial specifies the dial function for creating TCP connections. If
- // NetDial is nil, net.Dial is used.
- NetDial func(network, addr string) (net.Conn, error)
-
- // NetDialContext specifies the dial function for creating TCP connections. If
- // NetDialContext is nil, NetDial is used.
- NetDialContext func(ctx context.Context, network, addr string) (net.Conn, error)
-
- // NetDialTLSContext specifies the dial function for creating TLS/TCP connections. If
- // NetDialTLSContext is nil, NetDialContext is used.
- // If NetDialTLSContext is set, Dial assumes the TLS handshake is done there and
- // TLSClientConfig is ignored.
- NetDialTLSContext func(ctx context.Context, network, addr string) (net.Conn, error)
-
- // Proxy specifies a function to return a proxy for a given
- // Request. If the function returns a non-nil error, the
- // request is aborted with the provided error.
- // If Proxy is nil or returns a nil *URL, no proxy is used.
- Proxy func(*http.Request) (*url.URL, error)
-
- // TLSClientConfig specifies the TLS configuration to use with tls.Client.
- // If nil, the default configuration is used.
- // If either NetDialTLS or NetDialTLSContext are set, Dial assumes the TLS handshake
- // is done there and TLSClientConfig is ignored.
- TLSClientConfig *tls.Config
-
- // HandshakeTimeout specifies the duration for the handshake to complete.
- HandshakeTimeout time.Duration
-
- // ReadBufferSize and WriteBufferSize specify I/O buffer sizes in bytes. If a buffer
- // size is zero, then a useful default size is used. The I/O buffer sizes
- // do not limit the size of the messages that can be sent or received.
- ReadBufferSize, WriteBufferSize int
-
- // WriteBufferPool is a pool of buffers for write operations. If the value
- // is not set, then write buffers are allocated to the connection for the
- // lifetime of the connection.
- //
- // A pool is most useful when the application has a modest volume of writes
- // across a large number of connections.
- //
- // Applications should use a single pool for each unique value of
- // WriteBufferSize.
- WriteBufferPool BufferPool
-
- // Subprotocols specifies the client's requested subprotocols.
- Subprotocols []string
-
- // EnableCompression specifies if the client should attempt to negotiate
- // per message compression (RFC 7692). Setting this value to true does not
- // guarantee that compression will be supported. Currently only "no context
- // takeover" modes are supported.
- EnableCompression bool
-
- // Jar specifies the cookie jar.
- // If Jar is nil, cookies are not sent in requests and ignored
- // in responses.
- Jar http.CookieJar
-}
-
-// Dial creates a new client connection by calling DialContext with a background context.
-func (d *Dialer) Dial(urlStr string, requestHeader http.Header) (*Conn, *http.Response, error) {
- return d.DialContext(context.Background(), urlStr, requestHeader)
-}
-
-var errMalformedURL = errors.New("malformed ws or wss URL")
-
-func hostPortNoPort(u *url.URL) (hostPort, hostNoPort string) {
- hostPort = u.Host
- hostNoPort = u.Host
- if i := strings.LastIndex(u.Host, ":"); i > strings.LastIndex(u.Host, "]") {
- hostNoPort = hostNoPort[:i]
- } else {
- switch u.Scheme {
- case "wss":
- hostPort += ":443"
- case "https":
- hostPort += ":443"
- default:
- hostPort += ":80"
- }
- }
- return hostPort, hostNoPort
-}
-
-// DefaultDialer is a dialer with all fields set to the default values.
-var DefaultDialer = &Dialer{
- Proxy: http.ProxyFromEnvironment,
- HandshakeTimeout: 45 * time.Second,
-}
-
-// nilDialer is dialer to use when receiver is nil.
-var nilDialer = *DefaultDialer
-
-// DialContext creates a new client connection. Use requestHeader to specify the
-// origin (Origin), subprotocols (Sec-WebSocket-Protocol) and cookies (Cookie).
-// Use the response.Header to get the selected subprotocol
-// (Sec-WebSocket-Protocol) and cookies (Set-Cookie).
-//
-// The context will be used in the request and in the Dialer.
-//
-// If the WebSocket handshake fails, ErrBadHandshake is returned along with a
-// non-nil *http.Response so that callers can handle redirects, authentication,
-// etcetera. The response body may not contain the entire response and does not
-// need to be closed by the application.
-func (d *Dialer) DialContext(ctx context.Context, urlStr string, requestHeader http.Header) (*Conn, *http.Response, error) {
- if d == nil {
- d = &nilDialer
- }
-
- challengeKey, err := generateChallengeKey()
- if err != nil {
- return nil, nil, err
- }
-
- u, err := url.Parse(urlStr)
- if err != nil {
- return nil, nil, err
- }
-
- switch u.Scheme {
- case "ws":
- u.Scheme = "http"
- case "wss":
- u.Scheme = "https"
- default:
- return nil, nil, errMalformedURL
- }
-
- if u.User != nil {
- // User name and password are not allowed in websocket URIs.
- return nil, nil, errMalformedURL
- }
-
- req := &http.Request{
- Method: http.MethodGet,
- URL: u,
- Proto: "HTTP/1.1",
- ProtoMajor: 1,
- ProtoMinor: 1,
- Header: make(http.Header),
- Host: u.Host,
- }
- req = req.WithContext(ctx)
-
- // Set the cookies present in the cookie jar of the dialer
- if d.Jar != nil {
- for _, cookie := range d.Jar.Cookies(u) {
- req.AddCookie(cookie)
- }
- }
-
- // Set the request headers using the capitalization for names and values in
- // RFC examples. Although the capitalization shouldn't matter, there are
- // servers that depend on it. The Header.Set method is not used because the
- // method canonicalizes the header names.
- req.Header["Upgrade"] = []string{"websocket"}
- req.Header["Connection"] = []string{"Upgrade"}
- req.Header["Sec-WebSocket-Key"] = []string{challengeKey}
- req.Header["Sec-WebSocket-Version"] = []string{"13"}
- if len(d.Subprotocols) > 0 {
- req.Header["Sec-WebSocket-Protocol"] = []string{strings.Join(d.Subprotocols, ", ")}
- }
- for k, vs := range requestHeader {
- switch {
- case k == "Host":
- if len(vs) > 0 {
- req.Host = vs[0]
- }
- case k == "Upgrade" ||
- k == "Connection" ||
- k == "Sec-Websocket-Key" ||
- k == "Sec-Websocket-Version" ||
- k == "Sec-Websocket-Extensions" ||
- (k == "Sec-Websocket-Protocol" && len(d.Subprotocols) > 0):
- return nil, nil, errors.New("websocket: duplicate header not allowed: " + k)
- case k == "Sec-Websocket-Protocol":
- req.Header["Sec-WebSocket-Protocol"] = vs
- default:
- req.Header[k] = vs
- }
- }
-
- if d.EnableCompression {
- req.Header["Sec-WebSocket-Extensions"] = []string{"permessage-deflate; server_no_context_takeover; client_no_context_takeover"}
- }
-
- if d.HandshakeTimeout != 0 {
- var cancel func()
- ctx, cancel = context.WithTimeout(ctx, d.HandshakeTimeout)
- defer cancel()
- }
-
- // Get network dial function.
- var netDial func(network, add string) (net.Conn, error)
-
- switch u.Scheme {
- case "http":
- if d.NetDialContext != nil {
- netDial = func(network, addr string) (net.Conn, error) {
- return d.NetDialContext(ctx, network, addr)
- }
- } else if d.NetDial != nil {
- netDial = d.NetDial
- }
- case "https":
- if d.NetDialTLSContext != nil {
- netDial = func(network, addr string) (net.Conn, error) {
- return d.NetDialTLSContext(ctx, network, addr)
- }
- } else if d.NetDialContext != nil {
- netDial = func(network, addr string) (net.Conn, error) {
- return d.NetDialContext(ctx, network, addr)
- }
- } else if d.NetDial != nil {
- netDial = d.NetDial
- }
- default:
- return nil, nil, errMalformedURL
- }
-
- if netDial == nil {
- netDialer := &net.Dialer{}
- netDial = func(network, addr string) (net.Conn, error) {
- return netDialer.DialContext(ctx, network, addr)
- }
- }
-
- // If needed, wrap the dial function to set the connection deadline.
- if deadline, ok := ctx.Deadline(); ok {
- forwardDial := netDial
- netDial = func(network, addr string) (net.Conn, error) {
- c, err := forwardDial(network, addr)
- if err != nil {
- return nil, err
- }
- err = c.SetDeadline(deadline)
- if err != nil {
- c.Close()
- return nil, err
- }
- return c, nil
- }
- }
-
- // If needed, wrap the dial function to connect through a proxy.
- if d.Proxy != nil {
- proxyURL, err := d.Proxy(req)
- if err != nil {
- return nil, nil, err
- }
- if proxyURL != nil {
- dialer, err := proxy_FromURL(proxyURL, netDialerFunc(netDial))
- if err != nil {
- return nil, nil, err
- }
- netDial = dialer.Dial
- }
- }
-
- hostPort, hostNoPort := hostPortNoPort(u)
- trace := httptrace.ContextClientTrace(ctx)
- if trace != nil && trace.GetConn != nil {
- trace.GetConn(hostPort)
- }
-
- netConn, err := netDial("tcp", hostPort)
- if trace != nil && trace.GotConn != nil {
- trace.GotConn(httptrace.GotConnInfo{
- Conn: netConn,
- })
- }
- if err != nil {
- return nil, nil, err
- }
-
- defer func() {
- if netConn != nil {
- netConn.Close()
- }
- }()
-
- if u.Scheme == "https" && d.NetDialTLSContext == nil {
- // If NetDialTLSContext is set, assume that the TLS handshake has already been done
-
- cfg := cloneTLSConfig(d.TLSClientConfig)
- if cfg.ServerName == "" {
- cfg.ServerName = hostNoPort
- }
- tlsConn := tls.Client(netConn, cfg)
- netConn = tlsConn
-
- if trace != nil && trace.TLSHandshakeStart != nil {
- trace.TLSHandshakeStart()
- }
- err := doHandshake(ctx, tlsConn, cfg)
- if trace != nil && trace.TLSHandshakeDone != nil {
- trace.TLSHandshakeDone(tlsConn.ConnectionState(), err)
- }
-
- if err != nil {
- return nil, nil, err
- }
- }
-
- conn := newConn(netConn, false, d.ReadBufferSize, d.WriteBufferSize, d.WriteBufferPool, nil, nil)
-
- if err := req.Write(netConn); err != nil {
- return nil, nil, err
- }
-
- if trace != nil && trace.GotFirstResponseByte != nil {
- if peek, err := conn.br.Peek(1); err == nil && len(peek) == 1 {
- trace.GotFirstResponseByte()
- }
- }
-
- resp, err := http.ReadResponse(conn.br, req)
- if err != nil {
- return nil, nil, err
- }
-
- if d.Jar != nil {
- if rc := resp.Cookies(); len(rc) > 0 {
- d.Jar.SetCookies(u, rc)
- }
- }
-
- if resp.StatusCode != 101 ||
- !tokenListContainsValue(resp.Header, "Upgrade", "websocket") ||
- !tokenListContainsValue(resp.Header, "Connection", "upgrade") ||
- resp.Header.Get("Sec-Websocket-Accept") != computeAcceptKey(challengeKey) {
- // Before closing the network connection on return from this
- // function, slurp up some of the response to aid application
- // debugging.
- buf := make([]byte, 1024)
- n, _ := io.ReadFull(resp.Body, buf)
- resp.Body = ioutil.NopCloser(bytes.NewReader(buf[:n]))
- return nil, resp, ErrBadHandshake
- }
-
- for _, ext := range parseExtensions(resp.Header) {
- if ext[""] != "permessage-deflate" {
- continue
- }
- _, snct := ext["server_no_context_takeover"]
- _, cnct := ext["client_no_context_takeover"]
- if !snct || !cnct {
- return nil, resp, errInvalidCompression
- }
- conn.newCompressionWriter = compressNoContextTakeover
- conn.newDecompressionReader = decompressNoContextTakeover
- break
- }
-
- resp.Body = ioutil.NopCloser(bytes.NewReader([]byte{}))
- conn.subprotocol = resp.Header.Get("Sec-Websocket-Protocol")
-
- netConn.SetDeadline(time.Time{})
- netConn = nil // to avoid close in defer.
- return conn, resp, nil
-}
-
-func cloneTLSConfig(cfg *tls.Config) *tls.Config {
- if cfg == nil {
- return &tls.Config{}
- }
- return cfg.Clone()
-}
diff --git a/vendor/github.com/gorilla/websocket/compression.go b/vendor/github.com/gorilla/websocket/compression.go
deleted file mode 100644
index 813ffb1e..00000000
--- a/vendor/github.com/gorilla/websocket/compression.go
+++ /dev/null
@@ -1,148 +0,0 @@
-// Copyright 2017 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "compress/flate"
- "errors"
- "io"
- "strings"
- "sync"
-)
-
-const (
- minCompressionLevel = -2 // flate.HuffmanOnly not defined in Go < 1.6
- maxCompressionLevel = flate.BestCompression
- defaultCompressionLevel = 1
-)
-
-var (
- flateWriterPools [maxCompressionLevel - minCompressionLevel + 1]sync.Pool
- flateReaderPool = sync.Pool{New: func() interface{} {
- return flate.NewReader(nil)
- }}
-)
-
-func decompressNoContextTakeover(r io.Reader) io.ReadCloser {
- const tail =
- // Add four bytes as specified in RFC
- "\x00\x00\xff\xff" +
- // Add final block to squelch unexpected EOF error from flate reader.
- "\x01\x00\x00\xff\xff"
-
- fr, _ := flateReaderPool.Get().(io.ReadCloser)
- fr.(flate.Resetter).Reset(io.MultiReader(r, strings.NewReader(tail)), nil)
- return &flateReadWrapper{fr}
-}
-
-func isValidCompressionLevel(level int) bool {
- return minCompressionLevel <= level && level <= maxCompressionLevel
-}
-
-func compressNoContextTakeover(w io.WriteCloser, level int) io.WriteCloser {
- p := &flateWriterPools[level-minCompressionLevel]
- tw := &truncWriter{w: w}
- fw, _ := p.Get().(*flate.Writer)
- if fw == nil {
- fw, _ = flate.NewWriter(tw, level)
- } else {
- fw.Reset(tw)
- }
- return &flateWriteWrapper{fw: fw, tw: tw, p: p}
-}
-
-// truncWriter is an io.Writer that writes all but the last four bytes of the
-// stream to another io.Writer.
-type truncWriter struct {
- w io.WriteCloser
- n int
- p [4]byte
-}
-
-func (w *truncWriter) Write(p []byte) (int, error) {
- n := 0
-
- // fill buffer first for simplicity.
- if w.n < len(w.p) {
- n = copy(w.p[w.n:], p)
- p = p[n:]
- w.n += n
- if len(p) == 0 {
- return n, nil
- }
- }
-
- m := len(p)
- if m > len(w.p) {
- m = len(w.p)
- }
-
- if nn, err := w.w.Write(w.p[:m]); err != nil {
- return n + nn, err
- }
-
- copy(w.p[:], w.p[m:])
- copy(w.p[len(w.p)-m:], p[len(p)-m:])
- nn, err := w.w.Write(p[:len(p)-m])
- return n + nn, err
-}
-
-type flateWriteWrapper struct {
- fw *flate.Writer
- tw *truncWriter
- p *sync.Pool
-}
-
-func (w *flateWriteWrapper) Write(p []byte) (int, error) {
- if w.fw == nil {
- return 0, errWriteClosed
- }
- return w.fw.Write(p)
-}
-
-func (w *flateWriteWrapper) Close() error {
- if w.fw == nil {
- return errWriteClosed
- }
- err1 := w.fw.Flush()
- w.p.Put(w.fw)
- w.fw = nil
- if w.tw.p != [4]byte{0, 0, 0xff, 0xff} {
- return errors.New("websocket: internal error, unexpected bytes at end of flate stream")
- }
- err2 := w.tw.w.Close()
- if err1 != nil {
- return err1
- }
- return err2
-}
-
-type flateReadWrapper struct {
- fr io.ReadCloser
-}
-
-func (r *flateReadWrapper) Read(p []byte) (int, error) {
- if r.fr == nil {
- return 0, io.ErrClosedPipe
- }
- n, err := r.fr.Read(p)
- if err == io.EOF {
- // Preemptively place the reader back in the pool. This helps with
- // scenarios where the application does not call NextReader() soon after
- // this final read.
- r.Close()
- }
- return n, err
-}
-
-func (r *flateReadWrapper) Close() error {
- if r.fr == nil {
- return io.ErrClosedPipe
- }
- err := r.fr.Close()
- flateReaderPool.Put(r.fr)
- r.fr = nil
- return err
-}
diff --git a/vendor/github.com/gorilla/websocket/conn.go b/vendor/github.com/gorilla/websocket/conn.go
deleted file mode 100644
index 331eebc8..00000000
--- a/vendor/github.com/gorilla/websocket/conn.go
+++ /dev/null
@@ -1,1230 +0,0 @@
-// Copyright 2013 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "bufio"
- "encoding/binary"
- "errors"
- "io"
- "io/ioutil"
- "math/rand"
- "net"
- "strconv"
- "strings"
- "sync"
- "time"
- "unicode/utf8"
-)
-
-const (
- // Frame header byte 0 bits from Section 5.2 of RFC 6455
- finalBit = 1 << 7
- rsv1Bit = 1 << 6
- rsv2Bit = 1 << 5
- rsv3Bit = 1 << 4
-
- // Frame header byte 1 bits from Section 5.2 of RFC 6455
- maskBit = 1 << 7
-
- maxFrameHeaderSize = 2 + 8 + 4 // Fixed header + length + mask
- maxControlFramePayloadSize = 125
-
- writeWait = time.Second
-
- defaultReadBufferSize = 4096
- defaultWriteBufferSize = 4096
-
- continuationFrame = 0
- noFrame = -1
-)
-
-// Close codes defined in RFC 6455, section 11.7.
-const (
- CloseNormalClosure = 1000
- CloseGoingAway = 1001
- CloseProtocolError = 1002
- CloseUnsupportedData = 1003
- CloseNoStatusReceived = 1005
- CloseAbnormalClosure = 1006
- CloseInvalidFramePayloadData = 1007
- ClosePolicyViolation = 1008
- CloseMessageTooBig = 1009
- CloseMandatoryExtension = 1010
- CloseInternalServerErr = 1011
- CloseServiceRestart = 1012
- CloseTryAgainLater = 1013
- CloseTLSHandshake = 1015
-)
-
-// The message types are defined in RFC 6455, section 11.8.
-const (
- // TextMessage denotes a text data message. The text message payload is
- // interpreted as UTF-8 encoded text data.
- TextMessage = 1
-
- // BinaryMessage denotes a binary data message.
- BinaryMessage = 2
-
- // CloseMessage denotes a close control message. The optional message
- // payload contains a numeric code and text. Use the FormatCloseMessage
- // function to format a close message payload.
- CloseMessage = 8
-
- // PingMessage denotes a ping control message. The optional message payload
- // is UTF-8 encoded text.
- PingMessage = 9
-
- // PongMessage denotes a pong control message. The optional message payload
- // is UTF-8 encoded text.
- PongMessage = 10
-)
-
-// ErrCloseSent is returned when the application writes a message to the
-// connection after sending a close message.
-var ErrCloseSent = errors.New("websocket: close sent")
-
-// ErrReadLimit is returned when reading a message that is larger than the
-// read limit set for the connection.
-var ErrReadLimit = errors.New("websocket: read limit exceeded")
-
-// netError satisfies the net Error interface.
-type netError struct {
- msg string
- temporary bool
- timeout bool
-}
-
-func (e *netError) Error() string { return e.msg }
-func (e *netError) Temporary() bool { return e.temporary }
-func (e *netError) Timeout() bool { return e.timeout }
-
-// CloseError represents a close message.
-type CloseError struct {
- // Code is defined in RFC 6455, section 11.7.
- Code int
-
- // Text is the optional text payload.
- Text string
-}
-
-func (e *CloseError) Error() string {
- s := []byte("websocket: close ")
- s = strconv.AppendInt(s, int64(e.Code), 10)
- switch e.Code {
- case CloseNormalClosure:
- s = append(s, " (normal)"...)
- case CloseGoingAway:
- s = append(s, " (going away)"...)
- case CloseProtocolError:
- s = append(s, " (protocol error)"...)
- case CloseUnsupportedData:
- s = append(s, " (unsupported data)"...)
- case CloseNoStatusReceived:
- s = append(s, " (no status)"...)
- case CloseAbnormalClosure:
- s = append(s, " (abnormal closure)"...)
- case CloseInvalidFramePayloadData:
- s = append(s, " (invalid payload data)"...)
- case ClosePolicyViolation:
- s = append(s, " (policy violation)"...)
- case CloseMessageTooBig:
- s = append(s, " (message too big)"...)
- case CloseMandatoryExtension:
- s = append(s, " (mandatory extension missing)"...)
- case CloseInternalServerErr:
- s = append(s, " (internal server error)"...)
- case CloseTLSHandshake:
- s = append(s, " (TLS handshake error)"...)
- }
- if e.Text != "" {
- s = append(s, ": "...)
- s = append(s, e.Text...)
- }
- return string(s)
-}
-
-// IsCloseError returns boolean indicating whether the error is a *CloseError
-// with one of the specified codes.
-func IsCloseError(err error, codes ...int) bool {
- if e, ok := err.(*CloseError); ok {
- for _, code := range codes {
- if e.Code == code {
- return true
- }
- }
- }
- return false
-}
-
-// IsUnexpectedCloseError returns boolean indicating whether the error is a
-// *CloseError with a code not in the list of expected codes.
-func IsUnexpectedCloseError(err error, expectedCodes ...int) bool {
- if e, ok := err.(*CloseError); ok {
- for _, code := range expectedCodes {
- if e.Code == code {
- return false
- }
- }
- return true
- }
- return false
-}
-
-var (
- errWriteTimeout = &netError{msg: "websocket: write timeout", timeout: true, temporary: true}
- errUnexpectedEOF = &CloseError{Code: CloseAbnormalClosure, Text: io.ErrUnexpectedEOF.Error()}
- errBadWriteOpCode = errors.New("websocket: bad write message type")
- errWriteClosed = errors.New("websocket: write closed")
- errInvalidControlFrame = errors.New("websocket: invalid control frame")
-)
-
-func newMaskKey() [4]byte {
- n := rand.Uint32()
- return [4]byte{byte(n), byte(n >> 8), byte(n >> 16), byte(n >> 24)}
-}
-
-func hideTempErr(err error) error {
- if e, ok := err.(net.Error); ok && e.Temporary() {
- err = &netError{msg: e.Error(), timeout: e.Timeout()}
- }
- return err
-}
-
-func isControl(frameType int) bool {
- return frameType == CloseMessage || frameType == PingMessage || frameType == PongMessage
-}
-
-func isData(frameType int) bool {
- return frameType == TextMessage || frameType == BinaryMessage
-}
-
-var validReceivedCloseCodes = map[int]bool{
- // see http://www.iana.org/assignments/websocket/websocket.xhtml#close-code-number
-
- CloseNormalClosure: true,
- CloseGoingAway: true,
- CloseProtocolError: true,
- CloseUnsupportedData: true,
- CloseNoStatusReceived: false,
- CloseAbnormalClosure: false,
- CloseInvalidFramePayloadData: true,
- ClosePolicyViolation: true,
- CloseMessageTooBig: true,
- CloseMandatoryExtension: true,
- CloseInternalServerErr: true,
- CloseServiceRestart: true,
- CloseTryAgainLater: true,
- CloseTLSHandshake: false,
-}
-
-func isValidReceivedCloseCode(code int) bool {
- return validReceivedCloseCodes[code] || (code >= 3000 && code <= 4999)
-}
-
-// BufferPool represents a pool of buffers. The *sync.Pool type satisfies this
-// interface. The type of the value stored in a pool is not specified.
-type BufferPool interface {
- // Get gets a value from the pool or returns nil if the pool is empty.
- Get() interface{}
- // Put adds a value to the pool.
- Put(interface{})
-}
-
-// writePoolData is the type added to the write buffer pool. This wrapper is
-// used to prevent applications from peeking at and depending on the values
-// added to the pool.
-type writePoolData struct{ buf []byte }
-
-// The Conn type represents a WebSocket connection.
-type Conn struct {
- conn net.Conn
- isServer bool
- subprotocol string
-
- // Write fields
- mu chan struct{} // used as mutex to protect write to conn
- writeBuf []byte // frame is constructed in this buffer.
- writePool BufferPool
- writeBufSize int
- writeDeadline time.Time
- writer io.WriteCloser // the current writer returned to the application
- isWriting bool // for best-effort concurrent write detection
-
- writeErrMu sync.Mutex
- writeErr error
-
- enableWriteCompression bool
- compressionLevel int
- newCompressionWriter func(io.WriteCloser, int) io.WriteCloser
-
- // Read fields
- reader io.ReadCloser // the current reader returned to the application
- readErr error
- br *bufio.Reader
- // bytes remaining in current frame.
- // set setReadRemaining to safely update this value and prevent overflow
- readRemaining int64
- readFinal bool // true the current message has more frames.
- readLength int64 // Message size.
- readLimit int64 // Maximum message size.
- readMaskPos int
- readMaskKey [4]byte
- handlePong func(string) error
- handlePing func(string) error
- handleClose func(int, string) error
- readErrCount int
- messageReader *messageReader // the current low-level reader
-
- readDecompress bool // whether last read frame had RSV1 set
- newDecompressionReader func(io.Reader) io.ReadCloser
-}
-
-func newConn(conn net.Conn, isServer bool, readBufferSize, writeBufferSize int, writeBufferPool BufferPool, br *bufio.Reader, writeBuf []byte) *Conn {
-
- if br == nil {
- if readBufferSize == 0 {
- readBufferSize = defaultReadBufferSize
- } else if readBufferSize < maxControlFramePayloadSize {
- // must be large enough for control frame
- readBufferSize = maxControlFramePayloadSize
- }
- br = bufio.NewReaderSize(conn, readBufferSize)
- }
-
- if writeBufferSize <= 0 {
- writeBufferSize = defaultWriteBufferSize
- }
- writeBufferSize += maxFrameHeaderSize
-
- if writeBuf == nil && writeBufferPool == nil {
- writeBuf = make([]byte, writeBufferSize)
- }
-
- mu := make(chan struct{}, 1)
- mu <- struct{}{}
- c := &Conn{
- isServer: isServer,
- br: br,
- conn: conn,
- mu: mu,
- readFinal: true,
- writeBuf: writeBuf,
- writePool: writeBufferPool,
- writeBufSize: writeBufferSize,
- enableWriteCompression: true,
- compressionLevel: defaultCompressionLevel,
- }
- c.SetCloseHandler(nil)
- c.SetPingHandler(nil)
- c.SetPongHandler(nil)
- return c
-}
-
-// setReadRemaining tracks the number of bytes remaining on the connection. If n
-// overflows, an ErrReadLimit is returned.
-func (c *Conn) setReadRemaining(n int64) error {
- if n < 0 {
- return ErrReadLimit
- }
-
- c.readRemaining = n
- return nil
-}
-
-// Subprotocol returns the negotiated protocol for the connection.
-func (c *Conn) Subprotocol() string {
- return c.subprotocol
-}
-
-// Close closes the underlying network connection without sending or waiting
-// for a close message.
-func (c *Conn) Close() error {
- return c.conn.Close()
-}
-
-// LocalAddr returns the local network address.
-func (c *Conn) LocalAddr() net.Addr {
- return c.conn.LocalAddr()
-}
-
-// RemoteAddr returns the remote network address.
-func (c *Conn) RemoteAddr() net.Addr {
- return c.conn.RemoteAddr()
-}
-
-// Write methods
-
-func (c *Conn) writeFatal(err error) error {
- err = hideTempErr(err)
- c.writeErrMu.Lock()
- if c.writeErr == nil {
- c.writeErr = err
- }
- c.writeErrMu.Unlock()
- return err
-}
-
-func (c *Conn) read(n int) ([]byte, error) {
- p, err := c.br.Peek(n)
- if err == io.EOF {
- err = errUnexpectedEOF
- }
- c.br.Discard(len(p))
- return p, err
-}
-
-func (c *Conn) write(frameType int, deadline time.Time, buf0, buf1 []byte) error {
- <-c.mu
- defer func() { c.mu <- struct{}{} }()
-
- c.writeErrMu.Lock()
- err := c.writeErr
- c.writeErrMu.Unlock()
- if err != nil {
- return err
- }
-
- c.conn.SetWriteDeadline(deadline)
- if len(buf1) == 0 {
- _, err = c.conn.Write(buf0)
- } else {
- err = c.writeBufs(buf0, buf1)
- }
- if err != nil {
- return c.writeFatal(err)
- }
- if frameType == CloseMessage {
- c.writeFatal(ErrCloseSent)
- }
- return nil
-}
-
-func (c *Conn) writeBufs(bufs ...[]byte) error {
- b := net.Buffers(bufs)
- _, err := b.WriteTo(c.conn)
- return err
-}
-
-// WriteControl writes a control message with the given deadline. The allowed
-// message types are CloseMessage, PingMessage and PongMessage.
-func (c *Conn) WriteControl(messageType int, data []byte, deadline time.Time) error {
- if !isControl(messageType) {
- return errBadWriteOpCode
- }
- if len(data) > maxControlFramePayloadSize {
- return errInvalidControlFrame
- }
-
- b0 := byte(messageType) | finalBit
- b1 := byte(len(data))
- if !c.isServer {
- b1 |= maskBit
- }
-
- buf := make([]byte, 0, maxFrameHeaderSize+maxControlFramePayloadSize)
- buf = append(buf, b0, b1)
-
- if c.isServer {
- buf = append(buf, data...)
- } else {
- key := newMaskKey()
- buf = append(buf, key[:]...)
- buf = append(buf, data...)
- maskBytes(key, 0, buf[6:])
- }
-
- d := 1000 * time.Hour
- if !deadline.IsZero() {
- d = deadline.Sub(time.Now())
- if d < 0 {
- return errWriteTimeout
- }
- }
-
- timer := time.NewTimer(d)
- select {
- case <-c.mu:
- timer.Stop()
- case <-timer.C:
- return errWriteTimeout
- }
- defer func() { c.mu <- struct{}{} }()
-
- c.writeErrMu.Lock()
- err := c.writeErr
- c.writeErrMu.Unlock()
- if err != nil {
- return err
- }
-
- c.conn.SetWriteDeadline(deadline)
- _, err = c.conn.Write(buf)
- if err != nil {
- return c.writeFatal(err)
- }
- if messageType == CloseMessage {
- c.writeFatal(ErrCloseSent)
- }
- return err
-}
-
-// beginMessage prepares a connection and message writer for a new message.
-func (c *Conn) beginMessage(mw *messageWriter, messageType int) error {
- // Close previous writer if not already closed by the application. It's
- // probably better to return an error in this situation, but we cannot
- // change this without breaking existing applications.
- if c.writer != nil {
- c.writer.Close()
- c.writer = nil
- }
-
- if !isControl(messageType) && !isData(messageType) {
- return errBadWriteOpCode
- }
-
- c.writeErrMu.Lock()
- err := c.writeErr
- c.writeErrMu.Unlock()
- if err != nil {
- return err
- }
-
- mw.c = c
- mw.frameType = messageType
- mw.pos = maxFrameHeaderSize
-
- if c.writeBuf == nil {
- wpd, ok := c.writePool.Get().(writePoolData)
- if ok {
- c.writeBuf = wpd.buf
- } else {
- c.writeBuf = make([]byte, c.writeBufSize)
- }
- }
- return nil
-}
-
-// NextWriter returns a writer for the next message to send. The writer's Close
-// method flushes the complete message to the network.
-//
-// There can be at most one open writer on a connection. NextWriter closes the
-// previous writer if the application has not already done so.
-//
-// All message types (TextMessage, BinaryMessage, CloseMessage, PingMessage and
-// PongMessage) are supported.
-func (c *Conn) NextWriter(messageType int) (io.WriteCloser, error) {
- var mw messageWriter
- if err := c.beginMessage(&mw, messageType); err != nil {
- return nil, err
- }
- c.writer = &mw
- if c.newCompressionWriter != nil && c.enableWriteCompression && isData(messageType) {
- w := c.newCompressionWriter(c.writer, c.compressionLevel)
- mw.compress = true
- c.writer = w
- }
- return c.writer, nil
-}
-
-type messageWriter struct {
- c *Conn
- compress bool // whether next call to flushFrame should set RSV1
- pos int // end of data in writeBuf.
- frameType int // type of the current frame.
- err error
-}
-
-func (w *messageWriter) endMessage(err error) error {
- if w.err != nil {
- return err
- }
- c := w.c
- w.err = err
- c.writer = nil
- if c.writePool != nil {
- c.writePool.Put(writePoolData{buf: c.writeBuf})
- c.writeBuf = nil
- }
- return err
-}
-
-// flushFrame writes buffered data and extra as a frame to the network. The
-// final argument indicates that this is the last frame in the message.
-func (w *messageWriter) flushFrame(final bool, extra []byte) error {
- c := w.c
- length := w.pos - maxFrameHeaderSize + len(extra)
-
- // Check for invalid control frames.
- if isControl(w.frameType) &&
- (!final || length > maxControlFramePayloadSize) {
- return w.endMessage(errInvalidControlFrame)
- }
-
- b0 := byte(w.frameType)
- if final {
- b0 |= finalBit
- }
- if w.compress {
- b0 |= rsv1Bit
- }
- w.compress = false
-
- b1 := byte(0)
- if !c.isServer {
- b1 |= maskBit
- }
-
- // Assume that the frame starts at beginning of c.writeBuf.
- framePos := 0
- if c.isServer {
- // Adjust up if mask not included in the header.
- framePos = 4
- }
-
- switch {
- case length >= 65536:
- c.writeBuf[framePos] = b0
- c.writeBuf[framePos+1] = b1 | 127
- binary.BigEndian.PutUint64(c.writeBuf[framePos+2:], uint64(length))
- case length > 125:
- framePos += 6
- c.writeBuf[framePos] = b0
- c.writeBuf[framePos+1] = b1 | 126
- binary.BigEndian.PutUint16(c.writeBuf[framePos+2:], uint16(length))
- default:
- framePos += 8
- c.writeBuf[framePos] = b0
- c.writeBuf[framePos+1] = b1 | byte(length)
- }
-
- if !c.isServer {
- key := newMaskKey()
- copy(c.writeBuf[maxFrameHeaderSize-4:], key[:])
- maskBytes(key, 0, c.writeBuf[maxFrameHeaderSize:w.pos])
- if len(extra) > 0 {
- return w.endMessage(c.writeFatal(errors.New("websocket: internal error, extra used in client mode")))
- }
- }
-
- // Write the buffers to the connection with best-effort detection of
- // concurrent writes. See the concurrency section in the package
- // documentation for more info.
-
- if c.isWriting {
- panic("concurrent write to websocket connection")
- }
- c.isWriting = true
-
- err := c.write(w.frameType, c.writeDeadline, c.writeBuf[framePos:w.pos], extra)
-
- if !c.isWriting {
- panic("concurrent write to websocket connection")
- }
- c.isWriting = false
-
- if err != nil {
- return w.endMessage(err)
- }
-
- if final {
- w.endMessage(errWriteClosed)
- return nil
- }
-
- // Setup for next frame.
- w.pos = maxFrameHeaderSize
- w.frameType = continuationFrame
- return nil
-}
-
-func (w *messageWriter) ncopy(max int) (int, error) {
- n := len(w.c.writeBuf) - w.pos
- if n <= 0 {
- if err := w.flushFrame(false, nil); err != nil {
- return 0, err
- }
- n = len(w.c.writeBuf) - w.pos
- }
- if n > max {
- n = max
- }
- return n, nil
-}
-
-func (w *messageWriter) Write(p []byte) (int, error) {
- if w.err != nil {
- return 0, w.err
- }
-
- if len(p) > 2*len(w.c.writeBuf) && w.c.isServer {
- // Don't buffer large messages.
- err := w.flushFrame(false, p)
- if err != nil {
- return 0, err
- }
- return len(p), nil
- }
-
- nn := len(p)
- for len(p) > 0 {
- n, err := w.ncopy(len(p))
- if err != nil {
- return 0, err
- }
- copy(w.c.writeBuf[w.pos:], p[:n])
- w.pos += n
- p = p[n:]
- }
- return nn, nil
-}
-
-func (w *messageWriter) WriteString(p string) (int, error) {
- if w.err != nil {
- return 0, w.err
- }
-
- nn := len(p)
- for len(p) > 0 {
- n, err := w.ncopy(len(p))
- if err != nil {
- return 0, err
- }
- copy(w.c.writeBuf[w.pos:], p[:n])
- w.pos += n
- p = p[n:]
- }
- return nn, nil
-}
-
-func (w *messageWriter) ReadFrom(r io.Reader) (nn int64, err error) {
- if w.err != nil {
- return 0, w.err
- }
- for {
- if w.pos == len(w.c.writeBuf) {
- err = w.flushFrame(false, nil)
- if err != nil {
- break
- }
- }
- var n int
- n, err = r.Read(w.c.writeBuf[w.pos:])
- w.pos += n
- nn += int64(n)
- if err != nil {
- if err == io.EOF {
- err = nil
- }
- break
- }
- }
- return nn, err
-}
-
-func (w *messageWriter) Close() error {
- if w.err != nil {
- return w.err
- }
- return w.flushFrame(true, nil)
-}
-
-// WritePreparedMessage writes prepared message into connection.
-func (c *Conn) WritePreparedMessage(pm *PreparedMessage) error {
- frameType, frameData, err := pm.frame(prepareKey{
- isServer: c.isServer,
- compress: c.newCompressionWriter != nil && c.enableWriteCompression && isData(pm.messageType),
- compressionLevel: c.compressionLevel,
- })
- if err != nil {
- return err
- }
- if c.isWriting {
- panic("concurrent write to websocket connection")
- }
- c.isWriting = true
- err = c.write(frameType, c.writeDeadline, frameData, nil)
- if !c.isWriting {
- panic("concurrent write to websocket connection")
- }
- c.isWriting = false
- return err
-}
-
-// WriteMessage is a helper method for getting a writer using NextWriter,
-// writing the message and closing the writer.
-func (c *Conn) WriteMessage(messageType int, data []byte) error {
-
- if c.isServer && (c.newCompressionWriter == nil || !c.enableWriteCompression) {
- // Fast path with no allocations and single frame.
-
- var mw messageWriter
- if err := c.beginMessage(&mw, messageType); err != nil {
- return err
- }
- n := copy(c.writeBuf[mw.pos:], data)
- mw.pos += n
- data = data[n:]
- return mw.flushFrame(true, data)
- }
-
- w, err := c.NextWriter(messageType)
- if err != nil {
- return err
- }
- if _, err = w.Write(data); err != nil {
- return err
- }
- return w.Close()
-}
-
-// SetWriteDeadline sets the write deadline on the underlying network
-// connection. After a write has timed out, the websocket state is corrupt and
-// all future writes will return an error. A zero value for t means writes will
-// not time out.
-func (c *Conn) SetWriteDeadline(t time.Time) error {
- c.writeDeadline = t
- return nil
-}
-
-// Read methods
-
-func (c *Conn) advanceFrame() (int, error) {
- // 1. Skip remainder of previous frame.
-
- if c.readRemaining > 0 {
- if _, err := io.CopyN(ioutil.Discard, c.br, c.readRemaining); err != nil {
- return noFrame, err
- }
- }
-
- // 2. Read and parse first two bytes of frame header.
- // To aid debugging, collect and report all errors in the first two bytes
- // of the header.
-
- var errors []string
-
- p, err := c.read(2)
- if err != nil {
- return noFrame, err
- }
-
- frameType := int(p[0] & 0xf)
- final := p[0]&finalBit != 0
- rsv1 := p[0]&rsv1Bit != 0
- rsv2 := p[0]&rsv2Bit != 0
- rsv3 := p[0]&rsv3Bit != 0
- mask := p[1]&maskBit != 0
- c.setReadRemaining(int64(p[1] & 0x7f))
-
- c.readDecompress = false
- if rsv1 {
- if c.newDecompressionReader != nil {
- c.readDecompress = true
- } else {
- errors = append(errors, "RSV1 set")
- }
- }
-
- if rsv2 {
- errors = append(errors, "RSV2 set")
- }
-
- if rsv3 {
- errors = append(errors, "RSV3 set")
- }
-
- switch frameType {
- case CloseMessage, PingMessage, PongMessage:
- if c.readRemaining > maxControlFramePayloadSize {
- errors = append(errors, "len > 125 for control")
- }
- if !final {
- errors = append(errors, "FIN not set on control")
- }
- case TextMessage, BinaryMessage:
- if !c.readFinal {
- errors = append(errors, "data before FIN")
- }
- c.readFinal = final
- case continuationFrame:
- if c.readFinal {
- errors = append(errors, "continuation after FIN")
- }
- c.readFinal = final
- default:
- errors = append(errors, "bad opcode "+strconv.Itoa(frameType))
- }
-
- if mask != c.isServer {
- errors = append(errors, "bad MASK")
- }
-
- if len(errors) > 0 {
- return noFrame, c.handleProtocolError(strings.Join(errors, ", "))
- }
-
- // 3. Read and parse frame length as per
- // https://tools.ietf.org/html/rfc6455#section-5.2
- //
- // The length of the "Payload data", in bytes: if 0-125, that is the payload
- // length.
- // - If 126, the following 2 bytes interpreted as a 16-bit unsigned
- // integer are the payload length.
- // - If 127, the following 8 bytes interpreted as
- // a 64-bit unsigned integer (the most significant bit MUST be 0) are the
- // payload length. Multibyte length quantities are expressed in network byte
- // order.
-
- switch c.readRemaining {
- case 126:
- p, err := c.read(2)
- if err != nil {
- return noFrame, err
- }
-
- if err := c.setReadRemaining(int64(binary.BigEndian.Uint16(p))); err != nil {
- return noFrame, err
- }
- case 127:
- p, err := c.read(8)
- if err != nil {
- return noFrame, err
- }
-
- if err := c.setReadRemaining(int64(binary.BigEndian.Uint64(p))); err != nil {
- return noFrame, err
- }
- }
-
- // 4. Handle frame masking.
-
- if mask {
- c.readMaskPos = 0
- p, err := c.read(len(c.readMaskKey))
- if err != nil {
- return noFrame, err
- }
- copy(c.readMaskKey[:], p)
- }
-
- // 5. For text and binary messages, enforce read limit and return.
-
- if frameType == continuationFrame || frameType == TextMessage || frameType == BinaryMessage {
-
- c.readLength += c.readRemaining
- // Don't allow readLength to overflow in the presence of a large readRemaining
- // counter.
- if c.readLength < 0 {
- return noFrame, ErrReadLimit
- }
-
- if c.readLimit > 0 && c.readLength > c.readLimit {
- c.WriteControl(CloseMessage, FormatCloseMessage(CloseMessageTooBig, ""), time.Now().Add(writeWait))
- return noFrame, ErrReadLimit
- }
-
- return frameType, nil
- }
-
- // 6. Read control frame payload.
-
- var payload []byte
- if c.readRemaining > 0 {
- payload, err = c.read(int(c.readRemaining))
- c.setReadRemaining(0)
- if err != nil {
- return noFrame, err
- }
- if c.isServer {
- maskBytes(c.readMaskKey, 0, payload)
- }
- }
-
- // 7. Process control frame payload.
-
- switch frameType {
- case PongMessage:
- if err := c.handlePong(string(payload)); err != nil {
- return noFrame, err
- }
- case PingMessage:
- if err := c.handlePing(string(payload)); err != nil {
- return noFrame, err
- }
- case CloseMessage:
- closeCode := CloseNoStatusReceived
- closeText := ""
- if len(payload) >= 2 {
- closeCode = int(binary.BigEndian.Uint16(payload))
- if !isValidReceivedCloseCode(closeCode) {
- return noFrame, c.handleProtocolError("bad close code " + strconv.Itoa(closeCode))
- }
- closeText = string(payload[2:])
- if !utf8.ValidString(closeText) {
- return noFrame, c.handleProtocolError("invalid utf8 payload in close frame")
- }
- }
- if err := c.handleClose(closeCode, closeText); err != nil {
- return noFrame, err
- }
- return noFrame, &CloseError{Code: closeCode, Text: closeText}
- }
-
- return frameType, nil
-}
-
-func (c *Conn) handleProtocolError(message string) error {
- data := FormatCloseMessage(CloseProtocolError, message)
- if len(data) > maxControlFramePayloadSize {
- data = data[:maxControlFramePayloadSize]
- }
- c.WriteControl(CloseMessage, data, time.Now().Add(writeWait))
- return errors.New("websocket: " + message)
-}
-
-// NextReader returns the next data message received from the peer. The
-// returned messageType is either TextMessage or BinaryMessage.
-//
-// There can be at most one open reader on a connection. NextReader discards
-// the previous message if the application has not already consumed it.
-//
-// Applications must break out of the application's read loop when this method
-// returns a non-nil error value. Errors returned from this method are
-// permanent. Once this method returns a non-nil error, all subsequent calls to
-// this method return the same error.
-func (c *Conn) NextReader() (messageType int, r io.Reader, err error) {
- // Close previous reader, only relevant for decompression.
- if c.reader != nil {
- c.reader.Close()
- c.reader = nil
- }
-
- c.messageReader = nil
- c.readLength = 0
-
- for c.readErr == nil {
- frameType, err := c.advanceFrame()
- if err != nil {
- c.readErr = hideTempErr(err)
- break
- }
-
- if frameType == TextMessage || frameType == BinaryMessage {
- c.messageReader = &messageReader{c}
- c.reader = c.messageReader
- if c.readDecompress {
- c.reader = c.newDecompressionReader(c.reader)
- }
- return frameType, c.reader, nil
- }
- }
-
- // Applications that do handle the error returned from this method spin in
- // tight loop on connection failure. To help application developers detect
- // this error, panic on repeated reads to the failed connection.
- c.readErrCount++
- if c.readErrCount >= 1000 {
- panic("repeated read on failed websocket connection")
- }
-
- return noFrame, nil, c.readErr
-}
-
-type messageReader struct{ c *Conn }
-
-func (r *messageReader) Read(b []byte) (int, error) {
- c := r.c
- if c.messageReader != r {
- return 0, io.EOF
- }
-
- for c.readErr == nil {
-
- if c.readRemaining > 0 {
- if int64(len(b)) > c.readRemaining {
- b = b[:c.readRemaining]
- }
- n, err := c.br.Read(b)
- c.readErr = hideTempErr(err)
- if c.isServer {
- c.readMaskPos = maskBytes(c.readMaskKey, c.readMaskPos, b[:n])
- }
- rem := c.readRemaining
- rem -= int64(n)
- c.setReadRemaining(rem)
- if c.readRemaining > 0 && c.readErr == io.EOF {
- c.readErr = errUnexpectedEOF
- }
- return n, c.readErr
- }
-
- if c.readFinal {
- c.messageReader = nil
- return 0, io.EOF
- }
-
- frameType, err := c.advanceFrame()
- switch {
- case err != nil:
- c.readErr = hideTempErr(err)
- case frameType == TextMessage || frameType == BinaryMessage:
- c.readErr = errors.New("websocket: internal error, unexpected text or binary in Reader")
- }
- }
-
- err := c.readErr
- if err == io.EOF && c.messageReader == r {
- err = errUnexpectedEOF
- }
- return 0, err
-}
-
-func (r *messageReader) Close() error {
- return nil
-}
-
-// ReadMessage is a helper method for getting a reader using NextReader and
-// reading from that reader to a buffer.
-func (c *Conn) ReadMessage() (messageType int, p []byte, err error) {
- var r io.Reader
- messageType, r, err = c.NextReader()
- if err != nil {
- return messageType, nil, err
- }
- p, err = ioutil.ReadAll(r)
- return messageType, p, err
-}
-
-// SetReadDeadline sets the read deadline on the underlying network connection.
-// After a read has timed out, the websocket connection state is corrupt and
-// all future reads will return an error. A zero value for t means reads will
-// not time out.
-func (c *Conn) SetReadDeadline(t time.Time) error {
- return c.conn.SetReadDeadline(t)
-}
-
-// SetReadLimit sets the maximum size in bytes for a message read from the peer. If a
-// message exceeds the limit, the connection sends a close message to the peer
-// and returns ErrReadLimit to the application.
-func (c *Conn) SetReadLimit(limit int64) {
- c.readLimit = limit
-}
-
-// CloseHandler returns the current close handler
-func (c *Conn) CloseHandler() func(code int, text string) error {
- return c.handleClose
-}
-
-// SetCloseHandler sets the handler for close messages received from the peer.
-// The code argument to h is the received close code or CloseNoStatusReceived
-// if the close message is empty. The default close handler sends a close
-// message back to the peer.
-//
-// The handler function is called from the NextReader, ReadMessage and message
-// reader Read methods. The application must read the connection to process
-// close messages as described in the section on Control Messages above.
-//
-// The connection read methods return a CloseError when a close message is
-// received. Most applications should handle close messages as part of their
-// normal error handling. Applications should only set a close handler when the
-// application must perform some action before sending a close message back to
-// the peer.
-func (c *Conn) SetCloseHandler(h func(code int, text string) error) {
- if h == nil {
- h = func(code int, text string) error {
- message := FormatCloseMessage(code, "")
- c.WriteControl(CloseMessage, message, time.Now().Add(writeWait))
- return nil
- }
- }
- c.handleClose = h
-}
-
-// PingHandler returns the current ping handler
-func (c *Conn) PingHandler() func(appData string) error {
- return c.handlePing
-}
-
-// SetPingHandler sets the handler for ping messages received from the peer.
-// The appData argument to h is the PING message application data. The default
-// ping handler sends a pong to the peer.
-//
-// The handler function is called from the NextReader, ReadMessage and message
-// reader Read methods. The application must read the connection to process
-// ping messages as described in the section on Control Messages above.
-func (c *Conn) SetPingHandler(h func(appData string) error) {
- if h == nil {
- h = func(message string) error {
- err := c.WriteControl(PongMessage, []byte(message), time.Now().Add(writeWait))
- if err == ErrCloseSent {
- return nil
- } else if e, ok := err.(net.Error); ok && e.Temporary() {
- return nil
- }
- return err
- }
- }
- c.handlePing = h
-}
-
-// PongHandler returns the current pong handler
-func (c *Conn) PongHandler() func(appData string) error {
- return c.handlePong
-}
-
-// SetPongHandler sets the handler for pong messages received from the peer.
-// The appData argument to h is the PONG message application data. The default
-// pong handler does nothing.
-//
-// The handler function is called from the NextReader, ReadMessage and message
-// reader Read methods. The application must read the connection to process
-// pong messages as described in the section on Control Messages above.
-func (c *Conn) SetPongHandler(h func(appData string) error) {
- if h == nil {
- h = func(string) error { return nil }
- }
- c.handlePong = h
-}
-
-// UnderlyingConn returns the internal net.Conn. This can be used to further
-// modifications to connection specific flags.
-func (c *Conn) UnderlyingConn() net.Conn {
- return c.conn
-}
-
-// EnableWriteCompression enables and disables write compression of
-// subsequent text and binary messages. This function is a noop if
-// compression was not negotiated with the peer.
-func (c *Conn) EnableWriteCompression(enable bool) {
- c.enableWriteCompression = enable
-}
-
-// SetCompressionLevel sets the flate compression level for subsequent text and
-// binary messages. This function is a noop if compression was not negotiated
-// with the peer. See the compress/flate package for a description of
-// compression levels.
-func (c *Conn) SetCompressionLevel(level int) error {
- if !isValidCompressionLevel(level) {
- return errors.New("websocket: invalid compression level")
- }
- c.compressionLevel = level
- return nil
-}
-
-// FormatCloseMessage formats closeCode and text as a WebSocket close message.
-// An empty message is returned for code CloseNoStatusReceived.
-func FormatCloseMessage(closeCode int, text string) []byte {
- if closeCode == CloseNoStatusReceived {
- // Return empty message because it's illegal to send
- // CloseNoStatusReceived. Return non-nil value in case application
- // checks for nil.
- return []byte{}
- }
- buf := make([]byte, 2+len(text))
- binary.BigEndian.PutUint16(buf, uint16(closeCode))
- copy(buf[2:], text)
- return buf
-}
diff --git a/vendor/github.com/gorilla/websocket/doc.go b/vendor/github.com/gorilla/websocket/doc.go
deleted file mode 100644
index 8db0cef9..00000000
--- a/vendor/github.com/gorilla/websocket/doc.go
+++ /dev/null
@@ -1,227 +0,0 @@
-// Copyright 2013 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package websocket implements the WebSocket protocol defined in RFC 6455.
-//
-// Overview
-//
-// The Conn type represents a WebSocket connection. A server application calls
-// the Upgrader.Upgrade method from an HTTP request handler to get a *Conn:
-//
-// var upgrader = websocket.Upgrader{
-// ReadBufferSize: 1024,
-// WriteBufferSize: 1024,
-// }
-//
-// func handler(w http.ResponseWriter, r *http.Request) {
-// conn, err := upgrader.Upgrade(w, r, nil)
-// if err != nil {
-// log.Println(err)
-// return
-// }
-// ... Use conn to send and receive messages.
-// }
-//
-// Call the connection's WriteMessage and ReadMessage methods to send and
-// receive messages as a slice of bytes. This snippet of code shows how to echo
-// messages using these methods:
-//
-// for {
-// messageType, p, err := conn.ReadMessage()
-// if err != nil {
-// log.Println(err)
-// return
-// }
-// if err := conn.WriteMessage(messageType, p); err != nil {
-// log.Println(err)
-// return
-// }
-// }
-//
-// In above snippet of code, p is a []byte and messageType is an int with value
-// websocket.BinaryMessage or websocket.TextMessage.
-//
-// An application can also send and receive messages using the io.WriteCloser
-// and io.Reader interfaces. To send a message, call the connection NextWriter
-// method to get an io.WriteCloser, write the message to the writer and close
-// the writer when done. To receive a message, call the connection NextReader
-// method to get an io.Reader and read until io.EOF is returned. This snippet
-// shows how to echo messages using the NextWriter and NextReader methods:
-//
-// for {
-// messageType, r, err := conn.NextReader()
-// if err != nil {
-// return
-// }
-// w, err := conn.NextWriter(messageType)
-// if err != nil {
-// return err
-// }
-// if _, err := io.Copy(w, r); err != nil {
-// return err
-// }
-// if err := w.Close(); err != nil {
-// return err
-// }
-// }
-//
-// Data Messages
-//
-// The WebSocket protocol distinguishes between text and binary data messages.
-// Text messages are interpreted as UTF-8 encoded text. The interpretation of
-// binary messages is left to the application.
-//
-// This package uses the TextMessage and BinaryMessage integer constants to
-// identify the two data message types. The ReadMessage and NextReader methods
-// return the type of the received message. The messageType argument to the
-// WriteMessage and NextWriter methods specifies the type of a sent message.
-//
-// It is the application's responsibility to ensure that text messages are
-// valid UTF-8 encoded text.
-//
-// Control Messages
-//
-// The WebSocket protocol defines three types of control messages: close, ping
-// and pong. Call the connection WriteControl, WriteMessage or NextWriter
-// methods to send a control message to the peer.
-//
-// Connections handle received close messages by calling the handler function
-// set with the SetCloseHandler method and by returning a *CloseError from the
-// NextReader, ReadMessage or the message Read method. The default close
-// handler sends a close message to the peer.
-//
-// Connections handle received ping messages by calling the handler function
-// set with the SetPingHandler method. The default ping handler sends a pong
-// message to the peer.
-//
-// Connections handle received pong messages by calling the handler function
-// set with the SetPongHandler method. The default pong handler does nothing.
-// If an application sends ping messages, then the application should set a
-// pong handler to receive the corresponding pong.
-//
-// The control message handler functions are called from the NextReader,
-// ReadMessage and message reader Read methods. The default close and ping
-// handlers can block these methods for a short time when the handler writes to
-// the connection.
-//
-// The application must read the connection to process close, ping and pong
-// messages sent from the peer. If the application is not otherwise interested
-// in messages from the peer, then the application should start a goroutine to
-// read and discard messages from the peer. A simple example is:
-//
-// func readLoop(c *websocket.Conn) {
-// for {
-// if _, _, err := c.NextReader(); err != nil {
-// c.Close()
-// break
-// }
-// }
-// }
-//
-// Concurrency
-//
-// Connections support one concurrent reader and one concurrent writer.
-//
-// Applications are responsible for ensuring that no more than one goroutine
-// calls the write methods (NextWriter, SetWriteDeadline, WriteMessage,
-// WriteJSON, EnableWriteCompression, SetCompressionLevel) concurrently and
-// that no more than one goroutine calls the read methods (NextReader,
-// SetReadDeadline, ReadMessage, ReadJSON, SetPongHandler, SetPingHandler)
-// concurrently.
-//
-// The Close and WriteControl methods can be called concurrently with all other
-// methods.
-//
-// Origin Considerations
-//
-// Web browsers allow Javascript applications to open a WebSocket connection to
-// any host. It's up to the server to enforce an origin policy using the Origin
-// request header sent by the browser.
-//
-// The Upgrader calls the function specified in the CheckOrigin field to check
-// the origin. If the CheckOrigin function returns false, then the Upgrade
-// method fails the WebSocket handshake with HTTP status 403.
-//
-// If the CheckOrigin field is nil, then the Upgrader uses a safe default: fail
-// the handshake if the Origin request header is present and the Origin host is
-// not equal to the Host request header.
-//
-// The deprecated package-level Upgrade function does not perform origin
-// checking. The application is responsible for checking the Origin header
-// before calling the Upgrade function.
-//
-// Buffers
-//
-// Connections buffer network input and output to reduce the number
-// of system calls when reading or writing messages.
-//
-// Write buffers are also used for constructing WebSocket frames. See RFC 6455,
-// Section 5 for a discussion of message framing. A WebSocket frame header is
-// written to the network each time a write buffer is flushed to the network.
-// Decreasing the size of the write buffer can increase the amount of framing
-// overhead on the connection.
-//
-// The buffer sizes in bytes are specified by the ReadBufferSize and
-// WriteBufferSize fields in the Dialer and Upgrader. The Dialer uses a default
-// size of 4096 when a buffer size field is set to zero. The Upgrader reuses
-// buffers created by the HTTP server when a buffer size field is set to zero.
-// The HTTP server buffers have a size of 4096 at the time of this writing.
-//
-// The buffer sizes do not limit the size of a message that can be read or
-// written by a connection.
-//
-// Buffers are held for the lifetime of the connection by default. If the
-// Dialer or Upgrader WriteBufferPool field is set, then a connection holds the
-// write buffer only when writing a message.
-//
-// Applications should tune the buffer sizes to balance memory use and
-// performance. Increasing the buffer size uses more memory, but can reduce the
-// number of system calls to read or write the network. In the case of writing,
-// increasing the buffer size can reduce the number of frame headers written to
-// the network.
-//
-// Some guidelines for setting buffer parameters are:
-//
-// Limit the buffer sizes to the maximum expected message size. Buffers larger
-// than the largest message do not provide any benefit.
-//
-// Depending on the distribution of message sizes, setting the buffer size to
-// a value less than the maximum expected message size can greatly reduce memory
-// use with a small impact on performance. Here's an example: If 99% of the
-// messages are smaller than 256 bytes and the maximum message size is 512
-// bytes, then a buffer size of 256 bytes will result in 1.01 more system calls
-// than a buffer size of 512 bytes. The memory savings is 50%.
-//
-// A write buffer pool is useful when the application has a modest number
-// writes over a large number of connections. when buffers are pooled, a larger
-// buffer size has a reduced impact on total memory use and has the benefit of
-// reducing system calls and frame overhead.
-//
-// Compression EXPERIMENTAL
-//
-// Per message compression extensions (RFC 7692) are experimentally supported
-// by this package in a limited capacity. Setting the EnableCompression option
-// to true in Dialer or Upgrader will attempt to negotiate per message deflate
-// support.
-//
-// var upgrader = websocket.Upgrader{
-// EnableCompression: true,
-// }
-//
-// If compression was successfully negotiated with the connection's peer, any
-// message received in compressed form will be automatically decompressed.
-// All Read methods will return uncompressed bytes.
-//
-// Per message compression of messages written to a connection can be enabled
-// or disabled by calling the corresponding Conn method:
-//
-// conn.EnableWriteCompression(false)
-//
-// Currently this package does not support compression with "context takeover".
-// This means that messages must be compressed and decompressed in isolation,
-// without retaining sliding window or dictionary state across messages. For
-// more details refer to RFC 7692.
-//
-// Use of compression is experimental and may result in decreased performance.
-package websocket
diff --git a/vendor/github.com/gorilla/websocket/join.go b/vendor/github.com/gorilla/websocket/join.go
deleted file mode 100644
index c64f8c82..00000000
--- a/vendor/github.com/gorilla/websocket/join.go
+++ /dev/null
@@ -1,42 +0,0 @@
-// Copyright 2019 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "io"
- "strings"
-)
-
-// JoinMessages concatenates received messages to create a single io.Reader.
-// The string term is appended to each message. The returned reader does not
-// support concurrent calls to the Read method.
-func JoinMessages(c *Conn, term string) io.Reader {
- return &joinReader{c: c, term: term}
-}
-
-type joinReader struct {
- c *Conn
- term string
- r io.Reader
-}
-
-func (r *joinReader) Read(p []byte) (int, error) {
- if r.r == nil {
- var err error
- _, r.r, err = r.c.NextReader()
- if err != nil {
- return 0, err
- }
- if r.term != "" {
- r.r = io.MultiReader(r.r, strings.NewReader(r.term))
- }
- }
- n, err := r.r.Read(p)
- if err == io.EOF {
- err = nil
- r.r = nil
- }
- return n, err
-}
diff --git a/vendor/github.com/gorilla/websocket/json.go b/vendor/github.com/gorilla/websocket/json.go
deleted file mode 100644
index dc2c1f64..00000000
--- a/vendor/github.com/gorilla/websocket/json.go
+++ /dev/null
@@ -1,60 +0,0 @@
-// Copyright 2013 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "encoding/json"
- "io"
-)
-
-// WriteJSON writes the JSON encoding of v as a message.
-//
-// Deprecated: Use c.WriteJSON instead.
-func WriteJSON(c *Conn, v interface{}) error {
- return c.WriteJSON(v)
-}
-
-// WriteJSON writes the JSON encoding of v as a message.
-//
-// See the documentation for encoding/json Marshal for details about the
-// conversion of Go values to JSON.
-func (c *Conn) WriteJSON(v interface{}) error {
- w, err := c.NextWriter(TextMessage)
- if err != nil {
- return err
- }
- err1 := json.NewEncoder(w).Encode(v)
- err2 := w.Close()
- if err1 != nil {
- return err1
- }
- return err2
-}
-
-// ReadJSON reads the next JSON-encoded message from the connection and stores
-// it in the value pointed to by v.
-//
-// Deprecated: Use c.ReadJSON instead.
-func ReadJSON(c *Conn, v interface{}) error {
- return c.ReadJSON(v)
-}
-
-// ReadJSON reads the next JSON-encoded message from the connection and stores
-// it in the value pointed to by v.
-//
-// See the documentation for the encoding/json Unmarshal function for details
-// about the conversion of JSON to a Go value.
-func (c *Conn) ReadJSON(v interface{}) error {
- _, r, err := c.NextReader()
- if err != nil {
- return err
- }
- err = json.NewDecoder(r).Decode(v)
- if err == io.EOF {
- // One value is expected in the message.
- err = io.ErrUnexpectedEOF
- }
- return err
-}
diff --git a/vendor/github.com/gorilla/websocket/mask.go b/vendor/github.com/gorilla/websocket/mask.go
deleted file mode 100644
index d0742bf2..00000000
--- a/vendor/github.com/gorilla/websocket/mask.go
+++ /dev/null
@@ -1,55 +0,0 @@
-// Copyright 2016 The Gorilla WebSocket Authors. All rights reserved. Use of
-// this source code is governed by a BSD-style license that can be found in the
-// LICENSE file.
-
-//go:build !appengine
-// +build !appengine
-
-package websocket
-
-import "unsafe"
-
-const wordSize = int(unsafe.Sizeof(uintptr(0)))
-
-func maskBytes(key [4]byte, pos int, b []byte) int {
- // Mask one byte at a time for small buffers.
- if len(b) < 2*wordSize {
- for i := range b {
- b[i] ^= key[pos&3]
- pos++
- }
- return pos & 3
- }
-
- // Mask one byte at a time to word boundary.
- if n := int(uintptr(unsafe.Pointer(&b[0]))) % wordSize; n != 0 {
- n = wordSize - n
- for i := range b[:n] {
- b[i] ^= key[pos&3]
- pos++
- }
- b = b[n:]
- }
-
- // Create aligned word size key.
- var k [wordSize]byte
- for i := range k {
- k[i] = key[(pos+i)&3]
- }
- kw := *(*uintptr)(unsafe.Pointer(&k))
-
- // Mask one word at a time.
- n := (len(b) / wordSize) * wordSize
- for i := 0; i < n; i += wordSize {
- *(*uintptr)(unsafe.Pointer(uintptr(unsafe.Pointer(&b[0])) + uintptr(i))) ^= kw
- }
-
- // Mask one byte at a time for remaining bytes.
- b = b[n:]
- for i := range b {
- b[i] ^= key[pos&3]
- pos++
- }
-
- return pos & 3
-}
diff --git a/vendor/github.com/gorilla/websocket/mask_safe.go b/vendor/github.com/gorilla/websocket/mask_safe.go
deleted file mode 100644
index 36250ca7..00000000
--- a/vendor/github.com/gorilla/websocket/mask_safe.go
+++ /dev/null
@@ -1,16 +0,0 @@
-// Copyright 2016 The Gorilla WebSocket Authors. All rights reserved. Use of
-// this source code is governed by a BSD-style license that can be found in the
-// LICENSE file.
-
-//go:build appengine
-// +build appengine
-
-package websocket
-
-func maskBytes(key [4]byte, pos int, b []byte) int {
- for i := range b {
- b[i] ^= key[pos&3]
- pos++
- }
- return pos & 3
-}
diff --git a/vendor/github.com/gorilla/websocket/prepared.go b/vendor/github.com/gorilla/websocket/prepared.go
deleted file mode 100644
index c854225e..00000000
--- a/vendor/github.com/gorilla/websocket/prepared.go
+++ /dev/null
@@ -1,102 +0,0 @@
-// Copyright 2017 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "bytes"
- "net"
- "sync"
- "time"
-)
-
-// PreparedMessage caches on the wire representations of a message payload.
-// Use PreparedMessage to efficiently send a message payload to multiple
-// connections. PreparedMessage is especially useful when compression is used
-// because the CPU and memory expensive compression operation can be executed
-// once for a given set of compression options.
-type PreparedMessage struct {
- messageType int
- data []byte
- mu sync.Mutex
- frames map[prepareKey]*preparedFrame
-}
-
-// prepareKey defines a unique set of options to cache prepared frames in PreparedMessage.
-type prepareKey struct {
- isServer bool
- compress bool
- compressionLevel int
-}
-
-// preparedFrame contains data in wire representation.
-type preparedFrame struct {
- once sync.Once
- data []byte
-}
-
-// NewPreparedMessage returns an initialized PreparedMessage. You can then send
-// it to connection using WritePreparedMessage method. Valid wire
-// representation will be calculated lazily only once for a set of current
-// connection options.
-func NewPreparedMessage(messageType int, data []byte) (*PreparedMessage, error) {
- pm := &PreparedMessage{
- messageType: messageType,
- frames: make(map[prepareKey]*preparedFrame),
- data: data,
- }
-
- // Prepare a plain server frame.
- _, frameData, err := pm.frame(prepareKey{isServer: true, compress: false})
- if err != nil {
- return nil, err
- }
-
- // To protect against caller modifying the data argument, remember the data
- // copied to the plain server frame.
- pm.data = frameData[len(frameData)-len(data):]
- return pm, nil
-}
-
-func (pm *PreparedMessage) frame(key prepareKey) (int, []byte, error) {
- pm.mu.Lock()
- frame, ok := pm.frames[key]
- if !ok {
- frame = &preparedFrame{}
- pm.frames[key] = frame
- }
- pm.mu.Unlock()
-
- var err error
- frame.once.Do(func() {
- // Prepare a frame using a 'fake' connection.
- // TODO: Refactor code in conn.go to allow more direct construction of
- // the frame.
- mu := make(chan struct{}, 1)
- mu <- struct{}{}
- var nc prepareConn
- c := &Conn{
- conn: &nc,
- mu: mu,
- isServer: key.isServer,
- compressionLevel: key.compressionLevel,
- enableWriteCompression: true,
- writeBuf: make([]byte, defaultWriteBufferSize+maxFrameHeaderSize),
- }
- if key.compress {
- c.newCompressionWriter = compressNoContextTakeover
- }
- err = c.WriteMessage(pm.messageType, pm.data)
- frame.data = nc.buf.Bytes()
- })
- return pm.messageType, frame.data, err
-}
-
-type prepareConn struct {
- buf bytes.Buffer
- net.Conn
-}
-
-func (pc *prepareConn) Write(p []byte) (int, error) { return pc.buf.Write(p) }
-func (pc *prepareConn) SetWriteDeadline(t time.Time) error { return nil }
diff --git a/vendor/github.com/gorilla/websocket/proxy.go b/vendor/github.com/gorilla/websocket/proxy.go
deleted file mode 100644
index e0f466b7..00000000
--- a/vendor/github.com/gorilla/websocket/proxy.go
+++ /dev/null
@@ -1,77 +0,0 @@
-// Copyright 2017 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "bufio"
- "encoding/base64"
- "errors"
- "net"
- "net/http"
- "net/url"
- "strings"
-)
-
-type netDialerFunc func(network, addr string) (net.Conn, error)
-
-func (fn netDialerFunc) Dial(network, addr string) (net.Conn, error) {
- return fn(network, addr)
-}
-
-func init() {
- proxy_RegisterDialerType("http", func(proxyURL *url.URL, forwardDialer proxy_Dialer) (proxy_Dialer, error) {
- return &httpProxyDialer{proxyURL: proxyURL, forwardDial: forwardDialer.Dial}, nil
- })
-}
-
-type httpProxyDialer struct {
- proxyURL *url.URL
- forwardDial func(network, addr string) (net.Conn, error)
-}
-
-func (hpd *httpProxyDialer) Dial(network string, addr string) (net.Conn, error) {
- hostPort, _ := hostPortNoPort(hpd.proxyURL)
- conn, err := hpd.forwardDial(network, hostPort)
- if err != nil {
- return nil, err
- }
-
- connectHeader := make(http.Header)
- if user := hpd.proxyURL.User; user != nil {
- proxyUser := user.Username()
- if proxyPassword, passwordSet := user.Password(); passwordSet {
- credential := base64.StdEncoding.EncodeToString([]byte(proxyUser + ":" + proxyPassword))
- connectHeader.Set("Proxy-Authorization", "Basic "+credential)
- }
- }
-
- connectReq := &http.Request{
- Method: http.MethodConnect,
- URL: &url.URL{Opaque: addr},
- Host: addr,
- Header: connectHeader,
- }
-
- if err := connectReq.Write(conn); err != nil {
- conn.Close()
- return nil, err
- }
-
- // Read response. It's OK to use and discard buffered reader here becaue
- // the remote server does not speak until spoken to.
- br := bufio.NewReader(conn)
- resp, err := http.ReadResponse(br, connectReq)
- if err != nil {
- conn.Close()
- return nil, err
- }
-
- if resp.StatusCode != 200 {
- conn.Close()
- f := strings.SplitN(resp.Status, " ", 2)
- return nil, errors.New(f[1])
- }
- return conn, nil
-}
diff --git a/vendor/github.com/gorilla/websocket/server.go b/vendor/github.com/gorilla/websocket/server.go
deleted file mode 100644
index 24d53b38..00000000
--- a/vendor/github.com/gorilla/websocket/server.go
+++ /dev/null
@@ -1,365 +0,0 @@
-// Copyright 2013 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "bufio"
- "errors"
- "io"
- "net/http"
- "net/url"
- "strings"
- "time"
-)
-
-// HandshakeError describes an error with the handshake from the peer.
-type HandshakeError struct {
- message string
-}
-
-func (e HandshakeError) Error() string { return e.message }
-
-// Upgrader specifies parameters for upgrading an HTTP connection to a
-// WebSocket connection.
-//
-// It is safe to call Upgrader's methods concurrently.
-type Upgrader struct {
- // HandshakeTimeout specifies the duration for the handshake to complete.
- HandshakeTimeout time.Duration
-
- // ReadBufferSize and WriteBufferSize specify I/O buffer sizes in bytes. If a buffer
- // size is zero, then buffers allocated by the HTTP server are used. The
- // I/O buffer sizes do not limit the size of the messages that can be sent
- // or received.
- ReadBufferSize, WriteBufferSize int
-
- // WriteBufferPool is a pool of buffers for write operations. If the value
- // is not set, then write buffers are allocated to the connection for the
- // lifetime of the connection.
- //
- // A pool is most useful when the application has a modest volume of writes
- // across a large number of connections.
- //
- // Applications should use a single pool for each unique value of
- // WriteBufferSize.
- WriteBufferPool BufferPool
-
- // Subprotocols specifies the server's supported protocols in order of
- // preference. If this field is not nil, then the Upgrade method negotiates a
- // subprotocol by selecting the first match in this list with a protocol
- // requested by the client. If there's no match, then no protocol is
- // negotiated (the Sec-Websocket-Protocol header is not included in the
- // handshake response).
- Subprotocols []string
-
- // Error specifies the function for generating HTTP error responses. If Error
- // is nil, then http.Error is used to generate the HTTP response.
- Error func(w http.ResponseWriter, r *http.Request, status int, reason error)
-
- // CheckOrigin returns true if the request Origin header is acceptable. If
- // CheckOrigin is nil, then a safe default is used: return false if the
- // Origin request header is present and the origin host is not equal to
- // request Host header.
- //
- // A CheckOrigin function should carefully validate the request origin to
- // prevent cross-site request forgery.
- CheckOrigin func(r *http.Request) bool
-
- // EnableCompression specify if the server should attempt to negotiate per
- // message compression (RFC 7692). Setting this value to true does not
- // guarantee that compression will be supported. Currently only "no context
- // takeover" modes are supported.
- EnableCompression bool
-}
-
-func (u *Upgrader) returnError(w http.ResponseWriter, r *http.Request, status int, reason string) (*Conn, error) {
- err := HandshakeError{reason}
- if u.Error != nil {
- u.Error(w, r, status, err)
- } else {
- w.Header().Set("Sec-Websocket-Version", "13")
- http.Error(w, http.StatusText(status), status)
- }
- return nil, err
-}
-
-// checkSameOrigin returns true if the origin is not set or is equal to the request host.
-func checkSameOrigin(r *http.Request) bool {
- origin := r.Header["Origin"]
- if len(origin) == 0 {
- return true
- }
- u, err := url.Parse(origin[0])
- if err != nil {
- return false
- }
- return equalASCIIFold(u.Host, r.Host)
-}
-
-func (u *Upgrader) selectSubprotocol(r *http.Request, responseHeader http.Header) string {
- if u.Subprotocols != nil {
- clientProtocols := Subprotocols(r)
- for _, serverProtocol := range u.Subprotocols {
- for _, clientProtocol := range clientProtocols {
- if clientProtocol == serverProtocol {
- return clientProtocol
- }
- }
- }
- } else if responseHeader != nil {
- return responseHeader.Get("Sec-Websocket-Protocol")
- }
- return ""
-}
-
-// Upgrade upgrades the HTTP server connection to the WebSocket protocol.
-//
-// The responseHeader is included in the response to the client's upgrade
-// request. Use the responseHeader to specify cookies (Set-Cookie). To specify
-// subprotocols supported by the server, set Upgrader.Subprotocols directly.
-//
-// If the upgrade fails, then Upgrade replies to the client with an HTTP error
-// response.
-func (u *Upgrader) Upgrade(w http.ResponseWriter, r *http.Request, responseHeader http.Header) (*Conn, error) {
- const badHandshake = "websocket: the client is not using the websocket protocol: "
-
- if !tokenListContainsValue(r.Header, "Connection", "upgrade") {
- return u.returnError(w, r, http.StatusBadRequest, badHandshake+"'upgrade' token not found in 'Connection' header")
- }
-
- if !tokenListContainsValue(r.Header, "Upgrade", "websocket") {
- return u.returnError(w, r, http.StatusBadRequest, badHandshake+"'websocket' token not found in 'Upgrade' header")
- }
-
- if r.Method != http.MethodGet {
- return u.returnError(w, r, http.StatusMethodNotAllowed, badHandshake+"request method is not GET")
- }
-
- if !tokenListContainsValue(r.Header, "Sec-Websocket-Version", "13") {
- return u.returnError(w, r, http.StatusBadRequest, "websocket: unsupported version: 13 not found in 'Sec-Websocket-Version' header")
- }
-
- if _, ok := responseHeader["Sec-Websocket-Extensions"]; ok {
- return u.returnError(w, r, http.StatusInternalServerError, "websocket: application specific 'Sec-WebSocket-Extensions' headers are unsupported")
- }
-
- checkOrigin := u.CheckOrigin
- if checkOrigin == nil {
- checkOrigin = checkSameOrigin
- }
- if !checkOrigin(r) {
- return u.returnError(w, r, http.StatusForbidden, "websocket: request origin not allowed by Upgrader.CheckOrigin")
- }
-
- challengeKey := r.Header.Get("Sec-Websocket-Key")
- if challengeKey == "" {
- return u.returnError(w, r, http.StatusBadRequest, "websocket: not a websocket handshake: 'Sec-WebSocket-Key' header is missing or blank")
- }
-
- subprotocol := u.selectSubprotocol(r, responseHeader)
-
- // Negotiate PMCE
- var compress bool
- if u.EnableCompression {
- for _, ext := range parseExtensions(r.Header) {
- if ext[""] != "permessage-deflate" {
- continue
- }
- compress = true
- break
- }
- }
-
- h, ok := w.(http.Hijacker)
- if !ok {
- return u.returnError(w, r, http.StatusInternalServerError, "websocket: response does not implement http.Hijacker")
- }
- var brw *bufio.ReadWriter
- netConn, brw, err := h.Hijack()
- if err != nil {
- return u.returnError(w, r, http.StatusInternalServerError, err.Error())
- }
-
- if brw.Reader.Buffered() > 0 {
- netConn.Close()
- return nil, errors.New("websocket: client sent data before handshake is complete")
- }
-
- var br *bufio.Reader
- if u.ReadBufferSize == 0 && bufioReaderSize(netConn, brw.Reader) > 256 {
- // Reuse hijacked buffered reader as connection reader.
- br = brw.Reader
- }
-
- buf := bufioWriterBuffer(netConn, brw.Writer)
-
- var writeBuf []byte
- if u.WriteBufferPool == nil && u.WriteBufferSize == 0 && len(buf) >= maxFrameHeaderSize+256 {
- // Reuse hijacked write buffer as connection buffer.
- writeBuf = buf
- }
-
- c := newConn(netConn, true, u.ReadBufferSize, u.WriteBufferSize, u.WriteBufferPool, br, writeBuf)
- c.subprotocol = subprotocol
-
- if compress {
- c.newCompressionWriter = compressNoContextTakeover
- c.newDecompressionReader = decompressNoContextTakeover
- }
-
- // Use larger of hijacked buffer and connection write buffer for header.
- p := buf
- if len(c.writeBuf) > len(p) {
- p = c.writeBuf
- }
- p = p[:0]
-
- p = append(p, "HTTP/1.1 101 Switching Protocols\r\nUpgrade: websocket\r\nConnection: Upgrade\r\nSec-WebSocket-Accept: "...)
- p = append(p, computeAcceptKey(challengeKey)...)
- p = append(p, "\r\n"...)
- if c.subprotocol != "" {
- p = append(p, "Sec-WebSocket-Protocol: "...)
- p = append(p, c.subprotocol...)
- p = append(p, "\r\n"...)
- }
- if compress {
- p = append(p, "Sec-WebSocket-Extensions: permessage-deflate; server_no_context_takeover; client_no_context_takeover\r\n"...)
- }
- for k, vs := range responseHeader {
- if k == "Sec-Websocket-Protocol" {
- continue
- }
- for _, v := range vs {
- p = append(p, k...)
- p = append(p, ": "...)
- for i := 0; i < len(v); i++ {
- b := v[i]
- if b <= 31 {
- // prevent response splitting.
- b = ' '
- }
- p = append(p, b)
- }
- p = append(p, "\r\n"...)
- }
- }
- p = append(p, "\r\n"...)
-
- // Clear deadlines set by HTTP server.
- netConn.SetDeadline(time.Time{})
-
- if u.HandshakeTimeout > 0 {
- netConn.SetWriteDeadline(time.Now().Add(u.HandshakeTimeout))
- }
- if _, err = netConn.Write(p); err != nil {
- netConn.Close()
- return nil, err
- }
- if u.HandshakeTimeout > 0 {
- netConn.SetWriteDeadline(time.Time{})
- }
-
- return c, nil
-}
-
-// Upgrade upgrades the HTTP server connection to the WebSocket protocol.
-//
-// Deprecated: Use websocket.Upgrader instead.
-//
-// Upgrade does not perform origin checking. The application is responsible for
-// checking the Origin header before calling Upgrade. An example implementation
-// of the same origin policy check is:
-//
-// if req.Header.Get("Origin") != "http://"+req.Host {
-// http.Error(w, "Origin not allowed", http.StatusForbidden)
-// return
-// }
-//
-// If the endpoint supports subprotocols, then the application is responsible
-// for negotiating the protocol used on the connection. Use the Subprotocols()
-// function to get the subprotocols requested by the client. Use the
-// Sec-Websocket-Protocol response header to specify the subprotocol selected
-// by the application.
-//
-// The responseHeader is included in the response to the client's upgrade
-// request. Use the responseHeader to specify cookies (Set-Cookie) and the
-// negotiated subprotocol (Sec-Websocket-Protocol).
-//
-// The connection buffers IO to the underlying network connection. The
-// readBufSize and writeBufSize parameters specify the size of the buffers to
-// use. Messages can be larger than the buffers.
-//
-// If the request is not a valid WebSocket handshake, then Upgrade returns an
-// error of type HandshakeError. Applications should handle this error by
-// replying to the client with an HTTP error response.
-func Upgrade(w http.ResponseWriter, r *http.Request, responseHeader http.Header, readBufSize, writeBufSize int) (*Conn, error) {
- u := Upgrader{ReadBufferSize: readBufSize, WriteBufferSize: writeBufSize}
- u.Error = func(w http.ResponseWriter, r *http.Request, status int, reason error) {
- // don't return errors to maintain backwards compatibility
- }
- u.CheckOrigin = func(r *http.Request) bool {
- // allow all connections by default
- return true
- }
- return u.Upgrade(w, r, responseHeader)
-}
-
-// Subprotocols returns the subprotocols requested by the client in the
-// Sec-Websocket-Protocol header.
-func Subprotocols(r *http.Request) []string {
- h := strings.TrimSpace(r.Header.Get("Sec-Websocket-Protocol"))
- if h == "" {
- return nil
- }
- protocols := strings.Split(h, ",")
- for i := range protocols {
- protocols[i] = strings.TrimSpace(protocols[i])
- }
- return protocols
-}
-
-// IsWebSocketUpgrade returns true if the client requested upgrade to the
-// WebSocket protocol.
-func IsWebSocketUpgrade(r *http.Request) bool {
- return tokenListContainsValue(r.Header, "Connection", "upgrade") &&
- tokenListContainsValue(r.Header, "Upgrade", "websocket")
-}
-
-// bufioReaderSize size returns the size of a bufio.Reader.
-func bufioReaderSize(originalReader io.Reader, br *bufio.Reader) int {
- // This code assumes that peek on a reset reader returns
- // bufio.Reader.buf[:0].
- // TODO: Use bufio.Reader.Size() after Go 1.10
- br.Reset(originalReader)
- if p, err := br.Peek(0); err == nil {
- return cap(p)
- }
- return 0
-}
-
-// writeHook is an io.Writer that records the last slice passed to it vio
-// io.Writer.Write.
-type writeHook struct {
- p []byte
-}
-
-func (wh *writeHook) Write(p []byte) (int, error) {
- wh.p = p
- return len(p), nil
-}
-
-// bufioWriterBuffer grabs the buffer from a bufio.Writer.
-func bufioWriterBuffer(originalWriter io.Writer, bw *bufio.Writer) []byte {
- // This code assumes that bufio.Writer.buf[:1] is passed to the
- // bufio.Writer's underlying writer.
- var wh writeHook
- bw.Reset(&wh)
- bw.WriteByte(0)
- bw.Flush()
-
- bw.Reset(originalWriter)
-
- return wh.p[:cap(wh.p)]
-}
diff --git a/vendor/github.com/gorilla/websocket/tls_handshake.go b/vendor/github.com/gorilla/websocket/tls_handshake.go
deleted file mode 100644
index a62b68cc..00000000
--- a/vendor/github.com/gorilla/websocket/tls_handshake.go
+++ /dev/null
@@ -1,21 +0,0 @@
-//go:build go1.17
-// +build go1.17
-
-package websocket
-
-import (
- "context"
- "crypto/tls"
-)
-
-func doHandshake(ctx context.Context, tlsConn *tls.Conn, cfg *tls.Config) error {
- if err := tlsConn.HandshakeContext(ctx); err != nil {
- return err
- }
- if !cfg.InsecureSkipVerify {
- if err := tlsConn.VerifyHostname(cfg.ServerName); err != nil {
- return err
- }
- }
- return nil
-}
diff --git a/vendor/github.com/gorilla/websocket/tls_handshake_116.go b/vendor/github.com/gorilla/websocket/tls_handshake_116.go
deleted file mode 100644
index e1b2b44f..00000000
--- a/vendor/github.com/gorilla/websocket/tls_handshake_116.go
+++ /dev/null
@@ -1,21 +0,0 @@
-//go:build !go1.17
-// +build !go1.17
-
-package websocket
-
-import (
- "context"
- "crypto/tls"
-)
-
-func doHandshake(ctx context.Context, tlsConn *tls.Conn, cfg *tls.Config) error {
- if err := tlsConn.Handshake(); err != nil {
- return err
- }
- if !cfg.InsecureSkipVerify {
- if err := tlsConn.VerifyHostname(cfg.ServerName); err != nil {
- return err
- }
- }
- return nil
-}
diff --git a/vendor/github.com/gorilla/websocket/util.go b/vendor/github.com/gorilla/websocket/util.go
deleted file mode 100644
index 7bf2f66c..00000000
--- a/vendor/github.com/gorilla/websocket/util.go
+++ /dev/null
@@ -1,283 +0,0 @@
-// Copyright 2013 The Gorilla WebSocket Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package websocket
-
-import (
- "crypto/rand"
- "crypto/sha1"
- "encoding/base64"
- "io"
- "net/http"
- "strings"
- "unicode/utf8"
-)
-
-var keyGUID = []byte("258EAFA5-E914-47DA-95CA-C5AB0DC85B11")
-
-func computeAcceptKey(challengeKey string) string {
- h := sha1.New()
- h.Write([]byte(challengeKey))
- h.Write(keyGUID)
- return base64.StdEncoding.EncodeToString(h.Sum(nil))
-}
-
-func generateChallengeKey() (string, error) {
- p := make([]byte, 16)
- if _, err := io.ReadFull(rand.Reader, p); err != nil {
- return "", err
- }
- return base64.StdEncoding.EncodeToString(p), nil
-}
-
-// Token octets per RFC 2616.
-var isTokenOctet = [256]bool{
- '!': true,
- '#': true,
- '$': true,
- '%': true,
- '&': true,
- '\'': true,
- '*': true,
- '+': true,
- '-': true,
- '.': true,
- '0': true,
- '1': true,
- '2': true,
- '3': true,
- '4': true,
- '5': true,
- '6': true,
- '7': true,
- '8': true,
- '9': true,
- 'A': true,
- 'B': true,
- 'C': true,
- 'D': true,
- 'E': true,
- 'F': true,
- 'G': true,
- 'H': true,
- 'I': true,
- 'J': true,
- 'K': true,
- 'L': true,
- 'M': true,
- 'N': true,
- 'O': true,
- 'P': true,
- 'Q': true,
- 'R': true,
- 'S': true,
- 'T': true,
- 'U': true,
- 'W': true,
- 'V': true,
- 'X': true,
- 'Y': true,
- 'Z': true,
- '^': true,
- '_': true,
- '`': true,
- 'a': true,
- 'b': true,
- 'c': true,
- 'd': true,
- 'e': true,
- 'f': true,
- 'g': true,
- 'h': true,
- 'i': true,
- 'j': true,
- 'k': true,
- 'l': true,
- 'm': true,
- 'n': true,
- 'o': true,
- 'p': true,
- 'q': true,
- 'r': true,
- 's': true,
- 't': true,
- 'u': true,
- 'v': true,
- 'w': true,
- 'x': true,
- 'y': true,
- 'z': true,
- '|': true,
- '~': true,
-}
-
-// skipSpace returns a slice of the string s with all leading RFC 2616 linear
-// whitespace removed.
-func skipSpace(s string) (rest string) {
- i := 0
- for ; i < len(s); i++ {
- if b := s[i]; b != ' ' && b != '\t' {
- break
- }
- }
- return s[i:]
-}
-
-// nextToken returns the leading RFC 2616 token of s and the string following
-// the token.
-func nextToken(s string) (token, rest string) {
- i := 0
- for ; i < len(s); i++ {
- if !isTokenOctet[s[i]] {
- break
- }
- }
- return s[:i], s[i:]
-}
-
-// nextTokenOrQuoted returns the leading token or quoted string per RFC 2616
-// and the string following the token or quoted string.
-func nextTokenOrQuoted(s string) (value string, rest string) {
- if !strings.HasPrefix(s, "\"") {
- return nextToken(s)
- }
- s = s[1:]
- for i := 0; i < len(s); i++ {
- switch s[i] {
- case '"':
- return s[:i], s[i+1:]
- case '\\':
- p := make([]byte, len(s)-1)
- j := copy(p, s[:i])
- escape := true
- for i = i + 1; i < len(s); i++ {
- b := s[i]
- switch {
- case escape:
- escape = false
- p[j] = b
- j++
- case b == '\\':
- escape = true
- case b == '"':
- return string(p[:j]), s[i+1:]
- default:
- p[j] = b
- j++
- }
- }
- return "", ""
- }
- }
- return "", ""
-}
-
-// equalASCIIFold returns true if s is equal to t with ASCII case folding as
-// defined in RFC 4790.
-func equalASCIIFold(s, t string) bool {
- for s != "" && t != "" {
- sr, size := utf8.DecodeRuneInString(s)
- s = s[size:]
- tr, size := utf8.DecodeRuneInString(t)
- t = t[size:]
- if sr == tr {
- continue
- }
- if 'A' <= sr && sr <= 'Z' {
- sr = sr + 'a' - 'A'
- }
- if 'A' <= tr && tr <= 'Z' {
- tr = tr + 'a' - 'A'
- }
- if sr != tr {
- return false
- }
- }
- return s == t
-}
-
-// tokenListContainsValue returns true if the 1#token header with the given
-// name contains a token equal to value with ASCII case folding.
-func tokenListContainsValue(header http.Header, name string, value string) bool {
-headers:
- for _, s := range header[name] {
- for {
- var t string
- t, s = nextToken(skipSpace(s))
- if t == "" {
- continue headers
- }
- s = skipSpace(s)
- if s != "" && s[0] != ',' {
- continue headers
- }
- if equalASCIIFold(t, value) {
- return true
- }
- if s == "" {
- continue headers
- }
- s = s[1:]
- }
- }
- return false
-}
-
-// parseExtensions parses WebSocket extensions from a header.
-func parseExtensions(header http.Header) []map[string]string {
- // From RFC 6455:
- //
- // Sec-WebSocket-Extensions = extension-list
- // extension-list = 1#extension
- // extension = extension-token *( ";" extension-param )
- // extension-token = registered-token
- // registered-token = token
- // extension-param = token [ "=" (token | quoted-string) ]
- // ;When using the quoted-string syntax variant, the value
- // ;after quoted-string unescaping MUST conform to the
- // ;'token' ABNF.
-
- var result []map[string]string
-headers:
- for _, s := range header["Sec-Websocket-Extensions"] {
- for {
- var t string
- t, s = nextToken(skipSpace(s))
- if t == "" {
- continue headers
- }
- ext := map[string]string{"": t}
- for {
- s = skipSpace(s)
- if !strings.HasPrefix(s, ";") {
- break
- }
- var k string
- k, s = nextToken(skipSpace(s[1:]))
- if k == "" {
- continue headers
- }
- s = skipSpace(s)
- var v string
- if strings.HasPrefix(s, "=") {
- v, s = nextTokenOrQuoted(skipSpace(s[1:]))
- s = skipSpace(s)
- }
- if s != "" && s[0] != ',' && s[0] != ';' {
- continue headers
- }
- ext[k] = v
- }
- if s != "" && s[0] != ',' {
- continue headers
- }
- result = append(result, ext)
- if s == "" {
- continue headers
- }
- s = s[1:]
- }
- }
- return result
-}
diff --git a/vendor/github.com/gorilla/websocket/x_net_proxy.go b/vendor/github.com/gorilla/websocket/x_net_proxy.go
deleted file mode 100644
index 2e668f6b..00000000
--- a/vendor/github.com/gorilla/websocket/x_net_proxy.go
+++ /dev/null
@@ -1,473 +0,0 @@
-// Code generated by golang.org/x/tools/cmd/bundle. DO NOT EDIT.
-//go:generate bundle -o x_net_proxy.go golang.org/x/net/proxy
-
-// Package proxy provides support for a variety of protocols to proxy network
-// data.
-//
-
-package websocket
-
-import (
- "errors"
- "io"
- "net"
- "net/url"
- "os"
- "strconv"
- "strings"
- "sync"
-)
-
-type proxy_direct struct{}
-
-// Direct is a direct proxy: one that makes network connections directly.
-var proxy_Direct = proxy_direct{}
-
-func (proxy_direct) Dial(network, addr string) (net.Conn, error) {
- return net.Dial(network, addr)
-}
-
-// A PerHost directs connections to a default Dialer unless the host name
-// requested matches one of a number of exceptions.
-type proxy_PerHost struct {
- def, bypass proxy_Dialer
-
- bypassNetworks []*net.IPNet
- bypassIPs []net.IP
- bypassZones []string
- bypassHosts []string
-}
-
-// NewPerHost returns a PerHost Dialer that directs connections to either
-// defaultDialer or bypass, depending on whether the connection matches one of
-// the configured rules.
-func proxy_NewPerHost(defaultDialer, bypass proxy_Dialer) *proxy_PerHost {
- return &proxy_PerHost{
- def: defaultDialer,
- bypass: bypass,
- }
-}
-
-// Dial connects to the address addr on the given network through either
-// defaultDialer or bypass.
-func (p *proxy_PerHost) Dial(network, addr string) (c net.Conn, err error) {
- host, _, err := net.SplitHostPort(addr)
- if err != nil {
- return nil, err
- }
-
- return p.dialerForRequest(host).Dial(network, addr)
-}
-
-func (p *proxy_PerHost) dialerForRequest(host string) proxy_Dialer {
- if ip := net.ParseIP(host); ip != nil {
- for _, net := range p.bypassNetworks {
- if net.Contains(ip) {
- return p.bypass
- }
- }
- for _, bypassIP := range p.bypassIPs {
- if bypassIP.Equal(ip) {
- return p.bypass
- }
- }
- return p.def
- }
-
- for _, zone := range p.bypassZones {
- if strings.HasSuffix(host, zone) {
- return p.bypass
- }
- if host == zone[1:] {
- // For a zone ".example.com", we match "example.com"
- // too.
- return p.bypass
- }
- }
- for _, bypassHost := range p.bypassHosts {
- if bypassHost == host {
- return p.bypass
- }
- }
- return p.def
-}
-
-// AddFromString parses a string that contains comma-separated values
-// specifying hosts that should use the bypass proxy. Each value is either an
-// IP address, a CIDR range, a zone (*.example.com) or a host name
-// (localhost). A best effort is made to parse the string and errors are
-// ignored.
-func (p *proxy_PerHost) AddFromString(s string) {
- hosts := strings.Split(s, ",")
- for _, host := range hosts {
- host = strings.TrimSpace(host)
- if len(host) == 0 {
- continue
- }
- if strings.Contains(host, "/") {
- // We assume that it's a CIDR address like 127.0.0.0/8
- if _, net, err := net.ParseCIDR(host); err == nil {
- p.AddNetwork(net)
- }
- continue
- }
- if ip := net.ParseIP(host); ip != nil {
- p.AddIP(ip)
- continue
- }
- if strings.HasPrefix(host, "*.") {
- p.AddZone(host[1:])
- continue
- }
- p.AddHost(host)
- }
-}
-
-// AddIP specifies an IP address that will use the bypass proxy. Note that
-// this will only take effect if a literal IP address is dialed. A connection
-// to a named host will never match an IP.
-func (p *proxy_PerHost) AddIP(ip net.IP) {
- p.bypassIPs = append(p.bypassIPs, ip)
-}
-
-// AddNetwork specifies an IP range that will use the bypass proxy. Note that
-// this will only take effect if a literal IP address is dialed. A connection
-// to a named host will never match.
-func (p *proxy_PerHost) AddNetwork(net *net.IPNet) {
- p.bypassNetworks = append(p.bypassNetworks, net)
-}
-
-// AddZone specifies a DNS suffix that will use the bypass proxy. A zone of
-// "example.com" matches "example.com" and all of its subdomains.
-func (p *proxy_PerHost) AddZone(zone string) {
- if strings.HasSuffix(zone, ".") {
- zone = zone[:len(zone)-1]
- }
- if !strings.HasPrefix(zone, ".") {
- zone = "." + zone
- }
- p.bypassZones = append(p.bypassZones, zone)
-}
-
-// AddHost specifies a host name that will use the bypass proxy.
-func (p *proxy_PerHost) AddHost(host string) {
- if strings.HasSuffix(host, ".") {
- host = host[:len(host)-1]
- }
- p.bypassHosts = append(p.bypassHosts, host)
-}
-
-// A Dialer is a means to establish a connection.
-type proxy_Dialer interface {
- // Dial connects to the given address via the proxy.
- Dial(network, addr string) (c net.Conn, err error)
-}
-
-// Auth contains authentication parameters that specific Dialers may require.
-type proxy_Auth struct {
- User, Password string
-}
-
-// FromEnvironment returns the dialer specified by the proxy related variables in
-// the environment.
-func proxy_FromEnvironment() proxy_Dialer {
- allProxy := proxy_allProxyEnv.Get()
- if len(allProxy) == 0 {
- return proxy_Direct
- }
-
- proxyURL, err := url.Parse(allProxy)
- if err != nil {
- return proxy_Direct
- }
- proxy, err := proxy_FromURL(proxyURL, proxy_Direct)
- if err != nil {
- return proxy_Direct
- }
-
- noProxy := proxy_noProxyEnv.Get()
- if len(noProxy) == 0 {
- return proxy
- }
-
- perHost := proxy_NewPerHost(proxy, proxy_Direct)
- perHost.AddFromString(noProxy)
- return perHost
-}
-
-// proxySchemes is a map from URL schemes to a function that creates a Dialer
-// from a URL with such a scheme.
-var proxy_proxySchemes map[string]func(*url.URL, proxy_Dialer) (proxy_Dialer, error)
-
-// RegisterDialerType takes a URL scheme and a function to generate Dialers from
-// a URL with that scheme and a forwarding Dialer. Registered schemes are used
-// by FromURL.
-func proxy_RegisterDialerType(scheme string, f func(*url.URL, proxy_Dialer) (proxy_Dialer, error)) {
- if proxy_proxySchemes == nil {
- proxy_proxySchemes = make(map[string]func(*url.URL, proxy_Dialer) (proxy_Dialer, error))
- }
- proxy_proxySchemes[scheme] = f
-}
-
-// FromURL returns a Dialer given a URL specification and an underlying
-// Dialer for it to make network requests.
-func proxy_FromURL(u *url.URL, forward proxy_Dialer) (proxy_Dialer, error) {
- var auth *proxy_Auth
- if u.User != nil {
- auth = new(proxy_Auth)
- auth.User = u.User.Username()
- if p, ok := u.User.Password(); ok {
- auth.Password = p
- }
- }
-
- switch u.Scheme {
- case "socks5":
- return proxy_SOCKS5("tcp", u.Host, auth, forward)
- }
-
- // If the scheme doesn't match any of the built-in schemes, see if it
- // was registered by another package.
- if proxy_proxySchemes != nil {
- if f, ok := proxy_proxySchemes[u.Scheme]; ok {
- return f(u, forward)
- }
- }
-
- return nil, errors.New("proxy: unknown scheme: " + u.Scheme)
-}
-
-var (
- proxy_allProxyEnv = &proxy_envOnce{
- names: []string{"ALL_PROXY", "all_proxy"},
- }
- proxy_noProxyEnv = &proxy_envOnce{
- names: []string{"NO_PROXY", "no_proxy"},
- }
-)
-
-// envOnce looks up an environment variable (optionally by multiple
-// names) once. It mitigates expensive lookups on some platforms
-// (e.g. Windows).
-// (Borrowed from net/http/transport.go)
-type proxy_envOnce struct {
- names []string
- once sync.Once
- val string
-}
-
-func (e *proxy_envOnce) Get() string {
- e.once.Do(e.init)
- return e.val
-}
-
-func (e *proxy_envOnce) init() {
- for _, n := range e.names {
- e.val = os.Getenv(n)
- if e.val != "" {
- return
- }
- }
-}
-
-// SOCKS5 returns a Dialer that makes SOCKSv5 connections to the given address
-// with an optional username and password. See RFC 1928 and RFC 1929.
-func proxy_SOCKS5(network, addr string, auth *proxy_Auth, forward proxy_Dialer) (proxy_Dialer, error) {
- s := &proxy_socks5{
- network: network,
- addr: addr,
- forward: forward,
- }
- if auth != nil {
- s.user = auth.User
- s.password = auth.Password
- }
-
- return s, nil
-}
-
-type proxy_socks5 struct {
- user, password string
- network, addr string
- forward proxy_Dialer
-}
-
-const proxy_socks5Version = 5
-
-const (
- proxy_socks5AuthNone = 0
- proxy_socks5AuthPassword = 2
-)
-
-const proxy_socks5Connect = 1
-
-const (
- proxy_socks5IP4 = 1
- proxy_socks5Domain = 3
- proxy_socks5IP6 = 4
-)
-
-var proxy_socks5Errors = []string{
- "",
- "general failure",
- "connection forbidden",
- "network unreachable",
- "host unreachable",
- "connection refused",
- "TTL expired",
- "command not supported",
- "address type not supported",
-}
-
-// Dial connects to the address addr on the given network via the SOCKS5 proxy.
-func (s *proxy_socks5) Dial(network, addr string) (net.Conn, error) {
- switch network {
- case "tcp", "tcp6", "tcp4":
- default:
- return nil, errors.New("proxy: no support for SOCKS5 proxy connections of type " + network)
- }
-
- conn, err := s.forward.Dial(s.network, s.addr)
- if err != nil {
- return nil, err
- }
- if err := s.connect(conn, addr); err != nil {
- conn.Close()
- return nil, err
- }
- return conn, nil
-}
-
-// connect takes an existing connection to a socks5 proxy server,
-// and commands the server to extend that connection to target,
-// which must be a canonical address with a host and port.
-func (s *proxy_socks5) connect(conn net.Conn, target string) error {
- host, portStr, err := net.SplitHostPort(target)
- if err != nil {
- return err
- }
-
- port, err := strconv.Atoi(portStr)
- if err != nil {
- return errors.New("proxy: failed to parse port number: " + portStr)
- }
- if port < 1 || port > 0xffff {
- return errors.New("proxy: port number out of range: " + portStr)
- }
-
- // the size here is just an estimate
- buf := make([]byte, 0, 6+len(host))
-
- buf = append(buf, proxy_socks5Version)
- if len(s.user) > 0 && len(s.user) < 256 && len(s.password) < 256 {
- buf = append(buf, 2 /* num auth methods */, proxy_socks5AuthNone, proxy_socks5AuthPassword)
- } else {
- buf = append(buf, 1 /* num auth methods */, proxy_socks5AuthNone)
- }
-
- if _, err := conn.Write(buf); err != nil {
- return errors.New("proxy: failed to write greeting to SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- if _, err := io.ReadFull(conn, buf[:2]); err != nil {
- return errors.New("proxy: failed to read greeting from SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
- if buf[0] != 5 {
- return errors.New("proxy: SOCKS5 proxy at " + s.addr + " has unexpected version " + strconv.Itoa(int(buf[0])))
- }
- if buf[1] == 0xff {
- return errors.New("proxy: SOCKS5 proxy at " + s.addr + " requires authentication")
- }
-
- // See RFC 1929
- if buf[1] == proxy_socks5AuthPassword {
- buf = buf[:0]
- buf = append(buf, 1 /* password protocol version */)
- buf = append(buf, uint8(len(s.user)))
- buf = append(buf, s.user...)
- buf = append(buf, uint8(len(s.password)))
- buf = append(buf, s.password...)
-
- if _, err := conn.Write(buf); err != nil {
- return errors.New("proxy: failed to write authentication request to SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- if _, err := io.ReadFull(conn, buf[:2]); err != nil {
- return errors.New("proxy: failed to read authentication reply from SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- if buf[1] != 0 {
- return errors.New("proxy: SOCKS5 proxy at " + s.addr + " rejected username/password")
- }
- }
-
- buf = buf[:0]
- buf = append(buf, proxy_socks5Version, proxy_socks5Connect, 0 /* reserved */)
-
- if ip := net.ParseIP(host); ip != nil {
- if ip4 := ip.To4(); ip4 != nil {
- buf = append(buf, proxy_socks5IP4)
- ip = ip4
- } else {
- buf = append(buf, proxy_socks5IP6)
- }
- buf = append(buf, ip...)
- } else {
- if len(host) > 255 {
- return errors.New("proxy: destination host name too long: " + host)
- }
- buf = append(buf, proxy_socks5Domain)
- buf = append(buf, byte(len(host)))
- buf = append(buf, host...)
- }
- buf = append(buf, byte(port>>8), byte(port))
-
- if _, err := conn.Write(buf); err != nil {
- return errors.New("proxy: failed to write connect request to SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- if _, err := io.ReadFull(conn, buf[:4]); err != nil {
- return errors.New("proxy: failed to read connect reply from SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- failure := "unknown error"
- if int(buf[1]) < len(proxy_socks5Errors) {
- failure = proxy_socks5Errors[buf[1]]
- }
-
- if len(failure) > 0 {
- return errors.New("proxy: SOCKS5 proxy at " + s.addr + " failed to connect: " + failure)
- }
-
- bytesToDiscard := 0
- switch buf[3] {
- case proxy_socks5IP4:
- bytesToDiscard = net.IPv4len
- case proxy_socks5IP6:
- bytesToDiscard = net.IPv6len
- case proxy_socks5Domain:
- _, err := io.ReadFull(conn, buf[:1])
- if err != nil {
- return errors.New("proxy: failed to read domain length from SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
- bytesToDiscard = int(buf[0])
- default:
- return errors.New("proxy: got unknown address type " + strconv.Itoa(int(buf[3])) + " from SOCKS5 proxy at " + s.addr)
- }
-
- if cap(buf) < bytesToDiscard {
- buf = make([]byte, bytesToDiscard)
- } else {
- buf = buf[:bytesToDiscard]
- }
- if _, err := io.ReadFull(conn, buf); err != nil {
- return errors.New("proxy: failed to read address from SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- // Also need to discard the port number
- if _, err := io.ReadFull(conn, buf[:2]); err != nil {
- return errors.New("proxy: failed to read port from SOCKS5 proxy at " + s.addr + ": " + err.Error())
- }
-
- return nil
-}
diff --git a/vendor/github.com/hashicorp/errwrap/LICENSE b/vendor/github.com/hashicorp/errwrap/LICENSE
deleted file mode 100644
index c33dcc7c..00000000
--- a/vendor/github.com/hashicorp/errwrap/LICENSE
+++ /dev/null
@@ -1,354 +0,0 @@
-Mozilla Public License, version 2.0
-
-1. Definitions
-
-1.1. “Contributorâ€
-
- means each individual or legal entity that creates, contributes to the
- creation of, or owns Covered Software.
-
-1.2. “Contributor Versionâ€
-
- means the combination of the Contributions of others (if any) used by a
- Contributor and that particular Contributor’s Contribution.
-
-1.3. “Contributionâ€
-
- means Covered Software of a particular Contributor.
-
-1.4. “Covered Softwareâ€
-
- means Source Code Form to which the initial Contributor has attached the
- notice in Exhibit A, the Executable Form of such Source Code Form, and
- Modifications of such Source Code Form, in each case including portions
- thereof.
-
-1.5. “Incompatible With Secondary Licensesâ€
- means
-
- a. that the initial Contributor has attached the notice described in
- Exhibit B to the Covered Software; or
-
- b. that the Covered Software was made available under the terms of version
- 1.1 or earlier of the License, but not also under the terms of a
- Secondary License.
-
-1.6. “Executable Formâ€
-
- means any form of the work other than Source Code Form.
-
-1.7. “Larger Workâ€
-
- means a work that combines Covered Software with other material, in a separate
- file or files, that is not Covered Software.
-
-1.8. “Licenseâ€
-
- means this document.
-
-1.9. “Licensableâ€
-
- means having the right to grant, to the maximum extent possible, whether at the
- time of the initial grant or subsequently, any and all of the rights conveyed by
- this License.
-
-1.10. “Modificationsâ€
-
- means any of the following:
-
- a. any file in Source Code Form that results from an addition to, deletion
- from, or modification of the contents of Covered Software; or
-
- b. any new file in Source Code Form that contains any Covered Software.
-
-1.11. “Patent Claims†of a Contributor
-
- means any patent claim(s), including without limitation, method, process,
- and apparatus claims, in any patent Licensable by such Contributor that
- would be infringed, but for the grant of the License, by the making,
- using, selling, offering for sale, having made, import, or transfer of
- either its Contributions or its Contributor Version.
-
-1.12. “Secondary Licenseâ€
-
- means either the GNU General Public License, Version 2.0, the GNU Lesser
- General Public License, Version 2.1, the GNU Affero General Public
- License, Version 3.0, or any later versions of those licenses.
-
-1.13. “Source Code Formâ€
-
- means the form of the work preferred for making modifications.
-
-1.14. “You†(or “Yourâ€)
-
- means an individual or a legal entity exercising rights under this
- License. For legal entities, “You†includes any entity that controls, is
- controlled by, or is under common control with You. For purposes of this
- definition, “control†means (a) the power, direct or indirect, to cause
- the direction or management of such entity, whether by contract or
- otherwise, or (b) ownership of more than fifty percent (50%) of the
- outstanding shares or beneficial ownership of such entity.
-
-
-2. License Grants and Conditions
-
-2.1. Grants
-
- Each Contributor hereby grants You a world-wide, royalty-free,
- non-exclusive license:
-
- a. under intellectual property rights (other than patent or trademark)
- Licensable by such Contributor to use, reproduce, make available,
- modify, display, perform, distribute, and otherwise exploit its
- Contributions, either on an unmodified basis, with Modifications, or as
- part of a Larger Work; and
-
- b. under Patent Claims of such Contributor to make, use, sell, offer for
- sale, have made, import, and otherwise transfer either its Contributions
- or its Contributor Version.
-
-2.2. Effective Date
-
- The licenses granted in Section 2.1 with respect to any Contribution become
- effective for each Contribution on the date the Contributor first distributes
- such Contribution.
-
-2.3. Limitations on Grant Scope
-
- The licenses granted in this Section 2 are the only rights granted under this
- License. No additional rights or licenses will be implied from the distribution
- or licensing of Covered Software under this License. Notwithstanding Section
- 2.1(b) above, no patent license is granted by a Contributor:
-
- a. for any code that a Contributor has removed from Covered Software; or
-
- b. for infringements caused by: (i) Your and any other third party’s
- modifications of Covered Software, or (ii) the combination of its
- Contributions with other software (except as part of its Contributor
- Version); or
-
- c. under Patent Claims infringed by Covered Software in the absence of its
- Contributions.
-
- This License does not grant any rights in the trademarks, service marks, or
- logos of any Contributor (except as may be necessary to comply with the
- notice requirements in Section 3.4).
-
-2.4. Subsequent Licenses
-
- No Contributor makes additional grants as a result of Your choice to
- distribute the Covered Software under a subsequent version of this License
- (see Section 10.2) or under the terms of a Secondary License (if permitted
- under the terms of Section 3.3).
-
-2.5. Representation
-
- Each Contributor represents that the Contributor believes its Contributions
- are its original creation(s) or it has sufficient rights to grant the
- rights to its Contributions conveyed by this License.
-
-2.6. Fair Use
-
- This License is not intended to limit any rights You have under applicable
- copyright doctrines of fair use, fair dealing, or other equivalents.
-
-2.7. Conditions
-
- Sections 3.1, 3.2, 3.3, and 3.4 are conditions of the licenses granted in
- Section 2.1.
-
-
-3. Responsibilities
-
-3.1. Distribution of Source Form
-
- All distribution of Covered Software in Source Code Form, including any
- Modifications that You create or to which You contribute, must be under the
- terms of this License. You must inform recipients that the Source Code Form
- of the Covered Software is governed by the terms of this License, and how
- they can obtain a copy of this License. You may not attempt to alter or
- restrict the recipients’ rights in the Source Code Form.
-
-3.2. Distribution of Executable Form
-
- If You distribute Covered Software in Executable Form then:
-
- a. such Covered Software must also be made available in Source Code Form,
- as described in Section 3.1, and You must inform recipients of the
- Executable Form how they can obtain a copy of such Source Code Form by
- reasonable means in a timely manner, at a charge no more than the cost
- of distribution to the recipient; and
-
- b. You may distribute such Executable Form under the terms of this License,
- or sublicense it under different terms, provided that the license for
- the Executable Form does not attempt to limit or alter the recipients’
- rights in the Source Code Form under this License.
-
-3.3. Distribution of a Larger Work
-
- You may create and distribute a Larger Work under terms of Your choice,
- provided that You also comply with the requirements of this License for the
- Covered Software. If the Larger Work is a combination of Covered Software
- with a work governed by one or more Secondary Licenses, and the Covered
- Software is not Incompatible With Secondary Licenses, this License permits
- You to additionally distribute such Covered Software under the terms of
- such Secondary License(s), so that the recipient of the Larger Work may, at
- their option, further distribute the Covered Software under the terms of
- either this License or such Secondary License(s).
-
-3.4. Notices
-
- You may not remove or alter the substance of any license notices (including
- copyright notices, patent notices, disclaimers of warranty, or limitations
- of liability) contained within the Source Code Form of the Covered
- Software, except that You may alter any license notices to the extent
- required to remedy known factual inaccuracies.
-
-3.5. Application of Additional Terms
-
- You may choose to offer, and to charge a fee for, warranty, support,
- indemnity or liability obligations to one or more recipients of Covered
- Software. However, You may do so only on Your own behalf, and not on behalf
- of any Contributor. You must make it absolutely clear that any such
- warranty, support, indemnity, or liability obligation is offered by You
- alone, and You hereby agree to indemnify every Contributor for any
- liability incurred by such Contributor as a result of warranty, support,
- indemnity or liability terms You offer. You may include additional
- disclaimers of warranty and limitations of liability specific to any
- jurisdiction.
-
-4. Inability to Comply Due to Statute or Regulation
-
- If it is impossible for You to comply with any of the terms of this License
- with respect to some or all of the Covered Software due to statute, judicial
- order, or regulation then You must: (a) comply with the terms of this License
- to the maximum extent possible; and (b) describe the limitations and the code
- they affect. Such description must be placed in a text file included with all
- distributions of the Covered Software under this License. Except to the
- extent prohibited by statute or regulation, such description must be
- sufficiently detailed for a recipient of ordinary skill to be able to
- understand it.
-
-5. Termination
-
-5.1. The rights granted under this License will terminate automatically if You
- fail to comply with any of its terms. However, if You become compliant,
- then the rights granted under this License from a particular Contributor
- are reinstated (a) provisionally, unless and until such Contributor
- explicitly and finally terminates Your grants, and (b) on an ongoing basis,
- if such Contributor fails to notify You of the non-compliance by some
- reasonable means prior to 60 days after You have come back into compliance.
- Moreover, Your grants from a particular Contributor are reinstated on an
- ongoing basis if such Contributor notifies You of the non-compliance by
- some reasonable means, this is the first time You have received notice of
- non-compliance with this License from such Contributor, and You become
- compliant prior to 30 days after Your receipt of the notice.
-
-5.2. If You initiate litigation against any entity by asserting a patent
- infringement claim (excluding declaratory judgment actions, counter-claims,
- and cross-claims) alleging that a Contributor Version directly or
- indirectly infringes any patent, then the rights granted to You by any and
- all Contributors for the Covered Software under Section 2.1 of this License
- shall terminate.
-
-5.3. In the event of termination under Sections 5.1 or 5.2 above, all end user
- license agreements (excluding distributors and resellers) which have been
- validly granted by You or Your distributors under this License prior to
- termination shall survive termination.
-
-6. Disclaimer of Warranty
-
- Covered Software is provided under this License on an “as is†basis, without
- warranty of any kind, either expressed, implied, or statutory, including,
- without limitation, warranties that the Covered Software is free of defects,
- merchantable, fit for a particular purpose or non-infringing. The entire
- risk as to the quality and performance of the Covered Software is with You.
- Should any Covered Software prove defective in any respect, You (not any
- Contributor) assume the cost of any necessary servicing, repair, or
- correction. This disclaimer of warranty constitutes an essential part of this
- License. No use of any Covered Software is authorized under this License
- except under this disclaimer.
-
-7. Limitation of Liability
-
- Under no circumstances and under no legal theory, whether tort (including
- negligence), contract, or otherwise, shall any Contributor, or anyone who
- distributes Covered Software as permitted above, be liable to You for any
- direct, indirect, special, incidental, or consequential damages of any
- character including, without limitation, damages for lost profits, loss of
- goodwill, work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses, even if such party shall have been
- informed of the possibility of such damages. This limitation of liability
- shall not apply to liability for death or personal injury resulting from such
- party’s negligence to the extent applicable law prohibits such limitation.
- Some jurisdictions do not allow the exclusion or limitation of incidental or
- consequential damages, so this exclusion and limitation may not apply to You.
-
-8. Litigation
-
- Any litigation relating to this License may be brought only in the courts of
- a jurisdiction where the defendant maintains its principal place of business
- and such litigation shall be governed by laws of that jurisdiction, without
- reference to its conflict-of-law provisions. Nothing in this Section shall
- prevent a party’s ability to bring cross-claims or counter-claims.
-
-9. Miscellaneous
-
- This License represents the complete agreement concerning the subject matter
- hereof. If any provision of this License is held to be unenforceable, such
- provision shall be reformed only to the extent necessary to make it
- enforceable. Any law or regulation which provides that the language of a
- contract shall be construed against the drafter shall not be used to construe
- this License against a Contributor.
-
-
-10. Versions of the License
-
-10.1. New Versions
-
- Mozilla Foundation is the license steward. Except as provided in Section
- 10.3, no one other than the license steward has the right to modify or
- publish new versions of this License. Each version will be given a
- distinguishing version number.
-
-10.2. Effect of New Versions
-
- You may distribute the Covered Software under the terms of the version of
- the License under which You originally received the Covered Software, or
- under the terms of any subsequent version published by the license
- steward.
-
-10.3. Modified Versions
-
- If you create software not governed by this License, and you want to
- create a new license for such software, you may create and use a modified
- version of this License if you rename the license and remove any
- references to the name of the license steward (except to note that such
- modified license differs from this License).
-
-10.4. Distributing Source Code Form that is Incompatible With Secondary Licenses
- If You choose to distribute Source Code Form that is Incompatible With
- Secondary Licenses under the terms of this version of the License, the
- notice described in Exhibit B of this License must be attached.
-
-Exhibit A - Source Code Form License Notice
-
- This Source Code Form is subject to the
- terms of the Mozilla Public License, v.
- 2.0. If a copy of the MPL was not
- distributed with this file, You can
- obtain one at
- http://mozilla.org/MPL/2.0/.
-
-If it is not possible or desirable to put the notice in a particular file, then
-You may include the notice in a location (such as a LICENSE file in a relevant
-directory) where a recipient would be likely to look for such a notice.
-
-You may add additional accurate notices of copyright ownership.
-
-Exhibit B - “Incompatible With Secondary Licenses†Notice
-
- This Source Code Form is “Incompatible
- With Secondary Licensesâ€, as defined by
- the Mozilla Public License, v. 2.0.
-
diff --git a/vendor/github.com/hashicorp/errwrap/README.md b/vendor/github.com/hashicorp/errwrap/README.md
deleted file mode 100644
index 444df08f..00000000
--- a/vendor/github.com/hashicorp/errwrap/README.md
+++ /dev/null
@@ -1,89 +0,0 @@
-# errwrap
-
-`errwrap` is a package for Go that formalizes the pattern of wrapping errors
-and checking if an error contains another error.
-
-There is a common pattern in Go of taking a returned `error` value and
-then wrapping it (such as with `fmt.Errorf`) before returning it. The problem
-with this pattern is that you completely lose the original `error` structure.
-
-Arguably the _correct_ approach is that you should make a custom structure
-implementing the `error` interface, and have the original error as a field
-on that structure, such [as this example](http://golang.org/pkg/os/#PathError).
-This is a good approach, but you have to know the entire chain of possible
-rewrapping that happens, when you might just care about one.
-
-`errwrap` formalizes this pattern (it doesn't matter what approach you use
-above) by giving a single interface for wrapping errors, checking if a specific
-error is wrapped, and extracting that error.
-
-## Installation and Docs
-
-Install using `go get github.com/hashicorp/errwrap`.
-
-Full documentation is available at
-http://godoc.org/github.com/hashicorp/errwrap
-
-## Usage
-
-#### Basic Usage
-
-Below is a very basic example of its usage:
-
-```go
-// A function that always returns an error, but wraps it, like a real
-// function might.
-func tryOpen() error {
- _, err := os.Open("/i/dont/exist")
- if err != nil {
- return errwrap.Wrapf("Doesn't exist: {{err}}", err)
- }
-
- return nil
-}
-
-func main() {
- err := tryOpen()
-
- // We can use the Contains helpers to check if an error contains
- // another error. It is safe to do this with a nil error, or with
- // an error that doesn't even use the errwrap package.
- if errwrap.Contains(err, "does not exist") {
- // Do something
- }
- if errwrap.ContainsType(err, new(os.PathError)) {
- // Do something
- }
-
- // Or we can use the associated `Get` functions to just extract
- // a specific error. This would return nil if that specific error doesn't
- // exist.
- perr := errwrap.GetType(err, new(os.PathError))
-}
-```
-
-#### Custom Types
-
-If you're already making custom types that properly wrap errors, then
-you can get all the functionality of `errwraps.Contains` and such by
-implementing the `Wrapper` interface with just one function. Example:
-
-```go
-type AppError {
- Code ErrorCode
- Err error
-}
-
-func (e *AppError) WrappedErrors() []error {
- return []error{e.Err}
-}
-```
-
-Now this works:
-
-```go
-err := &AppError{Err: fmt.Errorf("an error")}
-if errwrap.ContainsType(err, fmt.Errorf("")) {
- // This will work!
-}
-```
diff --git a/vendor/github.com/hashicorp/errwrap/errwrap.go b/vendor/github.com/hashicorp/errwrap/errwrap.go
deleted file mode 100644
index a733bef1..00000000
--- a/vendor/github.com/hashicorp/errwrap/errwrap.go
+++ /dev/null
@@ -1,169 +0,0 @@
-// Package errwrap implements methods to formalize error wrapping in Go.
-//
-// All of the top-level functions that take an `error` are built to be able
-// to take any error, not just wrapped errors. This allows you to use errwrap
-// without having to type-check and type-cast everywhere.
-package errwrap
-
-import (
- "errors"
- "reflect"
- "strings"
-)
-
-// WalkFunc is the callback called for Walk.
-type WalkFunc func(error)
-
-// Wrapper is an interface that can be implemented by custom types to
-// have all the Contains, Get, etc. functions in errwrap work.
-//
-// When Walk reaches a Wrapper, it will call the callback for every
-// wrapped error in addition to the wrapper itself. Since all the top-level
-// functions in errwrap use Walk, this means that all those functions work
-// with your custom type.
-type Wrapper interface {
- WrappedErrors() []error
-}
-
-// Wrap defines that outer wraps inner, returning an error type that
-// can be cleanly used with the other methods in this package, such as
-// Contains, GetAll, etc.
-//
-// This function won't modify the error message at all (the outer message
-// will be used).
-func Wrap(outer, inner error) error {
- return &wrappedError{
- Outer: outer,
- Inner: inner,
- }
-}
-
-// Wrapf wraps an error with a formatting message. This is similar to using
-// `fmt.Errorf` to wrap an error. If you're using `fmt.Errorf` to wrap
-// errors, you should replace it with this.
-//
-// format is the format of the error message. The string '{{err}}' will
-// be replaced with the original error message.
-func Wrapf(format string, err error) error {
- outerMsg := ""
- if err != nil {
- outerMsg = err.Error()
- }
-
- outer := errors.New(strings.Replace(
- format, "{{err}}", outerMsg, -1))
-
- return Wrap(outer, err)
-}
-
-// Contains checks if the given error contains an error with the
-// message msg. If err is not a wrapped error, this will always return
-// false unless the error itself happens to match this msg.
-func Contains(err error, msg string) bool {
- return len(GetAll(err, msg)) > 0
-}
-
-// ContainsType checks if the given error contains an error with
-// the same concrete type as v. If err is not a wrapped error, this will
-// check the err itself.
-func ContainsType(err error, v interface{}) bool {
- return len(GetAllType(err, v)) > 0
-}
-
-// Get is the same as GetAll but returns the deepest matching error.
-func Get(err error, msg string) error {
- es := GetAll(err, msg)
- if len(es) > 0 {
- return es[len(es)-1]
- }
-
- return nil
-}
-
-// GetType is the same as GetAllType but returns the deepest matching error.
-func GetType(err error, v interface{}) error {
- es := GetAllType(err, v)
- if len(es) > 0 {
- return es[len(es)-1]
- }
-
- return nil
-}
-
-// GetAll gets all the errors that might be wrapped in err with the
-// given message. The order of the errors is such that the outermost
-// matching error (the most recent wrap) is index zero, and so on.
-func GetAll(err error, msg string) []error {
- var result []error
-
- Walk(err, func(err error) {
- if err.Error() == msg {
- result = append(result, err)
- }
- })
-
- return result
-}
-
-// GetAllType gets all the errors that are the same type as v.
-//
-// The order of the return value is the same as described in GetAll.
-func GetAllType(err error, v interface{}) []error {
- var result []error
-
- var search string
- if v != nil {
- search = reflect.TypeOf(v).String()
- }
- Walk(err, func(err error) {
- var needle string
- if err != nil {
- needle = reflect.TypeOf(err).String()
- }
-
- if needle == search {
- result = append(result, err)
- }
- })
-
- return result
-}
-
-// Walk walks all the wrapped errors in err and calls the callback. If
-// err isn't a wrapped error, this will be called once for err. If err
-// is a wrapped error, the callback will be called for both the wrapper
-// that implements error as well as the wrapped error itself.
-func Walk(err error, cb WalkFunc) {
- if err == nil {
- return
- }
-
- switch e := err.(type) {
- case *wrappedError:
- cb(e.Outer)
- Walk(e.Inner, cb)
- case Wrapper:
- cb(err)
-
- for _, err := range e.WrappedErrors() {
- Walk(err, cb)
- }
- default:
- cb(err)
- }
-}
-
-// wrappedError is an implementation of error that has both the
-// outer and inner errors.
-type wrappedError struct {
- Outer error
- Inner error
-}
-
-func (w *wrappedError) Error() string {
- return w.Outer.Error()
-}
-
-func (w *wrappedError) WrappedErrors() []error {
- return []error{w.Outer, w.Inner}
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/LICENSE b/vendor/github.com/hashicorp/go-multierror/LICENSE
deleted file mode 100644
index 82b4de97..00000000
--- a/vendor/github.com/hashicorp/go-multierror/LICENSE
+++ /dev/null
@@ -1,353 +0,0 @@
-Mozilla Public License, version 2.0
-
-1. Definitions
-
-1.1. “Contributorâ€
-
- means each individual or legal entity that creates, contributes to the
- creation of, or owns Covered Software.
-
-1.2. “Contributor Versionâ€
-
- means the combination of the Contributions of others (if any) used by a
- Contributor and that particular Contributor’s Contribution.
-
-1.3. “Contributionâ€
-
- means Covered Software of a particular Contributor.
-
-1.4. “Covered Softwareâ€
-
- means Source Code Form to which the initial Contributor has attached the
- notice in Exhibit A, the Executable Form of such Source Code Form, and
- Modifications of such Source Code Form, in each case including portions
- thereof.
-
-1.5. “Incompatible With Secondary Licensesâ€
- means
-
- a. that the initial Contributor has attached the notice described in
- Exhibit B to the Covered Software; or
-
- b. that the Covered Software was made available under the terms of version
- 1.1 or earlier of the License, but not also under the terms of a
- Secondary License.
-
-1.6. “Executable Formâ€
-
- means any form of the work other than Source Code Form.
-
-1.7. “Larger Workâ€
-
- means a work that combines Covered Software with other material, in a separate
- file or files, that is not Covered Software.
-
-1.8. “Licenseâ€
-
- means this document.
-
-1.9. “Licensableâ€
-
- means having the right to grant, to the maximum extent possible, whether at the
- time of the initial grant or subsequently, any and all of the rights conveyed by
- this License.
-
-1.10. “Modificationsâ€
-
- means any of the following:
-
- a. any file in Source Code Form that results from an addition to, deletion
- from, or modification of the contents of Covered Software; or
-
- b. any new file in Source Code Form that contains any Covered Software.
-
-1.11. “Patent Claims†of a Contributor
-
- means any patent claim(s), including without limitation, method, process,
- and apparatus claims, in any patent Licensable by such Contributor that
- would be infringed, but for the grant of the License, by the making,
- using, selling, offering for sale, having made, import, or transfer of
- either its Contributions or its Contributor Version.
-
-1.12. “Secondary Licenseâ€
-
- means either the GNU General Public License, Version 2.0, the GNU Lesser
- General Public License, Version 2.1, the GNU Affero General Public
- License, Version 3.0, or any later versions of those licenses.
-
-1.13. “Source Code Formâ€
-
- means the form of the work preferred for making modifications.
-
-1.14. “You†(or “Yourâ€)
-
- means an individual or a legal entity exercising rights under this
- License. For legal entities, “You†includes any entity that controls, is
- controlled by, or is under common control with You. For purposes of this
- definition, “control†means (a) the power, direct or indirect, to cause
- the direction or management of such entity, whether by contract or
- otherwise, or (b) ownership of more than fifty percent (50%) of the
- outstanding shares or beneficial ownership of such entity.
-
-
-2. License Grants and Conditions
-
-2.1. Grants
-
- Each Contributor hereby grants You a world-wide, royalty-free,
- non-exclusive license:
-
- a. under intellectual property rights (other than patent or trademark)
- Licensable by such Contributor to use, reproduce, make available,
- modify, display, perform, distribute, and otherwise exploit its
- Contributions, either on an unmodified basis, with Modifications, or as
- part of a Larger Work; and
-
- b. under Patent Claims of such Contributor to make, use, sell, offer for
- sale, have made, import, and otherwise transfer either its Contributions
- or its Contributor Version.
-
-2.2. Effective Date
-
- The licenses granted in Section 2.1 with respect to any Contribution become
- effective for each Contribution on the date the Contributor first distributes
- such Contribution.
-
-2.3. Limitations on Grant Scope
-
- The licenses granted in this Section 2 are the only rights granted under this
- License. No additional rights or licenses will be implied from the distribution
- or licensing of Covered Software under this License. Notwithstanding Section
- 2.1(b) above, no patent license is granted by a Contributor:
-
- a. for any code that a Contributor has removed from Covered Software; or
-
- b. for infringements caused by: (i) Your and any other third party’s
- modifications of Covered Software, or (ii) the combination of its
- Contributions with other software (except as part of its Contributor
- Version); or
-
- c. under Patent Claims infringed by Covered Software in the absence of its
- Contributions.
-
- This License does not grant any rights in the trademarks, service marks, or
- logos of any Contributor (except as may be necessary to comply with the
- notice requirements in Section 3.4).
-
-2.4. Subsequent Licenses
-
- No Contributor makes additional grants as a result of Your choice to
- distribute the Covered Software under a subsequent version of this License
- (see Section 10.2) or under the terms of a Secondary License (if permitted
- under the terms of Section 3.3).
-
-2.5. Representation
-
- Each Contributor represents that the Contributor believes its Contributions
- are its original creation(s) or it has sufficient rights to grant the
- rights to its Contributions conveyed by this License.
-
-2.6. Fair Use
-
- This License is not intended to limit any rights You have under applicable
- copyright doctrines of fair use, fair dealing, or other equivalents.
-
-2.7. Conditions
-
- Sections 3.1, 3.2, 3.3, and 3.4 are conditions of the licenses granted in
- Section 2.1.
-
-
-3. Responsibilities
-
-3.1. Distribution of Source Form
-
- All distribution of Covered Software in Source Code Form, including any
- Modifications that You create or to which You contribute, must be under the
- terms of this License. You must inform recipients that the Source Code Form
- of the Covered Software is governed by the terms of this License, and how
- they can obtain a copy of this License. You may not attempt to alter or
- restrict the recipients’ rights in the Source Code Form.
-
-3.2. Distribution of Executable Form
-
- If You distribute Covered Software in Executable Form then:
-
- a. such Covered Software must also be made available in Source Code Form,
- as described in Section 3.1, and You must inform recipients of the
- Executable Form how they can obtain a copy of such Source Code Form by
- reasonable means in a timely manner, at a charge no more than the cost
- of distribution to the recipient; and
-
- b. You may distribute such Executable Form under the terms of this License,
- or sublicense it under different terms, provided that the license for
- the Executable Form does not attempt to limit or alter the recipients’
- rights in the Source Code Form under this License.
-
-3.3. Distribution of a Larger Work
-
- You may create and distribute a Larger Work under terms of Your choice,
- provided that You also comply with the requirements of this License for the
- Covered Software. If the Larger Work is a combination of Covered Software
- with a work governed by one or more Secondary Licenses, and the Covered
- Software is not Incompatible With Secondary Licenses, this License permits
- You to additionally distribute such Covered Software under the terms of
- such Secondary License(s), so that the recipient of the Larger Work may, at
- their option, further distribute the Covered Software under the terms of
- either this License or such Secondary License(s).
-
-3.4. Notices
-
- You may not remove or alter the substance of any license notices (including
- copyright notices, patent notices, disclaimers of warranty, or limitations
- of liability) contained within the Source Code Form of the Covered
- Software, except that You may alter any license notices to the extent
- required to remedy known factual inaccuracies.
-
-3.5. Application of Additional Terms
-
- You may choose to offer, and to charge a fee for, warranty, support,
- indemnity or liability obligations to one or more recipients of Covered
- Software. However, You may do so only on Your own behalf, and not on behalf
- of any Contributor. You must make it absolutely clear that any such
- warranty, support, indemnity, or liability obligation is offered by You
- alone, and You hereby agree to indemnify every Contributor for any
- liability incurred by such Contributor as a result of warranty, support,
- indemnity or liability terms You offer. You may include additional
- disclaimers of warranty and limitations of liability specific to any
- jurisdiction.
-
-4. Inability to Comply Due to Statute or Regulation
-
- If it is impossible for You to comply with any of the terms of this License
- with respect to some or all of the Covered Software due to statute, judicial
- order, or regulation then You must: (a) comply with the terms of this License
- to the maximum extent possible; and (b) describe the limitations and the code
- they affect. Such description must be placed in a text file included with all
- distributions of the Covered Software under this License. Except to the
- extent prohibited by statute or regulation, such description must be
- sufficiently detailed for a recipient of ordinary skill to be able to
- understand it.
-
-5. Termination
-
-5.1. The rights granted under this License will terminate automatically if You
- fail to comply with any of its terms. However, if You become compliant,
- then the rights granted under this License from a particular Contributor
- are reinstated (a) provisionally, unless and until such Contributor
- explicitly and finally terminates Your grants, and (b) on an ongoing basis,
- if such Contributor fails to notify You of the non-compliance by some
- reasonable means prior to 60 days after You have come back into compliance.
- Moreover, Your grants from a particular Contributor are reinstated on an
- ongoing basis if such Contributor notifies You of the non-compliance by
- some reasonable means, this is the first time You have received notice of
- non-compliance with this License from such Contributor, and You become
- compliant prior to 30 days after Your receipt of the notice.
-
-5.2. If You initiate litigation against any entity by asserting a patent
- infringement claim (excluding declaratory judgment actions, counter-claims,
- and cross-claims) alleging that a Contributor Version directly or
- indirectly infringes any patent, then the rights granted to You by any and
- all Contributors for the Covered Software under Section 2.1 of this License
- shall terminate.
-
-5.3. In the event of termination under Sections 5.1 or 5.2 above, all end user
- license agreements (excluding distributors and resellers) which have been
- validly granted by You or Your distributors under this License prior to
- termination shall survive termination.
-
-6. Disclaimer of Warranty
-
- Covered Software is provided under this License on an “as is†basis, without
- warranty of any kind, either expressed, implied, or statutory, including,
- without limitation, warranties that the Covered Software is free of defects,
- merchantable, fit for a particular purpose or non-infringing. The entire
- risk as to the quality and performance of the Covered Software is with You.
- Should any Covered Software prove defective in any respect, You (not any
- Contributor) assume the cost of any necessary servicing, repair, or
- correction. This disclaimer of warranty constitutes an essential part of this
- License. No use of any Covered Software is authorized under this License
- except under this disclaimer.
-
-7. Limitation of Liability
-
- Under no circumstances and under no legal theory, whether tort (including
- negligence), contract, or otherwise, shall any Contributor, or anyone who
- distributes Covered Software as permitted above, be liable to You for any
- direct, indirect, special, incidental, or consequential damages of any
- character including, without limitation, damages for lost profits, loss of
- goodwill, work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses, even if such party shall have been
- informed of the possibility of such damages. This limitation of liability
- shall not apply to liability for death or personal injury resulting from such
- party’s negligence to the extent applicable law prohibits such limitation.
- Some jurisdictions do not allow the exclusion or limitation of incidental or
- consequential damages, so this exclusion and limitation may not apply to You.
-
-8. Litigation
-
- Any litigation relating to this License may be brought only in the courts of
- a jurisdiction where the defendant maintains its principal place of business
- and such litigation shall be governed by laws of that jurisdiction, without
- reference to its conflict-of-law provisions. Nothing in this Section shall
- prevent a party’s ability to bring cross-claims or counter-claims.
-
-9. Miscellaneous
-
- This License represents the complete agreement concerning the subject matter
- hereof. If any provision of this License is held to be unenforceable, such
- provision shall be reformed only to the extent necessary to make it
- enforceable. Any law or regulation which provides that the language of a
- contract shall be construed against the drafter shall not be used to construe
- this License against a Contributor.
-
-
-10. Versions of the License
-
-10.1. New Versions
-
- Mozilla Foundation is the license steward. Except as provided in Section
- 10.3, no one other than the license steward has the right to modify or
- publish new versions of this License. Each version will be given a
- distinguishing version number.
-
-10.2. Effect of New Versions
-
- You may distribute the Covered Software under the terms of the version of
- the License under which You originally received the Covered Software, or
- under the terms of any subsequent version published by the license
- steward.
-
-10.3. Modified Versions
-
- If you create software not governed by this License, and you want to
- create a new license for such software, you may create and use a modified
- version of this License if you rename the license and remove any
- references to the name of the license steward (except to note that such
- modified license differs from this License).
-
-10.4. Distributing Source Code Form that is Incompatible With Secondary Licenses
- If You choose to distribute Source Code Form that is Incompatible With
- Secondary Licenses under the terms of this version of the License, the
- notice described in Exhibit B of this License must be attached.
-
-Exhibit A - Source Code Form License Notice
-
- This Source Code Form is subject to the
- terms of the Mozilla Public License, v.
- 2.0. If a copy of the MPL was not
- distributed with this file, You can
- obtain one at
- http://mozilla.org/MPL/2.0/.
-
-If it is not possible or desirable to put the notice in a particular file, then
-You may include the notice in a location (such as a LICENSE file in a relevant
-directory) where a recipient would be likely to look for such a notice.
-
-You may add additional accurate notices of copyright ownership.
-
-Exhibit B - “Incompatible With Secondary Licenses†Notice
-
- This Source Code Form is “Incompatible
- With Secondary Licensesâ€, as defined by
- the Mozilla Public License, v. 2.0.
diff --git a/vendor/github.com/hashicorp/go-multierror/Makefile b/vendor/github.com/hashicorp/go-multierror/Makefile
deleted file mode 100644
index b97cd6ed..00000000
--- a/vendor/github.com/hashicorp/go-multierror/Makefile
+++ /dev/null
@@ -1,31 +0,0 @@
-TEST?=./...
-
-default: test
-
-# test runs the test suite and vets the code.
-test: generate
- @echo "==> Running tests..."
- @go list $(TEST) \
- | grep -v "/vendor/" \
- | xargs -n1 go test -timeout=60s -parallel=10 ${TESTARGS}
-
-# testrace runs the race checker
-testrace: generate
- @echo "==> Running tests (race)..."
- @go list $(TEST) \
- | grep -v "/vendor/" \
- | xargs -n1 go test -timeout=60s -race ${TESTARGS}
-
-# updatedeps installs all the dependencies needed to run and build.
-updatedeps:
- @sh -c "'${CURDIR}/scripts/deps.sh' '${NAME}'"
-
-# generate runs `go generate` to build the dynamically generated source files.
-generate:
- @echo "==> Generating..."
- @find . -type f -name '.DS_Store' -delete
- @go list ./... \
- | grep -v "/vendor/" \
- | xargs -n1 go generate
-
-.PHONY: default test testrace updatedeps generate
diff --git a/vendor/github.com/hashicorp/go-multierror/README.md b/vendor/github.com/hashicorp/go-multierror/README.md
deleted file mode 100644
index 71dd308e..00000000
--- a/vendor/github.com/hashicorp/go-multierror/README.md
+++ /dev/null
@@ -1,150 +0,0 @@
-# go-multierror
-
-[](https://circleci.com/gh/hashicorp/go-multierror)
-[](https://pkg.go.dev/github.com/hashicorp/go-multierror)
-
-
-[circleci]: https://app.circleci.com/pipelines/github/hashicorp/go-multierror
-[godocs]: https://pkg.go.dev/github.com/hashicorp/go-multierror
-
-`go-multierror` is a package for Go that provides a mechanism for
-representing a list of `error` values as a single `error`.
-
-This allows a function in Go to return an `error` that might actually
-be a list of errors. If the caller knows this, they can unwrap the
-list and access the errors. If the caller doesn't know, the error
-formats to a nice human-readable format.
-
-`go-multierror` is fully compatible with the Go standard library
-[errors](https://golang.org/pkg/errors/) package, including the
-functions `As`, `Is`, and `Unwrap`. This provides a standardized approach
-for introspecting on error values.
-
-## Installation and Docs
-
-Install using `go get github.com/hashicorp/go-multierror`.
-
-Full documentation is available at
-https://pkg.go.dev/github.com/hashicorp/go-multierror
-
-### Requires go version 1.13 or newer
-
-`go-multierror` requires go version 1.13 or newer. Go 1.13 introduced
-[error wrapping](https://golang.org/doc/go1.13#error_wrapping), which
-this library takes advantage of.
-
-If you need to use an earlier version of go, you can use the
-[v1.0.0](https://github.com/hashicorp/go-multierror/tree/v1.0.0)
-tag, which doesn't rely on features in go 1.13.
-
-If you see compile errors that look like the below, it's likely that
-you're on an older version of go:
-
-```
-/go/src/github.com/hashicorp/go-multierror/multierror.go:112:9: undefined: errors.As
-/go/src/github.com/hashicorp/go-multierror/multierror.go:117:9: undefined: errors.Is
-```
-
-## Usage
-
-go-multierror is easy to use and purposely built to be unobtrusive in
-existing Go applications/libraries that may not be aware of it.
-
-**Building a list of errors**
-
-The `Append` function is used to create a list of errors. This function
-behaves a lot like the Go built-in `append` function: it doesn't matter
-if the first argument is nil, a `multierror.Error`, or any other `error`,
-the function behaves as you would expect.
-
-```go
-var result error
-
-if err := step1(); err != nil {
- result = multierror.Append(result, err)
-}
-if err := step2(); err != nil {
- result = multierror.Append(result, err)
-}
-
-return result
-```
-
-**Customizing the formatting of the errors**
-
-By specifying a custom `ErrorFormat`, you can customize the format
-of the `Error() string` function:
-
-```go
-var result *multierror.Error
-
-// ... accumulate errors here, maybe using Append
-
-if result != nil {
- result.ErrorFormat = func([]error) string {
- return "errors!"
- }
-}
-```
-
-**Accessing the list of errors**
-
-`multierror.Error` implements `error` so if the caller doesn't know about
-multierror, it will work just fine. But if you're aware a multierror might
-be returned, you can use type switches to access the list of errors:
-
-```go
-if err := something(); err != nil {
- if merr, ok := err.(*multierror.Error); ok {
- // Use merr.Errors
- }
-}
-```
-
-You can also use the standard [`errors.Unwrap`](https://golang.org/pkg/errors/#Unwrap)
-function. This will continue to unwrap into subsequent errors until none exist.
-
-**Extracting an error**
-
-The standard library [`errors.As`](https://golang.org/pkg/errors/#As)
-function can be used directly with a multierror to extract a specific error:
-
-```go
-// Assume err is a multierror value
-err := somefunc()
-
-// We want to know if "err" has a "RichErrorType" in it and extract it.
-var errRich RichErrorType
-if errors.As(err, &errRich) {
- // It has it, and now errRich is populated.
-}
-```
-
-**Checking for an exact error value**
-
-Some errors are returned as exact errors such as the [`ErrNotExist`](https://golang.org/pkg/os/#pkg-variables)
-error in the `os` package. You can check if this error is present by using
-the standard [`errors.Is`](https://golang.org/pkg/errors/#Is) function.
-
-```go
-// Assume err is a multierror value
-err := somefunc()
-if errors.Is(err, os.ErrNotExist) {
- // err contains os.ErrNotExist
-}
-```
-
-**Returning a multierror only if there are errors**
-
-If you build a `multierror.Error`, you can use the `ErrorOrNil` function
-to return an `error` implementation only if there are errors to return:
-
-```go
-var result *multierror.Error
-
-// ... accumulate errors here
-
-// Return the `error` only if errors were added to the multierror, otherwise
-// return nil since there are no errors.
-return result.ErrorOrNil()
-```
diff --git a/vendor/github.com/hashicorp/go-multierror/append.go b/vendor/github.com/hashicorp/go-multierror/append.go
deleted file mode 100644
index 3e2589bf..00000000
--- a/vendor/github.com/hashicorp/go-multierror/append.go
+++ /dev/null
@@ -1,43 +0,0 @@
-package multierror
-
-// Append is a helper function that will append more errors
-// onto an Error in order to create a larger multi-error.
-//
-// If err is not a multierror.Error, then it will be turned into
-// one. If any of the errs are multierr.Error, they will be flattened
-// one level into err.
-// Any nil errors within errs will be ignored. If err is nil, a new
-// *Error will be returned.
-func Append(err error, errs ...error) *Error {
- switch err := err.(type) {
- case *Error:
- // Typed nils can reach here, so initialize if we are nil
- if err == nil {
- err = new(Error)
- }
-
- // Go through each error and flatten
- for _, e := range errs {
- switch e := e.(type) {
- case *Error:
- if e != nil {
- err.Errors = append(err.Errors, e.Errors...)
- }
- default:
- if e != nil {
- err.Errors = append(err.Errors, e)
- }
- }
- }
-
- return err
- default:
- newErrs := make([]error, 0, len(errs)+1)
- if err != nil {
- newErrs = append(newErrs, err)
- }
- newErrs = append(newErrs, errs...)
-
- return Append(&Error{}, newErrs...)
- }
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/flatten.go b/vendor/github.com/hashicorp/go-multierror/flatten.go
deleted file mode 100644
index aab8e9ab..00000000
--- a/vendor/github.com/hashicorp/go-multierror/flatten.go
+++ /dev/null
@@ -1,26 +0,0 @@
-package multierror
-
-// Flatten flattens the given error, merging any *Errors together into
-// a single *Error.
-func Flatten(err error) error {
- // If it isn't an *Error, just return the error as-is
- if _, ok := err.(*Error); !ok {
- return err
- }
-
- // Otherwise, make the result and flatten away!
- flatErr := new(Error)
- flatten(err, flatErr)
- return flatErr
-}
-
-func flatten(err error, flatErr *Error) {
- switch err := err.(type) {
- case *Error:
- for _, e := range err.Errors {
- flatten(e, flatErr)
- }
- default:
- flatErr.Errors = append(flatErr.Errors, err)
- }
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/format.go b/vendor/github.com/hashicorp/go-multierror/format.go
deleted file mode 100644
index 47f13c49..00000000
--- a/vendor/github.com/hashicorp/go-multierror/format.go
+++ /dev/null
@@ -1,27 +0,0 @@
-package multierror
-
-import (
- "fmt"
- "strings"
-)
-
-// ErrorFormatFunc is a function callback that is called by Error to
-// turn the list of errors into a string.
-type ErrorFormatFunc func([]error) string
-
-// ListFormatFunc is a basic formatter that outputs the number of errors
-// that occurred along with a bullet point list of the errors.
-func ListFormatFunc(es []error) string {
- if len(es) == 1 {
- return fmt.Sprintf("1 error occurred:\n\t* %s\n\n", es[0])
- }
-
- points := make([]string, len(es))
- for i, err := range es {
- points[i] = fmt.Sprintf("* %s", err)
- }
-
- return fmt.Sprintf(
- "%d errors occurred:\n\t%s\n\n",
- len(es), strings.Join(points, "\n\t"))
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/group.go b/vendor/github.com/hashicorp/go-multierror/group.go
deleted file mode 100644
index 9c29efb7..00000000
--- a/vendor/github.com/hashicorp/go-multierror/group.go
+++ /dev/null
@@ -1,38 +0,0 @@
-package multierror
-
-import "sync"
-
-// Group is a collection of goroutines which return errors that need to be
-// coalesced.
-type Group struct {
- mutex sync.Mutex
- err *Error
- wg sync.WaitGroup
-}
-
-// Go calls the given function in a new goroutine.
-//
-// If the function returns an error it is added to the group multierror which
-// is returned by Wait.
-func (g *Group) Go(f func() error) {
- g.wg.Add(1)
-
- go func() {
- defer g.wg.Done()
-
- if err := f(); err != nil {
- g.mutex.Lock()
- g.err = Append(g.err, err)
- g.mutex.Unlock()
- }
- }()
-}
-
-// Wait blocks until all function calls from the Go method have returned, then
-// returns the multierror.
-func (g *Group) Wait() *Error {
- g.wg.Wait()
- g.mutex.Lock()
- defer g.mutex.Unlock()
- return g.err
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/multierror.go b/vendor/github.com/hashicorp/go-multierror/multierror.go
deleted file mode 100644
index f5457432..00000000
--- a/vendor/github.com/hashicorp/go-multierror/multierror.go
+++ /dev/null
@@ -1,121 +0,0 @@
-package multierror
-
-import (
- "errors"
- "fmt"
-)
-
-// Error is an error type to track multiple errors. This is used to
-// accumulate errors in cases and return them as a single "error".
-type Error struct {
- Errors []error
- ErrorFormat ErrorFormatFunc
-}
-
-func (e *Error) Error() string {
- fn := e.ErrorFormat
- if fn == nil {
- fn = ListFormatFunc
- }
-
- return fn(e.Errors)
-}
-
-// ErrorOrNil returns an error interface if this Error represents
-// a list of errors, or returns nil if the list of errors is empty. This
-// function is useful at the end of accumulation to make sure that the value
-// returned represents the existence of errors.
-func (e *Error) ErrorOrNil() error {
- if e == nil {
- return nil
- }
- if len(e.Errors) == 0 {
- return nil
- }
-
- return e
-}
-
-func (e *Error) GoString() string {
- return fmt.Sprintf("*%#v", *e)
-}
-
-// WrappedErrors returns the list of errors that this Error is wrapping. It is
-// an implementation of the errwrap.Wrapper interface so that multierror.Error
-// can be used with that library.
-//
-// This method is not safe to be called concurrently. Unlike accessing the
-// Errors field directly, this function also checks if the multierror is nil to
-// prevent a null-pointer panic. It satisfies the errwrap.Wrapper interface.
-func (e *Error) WrappedErrors() []error {
- if e == nil {
- return nil
- }
- return e.Errors
-}
-
-// Unwrap returns an error from Error (or nil if there are no errors).
-// This error returned will further support Unwrap to get the next error,
-// etc. The order will match the order of Errors in the multierror.Error
-// at the time of calling.
-//
-// The resulting error supports errors.As/Is/Unwrap so you can continue
-// to use the stdlib errors package to introspect further.
-//
-// This will perform a shallow copy of the errors slice. Any errors appended
-// to this error after calling Unwrap will not be available until a new
-// Unwrap is called on the multierror.Error.
-func (e *Error) Unwrap() error {
- // If we have no errors then we do nothing
- if e == nil || len(e.Errors) == 0 {
- return nil
- }
-
- // If we have exactly one error, we can just return that directly.
- if len(e.Errors) == 1 {
- return e.Errors[0]
- }
-
- // Shallow copy the slice
- errs := make([]error, len(e.Errors))
- copy(errs, e.Errors)
- return chain(errs)
-}
-
-// chain implements the interfaces necessary for errors.Is/As/Unwrap to
-// work in a deterministic way with multierror. A chain tracks a list of
-// errors while accounting for the current represented error. This lets
-// Is/As be meaningful.
-//
-// Unwrap returns the next error. In the cleanest form, Unwrap would return
-// the wrapped error here but we can't do that if we want to properly
-// get access to all the errors. Instead, users are recommended to use
-// Is/As to get the correct error type out.
-//
-// Precondition: []error is non-empty (len > 0)
-type chain []error
-
-// Error implements the error interface
-func (e chain) Error() string {
- return e[0].Error()
-}
-
-// Unwrap implements errors.Unwrap by returning the next error in the
-// chain or nil if there are no more errors.
-func (e chain) Unwrap() error {
- if len(e) == 1 {
- return nil
- }
-
- return e[1:]
-}
-
-// As implements errors.As by attempting to map to the current value.
-func (e chain) As(target interface{}) bool {
- return errors.As(e[0], target)
-}
-
-// Is implements errors.Is by comparing the current value directly.
-func (e chain) Is(target error) bool {
- return errors.Is(e[0], target)
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/prefix.go b/vendor/github.com/hashicorp/go-multierror/prefix.go
deleted file mode 100644
index 5c477abe..00000000
--- a/vendor/github.com/hashicorp/go-multierror/prefix.go
+++ /dev/null
@@ -1,37 +0,0 @@
-package multierror
-
-import (
- "fmt"
-
- "github.com/hashicorp/errwrap"
-)
-
-// Prefix is a helper function that will prefix some text
-// to the given error. If the error is a multierror.Error, then
-// it will be prefixed to each wrapped error.
-//
-// This is useful to use when appending multiple multierrors
-// together in order to give better scoping.
-func Prefix(err error, prefix string) error {
- if err == nil {
- return nil
- }
-
- format := fmt.Sprintf("%s {{err}}", prefix)
- switch err := err.(type) {
- case *Error:
- // Typed nils can reach here, so initialize if we are nil
- if err == nil {
- err = new(Error)
- }
-
- // Wrap each of the errors
- for i, e := range err.Errors {
- err.Errors[i] = errwrap.Wrapf(format, e)
- }
-
- return err
- default:
- return errwrap.Wrapf(format, err)
- }
-}
diff --git a/vendor/github.com/hashicorp/go-multierror/sort.go b/vendor/github.com/hashicorp/go-multierror/sort.go
deleted file mode 100644
index fecb14e8..00000000
--- a/vendor/github.com/hashicorp/go-multierror/sort.go
+++ /dev/null
@@ -1,16 +0,0 @@
-package multierror
-
-// Len implements sort.Interface function for length
-func (err Error) Len() int {
- return len(err.Errors)
-}
-
-// Swap implements sort.Interface function for swapping elements
-func (err Error) Swap(i, j int) {
- err.Errors[i], err.Errors[j] = err.Errors[j], err.Errors[i]
-}
-
-// Less implements sort.Interface function for determining order
-func (err Error) Less(i, j int) bool {
- return err.Errors[i].Error() < err.Errors[j].Error()
-}
diff --git a/vendor/github.com/hashicorp/hcl/.gitignore b/vendor/github.com/hashicorp/hcl/.gitignore
deleted file mode 100644
index 15586a2b..00000000
--- a/vendor/github.com/hashicorp/hcl/.gitignore
+++ /dev/null
@@ -1,9 +0,0 @@
-y.output
-
-# ignore intellij files
-.idea
-*.iml
-*.ipr
-*.iws
-
-*.test
diff --git a/vendor/github.com/hashicorp/hcl/.travis.yml b/vendor/github.com/hashicorp/hcl/.travis.yml
deleted file mode 100644
index cb63a321..00000000
--- a/vendor/github.com/hashicorp/hcl/.travis.yml
+++ /dev/null
@@ -1,13 +0,0 @@
-sudo: false
-
-language: go
-
-go:
- - 1.x
- - tip
-
-branches:
- only:
- - master
-
-script: make test
diff --git a/vendor/github.com/hashicorp/hcl/LICENSE b/vendor/github.com/hashicorp/hcl/LICENSE
deleted file mode 100644
index c33dcc7c..00000000
--- a/vendor/github.com/hashicorp/hcl/LICENSE
+++ /dev/null
@@ -1,354 +0,0 @@
-Mozilla Public License, version 2.0
-
-1. Definitions
-
-1.1. “Contributorâ€
-
- means each individual or legal entity that creates, contributes to the
- creation of, or owns Covered Software.
-
-1.2. “Contributor Versionâ€
-
- means the combination of the Contributions of others (if any) used by a
- Contributor and that particular Contributor’s Contribution.
-
-1.3. “Contributionâ€
-
- means Covered Software of a particular Contributor.
-
-1.4. “Covered Softwareâ€
-
- means Source Code Form to which the initial Contributor has attached the
- notice in Exhibit A, the Executable Form of such Source Code Form, and
- Modifications of such Source Code Form, in each case including portions
- thereof.
-
-1.5. “Incompatible With Secondary Licensesâ€
- means
-
- a. that the initial Contributor has attached the notice described in
- Exhibit B to the Covered Software; or
-
- b. that the Covered Software was made available under the terms of version
- 1.1 or earlier of the License, but not also under the terms of a
- Secondary License.
-
-1.6. “Executable Formâ€
-
- means any form of the work other than Source Code Form.
-
-1.7. “Larger Workâ€
-
- means a work that combines Covered Software with other material, in a separate
- file or files, that is not Covered Software.
-
-1.8. “Licenseâ€
-
- means this document.
-
-1.9. “Licensableâ€
-
- means having the right to grant, to the maximum extent possible, whether at the
- time of the initial grant or subsequently, any and all of the rights conveyed by
- this License.
-
-1.10. “Modificationsâ€
-
- means any of the following:
-
- a. any file in Source Code Form that results from an addition to, deletion
- from, or modification of the contents of Covered Software; or
-
- b. any new file in Source Code Form that contains any Covered Software.
-
-1.11. “Patent Claims†of a Contributor
-
- means any patent claim(s), including without limitation, method, process,
- and apparatus claims, in any patent Licensable by such Contributor that
- would be infringed, but for the grant of the License, by the making,
- using, selling, offering for sale, having made, import, or transfer of
- either its Contributions or its Contributor Version.
-
-1.12. “Secondary Licenseâ€
-
- means either the GNU General Public License, Version 2.0, the GNU Lesser
- General Public License, Version 2.1, the GNU Affero General Public
- License, Version 3.0, or any later versions of those licenses.
-
-1.13. “Source Code Formâ€
-
- means the form of the work preferred for making modifications.
-
-1.14. “You†(or “Yourâ€)
-
- means an individual or a legal entity exercising rights under this
- License. For legal entities, “You†includes any entity that controls, is
- controlled by, or is under common control with You. For purposes of this
- definition, “control†means (a) the power, direct or indirect, to cause
- the direction or management of such entity, whether by contract or
- otherwise, or (b) ownership of more than fifty percent (50%) of the
- outstanding shares or beneficial ownership of such entity.
-
-
-2. License Grants and Conditions
-
-2.1. Grants
-
- Each Contributor hereby grants You a world-wide, royalty-free,
- non-exclusive license:
-
- a. under intellectual property rights (other than patent or trademark)
- Licensable by such Contributor to use, reproduce, make available,
- modify, display, perform, distribute, and otherwise exploit its
- Contributions, either on an unmodified basis, with Modifications, or as
- part of a Larger Work; and
-
- b. under Patent Claims of such Contributor to make, use, sell, offer for
- sale, have made, import, and otherwise transfer either its Contributions
- or its Contributor Version.
-
-2.2. Effective Date
-
- The licenses granted in Section 2.1 with respect to any Contribution become
- effective for each Contribution on the date the Contributor first distributes
- such Contribution.
-
-2.3. Limitations on Grant Scope
-
- The licenses granted in this Section 2 are the only rights granted under this
- License. No additional rights or licenses will be implied from the distribution
- or licensing of Covered Software under this License. Notwithstanding Section
- 2.1(b) above, no patent license is granted by a Contributor:
-
- a. for any code that a Contributor has removed from Covered Software; or
-
- b. for infringements caused by: (i) Your and any other third party’s
- modifications of Covered Software, or (ii) the combination of its
- Contributions with other software (except as part of its Contributor
- Version); or
-
- c. under Patent Claims infringed by Covered Software in the absence of its
- Contributions.
-
- This License does not grant any rights in the trademarks, service marks, or
- logos of any Contributor (except as may be necessary to comply with the
- notice requirements in Section 3.4).
-
-2.4. Subsequent Licenses
-
- No Contributor makes additional grants as a result of Your choice to
- distribute the Covered Software under a subsequent version of this License
- (see Section 10.2) or under the terms of a Secondary License (if permitted
- under the terms of Section 3.3).
-
-2.5. Representation
-
- Each Contributor represents that the Contributor believes its Contributions
- are its original creation(s) or it has sufficient rights to grant the
- rights to its Contributions conveyed by this License.
-
-2.6. Fair Use
-
- This License is not intended to limit any rights You have under applicable
- copyright doctrines of fair use, fair dealing, or other equivalents.
-
-2.7. Conditions
-
- Sections 3.1, 3.2, 3.3, and 3.4 are conditions of the licenses granted in
- Section 2.1.
-
-
-3. Responsibilities
-
-3.1. Distribution of Source Form
-
- All distribution of Covered Software in Source Code Form, including any
- Modifications that You create or to which You contribute, must be under the
- terms of this License. You must inform recipients that the Source Code Form
- of the Covered Software is governed by the terms of this License, and how
- they can obtain a copy of this License. You may not attempt to alter or
- restrict the recipients’ rights in the Source Code Form.
-
-3.2. Distribution of Executable Form
-
- If You distribute Covered Software in Executable Form then:
-
- a. such Covered Software must also be made available in Source Code Form,
- as described in Section 3.1, and You must inform recipients of the
- Executable Form how they can obtain a copy of such Source Code Form by
- reasonable means in a timely manner, at a charge no more than the cost
- of distribution to the recipient; and
-
- b. You may distribute such Executable Form under the terms of this License,
- or sublicense it under different terms, provided that the license for
- the Executable Form does not attempt to limit or alter the recipients’
- rights in the Source Code Form under this License.
-
-3.3. Distribution of a Larger Work
-
- You may create and distribute a Larger Work under terms of Your choice,
- provided that You also comply with the requirements of this License for the
- Covered Software. If the Larger Work is a combination of Covered Software
- with a work governed by one or more Secondary Licenses, and the Covered
- Software is not Incompatible With Secondary Licenses, this License permits
- You to additionally distribute such Covered Software under the terms of
- such Secondary License(s), so that the recipient of the Larger Work may, at
- their option, further distribute the Covered Software under the terms of
- either this License or such Secondary License(s).
-
-3.4. Notices
-
- You may not remove or alter the substance of any license notices (including
- copyright notices, patent notices, disclaimers of warranty, or limitations
- of liability) contained within the Source Code Form of the Covered
- Software, except that You may alter any license notices to the extent
- required to remedy known factual inaccuracies.
-
-3.5. Application of Additional Terms
-
- You may choose to offer, and to charge a fee for, warranty, support,
- indemnity or liability obligations to one or more recipients of Covered
- Software. However, You may do so only on Your own behalf, and not on behalf
- of any Contributor. You must make it absolutely clear that any such
- warranty, support, indemnity, or liability obligation is offered by You
- alone, and You hereby agree to indemnify every Contributor for any
- liability incurred by such Contributor as a result of warranty, support,
- indemnity or liability terms You offer. You may include additional
- disclaimers of warranty and limitations of liability specific to any
- jurisdiction.
-
-4. Inability to Comply Due to Statute or Regulation
-
- If it is impossible for You to comply with any of the terms of this License
- with respect to some or all of the Covered Software due to statute, judicial
- order, or regulation then You must: (a) comply with the terms of this License
- to the maximum extent possible; and (b) describe the limitations and the code
- they affect. Such description must be placed in a text file included with all
- distributions of the Covered Software under this License. Except to the
- extent prohibited by statute or regulation, such description must be
- sufficiently detailed for a recipient of ordinary skill to be able to
- understand it.
-
-5. Termination
-
-5.1. The rights granted under this License will terminate automatically if You
- fail to comply with any of its terms. However, if You become compliant,
- then the rights granted under this License from a particular Contributor
- are reinstated (a) provisionally, unless and until such Contributor
- explicitly and finally terminates Your grants, and (b) on an ongoing basis,
- if such Contributor fails to notify You of the non-compliance by some
- reasonable means prior to 60 days after You have come back into compliance.
- Moreover, Your grants from a particular Contributor are reinstated on an
- ongoing basis if such Contributor notifies You of the non-compliance by
- some reasonable means, this is the first time You have received notice of
- non-compliance with this License from such Contributor, and You become
- compliant prior to 30 days after Your receipt of the notice.
-
-5.2. If You initiate litigation against any entity by asserting a patent
- infringement claim (excluding declaratory judgment actions, counter-claims,
- and cross-claims) alleging that a Contributor Version directly or
- indirectly infringes any patent, then the rights granted to You by any and
- all Contributors for the Covered Software under Section 2.1 of this License
- shall terminate.
-
-5.3. In the event of termination under Sections 5.1 or 5.2 above, all end user
- license agreements (excluding distributors and resellers) which have been
- validly granted by You or Your distributors under this License prior to
- termination shall survive termination.
-
-6. Disclaimer of Warranty
-
- Covered Software is provided under this License on an “as is†basis, without
- warranty of any kind, either expressed, implied, or statutory, including,
- without limitation, warranties that the Covered Software is free of defects,
- merchantable, fit for a particular purpose or non-infringing. The entire
- risk as to the quality and performance of the Covered Software is with You.
- Should any Covered Software prove defective in any respect, You (not any
- Contributor) assume the cost of any necessary servicing, repair, or
- correction. This disclaimer of warranty constitutes an essential part of this
- License. No use of any Covered Software is authorized under this License
- except under this disclaimer.
-
-7. Limitation of Liability
-
- Under no circumstances and under no legal theory, whether tort (including
- negligence), contract, or otherwise, shall any Contributor, or anyone who
- distributes Covered Software as permitted above, be liable to You for any
- direct, indirect, special, incidental, or consequential damages of any
- character including, without limitation, damages for lost profits, loss of
- goodwill, work stoppage, computer failure or malfunction, or any and all
- other commercial damages or losses, even if such party shall have been
- informed of the possibility of such damages. This limitation of liability
- shall not apply to liability for death or personal injury resulting from such
- party’s negligence to the extent applicable law prohibits such limitation.
- Some jurisdictions do not allow the exclusion or limitation of incidental or
- consequential damages, so this exclusion and limitation may not apply to You.
-
-8. Litigation
-
- Any litigation relating to this License may be brought only in the courts of
- a jurisdiction where the defendant maintains its principal place of business
- and such litigation shall be governed by laws of that jurisdiction, without
- reference to its conflict-of-law provisions. Nothing in this Section shall
- prevent a party’s ability to bring cross-claims or counter-claims.
-
-9. Miscellaneous
-
- This License represents the complete agreement concerning the subject matter
- hereof. If any provision of this License is held to be unenforceable, such
- provision shall be reformed only to the extent necessary to make it
- enforceable. Any law or regulation which provides that the language of a
- contract shall be construed against the drafter shall not be used to construe
- this License against a Contributor.
-
-
-10. Versions of the License
-
-10.1. New Versions
-
- Mozilla Foundation is the license steward. Except as provided in Section
- 10.3, no one other than the license steward has the right to modify or
- publish new versions of this License. Each version will be given a
- distinguishing version number.
-
-10.2. Effect of New Versions
-
- You may distribute the Covered Software under the terms of the version of
- the License under which You originally received the Covered Software, or
- under the terms of any subsequent version published by the license
- steward.
-
-10.3. Modified Versions
-
- If you create software not governed by this License, and you want to
- create a new license for such software, you may create and use a modified
- version of this License if you rename the license and remove any
- references to the name of the license steward (except to note that such
- modified license differs from this License).
-
-10.4. Distributing Source Code Form that is Incompatible With Secondary Licenses
- If You choose to distribute Source Code Form that is Incompatible With
- Secondary Licenses under the terms of this version of the License, the
- notice described in Exhibit B of this License must be attached.
-
-Exhibit A - Source Code Form License Notice
-
- This Source Code Form is subject to the
- terms of the Mozilla Public License, v.
- 2.0. If a copy of the MPL was not
- distributed with this file, You can
- obtain one at
- http://mozilla.org/MPL/2.0/.
-
-If it is not possible or desirable to put the notice in a particular file, then
-You may include the notice in a location (such as a LICENSE file in a relevant
-directory) where a recipient would be likely to look for such a notice.
-
-You may add additional accurate notices of copyright ownership.
-
-Exhibit B - “Incompatible With Secondary Licenses†Notice
-
- This Source Code Form is “Incompatible
- With Secondary Licensesâ€, as defined by
- the Mozilla Public License, v. 2.0.
-
diff --git a/vendor/github.com/hashicorp/hcl/Makefile b/vendor/github.com/hashicorp/hcl/Makefile
deleted file mode 100644
index 84fd743f..00000000
--- a/vendor/github.com/hashicorp/hcl/Makefile
+++ /dev/null
@@ -1,18 +0,0 @@
-TEST?=./...
-
-default: test
-
-fmt: generate
- go fmt ./...
-
-test: generate
- go get -t ./...
- go test $(TEST) $(TESTARGS)
-
-generate:
- go generate ./...
-
-updatedeps:
- go get -u golang.org/x/tools/cmd/stringer
-
-.PHONY: default generate test updatedeps
diff --git a/vendor/github.com/hashicorp/hcl/README.md b/vendor/github.com/hashicorp/hcl/README.md
deleted file mode 100644
index c8223326..00000000
--- a/vendor/github.com/hashicorp/hcl/README.md
+++ /dev/null
@@ -1,125 +0,0 @@
-# HCL
-
-[](https://godoc.org/github.com/hashicorp/hcl) [](https://travis-ci.org/hashicorp/hcl)
-
-HCL (HashiCorp Configuration Language) is a configuration language built
-by HashiCorp. The goal of HCL is to build a structured configuration language
-that is both human and machine friendly for use with command-line tools, but
-specifically targeted towards DevOps tools, servers, etc.
-
-HCL is also fully JSON compatible. That is, JSON can be used as completely
-valid input to a system expecting HCL. This helps makes systems
-interoperable with other systems.
-
-HCL is heavily inspired by
-[libucl](https://github.com/vstakhov/libucl),
-nginx configuration, and others similar.
-
-## Why?
-
-A common question when viewing HCL is to ask the question: why not
-JSON, YAML, etc.?
-
-Prior to HCL, the tools we built at [HashiCorp](http://www.hashicorp.com)
-used a variety of configuration languages from full programming languages
-such as Ruby to complete data structure languages such as JSON. What we
-learned is that some people wanted human-friendly configuration languages
-and some people wanted machine-friendly languages.
-
-JSON fits a nice balance in this, but is fairly verbose and most
-importantly doesn't support comments. With YAML, we found that beginners
-had a really hard time determining what the actual structure was, and
-ended up guessing more often than not whether to use a hyphen, colon, etc.
-in order to represent some configuration key.
-
-Full programming languages such as Ruby enable complex behavior
-a configuration language shouldn't usually allow, and also forces
-people to learn some set of Ruby.
-
-Because of this, we decided to create our own configuration language
-that is JSON-compatible. Our configuration language (HCL) is designed
-to be written and modified by humans. The API for HCL allows JSON
-as an input so that it is also machine-friendly (machines can generate
-JSON instead of trying to generate HCL).
-
-Our goal with HCL is not to alienate other configuration languages.
-It is instead to provide HCL as a specialized language for our tools,
-and JSON as the interoperability layer.
-
-## Syntax
-
-For a complete grammar, please see the parser itself. A high-level overview
-of the syntax and grammar is listed here.
-
- * Single line comments start with `#` or `//`
-
- * Multi-line comments are wrapped in `/*` and `*/`. Nested block comments
- are not allowed. A multi-line comment (also known as a block comment)
- terminates at the first `*/` found.
-
- * Values are assigned with the syntax `key = value` (whitespace doesn't
- matter). The value can be any primitive: a string, number, boolean,
- object, or list.
-
- * Strings are double-quoted and can contain any UTF-8 characters.
- Example: `"Hello, World"`
-
- * Multi-line strings start with `<-
- echo %Path%
-
- go version
-
- go env
-
- go get -t ./...
-
-build_script:
-- cmd: go test -v ./...
diff --git a/vendor/github.com/hashicorp/hcl/decoder.go b/vendor/github.com/hashicorp/hcl/decoder.go
deleted file mode 100644
index bed9ebbe..00000000
--- a/vendor/github.com/hashicorp/hcl/decoder.go
+++ /dev/null
@@ -1,729 +0,0 @@
-package hcl
-
-import (
- "errors"
- "fmt"
- "reflect"
- "sort"
- "strconv"
- "strings"
-
- "github.com/hashicorp/hcl/hcl/ast"
- "github.com/hashicorp/hcl/hcl/parser"
- "github.com/hashicorp/hcl/hcl/token"
-)
-
-// This is the tag to use with structures to have settings for HCL
-const tagName = "hcl"
-
-var (
- // nodeType holds a reference to the type of ast.Node
- nodeType reflect.Type = findNodeType()
-)
-
-// Unmarshal accepts a byte slice as input and writes the
-// data to the value pointed to by v.
-func Unmarshal(bs []byte, v interface{}) error {
- root, err := parse(bs)
- if err != nil {
- return err
- }
-
- return DecodeObject(v, root)
-}
-
-// Decode reads the given input and decodes it into the structure
-// given by `out`.
-func Decode(out interface{}, in string) error {
- obj, err := Parse(in)
- if err != nil {
- return err
- }
-
- return DecodeObject(out, obj)
-}
-
-// DecodeObject is a lower-level version of Decode. It decodes a
-// raw Object into the given output.
-func DecodeObject(out interface{}, n ast.Node) error {
- val := reflect.ValueOf(out)
- if val.Kind() != reflect.Ptr {
- return errors.New("result must be a pointer")
- }
-
- // If we have the file, we really decode the root node
- if f, ok := n.(*ast.File); ok {
- n = f.Node
- }
-
- var d decoder
- return d.decode("root", n, val.Elem())
-}
-
-type decoder struct {
- stack []reflect.Kind
-}
-
-func (d *decoder) decode(name string, node ast.Node, result reflect.Value) error {
- k := result
-
- // If we have an interface with a valid value, we use that
- // for the check.
- if result.Kind() == reflect.Interface {
- elem := result.Elem()
- if elem.IsValid() {
- k = elem
- }
- }
-
- // Push current onto stack unless it is an interface.
- if k.Kind() != reflect.Interface {
- d.stack = append(d.stack, k.Kind())
-
- // Schedule a pop
- defer func() {
- d.stack = d.stack[:len(d.stack)-1]
- }()
- }
-
- switch k.Kind() {
- case reflect.Bool:
- return d.decodeBool(name, node, result)
- case reflect.Float32, reflect.Float64:
- return d.decodeFloat(name, node, result)
- case reflect.Int, reflect.Int32, reflect.Int64:
- return d.decodeInt(name, node, result)
- case reflect.Interface:
- // When we see an interface, we make our own thing
- return d.decodeInterface(name, node, result)
- case reflect.Map:
- return d.decodeMap(name, node, result)
- case reflect.Ptr:
- return d.decodePtr(name, node, result)
- case reflect.Slice:
- return d.decodeSlice(name, node, result)
- case reflect.String:
- return d.decodeString(name, node, result)
- case reflect.Struct:
- return d.decodeStruct(name, node, result)
- default:
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: unknown kind to decode into: %s", name, k.Kind()),
- }
- }
-}
-
-func (d *decoder) decodeBool(name string, node ast.Node, result reflect.Value) error {
- switch n := node.(type) {
- case *ast.LiteralType:
- if n.Token.Type == token.BOOL {
- v, err := strconv.ParseBool(n.Token.Text)
- if err != nil {
- return err
- }
-
- result.Set(reflect.ValueOf(v))
- return nil
- }
- }
-
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: unknown type %T", name, node),
- }
-}
-
-func (d *decoder) decodeFloat(name string, node ast.Node, result reflect.Value) error {
- switch n := node.(type) {
- case *ast.LiteralType:
- if n.Token.Type == token.FLOAT || n.Token.Type == token.NUMBER {
- v, err := strconv.ParseFloat(n.Token.Text, 64)
- if err != nil {
- return err
- }
-
- result.Set(reflect.ValueOf(v).Convert(result.Type()))
- return nil
- }
- }
-
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: unknown type %T", name, node),
- }
-}
-
-func (d *decoder) decodeInt(name string, node ast.Node, result reflect.Value) error {
- switch n := node.(type) {
- case *ast.LiteralType:
- switch n.Token.Type {
- case token.NUMBER:
- v, err := strconv.ParseInt(n.Token.Text, 0, 0)
- if err != nil {
- return err
- }
-
- if result.Kind() == reflect.Interface {
- result.Set(reflect.ValueOf(int(v)))
- } else {
- result.SetInt(v)
- }
- return nil
- case token.STRING:
- v, err := strconv.ParseInt(n.Token.Value().(string), 0, 0)
- if err != nil {
- return err
- }
-
- if result.Kind() == reflect.Interface {
- result.Set(reflect.ValueOf(int(v)))
- } else {
- result.SetInt(v)
- }
- return nil
- }
- }
-
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: unknown type %T", name, node),
- }
-}
-
-func (d *decoder) decodeInterface(name string, node ast.Node, result reflect.Value) error {
- // When we see an ast.Node, we retain the value to enable deferred decoding.
- // Very useful in situations where we want to preserve ast.Node information
- // like Pos
- if result.Type() == nodeType && result.CanSet() {
- result.Set(reflect.ValueOf(node))
- return nil
- }
-
- var set reflect.Value
- redecode := true
-
- // For testing types, ObjectType should just be treated as a list. We
- // set this to a temporary var because we want to pass in the real node.
- testNode := node
- if ot, ok := node.(*ast.ObjectType); ok {
- testNode = ot.List
- }
-
- switch n := testNode.(type) {
- case *ast.ObjectList:
- // If we're at the root or we're directly within a slice, then we
- // decode objects into map[string]interface{}, otherwise we decode
- // them into lists.
- if len(d.stack) == 0 || d.stack[len(d.stack)-1] == reflect.Slice {
- var temp map[string]interface{}
- tempVal := reflect.ValueOf(temp)
- result := reflect.MakeMap(
- reflect.MapOf(
- reflect.TypeOf(""),
- tempVal.Type().Elem()))
-
- set = result
- } else {
- var temp []map[string]interface{}
- tempVal := reflect.ValueOf(temp)
- result := reflect.MakeSlice(
- reflect.SliceOf(tempVal.Type().Elem()), 0, len(n.Items))
- set = result
- }
- case *ast.ObjectType:
- // If we're at the root or we're directly within a slice, then we
- // decode objects into map[string]interface{}, otherwise we decode
- // them into lists.
- if len(d.stack) == 0 || d.stack[len(d.stack)-1] == reflect.Slice {
- var temp map[string]interface{}
- tempVal := reflect.ValueOf(temp)
- result := reflect.MakeMap(
- reflect.MapOf(
- reflect.TypeOf(""),
- tempVal.Type().Elem()))
-
- set = result
- } else {
- var temp []map[string]interface{}
- tempVal := reflect.ValueOf(temp)
- result := reflect.MakeSlice(
- reflect.SliceOf(tempVal.Type().Elem()), 0, 1)
- set = result
- }
- case *ast.ListType:
- var temp []interface{}
- tempVal := reflect.ValueOf(temp)
- result := reflect.MakeSlice(
- reflect.SliceOf(tempVal.Type().Elem()), 0, 0)
- set = result
- case *ast.LiteralType:
- switch n.Token.Type {
- case token.BOOL:
- var result bool
- set = reflect.Indirect(reflect.New(reflect.TypeOf(result)))
- case token.FLOAT:
- var result float64
- set = reflect.Indirect(reflect.New(reflect.TypeOf(result)))
- case token.NUMBER:
- var result int
- set = reflect.Indirect(reflect.New(reflect.TypeOf(result)))
- case token.STRING, token.HEREDOC:
- set = reflect.Indirect(reflect.New(reflect.TypeOf("")))
- default:
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: cannot decode into interface: %T", name, node),
- }
- }
- default:
- return fmt.Errorf(
- "%s: cannot decode into interface: %T",
- name, node)
- }
-
- // Set the result to what its supposed to be, then reset
- // result so we don't reflect into this method anymore.
- result.Set(set)
-
- if redecode {
- // Revisit the node so that we can use the newly instantiated
- // thing and populate it.
- if err := d.decode(name, node, result); err != nil {
- return err
- }
- }
-
- return nil
-}
-
-func (d *decoder) decodeMap(name string, node ast.Node, result reflect.Value) error {
- if item, ok := node.(*ast.ObjectItem); ok {
- node = &ast.ObjectList{Items: []*ast.ObjectItem{item}}
- }
-
- if ot, ok := node.(*ast.ObjectType); ok {
- node = ot.List
- }
-
- n, ok := node.(*ast.ObjectList)
- if !ok {
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: not an object type for map (%T)", name, node),
- }
- }
-
- // If we have an interface, then we can address the interface,
- // but not the slice itself, so get the element but set the interface
- set := result
- if result.Kind() == reflect.Interface {
- result = result.Elem()
- }
-
- resultType := result.Type()
- resultElemType := resultType.Elem()
- resultKeyType := resultType.Key()
- if resultKeyType.Kind() != reflect.String {
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: map must have string keys", name),
- }
- }
-
- // Make a map if it is nil
- resultMap := result
- if result.IsNil() {
- resultMap = reflect.MakeMap(
- reflect.MapOf(resultKeyType, resultElemType))
- }
-
- // Go through each element and decode it.
- done := make(map[string]struct{})
- for _, item := range n.Items {
- if item.Val == nil {
- continue
- }
-
- // github.com/hashicorp/terraform/issue/5740
- if len(item.Keys) == 0 {
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: map must have string keys", name),
- }
- }
-
- // Get the key we're dealing with, which is the first item
- keyStr := item.Keys[0].Token.Value().(string)
-
- // If we've already processed this key, then ignore it
- if _, ok := done[keyStr]; ok {
- continue
- }
-
- // Determine the value. If we have more than one key, then we
- // get the objectlist of only these keys.
- itemVal := item.Val
- if len(item.Keys) > 1 {
- itemVal = n.Filter(keyStr)
- done[keyStr] = struct{}{}
- }
-
- // Make the field name
- fieldName := fmt.Sprintf("%s.%s", name, keyStr)
-
- // Get the key/value as reflection values
- key := reflect.ValueOf(keyStr)
- val := reflect.Indirect(reflect.New(resultElemType))
-
- // If we have a pre-existing value in the map, use that
- oldVal := resultMap.MapIndex(key)
- if oldVal.IsValid() {
- val.Set(oldVal)
- }
-
- // Decode!
- if err := d.decode(fieldName, itemVal, val); err != nil {
- return err
- }
-
- // Set the value on the map
- resultMap.SetMapIndex(key, val)
- }
-
- // Set the final map if we can
- set.Set(resultMap)
- return nil
-}
-
-func (d *decoder) decodePtr(name string, node ast.Node, result reflect.Value) error {
- // Create an element of the concrete (non pointer) type and decode
- // into that. Then set the value of the pointer to this type.
- resultType := result.Type()
- resultElemType := resultType.Elem()
- val := reflect.New(resultElemType)
- if err := d.decode(name, node, reflect.Indirect(val)); err != nil {
- return err
- }
-
- result.Set(val)
- return nil
-}
-
-func (d *decoder) decodeSlice(name string, node ast.Node, result reflect.Value) error {
- // If we have an interface, then we can address the interface,
- // but not the slice itself, so get the element but set the interface
- set := result
- if result.Kind() == reflect.Interface {
- result = result.Elem()
- }
- // Create the slice if it isn't nil
- resultType := result.Type()
- resultElemType := resultType.Elem()
- if result.IsNil() {
- resultSliceType := reflect.SliceOf(resultElemType)
- result = reflect.MakeSlice(
- resultSliceType, 0, 0)
- }
-
- // Figure out the items we'll be copying into the slice
- var items []ast.Node
- switch n := node.(type) {
- case *ast.ObjectList:
- items = make([]ast.Node, len(n.Items))
- for i, item := range n.Items {
- items[i] = item
- }
- case *ast.ObjectType:
- items = []ast.Node{n}
- case *ast.ListType:
- items = n.List
- default:
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("unknown slice type: %T", node),
- }
- }
-
- for i, item := range items {
- fieldName := fmt.Sprintf("%s[%d]", name, i)
-
- // Decode
- val := reflect.Indirect(reflect.New(resultElemType))
-
- // if item is an object that was decoded from ambiguous JSON and
- // flattened, make sure it's expanded if it needs to decode into a
- // defined structure.
- item := expandObject(item, val)
-
- if err := d.decode(fieldName, item, val); err != nil {
- return err
- }
-
- // Append it onto the slice
- result = reflect.Append(result, val)
- }
-
- set.Set(result)
- return nil
-}
-
-// expandObject detects if an ambiguous JSON object was flattened to a List which
-// should be decoded into a struct, and expands the ast to properly deocode.
-func expandObject(node ast.Node, result reflect.Value) ast.Node {
- item, ok := node.(*ast.ObjectItem)
- if !ok {
- return node
- }
-
- elemType := result.Type()
-
- // our target type must be a struct
- switch elemType.Kind() {
- case reflect.Ptr:
- switch elemType.Elem().Kind() {
- case reflect.Struct:
- //OK
- default:
- return node
- }
- case reflect.Struct:
- //OK
- default:
- return node
- }
-
- // A list value will have a key and field name. If it had more fields,
- // it wouldn't have been flattened.
- if len(item.Keys) != 2 {
- return node
- }
-
- keyToken := item.Keys[0].Token
- item.Keys = item.Keys[1:]
-
- // we need to un-flatten the ast enough to decode
- newNode := &ast.ObjectItem{
- Keys: []*ast.ObjectKey{
- &ast.ObjectKey{
- Token: keyToken,
- },
- },
- Val: &ast.ObjectType{
- List: &ast.ObjectList{
- Items: []*ast.ObjectItem{item},
- },
- },
- }
-
- return newNode
-}
-
-func (d *decoder) decodeString(name string, node ast.Node, result reflect.Value) error {
- switch n := node.(type) {
- case *ast.LiteralType:
- switch n.Token.Type {
- case token.NUMBER:
- result.Set(reflect.ValueOf(n.Token.Text).Convert(result.Type()))
- return nil
- case token.STRING, token.HEREDOC:
- result.Set(reflect.ValueOf(n.Token.Value()).Convert(result.Type()))
- return nil
- }
- }
-
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: unknown type for string %T", name, node),
- }
-}
-
-func (d *decoder) decodeStruct(name string, node ast.Node, result reflect.Value) error {
- var item *ast.ObjectItem
- if it, ok := node.(*ast.ObjectItem); ok {
- item = it
- node = it.Val
- }
-
- if ot, ok := node.(*ast.ObjectType); ok {
- node = ot.List
- }
-
- // Handle the special case where the object itself is a literal. Previously
- // the yacc parser would always ensure top-level elements were arrays. The new
- // parser does not make the same guarantees, thus we need to convert any
- // top-level literal elements into a list.
- if _, ok := node.(*ast.LiteralType); ok && item != nil {
- node = &ast.ObjectList{Items: []*ast.ObjectItem{item}}
- }
-
- list, ok := node.(*ast.ObjectList)
- if !ok {
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: not an object type for struct (%T)", name, node),
- }
- }
-
- // This slice will keep track of all the structs we'll be decoding.
- // There can be more than one struct if there are embedded structs
- // that are squashed.
- structs := make([]reflect.Value, 1, 5)
- structs[0] = result
-
- // Compile the list of all the fields that we're going to be decoding
- // from all the structs.
- type field struct {
- field reflect.StructField
- val reflect.Value
- }
- fields := []field{}
- for len(structs) > 0 {
- structVal := structs[0]
- structs = structs[1:]
-
- structType := structVal.Type()
- for i := 0; i < structType.NumField(); i++ {
- fieldType := structType.Field(i)
- tagParts := strings.Split(fieldType.Tag.Get(tagName), ",")
-
- // Ignore fields with tag name "-"
- if tagParts[0] == "-" {
- continue
- }
-
- if fieldType.Anonymous {
- fieldKind := fieldType.Type.Kind()
- if fieldKind != reflect.Struct {
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: unsupported type to struct: %s",
- fieldType.Name, fieldKind),
- }
- }
-
- // We have an embedded field. We "squash" the fields down
- // if specified in the tag.
- squash := false
- for _, tag := range tagParts[1:] {
- if tag == "squash" {
- squash = true
- break
- }
- }
-
- if squash {
- structs = append(
- structs, result.FieldByName(fieldType.Name))
- continue
- }
- }
-
- // Normal struct field, store it away
- fields = append(fields, field{fieldType, structVal.Field(i)})
- }
- }
-
- usedKeys := make(map[string]struct{})
- decodedFields := make([]string, 0, len(fields))
- decodedFieldsVal := make([]reflect.Value, 0)
- unusedKeysVal := make([]reflect.Value, 0)
- for _, f := range fields {
- field, fieldValue := f.field, f.val
- if !fieldValue.IsValid() {
- // This should never happen
- panic("field is not valid")
- }
-
- // If we can't set the field, then it is unexported or something,
- // and we just continue onwards.
- if !fieldValue.CanSet() {
- continue
- }
-
- fieldName := field.Name
-
- tagValue := field.Tag.Get(tagName)
- tagParts := strings.SplitN(tagValue, ",", 2)
- if len(tagParts) >= 2 {
- switch tagParts[1] {
- case "decodedFields":
- decodedFieldsVal = append(decodedFieldsVal, fieldValue)
- continue
- case "key":
- if item == nil {
- return &parser.PosError{
- Pos: node.Pos(),
- Err: fmt.Errorf("%s: %s asked for 'key', impossible",
- name, fieldName),
- }
- }
-
- fieldValue.SetString(item.Keys[0].Token.Value().(string))
- continue
- case "unusedKeys":
- unusedKeysVal = append(unusedKeysVal, fieldValue)
- continue
- }
- }
-
- if tagParts[0] != "" {
- fieldName = tagParts[0]
- }
-
- // Determine the element we'll use to decode. If it is a single
- // match (only object with the field), then we decode it exactly.
- // If it is a prefix match, then we decode the matches.
- filter := list.Filter(fieldName)
-
- prefixMatches := filter.Children()
- matches := filter.Elem()
- if len(matches.Items) == 0 && len(prefixMatches.Items) == 0 {
- continue
- }
-
- // Track the used key
- usedKeys[fieldName] = struct{}{}
-
- // Create the field name and decode. We range over the elements
- // because we actually want the value.
- fieldName = fmt.Sprintf("%s.%s", name, fieldName)
- if len(prefixMatches.Items) > 0 {
- if err := d.decode(fieldName, prefixMatches, fieldValue); err != nil {
- return err
- }
- }
- for _, match := range matches.Items {
- var decodeNode ast.Node = match.Val
- if ot, ok := decodeNode.(*ast.ObjectType); ok {
- decodeNode = &ast.ObjectList{Items: ot.List.Items}
- }
-
- if err := d.decode(fieldName, decodeNode, fieldValue); err != nil {
- return err
- }
- }
-
- decodedFields = append(decodedFields, field.Name)
- }
-
- if len(decodedFieldsVal) > 0 {
- // Sort it so that it is deterministic
- sort.Strings(decodedFields)
-
- for _, v := range decodedFieldsVal {
- v.Set(reflect.ValueOf(decodedFields))
- }
- }
-
- return nil
-}
-
-// findNodeType returns the type of ast.Node
-func findNodeType() reflect.Type {
- var nodeContainer struct {
- Node ast.Node
- }
- value := reflect.ValueOf(nodeContainer).FieldByName("Node")
- return value.Type()
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl.go b/vendor/github.com/hashicorp/hcl/hcl.go
deleted file mode 100644
index 575a20b5..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl.go
+++ /dev/null
@@ -1,11 +0,0 @@
-// Package hcl decodes HCL into usable Go structures.
-//
-// hcl input can come in either pure HCL format or JSON format.
-// It can be parsed into an AST, and then decoded into a structure,
-// or it can be decoded directly from a string into a structure.
-//
-// If you choose to parse HCL into a raw AST, the benefit is that you
-// can write custom visitor implementations to implement custom
-// semantic checks. By default, HCL does not perform any semantic
-// checks.
-package hcl
diff --git a/vendor/github.com/hashicorp/hcl/hcl/ast/ast.go b/vendor/github.com/hashicorp/hcl/hcl/ast/ast.go
deleted file mode 100644
index 6e5ef654..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/ast/ast.go
+++ /dev/null
@@ -1,219 +0,0 @@
-// Package ast declares the types used to represent syntax trees for HCL
-// (HashiCorp Configuration Language)
-package ast
-
-import (
- "fmt"
- "strings"
-
- "github.com/hashicorp/hcl/hcl/token"
-)
-
-// Node is an element in the abstract syntax tree.
-type Node interface {
- node()
- Pos() token.Pos
-}
-
-func (File) node() {}
-func (ObjectList) node() {}
-func (ObjectKey) node() {}
-func (ObjectItem) node() {}
-func (Comment) node() {}
-func (CommentGroup) node() {}
-func (ObjectType) node() {}
-func (LiteralType) node() {}
-func (ListType) node() {}
-
-// File represents a single HCL file
-type File struct {
- Node Node // usually a *ObjectList
- Comments []*CommentGroup // list of all comments in the source
-}
-
-func (f *File) Pos() token.Pos {
- return f.Node.Pos()
-}
-
-// ObjectList represents a list of ObjectItems. An HCL file itself is an
-// ObjectList.
-type ObjectList struct {
- Items []*ObjectItem
-}
-
-func (o *ObjectList) Add(item *ObjectItem) {
- o.Items = append(o.Items, item)
-}
-
-// Filter filters out the objects with the given key list as a prefix.
-//
-// The returned list of objects contain ObjectItems where the keys have
-// this prefix already stripped off. This might result in objects with
-// zero-length key lists if they have no children.
-//
-// If no matches are found, an empty ObjectList (non-nil) is returned.
-func (o *ObjectList) Filter(keys ...string) *ObjectList {
- var result ObjectList
- for _, item := range o.Items {
- // If there aren't enough keys, then ignore this
- if len(item.Keys) < len(keys) {
- continue
- }
-
- match := true
- for i, key := range item.Keys[:len(keys)] {
- key := key.Token.Value().(string)
- if key != keys[i] && !strings.EqualFold(key, keys[i]) {
- match = false
- break
- }
- }
- if !match {
- continue
- }
-
- // Strip off the prefix from the children
- newItem := *item
- newItem.Keys = newItem.Keys[len(keys):]
- result.Add(&newItem)
- }
-
- return &result
-}
-
-// Children returns further nested objects (key length > 0) within this
-// ObjectList. This should be used with Filter to get at child items.
-func (o *ObjectList) Children() *ObjectList {
- var result ObjectList
- for _, item := range o.Items {
- if len(item.Keys) > 0 {
- result.Add(item)
- }
- }
-
- return &result
-}
-
-// Elem returns items in the list that are direct element assignments
-// (key length == 0). This should be used with Filter to get at elements.
-func (o *ObjectList) Elem() *ObjectList {
- var result ObjectList
- for _, item := range o.Items {
- if len(item.Keys) == 0 {
- result.Add(item)
- }
- }
-
- return &result
-}
-
-func (o *ObjectList) Pos() token.Pos {
- // always returns the uninitiliazed position
- return o.Items[0].Pos()
-}
-
-// ObjectItem represents a HCL Object Item. An item is represented with a key
-// (or keys). It can be an assignment or an object (both normal and nested)
-type ObjectItem struct {
- // keys is only one length long if it's of type assignment. If it's a
- // nested object it can be larger than one. In that case "assign" is
- // invalid as there is no assignments for a nested object.
- Keys []*ObjectKey
-
- // assign contains the position of "=", if any
- Assign token.Pos
-
- // val is the item itself. It can be an object,list, number, bool or a
- // string. If key length is larger than one, val can be only of type
- // Object.
- Val Node
-
- LeadComment *CommentGroup // associated lead comment
- LineComment *CommentGroup // associated line comment
-}
-
-func (o *ObjectItem) Pos() token.Pos {
- // I'm not entirely sure what causes this, but removing this causes
- // a test failure. We should investigate at some point.
- if len(o.Keys) == 0 {
- return token.Pos{}
- }
-
- return o.Keys[0].Pos()
-}
-
-// ObjectKeys are either an identifier or of type string.
-type ObjectKey struct {
- Token token.Token
-}
-
-func (o *ObjectKey) Pos() token.Pos {
- return o.Token.Pos
-}
-
-// LiteralType represents a literal of basic type. Valid types are:
-// token.NUMBER, token.FLOAT, token.BOOL and token.STRING
-type LiteralType struct {
- Token token.Token
-
- // comment types, only used when in a list
- LeadComment *CommentGroup
- LineComment *CommentGroup
-}
-
-func (l *LiteralType) Pos() token.Pos {
- return l.Token.Pos
-}
-
-// ListStatement represents a HCL List type
-type ListType struct {
- Lbrack token.Pos // position of "["
- Rbrack token.Pos // position of "]"
- List []Node // the elements in lexical order
-}
-
-func (l *ListType) Pos() token.Pos {
- return l.Lbrack
-}
-
-func (l *ListType) Add(node Node) {
- l.List = append(l.List, node)
-}
-
-// ObjectType represents a HCL Object Type
-type ObjectType struct {
- Lbrace token.Pos // position of "{"
- Rbrace token.Pos // position of "}"
- List *ObjectList // the nodes in lexical order
-}
-
-func (o *ObjectType) Pos() token.Pos {
- return o.Lbrace
-}
-
-// Comment node represents a single //, # style or /*- style commment
-type Comment struct {
- Start token.Pos // position of / or #
- Text string
-}
-
-func (c *Comment) Pos() token.Pos {
- return c.Start
-}
-
-// CommentGroup node represents a sequence of comments with no other tokens and
-// no empty lines between.
-type CommentGroup struct {
- List []*Comment // len(List) > 0
-}
-
-func (c *CommentGroup) Pos() token.Pos {
- return c.List[0].Pos()
-}
-
-//-------------------------------------------------------------------
-// GoStringer
-//-------------------------------------------------------------------
-
-func (o *ObjectKey) GoString() string { return fmt.Sprintf("*%#v", *o) }
-func (o *ObjectList) GoString() string { return fmt.Sprintf("*%#v", *o) }
diff --git a/vendor/github.com/hashicorp/hcl/hcl/ast/walk.go b/vendor/github.com/hashicorp/hcl/hcl/ast/walk.go
deleted file mode 100644
index ba07ad42..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/ast/walk.go
+++ /dev/null
@@ -1,52 +0,0 @@
-package ast
-
-import "fmt"
-
-// WalkFunc describes a function to be called for each node during a Walk. The
-// returned node can be used to rewrite the AST. Walking stops the returned
-// bool is false.
-type WalkFunc func(Node) (Node, bool)
-
-// Walk traverses an AST in depth-first order: It starts by calling fn(node);
-// node must not be nil. If fn returns true, Walk invokes fn recursively for
-// each of the non-nil children of node, followed by a call of fn(nil). The
-// returned node of fn can be used to rewrite the passed node to fn.
-func Walk(node Node, fn WalkFunc) Node {
- rewritten, ok := fn(node)
- if !ok {
- return rewritten
- }
-
- switch n := node.(type) {
- case *File:
- n.Node = Walk(n.Node, fn)
- case *ObjectList:
- for i, item := range n.Items {
- n.Items[i] = Walk(item, fn).(*ObjectItem)
- }
- case *ObjectKey:
- // nothing to do
- case *ObjectItem:
- for i, k := range n.Keys {
- n.Keys[i] = Walk(k, fn).(*ObjectKey)
- }
-
- if n.Val != nil {
- n.Val = Walk(n.Val, fn)
- }
- case *LiteralType:
- // nothing to do
- case *ListType:
- for i, l := range n.List {
- n.List[i] = Walk(l, fn)
- }
- case *ObjectType:
- n.List = Walk(n.List, fn).(*ObjectList)
- default:
- // should we panic here?
- fmt.Printf("unknown type: %T\n", n)
- }
-
- fn(nil)
- return rewritten
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/parser/error.go b/vendor/github.com/hashicorp/hcl/hcl/parser/error.go
deleted file mode 100644
index 5c99381d..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/parser/error.go
+++ /dev/null
@@ -1,17 +0,0 @@
-package parser
-
-import (
- "fmt"
-
- "github.com/hashicorp/hcl/hcl/token"
-)
-
-// PosError is a parse error that contains a position.
-type PosError struct {
- Pos token.Pos
- Err error
-}
-
-func (e *PosError) Error() string {
- return fmt.Sprintf("At %s: %s", e.Pos, e.Err)
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/parser/parser.go b/vendor/github.com/hashicorp/hcl/hcl/parser/parser.go
deleted file mode 100644
index 64c83bcf..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/parser/parser.go
+++ /dev/null
@@ -1,532 +0,0 @@
-// Package parser implements a parser for HCL (HashiCorp Configuration
-// Language)
-package parser
-
-import (
- "bytes"
- "errors"
- "fmt"
- "strings"
-
- "github.com/hashicorp/hcl/hcl/ast"
- "github.com/hashicorp/hcl/hcl/scanner"
- "github.com/hashicorp/hcl/hcl/token"
-)
-
-type Parser struct {
- sc *scanner.Scanner
-
- // Last read token
- tok token.Token
- commaPrev token.Token
-
- comments []*ast.CommentGroup
- leadComment *ast.CommentGroup // last lead comment
- lineComment *ast.CommentGroup // last line comment
-
- enableTrace bool
- indent int
- n int // buffer size (max = 1)
-}
-
-func newParser(src []byte) *Parser {
- return &Parser{
- sc: scanner.New(src),
- }
-}
-
-// Parse returns the fully parsed source and returns the abstract syntax tree.
-func Parse(src []byte) (*ast.File, error) {
- // normalize all line endings
- // since the scanner and output only work with "\n" line endings, we may
- // end up with dangling "\r" characters in the parsed data.
- src = bytes.Replace(src, []byte("\r\n"), []byte("\n"), -1)
-
- p := newParser(src)
- return p.Parse()
-}
-
-var errEofToken = errors.New("EOF token found")
-
-// Parse returns the fully parsed source and returns the abstract syntax tree.
-func (p *Parser) Parse() (*ast.File, error) {
- f := &ast.File{}
- var err, scerr error
- p.sc.Error = func(pos token.Pos, msg string) {
- scerr = &PosError{Pos: pos, Err: errors.New(msg)}
- }
-
- f.Node, err = p.objectList(false)
- if scerr != nil {
- return nil, scerr
- }
- if err != nil {
- return nil, err
- }
-
- f.Comments = p.comments
- return f, nil
-}
-
-// objectList parses a list of items within an object (generally k/v pairs).
-// The parameter" obj" tells this whether to we are within an object (braces:
-// '{', '}') or just at the top level. If we're within an object, we end
-// at an RBRACE.
-func (p *Parser) objectList(obj bool) (*ast.ObjectList, error) {
- defer un(trace(p, "ParseObjectList"))
- node := &ast.ObjectList{}
-
- for {
- if obj {
- tok := p.scan()
- p.unscan()
- if tok.Type == token.RBRACE {
- break
- }
- }
-
- n, err := p.objectItem()
- if err == errEofToken {
- break // we are finished
- }
-
- // we don't return a nil node, because might want to use already
- // collected items.
- if err != nil {
- return node, err
- }
-
- node.Add(n)
-
- // object lists can be optionally comma-delimited e.g. when a list of maps
- // is being expressed, so a comma is allowed here - it's simply consumed
- tok := p.scan()
- if tok.Type != token.COMMA {
- p.unscan()
- }
- }
- return node, nil
-}
-
-func (p *Parser) consumeComment() (comment *ast.Comment, endline int) {
- endline = p.tok.Pos.Line
-
- // count the endline if it's multiline comment, ie starting with /*
- if len(p.tok.Text) > 1 && p.tok.Text[1] == '*' {
- // don't use range here - no need to decode Unicode code points
- for i := 0; i < len(p.tok.Text); i++ {
- if p.tok.Text[i] == '\n' {
- endline++
- }
- }
- }
-
- comment = &ast.Comment{Start: p.tok.Pos, Text: p.tok.Text}
- p.tok = p.sc.Scan()
- return
-}
-
-func (p *Parser) consumeCommentGroup(n int) (comments *ast.CommentGroup, endline int) {
- var list []*ast.Comment
- endline = p.tok.Pos.Line
-
- for p.tok.Type == token.COMMENT && p.tok.Pos.Line <= endline+n {
- var comment *ast.Comment
- comment, endline = p.consumeComment()
- list = append(list, comment)
- }
-
- // add comment group to the comments list
- comments = &ast.CommentGroup{List: list}
- p.comments = append(p.comments, comments)
-
- return
-}
-
-// objectItem parses a single object item
-func (p *Parser) objectItem() (*ast.ObjectItem, error) {
- defer un(trace(p, "ParseObjectItem"))
-
- keys, err := p.objectKey()
- if len(keys) > 0 && err == errEofToken {
- // We ignore eof token here since it is an error if we didn't
- // receive a value (but we did receive a key) for the item.
- err = nil
- }
- if len(keys) > 0 && err != nil && p.tok.Type == token.RBRACE {
- // This is a strange boolean statement, but what it means is:
- // We have keys with no value, and we're likely in an object
- // (since RBrace ends an object). For this, we set err to nil so
- // we continue and get the error below of having the wrong value
- // type.
- err = nil
-
- // Reset the token type so we don't think it completed fine. See
- // objectType which uses p.tok.Type to check if we're done with
- // the object.
- p.tok.Type = token.EOF
- }
- if err != nil {
- return nil, err
- }
-
- o := &ast.ObjectItem{
- Keys: keys,
- }
-
- if p.leadComment != nil {
- o.LeadComment = p.leadComment
- p.leadComment = nil
- }
-
- switch p.tok.Type {
- case token.ASSIGN:
- o.Assign = p.tok.Pos
- o.Val, err = p.object()
- if err != nil {
- return nil, err
- }
- case token.LBRACE:
- o.Val, err = p.objectType()
- if err != nil {
- return nil, err
- }
- default:
- keyStr := make([]string, 0, len(keys))
- for _, k := range keys {
- keyStr = append(keyStr, k.Token.Text)
- }
-
- return nil, &PosError{
- Pos: p.tok.Pos,
- Err: fmt.Errorf(
- "key '%s' expected start of object ('{') or assignment ('=')",
- strings.Join(keyStr, " ")),
- }
- }
-
- // key=#comment
- // val
- if p.lineComment != nil {
- o.LineComment, p.lineComment = p.lineComment, nil
- }
-
- // do a look-ahead for line comment
- p.scan()
- if len(keys) > 0 && o.Val.Pos().Line == keys[0].Pos().Line && p.lineComment != nil {
- o.LineComment = p.lineComment
- p.lineComment = nil
- }
- p.unscan()
- return o, nil
-}
-
-// objectKey parses an object key and returns a ObjectKey AST
-func (p *Parser) objectKey() ([]*ast.ObjectKey, error) {
- keyCount := 0
- keys := make([]*ast.ObjectKey, 0)
-
- for {
- tok := p.scan()
- switch tok.Type {
- case token.EOF:
- // It is very important to also return the keys here as well as
- // the error. This is because we need to be able to tell if we
- // did parse keys prior to finding the EOF, or if we just found
- // a bare EOF.
- return keys, errEofToken
- case token.ASSIGN:
- // assignment or object only, but not nested objects. this is not
- // allowed: `foo bar = {}`
- if keyCount > 1 {
- return nil, &PosError{
- Pos: p.tok.Pos,
- Err: fmt.Errorf("nested object expected: LBRACE got: %s", p.tok.Type),
- }
- }
-
- if keyCount == 0 {
- return nil, &PosError{
- Pos: p.tok.Pos,
- Err: errors.New("no object keys found!"),
- }
- }
-
- return keys, nil
- case token.LBRACE:
- var err error
-
- // If we have no keys, then it is a syntax error. i.e. {{}} is not
- // allowed.
- if len(keys) == 0 {
- err = &PosError{
- Pos: p.tok.Pos,
- Err: fmt.Errorf("expected: IDENT | STRING got: %s", p.tok.Type),
- }
- }
-
- // object
- return keys, err
- case token.IDENT, token.STRING:
- keyCount++
- keys = append(keys, &ast.ObjectKey{Token: p.tok})
- case token.ILLEGAL:
- return keys, &PosError{
- Pos: p.tok.Pos,
- Err: fmt.Errorf("illegal character"),
- }
- default:
- return keys, &PosError{
- Pos: p.tok.Pos,
- Err: fmt.Errorf("expected: IDENT | STRING | ASSIGN | LBRACE got: %s", p.tok.Type),
- }
- }
- }
-}
-
-// object parses any type of object, such as number, bool, string, object or
-// list.
-func (p *Parser) object() (ast.Node, error) {
- defer un(trace(p, "ParseType"))
- tok := p.scan()
-
- switch tok.Type {
- case token.NUMBER, token.FLOAT, token.BOOL, token.STRING, token.HEREDOC:
- return p.literalType()
- case token.LBRACE:
- return p.objectType()
- case token.LBRACK:
- return p.listType()
- case token.COMMENT:
- // implement comment
- case token.EOF:
- return nil, errEofToken
- }
-
- return nil, &PosError{
- Pos: tok.Pos,
- Err: fmt.Errorf("Unknown token: %+v", tok),
- }
-}
-
-// objectType parses an object type and returns a ObjectType AST
-func (p *Parser) objectType() (*ast.ObjectType, error) {
- defer un(trace(p, "ParseObjectType"))
-
- // we assume that the currently scanned token is a LBRACE
- o := &ast.ObjectType{
- Lbrace: p.tok.Pos,
- }
-
- l, err := p.objectList(true)
-
- // if we hit RBRACE, we are good to go (means we parsed all Items), if it's
- // not a RBRACE, it's an syntax error and we just return it.
- if err != nil && p.tok.Type != token.RBRACE {
- return nil, err
- }
-
- // No error, scan and expect the ending to be a brace
- if tok := p.scan(); tok.Type != token.RBRACE {
- return nil, &PosError{
- Pos: tok.Pos,
- Err: fmt.Errorf("object expected closing RBRACE got: %s", tok.Type),
- }
- }
-
- o.List = l
- o.Rbrace = p.tok.Pos // advanced via parseObjectList
- return o, nil
-}
-
-// listType parses a list type and returns a ListType AST
-func (p *Parser) listType() (*ast.ListType, error) {
- defer un(trace(p, "ParseListType"))
-
- // we assume that the currently scanned token is a LBRACK
- l := &ast.ListType{
- Lbrack: p.tok.Pos,
- }
-
- needComma := false
- for {
- tok := p.scan()
- if needComma {
- switch tok.Type {
- case token.COMMA, token.RBRACK:
- default:
- return nil, &PosError{
- Pos: tok.Pos,
- Err: fmt.Errorf(
- "error parsing list, expected comma or list end, got: %s",
- tok.Type),
- }
- }
- }
- switch tok.Type {
- case token.BOOL, token.NUMBER, token.FLOAT, token.STRING, token.HEREDOC:
- node, err := p.literalType()
- if err != nil {
- return nil, err
- }
-
- // If there is a lead comment, apply it
- if p.leadComment != nil {
- node.LeadComment = p.leadComment
- p.leadComment = nil
- }
-
- l.Add(node)
- needComma = true
- case token.COMMA:
- // get next list item or we are at the end
- // do a look-ahead for line comment
- p.scan()
- if p.lineComment != nil && len(l.List) > 0 {
- lit, ok := l.List[len(l.List)-1].(*ast.LiteralType)
- if ok {
- lit.LineComment = p.lineComment
- l.List[len(l.List)-1] = lit
- p.lineComment = nil
- }
- }
- p.unscan()
-
- needComma = false
- continue
- case token.LBRACE:
- // Looks like a nested object, so parse it out
- node, err := p.objectType()
- if err != nil {
- return nil, &PosError{
- Pos: tok.Pos,
- Err: fmt.Errorf(
- "error while trying to parse object within list: %s", err),
- }
- }
- l.Add(node)
- needComma = true
- case token.LBRACK:
- node, err := p.listType()
- if err != nil {
- return nil, &PosError{
- Pos: tok.Pos,
- Err: fmt.Errorf(
- "error while trying to parse list within list: %s", err),
- }
- }
- l.Add(node)
- case token.RBRACK:
- // finished
- l.Rbrack = p.tok.Pos
- return l, nil
- default:
- return nil, &PosError{
- Pos: tok.Pos,
- Err: fmt.Errorf("unexpected token while parsing list: %s", tok.Type),
- }
- }
- }
-}
-
-// literalType parses a literal type and returns a LiteralType AST
-func (p *Parser) literalType() (*ast.LiteralType, error) {
- defer un(trace(p, "ParseLiteral"))
-
- return &ast.LiteralType{
- Token: p.tok,
- }, nil
-}
-
-// scan returns the next token from the underlying scanner. If a token has
-// been unscanned then read that instead. In the process, it collects any
-// comment groups encountered, and remembers the last lead and line comments.
-func (p *Parser) scan() token.Token {
- // If we have a token on the buffer, then return it.
- if p.n != 0 {
- p.n = 0
- return p.tok
- }
-
- // Otherwise read the next token from the scanner and Save it to the buffer
- // in case we unscan later.
- prev := p.tok
- p.tok = p.sc.Scan()
-
- if p.tok.Type == token.COMMENT {
- var comment *ast.CommentGroup
- var endline int
-
- // fmt.Printf("p.tok.Pos.Line = %+v prev: %d endline %d \n",
- // p.tok.Pos.Line, prev.Pos.Line, endline)
- if p.tok.Pos.Line == prev.Pos.Line {
- // The comment is on same line as the previous token; it
- // cannot be a lead comment but may be a line comment.
- comment, endline = p.consumeCommentGroup(0)
- if p.tok.Pos.Line != endline {
- // The next token is on a different line, thus
- // the last comment group is a line comment.
- p.lineComment = comment
- }
- }
-
- // consume successor comments, if any
- endline = -1
- for p.tok.Type == token.COMMENT {
- comment, endline = p.consumeCommentGroup(1)
- }
-
- if endline+1 == p.tok.Pos.Line && p.tok.Type != token.RBRACE {
- switch p.tok.Type {
- case token.RBRACE, token.RBRACK:
- // Do not count for these cases
- default:
- // The next token is following on the line immediately after the
- // comment group, thus the last comment group is a lead comment.
- p.leadComment = comment
- }
- }
-
- }
-
- return p.tok
-}
-
-// unscan pushes the previously read token back onto the buffer.
-func (p *Parser) unscan() {
- p.n = 1
-}
-
-// ----------------------------------------------------------------------------
-// Parsing support
-
-func (p *Parser) printTrace(a ...interface{}) {
- if !p.enableTrace {
- return
- }
-
- const dots = ". . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . "
- const n = len(dots)
- fmt.Printf("%5d:%3d: ", p.tok.Pos.Line, p.tok.Pos.Column)
-
- i := 2 * p.indent
- for i > n {
- fmt.Print(dots)
- i -= n
- }
- // i <= n
- fmt.Print(dots[0:i])
- fmt.Println(a...)
-}
-
-func trace(p *Parser, msg string) *Parser {
- p.printTrace(msg, "(")
- p.indent++
- return p
-}
-
-// Usage pattern: defer un(trace(p, "..."))
-func un(p *Parser) {
- p.indent--
- p.printTrace(")")
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/printer/nodes.go b/vendor/github.com/hashicorp/hcl/hcl/printer/nodes.go
deleted file mode 100644
index 7c038d12..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/printer/nodes.go
+++ /dev/null
@@ -1,789 +0,0 @@
-package printer
-
-import (
- "bytes"
- "fmt"
- "sort"
-
- "github.com/hashicorp/hcl/hcl/ast"
- "github.com/hashicorp/hcl/hcl/token"
-)
-
-const (
- blank = byte(' ')
- newline = byte('\n')
- tab = byte('\t')
- infinity = 1 << 30 // offset or line
-)
-
-var (
- unindent = []byte("\uE123") // in the private use space
-)
-
-type printer struct {
- cfg Config
- prev token.Pos
-
- comments []*ast.CommentGroup // may be nil, contains all comments
- standaloneComments []*ast.CommentGroup // contains all standalone comments (not assigned to any node)
-
- enableTrace bool
- indentTrace int
-}
-
-type ByPosition []*ast.CommentGroup
-
-func (b ByPosition) Len() int { return len(b) }
-func (b ByPosition) Swap(i, j int) { b[i], b[j] = b[j], b[i] }
-func (b ByPosition) Less(i, j int) bool { return b[i].Pos().Before(b[j].Pos()) }
-
-// collectComments comments all standalone comments which are not lead or line
-// comment
-func (p *printer) collectComments(node ast.Node) {
- // first collect all comments. This is already stored in
- // ast.File.(comments)
- ast.Walk(node, func(nn ast.Node) (ast.Node, bool) {
- switch t := nn.(type) {
- case *ast.File:
- p.comments = t.Comments
- return nn, false
- }
- return nn, true
- })
-
- standaloneComments := make(map[token.Pos]*ast.CommentGroup, 0)
- for _, c := range p.comments {
- standaloneComments[c.Pos()] = c
- }
-
- // next remove all lead and line comments from the overall comment map.
- // This will give us comments which are standalone, comments which are not
- // assigned to any kind of node.
- ast.Walk(node, func(nn ast.Node) (ast.Node, bool) {
- switch t := nn.(type) {
- case *ast.LiteralType:
- if t.LeadComment != nil {
- for _, comment := range t.LeadComment.List {
- if _, ok := standaloneComments[comment.Pos()]; ok {
- delete(standaloneComments, comment.Pos())
- }
- }
- }
-
- if t.LineComment != nil {
- for _, comment := range t.LineComment.List {
- if _, ok := standaloneComments[comment.Pos()]; ok {
- delete(standaloneComments, comment.Pos())
- }
- }
- }
- case *ast.ObjectItem:
- if t.LeadComment != nil {
- for _, comment := range t.LeadComment.List {
- if _, ok := standaloneComments[comment.Pos()]; ok {
- delete(standaloneComments, comment.Pos())
- }
- }
- }
-
- if t.LineComment != nil {
- for _, comment := range t.LineComment.List {
- if _, ok := standaloneComments[comment.Pos()]; ok {
- delete(standaloneComments, comment.Pos())
- }
- }
- }
- }
-
- return nn, true
- })
-
- for _, c := range standaloneComments {
- p.standaloneComments = append(p.standaloneComments, c)
- }
-
- sort.Sort(ByPosition(p.standaloneComments))
-}
-
-// output prints creates b printable HCL output and returns it.
-func (p *printer) output(n interface{}) []byte {
- var buf bytes.Buffer
-
- switch t := n.(type) {
- case *ast.File:
- // File doesn't trace so we add the tracing here
- defer un(trace(p, "File"))
- return p.output(t.Node)
- case *ast.ObjectList:
- defer un(trace(p, "ObjectList"))
-
- var index int
- for {
- // Determine the location of the next actual non-comment
- // item. If we're at the end, the next item is at "infinity"
- var nextItem token.Pos
- if index != len(t.Items) {
- nextItem = t.Items[index].Pos()
- } else {
- nextItem = token.Pos{Offset: infinity, Line: infinity}
- }
-
- // Go through the standalone comments in the file and print out
- // the comments that we should be for this object item.
- for _, c := range p.standaloneComments {
- // Go through all the comments in the group. The group
- // should be printed together, not separated by double newlines.
- printed := false
- newlinePrinted := false
- for _, comment := range c.List {
- // We only care about comments after the previous item
- // we've printed so that comments are printed in the
- // correct locations (between two objects for example).
- // And before the next item.
- if comment.Pos().After(p.prev) && comment.Pos().Before(nextItem) {
- // if we hit the end add newlines so we can print the comment
- // we don't do this if prev is invalid which means the
- // beginning of the file since the first comment should
- // be at the first line.
- if !newlinePrinted && p.prev.IsValid() && index == len(t.Items) {
- buf.Write([]byte{newline, newline})
- newlinePrinted = true
- }
-
- // Write the actual comment.
- buf.WriteString(comment.Text)
- buf.WriteByte(newline)
-
- // Set printed to true to note that we printed something
- printed = true
- }
- }
-
- // If we're not at the last item, write a new line so
- // that there is a newline separating this comment from
- // the next object.
- if printed && index != len(t.Items) {
- buf.WriteByte(newline)
- }
- }
-
- if index == len(t.Items) {
- break
- }
-
- buf.Write(p.output(t.Items[index]))
- if index != len(t.Items)-1 {
- // Always write a newline to separate us from the next item
- buf.WriteByte(newline)
-
- // Need to determine if we're going to separate the next item
- // with a blank line. The logic here is simple, though there
- // are a few conditions:
- //
- // 1. The next object is more than one line away anyways,
- // so we need an empty line.
- //
- // 2. The next object is not a "single line" object, so
- // we need an empty line.
- //
- // 3. This current object is not a single line object,
- // so we need an empty line.
- current := t.Items[index]
- next := t.Items[index+1]
- if next.Pos().Line != t.Items[index].Pos().Line+1 ||
- !p.isSingleLineObject(next) ||
- !p.isSingleLineObject(current) {
- buf.WriteByte(newline)
- }
- }
- index++
- }
- case *ast.ObjectKey:
- buf.WriteString(t.Token.Text)
- case *ast.ObjectItem:
- p.prev = t.Pos()
- buf.Write(p.objectItem(t))
- case *ast.LiteralType:
- buf.Write(p.literalType(t))
- case *ast.ListType:
- buf.Write(p.list(t))
- case *ast.ObjectType:
- buf.Write(p.objectType(t))
- default:
- fmt.Printf(" unknown type: %T\n", n)
- }
-
- return buf.Bytes()
-}
-
-func (p *printer) literalType(lit *ast.LiteralType) []byte {
- result := []byte(lit.Token.Text)
- switch lit.Token.Type {
- case token.HEREDOC:
- // Clear the trailing newline from heredocs
- if result[len(result)-1] == '\n' {
- result = result[:len(result)-1]
- }
-
- // Poison lines 2+ so that we don't indent them
- result = p.heredocIndent(result)
- case token.STRING:
- // If this is a multiline string, poison lines 2+ so we don't
- // indent them.
- if bytes.IndexRune(result, '\n') >= 0 {
- result = p.heredocIndent(result)
- }
- }
-
- return result
-}
-
-// objectItem returns the printable HCL form of an object item. An object type
-// starts with one/multiple keys and has a value. The value might be of any
-// type.
-func (p *printer) objectItem(o *ast.ObjectItem) []byte {
- defer un(trace(p, fmt.Sprintf("ObjectItem: %s", o.Keys[0].Token.Text)))
- var buf bytes.Buffer
-
- if o.LeadComment != nil {
- for _, comment := range o.LeadComment.List {
- buf.WriteString(comment.Text)
- buf.WriteByte(newline)
- }
- }
-
- // If key and val are on different lines, treat line comments like lead comments.
- if o.LineComment != nil && o.Val.Pos().Line != o.Keys[0].Pos().Line {
- for _, comment := range o.LineComment.List {
- buf.WriteString(comment.Text)
- buf.WriteByte(newline)
- }
- }
-
- for i, k := range o.Keys {
- buf.WriteString(k.Token.Text)
- buf.WriteByte(blank)
-
- // reach end of key
- if o.Assign.IsValid() && i == len(o.Keys)-1 && len(o.Keys) == 1 {
- buf.WriteString("=")
- buf.WriteByte(blank)
- }
- }
-
- buf.Write(p.output(o.Val))
-
- if o.LineComment != nil && o.Val.Pos().Line == o.Keys[0].Pos().Line {
- buf.WriteByte(blank)
- for _, comment := range o.LineComment.List {
- buf.WriteString(comment.Text)
- }
- }
-
- return buf.Bytes()
-}
-
-// objectType returns the printable HCL form of an object type. An object type
-// begins with a brace and ends with a brace.
-func (p *printer) objectType(o *ast.ObjectType) []byte {
- defer un(trace(p, "ObjectType"))
- var buf bytes.Buffer
- buf.WriteString("{")
-
- var index int
- var nextItem token.Pos
- var commented, newlinePrinted bool
- for {
- // Determine the location of the next actual non-comment
- // item. If we're at the end, the next item is the closing brace
- if index != len(o.List.Items) {
- nextItem = o.List.Items[index].Pos()
- } else {
- nextItem = o.Rbrace
- }
-
- // Go through the standalone comments in the file and print out
- // the comments that we should be for this object item.
- for _, c := range p.standaloneComments {
- printed := false
- var lastCommentPos token.Pos
- for _, comment := range c.List {
- // We only care about comments after the previous item
- // we've printed so that comments are printed in the
- // correct locations (between two objects for example).
- // And before the next item.
- if comment.Pos().After(p.prev) && comment.Pos().Before(nextItem) {
- // If there are standalone comments and the initial newline has not
- // been printed yet, do it now.
- if !newlinePrinted {
- newlinePrinted = true
- buf.WriteByte(newline)
- }
-
- // add newline if it's between other printed nodes
- if index > 0 {
- commented = true
- buf.WriteByte(newline)
- }
-
- // Store this position
- lastCommentPos = comment.Pos()
-
- // output the comment itself
- buf.Write(p.indent(p.heredocIndent([]byte(comment.Text))))
-
- // Set printed to true to note that we printed something
- printed = true
-
- /*
- if index != len(o.List.Items) {
- buf.WriteByte(newline) // do not print on the end
- }
- */
- }
- }
-
- // Stuff to do if we had comments
- if printed {
- // Always write a newline
- buf.WriteByte(newline)
-
- // If there is another item in the object and our comment
- // didn't hug it directly, then make sure there is a blank
- // line separating them.
- if nextItem != o.Rbrace && nextItem.Line != lastCommentPos.Line+1 {
- buf.WriteByte(newline)
- }
- }
- }
-
- if index == len(o.List.Items) {
- p.prev = o.Rbrace
- break
- }
-
- // At this point we are sure that it's not a totally empty block: print
- // the initial newline if it hasn't been printed yet by the previous
- // block about standalone comments.
- if !newlinePrinted {
- buf.WriteByte(newline)
- newlinePrinted = true
- }
-
- // check if we have adjacent one liner items. If yes we'll going to align
- // the comments.
- var aligned []*ast.ObjectItem
- for _, item := range o.List.Items[index:] {
- // we don't group one line lists
- if len(o.List.Items) == 1 {
- break
- }
-
- // one means a oneliner with out any lead comment
- // two means a oneliner with lead comment
- // anything else might be something else
- cur := lines(string(p.objectItem(item)))
- if cur > 2 {
- break
- }
-
- curPos := item.Pos()
-
- nextPos := token.Pos{}
- if index != len(o.List.Items)-1 {
- nextPos = o.List.Items[index+1].Pos()
- }
-
- prevPos := token.Pos{}
- if index != 0 {
- prevPos = o.List.Items[index-1].Pos()
- }
-
- // fmt.Println("DEBUG ----------------")
- // fmt.Printf("prev = %+v prevPos: %s\n", prev, prevPos)
- // fmt.Printf("cur = %+v curPos: %s\n", cur, curPos)
- // fmt.Printf("next = %+v nextPos: %s\n", next, nextPos)
-
- if curPos.Line+1 == nextPos.Line {
- aligned = append(aligned, item)
- index++
- continue
- }
-
- if curPos.Line-1 == prevPos.Line {
- aligned = append(aligned, item)
- index++
-
- // finish if we have a new line or comment next. This happens
- // if the next item is not adjacent
- if curPos.Line+1 != nextPos.Line {
- break
- }
- continue
- }
-
- break
- }
-
- // put newlines if the items are between other non aligned items.
- // newlines are also added if there is a standalone comment already, so
- // check it too
- if !commented && index != len(aligned) {
- buf.WriteByte(newline)
- }
-
- if len(aligned) >= 1 {
- p.prev = aligned[len(aligned)-1].Pos()
-
- items := p.alignedItems(aligned)
- buf.Write(p.indent(items))
- } else {
- p.prev = o.List.Items[index].Pos()
-
- buf.Write(p.indent(p.objectItem(o.List.Items[index])))
- index++
- }
-
- buf.WriteByte(newline)
- }
-
- buf.WriteString("}")
- return buf.Bytes()
-}
-
-func (p *printer) alignedItems(items []*ast.ObjectItem) []byte {
- var buf bytes.Buffer
-
- // find the longest key and value length, needed for alignment
- var longestKeyLen int // longest key length
- var longestValLen int // longest value length
- for _, item := range items {
- key := len(item.Keys[0].Token.Text)
- val := len(p.output(item.Val))
-
- if key > longestKeyLen {
- longestKeyLen = key
- }
-
- if val > longestValLen {
- longestValLen = val
- }
- }
-
- for i, item := range items {
- if item.LeadComment != nil {
- for _, comment := range item.LeadComment.List {
- buf.WriteString(comment.Text)
- buf.WriteByte(newline)
- }
- }
-
- for i, k := range item.Keys {
- keyLen := len(k.Token.Text)
- buf.WriteString(k.Token.Text)
- for i := 0; i < longestKeyLen-keyLen+1; i++ {
- buf.WriteByte(blank)
- }
-
- // reach end of key
- if i == len(item.Keys)-1 && len(item.Keys) == 1 {
- buf.WriteString("=")
- buf.WriteByte(blank)
- }
- }
-
- val := p.output(item.Val)
- valLen := len(val)
- buf.Write(val)
-
- if item.Val.Pos().Line == item.Keys[0].Pos().Line && item.LineComment != nil {
- for i := 0; i < longestValLen-valLen+1; i++ {
- buf.WriteByte(blank)
- }
-
- for _, comment := range item.LineComment.List {
- buf.WriteString(comment.Text)
- }
- }
-
- // do not print for the last item
- if i != len(items)-1 {
- buf.WriteByte(newline)
- }
- }
-
- return buf.Bytes()
-}
-
-// list returns the printable HCL form of an list type.
-func (p *printer) list(l *ast.ListType) []byte {
- if p.isSingleLineList(l) {
- return p.singleLineList(l)
- }
-
- var buf bytes.Buffer
- buf.WriteString("[")
- buf.WriteByte(newline)
-
- var longestLine int
- for _, item := range l.List {
- // for now we assume that the list only contains literal types
- if lit, ok := item.(*ast.LiteralType); ok {
- lineLen := len(lit.Token.Text)
- if lineLen > longestLine {
- longestLine = lineLen
- }
- }
- }
-
- haveEmptyLine := false
- for i, item := range l.List {
- // If we have a lead comment, then we want to write that first
- leadComment := false
- if lit, ok := item.(*ast.LiteralType); ok && lit.LeadComment != nil {
- leadComment = true
-
- // Ensure an empty line before every element with a
- // lead comment (except the first item in a list).
- if !haveEmptyLine && i != 0 {
- buf.WriteByte(newline)
- }
-
- for _, comment := range lit.LeadComment.List {
- buf.Write(p.indent([]byte(comment.Text)))
- buf.WriteByte(newline)
- }
- }
-
- // also indent each line
- val := p.output(item)
- curLen := len(val)
- buf.Write(p.indent(val))
-
- // if this item is a heredoc, then we output the comma on
- // the next line. This is the only case this happens.
- comma := []byte{','}
- if lit, ok := item.(*ast.LiteralType); ok && lit.Token.Type == token.HEREDOC {
- buf.WriteByte(newline)
- comma = p.indent(comma)
- }
-
- buf.Write(comma)
-
- if lit, ok := item.(*ast.LiteralType); ok && lit.LineComment != nil {
- // if the next item doesn't have any comments, do not align
- buf.WriteByte(blank) // align one space
- for i := 0; i < longestLine-curLen; i++ {
- buf.WriteByte(blank)
- }
-
- for _, comment := range lit.LineComment.List {
- buf.WriteString(comment.Text)
- }
- }
-
- buf.WriteByte(newline)
-
- // Ensure an empty line after every element with a
- // lead comment (except the first item in a list).
- haveEmptyLine = leadComment && i != len(l.List)-1
- if haveEmptyLine {
- buf.WriteByte(newline)
- }
- }
-
- buf.WriteString("]")
- return buf.Bytes()
-}
-
-// isSingleLineList returns true if:
-// * they were previously formatted entirely on one line
-// * they consist entirely of literals
-// * there are either no heredoc strings or the list has exactly one element
-// * there are no line comments
-func (printer) isSingleLineList(l *ast.ListType) bool {
- for _, item := range l.List {
- if item.Pos().Line != l.Lbrack.Line {
- return false
- }
-
- lit, ok := item.(*ast.LiteralType)
- if !ok {
- return false
- }
-
- if lit.Token.Type == token.HEREDOC && len(l.List) != 1 {
- return false
- }
-
- if lit.LineComment != nil {
- return false
- }
- }
-
- return true
-}
-
-// singleLineList prints a simple single line list.
-// For a definition of "simple", see isSingleLineList above.
-func (p *printer) singleLineList(l *ast.ListType) []byte {
- buf := &bytes.Buffer{}
-
- buf.WriteString("[")
- for i, item := range l.List {
- if i != 0 {
- buf.WriteString(", ")
- }
-
- // Output the item itself
- buf.Write(p.output(item))
-
- // The heredoc marker needs to be at the end of line.
- if lit, ok := item.(*ast.LiteralType); ok && lit.Token.Type == token.HEREDOC {
- buf.WriteByte(newline)
- }
- }
-
- buf.WriteString("]")
- return buf.Bytes()
-}
-
-// indent indents the lines of the given buffer for each non-empty line
-func (p *printer) indent(buf []byte) []byte {
- var prefix []byte
- if p.cfg.SpacesWidth != 0 {
- for i := 0; i < p.cfg.SpacesWidth; i++ {
- prefix = append(prefix, blank)
- }
- } else {
- prefix = []byte{tab}
- }
-
- var res []byte
- bol := true
- for _, c := range buf {
- if bol && c != '\n' {
- res = append(res, prefix...)
- }
-
- res = append(res, c)
- bol = c == '\n'
- }
- return res
-}
-
-// unindent removes all the indentation from the tombstoned lines
-func (p *printer) unindent(buf []byte) []byte {
- var res []byte
- for i := 0; i < len(buf); i++ {
- skip := len(buf)-i <= len(unindent)
- if !skip {
- skip = !bytes.Equal(unindent, buf[i:i+len(unindent)])
- }
- if skip {
- res = append(res, buf[i])
- continue
- }
-
- // We have a marker. we have to backtrace here and clean out
- // any whitespace ahead of our tombstone up to a \n
- for j := len(res) - 1; j >= 0; j-- {
- if res[j] == '\n' {
- break
- }
-
- res = res[:j]
- }
-
- // Skip the entire unindent marker
- i += len(unindent) - 1
- }
-
- return res
-}
-
-// heredocIndent marks all the 2nd and further lines as unindentable
-func (p *printer) heredocIndent(buf []byte) []byte {
- var res []byte
- bol := false
- for _, c := range buf {
- if bol && c != '\n' {
- res = append(res, unindent...)
- }
- res = append(res, c)
- bol = c == '\n'
- }
- return res
-}
-
-// isSingleLineObject tells whether the given object item is a single
-// line object such as "obj {}".
-//
-// A single line object:
-//
-// * has no lead comments (hence multi-line)
-// * has no assignment
-// * has no values in the stanza (within {})
-//
-func (p *printer) isSingleLineObject(val *ast.ObjectItem) bool {
- // If there is a lead comment, can't be one line
- if val.LeadComment != nil {
- return false
- }
-
- // If there is assignment, we always break by line
- if val.Assign.IsValid() {
- return false
- }
-
- // If it isn't an object type, then its not a single line object
- ot, ok := val.Val.(*ast.ObjectType)
- if !ok {
- return false
- }
-
- // If the object has no items, it is single line!
- return len(ot.List.Items) == 0
-}
-
-func lines(txt string) int {
- endline := 1
- for i := 0; i < len(txt); i++ {
- if txt[i] == '\n' {
- endline++
- }
- }
- return endline
-}
-
-// ----------------------------------------------------------------------------
-// Tracing support
-
-func (p *printer) printTrace(a ...interface{}) {
- if !p.enableTrace {
- return
- }
-
- const dots = ". . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . "
- const n = len(dots)
- i := 2 * p.indentTrace
- for i > n {
- fmt.Print(dots)
- i -= n
- }
- // i <= n
- fmt.Print(dots[0:i])
- fmt.Println(a...)
-}
-
-func trace(p *printer, msg string) *printer {
- p.printTrace(msg, "(")
- p.indentTrace++
- return p
-}
-
-// Usage pattern: defer un(trace(p, "..."))
-func un(p *printer) {
- p.indentTrace--
- p.printTrace(")")
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/printer/printer.go b/vendor/github.com/hashicorp/hcl/hcl/printer/printer.go
deleted file mode 100644
index 6617ab8e..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/printer/printer.go
+++ /dev/null
@@ -1,66 +0,0 @@
-// Package printer implements printing of AST nodes to HCL format.
-package printer
-
-import (
- "bytes"
- "io"
- "text/tabwriter"
-
- "github.com/hashicorp/hcl/hcl/ast"
- "github.com/hashicorp/hcl/hcl/parser"
-)
-
-var DefaultConfig = Config{
- SpacesWidth: 2,
-}
-
-// A Config node controls the output of Fprint.
-type Config struct {
- SpacesWidth int // if set, it will use spaces instead of tabs for alignment
-}
-
-func (c *Config) Fprint(output io.Writer, node ast.Node) error {
- p := &printer{
- cfg: *c,
- comments: make([]*ast.CommentGroup, 0),
- standaloneComments: make([]*ast.CommentGroup, 0),
- // enableTrace: true,
- }
-
- p.collectComments(node)
-
- if _, err := output.Write(p.unindent(p.output(node))); err != nil {
- return err
- }
-
- // flush tabwriter, if any
- var err error
- if tw, _ := output.(*tabwriter.Writer); tw != nil {
- err = tw.Flush()
- }
-
- return err
-}
-
-// Fprint "pretty-prints" an HCL node to output
-// It calls Config.Fprint with default settings.
-func Fprint(output io.Writer, node ast.Node) error {
- return DefaultConfig.Fprint(output, node)
-}
-
-// Format formats src HCL and returns the result.
-func Format(src []byte) ([]byte, error) {
- node, err := parser.Parse(src)
- if err != nil {
- return nil, err
- }
-
- var buf bytes.Buffer
- if err := DefaultConfig.Fprint(&buf, node); err != nil {
- return nil, err
- }
-
- // Add trailing newline to result
- buf.WriteString("\n")
- return buf.Bytes(), nil
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/scanner/scanner.go b/vendor/github.com/hashicorp/hcl/hcl/scanner/scanner.go
deleted file mode 100644
index 624a18fe..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/scanner/scanner.go
+++ /dev/null
@@ -1,652 +0,0 @@
-// Package scanner implements a scanner for HCL (HashiCorp Configuration
-// Language) source text.
-package scanner
-
-import (
- "bytes"
- "fmt"
- "os"
- "regexp"
- "unicode"
- "unicode/utf8"
-
- "github.com/hashicorp/hcl/hcl/token"
-)
-
-// eof represents a marker rune for the end of the reader.
-const eof = rune(0)
-
-// Scanner defines a lexical scanner
-type Scanner struct {
- buf *bytes.Buffer // Source buffer for advancing and scanning
- src []byte // Source buffer for immutable access
-
- // Source Position
- srcPos token.Pos // current position
- prevPos token.Pos // previous position, used for peek() method
-
- lastCharLen int // length of last character in bytes
- lastLineLen int // length of last line in characters (for correct column reporting)
-
- tokStart int // token text start position
- tokEnd int // token text end position
-
- // Error is called for each error encountered. If no Error
- // function is set, the error is reported to os.Stderr.
- Error func(pos token.Pos, msg string)
-
- // ErrorCount is incremented by one for each error encountered.
- ErrorCount int
-
- // tokPos is the start position of most recently scanned token; set by
- // Scan. The Filename field is always left untouched by the Scanner. If
- // an error is reported (via Error) and Position is invalid, the scanner is
- // not inside a token.
- tokPos token.Pos
-}
-
-// New creates and initializes a new instance of Scanner using src as
-// its source content.
-func New(src []byte) *Scanner {
- // even though we accept a src, we read from a io.Reader compatible type
- // (*bytes.Buffer). So in the future we might easily change it to streaming
- // read.
- b := bytes.NewBuffer(src)
- s := &Scanner{
- buf: b,
- src: src,
- }
-
- // srcPosition always starts with 1
- s.srcPos.Line = 1
- return s
-}
-
-// next reads the next rune from the bufferred reader. Returns the rune(0) if
-// an error occurs (or io.EOF is returned).
-func (s *Scanner) next() rune {
- ch, size, err := s.buf.ReadRune()
- if err != nil {
- // advance for error reporting
- s.srcPos.Column++
- s.srcPos.Offset += size
- s.lastCharLen = size
- return eof
- }
-
- // remember last position
- s.prevPos = s.srcPos
-
- s.srcPos.Column++
- s.lastCharLen = size
- s.srcPos.Offset += size
-
- if ch == utf8.RuneError && size == 1 {
- s.err("illegal UTF-8 encoding")
- return ch
- }
-
- if ch == '\n' {
- s.srcPos.Line++
- s.lastLineLen = s.srcPos.Column
- s.srcPos.Column = 0
- }
-
- if ch == '\x00' {
- s.err("unexpected null character (0x00)")
- return eof
- }
-
- if ch == '\uE123' {
- s.err("unicode code point U+E123 reserved for internal use")
- return utf8.RuneError
- }
-
- // debug
- // fmt.Printf("ch: %q, offset:column: %d:%d\n", ch, s.srcPos.Offset, s.srcPos.Column)
- return ch
-}
-
-// unread unreads the previous read Rune and updates the source position
-func (s *Scanner) unread() {
- if err := s.buf.UnreadRune(); err != nil {
- panic(err) // this is user fault, we should catch it
- }
- s.srcPos = s.prevPos // put back last position
-}
-
-// peek returns the next rune without advancing the reader.
-func (s *Scanner) peek() rune {
- peek, _, err := s.buf.ReadRune()
- if err != nil {
- return eof
- }
-
- s.buf.UnreadRune()
- return peek
-}
-
-// Scan scans the next token and returns the token.
-func (s *Scanner) Scan() token.Token {
- ch := s.next()
-
- // skip white space
- for isWhitespace(ch) {
- ch = s.next()
- }
-
- var tok token.Type
-
- // token text markings
- s.tokStart = s.srcPos.Offset - s.lastCharLen
-
- // token position, initial next() is moving the offset by one(size of rune
- // actually), though we are interested with the starting point
- s.tokPos.Offset = s.srcPos.Offset - s.lastCharLen
- if s.srcPos.Column > 0 {
- // common case: last character was not a '\n'
- s.tokPos.Line = s.srcPos.Line
- s.tokPos.Column = s.srcPos.Column
- } else {
- // last character was a '\n'
- // (we cannot be at the beginning of the source
- // since we have called next() at least once)
- s.tokPos.Line = s.srcPos.Line - 1
- s.tokPos.Column = s.lastLineLen
- }
-
- switch {
- case isLetter(ch):
- tok = token.IDENT
- lit := s.scanIdentifier()
- if lit == "true" || lit == "false" {
- tok = token.BOOL
- }
- case isDecimal(ch):
- tok = s.scanNumber(ch)
- default:
- switch ch {
- case eof:
- tok = token.EOF
- case '"':
- tok = token.STRING
- s.scanString()
- case '#', '/':
- tok = token.COMMENT
- s.scanComment(ch)
- case '.':
- tok = token.PERIOD
- ch = s.peek()
- if isDecimal(ch) {
- tok = token.FLOAT
- ch = s.scanMantissa(ch)
- ch = s.scanExponent(ch)
- }
- case '<':
- tok = token.HEREDOC
- s.scanHeredoc()
- case '[':
- tok = token.LBRACK
- case ']':
- tok = token.RBRACK
- case '{':
- tok = token.LBRACE
- case '}':
- tok = token.RBRACE
- case ',':
- tok = token.COMMA
- case '=':
- tok = token.ASSIGN
- case '+':
- tok = token.ADD
- case '-':
- if isDecimal(s.peek()) {
- ch := s.next()
- tok = s.scanNumber(ch)
- } else {
- tok = token.SUB
- }
- default:
- s.err("illegal char")
- }
- }
-
- // finish token ending
- s.tokEnd = s.srcPos.Offset
-
- // create token literal
- var tokenText string
- if s.tokStart >= 0 {
- tokenText = string(s.src[s.tokStart:s.tokEnd])
- }
- s.tokStart = s.tokEnd // ensure idempotency of tokenText() call
-
- return token.Token{
- Type: tok,
- Pos: s.tokPos,
- Text: tokenText,
- }
-}
-
-func (s *Scanner) scanComment(ch rune) {
- // single line comments
- if ch == '#' || (ch == '/' && s.peek() != '*') {
- if ch == '/' && s.peek() != '/' {
- s.err("expected '/' for comment")
- return
- }
-
- ch = s.next()
- for ch != '\n' && ch >= 0 && ch != eof {
- ch = s.next()
- }
- if ch != eof && ch >= 0 {
- s.unread()
- }
- return
- }
-
- // be sure we get the character after /* This allows us to find comment's
- // that are not erminated
- if ch == '/' {
- s.next()
- ch = s.next() // read character after "/*"
- }
-
- // look for /* - style comments
- for {
- if ch < 0 || ch == eof {
- s.err("comment not terminated")
- break
- }
-
- ch0 := ch
- ch = s.next()
- if ch0 == '*' && ch == '/' {
- break
- }
- }
-}
-
-// scanNumber scans a HCL number definition starting with the given rune
-func (s *Scanner) scanNumber(ch rune) token.Type {
- if ch == '0' {
- // check for hexadecimal, octal or float
- ch = s.next()
- if ch == 'x' || ch == 'X' {
- // hexadecimal
- ch = s.next()
- found := false
- for isHexadecimal(ch) {
- ch = s.next()
- found = true
- }
-
- if !found {
- s.err("illegal hexadecimal number")
- }
-
- if ch != eof {
- s.unread()
- }
-
- return token.NUMBER
- }
-
- // now it's either something like: 0421(octal) or 0.1231(float)
- illegalOctal := false
- for isDecimal(ch) {
- ch = s.next()
- if ch == '8' || ch == '9' {
- // this is just a possibility. For example 0159 is illegal, but
- // 0159.23 is valid. So we mark a possible illegal octal. If
- // the next character is not a period, we'll print the error.
- illegalOctal = true
- }
- }
-
- if ch == 'e' || ch == 'E' {
- ch = s.scanExponent(ch)
- return token.FLOAT
- }
-
- if ch == '.' {
- ch = s.scanFraction(ch)
-
- if ch == 'e' || ch == 'E' {
- ch = s.next()
- ch = s.scanExponent(ch)
- }
- return token.FLOAT
- }
-
- if illegalOctal {
- s.err("illegal octal number")
- }
-
- if ch != eof {
- s.unread()
- }
- return token.NUMBER
- }
-
- s.scanMantissa(ch)
- ch = s.next() // seek forward
- if ch == 'e' || ch == 'E' {
- ch = s.scanExponent(ch)
- return token.FLOAT
- }
-
- if ch == '.' {
- ch = s.scanFraction(ch)
- if ch == 'e' || ch == 'E' {
- ch = s.next()
- ch = s.scanExponent(ch)
- }
- return token.FLOAT
- }
-
- if ch != eof {
- s.unread()
- }
- return token.NUMBER
-}
-
-// scanMantissa scans the mantissa beginning from the rune. It returns the next
-// non decimal rune. It's used to determine wheter it's a fraction or exponent.
-func (s *Scanner) scanMantissa(ch rune) rune {
- scanned := false
- for isDecimal(ch) {
- ch = s.next()
- scanned = true
- }
-
- if scanned && ch != eof {
- s.unread()
- }
- return ch
-}
-
-// scanFraction scans the fraction after the '.' rune
-func (s *Scanner) scanFraction(ch rune) rune {
- if ch == '.' {
- ch = s.peek() // we peek just to see if we can move forward
- ch = s.scanMantissa(ch)
- }
- return ch
-}
-
-// scanExponent scans the remaining parts of an exponent after the 'e' or 'E'
-// rune.
-func (s *Scanner) scanExponent(ch rune) rune {
- if ch == 'e' || ch == 'E' {
- ch = s.next()
- if ch == '-' || ch == '+' {
- ch = s.next()
- }
- ch = s.scanMantissa(ch)
- }
- return ch
-}
-
-// scanHeredoc scans a heredoc string
-func (s *Scanner) scanHeredoc() {
- // Scan the second '<' in example: '<= len(identBytes) && identRegexp.Match(s.src[lineStart:s.srcPos.Offset-s.lastCharLen]) {
- break
- }
-
- // Not an anchor match, record the start of a new line
- lineStart = s.srcPos.Offset
- }
-
- if ch == eof {
- s.err("heredoc not terminated")
- return
- }
- }
-
- return
-}
-
-// scanString scans a quoted string
-func (s *Scanner) scanString() {
- braces := 0
- for {
- // '"' opening already consumed
- // read character after quote
- ch := s.next()
-
- if (ch == '\n' && braces == 0) || ch < 0 || ch == eof {
- s.err("literal not terminated")
- return
- }
-
- if ch == '"' && braces == 0 {
- break
- }
-
- // If we're going into a ${} then we can ignore quotes for awhile
- if braces == 0 && ch == '$' && s.peek() == '{' {
- braces++
- s.next()
- } else if braces > 0 && ch == '{' {
- braces++
- }
- if braces > 0 && ch == '}' {
- braces--
- }
-
- if ch == '\\' {
- s.scanEscape()
- }
- }
-
- return
-}
-
-// scanEscape scans an escape sequence
-func (s *Scanner) scanEscape() rune {
- // http://en.cppreference.com/w/cpp/language/escape
- ch := s.next() // read character after '/'
- switch ch {
- case 'a', 'b', 'f', 'n', 'r', 't', 'v', '\\', '"':
- // nothing to do
- case '0', '1', '2', '3', '4', '5', '6', '7':
- // octal notation
- ch = s.scanDigits(ch, 8, 3)
- case 'x':
- // hexademical notation
- ch = s.scanDigits(s.next(), 16, 2)
- case 'u':
- // universal character name
- ch = s.scanDigits(s.next(), 16, 4)
- case 'U':
- // universal character name
- ch = s.scanDigits(s.next(), 16, 8)
- default:
- s.err("illegal char escape")
- }
- return ch
-}
-
-// scanDigits scans a rune with the given base for n times. For example an
-// octal notation \184 would yield in scanDigits(ch, 8, 3)
-func (s *Scanner) scanDigits(ch rune, base, n int) rune {
- start := n
- for n > 0 && digitVal(ch) < base {
- ch = s.next()
- if ch == eof {
- // If we see an EOF, we halt any more scanning of digits
- // immediately.
- break
- }
-
- n--
- }
- if n > 0 {
- s.err("illegal char escape")
- }
-
- if n != start && ch != eof {
- // we scanned all digits, put the last non digit char back,
- // only if we read anything at all
- s.unread()
- }
-
- return ch
-}
-
-// scanIdentifier scans an identifier and returns the literal string
-func (s *Scanner) scanIdentifier() string {
- offs := s.srcPos.Offset - s.lastCharLen
- ch := s.next()
- for isLetter(ch) || isDigit(ch) || ch == '-' || ch == '.' {
- ch = s.next()
- }
-
- if ch != eof {
- s.unread() // we got identifier, put back latest char
- }
-
- return string(s.src[offs:s.srcPos.Offset])
-}
-
-// recentPosition returns the position of the character immediately after the
-// character or token returned by the last call to Scan.
-func (s *Scanner) recentPosition() (pos token.Pos) {
- pos.Offset = s.srcPos.Offset - s.lastCharLen
- switch {
- case s.srcPos.Column > 0:
- // common case: last character was not a '\n'
- pos.Line = s.srcPos.Line
- pos.Column = s.srcPos.Column
- case s.lastLineLen > 0:
- // last character was a '\n'
- // (we cannot be at the beginning of the source
- // since we have called next() at least once)
- pos.Line = s.srcPos.Line - 1
- pos.Column = s.lastLineLen
- default:
- // at the beginning of the source
- pos.Line = 1
- pos.Column = 1
- }
- return
-}
-
-// err prints the error of any scanning to s.Error function. If the function is
-// not defined, by default it prints them to os.Stderr
-func (s *Scanner) err(msg string) {
- s.ErrorCount++
- pos := s.recentPosition()
-
- if s.Error != nil {
- s.Error(pos, msg)
- return
- }
-
- fmt.Fprintf(os.Stderr, "%s: %s\n", pos, msg)
-}
-
-// isHexadecimal returns true if the given rune is a letter
-func isLetter(ch rune) bool {
- return 'a' <= ch && ch <= 'z' || 'A' <= ch && ch <= 'Z' || ch == '_' || ch >= 0x80 && unicode.IsLetter(ch)
-}
-
-// isDigit returns true if the given rune is a decimal digit
-func isDigit(ch rune) bool {
- return '0' <= ch && ch <= '9' || ch >= 0x80 && unicode.IsDigit(ch)
-}
-
-// isDecimal returns true if the given rune is a decimal number
-func isDecimal(ch rune) bool {
- return '0' <= ch && ch <= '9'
-}
-
-// isHexadecimal returns true if the given rune is an hexadecimal number
-func isHexadecimal(ch rune) bool {
- return '0' <= ch && ch <= '9' || 'a' <= ch && ch <= 'f' || 'A' <= ch && ch <= 'F'
-}
-
-// isWhitespace returns true if the rune is a space, tab, newline or carriage return
-func isWhitespace(ch rune) bool {
- return ch == ' ' || ch == '\t' || ch == '\n' || ch == '\r'
-}
-
-// digitVal returns the integer value of a given octal,decimal or hexadecimal rune
-func digitVal(ch rune) int {
- switch {
- case '0' <= ch && ch <= '9':
- return int(ch - '0')
- case 'a' <= ch && ch <= 'f':
- return int(ch - 'a' + 10)
- case 'A' <= ch && ch <= 'F':
- return int(ch - 'A' + 10)
- }
- return 16 // larger than any legal digit val
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/strconv/quote.go b/vendor/github.com/hashicorp/hcl/hcl/strconv/quote.go
deleted file mode 100644
index 5f981eaa..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/strconv/quote.go
+++ /dev/null
@@ -1,241 +0,0 @@
-package strconv
-
-import (
- "errors"
- "unicode/utf8"
-)
-
-// ErrSyntax indicates that a value does not have the right syntax for the target type.
-var ErrSyntax = errors.New("invalid syntax")
-
-// Unquote interprets s as a single-quoted, double-quoted,
-// or backquoted Go string literal, returning the string value
-// that s quotes. (If s is single-quoted, it would be a Go
-// character literal; Unquote returns the corresponding
-// one-character string.)
-func Unquote(s string) (t string, err error) {
- n := len(s)
- if n < 2 {
- return "", ErrSyntax
- }
- quote := s[0]
- if quote != s[n-1] {
- return "", ErrSyntax
- }
- s = s[1 : n-1]
-
- if quote != '"' {
- return "", ErrSyntax
- }
- if !contains(s, '$') && !contains(s, '{') && contains(s, '\n') {
- return "", ErrSyntax
- }
-
- // Is it trivial? Avoid allocation.
- if !contains(s, '\\') && !contains(s, quote) && !contains(s, '$') {
- switch quote {
- case '"':
- return s, nil
- case '\'':
- r, size := utf8.DecodeRuneInString(s)
- if size == len(s) && (r != utf8.RuneError || size != 1) {
- return s, nil
- }
- }
- }
-
- var runeTmp [utf8.UTFMax]byte
- buf := make([]byte, 0, 3*len(s)/2) // Try to avoid more allocations.
- for len(s) > 0 {
- // If we're starting a '${}' then let it through un-unquoted.
- // Specifically: we don't unquote any characters within the `${}`
- // section.
- if s[0] == '$' && len(s) > 1 && s[1] == '{' {
- buf = append(buf, '$', '{')
- s = s[2:]
-
- // Continue reading until we find the closing brace, copying as-is
- braces := 1
- for len(s) > 0 && braces > 0 {
- r, size := utf8.DecodeRuneInString(s)
- if r == utf8.RuneError {
- return "", ErrSyntax
- }
-
- s = s[size:]
-
- n := utf8.EncodeRune(runeTmp[:], r)
- buf = append(buf, runeTmp[:n]...)
-
- switch r {
- case '{':
- braces++
- case '}':
- braces--
- }
- }
- if braces != 0 {
- return "", ErrSyntax
- }
- if len(s) == 0 {
- // If there's no string left, we're done!
- break
- } else {
- // If there's more left, we need to pop back up to the top of the loop
- // in case there's another interpolation in this string.
- continue
- }
- }
-
- if s[0] == '\n' {
- return "", ErrSyntax
- }
-
- c, multibyte, ss, err := unquoteChar(s, quote)
- if err != nil {
- return "", err
- }
- s = ss
- if c < utf8.RuneSelf || !multibyte {
- buf = append(buf, byte(c))
- } else {
- n := utf8.EncodeRune(runeTmp[:], c)
- buf = append(buf, runeTmp[:n]...)
- }
- if quote == '\'' && len(s) != 0 {
- // single-quoted must be single character
- return "", ErrSyntax
- }
- }
- return string(buf), nil
-}
-
-// contains reports whether the string contains the byte c.
-func contains(s string, c byte) bool {
- for i := 0; i < len(s); i++ {
- if s[i] == c {
- return true
- }
- }
- return false
-}
-
-func unhex(b byte) (v rune, ok bool) {
- c := rune(b)
- switch {
- case '0' <= c && c <= '9':
- return c - '0', true
- case 'a' <= c && c <= 'f':
- return c - 'a' + 10, true
- case 'A' <= c && c <= 'F':
- return c - 'A' + 10, true
- }
- return
-}
-
-func unquoteChar(s string, quote byte) (value rune, multibyte bool, tail string, err error) {
- // easy cases
- switch c := s[0]; {
- case c == quote && (quote == '\'' || quote == '"'):
- err = ErrSyntax
- return
- case c >= utf8.RuneSelf:
- r, size := utf8.DecodeRuneInString(s)
- return r, true, s[size:], nil
- case c != '\\':
- return rune(s[0]), false, s[1:], nil
- }
-
- // hard case: c is backslash
- if len(s) <= 1 {
- err = ErrSyntax
- return
- }
- c := s[1]
- s = s[2:]
-
- switch c {
- case 'a':
- value = '\a'
- case 'b':
- value = '\b'
- case 'f':
- value = '\f'
- case 'n':
- value = '\n'
- case 'r':
- value = '\r'
- case 't':
- value = '\t'
- case 'v':
- value = '\v'
- case 'x', 'u', 'U':
- n := 0
- switch c {
- case 'x':
- n = 2
- case 'u':
- n = 4
- case 'U':
- n = 8
- }
- var v rune
- if len(s) < n {
- err = ErrSyntax
- return
- }
- for j := 0; j < n; j++ {
- x, ok := unhex(s[j])
- if !ok {
- err = ErrSyntax
- return
- }
- v = v<<4 | x
- }
- s = s[n:]
- if c == 'x' {
- // single-byte string, possibly not UTF-8
- value = v
- break
- }
- if v > utf8.MaxRune {
- err = ErrSyntax
- return
- }
- value = v
- multibyte = true
- case '0', '1', '2', '3', '4', '5', '6', '7':
- v := rune(c) - '0'
- if len(s) < 2 {
- err = ErrSyntax
- return
- }
- for j := 0; j < 2; j++ { // one digit already; two more
- x := rune(s[j]) - '0'
- if x < 0 || x > 7 {
- err = ErrSyntax
- return
- }
- v = (v << 3) | x
- }
- s = s[2:]
- if v > 255 {
- err = ErrSyntax
- return
- }
- value = v
- case '\\':
- value = '\\'
- case '\'', '"':
- if c != quote {
- err = ErrSyntax
- return
- }
- value = rune(c)
- default:
- err = ErrSyntax
- return
- }
- tail = s
- return
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/token/position.go b/vendor/github.com/hashicorp/hcl/hcl/token/position.go
deleted file mode 100644
index 59c1bb72..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/token/position.go
+++ /dev/null
@@ -1,46 +0,0 @@
-package token
-
-import "fmt"
-
-// Pos describes an arbitrary source position
-// including the file, line, and column location.
-// A Position is valid if the line number is > 0.
-type Pos struct {
- Filename string // filename, if any
- Offset int // offset, starting at 0
- Line int // line number, starting at 1
- Column int // column number, starting at 1 (character count)
-}
-
-// IsValid returns true if the position is valid.
-func (p *Pos) IsValid() bool { return p.Line > 0 }
-
-// String returns a string in one of several forms:
-//
-// file:line:column valid position with file name
-// line:column valid position without file name
-// file invalid position with file name
-// - invalid position without file name
-func (p Pos) String() string {
- s := p.Filename
- if p.IsValid() {
- if s != "" {
- s += ":"
- }
- s += fmt.Sprintf("%d:%d", p.Line, p.Column)
- }
- if s == "" {
- s = "-"
- }
- return s
-}
-
-// Before reports whether the position p is before u.
-func (p Pos) Before(u Pos) bool {
- return u.Offset > p.Offset || u.Line > p.Line
-}
-
-// After reports whether the position p is after u.
-func (p Pos) After(u Pos) bool {
- return u.Offset < p.Offset || u.Line < p.Line
-}
diff --git a/vendor/github.com/hashicorp/hcl/hcl/token/token.go b/vendor/github.com/hashicorp/hcl/hcl/token/token.go
deleted file mode 100644
index e37c0664..00000000
--- a/vendor/github.com/hashicorp/hcl/hcl/token/token.go
+++ /dev/null
@@ -1,219 +0,0 @@
-// Package token defines constants representing the lexical tokens for HCL
-// (HashiCorp Configuration Language)
-package token
-
-import (
- "fmt"
- "strconv"
- "strings"
-
- hclstrconv "github.com/hashicorp/hcl/hcl/strconv"
-)
-
-// Token defines a single HCL token which can be obtained via the Scanner
-type Token struct {
- Type Type
- Pos Pos
- Text string
- JSON bool
-}
-
-// Type is the set of lexical tokens of the HCL (HashiCorp Configuration Language)
-type Type int
-
-const (
- // Special tokens
- ILLEGAL Type = iota
- EOF
- COMMENT
-
- identifier_beg
- IDENT // literals
- literal_beg
- NUMBER // 12345
- FLOAT // 123.45
- BOOL // true,false
- STRING // "abc"
- HEREDOC // < 0 {
- // Pop the current item
- n := len(frontier)
- item := frontier[n-1]
- frontier = frontier[:n-1]
-
- switch v := item.Val.(type) {
- case *ast.ObjectType:
- items, frontier = flattenObjectType(v, item, items, frontier)
- case *ast.ListType:
- items, frontier = flattenListType(v, item, items, frontier)
- default:
- items = append(items, item)
- }
- }
-
- // Reverse the list since the frontier model runs things backwards
- for i := len(items)/2 - 1; i >= 0; i-- {
- opp := len(items) - 1 - i
- items[i], items[opp] = items[opp], items[i]
- }
-
- // Done! Set the original items
- list.Items = items
- return n, true
- })
-}
-
-func flattenListType(
- ot *ast.ListType,
- item *ast.ObjectItem,
- items []*ast.ObjectItem,
- frontier []*ast.ObjectItem) ([]*ast.ObjectItem, []*ast.ObjectItem) {
- // If the list is empty, keep the original list
- if len(ot.List) == 0 {
- items = append(items, item)
- return items, frontier
- }
-
- // All the elements of this object must also be objects!
- for _, subitem := range ot.List {
- if _, ok := subitem.(*ast.ObjectType); !ok {
- items = append(items, item)
- return items, frontier
- }
- }
-
- // Great! We have a match go through all the items and flatten
- for _, elem := range ot.List {
- // Add it to the frontier so that we can recurse
- frontier = append(frontier, &ast.ObjectItem{
- Keys: item.Keys,
- Assign: item.Assign,
- Val: elem,
- LeadComment: item.LeadComment,
- LineComment: item.LineComment,
- })
- }
-
- return items, frontier
-}
-
-func flattenObjectType(
- ot *ast.ObjectType,
- item *ast.ObjectItem,
- items []*ast.ObjectItem,
- frontier []*ast.ObjectItem) ([]*ast.ObjectItem, []*ast.ObjectItem) {
- // If the list has no items we do not have to flatten anything
- if ot.List.Items == nil {
- items = append(items, item)
- return items, frontier
- }
-
- // All the elements of this object must also be objects!
- for _, subitem := range ot.List.Items {
- if _, ok := subitem.Val.(*ast.ObjectType); !ok {
- items = append(items, item)
- return items, frontier
- }
- }
-
- // Great! We have a match go through all the items and flatten
- for _, subitem := range ot.List.Items {
- // Copy the new key
- keys := make([]*ast.ObjectKey, len(item.Keys)+len(subitem.Keys))
- copy(keys, item.Keys)
- copy(keys[len(item.Keys):], subitem.Keys)
-
- // Add it to the frontier so that we can recurse
- frontier = append(frontier, &ast.ObjectItem{
- Keys: keys,
- Assign: item.Assign,
- Val: subitem.Val,
- LeadComment: item.LeadComment,
- LineComment: item.LineComment,
- })
- }
-
- return items, frontier
-}
diff --git a/vendor/github.com/hashicorp/hcl/json/parser/parser.go b/vendor/github.com/hashicorp/hcl/json/parser/parser.go
deleted file mode 100644
index 125a5f07..00000000
--- a/vendor/github.com/hashicorp/hcl/json/parser/parser.go
+++ /dev/null
@@ -1,313 +0,0 @@
-package parser
-
-import (
- "errors"
- "fmt"
-
- "github.com/hashicorp/hcl/hcl/ast"
- hcltoken "github.com/hashicorp/hcl/hcl/token"
- "github.com/hashicorp/hcl/json/scanner"
- "github.com/hashicorp/hcl/json/token"
-)
-
-type Parser struct {
- sc *scanner.Scanner
-
- // Last read token
- tok token.Token
- commaPrev token.Token
-
- enableTrace bool
- indent int
- n int // buffer size (max = 1)
-}
-
-func newParser(src []byte) *Parser {
- return &Parser{
- sc: scanner.New(src),
- }
-}
-
-// Parse returns the fully parsed source and returns the abstract syntax tree.
-func Parse(src []byte) (*ast.File, error) {
- p := newParser(src)
- return p.Parse()
-}
-
-var errEofToken = errors.New("EOF token found")
-
-// Parse returns the fully parsed source and returns the abstract syntax tree.
-func (p *Parser) Parse() (*ast.File, error) {
- f := &ast.File{}
- var err, scerr error
- p.sc.Error = func(pos token.Pos, msg string) {
- scerr = fmt.Errorf("%s: %s", pos, msg)
- }
-
- // The root must be an object in JSON
- object, err := p.object()
- if scerr != nil {
- return nil, scerr
- }
- if err != nil {
- return nil, err
- }
-
- // We make our final node an object list so it is more HCL compatible
- f.Node = object.List
-
- // Flatten it, which finds patterns and turns them into more HCL-like
- // AST trees.
- flattenObjects(f.Node)
-
- return f, nil
-}
-
-func (p *Parser) objectList() (*ast.ObjectList, error) {
- defer un(trace(p, "ParseObjectList"))
- node := &ast.ObjectList{}
-
- for {
- n, err := p.objectItem()
- if err == errEofToken {
- break // we are finished
- }
-
- // we don't return a nil node, because might want to use already
- // collected items.
- if err != nil {
- return node, err
- }
-
- node.Add(n)
-
- // Check for a followup comma. If it isn't a comma, then we're done
- if tok := p.scan(); tok.Type != token.COMMA {
- break
- }
- }
-
- return node, nil
-}
-
-// objectItem parses a single object item
-func (p *Parser) objectItem() (*ast.ObjectItem, error) {
- defer un(trace(p, "ParseObjectItem"))
-
- keys, err := p.objectKey()
- if err != nil {
- return nil, err
- }
-
- o := &ast.ObjectItem{
- Keys: keys,
- }
-
- switch p.tok.Type {
- case token.COLON:
- pos := p.tok.Pos
- o.Assign = hcltoken.Pos{
- Filename: pos.Filename,
- Offset: pos.Offset,
- Line: pos.Line,
- Column: pos.Column,
- }
-
- o.Val, err = p.objectValue()
- if err != nil {
- return nil, err
- }
- }
-
- return o, nil
-}
-
-// objectKey parses an object key and returns a ObjectKey AST
-func (p *Parser) objectKey() ([]*ast.ObjectKey, error) {
- keyCount := 0
- keys := make([]*ast.ObjectKey, 0)
-
- for {
- tok := p.scan()
- switch tok.Type {
- case token.EOF:
- return nil, errEofToken
- case token.STRING:
- keyCount++
- keys = append(keys, &ast.ObjectKey{
- Token: p.tok.HCLToken(),
- })
- case token.COLON:
- // If we have a zero keycount it means that we never got
- // an object key, i.e. `{ :`. This is a syntax error.
- if keyCount == 0 {
- return nil, fmt.Errorf("expected: STRING got: %s", p.tok.Type)
- }
-
- // Done
- return keys, nil
- case token.ILLEGAL:
- return nil, errors.New("illegal")
- default:
- return nil, fmt.Errorf("expected: STRING got: %s", p.tok.Type)
- }
- }
-}
-
-// object parses any type of object, such as number, bool, string, object or
-// list.
-func (p *Parser) objectValue() (ast.Node, error) {
- defer un(trace(p, "ParseObjectValue"))
- tok := p.scan()
-
- switch tok.Type {
- case token.NUMBER, token.FLOAT, token.BOOL, token.NULL, token.STRING:
- return p.literalType()
- case token.LBRACE:
- return p.objectType()
- case token.LBRACK:
- return p.listType()
- case token.EOF:
- return nil, errEofToken
- }
-
- return nil, fmt.Errorf("Expected object value, got unknown token: %+v", tok)
-}
-
-// object parses any type of object, such as number, bool, string, object or
-// list.
-func (p *Parser) object() (*ast.ObjectType, error) {
- defer un(trace(p, "ParseType"))
- tok := p.scan()
-
- switch tok.Type {
- case token.LBRACE:
- return p.objectType()
- case token.EOF:
- return nil, errEofToken
- }
-
- return nil, fmt.Errorf("Expected object, got unknown token: %+v", tok)
-}
-
-// objectType parses an object type and returns a ObjectType AST
-func (p *Parser) objectType() (*ast.ObjectType, error) {
- defer un(trace(p, "ParseObjectType"))
-
- // we assume that the currently scanned token is a LBRACE
- o := &ast.ObjectType{}
-
- l, err := p.objectList()
-
- // if we hit RBRACE, we are good to go (means we parsed all Items), if it's
- // not a RBRACE, it's an syntax error and we just return it.
- if err != nil && p.tok.Type != token.RBRACE {
- return nil, err
- }
-
- o.List = l
- return o, nil
-}
-
-// listType parses a list type and returns a ListType AST
-func (p *Parser) listType() (*ast.ListType, error) {
- defer un(trace(p, "ParseListType"))
-
- // we assume that the currently scanned token is a LBRACK
- l := &ast.ListType{}
-
- for {
- tok := p.scan()
- switch tok.Type {
- case token.NUMBER, token.FLOAT, token.STRING:
- node, err := p.literalType()
- if err != nil {
- return nil, err
- }
-
- l.Add(node)
- case token.COMMA:
- continue
- case token.LBRACE:
- node, err := p.objectType()
- if err != nil {
- return nil, err
- }
-
- l.Add(node)
- case token.BOOL:
- // TODO(arslan) should we support? not supported by HCL yet
- case token.LBRACK:
- // TODO(arslan) should we support nested lists? Even though it's
- // written in README of HCL, it's not a part of the grammar
- // (not defined in parse.y)
- case token.RBRACK:
- // finished
- return l, nil
- default:
- return nil, fmt.Errorf("unexpected token while parsing list: %s", tok.Type)
- }
-
- }
-}
-
-// literalType parses a literal type and returns a LiteralType AST
-func (p *Parser) literalType() (*ast.LiteralType, error) {
- defer un(trace(p, "ParseLiteral"))
-
- return &ast.LiteralType{
- Token: p.tok.HCLToken(),
- }, nil
-}
-
-// scan returns the next token from the underlying scanner. If a token has
-// been unscanned then read that instead.
-func (p *Parser) scan() token.Token {
- // If we have a token on the buffer, then return it.
- if p.n != 0 {
- p.n = 0
- return p.tok
- }
-
- p.tok = p.sc.Scan()
- return p.tok
-}
-
-// unscan pushes the previously read token back onto the buffer.
-func (p *Parser) unscan() {
- p.n = 1
-}
-
-// ----------------------------------------------------------------------------
-// Parsing support
-
-func (p *Parser) printTrace(a ...interface{}) {
- if !p.enableTrace {
- return
- }
-
- const dots = ". . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . "
- const n = len(dots)
- fmt.Printf("%5d:%3d: ", p.tok.Pos.Line, p.tok.Pos.Column)
-
- i := 2 * p.indent
- for i > n {
- fmt.Print(dots)
- i -= n
- }
- // i <= n
- fmt.Print(dots[0:i])
- fmt.Println(a...)
-}
-
-func trace(p *Parser, msg string) *Parser {
- p.printTrace(msg, "(")
- p.indent++
- return p
-}
-
-// Usage pattern: defer un(trace(p, "..."))
-func un(p *Parser) {
- p.indent--
- p.printTrace(")")
-}
diff --git a/vendor/github.com/hashicorp/hcl/json/scanner/scanner.go b/vendor/github.com/hashicorp/hcl/json/scanner/scanner.go
deleted file mode 100644
index fe3f0f09..00000000
--- a/vendor/github.com/hashicorp/hcl/json/scanner/scanner.go
+++ /dev/null
@@ -1,451 +0,0 @@
-package scanner
-
-import (
- "bytes"
- "fmt"
- "os"
- "unicode"
- "unicode/utf8"
-
- "github.com/hashicorp/hcl/json/token"
-)
-
-// eof represents a marker rune for the end of the reader.
-const eof = rune(0)
-
-// Scanner defines a lexical scanner
-type Scanner struct {
- buf *bytes.Buffer // Source buffer for advancing and scanning
- src []byte // Source buffer for immutable access
-
- // Source Position
- srcPos token.Pos // current position
- prevPos token.Pos // previous position, used for peek() method
-
- lastCharLen int // length of last character in bytes
- lastLineLen int // length of last line in characters (for correct column reporting)
-
- tokStart int // token text start position
- tokEnd int // token text end position
-
- // Error is called for each error encountered. If no Error
- // function is set, the error is reported to os.Stderr.
- Error func(pos token.Pos, msg string)
-
- // ErrorCount is incremented by one for each error encountered.
- ErrorCount int
-
- // tokPos is the start position of most recently scanned token; set by
- // Scan. The Filename field is always left untouched by the Scanner. If
- // an error is reported (via Error) and Position is invalid, the scanner is
- // not inside a token.
- tokPos token.Pos
-}
-
-// New creates and initializes a new instance of Scanner using src as
-// its source content.
-func New(src []byte) *Scanner {
- // even though we accept a src, we read from a io.Reader compatible type
- // (*bytes.Buffer). So in the future we might easily change it to streaming
- // read.
- b := bytes.NewBuffer(src)
- s := &Scanner{
- buf: b,
- src: src,
- }
-
- // srcPosition always starts with 1
- s.srcPos.Line = 1
- return s
-}
-
-// next reads the next rune from the bufferred reader. Returns the rune(0) if
-// an error occurs (or io.EOF is returned).
-func (s *Scanner) next() rune {
- ch, size, err := s.buf.ReadRune()
- if err != nil {
- // advance for error reporting
- s.srcPos.Column++
- s.srcPos.Offset += size
- s.lastCharLen = size
- return eof
- }
-
- if ch == utf8.RuneError && size == 1 {
- s.srcPos.Column++
- s.srcPos.Offset += size
- s.lastCharLen = size
- s.err("illegal UTF-8 encoding")
- return ch
- }
-
- // remember last position
- s.prevPos = s.srcPos
-
- s.srcPos.Column++
- s.lastCharLen = size
- s.srcPos.Offset += size
-
- if ch == '\n' {
- s.srcPos.Line++
- s.lastLineLen = s.srcPos.Column
- s.srcPos.Column = 0
- }
-
- // debug
- // fmt.Printf("ch: %q, offset:column: %d:%d\n", ch, s.srcPos.Offset, s.srcPos.Column)
- return ch
-}
-
-// unread unreads the previous read Rune and updates the source position
-func (s *Scanner) unread() {
- if err := s.buf.UnreadRune(); err != nil {
- panic(err) // this is user fault, we should catch it
- }
- s.srcPos = s.prevPos // put back last position
-}
-
-// peek returns the next rune without advancing the reader.
-func (s *Scanner) peek() rune {
- peek, _, err := s.buf.ReadRune()
- if err != nil {
- return eof
- }
-
- s.buf.UnreadRune()
- return peek
-}
-
-// Scan scans the next token and returns the token.
-func (s *Scanner) Scan() token.Token {
- ch := s.next()
-
- // skip white space
- for isWhitespace(ch) {
- ch = s.next()
- }
-
- var tok token.Type
-
- // token text markings
- s.tokStart = s.srcPos.Offset - s.lastCharLen
-
- // token position, initial next() is moving the offset by one(size of rune
- // actually), though we are interested with the starting point
- s.tokPos.Offset = s.srcPos.Offset - s.lastCharLen
- if s.srcPos.Column > 0 {
- // common case: last character was not a '\n'
- s.tokPos.Line = s.srcPos.Line
- s.tokPos.Column = s.srcPos.Column
- } else {
- // last character was a '\n'
- // (we cannot be at the beginning of the source
- // since we have called next() at least once)
- s.tokPos.Line = s.srcPos.Line - 1
- s.tokPos.Column = s.lastLineLen
- }
-
- switch {
- case isLetter(ch):
- lit := s.scanIdentifier()
- if lit == "true" || lit == "false" {
- tok = token.BOOL
- } else if lit == "null" {
- tok = token.NULL
- } else {
- s.err("illegal char")
- }
- case isDecimal(ch):
- tok = s.scanNumber(ch)
- default:
- switch ch {
- case eof:
- tok = token.EOF
- case '"':
- tok = token.STRING
- s.scanString()
- case '.':
- tok = token.PERIOD
- ch = s.peek()
- if isDecimal(ch) {
- tok = token.FLOAT
- ch = s.scanMantissa(ch)
- ch = s.scanExponent(ch)
- }
- case '[':
- tok = token.LBRACK
- case ']':
- tok = token.RBRACK
- case '{':
- tok = token.LBRACE
- case '}':
- tok = token.RBRACE
- case ',':
- tok = token.COMMA
- case ':':
- tok = token.COLON
- case '-':
- if isDecimal(s.peek()) {
- ch := s.next()
- tok = s.scanNumber(ch)
- } else {
- s.err("illegal char")
- }
- default:
- s.err("illegal char: " + string(ch))
- }
- }
-
- // finish token ending
- s.tokEnd = s.srcPos.Offset
-
- // create token literal
- var tokenText string
- if s.tokStart >= 0 {
- tokenText = string(s.src[s.tokStart:s.tokEnd])
- }
- s.tokStart = s.tokEnd // ensure idempotency of tokenText() call
-
- return token.Token{
- Type: tok,
- Pos: s.tokPos,
- Text: tokenText,
- }
-}
-
-// scanNumber scans a HCL number definition starting with the given rune
-func (s *Scanner) scanNumber(ch rune) token.Type {
- zero := ch == '0'
- pos := s.srcPos
-
- s.scanMantissa(ch)
- ch = s.next() // seek forward
- if ch == 'e' || ch == 'E' {
- ch = s.scanExponent(ch)
- return token.FLOAT
- }
-
- if ch == '.' {
- ch = s.scanFraction(ch)
- if ch == 'e' || ch == 'E' {
- ch = s.next()
- ch = s.scanExponent(ch)
- }
- return token.FLOAT
- }
-
- if ch != eof {
- s.unread()
- }
-
- // If we have a larger number and this is zero, error
- if zero && pos != s.srcPos {
- s.err("numbers cannot start with 0")
- }
-
- return token.NUMBER
-}
-
-// scanMantissa scans the mantissa beginning from the rune. It returns the next
-// non decimal rune. It's used to determine wheter it's a fraction or exponent.
-func (s *Scanner) scanMantissa(ch rune) rune {
- scanned := false
- for isDecimal(ch) {
- ch = s.next()
- scanned = true
- }
-
- if scanned && ch != eof {
- s.unread()
- }
- return ch
-}
-
-// scanFraction scans the fraction after the '.' rune
-func (s *Scanner) scanFraction(ch rune) rune {
- if ch == '.' {
- ch = s.peek() // we peek just to see if we can move forward
- ch = s.scanMantissa(ch)
- }
- return ch
-}
-
-// scanExponent scans the remaining parts of an exponent after the 'e' or 'E'
-// rune.
-func (s *Scanner) scanExponent(ch rune) rune {
- if ch == 'e' || ch == 'E' {
- ch = s.next()
- if ch == '-' || ch == '+' {
- ch = s.next()
- }
- ch = s.scanMantissa(ch)
- }
- return ch
-}
-
-// scanString scans a quoted string
-func (s *Scanner) scanString() {
- braces := 0
- for {
- // '"' opening already consumed
- // read character after quote
- ch := s.next()
-
- if ch == '\n' || ch < 0 || ch == eof {
- s.err("literal not terminated")
- return
- }
-
- if ch == '"' {
- break
- }
-
- // If we're going into a ${} then we can ignore quotes for awhile
- if braces == 0 && ch == '$' && s.peek() == '{' {
- braces++
- s.next()
- } else if braces > 0 && ch == '{' {
- braces++
- }
- if braces > 0 && ch == '}' {
- braces--
- }
-
- if ch == '\\' {
- s.scanEscape()
- }
- }
-
- return
-}
-
-// scanEscape scans an escape sequence
-func (s *Scanner) scanEscape() rune {
- // http://en.cppreference.com/w/cpp/language/escape
- ch := s.next() // read character after '/'
- switch ch {
- case 'a', 'b', 'f', 'n', 'r', 't', 'v', '\\', '"':
- // nothing to do
- case '0', '1', '2', '3', '4', '5', '6', '7':
- // octal notation
- ch = s.scanDigits(ch, 8, 3)
- case 'x':
- // hexademical notation
- ch = s.scanDigits(s.next(), 16, 2)
- case 'u':
- // universal character name
- ch = s.scanDigits(s.next(), 16, 4)
- case 'U':
- // universal character name
- ch = s.scanDigits(s.next(), 16, 8)
- default:
- s.err("illegal char escape")
- }
- return ch
-}
-
-// scanDigits scans a rune with the given base for n times. For example an
-// octal notation \184 would yield in scanDigits(ch, 8, 3)
-func (s *Scanner) scanDigits(ch rune, base, n int) rune {
- for n > 0 && digitVal(ch) < base {
- ch = s.next()
- n--
- }
- if n > 0 {
- s.err("illegal char escape")
- }
-
- // we scanned all digits, put the last non digit char back
- s.unread()
- return ch
-}
-
-// scanIdentifier scans an identifier and returns the literal string
-func (s *Scanner) scanIdentifier() string {
- offs := s.srcPos.Offset - s.lastCharLen
- ch := s.next()
- for isLetter(ch) || isDigit(ch) || ch == '-' {
- ch = s.next()
- }
-
- if ch != eof {
- s.unread() // we got identifier, put back latest char
- }
-
- return string(s.src[offs:s.srcPos.Offset])
-}
-
-// recentPosition returns the position of the character immediately after the
-// character or token returned by the last call to Scan.
-func (s *Scanner) recentPosition() (pos token.Pos) {
- pos.Offset = s.srcPos.Offset - s.lastCharLen
- switch {
- case s.srcPos.Column > 0:
- // common case: last character was not a '\n'
- pos.Line = s.srcPos.Line
- pos.Column = s.srcPos.Column
- case s.lastLineLen > 0:
- // last character was a '\n'
- // (we cannot be at the beginning of the source
- // since we have called next() at least once)
- pos.Line = s.srcPos.Line - 1
- pos.Column = s.lastLineLen
- default:
- // at the beginning of the source
- pos.Line = 1
- pos.Column = 1
- }
- return
-}
-
-// err prints the error of any scanning to s.Error function. If the function is
-// not defined, by default it prints them to os.Stderr
-func (s *Scanner) err(msg string) {
- s.ErrorCount++
- pos := s.recentPosition()
-
- if s.Error != nil {
- s.Error(pos, msg)
- return
- }
-
- fmt.Fprintf(os.Stderr, "%s: %s\n", pos, msg)
-}
-
-// isHexadecimal returns true if the given rune is a letter
-func isLetter(ch rune) bool {
- return 'a' <= ch && ch <= 'z' || 'A' <= ch && ch <= 'Z' || ch == '_' || ch >= 0x80 && unicode.IsLetter(ch)
-}
-
-// isHexadecimal returns true if the given rune is a decimal digit
-func isDigit(ch rune) bool {
- return '0' <= ch && ch <= '9' || ch >= 0x80 && unicode.IsDigit(ch)
-}
-
-// isHexadecimal returns true if the given rune is a decimal number
-func isDecimal(ch rune) bool {
- return '0' <= ch && ch <= '9'
-}
-
-// isHexadecimal returns true if the given rune is an hexadecimal number
-func isHexadecimal(ch rune) bool {
- return '0' <= ch && ch <= '9' || 'a' <= ch && ch <= 'f' || 'A' <= ch && ch <= 'F'
-}
-
-// isWhitespace returns true if the rune is a space, tab, newline or carriage return
-func isWhitespace(ch rune) bool {
- return ch == ' ' || ch == '\t' || ch == '\n' || ch == '\r'
-}
-
-// digitVal returns the integer value of a given octal,decimal or hexadecimal rune
-func digitVal(ch rune) int {
- switch {
- case '0' <= ch && ch <= '9':
- return int(ch - '0')
- case 'a' <= ch && ch <= 'f':
- return int(ch - 'a' + 10)
- case 'A' <= ch && ch <= 'F':
- return int(ch - 'A' + 10)
- }
- return 16 // larger than any legal digit val
-}
diff --git a/vendor/github.com/hashicorp/hcl/json/token/position.go b/vendor/github.com/hashicorp/hcl/json/token/position.go
deleted file mode 100644
index 59c1bb72..00000000
--- a/vendor/github.com/hashicorp/hcl/json/token/position.go
+++ /dev/null
@@ -1,46 +0,0 @@
-package token
-
-import "fmt"
-
-// Pos describes an arbitrary source position
-// including the file, line, and column location.
-// A Position is valid if the line number is > 0.
-type Pos struct {
- Filename string // filename, if any
- Offset int // offset, starting at 0
- Line int // line number, starting at 1
- Column int // column number, starting at 1 (character count)
-}
-
-// IsValid returns true if the position is valid.
-func (p *Pos) IsValid() bool { return p.Line > 0 }
-
-// String returns a string in one of several forms:
-//
-// file:line:column valid position with file name
-// line:column valid position without file name
-// file invalid position with file name
-// - invalid position without file name
-func (p Pos) String() string {
- s := p.Filename
- if p.IsValid() {
- if s != "" {
- s += ":"
- }
- s += fmt.Sprintf("%d:%d", p.Line, p.Column)
- }
- if s == "" {
- s = "-"
- }
- return s
-}
-
-// Before reports whether the position p is before u.
-func (p Pos) Before(u Pos) bool {
- return u.Offset > p.Offset || u.Line > p.Line
-}
-
-// After reports whether the position p is after u.
-func (p Pos) After(u Pos) bool {
- return u.Offset < p.Offset || u.Line < p.Line
-}
diff --git a/vendor/github.com/hashicorp/hcl/json/token/token.go b/vendor/github.com/hashicorp/hcl/json/token/token.go
deleted file mode 100644
index 95a0c3ee..00000000
--- a/vendor/github.com/hashicorp/hcl/json/token/token.go
+++ /dev/null
@@ -1,118 +0,0 @@
-package token
-
-import (
- "fmt"
- "strconv"
-
- hcltoken "github.com/hashicorp/hcl/hcl/token"
-)
-
-// Token defines a single HCL token which can be obtained via the Scanner
-type Token struct {
- Type Type
- Pos Pos
- Text string
-}
-
-// Type is the set of lexical tokens of the HCL (HashiCorp Configuration Language)
-type Type int
-
-const (
- // Special tokens
- ILLEGAL Type = iota
- EOF
-
- identifier_beg
- literal_beg
- NUMBER // 12345
- FLOAT // 123.45
- BOOL // true,false
- STRING // "abc"
- NULL // null
- literal_end
- identifier_end
-
- operator_beg
- LBRACK // [
- LBRACE // {
- COMMA // ,
- PERIOD // .
- COLON // :
-
- RBRACK // ]
- RBRACE // }
-
- operator_end
-)
-
-var tokens = [...]string{
- ILLEGAL: "ILLEGAL",
-
- EOF: "EOF",
-
- NUMBER: "NUMBER",
- FLOAT: "FLOAT",
- BOOL: "BOOL",
- STRING: "STRING",
- NULL: "NULL",
-
- LBRACK: "LBRACK",
- LBRACE: "LBRACE",
- COMMA: "COMMA",
- PERIOD: "PERIOD",
- COLON: "COLON",
-
- RBRACK: "RBRACK",
- RBRACE: "RBRACE",
-}
-
-// String returns the string corresponding to the token tok.
-func (t Type) String() string {
- s := ""
- if 0 <= t && t < Type(len(tokens)) {
- s = tokens[t]
- }
- if s == "" {
- s = "token(" + strconv.Itoa(int(t)) + ")"
- }
- return s
-}
-
-// IsIdentifier returns true for tokens corresponding to identifiers and basic
-// type literals; it returns false otherwise.
-func (t Type) IsIdentifier() bool { return identifier_beg < t && t < identifier_end }
-
-// IsLiteral returns true for tokens corresponding to basic type literals; it
-// returns false otherwise.
-func (t Type) IsLiteral() bool { return literal_beg < t && t < literal_end }
-
-// IsOperator returns true for tokens corresponding to operators and
-// delimiters; it returns false otherwise.
-func (t Type) IsOperator() bool { return operator_beg < t && t < operator_end }
-
-// String returns the token's literal text. Note that this is only
-// applicable for certain token types, such as token.IDENT,
-// token.STRING, etc..
-func (t Token) String() string {
- return fmt.Sprintf("%s %s %s", t.Pos.String(), t.Type.String(), t.Text)
-}
-
-// HCLToken converts this token to an HCL token.
-//
-// The token type must be a literal type or this will panic.
-func (t Token) HCLToken() hcltoken.Token {
- switch t.Type {
- case BOOL:
- return hcltoken.Token{Type: hcltoken.BOOL, Text: t.Text}
- case FLOAT:
- return hcltoken.Token{Type: hcltoken.FLOAT, Text: t.Text}
- case NULL:
- return hcltoken.Token{Type: hcltoken.STRING, Text: ""}
- case NUMBER:
- return hcltoken.Token{Type: hcltoken.NUMBER, Text: t.Text}
- case STRING:
- return hcltoken.Token{Type: hcltoken.STRING, Text: t.Text, JSON: true}
- default:
- panic(fmt.Sprintf("unimplemented HCLToken for type: %s", t.Type))
- }
-}
diff --git a/vendor/github.com/hashicorp/hcl/lex.go b/vendor/github.com/hashicorp/hcl/lex.go
deleted file mode 100644
index d9993c29..00000000
--- a/vendor/github.com/hashicorp/hcl/lex.go
+++ /dev/null
@@ -1,38 +0,0 @@
-package hcl
-
-import (
- "unicode"
- "unicode/utf8"
-)
-
-type lexModeValue byte
-
-const (
- lexModeUnknown lexModeValue = iota
- lexModeHcl
- lexModeJson
-)
-
-// lexMode returns whether we're going to be parsing in JSON
-// mode or HCL mode.
-func lexMode(v []byte) lexModeValue {
- var (
- r rune
- w int
- offset int
- )
-
- for {
- r, w = utf8.DecodeRune(v[offset:])
- offset += w
- if unicode.IsSpace(r) {
- continue
- }
- if r == '{' {
- return lexModeJson
- }
- break
- }
-
- return lexModeHcl
-}
diff --git a/vendor/github.com/hashicorp/hcl/parse.go b/vendor/github.com/hashicorp/hcl/parse.go
deleted file mode 100644
index 1fca53c4..00000000
--- a/vendor/github.com/hashicorp/hcl/parse.go
+++ /dev/null
@@ -1,39 +0,0 @@
-package hcl
-
-import (
- "fmt"
-
- "github.com/hashicorp/hcl/hcl/ast"
- hclParser "github.com/hashicorp/hcl/hcl/parser"
- jsonParser "github.com/hashicorp/hcl/json/parser"
-)
-
-// ParseBytes accepts as input byte slice and returns ast tree.
-//
-// Input can be either JSON or HCL
-func ParseBytes(in []byte) (*ast.File, error) {
- return parse(in)
-}
-
-// ParseString accepts input as a string and returns ast tree.
-func ParseString(input string) (*ast.File, error) {
- return parse([]byte(input))
-}
-
-func parse(in []byte) (*ast.File, error) {
- switch lexMode(in) {
- case lexModeHcl:
- return hclParser.Parse(in)
- case lexModeJson:
- return jsonParser.Parse(in)
- }
-
- return nil, fmt.Errorf("unknown config format")
-}
-
-// Parse parses the given input and returns the root object.
-//
-// The input format can be either HCL or JSON.
-func Parse(input string) (*ast.File, error) {
- return parse([]byte(input))
-}
diff --git a/vendor/github.com/invopop/yaml/.gitignore b/vendor/github.com/invopop/yaml/.gitignore
deleted file mode 100644
index e256a31e..00000000
--- a/vendor/github.com/invopop/yaml/.gitignore
+++ /dev/null
@@ -1,20 +0,0 @@
-# OSX leaves these everywhere on SMB shares
-._*
-
-# Eclipse files
-.classpath
-.project
-.settings/**
-
-# Emacs save files
-*~
-
-# Vim-related files
-[._]*.s[a-w][a-z]
-[._]s[a-w][a-z]
-*.un~
-Session.vim
-.netrwhist
-
-# Go test binaries
-*.test
diff --git a/vendor/github.com/invopop/yaml/.golangci.toml b/vendor/github.com/invopop/yaml/.golangci.toml
deleted file mode 100644
index 4a438ca2..00000000
--- a/vendor/github.com/invopop/yaml/.golangci.toml
+++ /dev/null
@@ -1,15 +0,0 @@
-[run]
-timeout = "120s"
-
-[output]
-format = "colored-line-number"
-
-[linters]
-enable = [
- "gocyclo", "unconvert", "goimports", "unused", "varcheck",
- "vetshadow", "misspell", "nakedret", "errcheck", "revive", "ineffassign",
- "deadcode", "goconst", "vet", "unparam", "gofmt"
-]
-
-[issues]
-exclude-use-default = false
diff --git a/vendor/github.com/invopop/yaml/LICENSE b/vendor/github.com/invopop/yaml/LICENSE
deleted file mode 100644
index 7805d36d..00000000
--- a/vendor/github.com/invopop/yaml/LICENSE
+++ /dev/null
@@ -1,50 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2014 Sam Ghods
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
-
-
-Copyright (c) 2012 The Go Authors. All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are
-met:
-
- * Redistributions of source code must retain the above copyright
-notice, this list of conditions and the following disclaimer.
- * Redistributions in binary form must reproduce the above
-copyright notice, this list of conditions and the following disclaimer
-in the documentation and/or other materials provided with the
-distribution.
- * Neither the name of Google Inc. nor the names of its
-contributors may be used to endorse or promote products derived from
-this software without specific prior written permission.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
-"AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
-LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
-A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
-OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
-SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
-LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
-DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
-THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/invopop/yaml/README.md b/vendor/github.com/invopop/yaml/README.md
deleted file mode 100644
index 2c33dfe5..00000000
--- a/vendor/github.com/invopop/yaml/README.md
+++ /dev/null
@@ -1,128 +0,0 @@
-# YAML marshaling and unmarshaling support for Go
-
-[](https://github.com/invopop/yaml/actions/workflows/lint.yaml)
-[](https://github.com/invopop/yaml/actions/workflows/test.yaml)
-[](https://goreportcard.com/report/github.com/invopop/yaml)
-
-
-## Introduction
-
-A wrapper around [go-yaml](https://github.com/go-yaml/yaml) designed to enable a better way of handling YAML when marshaling to and from structs.
-
-This is a fork and split of the original [ghodss/yaml](https://github.com/ghodss/yaml) repository which no longer appears to be maintained.
-
-In short, this library first converts YAML to JSON using go-yaml and then uses `json.Marshal` and `json.Unmarshal` to convert to or from the struct. This means that it effectively reuses the JSON struct tags as well as the custom JSON methods `MarshalJSON` and `UnmarshalJSON` unlike go-yaml. For a detailed overview of the rationale behind this method, [see this blog post](https://web.archive.org/web/20150812020634/http://ghodss.com/2014/the-right-way-to-handle-yaml-in-golang/).
-
-## Compatibility
-
-This package uses [go-yaml](https://github.com/go-yaml/yaml) and therefore supports [everything go-yaml supports](https://github.com/go-yaml/yaml#compatibility).
-
-Tested against Go versions 1.14 and onwards.
-
-## Caveats
-
-**Caveat #1:** When using `yaml.Marshal` and `yaml.Unmarshal`, binary data should NOT be preceded with the `!!binary` YAML tag. If you do, go-yaml will convert the binary data from base64 to native binary data, which is not compatible with JSON. You can still use binary in your YAML files though - just store them without the `!!binary` tag and decode the base64 in your code (e.g. in the custom JSON methods `MarshalJSON` and `UnmarshalJSON`). This also has the benefit that your YAML and your JSON binary data will be decoded exactly the same way. As an example:
-
-```
-BAD:
- exampleKey: !!binary gIGC
-
-GOOD:
- exampleKey: gIGC
-... and decode the base64 data in your code.
-```
-
-**Caveat #2:** When using `YAMLToJSON` directly, maps with keys that are maps will result in an error since this is not supported by JSON. This error will occur in `Unmarshal` as well since you can't unmarshal map keys anyways since struct fields can't be keys.
-
-## Installation and usage
-
-To install, run:
-
-```
-$ go get github.com/invopop/yaml
-```
-
-And import using:
-
-```
-import "github.com/invopop/yaml"
-```
-
-Usage is very similar to the JSON library:
-
-```go
-package main
-
-import (
- "fmt"
-
- "github.com/invopop/yaml"
-)
-
-type Person struct {
- Name string `json:"name"` // Affects YAML field names too.
- Age int `json:"age"`
-}
-
-func main() {
- // Marshal a Person struct to YAML.
- p := Person{"John", 30}
- y, err := yaml.Marshal(p)
- if err != nil {
- fmt.Printf("err: %v\n", err)
- return
- }
- fmt.Println(string(y))
- /* Output:
- age: 30
- name: John
- */
-
- // Unmarshal the YAML back into a Person struct.
- var p2 Person
- err = yaml.Unmarshal(y, &p2)
- if err != nil {
- fmt.Printf("err: %v\n", err)
- return
- }
- fmt.Println(p2)
- /* Output:
- {John 30}
- */
-}
-```
-
-`yaml.YAMLToJSON` and `yaml.JSONToYAML` methods are also available:
-
-```go
-package main
-
-import (
- "fmt"
-
- "github.com/invopop/yaml"
-)
-
-func main() {
- j := []byte(`{"name": "John", "age": 30}`)
- y, err := yaml.JSONToYAML(j)
- if err != nil {
- fmt.Printf("err: %v\n", err)
- return
- }
- fmt.Println(string(y))
- /* Output:
- name: John
- age: 30
- */
- j2, err := yaml.YAMLToJSON(y)
- if err != nil {
- fmt.Printf("err: %v\n", err)
- return
- }
- fmt.Println(string(j2))
- /* Output:
- {"age":30,"name":"John"}
- */
-}
-```
diff --git a/vendor/github.com/invopop/yaml/fields.go b/vendor/github.com/invopop/yaml/fields.go
deleted file mode 100644
index 52b30c6b..00000000
--- a/vendor/github.com/invopop/yaml/fields.go
+++ /dev/null
@@ -1,498 +0,0 @@
-// Copyright 2013 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package yaml
-
-import (
- "bytes"
- "encoding"
- "encoding/json"
- "reflect"
- "sort"
- "strings"
- "sync"
- "unicode"
- "unicode/utf8"
-)
-
-// indirect walks down v allocating pointers as needed,
-// until it gets to a non-pointer.
-// if it encounters an Unmarshaler, indirect stops and returns that.
-// if decodingNull is true, indirect stops at the last pointer so it can be set to nil.
-func indirect(v reflect.Value, decodingNull bool) (json.Unmarshaler, encoding.TextUnmarshaler, reflect.Value) {
- // If v is a named type and is addressable,
- // start with its address, so that if the type has pointer methods,
- // we find them.
- if v.Kind() != reflect.Ptr && v.Type().Name() != "" && v.CanAddr() {
- v = v.Addr()
- }
- for {
- // Load value from interface, but only if the result will be
- // usefully addressable.
- if v.Kind() == reflect.Interface && !v.IsNil() {
- e := v.Elem()
- if e.Kind() == reflect.Ptr && !e.IsNil() && (!decodingNull || e.Elem().Kind() == reflect.Ptr) {
- v = e
- continue
- }
- }
-
- if v.Kind() != reflect.Ptr {
- break
- }
-
- if v.Elem().Kind() != reflect.Ptr && decodingNull && v.CanSet() {
- break
- }
- if v.IsNil() {
- if v.CanSet() {
- v.Set(reflect.New(v.Type().Elem()))
- } else {
- v = reflect.New(v.Type().Elem())
- }
- }
- if v.Type().NumMethod() > 0 {
- if u, ok := v.Interface().(json.Unmarshaler); ok {
- return u, nil, reflect.Value{}
- }
- if u, ok := v.Interface().(encoding.TextUnmarshaler); ok {
- return nil, u, reflect.Value{}
- }
- }
- v = v.Elem()
- }
- return nil, nil, v
-}
-
-// A field represents a single field found in a struct.
-type field struct {
- name string
- nameBytes []byte // []byte(name)
- equalFold func(s, t []byte) bool // bytes.EqualFold or equivalent
-
- tag bool
- index []int
- typ reflect.Type
- omitEmpty bool
- quoted bool
-}
-
-func fillField(f field) field {
- f.nameBytes = []byte(f.name)
- f.equalFold = foldFunc(f.nameBytes)
- return f
-}
-
-// byName sorts field by name, breaking ties with depth,
-// then breaking ties with "name came from json tag", then
-// breaking ties with index sequence.
-type byName []field
-
-func (x byName) Len() int { return len(x) }
-
-func (x byName) Swap(i, j int) { x[i], x[j] = x[j], x[i] }
-
-func (x byName) Less(i, j int) bool {
- if x[i].name != x[j].name {
- return x[i].name < x[j].name
- }
- if len(x[i].index) != len(x[j].index) {
- return len(x[i].index) < len(x[j].index)
- }
- if x[i].tag != x[j].tag {
- return x[i].tag
- }
- return byIndex(x).Less(i, j)
-}
-
-// byIndex sorts field by index sequence.
-type byIndex []field
-
-func (x byIndex) Len() int { return len(x) }
-
-func (x byIndex) Swap(i, j int) { x[i], x[j] = x[j], x[i] }
-
-func (x byIndex) Less(i, j int) bool {
- for k, xik := range x[i].index {
- if k >= len(x[j].index) {
- return false
- }
- if xik != x[j].index[k] {
- return xik < x[j].index[k]
- }
- }
- return len(x[i].index) < len(x[j].index)
-}
-
-// typeFields returns a list of fields that JSON should recognize for the given type.
-// The algorithm is breadth-first search over the set of structs to include - the top struct
-// and then any reachable anonymous structs.
-func typeFields(t reflect.Type) []field {
- // Anonymous fields to explore at the current level and the next.
- current := []field{}
- next := []field{{typ: t}}
-
- // Count of queued names for current level and the next.
- var count, nextCount map[reflect.Type]int
-
- // Types already visited at an earlier level.
- visited := map[reflect.Type]bool{}
-
- // Fields found.
- var fields []field
-
- for len(next) > 0 {
- current, next = next, current[:0]
- count, nextCount = nextCount, map[reflect.Type]int{}
-
- for _, f := range current {
- if visited[f.typ] {
- continue
- }
- visited[f.typ] = true
-
- // Scan f.typ for fields to include.
- for i := 0; i < f.typ.NumField(); i++ {
- sf := f.typ.Field(i)
- if sf.PkgPath != "" { // unexported
- continue
- }
- tag := sf.Tag.Get("json")
- if tag == "-" {
- continue
- }
- name, opts := parseTag(tag)
- if !isValidTag(name) {
- name = ""
- }
- index := make([]int, len(f.index)+1)
- copy(index, f.index)
- index[len(f.index)] = i
-
- ft := sf.Type
- if ft.Name() == "" && ft.Kind() == reflect.Ptr {
- // Follow pointer.
- ft = ft.Elem()
- }
-
- // Record found field and index sequence.
- if name != "" || !sf.Anonymous || ft.Kind() != reflect.Struct {
- tagged := name != ""
- if name == "" {
- name = sf.Name
- }
- fields = append(fields, fillField(field{
- name: name,
- tag: tagged,
- index: index,
- typ: ft,
- omitEmpty: opts.Contains("omitempty"),
- quoted: opts.Contains("string"),
- }))
- if count[f.typ] > 1 {
- // If there were multiple instances, add a second,
- // so that the annihilation code will see a duplicate.
- // It only cares about the distinction between 1 or 2,
- // so don't bother generating any more copies.
- fields = append(fields, fields[len(fields)-1])
- }
- continue
- }
-
- // Record new anonymous struct to explore in next round.
- nextCount[ft]++
- if nextCount[ft] == 1 {
- next = append(next, fillField(field{name: ft.Name(), index: index, typ: ft}))
- }
- }
- }
- }
-
- sort.Sort(byName(fields))
-
- // Delete all fields that are hidden by the Go rules for embedded fields,
- // except that fields with JSON tags are promoted.
-
- // The fields are sorted in primary order of name, secondary order
- // of field index length. Loop over names; for each name, delete
- // hidden fields by choosing the one dominant field that survives.
- out := fields[:0]
- for advance, i := 0, 0; i < len(fields); i += advance {
- // One iteration per name.
- // Find the sequence of fields with the name of this first field.
- fi := fields[i]
- name := fi.name
- for advance = 1; i+advance < len(fields); advance++ {
- fj := fields[i+advance]
- if fj.name != name {
- break
- }
- }
- if advance == 1 { // Only one field with this name
- out = append(out, fi)
- continue
- }
- dominant, ok := dominantField(fields[i : i+advance])
- if ok {
- out = append(out, dominant)
- }
- }
-
- fields = out
- sort.Sort(byIndex(fields))
-
- return fields
-}
-
-// dominantField looks through the fields, all of which are known to
-// have the same name, to find the single field that dominates the
-// others using Go's embedding rules, modified by the presence of
-// JSON tags. If there are multiple top-level fields, the boolean
-// will be false: This condition is an error in Go and we skip all
-// the fields.
-func dominantField(fields []field) (field, bool) {
- // The fields are sorted in increasing index-length order. The winner
- // must therefore be one with the shortest index length. Drop all
- // longer entries, which is easy: just truncate the slice.
- length := len(fields[0].index)
- tagged := -1 // Index of first tagged field.
- for i, f := range fields {
- if len(f.index) > length {
- fields = fields[:i]
- break
- }
- if f.tag {
- if tagged >= 0 {
- // Multiple tagged fields at the same level: conflict.
- // Return no field.
- return field{}, false
- }
- tagged = i
- }
- }
- if tagged >= 0 {
- return fields[tagged], true
- }
- // All remaining fields have the same length. If there's more than one,
- // we have a conflict (two fields named "X" at the same level) and we
- // return no field.
- if len(fields) > 1 {
- return field{}, false
- }
- return fields[0], true
-}
-
-var fieldCache struct {
- sync.RWMutex
- m map[reflect.Type][]field
-}
-
-// cachedTypeFields is like typeFields but uses a cache to avoid repeated work.
-func cachedTypeFields(t reflect.Type) []field {
- fieldCache.RLock()
- f := fieldCache.m[t]
- fieldCache.RUnlock()
- if f != nil {
- return f
- }
-
- // Compute fields without lock.
- // Might duplicate effort but won't hold other computations back.
- f = typeFields(t)
- if f == nil {
- f = []field{}
- }
-
- fieldCache.Lock()
- if fieldCache.m == nil {
- fieldCache.m = map[reflect.Type][]field{}
- }
- fieldCache.m[t] = f
- fieldCache.Unlock()
- return f
-}
-
-func isValidTag(s string) bool {
- if s == "" {
- return false
- }
- for _, c := range s {
- switch {
- case strings.ContainsRune("!#$%&()*+-./:<=>?@[]^_{|}~ ", c):
- // Backslash and quote chars are reserved, but
- // otherwise any punctuation chars are allowed
- // in a tag name.
- default:
- if !unicode.IsLetter(c) && !unicode.IsDigit(c) {
- return false
- }
- }
- }
- return true
-}
-
-const (
- caseMask = ^byte(0x20) // Mask to ignore case in ASCII.
- kelvin = '\u212a'
- smallLongEss = '\u017f'
-)
-
-// foldFunc returns one of four different case folding equivalence
-// functions, from most general (and slow) to fastest:
-//
-// 1) bytes.EqualFold, if the key s contains any non-ASCII UTF-8
-// 2) equalFoldRight, if s contains special folding ASCII ('k', 'K', 's', 'S')
-// 3) asciiEqualFold, no special, but includes non-letters (including _)
-// 4) simpleLetterEqualFold, no specials, no non-letters.
-//
-// The letters S and K are special because they map to 3 runes, not just 2:
-// * S maps to s and to U+017F 'Å¿' Latin small letter long s
-// * k maps to K and to U+212A 'K' Kelvin sign
-// See http://play.golang.org/p/tTxjOc0OGo
-//
-// The returned function is specialized for matching against s and
-// should only be given s. It's not curried for performance reasons.
-func foldFunc(s []byte) func(s, t []byte) bool {
- nonLetter := false
- special := false // special letter
- for _, b := range s {
- if b >= utf8.RuneSelf {
- return bytes.EqualFold
- }
- upper := b & caseMask
- if upper < 'A' || upper > 'Z' {
- nonLetter = true
- } else if upper == 'K' || upper == 'S' {
- // See above for why these letters are special.
- special = true
- }
- }
- if special {
- return equalFoldRight
- }
- if nonLetter {
- return asciiEqualFold
- }
- return simpleLetterEqualFold
-}
-
-// equalFoldRight is a specialization of bytes.EqualFold when s is
-// known to be all ASCII (including punctuation), but contains an 's',
-// 'S', 'k', or 'K', requiring a Unicode fold on the bytes in t.
-// See comments on foldFunc.
-func equalFoldRight(s, t []byte) bool {
- for _, sb := range s {
- if len(t) == 0 {
- return false
- }
- tb := t[0]
- if tb < utf8.RuneSelf {
- if sb != tb {
- sbUpper := sb & caseMask
- if 'A' <= sbUpper && sbUpper <= 'Z' {
- if sbUpper != tb&caseMask {
- return false
- }
- } else {
- return false
- }
- }
- t = t[1:]
- continue
- }
- // sb is ASCII and t is not. t must be either kelvin
- // sign or long s; sb must be s, S, k, or K.
- tr, size := utf8.DecodeRune(t)
- switch sb {
- case 's', 'S':
- if tr != smallLongEss {
- return false
- }
- case 'k', 'K':
- if tr != kelvin {
- return false
- }
- default:
- return false
- }
- t = t[size:]
-
- }
- return len(t) <= 0
-}
-
-// asciiEqualFold is a specialization of bytes.EqualFold for use when
-// s is all ASCII (but may contain non-letters) and contains no
-// special-folding letters.
-// See comments on foldFunc.
-func asciiEqualFold(s, t []byte) bool {
- if len(s) != len(t) {
- return false
- }
- for i, sb := range s {
- tb := t[i]
- if sb == tb {
- continue
- }
- if ('a' <= sb && sb <= 'z') || ('A' <= sb && sb <= 'Z') {
- if sb&caseMask != tb&caseMask {
- return false
- }
- } else {
- return false
- }
- }
- return true
-}
-
-// simpleLetterEqualFold is a specialization of bytes.EqualFold for
-// use when s is all ASCII letters (no underscores, etc) and also
-// doesn't contain 'k', 'K', 's', or 'S'.
-// See comments on foldFunc.
-func simpleLetterEqualFold(s, t []byte) bool {
- if len(s) != len(t) {
- return false
- }
- for i, b := range s {
- if b&caseMask != t[i]&caseMask {
- return false
- }
- }
- return true
-}
-
-// tagOptions is the string following a comma in a struct field's "json"
-// tag, or the empty string. It does not include the leading comma.
-type tagOptions string
-
-// parseTag splits a struct field's json tag into its name and
-// comma-separated options.
-func parseTag(tag string) (string, tagOptions) {
- if idx := strings.Index(tag, ","); idx != -1 {
- return tag[:idx], tagOptions(tag[idx+1:])
- }
- return tag, tagOptions("")
-}
-
-// Contains reports whether a comma-separated list of options
-// contains a particular substr flag. substr must be surrounded by a
-// string boundary or commas.
-func (o tagOptions) Contains(optionName string) bool {
- if len(o) == 0 {
- return false
- }
- s := string(o)
- for s != "" {
- var next string
- i := strings.Index(s, ",")
- if i >= 0 {
- s, next = s[:i], s[i+1:]
- }
- if s == optionName {
- return true
- }
- s = next
- }
- return false
-}
diff --git a/vendor/github.com/invopop/yaml/yaml.go b/vendor/github.com/invopop/yaml/yaml.go
deleted file mode 100644
index 66c5c668..00000000
--- a/vendor/github.com/invopop/yaml/yaml.go
+++ /dev/null
@@ -1,309 +0,0 @@
-// Package yaml provides a wrapper around go-yaml designed to enable a better
-// way of handling YAML when marshaling to and from structs.
-//
-// In short, this package first converts YAML to JSON using go-yaml and then
-// uses json.Marshal and json.Unmarshal to convert to or from the struct. This
-// means that it effectively reuses the JSON struct tags as well as the custom
-// JSON methods MarshalJSON and UnmarshalJSON unlike go-yaml.
-//
-package yaml // import "github.com/invopop/yaml"
-
-import (
- "bytes"
- "encoding/json"
- "fmt"
- "io"
- "reflect"
- "strconv"
-
- "gopkg.in/yaml.v3"
-)
-
-// Marshal the object into JSON then converts JSON to YAML and returns the
-// YAML.
-func Marshal(o interface{}) ([]byte, error) {
- j, err := json.Marshal(o)
- if err != nil {
- return nil, fmt.Errorf("error marshaling into JSON: %v", err)
- }
-
- y, err := JSONToYAML(j)
- if err != nil {
- return nil, fmt.Errorf("error converting JSON to YAML: %v", err)
- }
-
- return y, nil
-}
-
-// JSONOpt is a decoding option for decoding from JSON format.
-type JSONOpt func(*json.Decoder) *json.Decoder
-
-// Unmarshal converts YAML to JSON then uses JSON to unmarshal into an object,
-// optionally configuring the behavior of the JSON unmarshal.
-func Unmarshal(y []byte, o interface{}, opts ...JSONOpt) error {
- dec := yaml.NewDecoder(bytes.NewReader(y))
- return unmarshal(dec, o, opts)
-}
-
-func unmarshal(dec *yaml.Decoder, o interface{}, opts []JSONOpt) error {
- vo := reflect.ValueOf(o)
- j, err := yamlToJSON(dec, &vo)
- if err != nil {
- return fmt.Errorf("error converting YAML to JSON: %v", err)
- }
-
- err = jsonUnmarshal(bytes.NewReader(j), o, opts...)
- if err != nil {
- return fmt.Errorf("error unmarshaling JSON: %v", err)
- }
-
- return nil
-}
-
-// jsonUnmarshal unmarshals the JSON byte stream from the given reader into the
-// object, optionally applying decoder options prior to decoding. We are not
-// using json.Unmarshal directly as we want the chance to pass in non-default
-// options.
-func jsonUnmarshal(r io.Reader, o interface{}, opts ...JSONOpt) error {
- d := json.NewDecoder(r)
- for _, opt := range opts {
- d = opt(d)
- }
- if err := d.Decode(&o); err != nil {
- return fmt.Errorf("while decoding JSON: %v", err)
- }
- return nil
-}
-
-// JSONToYAML converts JSON to YAML.
-func JSONToYAML(j []byte) ([]byte, error) {
- // Convert the JSON to an object.
- var jsonObj interface{}
- // We are using yaml.Unmarshal here (instead of json.Unmarshal) because the
- // Go JSON library doesn't try to pick the right number type (int, float,
- // etc.) when unmarshalling to interface{}, it just picks float64
- // universally. go-yaml does go through the effort of picking the right
- // number type, so we can preserve number type throughout this process.
- err := yaml.Unmarshal(j, &jsonObj)
- if err != nil {
- return nil, err
- }
-
- // Marshal this object into YAML.
- return yaml.Marshal(jsonObj)
-}
-
-// YAMLToJSON converts YAML to JSON. Since JSON is a subset of YAML,
-// passing JSON through this method should be a no-op.
-//
-// Things YAML can do that are not supported by JSON:
-// * In YAML you can have binary and null keys in your maps. These are invalid
-// in JSON. (int and float keys are converted to strings.)
-// * Binary data in YAML with the !!binary tag is not supported. If you want to
-// use binary data with this library, encode the data as base64 as usual but do
-// not use the !!binary tag in your YAML. This will ensure the original base64
-// encoded data makes it all the way through to the JSON.
-//
-func YAMLToJSON(y []byte) ([]byte, error) { //nolint:revive
- dec := yaml.NewDecoder(bytes.NewReader(y))
- return yamlToJSON(dec, nil)
-}
-
-func yamlToJSON(dec *yaml.Decoder, jsonTarget *reflect.Value) ([]byte, error) {
- // Convert the YAML to an object.
- var yamlObj interface{}
- if err := dec.Decode(&yamlObj); err != nil {
- return nil, err
- }
-
- // YAML objects are not completely compatible with JSON objects (e.g. you
- // can have non-string keys in YAML). So, convert the YAML-compatible object
- // to a JSON-compatible object, failing with an error if irrecoverable
- // incompatibilities happen along the way.
- jsonObj, err := convertToJSONableObject(yamlObj, jsonTarget)
- if err != nil {
- return nil, err
- }
-
- // Convert this object to JSON and return the data.
- return json.Marshal(jsonObj)
-}
-
-func convertToJSONableObject(yamlObj interface{}, jsonTarget *reflect.Value) (interface{}, error) { //nolint:gocyclo
- var err error
-
- // Resolve jsonTarget to a concrete value (i.e. not a pointer or an
- // interface). We pass decodingNull as false because we're not actually
- // decoding into the value, we're just checking if the ultimate target is a
- // string.
- if jsonTarget != nil {
- ju, tu, pv := indirect(*jsonTarget, false)
- // We have a JSON or Text Umarshaler at this level, so we can't be trying
- // to decode into a string.
- if ju != nil || tu != nil {
- jsonTarget = nil
- } else {
- jsonTarget = &pv
- }
- }
-
- // go-yaml v3 changed from v2 and now will provide map[string]interface{} by
- // default and map[interface{}]interface{} when none of the keys strings.
- // To get around this, we run a pre-loop to convert the map.
- // JSON only supports strings as keys, so we must convert.
-
- switch typedYAMLObj := yamlObj.(type) {
- case map[interface{}]interface{}:
- // From my reading of go-yaml v2 (specifically the resolve function),
- // keys can only have the types string, int, int64, float64, binary
- // (unsupported), or null (unsupported).
- strMap := make(map[string]interface{})
- for k, v := range typedYAMLObj {
- // Resolve the key to a string first.
- var keyString string
- switch typedKey := k.(type) {
- case string:
- keyString = typedKey
- case int:
- keyString = strconv.Itoa(typedKey)
- case int64:
- // go-yaml will only return an int64 as a key if the system
- // architecture is 32-bit and the key's value is between 32-bit
- // and 64-bit. Otherwise the key type will simply be int.
- keyString = strconv.FormatInt(typedKey, 10)
- case float64:
- // Float64 is now supported in keys
- keyString = strconv.FormatFloat(typedKey, 'g', -1, 64)
- case bool:
- if typedKey {
- keyString = "true"
- } else {
- keyString = "false"
- }
- default:
- return nil, fmt.Errorf("unsupported map key of type: %s, key: %+#v, value: %+#v",
- reflect.TypeOf(k), k, v)
- }
- strMap[keyString] = v
- }
- // replace yamlObj with our new string map
- yamlObj = strMap
- }
-
- // If yamlObj is a number or a boolean, check if jsonTarget is a string -
- // if so, coerce. Else return normal.
- // If yamlObj is a map or array, find the field that each key is
- // unmarshaling to, and when you recurse pass the reflect.Value for that
- // field back into this function.
- switch typedYAMLObj := yamlObj.(type) {
- case map[string]interface{}:
- for k, v := range typedYAMLObj {
-
- // jsonTarget should be a struct or a map. If it's a struct, find
- // the field it's going to map to and pass its reflect.Value. If
- // it's a map, find the element type of the map and pass the
- // reflect.Value created from that type. If it's neither, just pass
- // nil - JSON conversion will error for us if it's a real issue.
- if jsonTarget != nil {
- t := *jsonTarget
- if t.Kind() == reflect.Struct {
- keyBytes := []byte(k)
- // Find the field that the JSON library would use.
- var f *field
- fields := cachedTypeFields(t.Type())
- for i := range fields {
- ff := &fields[i]
- if bytes.Equal(ff.nameBytes, keyBytes) {
- f = ff
- break
- }
- // Do case-insensitive comparison.
- if f == nil && ff.equalFold(ff.nameBytes, keyBytes) {
- f = ff
- }
- }
- if f != nil {
- // Find the reflect.Value of the most preferential
- // struct field.
- jtf := t.Field(f.index[0])
- typedYAMLObj[k], err = convertToJSONableObject(v, &jtf)
- if err != nil {
- return nil, err
- }
- continue
- }
- } else if t.Kind() == reflect.Map {
- // Create a zero value of the map's element type to use as
- // the JSON target.
- jtv := reflect.Zero(t.Type().Elem())
- typedYAMLObj[k], err = convertToJSONableObject(v, &jtv)
- if err != nil {
- return nil, err
- }
- continue
- }
- }
- typedYAMLObj[k], err = convertToJSONableObject(v, nil)
- if err != nil {
- return nil, err
- }
- }
- return typedYAMLObj, nil
- case []interface{}:
- // We need to recurse into arrays in case there are any
- // map[interface{}]interface{}'s inside and to convert any
- // numbers to strings.
-
- // If jsonTarget is a slice (which it really should be), find the
- // thing it's going to map to. If it's not a slice, just pass nil
- // - JSON conversion will error for us if it's a real issue.
- var jsonSliceElemValue *reflect.Value
- if jsonTarget != nil {
- t := *jsonTarget
- if t.Kind() == reflect.Slice {
- // By default slices point to nil, but we need a reflect.Value
- // pointing to a value of the slice type, so we create one here.
- ev := reflect.Indirect(reflect.New(t.Type().Elem()))
- jsonSliceElemValue = &ev
- }
- }
-
- // Make and use a new array.
- arr := make([]interface{}, len(typedYAMLObj))
- for i, v := range typedYAMLObj {
- arr[i], err = convertToJSONableObject(v, jsonSliceElemValue)
- if err != nil {
- return nil, err
- }
- }
- return arr, nil
- default:
- // If the target type is a string and the YAML type is a number,
- // convert the YAML type to a string.
- if jsonTarget != nil && (*jsonTarget).Kind() == reflect.String {
- // Based on my reading of go-yaml, it may return int, int64,
- // float64, or uint64.
- var s string
- switch typedVal := typedYAMLObj.(type) {
- case int:
- s = strconv.FormatInt(int64(typedVal), 10)
- case int64:
- s = strconv.FormatInt(typedVal, 10)
- case float64:
- s = strconv.FormatFloat(typedVal, 'g', -1, 64)
- case uint64:
- s = strconv.FormatUint(typedVal, 10)
- case bool:
- if typedVal {
- s = "true"
- } else {
- s = "false"
- }
- }
- if len(s) > 0 {
- yamlObj = interface{}(s)
- }
- }
- return yamlObj, nil
- }
-}
diff --git a/vendor/github.com/josharian/intern/README.md b/vendor/github.com/josharian/intern/README.md
deleted file mode 100644
index ffc44b21..00000000
--- a/vendor/github.com/josharian/intern/README.md
+++ /dev/null
@@ -1,5 +0,0 @@
-Docs: https://godoc.org/github.com/josharian/intern
-
-See also [Go issue 5160](https://golang.org/issue/5160).
-
-License: MIT
diff --git a/vendor/github.com/josharian/intern/intern.go b/vendor/github.com/josharian/intern/intern.go
deleted file mode 100644
index 7acb1fe9..00000000
--- a/vendor/github.com/josharian/intern/intern.go
+++ /dev/null
@@ -1,44 +0,0 @@
-// Package intern interns strings.
-// Interning is best effort only.
-// Interned strings may be removed automatically
-// at any time without notification.
-// All functions may be called concurrently
-// with themselves and each other.
-package intern
-
-import "sync"
-
-var (
- pool sync.Pool = sync.Pool{
- New: func() interface{} {
- return make(map[string]string)
- },
- }
-)
-
-// String returns s, interned.
-func String(s string) string {
- m := pool.Get().(map[string]string)
- c, ok := m[s]
- if ok {
- pool.Put(m)
- return c
- }
- m[s] = s
- pool.Put(m)
- return s
-}
-
-// Bytes returns b converted to a string, interned.
-func Bytes(b []byte) string {
- m := pool.Get().(map[string]string)
- c, ok := m[string(b)]
- if ok {
- pool.Put(m)
- return c
- }
- s := string(b)
- m[s] = s
- pool.Put(m)
- return s
-}
diff --git a/vendor/github.com/josharian/intern/license.md b/vendor/github.com/josharian/intern/license.md
deleted file mode 100644
index 353d3055..00000000
--- a/vendor/github.com/josharian/intern/license.md
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2019 Josh Bleecher Snyder
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/leanovate/gopter/.gitignore b/vendor/github.com/leanovate/gopter/.gitignore
deleted file mode 100644
index 45371a9f..00000000
--- a/vendor/github.com/leanovate/gopter/.gitignore
+++ /dev/null
@@ -1,30 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
-
-bin/
-*.iml
-.idea/
-coverage.txt
-.pkg.coverage
diff --git a/vendor/github.com/leanovate/gopter/.travis.yml b/vendor/github.com/leanovate/gopter/.travis.yml
deleted file mode 100644
index 954d5260..00000000
--- a/vendor/github.com/leanovate/gopter/.travis.yml
+++ /dev/null
@@ -1,12 +0,0 @@
-sudo: false
-language: go
-go:
-- 1.x
-script: make all coverage refreshGodoc
-before_install:
- - pip install --user codecov
-after_success:
- - codecov
-notifications:
- slack:
- secure: M0PgOUB0Kzn0maWtd6NNtiKYINxMY/7zgbbDpb8mAa6NTPYuypEYkUgmo6HC74BzDWSjkJaLQOeZrumrOuJUKbGdT+eEYR1pXColp2qb/WxnSCAwlL9iM/k7pj6nIRUdlP7l6WX0QB/DNh+BC/9STHrcSKjBpUu38oO9CwT7klSj2hfPMjzcx7EO4f8pjSfwCrIyYbANKxLzP0lr4PcbdY/ZeGbc8R5/m9torzPjS2YXDl0tQQ7pvSS8UVToLfL0m+omp9A/lOu0n6FpdNIkof2Eu9qWJqsI7jy+Pi+8DGbfEyxSLKAhDiTn0nfO/5nwqWIBhUaVACBDxpaH6ewpiuMbs4RO+wNaEEuVEH8QMKZOx9PGgnzNJ3zZ5Hfm+FP8zBrwrKlsjUoy31waGFjgua2ne4X0wa+Ld4iFEsj+XoMKa1oxRKRXYFhyEywalwgBVjXH2+ZCMlFGV3QxaV5gVuYcfEuNQ4pOlJpk+WSgm7yfXEX2qosOk2p91yGyX2Msbe3B7Ov3PXVzs2CshIsYasHr46pLplMvG6Z+712TPsrFS0zhb8FAsm/Vd7xX2xxmNS/uffh3RgFzeZxg8S9/ObVq+JBkZAtK4j0SwLVsOkjI4W3yUVgfxvhnAM1iLzzeSyD64BSo1VyUZu1eSJ9YxJ1+K6ldo0u0hj2VHwO1vUE=
diff --git a/vendor/github.com/leanovate/gopter/CHANGELOG.md b/vendor/github.com/leanovate/gopter/CHANGELOG.md
deleted file mode 100644
index 40e7cc3c..00000000
--- a/vendor/github.com/leanovate/gopter/CHANGELOG.md
+++ /dev/null
@@ -1,65 +0,0 @@
-# Change log
-
-## [Unreleased]
-### Additions
-- `gopter.GenParameters` now has a `CloneWithSeed(seed int64)` function to
- temparary copies to create rerunable sections of code.
-- Added `gopter.Gen.MapResult` for power-user mappings
-- Added `gopter.DeriveGen` to derive a generator and it's shrinker from a
- bi-directional mapping (`gopter.BiMapper`)
-
-### Changed
-- Refactored `commands` package under the hood to allow the use of mutable state.
- Re-runability of commands is provided by invoking the `commands.GenInitialState`
- generator with the same `gopter.GenParameters`. Of course `commands.GenInitialState`
- is supposed to create the same state for the same parameters every time.
-- Fixed a bug in `commands` that might lead to shrinked command sequences not
- satisfying the precondtions.
-- `commands.Command.PostCondition` was called with the state before running the command. It makes
- much more sense to first do `commands.Command.NextState` and then `commands.Command.PostCondition`
-- `commands.Commands.NewSystemUnderTest` now takes has an argument `initialState commands.State` to
- allow implementators to create/bootstrap a system under test based on an arbitrary initial state.
- So far examples were just using a constant initial state ... which is a bit boring.
-- Fixed: Actually use `commands.Commands.InitialPreCondition` as sieve for
- `commands.Commands.GenInitialState`
-- Gen.Map and Shrink.Map now accept `interface{}` instead of `func (interface{}) interface{}`
-
- This allows cleaner mapping functions without type conversion. E.g. instead of
-
- ```Go
- gen.AnyString().Map(function (v interface{}) interface{} {
- return strings.ToUpper(v.(string))
- })
- ```
- you can (and should) now write
-
- ```Go
- gen.AnyString().Map(function (v string) string {
- return strings.ToUpper(v)
- })
- ```
-- Correspondingly Gen.SuchThat now also ccept `interface{}` instead of `func (interface{}) bool`
-
- This allows cleaner sieve functions without type conversion. E.g. instead of
-
- ```Go
- gen.AnyString().SuchThat(function (v interface{}) bool {
- return HasPrefix(v.(string), "P")
- })
- ```
- you can (and should) now write
-
- ```Go
- gen.AnyString().SuchThat(function (v string) bool {
- return HasPrefix(v, "P")
- })
- ```
-- Gen.FlatMap now has a second parameter `resultType reflect.Type` defining the result type of the mapped generator
-- Reason for these changes: The original `Map` and `FlatMap` had a recurring issue with empty results. If the original generator created an empty result there was no clean way to determine the result type of the mapped generator. The new version fixes this by extracting the return type of the mapping functions.
-
-## [0.1] - 2016-04-30
-### Added
-- Initial implementation.
-
-[Unreleased]: https://github.com/leanovate/gopter/compare/v0.1...HEAD
-[0.1]: https://github.com/leanovate/gopter/tree/v0.1
diff --git a/vendor/github.com/leanovate/gopter/LICENSE b/vendor/github.com/leanovate/gopter/LICENSE
deleted file mode 100644
index 072084c0..00000000
--- a/vendor/github.com/leanovate/gopter/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2016 leanovate
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/leanovate/gopter/Makefile b/vendor/github.com/leanovate/gopter/Makefile
deleted file mode 100644
index 2846b248..00000000
--- a/vendor/github.com/leanovate/gopter/Makefile
+++ /dev/null
@@ -1,41 +0,0 @@
-PACKAGES=$(shell go list ./...)
-
-all: format
- @go get github.com/smartystreets/goconvey
- @go build -v ./...
-
-format:
- @echo "--> Running go fmt"
- @gofmt -s -w .
-
-test:
- @echo "--> Running tests"
- @go test -v ./... -count=1
- @$(MAKE) vet
-
-coverage:
- @echo "--> Running tests with coverage"
- @echo "" > coverage.txt
- for pkg in $(shell go list ./...); do \
- (go test -coverprofile=.pkg.coverage -covermode=atomic -v $$pkg && \
- cat .pkg.coverage >> coverage.txt) || exit 1; \
- done
- @rm .pkg.coverage
- @$(MAKE) vet
-
-vet:
- @go vet 2>/dev/null ; if [ $$? -eq 3 ]; then \
- go get golang.org/x/tools/cmd/vet; \
- fi
- @echo "--> Running go vet $(VETARGS)"
- @find . -name "*.go" | grep -v "./Godeps/" | xargs go vet $(VETARGS); if [ $$? -eq 1 ]; then \
- echo ""; \
- echo "Vet found suspicious constructs. Please check the reported constructs"; \
- echo "and fix them if necessary before submitting the code for reviewal."; \
- fi
-
-refreshGodoc:
- @echo "--> Refreshing godoc.org"
- for pkg in $(shell go list ./...); do \
- curl -d "path=$$pkg" https://godoc.org/-/refresh ; \
- done
diff --git a/vendor/github.com/leanovate/gopter/README.md b/vendor/github.com/leanovate/gopter/README.md
deleted file mode 100644
index e4b0dad3..00000000
--- a/vendor/github.com/leanovate/gopter/README.md
+++ /dev/null
@@ -1,45 +0,0 @@
-# GOPTER
-
-... the GOlang Property TestER
-[](https://travis-ci.org/leanovate/gopter)
-[](https://codecov.io/gh/leanovate/gopter)
-[](https://godoc.org/github.com/leanovate/gopter)
-[](https://goreportcard.com/report/github.com/leanovate/gopter)
-
-[Change Log](CHANGELOG.md)
-
-## Synopsis
-
-Gopter tries to bring the goodness of [ScalaCheck](https://www.scalacheck.org/) (and implicitly, the goodness of [QuickCheck](http://hackage.haskell.org/package/QuickCheck)) to Go.
-It can also be seen as a more sophisticated version of the testing/quick package.
-
-Main differences to ScalaCheck:
-
-* It is Go ... duh
-* ... nevertheless: Do not expect the same typesafety and elegance as in ScalaCheck.
-* For simplicity [Shrink](https://www.scalacheck.org/files/scalacheck_2.11-1.14.0-api/index.html#org.scalacheck.Shrink) has become part of the generators. They can still be easily changed if necessary.
-* There is no [Pretty](https://www.scalacheck.org/files/scalacheck_2.11-1.14.0-api/index.html#org.scalacheck.util.Pretty) ... so far gopter feels quite comfortable being ugly.
-* A generator for regex matches
-* No parallel commands ... yet?
-
-Main differences to the testing/quick package:
-
-* Much tighter control over generators
-* Shrinkers, i.e. automatically find the minimum value falsifying a property
-* A generator for regex matches (already mentioned that ... but it's cool)
-* Support for stateful tests
-
-## Documentation
-
-Current godocs:
-
-* [gopter](https://godoc.org/github.com/leanovate/gopter): Main interfaces
-* [gopter/gen](https://godoc.org/github.com/leanovate/gopter/gen): All commonly used generators
-* [gopter/prop](https://godoc.org/github.com/leanovate/gopter/prop): Common helpers to create properties from a condition function and specific generators
-* [gopter/arbitrary](https://godoc.org/github.com/leanovate/gopter/arbitrary): Helpers automatically combine generators for arbitrary types
-* [gopter/commands](https://godoc.org/github.com/leanovate/gopter/commands): Helpers to create stateful tests based on arbitrary commands
-* [gopter/convey](https://godoc.org/github.com/leanovate/gopter/convey): Helpers used by gopter inside goconvey tests
-
-## License
-
-[MIT Licence](http://opensource.org/licenses/MIT)
diff --git a/vendor/github.com/leanovate/gopter/bi_mapper.go b/vendor/github.com/leanovate/gopter/bi_mapper.go
deleted file mode 100644
index 9df11298..00000000
--- a/vendor/github.com/leanovate/gopter/bi_mapper.go
+++ /dev/null
@@ -1,111 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "reflect"
-)
-
-// BiMapper is a bi-directional (or bijective) mapper of a tuple of values (up)
-// to another tuple of values (down).
-type BiMapper struct {
- UpTypes []reflect.Type
- DownTypes []reflect.Type
- Downstream reflect.Value
- Upstream reflect.Value
-}
-
-// NewBiMapper creates a BiMapper of two functions `downstream` and its
-// inverse `upstream`.
-// That is: The return values of `downstream` must match the parameters of
-// `upstream` and vice versa.
-func NewBiMapper(downstream interface{}, upstream interface{}) *BiMapper {
- downstreamVal := reflect.ValueOf(downstream)
- if downstreamVal.Kind() != reflect.Func {
- panic("downstream has to be a function")
- }
- upstreamVal := reflect.ValueOf(upstream)
- if upstreamVal.Kind() != reflect.Func {
- panic("upstream has to be a function")
- }
-
- downstreamType := downstreamVal.Type()
- upTypes := make([]reflect.Type, downstreamType.NumIn())
- for i := 0; i < len(upTypes); i++ {
- upTypes[i] = downstreamType.In(i)
- }
- downTypes := make([]reflect.Type, downstreamType.NumOut())
- for i := 0; i < len(downTypes); i++ {
- downTypes[i] = downstreamType.Out(i)
- }
-
- upstreamType := upstreamVal.Type()
- if len(upTypes) != upstreamType.NumOut() {
- panic(fmt.Sprintf("upstream is expected to have %d return values", len(upTypes)))
- }
- for i, upType := range upTypes {
- if upstreamType.Out(i) != upType {
- panic(fmt.Sprintf("upstream has wrong return type %d: %v != %v", i, upstreamType.Out(i), upType))
- }
- }
- if len(downTypes) != upstreamType.NumIn() {
- panic(fmt.Sprintf("upstream is expected to have %d parameters", len(downTypes)))
- }
- for i, downType := range downTypes {
- if upstreamType.In(i) != downType {
- panic(fmt.Sprintf("upstream has wrong parameter type %d: %v != %v", i, upstreamType.In(i), downType))
- }
- }
-
- return &BiMapper{
- UpTypes: upTypes,
- DownTypes: downTypes,
- Downstream: downstreamVal,
- Upstream: upstreamVal,
- }
-}
-
-// ConvertUp calls the Upstream function on the arguments in the down array
-// and returns the results.
-func (b *BiMapper) ConvertUp(down []interface{}) []interface{} {
- if len(down) != len(b.DownTypes) {
- panic(fmt.Sprintf("Expected %d values != %d", len(b.DownTypes), len(down)))
- }
- downVals := make([]reflect.Value, len(b.DownTypes))
- for i, val := range down {
- if val == nil {
- downVals[i] = reflect.Zero(b.DownTypes[i])
- } else {
- downVals[i] = reflect.ValueOf(val)
- }
- }
- upVals := b.Upstream.Call(downVals)
- up := make([]interface{}, len(upVals))
- for i, upVal := range upVals {
- up[i] = upVal.Interface()
- }
-
- return up
-}
-
-// ConvertDown calls the Downstream function on the elements of the up array
-// and returns the results.
-func (b *BiMapper) ConvertDown(up []interface{}) []interface{} {
- if len(up) != len(b.UpTypes) {
- panic(fmt.Sprintf("Expected %d values != %d", len(b.UpTypes), len(up)))
- }
- upVals := make([]reflect.Value, len(b.UpTypes))
- for i, val := range up {
- if val == nil {
- upVals[i] = reflect.Zero(b.UpTypes[i])
- } else {
- upVals[i] = reflect.ValueOf(val)
- }
- }
- downVals := b.Downstream.Call(upVals)
- down := make([]interface{}, len(downVals))
- for i, downVal := range downVals {
- down[i] = downVal.Interface()
- }
-
- return down
-}
diff --git a/vendor/github.com/leanovate/gopter/derived_gen.go b/vendor/github.com/leanovate/gopter/derived_gen.go
deleted file mode 100644
index 74933b40..00000000
--- a/vendor/github.com/leanovate/gopter/derived_gen.go
+++ /dev/null
@@ -1,114 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "reflect"
-)
-
-type derivedGen struct {
- biMapper *BiMapper
- upGens []Gen
- resultType reflect.Type
-}
-
-func (d *derivedGen) Generate(genParams *GenParameters) *GenResult {
- labels := []string{}
- up := make([]interface{}, len(d.upGens))
- shrinkers := make([]Shrinker, len(d.upGens))
- sieves := make([]func(v interface{}) bool, len(d.upGens))
-
- var ok bool
- for i, gen := range d.upGens {
- result := gen(genParams)
- labels = append(labels, result.Labels...)
- shrinkers[i] = result.Shrinker
- sieves[i] = result.Sieve
- up[i], ok = result.Retrieve()
- if !ok {
- return &GenResult{
- Shrinker: d.Shrinker(result.Shrinker),
- Result: nil,
- Labels: result.Labels,
- ResultType: d.resultType,
- Sieve: d.Sieve(sieves...),
- }
- }
- }
- down := d.biMapper.ConvertDown(up)
- if len(down) == 1 {
- return &GenResult{
- Shrinker: d.Shrinker(CombineShrinker(shrinkers...)),
- Result: down[0],
- Labels: labels,
- ResultType: reflect.TypeOf(down[0]),
- Sieve: d.Sieve(sieves...),
- }
- }
- return &GenResult{
- Shrinker: d.Shrinker(CombineShrinker(shrinkers...)),
- Result: down,
- Labels: labels,
- ResultType: reflect.TypeOf(down),
- Sieve: d.Sieve(sieves...),
- }
-}
-
-func (d *derivedGen) Sieve(baseSieve ...func(interface{}) bool) func(interface{}) bool {
- return func(down interface{}) bool {
- if down == nil {
- return false
- }
- downs, ok := down.([]interface{})
- if !ok {
- downs = []interface{}{down}
- }
- ups := d.biMapper.ConvertUp(downs)
- for i, up := range ups {
- if baseSieve[i] != nil && !baseSieve[i](up) {
- return false
- }
- }
- return true
- }
-}
-
-func (d *derivedGen) Shrinker(baseShrinker Shrinker) func(down interface{}) Shrink {
- return func(down interface{}) Shrink {
- downs, ok := down.([]interface{})
- if !ok {
- downs = []interface{}{down}
- }
- ups := d.biMapper.ConvertUp(downs)
- upShrink := baseShrinker(ups)
-
- return upShrink.Map(func(shrunkUps []interface{}) interface{} {
- downs := d.biMapper.ConvertDown(shrunkUps)
- if len(downs) == 1 {
- return downs[0]
- }
- return downs
- })
- }
-}
-
-// DeriveGen derives a generator with shrinkers from a sequence of other
-// generators mapped by a bijective function (BiMapper)
-func DeriveGen(downstream interface{}, upstream interface{}, gens ...Gen) Gen {
- biMapper := NewBiMapper(downstream, upstream)
-
- if len(gens) != len(biMapper.UpTypes) {
- panic(fmt.Sprintf("Expected %d generators != %d", len(biMapper.UpTypes), len(gens)))
- }
-
- resultType := reflect.TypeOf([]interface{}{})
- if len(biMapper.DownTypes) == 1 {
- resultType = biMapper.DownTypes[0]
- }
-
- derived := &derivedGen{
- biMapper: biMapper,
- upGens: gens,
- resultType: resultType,
- }
- return derived.Generate
-}
diff --git a/vendor/github.com/leanovate/gopter/doc.go b/vendor/github.com/leanovate/gopter/doc.go
deleted file mode 100644
index cbb5f973..00000000
--- a/vendor/github.com/leanovate/gopter/doc.go
+++ /dev/null
@@ -1,35 +0,0 @@
-/*
-Package gopter contain the main interfaces of the GOlang Property TestER.
-
-A simple property test might look like this:
-
- func TestSqrt(t *testing.T) {
- properties := gopter.NewProperties(nil)
-
- properties.Property("greater one of all greater one", prop.ForAll(
- func(v float64) bool {
- return math.Sqrt(v) >= 1
- },
- gen.Float64Range(1, math.MaxFloat64),
- ))
-
- properties.Property("squared is equal to value", prop.ForAll(
- func(v float64) bool {
- r := math.Sqrt(v)
- return math.Abs(r*r-v) < 1e-10*v
- },
- gen.Float64Range(0, math.MaxFloat64),
- ))
-
- properties.TestingRun(t)
- }
-
-Generally a property is just a function that takes GenParameters and produces
-a PropResult:
-
- type Prop func(*GenParameters) *PropResult
-
-but usually you will use prop.ForAll, prop.ForAllNoShrink or arbitrary.ForAll.
-There is also the commands package, which can be helpful for stateful testing.
-*/
-package gopter
diff --git a/vendor/github.com/leanovate/gopter/flag.go b/vendor/github.com/leanovate/gopter/flag.go
deleted file mode 100644
index b32b5743..00000000
--- a/vendor/github.com/leanovate/gopter/flag.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package gopter
-
-import "sync/atomic"
-
-// Flag is a convenient helper for an atomic boolean
-type Flag struct {
- flag int32
-}
-
-// Get the value of the flag
-func (f *Flag) Get() bool {
- return atomic.LoadInt32(&f.flag) > 0
-}
-
-// Set the the flag
-func (f *Flag) Set() {
- atomic.StoreInt32(&f.flag, 1)
-}
-
-// Unset the flag
-func (f *Flag) Unset() {
- atomic.StoreInt32(&f.flag, 0)
-}
diff --git a/vendor/github.com/leanovate/gopter/formated_reporter.go b/vendor/github.com/leanovate/gopter/formated_reporter.go
deleted file mode 100644
index 280708f3..00000000
--- a/vendor/github.com/leanovate/gopter/formated_reporter.go
+++ /dev/null
@@ -1,144 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "io"
- "os"
- "strings"
- "unicode"
-)
-
-const newLine = "\n"
-
-// FormatedReporter reports test results in a human readable manager.
-type FormatedReporter struct {
- verbose bool
- width int
- output io.Writer
-}
-
-// NewFormatedReporter create a new formated reporter
-// verbose toggles verbose output of the property results
-// width is the maximal width per line
-// output is the writer were the report will be written to
-func NewFormatedReporter(verbose bool, width int, output io.Writer) Reporter {
- return &FormatedReporter{
- verbose: verbose,
- width: width,
- output: output,
- }
-}
-
-// ConsoleReporter creates a FormatedReporter writing to the console (i.e. stdout)
-func ConsoleReporter(verbose bool) Reporter {
- return NewFormatedReporter(verbose, 75, os.Stdout)
-}
-
-// ReportTestResult reports a single property result
-func (r *FormatedReporter) ReportTestResult(propName string, result *TestResult) {
- if result.Passed() {
- fmt.Fprintln(r.output, r.formatLines(fmt.Sprintf("+ %s: %s", propName, r.reportResult(result)), "", ""))
- } else {
- fmt.Fprintln(r.output, r.formatLines(fmt.Sprintf("! %s: %s", propName, r.reportResult(result)), "", ""))
- }
-}
-
-func (r *FormatedReporter) reportResult(result *TestResult) string {
- status := ""
- switch result.Status {
- case TestProved:
- status = "OK, proved property.\n" + r.reportPropArgs(result.Args)
- case TestPassed:
- status = fmt.Sprintf("OK, passed %d tests.", result.Succeeded)
- case TestFailed:
- status = fmt.Sprintf("Falsified after %d passed tests.\n%s%s", result.Succeeded, r.reportLabels(result.Labels), r.reportPropArgs(result.Args))
- case TestExhausted:
- status = fmt.Sprintf("Gave up after only %d passed tests. %d tests were discarded.", result.Succeeded, result.Discarded)
- case TestError:
- if r.verbose {
- status = fmt.Sprintf("Error on property evaluation after %d passed tests: %s\n%s\n%s", result.Succeeded, result.Error.Error(), result.ErrorStack, r.reportPropArgs(result.Args))
- } else {
- status = fmt.Sprintf("Error on property evaluation after %d passed tests: %s\n%s", result.Succeeded, result.Error.Error(), r.reportPropArgs(result.Args))
- }
- }
-
- if r.verbose {
- return concatLines(status, fmt.Sprintf("Elapsed time: %s", result.Time.String()))
- }
- return status
-}
-
-func (r *FormatedReporter) reportLabels(labels []string) string {
- if labels != nil && len(labels) > 0 {
- return fmt.Sprintf("> Labels of failing property: %s\n", strings.Join(labels, newLine))
- }
- return ""
-}
-
-func (r *FormatedReporter) reportPropArgs(p PropArgs) string {
- result := ""
- for i, arg := range p {
- if result != "" {
- result += newLine
- }
- result += r.reportPropArg(i, arg)
- }
- return result
-}
-
-func (r *FormatedReporter) reportPropArg(idx int, propArg *PropArg) string {
- label := propArg.Label
- if label == "" {
- label = fmt.Sprintf("ARG_%d", idx)
- }
- result := fmt.Sprintf("%s: %s", label, propArg.ArgFormatted)
- if propArg.Shrinks > 0 {
- result += fmt.Sprintf("\n%s_ORIGINAL (%d shrinks): %s", label, propArg.Shrinks, propArg.OrigArgFormatted)
- }
-
- return result
-}
-
-func (r *FormatedReporter) formatLines(str, lead, trail string) string {
- result := ""
- for _, line := range strings.Split(str, "\n") {
- if result != "" {
- result += newLine
- }
- result += r.breakLine(lead+line+trail, " ")
- }
- return result
-}
-
-func (r *FormatedReporter) breakLine(str, lead string) string {
- if len(str) <= r.width {
- return str
- }
-
- result := ""
- for len(str) > r.width {
- idx := strings.LastIndexFunc(str[0:r.width], func(ch rune) bool {
- return unicode.IsSpace(ch)
- })
- if idx <= 0 {
- idx = r.width
- }
- result += str[0:idx] + "\n" + lead
- str = str[idx:]
- }
- result += str
- return result
-}
-
-func concatLines(strs ...string) string {
- result := ""
- for _, str := range strs {
- if str != "" {
- if result != "" {
- result += "\n"
- }
- result += str
- }
- }
- return result
-}
diff --git a/vendor/github.com/leanovate/gopter/gen.go b/vendor/github.com/leanovate/gopter/gen.go
deleted file mode 100644
index 6d292efb..00000000
--- a/vendor/github.com/leanovate/gopter/gen.go
+++ /dev/null
@@ -1,260 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "reflect"
-)
-
-// Gen generator of arbitrary values.
-// Usually properties are checked by verifing a condition holds true for
-// arbitrary input parameters generated by a Gen.
-//
-// IMPORTANT: Even though a generator is supposed to generate random values, it
-// should do this in a reproducible way. Therefore a generator has to create the
-// same result for the same GenParameters, i.e. ensure that you just use the
-// RNG provided by GenParameters and no external one.
-// If you just plug generators together you do not have to worry about this.
-type Gen func(*GenParameters) *GenResult
-
-var (
- // DefaultGenParams can be used as default für *GenParameters
- DefaultGenParams = DefaultGenParameters()
- MinGenParams = MinGenParameters()
-)
-
-// Sample generate a sample value.
-// Depending on the state of the RNG the generate might fail to provide a sample
-func (g Gen) Sample() (interface{}, bool) {
- return g(DefaultGenParameters()).Retrieve()
-}
-
-// WithLabel adds a label to a generated value.
-// Labels are usually used for reporting for the arguments of a property check.
-func (g Gen) WithLabel(label string) Gen {
- return func(genParams *GenParameters) *GenResult {
- result := g(genParams)
- result.Labels = append(result.Labels, label)
- return result
- }
-}
-
-// SuchThat creates a derived generator by adding a sieve.
-// f: has to be a function with one parameter (matching the generated value) returning a bool.
-// All generated values are expected to satisfy
-// f(value) == true.
-// Use this care, if the sieve to to fine the generator will have many misses which results
-// in an undecided property.
-func (g Gen) SuchThat(f interface{}) Gen {
- checkVal := reflect.ValueOf(f)
- checkType := checkVal.Type()
-
- if checkVal.Kind() != reflect.Func {
- panic(fmt.Sprintf("Param of SuchThat has to be a func, but is %v", checkType.Kind()))
- }
- if checkType.NumIn() != 1 {
- panic(fmt.Sprintf("Param of SuchThat has to be a func with one param, but is %v", checkType.NumIn()))
- } else {
- genResultType := g(MinGenParams).ResultType
- if !genResultType.AssignableTo(checkType.In(0)) {
- panic(fmt.Sprintf("Param of SuchThat has to be a func with one param assignable to %v, but is %v", genResultType, checkType.In(0)))
- }
- }
- if checkType.NumOut() != 1 {
- panic(fmt.Sprintf("Param of SuchThat has to be a func with one return value, but is %v", checkType.NumOut()))
- } else if checkType.Out(0).Kind() != reflect.Bool {
- panic(fmt.Sprintf("Param of SuchThat has to be a func with one return value of bool, but is %v", checkType.Out(0).Kind()))
- }
- sieve := func(v interface{}) bool {
- valueOf := reflect.ValueOf(v)
- if !valueOf.IsValid() {
- return false
- }
- return checkVal.Call([]reflect.Value{valueOf})[0].Bool()
- }
-
- return func(genParams *GenParameters) *GenResult {
- result := g(genParams)
- prevSieve := result.Sieve
- if prevSieve == nil {
- result.Sieve = sieve
- } else {
- result.Sieve = func(value interface{}) bool {
- return prevSieve(value) && sieve(value)
- }
- }
- return result
- }
-}
-
-// WithShrinker creates a derived generator with a specific shrinker
-func (g Gen) WithShrinker(shrinker Shrinker) Gen {
- return func(genParams *GenParameters) *GenResult {
- result := g(genParams)
- if shrinker == nil {
- result.Shrinker = NoShrinker
- } else {
- result.Shrinker = shrinker
- }
- return result
- }
-}
-
-// Map creates a derived generator by mapping all generatored values with a given function.
-// f: has to be a function with one parameter (matching the generated value) and a single return.
-// Note: The derived generator will not have a sieve or shrinker unless you are mapping to the same type
-// Note: The mapping function may have a second parameter "*GenParameters"
-// Note: The first parameter of the mapping function and its return may be a *GenResult (this makes MapResult obsolete)
-func (g Gen) Map(f interface{}) Gen {
- mapperVal := reflect.ValueOf(f)
- mapperType := mapperVal.Type()
- needsGenParameters := false
- genResultInput := false
- genResultOutput := false
-
- if mapperVal.Kind() != reflect.Func {
- panic(fmt.Sprintf("Param of Map has to be a func, but is %v", mapperType.Kind()))
- }
- if mapperType.NumIn() != 1 && mapperType.NumIn() != 2 {
- panic(fmt.Sprintf("Param of Map has to be a func with one or two params, but is %v", mapperType.NumIn()))
- } else {
- if mapperType.NumIn() == 2 {
- if !reflect.TypeOf(&GenParameters{}).AssignableTo(mapperType.In(1)) {
- panic("Second parameter of mapper function has to be a *GenParameters")
- }
- needsGenParameters = true
- }
- genResultType := g(MinGenParams).ResultType
- if reflect.TypeOf(&GenResult{}).AssignableTo(mapperType.In(0)) {
- genResultInput = true
- } else if !genResultType.AssignableTo(mapperType.In(0)) {
- panic(fmt.Sprintf("Param of Map has to be a func with one param assignable to %v, but is %v", genResultType, mapperType.In(0)))
- }
- }
- if mapperType.NumOut() != 1 {
- panic(fmt.Sprintf("Param of Map has to be a func with one return value, but is %v", mapperType.NumOut()))
- } else if reflect.TypeOf(&GenResult{}).AssignableTo(mapperType.Out(0)) {
- genResultOutput = true
- }
-
- return func(genParams *GenParameters) *GenResult {
- result := g(genParams)
- if genResultInput {
- var mapped reflect.Value
- if needsGenParameters {
- mapped = mapperVal.Call([]reflect.Value{reflect.ValueOf(result), reflect.ValueOf(genParams)})[0]
- } else {
- mapped = mapperVal.Call([]reflect.Value{reflect.ValueOf(result)})[0]
- }
- if genResultOutput {
- return mapped.Interface().(*GenResult)
- }
- return &GenResult{
- Shrinker: NoShrinker,
- Result: mapped.Interface(),
- Labels: result.Labels,
- ResultType: mapperType.Out(0),
- }
- }
- value, ok := result.RetrieveAsValue()
- if ok {
- var mapped reflect.Value
- shrinker := NoShrinker
- if needsGenParameters {
- mapped = mapperVal.Call([]reflect.Value{value, reflect.ValueOf(genParams)})[0]
- } else {
- mapped = mapperVal.Call([]reflect.Value{value})[0]
- }
- if genResultOutput {
- return mapped.Interface().(*GenResult)
- }
- if mapperType.In(0) == mapperType.Out(0) {
- shrinker = result.Shrinker
- }
- return &GenResult{
- Shrinker: shrinker,
- Result: mapped.Interface(),
- Labels: result.Labels,
- ResultType: mapperType.Out(0),
- }
- }
- return &GenResult{
- Shrinker: NoShrinker,
- Result: nil,
- Labels: result.Labels,
- ResultType: mapperType.Out(0),
- }
- }
-}
-
-// FlatMap creates a derived generator by passing a generated value to a function which itself
-// creates a generator.
-func (g Gen) FlatMap(f func(interface{}) Gen, resultType reflect.Type) Gen {
- return func(genParams *GenParameters) *GenResult {
- result := g(genParams)
- value, ok := result.Retrieve()
- if ok {
- return f(value)(genParams)
- }
- return &GenResult{
- Shrinker: NoShrinker,
- Result: nil,
- Labels: result.Labels,
- ResultType: resultType,
- }
- }
-}
-
-// MapResult creates a derived generator by mapping the GenResult directly.
-// Contrary to `Map` and `FlatMap` this also allow the conversion of
-// shrinkers and sieves, but implementation is more cumbersome.
-// Deprecation note: Map now has the same functionality
-func (g Gen) MapResult(f func(*GenResult) *GenResult) Gen {
- return func(genParams *GenParameters) *GenResult {
- return f(g(genParams))
- }
-}
-
-// CombineGens creates a generators from a list of generators.
-// The result type will be a []interface{} containing the generated values of each generators in
-// the list.
-// Note: The combined generator will not have a sieve or shrinker.
-func CombineGens(gens ...Gen) Gen {
- return func(genParams *GenParameters) *GenResult {
- labels := []string{}
- values := make([]interface{}, len(gens))
- shrinkers := make([]Shrinker, len(gens))
- sieves := make([]func(v interface{}) bool, len(gens))
-
- var ok bool
- for i, gen := range gens {
- result := gen(genParams)
- labels = append(labels, result.Labels...)
- shrinkers[i] = result.Shrinker
- sieves[i] = result.Sieve
- values[i], ok = result.Retrieve()
- if !ok {
- return &GenResult{
- Shrinker: NoShrinker,
- Result: nil,
- Labels: result.Labels,
- ResultType: reflect.TypeOf(values),
- }
- }
- }
- return &GenResult{
- Shrinker: CombineShrinker(shrinkers...),
- Result: values,
- Labels: labels,
- ResultType: reflect.TypeOf(values),
- Sieve: func(v interface{}) bool {
- values := v.([]interface{})
- for i, value := range values {
- if sieves[i] != nil && !sieves[i](value) {
- return false
- }
- }
- return true
- },
- }
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/bool.go b/vendor/github.com/leanovate/gopter/gen/bool.go
deleted file mode 100644
index 019f93bc..00000000
--- a/vendor/github.com/leanovate/gopter/gen/bool.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package gen
-
-import "github.com/leanovate/gopter"
-
-// Bool generates an arbitrary bool value
-func Bool() gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- return gopter.NewGenResult(genParams.NextBool(), gopter.NoShrinker)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/complex.go b/vendor/github.com/leanovate/gopter/gen/complex.go
deleted file mode 100644
index 2bc57a08..00000000
--- a/vendor/github.com/leanovate/gopter/gen/complex.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package gen
-
-import "github.com/leanovate/gopter"
-
-// Complex128Box generate complex128 numbers within a rectangle/box in the complex plane
-func Complex128Box(min, max complex128) gopter.Gen {
- return gopter.CombineGens(
- Float64Range(real(min), real(max)),
- Float64Range(imag(min), imag(max)),
- ).Map(func(values []interface{}) complex128 {
- return complex(values[0].(float64), values[1].(float64))
- }).SuchThat(func(v complex128) bool {
- return real(v) >= real(min) && real(v) <= real(max) &&
- imag(v) >= imag(min) && imag(v) <= imag(max)
- }).WithShrinker(Complex128Shrinker)
-}
-
-// Complex128 generate arbitrary complex128 numbers
-func Complex128() gopter.Gen {
- return gopter.CombineGens(
- Float64(),
- Float64(),
- ).Map(func(values []interface{}) complex128 {
- return complex(values[0].(float64), values[1].(float64))
- }).WithShrinker(Complex128Shrinker)
-}
-
-// Complex64Box generate complex64 numbers within a rectangle/box in the complex plane
-func Complex64Box(min, max complex64) gopter.Gen {
- return gopter.CombineGens(
- Float32Range(real(min), real(max)),
- Float32Range(imag(min), imag(max)),
- ).Map(func(values []interface{}) complex64 {
- return complex(values[0].(float32), values[1].(float32))
- }).SuchThat(func(v complex64) bool {
- return real(v) >= real(min) && real(v) <= real(max) &&
- imag(v) >= imag(min) && imag(v) <= imag(max)
- }).WithShrinker(Complex64Shrinker)
-}
-
-// Complex64 generate arbitrary complex64 numbers
-func Complex64() gopter.Gen {
- return gopter.CombineGens(
- Float32(),
- Float32(),
- ).Map(func(values []interface{}) complex64 {
- return complex(values[0].(float32), values[1].(float32))
- }).WithShrinker(Complex64Shrinker)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/complex_shrink.go b/vendor/github.com/leanovate/gopter/gen/complex_shrink.go
deleted file mode 100644
index 68ec8bdc..00000000
--- a/vendor/github.com/leanovate/gopter/gen/complex_shrink.go
+++ /dev/null
@@ -1,27 +0,0 @@
-package gen
-
-import "github.com/leanovate/gopter"
-
-// Complex128Shrinker is a shrinker for complex128 numbers
-func Complex128Shrinker(v interface{}) gopter.Shrink {
- c := v.(complex128)
- realShrink := Float64Shrinker(real(c)).Map(func(r float64) complex128 {
- return complex(r, imag(c))
- })
- imagShrink := Float64Shrinker(imag(c)).Map(func(i float64) complex128 {
- return complex(real(c), i)
- })
- return realShrink.Interleave(imagShrink)
-}
-
-// Complex64Shrinker is a shrinker for complex64 numbers
-func Complex64Shrinker(v interface{}) gopter.Shrink {
- c := v.(complex64)
- realShrink := Float64Shrinker(float64(real(c))).Map(func(r float64) complex64 {
- return complex(float32(r), imag(c))
- })
- imagShrink := Float64Shrinker(float64(imag(c))).Map(func(i float64) complex64 {
- return complex(real(c), float32(i))
- })
- return realShrink.Interleave(imagShrink)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/const.go b/vendor/github.com/leanovate/gopter/gen/const.go
deleted file mode 100644
index c213e167..00000000
--- a/vendor/github.com/leanovate/gopter/gen/const.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package gen
-
-import "github.com/leanovate/gopter"
-
-// Const creates a generator for a constant value
-// Not the most exciting generator, but can be helpful from time to time
-func Const(value interface{}) gopter.Gen {
- return func(*gopter.GenParameters) *gopter.GenResult {
- return gopter.NewGenResult(value, gopter.NoShrinker)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/doc.go b/vendor/github.com/leanovate/gopter/gen/doc.go
deleted file mode 100644
index 48592392..00000000
--- a/vendor/github.com/leanovate/gopter/gen/doc.go
+++ /dev/null
@@ -1,4 +0,0 @@
-/*
-Package gen contains all commonly used generators and shrinkers.
-*/
-package gen
diff --git a/vendor/github.com/leanovate/gopter/gen/fail.go b/vendor/github.com/leanovate/gopter/gen/fail.go
deleted file mode 100644
index 9d0efc7e..00000000
--- a/vendor/github.com/leanovate/gopter/gen/fail.go
+++ /dev/null
@@ -1,15 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// Fail is a generator that always fails to generate a value
-// Useful as fallback
-func Fail(resultType reflect.Type) gopter.Gen {
- return func(*gopter.GenParameters) *gopter.GenResult {
- return gopter.NewEmptyResult(resultType)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/floats.go b/vendor/github.com/leanovate/gopter/gen/floats.go
deleted file mode 100644
index 901cac60..00000000
--- a/vendor/github.com/leanovate/gopter/gen/floats.go
+++ /dev/null
@@ -1,69 +0,0 @@
-package gen
-
-import (
- "math"
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// Float64Range generates float64 numbers within a given range
-func Float64Range(min, max float64) gopter.Gen {
- d := max - min
- if d < 0 || d > math.MaxFloat64 {
- return Fail(reflect.TypeOf(float64(0)))
- }
-
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- genResult := gopter.NewGenResult(min+genParams.Rng.Float64()*d, Float64Shrinker)
- genResult.Sieve = func(v interface{}) bool {
- return v.(float64) >= min && v.(float64) <= max
- }
- return genResult
- }
-}
-
-// Float64 generates arbitrary float64 numbers that do not contain NaN or Inf
-func Float64() gopter.Gen {
- return gopter.CombineGens(
- Int64Range(0, 1),
- Int64Range(0, 0x7fe),
- Int64Range(0, 0xfffffffffffff),
- ).Map(func(values []interface{}) float64 {
- sign := uint64(values[0].(int64))
- exponent := uint64(values[1].(int64))
- mantissa := uint64(values[2].(int64))
-
- return math.Float64frombits((sign << 63) | (exponent << 52) | mantissa)
- }).WithShrinker(Float64Shrinker)
-}
-
-// Float32Range generates float32 numbers within a given range
-func Float32Range(min, max float32) gopter.Gen {
- d := max - min
- if d < 0 || d > math.MaxFloat32 {
- return Fail(reflect.TypeOf(float32(0)))
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- genResult := gopter.NewGenResult(min+genParams.Rng.Float32()*d, Float32Shrinker)
- genResult.Sieve = func(v interface{}) bool {
- return v.(float32) >= min && v.(float32) <= max
- }
- return genResult
- }
-}
-
-// Float32 generates arbitrary float32 numbers that do not contain NaN or Inf
-func Float32() gopter.Gen {
- return gopter.CombineGens(
- Int32Range(0, 1),
- Int32Range(0, 0xfe),
- Int32Range(0, 0x7fffff),
- ).Map(func(values []interface{}) float32 {
- sign := uint32(values[0].(int32))
- exponent := uint32(values[1].(int32))
- mantissa := uint32(values[2].(int32))
-
- return math.Float32frombits((sign << 31) | (exponent << 23) | mantissa)
- }).WithShrinker(Float32Shrinker)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/floats_shrink.go b/vendor/github.com/leanovate/gopter/gen/floats_shrink.go
deleted file mode 100644
index 00cd540e..00000000
--- a/vendor/github.com/leanovate/gopter/gen/floats_shrink.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package gen
-
-import (
- "math"
-
- "github.com/leanovate/gopter"
-)
-
-type float64Shrink struct {
- original float64
- half float64
-}
-
-func (s *float64Shrink) isZeroOrVeryClose() bool {
- if s.half == 0 {
- return true
- }
- muliple := s.half * 100000
- return math.Abs(muliple) < 1 && muliple != 0
-}
-
-func (s *float64Shrink) Next() (interface{}, bool) {
- if s.isZeroOrVeryClose() {
- return nil, false
- }
- value := s.original - s.half
- s.half /= 2
- return value, true
-}
-
-// Float64Shrinker is a shrinker for float64 numbers
-func Float64Shrinker(v interface{}) gopter.Shrink {
- negShrink := float64Shrink{
- original: -v.(float64),
- half: -v.(float64),
- }
- posShrink := float64Shrink{
- original: v.(float64),
- half: v.(float64) / 2,
- }
- return gopter.Shrink(negShrink.Next).Interleave(gopter.Shrink(posShrink.Next))
-}
-
-// Float32Shrinker is a shrinker for float32 numbers
-func Float32Shrinker(v interface{}) gopter.Shrink {
- return Float64Shrinker(float64(v.(float32))).Map(func(e float64) float32 {
- return float32(e)
- })
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/frequency.go b/vendor/github.com/leanovate/gopter/gen/frequency.go
deleted file mode 100644
index 5f5c7075..00000000
--- a/vendor/github.com/leanovate/gopter/gen/frequency.go
+++ /dev/null
@@ -1,33 +0,0 @@
-package gen
-
-import (
- "sort"
-
- "github.com/leanovate/gopter"
-)
-
-// Frequency combines multiple weighted generators of the the same result type
-// The generators from weightedGens will be used accrding to the weight, i.e. generators
-// with a hight weight will be used more often than generators with a low weight.
-func Frequency(weightedGens map[int]gopter.Gen) gopter.Gen {
- if len(weightedGens) == 0 {
- return Fail(nil)
- }
- weights := make(sort.IntSlice, 0, len(weightedGens))
- max := 0
- for weight := range weightedGens {
- if weight > max {
- max = weight
- }
- weights = append(weights, weight)
- }
- weights.Sort()
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- idx := weights.Search(genParams.Rng.Intn(max + 1))
- gen := weightedGens[weights[idx]]
-
- result := gen(genParams)
- result.Sieve = nil
- return result
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/integers.go b/vendor/github.com/leanovate/gopter/gen/integers.go
deleted file mode 100644
index 518530ab..00000000
--- a/vendor/github.com/leanovate/gopter/gen/integers.go
+++ /dev/null
@@ -1,225 +0,0 @@
-package gen
-
-import (
- "math"
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// Int64Range generates int64 numbers within a given range
-func Int64Range(min, max int64) gopter.Gen {
- if max < min {
- return Fail(reflect.TypeOf(int64(0)))
- }
- if max == math.MaxInt64 && min == math.MinInt64 { // Check for range overflow
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- return gopter.NewGenResult(genParams.NextInt64(), Int64Shrinker)
- }
- }
-
- rangeSize := uint64(max - min + 1)
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- var nextResult = uint64(min) + (genParams.NextUint64() % rangeSize)
- genResult := gopter.NewGenResult(int64(nextResult), Int64Shrinker)
- genResult.Sieve = func(v interface{}) bool {
- return v.(int64) >= min && v.(int64) <= max
- }
- return genResult
- }
-}
-
-// UInt64Range generates uint64 numbers within a given range
-func UInt64Range(min, max uint64) gopter.Gen {
- if max < min {
- return Fail(reflect.TypeOf(uint64(0)))
- }
- d := max - min + 1
- if d == 0 { // Check overflow (i.e. max = MaxInt64, min = MinInt64)
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- return gopter.NewGenResult(genParams.NextUint64(), UInt64Shrinker)
- }
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- genResult := gopter.NewGenResult(min+genParams.NextUint64()%d, UInt64Shrinker)
- genResult.Sieve = func(v interface{}) bool {
- return v.(uint64) >= min && v.(uint64) <= max
- }
- return genResult
- }
-}
-
-// Int64 generates an arbitrary int64 number
-func Int64() gopter.Gen {
- return Int64Range(math.MinInt64, math.MaxInt64)
-}
-
-// UInt64 generates an arbitrary Uint64 number
-func UInt64() gopter.Gen {
- return UInt64Range(0, math.MaxUint64)
-}
-
-// Int32Range generates int32 numbers within a given range
-func Int32Range(min, max int32) gopter.Gen {
- return Int64Range(int64(min), int64(max)).
- Map(int64To32).
- WithShrinker(Int32Shrinker).
- SuchThat(func(v int32) bool {
- return v >= min && v <= max
- })
-}
-
-// UInt32Range generates uint32 numbers within a given range
-func UInt32Range(min, max uint32) gopter.Gen {
- return UInt64Range(uint64(min), uint64(max)).
- Map(uint64To32).
- WithShrinker(UInt32Shrinker).
- SuchThat(func(v uint32) bool {
- return v >= min && v <= max
- })
-}
-
-// Int32 generate arbitrary int32 numbers
-func Int32() gopter.Gen {
- return Int32Range(math.MinInt32, math.MaxInt32)
-}
-
-// UInt32 generate arbitrary int32 numbers
-func UInt32() gopter.Gen {
- return UInt32Range(0, math.MaxUint32)
-}
-
-// Int16Range generates int16 numbers within a given range
-func Int16Range(min, max int16) gopter.Gen {
- return Int64Range(int64(min), int64(max)).
- Map(int64To16).
- WithShrinker(Int16Shrinker).
- SuchThat(func(v int16) bool {
- return v >= min && v <= max
- })
-}
-
-// UInt16Range generates uint16 numbers within a given range
-func UInt16Range(min, max uint16) gopter.Gen {
- return UInt64Range(uint64(min), uint64(max)).
- Map(uint64To16).
- WithShrinker(UInt16Shrinker).
- SuchThat(func(v uint16) bool {
- return v >= min && v <= max
- })
-}
-
-// Int16 generate arbitrary int16 numbers
-func Int16() gopter.Gen {
- return Int16Range(math.MinInt16, math.MaxInt16)
-}
-
-// UInt16 generate arbitrary uint16 numbers
-func UInt16() gopter.Gen {
- return UInt16Range(0, math.MaxUint16)
-}
-
-// Int8Range generates int8 numbers within a given range
-func Int8Range(min, max int8) gopter.Gen {
- return Int64Range(int64(min), int64(max)).
- Map(int64To8).
- WithShrinker(Int8Shrinker).
- SuchThat(func(v int8) bool {
- return v >= min && v <= max
- })
-}
-
-// UInt8Range generates uint8 numbers within a given range
-func UInt8Range(min, max uint8) gopter.Gen {
- return UInt64Range(uint64(min), uint64(max)).
- Map(uint64To8).
- WithShrinker(UInt8Shrinker).
- SuchThat(func(v uint8) bool {
- return v >= min && v <= max
- })
-}
-
-// Int8 generate arbitrary int8 numbers
-func Int8() gopter.Gen {
- return Int8Range(math.MinInt8, math.MaxInt8)
-}
-
-// UInt8 generate arbitrary uint8 numbers
-func UInt8() gopter.Gen {
- return UInt8Range(0, math.MaxUint8)
-}
-
-// IntRange generates int numbers within a given range
-func IntRange(min, max int) gopter.Gen {
- return Int64Range(int64(min), int64(max)).
- Map(int64ToInt).
- WithShrinker(IntShrinker).
- SuchThat(func(v int) bool {
- return v >= min && v <= max
- })
-}
-
-// Int generate arbitrary int numbers
-func Int() gopter.Gen {
- return Int64Range(math.MinInt32, math.MaxInt32).
- Map(int64ToInt).
- WithShrinker(IntShrinker)
-}
-
-// UIntRange generates uint numbers within a given range
-func UIntRange(min, max uint) gopter.Gen {
- return UInt64Range(uint64(min), uint64(max)).
- Map(uint64ToUint).
- WithShrinker(UIntShrinker).
- SuchThat(func(v uint) bool {
- return v >= min && v <= max
- })
-}
-
-// UInt generate arbitrary uint numbers
-func UInt() gopter.Gen {
- return UInt64Range(0, math.MaxUint32).
- Map(uint64ToUint).
- WithShrinker(UIntShrinker)
-}
-
-// Size just extracts the MaxSize field of the GenParameters.
-// This can be helpful to generate limited integer value in a more structued
-// manner.
-func Size() gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- return gopter.NewGenResult(genParams.MaxSize, IntShrinker)
- }
-}
-
-func int64To32(value int64) int32 {
- return int32(value)
-}
-
-func uint64To32(value uint64) uint32 {
- return uint32(value)
-}
-
-func int64To16(value int64) int16 {
- return int16(value)
-}
-
-func uint64To16(value uint64) uint16 {
- return uint16(value)
-}
-
-func int64To8(value int64) int8 {
- return int8(value)
-}
-
-func uint64To8(value uint64) uint8 {
- return uint8(value)
-}
-
-func int64ToInt(value int64) int {
- return int(value)
-}
-
-func uint64ToUint(value uint64) uint {
- return uint(value)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/integers_shrink.go b/vendor/github.com/leanovate/gopter/gen/integers_shrink.go
deleted file mode 100644
index ede4189a..00000000
--- a/vendor/github.com/leanovate/gopter/gen/integers_shrink.go
+++ /dev/null
@@ -1,95 +0,0 @@
-package gen
-
-import (
- "github.com/leanovate/gopter"
-)
-
-type int64Shrink struct {
- original int64
- half int64
-}
-
-func (s *int64Shrink) Next() (interface{}, bool) {
- if s.half == 0 {
- return nil, false
- }
- value := s.original - s.half
- s.half /= 2
- return value, true
-}
-
-type uint64Shrink struct {
- original uint64
- half uint64
-}
-
-func (s *uint64Shrink) Next() (interface{}, bool) {
- if s.half == 0 {
- return nil, false
- }
- value := s.original - s.half
- s.half >>= 1
- return value, true
-}
-
-// Int64Shrinker is a shrinker for int64 numbers
-func Int64Shrinker(v interface{}) gopter.Shrink {
- negShrink := int64Shrink{
- original: -v.(int64),
- half: -v.(int64),
- }
- posShrink := int64Shrink{
- original: v.(int64),
- half: v.(int64) / 2,
- }
- return gopter.Shrink(negShrink.Next).Interleave(gopter.Shrink(posShrink.Next))
-}
-
-// UInt64Shrinker is a shrinker for uint64 numbers
-func UInt64Shrinker(v interface{}) gopter.Shrink {
- shrink := uint64Shrink{
- original: v.(uint64),
- half: v.(uint64),
- }
- return shrink.Next
-}
-
-// Int32Shrinker is a shrinker for int32 numbers
-func Int32Shrinker(v interface{}) gopter.Shrink {
- return Int64Shrinker(int64(v.(int32))).Map(int64To32)
-}
-
-// UInt32Shrinker is a shrinker for uint32 numbers
-func UInt32Shrinker(v interface{}) gopter.Shrink {
- return UInt64Shrinker(uint64(v.(uint32))).Map(uint64To32)
-}
-
-// Int16Shrinker is a shrinker for int16 numbers
-func Int16Shrinker(v interface{}) gopter.Shrink {
- return Int64Shrinker(int64(v.(int16))).Map(int64To16)
-}
-
-// UInt16Shrinker is a shrinker for uint16 numbers
-func UInt16Shrinker(v interface{}) gopter.Shrink {
- return UInt64Shrinker(uint64(v.(uint16))).Map(uint64To16)
-}
-
-// Int8Shrinker is a shrinker for int8 numbers
-func Int8Shrinker(v interface{}) gopter.Shrink {
- return Int64Shrinker(int64(v.(int8))).Map(int64To8)
-}
-
-// UInt8Shrinker is a shrinker for uint8 numbers
-func UInt8Shrinker(v interface{}) gopter.Shrink {
- return UInt64Shrinker(uint64(v.(uint8))).Map(uint64To8)
-}
-
-// IntShrinker is a shrinker for int numbers
-func IntShrinker(v interface{}) gopter.Shrink {
- return Int64Shrinker(int64(v.(int))).Map(int64ToInt)
-}
-
-// UIntShrinker is a shrinker for uint numbers
-func UIntShrinker(v interface{}) gopter.Shrink {
- return UInt64Shrinker(uint64(v.(uint))).Map(uint64ToUint)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/map_of.go b/vendor/github.com/leanovate/gopter/gen/map_of.go
deleted file mode 100644
index 17a3e211..00000000
--- a/vendor/github.com/leanovate/gopter/gen/map_of.go
+++ /dev/null
@@ -1,87 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// MapOf generates an arbitrary map of generated kay values.
-// genParams.MaxSize sets an (exclusive) upper limit on the size of the map
-// genParams.MinSize sets an (inclusive) lower limit on the size of the map
-func MapOf(keyGen, elementGen gopter.Gen) gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- len := 0
- if genParams.MaxSize > 0 || genParams.MinSize > 0 {
- if genParams.MinSize > genParams.MaxSize {
- panic("GenParameters.MinSize must be <= GenParameters.MaxSize")
- }
-
- if genParams.MaxSize == genParams.MinSize {
- len = genParams.MaxSize
- } else {
- len = genParams.Rng.Intn(genParams.MaxSize-genParams.MinSize) + genParams.MinSize
- }
- }
-
- result, keySieve, keyShrinker, elementSieve, elementShrinker := genMap(keyGen, elementGen, genParams, len)
-
- genResult := gopter.NewGenResult(result.Interface(), MapShrinker(keyShrinker, elementShrinker))
- if keySieve != nil || elementSieve != nil {
- genResult.Sieve = forAllKeyValueSieve(keySieve, elementSieve)
- }
- return genResult
- }
-}
-
-func genMap(keyGen, elementGen gopter.Gen, genParams *gopter.GenParameters, len int) (reflect.Value, func(interface{}) bool, gopter.Shrinker, func(interface{}) bool, gopter.Shrinker) {
- element := elementGen(genParams)
- elementSieve := element.Sieve
- elementShrinker := element.Shrinker
-
- key := keyGen(genParams)
- keySieve := key.Sieve
- keyShrinker := key.Shrinker
-
- result := reflect.MakeMapWithSize(reflect.MapOf(key.ResultType, element.ResultType), len)
-
- for i := 0; i < len; i++ {
- keyValue, keyOk := key.Retrieve()
- elementValue, elementOk := element.Retrieve()
-
- if keyOk && elementOk {
- if key == nil {
- if elementValue == nil {
- result.SetMapIndex(reflect.Zero(key.ResultType), reflect.Zero(element.ResultType))
- } else {
- result.SetMapIndex(reflect.Zero(key.ResultType), reflect.ValueOf(elementValue))
- }
- } else {
- if elementValue == nil {
- result.SetMapIndex(reflect.ValueOf(keyValue), reflect.Zero(element.ResultType))
- } else {
- result.SetMapIndex(reflect.ValueOf(keyValue), reflect.ValueOf(elementValue))
- }
- }
- }
- key = keyGen(genParams)
- element = elementGen(genParams)
- }
-
- return result, keySieve, keyShrinker, elementSieve, elementShrinker
-}
-
-func forAllKeyValueSieve(keySieve, elementSieve func(interface{}) bool) func(interface{}) bool {
- return func(v interface{}) bool {
- rv := reflect.ValueOf(v)
- for _, key := range rv.MapKeys() {
- if keySieve != nil && !keySieve(key.Interface()) {
- return false
- }
- if elementSieve != nil && !elementSieve(rv.MapIndex(key).Interface()) {
- return false
- }
- }
- return true
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/map_shrink.go b/vendor/github.com/leanovate/gopter/gen/map_shrink.go
deleted file mode 100644
index b0787fce..00000000
--- a/vendor/github.com/leanovate/gopter/gen/map_shrink.go
+++ /dev/null
@@ -1,149 +0,0 @@
-package gen
-
-import (
- "fmt"
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-type mapShrinkOne struct {
- original reflect.Value
- key reflect.Value
- keyShrink gopter.Shrink
- elementShrink gopter.Shrink
- state bool
- keyExhausted bool
- lastKey interface{}
- elementExhausted bool
- lastElement interface{}
-}
-
-func (s *mapShrinkOne) nextKeyValue() (interface{}, interface{}, bool) {
- for !s.keyExhausted && !s.elementExhausted {
- s.state = !s.state
- if s.state && !s.keyExhausted {
- value, ok := s.keyShrink()
- if ok {
- s.lastKey = value
- return s.lastKey, s.lastElement, true
- }
- s.keyExhausted = true
- } else if !s.state && !s.elementExhausted {
- value, ok := s.elementShrink()
- if ok {
- s.lastElement = value
- return s.lastKey, s.lastElement, true
- }
- s.elementExhausted = true
- }
- }
- return nil, nil, false
-}
-
-func (s *mapShrinkOne) Next() (interface{}, bool) {
- nextKey, nextValue, ok := s.nextKeyValue()
- if !ok {
- return nil, false
- }
- result := reflect.MakeMapWithSize(s.original.Type(), s.original.Len())
- for _, key := range s.original.MapKeys() {
- if !reflect.DeepEqual(key.Interface(), s.key.Interface()) {
- result.SetMapIndex(key, s.original.MapIndex(key))
- }
- }
- result.SetMapIndex(reflect.ValueOf(nextKey), reflect.ValueOf(nextValue))
-
- return result.Interface(), true
-}
-
-// MapShrinkerOne creates a map shrinker from a shrinker for the key values of a map.
-// The length of the map will remain (mostly) unchanged, instead each key value pair is
-// shrunk after the other.
-func MapShrinkerOne(keyShrinker, elementShrinker gopter.Shrinker) gopter.Shrinker {
- return func(v interface{}) gopter.Shrink {
- rv := reflect.ValueOf(v)
- if rv.Kind() != reflect.Map {
- panic(fmt.Sprintf("%#v is not a map", v))
- }
-
- keys := rv.MapKeys()
- shrinks := make([]gopter.Shrink, 0, len(keys))
- for _, key := range keys {
- mapShrinkOne := &mapShrinkOne{
- original: rv,
- key: key,
- keyShrink: keyShrinker(key.Interface()),
- lastKey: key.Interface(),
- elementShrink: elementShrinker(rv.MapIndex(key).Interface()),
- lastElement: rv.MapIndex(key).Interface(),
- }
- shrinks = append(shrinks, mapShrinkOne.Next)
- }
- return gopter.ConcatShrinks(shrinks...)
- }
-}
-
-type mapShrink struct {
- original reflect.Value
- originalKeys []reflect.Value
- length int
- offset int
- chunkLength int
-}
-
-func (s *mapShrink) Next() (interface{}, bool) {
- if s.chunkLength == 0 {
- return nil, false
- }
- keys := make([]reflect.Value, 0, s.length-s.chunkLength)
- keys = append(keys, s.originalKeys[0:s.offset]...)
- s.offset += s.chunkLength
- if s.offset < s.length {
- keys = append(keys, s.originalKeys[s.offset:s.length]...)
- } else {
- s.offset = 0
- s.chunkLength >>= 1
- }
-
- result := reflect.MakeMapWithSize(s.original.Type(), len(keys))
- for _, key := range keys {
- result.SetMapIndex(key, s.original.MapIndex(key))
- }
-
- return result.Interface(), true
-}
-
-// MapShrinker creates a map shrinker from shrinker for the key values.
-// The length of the map will be shrunk as well
-func MapShrinker(keyShrinker, elementShrinker gopter.Shrinker) gopter.Shrinker {
- return func(v interface{}) gopter.Shrink {
- rv := reflect.ValueOf(v)
- if rv.Kind() != reflect.Map {
- panic(fmt.Sprintf("%#v is not a Map", v))
- }
- keys := rv.MapKeys()
- mapShrink := &mapShrink{
- original: rv,
- originalKeys: keys,
- offset: 0,
- length: rv.Len(),
- chunkLength: rv.Len() >> 1,
- }
-
- shrinks := make([]gopter.Shrink, 0, rv.Len()+1)
- shrinks = append(shrinks, mapShrink.Next)
- for _, key := range keys {
- mapShrinkOne := &mapShrinkOne{
- original: rv,
- key: key,
- keyShrink: keyShrinker(key.Interface()),
- lastKey: key.Interface(),
- elementShrink: elementShrinker(rv.MapIndex(key).Interface()),
- lastElement: rv.MapIndex(key).Interface(),
- }
- shrinks = append(shrinks, mapShrinkOne.Next)
- }
- return gopter.ConcatShrinks(shrinks...)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/one_of.go b/vendor/github.com/leanovate/gopter/gen/one_of.go
deleted file mode 100644
index 9cb5370d..00000000
--- a/vendor/github.com/leanovate/gopter/gen/one_of.go
+++ /dev/null
@@ -1,29 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// OneConstOf generate one of a list of constant values
-func OneConstOf(consts ...interface{}) gopter.Gen {
- if len(consts) == 0 {
- return Fail(reflect.TypeOf(nil))
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- idx := genParams.Rng.Intn(len(consts))
- return gopter.NewGenResult(consts[idx], gopter.NoShrinker)
- }
-}
-
-// OneGenOf generate one value from a a list of generators
-func OneGenOf(gens ...gopter.Gen) gopter.Gen {
- if len(gens) == 0 {
- return Fail(reflect.TypeOf(nil))
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- idx := genParams.Rng.Intn(len(gens))
- return gens[idx](genParams)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/ptr_of.go b/vendor/github.com/leanovate/gopter/gen/ptr_of.go
deleted file mode 100644
index 24d5c3d8..00000000
--- a/vendor/github.com/leanovate/gopter/gen/ptr_of.go
+++ /dev/null
@@ -1,41 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// PtrOf generates either a pointer to a generated element or a nil pointer
-func PtrOf(elementGen gopter.Gen) gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- element := elementGen(genParams)
- elementShrinker := element.Shrinker
- elementSieve := element.Sieve
- value, ok := element.Retrieve()
- if !ok || genParams.NextBool() {
- result := gopter.NewEmptyResult(reflect.PtrTo(element.ResultType))
- result.Sieve = func(v interface{}) bool {
- if elementSieve == nil {
- return true
- }
- r := reflect.ValueOf(v)
- return !r.IsValid() || r.IsNil() || elementSieve(r.Elem().Interface())
- }
- return result
- }
- // To get the right pointer type we have to create a slice with one element
- slice := reflect.MakeSlice(reflect.SliceOf(element.ResultType), 0, 1)
- slice = reflect.Append(slice, reflect.ValueOf(value))
-
- result := gopter.NewGenResult(slice.Index(0).Addr().Interface(), PtrShrinker(elementShrinker))
- result.Sieve = func(v interface{}) bool {
- if elementSieve == nil {
- return true
- }
- r := reflect.ValueOf(v)
- return !r.IsValid() || r.IsNil() || elementSieve(r.Elem().Interface())
- }
- return result
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/ptr_shrink.go b/vendor/github.com/leanovate/gopter/gen/ptr_shrink.go
deleted file mode 100644
index f3cdc402..00000000
--- a/vendor/github.com/leanovate/gopter/gen/ptr_shrink.go
+++ /dev/null
@@ -1,45 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-type nilShrink struct {
- done bool
-}
-
-func (s *nilShrink) Next() (interface{}, bool) {
- if !s.done {
- s.done = true
- return nil, true
- }
- return nil, false
-}
-
-// PtrShrinker convert a value shrinker to a pointer to value shrinker
-func PtrShrinker(elementShrinker gopter.Shrinker) gopter.Shrinker {
- return func(v interface{}) gopter.Shrink {
- if v == nil {
- return gopter.NoShrink
- }
- elem := reflect.ValueOf(v).Elem()
- if !elem.IsValid() || !elem.CanInterface() {
- return gopter.NoShrink
- }
- rt := reflect.TypeOf(v)
- elementShink := elementShrinker(reflect.ValueOf(v).Elem().Interface())
-
- nilShrink := &nilShrink{}
- return gopter.ConcatShrinks(
- nilShrink.Next,
- elementShink.Map(func(elem interface{}) interface{} {
- slice := reflect.MakeSlice(reflect.SliceOf(rt.Elem()), 0, 1)
- slice = reflect.Append(slice, reflect.ValueOf(elem))
-
- return slice.Index(0).Addr().Interface()
- }),
- )
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/regex.go b/vendor/github.com/leanovate/gopter/gen/regex.go
deleted file mode 100644
index ec27512c..00000000
--- a/vendor/github.com/leanovate/gopter/gen/regex.go
+++ /dev/null
@@ -1,78 +0,0 @@
-package gen
-
-import (
- "reflect"
- "regexp"
- "regexp/syntax"
- "strings"
-
- "github.com/leanovate/gopter"
-)
-
-// RegexMatch generates matches for a given regular expression
-// regexStr is supposed to conform to the perl regular expression syntax
-func RegexMatch(regexStr string) gopter.Gen {
- regexSyntax, err1 := syntax.Parse(regexStr, syntax.Perl)
- regex, err2 := regexp.Compile(regexStr)
- if err1 != nil || err2 != nil {
- return Fail(reflect.TypeOf(""))
- }
- return regexMatchGen(regexSyntax.Simplify()).SuchThat(func(v string) bool {
- return regex.MatchString(v)
- }).WithShrinker(StringShrinker)
-}
-
-func regexMatchGen(regex *syntax.Regexp) gopter.Gen {
- switch regex.Op {
- case syntax.OpLiteral:
- return Const(string(regex.Rune))
- case syntax.OpCharClass:
- gens := make([]gopter.Gen, 0, len(regex.Rune)/2)
- for i := 0; i+1 < len(regex.Rune); i += 2 {
- gens = append(gens, RuneRange(regex.Rune[i], regex.Rune[i+1]).Map(runeToString))
- }
- return OneGenOf(gens...)
- case syntax.OpAnyChar:
- return Rune().Map(runeToString)
- case syntax.OpAnyCharNotNL:
- return RuneNoControl().Map(runeToString)
- case syntax.OpCapture:
- return regexMatchGen(regex.Sub[0])
- case syntax.OpStar:
- elementGen := regexMatchGen(regex.Sub[0])
- return SliceOf(elementGen).Map(func(v []string) string {
- return strings.Join(v, "")
- })
- case syntax.OpPlus:
- elementGen := regexMatchGen(regex.Sub[0])
- return gopter.CombineGens(elementGen, SliceOf(elementGen)).Map(func(vs []interface{}) string {
- return vs[0].(string) + strings.Join(vs[1].([]string), "")
- })
- case syntax.OpQuest:
- elementGen := regexMatchGen(regex.Sub[0])
- return OneGenOf(Const(""), elementGen)
- case syntax.OpConcat:
- gens := make([]gopter.Gen, len(regex.Sub))
- for i, sub := range regex.Sub {
- gens[i] = regexMatchGen(sub)
- }
- return gopter.CombineGens(gens...).Map(func(v []interface{}) string {
- result := ""
- for _, str := range v {
- result += str.(string)
- }
- return result
- })
- case syntax.OpAlternate:
- gens := make([]gopter.Gen, len(regex.Sub))
- for i, sub := range regex.Sub {
- gens[i] = regexMatchGen(sub)
- }
- return OneGenOf(gens...)
- }
- return Const("")
-}
-
-func runeToString(v rune) string {
- return string(v)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/retry_until.go b/vendor/github.com/leanovate/gopter/gen/retry_until.go
deleted file mode 100644
index de9cf1dc..00000000
--- a/vendor/github.com/leanovate/gopter/gen/retry_until.go
+++ /dev/null
@@ -1,21 +0,0 @@
-package gen
-
-import "github.com/leanovate/gopter"
-
-// RetryUntil creates a generator that retries a given generator until a condition in met.
-// condition: has to be a function with one parameter (matching the generated value of gen) returning a bool.
-// Note: The new generator will only create an empty result once maxRetries is reached.
-// Depending on the hit-ratio of the condition is may result in long running tests, use with care.
-func RetryUntil(gen gopter.Gen, condition interface{}, maxRetries int) gopter.Gen {
- genWithSieve := gen.SuchThat(condition)
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- for i := 0; i < maxRetries; i++ {
- result := genWithSieve(genParams)
- if _, ok := result.Retrieve(); ok {
- return result
- }
- }
- resultType := gen(genParams).ResultType
- return gopter.NewEmptyResult(resultType)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/sized.go b/vendor/github.com/leanovate/gopter/gen/sized.go
deleted file mode 100644
index ca46c272..00000000
--- a/vendor/github.com/leanovate/gopter/gen/sized.go
+++ /dev/null
@@ -1,20 +0,0 @@
-package gen
-
-import (
- "github.com/leanovate/gopter"
-)
-
-// Sized derives a generator from based on size
-// This honors the `MinSize` and `MaxSize` of the `GenParameters` of the test suite.
-// Keep an eye on memory consumption, by default MaxSize is 100.
-func Sized(f func(int) gopter.Gen) gopter.Gen {
- return func(params *gopter.GenParameters) *gopter.GenResult {
- var size int
- if params.MaxSize == params.MinSize {
- size = params.MaxSize
- } else {
- size = params.Rng.Intn(params.MaxSize-params.MinSize) + params.MinSize
- }
- return f(size)(params)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/slice_of.go b/vendor/github.com/leanovate/gopter/gen/slice_of.go
deleted file mode 100644
index fa18ad5b..00000000
--- a/vendor/github.com/leanovate/gopter/gen/slice_of.go
+++ /dev/null
@@ -1,106 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// SliceOf generates an arbitrary slice of generated elements
-// genParams.MaxSize sets an (exclusive) upper limit on the size of the slice
-// genParams.MinSize sets an (inclusive) lower limit on the size of the slice
-func SliceOf(elementGen gopter.Gen, typeOverrides ...reflect.Type) gopter.Gen {
- var typeOverride reflect.Type
- if len(typeOverrides) > 1 {
- panic("too many type overrides specified, at most 1 may be provided.")
- } else if len(typeOverrides) == 1 {
- typeOverride = typeOverrides[0]
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- len := 0
- if genParams.MaxSize > 0 || genParams.MinSize > 0 {
- if genParams.MinSize > genParams.MaxSize {
- panic("GenParameters.MinSize must be <= GenParameters.MaxSize")
- }
-
- if genParams.MaxSize == genParams.MinSize {
- len = genParams.MaxSize
- } else {
- len = genParams.Rng.Intn(genParams.MaxSize-genParams.MinSize) + genParams.MinSize
- }
- }
- result, elementSieve, elementShrinker := genSlice(elementGen, genParams, len, typeOverride)
-
- genResult := gopter.NewGenResult(result.Interface(), SliceShrinker(elementShrinker))
- if elementSieve != nil {
- genResult.Sieve = forAllSieve(elementSieve)
- }
- return genResult
- }
-}
-
-// SliceOfN generates a slice of generated elements with definied length
-func SliceOfN(desiredlen int, elementGen gopter.Gen, typeOverrides ...reflect.Type) gopter.Gen {
- var typeOverride reflect.Type
- if len(typeOverrides) > 1 {
- panic("too many type overrides specified, at most 1 may be provided.")
- } else if len(typeOverrides) == 1 {
- typeOverride = typeOverrides[0]
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- result, elementSieve, elementShrinker := genSlice(elementGen, genParams, desiredlen, typeOverride)
-
- genResult := gopter.NewGenResult(result.Interface(), SliceShrinkerOne(elementShrinker))
- if elementSieve != nil {
- genResult.Sieve = func(v interface{}) bool {
- rv := reflect.ValueOf(v)
- return rv.Len() == desiredlen && forAllSieve(elementSieve)(v)
- }
- } else {
- genResult.Sieve = func(v interface{}) bool {
- return reflect.ValueOf(v).Len() == desiredlen
- }
- }
- return genResult
- }
-}
-
-func genSlice(elementGen gopter.Gen, genParams *gopter.GenParameters, desiredlen int, typeOverride reflect.Type) (reflect.Value, func(interface{}) bool, gopter.Shrinker) {
- element := elementGen(genParams)
- elementSieve := element.Sieve
- elementShrinker := element.Shrinker
-
- sliceType := typeOverride
- if sliceType == nil {
- sliceType = element.ResultType
- }
-
- result := reflect.MakeSlice(reflect.SliceOf(sliceType), 0, desiredlen)
-
- for i := 0; i < desiredlen; i++ {
- value, ok := element.Retrieve()
-
- if ok {
- if value == nil {
- result = reflect.Append(result, reflect.Zero(sliceType))
- } else {
- result = reflect.Append(result, reflect.ValueOf(value))
- }
- }
- element = elementGen(genParams)
- }
-
- return result, elementSieve, elementShrinker
-}
-
-func forAllSieve(elementSieve func(interface{}) bool) func(interface{}) bool {
- return func(v interface{}) bool {
- rv := reflect.ValueOf(v)
- for i := rv.Len() - 1; i >= 0; i-- {
- if !elementSieve(rv.Index(i).Interface()) {
- return false
- }
- }
- return true
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/slice_shrink.go b/vendor/github.com/leanovate/gopter/gen/slice_shrink.go
deleted file mode 100644
index 5a056e41..00000000
--- a/vendor/github.com/leanovate/gopter/gen/slice_shrink.go
+++ /dev/null
@@ -1,105 +0,0 @@
-package gen
-
-import (
- "fmt"
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-type sliceShrinkOne struct {
- original reflect.Value
- index int
- elementShrink gopter.Shrink
-}
-
-func (s *sliceShrinkOne) Next() (interface{}, bool) {
- value, ok := s.elementShrink()
- if !ok {
- return nil, false
- }
- result := reflect.MakeSlice(s.original.Type(), s.original.Len(), s.original.Len())
- reflect.Copy(result, s.original)
- if value == nil {
- result.Index(s.index).Set(reflect.Zero(s.original.Type().Elem()))
- } else {
- result.Index(s.index).Set(reflect.ValueOf(value))
- }
-
- return result.Interface(), true
-}
-
-// SliceShrinkerOne creates a slice shrinker from a shrinker for the elements of the slice.
-// The length of the slice will remains unchanged, instead each element is shrunk after the
-// other.
-func SliceShrinkerOne(elementShrinker gopter.Shrinker) gopter.Shrinker {
- return func(v interface{}) gopter.Shrink {
- rv := reflect.ValueOf(v)
- if rv.Kind() != reflect.Slice {
- panic(fmt.Sprintf("%#v is not a slice", v))
- }
-
- shrinks := make([]gopter.Shrink, 0, rv.Len())
- for i := 0; i < rv.Len(); i++ {
- sliceShrinkOne := &sliceShrinkOne{
- original: rv,
- index: i,
- elementShrink: elementShrinker(rv.Index(i).Interface()),
- }
- shrinks = append(shrinks, sliceShrinkOne.Next)
- }
- return gopter.ConcatShrinks(shrinks...)
- }
-}
-
-type sliceShrink struct {
- original reflect.Value
- length int
- offset int
- chunkLength int
-}
-
-func (s *sliceShrink) Next() (interface{}, bool) {
- if s.chunkLength == 0 {
- return nil, false
- }
- value := reflect.AppendSlice(reflect.MakeSlice(s.original.Type(), 0, s.length-s.chunkLength), s.original.Slice(0, s.offset))
- s.offset += s.chunkLength
- if s.offset < s.length {
- value = reflect.AppendSlice(value, s.original.Slice(s.offset, s.length))
- } else {
- s.offset = 0
- s.chunkLength >>= 1
- }
-
- return value.Interface(), true
-}
-
-// SliceShrinker creates a slice shrinker from a shrinker for the elements of the slice.
-// The length of the slice will be shrunk as well
-func SliceShrinker(elementShrinker gopter.Shrinker) gopter.Shrinker {
- return func(v interface{}) gopter.Shrink {
- rv := reflect.ValueOf(v)
- if rv.Kind() != reflect.Slice {
- panic(fmt.Sprintf("%#v is not a slice", v))
- }
- sliceShrink := &sliceShrink{
- original: rv,
- offset: 0,
- length: rv.Len(),
- chunkLength: rv.Len() >> 1,
- }
-
- shrinks := make([]gopter.Shrink, 0, rv.Len()+1)
- shrinks = append(shrinks, sliceShrink.Next)
- for i := 0; i < rv.Len(); i++ {
- sliceShrinkOne := &sliceShrinkOne{
- original: rv,
- index: i,
- elementShrink: elementShrinker(rv.Index(i).Interface()),
- }
- shrinks = append(shrinks, sliceShrinkOne.Next)
- }
- return gopter.ConcatShrinks(shrinks...)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/string_shrink.go b/vendor/github.com/leanovate/gopter/gen/string_shrink.go
deleted file mode 100644
index 019ee138..00000000
--- a/vendor/github.com/leanovate/gopter/gen/string_shrink.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package gen
-
-import "github.com/leanovate/gopter"
-
-var runeSliceShrinker = SliceShrinker(gopter.NoShrinker)
-
-// StringShrinker is a shrinker for strings.
-// It is very similar to a slice shrinker just that the elements themselves will not be shrunk.
-func StringShrinker(v interface{}) gopter.Shrink {
- return runeSliceShrinker([]rune(v.(string))).Map(runesToString)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/strings.go b/vendor/github.com/leanovate/gopter/gen/strings.go
deleted file mode 100644
index 79a69ef6..00000000
--- a/vendor/github.com/leanovate/gopter/gen/strings.go
+++ /dev/null
@@ -1,158 +0,0 @@
-package gen
-
-import (
- "reflect"
- "unicode"
- "unicode/utf8"
-
- "github.com/leanovate/gopter"
-)
-
-// RuneRange generates runes within a given range
-func RuneRange(min, max rune) gopter.Gen {
- return genRune(Int64Range(int64(min), int64(max)))
-}
-
-// Rune generates an arbitrary character rune
-func Rune() gopter.Gen {
- return genRune(Frequency(map[int]gopter.Gen{
- 0xD800: Int64Range(0, 0xD800),
- utf8.MaxRune - 0xDFFF: Int64Range(0xDFFF, int64(utf8.MaxRune)),
- }))
-}
-
-// RuneNoControl generates an arbitrary character rune that is not a control character
-func RuneNoControl() gopter.Gen {
- return genRune(Frequency(map[int]gopter.Gen{
- 0xD800: Int64Range(32, 0xD800),
- utf8.MaxRune - 0xDFFF: Int64Range(0xDFFF, int64(utf8.MaxRune)),
- }))
-}
-
-func genRune(int64Gen gopter.Gen) gopter.Gen {
- return int64Gen.Map(func(value int64) rune {
- return rune(value)
- }).SuchThat(func(v rune) bool {
- return utf8.ValidRune(v)
- })
-}
-
-// NumChar generates arbitrary numberic character runes
-func NumChar() gopter.Gen {
- return RuneRange('0', '9')
-}
-
-// AlphaUpperChar generates arbitrary uppercase alpha character runes
-func AlphaUpperChar() gopter.Gen {
- return RuneRange('A', 'Z')
-}
-
-// AlphaLowerChar generates arbitrary lowercase alpha character runes
-func AlphaLowerChar() gopter.Gen {
- return RuneRange('a', 'z')
-}
-
-// AlphaChar generates arbitrary character runes (upper- and lowercase)
-func AlphaChar() gopter.Gen {
- return Frequency(map[int]gopter.Gen{
- 0: AlphaUpperChar(),
- 9: AlphaLowerChar(),
- })
-}
-
-// AlphaNumChar generates arbitrary alpha-numeric character runes
-func AlphaNumChar() gopter.Gen {
- return Frequency(map[int]gopter.Gen{
- 0: NumChar(),
- 9: AlphaChar(),
- })
-}
-
-// UnicodeChar generates arbitrary character runes with a given unicode table
-func UnicodeChar(table *unicode.RangeTable) gopter.Gen {
- if table == nil || len(table.R16)+len(table.R32) == 0 {
- return Fail(reflect.TypeOf(rune('a')))
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- tableIdx := genParams.Rng.Intn(len(table.R16) + len(table.R32))
-
- var selectedRune rune
- if tableIdx < len(table.R16) {
- r := table.R16[tableIdx]
- runeOffset := uint16(genParams.Rng.Int63n(int64((r.Hi-r.Lo+1)/r.Stride))) * r.Stride
- selectedRune = rune(runeOffset + r.Lo)
- } else {
- r := table.R32[tableIdx-len(table.R16)]
- runeOffset := uint32(genParams.Rng.Int63n(int64((r.Hi-r.Lo+1)/r.Stride))) * r.Stride
- selectedRune = rune(runeOffset + r.Lo)
- }
- genResult := gopter.NewGenResult(selectedRune, gopter.NoShrinker)
- genResult.Sieve = func(v interface{}) bool {
- return unicode.Is(table, v.(rune))
- }
- return genResult
- }
-}
-
-// AnyString generates an arbitrary string
-func AnyString() gopter.Gen {
- return genString(Rune(), utf8.ValidRune)
-}
-
-// AlphaString generates an arbitrary string with letters
-func AlphaString() gopter.Gen {
- return genString(AlphaChar(), unicode.IsLetter)
-}
-
-// NumString generates an arbitrary string with digits
-func NumString() gopter.Gen {
- return genString(NumChar(), unicode.IsDigit)
-}
-
-// Identifier generates an arbitrary identifier string
-// Identitiers are supporsed to start with a lowercase letter and contain only
-// letters and digits
-func Identifier() gopter.Gen {
- return gopter.CombineGens(
- AlphaLowerChar(),
- SliceOf(AlphaNumChar()),
- ).Map(func(values []interface{}) string {
- first := values[0].(rune)
- tail := values[1].([]rune)
- result := make([]rune, 0, len(tail)+1)
- return string(append(append(result, first), tail...))
- }).SuchThat(func(str string) bool {
- if len(str) < 1 || !unicode.IsLower(([]rune(str))[0]) {
- return false
- }
- for _, ch := range str {
- if !unicode.IsLetter(ch) && !unicode.IsDigit(ch) {
- return false
- }
- }
- return true
- }).WithShrinker(StringShrinker)
-}
-
-// UnicodeString generates an arbitrary string from a given
-// unicode table.
-func UnicodeString(table *unicode.RangeTable) gopter.Gen {
- return genString(UnicodeChar(table), func(ch rune) bool {
- return unicode.Is(table, ch)
- })
-}
-
-func genString(runeGen gopter.Gen, runeSieve func(ch rune) bool) gopter.Gen {
- return SliceOf(runeGen).Map(runesToString).SuchThat(func(v string) bool {
- for _, ch := range v {
- if !runeSieve(ch) {
- return false
- }
- }
- return true
- }).WithShrinker(StringShrinker)
-}
-
-func runesToString(v []rune) string {
- return string(v)
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/struct.go b/vendor/github.com/leanovate/gopter/gen/struct.go
deleted file mode 100644
index dca8f8e5..00000000
--- a/vendor/github.com/leanovate/gopter/gen/struct.go
+++ /dev/null
@@ -1,103 +0,0 @@
-package gen
-
-import (
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-// Struct generates a given struct type.
-// rt has to be the reflect type of the struct, gens contains a map of field generators.
-// Note that the result types of the generators in gen have to match the type of the correspoinding
-// field in the struct. Also note that only public fields of a struct can be generated
-func Struct(rt reflect.Type, gens map[string]gopter.Gen) gopter.Gen {
- if rt.Kind() == reflect.Ptr {
- rt = rt.Elem()
- }
- if rt.Kind() != reflect.Struct {
- return Fail(rt)
- }
- fieldGens := []gopter.Gen{}
- fieldTypes := []reflect.Type{}
- assignable := reflect.New(rt).Elem()
- for i := 0; i < rt.NumField(); i++ {
- fieldName := rt.Field(i).Name
- if !assignable.Field(i).CanSet() {
- continue
- }
-
- gen := gens[fieldName]
- if gen != nil {
- fieldGens = append(fieldGens, gen)
- fieldTypes = append(fieldTypes, rt.Field(i).Type)
- }
- }
-
- buildStructType := reflect.FuncOf(fieldTypes, []reflect.Type{rt}, false)
- unbuildStructType := reflect.FuncOf([]reflect.Type{rt}, fieldTypes, false)
-
- buildStructFunc := reflect.MakeFunc(buildStructType, func(args []reflect.Value) []reflect.Value {
- result := reflect.New(rt)
- for i := 0; i < rt.NumField(); i++ {
- if _, ok := gens[rt.Field(i).Name]; !ok {
- continue
- }
- if !assignable.Field(i).CanSet() {
- continue
- }
- result.Elem().Field(i).Set(args[0])
- args = args[1:]
- }
- return []reflect.Value{result.Elem()}
- })
- unbuildStructFunc := reflect.MakeFunc(unbuildStructType, func(args []reflect.Value) []reflect.Value {
- s := args[0]
- results := []reflect.Value{}
- for i := 0; i < s.NumField(); i++ {
- if _, ok := gens[rt.Field(i).Name]; !ok {
- continue
- }
- if !assignable.Field(i).CanSet() {
- continue
- }
- results = append(results, s.Field(i))
- }
- return results
- })
-
- return gopter.DeriveGen(
- buildStructFunc.Interface(),
- unbuildStructFunc.Interface(),
- fieldGens...,
- )
-}
-
-// StructPtr generates pointers to a given struct type.
-// Note that StructPtr does not generate nil, if you want to include nil in your
-// testing you should combine gen.PtrOf with gen.Struct.
-// rt has to be the reflect type of the struct, gens contains a map of field generators.
-// Note that the result types of the generators in gen have to match the type of the correspoinding
-// field in the struct. Also note that only public fields of a struct can be generated
-func StructPtr(rt reflect.Type, gens map[string]gopter.Gen) gopter.Gen {
- if rt.Kind() == reflect.Ptr {
- rt = rt.Elem()
- }
-
- buildPtrType := reflect.FuncOf([]reflect.Type{rt}, []reflect.Type{reflect.PtrTo(rt)}, false)
- unbuildPtrType := reflect.FuncOf([]reflect.Type{reflect.PtrTo(rt)}, []reflect.Type{rt}, false)
-
- buildPtrFunc := reflect.MakeFunc(buildPtrType, func(args []reflect.Value) []reflect.Value {
- sp := reflect.New(rt)
- sp.Elem().Set(args[0])
- return []reflect.Value{sp}
- })
- unbuildPtrFunc := reflect.MakeFunc(unbuildPtrType, func(args []reflect.Value) []reflect.Value {
- return []reflect.Value{args[0].Elem()}
- })
-
- return gopter.DeriveGen(
- buildPtrFunc.Interface(),
- unbuildPtrFunc.Interface(),
- Struct(rt, gens),
- )
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/time.go b/vendor/github.com/leanovate/gopter/gen/time.go
deleted file mode 100644
index 72e658c5..00000000
--- a/vendor/github.com/leanovate/gopter/gen/time.go
+++ /dev/null
@@ -1,37 +0,0 @@
-package gen
-
-import (
- "time"
-
- "github.com/leanovate/gopter"
-)
-
-// Time generates an arbitrary time.Time within year [0, 9999]
-func Time() gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- sec := genParams.Rng.Int63n(253402214400) // Ensure year in [0, 9999]
- usec := genParams.Rng.Int63n(1000000000)
-
- return gopter.NewGenResult(time.Unix(sec, usec), TimeShrinker)
- }
-}
-
-// AnyTime generates an arbitrary time.Time struct (might be way out of bounds of any reason)
-func AnyTime() gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- sec := genParams.NextInt64()
- usec := genParams.NextInt64()
-
- return gopter.NewGenResult(time.Unix(sec, usec), TimeShrinker)
- }
-}
-
-// TimeRange generates an arbitrary time.Time with a range
-// from defines the start of the time range
-// duration defines the overall duration of the time range
-func TimeRange(from time.Time, duration time.Duration) gopter.Gen {
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- v := from.Add(time.Duration(genParams.Rng.Int63n(int64(duration))))
- return gopter.NewGenResult(v, TimeShrinker)
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/time_shrink.go b/vendor/github.com/leanovate/gopter/gen/time_shrink.go
deleted file mode 100644
index f7b76e55..00000000
--- a/vendor/github.com/leanovate/gopter/gen/time_shrink.go
+++ /dev/null
@@ -1,27 +0,0 @@
-package gen
-
-import (
- "time"
-
- "github.com/leanovate/gopter"
-)
-
-// TimeShrinker is a shrinker for time.Time structs
-func TimeShrinker(v interface{}) gopter.Shrink {
- t := v.(time.Time)
- sec := t.Unix()
- nsec := int64(t.Nanosecond())
- secShrink := uint64Shrink{
- original: uint64(sec),
- half: uint64(sec),
- }
- nsecShrink := uint64Shrink{
- original: uint64(nsec),
- half: uint64(nsec),
- }
- return gopter.Shrink(secShrink.Next).Map(func(v uint64) time.Time {
- return time.Unix(int64(v), nsec)
- }).Interleave(gopter.Shrink(nsecShrink.Next).Map(func(v uint64) time.Time {
- return time.Unix(sec, int64(v))
- }))
-}
diff --git a/vendor/github.com/leanovate/gopter/gen/weighted.go b/vendor/github.com/leanovate/gopter/gen/weighted.go
deleted file mode 100644
index af97c505..00000000
--- a/vendor/github.com/leanovate/gopter/gen/weighted.go
+++ /dev/null
@@ -1,44 +0,0 @@
-package gen
-
-import (
- "fmt"
- "sort"
-
- "github.com/leanovate/gopter"
-)
-
-// WeightedGen adds a weight number to a generator.
-// To be used as parameter to gen.Weighted
-type WeightedGen struct {
- Weight int
- Gen gopter.Gen
-}
-
-// Weighted combines multiple generators, where each generator has a weight.
-// The weight of a generator is proportional to the probability that the
-// generator gets selected.
-func Weighted(weightedGens []WeightedGen) gopter.Gen {
- if len(weightedGens) == 0 {
- panic("weightedGens must be non-empty")
- }
- weights := make(sort.IntSlice, 0, len(weightedGens))
-
- totalWeight := 0
- for _, weightedGen := range weightedGens {
- w := weightedGen.Weight
- if w <= 0 {
- panic(fmt.Sprintf(
- "weightedGens must have positive weights; got %d",
- w))
- }
- totalWeight += weightedGen.Weight
- weights = append(weights, totalWeight)
- }
- return func(genParams *gopter.GenParameters) *gopter.GenResult {
- idx := weights.Search(1 + genParams.Rng.Intn(totalWeight))
- gen := weightedGens[idx].Gen
- result := gen(genParams)
- result.Sieve = nil
- return result
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen_parameters.go b/vendor/github.com/leanovate/gopter/gen_parameters.go
deleted file mode 100644
index ee004968..00000000
--- a/vendor/github.com/leanovate/gopter/gen_parameters.go
+++ /dev/null
@@ -1,81 +0,0 @@
-package gopter
-
-import (
- "math/rand"
- "time"
-)
-
-// GenParameters encapsulates the parameters for all generators.
-type GenParameters struct {
- MinSize int
- MaxSize int
- MaxShrinkCount int
- Rng *rand.Rand
-}
-
-// WithSize modifies the size parameter. The size parameter defines an upper bound for the size of
-// generated slices or strings.
-func (p *GenParameters) WithSize(size int) *GenParameters {
- newParameters := *p
- newParameters.MaxSize = size
- return &newParameters
-}
-
-// NextBool create a random boolean using the underlying Rng.
-func (p *GenParameters) NextBool() bool {
- return p.Rng.Int63()&1 == 0
-}
-
-// NextInt64 create a random int64 using the underlying Rng.
-func (p *GenParameters) NextInt64() int64 {
- v := p.Rng.Int63()
- if p.NextBool() {
- return -v
- }
- return v
-}
-
-// NextUint64 create a random uint64 using the underlying Rng.
-func (p *GenParameters) NextUint64() uint64 {
- first := uint64(p.Rng.Int63())
- second := uint64(p.Rng.Int63())
-
- return (first << 1) ^ second
-}
-
-// CloneWithSeed clone the current parameters with a new seed.
-// This is useful to create subsections that can rerun (provided you keep the
-// seed)
-func (p *GenParameters) CloneWithSeed(seed int64) *GenParameters {
- return &GenParameters{
- MinSize: p.MinSize,
- MaxSize: p.MaxSize,
- MaxShrinkCount: p.MaxShrinkCount,
- Rng: rand.New(NewLockedSource(seed)),
- }
-}
-
-// DefaultGenParameters creates default GenParameters.
-func DefaultGenParameters() *GenParameters {
- seed := time.Now().UnixNano()
-
- return &GenParameters{
- MinSize: 0,
- MaxSize: 100,
- MaxShrinkCount: 1000,
- Rng: rand.New(NewLockedSource(seed)),
- }
-}
-
-// MinGenParameters creates minimal GenParameters.
-// Note: Most likely you do not want to use these for actual testing
-func MinGenParameters() *GenParameters {
- seed := time.Now().UnixNano()
-
- return &GenParameters{
- MinSize: 0,
- MaxSize: 0,
- MaxShrinkCount: 0,
- Rng: rand.New(NewLockedSource(seed)),
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/gen_result.go b/vendor/github.com/leanovate/gopter/gen_result.go
deleted file mode 100644
index f692f4e4..00000000
--- a/vendor/github.com/leanovate/gopter/gen_result.go
+++ /dev/null
@@ -1,55 +0,0 @@
-package gopter
-
-import "reflect"
-
-// GenResult contains the result of a generator.
-type GenResult struct {
- Labels []string
- Shrinker Shrinker
- ResultType reflect.Type
- Result interface{}
- Sieve func(interface{}) bool
-}
-
-// NewGenResult creates a new generator result from for a concrete value and
-// shrinker.
-// Note: The concrete value "result" not be nil
-func NewGenResult(result interface{}, shrinker Shrinker) *GenResult {
- return &GenResult{
- Shrinker: shrinker,
- ResultType: reflect.TypeOf(result),
- Result: result,
- }
-}
-
-// NewEmptyResult creates an empty generator result.
-// Unless the sieve does not explicitly allow it, empty (i.e. nil-valued)
-// results are considered invalid.
-func NewEmptyResult(resultType reflect.Type) *GenResult {
- return &GenResult{
- ResultType: resultType,
- Shrinker: NoShrinker,
- }
-}
-
-// Retrieve gets the concrete generator result.
-// If the result is invalid or does not pass the sieve there is no concrete
-// value and the property using the generator should be undecided.
-func (r *GenResult) Retrieve() (interface{}, bool) {
- if (r.Sieve == nil && r.Result != nil) || (r.Sieve != nil && r.Sieve(r.Result)) {
- return r.Result, true
- }
- return nil, false
-}
-
-// RetrieveAsValue get the concrete generator result as reflect value.
-// If the result is invalid or does not pass the sieve there is no concrete
-// value and the property using the generator should be undecided.
-func (r *GenResult) RetrieveAsValue() (reflect.Value, bool) {
- if r.Result != nil && (r.Sieve == nil || r.Sieve(r.Result)) {
- return reflect.ValueOf(r.Result), true
- } else if r.Result == nil && r.Sieve != nil && r.Sieve(r.Result) {
- return reflect.Zero(r.ResultType), true
- }
- return reflect.Zero(r.ResultType), false
-}
diff --git a/vendor/github.com/leanovate/gopter/locked_source.go b/vendor/github.com/leanovate/gopter/locked_source.go
deleted file mode 100644
index 34f5241c..00000000
--- a/vendor/github.com/leanovate/gopter/locked_source.go
+++ /dev/null
@@ -1,77 +0,0 @@
-// Copyright 2009 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-// Taken from golang lockedSource implementation https://github.com/golang/go/blob/master/src/math/rand/rand.go#L371-L410
-
-package gopter
-
-import (
- "math/rand"
- "sync"
-)
-
-type lockedSource struct {
- lk sync.Mutex
- src rand.Source64
-}
-
-// NewLockedSource takes a seed and returns a new
-// lockedSource for use with rand.New
-func NewLockedSource(seed int64) *lockedSource {
- return &lockedSource{
- src: rand.NewSource(seed).(rand.Source64),
- }
-}
-
-func (r *lockedSource) Int63() (n int64) {
- r.lk.Lock()
- n = r.src.Int63()
- r.lk.Unlock()
- return
-}
-
-func (r *lockedSource) Uint64() (n uint64) {
- r.lk.Lock()
- n = r.src.Uint64()
- r.lk.Unlock()
- return
-}
-
-func (r *lockedSource) Seed(seed int64) {
- r.lk.Lock()
- r.src.Seed(seed)
- r.lk.Unlock()
-}
-
-// seedPos implements Seed for a lockedSource without a race condition.
-func (r *lockedSource) seedPos(seed int64, readPos *int8) {
- r.lk.Lock()
- r.src.Seed(seed)
- *readPos = 0
- r.lk.Unlock()
-}
-
-// read implements Read for a lockedSource without a race condition.
-func (r *lockedSource) read(p []byte, readVal *int64, readPos *int8) (n int, err error) {
- r.lk.Lock()
- n, err = read(p, r.src.Int63, readVal, readPos)
- r.lk.Unlock()
- return
-}
-
-func read(p []byte, int63 func() int64, readVal *int64, readPos *int8) (n int, err error) {
- pos := *readPos
- val := *readVal
- for n = 0; n < len(p); n++ {
- if pos == 0 {
- val = int63()
- pos = 7
- }
- p[n] = byte(val)
- val >>= 8
- pos--
- }
- *readPos = pos
- *readVal = val
- return
-}
diff --git a/vendor/github.com/leanovate/gopter/prop.go b/vendor/github.com/leanovate/gopter/prop.go
deleted file mode 100644
index 6ea5afc5..00000000
--- a/vendor/github.com/leanovate/gopter/prop.go
+++ /dev/null
@@ -1,113 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "math"
- "runtime/debug"
-)
-
-// Prop represent some kind of property that (drums please) can and should be checked
-type Prop func(*GenParameters) *PropResult
-
-// SaveProp creates s save property by handling all panics from an inner property
-func SaveProp(prop Prop) Prop {
- return func(genParams *GenParameters) (result *PropResult) {
- defer func() {
- if r := recover(); r != nil {
- result = &PropResult{
- Status: PropError,
- Error: fmt.Errorf("Check paniced: %v", r),
- ErrorStack: debug.Stack(),
- }
- }
- }()
-
- return prop(genParams)
- }
-}
-
-// Check the property using specific parameters
-func (prop Prop) Check(parameters *TestParameters) *TestResult {
- iterations := math.Ceil(float64(parameters.MinSuccessfulTests) / float64(parameters.Workers))
- sizeStep := float64(parameters.MaxSize-parameters.MinSize) / (iterations * float64(parameters.Workers))
-
- genParameters := GenParameters{
- MinSize: parameters.MinSize,
- MaxSize: parameters.MaxSize,
- MaxShrinkCount: parameters.MaxShrinkCount,
- Rng: parameters.Rng,
- }
- runner := &runner{
- parameters: parameters,
- worker: func(workerIdx int, shouldStop shouldStop) *TestResult {
- var n int
- var d int
-
- isExhaused := func() bool {
- return n+d > parameters.MinSuccessfulTests &&
- 1.0+float64(parameters.Workers*n)*parameters.MaxDiscardRatio < float64(d)
- }
-
- for !shouldStop() && n < int(iterations) {
- size := float64(parameters.MinSize) + (sizeStep * float64(workerIdx+(parameters.Workers*(n+d))))
- propResult := prop(genParameters.WithSize(int(size)))
-
- switch propResult.Status {
- case PropUndecided:
- d++
- if isExhaused() {
- return &TestResult{
- Status: TestExhausted,
- Succeeded: n,
- Discarded: d,
- }
- }
- case PropTrue:
- n++
- case PropProof:
- n++
- return &TestResult{
- Status: TestProved,
- Succeeded: n,
- Discarded: d,
- Labels: propResult.Labels,
- Args: propResult.Args,
- }
- case PropFalse:
- return &TestResult{
- Status: TestFailed,
- Succeeded: n,
- Discarded: d,
- Labels: propResult.Labels,
- Args: propResult.Args,
- }
- case PropError:
- return &TestResult{
- Status: TestError,
- Succeeded: n,
- Discarded: d,
- Labels: propResult.Labels,
- Error: propResult.Error,
- ErrorStack: propResult.ErrorStack,
- Args: propResult.Args,
- }
- }
- }
-
- if isExhaused() {
- return &TestResult{
- Status: TestExhausted,
- Succeeded: n,
- Discarded: d,
- }
- }
- return &TestResult{
- Status: TestPassed,
- Succeeded: n,
- Discarded: d,
- }
- },
- }
-
- return runner.runWorkers()
-}
diff --git a/vendor/github.com/leanovate/gopter/prop/check_condition_func.go b/vendor/github.com/leanovate/gopter/prop/check_condition_func.go
deleted file mode 100644
index ea3dbe90..00000000
--- a/vendor/github.com/leanovate/gopter/prop/check_condition_func.go
+++ /dev/null
@@ -1,50 +0,0 @@
-package prop
-
-import (
- "errors"
- "fmt"
- "reflect"
- "runtime/debug"
-
- "github.com/leanovate/gopter"
-)
-
-func checkConditionFunc(check interface{}, numArgs int) (func([]reflect.Value) *gopter.PropResult, error) {
- checkVal := reflect.ValueOf(check)
- checkType := checkVal.Type()
-
- if checkType.Kind() != reflect.Func {
- return nil, fmt.Errorf("First param of ForrAll has to be a func: %v", checkVal.Kind())
- }
- if checkType.NumIn() != numArgs {
- return nil, fmt.Errorf("Number of parameters does not match number of generators: %d != %d", checkType.NumIn(), numArgs)
- }
- if checkType.NumOut() == 0 {
- return nil, errors.New("At least one output parameters is required")
- } else if checkType.NumOut() > 2 {
- return nil, fmt.Errorf("No more than 2 output parameters are allowed: %d", checkType.NumOut())
- } else if checkType.NumOut() == 2 && !checkType.Out(1).Implements(typeOfError) {
- return nil, fmt.Errorf("No 2 output has to be error: %v", checkType.Out(1).Kind())
- } else if checkType.NumOut() == 2 {
- return func(values []reflect.Value) *gopter.PropResult {
- results := checkVal.Call(values)
- if results[1].IsNil() {
- return convertResult(results[0].Interface(), nil)
- }
- return convertResult(results[0].Interface(), results[1].Interface().(error))
- }, nil
- }
- return func(values []reflect.Value) (result *gopter.PropResult) {
- defer func() {
- if r := recover(); r != nil {
- result = &gopter.PropResult{
- Status: gopter.PropError,
- Error: fmt.Errorf("Check paniced: %v", r),
- ErrorStack: debug.Stack(),
- }
- }
- }()
- results := checkVal.Call(values)
- return convertResult(results[0].Interface(), nil)
- }, nil
-}
diff --git a/vendor/github.com/leanovate/gopter/prop/convert_result.go b/vendor/github.com/leanovate/gopter/prop/convert_result.go
deleted file mode 100644
index 12e38cec..00000000
--- a/vendor/github.com/leanovate/gopter/prop/convert_result.go
+++ /dev/null
@@ -1,37 +0,0 @@
-package prop
-
-import (
- "fmt"
-
- "github.com/leanovate/gopter"
-)
-
-func convertResult(result interface{}, err error) *gopter.PropResult {
- if err != nil {
- return &gopter.PropResult{
- Status: gopter.PropError,
- Error: err,
- }
- }
- switch result.(type) {
- case bool:
- if result.(bool) {
- return &gopter.PropResult{Status: gopter.PropTrue}
- }
- return &gopter.PropResult{Status: gopter.PropFalse}
- case string:
- if result.(string) == "" {
- return &gopter.PropResult{Status: gopter.PropTrue}
- }
- return &gopter.PropResult{
- Status: gopter.PropFalse,
- Labels: []string{result.(string)},
- }
- case *gopter.PropResult:
- return result.(*gopter.PropResult)
- }
- return &gopter.PropResult{
- Status: gopter.PropError,
- Error: fmt.Errorf("Invalid check result: %#v", result),
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/prop/doc.go b/vendor/github.com/leanovate/gopter/prop/doc.go
deleted file mode 100644
index 256ab2e7..00000000
--- a/vendor/github.com/leanovate/gopter/prop/doc.go
+++ /dev/null
@@ -1,4 +0,0 @@
-/*
-Package prop contains the most common implementations of a gopter.Prop.
-*/
-package prop
diff --git a/vendor/github.com/leanovate/gopter/prop/error.go b/vendor/github.com/leanovate/gopter/prop/error.go
deleted file mode 100644
index 0c91d535..00000000
--- a/vendor/github.com/leanovate/gopter/prop/error.go
+++ /dev/null
@@ -1,14 +0,0 @@
-package prop
-
-import "github.com/leanovate/gopter"
-
-// ErrorProp creates a property that will always fail with an error.
-// Mostly used as a fallback when setup/initialization fails
-func ErrorProp(err error) gopter.Prop {
- return func(genParams *gopter.GenParameters) *gopter.PropResult {
- return &gopter.PropResult{
- Status: gopter.PropError,
- Error: err,
- }
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/prop/forall.go b/vendor/github.com/leanovate/gopter/prop/forall.go
deleted file mode 100644
index 00c63436..00000000
--- a/vendor/github.com/leanovate/gopter/prop/forall.go
+++ /dev/null
@@ -1,130 +0,0 @@
-package prop
-
-import (
- "fmt"
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-var typeOfError = reflect.TypeOf((*error)(nil)).Elem()
-
-/*
-ForAll creates a property that requires the check condition to be true for all values, if the
-condition falsiies the generated values will be shrunk.
-
-"condition" has to be a function with the same number of parameters as the provided
-generators "gens". The function may return a simple bool (true means that the
-condition has passed), a string (empty string means that condition has passed),
-a *PropResult, or one of former combined with an error.
-*/
-func ForAll(condition interface{}, gens ...gopter.Gen) gopter.Prop {
- callCheck, err := checkConditionFunc(condition, len(gens))
- if err != nil {
- return ErrorProp(err)
- }
-
- return gopter.SaveProp(func(genParams *gopter.GenParameters) *gopter.PropResult {
- genResults := make([]*gopter.GenResult, len(gens))
- values := make([]reflect.Value, len(gens))
- valuesFormated := make([]string, len(gens))
- var ok bool
- for i, gen := range gens {
- result := gen(genParams)
- genResults[i] = result
- values[i], ok = result.RetrieveAsValue()
- if !ok {
- return &gopter.PropResult{
- Status: gopter.PropUndecided,
- }
- }
- valuesFormated[i] = fmt.Sprintf("%+v", values[i].Interface())
- }
- result := callCheck(values)
- if result.Success() {
- for i, genResult := range genResults {
- result = result.AddArgs(gopter.NewPropArg(genResult, 0, values[i].Interface(), valuesFormated[i], values[i].Interface(), valuesFormated[i]))
- }
- } else {
- for i, genResult := range genResults {
- nextResult, nextValue := shrinkValue(genParams.MaxShrinkCount, genResult, values[i].Interface(), valuesFormated[i], result,
- func(v interface{}) *gopter.PropResult {
- shrunkOne := make([]reflect.Value, len(values))
- copy(shrunkOne, values)
- if v == nil {
- shrunkOne[i] = reflect.Zero(values[i].Type())
- } else {
- shrunkOne[i] = reflect.ValueOf(v)
- }
- return callCheck(shrunkOne)
- })
- result = nextResult
- if nextValue == nil {
- values[i] = reflect.Zero(values[i].Type())
- } else {
- values[i] = reflect.ValueOf(nextValue)
- }
- }
- }
- return result
- })
-}
-
-// ForAll1 legacy interface to be removed in the future
-func ForAll1(gen gopter.Gen, check func(v interface{}) (interface{}, error)) gopter.Prop {
- checkFunc := func(v interface{}) *gopter.PropResult {
- return convertResult(check(v))
- }
- return gopter.SaveProp(func(genParams *gopter.GenParameters) *gopter.PropResult {
- genResult := gen(genParams)
- value, ok := genResult.Retrieve()
- if !ok {
- return &gopter.PropResult{
- Status: gopter.PropUndecided,
- }
- }
- valueFormated := fmt.Sprintf("%+v", value)
- result := checkFunc(value)
- if result.Success() {
- return result.AddArgs(gopter.NewPropArg(genResult, 0, value, valueFormated, value, valueFormated))
- }
-
- result, _ = shrinkValue(genParams.MaxShrinkCount, genResult, value, valueFormated, result, checkFunc)
- return result
- })
-}
-
-func shrinkValue(maxShrinkCount int, genResult *gopter.GenResult, origValue interface{}, orgiValueFormated string,
- firstFail *gopter.PropResult, check func(interface{}) *gopter.PropResult) (*gopter.PropResult, interface{}) {
- lastFail := firstFail
- lastValue := origValue
- lastValueFormated := orgiValueFormated
-
- shrinks := 0
- shrink := genResult.Shrinker(lastValue).Filter(genResult.Sieve)
- nextResult, nextValue, nextValueFormated := firstFailure(shrink, check)
- for nextResult != nil && shrinks < maxShrinkCount {
- shrinks++
- lastValue = nextValue
- lastValueFormated = nextValueFormated
- lastFail = nextResult
-
- shrink = genResult.Shrinker(lastValue).Filter(genResult.Sieve)
- nextResult, nextValue, nextValueFormated = firstFailure(shrink, check)
- }
-
- return lastFail.WithArgs(firstFail.Args).AddArgs(gopter.NewPropArg(genResult, shrinks, lastValue, lastValueFormated, origValue, orgiValueFormated)), lastValue
-}
-
-func firstFailure(shrink gopter.Shrink, check func(interface{}) *gopter.PropResult) (*gopter.PropResult, interface{}, string) {
- value, ok := shrink()
- for ok {
- valueFormated := fmt.Sprintf("%+v", value)
- result := check(value)
- if !result.Success() {
- return result, value, valueFormated
- }
- value, ok = shrink()
- }
- return nil, nil, ""
-}
diff --git a/vendor/github.com/leanovate/gopter/prop/forall_no_shrink.go b/vendor/github.com/leanovate/gopter/prop/forall_no_shrink.go
deleted file mode 100644
index affcd3bc..00000000
--- a/vendor/github.com/leanovate/gopter/prop/forall_no_shrink.go
+++ /dev/null
@@ -1,63 +0,0 @@
-package prop
-
-import (
- "fmt"
- "reflect"
-
- "github.com/leanovate/gopter"
-)
-
-/*
-ForAllNoShrink creates a property that requires the check condition to be true for all values.
-As the name suggests the generated values will not be shrunk if the condition falsiies.
-
-"condition" has to be a function with the same number of parameters as the provided
-generators "gens". The function may return a simple bool (true means that the
-condition has passed), a string (empty string means that condition has passed),
-a *PropResult, or one of former combined with an error.
-*/
-func ForAllNoShrink(condition interface{}, gens ...gopter.Gen) gopter.Prop {
- callCheck, err := checkConditionFunc(condition, len(gens))
- if err != nil {
- return ErrorProp(err)
- }
-
- return gopter.SaveProp(func(genParams *gopter.GenParameters) *gopter.PropResult {
- genResults := make([]*gopter.GenResult, len(gens))
- values := make([]reflect.Value, len(gens))
- valuesFormated := make([]string, len(gens))
- var ok bool
- for i, gen := range gens {
- result := gen(genParams)
- genResults[i] = result
- values[i], ok = result.RetrieveAsValue()
- if !ok {
- return &gopter.PropResult{
- Status: gopter.PropUndecided,
- }
- }
- valuesFormated[i] = fmt.Sprintf("%+v", values[i].Interface())
- }
- result := callCheck(values)
- for i, genResult := range genResults {
- result = result.AddArgs(gopter.NewPropArg(genResult, 0, values[i].Interface(), valuesFormated[i], values[i].Interface(), valuesFormated[i]))
- }
- return result
- })
-}
-
-// ForAllNoShrink1 creates a property that requires the check condition to be true for all values
-// As the name suggests the generated values will not be shrunk if the condition falsiies
-func ForAllNoShrink1(gen gopter.Gen, check func(interface{}) (interface{}, error)) gopter.Prop {
- return gopter.SaveProp(func(genParams *gopter.GenParameters) *gopter.PropResult {
- genResult := gen(genParams)
- value, ok := genResult.Retrieve()
- if !ok {
- return &gopter.PropResult{
- Status: gopter.PropUndecided,
- }
- }
- valueFormated := fmt.Sprintf("%+v", value)
- return convertResult(check(value)).AddArgs(gopter.NewPropArg(genResult, 0, value, valueFormated, value, valueFormated))
- })
-}
diff --git a/vendor/github.com/leanovate/gopter/prop_arg.go b/vendor/github.com/leanovate/gopter/prop_arg.go
deleted file mode 100644
index cd7af6fd..00000000
--- a/vendor/github.com/leanovate/gopter/prop_arg.go
+++ /dev/null
@@ -1,36 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "strings"
-)
-
-// PropArg contains information about the specific values for a certain property check.
-// This is mostly used for reporting when a property has falsified.
-type PropArg struct {
- Arg interface{}
- ArgFormatted string
- OrigArg interface{}
- OrigArgFormatted string
- Label string
- Shrinks int
-}
-
-func (p *PropArg) String() string {
- return fmt.Sprintf("%v", p.Arg)
-}
-
-// PropArgs is a list of PropArg.
-type PropArgs []*PropArg
-
-// NewPropArg creates a new PropArg.
-func NewPropArg(genResult *GenResult, shrinks int, value interface{}, valueFormated string, origValue interface{}, origValueFormated string) *PropArg {
- return &PropArg{
- Label: strings.Join(genResult.Labels, ", "),
- Arg: value,
- ArgFormatted: valueFormated,
- OrigArg: origValue,
- OrigArgFormatted: origValueFormated,
- Shrinks: shrinks,
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/prop_result.go b/vendor/github.com/leanovate/gopter/prop_result.go
deleted file mode 100644
index 0a8bbeba..00000000
--- a/vendor/github.com/leanovate/gopter/prop_result.go
+++ /dev/null
@@ -1,109 +0,0 @@
-package gopter
-
-type propStatus int
-
-const (
- // PropProof THe property was proved (i.e. it is known to be correct and will be always true)
- PropProof propStatus = iota
- // PropTrue The property was true this time
- PropTrue
- // PropFalse The property was false this time
- PropFalse
- // PropUndecided The property has no clear outcome this time
- PropUndecided
- // PropError The property has generated an error
- PropError
-)
-
-func (s propStatus) String() string {
- switch s {
- case PropProof:
- return "PROOF"
- case PropTrue:
- return "TRUE"
- case PropFalse:
- return "FALSE"
- case PropUndecided:
- return "UNDECIDED"
- case PropError:
- return "ERROR"
- }
- return ""
-}
-
-// PropResult contains the result of a property
-type PropResult struct {
- Status propStatus
- Error error
- ErrorStack []byte
- Args []*PropArg
- Labels []string
-}
-
-// NewPropResult create a PropResult with label
-func NewPropResult(success bool, label string) *PropResult {
- if success {
- return &PropResult{
- Status: PropTrue,
- Labels: []string{label},
- Args: make([]*PropArg, 0),
- }
- }
- return &PropResult{
- Status: PropFalse,
- Labels: []string{label},
- Args: make([]*PropArg, 0),
- }
-}
-
-// Success checks if the result was successful
-func (r *PropResult) Success() bool {
- return r.Status == PropTrue || r.Status == PropProof
-}
-
-// WithArgs sets argument descriptors to the PropResult for reporting
-func (r *PropResult) WithArgs(args []*PropArg) *PropResult {
- r.Args = args
- return r
-}
-
-// AddArgs add argument descriptors to the PropResult for reporting
-func (r *PropResult) AddArgs(args ...*PropArg) *PropResult {
- r.Args = append(r.Args, args...)
- return r
-}
-
-// And combines two PropResult by an and operation.
-// The resulting PropResult will be only true if both PropResults are true.
-func (r *PropResult) And(other *PropResult) *PropResult {
- switch {
- case r.Status == PropError:
- return r
- case other.Status == PropError:
- return other
- case r.Status == PropFalse:
- return r
- case other.Status == PropFalse:
- return other
- case r.Status == PropUndecided:
- return r
- case other.Status == PropUndecided:
- return other
- case r.Status == PropProof:
- return r.mergeWith(other, other.Status)
- case other.Status == PropProof:
- return r.mergeWith(other, r.Status)
- case r.Status == PropTrue && other.Status == PropTrue:
- return r.mergeWith(other, PropTrue)
- default:
- return r
- }
-}
-
-func (r *PropResult) mergeWith(other *PropResult, status propStatus) *PropResult {
- return &PropResult{
- Status: status,
- Args: append(append(make([]*PropArg, 0, len(r.Args)+len(other.Args)), r.Args...), other.Args...),
- Labels: append(append(make([]string, 0, len(r.Labels)+len(other.Labels)), r.Labels...), other.Labels...),
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/properties.go b/vendor/github.com/leanovate/gopter/properties.go
deleted file mode 100644
index af82354d..00000000
--- a/vendor/github.com/leanovate/gopter/properties.go
+++ /dev/null
@@ -1,59 +0,0 @@
-package gopter
-
-import "testing"
-
-// Properties is a collection of properties that should be checked in a test
-type Properties struct {
- parameters *TestParameters
- props map[string]Prop
- propNames []string
-}
-
-// NewProperties create new Properties with given test parameters.
-// If parameters is nil default test parameters will be used
-func NewProperties(parameters *TestParameters) *Properties {
- if parameters == nil {
- parameters = DefaultTestParameters()
- }
- return &Properties{
- parameters: parameters,
- props: make(map[string]Prop, 0),
- propNames: make([]string, 0),
- }
-}
-
-// Property add/defines a property in a test.
-func (p *Properties) Property(name string, prop Prop) {
- p.propNames = append(p.propNames, name)
- p.props[name] = prop
-}
-
-// Run checks all definied propertiesand reports the result
-func (p *Properties) Run(reporter Reporter) bool {
- success := true
- for _, propName := range p.propNames {
- prop := p.props[propName]
-
- result := prop.Check(p.parameters)
-
- reporter.ReportTestResult(propName, result)
- if !result.Passed() {
- success = false
- }
- }
- return success
-}
-
-// TestingRun checks all definied properties with a testing.T context.
-// This the preferred wait to run property tests as part of a go unit test.
-func (p *Properties) TestingRun(t *testing.T, opts ...interface{}) {
- reporter := ConsoleReporter(true)
- for _, opt := range opts {
- if r, ok := opt.(Reporter); ok {
- reporter = r
- }
- }
- if !p.Run(reporter) {
- t.Errorf("failed with initial seed: %d", p.parameters.Seed())
- }
-}
diff --git a/vendor/github.com/leanovate/gopter/reporter.go b/vendor/github.com/leanovate/gopter/reporter.go
deleted file mode 100644
index 40655aa2..00000000
--- a/vendor/github.com/leanovate/gopter/reporter.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package gopter
-
-// Reporter is a simple interface to report/format the results of a property check.
-type Reporter interface {
- // ReportTestResult reports a single property result
- ReportTestResult(propName string, result *TestResult)
-}
diff --git a/vendor/github.com/leanovate/gopter/runner.go b/vendor/github.com/leanovate/gopter/runner.go
deleted file mode 100644
index 2464f89d..00000000
--- a/vendor/github.com/leanovate/gopter/runner.go
+++ /dev/null
@@ -1,77 +0,0 @@
-package gopter
-
-import (
- "sync"
- "time"
-)
-
-type shouldStop func() bool
-
-type worker func(int, shouldStop) *TestResult
-
-type runner struct {
- sync.RWMutex
- parameters *TestParameters
- worker worker
-}
-
-func (r *runner) mergeCheckResults(r1, r2 *TestResult) *TestResult {
- var result TestResult
-
- switch {
- case r1 == nil:
- return r2
- case r1.Status != TestPassed && r1.Status != TestExhausted:
- result = *r1
- case r2.Status != TestPassed && r2.Status != TestExhausted:
- result = *r2
- default:
- result.Status = TestExhausted
-
- if r1.Succeeded+r2.Succeeded >= r.parameters.MinSuccessfulTests &&
- float64(r1.Discarded+r2.Discarded) <= float64(r1.Succeeded+r2.Succeeded)*r.parameters.MaxDiscardRatio {
- result.Status = TestPassed
- }
- }
-
- result.Succeeded = r1.Succeeded + r2.Succeeded
- result.Discarded = r1.Discarded + r2.Discarded
-
- return &result
-}
-
-func (r *runner) runWorkers() *TestResult {
- var stopFlag Flag
- defer stopFlag.Set()
-
- start := time.Now()
- if r.parameters.Workers < 2 {
- result := r.worker(0, stopFlag.Get)
- result.Time = time.Since(start)
- return result
- }
- var waitGroup sync.WaitGroup
- waitGroup.Add(r.parameters.Workers)
- results := make(chan *TestResult, r.parameters.Workers)
- combinedResult := make(chan *TestResult)
-
- go func() {
- var combined *TestResult
- for result := range results {
- combined = r.mergeCheckResults(combined, result)
- }
- combinedResult <- combined
- }()
- for i := 0; i < r.parameters.Workers; i++ {
- go func(workerIdx int) {
- defer waitGroup.Done()
- results <- r.worker(workerIdx, stopFlag.Get)
- }(i)
- }
- waitGroup.Wait()
- close(results)
-
- result := <-combinedResult
- result.Time = time.Since(start)
- return result
-}
diff --git a/vendor/github.com/leanovate/gopter/shrink.go b/vendor/github.com/leanovate/gopter/shrink.go
deleted file mode 100644
index 129d5ef1..00000000
--- a/vendor/github.com/leanovate/gopter/shrink.go
+++ /dev/null
@@ -1,185 +0,0 @@
-package gopter
-
-import (
- "fmt"
- "reflect"
-)
-
-// Shrink is a stream of shrunk down values.
-// Once the result of a shrink is false, it is considered to be exhausted.
-// Important notes for implementors:
-// * Ensure that the returned stream is finite, even though shrinking will
-// eventually be aborted, infinite streams may result in very slow running
-// test.
-// * Ensure that modifications to the returned value will not affect the
-// internal state of your Shrink. If in doubt return by value not by reference
-type Shrink func() (interface{}, bool)
-
-// Filter creates a shrink filtered by a condition
-func (s Shrink) Filter(condition func(interface{}) bool) Shrink {
- if condition == nil {
- return s
- }
- return func() (interface{}, bool) {
- value, ok := s()
- for ok && !condition(value) {
- value, ok = s()
- }
- return value, ok
- }
-}
-
-// Map creates a shrink by applying a converter to each element of a shrink.
-// f: has to be a function with one parameter (matching the generated value) and a single return.
-func (s Shrink) Map(f interface{}) Shrink {
- mapperVal := reflect.ValueOf(f)
- mapperType := mapperVal.Type()
-
- if mapperVal.Kind() != reflect.Func {
- panic(fmt.Sprintf("Param of Map has to be a func, but is %v", mapperType.Kind()))
- }
- if mapperType.NumIn() != 1 {
- panic(fmt.Sprintf("Param of Map has to be a func with one param, but is %v", mapperType.NumIn()))
- }
- if mapperType.NumOut() != 1 {
- panic(fmt.Sprintf("Param of Map has to be a func with one return value, but is %v", mapperType.NumOut()))
- }
-
- return func() (interface{}, bool) {
- value, ok := s()
- if ok {
- return mapperVal.Call([]reflect.Value{reflect.ValueOf(value)})[0].Interface(), ok
- }
- return nil, false
- }
-}
-
-// All collects all shrinks as a slice. Use with care as this might create
-// large results depending on the complexity of the shrink
-func (s Shrink) All() []interface{} {
- result := []interface{}{}
- value, ok := s()
- for ok {
- result = append(result, value)
- value, ok = s()
- }
- return result
-}
-
-type concatedShrink struct {
- index int
- shrinks []Shrink
-}
-
-func (c *concatedShrink) Next() (interface{}, bool) {
- for c.index < len(c.shrinks) {
- value, ok := c.shrinks[c.index]()
- if ok {
- return value, ok
- }
- c.index++
- }
- return nil, false
-}
-
-// ConcatShrinks concats an array of shrinks to a single shrinks
-func ConcatShrinks(shrinks ...Shrink) Shrink {
- concated := &concatedShrink{
- index: 0,
- shrinks: shrinks,
- }
- return concated.Next
-}
-
-type interleaved struct {
- first Shrink
- second Shrink
- firstExhausted bool
- secondExhaused bool
- state bool
-}
-
-func (i *interleaved) Next() (interface{}, bool) {
- for !i.firstExhausted || !i.secondExhaused {
- i.state = !i.state
- if i.state && !i.firstExhausted {
- value, ok := i.first()
- if ok {
- return value, true
- }
- i.firstExhausted = true
- } else if !i.state && !i.secondExhaused {
- value, ok := i.second()
- if ok {
- return value, true
- }
- i.secondExhaused = true
- }
- }
- return nil, false
-}
-
-// Interleave this shrink with another
-// Both shrinks are expected to produce the same result
-func (s Shrink) Interleave(other Shrink) Shrink {
- interleaved := &interleaved{
- first: s,
- second: other,
- }
- return interleaved.Next
-}
-
-// Shrinker creates a shrink for a given value
-type Shrinker func(value interface{}) Shrink
-
-type elementShrink struct {
- original []interface{}
- index int
- elementShrink Shrink
-}
-
-func (e *elementShrink) Next() (interface{}, bool) {
- element, ok := e.elementShrink()
- if !ok {
- return nil, false
- }
- shrunk := make([]interface{}, len(e.original))
- copy(shrunk, e.original)
- shrunk[e.index] = element
-
- return shrunk, true
-}
-
-// CombineShrinker create a shrinker by combining a list of shrinkers.
-// The resulting shrinker will shrink an []interface{} where each element will be shrunk by
-// the corresonding shrinker in 'shrinkers'.
-// This method is implicitly used by CombineGens.
-func CombineShrinker(shrinkers ...Shrinker) Shrinker {
- return func(v interface{}) Shrink {
- values := v.([]interface{})
- shrinks := make([]Shrink, 0, len(values))
- for i, shrinker := range shrinkers {
- if i >= len(values) {
- break
- }
- shrink := &elementShrink{
- original: values,
- index: i,
- elementShrink: shrinker(values[i]),
- }
- shrinks = append(shrinks, shrink.Next)
- }
- return ConcatShrinks(shrinks...)
- }
-}
-
-// NoShrink is an empty shrink.
-var NoShrink = Shrink(func() (interface{}, bool) {
- return nil, false
-})
-
-// NoShrinker is a shrinker for NoShrink, i.e. a Shrinker that will not shrink any values.
-// This is the default Shrinker if none is provided.
-var NoShrinker = Shrinker(func(value interface{}) Shrink {
- return NoShrink
-})
diff --git a/vendor/github.com/leanovate/gopter/test_parameters.go b/vendor/github.com/leanovate/gopter/test_parameters.go
deleted file mode 100644
index 5234c595..00000000
--- a/vendor/github.com/leanovate/gopter/test_parameters.go
+++ /dev/null
@@ -1,48 +0,0 @@
-package gopter
-
-import (
- "math/rand"
- "time"
-)
-
-// TestParameters to run property tests
-type TestParameters struct {
- MinSuccessfulTests int
- // MinSize is an (inclusive) lower limit on the size of the parameters
- MinSize int
- // MaxSize is an (exclusive) upper limit on the size of the parameters
- MaxSize int
- MaxShrinkCount int
- seed int64
- Rng *rand.Rand
- Workers int
- MaxDiscardRatio float64
-}
-
-func (t *TestParameters) Seed() int64 {
- return t.seed
-}
-
-func (t *TestParameters) SetSeed(seed int64) {
- t.seed = seed
- t.Rng.Seed(seed)
-}
-
-// DefaultTestParameterWithSeeds creates reasonable default Parameters for most cases based on a fixed RNG-seed
-func DefaultTestParametersWithSeed(seed int64) *TestParameters {
- return &TestParameters{
- MinSuccessfulTests: 100,
- MinSize: 0,
- MaxSize: 100,
- MaxShrinkCount: 1000,
- seed: seed,
- Rng: rand.New(NewLockedSource(seed)),
- Workers: 1,
- MaxDiscardRatio: 5,
- }
-}
-
-// DefaultTestParameterWithSeeds creates reasonable default Parameters for most cases with an undefined RNG-seed
-func DefaultTestParameters() *TestParameters {
- return DefaultTestParametersWithSeed(time.Now().UnixNano())
-}
diff --git a/vendor/github.com/leanovate/gopter/test_result.go b/vendor/github.com/leanovate/gopter/test_result.go
deleted file mode 100644
index ce4ac411..00000000
--- a/vendor/github.com/leanovate/gopter/test_result.go
+++ /dev/null
@@ -1,52 +0,0 @@
-package gopter
-
-import "time"
-
-type testStatus int
-
-const (
- // TestPassed indicates that the property check has passed.
- TestPassed testStatus = iota
- // TestProved indicates that the property has been proved.
- TestProved
- // TestFailed indicates that the property check has failed.
- TestFailed
- // TestExhausted indicates that the property check has exhausted, i.e. the generators have
- // generated too many empty results.
- TestExhausted
- // TestError indicates that the property check has finished with an error.
- TestError
-)
-
-func (s testStatus) String() string {
- switch s {
- case TestPassed:
- return "PASSED"
- case TestProved:
- return "PROVED"
- case TestFailed:
- return "FAILED"
- case TestExhausted:
- return "EXHAUSTED"
- case TestError:
- return "ERROR"
- }
- return ""
-}
-
-// TestResult contains the result of a property property check.
-type TestResult struct {
- Status testStatus
- Succeeded int
- Discarded int
- Labels []string
- Error error
- ErrorStack []byte
- Args PropArgs
- Time time.Duration
-}
-
-// Passed checks if the check has passed
-func (r *TestResult) Passed() bool {
- return r.Status == TestPassed || r.Status == TestProved
-}
diff --git a/vendor/github.com/leodido/go-urn/.gitignore b/vendor/github.com/leodido/go-urn/.gitignore
deleted file mode 100644
index 5bcf4bad..00000000
--- a/vendor/github.com/leodido/go-urn/.gitignore
+++ /dev/null
@@ -1,11 +0,0 @@
-*.exe
-*.dll
-*.so
-*.dylib
-
-*.test
-
-*.out
-*.txt
-
-vendor/
\ No newline at end of file
diff --git a/vendor/github.com/leodido/go-urn/.travis.yml b/vendor/github.com/leodido/go-urn/.travis.yml
deleted file mode 100644
index 21f348d6..00000000
--- a/vendor/github.com/leodido/go-urn/.travis.yml
+++ /dev/null
@@ -1,16 +0,0 @@
-language: go
-
-go:
- - 1.13.x
- - 1.14.x
- - 1.15.x
- - tip
-
-before_install:
- - go get -t -v ./...
-
-script:
- - go test -race -coverprofile=coverage.txt -covermode=atomic
-
-after_success:
- - bash <(curl -s https://codecov.io/bash)
\ No newline at end of file
diff --git a/vendor/github.com/leodido/go-urn/LICENSE b/vendor/github.com/leodido/go-urn/LICENSE
deleted file mode 100644
index 8c3504a5..00000000
--- a/vendor/github.com/leodido/go-urn/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2018 Leonardo Di Donato
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/leodido/go-urn/README.md b/vendor/github.com/leodido/go-urn/README.md
deleted file mode 100644
index cc902ec0..00000000
--- a/vendor/github.com/leodido/go-urn/README.md
+++ /dev/null
@@ -1,55 +0,0 @@
-[](https://travis-ci.org/leodido/go-urn) [](https://codecov.io/gh/leodido/go-urn) [](https://godoc.org/github.com/leodido/go-urn)
-
-**A parser for URNs**.
-
-> As seen on [RFC 2141](https://tools.ietf.org/html/rfc2141#ref-1).
-
-[API documentation](https://godoc.org/github.com/leodido/go-urn).
-
-## Installation
-
-```
-go get github.com/leodido/go-urn
-```
-
-## Performances
-
-This implementation results to be really fast.
-
-Usually below ½ microsecond on my machine[1](#mymachine).
-
-Notice it also performs, while parsing:
-
-1. fine-grained and informative erroring
-2. specific-string normalization
-
-```
-ok/00/urn:a:b______________________________________/-4 20000000 265 ns/op 182 B/op 6 allocs/op
-ok/01/URN:foo:a123,456_____________________________/-4 30000000 296 ns/op 200 B/op 6 allocs/op
-ok/02/urn:foo:a123%2c456___________________________/-4 20000000 331 ns/op 208 B/op 6 allocs/op
-ok/03/urn:ietf:params:scim:schemas:core:2.0:User___/-4 20000000 430 ns/op 280 B/op 6 allocs/op
-ok/04/urn:ietf:params:scim:schemas:extension:enterp/-4 20000000 411 ns/op 312 B/op 6 allocs/op
-ok/05/urn:ietf:params:scim:schemas:extension:enterp/-4 20000000 472 ns/op 344 B/op 6 allocs/op
-ok/06/urn:burnout:nss______________________________/-4 30000000 257 ns/op 192 B/op 6 allocs/op
-ok/07/urn:abcdefghilmnopqrstuvzabcdefghilm:x_______/-4 20000000 375 ns/op 213 B/op 6 allocs/op
-ok/08/urn:urnurnurn:urn____________________________/-4 30000000 265 ns/op 197 B/op 6 allocs/op
-ok/09/urn:ciao:@!=%2c(xyz)+a,b.*@g=$_'_____________/-4 20000000 307 ns/op 248 B/op 6 allocs/op
-ok/10/URN:x:abc%1dz%2f%3az_________________________/-4 30000000 259 ns/op 212 B/op 6 allocs/op
-no/11/URN:-xxx:x___________________________________/-4 20000000 445 ns/op 320 B/op 6 allocs/op
-no/12/urn::colon:nss_______________________________/-4 20000000 461 ns/op 320 B/op 6 allocs/op
-no/13/urn:abcdefghilmnopqrstuvzabcdefghilmn:specifi/-4 10000000 660 ns/op 320 B/op 6 allocs/op
-no/14/URN:a!?:x____________________________________/-4 20000000 507 ns/op 320 B/op 6 allocs/op
-no/15/urn:urn:NSS__________________________________/-4 20000000 429 ns/op 288 B/op 6 allocs/op
-no/16/urn:white_space:NSS__________________________/-4 20000000 482 ns/op 320 B/op 6 allocs/op
-no/17/urn:concat:no_spaces_________________________/-4 20000000 539 ns/op 328 B/op 7 allocs/op
-no/18/urn:a:/______________________________________/-4 20000000 470 ns/op 320 B/op 7 allocs/op
-no/19/urn:UrN:NSS__________________________________/-4 20000000 399 ns/op 288 B/op 6 allocs/op
-```
-
----
-
-* [1]: Intel Core i7-7600U CPU @ 2.80GHz
-
----
-
-[](https://github.com/igrigorik/ga-beacon)
\ No newline at end of file
diff --git a/vendor/github.com/leodido/go-urn/machine.go b/vendor/github.com/leodido/go-urn/machine.go
deleted file mode 100644
index fe5a0cc8..00000000
--- a/vendor/github.com/leodido/go-urn/machine.go
+++ /dev/null
@@ -1,1691 +0,0 @@
-package urn
-
-import (
- "fmt"
-)
-
-var (
- errPrefix = "expecting the prefix to be the \"urn\" string (whatever case) [col %d]"
- errIdentifier = "expecting the identifier to be string (1..31 alnum chars, also containing dashes but not at its start) [col %d]"
- errSpecificString = "expecting the specific string to be a string containing alnum, hex, or others ([()+,-.:=@;$_!*']) chars [col %d]"
- errNoUrnWithinID = "expecting the identifier to not contain the \"urn\" reserved string [col %d]"
- errHex = "expecting the specific string hex chars to be well-formed (%%alnum{2}) [col %d]"
- errParse = "parsing error [col %d]"
-)
-
-const start int = 1
-const firstFinal int = 44
-
-const enFail int = 46
-const enMain int = 1
-
-// Machine is the interface representing the FSM
-type Machine interface {
- Error() error
- Parse(input []byte) (*URN, error)
-}
-
-type machine struct {
- data []byte
- cs int
- p, pe, eof, pb int
- err error
- tolower []int
-}
-
-// NewMachine creates a new FSM able to parse RFC 2141 strings.
-func NewMachine() Machine {
- m := &machine{}
-
- return m
-}
-
-// Err returns the error that occurred on the last call to Parse.
-//
-// If the result is nil, then the line was parsed successfully.
-func (m *machine) Error() error {
- return m.err
-}
-
-func (m *machine) text() []byte {
- return m.data[m.pb:m.p]
-}
-
-// Parse parses the input byte array as a RFC 2141 string.
-func (m *machine) Parse(input []byte) (*URN, error) {
- m.data = input
- m.p = 0
- m.pb = 0
- m.pe = len(input)
- m.eof = len(input)
- m.err = nil
- m.tolower = []int{}
- output := &URN{}
-
- {
- m.cs = start
- }
-
- {
- if (m.p) == (m.pe) {
- goto _testEof
- }
- switch m.cs {
- case 1:
- goto stCase1
- case 0:
- goto stCase0
- case 2:
- goto stCase2
- case 3:
- goto stCase3
- case 4:
- goto stCase4
- case 5:
- goto stCase5
- case 6:
- goto stCase6
- case 7:
- goto stCase7
- case 8:
- goto stCase8
- case 9:
- goto stCase9
- case 10:
- goto stCase10
- case 11:
- goto stCase11
- case 12:
- goto stCase12
- case 13:
- goto stCase13
- case 14:
- goto stCase14
- case 15:
- goto stCase15
- case 16:
- goto stCase16
- case 17:
- goto stCase17
- case 18:
- goto stCase18
- case 19:
- goto stCase19
- case 20:
- goto stCase20
- case 21:
- goto stCase21
- case 22:
- goto stCase22
- case 23:
- goto stCase23
- case 24:
- goto stCase24
- case 25:
- goto stCase25
- case 26:
- goto stCase26
- case 27:
- goto stCase27
- case 28:
- goto stCase28
- case 29:
- goto stCase29
- case 30:
- goto stCase30
- case 31:
- goto stCase31
- case 32:
- goto stCase32
- case 33:
- goto stCase33
- case 34:
- goto stCase34
- case 35:
- goto stCase35
- case 36:
- goto stCase36
- case 37:
- goto stCase37
- case 38:
- goto stCase38
- case 44:
- goto stCase44
- case 39:
- goto stCase39
- case 40:
- goto stCase40
- case 45:
- goto stCase45
- case 41:
- goto stCase41
- case 42:
- goto stCase42
- case 43:
- goto stCase43
- case 46:
- goto stCase46
- }
- goto stOut
- stCase1:
- switch (m.data)[(m.p)] {
- case 85:
- goto tr1
- case 117:
- goto tr1
- }
- goto tr0
- tr0:
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- tr3:
-
- m.err = fmt.Errorf(errPrefix, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- tr6:
-
- m.err = fmt.Errorf(errIdentifier, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- tr41:
-
- m.err = fmt.Errorf(errSpecificString, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- tr44:
-
- m.err = fmt.Errorf(errHex, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errSpecificString, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- tr50:
-
- m.err = fmt.Errorf(errPrefix, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errIdentifier, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- tr52:
-
- m.err = fmt.Errorf(errNoUrnWithinID, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errIdentifier, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- goto st0
- stCase0:
- st0:
- m.cs = 0
- goto _out
- tr1:
-
- m.pb = m.p
-
- goto st2
- st2:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof2
- }
- stCase2:
- switch (m.data)[(m.p)] {
- case 82:
- goto st3
- case 114:
- goto st3
- }
- goto tr0
- st3:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof3
- }
- stCase3:
- switch (m.data)[(m.p)] {
- case 78:
- goto st4
- case 110:
- goto st4
- }
- goto tr3
- st4:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof4
- }
- stCase4:
- if (m.data)[(m.p)] == 58 {
- goto tr5
- }
- goto tr0
- tr5:
-
- output.prefix = string(m.text())
-
- goto st5
- st5:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof5
- }
- stCase5:
- switch (m.data)[(m.p)] {
- case 85:
- goto tr8
- case 117:
- goto tr8
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto tr7
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto tr7
- }
- default:
- goto tr7
- }
- goto tr6
- tr7:
-
- m.pb = m.p
-
- goto st6
- st6:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof6
- }
- stCase6:
- switch (m.data)[(m.p)] {
- case 45:
- goto st7
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st7
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st7
- }
- default:
- goto st7
- }
- goto tr6
- st7:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof7
- }
- stCase7:
- switch (m.data)[(m.p)] {
- case 45:
- goto st8
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st8
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st8
- }
- default:
- goto st8
- }
- goto tr6
- st8:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof8
- }
- stCase8:
- switch (m.data)[(m.p)] {
- case 45:
- goto st9
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st9
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st9
- }
- default:
- goto st9
- }
- goto tr6
- st9:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof9
- }
- stCase9:
- switch (m.data)[(m.p)] {
- case 45:
- goto st10
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st10
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st10
- }
- default:
- goto st10
- }
- goto tr6
- st10:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof10
- }
- stCase10:
- switch (m.data)[(m.p)] {
- case 45:
- goto st11
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st11
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st11
- }
- default:
- goto st11
- }
- goto tr6
- st11:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof11
- }
- stCase11:
- switch (m.data)[(m.p)] {
- case 45:
- goto st12
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st12
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st12
- }
- default:
- goto st12
- }
- goto tr6
- st12:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof12
- }
- stCase12:
- switch (m.data)[(m.p)] {
- case 45:
- goto st13
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st13
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st13
- }
- default:
- goto st13
- }
- goto tr6
- st13:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof13
- }
- stCase13:
- switch (m.data)[(m.p)] {
- case 45:
- goto st14
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st14
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st14
- }
- default:
- goto st14
- }
- goto tr6
- st14:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof14
- }
- stCase14:
- switch (m.data)[(m.p)] {
- case 45:
- goto st15
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st15
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st15
- }
- default:
- goto st15
- }
- goto tr6
- st15:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof15
- }
- stCase15:
- switch (m.data)[(m.p)] {
- case 45:
- goto st16
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st16
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st16
- }
- default:
- goto st16
- }
- goto tr6
- st16:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof16
- }
- stCase16:
- switch (m.data)[(m.p)] {
- case 45:
- goto st17
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st17
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st17
- }
- default:
- goto st17
- }
- goto tr6
- st17:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof17
- }
- stCase17:
- switch (m.data)[(m.p)] {
- case 45:
- goto st18
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st18
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st18
- }
- default:
- goto st18
- }
- goto tr6
- st18:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof18
- }
- stCase18:
- switch (m.data)[(m.p)] {
- case 45:
- goto st19
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st19
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st19
- }
- default:
- goto st19
- }
- goto tr6
- st19:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof19
- }
- stCase19:
- switch (m.data)[(m.p)] {
- case 45:
- goto st20
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st20
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st20
- }
- default:
- goto st20
- }
- goto tr6
- st20:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof20
- }
- stCase20:
- switch (m.data)[(m.p)] {
- case 45:
- goto st21
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st21
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st21
- }
- default:
- goto st21
- }
- goto tr6
- st21:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof21
- }
- stCase21:
- switch (m.data)[(m.p)] {
- case 45:
- goto st22
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st22
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st22
- }
- default:
- goto st22
- }
- goto tr6
- st22:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof22
- }
- stCase22:
- switch (m.data)[(m.p)] {
- case 45:
- goto st23
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st23
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st23
- }
- default:
- goto st23
- }
- goto tr6
- st23:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof23
- }
- stCase23:
- switch (m.data)[(m.p)] {
- case 45:
- goto st24
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st24
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st24
- }
- default:
- goto st24
- }
- goto tr6
- st24:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof24
- }
- stCase24:
- switch (m.data)[(m.p)] {
- case 45:
- goto st25
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st25
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st25
- }
- default:
- goto st25
- }
- goto tr6
- st25:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof25
- }
- stCase25:
- switch (m.data)[(m.p)] {
- case 45:
- goto st26
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st26
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st26
- }
- default:
- goto st26
- }
- goto tr6
- st26:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof26
- }
- stCase26:
- switch (m.data)[(m.p)] {
- case 45:
- goto st27
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st27
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st27
- }
- default:
- goto st27
- }
- goto tr6
- st27:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof27
- }
- stCase27:
- switch (m.data)[(m.p)] {
- case 45:
- goto st28
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st28
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st28
- }
- default:
- goto st28
- }
- goto tr6
- st28:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof28
- }
- stCase28:
- switch (m.data)[(m.p)] {
- case 45:
- goto st29
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st29
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st29
- }
- default:
- goto st29
- }
- goto tr6
- st29:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof29
- }
- stCase29:
- switch (m.data)[(m.p)] {
- case 45:
- goto st30
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st30
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st30
- }
- default:
- goto st30
- }
- goto tr6
- st30:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof30
- }
- stCase30:
- switch (m.data)[(m.p)] {
- case 45:
- goto st31
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st31
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st31
- }
- default:
- goto st31
- }
- goto tr6
- st31:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof31
- }
- stCase31:
- switch (m.data)[(m.p)] {
- case 45:
- goto st32
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st32
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st32
- }
- default:
- goto st32
- }
- goto tr6
- st32:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof32
- }
- stCase32:
- switch (m.data)[(m.p)] {
- case 45:
- goto st33
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st33
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st33
- }
- default:
- goto st33
- }
- goto tr6
- st33:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof33
- }
- stCase33:
- switch (m.data)[(m.p)] {
- case 45:
- goto st34
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st34
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st34
- }
- default:
- goto st34
- }
- goto tr6
- st34:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof34
- }
- stCase34:
- switch (m.data)[(m.p)] {
- case 45:
- goto st35
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st35
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st35
- }
- default:
- goto st35
- }
- goto tr6
- st35:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof35
- }
- stCase35:
- switch (m.data)[(m.p)] {
- case 45:
- goto st36
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st36
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st36
- }
- default:
- goto st36
- }
- goto tr6
- st36:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof36
- }
- stCase36:
- switch (m.data)[(m.p)] {
- case 45:
- goto st37
- case 58:
- goto tr10
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st37
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st37
- }
- default:
- goto st37
- }
- goto tr6
- st37:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof37
- }
- stCase37:
- if (m.data)[(m.p)] == 58 {
- goto tr10
- }
- goto tr6
- tr10:
-
- output.ID = string(m.text())
-
- goto st38
- st38:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof38
- }
- stCase38:
- switch (m.data)[(m.p)] {
- case 33:
- goto tr42
- case 36:
- goto tr42
- case 37:
- goto tr43
- case 61:
- goto tr42
- case 95:
- goto tr42
- }
- switch {
- case (m.data)[(m.p)] < 48:
- if 39 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 46 {
- goto tr42
- }
- case (m.data)[(m.p)] > 59:
- switch {
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto tr42
- }
- case (m.data)[(m.p)] >= 64:
- goto tr42
- }
- default:
- goto tr42
- }
- goto tr41
- tr42:
-
- m.pb = m.p
-
- goto st44
- st44:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof44
- }
- stCase44:
- switch (m.data)[(m.p)] {
- case 33:
- goto st44
- case 36:
- goto st44
- case 37:
- goto st39
- case 61:
- goto st44
- case 95:
- goto st44
- }
- switch {
- case (m.data)[(m.p)] < 48:
- if 39 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 46 {
- goto st44
- }
- case (m.data)[(m.p)] > 59:
- switch {
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st44
- }
- case (m.data)[(m.p)] >= 64:
- goto st44
- }
- default:
- goto st44
- }
- goto tr41
- tr43:
-
- m.pb = m.p
-
- goto st39
- st39:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof39
- }
- stCase39:
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st40
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st40
- }
- default:
- goto tr46
- }
- goto tr44
- tr46:
-
- m.tolower = append(m.tolower, m.p-m.pb)
-
- goto st40
- st40:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof40
- }
- stCase40:
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st45
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st45
- }
- default:
- goto tr48
- }
- goto tr44
- tr48:
-
- m.tolower = append(m.tolower, m.p-m.pb)
-
- goto st45
- st45:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof45
- }
- stCase45:
- switch (m.data)[(m.p)] {
- case 33:
- goto st44
- case 36:
- goto st44
- case 37:
- goto st39
- case 61:
- goto st44
- case 95:
- goto st44
- }
- switch {
- case (m.data)[(m.p)] < 48:
- if 39 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 46 {
- goto st44
- }
- case (m.data)[(m.p)] > 59:
- switch {
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st44
- }
- case (m.data)[(m.p)] >= 64:
- goto st44
- }
- default:
- goto st44
- }
- goto tr44
- tr8:
-
- m.pb = m.p
-
- goto st41
- st41:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof41
- }
- stCase41:
- switch (m.data)[(m.p)] {
- case 45:
- goto st7
- case 58:
- goto tr10
- case 82:
- goto st42
- case 114:
- goto st42
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st7
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st7
- }
- default:
- goto st7
- }
- goto tr6
- st42:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof42
- }
- stCase42:
- switch (m.data)[(m.p)] {
- case 45:
- goto st8
- case 58:
- goto tr10
- case 78:
- goto st43
- case 110:
- goto st43
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st8
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st8
- }
- default:
- goto st8
- }
- goto tr50
- st43:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof43
- }
- stCase43:
- if (m.data)[(m.p)] == 45 {
- goto st9
- }
- switch {
- case (m.data)[(m.p)] < 65:
- if 48 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 57 {
- goto st9
- }
- case (m.data)[(m.p)] > 90:
- if 97 <= (m.data)[(m.p)] && (m.data)[(m.p)] <= 122 {
- goto st9
- }
- default:
- goto st9
- }
- goto tr52
- st46:
- if (m.p)++; (m.p) == (m.pe) {
- goto _testEof46
- }
- stCase46:
- switch (m.data)[(m.p)] {
- case 10:
- goto st0
- case 13:
- goto st0
- }
- goto st46
- stOut:
- _testEof2:
- m.cs = 2
- goto _testEof
- _testEof3:
- m.cs = 3
- goto _testEof
- _testEof4:
- m.cs = 4
- goto _testEof
- _testEof5:
- m.cs = 5
- goto _testEof
- _testEof6:
- m.cs = 6
- goto _testEof
- _testEof7:
- m.cs = 7
- goto _testEof
- _testEof8:
- m.cs = 8
- goto _testEof
- _testEof9:
- m.cs = 9
- goto _testEof
- _testEof10:
- m.cs = 10
- goto _testEof
- _testEof11:
- m.cs = 11
- goto _testEof
- _testEof12:
- m.cs = 12
- goto _testEof
- _testEof13:
- m.cs = 13
- goto _testEof
- _testEof14:
- m.cs = 14
- goto _testEof
- _testEof15:
- m.cs = 15
- goto _testEof
- _testEof16:
- m.cs = 16
- goto _testEof
- _testEof17:
- m.cs = 17
- goto _testEof
- _testEof18:
- m.cs = 18
- goto _testEof
- _testEof19:
- m.cs = 19
- goto _testEof
- _testEof20:
- m.cs = 20
- goto _testEof
- _testEof21:
- m.cs = 21
- goto _testEof
- _testEof22:
- m.cs = 22
- goto _testEof
- _testEof23:
- m.cs = 23
- goto _testEof
- _testEof24:
- m.cs = 24
- goto _testEof
- _testEof25:
- m.cs = 25
- goto _testEof
- _testEof26:
- m.cs = 26
- goto _testEof
- _testEof27:
- m.cs = 27
- goto _testEof
- _testEof28:
- m.cs = 28
- goto _testEof
- _testEof29:
- m.cs = 29
- goto _testEof
- _testEof30:
- m.cs = 30
- goto _testEof
- _testEof31:
- m.cs = 31
- goto _testEof
- _testEof32:
- m.cs = 32
- goto _testEof
- _testEof33:
- m.cs = 33
- goto _testEof
- _testEof34:
- m.cs = 34
- goto _testEof
- _testEof35:
- m.cs = 35
- goto _testEof
- _testEof36:
- m.cs = 36
- goto _testEof
- _testEof37:
- m.cs = 37
- goto _testEof
- _testEof38:
- m.cs = 38
- goto _testEof
- _testEof44:
- m.cs = 44
- goto _testEof
- _testEof39:
- m.cs = 39
- goto _testEof
- _testEof40:
- m.cs = 40
- goto _testEof
- _testEof45:
- m.cs = 45
- goto _testEof
- _testEof41:
- m.cs = 41
- goto _testEof
- _testEof42:
- m.cs = 42
- goto _testEof
- _testEof43:
- m.cs = 43
- goto _testEof
- _testEof46:
- m.cs = 46
- goto _testEof
-
- _testEof:
- {
- }
- if (m.p) == (m.eof) {
- switch m.cs {
- case 44, 45:
-
- raw := m.text()
- output.SS = string(raw)
- // Iterate upper letters lowering them
- for _, i := range m.tolower {
- raw[i] = raw[i] + 32
- }
- output.norm = string(raw)
-
- case 1, 2, 4:
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- case 3:
-
- m.err = fmt.Errorf(errPrefix, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- case 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 41:
-
- m.err = fmt.Errorf(errIdentifier, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- case 38:
-
- m.err = fmt.Errorf(errSpecificString, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- case 42:
-
- m.err = fmt.Errorf(errPrefix, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errIdentifier, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- case 43:
-
- m.err = fmt.Errorf(errNoUrnWithinID, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errIdentifier, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- case 39, 40:
-
- m.err = fmt.Errorf(errHex, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errSpecificString, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- m.err = fmt.Errorf(errParse, m.p)
- (m.p)--
-
- {
- goto st46
- }
-
- }
- }
-
- _out:
- {
- }
- }
-
- if m.cs < firstFinal || m.cs == enFail {
- return nil, m.err
- }
-
- return output, nil
-}
diff --git a/vendor/github.com/leodido/go-urn/machine.go.rl b/vendor/github.com/leodido/go-urn/machine.go.rl
deleted file mode 100644
index 3bc05a65..00000000
--- a/vendor/github.com/leodido/go-urn/machine.go.rl
+++ /dev/null
@@ -1,159 +0,0 @@
-package urn
-
-import (
- "fmt"
-)
-
-var (
- errPrefix = "expecting the prefix to be the \"urn\" string (whatever case) [col %d]"
- errIdentifier = "expecting the identifier to be string (1..31 alnum chars, also containing dashes but not at its start) [col %d]"
- errSpecificString = "expecting the specific string to be a string containing alnum, hex, or others ([()+,-.:=@;$_!*']) chars [col %d]"
- errNoUrnWithinID = "expecting the identifier to not contain the \"urn\" reserved string [col %d]"
- errHex = "expecting the specific string hex chars to be well-formed (%%alnum{2}) [col %d]"
- errParse = "parsing error [col %d]"
-)
-
-%%{
-machine urn;
-
-# unsigned alphabet
-alphtype uint8;
-
-action mark {
- m.pb = m.p
-}
-
-action tolower {
- m.tolower = append(m.tolower, m.p - m.pb)
-}
-
-action set_pre {
- output.prefix = string(m.text())
-}
-
-action set_nid {
- output.ID = string(m.text())
-}
-
-action set_nss {
- raw := m.text()
- output.SS = string(raw)
- // Iterate upper letters lowering them
- for _, i := range m.tolower {
- raw[i] = raw[i] + 32
- }
- output.norm = string(raw)
-}
-
-action err_pre {
- m.err = fmt.Errorf(errPrefix, m.p)
- fhold;
- fgoto fail;
-}
-
-action err_nid {
- m.err = fmt.Errorf(errIdentifier, m.p)
- fhold;
- fgoto fail;
-}
-
-action err_nss {
- m.err = fmt.Errorf(errSpecificString, m.p)
- fhold;
- fgoto fail;
-}
-
-action err_urn {
- m.err = fmt.Errorf(errNoUrnWithinID, m.p)
- fhold;
- fgoto fail;
-}
-
-action err_hex {
- m.err = fmt.Errorf(errHex, m.p)
- fhold;
- fgoto fail;
-}
-
-action err_parse {
- m.err = fmt.Errorf(errParse, m.p)
- fhold;
- fgoto fail;
-}
-
-pre = ([uU][rR][nN] @err(err_pre)) >mark %set_pre;
-
-nid = (alnum >mark (alnum | '-'){0,31}) %set_nid;
-
-hex = '%' (digit | lower | upper >tolower){2} $err(err_hex);
-
-sss = (alnum | [()+,\-.:=@;$_!*']);
-
-nss = (sss | hex)+ $err(err_nss);
-
-fail := (any - [\n\r])* @err{ fgoto main; };
-
-main := (pre ':' (nid - pre %err(err_urn)) $err(err_nid) ':' nss >mark %set_nss) $err(err_parse);
-
-}%%
-
-%% write data noerror noprefix;
-
-// Machine is the interface representing the FSM
-type Machine interface {
- Error() error
- Parse(input []byte) (*URN, error)
-}
-
-type machine struct {
- data []byte
- cs int
- p, pe, eof, pb int
- err error
- tolower []int
-}
-
-// NewMachine creates a new FSM able to parse RFC 2141 strings.
-func NewMachine() Machine {
- m := &machine{}
-
- %% access m.;
- %% variable p m.p;
- %% variable pe m.pe;
- %% variable eof m.eof;
- %% variable data m.data;
-
- return m
-}
-
-// Err returns the error that occurred on the last call to Parse.
-//
-// If the result is nil, then the line was parsed successfully.
-func (m *machine) Error() error {
- return m.err
-}
-
-func (m *machine) text() []byte {
- return m.data[m.pb:m.p]
-}
-
-// Parse parses the input byte array as a RFC 2141 string.
-func (m *machine) Parse(input []byte) (*URN, error) {
- m.data = input
- m.p = 0
- m.pb = 0
- m.pe = len(input)
- m.eof = len(input)
- m.err = nil
- m.tolower = []int{}
- output := &URN{}
-
- %% write init;
- %% write exec;
-
- if m.cs < first_final || m.cs == en_fail {
- return nil, m.err
- }
-
- return output, nil
-}
diff --git a/vendor/github.com/leodido/go-urn/makefile b/vendor/github.com/leodido/go-urn/makefile
deleted file mode 100644
index 47026d50..00000000
--- a/vendor/github.com/leodido/go-urn/makefile
+++ /dev/null
@@ -1,39 +0,0 @@
-SHELL := /bin/bash
-
-build: machine.go
-
-images: docs/urn.png
-
-machine.go: machine.go.rl
- ragel -Z -G2 -e -o $@ $<
- @sed -i '/^\/\/line/d' $@
- @$(MAKE) -s file=$@ snake2camel
- @gofmt -w -s $@
-
-docs/urn.dot: machine.go.rl
- @mkdir -p docs
- ragel -Z -e -Vp $< -o $@
-
-docs/urn.png: docs/urn.dot
- dot $< -Tpng -o $@
-
-.PHONY: bench
-bench: *_test.go machine.go
- go test -bench=. -benchmem -benchtime=5s ./...
-
-.PHONY: tests
-tests: *_test.go machine.go
- go test -race -timeout 10s -coverprofile=coverage.out -covermode=atomic -v ./...
-
-.PHONY: clean
-clean:
- @rm -rf docs
- @rm -f machine.go
-
-.PHONY: snake2camel
-snake2camel:
- @awk -i inplace '{ \
- while ( match($$0, /(.*)([a-z]+[0-9]*)_([a-zA-Z0-9])(.*)/, cap) ) \
- $$0 = cap[1] cap[2] toupper(cap[3]) cap[4]; \
- print \
- }' $(file)
\ No newline at end of file
diff --git a/vendor/github.com/leodido/go-urn/urn.go b/vendor/github.com/leodido/go-urn/urn.go
deleted file mode 100644
index d51a6c91..00000000
--- a/vendor/github.com/leodido/go-urn/urn.go
+++ /dev/null
@@ -1,86 +0,0 @@
-package urn
-
-import (
- "encoding/json"
- "fmt"
- "strings"
-)
-
-const errInvalidURN = "invalid URN: %s"
-
-// URN represents an Uniform Resource Name.
-//
-// The general form represented is:
-//
-// urn::
-//
-// Details at https://tools.ietf.org/html/rfc2141.
-type URN struct {
- prefix string // Static prefix. Equal to "urn" when empty.
- ID string // Namespace identifier
- SS string // Namespace specific string
- norm string // Normalized namespace specific string
-}
-
-// Normalize turns the receiving URN into its norm version.
-//
-// Which means: lowercase prefix, lowercase namespace identifier, and immutate namespace specific string chars (except tokens which are lowercased).
-func (u *URN) Normalize() *URN {
- return &URN{
- prefix: "urn",
- ID: strings.ToLower(u.ID),
- SS: u.norm,
- }
-}
-
-// Equal checks the lexical equivalence of the current URN with another one.
-func (u *URN) Equal(x *URN) bool {
- return *u.Normalize() == *x.Normalize()
-}
-
-// String reassembles the URN into a valid URN string.
-//
-// This requires both ID and SS fields to be non-empty.
-// Otherwise it returns an empty string.
-//
-// Default URN prefix is "urn".
-func (u *URN) String() string {
- var res string
- if u.ID != "" && u.SS != "" {
- if u.prefix == "" {
- res += "urn"
- }
- res += u.prefix + ":" + u.ID + ":" + u.SS
- }
-
- return res
-}
-
-// Parse is responsible to create an URN instance from a byte array matching the correct URN syntax.
-func Parse(u []byte) (*URN, bool) {
- urn, err := NewMachine().Parse(u)
- if err != nil {
- return nil, false
- }
-
- return urn, true
-}
-
-// MarshalJSON marshals the URN to JSON string form (e.g. `"urn:oid:1.2.3.4"`).
-func (u URN) MarshalJSON() ([]byte, error) {
- return json.Marshal(u.String())
-}
-
-// MarshalJSON unmarshals a URN from JSON string form (e.g. `"urn:oid:1.2.3.4"`).
-func (u *URN) UnmarshalJSON(bytes []byte) error {
- var str string
- if err := json.Unmarshal(bytes, &str); err != nil {
- return err
- }
- if value, ok := Parse([]byte(str)); !ok {
- return fmt.Errorf(errInvalidURN, str)
- } else {
- *u = *value
- }
- return nil
-}
\ No newline at end of file
diff --git a/vendor/github.com/magiconair/properties/.gitignore b/vendor/github.com/magiconair/properties/.gitignore
deleted file mode 100644
index e7081ff5..00000000
--- a/vendor/github.com/magiconair/properties/.gitignore
+++ /dev/null
@@ -1,6 +0,0 @@
-*.sublime-project
-*.sublime-workspace
-*.un~
-*.swp
-.idea/
-*.iml
diff --git a/vendor/github.com/magiconair/properties/CHANGELOG.md b/vendor/github.com/magiconair/properties/CHANGELOG.md
deleted file mode 100644
index 842e8e24..00000000
--- a/vendor/github.com/magiconair/properties/CHANGELOG.md
+++ /dev/null
@@ -1,205 +0,0 @@
-## Changelog
-
-### [1.8.7](https://github.com/magiconair/properties/tree/v1.8.7) - 08 Dec 2022
-
- * [PR #65](https://github.com/magiconair/properties/pull/65): Speedup Merge
-
- Thanks to [@AdityaVallabh](https://github.com/AdityaVallabh) for the patch.
-
- * [PR #66](https://github.com/magiconair/properties/pull/66): use github actions
-
-### [1.8.6](https://github.com/magiconair/properties/tree/v1.8.6) - 23 Feb 2022
-
- * [PR #57](https://github.com/magiconair/properties/pull/57):Fix "unreachable code" lint error
-
- Thanks to [@ellie](https://github.com/ellie) for the patch.
-
- * [PR #63](https://github.com/magiconair/properties/pull/63): Make TestMustGetParsedDuration backwards compatible
-
- This patch ensures that the `TestMustGetParsedDuration` still works with `go1.3` to make the
- author happy until it affects real users.
-
- Thanks to [@maage](https://github.com/maage) for the patch.
-
-### [1.8.5](https://github.com/magiconair/properties/tree/v1.8.5) - 24 Mar 2021
-
- * [PR #55](https://github.com/magiconair/properties/pull/55): Fix: Encoding Bug in Comments
-
- When reading comments \ are loaded correctly, but when writing they are then
- replaced by \\. This leads to wrong comments when writing and reading multiple times.
-
- Thanks to [@doxsch](https://github.com/doxsch) for the patch.
-
-### [1.8.4](https://github.com/magiconair/properties/tree/v1.8.4) - 23 Sep 2020
-
- * [PR #50](https://github.com/magiconair/properties/pull/50): enhance error message for circular references
-
- Thanks to [@sriv](https://github.com/sriv) for the patch.
-
-### [1.8.3](https://github.com/magiconair/properties/tree/v1.8.3) - 14 Sep 2020
-
- * [PR #49](https://github.com/magiconair/properties/pull/49): Include the key in error message causing the circular reference
-
- The change is include the key in the error message which is causing the circular
- reference when parsing/loading the properties files.
-
- Thanks to [@haroon-sheikh](https://github.com/haroon-sheikh) for the patch.
-
-### [1.8.2](https://github.com/magiconair/properties/tree/v1.8.2) - 25 Aug 2020
-
- * [PR #36](https://github.com/magiconair/properties/pull/36): Escape backslash on write
-
- This patch ensures that backslashes are escaped on write. Existing applications which
- rely on the old behavior may need to be updated.
-
- Thanks to [@apesternikov](https://github.com/apesternikov) for the patch.
-
- * [PR #42](https://github.com/magiconair/properties/pull/42): Made Content-Type check whitespace agnostic in LoadURL()
-
- Thanks to [@aliras1](https://github.com/aliras1) for the patch.
-
- * [PR #41](https://github.com/magiconair/properties/pull/41): Make key/value separator configurable on Write()
-
- Thanks to [@mkjor](https://github.com/mkjor) for the patch.
-
- * [PR #40](https://github.com/magiconair/properties/pull/40): Add method to return a sorted list of keys
-
- Thanks to [@mkjor](https://github.com/mkjor) for the patch.
-
-### [1.8.1](https://github.com/magiconair/properties/tree/v1.8.1) - 10 May 2019
-
- * [PR #35](https://github.com/magiconair/properties/pull/35): Close body always after request
-
- This patch ensures that in `LoadURL` the response body is always closed.
-
- Thanks to [@liubog2008](https://github.com/liubog2008) for the patch.
-
-### [1.8](https://github.com/magiconair/properties/tree/v1.8) - 15 May 2018
-
- * [PR #26](https://github.com/magiconair/properties/pull/26): Disable expansion during loading
-
- This adds the option to disable property expansion during loading.
-
- Thanks to [@kmala](https://github.com/kmala) for the patch.
-
-### [1.7.6](https://github.com/magiconair/properties/tree/v1.7.6) - 14 Feb 2018
-
- * [PR #29](https://github.com/magiconair/properties/pull/29): Reworked expansion logic to handle more complex cases.
-
- See PR for an example.
-
- Thanks to [@yobert](https://github.com/yobert) for the fix.
-
-### [1.7.5](https://github.com/magiconair/properties/tree/v1.7.5) - 13 Feb 2018
-
- * [PR #28](https://github.com/magiconair/properties/pull/28): Support duplicate expansions in the same value
-
- Values which expand the same key multiple times (e.g. `key=${a} ${a}`) will no longer fail
- with a `circular reference error`.
-
- Thanks to [@yobert](https://github.com/yobert) for the fix.
-
-### [1.7.4](https://github.com/magiconair/properties/tree/v1.7.4) - 31 Oct 2017
-
- * [Issue #23](https://github.com/magiconair/properties/issues/23): Ignore blank lines with whitespaces
-
- * [PR #24](https://github.com/magiconair/properties/pull/24): Update keys when DisableExpansion is enabled
-
- Thanks to [@mgurov](https://github.com/mgurov) for the fix.
-
-### [1.7.3](https://github.com/magiconair/properties/tree/v1.7.3) - 10 Jul 2017
-
- * [Issue #17](https://github.com/magiconair/properties/issues/17): Add [SetValue()](http://godoc.org/github.com/magiconair/properties#Properties.SetValue) method to set values generically
- * [Issue #22](https://github.com/magiconair/properties/issues/22): Add [LoadMap()](http://godoc.org/github.com/magiconair/properties#LoadMap) function to load properties from a string map
-
-### [1.7.2](https://github.com/magiconair/properties/tree/v1.7.2) - 20 Mar 2017
-
- * [Issue #15](https://github.com/magiconair/properties/issues/15): Drop gocheck dependency
- * [PR #21](https://github.com/magiconair/properties/pull/21): Add [Map()](http://godoc.org/github.com/magiconair/properties#Properties.Map) and [FilterFunc()](http://godoc.org/github.com/magiconair/properties#Properties.FilterFunc)
-
-### [1.7.1](https://github.com/magiconair/properties/tree/v1.7.1) - 13 Jan 2017
-
- * [Issue #14](https://github.com/magiconair/properties/issues/14): Decouple TestLoadExpandedFile from `$USER`
- * [PR #12](https://github.com/magiconair/properties/pull/12): Load from files and URLs
- * [PR #16](https://github.com/magiconair/properties/pull/16): Keep gofmt happy
- * [PR #18](https://github.com/magiconair/properties/pull/18): Fix Delete() function
-
-### [1.7.0](https://github.com/magiconair/properties/tree/v1.7.0) - 20 Mar 2016
-
- * [Issue #10](https://github.com/magiconair/properties/issues/10): Add [LoadURL,LoadURLs,MustLoadURL,MustLoadURLs](http://godoc.org/github.com/magiconair/properties#LoadURL) method to load properties from a URL.
- * [Issue #11](https://github.com/magiconair/properties/issues/11): Add [LoadString,MustLoadString](http://godoc.org/github.com/magiconair/properties#LoadString) method to load properties from an UTF8 string.
- * [PR #8](https://github.com/magiconair/properties/pull/8): Add [MustFlag](http://godoc.org/github.com/magiconair/properties#Properties.MustFlag) method to provide overrides via command line flags. (@pascaldekloe)
-
-### [1.6.0](https://github.com/magiconair/properties/tree/v1.6.0) - 11 Dec 2015
-
- * Add [Decode](http://godoc.org/github.com/magiconair/properties#Properties.Decode) method to populate struct from properties via tags.
-
-### [1.5.6](https://github.com/magiconair/properties/tree/v1.5.6) - 18 Oct 2015
-
- * Vendored in gopkg.in/check.v1
-
-### [1.5.5](https://github.com/magiconair/properties/tree/v1.5.5) - 31 Jul 2015
-
- * [PR #6](https://github.com/magiconair/properties/pull/6): Add [Delete](http://godoc.org/github.com/magiconair/properties#Properties.Delete) method to remove keys including comments. (@gerbenjacobs)
-
-### [1.5.4](https://github.com/magiconair/properties/tree/v1.5.4) - 23 Jun 2015
-
- * [Issue #5](https://github.com/magiconair/properties/issues/5): Allow disabling of property expansion [DisableExpansion](http://godoc.org/github.com/magiconair/properties#Properties.DisableExpansion). When property expansion is disabled Properties become a simple key/value store and don't check for circular references.
-
-### [1.5.3](https://github.com/magiconair/properties/tree/v1.5.3) - 02 Jun 2015
-
- * [Issue #4](https://github.com/magiconair/properties/issues/4): Maintain key order in [Filter()](http://godoc.org/github.com/magiconair/properties#Properties.Filter), [FilterPrefix()](http://godoc.org/github.com/magiconair/properties#Properties.FilterPrefix) and [FilterRegexp()](http://godoc.org/github.com/magiconair/properties#Properties.FilterRegexp)
-
-### [1.5.2](https://github.com/magiconair/properties/tree/v1.5.2) - 10 Apr 2015
-
- * [Issue #3](https://github.com/magiconair/properties/issues/3): Don't print comments in [WriteComment()](http://godoc.org/github.com/magiconair/properties#Properties.WriteComment) if they are all empty
- * Add clickable links to README
-
-### [1.5.1](https://github.com/magiconair/properties/tree/v1.5.1) - 08 Dec 2014
-
- * Added [GetParsedDuration()](http://godoc.org/github.com/magiconair/properties#Properties.GetParsedDuration) and [MustGetParsedDuration()](http://godoc.org/github.com/magiconair/properties#Properties.MustGetParsedDuration) for values specified compatible with
- [time.ParseDuration()](http://golang.org/pkg/time/#ParseDuration).
-
-### [1.5.0](https://github.com/magiconair/properties/tree/v1.5.0) - 18 Nov 2014
-
- * Added support for single and multi-line comments (reading, writing and updating)
- * The order of keys is now preserved
- * Calling [Set()](http://godoc.org/github.com/magiconair/properties#Properties.Set) with an empty key now silently ignores the call and does not create a new entry
- * Added a [MustSet()](http://godoc.org/github.com/magiconair/properties#Properties.MustSet) method
- * Migrated test library from launchpad.net/gocheck to [gopkg.in/check.v1](http://gopkg.in/check.v1)
-
-### [1.4.2](https://github.com/magiconair/properties/tree/v1.4.2) - 15 Nov 2014
-
- * [Issue #2](https://github.com/magiconair/properties/issues/2): Fixed goroutine leak in parser which created two lexers but cleaned up only one
-
-### [1.4.1](https://github.com/magiconair/properties/tree/v1.4.1) - 13 Nov 2014
-
- * [Issue #1](https://github.com/magiconair/properties/issues/1): Fixed bug in Keys() method which returned an empty string
-
-### [1.4.0](https://github.com/magiconair/properties/tree/v1.4.0) - 23 Sep 2014
-
- * Added [Keys()](http://godoc.org/github.com/magiconair/properties#Properties.Keys) to get the keys
- * Added [Filter()](http://godoc.org/github.com/magiconair/properties#Properties.Filter), [FilterRegexp()](http://godoc.org/github.com/magiconair/properties#Properties.FilterRegexp) and [FilterPrefix()](http://godoc.org/github.com/magiconair/properties#Properties.FilterPrefix) to get a subset of the properties
-
-### [1.3.0](https://github.com/magiconair/properties/tree/v1.3.0) - 18 Mar 2014
-
-* Added support for time.Duration
-* Made MustXXX() failure beha[ior configurable (log.Fatal, panic](https://github.com/magiconair/properties/tree/vior configurable (log.Fatal, panic) - custom)
-* Changed default of MustXXX() failure from panic to log.Fatal
-
-### [1.2.0](https://github.com/magiconair/properties/tree/v1.2.0) - 05 Mar 2014
-
-* Added MustGet... functions
-* Added support for int and uint with range checks on 32 bit platforms
-
-### [1.1.0](https://github.com/magiconair/properties/tree/v1.1.0) - 20 Jan 2014
-
-* Renamed from goproperties to properties
-* Added support for expansion of environment vars in
- filenames and value expressions
-* Fixed bug where value expressions were not at the
- start of the string
-
-### [1.0.0](https://github.com/magiconair/properties/tree/v1.0.0) - 7 Jan 2014
-
-* Initial release
diff --git a/vendor/github.com/magiconair/properties/LICENSE.md b/vendor/github.com/magiconair/properties/LICENSE.md
deleted file mode 100644
index 79c87e3e..00000000
--- a/vendor/github.com/magiconair/properties/LICENSE.md
+++ /dev/null
@@ -1,24 +0,0 @@
-Copyright (c) 2013-2020, Frank Schroeder
-
-All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are met:
-
- * Redistributions of source code must retain the above copyright notice, this
- list of conditions and the following disclaimer.
-
- * Redistributions in binary form must reproduce the above copyright notice,
- this list of conditions and the following disclaimer in the documentation
- and/or other materials provided with the distribution.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
-ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
-WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
-ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
-(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
-LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
-ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
-SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/magiconair/properties/README.md b/vendor/github.com/magiconair/properties/README.md
deleted file mode 100644
index e2edda02..00000000
--- a/vendor/github.com/magiconair/properties/README.md
+++ /dev/null
@@ -1,128 +0,0 @@
-[](https://github.com/magiconair/properties/releases)
-[](https://travis-ci.org/magiconair/properties)
-[](https://raw.githubusercontent.com/magiconair/properties/master/LICENSE)
-[](http://godoc.org/github.com/magiconair/properties)
-
-# Overview
-
-#### Please run `git pull --tags` to update the tags. See [below](#updated-git-tags) why.
-
-properties is a Go library for reading and writing properties files.
-
-It supports reading from multiple files or URLs and Spring style recursive
-property expansion of expressions like `${key}` to their corresponding value.
-Value expressions can refer to other keys like in `${key}` or to environment
-variables like in `${USER}`. Filenames can also contain environment variables
-like in `/home/${USER}/myapp.properties`.
-
-Properties can be decoded into structs, maps, arrays and values through
-struct tags.
-
-Comments and the order of keys are preserved. Comments can be modified
-and can be written to the output.
-
-The properties library supports both ISO-8859-1 and UTF-8 encoded data.
-
-Starting from version 1.3.0 the behavior of the MustXXX() functions is
-configurable by providing a custom `ErrorHandler` function. The default has
-changed from `panic` to `log.Fatal` but this is configurable and custom
-error handling functions can be provided. See the package documentation for
-details.
-
-Read the full documentation on [](http://godoc.org/github.com/magiconair/properties)
-
-## Getting Started
-
-```go
-import (
- "flag"
- "github.com/magiconair/properties"
-)
-
-func main() {
- // init from a file
- p := properties.MustLoadFile("${HOME}/config.properties", properties.UTF8)
-
- // or multiple files
- p = properties.MustLoadFiles([]string{
- "${HOME}/config.properties",
- "${HOME}/config-${USER}.properties",
- }, properties.UTF8, true)
-
- // or from a map
- p = properties.LoadMap(map[string]string{"key": "value", "abc": "def"})
-
- // or from a string
- p = properties.MustLoadString("key=value\nabc=def")
-
- // or from a URL
- p = properties.MustLoadURL("http://host/path")
-
- // or from multiple URLs
- p = properties.MustLoadURL([]string{
- "http://host/config",
- "http://host/config-${USER}",
- }, true)
-
- // or from flags
- p.MustFlag(flag.CommandLine)
-
- // get values through getters
- host := p.MustGetString("host")
- port := p.GetInt("port", 8080)
-
- // or through Decode
- type Config struct {
- Host string `properties:"host"`
- Port int `properties:"port,default=9000"`
- Accept []string `properties:"accept,default=image/png;image;gif"`
- Timeout time.Duration `properties:"timeout,default=5s"`
- }
- var cfg Config
- if err := p.Decode(&cfg); err != nil {
- log.Fatal(err)
- }
-}
-
-```
-
-## Installation and Upgrade
-
-```
-$ go get -u github.com/magiconair/properties
-```
-
-## License
-
-2 clause BSD license. See [LICENSE](https://github.com/magiconair/properties/blob/master/LICENSE) file for details.
-
-## ToDo
-
-* Dump contents with passwords and secrets obscured
-
-## Updated Git tags
-
-#### 13 Feb 2018
-
-I realized that all of the git tags I had pushed before v1.7.5 were lightweight tags
-and I've only recently learned that this doesn't play well with `git describe` 😞
-
-I have replaced all lightweight tags with signed tags using this script which should
-retain the commit date, name and email address. Please run `git pull --tags` to update them.
-
-Worst case you have to reclone the repo.
-
-```shell
-#!/bin/bash
-tag=$1
-echo "Updating $tag"
-date=$(git show ${tag}^0 --format=%aD | head -1)
-email=$(git show ${tag}^0 --format=%aE | head -1)
-name=$(git show ${tag}^0 --format=%aN | head -1)
-GIT_COMMITTER_DATE="$date" GIT_COMMITTER_NAME="$name" GIT_COMMITTER_EMAIL="$email" git tag -s -f ${tag} ${tag}^0 -m ${tag}
-```
-
-I apologize for the inconvenience.
-
-Frank
-
diff --git a/vendor/github.com/magiconair/properties/decode.go b/vendor/github.com/magiconair/properties/decode.go
deleted file mode 100644
index 8e6aa441..00000000
--- a/vendor/github.com/magiconair/properties/decode.go
+++ /dev/null
@@ -1,289 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package properties
-
-import (
- "fmt"
- "reflect"
- "strconv"
- "strings"
- "time"
-)
-
-// Decode assigns property values to exported fields of a struct.
-//
-// Decode traverses v recursively and returns an error if a value cannot be
-// converted to the field type or a required value is missing for a field.
-//
-// The following type dependent decodings are used:
-//
-// String, boolean, numeric fields have the value of the property key assigned.
-// The property key name is the name of the field. A different key and a default
-// value can be set in the field's tag. Fields without default value are
-// required. If the value cannot be converted to the field type an error is
-// returned.
-//
-// time.Duration fields have the result of time.ParseDuration() assigned.
-//
-// time.Time fields have the vaule of time.Parse() assigned. The default layout
-// is time.RFC3339 but can be set in the field's tag.
-//
-// Arrays and slices of string, boolean, numeric, time.Duration and time.Time
-// fields have the value interpreted as a comma separated list of values. The
-// individual values are trimmed of whitespace and empty values are ignored. A
-// default value can be provided as a semicolon separated list in the field's
-// tag.
-//
-// Struct fields are decoded recursively using the field name plus "." as
-// prefix. The prefix (without dot) can be overridden in the field's tag.
-// Default values are not supported in the field's tag. Specify them on the
-// fields of the inner struct instead.
-//
-// Map fields must have a key of type string and are decoded recursively by
-// using the field's name plus ".' as prefix and the next element of the key
-// name as map key. The prefix (without dot) can be overridden in the field's
-// tag. Default values are not supported.
-//
-// Examples:
-//
-// // Field is ignored.
-// Field int `properties:"-"`
-//
-// // Field is assigned value of 'Field'.
-// Field int
-//
-// // Field is assigned value of 'myName'.
-// Field int `properties:"myName"`
-//
-// // Field is assigned value of key 'myName' and has a default
-// // value 15 if the key does not exist.
-// Field int `properties:"myName,default=15"`
-//
-// // Field is assigned value of key 'Field' and has a default
-// // value 15 if the key does not exist.
-// Field int `properties:",default=15"`
-//
-// // Field is assigned value of key 'date' and the date
-// // is in format 2006-01-02
-// Field time.Time `properties:"date,layout=2006-01-02"`
-//
-// // Field is assigned the non-empty and whitespace trimmed
-// // values of key 'Field' split by commas.
-// Field []string
-//
-// // Field is assigned the non-empty and whitespace trimmed
-// // values of key 'Field' split by commas and has a default
-// // value ["a", "b", "c"] if the key does not exist.
-// Field []string `properties:",default=a;b;c"`
-//
-// // Field is decoded recursively with "Field." as key prefix.
-// Field SomeStruct
-//
-// // Field is decoded recursively with "myName." as key prefix.
-// Field SomeStruct `properties:"myName"`
-//
-// // Field is decoded recursively with "Field." as key prefix
-// // and the next dotted element of the key as map key.
-// Field map[string]string
-//
-// // Field is decoded recursively with "myName." as key prefix
-// // and the next dotted element of the key as map key.
-// Field map[string]string `properties:"myName"`
-func (p *Properties) Decode(x interface{}) error {
- t, v := reflect.TypeOf(x), reflect.ValueOf(x)
- if t.Kind() != reflect.Ptr || v.Elem().Type().Kind() != reflect.Struct {
- return fmt.Errorf("not a pointer to struct: %s", t)
- }
- if err := dec(p, "", nil, nil, v); err != nil {
- return err
- }
- return nil
-}
-
-func dec(p *Properties, key string, def *string, opts map[string]string, v reflect.Value) error {
- t := v.Type()
-
- // value returns the property value for key or the default if provided.
- value := func() (string, error) {
- if val, ok := p.Get(key); ok {
- return val, nil
- }
- if def != nil {
- return *def, nil
- }
- return "", fmt.Errorf("missing required key %s", key)
- }
-
- // conv converts a string to a value of the given type.
- conv := func(s string, t reflect.Type) (val reflect.Value, err error) {
- var v interface{}
-
- switch {
- case isDuration(t):
- v, err = time.ParseDuration(s)
-
- case isTime(t):
- layout := opts["layout"]
- if layout == "" {
- layout = time.RFC3339
- }
- v, err = time.Parse(layout, s)
-
- case isBool(t):
- v, err = boolVal(s), nil
-
- case isString(t):
- v, err = s, nil
-
- case isFloat(t):
- v, err = strconv.ParseFloat(s, 64)
-
- case isInt(t):
- v, err = strconv.ParseInt(s, 10, 64)
-
- case isUint(t):
- v, err = strconv.ParseUint(s, 10, 64)
-
- default:
- return reflect.Zero(t), fmt.Errorf("unsupported type %s", t)
- }
- if err != nil {
- return reflect.Zero(t), err
- }
- return reflect.ValueOf(v).Convert(t), nil
- }
-
- // keydef returns the property key and the default value based on the
- // name of the struct field and the options in the tag.
- keydef := func(f reflect.StructField) (string, *string, map[string]string) {
- _key, _opts := parseTag(f.Tag.Get("properties"))
-
- var _def *string
- if d, ok := _opts["default"]; ok {
- _def = &d
- }
- if _key != "" {
- return _key, _def, _opts
- }
- return f.Name, _def, _opts
- }
-
- switch {
- case isDuration(t) || isTime(t) || isBool(t) || isString(t) || isFloat(t) || isInt(t) || isUint(t):
- s, err := value()
- if err != nil {
- return err
- }
- val, err := conv(s, t)
- if err != nil {
- return err
- }
- v.Set(val)
-
- case isPtr(t):
- return dec(p, key, def, opts, v.Elem())
-
- case isStruct(t):
- for i := 0; i < v.NumField(); i++ {
- fv := v.Field(i)
- fk, def, opts := keydef(t.Field(i))
- if !fv.CanSet() {
- return fmt.Errorf("cannot set %s", t.Field(i).Name)
- }
- if fk == "-" {
- continue
- }
- if key != "" {
- fk = key + "." + fk
- }
- if err := dec(p, fk, def, opts, fv); err != nil {
- return err
- }
- }
- return nil
-
- case isArray(t):
- val, err := value()
- if err != nil {
- return err
- }
- vals := split(val, ";")
- a := reflect.MakeSlice(t, 0, len(vals))
- for _, s := range vals {
- val, err := conv(s, t.Elem())
- if err != nil {
- return err
- }
- a = reflect.Append(a, val)
- }
- v.Set(a)
-
- case isMap(t):
- valT := t.Elem()
- m := reflect.MakeMap(t)
- for postfix := range p.FilterStripPrefix(key + ".").m {
- pp := strings.SplitN(postfix, ".", 2)
- mk, mv := pp[0], reflect.New(valT)
- if err := dec(p, key+"."+mk, nil, nil, mv); err != nil {
- return err
- }
- m.SetMapIndex(reflect.ValueOf(mk), mv.Elem())
- }
- v.Set(m)
-
- default:
- return fmt.Errorf("unsupported type %s", t)
- }
- return nil
-}
-
-// split splits a string on sep, trims whitespace of elements
-// and omits empty elements
-func split(s string, sep string) []string {
- var a []string
- for _, v := range strings.Split(s, sep) {
- if v = strings.TrimSpace(v); v != "" {
- a = append(a, v)
- }
- }
- return a
-}
-
-// parseTag parses a "key,k=v,k=v,..."
-func parseTag(tag string) (key string, opts map[string]string) {
- opts = map[string]string{}
- for i, s := range strings.Split(tag, ",") {
- if i == 0 {
- key = s
- continue
- }
-
- pp := strings.SplitN(s, "=", 2)
- if len(pp) == 1 {
- opts[pp[0]] = ""
- } else {
- opts[pp[0]] = pp[1]
- }
- }
- return key, opts
-}
-
-func isArray(t reflect.Type) bool { return t.Kind() == reflect.Array || t.Kind() == reflect.Slice }
-func isBool(t reflect.Type) bool { return t.Kind() == reflect.Bool }
-func isDuration(t reflect.Type) bool { return t == reflect.TypeOf(time.Second) }
-func isMap(t reflect.Type) bool { return t.Kind() == reflect.Map }
-func isPtr(t reflect.Type) bool { return t.Kind() == reflect.Ptr }
-func isString(t reflect.Type) bool { return t.Kind() == reflect.String }
-func isStruct(t reflect.Type) bool { return t.Kind() == reflect.Struct }
-func isTime(t reflect.Type) bool { return t == reflect.TypeOf(time.Time{}) }
-func isFloat(t reflect.Type) bool {
- return t.Kind() == reflect.Float32 || t.Kind() == reflect.Float64
-}
-func isInt(t reflect.Type) bool {
- return t.Kind() == reflect.Int || t.Kind() == reflect.Int8 || t.Kind() == reflect.Int16 || t.Kind() == reflect.Int32 || t.Kind() == reflect.Int64
-}
-func isUint(t reflect.Type) bool {
- return t.Kind() == reflect.Uint || t.Kind() == reflect.Uint8 || t.Kind() == reflect.Uint16 || t.Kind() == reflect.Uint32 || t.Kind() == reflect.Uint64
-}
diff --git a/vendor/github.com/magiconair/properties/doc.go b/vendor/github.com/magiconair/properties/doc.go
deleted file mode 100644
index 7c797931..00000000
--- a/vendor/github.com/magiconair/properties/doc.go
+++ /dev/null
@@ -1,155 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-// Package properties provides functions for reading and writing
-// ISO-8859-1 and UTF-8 encoded .properties files and has
-// support for recursive property expansion.
-//
-// Java properties files are ISO-8859-1 encoded and use Unicode
-// literals for characters outside the ISO character set. Unicode
-// literals can be used in UTF-8 encoded properties files but
-// aren't necessary.
-//
-// To load a single properties file use MustLoadFile():
-//
-// p := properties.MustLoadFile(filename, properties.UTF8)
-//
-// To load multiple properties files use MustLoadFiles()
-// which loads the files in the given order and merges the
-// result. Missing properties files can be ignored if the
-// 'ignoreMissing' flag is set to true.
-//
-// Filenames can contain environment variables which are expanded
-// before loading.
-//
-// f1 := "/etc/myapp/myapp.conf"
-// f2 := "/home/${USER}/myapp.conf"
-// p := MustLoadFiles([]string{f1, f2}, properties.UTF8, true)
-//
-// All of the different key/value delimiters ' ', ':' and '=' are
-// supported as well as the comment characters '!' and '#' and
-// multi-line values.
-//
-// ! this is a comment
-// # and so is this
-//
-// # the following expressions are equal
-// key value
-// key=value
-// key:value
-// key = value
-// key : value
-// key = val\
-// ue
-//
-// Properties stores all comments preceding a key and provides
-// GetComments() and SetComments() methods to retrieve and
-// update them. The convenience functions GetComment() and
-// SetComment() allow access to the last comment. The
-// WriteComment() method writes properties files including
-// the comments and with the keys in the original order.
-// This can be used for sanitizing properties files.
-//
-// Property expansion is recursive and circular references
-// and malformed expressions are not allowed and cause an
-// error. Expansion of environment variables is supported.
-//
-// # standard property
-// key = value
-//
-// # property expansion: key2 = value
-// key2 = ${key}
-//
-// # recursive expansion: key3 = value
-// key3 = ${key2}
-//
-// # circular reference (error)
-// key = ${key}
-//
-// # malformed expression (error)
-// key = ${ke
-//
-// # refers to the users' home dir
-// home = ${HOME}
-//
-// # local key takes precedence over env var: u = foo
-// USER = foo
-// u = ${USER}
-//
-// The default property expansion format is ${key} but can be
-// changed by setting different pre- and postfix values on the
-// Properties object.
-//
-// p := properties.NewProperties()
-// p.Prefix = "#["
-// p.Postfix = "]#"
-//
-// Properties provides convenience functions for getting typed
-// values with default values if the key does not exist or the
-// type conversion failed.
-//
-// # Returns true if the value is either "1", "on", "yes" or "true"
-// # Returns false for every other value and the default value if
-// # the key does not exist.
-// v = p.GetBool("key", false)
-//
-// # Returns the value if the key exists and the format conversion
-// # was successful. Otherwise, the default value is returned.
-// v = p.GetInt64("key", 999)
-// v = p.GetUint64("key", 999)
-// v = p.GetFloat64("key", 123.0)
-// v = p.GetString("key", "def")
-// v = p.GetDuration("key", 999)
-//
-// As an alternative properties may be applied with the standard
-// library's flag implementation at any time.
-//
-// # Standard configuration
-// v = flag.Int("key", 999, "help message")
-// flag.Parse()
-//
-// # Merge p into the flag set
-// p.MustFlag(flag.CommandLine)
-//
-// Properties provides several MustXXX() convenience functions
-// which will terminate the app if an error occurs. The behavior
-// of the failure is configurable and the default is to call
-// log.Fatal(err). To have the MustXXX() functions panic instead
-// of logging the error set a different ErrorHandler before
-// you use the Properties package.
-//
-// properties.ErrorHandler = properties.PanicHandler
-//
-// # Will panic instead of logging an error
-// p := properties.MustLoadFile("config.properties")
-//
-// You can also provide your own ErrorHandler function. The only requirement
-// is that the error handler function must exit after handling the error.
-//
-// properties.ErrorHandler = func(err error) {
-// fmt.Println(err)
-// os.Exit(1)
-// }
-//
-// # Will write to stdout and then exit
-// p := properties.MustLoadFile("config.properties")
-//
-// Properties can also be loaded into a struct via the `Decode`
-// method, e.g.
-//
-// type S struct {
-// A string `properties:"a,default=foo"`
-// D time.Duration `properties:"timeout,default=5s"`
-// E time.Time `properties:"expires,layout=2006-01-02,default=2015-01-01"`
-// }
-//
-// See `Decode()` method for the full documentation.
-//
-// The following documents provide a description of the properties
-// file format.
-//
-// http://en.wikipedia.org/wiki/.properties
-//
-// http://docs.oracle.com/javase/7/docs/api/java/util/Properties.html#load%28java.io.Reader%29
-package properties
diff --git a/vendor/github.com/magiconair/properties/integrate.go b/vendor/github.com/magiconair/properties/integrate.go
deleted file mode 100644
index 35d0ae97..00000000
--- a/vendor/github.com/magiconair/properties/integrate.go
+++ /dev/null
@@ -1,35 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package properties
-
-import "flag"
-
-// MustFlag sets flags that are skipped by dst.Parse when p contains
-// the respective key for flag.Flag.Name.
-//
-// It's use is recommended with command line arguments as in:
-//
-// flag.Parse()
-// p.MustFlag(flag.CommandLine)
-func (p *Properties) MustFlag(dst *flag.FlagSet) {
- m := make(map[string]*flag.Flag)
- dst.VisitAll(func(f *flag.Flag) {
- m[f.Name] = f
- })
- dst.Visit(func(f *flag.Flag) {
- delete(m, f.Name) // overridden
- })
-
- for name, f := range m {
- v, ok := p.Get(name)
- if !ok {
- continue
- }
-
- if err := f.Value.Set(v); err != nil {
- ErrorHandler(err)
- }
- }
-}
diff --git a/vendor/github.com/magiconair/properties/lex.go b/vendor/github.com/magiconair/properties/lex.go
deleted file mode 100644
index 3d15a1f6..00000000
--- a/vendor/github.com/magiconair/properties/lex.go
+++ /dev/null
@@ -1,395 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-//
-// Parts of the lexer are from the template/text/parser package
-// For these parts the following applies:
-//
-// Copyright 2011 The Go Authors. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file of the go 1.2
-// distribution.
-
-package properties
-
-import (
- "fmt"
- "strconv"
- "strings"
- "unicode/utf8"
-)
-
-// item represents a token or text string returned from the scanner.
-type item struct {
- typ itemType // The type of this item.
- pos int // The starting position, in bytes, of this item in the input string.
- val string // The value of this item.
-}
-
-func (i item) String() string {
- switch {
- case i.typ == itemEOF:
- return "EOF"
- case i.typ == itemError:
- return i.val
- case len(i.val) > 10:
- return fmt.Sprintf("%.10q...", i.val)
- }
- return fmt.Sprintf("%q", i.val)
-}
-
-// itemType identifies the type of lex items.
-type itemType int
-
-const (
- itemError itemType = iota // error occurred; value is text of error
- itemEOF
- itemKey // a key
- itemValue // a value
- itemComment // a comment
-)
-
-// defines a constant for EOF
-const eof = -1
-
-// permitted whitespace characters space, FF and TAB
-const whitespace = " \f\t"
-
-// stateFn represents the state of the scanner as a function that returns the next state.
-type stateFn func(*lexer) stateFn
-
-// lexer holds the state of the scanner.
-type lexer struct {
- input string // the string being scanned
- state stateFn // the next lexing function to enter
- pos int // current position in the input
- start int // start position of this item
- width int // width of last rune read from input
- lastPos int // position of most recent item returned by nextItem
- runes []rune // scanned runes for this item
- items chan item // channel of scanned items
-}
-
-// next returns the next rune in the input.
-func (l *lexer) next() rune {
- if l.pos >= len(l.input) {
- l.width = 0
- return eof
- }
- r, w := utf8.DecodeRuneInString(l.input[l.pos:])
- l.width = w
- l.pos += l.width
- return r
-}
-
-// peek returns but does not consume the next rune in the input.
-func (l *lexer) peek() rune {
- r := l.next()
- l.backup()
- return r
-}
-
-// backup steps back one rune. Can only be called once per call of next.
-func (l *lexer) backup() {
- l.pos -= l.width
-}
-
-// emit passes an item back to the client.
-func (l *lexer) emit(t itemType) {
- i := item{t, l.start, string(l.runes)}
- l.items <- i
- l.start = l.pos
- l.runes = l.runes[:0]
-}
-
-// ignore skips over the pending input before this point.
-func (l *lexer) ignore() {
- l.start = l.pos
-}
-
-// appends the rune to the current value
-func (l *lexer) appendRune(r rune) {
- l.runes = append(l.runes, r)
-}
-
-// accept consumes the next rune if it's from the valid set.
-func (l *lexer) accept(valid string) bool {
- if strings.ContainsRune(valid, l.next()) {
- return true
- }
- l.backup()
- return false
-}
-
-// acceptRun consumes a run of runes from the valid set.
-func (l *lexer) acceptRun(valid string) {
- for strings.ContainsRune(valid, l.next()) {
- }
- l.backup()
-}
-
-// lineNumber reports which line we're on, based on the position of
-// the previous item returned by nextItem. Doing it this way
-// means we don't have to worry about peek double counting.
-func (l *lexer) lineNumber() int {
- return 1 + strings.Count(l.input[:l.lastPos], "\n")
-}
-
-// errorf returns an error token and terminates the scan by passing
-// back a nil pointer that will be the next state, terminating l.nextItem.
-func (l *lexer) errorf(format string, args ...interface{}) stateFn {
- l.items <- item{itemError, l.start, fmt.Sprintf(format, args...)}
- return nil
-}
-
-// nextItem returns the next item from the input.
-func (l *lexer) nextItem() item {
- i := <-l.items
- l.lastPos = i.pos
- return i
-}
-
-// lex creates a new scanner for the input string.
-func lex(input string) *lexer {
- l := &lexer{
- input: input,
- items: make(chan item),
- runes: make([]rune, 0, 32),
- }
- go l.run()
- return l
-}
-
-// run runs the state machine for the lexer.
-func (l *lexer) run() {
- for l.state = lexBeforeKey(l); l.state != nil; {
- l.state = l.state(l)
- }
-}
-
-// state functions
-
-// lexBeforeKey scans until a key begins.
-func lexBeforeKey(l *lexer) stateFn {
- switch r := l.next(); {
- case isEOF(r):
- l.emit(itemEOF)
- return nil
-
- case isEOL(r):
- l.ignore()
- return lexBeforeKey
-
- case isComment(r):
- return lexComment
-
- case isWhitespace(r):
- l.ignore()
- return lexBeforeKey
-
- default:
- l.backup()
- return lexKey
- }
-}
-
-// lexComment scans a comment line. The comment character has already been scanned.
-func lexComment(l *lexer) stateFn {
- l.acceptRun(whitespace)
- l.ignore()
- for {
- switch r := l.next(); {
- case isEOF(r):
- l.ignore()
- l.emit(itemEOF)
- return nil
- case isEOL(r):
- l.emit(itemComment)
- return lexBeforeKey
- default:
- l.appendRune(r)
- }
- }
-}
-
-// lexKey scans the key up to a delimiter
-func lexKey(l *lexer) stateFn {
- var r rune
-
-Loop:
- for {
- switch r = l.next(); {
-
- case isEscape(r):
- err := l.scanEscapeSequence()
- if err != nil {
- return l.errorf(err.Error())
- }
-
- case isEndOfKey(r):
- l.backup()
- break Loop
-
- case isEOF(r):
- break Loop
-
- default:
- l.appendRune(r)
- }
- }
-
- if len(l.runes) > 0 {
- l.emit(itemKey)
- }
-
- if isEOF(r) {
- l.emit(itemEOF)
- return nil
- }
-
- return lexBeforeValue
-}
-
-// lexBeforeValue scans the delimiter between key and value.
-// Leading and trailing whitespace is ignored.
-// We expect to be just after the key.
-func lexBeforeValue(l *lexer) stateFn {
- l.acceptRun(whitespace)
- l.accept(":=")
- l.acceptRun(whitespace)
- l.ignore()
- return lexValue
-}
-
-// lexValue scans text until the end of the line. We expect to be just after the delimiter.
-func lexValue(l *lexer) stateFn {
- for {
- switch r := l.next(); {
- case isEscape(r):
- if isEOL(l.peek()) {
- l.next()
- l.acceptRun(whitespace)
- } else {
- err := l.scanEscapeSequence()
- if err != nil {
- return l.errorf(err.Error())
- }
- }
-
- case isEOL(r):
- l.emit(itemValue)
- l.ignore()
- return lexBeforeKey
-
- case isEOF(r):
- l.emit(itemValue)
- l.emit(itemEOF)
- return nil
-
- default:
- l.appendRune(r)
- }
- }
-}
-
-// scanEscapeSequence scans either one of the escaped characters
-// or a unicode literal. We expect to be after the escape character.
-func (l *lexer) scanEscapeSequence() error {
- switch r := l.next(); {
-
- case isEscapedCharacter(r):
- l.appendRune(decodeEscapedCharacter(r))
- return nil
-
- case atUnicodeLiteral(r):
- return l.scanUnicodeLiteral()
-
- case isEOF(r):
- return fmt.Errorf("premature EOF")
-
- // silently drop the escape character and append the rune as is
- default:
- l.appendRune(r)
- return nil
- }
-}
-
-// scans a unicode literal in the form \uXXXX. We expect to be after the \u.
-func (l *lexer) scanUnicodeLiteral() error {
- // scan the digits
- d := make([]rune, 4)
- for i := 0; i < 4; i++ {
- d[i] = l.next()
- if d[i] == eof || !strings.ContainsRune("0123456789abcdefABCDEF", d[i]) {
- return fmt.Errorf("invalid unicode literal")
- }
- }
-
- // decode the digits into a rune
- r, err := strconv.ParseInt(string(d), 16, 0)
- if err != nil {
- return err
- }
-
- l.appendRune(rune(r))
- return nil
-}
-
-// decodeEscapedCharacter returns the unescaped rune. We expect to be after the escape character.
-func decodeEscapedCharacter(r rune) rune {
- switch r {
- case 'f':
- return '\f'
- case 'n':
- return '\n'
- case 'r':
- return '\r'
- case 't':
- return '\t'
- default:
- return r
- }
-}
-
-// atUnicodeLiteral reports whether we are at a unicode literal.
-// The escape character has already been consumed.
-func atUnicodeLiteral(r rune) bool {
- return r == 'u'
-}
-
-// isComment reports whether we are at the start of a comment.
-func isComment(r rune) bool {
- return r == '#' || r == '!'
-}
-
-// isEndOfKey reports whether the rune terminates the current key.
-func isEndOfKey(r rune) bool {
- return strings.ContainsRune(" \f\t\r\n:=", r)
-}
-
-// isEOF reports whether we are at EOF.
-func isEOF(r rune) bool {
- return r == eof
-}
-
-// isEOL reports whether we are at a new line character.
-func isEOL(r rune) bool {
- return r == '\n' || r == '\r'
-}
-
-// isEscape reports whether the rune is the escape character which
-// prefixes unicode literals and other escaped characters.
-func isEscape(r rune) bool {
- return r == '\\'
-}
-
-// isEscapedCharacter reports whether we are at one of the characters that need escaping.
-// The escape character has already been consumed.
-func isEscapedCharacter(r rune) bool {
- return strings.ContainsRune(" :=fnrt", r)
-}
-
-// isWhitespace reports whether the rune is a whitespace character.
-func isWhitespace(r rune) bool {
- return strings.ContainsRune(whitespace, r)
-}
diff --git a/vendor/github.com/magiconair/properties/load.go b/vendor/github.com/magiconair/properties/load.go
deleted file mode 100644
index 635368dc..00000000
--- a/vendor/github.com/magiconair/properties/load.go
+++ /dev/null
@@ -1,293 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package properties
-
-import (
- "fmt"
- "io/ioutil"
- "net/http"
- "os"
- "strings"
-)
-
-// Encoding specifies encoding of the input data.
-type Encoding uint
-
-const (
- // utf8Default is a private placeholder for the zero value of Encoding to
- // ensure that it has the correct meaning. UTF8 is the default encoding but
- // was assigned a non-zero value which cannot be changed without breaking
- // existing code. Clients should continue to use the public constants.
- utf8Default Encoding = iota
-
- // UTF8 interprets the input data as UTF-8.
- UTF8
-
- // ISO_8859_1 interprets the input data as ISO-8859-1.
- ISO_8859_1
-)
-
-type Loader struct {
- // Encoding determines how the data from files and byte buffers
- // is interpreted. For URLs the Content-Type header is used
- // to determine the encoding of the data.
- Encoding Encoding
-
- // DisableExpansion configures the property expansion of the
- // returned property object. When set to true, the property values
- // will not be expanded and the Property object will not be checked
- // for invalid expansion expressions.
- DisableExpansion bool
-
- // IgnoreMissing configures whether missing files or URLs which return
- // 404 are reported as errors. When set to true, missing files and 404
- // status codes are not reported as errors.
- IgnoreMissing bool
-}
-
-// Load reads a buffer into a Properties struct.
-func (l *Loader) LoadBytes(buf []byte) (*Properties, error) {
- return l.loadBytes(buf, l.Encoding)
-}
-
-// LoadAll reads the content of multiple URLs or files in the given order into
-// a Properties struct. If IgnoreMissing is true then a 404 status code or
-// missing file will not be reported as error. Encoding sets the encoding for
-// files. For the URLs see LoadURL for the Content-Type header and the
-// encoding.
-func (l *Loader) LoadAll(names []string) (*Properties, error) {
- all := NewProperties()
- for _, name := range names {
- n, err := expandName(name)
- if err != nil {
- return nil, err
- }
-
- var p *Properties
- switch {
- case strings.HasPrefix(n, "http://"):
- p, err = l.LoadURL(n)
- case strings.HasPrefix(n, "https://"):
- p, err = l.LoadURL(n)
- default:
- p, err = l.LoadFile(n)
- }
- if err != nil {
- return nil, err
- }
- all.Merge(p)
- }
-
- all.DisableExpansion = l.DisableExpansion
- if all.DisableExpansion {
- return all, nil
- }
- return all, all.check()
-}
-
-// LoadFile reads a file into a Properties struct.
-// If IgnoreMissing is true then a missing file will not be
-// reported as error.
-func (l *Loader) LoadFile(filename string) (*Properties, error) {
- data, err := ioutil.ReadFile(filename)
- if err != nil {
- if l.IgnoreMissing && os.IsNotExist(err) {
- LogPrintf("properties: %s not found. skipping", filename)
- return NewProperties(), nil
- }
- return nil, err
- }
- return l.loadBytes(data, l.Encoding)
-}
-
-// LoadURL reads the content of the URL into a Properties struct.
-//
-// The encoding is determined via the Content-Type header which
-// should be set to 'text/plain'. If the 'charset' parameter is
-// missing, 'iso-8859-1' or 'latin1' the encoding is set to
-// ISO-8859-1. If the 'charset' parameter is set to 'utf-8' the
-// encoding is set to UTF-8. A missing content type header is
-// interpreted as 'text/plain; charset=utf-8'.
-func (l *Loader) LoadURL(url string) (*Properties, error) {
- resp, err := http.Get(url)
- if err != nil {
- return nil, fmt.Errorf("properties: error fetching %q. %s", url, err)
- }
- defer resp.Body.Close()
-
- if resp.StatusCode == 404 && l.IgnoreMissing {
- LogPrintf("properties: %s returned %d. skipping", url, resp.StatusCode)
- return NewProperties(), nil
- }
-
- if resp.StatusCode != 200 {
- return nil, fmt.Errorf("properties: %s returned %d", url, resp.StatusCode)
- }
-
- body, err := ioutil.ReadAll(resp.Body)
- if err != nil {
- return nil, fmt.Errorf("properties: %s error reading response. %s", url, err)
- }
-
- ct := resp.Header.Get("Content-Type")
- ct = strings.Join(strings.Fields(ct), "")
- var enc Encoding
- switch strings.ToLower(ct) {
- case "text/plain", "text/plain;charset=iso-8859-1", "text/plain;charset=latin1":
- enc = ISO_8859_1
- case "", "text/plain;charset=utf-8":
- enc = UTF8
- default:
- return nil, fmt.Errorf("properties: invalid content type %s", ct)
- }
-
- return l.loadBytes(body, enc)
-}
-
-func (l *Loader) loadBytes(buf []byte, enc Encoding) (*Properties, error) {
- p, err := parse(convert(buf, enc))
- if err != nil {
- return nil, err
- }
- p.DisableExpansion = l.DisableExpansion
- if p.DisableExpansion {
- return p, nil
- }
- return p, p.check()
-}
-
-// Load reads a buffer into a Properties struct.
-func Load(buf []byte, enc Encoding) (*Properties, error) {
- l := &Loader{Encoding: enc}
- return l.LoadBytes(buf)
-}
-
-// LoadString reads an UTF8 string into a properties struct.
-func LoadString(s string) (*Properties, error) {
- l := &Loader{Encoding: UTF8}
- return l.LoadBytes([]byte(s))
-}
-
-// LoadMap creates a new Properties struct from a string map.
-func LoadMap(m map[string]string) *Properties {
- p := NewProperties()
- for k, v := range m {
- p.Set(k, v)
- }
- return p
-}
-
-// LoadFile reads a file into a Properties struct.
-func LoadFile(filename string, enc Encoding) (*Properties, error) {
- l := &Loader{Encoding: enc}
- return l.LoadAll([]string{filename})
-}
-
-// LoadFiles reads multiple files in the given order into
-// a Properties struct. If 'ignoreMissing' is true then
-// non-existent files will not be reported as error.
-func LoadFiles(filenames []string, enc Encoding, ignoreMissing bool) (*Properties, error) {
- l := &Loader{Encoding: enc, IgnoreMissing: ignoreMissing}
- return l.LoadAll(filenames)
-}
-
-// LoadURL reads the content of the URL into a Properties struct.
-// See Loader#LoadURL for details.
-func LoadURL(url string) (*Properties, error) {
- l := &Loader{Encoding: UTF8}
- return l.LoadAll([]string{url})
-}
-
-// LoadURLs reads the content of multiple URLs in the given order into a
-// Properties struct. If IgnoreMissing is true then a 404 status code will
-// not be reported as error. See Loader#LoadURL for the Content-Type header
-// and the encoding.
-func LoadURLs(urls []string, ignoreMissing bool) (*Properties, error) {
- l := &Loader{Encoding: UTF8, IgnoreMissing: ignoreMissing}
- return l.LoadAll(urls)
-}
-
-// LoadAll reads the content of multiple URLs or files in the given order into a
-// Properties struct. If 'ignoreMissing' is true then a 404 status code or missing file will
-// not be reported as error. Encoding sets the encoding for files. For the URLs please see
-// LoadURL for the Content-Type header and the encoding.
-func LoadAll(names []string, enc Encoding, ignoreMissing bool) (*Properties, error) {
- l := &Loader{Encoding: enc, IgnoreMissing: ignoreMissing}
- return l.LoadAll(names)
-}
-
-// MustLoadString reads an UTF8 string into a Properties struct and
-// panics on error.
-func MustLoadString(s string) *Properties {
- return must(LoadString(s))
-}
-
-// MustLoadFile reads a file into a Properties struct and
-// panics on error.
-func MustLoadFile(filename string, enc Encoding) *Properties {
- return must(LoadFile(filename, enc))
-}
-
-// MustLoadFiles reads multiple files in the given order into
-// a Properties struct and panics on error. If 'ignoreMissing'
-// is true then non-existent files will not be reported as error.
-func MustLoadFiles(filenames []string, enc Encoding, ignoreMissing bool) *Properties {
- return must(LoadFiles(filenames, enc, ignoreMissing))
-}
-
-// MustLoadURL reads the content of a URL into a Properties struct and
-// panics on error.
-func MustLoadURL(url string) *Properties {
- return must(LoadURL(url))
-}
-
-// MustLoadURLs reads the content of multiple URLs in the given order into a
-// Properties struct and panics on error. If 'ignoreMissing' is true then a 404
-// status code will not be reported as error.
-func MustLoadURLs(urls []string, ignoreMissing bool) *Properties {
- return must(LoadURLs(urls, ignoreMissing))
-}
-
-// MustLoadAll reads the content of multiple URLs or files in the given order into a
-// Properties struct. If 'ignoreMissing' is true then a 404 status code or missing file will
-// not be reported as error. Encoding sets the encoding for files. For the URLs please see
-// LoadURL for the Content-Type header and the encoding. It panics on error.
-func MustLoadAll(names []string, enc Encoding, ignoreMissing bool) *Properties {
- return must(LoadAll(names, enc, ignoreMissing))
-}
-
-func must(p *Properties, err error) *Properties {
- if err != nil {
- ErrorHandler(err)
- }
- return p
-}
-
-// expandName expands ${ENV_VAR} expressions in a name.
-// If the environment variable does not exist then it will be replaced
-// with an empty string. Malformed expressions like "${ENV_VAR" will
-// be reported as error.
-func expandName(name string) (string, error) {
- return expand(name, []string{}, "${", "}", make(map[string]string))
-}
-
-// Interprets a byte buffer either as an ISO-8859-1 or UTF-8 encoded string.
-// For ISO-8859-1 we can convert each byte straight into a rune since the
-// first 256 unicode code points cover ISO-8859-1.
-func convert(buf []byte, enc Encoding) string {
- switch enc {
- case utf8Default, UTF8:
- return string(buf)
- case ISO_8859_1:
- runes := make([]rune, len(buf))
- for i, b := range buf {
- runes[i] = rune(b)
- }
- return string(runes)
- default:
- ErrorHandler(fmt.Errorf("unsupported encoding %v", enc))
- }
- panic("ErrorHandler should exit")
-}
diff --git a/vendor/github.com/magiconair/properties/parser.go b/vendor/github.com/magiconair/properties/parser.go
deleted file mode 100644
index fccfd39f..00000000
--- a/vendor/github.com/magiconair/properties/parser.go
+++ /dev/null
@@ -1,86 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package properties
-
-import (
- "fmt"
- "runtime"
-)
-
-type parser struct {
- lex *lexer
-}
-
-func parse(input string) (properties *Properties, err error) {
- p := &parser{lex: lex(input)}
- defer p.recover(&err)
-
- properties = NewProperties()
- key := ""
- comments := []string{}
-
- for {
- token := p.expectOneOf(itemComment, itemKey, itemEOF)
- switch token.typ {
- case itemEOF:
- goto done
- case itemComment:
- comments = append(comments, token.val)
- continue
- case itemKey:
- key = token.val
- if _, ok := properties.m[key]; !ok {
- properties.k = append(properties.k, key)
- }
- }
-
- token = p.expectOneOf(itemValue, itemEOF)
- if len(comments) > 0 {
- properties.c[key] = comments
- comments = []string{}
- }
- switch token.typ {
- case itemEOF:
- properties.m[key] = ""
- goto done
- case itemValue:
- properties.m[key] = token.val
- }
- }
-
-done:
- return properties, nil
-}
-
-func (p *parser) errorf(format string, args ...interface{}) {
- format = fmt.Sprintf("properties: Line %d: %s", p.lex.lineNumber(), format)
- panic(fmt.Errorf(format, args...))
-}
-
-func (p *parser) expectOneOf(expected ...itemType) (token item) {
- token = p.lex.nextItem()
- for _, v := range expected {
- if token.typ == v {
- return token
- }
- }
- p.unexpected(token)
- panic("unexpected token")
-}
-
-func (p *parser) unexpected(token item) {
- p.errorf(token.String())
-}
-
-// recover is the handler that turns panics into returns from the top level of Parse.
-func (p *parser) recover(errp *error) {
- e := recover()
- if e != nil {
- if _, ok := e.(runtime.Error); ok {
- panic(e)
- }
- *errp = e.(error)
- }
-}
diff --git a/vendor/github.com/magiconair/properties/properties.go b/vendor/github.com/magiconair/properties/properties.go
deleted file mode 100644
index fb2f7b40..00000000
--- a/vendor/github.com/magiconair/properties/properties.go
+++ /dev/null
@@ -1,848 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package properties
-
-// BUG(frank): Set() does not check for invalid unicode literals since this is currently handled by the lexer.
-// BUG(frank): Write() does not allow to configure the newline character. Therefore, on Windows LF is used.
-
-import (
- "bytes"
- "fmt"
- "io"
- "log"
- "os"
- "regexp"
- "sort"
- "strconv"
- "strings"
- "time"
- "unicode/utf8"
-)
-
-const maxExpansionDepth = 64
-
-// ErrorHandlerFunc defines the type of function which handles failures
-// of the MustXXX() functions. An error handler function must exit
-// the application after handling the error.
-type ErrorHandlerFunc func(error)
-
-// ErrorHandler is the function which handles failures of the MustXXX()
-// functions. The default is LogFatalHandler.
-var ErrorHandler ErrorHandlerFunc = LogFatalHandler
-
-// LogHandlerFunc defines the function prototype for logging errors.
-type LogHandlerFunc func(fmt string, args ...interface{})
-
-// LogPrintf defines a log handler which uses log.Printf.
-var LogPrintf LogHandlerFunc = log.Printf
-
-// LogFatalHandler handles the error by logging a fatal error and exiting.
-func LogFatalHandler(err error) {
- log.Fatal(err)
-}
-
-// PanicHandler handles the error by panicking.
-func PanicHandler(err error) {
- panic(err)
-}
-
-// -----------------------------------------------------------------------------
-
-// A Properties contains the key/value pairs from the properties input.
-// All values are stored in unexpanded form and are expanded at runtime
-type Properties struct {
- // Pre-/Postfix for property expansion.
- Prefix string
- Postfix string
-
- // DisableExpansion controls the expansion of properties on Get()
- // and the check for circular references on Set(). When set to
- // true Properties behaves like a simple key/value store and does
- // not check for circular references on Get() or on Set().
- DisableExpansion bool
-
- // Stores the key/value pairs
- m map[string]string
-
- // Stores the comments per key.
- c map[string][]string
-
- // Stores the keys in order of appearance.
- k []string
-
- // WriteSeparator specifies the separator of key and value while writing the properties.
- WriteSeparator string
-}
-
-// NewProperties creates a new Properties struct with the default
-// configuration for "${key}" expressions.
-func NewProperties() *Properties {
- return &Properties{
- Prefix: "${",
- Postfix: "}",
- m: map[string]string{},
- c: map[string][]string{},
- k: []string{},
- }
-}
-
-// Load reads a buffer into the given Properties struct.
-func (p *Properties) Load(buf []byte, enc Encoding) error {
- l := &Loader{Encoding: enc, DisableExpansion: p.DisableExpansion}
- newProperties, err := l.LoadBytes(buf)
- if err != nil {
- return err
- }
- p.Merge(newProperties)
- return nil
-}
-
-// Get returns the expanded value for the given key if exists.
-// Otherwise, ok is false.
-func (p *Properties) Get(key string) (value string, ok bool) {
- v, ok := p.m[key]
- if p.DisableExpansion {
- return v, ok
- }
- if !ok {
- return "", false
- }
-
- expanded, err := p.expand(key, v)
-
- // we guarantee that the expanded value is free of
- // circular references and malformed expressions
- // so we panic if we still get an error here.
- if err != nil {
- ErrorHandler(err)
- }
-
- return expanded, true
-}
-
-// MustGet returns the expanded value for the given key if exists.
-// Otherwise, it panics.
-func (p *Properties) MustGet(key string) string {
- if v, ok := p.Get(key); ok {
- return v
- }
- ErrorHandler(invalidKeyError(key))
- panic("ErrorHandler should exit")
-}
-
-// ----------------------------------------------------------------------------
-
-// ClearComments removes the comments for all keys.
-func (p *Properties) ClearComments() {
- p.c = map[string][]string{}
-}
-
-// ----------------------------------------------------------------------------
-
-// GetComment returns the last comment before the given key or an empty string.
-func (p *Properties) GetComment(key string) string {
- comments, ok := p.c[key]
- if !ok || len(comments) == 0 {
- return ""
- }
- return comments[len(comments)-1]
-}
-
-// ----------------------------------------------------------------------------
-
-// GetComments returns all comments that appeared before the given key or nil.
-func (p *Properties) GetComments(key string) []string {
- if comments, ok := p.c[key]; ok {
- return comments
- }
- return nil
-}
-
-// ----------------------------------------------------------------------------
-
-// SetComment sets the comment for the key.
-func (p *Properties) SetComment(key, comment string) {
- p.c[key] = []string{comment}
-}
-
-// ----------------------------------------------------------------------------
-
-// SetComments sets the comments for the key. If the comments are nil then
-// all comments for this key are deleted.
-func (p *Properties) SetComments(key string, comments []string) {
- if comments == nil {
- delete(p.c, key)
- return
- }
- p.c[key] = comments
-}
-
-// ----------------------------------------------------------------------------
-
-// GetBool checks if the expanded value is one of '1', 'yes',
-// 'true' or 'on' if the key exists. The comparison is case-insensitive.
-// If the key does not exist the default value is returned.
-func (p *Properties) GetBool(key string, def bool) bool {
- v, err := p.getBool(key)
- if err != nil {
- return def
- }
- return v
-}
-
-// MustGetBool checks if the expanded value is one of '1', 'yes',
-// 'true' or 'on' if the key exists. The comparison is case-insensitive.
-// If the key does not exist the function panics.
-func (p *Properties) MustGetBool(key string) bool {
- v, err := p.getBool(key)
- if err != nil {
- ErrorHandler(err)
- }
- return v
-}
-
-func (p *Properties) getBool(key string) (value bool, err error) {
- if v, ok := p.Get(key); ok {
- return boolVal(v), nil
- }
- return false, invalidKeyError(key)
-}
-
-func boolVal(v string) bool {
- v = strings.ToLower(v)
- return v == "1" || v == "true" || v == "yes" || v == "on"
-}
-
-// ----------------------------------------------------------------------------
-
-// GetDuration parses the expanded value as an time.Duration (in ns) if the
-// key exists. If key does not exist or the value cannot be parsed the default
-// value is returned. In almost all cases you want to use GetParsedDuration().
-func (p *Properties) GetDuration(key string, def time.Duration) time.Duration {
- v, err := p.getInt64(key)
- if err != nil {
- return def
- }
- return time.Duration(v)
-}
-
-// MustGetDuration parses the expanded value as an time.Duration (in ns) if
-// the key exists. If key does not exist or the value cannot be parsed the
-// function panics. In almost all cases you want to use MustGetParsedDuration().
-func (p *Properties) MustGetDuration(key string) time.Duration {
- v, err := p.getInt64(key)
- if err != nil {
- ErrorHandler(err)
- }
- return time.Duration(v)
-}
-
-// ----------------------------------------------------------------------------
-
-// GetParsedDuration parses the expanded value with time.ParseDuration() if the key exists.
-// If key does not exist or the value cannot be parsed the default
-// value is returned.
-func (p *Properties) GetParsedDuration(key string, def time.Duration) time.Duration {
- s, ok := p.Get(key)
- if !ok {
- return def
- }
- v, err := time.ParseDuration(s)
- if err != nil {
- return def
- }
- return v
-}
-
-// MustGetParsedDuration parses the expanded value with time.ParseDuration() if the key exists.
-// If key does not exist or the value cannot be parsed the function panics.
-func (p *Properties) MustGetParsedDuration(key string) time.Duration {
- s, ok := p.Get(key)
- if !ok {
- ErrorHandler(invalidKeyError(key))
- }
- v, err := time.ParseDuration(s)
- if err != nil {
- ErrorHandler(err)
- }
- return v
-}
-
-// ----------------------------------------------------------------------------
-
-// GetFloat64 parses the expanded value as a float64 if the key exists.
-// If key does not exist or the value cannot be parsed the default
-// value is returned.
-func (p *Properties) GetFloat64(key string, def float64) float64 {
- v, err := p.getFloat64(key)
- if err != nil {
- return def
- }
- return v
-}
-
-// MustGetFloat64 parses the expanded value as a float64 if the key exists.
-// If key does not exist or the value cannot be parsed the function panics.
-func (p *Properties) MustGetFloat64(key string) float64 {
- v, err := p.getFloat64(key)
- if err != nil {
- ErrorHandler(err)
- }
- return v
-}
-
-func (p *Properties) getFloat64(key string) (value float64, err error) {
- if v, ok := p.Get(key); ok {
- value, err = strconv.ParseFloat(v, 64)
- if err != nil {
- return 0, err
- }
- return value, nil
- }
- return 0, invalidKeyError(key)
-}
-
-// ----------------------------------------------------------------------------
-
-// GetInt parses the expanded value as an int if the key exists.
-// If key does not exist or the value cannot be parsed the default
-// value is returned. If the value does not fit into an int the
-// function panics with an out of range error.
-func (p *Properties) GetInt(key string, def int) int {
- v, err := p.getInt64(key)
- if err != nil {
- return def
- }
- return intRangeCheck(key, v)
-}
-
-// MustGetInt parses the expanded value as an int if the key exists.
-// If key does not exist or the value cannot be parsed the function panics.
-// If the value does not fit into an int the function panics with
-// an out of range error.
-func (p *Properties) MustGetInt(key string) int {
- v, err := p.getInt64(key)
- if err != nil {
- ErrorHandler(err)
- }
- return intRangeCheck(key, v)
-}
-
-// ----------------------------------------------------------------------------
-
-// GetInt64 parses the expanded value as an int64 if the key exists.
-// If key does not exist or the value cannot be parsed the default
-// value is returned.
-func (p *Properties) GetInt64(key string, def int64) int64 {
- v, err := p.getInt64(key)
- if err != nil {
- return def
- }
- return v
-}
-
-// MustGetInt64 parses the expanded value as an int if the key exists.
-// If key does not exist or the value cannot be parsed the function panics.
-func (p *Properties) MustGetInt64(key string) int64 {
- v, err := p.getInt64(key)
- if err != nil {
- ErrorHandler(err)
- }
- return v
-}
-
-func (p *Properties) getInt64(key string) (value int64, err error) {
- if v, ok := p.Get(key); ok {
- value, err = strconv.ParseInt(v, 10, 64)
- if err != nil {
- return 0, err
- }
- return value, nil
- }
- return 0, invalidKeyError(key)
-}
-
-// ----------------------------------------------------------------------------
-
-// GetUint parses the expanded value as an uint if the key exists.
-// If key does not exist or the value cannot be parsed the default
-// value is returned. If the value does not fit into an int the
-// function panics with an out of range error.
-func (p *Properties) GetUint(key string, def uint) uint {
- v, err := p.getUint64(key)
- if err != nil {
- return def
- }
- return uintRangeCheck(key, v)
-}
-
-// MustGetUint parses the expanded value as an int if the key exists.
-// If key does not exist or the value cannot be parsed the function panics.
-// If the value does not fit into an int the function panics with
-// an out of range error.
-func (p *Properties) MustGetUint(key string) uint {
- v, err := p.getUint64(key)
- if err != nil {
- ErrorHandler(err)
- }
- return uintRangeCheck(key, v)
-}
-
-// ----------------------------------------------------------------------------
-
-// GetUint64 parses the expanded value as an uint64 if the key exists.
-// If key does not exist or the value cannot be parsed the default
-// value is returned.
-func (p *Properties) GetUint64(key string, def uint64) uint64 {
- v, err := p.getUint64(key)
- if err != nil {
- return def
- }
- return v
-}
-
-// MustGetUint64 parses the expanded value as an int if the key exists.
-// If key does not exist or the value cannot be parsed the function panics.
-func (p *Properties) MustGetUint64(key string) uint64 {
- v, err := p.getUint64(key)
- if err != nil {
- ErrorHandler(err)
- }
- return v
-}
-
-func (p *Properties) getUint64(key string) (value uint64, err error) {
- if v, ok := p.Get(key); ok {
- value, err = strconv.ParseUint(v, 10, 64)
- if err != nil {
- return 0, err
- }
- return value, nil
- }
- return 0, invalidKeyError(key)
-}
-
-// ----------------------------------------------------------------------------
-
-// GetString returns the expanded value for the given key if exists or
-// the default value otherwise.
-func (p *Properties) GetString(key, def string) string {
- if v, ok := p.Get(key); ok {
- return v
- }
- return def
-}
-
-// MustGetString returns the expanded value for the given key if exists or
-// panics otherwise.
-func (p *Properties) MustGetString(key string) string {
- if v, ok := p.Get(key); ok {
- return v
- }
- ErrorHandler(invalidKeyError(key))
- panic("ErrorHandler should exit")
-}
-
-// ----------------------------------------------------------------------------
-
-// Filter returns a new properties object which contains all properties
-// for which the key matches the pattern.
-func (p *Properties) Filter(pattern string) (*Properties, error) {
- re, err := regexp.Compile(pattern)
- if err != nil {
- return nil, err
- }
-
- return p.FilterRegexp(re), nil
-}
-
-// FilterRegexp returns a new properties object which contains all properties
-// for which the key matches the regular expression.
-func (p *Properties) FilterRegexp(re *regexp.Regexp) *Properties {
- pp := NewProperties()
- for _, k := range p.k {
- if re.MatchString(k) {
- // TODO(fs): we are ignoring the error which flags a circular reference.
- // TODO(fs): since we are just copying a subset of keys this cannot happen (fingers crossed)
- pp.Set(k, p.m[k])
- }
- }
- return pp
-}
-
-// FilterPrefix returns a new properties object with a subset of all keys
-// with the given prefix.
-func (p *Properties) FilterPrefix(prefix string) *Properties {
- pp := NewProperties()
- for _, k := range p.k {
- if strings.HasPrefix(k, prefix) {
- // TODO(fs): we are ignoring the error which flags a circular reference.
- // TODO(fs): since we are just copying a subset of keys this cannot happen (fingers crossed)
- pp.Set(k, p.m[k])
- }
- }
- return pp
-}
-
-// FilterStripPrefix returns a new properties object with a subset of all keys
-// with the given prefix and the prefix removed from the keys.
-func (p *Properties) FilterStripPrefix(prefix string) *Properties {
- pp := NewProperties()
- n := len(prefix)
- for _, k := range p.k {
- if len(k) > len(prefix) && strings.HasPrefix(k, prefix) {
- // TODO(fs): we are ignoring the error which flags a circular reference.
- // TODO(fs): since we are modifying keys I am not entirely sure whether we can create a circular reference
- // TODO(fs): this function should probably return an error but the signature is fixed
- pp.Set(k[n:], p.m[k])
- }
- }
- return pp
-}
-
-// Len returns the number of keys.
-func (p *Properties) Len() int {
- return len(p.m)
-}
-
-// Keys returns all keys in the same order as in the input.
-func (p *Properties) Keys() []string {
- keys := make([]string, len(p.k))
- copy(keys, p.k)
- return keys
-}
-
-// Set sets the property key to the corresponding value.
-// If a value for key existed before then ok is true and prev
-// contains the previous value. If the value contains a
-// circular reference or a malformed expression then
-// an error is returned.
-// An empty key is silently ignored.
-func (p *Properties) Set(key, value string) (prev string, ok bool, err error) {
- if key == "" {
- return "", false, nil
- }
-
- // if expansion is disabled we allow circular references
- if p.DisableExpansion {
- prev, ok = p.Get(key)
- p.m[key] = value
- if !ok {
- p.k = append(p.k, key)
- }
- return prev, ok, nil
- }
-
- // to check for a circular reference we temporarily need
- // to set the new value. If there is an error then revert
- // to the previous state. Only if all tests are successful
- // then we add the key to the p.k list.
- prev, ok = p.Get(key)
- p.m[key] = value
-
- // now check for a circular reference
- _, err = p.expand(key, value)
- if err != nil {
-
- // revert to the previous state
- if ok {
- p.m[key] = prev
- } else {
- delete(p.m, key)
- }
-
- return "", false, err
- }
-
- if !ok {
- p.k = append(p.k, key)
- }
-
- return prev, ok, nil
-}
-
-// SetValue sets property key to the default string value
-// as defined by fmt.Sprintf("%v").
-func (p *Properties) SetValue(key string, value interface{}) error {
- _, _, err := p.Set(key, fmt.Sprintf("%v", value))
- return err
-}
-
-// MustSet sets the property key to the corresponding value.
-// If a value for key existed before then ok is true and prev
-// contains the previous value. An empty key is silently ignored.
-func (p *Properties) MustSet(key, value string) (prev string, ok bool) {
- prev, ok, err := p.Set(key, value)
- if err != nil {
- ErrorHandler(err)
- }
- return prev, ok
-}
-
-// String returns a string of all expanded 'key = value' pairs.
-func (p *Properties) String() string {
- var s string
- for _, key := range p.k {
- value, _ := p.Get(key)
- s = fmt.Sprintf("%s%s = %s\n", s, key, value)
- }
- return s
-}
-
-// Sort sorts the properties keys in alphabetical order.
-// This is helpfully before writing the properties.
-func (p *Properties) Sort() {
- sort.Strings(p.k)
-}
-
-// Write writes all unexpanded 'key = value' pairs to the given writer.
-// Write returns the number of bytes written and any write error encountered.
-func (p *Properties) Write(w io.Writer, enc Encoding) (n int, err error) {
- return p.WriteComment(w, "", enc)
-}
-
-// WriteComment writes all unexpanced 'key = value' pairs to the given writer.
-// If prefix is not empty then comments are written with a blank line and the
-// given prefix. The prefix should be either "# " or "! " to be compatible with
-// the properties file format. Otherwise, the properties parser will not be
-// able to read the file back in. It returns the number of bytes written and
-// any write error encountered.
-func (p *Properties) WriteComment(w io.Writer, prefix string, enc Encoding) (n int, err error) {
- var x int
-
- for _, key := range p.k {
- value := p.m[key]
-
- if prefix != "" {
- if comments, ok := p.c[key]; ok {
- // don't print comments if they are all empty
- allEmpty := true
- for _, c := range comments {
- if c != "" {
- allEmpty = false
- break
- }
- }
-
- if !allEmpty {
- // add a blank line between entries but not at the top
- if len(comments) > 0 && n > 0 {
- x, err = fmt.Fprintln(w)
- if err != nil {
- return
- }
- n += x
- }
-
- for _, c := range comments {
- x, err = fmt.Fprintf(w, "%s%s\n", prefix, c)
- if err != nil {
- return
- }
- n += x
- }
- }
- }
- }
- sep := " = "
- if p.WriteSeparator != "" {
- sep = p.WriteSeparator
- }
- x, err = fmt.Fprintf(w, "%s%s%s\n", encode(key, " :", enc), sep, encode(value, "", enc))
- if err != nil {
- return
- }
- n += x
- }
- return
-}
-
-// Map returns a copy of the properties as a map.
-func (p *Properties) Map() map[string]string {
- m := make(map[string]string)
- for k, v := range p.m {
- m[k] = v
- }
- return m
-}
-
-// FilterFunc returns a copy of the properties which includes the values which passed all filters.
-func (p *Properties) FilterFunc(filters ...func(k, v string) bool) *Properties {
- pp := NewProperties()
-outer:
- for k, v := range p.m {
- for _, f := range filters {
- if !f(k, v) {
- continue outer
- }
- pp.Set(k, v)
- }
- }
- return pp
-}
-
-// ----------------------------------------------------------------------------
-
-// Delete removes the key and its comments.
-func (p *Properties) Delete(key string) {
- delete(p.m, key)
- delete(p.c, key)
- newKeys := []string{}
- for _, k := range p.k {
- if k != key {
- newKeys = append(newKeys, k)
- }
- }
- p.k = newKeys
-}
-
-// Merge merges properties, comments and keys from other *Properties into p
-func (p *Properties) Merge(other *Properties) {
- for _, k := range other.k {
- if _, ok := p.m[k]; !ok {
- p.k = append(p.k, k)
- }
- }
- for k, v := range other.m {
- p.m[k] = v
- }
- for k, v := range other.c {
- p.c[k] = v
- }
-}
-
-// ----------------------------------------------------------------------------
-
-// check expands all values and returns an error if a circular reference or
-// a malformed expression was found.
-func (p *Properties) check() error {
- for key, value := range p.m {
- if _, err := p.expand(key, value); err != nil {
- return err
- }
- }
- return nil
-}
-
-func (p *Properties) expand(key, input string) (string, error) {
- // no pre/postfix -> nothing to expand
- if p.Prefix == "" && p.Postfix == "" {
- return input, nil
- }
-
- return expand(input, []string{key}, p.Prefix, p.Postfix, p.m)
-}
-
-// expand recursively expands expressions of '(prefix)key(postfix)' to their corresponding values.
-// The function keeps track of the keys that were already expanded and stops if it
-// detects a circular reference or a malformed expression of the form '(prefix)key'.
-func expand(s string, keys []string, prefix, postfix string, values map[string]string) (string, error) {
- if len(keys) > maxExpansionDepth {
- return "", fmt.Errorf("expansion too deep")
- }
-
- for {
- start := strings.Index(s, prefix)
- if start == -1 {
- return s, nil
- }
-
- keyStart := start + len(prefix)
- keyLen := strings.Index(s[keyStart:], postfix)
- if keyLen == -1 {
- return "", fmt.Errorf("malformed expression")
- }
-
- end := keyStart + keyLen + len(postfix) - 1
- key := s[keyStart : keyStart+keyLen]
-
- // fmt.Printf("s:%q pp:%q start:%d end:%d keyStart:%d keyLen:%d key:%q\n", s, prefix + "..." + postfix, start, end, keyStart, keyLen, key)
-
- for _, k := range keys {
- if key == k {
- var b bytes.Buffer
- b.WriteString("circular reference in:\n")
- for _, k1 := range keys {
- fmt.Fprintf(&b, "%s=%s\n", k1, values[k1])
- }
- return "", fmt.Errorf(b.String())
- }
- }
-
- val, ok := values[key]
- if !ok {
- val = os.Getenv(key)
- }
- new_val, err := expand(val, append(keys, key), prefix, postfix, values)
- if err != nil {
- return "", err
- }
- s = s[:start] + new_val + s[end+1:]
- }
-}
-
-// encode encodes a UTF-8 string to ISO-8859-1 and escapes some characters.
-func encode(s string, special string, enc Encoding) string {
- switch enc {
- case UTF8:
- return encodeUtf8(s, special)
- case ISO_8859_1:
- return encodeIso(s, special)
- default:
- panic(fmt.Sprintf("unsupported encoding %v", enc))
- }
-}
-
-func encodeUtf8(s string, special string) string {
- v := ""
- for pos := 0; pos < len(s); {
- r, w := utf8.DecodeRuneInString(s[pos:])
- pos += w
- v += escape(r, special)
- }
- return v
-}
-
-func encodeIso(s string, special string) string {
- var r rune
- var w int
- var v string
- for pos := 0; pos < len(s); {
- switch r, w = utf8.DecodeRuneInString(s[pos:]); {
- case r < 1<<8: // single byte rune -> escape special chars only
- v += escape(r, special)
- case r < 1<<16: // two byte rune -> unicode literal
- v += fmt.Sprintf("\\u%04x", r)
- default: // more than two bytes per rune -> can't encode
- v += "?"
- }
- pos += w
- }
- return v
-}
-
-func escape(r rune, special string) string {
- switch r {
- case '\f':
- return "\\f"
- case '\n':
- return "\\n"
- case '\r':
- return "\\r"
- case '\t':
- return "\\t"
- case '\\':
- return "\\\\"
- default:
- if strings.ContainsRune(special, r) {
- return "\\" + string(r)
- }
- return string(r)
- }
-}
-
-func invalidKeyError(key string) error {
- return fmt.Errorf("unknown property: %s", key)
-}
diff --git a/vendor/github.com/magiconair/properties/rangecheck.go b/vendor/github.com/magiconair/properties/rangecheck.go
deleted file mode 100644
index dbd60b36..00000000
--- a/vendor/github.com/magiconair/properties/rangecheck.go
+++ /dev/null
@@ -1,31 +0,0 @@
-// Copyright 2013-2022 Frank Schroeder. All rights reserved.
-// Use of this source code is governed by a BSD-style
-// license that can be found in the LICENSE file.
-
-package properties
-
-import (
- "fmt"
- "math"
-)
-
-// make this a var to overwrite it in a test
-var is32Bit = ^uint(0) == math.MaxUint32
-
-// intRangeCheck checks if the value fits into the int type and
-// panics if it does not.
-func intRangeCheck(key string, v int64) int {
- if is32Bit && (v < math.MinInt32 || v > math.MaxInt32) {
- panic(fmt.Sprintf("Value %d for key %s out of range", v, key))
- }
- return int(v)
-}
-
-// uintRangeCheck checks if the value fits into the uint type and
-// panics if it does not.
-func uintRangeCheck(key string, v uint64) uint {
- if is32Bit && v > math.MaxUint32 {
- panic(fmt.Sprintf("Value %d for key %s out of range", v, key))
- }
- return uint(v)
-}
diff --git a/vendor/github.com/mailru/easyjson/LICENSE b/vendor/github.com/mailru/easyjson/LICENSE
deleted file mode 100644
index fbff658f..00000000
--- a/vendor/github.com/mailru/easyjson/LICENSE
+++ /dev/null
@@ -1,7 +0,0 @@
-Copyright (c) 2016 Mail.Ru Group
-
-Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/vendor/github.com/mailru/easyjson/buffer/pool.go b/vendor/github.com/mailru/easyjson/buffer/pool.go
deleted file mode 100644
index 598a54af..00000000
--- a/vendor/github.com/mailru/easyjson/buffer/pool.go
+++ /dev/null
@@ -1,278 +0,0 @@
-// Package buffer implements a buffer for serialization, consisting of a chain of []byte-s to
-// reduce copying and to allow reuse of individual chunks.
-package buffer
-
-import (
- "io"
- "net"
- "sync"
-)
-
-// PoolConfig contains configuration for the allocation and reuse strategy.
-type PoolConfig struct {
- StartSize int // Minimum chunk size that is allocated.
- PooledSize int // Minimum chunk size that is reused, reusing chunks too small will result in overhead.
- MaxSize int // Maximum chunk size that will be allocated.
-}
-
-var config = PoolConfig{
- StartSize: 128,
- PooledSize: 512,
- MaxSize: 32768,
-}
-
-// Reuse pool: chunk size -> pool.
-var buffers = map[int]*sync.Pool{}
-
-func initBuffers() {
- for l := config.PooledSize; l <= config.MaxSize; l *= 2 {
- buffers[l] = new(sync.Pool)
- }
-}
-
-func init() {
- initBuffers()
-}
-
-// Init sets up a non-default pooling and allocation strategy. Should be run before serialization is done.
-func Init(cfg PoolConfig) {
- config = cfg
- initBuffers()
-}
-
-// putBuf puts a chunk to reuse pool if it can be reused.
-func putBuf(buf []byte) {
- size := cap(buf)
- if size < config.PooledSize {
- return
- }
- if c := buffers[size]; c != nil {
- c.Put(buf[:0])
- }
-}
-
-// getBuf gets a chunk from reuse pool or creates a new one if reuse failed.
-func getBuf(size int) []byte {
- if size >= config.PooledSize {
- if c := buffers[size]; c != nil {
- v := c.Get()
- if v != nil {
- return v.([]byte)
- }
- }
- }
- return make([]byte, 0, size)
-}
-
-// Buffer is a buffer optimized for serialization without extra copying.
-type Buffer struct {
-
- // Buf is the current chunk that can be used for serialization.
- Buf []byte
-
- toPool []byte
- bufs [][]byte
-}
-
-// EnsureSpace makes sure that the current chunk contains at least s free bytes,
-// possibly creating a new chunk.
-func (b *Buffer) EnsureSpace(s int) {
- if cap(b.Buf)-len(b.Buf) < s {
- b.ensureSpaceSlow(s)
- }
-}
-
-func (b *Buffer) ensureSpaceSlow(s int) {
- l := len(b.Buf)
- if l > 0 {
- if cap(b.toPool) != cap(b.Buf) {
- // Chunk was reallocated, toPool can be pooled.
- putBuf(b.toPool)
- }
- if cap(b.bufs) == 0 {
- b.bufs = make([][]byte, 0, 8)
- }
- b.bufs = append(b.bufs, b.Buf)
- l = cap(b.toPool) * 2
- } else {
- l = config.StartSize
- }
-
- if l > config.MaxSize {
- l = config.MaxSize
- }
- b.Buf = getBuf(l)
- b.toPool = b.Buf
-}
-
-// AppendByte appends a single byte to buffer.
-func (b *Buffer) AppendByte(data byte) {
- b.EnsureSpace(1)
- b.Buf = append(b.Buf, data)
-}
-
-// AppendBytes appends a byte slice to buffer.
-func (b *Buffer) AppendBytes(data []byte) {
- if len(data) <= cap(b.Buf)-len(b.Buf) {
- b.Buf = append(b.Buf, data...) // fast path
- } else {
- b.appendBytesSlow(data)
- }
-}
-
-func (b *Buffer) appendBytesSlow(data []byte) {
- for len(data) > 0 {
- b.EnsureSpace(1)
-
- sz := cap(b.Buf) - len(b.Buf)
- if sz > len(data) {
- sz = len(data)
- }
-
- b.Buf = append(b.Buf, data[:sz]...)
- data = data[sz:]
- }
-}
-
-// AppendString appends a string to buffer.
-func (b *Buffer) AppendString(data string) {
- if len(data) <= cap(b.Buf)-len(b.Buf) {
- b.Buf = append(b.Buf, data...) // fast path
- } else {
- b.appendStringSlow(data)
- }
-}
-
-func (b *Buffer) appendStringSlow(data string) {
- for len(data) > 0 {
- b.EnsureSpace(1)
-
- sz := cap(b.Buf) - len(b.Buf)
- if sz > len(data) {
- sz = len(data)
- }
-
- b.Buf = append(b.Buf, data[:sz]...)
- data = data[sz:]
- }
-}
-
-// Size computes the size of a buffer by adding sizes of every chunk.
-func (b *Buffer) Size() int {
- size := len(b.Buf)
- for _, buf := range b.bufs {
- size += len(buf)
- }
- return size
-}
-
-// DumpTo outputs the contents of a buffer to a writer and resets the buffer.
-func (b *Buffer) DumpTo(w io.Writer) (written int, err error) {
- bufs := net.Buffers(b.bufs)
- if len(b.Buf) > 0 {
- bufs = append(bufs, b.Buf)
- }
- n, err := bufs.WriteTo(w)
-
- for _, buf := range b.bufs {
- putBuf(buf)
- }
- putBuf(b.toPool)
-
- b.bufs = nil
- b.Buf = nil
- b.toPool = nil
-
- return int(n), err
-}
-
-// BuildBytes creates a single byte slice with all the contents of the buffer. Data is
-// copied if it does not fit in a single chunk. You can optionally provide one byte
-// slice as argument that it will try to reuse.
-func (b *Buffer) BuildBytes(reuse ...[]byte) []byte {
- if len(b.bufs) == 0 {
- ret := b.Buf
- b.toPool = nil
- b.Buf = nil
- return ret
- }
-
- var ret []byte
- size := b.Size()
-
- // If we got a buffer as argument and it is big enough, reuse it.
- if len(reuse) == 1 && cap(reuse[0]) >= size {
- ret = reuse[0][:0]
- } else {
- ret = make([]byte, 0, size)
- }
- for _, buf := range b.bufs {
- ret = append(ret, buf...)
- putBuf(buf)
- }
-
- ret = append(ret, b.Buf...)
- putBuf(b.toPool)
-
- b.bufs = nil
- b.toPool = nil
- b.Buf = nil
-
- return ret
-}
-
-type readCloser struct {
- offset int
- bufs [][]byte
-}
-
-func (r *readCloser) Read(p []byte) (n int, err error) {
- for _, buf := range r.bufs {
- // Copy as much as we can.
- x := copy(p[n:], buf[r.offset:])
- n += x // Increment how much we filled.
-
- // Did we empty the whole buffer?
- if r.offset+x == len(buf) {
- // On to the next buffer.
- r.offset = 0
- r.bufs = r.bufs[1:]
-
- // We can release this buffer.
- putBuf(buf)
- } else {
- r.offset += x
- }
-
- if n == len(p) {
- break
- }
- }
- // No buffers left or nothing read?
- if len(r.bufs) == 0 {
- err = io.EOF
- }
- return
-}
-
-func (r *readCloser) Close() error {
- // Release all remaining buffers.
- for _, buf := range r.bufs {
- putBuf(buf)
- }
- // In case Close gets called multiple times.
- r.bufs = nil
-
- return nil
-}
-
-// ReadCloser creates an io.ReadCloser with all the contents of the buffer.
-func (b *Buffer) ReadCloser() io.ReadCloser {
- ret := &readCloser{0, append(b.bufs, b.Buf)}
-
- b.bufs = nil
- b.toPool = nil
- b.Buf = nil
-
- return ret
-}
diff --git a/vendor/github.com/mailru/easyjson/jlexer/bytestostr.go b/vendor/github.com/mailru/easyjson/jlexer/bytestostr.go
deleted file mode 100644
index ff7b27c5..00000000
--- a/vendor/github.com/mailru/easyjson/jlexer/bytestostr.go
+++ /dev/null
@@ -1,24 +0,0 @@
-// This file will only be included to the build if neither
-// easyjson_nounsafe nor appengine build tag is set. See README notes
-// for more details.
-
-//+build !easyjson_nounsafe
-//+build !appengine
-
-package jlexer
-
-import (
- "reflect"
- "unsafe"
-)
-
-// bytesToStr creates a string pointing at the slice to avoid copying.
-//
-// Warning: the string returned by the function should be used with care, as the whole input data
-// chunk may be either blocked from being freed by GC because of a single string or the buffer.Data
-// may be garbage-collected even when the string exists.
-func bytesToStr(data []byte) string {
- h := (*reflect.SliceHeader)(unsafe.Pointer(&data))
- shdr := reflect.StringHeader{Data: h.Data, Len: h.Len}
- return *(*string)(unsafe.Pointer(&shdr))
-}
diff --git a/vendor/github.com/mailru/easyjson/jlexer/bytestostr_nounsafe.go b/vendor/github.com/mailru/easyjson/jlexer/bytestostr_nounsafe.go
deleted file mode 100644
index 864d1be6..00000000
--- a/vendor/github.com/mailru/easyjson/jlexer/bytestostr_nounsafe.go
+++ /dev/null
@@ -1,13 +0,0 @@
-// This file is included to the build if any of the buildtags below
-// are defined. Refer to README notes for more details.
-
-//+build easyjson_nounsafe appengine
-
-package jlexer
-
-// bytesToStr creates a string normally from []byte
-//
-// Note that this method is roughly 1.5x slower than using the 'unsafe' method.
-func bytesToStr(data []byte) string {
- return string(data)
-}
diff --git a/vendor/github.com/mailru/easyjson/jlexer/error.go b/vendor/github.com/mailru/easyjson/jlexer/error.go
deleted file mode 100644
index e90ec40d..00000000
--- a/vendor/github.com/mailru/easyjson/jlexer/error.go
+++ /dev/null
@@ -1,15 +0,0 @@
-package jlexer
-
-import "fmt"
-
-// LexerError implements the error interface and represents all possible errors that can be
-// generated during parsing the JSON data.
-type LexerError struct {
- Reason string
- Offset int
- Data string
-}
-
-func (l *LexerError) Error() string {
- return fmt.Sprintf("parse error: %s near offset %d of '%s'", l.Reason, l.Offset, l.Data)
-}
diff --git a/vendor/github.com/mailru/easyjson/jlexer/lexer.go b/vendor/github.com/mailru/easyjson/jlexer/lexer.go
deleted file mode 100644
index b5f5e261..00000000
--- a/vendor/github.com/mailru/easyjson/jlexer/lexer.go
+++ /dev/null
@@ -1,1244 +0,0 @@
-// Package jlexer contains a JSON lexer implementation.
-//
-// It is expected that it is mostly used with generated parser code, so the interface is tuned
-// for a parser that knows what kind of data is expected.
-package jlexer
-
-import (
- "bytes"
- "encoding/base64"
- "encoding/json"
- "errors"
- "fmt"
- "io"
- "strconv"
- "unicode"
- "unicode/utf16"
- "unicode/utf8"
-
- "github.com/josharian/intern"
-)
-
-// tokenKind determines type of a token.
-type tokenKind byte
-
-const (
- tokenUndef tokenKind = iota // No token.
- tokenDelim // Delimiter: one of '{', '}', '[' or ']'.
- tokenString // A string literal, e.g. "abc\u1234"
- tokenNumber // Number literal, e.g. 1.5e5
- tokenBool // Boolean literal: true or false.
- tokenNull // null keyword.
-)
-
-// token describes a single token: type, position in the input and value.
-type token struct {
- kind tokenKind // Type of a token.
-
- boolValue bool // Value if a boolean literal token.
- byteValueCloned bool // true if byteValue was allocated and does not refer to original json body
- byteValue []byte // Raw value of a token.
- delimValue byte
-}
-
-// Lexer is a JSON lexer: it iterates over JSON tokens in a byte slice.
-type Lexer struct {
- Data []byte // Input data given to the lexer.
-
- start int // Start of the current token.
- pos int // Current unscanned position in the input stream.
- token token // Last scanned token, if token.kind != tokenUndef.
-
- firstElement bool // Whether current element is the first in array or an object.
- wantSep byte // A comma or a colon character, which need to occur before a token.
-
- UseMultipleErrors bool // If we want to use multiple errors.
- fatalError error // Fatal error occurred during lexing. It is usually a syntax error.
- multipleErrors []*LexerError // Semantic errors occurred during lexing. Marshalling will be continued after finding this errors.
-}
-
-// FetchToken scans the input for the next token.
-func (r *Lexer) FetchToken() {
- r.token.kind = tokenUndef
- r.start = r.pos
-
- // Check if r.Data has r.pos element
- // If it doesn't, it mean corrupted input data
- if len(r.Data) < r.pos {
- r.errParse("Unexpected end of data")
- return
- }
- // Determine the type of a token by skipping whitespace and reading the
- // first character.
- for _, c := range r.Data[r.pos:] {
- switch c {
- case ':', ',':
- if r.wantSep == c {
- r.pos++
- r.start++
- r.wantSep = 0
- } else {
- r.errSyntax()
- }
-
- case ' ', '\t', '\r', '\n':
- r.pos++
- r.start++
-
- case '"':
- if r.wantSep != 0 {
- r.errSyntax()
- }
-
- r.token.kind = tokenString
- r.fetchString()
- return
-
- case '{', '[':
- if r.wantSep != 0 {
- r.errSyntax()
- }
- r.firstElement = true
- r.token.kind = tokenDelim
- r.token.delimValue = r.Data[r.pos]
- r.pos++
- return
-
- case '}', ']':
- if !r.firstElement && (r.wantSep != ',') {
- r.errSyntax()
- }
- r.wantSep = 0
- r.token.kind = tokenDelim
- r.token.delimValue = r.Data[r.pos]
- r.pos++
- return
-
- case '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '-':
- if r.wantSep != 0 {
- r.errSyntax()
- }
- r.token.kind = tokenNumber
- r.fetchNumber()
- return
-
- case 'n':
- if r.wantSep != 0 {
- r.errSyntax()
- }
-
- r.token.kind = tokenNull
- r.fetchNull()
- return
-
- case 't':
- if r.wantSep != 0 {
- r.errSyntax()
- }
-
- r.token.kind = tokenBool
- r.token.boolValue = true
- r.fetchTrue()
- return
-
- case 'f':
- if r.wantSep != 0 {
- r.errSyntax()
- }
-
- r.token.kind = tokenBool
- r.token.boolValue = false
- r.fetchFalse()
- return
-
- default:
- r.errSyntax()
- return
- }
- }
- r.fatalError = io.EOF
- return
-}
-
-// isTokenEnd returns true if the char can follow a non-delimiter token
-func isTokenEnd(c byte) bool {
- return c == ' ' || c == '\t' || c == '\r' || c == '\n' || c == '[' || c == ']' || c == '{' || c == '}' || c == ',' || c == ':'
-}
-
-// fetchNull fetches and checks remaining bytes of null keyword.
-func (r *Lexer) fetchNull() {
- r.pos += 4
- if r.pos > len(r.Data) ||
- r.Data[r.pos-3] != 'u' ||
- r.Data[r.pos-2] != 'l' ||
- r.Data[r.pos-1] != 'l' ||
- (r.pos != len(r.Data) && !isTokenEnd(r.Data[r.pos])) {
-
- r.pos -= 4
- r.errSyntax()
- }
-}
-
-// fetchTrue fetches and checks remaining bytes of true keyword.
-func (r *Lexer) fetchTrue() {
- r.pos += 4
- if r.pos > len(r.Data) ||
- r.Data[r.pos-3] != 'r' ||
- r.Data[r.pos-2] != 'u' ||
- r.Data[r.pos-1] != 'e' ||
- (r.pos != len(r.Data) && !isTokenEnd(r.Data[r.pos])) {
-
- r.pos -= 4
- r.errSyntax()
- }
-}
-
-// fetchFalse fetches and checks remaining bytes of false keyword.
-func (r *Lexer) fetchFalse() {
- r.pos += 5
- if r.pos > len(r.Data) ||
- r.Data[r.pos-4] != 'a' ||
- r.Data[r.pos-3] != 'l' ||
- r.Data[r.pos-2] != 's' ||
- r.Data[r.pos-1] != 'e' ||
- (r.pos != len(r.Data) && !isTokenEnd(r.Data[r.pos])) {
-
- r.pos -= 5
- r.errSyntax()
- }
-}
-
-// fetchNumber scans a number literal token.
-func (r *Lexer) fetchNumber() {
- hasE := false
- afterE := false
- hasDot := false
-
- r.pos++
- for i, c := range r.Data[r.pos:] {
- switch {
- case c >= '0' && c <= '9':
- afterE = false
- case c == '.' && !hasDot:
- hasDot = true
- case (c == 'e' || c == 'E') && !hasE:
- hasE = true
- hasDot = true
- afterE = true
- case (c == '+' || c == '-') && afterE:
- afterE = false
- default:
- r.pos += i
- if !isTokenEnd(c) {
- r.errSyntax()
- } else {
- r.token.byteValue = r.Data[r.start:r.pos]
- }
- return
- }
- }
-
- r.pos = len(r.Data)
- r.token.byteValue = r.Data[r.start:]
-}
-
-// findStringLen tries to scan into the string literal for ending quote char to determine required size.
-// The size will be exact if no escapes are present and may be inexact if there are escaped chars.
-func findStringLen(data []byte) (isValid bool, length int) {
- for {
- idx := bytes.IndexByte(data, '"')
- if idx == -1 {
- return false, len(data)
- }
- if idx == 0 || (idx > 0 && data[idx-1] != '\\') {
- return true, length + idx
- }
-
- // count \\\\\\\ sequences. even number of slashes means quote is not really escaped
- cnt := 1
- for idx-cnt-1 >= 0 && data[idx-cnt-1] == '\\' {
- cnt++
- }
- if cnt%2 == 0 {
- return true, length + idx
- }
-
- length += idx + 1
- data = data[idx+1:]
- }
-}
-
-// unescapeStringToken performs unescaping of string token.
-// if no escaping is needed, original string is returned, otherwise - a new one allocated
-func (r *Lexer) unescapeStringToken() (err error) {
- data := r.token.byteValue
- var unescapedData []byte
-
- for {
- i := bytes.IndexByte(data, '\\')
- if i == -1 {
- break
- }
-
- escapedRune, escapedBytes, err := decodeEscape(data[i:])
- if err != nil {
- r.errParse(err.Error())
- return err
- }
-
- if unescapedData == nil {
- unescapedData = make([]byte, 0, len(r.token.byteValue))
- }
-
- var d [4]byte
- s := utf8.EncodeRune(d[:], escapedRune)
- unescapedData = append(unescapedData, data[:i]...)
- unescapedData = append(unescapedData, d[:s]...)
-
- data = data[i+escapedBytes:]
- }
-
- if unescapedData != nil {
- r.token.byteValue = append(unescapedData, data...)
- r.token.byteValueCloned = true
- }
- return
-}
-
-// getu4 decodes \uXXXX from the beginning of s, returning the hex value,
-// or it returns -1.
-func getu4(s []byte) rune {
- if len(s) < 6 || s[0] != '\\' || s[1] != 'u' {
- return -1
- }
- var val rune
- for i := 2; i < len(s) && i < 6; i++ {
- var v byte
- c := s[i]
- switch c {
- case '0', '1', '2', '3', '4', '5', '6', '7', '8', '9':
- v = c - '0'
- case 'a', 'b', 'c', 'd', 'e', 'f':
- v = c - 'a' + 10
- case 'A', 'B', 'C', 'D', 'E', 'F':
- v = c - 'A' + 10
- default:
- return -1
- }
-
- val <<= 4
- val |= rune(v)
- }
- return val
-}
-
-// decodeEscape processes a single escape sequence and returns number of bytes processed.
-func decodeEscape(data []byte) (decoded rune, bytesProcessed int, err error) {
- if len(data) < 2 {
- return 0, 0, errors.New("incorrect escape symbol \\ at the end of token")
- }
-
- c := data[1]
- switch c {
- case '"', '/', '\\':
- return rune(c), 2, nil
- case 'b':
- return '\b', 2, nil
- case 'f':
- return '\f', 2, nil
- case 'n':
- return '\n', 2, nil
- case 'r':
- return '\r', 2, nil
- case 't':
- return '\t', 2, nil
- case 'u':
- rr := getu4(data)
- if rr < 0 {
- return 0, 0, errors.New("incorrectly escaped \\uXXXX sequence")
- }
-
- read := 6
- if utf16.IsSurrogate(rr) {
- rr1 := getu4(data[read:])
- if dec := utf16.DecodeRune(rr, rr1); dec != unicode.ReplacementChar {
- read += 6
- rr = dec
- } else {
- rr = unicode.ReplacementChar
- }
- }
- return rr, read, nil
- }
-
- return 0, 0, errors.New("incorrectly escaped bytes")
-}
-
-// fetchString scans a string literal token.
-func (r *Lexer) fetchString() {
- r.pos++
- data := r.Data[r.pos:]
-
- isValid, length := findStringLen(data)
- if !isValid {
- r.pos += length
- r.errParse("unterminated string literal")
- return
- }
- r.token.byteValue = data[:length]
- r.pos += length + 1 // skip closing '"' as well
-}
-
-// scanToken scans the next token if no token is currently available in the lexer.
-func (r *Lexer) scanToken() {
- if r.token.kind != tokenUndef || r.fatalError != nil {
- return
- }
-
- r.FetchToken()
-}
-
-// consume resets the current token to allow scanning the next one.
-func (r *Lexer) consume() {
- r.token.kind = tokenUndef
- r.token.byteValueCloned = false
- r.token.delimValue = 0
-}
-
-// Ok returns true if no error (including io.EOF) was encountered during scanning.
-func (r *Lexer) Ok() bool {
- return r.fatalError == nil
-}
-
-const maxErrorContextLen = 13
-
-func (r *Lexer) errParse(what string) {
- if r.fatalError == nil {
- var str string
- if len(r.Data)-r.pos <= maxErrorContextLen {
- str = string(r.Data)
- } else {
- str = string(r.Data[r.pos:r.pos+maxErrorContextLen-3]) + "..."
- }
- r.fatalError = &LexerError{
- Reason: what,
- Offset: r.pos,
- Data: str,
- }
- }
-}
-
-func (r *Lexer) errSyntax() {
- r.errParse("syntax error")
-}
-
-func (r *Lexer) errInvalidToken(expected string) {
- if r.fatalError != nil {
- return
- }
- if r.UseMultipleErrors {
- r.pos = r.start
- r.consume()
- r.SkipRecursive()
- switch expected {
- case "[":
- r.token.delimValue = ']'
- r.token.kind = tokenDelim
- case "{":
- r.token.delimValue = '}'
- r.token.kind = tokenDelim
- }
- r.addNonfatalError(&LexerError{
- Reason: fmt.Sprintf("expected %s", expected),
- Offset: r.start,
- Data: string(r.Data[r.start:r.pos]),
- })
- return
- }
-
- var str string
- if len(r.token.byteValue) <= maxErrorContextLen {
- str = string(r.token.byteValue)
- } else {
- str = string(r.token.byteValue[:maxErrorContextLen-3]) + "..."
- }
- r.fatalError = &LexerError{
- Reason: fmt.Sprintf("expected %s", expected),
- Offset: r.pos,
- Data: str,
- }
-}
-
-func (r *Lexer) GetPos() int {
- return r.pos
-}
-
-// Delim consumes a token and verifies that it is the given delimiter.
-func (r *Lexer) Delim(c byte) {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
-
- if !r.Ok() || r.token.delimValue != c {
- r.consume() // errInvalidToken can change token if UseMultipleErrors is enabled.
- r.errInvalidToken(string([]byte{c}))
- } else {
- r.consume()
- }
-}
-
-// IsDelim returns true if there was no scanning error and next token is the given delimiter.
-func (r *Lexer) IsDelim(c byte) bool {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- return !r.Ok() || r.token.delimValue == c
-}
-
-// Null verifies that the next token is null and consumes it.
-func (r *Lexer) Null() {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenNull {
- r.errInvalidToken("null")
- }
- r.consume()
-}
-
-// IsNull returns true if the next token is a null keyword.
-func (r *Lexer) IsNull() bool {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- return r.Ok() && r.token.kind == tokenNull
-}
-
-// Skip skips a single token.
-func (r *Lexer) Skip() {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- r.consume()
-}
-
-// SkipRecursive skips next array or object completely, or just skips a single token if not
-// an array/object.
-//
-// Note: no syntax validation is performed on the skipped data.
-func (r *Lexer) SkipRecursive() {
- r.scanToken()
- var start, end byte
- startPos := r.start
-
- switch r.token.delimValue {
- case '{':
- start, end = '{', '}'
- case '[':
- start, end = '[', ']'
- default:
- r.consume()
- return
- }
-
- r.consume()
-
- level := 1
- inQuotes := false
- wasEscape := false
-
- for i, c := range r.Data[r.pos:] {
- switch {
- case c == start && !inQuotes:
- level++
- case c == end && !inQuotes:
- level--
- if level == 0 {
- r.pos += i + 1
- if !json.Valid(r.Data[startPos:r.pos]) {
- r.pos = len(r.Data)
- r.fatalError = &LexerError{
- Reason: "skipped array/object json value is invalid",
- Offset: r.pos,
- Data: string(r.Data[r.pos:]),
- }
- }
- return
- }
- case c == '\\' && inQuotes:
- wasEscape = !wasEscape
- continue
- case c == '"' && inQuotes:
- inQuotes = wasEscape
- case c == '"':
- inQuotes = true
- }
- wasEscape = false
- }
- r.pos = len(r.Data)
- r.fatalError = &LexerError{
- Reason: "EOF reached while skipping array/object or token",
- Offset: r.pos,
- Data: string(r.Data[r.pos:]),
- }
-}
-
-// Raw fetches the next item recursively as a data slice
-func (r *Lexer) Raw() []byte {
- r.SkipRecursive()
- if !r.Ok() {
- return nil
- }
- return r.Data[r.start:r.pos]
-}
-
-// IsStart returns whether the lexer is positioned at the start
-// of an input string.
-func (r *Lexer) IsStart() bool {
- return r.pos == 0
-}
-
-// Consumed reads all remaining bytes from the input, publishing an error if
-// there is anything but whitespace remaining.
-func (r *Lexer) Consumed() {
- if r.pos > len(r.Data) || !r.Ok() {
- return
- }
-
- for _, c := range r.Data[r.pos:] {
- if c != ' ' && c != '\t' && c != '\r' && c != '\n' {
- r.AddError(&LexerError{
- Reason: "invalid character '" + string(c) + "' after top-level value",
- Offset: r.pos,
- Data: string(r.Data[r.pos:]),
- })
- return
- }
-
- r.pos++
- r.start++
- }
-}
-
-func (r *Lexer) unsafeString(skipUnescape bool) (string, []byte) {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenString {
- r.errInvalidToken("string")
- return "", nil
- }
- if !skipUnescape {
- if err := r.unescapeStringToken(); err != nil {
- r.errInvalidToken("string")
- return "", nil
- }
- }
-
- bytes := r.token.byteValue
- ret := bytesToStr(r.token.byteValue)
- r.consume()
- return ret, bytes
-}
-
-// UnsafeString returns the string value if the token is a string literal.
-//
-// Warning: returned string may point to the input buffer, so the string should not outlive
-// the input buffer. Intended pattern of usage is as an argument to a switch statement.
-func (r *Lexer) UnsafeString() string {
- ret, _ := r.unsafeString(false)
- return ret
-}
-
-// UnsafeBytes returns the byte slice if the token is a string literal.
-func (r *Lexer) UnsafeBytes() []byte {
- _, ret := r.unsafeString(false)
- return ret
-}
-
-// UnsafeFieldName returns current member name string token
-func (r *Lexer) UnsafeFieldName(skipUnescape bool) string {
- ret, _ := r.unsafeString(skipUnescape)
- return ret
-}
-
-// String reads a string literal.
-func (r *Lexer) String() string {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenString {
- r.errInvalidToken("string")
- return ""
- }
- if err := r.unescapeStringToken(); err != nil {
- r.errInvalidToken("string")
- return ""
- }
- var ret string
- if r.token.byteValueCloned {
- ret = bytesToStr(r.token.byteValue)
- } else {
- ret = string(r.token.byteValue)
- }
- r.consume()
- return ret
-}
-
-// StringIntern reads a string literal, and performs string interning on it.
-func (r *Lexer) StringIntern() string {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenString {
- r.errInvalidToken("string")
- return ""
- }
- if err := r.unescapeStringToken(); err != nil {
- r.errInvalidToken("string")
- return ""
- }
- ret := intern.Bytes(r.token.byteValue)
- r.consume()
- return ret
-}
-
-// Bytes reads a string literal and base64 decodes it into a byte slice.
-func (r *Lexer) Bytes() []byte {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenString {
- r.errInvalidToken("string")
- return nil
- }
- if err := r.unescapeStringToken(); err != nil {
- r.errInvalidToken("string")
- return nil
- }
- ret := make([]byte, base64.StdEncoding.DecodedLen(len(r.token.byteValue)))
- n, err := base64.StdEncoding.Decode(ret, r.token.byteValue)
- if err != nil {
- r.fatalError = &LexerError{
- Reason: err.Error(),
- }
- return nil
- }
-
- r.consume()
- return ret[:n]
-}
-
-// Bool reads a true or false boolean keyword.
-func (r *Lexer) Bool() bool {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenBool {
- r.errInvalidToken("bool")
- return false
- }
- ret := r.token.boolValue
- r.consume()
- return ret
-}
-
-func (r *Lexer) number() string {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() || r.token.kind != tokenNumber {
- r.errInvalidToken("number")
- return ""
- }
- ret := bytesToStr(r.token.byteValue)
- r.consume()
- return ret
-}
-
-func (r *Lexer) Uint8() uint8 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 8)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return uint8(n)
-}
-
-func (r *Lexer) Uint16() uint16 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 16)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return uint16(n)
-}
-
-func (r *Lexer) Uint32() uint32 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 32)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return uint32(n)
-}
-
-func (r *Lexer) Uint64() uint64 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 64)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return n
-}
-
-func (r *Lexer) Uint() uint {
- return uint(r.Uint64())
-}
-
-func (r *Lexer) Int8() int8 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 8)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return int8(n)
-}
-
-func (r *Lexer) Int16() int16 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 16)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return int16(n)
-}
-
-func (r *Lexer) Int32() int32 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 32)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return int32(n)
-}
-
-func (r *Lexer) Int64() int64 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 64)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return n
-}
-
-func (r *Lexer) Int() int {
- return int(r.Int64())
-}
-
-func (r *Lexer) Uint8Str() uint8 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 8)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return uint8(n)
-}
-
-func (r *Lexer) Uint16Str() uint16 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 16)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return uint16(n)
-}
-
-func (r *Lexer) Uint32Str() uint32 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 32)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return uint32(n)
-}
-
-func (r *Lexer) Uint64Str() uint64 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseUint(s, 10, 64)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return n
-}
-
-func (r *Lexer) UintStr() uint {
- return uint(r.Uint64Str())
-}
-
-func (r *Lexer) UintptrStr() uintptr {
- return uintptr(r.Uint64Str())
-}
-
-func (r *Lexer) Int8Str() int8 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 8)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return int8(n)
-}
-
-func (r *Lexer) Int16Str() int16 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 16)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return int16(n)
-}
-
-func (r *Lexer) Int32Str() int32 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 32)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return int32(n)
-}
-
-func (r *Lexer) Int64Str() int64 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseInt(s, 10, 64)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return n
-}
-
-func (r *Lexer) IntStr() int {
- return int(r.Int64Str())
-}
-
-func (r *Lexer) Float32() float32 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseFloat(s, 32)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return float32(n)
-}
-
-func (r *Lexer) Float32Str() float32 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
- n, err := strconv.ParseFloat(s, 32)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return float32(n)
-}
-
-func (r *Lexer) Float64() float64 {
- s := r.number()
- if !r.Ok() {
- return 0
- }
-
- n, err := strconv.ParseFloat(s, 64)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: s,
- })
- }
- return n
-}
-
-func (r *Lexer) Float64Str() float64 {
- s, b := r.unsafeString(false)
- if !r.Ok() {
- return 0
- }
- n, err := strconv.ParseFloat(s, 64)
- if err != nil {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Reason: err.Error(),
- Data: string(b),
- })
- }
- return n
-}
-
-func (r *Lexer) Error() error {
- return r.fatalError
-}
-
-func (r *Lexer) AddError(e error) {
- if r.fatalError == nil {
- r.fatalError = e
- }
-}
-
-func (r *Lexer) AddNonFatalError(e error) {
- r.addNonfatalError(&LexerError{
- Offset: r.start,
- Data: string(r.Data[r.start:r.pos]),
- Reason: e.Error(),
- })
-}
-
-func (r *Lexer) addNonfatalError(err *LexerError) {
- if r.UseMultipleErrors {
- // We don't want to add errors with the same offset.
- if len(r.multipleErrors) != 0 && r.multipleErrors[len(r.multipleErrors)-1].Offset == err.Offset {
- return
- }
- r.multipleErrors = append(r.multipleErrors, err)
- return
- }
- r.fatalError = err
-}
-
-func (r *Lexer) GetNonFatalErrors() []*LexerError {
- return r.multipleErrors
-}
-
-// JsonNumber fetches and json.Number from 'encoding/json' package.
-// Both int, float or string, contains them are valid values
-func (r *Lexer) JsonNumber() json.Number {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
- if !r.Ok() {
- r.errInvalidToken("json.Number")
- return json.Number("")
- }
-
- switch r.token.kind {
- case tokenString:
- return json.Number(r.String())
- case tokenNumber:
- return json.Number(r.Raw())
- case tokenNull:
- r.Null()
- return json.Number("")
- default:
- r.errSyntax()
- return json.Number("")
- }
-}
-
-// Interface fetches an interface{} analogous to the 'encoding/json' package.
-func (r *Lexer) Interface() interface{} {
- if r.token.kind == tokenUndef && r.Ok() {
- r.FetchToken()
- }
-
- if !r.Ok() {
- return nil
- }
- switch r.token.kind {
- case tokenString:
- return r.String()
- case tokenNumber:
- return r.Float64()
- case tokenBool:
- return r.Bool()
- case tokenNull:
- r.Null()
- return nil
- }
-
- if r.token.delimValue == '{' {
- r.consume()
-
- ret := map[string]interface{}{}
- for !r.IsDelim('}') {
- key := r.String()
- r.WantColon()
- ret[key] = r.Interface()
- r.WantComma()
- }
- r.Delim('}')
-
- if r.Ok() {
- return ret
- } else {
- return nil
- }
- } else if r.token.delimValue == '[' {
- r.consume()
-
- ret := []interface{}{}
- for !r.IsDelim(']') {
- ret = append(ret, r.Interface())
- r.WantComma()
- }
- r.Delim(']')
-
- if r.Ok() {
- return ret
- } else {
- return nil
- }
- }
- r.errSyntax()
- return nil
-}
-
-// WantComma requires a comma to be present before fetching next token.
-func (r *Lexer) WantComma() {
- r.wantSep = ','
- r.firstElement = false
-}
-
-// WantColon requires a colon to be present before fetching next token.
-func (r *Lexer) WantColon() {
- r.wantSep = ':'
- r.firstElement = false
-}
diff --git a/vendor/github.com/mailru/easyjson/jwriter/writer.go b/vendor/github.com/mailru/easyjson/jwriter/writer.go
deleted file mode 100644
index 2c5b2010..00000000
--- a/vendor/github.com/mailru/easyjson/jwriter/writer.go
+++ /dev/null
@@ -1,405 +0,0 @@
-// Package jwriter contains a JSON writer.
-package jwriter
-
-import (
- "io"
- "strconv"
- "unicode/utf8"
-
- "github.com/mailru/easyjson/buffer"
-)
-
-// Flags describe various encoding options. The behavior may be actually implemented in the encoder, but
-// Flags field in Writer is used to set and pass them around.
-type Flags int
-
-const (
- NilMapAsEmpty Flags = 1 << iota // Encode nil map as '{}' rather than 'null'.
- NilSliceAsEmpty // Encode nil slice as '[]' rather than 'null'.
-)
-
-// Writer is a JSON writer.
-type Writer struct {
- Flags Flags
-
- Error error
- Buffer buffer.Buffer
- NoEscapeHTML bool
-}
-
-// Size returns the size of the data that was written out.
-func (w *Writer) Size() int {
- return w.Buffer.Size()
-}
-
-// DumpTo outputs the data to given io.Writer, resetting the buffer.
-func (w *Writer) DumpTo(out io.Writer) (written int, err error) {
- return w.Buffer.DumpTo(out)
-}
-
-// BuildBytes returns writer data as a single byte slice. You can optionally provide one byte slice
-// as argument that it will try to reuse.
-func (w *Writer) BuildBytes(reuse ...[]byte) ([]byte, error) {
- if w.Error != nil {
- return nil, w.Error
- }
-
- return w.Buffer.BuildBytes(reuse...), nil
-}
-
-// ReadCloser returns an io.ReadCloser that can be used to read the data.
-// ReadCloser also resets the buffer.
-func (w *Writer) ReadCloser() (io.ReadCloser, error) {
- if w.Error != nil {
- return nil, w.Error
- }
-
- return w.Buffer.ReadCloser(), nil
-}
-
-// RawByte appends raw binary data to the buffer.
-func (w *Writer) RawByte(c byte) {
- w.Buffer.AppendByte(c)
-}
-
-// RawByte appends raw binary data to the buffer.
-func (w *Writer) RawString(s string) {
- w.Buffer.AppendString(s)
-}
-
-// Raw appends raw binary data to the buffer or sets the error if it is given. Useful for
-// calling with results of MarshalJSON-like functions.
-func (w *Writer) Raw(data []byte, err error) {
- switch {
- case w.Error != nil:
- return
- case err != nil:
- w.Error = err
- case len(data) > 0:
- w.Buffer.AppendBytes(data)
- default:
- w.RawString("null")
- }
-}
-
-// RawText encloses raw binary data in quotes and appends in to the buffer.
-// Useful for calling with results of MarshalText-like functions.
-func (w *Writer) RawText(data []byte, err error) {
- switch {
- case w.Error != nil:
- return
- case err != nil:
- w.Error = err
- case len(data) > 0:
- w.String(string(data))
- default:
- w.RawString("null")
- }
-}
-
-// Base64Bytes appends data to the buffer after base64 encoding it
-func (w *Writer) Base64Bytes(data []byte) {
- if data == nil {
- w.Buffer.AppendString("null")
- return
- }
- w.Buffer.AppendByte('"')
- w.base64(data)
- w.Buffer.AppendByte('"')
-}
-
-func (w *Writer) Uint8(n uint8) {
- w.Buffer.EnsureSpace(3)
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
-}
-
-func (w *Writer) Uint16(n uint16) {
- w.Buffer.EnsureSpace(5)
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
-}
-
-func (w *Writer) Uint32(n uint32) {
- w.Buffer.EnsureSpace(10)
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
-}
-
-func (w *Writer) Uint(n uint) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
-}
-
-func (w *Writer) Uint64(n uint64) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, n, 10)
-}
-
-func (w *Writer) Int8(n int8) {
- w.Buffer.EnsureSpace(4)
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
-}
-
-func (w *Writer) Int16(n int16) {
- w.Buffer.EnsureSpace(6)
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
-}
-
-func (w *Writer) Int32(n int32) {
- w.Buffer.EnsureSpace(11)
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
-}
-
-func (w *Writer) Int(n int) {
- w.Buffer.EnsureSpace(21)
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
-}
-
-func (w *Writer) Int64(n int64) {
- w.Buffer.EnsureSpace(21)
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, n, 10)
-}
-
-func (w *Writer) Uint8Str(n uint8) {
- w.Buffer.EnsureSpace(3)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Uint16Str(n uint16) {
- w.Buffer.EnsureSpace(5)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Uint32Str(n uint32) {
- w.Buffer.EnsureSpace(10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) UintStr(n uint) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Uint64Str(n uint64) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, n, 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) UintptrStr(n uintptr) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendUint(w.Buffer.Buf, uint64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Int8Str(n int8) {
- w.Buffer.EnsureSpace(4)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Int16Str(n int16) {
- w.Buffer.EnsureSpace(6)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Int32Str(n int32) {
- w.Buffer.EnsureSpace(11)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) IntStr(n int) {
- w.Buffer.EnsureSpace(21)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, int64(n), 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Int64Str(n int64) {
- w.Buffer.EnsureSpace(21)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendInt(w.Buffer.Buf, n, 10)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Float32(n float32) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = strconv.AppendFloat(w.Buffer.Buf, float64(n), 'g', -1, 32)
-}
-
-func (w *Writer) Float32Str(n float32) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendFloat(w.Buffer.Buf, float64(n), 'g', -1, 32)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Float64(n float64) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = strconv.AppendFloat(w.Buffer.Buf, n, 'g', -1, 64)
-}
-
-func (w *Writer) Float64Str(n float64) {
- w.Buffer.EnsureSpace(20)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
- w.Buffer.Buf = strconv.AppendFloat(w.Buffer.Buf, float64(n), 'g', -1, 64)
- w.Buffer.Buf = append(w.Buffer.Buf, '"')
-}
-
-func (w *Writer) Bool(v bool) {
- w.Buffer.EnsureSpace(5)
- if v {
- w.Buffer.Buf = append(w.Buffer.Buf, "true"...)
- } else {
- w.Buffer.Buf = append(w.Buffer.Buf, "false"...)
- }
-}
-
-const chars = "0123456789abcdef"
-
-func getTable(falseValues ...int) [128]bool {
- table := [128]bool{}
-
- for i := 0; i < 128; i++ {
- table[i] = true
- }
-
- for _, v := range falseValues {
- table[v] = false
- }
-
- return table
-}
-
-var (
- htmlEscapeTable = getTable(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, '"', '&', '<', '>', '\\')
- htmlNoEscapeTable = getTable(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, '"', '\\')
-)
-
-func (w *Writer) String(s string) {
- w.Buffer.AppendByte('"')
-
- // Portions of the string that contain no escapes are appended as
- // byte slices.
-
- p := 0 // last non-escape symbol
-
- escapeTable := &htmlEscapeTable
- if w.NoEscapeHTML {
- escapeTable = &htmlNoEscapeTable
- }
-
- for i := 0; i < len(s); {
- c := s[i]
-
- if c < utf8.RuneSelf {
- if escapeTable[c] {
- // single-width character, no escaping is required
- i++
- continue
- }
-
- w.Buffer.AppendString(s[p:i])
- switch c {
- case '\t':
- w.Buffer.AppendString(`\t`)
- case '\r':
- w.Buffer.AppendString(`\r`)
- case '\n':
- w.Buffer.AppendString(`\n`)
- case '\\':
- w.Buffer.AppendString(`\\`)
- case '"':
- w.Buffer.AppendString(`\"`)
- default:
- w.Buffer.AppendString(`\u00`)
- w.Buffer.AppendByte(chars[c>>4])
- w.Buffer.AppendByte(chars[c&0xf])
- }
-
- i++
- p = i
- continue
- }
-
- // broken utf
- runeValue, runeWidth := utf8.DecodeRuneInString(s[i:])
- if runeValue == utf8.RuneError && runeWidth == 1 {
- w.Buffer.AppendString(s[p:i])
- w.Buffer.AppendString(`\ufffd`)
- i++
- p = i
- continue
- }
-
- // jsonp stuff - tab separator and line separator
- if runeValue == '\u2028' || runeValue == '\u2029' {
- w.Buffer.AppendString(s[p:i])
- w.Buffer.AppendString(`\u202`)
- w.Buffer.AppendByte(chars[runeValue&0xf])
- i += runeWidth
- p = i
- continue
- }
- i += runeWidth
- }
- w.Buffer.AppendString(s[p:])
- w.Buffer.AppendByte('"')
-}
-
-const encode = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"
-const padChar = '='
-
-func (w *Writer) base64(in []byte) {
-
- if len(in) == 0 {
- return
- }
-
- w.Buffer.EnsureSpace(((len(in)-1)/3 + 1) * 4)
-
- si := 0
- n := (len(in) / 3) * 3
-
- for si < n {
- // Convert 3x 8bit source bytes into 4 bytes
- val := uint(in[si+0])<<16 | uint(in[si+1])<<8 | uint(in[si+2])
-
- w.Buffer.Buf = append(w.Buffer.Buf, encode[val>>18&0x3F], encode[val>>12&0x3F], encode[val>>6&0x3F], encode[val&0x3F])
-
- si += 3
- }
-
- remain := len(in) - si
- if remain == 0 {
- return
- }
-
- // Add the remaining small block
- val := uint(in[si+0]) << 16
- if remain == 2 {
- val |= uint(in[si+1]) << 8
- }
-
- w.Buffer.Buf = append(w.Buffer.Buf, encode[val>>18&0x3F], encode[val>>12&0x3F])
-
- switch remain {
- case 2:
- w.Buffer.Buf = append(w.Buffer.Buf, encode[val>>6&0x3F], byte(padChar))
- case 1:
- w.Buffer.Buf = append(w.Buffer.Buf, byte(padChar), byte(padChar))
- }
-}
diff --git a/vendor/github.com/mattn/go-runewidth/LICENSE b/vendor/github.com/mattn/go-runewidth/LICENSE
deleted file mode 100644
index 91b5cef3..00000000
--- a/vendor/github.com/mattn/go-runewidth/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2016 Yasuhiro Matsumoto
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/mattn/go-runewidth/README.md b/vendor/github.com/mattn/go-runewidth/README.md
deleted file mode 100644
index 5e2cfd98..00000000
--- a/vendor/github.com/mattn/go-runewidth/README.md
+++ /dev/null
@@ -1,27 +0,0 @@
-go-runewidth
-============
-
-[](https://github.com/mattn/go-runewidth/actions?query=workflow%3Atest)
-[](https://codecov.io/gh/mattn/go-runewidth)
-[](http://godoc.org/github.com/mattn/go-runewidth)
-[](https://goreportcard.com/report/github.com/mattn/go-runewidth)
-
-Provides functions to get fixed width of the character or string.
-
-Usage
------
-
-```go
-runewidth.StringWidth("ã¤ã®ã ☆HIRO") == 12
-```
-
-
-Author
-------
-
-Yasuhiro Matsumoto
-
-License
--------
-
-under the MIT License: http://mattn.mit-license.org/2013
diff --git a/vendor/github.com/mattn/go-runewidth/runewidth.go b/vendor/github.com/mattn/go-runewidth/runewidth.go
deleted file mode 100644
index 7dfbb3be..00000000
--- a/vendor/github.com/mattn/go-runewidth/runewidth.go
+++ /dev/null
@@ -1,358 +0,0 @@
-package runewidth
-
-import (
- "os"
- "strings"
-
- "github.com/rivo/uniseg"
-)
-
-//go:generate go run script/generate.go
-
-var (
- // EastAsianWidth will be set true if the current locale is CJK
- EastAsianWidth bool
-
- // StrictEmojiNeutral should be set false if handle broken fonts
- StrictEmojiNeutral bool = true
-
- // DefaultCondition is a condition in current locale
- DefaultCondition = &Condition{
- EastAsianWidth: false,
- StrictEmojiNeutral: true,
- }
-)
-
-func init() {
- handleEnv()
-}
-
-func handleEnv() {
- env := os.Getenv("RUNEWIDTH_EASTASIAN")
- if env == "" {
- EastAsianWidth = IsEastAsian()
- } else {
- EastAsianWidth = env == "1"
- }
- // update DefaultCondition
- if DefaultCondition.EastAsianWidth != EastAsianWidth {
- DefaultCondition.EastAsianWidth = EastAsianWidth
- if len(DefaultCondition.combinedLut) > 0 {
- DefaultCondition.combinedLut = DefaultCondition.combinedLut[:0]
- CreateLUT()
- }
- }
-}
-
-type interval struct {
- first rune
- last rune
-}
-
-type table []interval
-
-func inTables(r rune, ts ...table) bool {
- for _, t := range ts {
- if inTable(r, t) {
- return true
- }
- }
- return false
-}
-
-func inTable(r rune, t table) bool {
- if r < t[0].first {
- return false
- }
-
- bot := 0
- top := len(t) - 1
- for top >= bot {
- mid := (bot + top) >> 1
-
- switch {
- case t[mid].last < r:
- bot = mid + 1
- case t[mid].first > r:
- top = mid - 1
- default:
- return true
- }
- }
-
- return false
-}
-
-var private = table{
- {0x00E000, 0x00F8FF}, {0x0F0000, 0x0FFFFD}, {0x100000, 0x10FFFD},
-}
-
-var nonprint = table{
- {0x0000, 0x001F}, {0x007F, 0x009F}, {0x00AD, 0x00AD},
- {0x070F, 0x070F}, {0x180B, 0x180E}, {0x200B, 0x200F},
- {0x2028, 0x202E}, {0x206A, 0x206F}, {0xD800, 0xDFFF},
- {0xFEFF, 0xFEFF}, {0xFFF9, 0xFFFB}, {0xFFFE, 0xFFFF},
-}
-
-// Condition have flag EastAsianWidth whether the current locale is CJK or not.
-type Condition struct {
- combinedLut []byte
- EastAsianWidth bool
- StrictEmojiNeutral bool
-}
-
-// NewCondition return new instance of Condition which is current locale.
-func NewCondition() *Condition {
- return &Condition{
- EastAsianWidth: EastAsianWidth,
- StrictEmojiNeutral: StrictEmojiNeutral,
- }
-}
-
-// RuneWidth returns the number of cells in r.
-// See http://www.unicode.org/reports/tr11/
-func (c *Condition) RuneWidth(r rune) int {
- if r < 0 || r > 0x10FFFF {
- return 0
- }
- if len(c.combinedLut) > 0 {
- return int(c.combinedLut[r>>1]>>(uint(r&1)*4)) & 3
- }
- // optimized version, verified by TestRuneWidthChecksums()
- if !c.EastAsianWidth {
- switch {
- case r < 0x20:
- return 0
- case (r >= 0x7F && r <= 0x9F) || r == 0xAD: // nonprint
- return 0
- case r < 0x300:
- return 1
- case inTable(r, narrow):
- return 1
- case inTables(r, nonprint, combining):
- return 0
- case inTable(r, doublewidth):
- return 2
- default:
- return 1
- }
- } else {
- switch {
- case inTables(r, nonprint, combining):
- return 0
- case inTable(r, narrow):
- return 1
- case inTables(r, ambiguous, doublewidth):
- return 2
- case !c.StrictEmojiNeutral && inTables(r, ambiguous, emoji, narrow):
- return 2
- default:
- return 1
- }
- }
-}
-
-// CreateLUT will create an in-memory lookup table of 557056 bytes for faster operation.
-// This should not be called concurrently with other operations on c.
-// If options in c is changed, CreateLUT should be called again.
-func (c *Condition) CreateLUT() {
- const max = 0x110000
- lut := c.combinedLut
- if len(c.combinedLut) != 0 {
- // Remove so we don't use it.
- c.combinedLut = nil
- } else {
- lut = make([]byte, max/2)
- }
- for i := range lut {
- i32 := int32(i * 2)
- x0 := c.RuneWidth(i32)
- x1 := c.RuneWidth(i32 + 1)
- lut[i] = uint8(x0) | uint8(x1)<<4
- }
- c.combinedLut = lut
-}
-
-// StringWidth return width as you can see
-func (c *Condition) StringWidth(s string) (width int) {
- g := uniseg.NewGraphemes(s)
- for g.Next() {
- var chWidth int
- for _, r := range g.Runes() {
- chWidth = c.RuneWidth(r)
- if chWidth > 0 {
- break // Our best guess at this point is to use the width of the first non-zero-width rune.
- }
- }
- width += chWidth
- }
- return
-}
-
-// Truncate return string truncated with w cells
-func (c *Condition) Truncate(s string, w int, tail string) string {
- if c.StringWidth(s) <= w {
- return s
- }
- w -= c.StringWidth(tail)
- var width int
- pos := len(s)
- g := uniseg.NewGraphemes(s)
- for g.Next() {
- var chWidth int
- for _, r := range g.Runes() {
- chWidth = c.RuneWidth(r)
- if chWidth > 0 {
- break // See StringWidth() for details.
- }
- }
- if width+chWidth > w {
- pos, _ = g.Positions()
- break
- }
- width += chWidth
- }
- return s[:pos] + tail
-}
-
-// TruncateLeft cuts w cells from the beginning of the `s`.
-func (c *Condition) TruncateLeft(s string, w int, prefix string) string {
- if c.StringWidth(s) <= w {
- return prefix
- }
-
- var width int
- pos := len(s)
-
- g := uniseg.NewGraphemes(s)
- for g.Next() {
- var chWidth int
- for _, r := range g.Runes() {
- chWidth = c.RuneWidth(r)
- if chWidth > 0 {
- break // See StringWidth() for details.
- }
- }
-
- if width+chWidth > w {
- if width < w {
- _, pos = g.Positions()
- prefix += strings.Repeat(" ", width+chWidth-w)
- } else {
- pos, _ = g.Positions()
- }
-
- break
- }
-
- width += chWidth
- }
-
- return prefix + s[pos:]
-}
-
-// Wrap return string wrapped with w cells
-func (c *Condition) Wrap(s string, w int) string {
- width := 0
- out := ""
- for _, r := range s {
- cw := c.RuneWidth(r)
- if r == '\n' {
- out += string(r)
- width = 0
- continue
- } else if width+cw > w {
- out += "\n"
- width = 0
- out += string(r)
- width += cw
- continue
- }
- out += string(r)
- width += cw
- }
- return out
-}
-
-// FillLeft return string filled in left by spaces in w cells
-func (c *Condition) FillLeft(s string, w int) string {
- width := c.StringWidth(s)
- count := w - width
- if count > 0 {
- b := make([]byte, count)
- for i := range b {
- b[i] = ' '
- }
- return string(b) + s
- }
- return s
-}
-
-// FillRight return string filled in left by spaces in w cells
-func (c *Condition) FillRight(s string, w int) string {
- width := c.StringWidth(s)
- count := w - width
- if count > 0 {
- b := make([]byte, count)
- for i := range b {
- b[i] = ' '
- }
- return s + string(b)
- }
- return s
-}
-
-// RuneWidth returns the number of cells in r.
-// See http://www.unicode.org/reports/tr11/
-func RuneWidth(r rune) int {
- return DefaultCondition.RuneWidth(r)
-}
-
-// IsAmbiguousWidth returns whether is ambiguous width or not.
-func IsAmbiguousWidth(r rune) bool {
- return inTables(r, private, ambiguous)
-}
-
-// IsNeutralWidth returns whether is neutral width or not.
-func IsNeutralWidth(r rune) bool {
- return inTable(r, neutral)
-}
-
-// StringWidth return width as you can see
-func StringWidth(s string) (width int) {
- return DefaultCondition.StringWidth(s)
-}
-
-// Truncate return string truncated with w cells
-func Truncate(s string, w int, tail string) string {
- return DefaultCondition.Truncate(s, w, tail)
-}
-
-// TruncateLeft cuts w cells from the beginning of the `s`.
-func TruncateLeft(s string, w int, prefix string) string {
- return DefaultCondition.TruncateLeft(s, w, prefix)
-}
-
-// Wrap return string wrapped with w cells
-func Wrap(s string, w int) string {
- return DefaultCondition.Wrap(s, w)
-}
-
-// FillLeft return string filled in left by spaces in w cells
-func FillLeft(s string, w int) string {
- return DefaultCondition.FillLeft(s, w)
-}
-
-// FillRight return string filled in left by spaces in w cells
-func FillRight(s string, w int) string {
- return DefaultCondition.FillRight(s, w)
-}
-
-// CreateLUT will create an in-memory lookup table of 557055 bytes for faster operation.
-// This should not be called concurrently with other operations.
-func CreateLUT() {
- if len(DefaultCondition.combinedLut) > 0 {
- return
- }
- DefaultCondition.CreateLUT()
-}
diff --git a/vendor/github.com/mattn/go-runewidth/runewidth_appengine.go b/vendor/github.com/mattn/go-runewidth/runewidth_appengine.go
deleted file mode 100644
index 84b6528d..00000000
--- a/vendor/github.com/mattn/go-runewidth/runewidth_appengine.go
+++ /dev/null
@@ -1,9 +0,0 @@
-//go:build appengine
-// +build appengine
-
-package runewidth
-
-// IsEastAsian return true if the current locale is CJK
-func IsEastAsian() bool {
- return false
-}
diff --git a/vendor/github.com/mattn/go-runewidth/runewidth_js.go b/vendor/github.com/mattn/go-runewidth/runewidth_js.go
deleted file mode 100644
index c2abbc2d..00000000
--- a/vendor/github.com/mattn/go-runewidth/runewidth_js.go
+++ /dev/null
@@ -1,9 +0,0 @@
-//go:build js && !appengine
-// +build js,!appengine
-
-package runewidth
-
-func IsEastAsian() bool {
- // TODO: Implement this for the web. Detect east asian in a compatible way, and return true.
- return false
-}
diff --git a/vendor/github.com/mattn/go-runewidth/runewidth_posix.go b/vendor/github.com/mattn/go-runewidth/runewidth_posix.go
deleted file mode 100644
index 5a31d738..00000000
--- a/vendor/github.com/mattn/go-runewidth/runewidth_posix.go
+++ /dev/null
@@ -1,81 +0,0 @@
-//go:build !windows && !js && !appengine
-// +build !windows,!js,!appengine
-
-package runewidth
-
-import (
- "os"
- "regexp"
- "strings"
-)
-
-var reLoc = regexp.MustCompile(`^[a-z][a-z][a-z]?(?:_[A-Z][A-Z])?\.(.+)`)
-
-var mblenTable = map[string]int{
- "utf-8": 6,
- "utf8": 6,
- "jis": 8,
- "eucjp": 3,
- "euckr": 2,
- "euccn": 2,
- "sjis": 2,
- "cp932": 2,
- "cp51932": 2,
- "cp936": 2,
- "cp949": 2,
- "cp950": 2,
- "big5": 2,
- "gbk": 2,
- "gb2312": 2,
-}
-
-func isEastAsian(locale string) bool {
- charset := strings.ToLower(locale)
- r := reLoc.FindStringSubmatch(locale)
- if len(r) == 2 {
- charset = strings.ToLower(r[1])
- }
-
- if strings.HasSuffix(charset, "@cjk_narrow") {
- return false
- }
-
- for pos, b := range []byte(charset) {
- if b == '@' {
- charset = charset[:pos]
- break
- }
- }
- max := 1
- if m, ok := mblenTable[charset]; ok {
- max = m
- }
- if max > 1 && (charset[0] != 'u' ||
- strings.HasPrefix(locale, "ja") ||
- strings.HasPrefix(locale, "ko") ||
- strings.HasPrefix(locale, "zh")) {
- return true
- }
- return false
-}
-
-// IsEastAsian return true if the current locale is CJK
-func IsEastAsian() bool {
- locale := os.Getenv("LC_ALL")
- if locale == "" {
- locale = os.Getenv("LC_CTYPE")
- }
- if locale == "" {
- locale = os.Getenv("LANG")
- }
-
- // ignore C locale
- if locale == "POSIX" || locale == "C" {
- return false
- }
- if len(locale) > 1 && locale[0] == 'C' && (locale[1] == '.' || locale[1] == '-') {
- return false
- }
-
- return isEastAsian(locale)
-}
diff --git a/vendor/github.com/mattn/go-runewidth/runewidth_table.go b/vendor/github.com/mattn/go-runewidth/runewidth_table.go
deleted file mode 100644
index e5d890c2..00000000
--- a/vendor/github.com/mattn/go-runewidth/runewidth_table.go
+++ /dev/null
@@ -1,439 +0,0 @@
-// Code generated by script/generate.go. DO NOT EDIT.
-
-package runewidth
-
-var combining = table{
- {0x0300, 0x036F}, {0x0483, 0x0489}, {0x07EB, 0x07F3},
- {0x0C00, 0x0C00}, {0x0C04, 0x0C04}, {0x0D00, 0x0D01},
- {0x135D, 0x135F}, {0x1A7F, 0x1A7F}, {0x1AB0, 0x1AC0},
- {0x1B6B, 0x1B73}, {0x1DC0, 0x1DF9}, {0x1DFB, 0x1DFF},
- {0x20D0, 0x20F0}, {0x2CEF, 0x2CF1}, {0x2DE0, 0x2DFF},
- {0x3099, 0x309A}, {0xA66F, 0xA672}, {0xA674, 0xA67D},
- {0xA69E, 0xA69F}, {0xA6F0, 0xA6F1}, {0xA8E0, 0xA8F1},
- {0xFE20, 0xFE2F}, {0x101FD, 0x101FD}, {0x10376, 0x1037A},
- {0x10EAB, 0x10EAC}, {0x10F46, 0x10F50}, {0x11300, 0x11301},
- {0x1133B, 0x1133C}, {0x11366, 0x1136C}, {0x11370, 0x11374},
- {0x16AF0, 0x16AF4}, {0x1D165, 0x1D169}, {0x1D16D, 0x1D172},
- {0x1D17B, 0x1D182}, {0x1D185, 0x1D18B}, {0x1D1AA, 0x1D1AD},
- {0x1D242, 0x1D244}, {0x1E000, 0x1E006}, {0x1E008, 0x1E018},
- {0x1E01B, 0x1E021}, {0x1E023, 0x1E024}, {0x1E026, 0x1E02A},
- {0x1E8D0, 0x1E8D6},
-}
-
-var doublewidth = table{
- {0x1100, 0x115F}, {0x231A, 0x231B}, {0x2329, 0x232A},
- {0x23E9, 0x23EC}, {0x23F0, 0x23F0}, {0x23F3, 0x23F3},
- {0x25FD, 0x25FE}, {0x2614, 0x2615}, {0x2648, 0x2653},
- {0x267F, 0x267F}, {0x2693, 0x2693}, {0x26A1, 0x26A1},
- {0x26AA, 0x26AB}, {0x26BD, 0x26BE}, {0x26C4, 0x26C5},
- {0x26CE, 0x26CE}, {0x26D4, 0x26D4}, {0x26EA, 0x26EA},
- {0x26F2, 0x26F3}, {0x26F5, 0x26F5}, {0x26FA, 0x26FA},
- {0x26FD, 0x26FD}, {0x2705, 0x2705}, {0x270A, 0x270B},
- {0x2728, 0x2728}, {0x274C, 0x274C}, {0x274E, 0x274E},
- {0x2753, 0x2755}, {0x2757, 0x2757}, {0x2795, 0x2797},
- {0x27B0, 0x27B0}, {0x27BF, 0x27BF}, {0x2B1B, 0x2B1C},
- {0x2B50, 0x2B50}, {0x2B55, 0x2B55}, {0x2E80, 0x2E99},
- {0x2E9B, 0x2EF3}, {0x2F00, 0x2FD5}, {0x2FF0, 0x2FFB},
- {0x3000, 0x303E}, {0x3041, 0x3096}, {0x3099, 0x30FF},
- {0x3105, 0x312F}, {0x3131, 0x318E}, {0x3190, 0x31E3},
- {0x31F0, 0x321E}, {0x3220, 0x3247}, {0x3250, 0x4DBF},
- {0x4E00, 0xA48C}, {0xA490, 0xA4C6}, {0xA960, 0xA97C},
- {0xAC00, 0xD7A3}, {0xF900, 0xFAFF}, {0xFE10, 0xFE19},
- {0xFE30, 0xFE52}, {0xFE54, 0xFE66}, {0xFE68, 0xFE6B},
- {0xFF01, 0xFF60}, {0xFFE0, 0xFFE6}, {0x16FE0, 0x16FE4},
- {0x16FF0, 0x16FF1}, {0x17000, 0x187F7}, {0x18800, 0x18CD5},
- {0x18D00, 0x18D08}, {0x1B000, 0x1B11E}, {0x1B150, 0x1B152},
- {0x1B164, 0x1B167}, {0x1B170, 0x1B2FB}, {0x1F004, 0x1F004},
- {0x1F0CF, 0x1F0CF}, {0x1F18E, 0x1F18E}, {0x1F191, 0x1F19A},
- {0x1F200, 0x1F202}, {0x1F210, 0x1F23B}, {0x1F240, 0x1F248},
- {0x1F250, 0x1F251}, {0x1F260, 0x1F265}, {0x1F300, 0x1F320},
- {0x1F32D, 0x1F335}, {0x1F337, 0x1F37C}, {0x1F37E, 0x1F393},
- {0x1F3A0, 0x1F3CA}, {0x1F3CF, 0x1F3D3}, {0x1F3E0, 0x1F3F0},
- {0x1F3F4, 0x1F3F4}, {0x1F3F8, 0x1F43E}, {0x1F440, 0x1F440},
- {0x1F442, 0x1F4FC}, {0x1F4FF, 0x1F53D}, {0x1F54B, 0x1F54E},
- {0x1F550, 0x1F567}, {0x1F57A, 0x1F57A}, {0x1F595, 0x1F596},
- {0x1F5A4, 0x1F5A4}, {0x1F5FB, 0x1F64F}, {0x1F680, 0x1F6C5},
- {0x1F6CC, 0x1F6CC}, {0x1F6D0, 0x1F6D2}, {0x1F6D5, 0x1F6D7},
- {0x1F6EB, 0x1F6EC}, {0x1F6F4, 0x1F6FC}, {0x1F7E0, 0x1F7EB},
- {0x1F90C, 0x1F93A}, {0x1F93C, 0x1F945}, {0x1F947, 0x1F978},
- {0x1F97A, 0x1F9CB}, {0x1F9CD, 0x1F9FF}, {0x1FA70, 0x1FA74},
- {0x1FA78, 0x1FA7A}, {0x1FA80, 0x1FA86}, {0x1FA90, 0x1FAA8},
- {0x1FAB0, 0x1FAB6}, {0x1FAC0, 0x1FAC2}, {0x1FAD0, 0x1FAD6},
- {0x20000, 0x2FFFD}, {0x30000, 0x3FFFD},
-}
-
-var ambiguous = table{
- {0x00A1, 0x00A1}, {0x00A4, 0x00A4}, {0x00A7, 0x00A8},
- {0x00AA, 0x00AA}, {0x00AD, 0x00AE}, {0x00B0, 0x00B4},
- {0x00B6, 0x00BA}, {0x00BC, 0x00BF}, {0x00C6, 0x00C6},
- {0x00D0, 0x00D0}, {0x00D7, 0x00D8}, {0x00DE, 0x00E1},
- {0x00E6, 0x00E6}, {0x00E8, 0x00EA}, {0x00EC, 0x00ED},
- {0x00F0, 0x00F0}, {0x00F2, 0x00F3}, {0x00F7, 0x00FA},
- {0x00FC, 0x00FC}, {0x00FE, 0x00FE}, {0x0101, 0x0101},
- {0x0111, 0x0111}, {0x0113, 0x0113}, {0x011B, 0x011B},
- {0x0126, 0x0127}, {0x012B, 0x012B}, {0x0131, 0x0133},
- {0x0138, 0x0138}, {0x013F, 0x0142}, {0x0144, 0x0144},
- {0x0148, 0x014B}, {0x014D, 0x014D}, {0x0152, 0x0153},
- {0x0166, 0x0167}, {0x016B, 0x016B}, {0x01CE, 0x01CE},
- {0x01D0, 0x01D0}, {0x01D2, 0x01D2}, {0x01D4, 0x01D4},
- {0x01D6, 0x01D6}, {0x01D8, 0x01D8}, {0x01DA, 0x01DA},
- {0x01DC, 0x01DC}, {0x0251, 0x0251}, {0x0261, 0x0261},
- {0x02C4, 0x02C4}, {0x02C7, 0x02C7}, {0x02C9, 0x02CB},
- {0x02CD, 0x02CD}, {0x02D0, 0x02D0}, {0x02D8, 0x02DB},
- {0x02DD, 0x02DD}, {0x02DF, 0x02DF}, {0x0300, 0x036F},
- {0x0391, 0x03A1}, {0x03A3, 0x03A9}, {0x03B1, 0x03C1},
- {0x03C3, 0x03C9}, {0x0401, 0x0401}, {0x0410, 0x044F},
- {0x0451, 0x0451}, {0x2010, 0x2010}, {0x2013, 0x2016},
- {0x2018, 0x2019}, {0x201C, 0x201D}, {0x2020, 0x2022},
- {0x2024, 0x2027}, {0x2030, 0x2030}, {0x2032, 0x2033},
- {0x2035, 0x2035}, {0x203B, 0x203B}, {0x203E, 0x203E},
- {0x2074, 0x2074}, {0x207F, 0x207F}, {0x2081, 0x2084},
- {0x20AC, 0x20AC}, {0x2103, 0x2103}, {0x2105, 0x2105},
- {0x2109, 0x2109}, {0x2113, 0x2113}, {0x2116, 0x2116},
- {0x2121, 0x2122}, {0x2126, 0x2126}, {0x212B, 0x212B},
- {0x2153, 0x2154}, {0x215B, 0x215E}, {0x2160, 0x216B},
- {0x2170, 0x2179}, {0x2189, 0x2189}, {0x2190, 0x2199},
- {0x21B8, 0x21B9}, {0x21D2, 0x21D2}, {0x21D4, 0x21D4},
- {0x21E7, 0x21E7}, {0x2200, 0x2200}, {0x2202, 0x2203},
- {0x2207, 0x2208}, {0x220B, 0x220B}, {0x220F, 0x220F},
- {0x2211, 0x2211}, {0x2215, 0x2215}, {0x221A, 0x221A},
- {0x221D, 0x2220}, {0x2223, 0x2223}, {0x2225, 0x2225},
- {0x2227, 0x222C}, {0x222E, 0x222E}, {0x2234, 0x2237},
- {0x223C, 0x223D}, {0x2248, 0x2248}, {0x224C, 0x224C},
- {0x2252, 0x2252}, {0x2260, 0x2261}, {0x2264, 0x2267},
- {0x226A, 0x226B}, {0x226E, 0x226F}, {0x2282, 0x2283},
- {0x2286, 0x2287}, {0x2295, 0x2295}, {0x2299, 0x2299},
- {0x22A5, 0x22A5}, {0x22BF, 0x22BF}, {0x2312, 0x2312},
- {0x2460, 0x24E9}, {0x24EB, 0x254B}, {0x2550, 0x2573},
- {0x2580, 0x258F}, {0x2592, 0x2595}, {0x25A0, 0x25A1},
- {0x25A3, 0x25A9}, {0x25B2, 0x25B3}, {0x25B6, 0x25B7},
- {0x25BC, 0x25BD}, {0x25C0, 0x25C1}, {0x25C6, 0x25C8},
- {0x25CB, 0x25CB}, {0x25CE, 0x25D1}, {0x25E2, 0x25E5},
- {0x25EF, 0x25EF}, {0x2605, 0x2606}, {0x2609, 0x2609},
- {0x260E, 0x260F}, {0x261C, 0x261C}, {0x261E, 0x261E},
- {0x2640, 0x2640}, {0x2642, 0x2642}, {0x2660, 0x2661},
- {0x2663, 0x2665}, {0x2667, 0x266A}, {0x266C, 0x266D},
- {0x266F, 0x266F}, {0x269E, 0x269F}, {0x26BF, 0x26BF},
- {0x26C6, 0x26CD}, {0x26CF, 0x26D3}, {0x26D5, 0x26E1},
- {0x26E3, 0x26E3}, {0x26E8, 0x26E9}, {0x26EB, 0x26F1},
- {0x26F4, 0x26F4}, {0x26F6, 0x26F9}, {0x26FB, 0x26FC},
- {0x26FE, 0x26FF}, {0x273D, 0x273D}, {0x2776, 0x277F},
- {0x2B56, 0x2B59}, {0x3248, 0x324F}, {0xE000, 0xF8FF},
- {0xFE00, 0xFE0F}, {0xFFFD, 0xFFFD}, {0x1F100, 0x1F10A},
- {0x1F110, 0x1F12D}, {0x1F130, 0x1F169}, {0x1F170, 0x1F18D},
- {0x1F18F, 0x1F190}, {0x1F19B, 0x1F1AC}, {0xE0100, 0xE01EF},
- {0xF0000, 0xFFFFD}, {0x100000, 0x10FFFD},
-}
-var narrow = table{
- {0x0020, 0x007E}, {0x00A2, 0x00A3}, {0x00A5, 0x00A6},
- {0x00AC, 0x00AC}, {0x00AF, 0x00AF}, {0x27E6, 0x27ED},
- {0x2985, 0x2986},
-}
-
-var neutral = table{
- {0x0000, 0x001F}, {0x007F, 0x00A0}, {0x00A9, 0x00A9},
- {0x00AB, 0x00AB}, {0x00B5, 0x00B5}, {0x00BB, 0x00BB},
- {0x00C0, 0x00C5}, {0x00C7, 0x00CF}, {0x00D1, 0x00D6},
- {0x00D9, 0x00DD}, {0x00E2, 0x00E5}, {0x00E7, 0x00E7},
- {0x00EB, 0x00EB}, {0x00EE, 0x00EF}, {0x00F1, 0x00F1},
- {0x00F4, 0x00F6}, {0x00FB, 0x00FB}, {0x00FD, 0x00FD},
- {0x00FF, 0x0100}, {0x0102, 0x0110}, {0x0112, 0x0112},
- {0x0114, 0x011A}, {0x011C, 0x0125}, {0x0128, 0x012A},
- {0x012C, 0x0130}, {0x0134, 0x0137}, {0x0139, 0x013E},
- {0x0143, 0x0143}, {0x0145, 0x0147}, {0x014C, 0x014C},
- {0x014E, 0x0151}, {0x0154, 0x0165}, {0x0168, 0x016A},
- {0x016C, 0x01CD}, {0x01CF, 0x01CF}, {0x01D1, 0x01D1},
- {0x01D3, 0x01D3}, {0x01D5, 0x01D5}, {0x01D7, 0x01D7},
- {0x01D9, 0x01D9}, {0x01DB, 0x01DB}, {0x01DD, 0x0250},
- {0x0252, 0x0260}, {0x0262, 0x02C3}, {0x02C5, 0x02C6},
- {0x02C8, 0x02C8}, {0x02CC, 0x02CC}, {0x02CE, 0x02CF},
- {0x02D1, 0x02D7}, {0x02DC, 0x02DC}, {0x02DE, 0x02DE},
- {0x02E0, 0x02FF}, {0x0370, 0x0377}, {0x037A, 0x037F},
- {0x0384, 0x038A}, {0x038C, 0x038C}, {0x038E, 0x0390},
- {0x03AA, 0x03B0}, {0x03C2, 0x03C2}, {0x03CA, 0x0400},
- {0x0402, 0x040F}, {0x0450, 0x0450}, {0x0452, 0x052F},
- {0x0531, 0x0556}, {0x0559, 0x058A}, {0x058D, 0x058F},
- {0x0591, 0x05C7}, {0x05D0, 0x05EA}, {0x05EF, 0x05F4},
- {0x0600, 0x061C}, {0x061E, 0x070D}, {0x070F, 0x074A},
- {0x074D, 0x07B1}, {0x07C0, 0x07FA}, {0x07FD, 0x082D},
- {0x0830, 0x083E}, {0x0840, 0x085B}, {0x085E, 0x085E},
- {0x0860, 0x086A}, {0x08A0, 0x08B4}, {0x08B6, 0x08C7},
- {0x08D3, 0x0983}, {0x0985, 0x098C}, {0x098F, 0x0990},
- {0x0993, 0x09A8}, {0x09AA, 0x09B0}, {0x09B2, 0x09B2},
- {0x09B6, 0x09B9}, {0x09BC, 0x09C4}, {0x09C7, 0x09C8},
- {0x09CB, 0x09CE}, {0x09D7, 0x09D7}, {0x09DC, 0x09DD},
- {0x09DF, 0x09E3}, {0x09E6, 0x09FE}, {0x0A01, 0x0A03},
- {0x0A05, 0x0A0A}, {0x0A0F, 0x0A10}, {0x0A13, 0x0A28},
- {0x0A2A, 0x0A30}, {0x0A32, 0x0A33}, {0x0A35, 0x0A36},
- {0x0A38, 0x0A39}, {0x0A3C, 0x0A3C}, {0x0A3E, 0x0A42},
- {0x0A47, 0x0A48}, {0x0A4B, 0x0A4D}, {0x0A51, 0x0A51},
- {0x0A59, 0x0A5C}, {0x0A5E, 0x0A5E}, {0x0A66, 0x0A76},
- {0x0A81, 0x0A83}, {0x0A85, 0x0A8D}, {0x0A8F, 0x0A91},
- {0x0A93, 0x0AA8}, {0x0AAA, 0x0AB0}, {0x0AB2, 0x0AB3},
- {0x0AB5, 0x0AB9}, {0x0ABC, 0x0AC5}, {0x0AC7, 0x0AC9},
- {0x0ACB, 0x0ACD}, {0x0AD0, 0x0AD0}, {0x0AE0, 0x0AE3},
- {0x0AE6, 0x0AF1}, {0x0AF9, 0x0AFF}, {0x0B01, 0x0B03},
- {0x0B05, 0x0B0C}, {0x0B0F, 0x0B10}, {0x0B13, 0x0B28},
- {0x0B2A, 0x0B30}, {0x0B32, 0x0B33}, {0x0B35, 0x0B39},
- {0x0B3C, 0x0B44}, {0x0B47, 0x0B48}, {0x0B4B, 0x0B4D},
- {0x0B55, 0x0B57}, {0x0B5C, 0x0B5D}, {0x0B5F, 0x0B63},
- {0x0B66, 0x0B77}, {0x0B82, 0x0B83}, {0x0B85, 0x0B8A},
- {0x0B8E, 0x0B90}, {0x0B92, 0x0B95}, {0x0B99, 0x0B9A},
- {0x0B9C, 0x0B9C}, {0x0B9E, 0x0B9F}, {0x0BA3, 0x0BA4},
- {0x0BA8, 0x0BAA}, {0x0BAE, 0x0BB9}, {0x0BBE, 0x0BC2},
- {0x0BC6, 0x0BC8}, {0x0BCA, 0x0BCD}, {0x0BD0, 0x0BD0},
- {0x0BD7, 0x0BD7}, {0x0BE6, 0x0BFA}, {0x0C00, 0x0C0C},
- {0x0C0E, 0x0C10}, {0x0C12, 0x0C28}, {0x0C2A, 0x0C39},
- {0x0C3D, 0x0C44}, {0x0C46, 0x0C48}, {0x0C4A, 0x0C4D},
- {0x0C55, 0x0C56}, {0x0C58, 0x0C5A}, {0x0C60, 0x0C63},
- {0x0C66, 0x0C6F}, {0x0C77, 0x0C8C}, {0x0C8E, 0x0C90},
- {0x0C92, 0x0CA8}, {0x0CAA, 0x0CB3}, {0x0CB5, 0x0CB9},
- {0x0CBC, 0x0CC4}, {0x0CC6, 0x0CC8}, {0x0CCA, 0x0CCD},
- {0x0CD5, 0x0CD6}, {0x0CDE, 0x0CDE}, {0x0CE0, 0x0CE3},
- {0x0CE6, 0x0CEF}, {0x0CF1, 0x0CF2}, {0x0D00, 0x0D0C},
- {0x0D0E, 0x0D10}, {0x0D12, 0x0D44}, {0x0D46, 0x0D48},
- {0x0D4A, 0x0D4F}, {0x0D54, 0x0D63}, {0x0D66, 0x0D7F},
- {0x0D81, 0x0D83}, {0x0D85, 0x0D96}, {0x0D9A, 0x0DB1},
- {0x0DB3, 0x0DBB}, {0x0DBD, 0x0DBD}, {0x0DC0, 0x0DC6},
- {0x0DCA, 0x0DCA}, {0x0DCF, 0x0DD4}, {0x0DD6, 0x0DD6},
- {0x0DD8, 0x0DDF}, {0x0DE6, 0x0DEF}, {0x0DF2, 0x0DF4},
- {0x0E01, 0x0E3A}, {0x0E3F, 0x0E5B}, {0x0E81, 0x0E82},
- {0x0E84, 0x0E84}, {0x0E86, 0x0E8A}, {0x0E8C, 0x0EA3},
- {0x0EA5, 0x0EA5}, {0x0EA7, 0x0EBD}, {0x0EC0, 0x0EC4},
- {0x0EC6, 0x0EC6}, {0x0EC8, 0x0ECD}, {0x0ED0, 0x0ED9},
- {0x0EDC, 0x0EDF}, {0x0F00, 0x0F47}, {0x0F49, 0x0F6C},
- {0x0F71, 0x0F97}, {0x0F99, 0x0FBC}, {0x0FBE, 0x0FCC},
- {0x0FCE, 0x0FDA}, {0x1000, 0x10C5}, {0x10C7, 0x10C7},
- {0x10CD, 0x10CD}, {0x10D0, 0x10FF}, {0x1160, 0x1248},
- {0x124A, 0x124D}, {0x1250, 0x1256}, {0x1258, 0x1258},
- {0x125A, 0x125D}, {0x1260, 0x1288}, {0x128A, 0x128D},
- {0x1290, 0x12B0}, {0x12B2, 0x12B5}, {0x12B8, 0x12BE},
- {0x12C0, 0x12C0}, {0x12C2, 0x12C5}, {0x12C8, 0x12D6},
- {0x12D8, 0x1310}, {0x1312, 0x1315}, {0x1318, 0x135A},
- {0x135D, 0x137C}, {0x1380, 0x1399}, {0x13A0, 0x13F5},
- {0x13F8, 0x13FD}, {0x1400, 0x169C}, {0x16A0, 0x16F8},
- {0x1700, 0x170C}, {0x170E, 0x1714}, {0x1720, 0x1736},
- {0x1740, 0x1753}, {0x1760, 0x176C}, {0x176E, 0x1770},
- {0x1772, 0x1773}, {0x1780, 0x17DD}, {0x17E0, 0x17E9},
- {0x17F0, 0x17F9}, {0x1800, 0x180E}, {0x1810, 0x1819},
- {0x1820, 0x1878}, {0x1880, 0x18AA}, {0x18B0, 0x18F5},
- {0x1900, 0x191E}, {0x1920, 0x192B}, {0x1930, 0x193B},
- {0x1940, 0x1940}, {0x1944, 0x196D}, {0x1970, 0x1974},
- {0x1980, 0x19AB}, {0x19B0, 0x19C9}, {0x19D0, 0x19DA},
- {0x19DE, 0x1A1B}, {0x1A1E, 0x1A5E}, {0x1A60, 0x1A7C},
- {0x1A7F, 0x1A89}, {0x1A90, 0x1A99}, {0x1AA0, 0x1AAD},
- {0x1AB0, 0x1AC0}, {0x1B00, 0x1B4B}, {0x1B50, 0x1B7C},
- {0x1B80, 0x1BF3}, {0x1BFC, 0x1C37}, {0x1C3B, 0x1C49},
- {0x1C4D, 0x1C88}, {0x1C90, 0x1CBA}, {0x1CBD, 0x1CC7},
- {0x1CD0, 0x1CFA}, {0x1D00, 0x1DF9}, {0x1DFB, 0x1F15},
- {0x1F18, 0x1F1D}, {0x1F20, 0x1F45}, {0x1F48, 0x1F4D},
- {0x1F50, 0x1F57}, {0x1F59, 0x1F59}, {0x1F5B, 0x1F5B},
- {0x1F5D, 0x1F5D}, {0x1F5F, 0x1F7D}, {0x1F80, 0x1FB4},
- {0x1FB6, 0x1FC4}, {0x1FC6, 0x1FD3}, {0x1FD6, 0x1FDB},
- {0x1FDD, 0x1FEF}, {0x1FF2, 0x1FF4}, {0x1FF6, 0x1FFE},
- {0x2000, 0x200F}, {0x2011, 0x2012}, {0x2017, 0x2017},
- {0x201A, 0x201B}, {0x201E, 0x201F}, {0x2023, 0x2023},
- {0x2028, 0x202F}, {0x2031, 0x2031}, {0x2034, 0x2034},
- {0x2036, 0x203A}, {0x203C, 0x203D}, {0x203F, 0x2064},
- {0x2066, 0x2071}, {0x2075, 0x207E}, {0x2080, 0x2080},
- {0x2085, 0x208E}, {0x2090, 0x209C}, {0x20A0, 0x20A8},
- {0x20AA, 0x20AB}, {0x20AD, 0x20BF}, {0x20D0, 0x20F0},
- {0x2100, 0x2102}, {0x2104, 0x2104}, {0x2106, 0x2108},
- {0x210A, 0x2112}, {0x2114, 0x2115}, {0x2117, 0x2120},
- {0x2123, 0x2125}, {0x2127, 0x212A}, {0x212C, 0x2152},
- {0x2155, 0x215A}, {0x215F, 0x215F}, {0x216C, 0x216F},
- {0x217A, 0x2188}, {0x218A, 0x218B}, {0x219A, 0x21B7},
- {0x21BA, 0x21D1}, {0x21D3, 0x21D3}, {0x21D5, 0x21E6},
- {0x21E8, 0x21FF}, {0x2201, 0x2201}, {0x2204, 0x2206},
- {0x2209, 0x220A}, {0x220C, 0x220E}, {0x2210, 0x2210},
- {0x2212, 0x2214}, {0x2216, 0x2219}, {0x221B, 0x221C},
- {0x2221, 0x2222}, {0x2224, 0x2224}, {0x2226, 0x2226},
- {0x222D, 0x222D}, {0x222F, 0x2233}, {0x2238, 0x223B},
- {0x223E, 0x2247}, {0x2249, 0x224B}, {0x224D, 0x2251},
- {0x2253, 0x225F}, {0x2262, 0x2263}, {0x2268, 0x2269},
- {0x226C, 0x226D}, {0x2270, 0x2281}, {0x2284, 0x2285},
- {0x2288, 0x2294}, {0x2296, 0x2298}, {0x229A, 0x22A4},
- {0x22A6, 0x22BE}, {0x22C0, 0x2311}, {0x2313, 0x2319},
- {0x231C, 0x2328}, {0x232B, 0x23E8}, {0x23ED, 0x23EF},
- {0x23F1, 0x23F2}, {0x23F4, 0x2426}, {0x2440, 0x244A},
- {0x24EA, 0x24EA}, {0x254C, 0x254F}, {0x2574, 0x257F},
- {0x2590, 0x2591}, {0x2596, 0x259F}, {0x25A2, 0x25A2},
- {0x25AA, 0x25B1}, {0x25B4, 0x25B5}, {0x25B8, 0x25BB},
- {0x25BE, 0x25BF}, {0x25C2, 0x25C5}, {0x25C9, 0x25CA},
- {0x25CC, 0x25CD}, {0x25D2, 0x25E1}, {0x25E6, 0x25EE},
- {0x25F0, 0x25FC}, {0x25FF, 0x2604}, {0x2607, 0x2608},
- {0x260A, 0x260D}, {0x2610, 0x2613}, {0x2616, 0x261B},
- {0x261D, 0x261D}, {0x261F, 0x263F}, {0x2641, 0x2641},
- {0x2643, 0x2647}, {0x2654, 0x265F}, {0x2662, 0x2662},
- {0x2666, 0x2666}, {0x266B, 0x266B}, {0x266E, 0x266E},
- {0x2670, 0x267E}, {0x2680, 0x2692}, {0x2694, 0x269D},
- {0x26A0, 0x26A0}, {0x26A2, 0x26A9}, {0x26AC, 0x26BC},
- {0x26C0, 0x26C3}, {0x26E2, 0x26E2}, {0x26E4, 0x26E7},
- {0x2700, 0x2704}, {0x2706, 0x2709}, {0x270C, 0x2727},
- {0x2729, 0x273C}, {0x273E, 0x274B}, {0x274D, 0x274D},
- {0x274F, 0x2752}, {0x2756, 0x2756}, {0x2758, 0x2775},
- {0x2780, 0x2794}, {0x2798, 0x27AF}, {0x27B1, 0x27BE},
- {0x27C0, 0x27E5}, {0x27EE, 0x2984}, {0x2987, 0x2B1A},
- {0x2B1D, 0x2B4F}, {0x2B51, 0x2B54}, {0x2B5A, 0x2B73},
- {0x2B76, 0x2B95}, {0x2B97, 0x2C2E}, {0x2C30, 0x2C5E},
- {0x2C60, 0x2CF3}, {0x2CF9, 0x2D25}, {0x2D27, 0x2D27},
- {0x2D2D, 0x2D2D}, {0x2D30, 0x2D67}, {0x2D6F, 0x2D70},
- {0x2D7F, 0x2D96}, {0x2DA0, 0x2DA6}, {0x2DA8, 0x2DAE},
- {0x2DB0, 0x2DB6}, {0x2DB8, 0x2DBE}, {0x2DC0, 0x2DC6},
- {0x2DC8, 0x2DCE}, {0x2DD0, 0x2DD6}, {0x2DD8, 0x2DDE},
- {0x2DE0, 0x2E52}, {0x303F, 0x303F}, {0x4DC0, 0x4DFF},
- {0xA4D0, 0xA62B}, {0xA640, 0xA6F7}, {0xA700, 0xA7BF},
- {0xA7C2, 0xA7CA}, {0xA7F5, 0xA82C}, {0xA830, 0xA839},
- {0xA840, 0xA877}, {0xA880, 0xA8C5}, {0xA8CE, 0xA8D9},
- {0xA8E0, 0xA953}, {0xA95F, 0xA95F}, {0xA980, 0xA9CD},
- {0xA9CF, 0xA9D9}, {0xA9DE, 0xA9FE}, {0xAA00, 0xAA36},
- {0xAA40, 0xAA4D}, {0xAA50, 0xAA59}, {0xAA5C, 0xAAC2},
- {0xAADB, 0xAAF6}, {0xAB01, 0xAB06}, {0xAB09, 0xAB0E},
- {0xAB11, 0xAB16}, {0xAB20, 0xAB26}, {0xAB28, 0xAB2E},
- {0xAB30, 0xAB6B}, {0xAB70, 0xABED}, {0xABF0, 0xABF9},
- {0xD7B0, 0xD7C6}, {0xD7CB, 0xD7FB}, {0xD800, 0xDFFF},
- {0xFB00, 0xFB06}, {0xFB13, 0xFB17}, {0xFB1D, 0xFB36},
- {0xFB38, 0xFB3C}, {0xFB3E, 0xFB3E}, {0xFB40, 0xFB41},
- {0xFB43, 0xFB44}, {0xFB46, 0xFBC1}, {0xFBD3, 0xFD3F},
- {0xFD50, 0xFD8F}, {0xFD92, 0xFDC7}, {0xFDF0, 0xFDFD},
- {0xFE20, 0xFE2F}, {0xFE70, 0xFE74}, {0xFE76, 0xFEFC},
- {0xFEFF, 0xFEFF}, {0xFFF9, 0xFFFC}, {0x10000, 0x1000B},
- {0x1000D, 0x10026}, {0x10028, 0x1003A}, {0x1003C, 0x1003D},
- {0x1003F, 0x1004D}, {0x10050, 0x1005D}, {0x10080, 0x100FA},
- {0x10100, 0x10102}, {0x10107, 0x10133}, {0x10137, 0x1018E},
- {0x10190, 0x1019C}, {0x101A0, 0x101A0}, {0x101D0, 0x101FD},
- {0x10280, 0x1029C}, {0x102A0, 0x102D0}, {0x102E0, 0x102FB},
- {0x10300, 0x10323}, {0x1032D, 0x1034A}, {0x10350, 0x1037A},
- {0x10380, 0x1039D}, {0x1039F, 0x103C3}, {0x103C8, 0x103D5},
- {0x10400, 0x1049D}, {0x104A0, 0x104A9}, {0x104B0, 0x104D3},
- {0x104D8, 0x104FB}, {0x10500, 0x10527}, {0x10530, 0x10563},
- {0x1056F, 0x1056F}, {0x10600, 0x10736}, {0x10740, 0x10755},
- {0x10760, 0x10767}, {0x10800, 0x10805}, {0x10808, 0x10808},
- {0x1080A, 0x10835}, {0x10837, 0x10838}, {0x1083C, 0x1083C},
- {0x1083F, 0x10855}, {0x10857, 0x1089E}, {0x108A7, 0x108AF},
- {0x108E0, 0x108F2}, {0x108F4, 0x108F5}, {0x108FB, 0x1091B},
- {0x1091F, 0x10939}, {0x1093F, 0x1093F}, {0x10980, 0x109B7},
- {0x109BC, 0x109CF}, {0x109D2, 0x10A03}, {0x10A05, 0x10A06},
- {0x10A0C, 0x10A13}, {0x10A15, 0x10A17}, {0x10A19, 0x10A35},
- {0x10A38, 0x10A3A}, {0x10A3F, 0x10A48}, {0x10A50, 0x10A58},
- {0x10A60, 0x10A9F}, {0x10AC0, 0x10AE6}, {0x10AEB, 0x10AF6},
- {0x10B00, 0x10B35}, {0x10B39, 0x10B55}, {0x10B58, 0x10B72},
- {0x10B78, 0x10B91}, {0x10B99, 0x10B9C}, {0x10BA9, 0x10BAF},
- {0x10C00, 0x10C48}, {0x10C80, 0x10CB2}, {0x10CC0, 0x10CF2},
- {0x10CFA, 0x10D27}, {0x10D30, 0x10D39}, {0x10E60, 0x10E7E},
- {0x10E80, 0x10EA9}, {0x10EAB, 0x10EAD}, {0x10EB0, 0x10EB1},
- {0x10F00, 0x10F27}, {0x10F30, 0x10F59}, {0x10FB0, 0x10FCB},
- {0x10FE0, 0x10FF6}, {0x11000, 0x1104D}, {0x11052, 0x1106F},
- {0x1107F, 0x110C1}, {0x110CD, 0x110CD}, {0x110D0, 0x110E8},
- {0x110F0, 0x110F9}, {0x11100, 0x11134}, {0x11136, 0x11147},
- {0x11150, 0x11176}, {0x11180, 0x111DF}, {0x111E1, 0x111F4},
- {0x11200, 0x11211}, {0x11213, 0x1123E}, {0x11280, 0x11286},
- {0x11288, 0x11288}, {0x1128A, 0x1128D}, {0x1128F, 0x1129D},
- {0x1129F, 0x112A9}, {0x112B0, 0x112EA}, {0x112F0, 0x112F9},
- {0x11300, 0x11303}, {0x11305, 0x1130C}, {0x1130F, 0x11310},
- {0x11313, 0x11328}, {0x1132A, 0x11330}, {0x11332, 0x11333},
- {0x11335, 0x11339}, {0x1133B, 0x11344}, {0x11347, 0x11348},
- {0x1134B, 0x1134D}, {0x11350, 0x11350}, {0x11357, 0x11357},
- {0x1135D, 0x11363}, {0x11366, 0x1136C}, {0x11370, 0x11374},
- {0x11400, 0x1145B}, {0x1145D, 0x11461}, {0x11480, 0x114C7},
- {0x114D0, 0x114D9}, {0x11580, 0x115B5}, {0x115B8, 0x115DD},
- {0x11600, 0x11644}, {0x11650, 0x11659}, {0x11660, 0x1166C},
- {0x11680, 0x116B8}, {0x116C0, 0x116C9}, {0x11700, 0x1171A},
- {0x1171D, 0x1172B}, {0x11730, 0x1173F}, {0x11800, 0x1183B},
- {0x118A0, 0x118F2}, {0x118FF, 0x11906}, {0x11909, 0x11909},
- {0x1190C, 0x11913}, {0x11915, 0x11916}, {0x11918, 0x11935},
- {0x11937, 0x11938}, {0x1193B, 0x11946}, {0x11950, 0x11959},
- {0x119A0, 0x119A7}, {0x119AA, 0x119D7}, {0x119DA, 0x119E4},
- {0x11A00, 0x11A47}, {0x11A50, 0x11AA2}, {0x11AC0, 0x11AF8},
- {0x11C00, 0x11C08}, {0x11C0A, 0x11C36}, {0x11C38, 0x11C45},
- {0x11C50, 0x11C6C}, {0x11C70, 0x11C8F}, {0x11C92, 0x11CA7},
- {0x11CA9, 0x11CB6}, {0x11D00, 0x11D06}, {0x11D08, 0x11D09},
- {0x11D0B, 0x11D36}, {0x11D3A, 0x11D3A}, {0x11D3C, 0x11D3D},
- {0x11D3F, 0x11D47}, {0x11D50, 0x11D59}, {0x11D60, 0x11D65},
- {0x11D67, 0x11D68}, {0x11D6A, 0x11D8E}, {0x11D90, 0x11D91},
- {0x11D93, 0x11D98}, {0x11DA0, 0x11DA9}, {0x11EE0, 0x11EF8},
- {0x11FB0, 0x11FB0}, {0x11FC0, 0x11FF1}, {0x11FFF, 0x12399},
- {0x12400, 0x1246E}, {0x12470, 0x12474}, {0x12480, 0x12543},
- {0x13000, 0x1342E}, {0x13430, 0x13438}, {0x14400, 0x14646},
- {0x16800, 0x16A38}, {0x16A40, 0x16A5E}, {0x16A60, 0x16A69},
- {0x16A6E, 0x16A6F}, {0x16AD0, 0x16AED}, {0x16AF0, 0x16AF5},
- {0x16B00, 0x16B45}, {0x16B50, 0x16B59}, {0x16B5B, 0x16B61},
- {0x16B63, 0x16B77}, {0x16B7D, 0x16B8F}, {0x16E40, 0x16E9A},
- {0x16F00, 0x16F4A}, {0x16F4F, 0x16F87}, {0x16F8F, 0x16F9F},
- {0x1BC00, 0x1BC6A}, {0x1BC70, 0x1BC7C}, {0x1BC80, 0x1BC88},
- {0x1BC90, 0x1BC99}, {0x1BC9C, 0x1BCA3}, {0x1D000, 0x1D0F5},
- {0x1D100, 0x1D126}, {0x1D129, 0x1D1E8}, {0x1D200, 0x1D245},
- {0x1D2E0, 0x1D2F3}, {0x1D300, 0x1D356}, {0x1D360, 0x1D378},
- {0x1D400, 0x1D454}, {0x1D456, 0x1D49C}, {0x1D49E, 0x1D49F},
- {0x1D4A2, 0x1D4A2}, {0x1D4A5, 0x1D4A6}, {0x1D4A9, 0x1D4AC},
- {0x1D4AE, 0x1D4B9}, {0x1D4BB, 0x1D4BB}, {0x1D4BD, 0x1D4C3},
- {0x1D4C5, 0x1D505}, {0x1D507, 0x1D50A}, {0x1D50D, 0x1D514},
- {0x1D516, 0x1D51C}, {0x1D51E, 0x1D539}, {0x1D53B, 0x1D53E},
- {0x1D540, 0x1D544}, {0x1D546, 0x1D546}, {0x1D54A, 0x1D550},
- {0x1D552, 0x1D6A5}, {0x1D6A8, 0x1D7CB}, {0x1D7CE, 0x1DA8B},
- {0x1DA9B, 0x1DA9F}, {0x1DAA1, 0x1DAAF}, {0x1E000, 0x1E006},
- {0x1E008, 0x1E018}, {0x1E01B, 0x1E021}, {0x1E023, 0x1E024},
- {0x1E026, 0x1E02A}, {0x1E100, 0x1E12C}, {0x1E130, 0x1E13D},
- {0x1E140, 0x1E149}, {0x1E14E, 0x1E14F}, {0x1E2C0, 0x1E2F9},
- {0x1E2FF, 0x1E2FF}, {0x1E800, 0x1E8C4}, {0x1E8C7, 0x1E8D6},
- {0x1E900, 0x1E94B}, {0x1E950, 0x1E959}, {0x1E95E, 0x1E95F},
- {0x1EC71, 0x1ECB4}, {0x1ED01, 0x1ED3D}, {0x1EE00, 0x1EE03},
- {0x1EE05, 0x1EE1F}, {0x1EE21, 0x1EE22}, {0x1EE24, 0x1EE24},
- {0x1EE27, 0x1EE27}, {0x1EE29, 0x1EE32}, {0x1EE34, 0x1EE37},
- {0x1EE39, 0x1EE39}, {0x1EE3B, 0x1EE3B}, {0x1EE42, 0x1EE42},
- {0x1EE47, 0x1EE47}, {0x1EE49, 0x1EE49}, {0x1EE4B, 0x1EE4B},
- {0x1EE4D, 0x1EE4F}, {0x1EE51, 0x1EE52}, {0x1EE54, 0x1EE54},
- {0x1EE57, 0x1EE57}, {0x1EE59, 0x1EE59}, {0x1EE5B, 0x1EE5B},
- {0x1EE5D, 0x1EE5D}, {0x1EE5F, 0x1EE5F}, {0x1EE61, 0x1EE62},
- {0x1EE64, 0x1EE64}, {0x1EE67, 0x1EE6A}, {0x1EE6C, 0x1EE72},
- {0x1EE74, 0x1EE77}, {0x1EE79, 0x1EE7C}, {0x1EE7E, 0x1EE7E},
- {0x1EE80, 0x1EE89}, {0x1EE8B, 0x1EE9B}, {0x1EEA1, 0x1EEA3},
- {0x1EEA5, 0x1EEA9}, {0x1EEAB, 0x1EEBB}, {0x1EEF0, 0x1EEF1},
- {0x1F000, 0x1F003}, {0x1F005, 0x1F02B}, {0x1F030, 0x1F093},
- {0x1F0A0, 0x1F0AE}, {0x1F0B1, 0x1F0BF}, {0x1F0C1, 0x1F0CE},
- {0x1F0D1, 0x1F0F5}, {0x1F10B, 0x1F10F}, {0x1F12E, 0x1F12F},
- {0x1F16A, 0x1F16F}, {0x1F1AD, 0x1F1AD}, {0x1F1E6, 0x1F1FF},
- {0x1F321, 0x1F32C}, {0x1F336, 0x1F336}, {0x1F37D, 0x1F37D},
- {0x1F394, 0x1F39F}, {0x1F3CB, 0x1F3CE}, {0x1F3D4, 0x1F3DF},
- {0x1F3F1, 0x1F3F3}, {0x1F3F5, 0x1F3F7}, {0x1F43F, 0x1F43F},
- {0x1F441, 0x1F441}, {0x1F4FD, 0x1F4FE}, {0x1F53E, 0x1F54A},
- {0x1F54F, 0x1F54F}, {0x1F568, 0x1F579}, {0x1F57B, 0x1F594},
- {0x1F597, 0x1F5A3}, {0x1F5A5, 0x1F5FA}, {0x1F650, 0x1F67F},
- {0x1F6C6, 0x1F6CB}, {0x1F6CD, 0x1F6CF}, {0x1F6D3, 0x1F6D4},
- {0x1F6E0, 0x1F6EA}, {0x1F6F0, 0x1F6F3}, {0x1F700, 0x1F773},
- {0x1F780, 0x1F7D8}, {0x1F800, 0x1F80B}, {0x1F810, 0x1F847},
- {0x1F850, 0x1F859}, {0x1F860, 0x1F887}, {0x1F890, 0x1F8AD},
- {0x1F8B0, 0x1F8B1}, {0x1F900, 0x1F90B}, {0x1F93B, 0x1F93B},
- {0x1F946, 0x1F946}, {0x1FA00, 0x1FA53}, {0x1FA60, 0x1FA6D},
- {0x1FB00, 0x1FB92}, {0x1FB94, 0x1FBCA}, {0x1FBF0, 0x1FBF9},
- {0xE0001, 0xE0001}, {0xE0020, 0xE007F},
-}
-
-var emoji = table{
- {0x203C, 0x203C}, {0x2049, 0x2049}, {0x2122, 0x2122},
- {0x2139, 0x2139}, {0x2194, 0x2199}, {0x21A9, 0x21AA},
- {0x231A, 0x231B}, {0x2328, 0x2328}, {0x2388, 0x2388},
- {0x23CF, 0x23CF}, {0x23E9, 0x23F3}, {0x23F8, 0x23FA},
- {0x24C2, 0x24C2}, {0x25AA, 0x25AB}, {0x25B6, 0x25B6},
- {0x25C0, 0x25C0}, {0x25FB, 0x25FE}, {0x2600, 0x2605},
- {0x2607, 0x2612}, {0x2614, 0x2685}, {0x2690, 0x2705},
- {0x2708, 0x2712}, {0x2714, 0x2714}, {0x2716, 0x2716},
- {0x271D, 0x271D}, {0x2721, 0x2721}, {0x2728, 0x2728},
- {0x2733, 0x2734}, {0x2744, 0x2744}, {0x2747, 0x2747},
- {0x274C, 0x274C}, {0x274E, 0x274E}, {0x2753, 0x2755},
- {0x2757, 0x2757}, {0x2763, 0x2767}, {0x2795, 0x2797},
- {0x27A1, 0x27A1}, {0x27B0, 0x27B0}, {0x27BF, 0x27BF},
- {0x2934, 0x2935}, {0x2B05, 0x2B07}, {0x2B1B, 0x2B1C},
- {0x2B50, 0x2B50}, {0x2B55, 0x2B55}, {0x3030, 0x3030},
- {0x303D, 0x303D}, {0x3297, 0x3297}, {0x3299, 0x3299},
- {0x1F000, 0x1F0FF}, {0x1F10D, 0x1F10F}, {0x1F12F, 0x1F12F},
- {0x1F16C, 0x1F171}, {0x1F17E, 0x1F17F}, {0x1F18E, 0x1F18E},
- {0x1F191, 0x1F19A}, {0x1F1AD, 0x1F1E5}, {0x1F201, 0x1F20F},
- {0x1F21A, 0x1F21A}, {0x1F22F, 0x1F22F}, {0x1F232, 0x1F23A},
- {0x1F23C, 0x1F23F}, {0x1F249, 0x1F3FA}, {0x1F400, 0x1F53D},
- {0x1F546, 0x1F64F}, {0x1F680, 0x1F6FF}, {0x1F774, 0x1F77F},
- {0x1F7D5, 0x1F7FF}, {0x1F80C, 0x1F80F}, {0x1F848, 0x1F84F},
- {0x1F85A, 0x1F85F}, {0x1F888, 0x1F88F}, {0x1F8AE, 0x1F8FF},
- {0x1F90C, 0x1F93A}, {0x1F93C, 0x1F945}, {0x1F947, 0x1FAFF},
- {0x1FC00, 0x1FFFD},
-}
diff --git a/vendor/github.com/mattn/go-runewidth/runewidth_windows.go b/vendor/github.com/mattn/go-runewidth/runewidth_windows.go
deleted file mode 100644
index 5f987a31..00000000
--- a/vendor/github.com/mattn/go-runewidth/runewidth_windows.go
+++ /dev/null
@@ -1,28 +0,0 @@
-//go:build windows && !appengine
-// +build windows,!appengine
-
-package runewidth
-
-import (
- "syscall"
-)
-
-var (
- kernel32 = syscall.NewLazyDLL("kernel32")
- procGetConsoleOutputCP = kernel32.NewProc("GetConsoleOutputCP")
-)
-
-// IsEastAsian return true if the current locale is CJK
-func IsEastAsian() bool {
- r1, _, _ := procGetConsoleOutputCP.Call()
- if r1 == 0 {
- return false
- }
-
- switch int(r1) {
- case 932, 51932, 936, 949, 950:
- return true
- }
-
- return false
-}
diff --git a/vendor/github.com/mcnijman/go-emailaddress/.gitignore b/vendor/github.com/mcnijman/go-emailaddress/.gitignore
deleted file mode 100644
index c0613ec1..00000000
--- a/vendor/github.com/mcnijman/go-emailaddress/.gitignore
+++ /dev/null
@@ -1,2 +0,0 @@
-emailaddress
-coverage.*
\ No newline at end of file
diff --git a/vendor/github.com/mcnijman/go-emailaddress/.travis.yml b/vendor/github.com/mcnijman/go-emailaddress/.travis.yml
deleted file mode 100644
index e0799bad..00000000
--- a/vendor/github.com/mcnijman/go-emailaddress/.travis.yml
+++ /dev/null
@@ -1,25 +0,0 @@
-sudo: false
-language: go
-go:
- - "1.11.x"
- - "1.10.x"
- - "1.9.x"
- - tip
-env:
- global:
- - GO111MODULE=on
-matrix:
- allow_failures:
- - go: tip
- fast_finish: true
-install:
- - go get golang.org/x/tools/cmd/cover
- - go get github.com/mattn/goveralls
- - GO111MODULE=off go get -u github.com/securego/gosec/cmd/gosec/...
-script:
- - go get -t -v ./...
- - diff -u <(echo -n) <(gofmt -d -s .)
- - go tool vet .
- - $GOPATH/bin/gosec ./...
- - go test -race -covermode=atomic -coverprofile=coverage.txt ./...
- - $GOPATH/bin/goveralls -coverprofile=coverage.txt -service=travis-ci
diff --git a/vendor/github.com/mcnijman/go-emailaddress/LICENCE b/vendor/github.com/mcnijman/go-emailaddress/LICENCE
deleted file mode 100644
index 4dfcb588..00000000
--- a/vendor/github.com/mcnijman/go-emailaddress/LICENCE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2018 Thijs Nijman
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/mcnijman/go-emailaddress/README.md b/vendor/github.com/mcnijman/go-emailaddress/README.md
deleted file mode 100644
index 525cc431..00000000
--- a/vendor/github.com/mcnijman/go-emailaddress/README.md
+++ /dev/null
@@ -1,102 +0,0 @@
-# go-emailaddress #
-
-[](https://godoc.org/github.com/mcnijman/go-emailaddress) [](https://travis-ci.org/mcnijman/go-emailaddress) [](https://coveralls.io/github/mcnijman/go-emailaddress?branch=master) [](https://goreportcard.com/report/github.com/mcnijman/go-emailaddress)
-
-go-emailaddress is a tiny Go library for finding, parsing and validating email addresses. This
-library is tested for Go v1.9 and above.
-
-Note that there is no such thing as perfect email address validation other than sending an actual
-email (ie. with a confirmation token). This library however checks if the email format conforms to
-the spec and if the host (domain) is actually able to receive emails. You can also use this library
-to find emails in a byte array. This package was created as similar packages don't seem to be
-maintained anymore (ie contain bugs with pull requests still open), and/or use wrong local
-validation.
-
-## Usage ##
-
-```bash
-go get -u github.com/mcnijman/go-emailaddress
-```
-
-### Parsing and local validation ###
-
-Parse and validate the email locally using RFC 5322 regex, note that when `err == nil` it doesn't
-necessarily mean the email address actually exists.
-
-```go
-import "github.com/mcnijman/go-emailaddress"
-
-email, err := emailaddress.Parse("foo@bar.com")
-if err != nil {
- fmt.Println("invalid email")
-}
-
-fmt.Println(email.LocalPart) // foo
-fmt.Println(email.Domain) // bar.com
-fmt.Println(email) // foo@bar.com
-fmt.Println(email.String()) // foo@bar.com
-```
-
-### Validating the host ###
-
-Host validation will first attempt to resolve the domain and then verify if we can start a mail
-transaction with the host. This is relatively slow as it will contact the host several times.
-Note that when `err == nil` it doesn't necessarily mean the email address actually exists.
-
-```go
-import "github.com/mcnijman/go-emailaddress"
-
-email, err := emailaddress.Parse("foo@bar.com")
-if err != nil {
- fmt.Println("invalid email")
-}
-
-err := email.ValidateHost()
-if err != nil {
- fmt.Println("invalid host")
-}
-```
-
-### Finding emails ###
-
-This will look for emails in a byte array (ie text or an html response).
-
-```go
-import "github.com/mcnijman/go-emailaddress"
-
-text := []byte(`Send me an email at foo@bar.com.`)
-validateHost := false
-
-emails := emailaddress.Find(text, validateHost)
-
-for _, e := range emails {
- fmt.Println(e)
-}
-// foo@bar.com
-```
-
-As RFC 5322 is really broad this method will likely match images and urls that contain
-the '@' character (ie. !--logo@2x.png). For more reliable results, you can use the following method.
-
-```go
-import "github.com/mcnijman/go-emailaddress"
-
-text := []byte(`Send me an email at foo@bar.com or fake@domain.foobar.`)
-validateHost := false
-
-emails := emailaddress.FindWithIcannSuffix(text, validateHost)
-
-for _, e := range emails {
- fmt.Println(e)
-}
-// foo@bar.com
-```
-
-## Versioning ##
-
-This library uses [semantic versioning 2.0.0](https://semver.org/spec/v2.0.0.html).
-
-## License ##
-
-This library is distributed under the MIT license found in the [LICENSE](./LICENSE)
-file.
\ No newline at end of file
diff --git a/vendor/github.com/mcnijman/go-emailaddress/emailaddress.go b/vendor/github.com/mcnijman/go-emailaddress/emailaddress.go
deleted file mode 100644
index a78ae6a3..00000000
--- a/vendor/github.com/mcnijman/go-emailaddress/emailaddress.go
+++ /dev/null
@@ -1,224 +0,0 @@
-// Copyright 2018 The go-emailaddress AUTHORS. All rights reserved.
-//
-// Use of this source code is governed by a MIT
-// license that can be found in the LICENSE file.
-
-/*
-Package emailaddress provides a tiny library for finding, parsing and validation of email
-addresses. This library is tested for Go v1.9 and above.
-
- go get -u github.com/mcnijman/go-emailaddress
-
-Local validation
-
-Parse and validate the email locally using RFC 5322 regex, note that when err == nil it doesn't
-necessarily mean the email address actually exists.
-
- import "github.com/mcnijman/go-emailaddress"
-
- email, err := emailaddress.Parse("foo@bar.com")
- if err != nil {
- fmt.Println("invalid email")
- }
-
- fmt.Println(email.LocalPart) // foo
- fmt.Println(email.Domain) // bar.com
- fmt.Println(email) // foo@bar.com
- fmt.Println(email.String()) // foo@bar.com
-
-Host validation
-
-Host validation will first attempt to resolve the domain and then verify if we can start a mail
-transaction with the host. This is relatively slow as it will contact the host several times.
-Note that when err == nil it doesn't necessarily mean the email address actually exists.
-
- import "github.com/mcnijman/go-emailaddress"
-
- email, err := emailaddress.Parse("foo@bar.com")
- if err != nil {
- fmt.Println("invalid email")
- }
-
- err := email.ValidateHost()
- if err != nil {
- fmt.Println("invalid host")
- }
-
-Finding emails
-
-This will look for emails in a byte array (ie text or an html response).
-
- import "github.com/mcnijman/go-emailaddress"
-
- text := []byte(`Send me an email at foo@bar.com.`)
- validateHost := false
-
- emails := emailaddress.Find(text, validateHost)
-
- for _, e := range emails {
- fmt.Println(e)
- }
- // foo@bar.com
-
-As RFC 5322 is really broad this method will likely match images and urls that contain
-the '@' character (ie. !--logo@2x.png). For more reliable results, you can use the following method.
-
- import "github.com/mcnijman/go-emailaddress"
-
- text := []byte(`Send me an email at foo@bar.com or fake@domain.foobar.`)
- validateHost := false
-
- emails := emailaddress.FindWithIcannSuffix(text, validateHost)
-
- for _, e := range emails {
- fmt.Println(e)
- }
- // foo@bar.com
-
-*/
-package emailaddress
-
-import (
- "fmt"
- "net"
- "net/smtp"
- "regexp"
- "strings"
-
- "golang.org/x/net/publicsuffix"
-)
-
-var (
- // rfc5322 is a RFC 5322 regex, as per: https://stackoverflow.com/a/201378/5405453.
- // Note that this can't verify that the address is an actual working email address.
- // Use ValidateHost as a starter and/or send them one :-).
- rfc5322 = "(?i)(?:[a-z0-9!#$%&'*+/=?^_`{|}~-]+(?:\\.[a-z0-9!#$%&'*+/=?^_`{|}~-]+)*|\"(?:[\\x01-\\x08\\x0b\\x0c\\x0e-\\x1f\\x21\\x23-\\x5b\\x5d-\\x7f]|\\\\[\\x01-\\x09\\x0b\\x0c\\x0e-\\x7f])*\")@(?:(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?\\.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])?|\\[(?:(?:(2(5[0-5]|[0-4][0-9])|1[0-9][0-9]|[1-9]?[0-9]))\\.){3}(?:(2(5[0-5]|[0-4][0-9])|1[0-9][0-9]|[1-9]?[0-9])|[a-z0-9-]*[a-z0-9]:(?:[\\x01-\\x08\\x0b\\x0c\\x0e-\\x1f\\x21-\\x5a\\x53-\\x7f]|\\\\[\\x01-\\x09\\x0b\\x0c\\x0e-\\x7f])+)\\])"
- validEmailRegexp = regexp.MustCompile(fmt.Sprintf("^%s*$", rfc5322))
- findEmailRegexp = regexp.MustCompile(rfc5322)
-)
-
-// EmailAddress is a structure that stores the address local-part@domain parts.
-type EmailAddress struct {
- // LocalPart usually the username of an email address.
- LocalPart string
-
- // Domain is the part of the email address after the last @.
- // This should be DNS resolvable to an email server.
- Domain string
-}
-
-func (e EmailAddress) String() string {
- if e.LocalPart == "" || e.Domain == "" {
- return ""
- }
- return fmt.Sprintf("%s@%s", e.LocalPart, e.Domain)
-}
-
-// ValidateHost will test if the email address is actually reachable. It will first try to resolve
-// the host and then start a mail transaction.
-func (e EmailAddress) ValidateHost() error {
- host, err := lookupHost(e.Domain)
- if err != nil {
- return err
- }
- return tryHost(host, e)
-}
-
-// ValidateIcanSuffix will test if the public suffix of the domain is managed by ICANN using
-// the golang.org/x/net/publicsuffix package. If not it will return an error. Note that if this
-// method returns an error it does not necessarily mean that the email address is invalid. Also the
-// suffix list in the standard package is embedded and thereby not up to date.
-func (e EmailAddress) ValidateIcanSuffix() error {
- d := strings.ToLower(e.Domain)
- if s, icann := publicsuffix.PublicSuffix(d); !icann {
- return fmt.Errorf("public suffix is not managed by ICANN, got %s", s)
- }
- return nil
-}
-
-// Find uses the RFC 5322 regex to match, parse and validate any email addresses found in a string.
-// If the validateHost boolean is true it will call the validate host for every email address
-// encounterd. As RFC 5322 is really broad this method will likely match images and urls that
-// contain the '@' character.
-func Find(haystack []byte, validateHost bool) (emails []*EmailAddress) {
- results := findEmailRegexp.FindAll(haystack, -1)
- for _, r := range results {
- if e, err := Parse(string(r)); err == nil {
- if validateHost {
- if err := e.ValidateHost(); err != nil {
- continue
- }
- }
- emails = append(emails, e)
- }
- }
- return emails
-}
-
-// FindWithIcannSuffix uses the RFC 5322 regex to match, parse and validate any email addresses
-// found in a string. It will return emails if its eTLD is managed by the ICANN organization.
-// If the validateHost boolean is true it will call the validate host for every email address
-// encounterd. As RFC 5322 is really broad this method will likely match images and urls that
-// contain the '@' character.
-func FindWithIcannSuffix(haystack []byte, validateHost bool) (emails []*EmailAddress) {
- results := Find(haystack, false)
- for _, e := range results {
- if err := e.ValidateIcanSuffix(); err == nil {
- if validateHost {
- if err := e.ValidateHost(); err != nil {
- continue
- }
- }
- emails = append(emails, e)
- }
- }
- return emails
-}
-
-// Parse will parse the input and validate the email locally. If you want to validate the host of
-// this email address remotely call the ValidateHost method.
-func Parse(email string) (*EmailAddress, error) {
- if !validEmailRegexp.MatchString(email) {
- return nil, fmt.Errorf("format is incorrect for %s", email)
- }
-
- i := strings.LastIndexByte(email, '@')
- e := &EmailAddress{
- LocalPart: email[:i],
- Domain: email[i+1:],
- }
- return e, nil
-}
-
-// lookupHost first checks if any MX records are available and if not, it will check
-// if A records are available as they can resolve email server hosts. An error indicates
-// that non of the A or MX records are available.
-func lookupHost(domain string) (string, error) {
- if mx, err := net.LookupMX(domain); err == nil {
- return mx[0].Host, nil
- }
- if ips, err := net.LookupIP(domain); err == nil {
- return ips[0].String(), nil // randomly returns IPv4 or IPv6 (when available)
- }
- return "", fmt.Errorf("failed finding MX and A records for domain %s", domain)
-}
-
-// tryHost will verify if we can start a mail transaction with the host.
-func tryHost(host string, e EmailAddress) error {
- client, err := smtp.Dial(fmt.Sprintf("%s:%d", host, 25))
- if err != nil {
- return err
- }
- defer client.Close()
-
- if err = client.Hello(e.Domain); err == nil {
- if err = client.Mail(fmt.Sprintf("hello@%s", e.Domain)); err == nil {
- if err = client.Rcpt(e.String()); err == nil {
- client.Reset() // #nosec
- client.Quit() // #nosec
- return nil
- }
- }
- }
- return err
-}
diff --git a/vendor/github.com/mitchellh/colorstring/.travis.yml b/vendor/github.com/mitchellh/colorstring/.travis.yml
deleted file mode 100644
index 74e286ae..00000000
--- a/vendor/github.com/mitchellh/colorstring/.travis.yml
+++ /dev/null
@@ -1,15 +0,0 @@
-language: go
-
-go:
- - 1.0
- - 1.1
- - 1.2
- - 1.3
- - tip
-
-script:
- - go test
-
-matrix:
- allow_failures:
- - go: tip
diff --git a/vendor/github.com/mitchellh/colorstring/LICENSE b/vendor/github.com/mitchellh/colorstring/LICENSE
deleted file mode 100644
index 22985159..00000000
--- a/vendor/github.com/mitchellh/colorstring/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2014 Mitchell Hashimoto
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
diff --git a/vendor/github.com/mitchellh/colorstring/README.md b/vendor/github.com/mitchellh/colorstring/README.md
deleted file mode 100644
index 0654d454..00000000
--- a/vendor/github.com/mitchellh/colorstring/README.md
+++ /dev/null
@@ -1,30 +0,0 @@
-# colorstring [](https://travis-ci.org/mitchellh/colorstring)
-
-colorstring is a [Go](http://www.golang.org) library for outputting colored
-strings to a console using a simple inline syntax in your string to specify
-the color to print as.
-
-For example, the string `[blue]hello [red]world` would output the text
-"hello world" in two colors. The API of colorstring allows for easily disabling
-colors, adding aliases, etc.
-
-## Installation
-
-Standard `go get`:
-
-```
-$ go get github.com/mitchellh/colorstring
-```
-
-## Usage & Example
-
-For usage and examples see the [Godoc](http://godoc.org/github.com/mitchellh/colorstring).
-
-Usage is easy enough:
-
-```go
-colorstring.Println("[blue]Hello [red]World!")
-```
-
-Additionally, the `Colorize` struct can be used to set options such as
-custom colors, color disabling, etc.
diff --git a/vendor/github.com/mitchellh/colorstring/colorstring.go b/vendor/github.com/mitchellh/colorstring/colorstring.go
deleted file mode 100644
index 3de5b241..00000000
--- a/vendor/github.com/mitchellh/colorstring/colorstring.go
+++ /dev/null
@@ -1,244 +0,0 @@
-// colorstring provides functions for colorizing strings for terminal
-// output.
-package colorstring
-
-import (
- "bytes"
- "fmt"
- "io"
- "regexp"
- "strings"
-)
-
-// Color colorizes your strings using the default settings.
-//
-// Strings given to Color should use the syntax `[color]` to specify the
-// color for text following. For example: `[blue]Hello` will return "Hello"
-// in blue. See DefaultColors for all the supported colors and attributes.
-//
-// If an unrecognized color is given, it is ignored and assumed to be part
-// of the string. For example: `[hi]world` will result in "[hi]world".
-//
-// A color reset is appended to the end of every string. This will reset
-// the color of following strings when you output this text to the same
-// terminal session.
-//
-// If you want to customize any of this behavior, use the Colorize struct.
-func Color(v string) string {
- return def.Color(v)
-}
-
-// ColorPrefix returns the color sequence that prefixes the given text.
-//
-// This is useful when wrapping text if you want to inherit the color
-// of the wrapped text. For example, "[green]foo" will return "[green]".
-// If there is no color sequence, then this will return "".
-func ColorPrefix(v string) string {
- return def.ColorPrefix(v)
-}
-
-// Colorize colorizes your strings, giving you the ability to customize
-// some of the colorization process.
-//
-// The options in Colorize can be set to customize colorization. If you're
-// only interested in the defaults, just use the top Color function directly,
-// which creates a default Colorize.
-type Colorize struct {
- // Colors maps a color string to the code for that color. The code
- // is a string so that you can use more complex colors to set foreground,
- // background, attributes, etc. For example, "boldblue" might be
- // "1;34"
- Colors map[string]string
-
- // If true, color attributes will be ignored. This is useful if you're
- // outputting to a location that doesn't support colors and you just
- // want the strings returned.
- Disable bool
-
- // Reset, if true, will reset the color after each colorization by
- // adding a reset code at the end.
- Reset bool
-}
-
-// Color colorizes a string according to the settings setup in the struct.
-//
-// For more details on the syntax, see the top-level Color function.
-func (c *Colorize) Color(v string) string {
- matches := parseRe.FindAllStringIndex(v, -1)
- if len(matches) == 0 {
- return v
- }
-
- result := new(bytes.Buffer)
- colored := false
- m := []int{0, 0}
- for _, nm := range matches {
- // Write the text in between this match and the last
- result.WriteString(v[m[1]:nm[0]])
- m = nm
-
- var replace string
- if code, ok := c.Colors[v[m[0]+1:m[1]-1]]; ok {
- colored = true
-
- if !c.Disable {
- replace = fmt.Sprintf("\033[%sm", code)
- }
- } else {
- replace = v[m[0]:m[1]]
- }
-
- result.WriteString(replace)
- }
- result.WriteString(v[m[1]:])
-
- if colored && c.Reset && !c.Disable {
- // Write the clear byte at the end
- result.WriteString("\033[0m")
- }
-
- return result.String()
-}
-
-// ColorPrefix returns the first color sequence that exists in this string.
-//
-// For example: "[green]foo" would return "[green]". If no color sequence
-// exists, then "" is returned. This is especially useful when wrapping
-// colored texts to inherit the color of the wrapped text.
-func (c *Colorize) ColorPrefix(v string) string {
- return prefixRe.FindString(strings.TrimSpace(v))
-}
-
-// DefaultColors are the default colors used when colorizing.
-//
-// If the color is surrounded in underscores, such as "_blue_", then that
-// color will be used for the background color.
-var DefaultColors map[string]string
-
-func init() {
- DefaultColors = map[string]string{
- // Default foreground/background colors
- "default": "39",
- "_default_": "49",
-
- // Foreground colors
- "black": "30",
- "red": "31",
- "green": "32",
- "yellow": "33",
- "blue": "34",
- "magenta": "35",
- "cyan": "36",
- "light_gray": "37",
- "dark_gray": "90",
- "light_red": "91",
- "light_green": "92",
- "light_yellow": "93",
- "light_blue": "94",
- "light_magenta": "95",
- "light_cyan": "96",
- "white": "97",
-
- // Background colors
- "_black_": "40",
- "_red_": "41",
- "_green_": "42",
- "_yellow_": "43",
- "_blue_": "44",
- "_magenta_": "45",
- "_cyan_": "46",
- "_light_gray_": "47",
- "_dark_gray_": "100",
- "_light_red_": "101",
- "_light_green_": "102",
- "_light_yellow_": "103",
- "_light_blue_": "104",
- "_light_magenta_": "105",
- "_light_cyan_": "106",
- "_white_": "107",
-
- // Attributes
- "bold": "1",
- "dim": "2",
- "underline": "4",
- "blink_slow": "5",
- "blink_fast": "6",
- "invert": "7",
- "hidden": "8",
-
- // Reset to reset everything to their defaults
- "reset": "0",
- "reset_bold": "21",
- }
-
- def = Colorize{
- Colors: DefaultColors,
- Reset: true,
- }
-}
-
-var def Colorize
-var parseReRaw = `\[[a-z0-9_-]+\]`
-var parseRe = regexp.MustCompile(`(?i)` + parseReRaw)
-var prefixRe = regexp.MustCompile(`^(?i)(` + parseReRaw + `)+`)
-
-// Print is a convenience wrapper for fmt.Print with support for color codes.
-//
-// Print formats using the default formats for its operands and writes to
-// standard output with support for color codes. Spaces are added between
-// operands when neither is a string. It returns the number of bytes written
-// and any write error encountered.
-func Print(a string) (n int, err error) {
- return fmt.Print(Color(a))
-}
-
-// Println is a convenience wrapper for fmt.Println with support for color
-// codes.
-//
-// Println formats using the default formats for its operands and writes to
-// standard output with support for color codes. Spaces are always added
-// between operands and a newline is appended. It returns the number of bytes
-// written and any write error encountered.
-func Println(a string) (n int, err error) {
- return fmt.Println(Color(a))
-}
-
-// Printf is a convenience wrapper for fmt.Printf with support for color codes.
-//
-// Printf formats according to a format specifier and writes to standard output
-// with support for color codes. It returns the number of bytes written and any
-// write error encountered.
-func Printf(format string, a ...interface{}) (n int, err error) {
- return fmt.Printf(Color(format), a...)
-}
-
-// Fprint is a convenience wrapper for fmt.Fprint with support for color codes.
-//
-// Fprint formats using the default formats for its operands and writes to w
-// with support for color codes. Spaces are added between operands when neither
-// is a string. It returns the number of bytes written and any write error
-// encountered.
-func Fprint(w io.Writer, a string) (n int, err error) {
- return fmt.Fprint(w, Color(a))
-}
-
-// Fprintln is a convenience wrapper for fmt.Fprintln with support for color
-// codes.
-//
-// Fprintln formats using the default formats for its operands and writes to w
-// with support for color codes. Spaces are always added between operands and a
-// newline is appended. It returns the number of bytes written and any write
-// error encountered.
-func Fprintln(w io.Writer, a string) (n int, err error) {
- return fmt.Fprintln(w, Color(a))
-}
-
-// Fprintf is a convenience wrapper for fmt.Fprintf with support for color
-// codes.
-//
-// Fprintf formats according to a format specifier and writes to w with support
-// for color codes. It returns the number of bytes written and any write error
-// encountered.
-func Fprintf(w io.Writer, format string, a ...interface{}) (n int, err error) {
- return fmt.Fprintf(w, Color(format), a...)
-}
diff --git a/vendor/github.com/mitchellh/mapstructure/CHANGELOG.md b/vendor/github.com/mitchellh/mapstructure/CHANGELOG.md
deleted file mode 100644
index c7582349..00000000
--- a/vendor/github.com/mitchellh/mapstructure/CHANGELOG.md
+++ /dev/null
@@ -1,96 +0,0 @@
-## 1.5.0
-
-* New option `IgnoreUntaggedFields` to ignore decoding to any fields
- without `mapstructure` (or the configured tag name) set [GH-277]
-* New option `ErrorUnset` which makes it an error if any fields
- in a target struct are not set by the decoding process. [GH-225]
-* New function `OrComposeDecodeHookFunc` to help compose decode hooks. [GH-240]
-* Decoding to slice from array no longer crashes [GH-265]
-* Decode nested struct pointers to map [GH-271]
-* Fix issue where `,squash` was ignored if `Squash` option was set. [GH-280]
-* Fix issue where fields with `,omitempty` would sometimes decode
- into a map with an empty string key [GH-281]
-
-## 1.4.3
-
-* Fix cases where `json.Number` didn't decode properly [GH-261]
-
-## 1.4.2
-
-* Custom name matchers to support any sort of casing, formatting, etc. for
- field names. [GH-250]
-* Fix possible panic in ComposeDecodeHookFunc [GH-251]
-
-## 1.4.1
-
-* Fix regression where `*time.Time` value would be set to empty and not be sent
- to decode hooks properly [GH-232]
-
-## 1.4.0
-
-* A new decode hook type `DecodeHookFuncValue` has been added that has
- access to the full values. [GH-183]
-* Squash is now supported with embedded fields that are struct pointers [GH-205]
-* Empty strings will convert to 0 for all numeric types when weakly decoding [GH-206]
-
-## 1.3.3
-
-* Decoding maps from maps creates a settable value for decode hooks [GH-203]
-
-## 1.3.2
-
-* Decode into interface type with a struct value is supported [GH-187]
-
-## 1.3.1
-
-* Squash should only squash embedded structs. [GH-194]
-
-## 1.3.0
-
-* Added `",omitempty"` support. This will ignore zero values in the source
- structure when encoding. [GH-145]
-
-## 1.2.3
-
-* Fix duplicate entries in Keys list with pointer values. [GH-185]
-
-## 1.2.2
-
-* Do not add unsettable (unexported) values to the unused metadata key
- or "remain" value. [GH-150]
-
-## 1.2.1
-
-* Go modules checksum mismatch fix
-
-## 1.2.0
-
-* Added support to capture unused values in a field using the `",remain"` value
- in the mapstructure tag. There is an example to showcase usage.
-* Added `DecoderConfig` option to always squash embedded structs
-* `json.Number` can decode into `uint` types
-* Empty slices are preserved and not replaced with nil slices
-* Fix panic that can occur in when decoding a map into a nil slice of structs
-* Improved package documentation for godoc
-
-## 1.1.2
-
-* Fix error when decode hook decodes interface implementation into interface
- type. [GH-140]
-
-## 1.1.1
-
-* Fix panic that can happen in `decodePtr`
-
-## 1.1.0
-
-* Added `StringToIPHookFunc` to convert `string` to `net.IP` and `net.IPNet` [GH-133]
-* Support struct to struct decoding [GH-137]
-* If source map value is nil, then destination map value is nil (instead of empty)
-* If source slice value is nil, then destination slice value is nil (instead of empty)
-* If source pointer is nil, then destination pointer is set to nil (instead of
- allocated zero value of type)
-
-## 1.0.0
-
-* Initial tagged stable release.
diff --git a/vendor/github.com/mitchellh/mapstructure/LICENSE b/vendor/github.com/mitchellh/mapstructure/LICENSE
deleted file mode 100644
index f9c841a5..00000000
--- a/vendor/github.com/mitchellh/mapstructure/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2013 Mitchell Hashimoto
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
diff --git a/vendor/github.com/mitchellh/mapstructure/README.md b/vendor/github.com/mitchellh/mapstructure/README.md
deleted file mode 100644
index 0018dc7d..00000000
--- a/vendor/github.com/mitchellh/mapstructure/README.md
+++ /dev/null
@@ -1,46 +0,0 @@
-# mapstructure [](https://godoc.org/github.com/mitchellh/mapstructure)
-
-mapstructure is a Go library for decoding generic map values to structures
-and vice versa, while providing helpful error handling.
-
-This library is most useful when decoding values from some data stream (JSON,
-Gob, etc.) where you don't _quite_ know the structure of the underlying data
-until you read a part of it. You can therefore read a `map[string]interface{}`
-and use this library to decode it into the proper underlying native Go
-structure.
-
-## Installation
-
-Standard `go get`:
-
-```
-$ go get github.com/mitchellh/mapstructure
-```
-
-## Usage & Example
-
-For usage and examples see the [Godoc](http://godoc.org/github.com/mitchellh/mapstructure).
-
-The `Decode` function has examples associated with it there.
-
-## But Why?!
-
-Go offers fantastic standard libraries for decoding formats such as JSON.
-The standard method is to have a struct pre-created, and populate that struct
-from the bytes of the encoded format. This is great, but the problem is if
-you have configuration or an encoding that changes slightly depending on
-specific fields. For example, consider this JSON:
-
-```json
-{
- "type": "person",
- "name": "Mitchell"
-}
-```
-
-Perhaps we can't populate a specific structure without first reading
-the "type" field from the JSON. We could always do two passes over the
-decoding of the JSON (reading the "type" first, and the rest later).
-However, it is much simpler to just decode this into a `map[string]interface{}`
-structure, read the "type" key, then use something like this library
-to decode it into the proper structure.
diff --git a/vendor/github.com/mitchellh/mapstructure/decode_hooks.go b/vendor/github.com/mitchellh/mapstructure/decode_hooks.go
deleted file mode 100644
index 3a754ca7..00000000
--- a/vendor/github.com/mitchellh/mapstructure/decode_hooks.go
+++ /dev/null
@@ -1,279 +0,0 @@
-package mapstructure
-
-import (
- "encoding"
- "errors"
- "fmt"
- "net"
- "reflect"
- "strconv"
- "strings"
- "time"
-)
-
-// typedDecodeHook takes a raw DecodeHookFunc (an interface{}) and turns
-// it into the proper DecodeHookFunc type, such as DecodeHookFuncType.
-func typedDecodeHook(h DecodeHookFunc) DecodeHookFunc {
- // Create variables here so we can reference them with the reflect pkg
- var f1 DecodeHookFuncType
- var f2 DecodeHookFuncKind
- var f3 DecodeHookFuncValue
-
- // Fill in the variables into this interface and the rest is done
- // automatically using the reflect package.
- potential := []interface{}{f1, f2, f3}
-
- v := reflect.ValueOf(h)
- vt := v.Type()
- for _, raw := range potential {
- pt := reflect.ValueOf(raw).Type()
- if vt.ConvertibleTo(pt) {
- return v.Convert(pt).Interface()
- }
- }
-
- return nil
-}
-
-// DecodeHookExec executes the given decode hook. This should be used
-// since it'll naturally degrade to the older backwards compatible DecodeHookFunc
-// that took reflect.Kind instead of reflect.Type.
-func DecodeHookExec(
- raw DecodeHookFunc,
- from reflect.Value, to reflect.Value) (interface{}, error) {
-
- switch f := typedDecodeHook(raw).(type) {
- case DecodeHookFuncType:
- return f(from.Type(), to.Type(), from.Interface())
- case DecodeHookFuncKind:
- return f(from.Kind(), to.Kind(), from.Interface())
- case DecodeHookFuncValue:
- return f(from, to)
- default:
- return nil, errors.New("invalid decode hook signature")
- }
-}
-
-// ComposeDecodeHookFunc creates a single DecodeHookFunc that
-// automatically composes multiple DecodeHookFuncs.
-//
-// The composed funcs are called in order, with the result of the
-// previous transformation.
-func ComposeDecodeHookFunc(fs ...DecodeHookFunc) DecodeHookFunc {
- return func(f reflect.Value, t reflect.Value) (interface{}, error) {
- var err error
- data := f.Interface()
-
- newFrom := f
- for _, f1 := range fs {
- data, err = DecodeHookExec(f1, newFrom, t)
- if err != nil {
- return nil, err
- }
- newFrom = reflect.ValueOf(data)
- }
-
- return data, nil
- }
-}
-
-// OrComposeDecodeHookFunc executes all input hook functions until one of them returns no error. In that case its value is returned.
-// If all hooks return an error, OrComposeDecodeHookFunc returns an error concatenating all error messages.
-func OrComposeDecodeHookFunc(ff ...DecodeHookFunc) DecodeHookFunc {
- return func(a, b reflect.Value) (interface{}, error) {
- var allErrs string
- var out interface{}
- var err error
-
- for _, f := range ff {
- out, err = DecodeHookExec(f, a, b)
- if err != nil {
- allErrs += err.Error() + "\n"
- continue
- }
-
- return out, nil
- }
-
- return nil, errors.New(allErrs)
- }
-}
-
-// StringToSliceHookFunc returns a DecodeHookFunc that converts
-// string to []string by splitting on the given sep.
-func StringToSliceHookFunc(sep string) DecodeHookFunc {
- return func(
- f reflect.Kind,
- t reflect.Kind,
- data interface{}) (interface{}, error) {
- if f != reflect.String || t != reflect.Slice {
- return data, nil
- }
-
- raw := data.(string)
- if raw == "" {
- return []string{}, nil
- }
-
- return strings.Split(raw, sep), nil
- }
-}
-
-// StringToTimeDurationHookFunc returns a DecodeHookFunc that converts
-// strings to time.Duration.
-func StringToTimeDurationHookFunc() DecodeHookFunc {
- return func(
- f reflect.Type,
- t reflect.Type,
- data interface{}) (interface{}, error) {
- if f.Kind() != reflect.String {
- return data, nil
- }
- if t != reflect.TypeOf(time.Duration(5)) {
- return data, nil
- }
-
- // Convert it by parsing
- return time.ParseDuration(data.(string))
- }
-}
-
-// StringToIPHookFunc returns a DecodeHookFunc that converts
-// strings to net.IP
-func StringToIPHookFunc() DecodeHookFunc {
- return func(
- f reflect.Type,
- t reflect.Type,
- data interface{}) (interface{}, error) {
- if f.Kind() != reflect.String {
- return data, nil
- }
- if t != reflect.TypeOf(net.IP{}) {
- return data, nil
- }
-
- // Convert it by parsing
- ip := net.ParseIP(data.(string))
- if ip == nil {
- return net.IP{}, fmt.Errorf("failed parsing ip %v", data)
- }
-
- return ip, nil
- }
-}
-
-// StringToIPNetHookFunc returns a DecodeHookFunc that converts
-// strings to net.IPNet
-func StringToIPNetHookFunc() DecodeHookFunc {
- return func(
- f reflect.Type,
- t reflect.Type,
- data interface{}) (interface{}, error) {
- if f.Kind() != reflect.String {
- return data, nil
- }
- if t != reflect.TypeOf(net.IPNet{}) {
- return data, nil
- }
-
- // Convert it by parsing
- _, net, err := net.ParseCIDR(data.(string))
- return net, err
- }
-}
-
-// StringToTimeHookFunc returns a DecodeHookFunc that converts
-// strings to time.Time.
-func StringToTimeHookFunc(layout string) DecodeHookFunc {
- return func(
- f reflect.Type,
- t reflect.Type,
- data interface{}) (interface{}, error) {
- if f.Kind() != reflect.String {
- return data, nil
- }
- if t != reflect.TypeOf(time.Time{}) {
- return data, nil
- }
-
- // Convert it by parsing
- return time.Parse(layout, data.(string))
- }
-}
-
-// WeaklyTypedHook is a DecodeHookFunc which adds support for weak typing to
-// the decoder.
-//
-// Note that this is significantly different from the WeaklyTypedInput option
-// of the DecoderConfig.
-func WeaklyTypedHook(
- f reflect.Kind,
- t reflect.Kind,
- data interface{}) (interface{}, error) {
- dataVal := reflect.ValueOf(data)
- switch t {
- case reflect.String:
- switch f {
- case reflect.Bool:
- if dataVal.Bool() {
- return "1", nil
- }
- return "0", nil
- case reflect.Float32:
- return strconv.FormatFloat(dataVal.Float(), 'f', -1, 64), nil
- case reflect.Int:
- return strconv.FormatInt(dataVal.Int(), 10), nil
- case reflect.Slice:
- dataType := dataVal.Type()
- elemKind := dataType.Elem().Kind()
- if elemKind == reflect.Uint8 {
- return string(dataVal.Interface().([]uint8)), nil
- }
- case reflect.Uint:
- return strconv.FormatUint(dataVal.Uint(), 10), nil
- }
- }
-
- return data, nil
-}
-
-func RecursiveStructToMapHookFunc() DecodeHookFunc {
- return func(f reflect.Value, t reflect.Value) (interface{}, error) {
- if f.Kind() != reflect.Struct {
- return f.Interface(), nil
- }
-
- var i interface{} = struct{}{}
- if t.Type() != reflect.TypeOf(&i).Elem() {
- return f.Interface(), nil
- }
-
- m := make(map[string]interface{})
- t.Set(reflect.ValueOf(m))
-
- return f.Interface(), nil
- }
-}
-
-// TextUnmarshallerHookFunc returns a DecodeHookFunc that applies
-// strings to the UnmarshalText function, when the target type
-// implements the encoding.TextUnmarshaler interface
-func TextUnmarshallerHookFunc() DecodeHookFuncType {
- return func(
- f reflect.Type,
- t reflect.Type,
- data interface{}) (interface{}, error) {
- if f.Kind() != reflect.String {
- return data, nil
- }
- result := reflect.New(t).Interface()
- unmarshaller, ok := result.(encoding.TextUnmarshaler)
- if !ok {
- return data, nil
- }
- if err := unmarshaller.UnmarshalText([]byte(data.(string))); err != nil {
- return nil, err
- }
- return result, nil
- }
-}
diff --git a/vendor/github.com/mitchellh/mapstructure/error.go b/vendor/github.com/mitchellh/mapstructure/error.go
deleted file mode 100644
index 47a99e5a..00000000
--- a/vendor/github.com/mitchellh/mapstructure/error.go
+++ /dev/null
@@ -1,50 +0,0 @@
-package mapstructure
-
-import (
- "errors"
- "fmt"
- "sort"
- "strings"
-)
-
-// Error implements the error interface and can represents multiple
-// errors that occur in the course of a single decode.
-type Error struct {
- Errors []string
-}
-
-func (e *Error) Error() string {
- points := make([]string, len(e.Errors))
- for i, err := range e.Errors {
- points[i] = fmt.Sprintf("* %s", err)
- }
-
- sort.Strings(points)
- return fmt.Sprintf(
- "%d error(s) decoding:\n\n%s",
- len(e.Errors), strings.Join(points, "\n"))
-}
-
-// WrappedErrors implements the errwrap.Wrapper interface to make this
-// return value more useful with the errwrap and go-multierror libraries.
-func (e *Error) WrappedErrors() []error {
- if e == nil {
- return nil
- }
-
- result := make([]error, len(e.Errors))
- for i, e := range e.Errors {
- result[i] = errors.New(e)
- }
-
- return result
-}
-
-func appendErrors(errors []string, err error) []string {
- switch e := err.(type) {
- case *Error:
- return append(errors, e.Errors...)
- default:
- return append(errors, e.Error())
- }
-}
diff --git a/vendor/github.com/mitchellh/mapstructure/mapstructure.go b/vendor/github.com/mitchellh/mapstructure/mapstructure.go
deleted file mode 100644
index 1efb22ac..00000000
--- a/vendor/github.com/mitchellh/mapstructure/mapstructure.go
+++ /dev/null
@@ -1,1540 +0,0 @@
-// Package mapstructure exposes functionality to convert one arbitrary
-// Go type into another, typically to convert a map[string]interface{}
-// into a native Go structure.
-//
-// The Go structure can be arbitrarily complex, containing slices,
-// other structs, etc. and the decoder will properly decode nested
-// maps and so on into the proper structures in the native Go struct.
-// See the examples to see what the decoder is capable of.
-//
-// The simplest function to start with is Decode.
-//
-// Field Tags
-//
-// When decoding to a struct, mapstructure will use the field name by
-// default to perform the mapping. For example, if a struct has a field
-// "Username" then mapstructure will look for a key in the source value
-// of "username" (case insensitive).
-//
-// type User struct {
-// Username string
-// }
-//
-// You can change the behavior of mapstructure by using struct tags.
-// The default struct tag that mapstructure looks for is "mapstructure"
-// but you can customize it using DecoderConfig.
-//
-// Renaming Fields
-//
-// To rename the key that mapstructure looks for, use the "mapstructure"
-// tag and set a value directly. For example, to change the "username" example
-// above to "user":
-//
-// type User struct {
-// Username string `mapstructure:"user"`
-// }
-//
-// Embedded Structs and Squashing
-//
-// Embedded structs are treated as if they're another field with that name.
-// By default, the two structs below are equivalent when decoding with
-// mapstructure:
-//
-// type Person struct {
-// Name string
-// }
-//
-// type Friend struct {
-// Person
-// }
-//
-// type Friend struct {
-// Person Person
-// }
-//
-// This would require an input that looks like below:
-//
-// map[string]interface{}{
-// "person": map[string]interface{}{"name": "alice"},
-// }
-//
-// If your "person" value is NOT nested, then you can append ",squash" to
-// your tag value and mapstructure will treat it as if the embedded struct
-// were part of the struct directly. Example:
-//
-// type Friend struct {
-// Person `mapstructure:",squash"`
-// }
-//
-// Now the following input would be accepted:
-//
-// map[string]interface{}{
-// "name": "alice",
-// }
-//
-// When decoding from a struct to a map, the squash tag squashes the struct
-// fields into a single map. Using the example structs from above:
-//
-// Friend{Person: Person{Name: "alice"}}
-//
-// Will be decoded into a map:
-//
-// map[string]interface{}{
-// "name": "alice",
-// }
-//
-// DecoderConfig has a field that changes the behavior of mapstructure
-// to always squash embedded structs.
-//
-// Remainder Values
-//
-// If there are any unmapped keys in the source value, mapstructure by
-// default will silently ignore them. You can error by setting ErrorUnused
-// in DecoderConfig. If you're using Metadata you can also maintain a slice
-// of the unused keys.
-//
-// You can also use the ",remain" suffix on your tag to collect all unused
-// values in a map. The field with this tag MUST be a map type and should
-// probably be a "map[string]interface{}" or "map[interface{}]interface{}".
-// See example below:
-//
-// type Friend struct {
-// Name string
-// Other map[string]interface{} `mapstructure:",remain"`
-// }
-//
-// Given the input below, Other would be populated with the other
-// values that weren't used (everything but "name"):
-//
-// map[string]interface{}{
-// "name": "bob",
-// "address": "123 Maple St.",
-// }
-//
-// Omit Empty Values
-//
-// When decoding from a struct to any other value, you may use the
-// ",omitempty" suffix on your tag to omit that value if it equates to
-// the zero value. The zero value of all types is specified in the Go
-// specification.
-//
-// For example, the zero type of a numeric type is zero ("0"). If the struct
-// field value is zero and a numeric type, the field is empty, and it won't
-// be encoded into the destination type.
-//
-// type Source struct {
-// Age int `mapstructure:",omitempty"`
-// }
-//
-// Unexported fields
-//
-// Since unexported (private) struct fields cannot be set outside the package
-// where they are defined, the decoder will simply skip them.
-//
-// For this output type definition:
-//
-// type Exported struct {
-// private string // this unexported field will be skipped
-// Public string
-// }
-//
-// Using this map as input:
-//
-// map[string]interface{}{
-// "private": "I will be ignored",
-// "Public": "I made it through!",
-// }
-//
-// The following struct will be decoded:
-//
-// type Exported struct {
-// private: "" // field is left with an empty string (zero value)
-// Public: "I made it through!"
-// }
-//
-// Other Configuration
-//
-// mapstructure is highly configurable. See the DecoderConfig struct
-// for other features and options that are supported.
-package mapstructure
-
-import (
- "encoding/json"
- "errors"
- "fmt"
- "reflect"
- "sort"
- "strconv"
- "strings"
-)
-
-// DecodeHookFunc is the callback function that can be used for
-// data transformations. See "DecodeHook" in the DecoderConfig
-// struct.
-//
-// The type must be one of DecodeHookFuncType, DecodeHookFuncKind, or
-// DecodeHookFuncValue.
-// Values are a superset of Types (Values can return types), and Types are a
-// superset of Kinds (Types can return Kinds) and are generally a richer thing
-// to use, but Kinds are simpler if you only need those.
-//
-// The reason DecodeHookFunc is multi-typed is for backwards compatibility:
-// we started with Kinds and then realized Types were the better solution,
-// but have a promise to not break backwards compat so we now support
-// both.
-type DecodeHookFunc interface{}
-
-// DecodeHookFuncType is a DecodeHookFunc which has complete information about
-// the source and target types.
-type DecodeHookFuncType func(reflect.Type, reflect.Type, interface{}) (interface{}, error)
-
-// DecodeHookFuncKind is a DecodeHookFunc which knows only the Kinds of the
-// source and target types.
-type DecodeHookFuncKind func(reflect.Kind, reflect.Kind, interface{}) (interface{}, error)
-
-// DecodeHookFuncValue is a DecodeHookFunc which has complete access to both the source and target
-// values.
-type DecodeHookFuncValue func(from reflect.Value, to reflect.Value) (interface{}, error)
-
-// DecoderConfig is the configuration that is used to create a new decoder
-// and allows customization of various aspects of decoding.
-type DecoderConfig struct {
- // DecodeHook, if set, will be called before any decoding and any
- // type conversion (if WeaklyTypedInput is on). This lets you modify
- // the values before they're set down onto the resulting struct. The
- // DecodeHook is called for every map and value in the input. This means
- // that if a struct has embedded fields with squash tags the decode hook
- // is called only once with all of the input data, not once for each
- // embedded struct.
- //
- // If an error is returned, the entire decode will fail with that error.
- DecodeHook DecodeHookFunc
-
- // If ErrorUnused is true, then it is an error for there to exist
- // keys in the original map that were unused in the decoding process
- // (extra keys).
- ErrorUnused bool
-
- // If ErrorUnset is true, then it is an error for there to exist
- // fields in the result that were not set in the decoding process
- // (extra fields). This only applies to decoding to a struct. This
- // will affect all nested structs as well.
- ErrorUnset bool
-
- // ZeroFields, if set to true, will zero fields before writing them.
- // For example, a map will be emptied before decoded values are put in
- // it. If this is false, a map will be merged.
- ZeroFields bool
-
- // If WeaklyTypedInput is true, the decoder will make the following
- // "weak" conversions:
- //
- // - bools to string (true = "1", false = "0")
- // - numbers to string (base 10)
- // - bools to int/uint (true = 1, false = 0)
- // - strings to int/uint (base implied by prefix)
- // - int to bool (true if value != 0)
- // - string to bool (accepts: 1, t, T, TRUE, true, True, 0, f, F,
- // FALSE, false, False. Anything else is an error)
- // - empty array = empty map and vice versa
- // - negative numbers to overflowed uint values (base 10)
- // - slice of maps to a merged map
- // - single values are converted to slices if required. Each
- // element is weakly decoded. For example: "4" can become []int{4}
- // if the target type is an int slice.
- //
- WeaklyTypedInput bool
-
- // Squash will squash embedded structs. A squash tag may also be
- // added to an individual struct field using a tag. For example:
- //
- // type Parent struct {
- // Child `mapstructure:",squash"`
- // }
- Squash bool
-
- // Metadata is the struct that will contain extra metadata about
- // the decoding. If this is nil, then no metadata will be tracked.
- Metadata *Metadata
-
- // Result is a pointer to the struct that will contain the decoded
- // value.
- Result interface{}
-
- // The tag name that mapstructure reads for field names. This
- // defaults to "mapstructure"
- TagName string
-
- // IgnoreUntaggedFields ignores all struct fields without explicit
- // TagName, comparable to `mapstructure:"-"` as default behaviour.
- IgnoreUntaggedFields bool
-
- // MatchName is the function used to match the map key to the struct
- // field name or tag. Defaults to `strings.EqualFold`. This can be used
- // to implement case-sensitive tag values, support snake casing, etc.
- MatchName func(mapKey, fieldName string) bool
-}
-
-// A Decoder takes a raw interface value and turns it into structured
-// data, keeping track of rich error information along the way in case
-// anything goes wrong. Unlike the basic top-level Decode method, you can
-// more finely control how the Decoder behaves using the DecoderConfig
-// structure. The top-level Decode method is just a convenience that sets
-// up the most basic Decoder.
-type Decoder struct {
- config *DecoderConfig
-}
-
-// Metadata contains information about decoding a structure that
-// is tedious or difficult to get otherwise.
-type Metadata struct {
- // Keys are the keys of the structure which were successfully decoded
- Keys []string
-
- // Unused is a slice of keys that were found in the raw value but
- // weren't decoded since there was no matching field in the result interface
- Unused []string
-
- // Unset is a slice of field names that were found in the result interface
- // but weren't set in the decoding process since there was no matching value
- // in the input
- Unset []string
-}
-
-// Decode takes an input structure and uses reflection to translate it to
-// the output structure. output must be a pointer to a map or struct.
-func Decode(input interface{}, output interface{}) error {
- config := &DecoderConfig{
- Metadata: nil,
- Result: output,
- }
-
- decoder, err := NewDecoder(config)
- if err != nil {
- return err
- }
-
- return decoder.Decode(input)
-}
-
-// WeakDecode is the same as Decode but is shorthand to enable
-// WeaklyTypedInput. See DecoderConfig for more info.
-func WeakDecode(input, output interface{}) error {
- config := &DecoderConfig{
- Metadata: nil,
- Result: output,
- WeaklyTypedInput: true,
- }
-
- decoder, err := NewDecoder(config)
- if err != nil {
- return err
- }
-
- return decoder.Decode(input)
-}
-
-// DecodeMetadata is the same as Decode, but is shorthand to
-// enable metadata collection. See DecoderConfig for more info.
-func DecodeMetadata(input interface{}, output interface{}, metadata *Metadata) error {
- config := &DecoderConfig{
- Metadata: metadata,
- Result: output,
- }
-
- decoder, err := NewDecoder(config)
- if err != nil {
- return err
- }
-
- return decoder.Decode(input)
-}
-
-// WeakDecodeMetadata is the same as Decode, but is shorthand to
-// enable both WeaklyTypedInput and metadata collection. See
-// DecoderConfig for more info.
-func WeakDecodeMetadata(input interface{}, output interface{}, metadata *Metadata) error {
- config := &DecoderConfig{
- Metadata: metadata,
- Result: output,
- WeaklyTypedInput: true,
- }
-
- decoder, err := NewDecoder(config)
- if err != nil {
- return err
- }
-
- return decoder.Decode(input)
-}
-
-// NewDecoder returns a new decoder for the given configuration. Once
-// a decoder has been returned, the same configuration must not be used
-// again.
-func NewDecoder(config *DecoderConfig) (*Decoder, error) {
- val := reflect.ValueOf(config.Result)
- if val.Kind() != reflect.Ptr {
- return nil, errors.New("result must be a pointer")
- }
-
- val = val.Elem()
- if !val.CanAddr() {
- return nil, errors.New("result must be addressable (a pointer)")
- }
-
- if config.Metadata != nil {
- if config.Metadata.Keys == nil {
- config.Metadata.Keys = make([]string, 0)
- }
-
- if config.Metadata.Unused == nil {
- config.Metadata.Unused = make([]string, 0)
- }
-
- if config.Metadata.Unset == nil {
- config.Metadata.Unset = make([]string, 0)
- }
- }
-
- if config.TagName == "" {
- config.TagName = "mapstructure"
- }
-
- if config.MatchName == nil {
- config.MatchName = strings.EqualFold
- }
-
- result := &Decoder{
- config: config,
- }
-
- return result, nil
-}
-
-// Decode decodes the given raw interface to the target pointer specified
-// by the configuration.
-func (d *Decoder) Decode(input interface{}) error {
- return d.decode("", input, reflect.ValueOf(d.config.Result).Elem())
-}
-
-// Decodes an unknown data type into a specific reflection value.
-func (d *Decoder) decode(name string, input interface{}, outVal reflect.Value) error {
- var inputVal reflect.Value
- if input != nil {
- inputVal = reflect.ValueOf(input)
-
- // We need to check here if input is a typed nil. Typed nils won't
- // match the "input == nil" below so we check that here.
- if inputVal.Kind() == reflect.Ptr && inputVal.IsNil() {
- input = nil
- }
- }
-
- if input == nil {
- // If the data is nil, then we don't set anything, unless ZeroFields is set
- // to true.
- if d.config.ZeroFields {
- outVal.Set(reflect.Zero(outVal.Type()))
-
- if d.config.Metadata != nil && name != "" {
- d.config.Metadata.Keys = append(d.config.Metadata.Keys, name)
- }
- }
- return nil
- }
-
- if !inputVal.IsValid() {
- // If the input value is invalid, then we just set the value
- // to be the zero value.
- outVal.Set(reflect.Zero(outVal.Type()))
- if d.config.Metadata != nil && name != "" {
- d.config.Metadata.Keys = append(d.config.Metadata.Keys, name)
- }
- return nil
- }
-
- if d.config.DecodeHook != nil {
- // We have a DecodeHook, so let's pre-process the input.
- var err error
- input, err = DecodeHookExec(d.config.DecodeHook, inputVal, outVal)
- if err != nil {
- return fmt.Errorf("error decoding '%s': %s", name, err)
- }
- }
-
- var err error
- outputKind := getKind(outVal)
- addMetaKey := true
- switch outputKind {
- case reflect.Bool:
- err = d.decodeBool(name, input, outVal)
- case reflect.Interface:
- err = d.decodeBasic(name, input, outVal)
- case reflect.String:
- err = d.decodeString(name, input, outVal)
- case reflect.Int:
- err = d.decodeInt(name, input, outVal)
- case reflect.Uint:
- err = d.decodeUint(name, input, outVal)
- case reflect.Float32:
- err = d.decodeFloat(name, input, outVal)
- case reflect.Struct:
- err = d.decodeStruct(name, input, outVal)
- case reflect.Map:
- err = d.decodeMap(name, input, outVal)
- case reflect.Ptr:
- addMetaKey, err = d.decodePtr(name, input, outVal)
- case reflect.Slice:
- err = d.decodeSlice(name, input, outVal)
- case reflect.Array:
- err = d.decodeArray(name, input, outVal)
- case reflect.Func:
- err = d.decodeFunc(name, input, outVal)
- default:
- // If we reached this point then we weren't able to decode it
- return fmt.Errorf("%s: unsupported type: %s", name, outputKind)
- }
-
- // If we reached here, then we successfully decoded SOMETHING, so
- // mark the key as used if we're tracking metainput.
- if addMetaKey && d.config.Metadata != nil && name != "" {
- d.config.Metadata.Keys = append(d.config.Metadata.Keys, name)
- }
-
- return err
-}
-
-// This decodes a basic type (bool, int, string, etc.) and sets the
-// value to "data" of that type.
-func (d *Decoder) decodeBasic(name string, data interface{}, val reflect.Value) error {
- if val.IsValid() && val.Elem().IsValid() {
- elem := val.Elem()
-
- // If we can't address this element, then its not writable. Instead,
- // we make a copy of the value (which is a pointer and therefore
- // writable), decode into that, and replace the whole value.
- copied := false
- if !elem.CanAddr() {
- copied = true
-
- // Make *T
- copy := reflect.New(elem.Type())
-
- // *T = elem
- copy.Elem().Set(elem)
-
- // Set elem so we decode into it
- elem = copy
- }
-
- // Decode. If we have an error then return. We also return right
- // away if we're not a copy because that means we decoded directly.
- if err := d.decode(name, data, elem); err != nil || !copied {
- return err
- }
-
- // If we're a copy, we need to set te final result
- val.Set(elem.Elem())
- return nil
- }
-
- dataVal := reflect.ValueOf(data)
-
- // If the input data is a pointer, and the assigned type is the dereference
- // of that exact pointer, then indirect it so that we can assign it.
- // Example: *string to string
- if dataVal.Kind() == reflect.Ptr && dataVal.Type().Elem() == val.Type() {
- dataVal = reflect.Indirect(dataVal)
- }
-
- if !dataVal.IsValid() {
- dataVal = reflect.Zero(val.Type())
- }
-
- dataValType := dataVal.Type()
- if !dataValType.AssignableTo(val.Type()) {
- return fmt.Errorf(
- "'%s' expected type '%s', got '%s'",
- name, val.Type(), dataValType)
- }
-
- val.Set(dataVal)
- return nil
-}
-
-func (d *Decoder) decodeString(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataKind := getKind(dataVal)
-
- converted := true
- switch {
- case dataKind == reflect.String:
- val.SetString(dataVal.String())
- case dataKind == reflect.Bool && d.config.WeaklyTypedInput:
- if dataVal.Bool() {
- val.SetString("1")
- } else {
- val.SetString("0")
- }
- case dataKind == reflect.Int && d.config.WeaklyTypedInput:
- val.SetString(strconv.FormatInt(dataVal.Int(), 10))
- case dataKind == reflect.Uint && d.config.WeaklyTypedInput:
- val.SetString(strconv.FormatUint(dataVal.Uint(), 10))
- case dataKind == reflect.Float32 && d.config.WeaklyTypedInput:
- val.SetString(strconv.FormatFloat(dataVal.Float(), 'f', -1, 64))
- case dataKind == reflect.Slice && d.config.WeaklyTypedInput,
- dataKind == reflect.Array && d.config.WeaklyTypedInput:
- dataType := dataVal.Type()
- elemKind := dataType.Elem().Kind()
- switch elemKind {
- case reflect.Uint8:
- var uints []uint8
- if dataKind == reflect.Array {
- uints = make([]uint8, dataVal.Len(), dataVal.Len())
- for i := range uints {
- uints[i] = dataVal.Index(i).Interface().(uint8)
- }
- } else {
- uints = dataVal.Interface().([]uint8)
- }
- val.SetString(string(uints))
- default:
- converted = false
- }
- default:
- converted = false
- }
-
- if !converted {
- return fmt.Errorf(
- "'%s' expected type '%s', got unconvertible type '%s', value: '%v'",
- name, val.Type(), dataVal.Type(), data)
- }
-
- return nil
-}
-
-func (d *Decoder) decodeInt(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataKind := getKind(dataVal)
- dataType := dataVal.Type()
-
- switch {
- case dataKind == reflect.Int:
- val.SetInt(dataVal.Int())
- case dataKind == reflect.Uint:
- val.SetInt(int64(dataVal.Uint()))
- case dataKind == reflect.Float32:
- val.SetInt(int64(dataVal.Float()))
- case dataKind == reflect.Bool && d.config.WeaklyTypedInput:
- if dataVal.Bool() {
- val.SetInt(1)
- } else {
- val.SetInt(0)
- }
- case dataKind == reflect.String && d.config.WeaklyTypedInput:
- str := dataVal.String()
- if str == "" {
- str = "0"
- }
-
- i, err := strconv.ParseInt(str, 0, val.Type().Bits())
- if err == nil {
- val.SetInt(i)
- } else {
- return fmt.Errorf("cannot parse '%s' as int: %s", name, err)
- }
- case dataType.PkgPath() == "encoding/json" && dataType.Name() == "Number":
- jn := data.(json.Number)
- i, err := jn.Int64()
- if err != nil {
- return fmt.Errorf(
- "error decoding json.Number into %s: %s", name, err)
- }
- val.SetInt(i)
- default:
- return fmt.Errorf(
- "'%s' expected type '%s', got unconvertible type '%s', value: '%v'",
- name, val.Type(), dataVal.Type(), data)
- }
-
- return nil
-}
-
-func (d *Decoder) decodeUint(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataKind := getKind(dataVal)
- dataType := dataVal.Type()
-
- switch {
- case dataKind == reflect.Int:
- i := dataVal.Int()
- if i < 0 && !d.config.WeaklyTypedInput {
- return fmt.Errorf("cannot parse '%s', %d overflows uint",
- name, i)
- }
- val.SetUint(uint64(i))
- case dataKind == reflect.Uint:
- val.SetUint(dataVal.Uint())
- case dataKind == reflect.Float32:
- f := dataVal.Float()
- if f < 0 && !d.config.WeaklyTypedInput {
- return fmt.Errorf("cannot parse '%s', %f overflows uint",
- name, f)
- }
- val.SetUint(uint64(f))
- case dataKind == reflect.Bool && d.config.WeaklyTypedInput:
- if dataVal.Bool() {
- val.SetUint(1)
- } else {
- val.SetUint(0)
- }
- case dataKind == reflect.String && d.config.WeaklyTypedInput:
- str := dataVal.String()
- if str == "" {
- str = "0"
- }
-
- i, err := strconv.ParseUint(str, 0, val.Type().Bits())
- if err == nil {
- val.SetUint(i)
- } else {
- return fmt.Errorf("cannot parse '%s' as uint: %s", name, err)
- }
- case dataType.PkgPath() == "encoding/json" && dataType.Name() == "Number":
- jn := data.(json.Number)
- i, err := strconv.ParseUint(string(jn), 0, 64)
- if err != nil {
- return fmt.Errorf(
- "error decoding json.Number into %s: %s", name, err)
- }
- val.SetUint(i)
- default:
- return fmt.Errorf(
- "'%s' expected type '%s', got unconvertible type '%s', value: '%v'",
- name, val.Type(), dataVal.Type(), data)
- }
-
- return nil
-}
-
-func (d *Decoder) decodeBool(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataKind := getKind(dataVal)
-
- switch {
- case dataKind == reflect.Bool:
- val.SetBool(dataVal.Bool())
- case dataKind == reflect.Int && d.config.WeaklyTypedInput:
- val.SetBool(dataVal.Int() != 0)
- case dataKind == reflect.Uint && d.config.WeaklyTypedInput:
- val.SetBool(dataVal.Uint() != 0)
- case dataKind == reflect.Float32 && d.config.WeaklyTypedInput:
- val.SetBool(dataVal.Float() != 0)
- case dataKind == reflect.String && d.config.WeaklyTypedInput:
- b, err := strconv.ParseBool(dataVal.String())
- if err == nil {
- val.SetBool(b)
- } else if dataVal.String() == "" {
- val.SetBool(false)
- } else {
- return fmt.Errorf("cannot parse '%s' as bool: %s", name, err)
- }
- default:
- return fmt.Errorf(
- "'%s' expected type '%s', got unconvertible type '%s', value: '%v'",
- name, val.Type(), dataVal.Type(), data)
- }
-
- return nil
-}
-
-func (d *Decoder) decodeFloat(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataKind := getKind(dataVal)
- dataType := dataVal.Type()
-
- switch {
- case dataKind == reflect.Int:
- val.SetFloat(float64(dataVal.Int()))
- case dataKind == reflect.Uint:
- val.SetFloat(float64(dataVal.Uint()))
- case dataKind == reflect.Float32:
- val.SetFloat(dataVal.Float())
- case dataKind == reflect.Bool && d.config.WeaklyTypedInput:
- if dataVal.Bool() {
- val.SetFloat(1)
- } else {
- val.SetFloat(0)
- }
- case dataKind == reflect.String && d.config.WeaklyTypedInput:
- str := dataVal.String()
- if str == "" {
- str = "0"
- }
-
- f, err := strconv.ParseFloat(str, val.Type().Bits())
- if err == nil {
- val.SetFloat(f)
- } else {
- return fmt.Errorf("cannot parse '%s' as float: %s", name, err)
- }
- case dataType.PkgPath() == "encoding/json" && dataType.Name() == "Number":
- jn := data.(json.Number)
- i, err := jn.Float64()
- if err != nil {
- return fmt.Errorf(
- "error decoding json.Number into %s: %s", name, err)
- }
- val.SetFloat(i)
- default:
- return fmt.Errorf(
- "'%s' expected type '%s', got unconvertible type '%s', value: '%v'",
- name, val.Type(), dataVal.Type(), data)
- }
-
- return nil
-}
-
-func (d *Decoder) decodeMap(name string, data interface{}, val reflect.Value) error {
- valType := val.Type()
- valKeyType := valType.Key()
- valElemType := valType.Elem()
-
- // By default we overwrite keys in the current map
- valMap := val
-
- // If the map is nil or we're purposely zeroing fields, make a new map
- if valMap.IsNil() || d.config.ZeroFields {
- // Make a new map to hold our result
- mapType := reflect.MapOf(valKeyType, valElemType)
- valMap = reflect.MakeMap(mapType)
- }
-
- // Check input type and based on the input type jump to the proper func
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- switch dataVal.Kind() {
- case reflect.Map:
- return d.decodeMapFromMap(name, dataVal, val, valMap)
-
- case reflect.Struct:
- return d.decodeMapFromStruct(name, dataVal, val, valMap)
-
- case reflect.Array, reflect.Slice:
- if d.config.WeaklyTypedInput {
- return d.decodeMapFromSlice(name, dataVal, val, valMap)
- }
-
- fallthrough
-
- default:
- return fmt.Errorf("'%s' expected a map, got '%s'", name, dataVal.Kind())
- }
-}
-
-func (d *Decoder) decodeMapFromSlice(name string, dataVal reflect.Value, val reflect.Value, valMap reflect.Value) error {
- // Special case for BC reasons (covered by tests)
- if dataVal.Len() == 0 {
- val.Set(valMap)
- return nil
- }
-
- for i := 0; i < dataVal.Len(); i++ {
- err := d.decode(
- name+"["+strconv.Itoa(i)+"]",
- dataVal.Index(i).Interface(), val)
- if err != nil {
- return err
- }
- }
-
- return nil
-}
-
-func (d *Decoder) decodeMapFromMap(name string, dataVal reflect.Value, val reflect.Value, valMap reflect.Value) error {
- valType := val.Type()
- valKeyType := valType.Key()
- valElemType := valType.Elem()
-
- // Accumulate errors
- errors := make([]string, 0)
-
- // If the input data is empty, then we just match what the input data is.
- if dataVal.Len() == 0 {
- if dataVal.IsNil() {
- if !val.IsNil() {
- val.Set(dataVal)
- }
- } else {
- // Set to empty allocated value
- val.Set(valMap)
- }
-
- return nil
- }
-
- for _, k := range dataVal.MapKeys() {
- fieldName := name + "[" + k.String() + "]"
-
- // First decode the key into the proper type
- currentKey := reflect.Indirect(reflect.New(valKeyType))
- if err := d.decode(fieldName, k.Interface(), currentKey); err != nil {
- errors = appendErrors(errors, err)
- continue
- }
-
- // Next decode the data into the proper type
- v := dataVal.MapIndex(k).Interface()
- currentVal := reflect.Indirect(reflect.New(valElemType))
- if err := d.decode(fieldName, v, currentVal); err != nil {
- errors = appendErrors(errors, err)
- continue
- }
-
- valMap.SetMapIndex(currentKey, currentVal)
- }
-
- // Set the built up map to the value
- val.Set(valMap)
-
- // If we had errors, return those
- if len(errors) > 0 {
- return &Error{errors}
- }
-
- return nil
-}
-
-func (d *Decoder) decodeMapFromStruct(name string, dataVal reflect.Value, val reflect.Value, valMap reflect.Value) error {
- typ := dataVal.Type()
- for i := 0; i < typ.NumField(); i++ {
- // Get the StructField first since this is a cheap operation. If the
- // field is unexported, then ignore it.
- f := typ.Field(i)
- if f.PkgPath != "" {
- continue
- }
-
- // Next get the actual value of this field and verify it is assignable
- // to the map value.
- v := dataVal.Field(i)
- if !v.Type().AssignableTo(valMap.Type().Elem()) {
- return fmt.Errorf("cannot assign type '%s' to map value field of type '%s'", v.Type(), valMap.Type().Elem())
- }
-
- tagValue := f.Tag.Get(d.config.TagName)
- keyName := f.Name
-
- if tagValue == "" && d.config.IgnoreUntaggedFields {
- continue
- }
-
- // If Squash is set in the config, we squash the field down.
- squash := d.config.Squash && v.Kind() == reflect.Struct && f.Anonymous
-
- v = dereferencePtrToStructIfNeeded(v, d.config.TagName)
-
- // Determine the name of the key in the map
- if index := strings.Index(tagValue, ","); index != -1 {
- if tagValue[:index] == "-" {
- continue
- }
- // If "omitempty" is specified in the tag, it ignores empty values.
- if strings.Index(tagValue[index+1:], "omitempty") != -1 && isEmptyValue(v) {
- continue
- }
-
- // If "squash" is specified in the tag, we squash the field down.
- squash = squash || strings.Index(tagValue[index+1:], "squash") != -1
- if squash {
- // When squashing, the embedded type can be a pointer to a struct.
- if v.Kind() == reflect.Ptr && v.Elem().Kind() == reflect.Struct {
- v = v.Elem()
- }
-
- // The final type must be a struct
- if v.Kind() != reflect.Struct {
- return fmt.Errorf("cannot squash non-struct type '%s'", v.Type())
- }
- }
- if keyNameTagValue := tagValue[:index]; keyNameTagValue != "" {
- keyName = keyNameTagValue
- }
- } else if len(tagValue) > 0 {
- if tagValue == "-" {
- continue
- }
- keyName = tagValue
- }
-
- switch v.Kind() {
- // this is an embedded struct, so handle it differently
- case reflect.Struct:
- x := reflect.New(v.Type())
- x.Elem().Set(v)
-
- vType := valMap.Type()
- vKeyType := vType.Key()
- vElemType := vType.Elem()
- mType := reflect.MapOf(vKeyType, vElemType)
- vMap := reflect.MakeMap(mType)
-
- // Creating a pointer to a map so that other methods can completely
- // overwrite the map if need be (looking at you decodeMapFromMap). The
- // indirection allows the underlying map to be settable (CanSet() == true)
- // where as reflect.MakeMap returns an unsettable map.
- addrVal := reflect.New(vMap.Type())
- reflect.Indirect(addrVal).Set(vMap)
-
- err := d.decode(keyName, x.Interface(), reflect.Indirect(addrVal))
- if err != nil {
- return err
- }
-
- // the underlying map may have been completely overwritten so pull
- // it indirectly out of the enclosing value.
- vMap = reflect.Indirect(addrVal)
-
- if squash {
- for _, k := range vMap.MapKeys() {
- valMap.SetMapIndex(k, vMap.MapIndex(k))
- }
- } else {
- valMap.SetMapIndex(reflect.ValueOf(keyName), vMap)
- }
-
- default:
- valMap.SetMapIndex(reflect.ValueOf(keyName), v)
- }
- }
-
- if val.CanAddr() {
- val.Set(valMap)
- }
-
- return nil
-}
-
-func (d *Decoder) decodePtr(name string, data interface{}, val reflect.Value) (bool, error) {
- // If the input data is nil, then we want to just set the output
- // pointer to be nil as well.
- isNil := data == nil
- if !isNil {
- switch v := reflect.Indirect(reflect.ValueOf(data)); v.Kind() {
- case reflect.Chan,
- reflect.Func,
- reflect.Interface,
- reflect.Map,
- reflect.Ptr,
- reflect.Slice:
- isNil = v.IsNil()
- }
- }
- if isNil {
- if !val.IsNil() && val.CanSet() {
- nilValue := reflect.New(val.Type()).Elem()
- val.Set(nilValue)
- }
-
- return true, nil
- }
-
- // Create an element of the concrete (non pointer) type and decode
- // into that. Then set the value of the pointer to this type.
- valType := val.Type()
- valElemType := valType.Elem()
- if val.CanSet() {
- realVal := val
- if realVal.IsNil() || d.config.ZeroFields {
- realVal = reflect.New(valElemType)
- }
-
- if err := d.decode(name, data, reflect.Indirect(realVal)); err != nil {
- return false, err
- }
-
- val.Set(realVal)
- } else {
- if err := d.decode(name, data, reflect.Indirect(val)); err != nil {
- return false, err
- }
- }
- return false, nil
-}
-
-func (d *Decoder) decodeFunc(name string, data interface{}, val reflect.Value) error {
- // Create an element of the concrete (non pointer) type and decode
- // into that. Then set the value of the pointer to this type.
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- if val.Type() != dataVal.Type() {
- return fmt.Errorf(
- "'%s' expected type '%s', got unconvertible type '%s', value: '%v'",
- name, val.Type(), dataVal.Type(), data)
- }
- val.Set(dataVal)
- return nil
-}
-
-func (d *Decoder) decodeSlice(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataValKind := dataVal.Kind()
- valType := val.Type()
- valElemType := valType.Elem()
- sliceType := reflect.SliceOf(valElemType)
-
- // If we have a non array/slice type then we first attempt to convert.
- if dataValKind != reflect.Array && dataValKind != reflect.Slice {
- if d.config.WeaklyTypedInput {
- switch {
- // Slice and array we use the normal logic
- case dataValKind == reflect.Slice, dataValKind == reflect.Array:
- break
-
- // Empty maps turn into empty slices
- case dataValKind == reflect.Map:
- if dataVal.Len() == 0 {
- val.Set(reflect.MakeSlice(sliceType, 0, 0))
- return nil
- }
- // Create slice of maps of other sizes
- return d.decodeSlice(name, []interface{}{data}, val)
-
- case dataValKind == reflect.String && valElemType.Kind() == reflect.Uint8:
- return d.decodeSlice(name, []byte(dataVal.String()), val)
-
- // All other types we try to convert to the slice type
- // and "lift" it into it. i.e. a string becomes a string slice.
- default:
- // Just re-try this function with data as a slice.
- return d.decodeSlice(name, []interface{}{data}, val)
- }
- }
-
- return fmt.Errorf(
- "'%s': source data must be an array or slice, got %s", name, dataValKind)
- }
-
- // If the input value is nil, then don't allocate since empty != nil
- if dataValKind != reflect.Array && dataVal.IsNil() {
- return nil
- }
-
- valSlice := val
- if valSlice.IsNil() || d.config.ZeroFields {
- // Make a new slice to hold our result, same size as the original data.
- valSlice = reflect.MakeSlice(sliceType, dataVal.Len(), dataVal.Len())
- }
-
- // Accumulate any errors
- errors := make([]string, 0)
-
- for i := 0; i < dataVal.Len(); i++ {
- currentData := dataVal.Index(i).Interface()
- for valSlice.Len() <= i {
- valSlice = reflect.Append(valSlice, reflect.Zero(valElemType))
- }
- currentField := valSlice.Index(i)
-
- fieldName := name + "[" + strconv.Itoa(i) + "]"
- if err := d.decode(fieldName, currentData, currentField); err != nil {
- errors = appendErrors(errors, err)
- }
- }
-
- // Finally, set the value to the slice we built up
- val.Set(valSlice)
-
- // If there were errors, we return those
- if len(errors) > 0 {
- return &Error{errors}
- }
-
- return nil
-}
-
-func (d *Decoder) decodeArray(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
- dataValKind := dataVal.Kind()
- valType := val.Type()
- valElemType := valType.Elem()
- arrayType := reflect.ArrayOf(valType.Len(), valElemType)
-
- valArray := val
-
- if valArray.Interface() == reflect.Zero(valArray.Type()).Interface() || d.config.ZeroFields {
- // Check input type
- if dataValKind != reflect.Array && dataValKind != reflect.Slice {
- if d.config.WeaklyTypedInput {
- switch {
- // Empty maps turn into empty arrays
- case dataValKind == reflect.Map:
- if dataVal.Len() == 0 {
- val.Set(reflect.Zero(arrayType))
- return nil
- }
-
- // All other types we try to convert to the array type
- // and "lift" it into it. i.e. a string becomes a string array.
- default:
- // Just re-try this function with data as a slice.
- return d.decodeArray(name, []interface{}{data}, val)
- }
- }
-
- return fmt.Errorf(
- "'%s': source data must be an array or slice, got %s", name, dataValKind)
-
- }
- if dataVal.Len() > arrayType.Len() {
- return fmt.Errorf(
- "'%s': expected source data to have length less or equal to %d, got %d", name, arrayType.Len(), dataVal.Len())
-
- }
-
- // Make a new array to hold our result, same size as the original data.
- valArray = reflect.New(arrayType).Elem()
- }
-
- // Accumulate any errors
- errors := make([]string, 0)
-
- for i := 0; i < dataVal.Len(); i++ {
- currentData := dataVal.Index(i).Interface()
- currentField := valArray.Index(i)
-
- fieldName := name + "[" + strconv.Itoa(i) + "]"
- if err := d.decode(fieldName, currentData, currentField); err != nil {
- errors = appendErrors(errors, err)
- }
- }
-
- // Finally, set the value to the array we built up
- val.Set(valArray)
-
- // If there were errors, we return those
- if len(errors) > 0 {
- return &Error{errors}
- }
-
- return nil
-}
-
-func (d *Decoder) decodeStruct(name string, data interface{}, val reflect.Value) error {
- dataVal := reflect.Indirect(reflect.ValueOf(data))
-
- // If the type of the value to write to and the data match directly,
- // then we just set it directly instead of recursing into the structure.
- if dataVal.Type() == val.Type() {
- val.Set(dataVal)
- return nil
- }
-
- dataValKind := dataVal.Kind()
- switch dataValKind {
- case reflect.Map:
- return d.decodeStructFromMap(name, dataVal, val)
-
- case reflect.Struct:
- // Not the most efficient way to do this but we can optimize later if
- // we want to. To convert from struct to struct we go to map first
- // as an intermediary.
-
- // Make a new map to hold our result
- mapType := reflect.TypeOf((map[string]interface{})(nil))
- mval := reflect.MakeMap(mapType)
-
- // Creating a pointer to a map so that other methods can completely
- // overwrite the map if need be (looking at you decodeMapFromMap). The
- // indirection allows the underlying map to be settable (CanSet() == true)
- // where as reflect.MakeMap returns an unsettable map.
- addrVal := reflect.New(mval.Type())
-
- reflect.Indirect(addrVal).Set(mval)
- if err := d.decodeMapFromStruct(name, dataVal, reflect.Indirect(addrVal), mval); err != nil {
- return err
- }
-
- result := d.decodeStructFromMap(name, reflect.Indirect(addrVal), val)
- return result
-
- default:
- return fmt.Errorf("'%s' expected a map, got '%s'", name, dataVal.Kind())
- }
-}
-
-func (d *Decoder) decodeStructFromMap(name string, dataVal, val reflect.Value) error {
- dataValType := dataVal.Type()
- if kind := dataValType.Key().Kind(); kind != reflect.String && kind != reflect.Interface {
- return fmt.Errorf(
- "'%s' needs a map with string keys, has '%s' keys",
- name, dataValType.Key().Kind())
- }
-
- dataValKeys := make(map[reflect.Value]struct{})
- dataValKeysUnused := make(map[interface{}]struct{})
- for _, dataValKey := range dataVal.MapKeys() {
- dataValKeys[dataValKey] = struct{}{}
- dataValKeysUnused[dataValKey.Interface()] = struct{}{}
- }
-
- targetValKeysUnused := make(map[interface{}]struct{})
- errors := make([]string, 0)
-
- // This slice will keep track of all the structs we'll be decoding.
- // There can be more than one struct if there are embedded structs
- // that are squashed.
- structs := make([]reflect.Value, 1, 5)
- structs[0] = val
-
- // Compile the list of all the fields that we're going to be decoding
- // from all the structs.
- type field struct {
- field reflect.StructField
- val reflect.Value
- }
-
- // remainField is set to a valid field set with the "remain" tag if
- // we are keeping track of remaining values.
- var remainField *field
-
- fields := []field{}
- for len(structs) > 0 {
- structVal := structs[0]
- structs = structs[1:]
-
- structType := structVal.Type()
-
- for i := 0; i < structType.NumField(); i++ {
- fieldType := structType.Field(i)
- fieldVal := structVal.Field(i)
- if fieldVal.Kind() == reflect.Ptr && fieldVal.Elem().Kind() == reflect.Struct {
- // Handle embedded struct pointers as embedded structs.
- fieldVal = fieldVal.Elem()
- }
-
- // If "squash" is specified in the tag, we squash the field down.
- squash := d.config.Squash && fieldVal.Kind() == reflect.Struct && fieldType.Anonymous
- remain := false
-
- // We always parse the tags cause we're looking for other tags too
- tagParts := strings.Split(fieldType.Tag.Get(d.config.TagName), ",")
- for _, tag := range tagParts[1:] {
- if tag == "squash" {
- squash = true
- break
- }
-
- if tag == "remain" {
- remain = true
- break
- }
- }
-
- if squash {
- if fieldVal.Kind() != reflect.Struct {
- errors = appendErrors(errors,
- fmt.Errorf("%s: unsupported type for squash: %s", fieldType.Name, fieldVal.Kind()))
- } else {
- structs = append(structs, fieldVal)
- }
- continue
- }
-
- // Build our field
- if remain {
- remainField = &field{fieldType, fieldVal}
- } else {
- // Normal struct field, store it away
- fields = append(fields, field{fieldType, fieldVal})
- }
- }
- }
-
- // for fieldType, field := range fields {
- for _, f := range fields {
- field, fieldValue := f.field, f.val
- fieldName := field.Name
-
- tagValue := field.Tag.Get(d.config.TagName)
- tagValue = strings.SplitN(tagValue, ",", 2)[0]
- if tagValue != "" {
- fieldName = tagValue
- }
-
- rawMapKey := reflect.ValueOf(fieldName)
- rawMapVal := dataVal.MapIndex(rawMapKey)
- if !rawMapVal.IsValid() {
- // Do a slower search by iterating over each key and
- // doing case-insensitive search.
- for dataValKey := range dataValKeys {
- mK, ok := dataValKey.Interface().(string)
- if !ok {
- // Not a string key
- continue
- }
-
- if d.config.MatchName(mK, fieldName) {
- rawMapKey = dataValKey
- rawMapVal = dataVal.MapIndex(dataValKey)
- break
- }
- }
-
- if !rawMapVal.IsValid() {
- // There was no matching key in the map for the value in
- // the struct. Remember it for potential errors and metadata.
- targetValKeysUnused[fieldName] = struct{}{}
- continue
- }
- }
-
- if !fieldValue.IsValid() {
- // This should never happen
- panic("field is not valid")
- }
-
- // If we can't set the field, then it is unexported or something,
- // and we just continue onwards.
- if !fieldValue.CanSet() {
- continue
- }
-
- // Delete the key we're using from the unused map so we stop tracking
- delete(dataValKeysUnused, rawMapKey.Interface())
-
- // If the name is empty string, then we're at the root, and we
- // don't dot-join the fields.
- if name != "" {
- fieldName = name + "." + fieldName
- }
-
- if err := d.decode(fieldName, rawMapVal.Interface(), fieldValue); err != nil {
- errors = appendErrors(errors, err)
- }
- }
-
- // If we have a "remain"-tagged field and we have unused keys then
- // we put the unused keys directly into the remain field.
- if remainField != nil && len(dataValKeysUnused) > 0 {
- // Build a map of only the unused values
- remain := map[interface{}]interface{}{}
- for key := range dataValKeysUnused {
- remain[key] = dataVal.MapIndex(reflect.ValueOf(key)).Interface()
- }
-
- // Decode it as-if we were just decoding this map onto our map.
- if err := d.decodeMap(name, remain, remainField.val); err != nil {
- errors = appendErrors(errors, err)
- }
-
- // Set the map to nil so we have none so that the next check will
- // not error (ErrorUnused)
- dataValKeysUnused = nil
- }
-
- if d.config.ErrorUnused && len(dataValKeysUnused) > 0 {
- keys := make([]string, 0, len(dataValKeysUnused))
- for rawKey := range dataValKeysUnused {
- keys = append(keys, rawKey.(string))
- }
- sort.Strings(keys)
-
- err := fmt.Errorf("'%s' has invalid keys: %s", name, strings.Join(keys, ", "))
- errors = appendErrors(errors, err)
- }
-
- if d.config.ErrorUnset && len(targetValKeysUnused) > 0 {
- keys := make([]string, 0, len(targetValKeysUnused))
- for rawKey := range targetValKeysUnused {
- keys = append(keys, rawKey.(string))
- }
- sort.Strings(keys)
-
- err := fmt.Errorf("'%s' has unset fields: %s", name, strings.Join(keys, ", "))
- errors = appendErrors(errors, err)
- }
-
- if len(errors) > 0 {
- return &Error{errors}
- }
-
- // Add the unused keys to the list of unused keys if we're tracking metadata
- if d.config.Metadata != nil {
- for rawKey := range dataValKeysUnused {
- key := rawKey.(string)
- if name != "" {
- key = name + "." + key
- }
-
- d.config.Metadata.Unused = append(d.config.Metadata.Unused, key)
- }
- for rawKey := range targetValKeysUnused {
- key := rawKey.(string)
- if name != "" {
- key = name + "." + key
- }
-
- d.config.Metadata.Unset = append(d.config.Metadata.Unset, key)
- }
- }
-
- return nil
-}
-
-func isEmptyValue(v reflect.Value) bool {
- switch getKind(v) {
- case reflect.Array, reflect.Map, reflect.Slice, reflect.String:
- return v.Len() == 0
- case reflect.Bool:
- return !v.Bool()
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return v.Int() == 0
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return v.Uint() == 0
- case reflect.Float32, reflect.Float64:
- return v.Float() == 0
- case reflect.Interface, reflect.Ptr:
- return v.IsNil()
- }
- return false
-}
-
-func getKind(val reflect.Value) reflect.Kind {
- kind := val.Kind()
-
- switch {
- case kind >= reflect.Int && kind <= reflect.Int64:
- return reflect.Int
- case kind >= reflect.Uint && kind <= reflect.Uint64:
- return reflect.Uint
- case kind >= reflect.Float32 && kind <= reflect.Float64:
- return reflect.Float32
- default:
- return kind
- }
-}
-
-func isStructTypeConvertibleToMap(typ reflect.Type, checkMapstructureTags bool, tagName string) bool {
- for i := 0; i < typ.NumField(); i++ {
- f := typ.Field(i)
- if f.PkgPath == "" && !checkMapstructureTags { // check for unexported fields
- return true
- }
- if checkMapstructureTags && f.Tag.Get(tagName) != "" { // check for mapstructure tags inside
- return true
- }
- }
- return false
-}
-
-func dereferencePtrToStructIfNeeded(v reflect.Value, tagName string) reflect.Value {
- if v.Kind() != reflect.Ptr || v.Elem().Kind() != reflect.Struct {
- return v
- }
- deref := v.Elem()
- derefT := deref.Type()
- if isStructTypeConvertibleToMap(derefT, true, tagName) {
- return deref
- }
- return v
-}
diff --git a/vendor/github.com/mohae/deepcopy/.gitignore b/vendor/github.com/mohae/deepcopy/.gitignore
deleted file mode 100644
index 5846dd15..00000000
--- a/vendor/github.com/mohae/deepcopy/.gitignore
+++ /dev/null
@@ -1,26 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*~
-*.out
-*.log
diff --git a/vendor/github.com/mohae/deepcopy/.travis.yml b/vendor/github.com/mohae/deepcopy/.travis.yml
deleted file mode 100644
index fd47a8cf..00000000
--- a/vendor/github.com/mohae/deepcopy/.travis.yml
+++ /dev/null
@@ -1,11 +0,0 @@
-language: go
-
-go:
- - tip
-
-matrix:
- allow_failures:
- - go: tip
-
-script:
- - go test ./...
diff --git a/vendor/github.com/mohae/deepcopy/LICENSE b/vendor/github.com/mohae/deepcopy/LICENSE
deleted file mode 100644
index 419673f0..00000000
--- a/vendor/github.com/mohae/deepcopy/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2014 Joel
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/mohae/deepcopy/README.md b/vendor/github.com/mohae/deepcopy/README.md
deleted file mode 100644
index f8184188..00000000
--- a/vendor/github.com/mohae/deepcopy/README.md
+++ /dev/null
@@ -1,8 +0,0 @@
-deepCopy
-========
-[](https://godoc.org/github.com/mohae/deepcopy)[](https://travis-ci.org/mohae/deepcopy)
-
-DeepCopy makes deep copies of things: unexported field values are not copied.
-
-## Usage
- cpy := deepcopy.Copy(orig)
diff --git a/vendor/github.com/mohae/deepcopy/deepcopy.go b/vendor/github.com/mohae/deepcopy/deepcopy.go
deleted file mode 100644
index ba763ad0..00000000
--- a/vendor/github.com/mohae/deepcopy/deepcopy.go
+++ /dev/null
@@ -1,125 +0,0 @@
-// deepcopy makes deep copies of things. A standard copy will copy the
-// pointers: deep copy copies the values pointed to. Unexported field
-// values are not copied.
-//
-// Copyright (c)2014-2016, Joel Scoble (github.com/mohae), all rights reserved.
-// License: MIT, for more details check the included LICENSE file.
-package deepcopy
-
-import (
- "reflect"
- "time"
-)
-
-// Interface for delegating copy process to type
-type Interface interface {
- DeepCopy() interface{}
-}
-
-// Iface is an alias to Copy; this exists for backwards compatibility reasons.
-func Iface(iface interface{}) interface{} {
- return Copy(iface)
-}
-
-// Copy creates a deep copy of whatever is passed to it and returns the copy
-// in an interface{}. The returned value will need to be asserted to the
-// correct type.
-func Copy(src interface{}) interface{} {
- if src == nil {
- return nil
- }
-
- // Make the interface a reflect.Value
- original := reflect.ValueOf(src)
-
- // Make a copy of the same type as the original.
- cpy := reflect.New(original.Type()).Elem()
-
- // Recursively copy the original.
- copyRecursive(original, cpy)
-
- // Return the copy as an interface.
- return cpy.Interface()
-}
-
-// copyRecursive does the actual copying of the interface. It currently has
-// limited support for what it can handle. Add as needed.
-func copyRecursive(original, cpy reflect.Value) {
- // check for implement deepcopy.Interface
- if original.CanInterface() {
- if copier, ok := original.Interface().(Interface); ok {
- cpy.Set(reflect.ValueOf(copier.DeepCopy()))
- return
- }
- }
-
- // handle according to original's Kind
- switch original.Kind() {
- case reflect.Ptr:
- // Get the actual value being pointed to.
- originalValue := original.Elem()
-
- // if it isn't valid, return.
- if !originalValue.IsValid() {
- return
- }
- cpy.Set(reflect.New(originalValue.Type()))
- copyRecursive(originalValue, cpy.Elem())
-
- case reflect.Interface:
- // If this is a nil, don't do anything
- if original.IsNil() {
- return
- }
- // Get the value for the interface, not the pointer.
- originalValue := original.Elem()
-
- // Get the value by calling Elem().
- copyValue := reflect.New(originalValue.Type()).Elem()
- copyRecursive(originalValue, copyValue)
- cpy.Set(copyValue)
-
- case reflect.Struct:
- t, ok := original.Interface().(time.Time)
- if ok {
- cpy.Set(reflect.ValueOf(t))
- return
- }
- // Go through each field of the struct and copy it.
- for i := 0; i < original.NumField(); i++ {
- // The Type's StructField for a given field is checked to see if StructField.PkgPath
- // is set to determine if the field is exported or not because CanSet() returns false
- // for settable fields. I'm not sure why. -mohae
- if original.Type().Field(i).PkgPath != "" {
- continue
- }
- copyRecursive(original.Field(i), cpy.Field(i))
- }
-
- case reflect.Slice:
- if original.IsNil() {
- return
- }
- // Make a new slice and copy each element.
- cpy.Set(reflect.MakeSlice(original.Type(), original.Len(), original.Cap()))
- for i := 0; i < original.Len(); i++ {
- copyRecursive(original.Index(i), cpy.Index(i))
- }
-
- case reflect.Map:
- if original.IsNil() {
- return
- }
- cpy.Set(reflect.MakeMap(original.Type()))
- for _, key := range original.MapKeys() {
- originalValue := original.MapIndex(key)
- copyValue := reflect.New(originalValue.Type()).Elem()
- copyRecursive(originalValue, copyValue)
- copyKey := Copy(key.Interface())
- cpy.SetMapIndex(reflect.ValueOf(copyKey), copyValue)
- }
-
- default:
- cpy.Set(original)
- }
-}
diff --git a/vendor/github.com/olekukonko/tablewriter/.gitignore b/vendor/github.com/olekukonko/tablewriter/.gitignore
deleted file mode 100644
index b66cec63..00000000
--- a/vendor/github.com/olekukonko/tablewriter/.gitignore
+++ /dev/null
@@ -1,15 +0,0 @@
-# Created by .ignore support plugin (hsz.mobi)
-### Go template
-# Binaries for programs and plugins
-*.exe
-*.exe~
-*.dll
-*.so
-*.dylib
-
-# Test binary, build with `go test -c`
-*.test
-
-# Output of the go coverage tool, specifically when used with LiteIDE
-*.out
-
diff --git a/vendor/github.com/olekukonko/tablewriter/.travis.yml b/vendor/github.com/olekukonko/tablewriter/.travis.yml
deleted file mode 100644
index 366d48a3..00000000
--- a/vendor/github.com/olekukonko/tablewriter/.travis.yml
+++ /dev/null
@@ -1,22 +0,0 @@
-language: go
-arch:
- - ppc64le
- - amd64
-go:
- - 1.3
- - 1.4
- - 1.5
- - 1.6
- - 1.7
- - 1.8
- - 1.9
- - "1.10"
- - tip
-jobs:
- exclude :
- - arch : ppc64le
- go :
- - 1.3
- - arch : ppc64le
- go :
- - 1.4
diff --git a/vendor/github.com/olekukonko/tablewriter/LICENSE.md b/vendor/github.com/olekukonko/tablewriter/LICENSE.md
deleted file mode 100644
index a0769b5c..00000000
--- a/vendor/github.com/olekukonko/tablewriter/LICENSE.md
+++ /dev/null
@@ -1,19 +0,0 @@
-Copyright (C) 2014 by Oleku Konko
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
diff --git a/vendor/github.com/olekukonko/tablewriter/README.md b/vendor/github.com/olekukonko/tablewriter/README.md
deleted file mode 100644
index f06530d7..00000000
--- a/vendor/github.com/olekukonko/tablewriter/README.md
+++ /dev/null
@@ -1,431 +0,0 @@
-ASCII Table Writer
-=========
-
-[](https://travis-ci.org/olekukonko/tablewriter)
-[](https://sourcegraph.com/github.com/olekukonko/tablewriter)
-[](https://godoc.org/github.com/olekukonko/tablewriter)
-
-Generate ASCII table on the fly ... Installation is simple as
-
- go get github.com/olekukonko/tablewriter
-
-
-#### Features
-- Automatic Padding
-- Support Multiple Lines
-- Supports Alignment
-- Support Custom Separators
-- Automatic Alignment of numbers & percentage
-- Write directly to http , file etc via `io.Writer`
-- Read directly from CSV file
-- Optional row line via `SetRowLine`
-- Normalise table header
-- Make CSV Headers optional
-- Enable or disable table border
-- Set custom footer support
-- Optional identical cells merging
-- Set custom caption
-- Optional reflowing of paragraphs in multi-line cells.
-
-#### Example 1 - Basic
-```go
-data := [][]string{
- []string{"A", "The Good", "500"},
- []string{"B", "The Very very Bad Man", "288"},
- []string{"C", "The Ugly", "120"},
- []string{"D", "The Gopher", "800"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Name", "Sign", "Rating"})
-
-for _, v := range data {
- table.Append(v)
-}
-table.Render() // Send output
-```
-
-##### Output 1
-```
-+------+-----------------------+--------+
-| NAME | SIGN | RATING |
-+------+-----------------------+--------+
-| A | The Good | 500 |
-| B | The Very very Bad Man | 288 |
-| C | The Ugly | 120 |
-| D | The Gopher | 800 |
-+------+-----------------------+--------+
-```
-
-#### Example 2 - Without Border / Footer / Bulk Append
-```go
-data := [][]string{
- []string{"1/1/2014", "Domain name", "2233", "$10.98"},
- []string{"1/1/2014", "January Hosting", "2233", "$54.95"},
- []string{"1/4/2014", "February Hosting", "2233", "$51.00"},
- []string{"1/4/2014", "February Extra Bandwidth", "2233", "$30.00"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Date", "Description", "CV2", "Amount"})
-table.SetFooter([]string{"", "", "Total", "$146.93"}) // Add Footer
-table.SetBorder(false) // Set Border to false
-table.AppendBulk(data) // Add Bulk Data
-table.Render()
-```
-
-##### Output 2
-```
-
- DATE | DESCRIPTION | CV2 | AMOUNT
------------+--------------------------+-------+----------
- 1/1/2014 | Domain name | 2233 | $10.98
- 1/1/2014 | January Hosting | 2233 | $54.95
- 1/4/2014 | February Hosting | 2233 | $51.00
- 1/4/2014 | February Extra Bandwidth | 2233 | $30.00
------------+--------------------------+-------+----------
- TOTAL | $146 93
- --------+----------
-
-```
-
-
-#### Example 3 - CSV
-```go
-table, _ := tablewriter.NewCSV(os.Stdout, "testdata/test_info.csv", true)
-table.SetAlignment(tablewriter.ALIGN_LEFT) // Set Alignment
-table.Render()
-```
-
-##### Output 3
-```
-+----------+--------------+------+-----+---------+----------------+
-| FIELD | TYPE | NULL | KEY | DEFAULT | EXTRA |
-+----------+--------------+------+-----+---------+----------------+
-| user_id | smallint(5) | NO | PRI | NULL | auto_increment |
-| username | varchar(10) | NO | | NULL | |
-| password | varchar(100) | NO | | NULL | |
-+----------+--------------+------+-----+---------+----------------+
-```
-
-#### Example 4 - Custom Separator
-```go
-table, _ := tablewriter.NewCSV(os.Stdout, "testdata/test.csv", true)
-table.SetRowLine(true) // Enable row line
-
-// Change table lines
-table.SetCenterSeparator("*")
-table.SetColumnSeparator("╪")
-table.SetRowSeparator("-")
-
-table.SetAlignment(tablewriter.ALIGN_LEFT)
-table.Render()
-```
-
-##### Output 4
-```
-*------------*-----------*---------*
-╪ FIRST NAME ╪ LAST NAME ╪ SSN ╪
-*------------*-----------*---------*
-╪ John ╪ Barry ╪ 123456 ╪
-*------------*-----------*---------*
-╪ Kathy ╪ Smith ╪ 687987 ╪
-*------------*-----------*---------*
-╪ Bob ╪ McCornick ╪ 3979870 ╪
-*------------*-----------*---------*
-```
-
-#### Example 5 - Markdown Format
-```go
-data := [][]string{
- []string{"1/1/2014", "Domain name", "2233", "$10.98"},
- []string{"1/1/2014", "January Hosting", "2233", "$54.95"},
- []string{"1/4/2014", "February Hosting", "2233", "$51.00"},
- []string{"1/4/2014", "February Extra Bandwidth", "2233", "$30.00"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Date", "Description", "CV2", "Amount"})
-table.SetBorders(tablewriter.Border{Left: true, Top: false, Right: true, Bottom: false})
-table.SetCenterSeparator("|")
-table.AppendBulk(data) // Add Bulk Data
-table.Render()
-```
-
-##### Output 5
-```
-| DATE | DESCRIPTION | CV2 | AMOUNT |
-|----------|--------------------------|------|--------|
-| 1/1/2014 | Domain name | 2233 | $10.98 |
-| 1/1/2014 | January Hosting | 2233 | $54.95 |
-| 1/4/2014 | February Hosting | 2233 | $51.00 |
-| 1/4/2014 | February Extra Bandwidth | 2233 | $30.00 |
-```
-
-#### Example 6 - Identical cells merging
-```go
-data := [][]string{
- []string{"1/1/2014", "Domain name", "1234", "$10.98"},
- []string{"1/1/2014", "January Hosting", "2345", "$54.95"},
- []string{"1/4/2014", "February Hosting", "3456", "$51.00"},
- []string{"1/4/2014", "February Extra Bandwidth", "4567", "$30.00"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Date", "Description", "CV2", "Amount"})
-table.SetFooter([]string{"", "", "Total", "$146.93"})
-table.SetAutoMergeCells(true)
-table.SetRowLine(true)
-table.AppendBulk(data)
-table.Render()
-```
-
-##### Output 6
-```
-+----------+--------------------------+-------+---------+
-| DATE | DESCRIPTION | CV2 | AMOUNT |
-+----------+--------------------------+-------+---------+
-| 1/1/2014 | Domain name | 1234 | $10.98 |
-+ +--------------------------+-------+---------+
-| | January Hosting | 2345 | $54.95 |
-+----------+--------------------------+-------+---------+
-| 1/4/2014 | February Hosting | 3456 | $51.00 |
-+ +--------------------------+-------+---------+
-| | February Extra Bandwidth | 4567 | $30.00 |
-+----------+--------------------------+-------+---------+
-| TOTAL | $146 93 |
-+----------+--------------------------+-------+---------+
-```
-
-#### Example 7 - Identical cells merging (specify the column index to merge)
-```go
-data := [][]string{
- []string{"1/1/2014", "Domain name", "1234", "$10.98"},
- []string{"1/1/2014", "January Hosting", "1234", "$10.98"},
- []string{"1/4/2014", "February Hosting", "3456", "$51.00"},
- []string{"1/4/2014", "February Extra Bandwidth", "4567", "$30.00"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Date", "Description", "CV2", "Amount"})
-table.SetFooter([]string{"", "", "Total", "$146.93"})
-table.SetAutoMergeCellsByColumnIndex([]int{2, 3})
-table.SetRowLine(true)
-table.AppendBulk(data)
-table.Render()
-```
-
-##### Output 7
-```
-+----------+--------------------------+-------+---------+
-| DATE | DESCRIPTION | CV2 | AMOUNT |
-+----------+--------------------------+-------+---------+
-| 1/1/2014 | Domain name | 1234 | $10.98 |
-+----------+--------------------------+ + +
-| 1/1/2014 | January Hosting | | |
-+----------+--------------------------+-------+---------+
-| 1/4/2014 | February Hosting | 3456 | $51.00 |
-+----------+--------------------------+-------+---------+
-| 1/4/2014 | February Extra Bandwidth | 4567 | $30.00 |
-+----------+--------------------------+-------+---------+
-| TOTAL | $146.93 |
-+----------+--------------------------+-------+---------+
-```
-
-
-#### Table with color
-```go
-data := [][]string{
- []string{"1/1/2014", "Domain name", "2233", "$10.98"},
- []string{"1/1/2014", "January Hosting", "2233", "$54.95"},
- []string{"1/4/2014", "February Hosting", "2233", "$51.00"},
- []string{"1/4/2014", "February Extra Bandwidth", "2233", "$30.00"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Date", "Description", "CV2", "Amount"})
-table.SetFooter([]string{"", "", "Total", "$146.93"}) // Add Footer
-table.SetBorder(false) // Set Border to false
-
-table.SetHeaderColor(tablewriter.Colors{tablewriter.Bold, tablewriter.BgGreenColor},
- tablewriter.Colors{tablewriter.FgHiRedColor, tablewriter.Bold, tablewriter.BgBlackColor},
- tablewriter.Colors{tablewriter.BgRedColor, tablewriter.FgWhiteColor},
- tablewriter.Colors{tablewriter.BgCyanColor, tablewriter.FgWhiteColor})
-
-table.SetColumnColor(tablewriter.Colors{tablewriter.Bold, tablewriter.FgHiBlackColor},
- tablewriter.Colors{tablewriter.Bold, tablewriter.FgHiRedColor},
- tablewriter.Colors{tablewriter.Bold, tablewriter.FgHiBlackColor},
- tablewriter.Colors{tablewriter.Bold, tablewriter.FgBlackColor})
-
-table.SetFooterColor(tablewriter.Colors{}, tablewriter.Colors{},
- tablewriter.Colors{tablewriter.Bold},
- tablewriter.Colors{tablewriter.FgHiRedColor})
-
-table.AppendBulk(data)
-table.Render()
-```
-
-#### Table with color Output
-
-
-#### Example - 8 Table Cells with Color
-
-Individual Cell Colors from `func Rich` take precedence over Column Colors
-
-```go
-data := [][]string{
- []string{"Test1Merge", "HelloCol2 - 1", "HelloCol3 - 1", "HelloCol4 - 1"},
- []string{"Test1Merge", "HelloCol2 - 2", "HelloCol3 - 2", "HelloCol4 - 2"},
- []string{"Test1Merge", "HelloCol2 - 3", "HelloCol3 - 3", "HelloCol4 - 3"},
- []string{"Test2Merge", "HelloCol2 - 4", "HelloCol3 - 4", "HelloCol4 - 4"},
- []string{"Test2Merge", "HelloCol2 - 5", "HelloCol3 - 5", "HelloCol4 - 5"},
- []string{"Test2Merge", "HelloCol2 - 6", "HelloCol3 - 6", "HelloCol4 - 6"},
- []string{"Test2Merge", "HelloCol2 - 7", "HelloCol3 - 7", "HelloCol4 - 7"},
- []string{"Test3Merge", "HelloCol2 - 8", "HelloCol3 - 8", "HelloCol4 - 8"},
- []string{"Test3Merge", "HelloCol2 - 9", "HelloCol3 - 9", "HelloCol4 - 9"},
- []string{"Test3Merge", "HelloCol2 - 10", "HelloCol3 -10", "HelloCol4 - 10"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Col1", "Col2", "Col3", "Col4"})
-table.SetFooter([]string{"", "", "Footer3", "Footer4"})
-table.SetBorder(false)
-
-table.SetHeaderColor(tablewriter.Colors{tablewriter.Bold, tablewriter.BgGreenColor},
- tablewriter.Colors{tablewriter.FgHiRedColor, tablewriter.Bold, tablewriter.BgBlackColor},
- tablewriter.Colors{tablewriter.BgRedColor, tablewriter.FgWhiteColor},
- tablewriter.Colors{tablewriter.BgCyanColor, tablewriter.FgWhiteColor})
-
-table.SetColumnColor(tablewriter.Colors{tablewriter.Bold, tablewriter.FgHiBlackColor},
- tablewriter.Colors{tablewriter.Bold, tablewriter.FgHiRedColor},
- tablewriter.Colors{tablewriter.Bold, tablewriter.FgHiBlackColor},
- tablewriter.Colors{tablewriter.Bold, tablewriter.FgBlackColor})
-
-table.SetFooterColor(tablewriter.Colors{}, tablewriter.Colors{},
- tablewriter.Colors{tablewriter.Bold},
- tablewriter.Colors{tablewriter.FgHiRedColor})
-
-colorData1 := []string{"TestCOLOR1Merge", "HelloCol2 - COLOR1", "HelloCol3 - COLOR1", "HelloCol4 - COLOR1"}
-colorData2 := []string{"TestCOLOR2Merge", "HelloCol2 - COLOR2", "HelloCol3 - COLOR2", "HelloCol4 - COLOR2"}
-
-for i, row := range data {
- if i == 4 {
- table.Rich(colorData1, []tablewriter.Colors{tablewriter.Colors{}, tablewriter.Colors{tablewriter.Normal, tablewriter.FgCyanColor}, tablewriter.Colors{tablewriter.Bold, tablewriter.FgWhiteColor}, tablewriter.Colors{}})
- table.Rich(colorData2, []tablewriter.Colors{tablewriter.Colors{tablewriter.Normal, tablewriter.FgMagentaColor}, tablewriter.Colors{}, tablewriter.Colors{tablewriter.Bold, tablewriter.BgRedColor}, tablewriter.Colors{tablewriter.FgHiGreenColor, tablewriter.Italic, tablewriter.BgHiCyanColor}})
- }
- table.Append(row)
-}
-
-table.SetAutoMergeCells(true)
-table.Render()
-
-```
-
-##### Table cells with color Output
-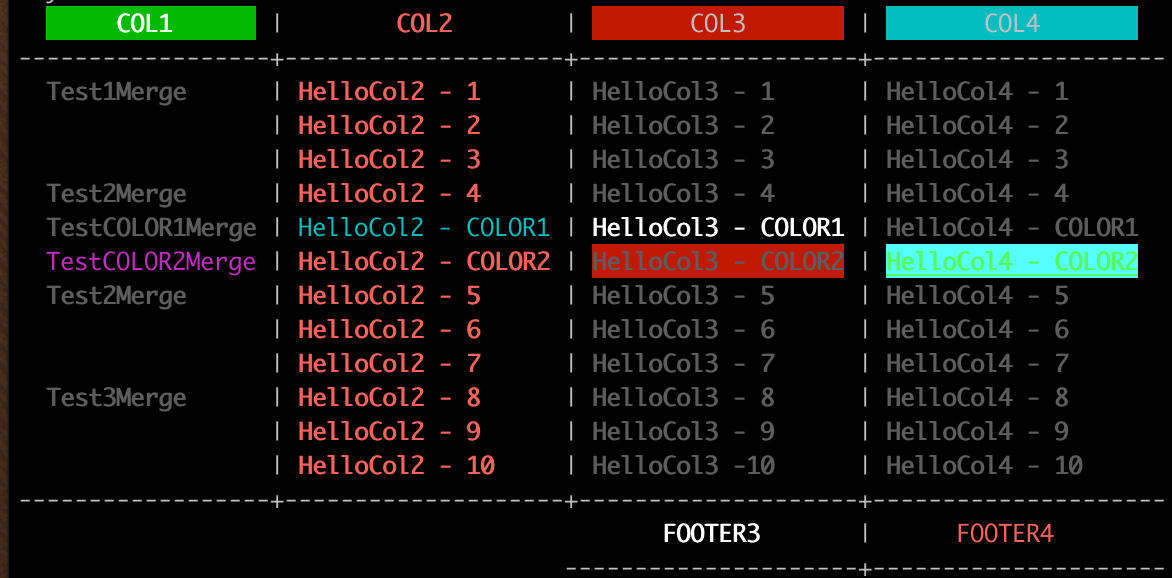
-
-#### Example 9 - Set table caption
-```go
-data := [][]string{
- []string{"A", "The Good", "500"},
- []string{"B", "The Very very Bad Man", "288"},
- []string{"C", "The Ugly", "120"},
- []string{"D", "The Gopher", "800"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Name", "Sign", "Rating"})
-table.SetCaption(true, "Movie ratings.")
-
-for _, v := range data {
- table.Append(v)
-}
-table.Render() // Send output
-```
-
-Note: Caption text will wrap with total width of rendered table.
-
-##### Output 9
-```
-+------+-----------------------+--------+
-| NAME | SIGN | RATING |
-+------+-----------------------+--------+
-| A | The Good | 500 |
-| B | The Very very Bad Man | 288 |
-| C | The Ugly | 120 |
-| D | The Gopher | 800 |
-+------+-----------------------+--------+
-Movie ratings.
-```
-
-#### Example 10 - Set NoWhiteSpace and TablePadding option
-```go
-data := [][]string{
- {"node1.example.com", "Ready", "compute", "1.11"},
- {"node2.example.com", "Ready", "compute", "1.11"},
- {"node3.example.com", "Ready", "compute", "1.11"},
- {"node4.example.com", "NotReady", "compute", "1.11"},
-}
-
-table := tablewriter.NewWriter(os.Stdout)
-table.SetHeader([]string{"Name", "Status", "Role", "Version"})
-table.SetAutoWrapText(false)
-table.SetAutoFormatHeaders(true)
-table.SetHeaderAlignment(ALIGN_LEFT)
-table.SetAlignment(ALIGN_LEFT)
-table.SetCenterSeparator("")
-table.SetColumnSeparator("")
-table.SetRowSeparator("")
-table.SetHeaderLine(false)
-table.SetBorder(false)
-table.SetTablePadding("\t") // pad with tabs
-table.SetNoWhiteSpace(true)
-table.AppendBulk(data) // Add Bulk Data
-table.Render()
-```
-
-##### Output 10
-```
-NAME STATUS ROLE VERSION
-node1.example.com Ready compute 1.11
-node2.example.com Ready compute 1.11
-node3.example.com Ready compute 1.11
-node4.example.com NotReady compute 1.11
-```
-
-#### Render table into a string
-
-Instead of rendering the table to `io.Stdout` you can also render it into a string. Go 1.10 introduced the `strings.Builder` type which implements the `io.Writer` interface and can therefore be used for this task. Example:
-
-```go
-package main
-
-import (
- "strings"
- "fmt"
-
- "github.com/olekukonko/tablewriter"
-)
-
-func main() {
- tableString := &strings.Builder{}
- table := tablewriter.NewWriter(tableString)
-
- /*
- * Code to fill the table
- */
-
- table.Render()
-
- fmt.Println(tableString.String())
-}
-```
-
-#### TODO
-- ~~Import Directly from CSV~~ - `done`
-- ~~Support for `SetFooter`~~ - `done`
-- ~~Support for `SetBorder`~~ - `done`
-- ~~Support table with uneven rows~~ - `done`
-- ~~Support custom alignment~~
-- General Improvement & Optimisation
-- `NewHTML` Parse table from HTML
diff --git a/vendor/github.com/olekukonko/tablewriter/csv.go b/vendor/github.com/olekukonko/tablewriter/csv.go
deleted file mode 100644
index 98878303..00000000
--- a/vendor/github.com/olekukonko/tablewriter/csv.go
+++ /dev/null
@@ -1,52 +0,0 @@
-// Copyright 2014 Oleku Konko All rights reserved.
-// Use of this source code is governed by a MIT
-// license that can be found in the LICENSE file.
-
-// This module is a Table Writer API for the Go Programming Language.
-// The protocols were written in pure Go and works on windows and unix systems
-
-package tablewriter
-
-import (
- "encoding/csv"
- "io"
- "os"
-)
-
-// Start A new table by importing from a CSV file
-// Takes io.Writer and csv File name
-func NewCSV(writer io.Writer, fileName string, hasHeader bool) (*Table, error) {
- file, err := os.Open(fileName)
- if err != nil {
- return &Table{}, err
- }
- defer file.Close()
- csvReader := csv.NewReader(file)
- t, err := NewCSVReader(writer, csvReader, hasHeader)
- return t, err
-}
-
-// Start a New Table Writer with csv.Reader
-// This enables customisation such as reader.Comma = ';'
-// See http://golang.org/src/pkg/encoding/csv/reader.go?s=3213:3671#L94
-func NewCSVReader(writer io.Writer, csvReader *csv.Reader, hasHeader bool) (*Table, error) {
- t := NewWriter(writer)
- if hasHeader {
- // Read the first row
- headers, err := csvReader.Read()
- if err != nil {
- return &Table{}, err
- }
- t.SetHeader(headers)
- }
- for {
- record, err := csvReader.Read()
- if err == io.EOF {
- break
- } else if err != nil {
- return &Table{}, err
- }
- t.Append(record)
- }
- return t, nil
-}
diff --git a/vendor/github.com/olekukonko/tablewriter/table.go b/vendor/github.com/olekukonko/tablewriter/table.go
deleted file mode 100644
index f913149c..00000000
--- a/vendor/github.com/olekukonko/tablewriter/table.go
+++ /dev/null
@@ -1,967 +0,0 @@
-// Copyright 2014 Oleku Konko All rights reserved.
-// Use of this source code is governed by a MIT
-// license that can be found in the LICENSE file.
-
-// This module is a Table Writer API for the Go Programming Language.
-// The protocols were written in pure Go and works on windows and unix systems
-
-// Create & Generate text based table
-package tablewriter
-
-import (
- "bytes"
- "fmt"
- "io"
- "regexp"
- "strings"
-)
-
-const (
- MAX_ROW_WIDTH = 30
-)
-
-const (
- CENTER = "+"
- ROW = "-"
- COLUMN = "|"
- SPACE = " "
- NEWLINE = "\n"
-)
-
-const (
- ALIGN_DEFAULT = iota
- ALIGN_CENTER
- ALIGN_RIGHT
- ALIGN_LEFT
-)
-
-var (
- decimal = regexp.MustCompile(`^-?(?:\d{1,3}(?:,\d{3})*|\d+)(?:\.\d+)?$`)
- percent = regexp.MustCompile(`^-?\d+\.?\d*$%$`)
-)
-
-type Border struct {
- Left bool
- Right bool
- Top bool
- Bottom bool
-}
-
-type Table struct {
- out io.Writer
- rows [][]string
- lines [][][]string
- cs map[int]int
- rs map[int]int
- headers [][]string
- footers [][]string
- caption bool
- captionText string
- autoFmt bool
- autoWrap bool
- reflowText bool
- mW int
- pCenter string
- pRow string
- pColumn string
- tColumn int
- tRow int
- hAlign int
- fAlign int
- align int
- newLine string
- rowLine bool
- autoMergeCells bool
- columnsToAutoMergeCells map[int]bool
- noWhiteSpace bool
- tablePadding string
- hdrLine bool
- borders Border
- colSize int
- headerParams []string
- columnsParams []string
- footerParams []string
- columnsAlign []int
-}
-
-// Start New Table
-// Take io.Writer Directly
-func NewWriter(writer io.Writer) *Table {
- t := &Table{
- out: writer,
- rows: [][]string{},
- lines: [][][]string{},
- cs: make(map[int]int),
- rs: make(map[int]int),
- headers: [][]string{},
- footers: [][]string{},
- caption: false,
- captionText: "Table caption.",
- autoFmt: true,
- autoWrap: true,
- reflowText: true,
- mW: MAX_ROW_WIDTH,
- pCenter: CENTER,
- pRow: ROW,
- pColumn: COLUMN,
- tColumn: -1,
- tRow: -1,
- hAlign: ALIGN_DEFAULT,
- fAlign: ALIGN_DEFAULT,
- align: ALIGN_DEFAULT,
- newLine: NEWLINE,
- rowLine: false,
- hdrLine: true,
- borders: Border{Left: true, Right: true, Bottom: true, Top: true},
- colSize: -1,
- headerParams: []string{},
- columnsParams: []string{},
- footerParams: []string{},
- columnsAlign: []int{}}
- return t
-}
-
-// Render table output
-func (t *Table) Render() {
- if t.borders.Top {
- t.printLine(true)
- }
- t.printHeading()
- if t.autoMergeCells {
- t.printRowsMergeCells()
- } else {
- t.printRows()
- }
- if !t.rowLine && t.borders.Bottom {
- t.printLine(true)
- }
- t.printFooter()
-
- if t.caption {
- t.printCaption()
- }
-}
-
-const (
- headerRowIdx = -1
- footerRowIdx = -2
-)
-
-// Set table header
-func (t *Table) SetHeader(keys []string) {
- t.colSize = len(keys)
- for i, v := range keys {
- lines := t.parseDimension(v, i, headerRowIdx)
- t.headers = append(t.headers, lines)
- }
-}
-
-// Set table Footer
-func (t *Table) SetFooter(keys []string) {
- //t.colSize = len(keys)
- for i, v := range keys {
- lines := t.parseDimension(v, i, footerRowIdx)
- t.footers = append(t.footers, lines)
- }
-}
-
-// Set table Caption
-func (t *Table) SetCaption(caption bool, captionText ...string) {
- t.caption = caption
- if len(captionText) == 1 {
- t.captionText = captionText[0]
- }
-}
-
-// Turn header autoformatting on/off. Default is on (true).
-func (t *Table) SetAutoFormatHeaders(auto bool) {
- t.autoFmt = auto
-}
-
-// Turn automatic multiline text adjustment on/off. Default is on (true).
-func (t *Table) SetAutoWrapText(auto bool) {
- t.autoWrap = auto
-}
-
-// Turn automatic reflowing of multiline text when rewrapping. Default is on (true).
-func (t *Table) SetReflowDuringAutoWrap(auto bool) {
- t.reflowText = auto
-}
-
-// Set the Default column width
-func (t *Table) SetColWidth(width int) {
- t.mW = width
-}
-
-// Set the minimal width for a column
-func (t *Table) SetColMinWidth(column int, width int) {
- t.cs[column] = width
-}
-
-// Set the Column Separator
-func (t *Table) SetColumnSeparator(sep string) {
- t.pColumn = sep
-}
-
-// Set the Row Separator
-func (t *Table) SetRowSeparator(sep string) {
- t.pRow = sep
-}
-
-// Set the center Separator
-func (t *Table) SetCenterSeparator(sep string) {
- t.pCenter = sep
-}
-
-// Set Header Alignment
-func (t *Table) SetHeaderAlignment(hAlign int) {
- t.hAlign = hAlign
-}
-
-// Set Footer Alignment
-func (t *Table) SetFooterAlignment(fAlign int) {
- t.fAlign = fAlign
-}
-
-// Set Table Alignment
-func (t *Table) SetAlignment(align int) {
- t.align = align
-}
-
-// Set No White Space
-func (t *Table) SetNoWhiteSpace(allow bool) {
- t.noWhiteSpace = allow
-}
-
-// Set Table Padding
-func (t *Table) SetTablePadding(padding string) {
- t.tablePadding = padding
-}
-
-func (t *Table) SetColumnAlignment(keys []int) {
- for _, v := range keys {
- switch v {
- case ALIGN_CENTER:
- break
- case ALIGN_LEFT:
- break
- case ALIGN_RIGHT:
- break
- default:
- v = ALIGN_DEFAULT
- }
- t.columnsAlign = append(t.columnsAlign, v)
- }
-}
-
-// Set New Line
-func (t *Table) SetNewLine(nl string) {
- t.newLine = nl
-}
-
-// Set Header Line
-// This would enable / disable a line after the header
-func (t *Table) SetHeaderLine(line bool) {
- t.hdrLine = line
-}
-
-// Set Row Line
-// This would enable / disable a line on each row of the table
-func (t *Table) SetRowLine(line bool) {
- t.rowLine = line
-}
-
-// Set Auto Merge Cells
-// This would enable / disable the merge of cells with identical values
-func (t *Table) SetAutoMergeCells(auto bool) {
- t.autoMergeCells = auto
-}
-
-// Set Auto Merge Cells By Column Index
-// This would enable / disable the merge of cells with identical values for specific columns
-// If cols is empty, it is the same as `SetAutoMergeCells(true)`.
-func (t *Table) SetAutoMergeCellsByColumnIndex(cols []int) {
- t.autoMergeCells = true
-
- if len(cols) > 0 {
- m := make(map[int]bool)
- for _, col := range cols {
- m[col] = true
- }
- t.columnsToAutoMergeCells = m
- }
-}
-
-// Set Table Border
-// This would enable / disable line around the table
-func (t *Table) SetBorder(border bool) {
- t.SetBorders(Border{border, border, border, border})
-}
-
-func (t *Table) SetBorders(border Border) {
- t.borders = border
-}
-
-// Append row to table
-func (t *Table) Append(row []string) {
- rowSize := len(t.headers)
- if rowSize > t.colSize {
- t.colSize = rowSize
- }
-
- n := len(t.lines)
- line := [][]string{}
- for i, v := range row {
-
- // Detect string width
- // Detect String height
- // Break strings into words
- out := t.parseDimension(v, i, n)
-
- // Append broken words
- line = append(line, out)
- }
- t.lines = append(t.lines, line)
-}
-
-// Append row to table with color attributes
-func (t *Table) Rich(row []string, colors []Colors) {
- rowSize := len(t.headers)
- if rowSize > t.colSize {
- t.colSize = rowSize
- }
-
- n := len(t.lines)
- line := [][]string{}
- for i, v := range row {
-
- // Detect string width
- // Detect String height
- // Break strings into words
- out := t.parseDimension(v, i, n)
-
- if len(colors) > i {
- color := colors[i]
- out[0] = format(out[0], color)
- }
-
- // Append broken words
- line = append(line, out)
- }
- t.lines = append(t.lines, line)
-}
-
-// Allow Support for Bulk Append
-// Eliminates repeated for loops
-func (t *Table) AppendBulk(rows [][]string) {
- for _, row := range rows {
- t.Append(row)
- }
-}
-
-// NumLines to get the number of lines
-func (t *Table) NumLines() int {
- return len(t.lines)
-}
-
-// Clear rows
-func (t *Table) ClearRows() {
- t.lines = [][][]string{}
-}
-
-// Clear footer
-func (t *Table) ClearFooter() {
- t.footers = [][]string{}
-}
-
-// Center based on position and border.
-func (t *Table) center(i int) string {
- if i == -1 && !t.borders.Left {
- return t.pRow
- }
-
- if i == len(t.cs)-1 && !t.borders.Right {
- return t.pRow
- }
-
- return t.pCenter
-}
-
-// Print line based on row width
-func (t *Table) printLine(nl bool) {
- fmt.Fprint(t.out, t.center(-1))
- for i := 0; i < len(t.cs); i++ {
- v := t.cs[i]
- fmt.Fprintf(t.out, "%s%s%s%s",
- t.pRow,
- strings.Repeat(string(t.pRow), v),
- t.pRow,
- t.center(i))
- }
- if nl {
- fmt.Fprint(t.out, t.newLine)
- }
-}
-
-// Print line based on row width with our without cell separator
-func (t *Table) printLineOptionalCellSeparators(nl bool, displayCellSeparator []bool) {
- fmt.Fprint(t.out, t.pCenter)
- for i := 0; i < len(t.cs); i++ {
- v := t.cs[i]
- if i > len(displayCellSeparator) || displayCellSeparator[i] {
- // Display the cell separator
- fmt.Fprintf(t.out, "%s%s%s%s",
- t.pRow,
- strings.Repeat(string(t.pRow), v),
- t.pRow,
- t.pCenter)
- } else {
- // Don't display the cell separator for this cell
- fmt.Fprintf(t.out, "%s%s",
- strings.Repeat(" ", v+2),
- t.pCenter)
- }
- }
- if nl {
- fmt.Fprint(t.out, t.newLine)
- }
-}
-
-// Return the PadRight function if align is left, PadLeft if align is right,
-// and Pad by default
-func pad(align int) func(string, string, int) string {
- padFunc := Pad
- switch align {
- case ALIGN_LEFT:
- padFunc = PadRight
- case ALIGN_RIGHT:
- padFunc = PadLeft
- }
- return padFunc
-}
-
-// Print heading information
-func (t *Table) printHeading() {
- // Check if headers is available
- if len(t.headers) < 1 {
- return
- }
-
- // Identify last column
- end := len(t.cs) - 1
-
- // Get pad function
- padFunc := pad(t.hAlign)
-
- // Checking for ANSI escape sequences for header
- is_esc_seq := false
- if len(t.headerParams) > 0 {
- is_esc_seq = true
- }
-
- // Maximum height.
- max := t.rs[headerRowIdx]
-
- // Print Heading
- for x := 0; x < max; x++ {
- // Check if border is set
- // Replace with space if not set
- if !t.noWhiteSpace {
- fmt.Fprint(t.out, ConditionString(t.borders.Left, t.pColumn, SPACE))
- }
-
- for y := 0; y <= end; y++ {
- v := t.cs[y]
- h := ""
-
- if y < len(t.headers) && x < len(t.headers[y]) {
- h = t.headers[y][x]
- }
- if t.autoFmt {
- h = Title(h)
- }
- pad := ConditionString((y == end && !t.borders.Left), SPACE, t.pColumn)
- if t.noWhiteSpace {
- pad = ConditionString((y == end && !t.borders.Left), SPACE, t.tablePadding)
- }
- if is_esc_seq {
- if !t.noWhiteSpace {
- fmt.Fprintf(t.out, " %s %s",
- format(padFunc(h, SPACE, v),
- t.headerParams[y]), pad)
- } else {
- fmt.Fprintf(t.out, "%s %s",
- format(padFunc(h, SPACE, v),
- t.headerParams[y]), pad)
- }
- } else {
- if !t.noWhiteSpace {
- fmt.Fprintf(t.out, " %s %s",
- padFunc(h, SPACE, v),
- pad)
- } else {
- // the spaces between breaks the kube formatting
- fmt.Fprintf(t.out, "%s%s",
- padFunc(h, SPACE, v),
- pad)
- }
- }
- }
- // Next line
- fmt.Fprint(t.out, t.newLine)
- }
- if t.hdrLine {
- t.printLine(true)
- }
-}
-
-// Print heading information
-func (t *Table) printFooter() {
- // Check if headers is available
- if len(t.footers) < 1 {
- return
- }
-
- // Only print line if border is not set
- if !t.borders.Bottom {
- t.printLine(true)
- }
-
- // Identify last column
- end := len(t.cs) - 1
-
- // Get pad function
- padFunc := pad(t.fAlign)
-
- // Checking for ANSI escape sequences for header
- is_esc_seq := false
- if len(t.footerParams) > 0 {
- is_esc_seq = true
- }
-
- // Maximum height.
- max := t.rs[footerRowIdx]
-
- // Print Footer
- erasePad := make([]bool, len(t.footers))
- for x := 0; x < max; x++ {
- // Check if border is set
- // Replace with space if not set
- fmt.Fprint(t.out, ConditionString(t.borders.Bottom, t.pColumn, SPACE))
-
- for y := 0; y <= end; y++ {
- v := t.cs[y]
- f := ""
- if y < len(t.footers) && x < len(t.footers[y]) {
- f = t.footers[y][x]
- }
- if t.autoFmt {
- f = Title(f)
- }
- pad := ConditionString((y == end && !t.borders.Top), SPACE, t.pColumn)
-
- if erasePad[y] || (x == 0 && len(f) == 0) {
- pad = SPACE
- erasePad[y] = true
- }
-
- if is_esc_seq {
- fmt.Fprintf(t.out, " %s %s",
- format(padFunc(f, SPACE, v),
- t.footerParams[y]), pad)
- } else {
- fmt.Fprintf(t.out, " %s %s",
- padFunc(f, SPACE, v),
- pad)
- }
-
- //fmt.Fprintf(t.out, " %s %s",
- // padFunc(f, SPACE, v),
- // pad)
- }
- // Next line
- fmt.Fprint(t.out, t.newLine)
- //t.printLine(true)
- }
-
- hasPrinted := false
-
- for i := 0; i <= end; i++ {
- v := t.cs[i]
- pad := t.pRow
- center := t.pCenter
- length := len(t.footers[i][0])
-
- if length > 0 {
- hasPrinted = true
- }
-
- // Set center to be space if length is 0
- if length == 0 && !t.borders.Right {
- center = SPACE
- }
-
- // Print first junction
- if i == 0 {
- if length > 0 && !t.borders.Left {
- center = t.pRow
- }
- fmt.Fprint(t.out, center)
- }
-
- // Pad With space of length is 0
- if length == 0 {
- pad = SPACE
- }
- // Ignore left space as it has printed before
- if hasPrinted || t.borders.Left {
- pad = t.pRow
- center = t.pCenter
- }
-
- // Change Center end position
- if center != SPACE {
- if i == end && !t.borders.Right {
- center = t.pRow
- }
- }
-
- // Change Center start position
- if center == SPACE {
- if i < end && len(t.footers[i+1][0]) != 0 {
- if !t.borders.Left {
- center = t.pRow
- } else {
- center = t.pCenter
- }
- }
- }
-
- // Print the footer
- fmt.Fprintf(t.out, "%s%s%s%s",
- pad,
- strings.Repeat(string(pad), v),
- pad,
- center)
-
- }
-
- fmt.Fprint(t.out, t.newLine)
-}
-
-// Print caption text
-func (t Table) printCaption() {
- width := t.getTableWidth()
- paragraph, _ := WrapString(t.captionText, width)
- for linecount := 0; linecount < len(paragraph); linecount++ {
- fmt.Fprintln(t.out, paragraph[linecount])
- }
-}
-
-// Calculate the total number of characters in a row
-func (t Table) getTableWidth() int {
- var chars int
- for _, v := range t.cs {
- chars += v
- }
-
- // Add chars, spaces, seperators to calculate the total width of the table.
- // ncols := t.colSize
- // spaces := ncols * 2
- // seps := ncols + 1
-
- return (chars + (3 * t.colSize) + 2)
-}
-
-func (t Table) printRows() {
- for i, lines := range t.lines {
- t.printRow(lines, i)
- }
-}
-
-func (t *Table) fillAlignment(num int) {
- if len(t.columnsAlign) < num {
- t.columnsAlign = make([]int, num)
- for i := range t.columnsAlign {
- t.columnsAlign[i] = t.align
- }
- }
-}
-
-// Print Row Information
-// Adjust column alignment based on type
-
-func (t *Table) printRow(columns [][]string, rowIdx int) {
- // Get Maximum Height
- max := t.rs[rowIdx]
- total := len(columns)
-
- // TODO Fix uneven col size
- // if total < t.colSize {
- // for n := t.colSize - total; n < t.colSize ; n++ {
- // columns = append(columns, []string{SPACE})
- // t.cs[n] = t.mW
- // }
- //}
-
- // Pad Each Height
- pads := []int{}
-
- // Checking for ANSI escape sequences for columns
- is_esc_seq := false
- if len(t.columnsParams) > 0 {
- is_esc_seq = true
- }
- t.fillAlignment(total)
-
- for i, line := range columns {
- length := len(line)
- pad := max - length
- pads = append(pads, pad)
- for n := 0; n < pad; n++ {
- columns[i] = append(columns[i], " ")
- }
- }
- //fmt.Println(max, "\n")
- for x := 0; x < max; x++ {
- for y := 0; y < total; y++ {
-
- // Check if border is set
- if !t.noWhiteSpace {
- fmt.Fprint(t.out, ConditionString((!t.borders.Left && y == 0), SPACE, t.pColumn))
- fmt.Fprintf(t.out, SPACE)
- }
-
- str := columns[y][x]
-
- // Embedding escape sequence with column value
- if is_esc_seq {
- str = format(str, t.columnsParams[y])
- }
-
- // This would print alignment
- // Default alignment would use multiple configuration
- switch t.columnsAlign[y] {
- case ALIGN_CENTER: //
- fmt.Fprintf(t.out, "%s", Pad(str, SPACE, t.cs[y]))
- case ALIGN_RIGHT:
- fmt.Fprintf(t.out, "%s", PadLeft(str, SPACE, t.cs[y]))
- case ALIGN_LEFT:
- fmt.Fprintf(t.out, "%s", PadRight(str, SPACE, t.cs[y]))
- default:
- if decimal.MatchString(strings.TrimSpace(str)) || percent.MatchString(strings.TrimSpace(str)) {
- fmt.Fprintf(t.out, "%s", PadLeft(str, SPACE, t.cs[y]))
- } else {
- fmt.Fprintf(t.out, "%s", PadRight(str, SPACE, t.cs[y]))
-
- // TODO Custom alignment per column
- //if max == 1 || pads[y] > 0 {
- // fmt.Fprintf(t.out, "%s", Pad(str, SPACE, t.cs[y]))
- //} else {
- // fmt.Fprintf(t.out, "%s", PadRight(str, SPACE, t.cs[y]))
- //}
-
- }
- }
- if !t.noWhiteSpace {
- fmt.Fprintf(t.out, SPACE)
- } else {
- fmt.Fprintf(t.out, t.tablePadding)
- }
- }
- // Check if border is set
- // Replace with space if not set
- if !t.noWhiteSpace {
- fmt.Fprint(t.out, ConditionString(t.borders.Left, t.pColumn, SPACE))
- }
- fmt.Fprint(t.out, t.newLine)
- }
-
- if t.rowLine {
- t.printLine(true)
- }
-}
-
-// Print the rows of the table and merge the cells that are identical
-func (t *Table) printRowsMergeCells() {
- var previousLine []string
- var displayCellBorder []bool
- var tmpWriter bytes.Buffer
- for i, lines := range t.lines {
- // We store the display of the current line in a tmp writer, as we need to know which border needs to be print above
- previousLine, displayCellBorder = t.printRowMergeCells(&tmpWriter, lines, i, previousLine)
- if i > 0 { //We don't need to print borders above first line
- if t.rowLine {
- t.printLineOptionalCellSeparators(true, displayCellBorder)
- }
- }
- tmpWriter.WriteTo(t.out)
- }
- //Print the end of the table
- if t.rowLine {
- t.printLine(true)
- }
-}
-
-// Print Row Information to a writer and merge identical cells.
-// Adjust column alignment based on type
-
-func (t *Table) printRowMergeCells(writer io.Writer, columns [][]string, rowIdx int, previousLine []string) ([]string, []bool) {
- // Get Maximum Height
- max := t.rs[rowIdx]
- total := len(columns)
-
- // Pad Each Height
- pads := []int{}
-
- // Checking for ANSI escape sequences for columns
- is_esc_seq := false
- if len(t.columnsParams) > 0 {
- is_esc_seq = true
- }
- for i, line := range columns {
- length := len(line)
- pad := max - length
- pads = append(pads, pad)
- for n := 0; n < pad; n++ {
- columns[i] = append(columns[i], " ")
- }
- }
-
- var displayCellBorder []bool
- t.fillAlignment(total)
- for x := 0; x < max; x++ {
- for y := 0; y < total; y++ {
-
- // Check if border is set
- fmt.Fprint(writer, ConditionString((!t.borders.Left && y == 0), SPACE, t.pColumn))
-
- fmt.Fprintf(writer, SPACE)
-
- str := columns[y][x]
-
- // Embedding escape sequence with column value
- if is_esc_seq {
- str = format(str, t.columnsParams[y])
- }
-
- if t.autoMergeCells {
- var mergeCell bool
- if t.columnsToAutoMergeCells != nil {
- // Check to see if the column index is in columnsToAutoMergeCells.
- if t.columnsToAutoMergeCells[y] {
- mergeCell = true
- }
- } else {
- // columnsToAutoMergeCells was not set.
- mergeCell = true
- }
- //Store the full line to merge mutli-lines cells
- fullLine := strings.TrimRight(strings.Join(columns[y], " "), " ")
- if len(previousLine) > y && fullLine == previousLine[y] && fullLine != "" && mergeCell {
- // If this cell is identical to the one above but not empty, we don't display the border and keep the cell empty.
- displayCellBorder = append(displayCellBorder, false)
- str = ""
- } else {
- // First line or different content, keep the content and print the cell border
- displayCellBorder = append(displayCellBorder, true)
- }
- }
-
- // This would print alignment
- // Default alignment would use multiple configuration
- switch t.columnsAlign[y] {
- case ALIGN_CENTER: //
- fmt.Fprintf(writer, "%s", Pad(str, SPACE, t.cs[y]))
- case ALIGN_RIGHT:
- fmt.Fprintf(writer, "%s", PadLeft(str, SPACE, t.cs[y]))
- case ALIGN_LEFT:
- fmt.Fprintf(writer, "%s", PadRight(str, SPACE, t.cs[y]))
- default:
- if decimal.MatchString(strings.TrimSpace(str)) || percent.MatchString(strings.TrimSpace(str)) {
- fmt.Fprintf(writer, "%s", PadLeft(str, SPACE, t.cs[y]))
- } else {
- fmt.Fprintf(writer, "%s", PadRight(str, SPACE, t.cs[y]))
- }
- }
- fmt.Fprintf(writer, SPACE)
- }
- // Check if border is set
- // Replace with space if not set
- fmt.Fprint(writer, ConditionString(t.borders.Left, t.pColumn, SPACE))
- fmt.Fprint(writer, t.newLine)
- }
-
- //The new previous line is the current one
- previousLine = make([]string, total)
- for y := 0; y < total; y++ {
- previousLine[y] = strings.TrimRight(strings.Join(columns[y], " "), " ") //Store the full line for multi-lines cells
- }
- //Returns the newly added line and wether or not a border should be displayed above.
- return previousLine, displayCellBorder
-}
-
-func (t *Table) parseDimension(str string, colKey, rowKey int) []string {
- var (
- raw []string
- maxWidth int
- )
-
- raw = getLines(str)
- maxWidth = 0
- for _, line := range raw {
- if w := DisplayWidth(line); w > maxWidth {
- maxWidth = w
- }
- }
-
- // If wrapping, ensure that all paragraphs in the cell fit in the
- // specified width.
- if t.autoWrap {
- // If there's a maximum allowed width for wrapping, use that.
- if maxWidth > t.mW {
- maxWidth = t.mW
- }
-
- // In the process of doing so, we need to recompute maxWidth. This
- // is because perhaps a word in the cell is longer than the
- // allowed maximum width in t.mW.
- newMaxWidth := maxWidth
- newRaw := make([]string, 0, len(raw))
-
- if t.reflowText {
- // Make a single paragraph of everything.
- raw = []string{strings.Join(raw, " ")}
- }
- for i, para := range raw {
- paraLines, _ := WrapString(para, maxWidth)
- for _, line := range paraLines {
- if w := DisplayWidth(line); w > newMaxWidth {
- newMaxWidth = w
- }
- }
- if i > 0 {
- newRaw = append(newRaw, " ")
- }
- newRaw = append(newRaw, paraLines...)
- }
- raw = newRaw
- maxWidth = newMaxWidth
- }
-
- // Store the new known maximum width.
- v, ok := t.cs[colKey]
- if !ok || v < maxWidth || v == 0 {
- t.cs[colKey] = maxWidth
- }
-
- // Remember the number of lines for the row printer.
- h := len(raw)
- v, ok = t.rs[rowKey]
-
- if !ok || v < h || v == 0 {
- t.rs[rowKey] = h
- }
- //fmt.Printf("Raw %+v %d\n", raw, len(raw))
- return raw
-}
diff --git a/vendor/github.com/olekukonko/tablewriter/table_with_color.go b/vendor/github.com/olekukonko/tablewriter/table_with_color.go
deleted file mode 100644
index ae7a364a..00000000
--- a/vendor/github.com/olekukonko/tablewriter/table_with_color.go
+++ /dev/null
@@ -1,136 +0,0 @@
-package tablewriter
-
-import (
- "fmt"
- "strconv"
- "strings"
-)
-
-const ESC = "\033"
-const SEP = ";"
-
-const (
- BgBlackColor int = iota + 40
- BgRedColor
- BgGreenColor
- BgYellowColor
- BgBlueColor
- BgMagentaColor
- BgCyanColor
- BgWhiteColor
-)
-
-const (
- FgBlackColor int = iota + 30
- FgRedColor
- FgGreenColor
- FgYellowColor
- FgBlueColor
- FgMagentaColor
- FgCyanColor
- FgWhiteColor
-)
-
-const (
- BgHiBlackColor int = iota + 100
- BgHiRedColor
- BgHiGreenColor
- BgHiYellowColor
- BgHiBlueColor
- BgHiMagentaColor
- BgHiCyanColor
- BgHiWhiteColor
-)
-
-const (
- FgHiBlackColor int = iota + 90
- FgHiRedColor
- FgHiGreenColor
- FgHiYellowColor
- FgHiBlueColor
- FgHiMagentaColor
- FgHiCyanColor
- FgHiWhiteColor
-)
-
-const (
- Normal = 0
- Bold = 1
- UnderlineSingle = 4
- Italic
-)
-
-type Colors []int
-
-func startFormat(seq string) string {
- return fmt.Sprintf("%s[%sm", ESC, seq)
-}
-
-func stopFormat() string {
- return fmt.Sprintf("%s[%dm", ESC, Normal)
-}
-
-// Making the SGR (Select Graphic Rendition) sequence.
-func makeSequence(codes []int) string {
- codesInString := []string{}
- for _, code := range codes {
- codesInString = append(codesInString, strconv.Itoa(code))
- }
- return strings.Join(codesInString, SEP)
-}
-
-// Adding ANSI escape sequences before and after string
-func format(s string, codes interface{}) string {
- var seq string
-
- switch v := codes.(type) {
-
- case string:
- seq = v
- case []int:
- seq = makeSequence(v)
- case Colors:
- seq = makeSequence(v)
- default:
- return s
- }
-
- if len(seq) == 0 {
- return s
- }
- return startFormat(seq) + s + stopFormat()
-}
-
-// Adding header colors (ANSI codes)
-func (t *Table) SetHeaderColor(colors ...Colors) {
- if t.colSize != len(colors) {
- panic("Number of header colors must be equal to number of headers.")
- }
- for i := 0; i < len(colors); i++ {
- t.headerParams = append(t.headerParams, makeSequence(colors[i]))
- }
-}
-
-// Adding column colors (ANSI codes)
-func (t *Table) SetColumnColor(colors ...Colors) {
- if t.colSize != len(colors) {
- panic("Number of column colors must be equal to number of headers.")
- }
- for i := 0; i < len(colors); i++ {
- t.columnsParams = append(t.columnsParams, makeSequence(colors[i]))
- }
-}
-
-// Adding column colors (ANSI codes)
-func (t *Table) SetFooterColor(colors ...Colors) {
- if len(t.footers) != len(colors) {
- panic("Number of footer colors must be equal to number of footer.")
- }
- for i := 0; i < len(colors); i++ {
- t.footerParams = append(t.footerParams, makeSequence(colors[i]))
- }
-}
-
-func Color(colors ...int) []int {
- return colors
-}
diff --git a/vendor/github.com/olekukonko/tablewriter/util.go b/vendor/github.com/olekukonko/tablewriter/util.go
deleted file mode 100644
index 380e7ab3..00000000
--- a/vendor/github.com/olekukonko/tablewriter/util.go
+++ /dev/null
@@ -1,93 +0,0 @@
-// Copyright 2014 Oleku Konko All rights reserved.
-// Use of this source code is governed by a MIT
-// license that can be found in the LICENSE file.
-
-// This module is a Table Writer API for the Go Programming Language.
-// The protocols were written in pure Go and works on windows and unix systems
-
-package tablewriter
-
-import (
- "math"
- "regexp"
- "strings"
-
- "github.com/mattn/go-runewidth"
-)
-
-var ansi = regexp.MustCompile("\033\\[(?:[0-9]{1,3}(?:;[0-9]{1,3})*)?[m|K]")
-
-func DisplayWidth(str string) int {
- return runewidth.StringWidth(ansi.ReplaceAllLiteralString(str, ""))
-}
-
-// Simple Condition for string
-// Returns value based on condition
-func ConditionString(cond bool, valid, inValid string) string {
- if cond {
- return valid
- }
- return inValid
-}
-
-func isNumOrSpace(r rune) bool {
- return ('0' <= r && r <= '9') || r == ' '
-}
-
-// Format Table Header
-// Replace _ , . and spaces
-func Title(name string) string {
- origLen := len(name)
- rs := []rune(name)
- for i, r := range rs {
- switch r {
- case '_':
- rs[i] = ' '
- case '.':
- // ignore floating number 0.0
- if (i != 0 && !isNumOrSpace(rs[i-1])) || (i != len(rs)-1 && !isNumOrSpace(rs[i+1])) {
- rs[i] = ' '
- }
- }
- }
- name = string(rs)
- name = strings.TrimSpace(name)
- if len(name) == 0 && origLen > 0 {
- // Keep at least one character. This is important to preserve
- // empty lines in multi-line headers/footers.
- name = " "
- }
- return strings.ToUpper(name)
-}
-
-// Pad String
-// Attempts to place string in the center
-func Pad(s, pad string, width int) string {
- gap := width - DisplayWidth(s)
- if gap > 0 {
- gapLeft := int(math.Ceil(float64(gap / 2)))
- gapRight := gap - gapLeft
- return strings.Repeat(string(pad), gapLeft) + s + strings.Repeat(string(pad), gapRight)
- }
- return s
-}
-
-// Pad String Right position
-// This would place string at the left side of the screen
-func PadRight(s, pad string, width int) string {
- gap := width - DisplayWidth(s)
- if gap > 0 {
- return s + strings.Repeat(string(pad), gap)
- }
- return s
-}
-
-// Pad String Left position
-// This would place string at the right side of the screen
-func PadLeft(s, pad string, width int) string {
- gap := width - DisplayWidth(s)
- if gap > 0 {
- return strings.Repeat(string(pad), gap) + s
- }
- return s
-}
diff --git a/vendor/github.com/olekukonko/tablewriter/wrap.go b/vendor/github.com/olekukonko/tablewriter/wrap.go
deleted file mode 100644
index a092ee1f..00000000
--- a/vendor/github.com/olekukonko/tablewriter/wrap.go
+++ /dev/null
@@ -1,99 +0,0 @@
-// Copyright 2014 Oleku Konko All rights reserved.
-// Use of this source code is governed by a MIT
-// license that can be found in the LICENSE file.
-
-// This module is a Table Writer API for the Go Programming Language.
-// The protocols were written in pure Go and works on windows and unix systems
-
-package tablewriter
-
-import (
- "math"
- "strings"
-
- "github.com/mattn/go-runewidth"
-)
-
-var (
- nl = "\n"
- sp = " "
-)
-
-const defaultPenalty = 1e5
-
-// Wrap wraps s into a paragraph of lines of length lim, with minimal
-// raggedness.
-func WrapString(s string, lim int) ([]string, int) {
- words := strings.Split(strings.Replace(s, nl, sp, -1), sp)
- var lines []string
- max := 0
- for _, v := range words {
- max = runewidth.StringWidth(v)
- if max > lim {
- lim = max
- }
- }
- for _, line := range WrapWords(words, 1, lim, defaultPenalty) {
- lines = append(lines, strings.Join(line, sp))
- }
- return lines, lim
-}
-
-// WrapWords is the low-level line-breaking algorithm, useful if you need more
-// control over the details of the text wrapping process. For most uses,
-// WrapString will be sufficient and more convenient.
-//
-// WrapWords splits a list of words into lines with minimal "raggedness",
-// treating each rune as one unit, accounting for spc units between adjacent
-// words on each line, and attempting to limit lines to lim units. Raggedness
-// is the total error over all lines, where error is the square of the
-// difference of the length of the line and lim. Too-long lines (which only
-// happen when a single word is longer than lim units) have pen penalty units
-// added to the error.
-func WrapWords(words []string, spc, lim, pen int) [][]string {
- n := len(words)
-
- length := make([][]int, n)
- for i := 0; i < n; i++ {
- length[i] = make([]int, n)
- length[i][i] = runewidth.StringWidth(words[i])
- for j := i + 1; j < n; j++ {
- length[i][j] = length[i][j-1] + spc + runewidth.StringWidth(words[j])
- }
- }
- nbrk := make([]int, n)
- cost := make([]int, n)
- for i := range cost {
- cost[i] = math.MaxInt32
- }
- for i := n - 1; i >= 0; i-- {
- if length[i][n-1] <= lim {
- cost[i] = 0
- nbrk[i] = n
- } else {
- for j := i + 1; j < n; j++ {
- d := lim - length[i][j-1]
- c := d*d + cost[j]
- if length[i][j-1] > lim {
- c += pen // too-long lines get a worse penalty
- }
- if c < cost[i] {
- cost[i] = c
- nbrk[i] = j
- }
- }
- }
- }
- var lines [][]string
- i := 0
- for i < n {
- lines = append(lines, words[i:nbrk[i]])
- i = nbrk[i]
- }
- return lines
-}
-
-// getLines decomposes a multiline string into a slice of strings.
-func getLines(s string) []string {
- return strings.Split(s, nl)
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/.dockerignore b/vendor/github.com/pelletier/go-toml/v2/.dockerignore
deleted file mode 100644
index 7b588347..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/.dockerignore
+++ /dev/null
@@ -1,2 +0,0 @@
-cmd/tomll/tomll
-cmd/tomljson/tomljson
diff --git a/vendor/github.com/pelletier/go-toml/v2/.gitattributes b/vendor/github.com/pelletier/go-toml/v2/.gitattributes
deleted file mode 100644
index 34a0a21a..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/.gitattributes
+++ /dev/null
@@ -1,4 +0,0 @@
-* text=auto
-
-benchmark/benchmark.toml text eol=lf
-testdata/** text eol=lf
diff --git a/vendor/github.com/pelletier/go-toml/v2/.gitignore b/vendor/github.com/pelletier/go-toml/v2/.gitignore
deleted file mode 100644
index a69e2b0e..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/.gitignore
+++ /dev/null
@@ -1,6 +0,0 @@
-test_program/test_program_bin
-fuzz/
-cmd/tomll/tomll
-cmd/tomljson/tomljson
-cmd/tomltestgen/tomltestgen
-dist
\ No newline at end of file
diff --git a/vendor/github.com/pelletier/go-toml/v2/.golangci.toml b/vendor/github.com/pelletier/go-toml/v2/.golangci.toml
deleted file mode 100644
index 067db551..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/.golangci.toml
+++ /dev/null
@@ -1,84 +0,0 @@
-[service]
-golangci-lint-version = "1.39.0"
-
-[linters-settings.wsl]
-allow-assign-and-anything = true
-
-[linters-settings.exhaustive]
-default-signifies-exhaustive = true
-
-[linters]
-disable-all = true
-enable = [
- "asciicheck",
- "bodyclose",
- "cyclop",
- "deadcode",
- "depguard",
- "dogsled",
- "dupl",
- "durationcheck",
- "errcheck",
- "errorlint",
- "exhaustive",
- # "exhaustivestruct",
- "exportloopref",
- "forbidigo",
- # "forcetypeassert",
- "funlen",
- "gci",
- # "gochecknoglobals",
- "gochecknoinits",
- "gocognit",
- "goconst",
- "gocritic",
- "gocyclo",
- "godot",
- "godox",
- # "goerr113",
- "gofmt",
- "gofumpt",
- "goheader",
- "goimports",
- "golint",
- "gomnd",
- # "gomoddirectives",
- "gomodguard",
- "goprintffuncname",
- "gosec",
- "gosimple",
- "govet",
- # "ifshort",
- "importas",
- "ineffassign",
- "lll",
- "makezero",
- "misspell",
- "nakedret",
- "nestif",
- "nilerr",
- # "nlreturn",
- "noctx",
- "nolintlint",
- #"paralleltest",
- "prealloc",
- "predeclared",
- "revive",
- "rowserrcheck",
- "sqlclosecheck",
- "staticcheck",
- "structcheck",
- "stylecheck",
- # "testpackage",
- "thelper",
- "tparallel",
- "typecheck",
- "unconvert",
- "unparam",
- "unused",
- "varcheck",
- "wastedassign",
- "whitespace",
- # "wrapcheck",
- # "wsl"
-]
diff --git a/vendor/github.com/pelletier/go-toml/v2/.goreleaser.yaml b/vendor/github.com/pelletier/go-toml/v2/.goreleaser.yaml
deleted file mode 100644
index 3aa1840e..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/.goreleaser.yaml
+++ /dev/null
@@ -1,123 +0,0 @@
-before:
- hooks:
- - go mod tidy
- - go fmt ./...
- - go test ./...
-builds:
- - id: tomll
- main: ./cmd/tomll
- binary: tomll
- env:
- - CGO_ENABLED=0
- flags:
- - -trimpath
- ldflags:
- - -X main.version={{.Version}} -X main.commit={{.Commit}} -X main.date={{.CommitDate}}
- mod_timestamp: '{{ .CommitTimestamp }}'
- targets:
- - linux_amd64
- - linux_arm64
- - linux_arm
- - windows_amd64
- - windows_arm64
- - windows_arm
- - darwin_amd64
- - darwin_arm64
- - id: tomljson
- main: ./cmd/tomljson
- binary: tomljson
- env:
- - CGO_ENABLED=0
- flags:
- - -trimpath
- ldflags:
- - -X main.version={{.Version}} -X main.commit={{.Commit}} -X main.date={{.CommitDate}}
- mod_timestamp: '{{ .CommitTimestamp }}'
- targets:
- - linux_amd64
- - linux_arm64
- - linux_arm
- - windows_amd64
- - windows_arm64
- - windows_arm
- - darwin_amd64
- - darwin_arm64
- - id: jsontoml
- main: ./cmd/jsontoml
- binary: jsontoml
- env:
- - CGO_ENABLED=0
- flags:
- - -trimpath
- ldflags:
- - -X main.version={{.Version}} -X main.commit={{.Commit}} -X main.date={{.CommitDate}}
- mod_timestamp: '{{ .CommitTimestamp }}'
- targets:
- - linux_amd64
- - linux_arm64
- - linux_arm
- - windows_amd64
- - windows_arm64
- - windows_arm
- - darwin_amd64
- - darwin_arm64
-universal_binaries:
- - id: tomll
- replace: true
- name_template: tomll
- - id: tomljson
- replace: true
- name_template: tomljson
- - id: jsontoml
- replace: true
- name_template: jsontoml
-archives:
-- id: jsontoml
- format: tar.xz
- builds:
- - jsontoml
- files:
- - none*
- name_template: "{{ .Binary }}_{{.Version}}_{{ .Os }}_{{ .Arch }}"
-- id: tomljson
- format: tar.xz
- builds:
- - tomljson
- files:
- - none*
- name_template: "{{ .Binary }}_{{.Version}}_{{ .Os }}_{{ .Arch }}"
-- id: tomll
- format: tar.xz
- builds:
- - tomll
- files:
- - none*
- name_template: "{{ .Binary }}_{{.Version}}_{{ .Os }}_{{ .Arch }}"
-dockers:
- - id: tools
- goos: linux
- goarch: amd64
- ids:
- - jsontoml
- - tomljson
- - tomll
- image_templates:
- - "ghcr.io/pelletier/go-toml:latest"
- - "ghcr.io/pelletier/go-toml:{{ .Tag }}"
- - "ghcr.io/pelletier/go-toml:v{{ .Major }}"
- skip_push: false
-checksum:
- name_template: 'sha256sums.txt'
-snapshot:
- name_template: "{{ incpatch .Version }}-next"
-release:
- github:
- owner: pelletier
- name: go-toml
- draft: true
- prerelease: auto
- mode: replace
-changelog:
- use: github-native
-announce:
- skip: true
diff --git a/vendor/github.com/pelletier/go-toml/v2/CONTRIBUTING.md b/vendor/github.com/pelletier/go-toml/v2/CONTRIBUTING.md
deleted file mode 100644
index 04dd12bc..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/CONTRIBUTING.md
+++ /dev/null
@@ -1,196 +0,0 @@
-# Contributing
-
-Thank you for your interest in go-toml! We appreciate you considering
-contributing to go-toml!
-
-The main goal is the project is to provide an easy-to-use and efficient TOML
-implementation for Go that gets the job done and gets out of your way – dealing
-with TOML is probably not the central piece of your project.
-
-As the single maintainer of go-toml, time is scarce. All help, big or small, is
-more than welcomed!
-
-## Ask questions
-
-Any question you may have, somebody else might have it too. Always feel free to
-ask them on the [discussion board][discussions]. We will try to answer them as
-clearly and quickly as possible, time permitting.
-
-Asking questions also helps us identify areas where the documentation needs
-improvement, or new features that weren't envisioned before. Sometimes, a
-seemingly innocent question leads to the fix of a bug. Don't hesitate and ask
-away!
-
-[discussions]: https://github.com/pelletier/go-toml/discussions
-
-## Improve the documentation
-
-The best way to share your knowledge and experience with go-toml is to improve
-the documentation. Fix a typo, clarify an interface, add an example, anything
-goes!
-
-The documentation is present in the [README][readme] and thorough the source
-code. On release, it gets updated on [pkg.go.dev][pkg.go.dev]. To make a change
-to the documentation, create a pull request with your proposed changes. For
-simple changes like that, the easiest way to go is probably the "Fork this
-project and edit the file" button on Github, displayed at the top right of the
-file. Unless it's a trivial change (for example a typo), provide a little bit of
-context in your pull request description or commit message.
-
-## Report a bug
-
-Found a bug! Sorry to hear that :(. Help us and other track them down and fix by
-reporting it. [File a new bug report][bug-report] on the [issues
-tracker][issues-tracker]. The template should provide enough guidance on what to
-include. When in doubt: add more details! By reducing ambiguity and providing
-more information, it decreases back and forth and saves everyone time.
-
-## Code changes
-
-Want to contribute a patch? Very happy to hear that!
-
-First, some high-level rules:
-
-- A short proposal with some POC code is better than a lengthy piece of text
- with no code. Code speaks louder than words. That being said, bigger changes
- should probably start with a [discussion][discussions].
-- No backward-incompatible patch will be accepted unless discussed. Sometimes
- it's hard, but we try not to break people's programs unless we absolutely have
- to.
-- If you are writing a new feature or extending an existing one, make sure to
- write some documentation.
-- Bug fixes need to be accompanied with regression tests.
-- New code needs to be tested.
-- Your commit messages need to explain why the change is needed, even if already
- included in the PR description.
-
-It does sound like a lot, but those best practices are here to save time overall
-and continuously improve the quality of the project, which is something everyone
-benefits from.
-
-### Get started
-
-The fairly standard code contribution process looks like that:
-
-1. [Fork the project][fork].
-2. Make your changes, commit on any branch you like.
-3. [Open up a pull request][pull-request]
-4. Review, potential ask for changes.
-5. Merge.
-
-Feel free to ask for help! You can create draft pull requests to gather
-some early feedback!
-
-### Run the tests
-
-You can run tests for go-toml using Go's test tool: `go test -race ./...`.
-
-During the pull request process, all tests will be ran on Linux, Windows, and
-MacOS on the last two versions of Go.
-
-However, given GitHub's new policy to _not_ run Actions on pull requests until a
-maintainer clicks on button, it is highly recommended that you run them locally
-as you make changes.
-
-### Check coverage
-
-We use `go tool cover` to compute test coverage. Most code editors have a way to
-run and display code coverage, but at the end of the day, we do this:
-
-```
-go test -covermode=atomic -coverprofile=coverage.out
-go tool cover -func=coverage.out
-```
-
-and verify that the overall percentage of tested code does not go down. This is
-a requirement. As a rule of thumb, all lines of code touched by your changes
-should be covered. On Unix you can use `./ci.sh coverage -d v2` to check if your
-code lowers the coverage.
-
-### Verify performance
-
-Go-toml aims to stay efficient. We rely on a set of scenarios executed with Go's
-builtin benchmark systems. Because of their noisy nature, containers provided by
-Github Actions cannot be reliably used for benchmarking. As a result, you are
-responsible for checking that your changes do not incur a performance penalty.
-You can run their following to execute benchmarks:
-
-```
-go test ./... -bench=. -count=10
-```
-
-Benchmark results should be compared against each other with
-[benchstat][benchstat]. Typical flow looks like this:
-
-1. On the `v2` branch, run `go test ./... -bench=. -count 10` and save output to
- a file (for example `old.txt`).
-2. Make some code changes.
-3. Run `go test ....` again, and save the output to an other file (for example
- `new.txt`).
-4. Run `benchstat old.txt new.txt` to check that time/op does not go up in any
- test.
-
-On Unix you can use `./ci.sh benchmark -d v2` to verify how your code impacts
-performance.
-
-It is highly encouraged to add the benchstat results to your pull request
-description. Pull requests that lower performance will receive more scrutiny.
-
-[benchstat]: https://pkg.go.dev/golang.org/x/perf/cmd/benchstat
-
-### Style
-
-Try to look around and follow the same format and structure as the rest of the
-code. We enforce using `go fmt` on the whole code base.
-
----
-
-## Maintainers-only
-
-### Merge pull request
-
-Checklist:
-
-- Passing CI.
-- Does not introduce backward-incompatible changes (unless discussed).
-- Has relevant doc changes.
-- Benchstat does not show performance regression.
-- Pull request is [labeled appropriately][pr-labels].
-- Title will be understandable in the changelog.
-
-1. Merge using "squash and merge".
-2. Make sure to edit the commit message to keep all the useful information
- nice and clean.
-3. Make sure the commit title is clear and contains the PR number (#123).
-
-### New release
-
-1. Decide on the next version number. Use semver.
-2. Generate release notes using [`gh`][gh]. Example:
-```
-$ gh api -X POST \
- -F tag_name='v2.0.0-beta.5' \
- -F target_commitish='v2' \
- -F previous_tag_name='v2.0.0-beta.4' \
- --jq '.body' \
- repos/pelletier/go-toml/releases/generate-notes
-```
-3. Look for "Other changes". That would indicate a pull request not labeled
- properly. Tweak labels and pull request titles until changelog looks good for
- users.
-4. [Draft new release][new-release].
-5. Fill tag and target with the same value used to generate the changelog.
-6. Set title to the new tag value.
-7. Paste the generated changelog.
-8. Check "create discussion", in the "Releases" category.
-9. Check pre-release if new version is an alpha or beta.
-
-[issues-tracker]: https://github.com/pelletier/go-toml/issues
-[bug-report]: https://github.com/pelletier/go-toml/issues/new?template=bug_report.md
-[pkg.go.dev]: https://pkg.go.dev/github.com/pelletier/go-toml
-[readme]: ./README.md
-[fork]: https://help.github.com/articles/fork-a-repo
-[pull-request]: https://help.github.com/en/articles/creating-a-pull-request
-[new-release]: https://github.com/pelletier/go-toml/releases/new
-[gh]: https://github.com/cli/cli
-[pr-labels]: https://github.com/pelletier/go-toml/blob/v2/.github/release.yml
diff --git a/vendor/github.com/pelletier/go-toml/v2/Dockerfile b/vendor/github.com/pelletier/go-toml/v2/Dockerfile
deleted file mode 100644
index b9e93323..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/Dockerfile
+++ /dev/null
@@ -1,5 +0,0 @@
-FROM scratch
-ENV PATH "$PATH:/bin"
-COPY tomll /bin/tomll
-COPY tomljson /bin/tomljson
-COPY jsontoml /bin/jsontoml
diff --git a/vendor/github.com/pelletier/go-toml/v2/LICENSE b/vendor/github.com/pelletier/go-toml/v2/LICENSE
deleted file mode 100644
index 6839d51c..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2013 - 2022 Thomas Pelletier, Eric Anderton
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/pelletier/go-toml/v2/README.md b/vendor/github.com/pelletier/go-toml/v2/README.md
deleted file mode 100644
index 9f8439cc..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/README.md
+++ /dev/null
@@ -1,563 +0,0 @@
-# go-toml v2
-
-Go library for the [TOML](https://toml.io/en/) format.
-
-This library supports [TOML v1.0.0](https://toml.io/en/v1.0.0).
-
-[🞠Bug Reports](https://github.com/pelletier/go-toml/issues)
-
-[💬 Anything else](https://github.com/pelletier/go-toml/discussions)
-
-## Documentation
-
-Full API, examples, and implementation notes are available in the Go
-documentation.
-
-[](https://pkg.go.dev/github.com/pelletier/go-toml/v2)
-
-## Import
-
-```go
-import "github.com/pelletier/go-toml/v2"
-```
-
-See [Modules](#Modules).
-
-## Features
-
-### Stdlib behavior
-
-As much as possible, this library is designed to behave similarly as the
-standard library's `encoding/json`.
-
-### Performance
-
-While go-toml favors usability, it is written with performance in mind. Most
-operations should not be shockingly slow. See [benchmarks](#benchmarks).
-
-### Strict mode
-
-`Decoder` can be set to "strict mode", which makes it error when some parts of
-the TOML document was not present in the target structure. This is a great way
-to check for typos. [See example in the documentation][strict].
-
-[strict]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#example-Decoder.DisallowUnknownFields
-
-### Contextualized errors
-
-When most decoding errors occur, go-toml returns [`DecodeError`][decode-err]),
-which contains a human readable contextualized version of the error. For
-example:
-
-```
-2| key1 = "value1"
-3| key2 = "missing2"
- | ~~~~ missing field
-4| key3 = "missing3"
-5| key4 = "value4"
-```
-
-[decode-err]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#DecodeError
-
-### Local date and time support
-
-TOML supports native [local date/times][ldt]. It allows to represent a given
-date, time, or date-time without relation to a timezone or offset. To support
-this use-case, go-toml provides [`LocalDate`][tld], [`LocalTime`][tlt], and
-[`LocalDateTime`][tldt]. Those types can be transformed to and from `time.Time`,
-making them convenient yet unambiguous structures for their respective TOML
-representation.
-
-[ldt]: https://toml.io/en/v1.0.0#local-date-time
-[tld]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#LocalDate
-[tlt]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#LocalTime
-[tldt]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#LocalDateTime
-
-## Getting started
-
-Given the following struct, let's see how to read it and write it as TOML:
-
-```go
-type MyConfig struct {
- Version int
- Name string
- Tags []string
-}
-```
-
-### Unmarshaling
-
-[`Unmarshal`][unmarshal] reads a TOML document and fills a Go structure with its
-content. For example:
-
-```go
-doc := `
-version = 2
-name = "go-toml"
-tags = ["go", "toml"]
-`
-
-var cfg MyConfig
-err := toml.Unmarshal([]byte(doc), &cfg)
-if err != nil {
- panic(err)
-}
-fmt.Println("version:", cfg.Version)
-fmt.Println("name:", cfg.Name)
-fmt.Println("tags:", cfg.Tags)
-
-// Output:
-// version: 2
-// name: go-toml
-// tags: [go toml]
-```
-
-[unmarshal]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#Unmarshal
-
-### Marshaling
-
-[`Marshal`][marshal] is the opposite of Unmarshal: it represents a Go structure
-as a TOML document:
-
-```go
-cfg := MyConfig{
- Version: 2,
- Name: "go-toml",
- Tags: []string{"go", "toml"},
-}
-
-b, err := toml.Marshal(cfg)
-if err != nil {
- panic(err)
-}
-fmt.Println(string(b))
-
-// Output:
-// Version = 2
-// Name = 'go-toml'
-// Tags = ['go', 'toml']
-```
-
-[marshal]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#Marshal
-
-## Unstable API
-
-This API does not yet follow the backward compatibility guarantees of this
-library. They provide early access to features that may have rough edges or an
-API subject to change.
-
-### Parser
-
-Parser is the unstable API that allows iterative parsing of a TOML document at
-the AST level. See https://pkg.go.dev/github.com/pelletier/go-toml/v2/unstable.
-
-## Benchmarks
-
-Execution time speedup compared to other Go TOML libraries:
-
-
-
- Benchmark | go-toml v1 | BurntSushi/toml |
-
-
- Marshal/HugoFrontMatter-2 | 1.9x | 1.9x |
- Marshal/ReferenceFile/map-2 | 1.7x | 1.8x |
- Marshal/ReferenceFile/struct-2 | 2.2x | 2.5x |
- Unmarshal/HugoFrontMatter-2 | 2.9x | 2.9x |
- Unmarshal/ReferenceFile/map-2 | 2.6x | 2.9x |
- Unmarshal/ReferenceFile/struct-2 | 4.4x | 5.3x |
-
-
-See more
-The table above has the results of the most common use-cases. The table below
-contains the results of all benchmarks, including unrealistic ones. It is
-provided for completeness.
-
-
-
- Benchmark | go-toml v1 | BurntSushi/toml |
-
-
- Marshal/SimpleDocument/map-2 | 1.8x | 2.9x |
- Marshal/SimpleDocument/struct-2 | 2.7x | 4.2x |
- Unmarshal/SimpleDocument/map-2 | 4.5x | 3.1x |
- Unmarshal/SimpleDocument/struct-2 | 6.2x | 3.9x |
- UnmarshalDataset/example-2 | 3.1x | 3.5x |
- UnmarshalDataset/code-2 | 2.3x | 3.1x |
- UnmarshalDataset/twitter-2 | 2.5x | 2.6x |
- UnmarshalDataset/citm_catalog-2 | 2.1x | 2.2x |
- UnmarshalDataset/canada-2 | 1.6x | 1.3x |
- UnmarshalDataset/config-2 | 4.3x | 3.2x |
- [Geo mean] | 2.7x | 2.8x |
-
-
-This table can be generated with ./ci.sh benchmark -a -html
.
-
-
-## Modules
-
-go-toml uses Go's standard modules system.
-
-Installation instructions:
-
-- Go ≥ 1.16: Nothing to do. Use the import in your code. The `go` command deals
- with it automatically.
-- Go ≥ 1.13: `GO111MODULE=on go get github.com/pelletier/go-toml/v2`.
-
-In case of trouble: [Go Modules FAQ][mod-faq].
-
-[mod-faq]: https://github.com/golang/go/wiki/Modules#why-does-installing-a-tool-via-go-get-fail-with-error-cannot-find-main-module
-
-## Tools
-
-Go-toml provides three handy command line tools:
-
- * `tomljson`: Reads a TOML file and outputs its JSON representation.
-
- ```
- $ go install github.com/pelletier/go-toml/v2/cmd/tomljson@latest
- $ tomljson --help
- ```
-
- * `jsontoml`: Reads a JSON file and outputs a TOML representation.
-
- ```
- $ go install github.com/pelletier/go-toml/v2/cmd/jsontoml@latest
- $ jsontoml --help
- ```
-
- * `tomll`: Lints and reformats a TOML file.
-
- ```
- $ go install github.com/pelletier/go-toml/v2/cmd/tomll@latest
- $ tomll --help
- ```
-
-### Docker image
-
-Those tools are also available as a [Docker image][docker]. For example, to use
-`tomljson`:
-
-```
-docker run -i ghcr.io/pelletier/go-toml:v2 tomljson < example.toml
-```
-
-Multiple versions are availble on [ghcr.io][docker].
-
-[docker]: https://github.com/pelletier/go-toml/pkgs/container/go-toml
-
-## Migrating from v1
-
-This section describes the differences between v1 and v2, with some pointers on
-how to get the original behavior when possible.
-
-### Decoding / Unmarshal
-
-#### Automatic field name guessing
-
-When unmarshaling to a struct, if a key in the TOML document does not exactly
-match the name of a struct field or any of the `toml`-tagged field, v1 tries
-multiple variations of the key ([code][v1-keys]).
-
-V2 instead does a case-insensitive matching, like `encoding/json`.
-
-This could impact you if you are relying on casing to differentiate two fields,
-and one of them is a not using the `toml` struct tag. The recommended solution
-is to be specific about tag names for those fields using the `toml` struct tag.
-
-[v1-keys]: https://github.com/pelletier/go-toml/blob/a2e52561804c6cd9392ebf0048ca64fe4af67a43/marshal.go#L775-L781
-
-#### Ignore preexisting value in interface
-
-When decoding into a non-nil `interface{}`, go-toml v1 uses the type of the
-element in the interface to decode the object. For example:
-
-```go
-type inner struct {
- B interface{}
-}
-type doc struct {
- A interface{}
-}
-
-d := doc{
- A: inner{
- B: "Before",
- },
-}
-
-data := `
-[A]
-B = "After"
-`
-
-toml.Unmarshal([]byte(data), &d)
-fmt.Printf("toml v1: %#v\n", d)
-
-// toml v1: main.doc{A:main.inner{B:"After"}}
-```
-
-In this case, field `A` is of type `interface{}`, containing a `inner` struct.
-V1 sees that type and uses it when decoding the object.
-
-When decoding an object into an `interface{}`, V2 instead disregards whatever
-value the `interface{}` may contain and replaces it with a
-`map[string]interface{}`. With the same data structure as above, here is what
-the result looks like:
-
-```go
-toml.Unmarshal([]byte(data), &d)
-fmt.Printf("toml v2: %#v\n", d)
-
-// toml v2: main.doc{A:map[string]interface {}{"B":"After"}}
-```
-
-This is to match `encoding/json`'s behavior. There is no way to make the v2
-decoder behave like v1.
-
-#### Values out of array bounds ignored
-
-When decoding into an array, v1 returns an error when the number of elements
-contained in the doc is superior to the capacity of the array. For example:
-
-```go
-type doc struct {
- A [2]string
-}
-d := doc{}
-err := toml.Unmarshal([]byte(`A = ["one", "two", "many"]`), &d)
-fmt.Println(err)
-
-// (1, 1): unmarshal: TOML array length (3) exceeds destination array length (2)
-```
-
-In the same situation, v2 ignores the last value:
-
-```go
-err := toml.Unmarshal([]byte(`A = ["one", "two", "many"]`), &d)
-fmt.Println("err:", err, "d:", d)
-// err: d: {[one two]}
-```
-
-This is to match `encoding/json`'s behavior. There is no way to make the v2
-decoder behave like v1.
-
-#### Support for `toml.Unmarshaler` has been dropped
-
-This method was not widely used, poorly defined, and added a lot of complexity.
-A similar effect can be achieved by implementing the `encoding.TextUnmarshaler`
-interface and use strings.
-
-#### Support for `default` struct tag has been dropped
-
-This feature adds complexity and a poorly defined API for an effect that can be
-accomplished outside of the library.
-
-It does not seem like other format parsers in Go support that feature (the
-project referenced in the original ticket #202 has not been updated since 2017).
-Given that go-toml v2 should not touch values not in the document, the same
-effect can be achieved by pre-filling the struct with defaults (libraries like
-[go-defaults][go-defaults] can help). Also, string representation is not well
-defined for all types: it creates issues like #278.
-
-The recommended replacement is pre-filling the struct before unmarshaling.
-
-[go-defaults]: https://github.com/mcuadros/go-defaults
-
-#### `toml.Tree` replacement
-
-This structure was the initial attempt at providing a document model for
-go-toml. It allows manipulating the structure of any document, encoding and
-decoding from their TOML representation. While a more robust feature was
-initially planned in go-toml v2, this has been ultimately [removed from
-scope][nodoc] of this library, with no plan to add it back at the moment. The
-closest equivalent at the moment would be to unmarshal into an `interface{}` and
-use type assertions and/or reflection to manipulate the arbitrary
-structure. However this would fall short of providing all of the TOML features
-such as adding comments and be specific about whitespace.
-
-
-#### `toml.Position` are not retrievable anymore
-
-The API for retrieving the position (line, column) of a specific TOML element do
-not exist anymore. This was done to minimize the amount of concepts introduced
-by the library (query path), and avoid the performance hit related to storing
-positions in the absence of a document model, for a feature that seemed to have
-little use. Errors however have gained more detailed position
-information. Position retrieval seems better fitted for a document model, which
-has been [removed from the scope][nodoc] of go-toml v2 at the moment.
-
-### Encoding / Marshal
-
-#### Default struct fields order
-
-V1 emits struct fields order alphabetically by default. V2 struct fields are
-emitted in order they are defined. For example:
-
-```go
-type S struct {
- B string
- A string
-}
-
-data := S{
- B: "B",
- A: "A",
-}
-
-b, _ := tomlv1.Marshal(data)
-fmt.Println("v1:\n" + string(b))
-
-b, _ = tomlv2.Marshal(data)
-fmt.Println("v2:\n" + string(b))
-
-// Output:
-// v1:
-// A = "A"
-// B = "B"
-
-// v2:
-// B = 'B'
-// A = 'A'
-```
-
-There is no way to make v2 encoder behave like v1. A workaround could be to
-manually sort the fields alphabetically in the struct definition, or generate
-struct types using `reflect.StructOf`.
-
-#### No indentation by default
-
-V1 automatically indents content of tables by default. V2 does not. However the
-same behavior can be obtained using [`Encoder.SetIndentTables`][sit]. For example:
-
-```go
-data := map[string]interface{}{
- "table": map[string]string{
- "key": "value",
- },
-}
-
-b, _ := tomlv1.Marshal(data)
-fmt.Println("v1:\n" + string(b))
-
-b, _ = tomlv2.Marshal(data)
-fmt.Println("v2:\n" + string(b))
-
-buf := bytes.Buffer{}
-enc := tomlv2.NewEncoder(&buf)
-enc.SetIndentTables(true)
-enc.Encode(data)
-fmt.Println("v2 Encoder:\n" + string(buf.Bytes()))
-
-// Output:
-// v1:
-//
-// [table]
-// key = "value"
-//
-// v2:
-// [table]
-// key = 'value'
-//
-//
-// v2 Encoder:
-// [table]
-// key = 'value'
-```
-
-[sit]: https://pkg.go.dev/github.com/pelletier/go-toml/v2#Encoder.SetIndentTables
-
-#### Keys and strings are single quoted
-
-V1 always uses double quotes (`"`) around strings and keys that cannot be
-represented bare (unquoted). V2 uses single quotes instead by default (`'`),
-unless a character cannot be represented, then falls back to double quotes. As a
-result of this change, `Encoder.QuoteMapKeys` has been removed, as it is not
-useful anymore.
-
-There is no way to make v2 encoder behave like v1.
-
-#### `TextMarshaler` emits as a string, not TOML
-
-Types that implement [`encoding.TextMarshaler`][tm] can emit arbitrary TOML in
-v1. The encoder would append the result to the output directly. In v2 the result
-is wrapped in a string. As a result, this interface cannot be implemented by the
-root object.
-
-There is no way to make v2 encoder behave like v1.
-
-[tm]: https://golang.org/pkg/encoding/#TextMarshaler
-
-#### `Encoder.CompactComments` has been removed
-
-Emitting compact comments is now the default behavior of go-toml. This option
-is not necessary anymore.
-
-#### Struct tags have been merged
-
-V1 used to provide multiple struct tags: `comment`, `commented`, `multiline`,
-`toml`, and `omitempty`. To behave more like the standard library, v2 has merged
-`toml`, `multiline`, and `omitempty`. For example:
-
-```go
-type doc struct {
- // v1
- F string `toml:"field" multiline:"true" omitempty:"true"`
- // v2
- F string `toml:"field,multiline,omitempty"`
-}
-```
-
-Has a result, the `Encoder.SetTag*` methods have been removed, as there is just
-one tag now.
-
-
-#### `commented` tag has been removed
-
-There is no replacement for the `commented` tag. This feature would be better
-suited in a proper document model for go-toml v2, which has been [cut from
-scope][nodoc] at the moment.
-
-#### `Encoder.ArraysWithOneElementPerLine` has been renamed
-
-The new name is `Encoder.SetArraysMultiline`. The behavior should be the same.
-
-#### `Encoder.Indentation` has been renamed
-
-The new name is `Encoder.SetIndentSymbol`. The behavior should be the same.
-
-
-#### Embedded structs behave like stdlib
-
-V1 defaults to merging embedded struct fields into the embedding struct. This
-behavior was unexpected because it does not follow the standard library. To
-avoid breaking backward compatibility, the `Encoder.PromoteAnonymous` method was
-added to make the encoder behave correctly. Given backward compatibility is not
-a problem anymore, v2 does the right thing by default: it follows the behavior
-of `encoding/json`. `Encoder.PromoteAnonymous` has been removed.
-
-[nodoc]: https://github.com/pelletier/go-toml/discussions/506#discussioncomment-1526038
-
-### `query`
-
-go-toml v1 provided the [`go-toml/query`][query] package. It allowed to run
-JSONPath-style queries on TOML files. This feature is not available in v2. For a
-replacement, check out [dasel][dasel].
-
-This package has been removed because it was essentially not supported anymore
-(last commit May 2020), increased the complexity of the code base, and more
-complete solutions exist out there.
-
-[query]: https://github.com/pelletier/go-toml/tree/f99d6bbca119636aeafcf351ee52b3d202782627/query
-[dasel]: https://github.com/TomWright/dasel
-
-## Versioning
-
-Go-toml follows [Semantic Versioning](http://semver.org/). The supported version
-of [TOML](https://github.com/toml-lang/toml) is indicated at the beginning of
-this document. The last two major versions of Go are supported
-(see [Go Release Policy](https://golang.org/doc/devel/release.html#policy)).
-
-## License
-
-The MIT License (MIT). Read [LICENSE](LICENSE).
diff --git a/vendor/github.com/pelletier/go-toml/v2/SECURITY.md b/vendor/github.com/pelletier/go-toml/v2/SECURITY.md
deleted file mode 100644
index b2f21cfc..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/SECURITY.md
+++ /dev/null
@@ -1,19 +0,0 @@
-# Security Policy
-
-## Supported Versions
-
-Use this section to tell people about which versions of your project are
-currently being supported with security updates.
-
-| Version | Supported |
-| ---------- | ------------------ |
-| Latest 2.x | :white_check_mark: |
-| All 1.x | :x: |
-| All 0.x | :x: |
-
-## Reporting a Vulnerability
-
-Email a vulnerability report to `security@pelletier.codes`. Make sure to include
-as many details as possible to reproduce the vulnerability. This is a
-side-project: I will try to get back to you as quickly as possible, time
-permitting in my personal life. Providing a working patch helps very much!
diff --git a/vendor/github.com/pelletier/go-toml/v2/ci.sh b/vendor/github.com/pelletier/go-toml/v2/ci.sh
deleted file mode 100644
index d916c5f2..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/ci.sh
+++ /dev/null
@@ -1,279 +0,0 @@
-#!/usr/bin/env bash
-
-
-stderr() {
- echo "$@" 1>&2
-}
-
-usage() {
- b=$(basename "$0")
- echo $b: ERROR: "$@" 1>&2
-
- cat 1>&2 < coverage.out
- go tool cover -func=coverage.out
- popd
-
- if [ "${branch}" != "HEAD" ]; then
- git worktree remove --force "$dir"
- fi
-}
-
-coverage() {
- case "$1" in
- -d)
- shift
- target="${1?Need to provide a target branch argument}"
-
- output_dir="$(mktemp -d)"
- target_out="${output_dir}/target.txt"
- head_out="${output_dir}/head.txt"
-
- cover "${target}" > "${target_out}"
- cover "HEAD" > "${head_out}"
-
- cat "${target_out}"
- cat "${head_out}"
-
- echo ""
-
- target_pct="$(tail -n2 ${target_out} | head -n1 | sed -E 's/.*total.*\t([0-9.]+)%.*/\1/')"
- head_pct="$(tail -n2 ${head_out} | head -n1 | sed -E 's/.*total.*\t([0-9.]+)%/\1/')"
- echo "Results: ${target} ${target_pct}% HEAD ${head_pct}%"
-
- delta_pct=$(echo "$head_pct - $target_pct" | bc -l)
- echo "Delta: ${delta_pct}"
-
- if [[ $delta_pct = \-* ]]; then
- echo "Regression!";
-
- target_diff="${output_dir}/target.diff.txt"
- head_diff="${output_dir}/head.diff.txt"
- cat "${target_out}" | grep -E '^github.com/pelletier/go-toml' | tr -s "\t " | cut -f 2,3 | sort > "${target_diff}"
- cat "${head_out}" | grep -E '^github.com/pelletier/go-toml' | tr -s "\t " | cut -f 2,3 | sort > "${head_diff}"
-
- diff --side-by-side --suppress-common-lines "${target_diff}" "${head_diff}"
- return 1
- fi
- return 0
- ;;
- esac
-
- cover "${1-HEAD}"
-}
-
-bench() {
- branch="${1}"
- out="${2}"
- replace="${3}"
- dir="$(mktemp -d)"
-
- stderr "Executing benchmark for ${branch} at ${dir}"
-
- if [ "${branch}" = "HEAD" ]; then
- cp -r . "${dir}/"
- else
- git worktree add "$dir" "$branch"
- fi
-
- pushd "$dir"
-
- if [ "${replace}" != "" ]; then
- find ./benchmark/ -iname '*.go' -exec sed -i -E "s|github.com/pelletier/go-toml/v2|${replace}|g" {} \;
- go get "${replace}"
- fi
-
- export GOMAXPROCS=2
- nice -n -19 taskset --cpu-list 0,1 go test '-bench=^Benchmark(Un)?[mM]arshal' -count=5 -run=Nothing ./... | tee "${out}"
- popd
-
- if [ "${branch}" != "HEAD" ]; then
- git worktree remove --force "$dir"
- fi
-}
-
-fmktemp() {
- if mktemp --version|grep GNU >/dev/null; then
- mktemp --suffix=-$1;
- else
- mktemp -t $1;
- fi
-}
-
-benchstathtml() {
-python3 - $1 <<'EOF'
-import sys
-
-lines = []
-stop = False
-
-with open(sys.argv[1]) as f:
- for line in f.readlines():
- line = line.strip()
- if line == "":
- stop = True
- if not stop:
- lines.append(line.split(','))
-
-results = []
-for line in reversed(lines[1:]):
- v2 = float(line[1])
- results.append([
- line[0].replace("-32", ""),
- "%.1fx" % (float(line[3])/v2), # v1
- "%.1fx" % (float(line[5])/v2), # bs
- ])
-# move geomean to the end
-results.append(results[0])
-del results[0]
-
-
-def printtable(data):
- print("""
-
-
- Benchmark | go-toml v1 | BurntSushi/toml |
-
- """)
-
- for r in data:
- print(" {} | {} | {} |
".format(*r))
-
- print("""
-
""")
-
-
-def match(x):
- return "ReferenceFile" in x[0] or "HugoFrontMatter" in x[0]
-
-above = [x for x in results if match(x)]
-below = [x for x in results if not match(x)]
-
-printtable(above)
-print("See more
")
-print("""The table above has the results of the most common use-cases. The table below
-contains the results of all benchmarks, including unrealistic ones. It is
-provided for completeness.
""")
-printtable(below)
-print('This table can be generated with ./ci.sh benchmark -a -html
.
')
-print(" ")
-
-EOF
-}
-
-benchmark() {
- case "$1" in
- -d)
- shift
- target="${1?Need to provide a target branch argument}"
-
- old=`fmktemp ${target}`
- bench "${target}" "${old}"
-
- new=`fmktemp HEAD`
- bench HEAD "${new}"
-
- benchstat "${old}" "${new}"
- return 0
- ;;
- -a)
- shift
-
- v2stats=`fmktemp go-toml-v2`
- bench HEAD "${v2stats}" "github.com/pelletier/go-toml/v2"
- v1stats=`fmktemp go-toml-v1`
- bench HEAD "${v1stats}" "github.com/pelletier/go-toml"
- bsstats=`fmktemp bs-toml`
- bench HEAD "${bsstats}" "github.com/BurntSushi/toml"
-
- cp "${v2stats}" go-toml-v2.txt
- cp "${v1stats}" go-toml-v1.txt
- cp "${bsstats}" bs-toml.txt
-
- if [ "$1" = "-html" ]; then
- tmpcsv=`fmktemp csv`
- benchstat -csv -geomean go-toml-v2.txt go-toml-v1.txt bs-toml.txt > $tmpcsv
- benchstathtml $tmpcsv
- else
- benchstat -geomean go-toml-v2.txt go-toml-v1.txt bs-toml.txt
- fi
-
- rm -f go-toml-v2.txt go-toml-v1.txt bs-toml.txt
- return $?
- esac
-
- bench "${1-HEAD}" `mktemp`
-}
-
-case "$1" in
- coverage) shift; coverage $@;;
- benchmark) shift; benchmark $@;;
- *) usage "bad argument $1";;
-esac
diff --git a/vendor/github.com/pelletier/go-toml/v2/decode.go b/vendor/github.com/pelletier/go-toml/v2/decode.go
deleted file mode 100644
index 3a860d0f..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/decode.go
+++ /dev/null
@@ -1,550 +0,0 @@
-package toml
-
-import (
- "fmt"
- "math"
- "strconv"
- "time"
-
- "github.com/pelletier/go-toml/v2/unstable"
-)
-
-func parseInteger(b []byte) (int64, error) {
- if len(b) > 2 && b[0] == '0' {
- switch b[1] {
- case 'x':
- return parseIntHex(b)
- case 'b':
- return parseIntBin(b)
- case 'o':
- return parseIntOct(b)
- default:
- panic(fmt.Errorf("invalid base '%c', should have been checked by scanIntOrFloat", b[1]))
- }
- }
-
- return parseIntDec(b)
-}
-
-func parseLocalDate(b []byte) (LocalDate, error) {
- // full-date = date-fullyear "-" date-month "-" date-mday
- // date-fullyear = 4DIGIT
- // date-month = 2DIGIT ; 01-12
- // date-mday = 2DIGIT ; 01-28, 01-29, 01-30, 01-31 based on month/year
- var date LocalDate
-
- if len(b) != 10 || b[4] != '-' || b[7] != '-' {
- return date, unstable.NewParserError(b, "dates are expected to have the format YYYY-MM-DD")
- }
-
- var err error
-
- date.Year, err = parseDecimalDigits(b[0:4])
- if err != nil {
- return LocalDate{}, err
- }
-
- date.Month, err = parseDecimalDigits(b[5:7])
- if err != nil {
- return LocalDate{}, err
- }
-
- date.Day, err = parseDecimalDigits(b[8:10])
- if err != nil {
- return LocalDate{}, err
- }
-
- if !isValidDate(date.Year, date.Month, date.Day) {
- return LocalDate{}, unstable.NewParserError(b, "impossible date")
- }
-
- return date, nil
-}
-
-func parseDecimalDigits(b []byte) (int, error) {
- v := 0
-
- for i, c := range b {
- if c < '0' || c > '9' {
- return 0, unstable.NewParserError(b[i:i+1], "expected digit (0-9)")
- }
- v *= 10
- v += int(c - '0')
- }
-
- return v, nil
-}
-
-func parseDateTime(b []byte) (time.Time, error) {
- // offset-date-time = full-date time-delim full-time
- // full-time = partial-time time-offset
- // time-offset = "Z" / time-numoffset
- // time-numoffset = ( "+" / "-" ) time-hour ":" time-minute
-
- dt, b, err := parseLocalDateTime(b)
- if err != nil {
- return time.Time{}, err
- }
-
- var zone *time.Location
-
- if len(b) == 0 {
- // parser should have checked that when assigning the date time node
- panic("date time should have a timezone")
- }
-
- if b[0] == 'Z' || b[0] == 'z' {
- b = b[1:]
- zone = time.UTC
- } else {
- const dateTimeByteLen = 6
- if len(b) != dateTimeByteLen {
- return time.Time{}, unstable.NewParserError(b, "invalid date-time timezone")
- }
- var direction int
- switch b[0] {
- case '-':
- direction = -1
- case '+':
- direction = +1
- default:
- return time.Time{}, unstable.NewParserError(b[:1], "invalid timezone offset character")
- }
-
- if b[3] != ':' {
- return time.Time{}, unstable.NewParserError(b[3:4], "expected a : separator")
- }
-
- hours, err := parseDecimalDigits(b[1:3])
- if err != nil {
- return time.Time{}, err
- }
- if hours > 23 {
- return time.Time{}, unstable.NewParserError(b[:1], "invalid timezone offset hours")
- }
-
- minutes, err := parseDecimalDigits(b[4:6])
- if err != nil {
- return time.Time{}, err
- }
- if minutes > 59 {
- return time.Time{}, unstable.NewParserError(b[:1], "invalid timezone offset minutes")
- }
-
- seconds := direction * (hours*3600 + minutes*60)
- if seconds == 0 {
- zone = time.UTC
- } else {
- zone = time.FixedZone("", seconds)
- }
- b = b[dateTimeByteLen:]
- }
-
- if len(b) > 0 {
- return time.Time{}, unstable.NewParserError(b, "extra bytes at the end of the timezone")
- }
-
- t := time.Date(
- dt.Year,
- time.Month(dt.Month),
- dt.Day,
- dt.Hour,
- dt.Minute,
- dt.Second,
- dt.Nanosecond,
- zone)
-
- return t, nil
-}
-
-func parseLocalDateTime(b []byte) (LocalDateTime, []byte, error) {
- var dt LocalDateTime
-
- const localDateTimeByteMinLen = 11
- if len(b) < localDateTimeByteMinLen {
- return dt, nil, unstable.NewParserError(b, "local datetimes are expected to have the format YYYY-MM-DDTHH:MM:SS[.NNNNNNNNN]")
- }
-
- date, err := parseLocalDate(b[:10])
- if err != nil {
- return dt, nil, err
- }
- dt.LocalDate = date
-
- sep := b[10]
- if sep != 'T' && sep != ' ' && sep != 't' {
- return dt, nil, unstable.NewParserError(b[10:11], "datetime separator is expected to be T or a space")
- }
-
- t, rest, err := parseLocalTime(b[11:])
- if err != nil {
- return dt, nil, err
- }
- dt.LocalTime = t
-
- return dt, rest, nil
-}
-
-// parseLocalTime is a bit different because it also returns the remaining
-// []byte that is didn't need. This is to allow parseDateTime to parse those
-// remaining bytes as a timezone.
-func parseLocalTime(b []byte) (LocalTime, []byte, error) {
- var (
- nspow = [10]int{0, 1e8, 1e7, 1e6, 1e5, 1e4, 1e3, 1e2, 1e1, 1e0}
- t LocalTime
- )
-
- // check if b matches to have expected format HH:MM:SS[.NNNNNN]
- const localTimeByteLen = 8
- if len(b) < localTimeByteLen {
- return t, nil, unstable.NewParserError(b, "times are expected to have the format HH:MM:SS[.NNNNNN]")
- }
-
- var err error
-
- t.Hour, err = parseDecimalDigits(b[0:2])
- if err != nil {
- return t, nil, err
- }
-
- if t.Hour > 23 {
- return t, nil, unstable.NewParserError(b[0:2], "hour cannot be greater 23")
- }
- if b[2] != ':' {
- return t, nil, unstable.NewParserError(b[2:3], "expecting colon between hours and minutes")
- }
-
- t.Minute, err = parseDecimalDigits(b[3:5])
- if err != nil {
- return t, nil, err
- }
- if t.Minute > 59 {
- return t, nil, unstable.NewParserError(b[3:5], "minutes cannot be greater 59")
- }
- if b[5] != ':' {
- return t, nil, unstable.NewParserError(b[5:6], "expecting colon between minutes and seconds")
- }
-
- t.Second, err = parseDecimalDigits(b[6:8])
- if err != nil {
- return t, nil, err
- }
-
- if t.Second > 60 {
- return t, nil, unstable.NewParserError(b[6:8], "seconds cannot be greater 60")
- }
-
- b = b[8:]
-
- if len(b) >= 1 && b[0] == '.' {
- frac := 0
- precision := 0
- digits := 0
-
- for i, c := range b[1:] {
- if !isDigit(c) {
- if i == 0 {
- return t, nil, unstable.NewParserError(b[0:1], "need at least one digit after fraction point")
- }
- break
- }
- digits++
-
- const maxFracPrecision = 9
- if i >= maxFracPrecision {
- // go-toml allows decoding fractional seconds
- // beyond the supported precision of 9
- // digits. It truncates the fractional component
- // to the supported precision and ignores the
- // remaining digits.
- //
- // https://github.com/pelletier/go-toml/discussions/707
- continue
- }
-
- frac *= 10
- frac += int(c - '0')
- precision++
- }
-
- if precision == 0 {
- return t, nil, unstable.NewParserError(b[:1], "nanoseconds need at least one digit")
- }
-
- t.Nanosecond = frac * nspow[precision]
- t.Precision = precision
-
- return t, b[1+digits:], nil
- }
- return t, b, nil
-}
-
-//nolint:cyclop
-func parseFloat(b []byte) (float64, error) {
- if len(b) == 4 && (b[0] == '+' || b[0] == '-') && b[1] == 'n' && b[2] == 'a' && b[3] == 'n' {
- return math.NaN(), nil
- }
-
- cleaned, err := checkAndRemoveUnderscoresFloats(b)
- if err != nil {
- return 0, err
- }
-
- if cleaned[0] == '.' {
- return 0, unstable.NewParserError(b, "float cannot start with a dot")
- }
-
- if cleaned[len(cleaned)-1] == '.' {
- return 0, unstable.NewParserError(b, "float cannot end with a dot")
- }
-
- dotAlreadySeen := false
- for i, c := range cleaned {
- if c == '.' {
- if dotAlreadySeen {
- return 0, unstable.NewParserError(b[i:i+1], "float can have at most one decimal point")
- }
- if !isDigit(cleaned[i-1]) {
- return 0, unstable.NewParserError(b[i-1:i+1], "float decimal point must be preceded by a digit")
- }
- if !isDigit(cleaned[i+1]) {
- return 0, unstable.NewParserError(b[i:i+2], "float decimal point must be followed by a digit")
- }
- dotAlreadySeen = true
- }
- }
-
- start := 0
- if cleaned[0] == '+' || cleaned[0] == '-' {
- start = 1
- }
- if cleaned[start] == '0' && isDigit(cleaned[start+1]) {
- return 0, unstable.NewParserError(b, "float integer part cannot have leading zeroes")
- }
-
- f, err := strconv.ParseFloat(string(cleaned), 64)
- if err != nil {
- return 0, unstable.NewParserError(b, "unable to parse float: %w", err)
- }
-
- return f, nil
-}
-
-func parseIntHex(b []byte) (int64, error) {
- cleaned, err := checkAndRemoveUnderscoresIntegers(b[2:])
- if err != nil {
- return 0, err
- }
-
- i, err := strconv.ParseInt(string(cleaned), 16, 64)
- if err != nil {
- return 0, unstable.NewParserError(b, "couldn't parse hexadecimal number: %w", err)
- }
-
- return i, nil
-}
-
-func parseIntOct(b []byte) (int64, error) {
- cleaned, err := checkAndRemoveUnderscoresIntegers(b[2:])
- if err != nil {
- return 0, err
- }
-
- i, err := strconv.ParseInt(string(cleaned), 8, 64)
- if err != nil {
- return 0, unstable.NewParserError(b, "couldn't parse octal number: %w", err)
- }
-
- return i, nil
-}
-
-func parseIntBin(b []byte) (int64, error) {
- cleaned, err := checkAndRemoveUnderscoresIntegers(b[2:])
- if err != nil {
- return 0, err
- }
-
- i, err := strconv.ParseInt(string(cleaned), 2, 64)
- if err != nil {
- return 0, unstable.NewParserError(b, "couldn't parse binary number: %w", err)
- }
-
- return i, nil
-}
-
-func isSign(b byte) bool {
- return b == '+' || b == '-'
-}
-
-func parseIntDec(b []byte) (int64, error) {
- cleaned, err := checkAndRemoveUnderscoresIntegers(b)
- if err != nil {
- return 0, err
- }
-
- startIdx := 0
-
- if isSign(cleaned[0]) {
- startIdx++
- }
-
- if len(cleaned) > startIdx+1 && cleaned[startIdx] == '0' {
- return 0, unstable.NewParserError(b, "leading zero not allowed on decimal number")
- }
-
- i, err := strconv.ParseInt(string(cleaned), 10, 64)
- if err != nil {
- return 0, unstable.NewParserError(b, "couldn't parse decimal number: %w", err)
- }
-
- return i, nil
-}
-
-func checkAndRemoveUnderscoresIntegers(b []byte) ([]byte, error) {
- start := 0
- if b[start] == '+' || b[start] == '-' {
- start++
- }
-
- if len(b) == start {
- return b, nil
- }
-
- if b[start] == '_' {
- return nil, unstable.NewParserError(b[start:start+1], "number cannot start with underscore")
- }
-
- if b[len(b)-1] == '_' {
- return nil, unstable.NewParserError(b[len(b)-1:], "number cannot end with underscore")
- }
-
- // fast path
- i := 0
- for ; i < len(b); i++ {
- if b[i] == '_' {
- break
- }
- }
- if i == len(b) {
- return b, nil
- }
-
- before := false
- cleaned := make([]byte, i, len(b))
- copy(cleaned, b)
-
- for i++; i < len(b); i++ {
- c := b[i]
- if c == '_' {
- if !before {
- return nil, unstable.NewParserError(b[i-1:i+1], "number must have at least one digit between underscores")
- }
- before = false
- } else {
- before = true
- cleaned = append(cleaned, c)
- }
- }
-
- return cleaned, nil
-}
-
-func checkAndRemoveUnderscoresFloats(b []byte) ([]byte, error) {
- if b[0] == '_' {
- return nil, unstable.NewParserError(b[0:1], "number cannot start with underscore")
- }
-
- if b[len(b)-1] == '_' {
- return nil, unstable.NewParserError(b[len(b)-1:], "number cannot end with underscore")
- }
-
- // fast path
- i := 0
- for ; i < len(b); i++ {
- if b[i] == '_' {
- break
- }
- }
- if i == len(b) {
- return b, nil
- }
-
- before := false
- cleaned := make([]byte, 0, len(b))
-
- for i := 0; i < len(b); i++ {
- c := b[i]
-
- switch c {
- case '_':
- if !before {
- return nil, unstable.NewParserError(b[i-1:i+1], "number must have at least one digit between underscores")
- }
- if i < len(b)-1 && (b[i+1] == 'e' || b[i+1] == 'E') {
- return nil, unstable.NewParserError(b[i+1:i+2], "cannot have underscore before exponent")
- }
- before = false
- case '+', '-':
- // signed exponents
- cleaned = append(cleaned, c)
- before = false
- case 'e', 'E':
- if i < len(b)-1 && b[i+1] == '_' {
- return nil, unstable.NewParserError(b[i+1:i+2], "cannot have underscore after exponent")
- }
- cleaned = append(cleaned, c)
- case '.':
- if i < len(b)-1 && b[i+1] == '_' {
- return nil, unstable.NewParserError(b[i+1:i+2], "cannot have underscore after decimal point")
- }
- if i > 0 && b[i-1] == '_' {
- return nil, unstable.NewParserError(b[i-1:i], "cannot have underscore before decimal point")
- }
- cleaned = append(cleaned, c)
- default:
- before = true
- cleaned = append(cleaned, c)
- }
- }
-
- return cleaned, nil
-}
-
-// isValidDate checks if a provided date is a date that exists.
-func isValidDate(year int, month int, day int) bool {
- return month > 0 && month < 13 && day > 0 && day <= daysIn(month, year)
-}
-
-// daysBefore[m] counts the number of days in a non-leap year
-// before month m begins. There is an entry for m=12, counting
-// the number of days before January of next year (365).
-var daysBefore = [...]int32{
- 0,
- 31,
- 31 + 28,
- 31 + 28 + 31,
- 31 + 28 + 31 + 30,
- 31 + 28 + 31 + 30 + 31,
- 31 + 28 + 31 + 30 + 31 + 30,
- 31 + 28 + 31 + 30 + 31 + 30 + 31,
- 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31,
- 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30,
- 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31,
- 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30,
- 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30 + 31,
-}
-
-func daysIn(m int, year int) int {
- if m == 2 && isLeap(year) {
- return 29
- }
- return int(daysBefore[m] - daysBefore[m-1])
-}
-
-func isLeap(year int) bool {
- return year%4 == 0 && (year%100 != 0 || year%400 == 0)
-}
-
-func isDigit(r byte) bool {
- return r >= '0' && r <= '9'
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/doc.go b/vendor/github.com/pelletier/go-toml/v2/doc.go
deleted file mode 100644
index b7bc599b..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/doc.go
+++ /dev/null
@@ -1,2 +0,0 @@
-// Package toml is a library to read and write TOML documents.
-package toml
diff --git a/vendor/github.com/pelletier/go-toml/v2/errors.go b/vendor/github.com/pelletier/go-toml/v2/errors.go
deleted file mode 100644
index 309733f1..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/errors.go
+++ /dev/null
@@ -1,252 +0,0 @@
-package toml
-
-import (
- "fmt"
- "strconv"
- "strings"
-
- "github.com/pelletier/go-toml/v2/internal/danger"
- "github.com/pelletier/go-toml/v2/unstable"
-)
-
-// DecodeError represents an error encountered during the parsing or decoding
-// of a TOML document.
-//
-// In addition to the error message, it contains the position in the document
-// where it happened, as well as a human-readable representation that shows
-// where the error occurred in the document.
-type DecodeError struct {
- message string
- line int
- column int
- key Key
-
- human string
-}
-
-// StrictMissingError occurs in a TOML document that does not have a
-// corresponding field in the target value. It contains all the missing fields
-// in Errors.
-//
-// Emitted by Decoder when DisallowUnknownFields() was called.
-type StrictMissingError struct {
- // One error per field that could not be found.
- Errors []DecodeError
-}
-
-// Error returns the canonical string for this error.
-func (s *StrictMissingError) Error() string {
- return "strict mode: fields in the document are missing in the target struct"
-}
-
-// String returns a human readable description of all errors.
-func (s *StrictMissingError) String() string {
- var buf strings.Builder
-
- for i, e := range s.Errors {
- if i > 0 {
- buf.WriteString("\n---\n")
- }
-
- buf.WriteString(e.String())
- }
-
- return buf.String()
-}
-
-type Key []string
-
-// Error returns the error message contained in the DecodeError.
-func (e *DecodeError) Error() string {
- return "toml: " + e.message
-}
-
-// String returns the human-readable contextualized error. This string is multi-line.
-func (e *DecodeError) String() string {
- return e.human
-}
-
-// Position returns the (line, column) pair indicating where the error
-// occurred in the document. Positions are 1-indexed.
-func (e *DecodeError) Position() (row int, column int) {
- return e.line, e.column
-}
-
-// Key that was being processed when the error occurred. The key is present only
-// if this DecodeError is part of a StrictMissingError.
-func (e *DecodeError) Key() Key {
- return e.key
-}
-
-// decodeErrorFromHighlight creates a DecodeError referencing a highlighted
-// range of bytes from document.
-//
-// highlight needs to be a sub-slice of document, or this function panics.
-//
-// The function copies all bytes used in DecodeError, so that document and
-// highlight can be freely deallocated.
-//
-//nolint:funlen
-func wrapDecodeError(document []byte, de *unstable.ParserError) *DecodeError {
- offset := danger.SubsliceOffset(document, de.Highlight)
-
- errMessage := de.Error()
- errLine, errColumn := positionAtEnd(document[:offset])
- before, after := linesOfContext(document, de.Highlight, offset, 3)
-
- var buf strings.Builder
-
- maxLine := errLine + len(after) - 1
- lineColumnWidth := len(strconv.Itoa(maxLine))
-
- // Write the lines of context strictly before the error.
- for i := len(before) - 1; i > 0; i-- {
- line := errLine - i
- buf.WriteString(formatLineNumber(line, lineColumnWidth))
- buf.WriteString("|")
-
- if len(before[i]) > 0 {
- buf.WriteString(" ")
- buf.Write(before[i])
- }
-
- buf.WriteRune('\n')
- }
-
- // Write the document line that contains the error.
-
- buf.WriteString(formatLineNumber(errLine, lineColumnWidth))
- buf.WriteString("| ")
-
- if len(before) > 0 {
- buf.Write(before[0])
- }
-
- buf.Write(de.Highlight)
-
- if len(after) > 0 {
- buf.Write(after[0])
- }
-
- buf.WriteRune('\n')
-
- // Write the line with the error message itself (so it does not have a line
- // number).
-
- buf.WriteString(strings.Repeat(" ", lineColumnWidth))
- buf.WriteString("| ")
-
- if len(before) > 0 {
- buf.WriteString(strings.Repeat(" ", len(before[0])))
- }
-
- buf.WriteString(strings.Repeat("~", len(de.Highlight)))
-
- if len(errMessage) > 0 {
- buf.WriteString(" ")
- buf.WriteString(errMessage)
- }
-
- // Write the lines of context strictly after the error.
-
- for i := 1; i < len(after); i++ {
- buf.WriteRune('\n')
- line := errLine + i
- buf.WriteString(formatLineNumber(line, lineColumnWidth))
- buf.WriteString("|")
-
- if len(after[i]) > 0 {
- buf.WriteString(" ")
- buf.Write(after[i])
- }
- }
-
- return &DecodeError{
- message: errMessage,
- line: errLine,
- column: errColumn,
- key: de.Key,
- human: buf.String(),
- }
-}
-
-func formatLineNumber(line int, width int) string {
- format := "%" + strconv.Itoa(width) + "d"
-
- return fmt.Sprintf(format, line)
-}
-
-func linesOfContext(document []byte, highlight []byte, offset int, linesAround int) ([][]byte, [][]byte) {
- return beforeLines(document, offset, linesAround), afterLines(document, highlight, offset, linesAround)
-}
-
-func beforeLines(document []byte, offset int, linesAround int) [][]byte {
- var beforeLines [][]byte
-
- // Walk the document backward from the highlight to find previous lines
- // of context.
- rest := document[:offset]
-backward:
- for o := len(rest) - 1; o >= 0 && len(beforeLines) <= linesAround && len(rest) > 0; {
- switch {
- case rest[o] == '\n':
- // handle individual lines
- beforeLines = append(beforeLines, rest[o+1:])
- rest = rest[:o]
- o = len(rest) - 1
- case o == 0:
- // add the first line only if it's non-empty
- beforeLines = append(beforeLines, rest)
-
- break backward
- default:
- o--
- }
- }
-
- return beforeLines
-}
-
-func afterLines(document []byte, highlight []byte, offset int, linesAround int) [][]byte {
- var afterLines [][]byte
-
- // Walk the document forward from the highlight to find the following
- // lines of context.
- rest := document[offset+len(highlight):]
-forward:
- for o := 0; o < len(rest) && len(afterLines) <= linesAround; {
- switch {
- case rest[o] == '\n':
- // handle individual lines
- afterLines = append(afterLines, rest[:o])
- rest = rest[o+1:]
- o = 0
-
- case o == len(rest)-1:
- // add last line only if it's non-empty
- afterLines = append(afterLines, rest)
-
- break forward
- default:
- o++
- }
- }
-
- return afterLines
-}
-
-func positionAtEnd(b []byte) (row int, column int) {
- row = 1
- column = 1
-
- for _, c := range b {
- if c == '\n' {
- row++
- column = 1
- } else {
- column++
- }
- }
-
- return
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/characters/ascii.go b/vendor/github.com/pelletier/go-toml/v2/internal/characters/ascii.go
deleted file mode 100644
index 80f698db..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/characters/ascii.go
+++ /dev/null
@@ -1,42 +0,0 @@
-package characters
-
-var invalidAsciiTable = [256]bool{
- 0x00: true,
- 0x01: true,
- 0x02: true,
- 0x03: true,
- 0x04: true,
- 0x05: true,
- 0x06: true,
- 0x07: true,
- 0x08: true,
- // 0x09 TAB
- // 0x0A LF
- 0x0B: true,
- 0x0C: true,
- // 0x0D CR
- 0x0E: true,
- 0x0F: true,
- 0x10: true,
- 0x11: true,
- 0x12: true,
- 0x13: true,
- 0x14: true,
- 0x15: true,
- 0x16: true,
- 0x17: true,
- 0x18: true,
- 0x19: true,
- 0x1A: true,
- 0x1B: true,
- 0x1C: true,
- 0x1D: true,
- 0x1E: true,
- 0x1F: true,
- // 0x20 - 0x7E Printable ASCII characters
- 0x7F: true,
-}
-
-func InvalidAscii(b byte) bool {
- return invalidAsciiTable[b]
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/characters/utf8.go b/vendor/github.com/pelletier/go-toml/v2/internal/characters/utf8.go
deleted file mode 100644
index db4f45ac..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/characters/utf8.go
+++ /dev/null
@@ -1,199 +0,0 @@
-package characters
-
-import (
- "unicode/utf8"
-)
-
-type utf8Err struct {
- Index int
- Size int
-}
-
-func (u utf8Err) Zero() bool {
- return u.Size == 0
-}
-
-// Verified that a given string is only made of valid UTF-8 characters allowed
-// by the TOML spec:
-//
-// Any Unicode character may be used except those that must be escaped:
-// quotation mark, backslash, and the control characters other than tab (U+0000
-// to U+0008, U+000A to U+001F, U+007F).
-//
-// It is a copy of the Go 1.17 utf8.Valid implementation, tweaked to exit early
-// when a character is not allowed.
-//
-// The returned utf8Err is Zero() if the string is valid, or contains the byte
-// index and size of the invalid character.
-//
-// quotation mark => already checked
-// backslash => already checked
-// 0-0x8 => invalid
-// 0x9 => tab, ok
-// 0xA - 0x1F => invalid
-// 0x7F => invalid
-func Utf8TomlValidAlreadyEscaped(p []byte) (err utf8Err) {
- // Fast path. Check for and skip 8 bytes of ASCII characters per iteration.
- offset := 0
- for len(p) >= 8 {
- // Combining two 32 bit loads allows the same code to be used
- // for 32 and 64 bit platforms.
- // The compiler can generate a 32bit load for first32 and second32
- // on many platforms. See test/codegen/memcombine.go.
- first32 := uint32(p[0]) | uint32(p[1])<<8 | uint32(p[2])<<16 | uint32(p[3])<<24
- second32 := uint32(p[4]) | uint32(p[5])<<8 | uint32(p[6])<<16 | uint32(p[7])<<24
- if (first32|second32)&0x80808080 != 0 {
- // Found a non ASCII byte (>= RuneSelf).
- break
- }
-
- for i, b := range p[:8] {
- if InvalidAscii(b) {
- err.Index = offset + i
- err.Size = 1
- return
- }
- }
-
- p = p[8:]
- offset += 8
- }
- n := len(p)
- for i := 0; i < n; {
- pi := p[i]
- if pi < utf8.RuneSelf {
- if InvalidAscii(pi) {
- err.Index = offset + i
- err.Size = 1
- return
- }
- i++
- continue
- }
- x := first[pi]
- if x == xx {
- // Illegal starter byte.
- err.Index = offset + i
- err.Size = 1
- return
- }
- size := int(x & 7)
- if i+size > n {
- // Short or invalid.
- err.Index = offset + i
- err.Size = n - i
- return
- }
- accept := acceptRanges[x>>4]
- if c := p[i+1]; c < accept.lo || accept.hi < c {
- err.Index = offset + i
- err.Size = 2
- return
- } else if size == 2 {
- } else if c := p[i+2]; c < locb || hicb < c {
- err.Index = offset + i
- err.Size = 3
- return
- } else if size == 3 {
- } else if c := p[i+3]; c < locb || hicb < c {
- err.Index = offset + i
- err.Size = 4
- return
- }
- i += size
- }
- return
-}
-
-// Return the size of the next rune if valid, 0 otherwise.
-func Utf8ValidNext(p []byte) int {
- c := p[0]
-
- if c < utf8.RuneSelf {
- if InvalidAscii(c) {
- return 0
- }
- return 1
- }
-
- x := first[c]
- if x == xx {
- // Illegal starter byte.
- return 0
- }
- size := int(x & 7)
- if size > len(p) {
- // Short or invalid.
- return 0
- }
- accept := acceptRanges[x>>4]
- if c := p[1]; c < accept.lo || accept.hi < c {
- return 0
- } else if size == 2 {
- } else if c := p[2]; c < locb || hicb < c {
- return 0
- } else if size == 3 {
- } else if c := p[3]; c < locb || hicb < c {
- return 0
- }
-
- return size
-}
-
-// acceptRange gives the range of valid values for the second byte in a UTF-8
-// sequence.
-type acceptRange struct {
- lo uint8 // lowest value for second byte.
- hi uint8 // highest value for second byte.
-}
-
-// acceptRanges has size 16 to avoid bounds checks in the code that uses it.
-var acceptRanges = [16]acceptRange{
- 0: {locb, hicb},
- 1: {0xA0, hicb},
- 2: {locb, 0x9F},
- 3: {0x90, hicb},
- 4: {locb, 0x8F},
-}
-
-// first is information about the first byte in a UTF-8 sequence.
-var first = [256]uint8{
- // 1 2 3 4 5 6 7 8 9 A B C D E F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x00-0x0F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x10-0x1F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x20-0x2F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x30-0x3F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x40-0x4F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x50-0x5F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x60-0x6F
- as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, as, // 0x70-0x7F
- // 1 2 3 4 5 6 7 8 9 A B C D E F
- xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, // 0x80-0x8F
- xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, // 0x90-0x9F
- xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, // 0xA0-0xAF
- xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, // 0xB0-0xBF
- xx, xx, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, // 0xC0-0xCF
- s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, s1, // 0xD0-0xDF
- s2, s3, s3, s3, s3, s3, s3, s3, s3, s3, s3, s3, s3, s4, s3, s3, // 0xE0-0xEF
- s5, s6, s6, s6, s7, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, xx, // 0xF0-0xFF
-}
-
-const (
- // The default lowest and highest continuation byte.
- locb = 0b10000000
- hicb = 0b10111111
-
- // These names of these constants are chosen to give nice alignment in the
- // table below. The first nibble is an index into acceptRanges or F for
- // special one-byte cases. The second nibble is the Rune length or the
- // Status for the special one-byte case.
- xx = 0xF1 // invalid: size 1
- as = 0xF0 // ASCII: size 1
- s1 = 0x02 // accept 0, size 2
- s2 = 0x13 // accept 1, size 3
- s3 = 0x03 // accept 0, size 3
- s4 = 0x23 // accept 2, size 3
- s5 = 0x34 // accept 3, size 4
- s6 = 0x04 // accept 0, size 4
- s7 = 0x44 // accept 4, size 4
-)
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/danger/danger.go b/vendor/github.com/pelletier/go-toml/v2/internal/danger/danger.go
deleted file mode 100644
index e38e1131..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/danger/danger.go
+++ /dev/null
@@ -1,65 +0,0 @@
-package danger
-
-import (
- "fmt"
- "reflect"
- "unsafe"
-)
-
-const maxInt = uintptr(int(^uint(0) >> 1))
-
-func SubsliceOffset(data []byte, subslice []byte) int {
- datap := (*reflect.SliceHeader)(unsafe.Pointer(&data))
- hlp := (*reflect.SliceHeader)(unsafe.Pointer(&subslice))
-
- if hlp.Data < datap.Data {
- panic(fmt.Errorf("subslice address (%d) is before data address (%d)", hlp.Data, datap.Data))
- }
- offset := hlp.Data - datap.Data
-
- if offset > maxInt {
- panic(fmt.Errorf("slice offset larger than int (%d)", offset))
- }
-
- intoffset := int(offset)
-
- if intoffset > datap.Len {
- panic(fmt.Errorf("slice offset (%d) is farther than data length (%d)", intoffset, datap.Len))
- }
-
- if intoffset+hlp.Len > datap.Len {
- panic(fmt.Errorf("slice ends (%d+%d) is farther than data length (%d)", intoffset, hlp.Len, datap.Len))
- }
-
- return intoffset
-}
-
-func BytesRange(start []byte, end []byte) []byte {
- if start == nil || end == nil {
- panic("cannot call BytesRange with nil")
- }
- startp := (*reflect.SliceHeader)(unsafe.Pointer(&start))
- endp := (*reflect.SliceHeader)(unsafe.Pointer(&end))
-
- if startp.Data > endp.Data {
- panic(fmt.Errorf("start pointer address (%d) is after end pointer address (%d)", startp.Data, endp.Data))
- }
-
- l := startp.Len
- endLen := int(endp.Data-startp.Data) + endp.Len
- if endLen > l {
- l = endLen
- }
-
- if l > startp.Cap {
- panic(fmt.Errorf("range length is larger than capacity"))
- }
-
- return start[:l]
-}
-
-func Stride(ptr unsafe.Pointer, size uintptr, offset int) unsafe.Pointer {
- // TODO: replace with unsafe.Add when Go 1.17 is released
- // https://github.com/golang/go/issues/40481
- return unsafe.Pointer(uintptr(ptr) + uintptr(int(size)*offset))
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/danger/typeid.go b/vendor/github.com/pelletier/go-toml/v2/internal/danger/typeid.go
deleted file mode 100644
index 9d41c28a..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/danger/typeid.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package danger
-
-import (
- "reflect"
- "unsafe"
-)
-
-// typeID is used as key in encoder and decoder caches to enable using
-// the optimize runtime.mapaccess2_fast64 function instead of the more
-// expensive lookup if we were to use reflect.Type as map key.
-//
-// typeID holds the pointer to the reflect.Type value, which is unique
-// in the program.
-//
-// https://github.com/segmentio/encoding/blob/master/json/codec.go#L59-L61
-type TypeID unsafe.Pointer
-
-func MakeTypeID(t reflect.Type) TypeID {
- // reflect.Type has the fields:
- // typ unsafe.Pointer
- // ptr unsafe.Pointer
- return TypeID((*[2]unsafe.Pointer)(unsafe.Pointer(&t))[1])
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/tracker/key.go b/vendor/github.com/pelletier/go-toml/v2/internal/tracker/key.go
deleted file mode 100644
index 149b17f5..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/tracker/key.go
+++ /dev/null
@@ -1,48 +0,0 @@
-package tracker
-
-import "github.com/pelletier/go-toml/v2/unstable"
-
-// KeyTracker is a tracker that keeps track of the current Key as the AST is
-// walked.
-type KeyTracker struct {
- k []string
-}
-
-// UpdateTable sets the state of the tracker with the AST table node.
-func (t *KeyTracker) UpdateTable(node *unstable.Node) {
- t.reset()
- t.Push(node)
-}
-
-// UpdateArrayTable sets the state of the tracker with the AST array table node.
-func (t *KeyTracker) UpdateArrayTable(node *unstable.Node) {
- t.reset()
- t.Push(node)
-}
-
-// Push the given key on the stack.
-func (t *KeyTracker) Push(node *unstable.Node) {
- it := node.Key()
- for it.Next() {
- t.k = append(t.k, string(it.Node().Data))
- }
-}
-
-// Pop key from stack.
-func (t *KeyTracker) Pop(node *unstable.Node) {
- it := node.Key()
- for it.Next() {
- t.k = t.k[:len(t.k)-1]
- }
-}
-
-// Key returns the current key
-func (t *KeyTracker) Key() []string {
- k := make([]string, len(t.k))
- copy(k, t.k)
- return k
-}
-
-func (t *KeyTracker) reset() {
- t.k = t.k[:0]
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/tracker/seen.go b/vendor/github.com/pelletier/go-toml/v2/internal/tracker/seen.go
deleted file mode 100644
index 40e23f83..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/tracker/seen.go
+++ /dev/null
@@ -1,356 +0,0 @@
-package tracker
-
-import (
- "bytes"
- "fmt"
- "sync"
-
- "github.com/pelletier/go-toml/v2/unstable"
-)
-
-type keyKind uint8
-
-const (
- invalidKind keyKind = iota
- valueKind
- tableKind
- arrayTableKind
-)
-
-func (k keyKind) String() string {
- switch k {
- case invalidKind:
- return "invalid"
- case valueKind:
- return "value"
- case tableKind:
- return "table"
- case arrayTableKind:
- return "array table"
- }
- panic("missing keyKind string mapping")
-}
-
-// SeenTracker tracks which keys have been seen with which TOML type to flag
-// duplicates and mismatches according to the spec.
-//
-// Each node in the visited tree is represented by an entry. Each entry has an
-// identifier, which is provided by a counter. Entries are stored in the array
-// entries. As new nodes are discovered (referenced for the first time in the
-// TOML document), entries are created and appended to the array. An entry
-// points to its parent using its id.
-//
-// To find whether a given key (sequence of []byte) has already been visited,
-// the entries are linearly searched, looking for one with the right name and
-// parent id.
-//
-// Given that all keys appear in the document after their parent, it is
-// guaranteed that all descendants of a node are stored after the node, this
-// speeds up the search process.
-//
-// When encountering [[array tables]], the descendants of that node are removed
-// to allow that branch of the tree to be "rediscovered". To maintain the
-// invariant above, the deletion process needs to keep the order of entries.
-// This results in more copies in that case.
-type SeenTracker struct {
- entries []entry
- currentIdx int
-}
-
-var pool sync.Pool
-
-func (s *SeenTracker) reset() {
- // Always contains a root element at index 0.
- s.currentIdx = 0
- if len(s.entries) == 0 {
- s.entries = make([]entry, 1, 2)
- } else {
- s.entries = s.entries[:1]
- }
- s.entries[0].child = -1
- s.entries[0].next = -1
-}
-
-type entry struct {
- // Use -1 to indicate no child or no sibling.
- child int
- next int
-
- name []byte
- kind keyKind
- explicit bool
- kv bool
-}
-
-// Find the index of the child of parentIdx with key k. Returns -1 if
-// it does not exist.
-func (s *SeenTracker) find(parentIdx int, k []byte) int {
- for i := s.entries[parentIdx].child; i >= 0; i = s.entries[i].next {
- if bytes.Equal(s.entries[i].name, k) {
- return i
- }
- }
- return -1
-}
-
-// Remove all descendants of node at position idx.
-func (s *SeenTracker) clear(idx int) {
- if idx >= len(s.entries) {
- return
- }
-
- for i := s.entries[idx].child; i >= 0; {
- next := s.entries[i].next
- n := s.entries[0].next
- s.entries[0].next = i
- s.entries[i].next = n
- s.entries[i].name = nil
- s.clear(i)
- i = next
- }
-
- s.entries[idx].child = -1
-}
-
-func (s *SeenTracker) create(parentIdx int, name []byte, kind keyKind, explicit bool, kv bool) int {
- e := entry{
- child: -1,
- next: s.entries[parentIdx].child,
-
- name: name,
- kind: kind,
- explicit: explicit,
- kv: kv,
- }
- var idx int
- if s.entries[0].next >= 0 {
- idx = s.entries[0].next
- s.entries[0].next = s.entries[idx].next
- s.entries[idx] = e
- } else {
- idx = len(s.entries)
- s.entries = append(s.entries, e)
- }
-
- s.entries[parentIdx].child = idx
-
- return idx
-}
-
-func (s *SeenTracker) setExplicitFlag(parentIdx int) {
- for i := s.entries[parentIdx].child; i >= 0; i = s.entries[i].next {
- if s.entries[i].kv {
- s.entries[i].explicit = true
- s.entries[i].kv = false
- }
- s.setExplicitFlag(i)
- }
-}
-
-// CheckExpression takes a top-level node and checks that it does not contain
-// keys that have been seen in previous calls, and validates that types are
-// consistent.
-func (s *SeenTracker) CheckExpression(node *unstable.Node) error {
- if s.entries == nil {
- s.reset()
- }
- switch node.Kind {
- case unstable.KeyValue:
- return s.checkKeyValue(node)
- case unstable.Table:
- return s.checkTable(node)
- case unstable.ArrayTable:
- return s.checkArrayTable(node)
- default:
- panic(fmt.Errorf("this should not be a top level node type: %s", node.Kind))
- }
-}
-
-func (s *SeenTracker) checkTable(node *unstable.Node) error {
- if s.currentIdx >= 0 {
- s.setExplicitFlag(s.currentIdx)
- }
-
- it := node.Key()
-
- parentIdx := 0
-
- // This code is duplicated in checkArrayTable. This is because factoring
- // it in a function requires to copy the iterator, or allocate it to the
- // heap, which is not cheap.
- for it.Next() {
- if it.IsLast() {
- break
- }
-
- k := it.Node().Data
-
- idx := s.find(parentIdx, k)
-
- if idx < 0 {
- idx = s.create(parentIdx, k, tableKind, false, false)
- } else {
- entry := s.entries[idx]
- if entry.kind == valueKind {
- return fmt.Errorf("toml: expected %s to be a table, not a %s", string(k), entry.kind)
- }
- }
- parentIdx = idx
- }
-
- k := it.Node().Data
- idx := s.find(parentIdx, k)
-
- if idx >= 0 {
- kind := s.entries[idx].kind
- if kind != tableKind {
- return fmt.Errorf("toml: key %s should be a table, not a %s", string(k), kind)
- }
- if s.entries[idx].explicit {
- return fmt.Errorf("toml: table %s already exists", string(k))
- }
- s.entries[idx].explicit = true
- } else {
- idx = s.create(parentIdx, k, tableKind, true, false)
- }
-
- s.currentIdx = idx
-
- return nil
-}
-
-func (s *SeenTracker) checkArrayTable(node *unstable.Node) error {
- if s.currentIdx >= 0 {
- s.setExplicitFlag(s.currentIdx)
- }
-
- it := node.Key()
-
- parentIdx := 0
-
- for it.Next() {
- if it.IsLast() {
- break
- }
-
- k := it.Node().Data
-
- idx := s.find(parentIdx, k)
-
- if idx < 0 {
- idx = s.create(parentIdx, k, tableKind, false, false)
- } else {
- entry := s.entries[idx]
- if entry.kind == valueKind {
- return fmt.Errorf("toml: expected %s to be a table, not a %s", string(k), entry.kind)
- }
- }
-
- parentIdx = idx
- }
-
- k := it.Node().Data
- idx := s.find(parentIdx, k)
-
- if idx >= 0 {
- kind := s.entries[idx].kind
- if kind != arrayTableKind {
- return fmt.Errorf("toml: key %s already exists as a %s, but should be an array table", kind, string(k))
- }
- s.clear(idx)
- } else {
- idx = s.create(parentIdx, k, arrayTableKind, true, false)
- }
-
- s.currentIdx = idx
-
- return nil
-}
-
-func (s *SeenTracker) checkKeyValue(node *unstable.Node) error {
- parentIdx := s.currentIdx
- it := node.Key()
-
- for it.Next() {
- k := it.Node().Data
-
- idx := s.find(parentIdx, k)
-
- if idx < 0 {
- idx = s.create(parentIdx, k, tableKind, false, true)
- } else {
- entry := s.entries[idx]
- if it.IsLast() {
- return fmt.Errorf("toml: key %s is already defined", string(k))
- } else if entry.kind != tableKind {
- return fmt.Errorf("toml: expected %s to be a table, not a %s", string(k), entry.kind)
- } else if entry.explicit {
- return fmt.Errorf("toml: cannot redefine table %s that has already been explicitly defined", string(k))
- }
- }
-
- parentIdx = idx
- }
-
- s.entries[parentIdx].kind = valueKind
-
- value := node.Value()
-
- switch value.Kind {
- case unstable.InlineTable:
- return s.checkInlineTable(value)
- case unstable.Array:
- return s.checkArray(value)
- }
-
- return nil
-}
-
-func (s *SeenTracker) checkArray(node *unstable.Node) error {
- it := node.Children()
- for it.Next() {
- n := it.Node()
- switch n.Kind {
- case unstable.InlineTable:
- err := s.checkInlineTable(n)
- if err != nil {
- return err
- }
- case unstable.Array:
- err := s.checkArray(n)
- if err != nil {
- return err
- }
- }
- }
- return nil
-}
-
-func (s *SeenTracker) checkInlineTable(node *unstable.Node) error {
- if pool.New == nil {
- pool.New = func() interface{} {
- return &SeenTracker{}
- }
- }
-
- s = pool.Get().(*SeenTracker)
- s.reset()
-
- it := node.Children()
- for it.Next() {
- n := it.Node()
- err := s.checkKeyValue(n)
- if err != nil {
- return err
- }
- }
-
- // As inline tables are self-contained, the tracker does not
- // need to retain the details of what they contain. The
- // keyValue element that creates the inline table is kept to
- // mark the presence of the inline table and prevent
- // redefinition of its keys: check* functions cannot walk into
- // a value.
- pool.Put(s)
- return nil
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/internal/tracker/tracker.go b/vendor/github.com/pelletier/go-toml/v2/internal/tracker/tracker.go
deleted file mode 100644
index bf031739..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/internal/tracker/tracker.go
+++ /dev/null
@@ -1 +0,0 @@
-package tracker
diff --git a/vendor/github.com/pelletier/go-toml/v2/localtime.go b/vendor/github.com/pelletier/go-toml/v2/localtime.go
deleted file mode 100644
index a856bfdb..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/localtime.go
+++ /dev/null
@@ -1,122 +0,0 @@
-package toml
-
-import (
- "fmt"
- "strings"
- "time"
-
- "github.com/pelletier/go-toml/v2/unstable"
-)
-
-// LocalDate represents a calendar day in no specific timezone.
-type LocalDate struct {
- Year int
- Month int
- Day int
-}
-
-// AsTime converts d into a specific time instance at midnight in zone.
-func (d LocalDate) AsTime(zone *time.Location) time.Time {
- return time.Date(d.Year, time.Month(d.Month), d.Day, 0, 0, 0, 0, zone)
-}
-
-// String returns RFC 3339 representation of d.
-func (d LocalDate) String() string {
- return fmt.Sprintf("%04d-%02d-%02d", d.Year, d.Month, d.Day)
-}
-
-// MarshalText returns RFC 3339 representation of d.
-func (d LocalDate) MarshalText() ([]byte, error) {
- return []byte(d.String()), nil
-}
-
-// UnmarshalText parses b using RFC 3339 to fill d.
-func (d *LocalDate) UnmarshalText(b []byte) error {
- res, err := parseLocalDate(b)
- if err != nil {
- return err
- }
- *d = res
- return nil
-}
-
-// LocalTime represents a time of day of no specific day in no specific
-// timezone.
-type LocalTime struct {
- Hour int // Hour of the day: [0; 24[
- Minute int // Minute of the hour: [0; 60[
- Second int // Second of the minute: [0; 60[
- Nanosecond int // Nanoseconds within the second: [0, 1000000000[
- Precision int // Number of digits to display for Nanosecond.
-}
-
-// String returns RFC 3339 representation of d.
-// If d.Nanosecond and d.Precision are zero, the time won't have a nanosecond
-// component. If d.Nanosecond > 0 but d.Precision = 0, then the minimum number
-// of digits for nanoseconds is provided.
-func (d LocalTime) String() string {
- s := fmt.Sprintf("%02d:%02d:%02d", d.Hour, d.Minute, d.Second)
-
- if d.Precision > 0 {
- s += fmt.Sprintf(".%09d", d.Nanosecond)[:d.Precision+1]
- } else if d.Nanosecond > 0 {
- // Nanoseconds are specified, but precision is not provided. Use the
- // minimum.
- s += strings.Trim(fmt.Sprintf(".%09d", d.Nanosecond), "0")
- }
-
- return s
-}
-
-// MarshalText returns RFC 3339 representation of d.
-func (d LocalTime) MarshalText() ([]byte, error) {
- return []byte(d.String()), nil
-}
-
-// UnmarshalText parses b using RFC 3339 to fill d.
-func (d *LocalTime) UnmarshalText(b []byte) error {
- res, left, err := parseLocalTime(b)
- if err == nil && len(left) != 0 {
- err = unstable.NewParserError(left, "extra characters")
- }
- if err != nil {
- return err
- }
- *d = res
- return nil
-}
-
-// LocalDateTime represents a time of a specific day in no specific timezone.
-type LocalDateTime struct {
- LocalDate
- LocalTime
-}
-
-// AsTime converts d into a specific time instance in zone.
-func (d LocalDateTime) AsTime(zone *time.Location) time.Time {
- return time.Date(d.Year, time.Month(d.Month), d.Day, d.Hour, d.Minute, d.Second, d.Nanosecond, zone)
-}
-
-// String returns RFC 3339 representation of d.
-func (d LocalDateTime) String() string {
- return d.LocalDate.String() + "T" + d.LocalTime.String()
-}
-
-// MarshalText returns RFC 3339 representation of d.
-func (d LocalDateTime) MarshalText() ([]byte, error) {
- return []byte(d.String()), nil
-}
-
-// UnmarshalText parses b using RFC 3339 to fill d.
-func (d *LocalDateTime) UnmarshalText(data []byte) error {
- res, left, err := parseLocalDateTime(data)
- if err == nil && len(left) != 0 {
- err = unstable.NewParserError(left, "extra characters")
- }
- if err != nil {
- return err
- }
-
- *d = res
- return nil
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/marshaler.go b/vendor/github.com/pelletier/go-toml/v2/marshaler.go
deleted file mode 100644
index 07aceb90..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/marshaler.go
+++ /dev/null
@@ -1,1042 +0,0 @@
-package toml
-
-import (
- "bytes"
- "encoding"
- "fmt"
- "io"
- "math"
- "reflect"
- "sort"
- "strconv"
- "strings"
- "time"
- "unicode"
-
- "github.com/pelletier/go-toml/v2/internal/characters"
-)
-
-// Marshal serializes a Go value as a TOML document.
-//
-// It is a shortcut for Encoder.Encode() with the default options.
-func Marshal(v interface{}) ([]byte, error) {
- var buf bytes.Buffer
- enc := NewEncoder(&buf)
-
- err := enc.Encode(v)
- if err != nil {
- return nil, err
- }
-
- return buf.Bytes(), nil
-}
-
-// Encoder writes a TOML document to an output stream.
-type Encoder struct {
- // output
- w io.Writer
-
- // global settings
- tablesInline bool
- arraysMultiline bool
- indentSymbol string
- indentTables bool
-}
-
-// NewEncoder returns a new Encoder that writes to w.
-func NewEncoder(w io.Writer) *Encoder {
- return &Encoder{
- w: w,
- indentSymbol: " ",
- }
-}
-
-// SetTablesInline forces the encoder to emit all tables inline.
-//
-// This behavior can be controlled on an individual struct field basis with the
-// inline tag:
-//
-// MyField `toml:",inline"`
-func (enc *Encoder) SetTablesInline(inline bool) *Encoder {
- enc.tablesInline = inline
- return enc
-}
-
-// SetArraysMultiline forces the encoder to emit all arrays with one element per
-// line.
-//
-// This behavior can be controlled on an individual struct field basis with the multiline tag:
-//
-// MyField `multiline:"true"`
-func (enc *Encoder) SetArraysMultiline(multiline bool) *Encoder {
- enc.arraysMultiline = multiline
- return enc
-}
-
-// SetIndentSymbol defines the string that should be used for indentation. The
-// provided string is repeated for each indentation level. Defaults to two
-// spaces.
-func (enc *Encoder) SetIndentSymbol(s string) *Encoder {
- enc.indentSymbol = s
- return enc
-}
-
-// SetIndentTables forces the encoder to intent tables and array tables.
-func (enc *Encoder) SetIndentTables(indent bool) *Encoder {
- enc.indentTables = indent
- return enc
-}
-
-// Encode writes a TOML representation of v to the stream.
-//
-// If v cannot be represented to TOML it returns an error.
-//
-// # Encoding rules
-//
-// A top level slice containing only maps or structs is encoded as [[table
-// array]].
-//
-// All slices not matching rule 1 are encoded as [array]. As a result, any map
-// or struct they contain is encoded as an {inline table}.
-//
-// Nil interfaces and nil pointers are not supported.
-//
-// Keys in key-values always have one part.
-//
-// Intermediate tables are always printed.
-//
-// By default, strings are encoded as literal string, unless they contain either
-// a newline character or a single quote. In that case they are emitted as
-// quoted strings.
-//
-// Unsigned integers larger than math.MaxInt64 cannot be encoded. Doing so
-// results in an error. This rule exists because the TOML specification only
-// requires parsers to support at least the 64 bits integer range. Allowing
-// larger numbers would create non-standard TOML documents, which may not be
-// readable (at best) by other implementations. To encode such numbers, a
-// solution is a custom type that implements encoding.TextMarshaler.
-//
-// When encoding structs, fields are encoded in order of definition, with their
-// exact name.
-//
-// Tables and array tables are separated by empty lines. However, consecutive
-// subtables definitions are not. For example:
-//
-// [top1]
-//
-// [top2]
-// [top2.child1]
-//
-// [[array]]
-//
-// [[array]]
-// [array.child2]
-//
-// # Struct tags
-//
-// The encoding of each public struct field can be customized by the format
-// string in the "toml" key of the struct field's tag. This follows
-// encoding/json's convention. The format string starts with the name of the
-// field, optionally followed by a comma-separated list of options. The name may
-// be empty in order to provide options without overriding the default name.
-//
-// The "multiline" option emits strings as quoted multi-line TOML strings. It
-// has no effect on fields that would not be encoded as strings.
-//
-// The "inline" option turns fields that would be emitted as tables into inline
-// tables instead. It has no effect on other fields.
-//
-// The "omitempty" option prevents empty values or groups from being emitted.
-//
-// In addition to the "toml" tag struct tag, a "comment" tag can be used to emit
-// a TOML comment before the value being annotated. Comments are ignored inside
-// inline tables. For array tables, the comment is only present before the first
-// element of the array.
-func (enc *Encoder) Encode(v interface{}) error {
- var (
- b []byte
- ctx encoderCtx
- )
-
- ctx.inline = enc.tablesInline
-
- if v == nil {
- return fmt.Errorf("toml: cannot encode a nil interface")
- }
-
- b, err := enc.encode(b, ctx, reflect.ValueOf(v))
- if err != nil {
- return err
- }
-
- _, err = enc.w.Write(b)
- if err != nil {
- return fmt.Errorf("toml: cannot write: %w", err)
- }
-
- return nil
-}
-
-type valueOptions struct {
- multiline bool
- omitempty bool
- comment string
-}
-
-type encoderCtx struct {
- // Current top-level key.
- parentKey []string
-
- // Key that should be used for a KV.
- key string
- // Extra flag to account for the empty string
- hasKey bool
-
- // Set to true to indicate that the encoder is inside a KV, so that all
- // tables need to be inlined.
- insideKv bool
-
- // Set to true to skip the first table header in an array table.
- skipTableHeader bool
-
- // Should the next table be encoded as inline
- inline bool
-
- // Indentation level
- indent int
-
- // Options coming from struct tags
- options valueOptions
-}
-
-func (ctx *encoderCtx) shiftKey() {
- if ctx.hasKey {
- ctx.parentKey = append(ctx.parentKey, ctx.key)
- ctx.clearKey()
- }
-}
-
-func (ctx *encoderCtx) setKey(k string) {
- ctx.key = k
- ctx.hasKey = true
-}
-
-func (ctx *encoderCtx) clearKey() {
- ctx.key = ""
- ctx.hasKey = false
-}
-
-func (ctx *encoderCtx) isRoot() bool {
- return len(ctx.parentKey) == 0 && !ctx.hasKey
-}
-
-func (enc *Encoder) encode(b []byte, ctx encoderCtx, v reflect.Value) ([]byte, error) {
- i := v.Interface()
-
- switch x := i.(type) {
- case time.Time:
- if x.Nanosecond() > 0 {
- return x.AppendFormat(b, time.RFC3339Nano), nil
- }
- return x.AppendFormat(b, time.RFC3339), nil
- case LocalTime:
- return append(b, x.String()...), nil
- case LocalDate:
- return append(b, x.String()...), nil
- case LocalDateTime:
- return append(b, x.String()...), nil
- }
-
- hasTextMarshaler := v.Type().Implements(textMarshalerType)
- if hasTextMarshaler || (v.CanAddr() && reflect.PtrTo(v.Type()).Implements(textMarshalerType)) {
- if !hasTextMarshaler {
- v = v.Addr()
- }
-
- if ctx.isRoot() {
- return nil, fmt.Errorf("toml: type %s implementing the TextMarshaler interface cannot be a root element", v.Type())
- }
-
- text, err := v.Interface().(encoding.TextMarshaler).MarshalText()
- if err != nil {
- return nil, err
- }
-
- b = enc.encodeString(b, string(text), ctx.options)
-
- return b, nil
- }
-
- switch v.Kind() {
- // containers
- case reflect.Map:
- return enc.encodeMap(b, ctx, v)
- case reflect.Struct:
- return enc.encodeStruct(b, ctx, v)
- case reflect.Slice:
- return enc.encodeSlice(b, ctx, v)
- case reflect.Interface:
- if v.IsNil() {
- return nil, fmt.Errorf("toml: encoding a nil interface is not supported")
- }
-
- return enc.encode(b, ctx, v.Elem())
- case reflect.Ptr:
- if v.IsNil() {
- return enc.encode(b, ctx, reflect.Zero(v.Type().Elem()))
- }
-
- return enc.encode(b, ctx, v.Elem())
-
- // values
- case reflect.String:
- b = enc.encodeString(b, v.String(), ctx.options)
- case reflect.Float32:
- f := v.Float()
-
- if math.IsNaN(f) {
- b = append(b, "nan"...)
- } else if f > math.MaxFloat32 {
- b = append(b, "inf"...)
- } else if f < -math.MaxFloat32 {
- b = append(b, "-inf"...)
- } else if math.Trunc(f) == f {
- b = strconv.AppendFloat(b, f, 'f', 1, 32)
- } else {
- b = strconv.AppendFloat(b, f, 'f', -1, 32)
- }
- case reflect.Float64:
- f := v.Float()
- if math.IsNaN(f) {
- b = append(b, "nan"...)
- } else if f > math.MaxFloat64 {
- b = append(b, "inf"...)
- } else if f < -math.MaxFloat64 {
- b = append(b, "-inf"...)
- } else if math.Trunc(f) == f {
- b = strconv.AppendFloat(b, f, 'f', 1, 64)
- } else {
- b = strconv.AppendFloat(b, f, 'f', -1, 64)
- }
- case reflect.Bool:
- if v.Bool() {
- b = append(b, "true"...)
- } else {
- b = append(b, "false"...)
- }
- case reflect.Uint64, reflect.Uint32, reflect.Uint16, reflect.Uint8, reflect.Uint:
- x := v.Uint()
- if x > uint64(math.MaxInt64) {
- return nil, fmt.Errorf("toml: not encoding uint (%d) greater than max int64 (%d)", x, int64(math.MaxInt64))
- }
- b = strconv.AppendUint(b, x, 10)
- case reflect.Int64, reflect.Int32, reflect.Int16, reflect.Int8, reflect.Int:
- b = strconv.AppendInt(b, v.Int(), 10)
- default:
- return nil, fmt.Errorf("toml: cannot encode value of type %s", v.Kind())
- }
-
- return b, nil
-}
-
-func isNil(v reflect.Value) bool {
- switch v.Kind() {
- case reflect.Ptr, reflect.Interface, reflect.Map:
- return v.IsNil()
- default:
- return false
- }
-}
-
-func shouldOmitEmpty(options valueOptions, v reflect.Value) bool {
- return options.omitempty && isEmptyValue(v)
-}
-
-func (enc *Encoder) encodeKv(b []byte, ctx encoderCtx, options valueOptions, v reflect.Value) ([]byte, error) {
- var err error
-
- if !ctx.inline {
- b = enc.encodeComment(ctx.indent, options.comment, b)
- }
-
- b = enc.indent(ctx.indent, b)
- b = enc.encodeKey(b, ctx.key)
- b = append(b, " = "...)
-
- // create a copy of the context because the value of a KV shouldn't
- // modify the global context.
- subctx := ctx
- subctx.insideKv = true
- subctx.shiftKey()
- subctx.options = options
-
- b, err = enc.encode(b, subctx, v)
- if err != nil {
- return nil, err
- }
-
- return b, nil
-}
-
-func isEmptyValue(v reflect.Value) bool {
- switch v.Kind() {
- case reflect.Struct:
- return isEmptyStruct(v)
- case reflect.Array, reflect.Map, reflect.Slice, reflect.String:
- return v.Len() == 0
- case reflect.Bool:
- return !v.Bool()
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64:
- return v.Int() == 0
- case reflect.Uint, reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr:
- return v.Uint() == 0
- case reflect.Float32, reflect.Float64:
- return v.Float() == 0
- case reflect.Interface, reflect.Ptr:
- return v.IsNil()
- }
- return false
-}
-
-func isEmptyStruct(v reflect.Value) bool {
- // TODO: merge with walkStruct and cache.
- typ := v.Type()
- for i := 0; i < typ.NumField(); i++ {
- fieldType := typ.Field(i)
-
- // only consider exported fields
- if fieldType.PkgPath != "" {
- continue
- }
-
- tag := fieldType.Tag.Get("toml")
-
- // special field name to skip field
- if tag == "-" {
- continue
- }
-
- f := v.Field(i)
-
- if !isEmptyValue(f) {
- return false
- }
- }
-
- return true
-}
-
-const literalQuote = '\''
-
-func (enc *Encoder) encodeString(b []byte, v string, options valueOptions) []byte {
- if needsQuoting(v) {
- return enc.encodeQuotedString(options.multiline, b, v)
- }
-
- return enc.encodeLiteralString(b, v)
-}
-
-func needsQuoting(v string) bool {
- // TODO: vectorize
- for _, b := range []byte(v) {
- if b == '\'' || b == '\r' || b == '\n' || characters.InvalidAscii(b) {
- return true
- }
- }
- return false
-}
-
-// caller should have checked that the string does not contain new lines or ' .
-func (enc *Encoder) encodeLiteralString(b []byte, v string) []byte {
- b = append(b, literalQuote)
- b = append(b, v...)
- b = append(b, literalQuote)
-
- return b
-}
-
-func (enc *Encoder) encodeQuotedString(multiline bool, b []byte, v string) []byte {
- stringQuote := `"`
-
- if multiline {
- stringQuote = `"""`
- }
-
- b = append(b, stringQuote...)
- if multiline {
- b = append(b, '\n')
- }
-
- const (
- hextable = "0123456789ABCDEF"
- // U+0000 to U+0008, U+000A to U+001F, U+007F
- nul = 0x0
- bs = 0x8
- lf = 0xa
- us = 0x1f
- del = 0x7f
- )
-
- for _, r := range []byte(v) {
- switch r {
- case '\\':
- b = append(b, `\\`...)
- case '"':
- b = append(b, `\"`...)
- case '\b':
- b = append(b, `\b`...)
- case '\f':
- b = append(b, `\f`...)
- case '\n':
- if multiline {
- b = append(b, r)
- } else {
- b = append(b, `\n`...)
- }
- case '\r':
- b = append(b, `\r`...)
- case '\t':
- b = append(b, `\t`...)
- default:
- switch {
- case r >= nul && r <= bs, r >= lf && r <= us, r == del:
- b = append(b, `\u00`...)
- b = append(b, hextable[r>>4])
- b = append(b, hextable[r&0x0f])
- default:
- b = append(b, r)
- }
- }
- }
-
- b = append(b, stringQuote...)
-
- return b
-}
-
-// caller should have checked that the string is in A-Z / a-z / 0-9 / - / _ .
-func (enc *Encoder) encodeUnquotedKey(b []byte, v string) []byte {
- return append(b, v...)
-}
-
-func (enc *Encoder) encodeTableHeader(ctx encoderCtx, b []byte) ([]byte, error) {
- if len(ctx.parentKey) == 0 {
- return b, nil
- }
-
- b = enc.encodeComment(ctx.indent, ctx.options.comment, b)
-
- b = enc.indent(ctx.indent, b)
-
- b = append(b, '[')
-
- b = enc.encodeKey(b, ctx.parentKey[0])
-
- for _, k := range ctx.parentKey[1:] {
- b = append(b, '.')
- b = enc.encodeKey(b, k)
- }
-
- b = append(b, "]\n"...)
-
- return b, nil
-}
-
-//nolint:cyclop
-func (enc *Encoder) encodeKey(b []byte, k string) []byte {
- needsQuotation := false
- cannotUseLiteral := false
-
- if len(k) == 0 {
- return append(b, "''"...)
- }
-
- for _, c := range k {
- if (c >= 'A' && c <= 'Z') || (c >= 'a' && c <= 'z') || (c >= '0' && c <= '9') || c == '-' || c == '_' {
- continue
- }
-
- if c == literalQuote {
- cannotUseLiteral = true
- }
-
- needsQuotation = true
- }
-
- if needsQuotation && needsQuoting(k) {
- cannotUseLiteral = true
- }
-
- switch {
- case cannotUseLiteral:
- return enc.encodeQuotedString(false, b, k)
- case needsQuotation:
- return enc.encodeLiteralString(b, k)
- default:
- return enc.encodeUnquotedKey(b, k)
- }
-}
-
-func (enc *Encoder) encodeMap(b []byte, ctx encoderCtx, v reflect.Value) ([]byte, error) {
- if v.Type().Key().Kind() != reflect.String {
- return nil, fmt.Errorf("toml: type %s is not supported as a map key", v.Type().Key().Kind())
- }
-
- var (
- t table
- emptyValueOptions valueOptions
- )
-
- iter := v.MapRange()
- for iter.Next() {
- k := iter.Key().String()
- v := iter.Value()
-
- if isNil(v) {
- continue
- }
-
- if willConvertToTableOrArrayTable(ctx, v) {
- t.pushTable(k, v, emptyValueOptions)
- } else {
- t.pushKV(k, v, emptyValueOptions)
- }
- }
-
- sortEntriesByKey(t.kvs)
- sortEntriesByKey(t.tables)
-
- return enc.encodeTable(b, ctx, t)
-}
-
-func sortEntriesByKey(e []entry) {
- sort.Slice(e, func(i, j int) bool {
- return e[i].Key < e[j].Key
- })
-}
-
-type entry struct {
- Key string
- Value reflect.Value
- Options valueOptions
-}
-
-type table struct {
- kvs []entry
- tables []entry
-}
-
-func (t *table) pushKV(k string, v reflect.Value, options valueOptions) {
- for _, e := range t.kvs {
- if e.Key == k {
- return
- }
- }
-
- t.kvs = append(t.kvs, entry{Key: k, Value: v, Options: options})
-}
-
-func (t *table) pushTable(k string, v reflect.Value, options valueOptions) {
- for _, e := range t.tables {
- if e.Key == k {
- return
- }
- }
- t.tables = append(t.tables, entry{Key: k, Value: v, Options: options})
-}
-
-func walkStruct(ctx encoderCtx, t *table, v reflect.Value) {
- // TODO: cache this
- typ := v.Type()
- for i := 0; i < typ.NumField(); i++ {
- fieldType := typ.Field(i)
-
- // only consider exported fields
- if fieldType.PkgPath != "" {
- continue
- }
-
- tag := fieldType.Tag.Get("toml")
-
- // special field name to skip field
- if tag == "-" {
- continue
- }
-
- k, opts := parseTag(tag)
- if !isValidName(k) {
- k = ""
- }
-
- f := v.Field(i)
-
- if k == "" {
- if fieldType.Anonymous {
- if fieldType.Type.Kind() == reflect.Struct {
- walkStruct(ctx, t, f)
- }
- continue
- } else {
- k = fieldType.Name
- }
- }
-
- if isNil(f) {
- continue
- }
-
- options := valueOptions{
- multiline: opts.multiline,
- omitempty: opts.omitempty,
- comment: fieldType.Tag.Get("comment"),
- }
-
- if opts.inline || !willConvertToTableOrArrayTable(ctx, f) {
- t.pushKV(k, f, options)
- } else {
- t.pushTable(k, f, options)
- }
- }
-}
-
-func (enc *Encoder) encodeStruct(b []byte, ctx encoderCtx, v reflect.Value) ([]byte, error) {
- var t table
-
- walkStruct(ctx, &t, v)
-
- return enc.encodeTable(b, ctx, t)
-}
-
-func (enc *Encoder) encodeComment(indent int, comment string, b []byte) []byte {
- for len(comment) > 0 {
- var line string
- idx := strings.IndexByte(comment, '\n')
- if idx >= 0 {
- line = comment[:idx]
- comment = comment[idx+1:]
- } else {
- line = comment
- comment = ""
- }
- b = enc.indent(indent, b)
- b = append(b, "# "...)
- b = append(b, line...)
- b = append(b, '\n')
- }
- return b
-}
-
-func isValidName(s string) bool {
- if s == "" {
- return false
- }
- for _, c := range s {
- switch {
- case strings.ContainsRune("!#$%&()*+-./:;<=>?@[]^_{|}~ ", c):
- // Backslash and quote chars are reserved, but
- // otherwise any punctuation chars are allowed
- // in a tag name.
- case !unicode.IsLetter(c) && !unicode.IsDigit(c):
- return false
- }
- }
- return true
-}
-
-type tagOptions struct {
- multiline bool
- inline bool
- omitempty bool
-}
-
-func parseTag(tag string) (string, tagOptions) {
- opts := tagOptions{}
-
- idx := strings.Index(tag, ",")
- if idx == -1 {
- return tag, opts
- }
-
- raw := tag[idx+1:]
- tag = string(tag[:idx])
- for raw != "" {
- var o string
- i := strings.Index(raw, ",")
- if i >= 0 {
- o, raw = raw[:i], raw[i+1:]
- } else {
- o, raw = raw, ""
- }
- switch o {
- case "multiline":
- opts.multiline = true
- case "inline":
- opts.inline = true
- case "omitempty":
- opts.omitempty = true
- }
- }
-
- return tag, opts
-}
-
-func (enc *Encoder) encodeTable(b []byte, ctx encoderCtx, t table) ([]byte, error) {
- var err error
-
- ctx.shiftKey()
-
- if ctx.insideKv || (ctx.inline && !ctx.isRoot()) {
- return enc.encodeTableInline(b, ctx, t)
- }
-
- if !ctx.skipTableHeader {
- b, err = enc.encodeTableHeader(ctx, b)
- if err != nil {
- return nil, err
- }
-
- if enc.indentTables && len(ctx.parentKey) > 0 {
- ctx.indent++
- }
- }
- ctx.skipTableHeader = false
-
- hasNonEmptyKV := false
- for _, kv := range t.kvs {
- if shouldOmitEmpty(kv.Options, kv.Value) {
- continue
- }
- hasNonEmptyKV = true
-
- ctx.setKey(kv.Key)
-
- b, err = enc.encodeKv(b, ctx, kv.Options, kv.Value)
- if err != nil {
- return nil, err
- }
-
- b = append(b, '\n')
- }
-
- first := true
- for _, table := range t.tables {
- if shouldOmitEmpty(table.Options, table.Value) {
- continue
- }
- if first {
- first = false
- if hasNonEmptyKV {
- b = append(b, '\n')
- }
- } else {
- b = append(b, "\n"...)
- }
-
- ctx.setKey(table.Key)
-
- ctx.options = table.Options
-
- b, err = enc.encode(b, ctx, table.Value)
- if err != nil {
- return nil, err
- }
- }
-
- return b, nil
-}
-
-func (enc *Encoder) encodeTableInline(b []byte, ctx encoderCtx, t table) ([]byte, error) {
- var err error
-
- b = append(b, '{')
-
- first := true
- for _, kv := range t.kvs {
- if shouldOmitEmpty(kv.Options, kv.Value) {
- continue
- }
-
- if first {
- first = false
- } else {
- b = append(b, `, `...)
- }
-
- ctx.setKey(kv.Key)
-
- b, err = enc.encodeKv(b, ctx, kv.Options, kv.Value)
- if err != nil {
- return nil, err
- }
- }
-
- if len(t.tables) > 0 {
- panic("inline table cannot contain nested tables, only key-values")
- }
-
- b = append(b, "}"...)
-
- return b, nil
-}
-
-func willConvertToTable(ctx encoderCtx, v reflect.Value) bool {
- if !v.IsValid() {
- return false
- }
- if v.Type() == timeType || v.Type().Implements(textMarshalerType) || (v.Kind() != reflect.Ptr && v.CanAddr() && reflect.PtrTo(v.Type()).Implements(textMarshalerType)) {
- return false
- }
-
- t := v.Type()
- switch t.Kind() {
- case reflect.Map, reflect.Struct:
- return !ctx.inline
- case reflect.Interface:
- return willConvertToTable(ctx, v.Elem())
- case reflect.Ptr:
- if v.IsNil() {
- return false
- }
-
- return willConvertToTable(ctx, v.Elem())
- default:
- return false
- }
-}
-
-func willConvertToTableOrArrayTable(ctx encoderCtx, v reflect.Value) bool {
- if ctx.insideKv {
- return false
- }
- t := v.Type()
-
- if t.Kind() == reflect.Interface {
- return willConvertToTableOrArrayTable(ctx, v.Elem())
- }
-
- if t.Kind() == reflect.Slice {
- if v.Len() == 0 {
- // An empty slice should be a kv = [].
- return false
- }
-
- for i := 0; i < v.Len(); i++ {
- t := willConvertToTable(ctx, v.Index(i))
-
- if !t {
- return false
- }
- }
-
- return true
- }
-
- return willConvertToTable(ctx, v)
-}
-
-func (enc *Encoder) encodeSlice(b []byte, ctx encoderCtx, v reflect.Value) ([]byte, error) {
- if v.Len() == 0 {
- b = append(b, "[]"...)
-
- return b, nil
- }
-
- if willConvertToTableOrArrayTable(ctx, v) {
- return enc.encodeSliceAsArrayTable(b, ctx, v)
- }
-
- return enc.encodeSliceAsArray(b, ctx, v)
-}
-
-// caller should have checked that v is a slice that only contains values that
-// encode into tables.
-func (enc *Encoder) encodeSliceAsArrayTable(b []byte, ctx encoderCtx, v reflect.Value) ([]byte, error) {
- ctx.shiftKey()
-
- scratch := make([]byte, 0, 64)
- scratch = append(scratch, "[["...)
-
- for i, k := range ctx.parentKey {
- if i > 0 {
- scratch = append(scratch, '.')
- }
-
- scratch = enc.encodeKey(scratch, k)
- }
-
- scratch = append(scratch, "]]\n"...)
- ctx.skipTableHeader = true
-
- b = enc.encodeComment(ctx.indent, ctx.options.comment, b)
-
- for i := 0; i < v.Len(); i++ {
- if i != 0 {
- b = append(b, "\n"...)
- }
-
- b = append(b, scratch...)
-
- var err error
- b, err = enc.encode(b, ctx, v.Index(i))
- if err != nil {
- return nil, err
- }
- }
-
- return b, nil
-}
-
-func (enc *Encoder) encodeSliceAsArray(b []byte, ctx encoderCtx, v reflect.Value) ([]byte, error) {
- multiline := ctx.options.multiline || enc.arraysMultiline
- separator := ", "
-
- b = append(b, '[')
-
- subCtx := ctx
- subCtx.options = valueOptions{}
-
- if multiline {
- separator = ",\n"
-
- b = append(b, '\n')
-
- subCtx.indent++
- }
-
- var err error
- first := true
-
- for i := 0; i < v.Len(); i++ {
- if first {
- first = false
- } else {
- b = append(b, separator...)
- }
-
- if multiline {
- b = enc.indent(subCtx.indent, b)
- }
-
- b, err = enc.encode(b, subCtx, v.Index(i))
- if err != nil {
- return nil, err
- }
- }
-
- if multiline {
- b = append(b, '\n')
- b = enc.indent(ctx.indent, b)
- }
-
- b = append(b, ']')
-
- return b, nil
-}
-
-func (enc *Encoder) indent(level int, b []byte) []byte {
- for i := 0; i < level; i++ {
- b = append(b, enc.indentSymbol...)
- }
-
- return b
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/strict.go b/vendor/github.com/pelletier/go-toml/v2/strict.go
deleted file mode 100644
index 802e7e4d..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/strict.go
+++ /dev/null
@@ -1,107 +0,0 @@
-package toml
-
-import (
- "github.com/pelletier/go-toml/v2/internal/danger"
- "github.com/pelletier/go-toml/v2/internal/tracker"
- "github.com/pelletier/go-toml/v2/unstable"
-)
-
-type strict struct {
- Enabled bool
-
- // Tracks the current key being processed.
- key tracker.KeyTracker
-
- missing []unstable.ParserError
-}
-
-func (s *strict) EnterTable(node *unstable.Node) {
- if !s.Enabled {
- return
- }
-
- s.key.UpdateTable(node)
-}
-
-func (s *strict) EnterArrayTable(node *unstable.Node) {
- if !s.Enabled {
- return
- }
-
- s.key.UpdateArrayTable(node)
-}
-
-func (s *strict) EnterKeyValue(node *unstable.Node) {
- if !s.Enabled {
- return
- }
-
- s.key.Push(node)
-}
-
-func (s *strict) ExitKeyValue(node *unstable.Node) {
- if !s.Enabled {
- return
- }
-
- s.key.Pop(node)
-}
-
-func (s *strict) MissingTable(node *unstable.Node) {
- if !s.Enabled {
- return
- }
-
- s.missing = append(s.missing, unstable.ParserError{
- Highlight: keyLocation(node),
- Message: "missing table",
- Key: s.key.Key(),
- })
-}
-
-func (s *strict) MissingField(node *unstable.Node) {
- if !s.Enabled {
- return
- }
-
- s.missing = append(s.missing, unstable.ParserError{
- Highlight: keyLocation(node),
- Message: "missing field",
- Key: s.key.Key(),
- })
-}
-
-func (s *strict) Error(doc []byte) error {
- if !s.Enabled || len(s.missing) == 0 {
- return nil
- }
-
- err := &StrictMissingError{
- Errors: make([]DecodeError, 0, len(s.missing)),
- }
-
- for _, derr := range s.missing {
- derr := derr
- err.Errors = append(err.Errors, *wrapDecodeError(doc, &derr))
- }
-
- return err
-}
-
-func keyLocation(node *unstable.Node) []byte {
- k := node.Key()
-
- hasOne := k.Next()
- if !hasOne {
- panic("should not be called with empty key")
- }
-
- start := k.Node().Data
- end := k.Node().Data
-
- for k.Next() {
- end = k.Node().Data
- }
-
- return danger.BytesRange(start, end)
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/toml.abnf b/vendor/github.com/pelletier/go-toml/v2/toml.abnf
deleted file mode 100644
index 473f3749..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/toml.abnf
+++ /dev/null
@@ -1,243 +0,0 @@
-;; This document describes TOML's syntax, using the ABNF format (defined in
-;; RFC 5234 -- https://www.ietf.org/rfc/rfc5234.txt).
-;;
-;; All valid TOML documents will match this description, however certain
-;; invalid documents would need to be rejected as per the semantics described
-;; in the supporting text description.
-
-;; It is possible to try this grammar interactively, using instaparse.
-;; http://instaparse.mojombo.com/
-;;
-;; To do so, in the lower right, click on Options and change `:input-format` to
-;; ':abnf'. Then paste this entire ABNF document into the grammar entry box
-;; (above the options). Then you can type or paste a sample TOML document into
-;; the beige box on the left. Tada!
-
-;; Overall Structure
-
-toml = expression *( newline expression )
-
-expression = ws [ comment ]
-expression =/ ws keyval ws [ comment ]
-expression =/ ws table ws [ comment ]
-
-;; Whitespace
-
-ws = *wschar
-wschar = %x20 ; Space
-wschar =/ %x09 ; Horizontal tab
-
-;; Newline
-
-newline = %x0A ; LF
-newline =/ %x0D.0A ; CRLF
-
-;; Comment
-
-comment-start-symbol = %x23 ; #
-non-ascii = %x80-D7FF / %xE000-10FFFF
-non-eol = %x09 / %x20-7F / non-ascii
-
-comment = comment-start-symbol *non-eol
-
-;; Key-Value pairs
-
-keyval = key keyval-sep val
-
-key = simple-key / dotted-key
-simple-key = quoted-key / unquoted-key
-
-unquoted-key = 1*( ALPHA / DIGIT / %x2D / %x5F ) ; A-Z / a-z / 0-9 / - / _
-quoted-key = basic-string / literal-string
-dotted-key = simple-key 1*( dot-sep simple-key )
-
-dot-sep = ws %x2E ws ; . Period
-keyval-sep = ws %x3D ws ; =
-
-val = string / boolean / array / inline-table / date-time / float / integer
-
-;; String
-
-string = ml-basic-string / basic-string / ml-literal-string / literal-string
-
-;; Basic String
-
-basic-string = quotation-mark *basic-char quotation-mark
-
-quotation-mark = %x22 ; "
-
-basic-char = basic-unescaped / escaped
-basic-unescaped = wschar / %x21 / %x23-5B / %x5D-7E / non-ascii
-escaped = escape escape-seq-char
-
-escape = %x5C ; \
-escape-seq-char = %x22 ; " quotation mark U+0022
-escape-seq-char =/ %x5C ; \ reverse solidus U+005C
-escape-seq-char =/ %x62 ; b backspace U+0008
-escape-seq-char =/ %x66 ; f form feed U+000C
-escape-seq-char =/ %x6E ; n line feed U+000A
-escape-seq-char =/ %x72 ; r carriage return U+000D
-escape-seq-char =/ %x74 ; t tab U+0009
-escape-seq-char =/ %x75 4HEXDIG ; uXXXX U+XXXX
-escape-seq-char =/ %x55 8HEXDIG ; UXXXXXXXX U+XXXXXXXX
-
-;; Multiline Basic String
-
-ml-basic-string = ml-basic-string-delim [ newline ] ml-basic-body
- ml-basic-string-delim
-ml-basic-string-delim = 3quotation-mark
-ml-basic-body = *mlb-content *( mlb-quotes 1*mlb-content ) [ mlb-quotes ]
-
-mlb-content = mlb-char / newline / mlb-escaped-nl
-mlb-char = mlb-unescaped / escaped
-mlb-quotes = 1*2quotation-mark
-mlb-unescaped = wschar / %x21 / %x23-5B / %x5D-7E / non-ascii
-mlb-escaped-nl = escape ws newline *( wschar / newline )
-
-;; Literal String
-
-literal-string = apostrophe *literal-char apostrophe
-
-apostrophe = %x27 ; ' apostrophe
-
-literal-char = %x09 / %x20-26 / %x28-7E / non-ascii
-
-;; Multiline Literal String
-
-ml-literal-string = ml-literal-string-delim [ newline ] ml-literal-body
- ml-literal-string-delim
-ml-literal-string-delim = 3apostrophe
-ml-literal-body = *mll-content *( mll-quotes 1*mll-content ) [ mll-quotes ]
-
-mll-content = mll-char / newline
-mll-char = %x09 / %x20-26 / %x28-7E / non-ascii
-mll-quotes = 1*2apostrophe
-
-;; Integer
-
-integer = dec-int / hex-int / oct-int / bin-int
-
-minus = %x2D ; -
-plus = %x2B ; +
-underscore = %x5F ; _
-digit1-9 = %x31-39 ; 1-9
-digit0-7 = %x30-37 ; 0-7
-digit0-1 = %x30-31 ; 0-1
-
-hex-prefix = %x30.78 ; 0x
-oct-prefix = %x30.6F ; 0o
-bin-prefix = %x30.62 ; 0b
-
-dec-int = [ minus / plus ] unsigned-dec-int
-unsigned-dec-int = DIGIT / digit1-9 1*( DIGIT / underscore DIGIT )
-
-hex-int = hex-prefix HEXDIG *( HEXDIG / underscore HEXDIG )
-oct-int = oct-prefix digit0-7 *( digit0-7 / underscore digit0-7 )
-bin-int = bin-prefix digit0-1 *( digit0-1 / underscore digit0-1 )
-
-;; Float
-
-float = float-int-part ( exp / frac [ exp ] )
-float =/ special-float
-
-float-int-part = dec-int
-frac = decimal-point zero-prefixable-int
-decimal-point = %x2E ; .
-zero-prefixable-int = DIGIT *( DIGIT / underscore DIGIT )
-
-exp = "e" float-exp-part
-float-exp-part = [ minus / plus ] zero-prefixable-int
-
-special-float = [ minus / plus ] ( inf / nan )
-inf = %x69.6e.66 ; inf
-nan = %x6e.61.6e ; nan
-
-;; Boolean
-
-boolean = true / false
-
-true = %x74.72.75.65 ; true
-false = %x66.61.6C.73.65 ; false
-
-;; Date and Time (as defined in RFC 3339)
-
-date-time = offset-date-time / local-date-time / local-date / local-time
-
-date-fullyear = 4DIGIT
-date-month = 2DIGIT ; 01-12
-date-mday = 2DIGIT ; 01-28, 01-29, 01-30, 01-31 based on month/year
-time-delim = "T" / %x20 ; T, t, or space
-time-hour = 2DIGIT ; 00-23
-time-minute = 2DIGIT ; 00-59
-time-second = 2DIGIT ; 00-58, 00-59, 00-60 based on leap second rules
-time-secfrac = "." 1*DIGIT
-time-numoffset = ( "+" / "-" ) time-hour ":" time-minute
-time-offset = "Z" / time-numoffset
-
-partial-time = time-hour ":" time-minute ":" time-second [ time-secfrac ]
-full-date = date-fullyear "-" date-month "-" date-mday
-full-time = partial-time time-offset
-
-;; Offset Date-Time
-
-offset-date-time = full-date time-delim full-time
-
-;; Local Date-Time
-
-local-date-time = full-date time-delim partial-time
-
-;; Local Date
-
-local-date = full-date
-
-;; Local Time
-
-local-time = partial-time
-
-;; Array
-
-array = array-open [ array-values ] ws-comment-newline array-close
-
-array-open = %x5B ; [
-array-close = %x5D ; ]
-
-array-values = ws-comment-newline val ws-comment-newline array-sep array-values
-array-values =/ ws-comment-newline val ws-comment-newline [ array-sep ]
-
-array-sep = %x2C ; , Comma
-
-ws-comment-newline = *( wschar / [ comment ] newline )
-
-;; Table
-
-table = std-table / array-table
-
-;; Standard Table
-
-std-table = std-table-open key std-table-close
-
-std-table-open = %x5B ws ; [ Left square bracket
-std-table-close = ws %x5D ; ] Right square bracket
-
-;; Inline Table
-
-inline-table = inline-table-open [ inline-table-keyvals ] inline-table-close
-
-inline-table-open = %x7B ws ; {
-inline-table-close = ws %x7D ; }
-inline-table-sep = ws %x2C ws ; , Comma
-
-inline-table-keyvals = keyval [ inline-table-sep inline-table-keyvals ]
-
-;; Array Table
-
-array-table = array-table-open key array-table-close
-
-array-table-open = %x5B.5B ws ; [[ Double left square bracket
-array-table-close = ws %x5D.5D ; ]] Double right square bracket
-
-;; Built-in ABNF terms, reproduced here for clarity
-
-ALPHA = %x41-5A / %x61-7A ; A-Z / a-z
-DIGIT = %x30-39 ; 0-9
-HEXDIG = DIGIT / "A" / "B" / "C" / "D" / "E" / "F"
diff --git a/vendor/github.com/pelletier/go-toml/v2/types.go b/vendor/github.com/pelletier/go-toml/v2/types.go
deleted file mode 100644
index 3c6b8fe5..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/types.go
+++ /dev/null
@@ -1,14 +0,0 @@
-package toml
-
-import (
- "encoding"
- "reflect"
- "time"
-)
-
-var timeType = reflect.TypeOf((*time.Time)(nil)).Elem()
-var textMarshalerType = reflect.TypeOf((*encoding.TextMarshaler)(nil)).Elem()
-var textUnmarshalerType = reflect.TypeOf((*encoding.TextUnmarshaler)(nil)).Elem()
-var mapStringInterfaceType = reflect.TypeOf(map[string]interface{}(nil))
-var sliceInterfaceType = reflect.TypeOf([]interface{}(nil))
-var stringType = reflect.TypeOf("")
diff --git a/vendor/github.com/pelletier/go-toml/v2/unmarshaler.go b/vendor/github.com/pelletier/go-toml/v2/unmarshaler.go
deleted file mode 100644
index 70f6ec57..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unmarshaler.go
+++ /dev/null
@@ -1,1227 +0,0 @@
-package toml
-
-import (
- "encoding"
- "errors"
- "fmt"
- "io"
- "io/ioutil"
- "math"
- "reflect"
- "strings"
- "sync/atomic"
- "time"
-
- "github.com/pelletier/go-toml/v2/internal/danger"
- "github.com/pelletier/go-toml/v2/internal/tracker"
- "github.com/pelletier/go-toml/v2/unstable"
-)
-
-// Unmarshal deserializes a TOML document into a Go value.
-//
-// It is a shortcut for Decoder.Decode() with the default options.
-func Unmarshal(data []byte, v interface{}) error {
- p := unstable.Parser{}
- p.Reset(data)
- d := decoder{p: &p}
-
- return d.FromParser(v)
-}
-
-// Decoder reads and decode a TOML document from an input stream.
-type Decoder struct {
- // input
- r io.Reader
-
- // global settings
- strict bool
-}
-
-// NewDecoder creates a new Decoder that will read from r.
-func NewDecoder(r io.Reader) *Decoder {
- return &Decoder{r: r}
-}
-
-// DisallowUnknownFields causes the Decoder to return an error when the
-// destination is a struct and the input contains a key that does not match a
-// non-ignored field.
-//
-// In that case, the Decoder returns a StrictMissingError that can be used to
-// retrieve the individual errors as well as generate a human readable
-// description of the missing fields.
-func (d *Decoder) DisallowUnknownFields() *Decoder {
- d.strict = true
- return d
-}
-
-// Decode the whole content of r into v.
-//
-// By default, values in the document that don't exist in the target Go value
-// are ignored. See Decoder.DisallowUnknownFields() to change this behavior.
-//
-// When a TOML local date, time, or date-time is decoded into a time.Time, its
-// value is represented in time.Local timezone. Otherwise the approriate Local*
-// structure is used. For time values, precision up to the nanosecond is
-// supported by truncating extra digits.
-//
-// Empty tables decoded in an interface{} create an empty initialized
-// map[string]interface{}.
-//
-// Types implementing the encoding.TextUnmarshaler interface are decoded from a
-// TOML string.
-//
-// When decoding a number, go-toml will return an error if the number is out of
-// bounds for the target type (which includes negative numbers when decoding
-// into an unsigned int).
-//
-// If an error occurs while decoding the content of the document, this function
-// returns a toml.DecodeError, providing context about the issue. When using
-// strict mode and a field is missing, a `toml.StrictMissingError` is
-// returned. In any other case, this function returns a standard Go error.
-//
-// # Type mapping
-//
-// List of supported TOML types and their associated accepted Go types:
-//
-// String -> string
-// Integer -> uint*, int*, depending on size
-// Float -> float*, depending on size
-// Boolean -> bool
-// Offset Date-Time -> time.Time
-// Local Date-time -> LocalDateTime, time.Time
-// Local Date -> LocalDate, time.Time
-// Local Time -> LocalTime, time.Time
-// Array -> slice and array, depending on elements types
-// Table -> map and struct
-// Inline Table -> same as Table
-// Array of Tables -> same as Array and Table
-func (d *Decoder) Decode(v interface{}) error {
- b, err := ioutil.ReadAll(d.r)
- if err != nil {
- return fmt.Errorf("toml: %w", err)
- }
-
- p := unstable.Parser{}
- p.Reset(b)
- dec := decoder{
- p: &p,
- strict: strict{
- Enabled: d.strict,
- },
- }
-
- return dec.FromParser(v)
-}
-
-type decoder struct {
- // Which parser instance in use for this decoding session.
- p *unstable.Parser
-
- // Flag indicating that the current expression is stashed.
- // If set to true, calling nextExpr will not actually pull a new expression
- // but turn off the flag instead.
- stashedExpr bool
-
- // Skip expressions until a table is found. This is set to true when a
- // table could not be created (missing field in map), so all KV expressions
- // need to be skipped.
- skipUntilTable bool
-
- // Tracks position in Go arrays.
- // This is used when decoding [[array tables]] into Go arrays. Given array
- // tables are separate TOML expression, we need to keep track of where we
- // are at in the Go array, as we can't just introspect its size.
- arrayIndexes map[reflect.Value]int
-
- // Tracks keys that have been seen, with which type.
- seen tracker.SeenTracker
-
- // Strict mode
- strict strict
-
- // Current context for the error.
- errorContext *errorContext
-}
-
-type errorContext struct {
- Struct reflect.Type
- Field []int
-}
-
-func (d *decoder) typeMismatchError(toml string, target reflect.Type) error {
- if d.errorContext != nil && d.errorContext.Struct != nil {
- ctx := d.errorContext
- f := ctx.Struct.FieldByIndex(ctx.Field)
- return fmt.Errorf("toml: cannot decode TOML %s into struct field %s.%s of type %s", toml, ctx.Struct, f.Name, f.Type)
- }
- return fmt.Errorf("toml: cannot decode TOML %s into a Go value of type %s", toml, target)
-}
-
-func (d *decoder) expr() *unstable.Node {
- return d.p.Expression()
-}
-
-func (d *decoder) nextExpr() bool {
- if d.stashedExpr {
- d.stashedExpr = false
- return true
- }
- return d.p.NextExpression()
-}
-
-func (d *decoder) stashExpr() {
- d.stashedExpr = true
-}
-
-func (d *decoder) arrayIndex(shouldAppend bool, v reflect.Value) int {
- if d.arrayIndexes == nil {
- d.arrayIndexes = make(map[reflect.Value]int, 1)
- }
-
- idx, ok := d.arrayIndexes[v]
-
- if !ok {
- d.arrayIndexes[v] = 0
- } else if shouldAppend {
- idx++
- d.arrayIndexes[v] = idx
- }
-
- return idx
-}
-
-func (d *decoder) FromParser(v interface{}) error {
- r := reflect.ValueOf(v)
- if r.Kind() != reflect.Ptr {
- return fmt.Errorf("toml: decoding can only be performed into a pointer, not %s", r.Kind())
- }
-
- if r.IsNil() {
- return fmt.Errorf("toml: decoding pointer target cannot be nil")
- }
-
- r = r.Elem()
- if r.Kind() == reflect.Interface && r.IsNil() {
- newMap := map[string]interface{}{}
- r.Set(reflect.ValueOf(newMap))
- }
-
- err := d.fromParser(r)
- if err == nil {
- return d.strict.Error(d.p.Data())
- }
-
- var e *unstable.ParserError
- if errors.As(err, &e) {
- return wrapDecodeError(d.p.Data(), e)
- }
-
- return err
-}
-
-func (d *decoder) fromParser(root reflect.Value) error {
- for d.nextExpr() {
- err := d.handleRootExpression(d.expr(), root)
- if err != nil {
- return err
- }
- }
-
- return d.p.Error()
-}
-
-/*
-Rules for the unmarshal code:
-
-- The stack is used to keep track of which values need to be set where.
-- handle* functions <=> switch on a given unstable.Kind.
-- unmarshalX* functions need to unmarshal a node of kind X.
-- An "object" is either a struct or a map.
-*/
-
-func (d *decoder) handleRootExpression(expr *unstable.Node, v reflect.Value) error {
- var x reflect.Value
- var err error
-
- if !(d.skipUntilTable && expr.Kind == unstable.KeyValue) {
- err = d.seen.CheckExpression(expr)
- if err != nil {
- return err
- }
- }
-
- switch expr.Kind {
- case unstable.KeyValue:
- if d.skipUntilTable {
- return nil
- }
- x, err = d.handleKeyValue(expr, v)
- case unstable.Table:
- d.skipUntilTable = false
- d.strict.EnterTable(expr)
- x, err = d.handleTable(expr.Key(), v)
- case unstable.ArrayTable:
- d.skipUntilTable = false
- d.strict.EnterArrayTable(expr)
- x, err = d.handleArrayTable(expr.Key(), v)
- default:
- panic(fmt.Errorf("parser should not permit expression of kind %s at document root", expr.Kind))
- }
-
- if d.skipUntilTable {
- if expr.Kind == unstable.Table || expr.Kind == unstable.ArrayTable {
- d.strict.MissingTable(expr)
- }
- } else if err == nil && x.IsValid() {
- v.Set(x)
- }
-
- return err
-}
-
-func (d *decoder) handleArrayTable(key unstable.Iterator, v reflect.Value) (reflect.Value, error) {
- if key.Next() {
- return d.handleArrayTablePart(key, v)
- }
- return d.handleKeyValues(v)
-}
-
-func (d *decoder) handleArrayTableCollectionLast(key unstable.Iterator, v reflect.Value) (reflect.Value, error) {
- switch v.Kind() {
- case reflect.Interface:
- elem := v.Elem()
- if !elem.IsValid() {
- elem = reflect.New(sliceInterfaceType).Elem()
- elem.Set(reflect.MakeSlice(sliceInterfaceType, 0, 16))
- } else if elem.Kind() == reflect.Slice {
- if elem.Type() != sliceInterfaceType {
- elem = reflect.New(sliceInterfaceType).Elem()
- elem.Set(reflect.MakeSlice(sliceInterfaceType, 0, 16))
- } else if !elem.CanSet() {
- nelem := reflect.New(sliceInterfaceType).Elem()
- nelem.Set(reflect.MakeSlice(sliceInterfaceType, elem.Len(), elem.Cap()))
- reflect.Copy(nelem, elem)
- elem = nelem
- }
- }
- return d.handleArrayTableCollectionLast(key, elem)
- case reflect.Ptr:
- elem := v.Elem()
- if !elem.IsValid() {
- ptr := reflect.New(v.Type().Elem())
- v.Set(ptr)
- elem = ptr.Elem()
- }
-
- elem, err := d.handleArrayTableCollectionLast(key, elem)
- if err != nil {
- return reflect.Value{}, err
- }
- v.Elem().Set(elem)
-
- return v, nil
- case reflect.Slice:
- elemType := v.Type().Elem()
- var elem reflect.Value
- if elemType.Kind() == reflect.Interface {
- elem = makeMapStringInterface()
- } else {
- elem = reflect.New(elemType).Elem()
- }
- elem2, err := d.handleArrayTable(key, elem)
- if err != nil {
- return reflect.Value{}, err
- }
- if elem2.IsValid() {
- elem = elem2
- }
- return reflect.Append(v, elem), nil
- case reflect.Array:
- idx := d.arrayIndex(true, v)
- if idx >= v.Len() {
- return v, fmt.Errorf("%s at position %d", d.typeMismatchError("array table", v.Type()), idx)
- }
- elem := v.Index(idx)
- _, err := d.handleArrayTable(key, elem)
- return v, err
- default:
- return reflect.Value{}, d.typeMismatchError("array table", v.Type())
- }
-}
-
-// When parsing an array table expression, each part of the key needs to be
-// evaluated like a normal key, but if it returns a collection, it also needs to
-// point to the last element of the collection. Unless it is the last part of
-// the key, then it needs to create a new element at the end.
-func (d *decoder) handleArrayTableCollection(key unstable.Iterator, v reflect.Value) (reflect.Value, error) {
- if key.IsLast() {
- return d.handleArrayTableCollectionLast(key, v)
- }
-
- switch v.Kind() {
- case reflect.Ptr:
- elem := v.Elem()
- if !elem.IsValid() {
- ptr := reflect.New(v.Type().Elem())
- v.Set(ptr)
- elem = ptr.Elem()
- }
-
- elem, err := d.handleArrayTableCollection(key, elem)
- if err != nil {
- return reflect.Value{}, err
- }
- if elem.IsValid() {
- v.Elem().Set(elem)
- }
-
- return v, nil
- case reflect.Slice:
- elem := v.Index(v.Len() - 1)
- x, err := d.handleArrayTable(key, elem)
- if err != nil || d.skipUntilTable {
- return reflect.Value{}, err
- }
- if x.IsValid() {
- elem.Set(x)
- }
-
- return v, err
- case reflect.Array:
- idx := d.arrayIndex(false, v)
- if idx >= v.Len() {
- return v, fmt.Errorf("%s at position %d", d.typeMismatchError("array table", v.Type()), idx)
- }
- elem := v.Index(idx)
- _, err := d.handleArrayTable(key, elem)
- return v, err
- }
-
- return d.handleArrayTable(key, v)
-}
-
-func (d *decoder) handleKeyPart(key unstable.Iterator, v reflect.Value, nextFn handlerFn, makeFn valueMakerFn) (reflect.Value, error) {
- var rv reflect.Value
-
- // First, dispatch over v to make sure it is a valid object.
- // There is no guarantee over what it could be.
- switch v.Kind() {
- case reflect.Ptr:
- elem := v.Elem()
- if !elem.IsValid() {
- v.Set(reflect.New(v.Type().Elem()))
- }
- elem = v.Elem()
- return d.handleKeyPart(key, elem, nextFn, makeFn)
- case reflect.Map:
- vt := v.Type()
-
- // Create the key for the map element. Convert to key type.
- mk := reflect.ValueOf(string(key.Node().Data)).Convert(vt.Key())
-
- // If the map does not exist, create it.
- if v.IsNil() {
- vt := v.Type()
- v = reflect.MakeMap(vt)
- rv = v
- }
-
- mv := v.MapIndex(mk)
- set := false
- if !mv.IsValid() {
- // If there is no value in the map, create a new one according to
- // the map type. If the element type is interface, create either a
- // map[string]interface{} or a []interface{} depending on whether
- // this is the last part of the array table key.
-
- t := vt.Elem()
- if t.Kind() == reflect.Interface {
- mv = makeFn()
- } else {
- mv = reflect.New(t).Elem()
- }
- set = true
- } else if mv.Kind() == reflect.Interface {
- mv = mv.Elem()
- if !mv.IsValid() {
- mv = makeFn()
- }
- set = true
- } else if !mv.CanAddr() {
- vt := v.Type()
- t := vt.Elem()
- oldmv := mv
- mv = reflect.New(t).Elem()
- mv.Set(oldmv)
- set = true
- }
-
- x, err := nextFn(key, mv)
- if err != nil {
- return reflect.Value{}, err
- }
-
- if x.IsValid() {
- mv = x
- set = true
- }
-
- if set {
- v.SetMapIndex(mk, mv)
- }
- case reflect.Struct:
- path, found := structFieldPath(v, string(key.Node().Data))
- if !found {
- d.skipUntilTable = true
- return reflect.Value{}, nil
- }
-
- if d.errorContext == nil {
- d.errorContext = new(errorContext)
- }
- t := v.Type()
- d.errorContext.Struct = t
- d.errorContext.Field = path
-
- f := fieldByIndex(v, path)
- x, err := nextFn(key, f)
- if err != nil || d.skipUntilTable {
- return reflect.Value{}, err
- }
- if x.IsValid() {
- f.Set(x)
- }
- d.errorContext.Field = nil
- d.errorContext.Struct = nil
- case reflect.Interface:
- if v.Elem().IsValid() {
- v = v.Elem()
- } else {
- v = makeMapStringInterface()
- }
-
- x, err := d.handleKeyPart(key, v, nextFn, makeFn)
- if err != nil {
- return reflect.Value{}, err
- }
- if x.IsValid() {
- v = x
- }
- rv = v
- default:
- panic(fmt.Errorf("unhandled part: %s", v.Kind()))
- }
-
- return rv, nil
-}
-
-// HandleArrayTablePart navigates the Go structure v using the key v. It is
-// only used for the prefix (non-last) parts of an array-table. When
-// encountering a collection, it should go to the last element.
-func (d *decoder) handleArrayTablePart(key unstable.Iterator, v reflect.Value) (reflect.Value, error) {
- var makeFn valueMakerFn
- if key.IsLast() {
- makeFn = makeSliceInterface
- } else {
- makeFn = makeMapStringInterface
- }
- return d.handleKeyPart(key, v, d.handleArrayTableCollection, makeFn)
-}
-
-// HandleTable returns a reference when it has checked the next expression but
-// cannot handle it.
-func (d *decoder) handleTable(key unstable.Iterator, v reflect.Value) (reflect.Value, error) {
- if v.Kind() == reflect.Slice {
- if v.Len() == 0 {
- return reflect.Value{}, unstable.NewParserError(key.Node().Data, "cannot store a table in a slice")
- }
- elem := v.Index(v.Len() - 1)
- x, err := d.handleTable(key, elem)
- if err != nil {
- return reflect.Value{}, err
- }
- if x.IsValid() {
- elem.Set(x)
- }
- return reflect.Value{}, nil
- }
- if key.Next() {
- // Still scoping the key
- return d.handleTablePart(key, v)
- }
- // Done scoping the key.
- // Now handle all the key-value expressions in this table.
- return d.handleKeyValues(v)
-}
-
-// Handle root expressions until the end of the document or the next
-// non-key-value.
-func (d *decoder) handleKeyValues(v reflect.Value) (reflect.Value, error) {
- var rv reflect.Value
- for d.nextExpr() {
- expr := d.expr()
- if expr.Kind != unstable.KeyValue {
- // Stash the expression so that fromParser can just loop and use
- // the right handler.
- // We could just recurse ourselves here, but at least this gives a
- // chance to pop the stack a bit.
- d.stashExpr()
- break
- }
-
- err := d.seen.CheckExpression(expr)
- if err != nil {
- return reflect.Value{}, err
- }
-
- x, err := d.handleKeyValue(expr, v)
- if err != nil {
- return reflect.Value{}, err
- }
- if x.IsValid() {
- v = x
- rv = x
- }
- }
- return rv, nil
-}
-
-type (
- handlerFn func(key unstable.Iterator, v reflect.Value) (reflect.Value, error)
- valueMakerFn func() reflect.Value
-)
-
-func makeMapStringInterface() reflect.Value {
- return reflect.MakeMap(mapStringInterfaceType)
-}
-
-func makeSliceInterface() reflect.Value {
- return reflect.MakeSlice(sliceInterfaceType, 0, 16)
-}
-
-func (d *decoder) handleTablePart(key unstable.Iterator, v reflect.Value) (reflect.Value, error) {
- return d.handleKeyPart(key, v, d.handleTable, makeMapStringInterface)
-}
-
-func (d *decoder) tryTextUnmarshaler(node *unstable.Node, v reflect.Value) (bool, error) {
- // Special case for time, because we allow to unmarshal to it from
- // different kind of AST nodes.
- if v.Type() == timeType {
- return false, nil
- }
-
- if v.CanAddr() && v.Addr().Type().Implements(textUnmarshalerType) {
- err := v.Addr().Interface().(encoding.TextUnmarshaler).UnmarshalText(node.Data)
- if err != nil {
- return false, unstable.NewParserError(d.p.Raw(node.Raw), "%w", err)
- }
-
- return true, nil
- }
-
- return false, nil
-}
-
-func (d *decoder) handleValue(value *unstable.Node, v reflect.Value) error {
- for v.Kind() == reflect.Ptr {
- v = initAndDereferencePointer(v)
- }
-
- ok, err := d.tryTextUnmarshaler(value, v)
- if ok || err != nil {
- return err
- }
-
- switch value.Kind {
- case unstable.String:
- return d.unmarshalString(value, v)
- case unstable.Integer:
- return d.unmarshalInteger(value, v)
- case unstable.Float:
- return d.unmarshalFloat(value, v)
- case unstable.Bool:
- return d.unmarshalBool(value, v)
- case unstable.DateTime:
- return d.unmarshalDateTime(value, v)
- case unstable.LocalDate:
- return d.unmarshalLocalDate(value, v)
- case unstable.LocalTime:
- return d.unmarshalLocalTime(value, v)
- case unstable.LocalDateTime:
- return d.unmarshalLocalDateTime(value, v)
- case unstable.InlineTable:
- return d.unmarshalInlineTable(value, v)
- case unstable.Array:
- return d.unmarshalArray(value, v)
- default:
- panic(fmt.Errorf("handleValue not implemented for %s", value.Kind))
- }
-}
-
-func (d *decoder) unmarshalArray(array *unstable.Node, v reflect.Value) error {
- switch v.Kind() {
- case reflect.Slice:
- if v.IsNil() {
- v.Set(reflect.MakeSlice(v.Type(), 0, 16))
- } else {
- v.SetLen(0)
- }
- case reflect.Array:
- // arrays are always initialized
- case reflect.Interface:
- elem := v.Elem()
- if !elem.IsValid() {
- elem = reflect.New(sliceInterfaceType).Elem()
- elem.Set(reflect.MakeSlice(sliceInterfaceType, 0, 16))
- } else if elem.Kind() == reflect.Slice {
- if elem.Type() != sliceInterfaceType {
- elem = reflect.New(sliceInterfaceType).Elem()
- elem.Set(reflect.MakeSlice(sliceInterfaceType, 0, 16))
- } else if !elem.CanSet() {
- nelem := reflect.New(sliceInterfaceType).Elem()
- nelem.Set(reflect.MakeSlice(sliceInterfaceType, elem.Len(), elem.Cap()))
- reflect.Copy(nelem, elem)
- elem = nelem
- }
- }
- err := d.unmarshalArray(array, elem)
- if err != nil {
- return err
- }
- v.Set(elem)
- return nil
- default:
- // TODO: use newDecodeError, but first the parser needs to fill
- // array.Data.
- return d.typeMismatchError("array", v.Type())
- }
-
- elemType := v.Type().Elem()
-
- it := array.Children()
- idx := 0
- for it.Next() {
- n := it.Node()
-
- // TODO: optimize
- if v.Kind() == reflect.Slice {
- elem := reflect.New(elemType).Elem()
-
- err := d.handleValue(n, elem)
- if err != nil {
- return err
- }
-
- v.Set(reflect.Append(v, elem))
- } else { // array
- if idx >= v.Len() {
- return nil
- }
- elem := v.Index(idx)
- err := d.handleValue(n, elem)
- if err != nil {
- return err
- }
- idx++
- }
- }
-
- return nil
-}
-
-func (d *decoder) unmarshalInlineTable(itable *unstable.Node, v reflect.Value) error {
- // Make sure v is an initialized object.
- switch v.Kind() {
- case reflect.Map:
- if v.IsNil() {
- v.Set(reflect.MakeMap(v.Type()))
- }
- case reflect.Struct:
- // structs are always initialized.
- case reflect.Interface:
- elem := v.Elem()
- if !elem.IsValid() {
- elem = makeMapStringInterface()
- v.Set(elem)
- }
- return d.unmarshalInlineTable(itable, elem)
- default:
- return unstable.NewParserError(itable.Data, "cannot store inline table in Go type %s", v.Kind())
- }
-
- it := itable.Children()
- for it.Next() {
- n := it.Node()
-
- x, err := d.handleKeyValue(n, v)
- if err != nil {
- return err
- }
- if x.IsValid() {
- v = x
- }
- }
-
- return nil
-}
-
-func (d *decoder) unmarshalDateTime(value *unstable.Node, v reflect.Value) error {
- dt, err := parseDateTime(value.Data)
- if err != nil {
- return err
- }
-
- v.Set(reflect.ValueOf(dt))
- return nil
-}
-
-func (d *decoder) unmarshalLocalDate(value *unstable.Node, v reflect.Value) error {
- ld, err := parseLocalDate(value.Data)
- if err != nil {
- return err
- }
-
- if v.Type() == timeType {
- cast := ld.AsTime(time.Local)
- v.Set(reflect.ValueOf(cast))
- return nil
- }
-
- v.Set(reflect.ValueOf(ld))
-
- return nil
-}
-
-func (d *decoder) unmarshalLocalTime(value *unstable.Node, v reflect.Value) error {
- lt, rest, err := parseLocalTime(value.Data)
- if err != nil {
- return err
- }
-
- if len(rest) > 0 {
- return unstable.NewParserError(rest, "extra characters at the end of a local time")
- }
-
- v.Set(reflect.ValueOf(lt))
- return nil
-}
-
-func (d *decoder) unmarshalLocalDateTime(value *unstable.Node, v reflect.Value) error {
- ldt, rest, err := parseLocalDateTime(value.Data)
- if err != nil {
- return err
- }
-
- if len(rest) > 0 {
- return unstable.NewParserError(rest, "extra characters at the end of a local date time")
- }
-
- if v.Type() == timeType {
- cast := ldt.AsTime(time.Local)
-
- v.Set(reflect.ValueOf(cast))
- return nil
- }
-
- v.Set(reflect.ValueOf(ldt))
-
- return nil
-}
-
-func (d *decoder) unmarshalBool(value *unstable.Node, v reflect.Value) error {
- b := value.Data[0] == 't'
-
- switch v.Kind() {
- case reflect.Bool:
- v.SetBool(b)
- case reflect.Interface:
- v.Set(reflect.ValueOf(b))
- default:
- return unstable.NewParserError(value.Data, "cannot assign boolean to a %t", b)
- }
-
- return nil
-}
-
-func (d *decoder) unmarshalFloat(value *unstable.Node, v reflect.Value) error {
- f, err := parseFloat(value.Data)
- if err != nil {
- return err
- }
-
- switch v.Kind() {
- case reflect.Float64:
- v.SetFloat(f)
- case reflect.Float32:
- if f > math.MaxFloat32 {
- return unstable.NewParserError(value.Data, "number %f does not fit in a float32", f)
- }
- v.SetFloat(f)
- case reflect.Interface:
- v.Set(reflect.ValueOf(f))
- default:
- return unstable.NewParserError(value.Data, "float cannot be assigned to %s", v.Kind())
- }
-
- return nil
-}
-
-const (
- maxInt = int64(^uint(0) >> 1)
- minInt = -maxInt - 1
-)
-
-// Maximum value of uint for decoding. Currently the decoder parses the integer
-// into an int64. As a result, on architectures where uint is 64 bits, the
-// effective maximum uint we can decode is the maximum of int64. On
-// architectures where uint is 32 bits, the maximum value we can decode is
-// lower: the maximum of uint32. I didn't find a way to figure out this value at
-// compile time, so it is computed during initialization.
-var maxUint int64 = math.MaxInt64
-
-func init() {
- m := uint64(^uint(0))
- if m < uint64(maxUint) {
- maxUint = int64(m)
- }
-}
-
-func (d *decoder) unmarshalInteger(value *unstable.Node, v reflect.Value) error {
- i, err := parseInteger(value.Data)
- if err != nil {
- return err
- }
-
- var r reflect.Value
-
- switch v.Kind() {
- case reflect.Int64:
- v.SetInt(i)
- return nil
- case reflect.Int32:
- if i < math.MinInt32 || i > math.MaxInt32 {
- return fmt.Errorf("toml: number %d does not fit in an int32", i)
- }
-
- r = reflect.ValueOf(int32(i))
- case reflect.Int16:
- if i < math.MinInt16 || i > math.MaxInt16 {
- return fmt.Errorf("toml: number %d does not fit in an int16", i)
- }
-
- r = reflect.ValueOf(int16(i))
- case reflect.Int8:
- if i < math.MinInt8 || i > math.MaxInt8 {
- return fmt.Errorf("toml: number %d does not fit in an int8", i)
- }
-
- r = reflect.ValueOf(int8(i))
- case reflect.Int:
- if i < minInt || i > maxInt {
- return fmt.Errorf("toml: number %d does not fit in an int", i)
- }
-
- r = reflect.ValueOf(int(i))
- case reflect.Uint64:
- if i < 0 {
- return fmt.Errorf("toml: negative number %d does not fit in an uint64", i)
- }
-
- r = reflect.ValueOf(uint64(i))
- case reflect.Uint32:
- if i < 0 || i > math.MaxUint32 {
- return fmt.Errorf("toml: negative number %d does not fit in an uint32", i)
- }
-
- r = reflect.ValueOf(uint32(i))
- case reflect.Uint16:
- if i < 0 || i > math.MaxUint16 {
- return fmt.Errorf("toml: negative number %d does not fit in an uint16", i)
- }
-
- r = reflect.ValueOf(uint16(i))
- case reflect.Uint8:
- if i < 0 || i > math.MaxUint8 {
- return fmt.Errorf("toml: negative number %d does not fit in an uint8", i)
- }
-
- r = reflect.ValueOf(uint8(i))
- case reflect.Uint:
- if i < 0 || i > maxUint {
- return fmt.Errorf("toml: negative number %d does not fit in an uint", i)
- }
-
- r = reflect.ValueOf(uint(i))
- case reflect.Interface:
- r = reflect.ValueOf(i)
- default:
- return d.typeMismatchError("integer", v.Type())
- }
-
- if !r.Type().AssignableTo(v.Type()) {
- r = r.Convert(v.Type())
- }
-
- v.Set(r)
-
- return nil
-}
-
-func (d *decoder) unmarshalString(value *unstable.Node, v reflect.Value) error {
- switch v.Kind() {
- case reflect.String:
- v.SetString(string(value.Data))
- case reflect.Interface:
- v.Set(reflect.ValueOf(string(value.Data)))
- default:
- return unstable.NewParserError(d.p.Raw(value.Raw), "cannot store TOML string into a Go %s", v.Kind())
- }
-
- return nil
-}
-
-func (d *decoder) handleKeyValue(expr *unstable.Node, v reflect.Value) (reflect.Value, error) {
- d.strict.EnterKeyValue(expr)
-
- v, err := d.handleKeyValueInner(expr.Key(), expr.Value(), v)
- if d.skipUntilTable {
- d.strict.MissingField(expr)
- d.skipUntilTable = false
- }
-
- d.strict.ExitKeyValue(expr)
-
- return v, err
-}
-
-func (d *decoder) handleKeyValueInner(key unstable.Iterator, value *unstable.Node, v reflect.Value) (reflect.Value, error) {
- if key.Next() {
- // Still scoping the key
- return d.handleKeyValuePart(key, value, v)
- }
- // Done scoping the key.
- // v is whatever Go value we need to fill.
- return reflect.Value{}, d.handleValue(value, v)
-}
-
-func (d *decoder) handleKeyValuePart(key unstable.Iterator, value *unstable.Node, v reflect.Value) (reflect.Value, error) {
- // contains the replacement for v
- var rv reflect.Value
-
- // First, dispatch over v to make sure it is a valid object.
- // There is no guarantee over what it could be.
- switch v.Kind() {
- case reflect.Map:
- vt := v.Type()
-
- mk := reflect.ValueOf(string(key.Node().Data))
- mkt := stringType
-
- keyType := vt.Key()
- if !mkt.AssignableTo(keyType) {
- if !mkt.ConvertibleTo(keyType) {
- return reflect.Value{}, fmt.Errorf("toml: cannot convert map key of type %s to expected type %s", mkt, keyType)
- }
-
- mk = mk.Convert(keyType)
- }
-
- // If the map does not exist, create it.
- if v.IsNil() {
- v = reflect.MakeMap(vt)
- rv = v
- }
-
- mv := v.MapIndex(mk)
- set := false
- if !mv.IsValid() {
- set = true
- mv = reflect.New(v.Type().Elem()).Elem()
- } else {
- if key.IsLast() {
- var x interface{}
- mv = reflect.ValueOf(&x).Elem()
- set = true
- }
- }
-
- nv, err := d.handleKeyValueInner(key, value, mv)
- if err != nil {
- return reflect.Value{}, err
- }
- if nv.IsValid() {
- mv = nv
- set = true
- }
-
- if set {
- v.SetMapIndex(mk, mv)
- }
- case reflect.Struct:
- path, found := structFieldPath(v, string(key.Node().Data))
- if !found {
- d.skipUntilTable = true
- break
- }
-
- if d.errorContext == nil {
- d.errorContext = new(errorContext)
- }
- t := v.Type()
- d.errorContext.Struct = t
- d.errorContext.Field = path
-
- f := fieldByIndex(v, path)
- x, err := d.handleKeyValueInner(key, value, f)
- if err != nil {
- return reflect.Value{}, err
- }
-
- if x.IsValid() {
- f.Set(x)
- }
- d.errorContext.Struct = nil
- d.errorContext.Field = nil
- case reflect.Interface:
- v = v.Elem()
-
- // Following encoding/json: decoding an object into an
- // interface{}, it needs to always hold a
- // map[string]interface{}. This is for the types to be
- // consistent whether a previous value was set or not.
- if !v.IsValid() || v.Type() != mapStringInterfaceType {
- v = makeMapStringInterface()
- }
-
- x, err := d.handleKeyValuePart(key, value, v)
- if err != nil {
- return reflect.Value{}, err
- }
- if x.IsValid() {
- v = x
- }
- rv = v
- case reflect.Ptr:
- elem := v.Elem()
- if !elem.IsValid() {
- ptr := reflect.New(v.Type().Elem())
- v.Set(ptr)
- rv = v
- elem = ptr.Elem()
- }
-
- elem2, err := d.handleKeyValuePart(key, value, elem)
- if err != nil {
- return reflect.Value{}, err
- }
- if elem2.IsValid() {
- elem = elem2
- }
- v.Elem().Set(elem)
- default:
- return reflect.Value{}, fmt.Errorf("unhandled kv part: %s", v.Kind())
- }
-
- return rv, nil
-}
-
-func initAndDereferencePointer(v reflect.Value) reflect.Value {
- var elem reflect.Value
- if v.IsNil() {
- ptr := reflect.New(v.Type().Elem())
- v.Set(ptr)
- }
- elem = v.Elem()
- return elem
-}
-
-// Same as reflect.Value.FieldByIndex, but creates pointers if needed.
-func fieldByIndex(v reflect.Value, path []int) reflect.Value {
- for i, x := range path {
- v = v.Field(x)
-
- if i < len(path)-1 && v.Kind() == reflect.Ptr {
- if v.IsNil() {
- v.Set(reflect.New(v.Type().Elem()))
- }
- v = v.Elem()
- }
- }
- return v
-}
-
-type fieldPathsMap = map[string][]int
-
-var globalFieldPathsCache atomic.Value // map[danger.TypeID]fieldPathsMap
-
-func structFieldPath(v reflect.Value, name string) ([]int, bool) {
- t := v.Type()
-
- cache, _ := globalFieldPathsCache.Load().(map[danger.TypeID]fieldPathsMap)
- fieldPaths, ok := cache[danger.MakeTypeID(t)]
-
- if !ok {
- fieldPaths = map[string][]int{}
-
- forEachField(t, nil, func(name string, path []int) {
- fieldPaths[name] = path
- // extra copy for the case-insensitive match
- fieldPaths[strings.ToLower(name)] = path
- })
-
- newCache := make(map[danger.TypeID]fieldPathsMap, len(cache)+1)
- newCache[danger.MakeTypeID(t)] = fieldPaths
- for k, v := range cache {
- newCache[k] = v
- }
- globalFieldPathsCache.Store(newCache)
- }
-
- path, ok := fieldPaths[name]
- if !ok {
- path, ok = fieldPaths[strings.ToLower(name)]
- }
- return path, ok
-}
-
-func forEachField(t reflect.Type, path []int, do func(name string, path []int)) {
- n := t.NumField()
- for i := 0; i < n; i++ {
- f := t.Field(i)
-
- if !f.Anonymous && f.PkgPath != "" {
- // only consider exported fields.
- continue
- }
-
- fieldPath := append(path, i)
- fieldPath = fieldPath[:len(fieldPath):len(fieldPath)]
-
- name := f.Tag.Get("toml")
- if name == "-" {
- continue
- }
-
- if i := strings.IndexByte(name, ','); i >= 0 {
- name = name[:i]
- }
-
- if f.Anonymous && name == "" {
- t2 := f.Type
- if t2.Kind() == reflect.Ptr {
- t2 = t2.Elem()
- }
-
- if t2.Kind() == reflect.Struct {
- forEachField(t2, fieldPath, do)
- }
- continue
- }
-
- if name == "" {
- name = f.Name
- }
-
- do(name, fieldPath)
- }
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/unstable/ast.go b/vendor/github.com/pelletier/go-toml/v2/unstable/ast.go
deleted file mode 100644
index b60d9bfd..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unstable/ast.go
+++ /dev/null
@@ -1,136 +0,0 @@
-package unstable
-
-import (
- "fmt"
- "unsafe"
-
- "github.com/pelletier/go-toml/v2/internal/danger"
-)
-
-// Iterator over a sequence of nodes.
-//
-// Starts uninitialized, you need to call Next() first.
-//
-// For example:
-//
-// it := n.Children()
-// for it.Next() {
-// n := it.Node()
-// // do something with n
-// }
-type Iterator struct {
- started bool
- node *Node
-}
-
-// Next moves the iterator forward and returns true if points to a
-// node, false otherwise.
-func (c *Iterator) Next() bool {
- if !c.started {
- c.started = true
- } else if c.node.Valid() {
- c.node = c.node.Next()
- }
- return c.node.Valid()
-}
-
-// IsLast returns true if the current node of the iterator is the last
-// one. Subsequent calls to Next() will return false.
-func (c *Iterator) IsLast() bool {
- return c.node.next == 0
-}
-
-// Node returns a pointer to the node pointed at by the iterator.
-func (c *Iterator) Node() *Node {
- return c.node
-}
-
-// Node in a TOML expression AST.
-//
-// Depending on Kind, its sequence of children should be interpreted
-// differently.
-//
-// - Array have one child per element in the array.
-// - InlineTable have one child per key-value in the table (each of kind
-// InlineTable).
-// - KeyValue have at least two children. The first one is the value. The rest
-// make a potentially dotted key.
-// - Table and ArrayTable's children represent a dotted key (same as
-// KeyValue, but without the first node being the value).
-//
-// When relevant, Raw describes the range of bytes this node is refering to in
-// the input document. Use Parser.Raw() to retrieve the actual bytes.
-type Node struct {
- Kind Kind
- Raw Range // Raw bytes from the input.
- Data []byte // Node value (either allocated or referencing the input).
-
- // References to other nodes, as offsets in the backing array
- // from this node. References can go backward, so those can be
- // negative.
- next int // 0 if last element
- child int // 0 if no child
-}
-
-// Range of bytes in the document.
-type Range struct {
- Offset uint32
- Length uint32
-}
-
-// Next returns a pointer to the next node, or nil if there is no next node.
-func (n *Node) Next() *Node {
- if n.next == 0 {
- return nil
- }
- ptr := unsafe.Pointer(n)
- size := unsafe.Sizeof(Node{})
- return (*Node)(danger.Stride(ptr, size, n.next))
-}
-
-// Child returns a pointer to the first child node of this node. Other children
-// can be accessed calling Next on the first child. Returns an nil if this Node
-// has no child.
-func (n *Node) Child() *Node {
- if n.child == 0 {
- return nil
- }
- ptr := unsafe.Pointer(n)
- size := unsafe.Sizeof(Node{})
- return (*Node)(danger.Stride(ptr, size, n.child))
-}
-
-// Valid returns true if the node's kind is set (not to Invalid).
-func (n *Node) Valid() bool {
- return n != nil
-}
-
-// Key returns the children nodes making the Key on a supported node. Panics
-// otherwise. They are guaranteed to be all be of the Kind Key. A simple key
-// would return just one element.
-func (n *Node) Key() Iterator {
- switch n.Kind {
- case KeyValue:
- value := n.Child()
- if !value.Valid() {
- panic(fmt.Errorf("KeyValue should have at least two children"))
- }
- return Iterator{node: value.Next()}
- case Table, ArrayTable:
- return Iterator{node: n.Child()}
- default:
- panic(fmt.Errorf("Key() is not supported on a %s", n.Kind))
- }
-}
-
-// Value returns a pointer to the value node of a KeyValue.
-// Guaranteed to be non-nil. Panics if not called on a KeyValue node,
-// or if the Children are malformed.
-func (n *Node) Value() *Node {
- return n.Child()
-}
-
-// Children returns an iterator over a node's children.
-func (n *Node) Children() Iterator {
- return Iterator{node: n.Child()}
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/unstable/builder.go b/vendor/github.com/pelletier/go-toml/v2/unstable/builder.go
deleted file mode 100644
index 9538e30d..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unstable/builder.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package unstable
-
-// root contains a full AST.
-//
-// It is immutable once constructed with Builder.
-type root struct {
- nodes []Node
-}
-
-// Iterator over the top level nodes.
-func (r *root) Iterator() Iterator {
- it := Iterator{}
- if len(r.nodes) > 0 {
- it.node = &r.nodes[0]
- }
- return it
-}
-
-func (r *root) at(idx reference) *Node {
- return &r.nodes[idx]
-}
-
-type reference int
-
-const invalidReference reference = -1
-
-func (r reference) Valid() bool {
- return r != invalidReference
-}
-
-type builder struct {
- tree root
- lastIdx int
-}
-
-func (b *builder) Tree() *root {
- return &b.tree
-}
-
-func (b *builder) NodeAt(ref reference) *Node {
- return b.tree.at(ref)
-}
-
-func (b *builder) Reset() {
- b.tree.nodes = b.tree.nodes[:0]
- b.lastIdx = 0
-}
-
-func (b *builder) Push(n Node) reference {
- b.lastIdx = len(b.tree.nodes)
- b.tree.nodes = append(b.tree.nodes, n)
- return reference(b.lastIdx)
-}
-
-func (b *builder) PushAndChain(n Node) reference {
- newIdx := len(b.tree.nodes)
- b.tree.nodes = append(b.tree.nodes, n)
- if b.lastIdx >= 0 {
- b.tree.nodes[b.lastIdx].next = newIdx - b.lastIdx
- }
- b.lastIdx = newIdx
- return reference(b.lastIdx)
-}
-
-func (b *builder) AttachChild(parent reference, child reference) {
- b.tree.nodes[parent].child = int(child) - int(parent)
-}
-
-func (b *builder) Chain(from reference, to reference) {
- b.tree.nodes[from].next = int(to) - int(from)
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/unstable/doc.go b/vendor/github.com/pelletier/go-toml/v2/unstable/doc.go
deleted file mode 100644
index 7ff26c53..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unstable/doc.go
+++ /dev/null
@@ -1,3 +0,0 @@
-// Package unstable provides APIs that do not meet the backward compatibility
-// guarantees yet.
-package unstable
diff --git a/vendor/github.com/pelletier/go-toml/v2/unstable/kind.go b/vendor/github.com/pelletier/go-toml/v2/unstable/kind.go
deleted file mode 100644
index ff9df1be..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unstable/kind.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package unstable
-
-import "fmt"
-
-// Kind represents the type of TOML structure contained in a given Node.
-type Kind int
-
-const (
- // Meta
- Invalid Kind = iota
- Comment
- Key
-
- // Top level structures
- Table
- ArrayTable
- KeyValue
-
- // Containers values
- Array
- InlineTable
-
- // Values
- String
- Bool
- Float
- Integer
- LocalDate
- LocalTime
- LocalDateTime
- DateTime
-)
-
-// String implementation of fmt.Stringer.
-func (k Kind) String() string {
- switch k {
- case Invalid:
- return "Invalid"
- case Comment:
- return "Comment"
- case Key:
- return "Key"
- case Table:
- return "Table"
- case ArrayTable:
- return "ArrayTable"
- case KeyValue:
- return "KeyValue"
- case Array:
- return "Array"
- case InlineTable:
- return "InlineTable"
- case String:
- return "String"
- case Bool:
- return "Bool"
- case Float:
- return "Float"
- case Integer:
- return "Integer"
- case LocalDate:
- return "LocalDate"
- case LocalTime:
- return "LocalTime"
- case LocalDateTime:
- return "LocalDateTime"
- case DateTime:
- return "DateTime"
- }
- panic(fmt.Errorf("Kind.String() not implemented for '%d'", k))
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/unstable/parser.go b/vendor/github.com/pelletier/go-toml/v2/unstable/parser.go
deleted file mode 100644
index 52db88e7..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unstable/parser.go
+++ /dev/null
@@ -1,1147 +0,0 @@
-package unstable
-
-import (
- "bytes"
- "fmt"
- "unicode"
-
- "github.com/pelletier/go-toml/v2/internal/characters"
- "github.com/pelletier/go-toml/v2/internal/danger"
-)
-
-// ParserError describes an error relative to the content of the document.
-//
-// It cannot outlive the instance of Parser it refers to, and may cause panics
-// if the parser is reset.
-type ParserError struct {
- Highlight []byte
- Message string
- Key []string // optional
-}
-
-// Error is the implementation of the error interface.
-func (e *ParserError) Error() string {
- return e.Message
-}
-
-// NewParserError is a convenience function to create a ParserError
-//
-// Warning: Highlight needs to be a subslice of Parser.data, so only slices
-// returned by Parser.Raw are valid candidates.
-func NewParserError(highlight []byte, format string, args ...interface{}) error {
- return &ParserError{
- Highlight: highlight,
- Message: fmt.Errorf(format, args...).Error(),
- }
-}
-
-// Parser scans over a TOML-encoded document and generates an iterative AST.
-//
-// To prime the Parser, first reset it with the contents of a TOML document.
-// Then, process all top-level expressions sequentially. See Example.
-//
-// Don't forget to check Error() after you're done parsing.
-//
-// Each top-level expression needs to be fully processed before calling
-// NextExpression() again. Otherwise, calls to various Node methods may panic if
-// the parser has moved on the next expression.
-//
-// For performance reasons, go-toml doesn't make a copy of the input bytes to
-// the parser. Make sure to copy all the bytes you need to outlive the slice
-// given to the parser.
-//
-// The parser doesn't provide nodes for comments yet, nor for whitespace.
-type Parser struct {
- data []byte
- builder builder
- ref reference
- left []byte
- err error
- first bool
-}
-
-// Data returns the slice provided to the last call to Reset.
-func (p *Parser) Data() []byte {
- return p.data
-}
-
-// Range returns a range description that corresponds to a given slice of the
-// input. If the argument is not a subslice of the parser input, this function
-// panics.
-func (p *Parser) Range(b []byte) Range {
- return Range{
- Offset: uint32(danger.SubsliceOffset(p.data, b)),
- Length: uint32(len(b)),
- }
-}
-
-// Raw returns the slice corresponding to the bytes in the given range.
-func (p *Parser) Raw(raw Range) []byte {
- return p.data[raw.Offset : raw.Offset+raw.Length]
-}
-
-// Reset brings the parser to its initial state for a given input. It wipes an
-// reuses internal storage to reduce allocation.
-func (p *Parser) Reset(b []byte) {
- p.builder.Reset()
- p.ref = invalidReference
- p.data = b
- p.left = b
- p.err = nil
- p.first = true
-}
-
-// NextExpression parses the next top-level expression. If an expression was
-// successfully parsed, it returns true. If the parser is at the end of the
-// document or an error occurred, it returns false.
-//
-// Retrieve the parsed expression with Expression().
-func (p *Parser) NextExpression() bool {
- if len(p.left) == 0 || p.err != nil {
- return false
- }
-
- p.builder.Reset()
- p.ref = invalidReference
-
- for {
- if len(p.left) == 0 || p.err != nil {
- return false
- }
-
- if !p.first {
- p.left, p.err = p.parseNewline(p.left)
- }
-
- if len(p.left) == 0 || p.err != nil {
- return false
- }
-
- p.ref, p.left, p.err = p.parseExpression(p.left)
-
- if p.err != nil {
- return false
- }
-
- p.first = false
-
- if p.ref.Valid() {
- return true
- }
- }
-}
-
-// Expression returns a pointer to the node representing the last successfully
-// parsed expresion.
-func (p *Parser) Expression() *Node {
- return p.builder.NodeAt(p.ref)
-}
-
-// Error returns any error that has occured during parsing.
-func (p *Parser) Error() error {
- return p.err
-}
-
-func (p *Parser) parseNewline(b []byte) ([]byte, error) {
- if b[0] == '\n' {
- return b[1:], nil
- }
-
- if b[0] == '\r' {
- _, rest, err := scanWindowsNewline(b)
- return rest, err
- }
-
- return nil, NewParserError(b[0:1], "expected newline but got %#U", b[0])
-}
-
-func (p *Parser) parseExpression(b []byte) (reference, []byte, error) {
- // expression = ws [ comment ]
- // expression =/ ws keyval ws [ comment ]
- // expression =/ ws table ws [ comment ]
- ref := invalidReference
-
- b = p.parseWhitespace(b)
-
- if len(b) == 0 {
- return ref, b, nil
- }
-
- if b[0] == '#' {
- _, rest, err := scanComment(b)
- return ref, rest, err
- }
-
- if b[0] == '\n' || b[0] == '\r' {
- return ref, b, nil
- }
-
- var err error
- if b[0] == '[' {
- ref, b, err = p.parseTable(b)
- } else {
- ref, b, err = p.parseKeyval(b)
- }
-
- if err != nil {
- return ref, nil, err
- }
-
- b = p.parseWhitespace(b)
-
- if len(b) > 0 && b[0] == '#' {
- _, rest, err := scanComment(b)
- return ref, rest, err
- }
-
- return ref, b, nil
-}
-
-func (p *Parser) parseTable(b []byte) (reference, []byte, error) {
- // table = std-table / array-table
- if len(b) > 1 && b[1] == '[' {
- return p.parseArrayTable(b)
- }
-
- return p.parseStdTable(b)
-}
-
-func (p *Parser) parseArrayTable(b []byte) (reference, []byte, error) {
- // array-table = array-table-open key array-table-close
- // array-table-open = %x5B.5B ws ; [[ Double left square bracket
- // array-table-close = ws %x5D.5D ; ]] Double right square bracket
- ref := p.builder.Push(Node{
- Kind: ArrayTable,
- })
-
- b = b[2:]
- b = p.parseWhitespace(b)
-
- k, b, err := p.parseKey(b)
- if err != nil {
- return ref, nil, err
- }
-
- p.builder.AttachChild(ref, k)
- b = p.parseWhitespace(b)
-
- b, err = expect(']', b)
- if err != nil {
- return ref, nil, err
- }
-
- b, err = expect(']', b)
-
- return ref, b, err
-}
-
-func (p *Parser) parseStdTable(b []byte) (reference, []byte, error) {
- // std-table = std-table-open key std-table-close
- // std-table-open = %x5B ws ; [ Left square bracket
- // std-table-close = ws %x5D ; ] Right square bracket
- ref := p.builder.Push(Node{
- Kind: Table,
- })
-
- b = b[1:]
- b = p.parseWhitespace(b)
-
- key, b, err := p.parseKey(b)
- if err != nil {
- return ref, nil, err
- }
-
- p.builder.AttachChild(ref, key)
-
- b = p.parseWhitespace(b)
-
- b, err = expect(']', b)
-
- return ref, b, err
-}
-
-func (p *Parser) parseKeyval(b []byte) (reference, []byte, error) {
- // keyval = key keyval-sep val
- ref := p.builder.Push(Node{
- Kind: KeyValue,
- })
-
- key, b, err := p.parseKey(b)
- if err != nil {
- return invalidReference, nil, err
- }
-
- // keyval-sep = ws %x3D ws ; =
-
- b = p.parseWhitespace(b)
-
- if len(b) == 0 {
- return invalidReference, nil, NewParserError(b, "expected = after a key, but the document ends there")
- }
-
- b, err = expect('=', b)
- if err != nil {
- return invalidReference, nil, err
- }
-
- b = p.parseWhitespace(b)
-
- valRef, b, err := p.parseVal(b)
- if err != nil {
- return ref, b, err
- }
-
- p.builder.Chain(valRef, key)
- p.builder.AttachChild(ref, valRef)
-
- return ref, b, err
-}
-
-//nolint:cyclop,funlen
-func (p *Parser) parseVal(b []byte) (reference, []byte, error) {
- // val = string / boolean / array / inline-table / date-time / float / integer
- ref := invalidReference
-
- if len(b) == 0 {
- return ref, nil, NewParserError(b, "expected value, not eof")
- }
-
- var err error
- c := b[0]
-
- switch c {
- case '"':
- var raw []byte
- var v []byte
- if scanFollowsMultilineBasicStringDelimiter(b) {
- raw, v, b, err = p.parseMultilineBasicString(b)
- } else {
- raw, v, b, err = p.parseBasicString(b)
- }
-
- if err == nil {
- ref = p.builder.Push(Node{
- Kind: String,
- Raw: p.Range(raw),
- Data: v,
- })
- }
-
- return ref, b, err
- case '\'':
- var raw []byte
- var v []byte
- if scanFollowsMultilineLiteralStringDelimiter(b) {
- raw, v, b, err = p.parseMultilineLiteralString(b)
- } else {
- raw, v, b, err = p.parseLiteralString(b)
- }
-
- if err == nil {
- ref = p.builder.Push(Node{
- Kind: String,
- Raw: p.Range(raw),
- Data: v,
- })
- }
-
- return ref, b, err
- case 't':
- if !scanFollowsTrue(b) {
- return ref, nil, NewParserError(atmost(b, 4), "expected 'true'")
- }
-
- ref = p.builder.Push(Node{
- Kind: Bool,
- Data: b[:4],
- })
-
- return ref, b[4:], nil
- case 'f':
- if !scanFollowsFalse(b) {
- return ref, nil, NewParserError(atmost(b, 5), "expected 'false'")
- }
-
- ref = p.builder.Push(Node{
- Kind: Bool,
- Data: b[:5],
- })
-
- return ref, b[5:], nil
- case '[':
- return p.parseValArray(b)
- case '{':
- return p.parseInlineTable(b)
- default:
- return p.parseIntOrFloatOrDateTime(b)
- }
-}
-
-func atmost(b []byte, n int) []byte {
- if n >= len(b) {
- return b
- }
-
- return b[:n]
-}
-
-func (p *Parser) parseLiteralString(b []byte) ([]byte, []byte, []byte, error) {
- v, rest, err := scanLiteralString(b)
- if err != nil {
- return nil, nil, nil, err
- }
-
- return v, v[1 : len(v)-1], rest, nil
-}
-
-func (p *Parser) parseInlineTable(b []byte) (reference, []byte, error) {
- // inline-table = inline-table-open [ inline-table-keyvals ] inline-table-close
- // inline-table-open = %x7B ws ; {
- // inline-table-close = ws %x7D ; }
- // inline-table-sep = ws %x2C ws ; , Comma
- // inline-table-keyvals = keyval [ inline-table-sep inline-table-keyvals ]
- parent := p.builder.Push(Node{
- Kind: InlineTable,
- })
-
- first := true
-
- var child reference
-
- b = b[1:]
-
- var err error
-
- for len(b) > 0 {
- previousB := b
- b = p.parseWhitespace(b)
-
- if len(b) == 0 {
- return parent, nil, NewParserError(previousB[:1], "inline table is incomplete")
- }
-
- if b[0] == '}' {
- break
- }
-
- if !first {
- b, err = expect(',', b)
- if err != nil {
- return parent, nil, err
- }
- b = p.parseWhitespace(b)
- }
-
- var kv reference
-
- kv, b, err = p.parseKeyval(b)
- if err != nil {
- return parent, nil, err
- }
-
- if first {
- p.builder.AttachChild(parent, kv)
- } else {
- p.builder.Chain(child, kv)
- }
- child = kv
-
- first = false
- }
-
- rest, err := expect('}', b)
-
- return parent, rest, err
-}
-
-//nolint:funlen,cyclop
-func (p *Parser) parseValArray(b []byte) (reference, []byte, error) {
- // array = array-open [ array-values ] ws-comment-newline array-close
- // array-open = %x5B ; [
- // array-close = %x5D ; ]
- // array-values = ws-comment-newline val ws-comment-newline array-sep array-values
- // array-values =/ ws-comment-newline val ws-comment-newline [ array-sep ]
- // array-sep = %x2C ; , Comma
- // ws-comment-newline = *( wschar / [ comment ] newline )
- arrayStart := b
- b = b[1:]
-
- parent := p.builder.Push(Node{
- Kind: Array,
- })
-
- first := true
-
- var lastChild reference
-
- var err error
- for len(b) > 0 {
- b, err = p.parseOptionalWhitespaceCommentNewline(b)
- if err != nil {
- return parent, nil, err
- }
-
- if len(b) == 0 {
- return parent, nil, NewParserError(arrayStart[:1], "array is incomplete")
- }
-
- if b[0] == ']' {
- break
- }
-
- if b[0] == ',' {
- if first {
- return parent, nil, NewParserError(b[0:1], "array cannot start with comma")
- }
- b = b[1:]
-
- b, err = p.parseOptionalWhitespaceCommentNewline(b)
- if err != nil {
- return parent, nil, err
- }
- } else if !first {
- return parent, nil, NewParserError(b[0:1], "array elements must be separated by commas")
- }
-
- // TOML allows trailing commas in arrays.
- if len(b) > 0 && b[0] == ']' {
- break
- }
-
- var valueRef reference
- valueRef, b, err = p.parseVal(b)
- if err != nil {
- return parent, nil, err
- }
-
- if first {
- p.builder.AttachChild(parent, valueRef)
- } else {
- p.builder.Chain(lastChild, valueRef)
- }
- lastChild = valueRef
-
- b, err = p.parseOptionalWhitespaceCommentNewline(b)
- if err != nil {
- return parent, nil, err
- }
- first = false
- }
-
- rest, err := expect(']', b)
-
- return parent, rest, err
-}
-
-func (p *Parser) parseOptionalWhitespaceCommentNewline(b []byte) ([]byte, error) {
- for len(b) > 0 {
- var err error
- b = p.parseWhitespace(b)
-
- if len(b) > 0 && b[0] == '#' {
- _, b, err = scanComment(b)
- if err != nil {
- return nil, err
- }
- }
-
- if len(b) == 0 {
- break
- }
-
- if b[0] == '\n' || b[0] == '\r' {
- b, err = p.parseNewline(b)
- if err != nil {
- return nil, err
- }
- } else {
- break
- }
- }
-
- return b, nil
-}
-
-func (p *Parser) parseMultilineLiteralString(b []byte) ([]byte, []byte, []byte, error) {
- token, rest, err := scanMultilineLiteralString(b)
- if err != nil {
- return nil, nil, nil, err
- }
-
- i := 3
-
- // skip the immediate new line
- if token[i] == '\n' {
- i++
- } else if token[i] == '\r' && token[i+1] == '\n' {
- i += 2
- }
-
- return token, token[i : len(token)-3], rest, err
-}
-
-//nolint:funlen,gocognit,cyclop
-func (p *Parser) parseMultilineBasicString(b []byte) ([]byte, []byte, []byte, error) {
- // ml-basic-string = ml-basic-string-delim [ newline ] ml-basic-body
- // ml-basic-string-delim
- // ml-basic-string-delim = 3quotation-mark
- // ml-basic-body = *mlb-content *( mlb-quotes 1*mlb-content ) [ mlb-quotes ]
- //
- // mlb-content = mlb-char / newline / mlb-escaped-nl
- // mlb-char = mlb-unescaped / escaped
- // mlb-quotes = 1*2quotation-mark
- // mlb-unescaped = wschar / %x21 / %x23-5B / %x5D-7E / non-ascii
- // mlb-escaped-nl = escape ws newline *( wschar / newline )
- token, escaped, rest, err := scanMultilineBasicString(b)
- if err != nil {
- return nil, nil, nil, err
- }
-
- i := 3
-
- // skip the immediate new line
- if token[i] == '\n' {
- i++
- } else if token[i] == '\r' && token[i+1] == '\n' {
- i += 2
- }
-
- // fast path
- startIdx := i
- endIdx := len(token) - len(`"""`)
-
- if !escaped {
- str := token[startIdx:endIdx]
- verr := characters.Utf8TomlValidAlreadyEscaped(str)
- if verr.Zero() {
- return token, str, rest, nil
- }
- return nil, nil, nil, NewParserError(str[verr.Index:verr.Index+verr.Size], "invalid UTF-8")
- }
-
- var builder bytes.Buffer
-
- // The scanner ensures that the token starts and ends with quotes and that
- // escapes are balanced.
- for i < len(token)-3 {
- c := token[i]
-
- //nolint:nestif
- if c == '\\' {
- // When the last non-whitespace character on a line is an unescaped \,
- // it will be trimmed along with all whitespace (including newlines) up
- // to the next non-whitespace character or closing delimiter.
-
- isLastNonWhitespaceOnLine := false
- j := 1
- findEOLLoop:
- for ; j < len(token)-3-i; j++ {
- switch token[i+j] {
- case ' ', '\t':
- continue
- case '\r':
- if token[i+j+1] == '\n' {
- continue
- }
- case '\n':
- isLastNonWhitespaceOnLine = true
- }
- break findEOLLoop
- }
- if isLastNonWhitespaceOnLine {
- i += j
- for ; i < len(token)-3; i++ {
- c := token[i]
- if !(c == '\n' || c == '\r' || c == ' ' || c == '\t') {
- i--
- break
- }
- }
- i++
- continue
- }
-
- // handle escaping
- i++
- c = token[i]
-
- switch c {
- case '"', '\\':
- builder.WriteByte(c)
- case 'b':
- builder.WriteByte('\b')
- case 'f':
- builder.WriteByte('\f')
- case 'n':
- builder.WriteByte('\n')
- case 'r':
- builder.WriteByte('\r')
- case 't':
- builder.WriteByte('\t')
- case 'e':
- builder.WriteByte(0x1B)
- case 'u':
- x, err := hexToRune(atmost(token[i+1:], 4), 4)
- if err != nil {
- return nil, nil, nil, err
- }
- builder.WriteRune(x)
- i += 4
- case 'U':
- x, err := hexToRune(atmost(token[i+1:], 8), 8)
- if err != nil {
- return nil, nil, nil, err
- }
-
- builder.WriteRune(x)
- i += 8
- default:
- return nil, nil, nil, NewParserError(token[i:i+1], "invalid escaped character %#U", c)
- }
- i++
- } else {
- size := characters.Utf8ValidNext(token[i:])
- if size == 0 {
- return nil, nil, nil, NewParserError(token[i:i+1], "invalid character %#U", c)
- }
- builder.Write(token[i : i+size])
- i += size
- }
- }
-
- return token, builder.Bytes(), rest, nil
-}
-
-func (p *Parser) parseKey(b []byte) (reference, []byte, error) {
- // key = simple-key / dotted-key
- // simple-key = quoted-key / unquoted-key
- //
- // unquoted-key = 1*( ALPHA / DIGIT / %x2D / %x5F ) ; A-Z / a-z / 0-9 / - / _
- // quoted-key = basic-string / literal-string
- // dotted-key = simple-key 1*( dot-sep simple-key )
- //
- // dot-sep = ws %x2E ws ; . Period
- raw, key, b, err := p.parseSimpleKey(b)
- if err != nil {
- return invalidReference, nil, err
- }
-
- ref := p.builder.Push(Node{
- Kind: Key,
- Raw: p.Range(raw),
- Data: key,
- })
-
- for {
- b = p.parseWhitespace(b)
- if len(b) > 0 && b[0] == '.' {
- b = p.parseWhitespace(b[1:])
-
- raw, key, b, err = p.parseSimpleKey(b)
- if err != nil {
- return ref, nil, err
- }
-
- p.builder.PushAndChain(Node{
- Kind: Key,
- Raw: p.Range(raw),
- Data: key,
- })
- } else {
- break
- }
- }
-
- return ref, b, nil
-}
-
-func (p *Parser) parseSimpleKey(b []byte) (raw, key, rest []byte, err error) {
- if len(b) == 0 {
- return nil, nil, nil, NewParserError(b, "expected key but found none")
- }
-
- // simple-key = quoted-key / unquoted-key
- // unquoted-key = 1*( ALPHA / DIGIT / %x2D / %x5F ) ; A-Z / a-z / 0-9 / - / _
- // quoted-key = basic-string / literal-string
- switch {
- case b[0] == '\'':
- return p.parseLiteralString(b)
- case b[0] == '"':
- return p.parseBasicString(b)
- case isUnquotedKeyChar(b[0]):
- key, rest = scanUnquotedKey(b)
- return key, key, rest, nil
- default:
- return nil, nil, nil, NewParserError(b[0:1], "invalid character at start of key: %c", b[0])
- }
-}
-
-//nolint:funlen,cyclop
-func (p *Parser) parseBasicString(b []byte) ([]byte, []byte, []byte, error) {
- // basic-string = quotation-mark *basic-char quotation-mark
- // quotation-mark = %x22 ; "
- // basic-char = basic-unescaped / escaped
- // basic-unescaped = wschar / %x21 / %x23-5B / %x5D-7E / non-ascii
- // escaped = escape escape-seq-char
- // escape-seq-char = %x22 ; " quotation mark U+0022
- // escape-seq-char =/ %x5C ; \ reverse solidus U+005C
- // escape-seq-char =/ %x62 ; b backspace U+0008
- // escape-seq-char =/ %x66 ; f form feed U+000C
- // escape-seq-char =/ %x6E ; n line feed U+000A
- // escape-seq-char =/ %x72 ; r carriage return U+000D
- // escape-seq-char =/ %x74 ; t tab U+0009
- // escape-seq-char =/ %x75 4HEXDIG ; uXXXX U+XXXX
- // escape-seq-char =/ %x55 8HEXDIG ; UXXXXXXXX U+XXXXXXXX
- token, escaped, rest, err := scanBasicString(b)
- if err != nil {
- return nil, nil, nil, err
- }
-
- startIdx := len(`"`)
- endIdx := len(token) - len(`"`)
-
- // Fast path. If there is no escape sequence, the string should just be
- // an UTF-8 encoded string, which is the same as Go. In that case,
- // validate the string and return a direct reference to the buffer.
- if !escaped {
- str := token[startIdx:endIdx]
- verr := characters.Utf8TomlValidAlreadyEscaped(str)
- if verr.Zero() {
- return token, str, rest, nil
- }
- return nil, nil, nil, NewParserError(str[verr.Index:verr.Index+verr.Size], "invalid UTF-8")
- }
-
- i := startIdx
-
- var builder bytes.Buffer
-
- // The scanner ensures that the token starts and ends with quotes and that
- // escapes are balanced.
- for i < len(token)-1 {
- c := token[i]
- if c == '\\' {
- i++
- c = token[i]
-
- switch c {
- case '"', '\\':
- builder.WriteByte(c)
- case 'b':
- builder.WriteByte('\b')
- case 'f':
- builder.WriteByte('\f')
- case 'n':
- builder.WriteByte('\n')
- case 'r':
- builder.WriteByte('\r')
- case 't':
- builder.WriteByte('\t')
- case 'e':
- builder.WriteByte(0x1B)
- case 'u':
- x, err := hexToRune(token[i+1:len(token)-1], 4)
- if err != nil {
- return nil, nil, nil, err
- }
-
- builder.WriteRune(x)
- i += 4
- case 'U':
- x, err := hexToRune(token[i+1:len(token)-1], 8)
- if err != nil {
- return nil, nil, nil, err
- }
-
- builder.WriteRune(x)
- i += 8
- default:
- return nil, nil, nil, NewParserError(token[i:i+1], "invalid escaped character %#U", c)
- }
- i++
- } else {
- size := characters.Utf8ValidNext(token[i:])
- if size == 0 {
- return nil, nil, nil, NewParserError(token[i:i+1], "invalid character %#U", c)
- }
- builder.Write(token[i : i+size])
- i += size
- }
- }
-
- return token, builder.Bytes(), rest, nil
-}
-
-func hexToRune(b []byte, length int) (rune, error) {
- if len(b) < length {
- return -1, NewParserError(b, "unicode point needs %d character, not %d", length, len(b))
- }
- b = b[:length]
-
- var r uint32
- for i, c := range b {
- d := uint32(0)
- switch {
- case '0' <= c && c <= '9':
- d = uint32(c - '0')
- case 'a' <= c && c <= 'f':
- d = uint32(c - 'a' + 10)
- case 'A' <= c && c <= 'F':
- d = uint32(c - 'A' + 10)
- default:
- return -1, NewParserError(b[i:i+1], "non-hex character")
- }
- r = r*16 + d
- }
-
- if r > unicode.MaxRune || 0xD800 <= r && r < 0xE000 {
- return -1, NewParserError(b, "escape sequence is invalid Unicode code point")
- }
-
- return rune(r), nil
-}
-
-func (p *Parser) parseWhitespace(b []byte) []byte {
- // ws = *wschar
- // wschar = %x20 ; Space
- // wschar =/ %x09 ; Horizontal tab
- _, rest := scanWhitespace(b)
-
- return rest
-}
-
-//nolint:cyclop
-func (p *Parser) parseIntOrFloatOrDateTime(b []byte) (reference, []byte, error) {
- switch b[0] {
- case 'i':
- if !scanFollowsInf(b) {
- return invalidReference, nil, NewParserError(atmost(b, 3), "expected 'inf'")
- }
-
- return p.builder.Push(Node{
- Kind: Float,
- Data: b[:3],
- }), b[3:], nil
- case 'n':
- if !scanFollowsNan(b) {
- return invalidReference, nil, NewParserError(atmost(b, 3), "expected 'nan'")
- }
-
- return p.builder.Push(Node{
- Kind: Float,
- Data: b[:3],
- }), b[3:], nil
- case '+', '-':
- return p.scanIntOrFloat(b)
- }
-
- if len(b) < 3 {
- return p.scanIntOrFloat(b)
- }
-
- s := 5
- if len(b) < s {
- s = len(b)
- }
-
- for idx, c := range b[:s] {
- if isDigit(c) {
- continue
- }
-
- if idx == 2 && c == ':' || (idx == 4 && c == '-') {
- return p.scanDateTime(b)
- }
-
- break
- }
-
- return p.scanIntOrFloat(b)
-}
-
-func (p *Parser) scanDateTime(b []byte) (reference, []byte, error) {
- // scans for contiguous characters in [0-9T:Z.+-], and up to one space if
- // followed by a digit.
- hasDate := false
- hasTime := false
- hasTz := false
- seenSpace := false
-
- i := 0
-byteLoop:
- for ; i < len(b); i++ {
- c := b[i]
-
- switch {
- case isDigit(c):
- case c == '-':
- hasDate = true
- const minOffsetOfTz = 8
- if i >= minOffsetOfTz {
- hasTz = true
- }
- case c == 'T' || c == 't' || c == ':' || c == '.':
- hasTime = true
- case c == '+' || c == '-' || c == 'Z' || c == 'z':
- hasTz = true
- case c == ' ':
- if !seenSpace && i+1 < len(b) && isDigit(b[i+1]) {
- i += 2
- // Avoid reaching past the end of the document in case the time
- // is malformed. See TestIssue585.
- if i >= len(b) {
- i--
- }
- seenSpace = true
- hasTime = true
- } else {
- break byteLoop
- }
- default:
- break byteLoop
- }
- }
-
- var kind Kind
-
- if hasTime {
- if hasDate {
- if hasTz {
- kind = DateTime
- } else {
- kind = LocalDateTime
- }
- } else {
- kind = LocalTime
- }
- } else {
- kind = LocalDate
- }
-
- return p.builder.Push(Node{
- Kind: kind,
- Data: b[:i],
- }), b[i:], nil
-}
-
-//nolint:funlen,gocognit,cyclop
-func (p *Parser) scanIntOrFloat(b []byte) (reference, []byte, error) {
- i := 0
-
- if len(b) > 2 && b[0] == '0' && b[1] != '.' && b[1] != 'e' && b[1] != 'E' {
- var isValidRune validRuneFn
-
- switch b[1] {
- case 'x':
- isValidRune = isValidHexRune
- case 'o':
- isValidRune = isValidOctalRune
- case 'b':
- isValidRune = isValidBinaryRune
- default:
- i++
- }
-
- if isValidRune != nil {
- i += 2
- for ; i < len(b); i++ {
- if !isValidRune(b[i]) {
- break
- }
- }
- }
-
- return p.builder.Push(Node{
- Kind: Integer,
- Data: b[:i],
- }), b[i:], nil
- }
-
- isFloat := false
-
- for ; i < len(b); i++ {
- c := b[i]
-
- if c >= '0' && c <= '9' || c == '+' || c == '-' || c == '_' {
- continue
- }
-
- if c == '.' || c == 'e' || c == 'E' {
- isFloat = true
-
- continue
- }
-
- if c == 'i' {
- if scanFollowsInf(b[i:]) {
- return p.builder.Push(Node{
- Kind: Float,
- Data: b[:i+3],
- }), b[i+3:], nil
- }
-
- return invalidReference, nil, NewParserError(b[i:i+1], "unexpected character 'i' while scanning for a number")
- }
-
- if c == 'n' {
- if scanFollowsNan(b[i:]) {
- return p.builder.Push(Node{
- Kind: Float,
- Data: b[:i+3],
- }), b[i+3:], nil
- }
-
- return invalidReference, nil, NewParserError(b[i:i+1], "unexpected character 'n' while scanning for a number")
- }
-
- break
- }
-
- if i == 0 {
- return invalidReference, b, NewParserError(b, "incomplete number")
- }
-
- kind := Integer
-
- if isFloat {
- kind = Float
- }
-
- return p.builder.Push(Node{
- Kind: kind,
- Data: b[:i],
- }), b[i:], nil
-}
-
-func isDigit(r byte) bool {
- return r >= '0' && r <= '9'
-}
-
-type validRuneFn func(r byte) bool
-
-func isValidHexRune(r byte) bool {
- return r >= 'a' && r <= 'f' ||
- r >= 'A' && r <= 'F' ||
- r >= '0' && r <= '9' ||
- r == '_'
-}
-
-func isValidOctalRune(r byte) bool {
- return r >= '0' && r <= '7' || r == '_'
-}
-
-func isValidBinaryRune(r byte) bool {
- return r == '0' || r == '1' || r == '_'
-}
-
-func expect(x byte, b []byte) ([]byte, error) {
- if len(b) == 0 {
- return nil, NewParserError(b, "expected character %c but the document ended here", x)
- }
-
- if b[0] != x {
- return nil, NewParserError(b[0:1], "expected character %c", x)
- }
-
- return b[1:], nil
-}
diff --git a/vendor/github.com/pelletier/go-toml/v2/unstable/scanner.go b/vendor/github.com/pelletier/go-toml/v2/unstable/scanner.go
deleted file mode 100644
index af22ebbe..00000000
--- a/vendor/github.com/pelletier/go-toml/v2/unstable/scanner.go
+++ /dev/null
@@ -1,271 +0,0 @@
-package unstable
-
-import "github.com/pelletier/go-toml/v2/internal/characters"
-
-func scanFollows(b []byte, pattern string) bool {
- n := len(pattern)
-
- return len(b) >= n && string(b[:n]) == pattern
-}
-
-func scanFollowsMultilineBasicStringDelimiter(b []byte) bool {
- return scanFollows(b, `"""`)
-}
-
-func scanFollowsMultilineLiteralStringDelimiter(b []byte) bool {
- return scanFollows(b, `'''`)
-}
-
-func scanFollowsTrue(b []byte) bool {
- return scanFollows(b, `true`)
-}
-
-func scanFollowsFalse(b []byte) bool {
- return scanFollows(b, `false`)
-}
-
-func scanFollowsInf(b []byte) bool {
- return scanFollows(b, `inf`)
-}
-
-func scanFollowsNan(b []byte) bool {
- return scanFollows(b, `nan`)
-}
-
-func scanUnquotedKey(b []byte) ([]byte, []byte) {
- // unquoted-key = 1*( ALPHA / DIGIT / %x2D / %x5F ) ; A-Z / a-z / 0-9 / - / _
- for i := 0; i < len(b); i++ {
- if !isUnquotedKeyChar(b[i]) {
- return b[:i], b[i:]
- }
- }
-
- return b, b[len(b):]
-}
-
-func isUnquotedKeyChar(r byte) bool {
- return (r >= 'A' && r <= 'Z') || (r >= 'a' && r <= 'z') || (r >= '0' && r <= '9') || r == '-' || r == '_'
-}
-
-func scanLiteralString(b []byte) ([]byte, []byte, error) {
- // literal-string = apostrophe *literal-char apostrophe
- // apostrophe = %x27 ; ' apostrophe
- // literal-char = %x09 / %x20-26 / %x28-7E / non-ascii
- for i := 1; i < len(b); {
- switch b[i] {
- case '\'':
- return b[:i+1], b[i+1:], nil
- case '\n', '\r':
- return nil, nil, NewParserError(b[i:i+1], "literal strings cannot have new lines")
- }
- size := characters.Utf8ValidNext(b[i:])
- if size == 0 {
- return nil, nil, NewParserError(b[i:i+1], "invalid character")
- }
- i += size
- }
-
- return nil, nil, NewParserError(b[len(b):], "unterminated literal string")
-}
-
-func scanMultilineLiteralString(b []byte) ([]byte, []byte, error) {
- // ml-literal-string = ml-literal-string-delim [ newline ] ml-literal-body
- // ml-literal-string-delim
- // ml-literal-string-delim = 3apostrophe
- // ml-literal-body = *mll-content *( mll-quotes 1*mll-content ) [ mll-quotes ]
- //
- // mll-content = mll-char / newline
- // mll-char = %x09 / %x20-26 / %x28-7E / non-ascii
- // mll-quotes = 1*2apostrophe
- for i := 3; i < len(b); {
- switch b[i] {
- case '\'':
- if scanFollowsMultilineLiteralStringDelimiter(b[i:]) {
- i += 3
-
- // At that point we found 3 apostrophe, and i is the
- // index of the byte after the third one. The scanner
- // needs to be eager, because there can be an extra 2
- // apostrophe that can be accepted at the end of the
- // string.
-
- if i >= len(b) || b[i] != '\'' {
- return b[:i], b[i:], nil
- }
- i++
-
- if i >= len(b) || b[i] != '\'' {
- return b[:i], b[i:], nil
- }
- i++
-
- if i < len(b) && b[i] == '\'' {
- return nil, nil, NewParserError(b[i-3:i+1], "''' not allowed in multiline literal string")
- }
-
- return b[:i], b[i:], nil
- }
- case '\r':
- if len(b) < i+2 {
- return nil, nil, NewParserError(b[len(b):], `need a \n after \r`)
- }
- if b[i+1] != '\n' {
- return nil, nil, NewParserError(b[i:i+2], `need a \n after \r`)
- }
- i += 2 // skip the \n
- continue
- }
- size := characters.Utf8ValidNext(b[i:])
- if size == 0 {
- return nil, nil, NewParserError(b[i:i+1], "invalid character")
- }
- i += size
- }
-
- return nil, nil, NewParserError(b[len(b):], `multiline literal string not terminated by '''`)
-}
-
-func scanWindowsNewline(b []byte) ([]byte, []byte, error) {
- const lenCRLF = 2
- if len(b) < lenCRLF {
- return nil, nil, NewParserError(b, "windows new line expected")
- }
-
- if b[1] != '\n' {
- return nil, nil, NewParserError(b, `windows new line should be \r\n`)
- }
-
- return b[:lenCRLF], b[lenCRLF:], nil
-}
-
-func scanWhitespace(b []byte) ([]byte, []byte) {
- for i := 0; i < len(b); i++ {
- switch b[i] {
- case ' ', '\t':
- continue
- default:
- return b[:i], b[i:]
- }
- }
-
- return b, b[len(b):]
-}
-
-//nolint:unparam
-func scanComment(b []byte) ([]byte, []byte, error) {
- // comment-start-symbol = %x23 ; #
- // non-ascii = %x80-D7FF / %xE000-10FFFF
- // non-eol = %x09 / %x20-7F / non-ascii
- //
- // comment = comment-start-symbol *non-eol
-
- for i := 1; i < len(b); {
- if b[i] == '\n' {
- return b[:i], b[i:], nil
- }
- if b[i] == '\r' {
- if i+1 < len(b) && b[i+1] == '\n' {
- return b[:i+1], b[i+1:], nil
- }
- return nil, nil, NewParserError(b[i:i+1], "invalid character in comment")
- }
- size := characters.Utf8ValidNext(b[i:])
- if size == 0 {
- return nil, nil, NewParserError(b[i:i+1], "invalid character in comment")
- }
-
- i += size
- }
-
- return b, b[len(b):], nil
-}
-
-func scanBasicString(b []byte) ([]byte, bool, []byte, error) {
- // basic-string = quotation-mark *basic-char quotation-mark
- // quotation-mark = %x22 ; "
- // basic-char = basic-unescaped / escaped
- // basic-unescaped = wschar / %x21 / %x23-5B / %x5D-7E / non-ascii
- // escaped = escape escape-seq-char
- escaped := false
- i := 1
-
- for ; i < len(b); i++ {
- switch b[i] {
- case '"':
- return b[:i+1], escaped, b[i+1:], nil
- case '\n', '\r':
- return nil, escaped, nil, NewParserError(b[i:i+1], "basic strings cannot have new lines")
- case '\\':
- if len(b) < i+2 {
- return nil, escaped, nil, NewParserError(b[i:i+1], "need a character after \\")
- }
- escaped = true
- i++ // skip the next character
- }
- }
-
- return nil, escaped, nil, NewParserError(b[len(b):], `basic string not terminated by "`)
-}
-
-func scanMultilineBasicString(b []byte) ([]byte, bool, []byte, error) {
- // ml-basic-string = ml-basic-string-delim [ newline ] ml-basic-body
- // ml-basic-string-delim
- // ml-basic-string-delim = 3quotation-mark
- // ml-basic-body = *mlb-content *( mlb-quotes 1*mlb-content ) [ mlb-quotes ]
- //
- // mlb-content = mlb-char / newline / mlb-escaped-nl
- // mlb-char = mlb-unescaped / escaped
- // mlb-quotes = 1*2quotation-mark
- // mlb-unescaped = wschar / %x21 / %x23-5B / %x5D-7E / non-ascii
- // mlb-escaped-nl = escape ws newline *( wschar / newline )
-
- escaped := false
- i := 3
-
- for ; i < len(b); i++ {
- switch b[i] {
- case '"':
- if scanFollowsMultilineBasicStringDelimiter(b[i:]) {
- i += 3
-
- // At that point we found 3 apostrophe, and i is the
- // index of the byte after the third one. The scanner
- // needs to be eager, because there can be an extra 2
- // apostrophe that can be accepted at the end of the
- // string.
-
- if i >= len(b) || b[i] != '"' {
- return b[:i], escaped, b[i:], nil
- }
- i++
-
- if i >= len(b) || b[i] != '"' {
- return b[:i], escaped, b[i:], nil
- }
- i++
-
- if i < len(b) && b[i] == '"' {
- return nil, escaped, nil, NewParserError(b[i-3:i+1], `""" not allowed in multiline basic string`)
- }
-
- return b[:i], escaped, b[i:], nil
- }
- case '\\':
- if len(b) < i+2 {
- return nil, escaped, nil, NewParserError(b[len(b):], "need a character after \\")
- }
- escaped = true
- i++ // skip the next character
- case '\r':
- if len(b) < i+2 {
- return nil, escaped, nil, NewParserError(b[len(b):], `need a \n after \r`)
- }
- if b[i+1] != '\n' {
- return nil, escaped, nil, NewParserError(b[i:i+2], `need a \n after \r`)
- }
- i++ // skip the \n
- }
- }
-
- return nil, escaped, nil, NewParserError(b[len(b):], `multiline basic string not terminated by """`)
-}
diff --git a/vendor/github.com/perimeterx/marshmallow/.gitignore b/vendor/github.com/perimeterx/marshmallow/.gitignore
deleted file mode 100644
index cf53c0a1..00000000
--- a/vendor/github.com/perimeterx/marshmallow/.gitignore
+++ /dev/null
@@ -1,4 +0,0 @@
-/.idea
-
-coverage.out
-profile.out
diff --git a/vendor/github.com/perimeterx/marshmallow/LICENSE b/vendor/github.com/perimeterx/marshmallow/LICENSE
deleted file mode 100644
index 8ffe8691..00000000
--- a/vendor/github.com/perimeterx/marshmallow/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2022 PerimeterX
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/perimeterx/marshmallow/README.md b/vendor/github.com/perimeterx/marshmallow/README.md
deleted file mode 100644
index 2eee63fe..00000000
--- a/vendor/github.com/perimeterx/marshmallow/README.md
+++ /dev/null
@@ -1,196 +0,0 @@
-# Marshmallow
-
-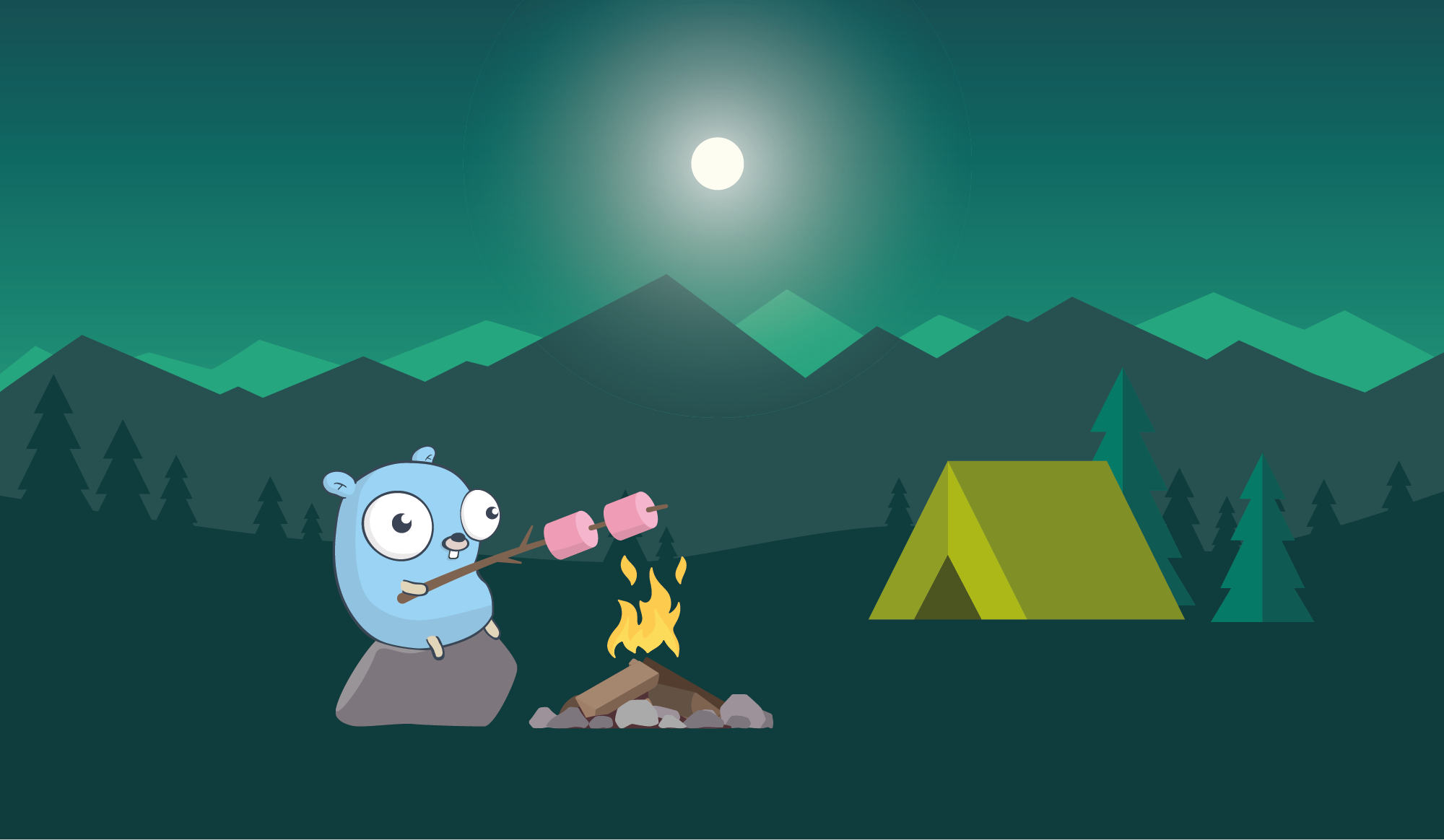
-
-[](https://github.com/PerimeterX/marshmallow/actions/workflows/codeql.yml?query=branch%3Amain++)
-[](https://github.com/PerimeterX/marshmallow/actions/workflows/go.yml?query=branch%3Amain)
-[](https://github.com/PerimeterX/marshmallow/actions/workflows/dependency-review.yml?query=branch%3Amain)
-[](https://goreportcard.com/report/github.com/perimeterx/marshmallow)
-
-[](https://pkg.go.dev/github.com/perimeterx/marshmallow)
-[](LICENSE)
-[](https://github.com/PerimeterX/marshmallow/releases)
-
-[](https://github.com/PerimeterX/marshmallow/issues)
-[](https://github.com/PerimeterX/marshmallow/pulls)
-[](https://github.com/PerimeterX/marshmallow/commits/main)
-
-
-
-Marshmallow package provides a simple API to perform flexible and performant JSON unmarshalling in Go.
-
-Marshmallow specializes in dealing with **unstructured struct** - when some fields are known and some aren't,
-with zero performance overhead nor extra coding needed.
-While unmarshalling, marshmallow allows fully retaining the original data and access
-it via a typed struct and a dynamic map.
-
-## Contents
-
-- [Install](#install)
-- [Usage](#usage)
-- [Performance Benchmark And Alternatives](#performance-benchmark-and-alternatives)
-- [When Should I Use Marshmallow](#when-should-i-use-marshmallow)
-- [API](#api)
-
-## Install
-
-```sh
-go get -u github.com/perimeterx/marshmallow
-```
-
-## Usage
-
-```go
-package main
-
-import (
- "fmt"
- "github.com/perimeterx/marshmallow"
-)
-
-func main() {
- marshmallow.EnableCache() // this is used to boost performance, read more below
- v := struct {
- Foo string `json:"foo"`
- Boo []int `json:"boo"`
- }{}
- result, err := marshmallow.Unmarshal([]byte(`{"foo":"bar","boo":[1,2,3],"goo":12.6}`), &v)
- fmt.Printf("v=%+v, result=%+v, err=%v", v, result, err)
- // Output: v={Foo:bar Boo:[1 2 3]}, result=map[boo:[1 2 3] foo:bar goo:12.6], err=
-}
-```
-
-## Performance Benchmark And Alternatives
-
-Marshmallow performs best when dealing with mixed data - when some fields are known and some are unknown.
-More info [below](#when-should-i-use-marshmallow).
-Other solutions are available for this kind of use case, each solution is explained and documented in the link below.
-The full benchmark test can be found
-[here](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go).
-
-|Benchmark|Iterations|Time/Iteration|Bytes Allocated|Allocations|
-|--|--|--|--|--|
-|[unmarshall twice](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L40)|228693|5164 ns/op|1640 B/op|51 allocs/op|
-|[raw map](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L66)|232236|5116 ns/op|2296 B/op|53 allocs/op|
-|[go codec](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L121)|388442|3077 ns/op|2512 B/op|37 allocs/op|
-|[marshmallow](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L16)|626168|1853 ns/op|608 B/op|18 allocs/op|
-|[marshmallow without populating struct](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L162)|678616|1751 ns/op|608 B/op|18 allocs/op|
-
-
-
-**Marshmallow provides the best performance (up to X3 faster) while not requiring any extra coding.**
-In fact, marshmallow performs as fast as normal `json.Unmarshal` call, however, such a call causes loss of data for all
-the fields that did not match the given struct. With marshmallow you never lose any data.
-
-|Benchmark|Iterations|Time/Iteration|Bytes Allocated|Allocations|
-|--|--|--|--|--|
-|[marshmallow](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L16)|626168|1853 ns/op|608 B/op|18 allocs/op|
-|[native library](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L143)|652106|1845 ns/op|304 B/op|11 allocs/op|
-|[marshmallow without populating struct](https://github.com/PerimeterX/marshmallow/blob/8c5bba9e6dc0033f4324eca554737089a99f6e5e/benchmark_test.go#L162)|678616|1751 ns/op|608 B/op|18 allocs/op|
-
-## When Should I Use Marshmallow
-
-Marshmallow is best suited for use cases where you are interested in all the input data, but you have predetermined
-information only about a subset of it. For instance, if you plan to reference two specific fields from the data, then
-iterate all the data and apply some generic logic. How does it look with the native library:
-
-```go
-func isAllowedToDrive(data []byte) (bool, error) {
- result := make(map[string]interface{})
- err := json.Unmarshal(data, &result)
- if err != nil {
- return false, err
- }
-
- age, ok := result["age"]
- if !ok {
- return false, nil
- }
- a, ok := age.(float64)
- if !ok {
- return false, nil
- }
- if a < 17 {
- return false, nil
- }
-
- hasDriversLicense, ok := result["has_drivers_license"]
- if !ok {
- return false, nil
- }
- h, ok := hasDriversLicense.(bool)
- if !ok {
- return false, nil
- }
- if !h {
- return false, nil
- }
-
- for key := range result {
- if strings.Contains(key, "prior_conviction") {
- return false, nil
- }
- }
-
- return true, nil
-}
-```
-
-And with marshmallow:
-
-```go
-func isAllowedToDrive(data []byte) (bool, error) {
- v := struct {
- Age int `json:"age"`
- HasDriversLicense bool `json:"has_drivers_license"`
- }{}
- result, err := marshmallow.Unmarshal(data, &v)
- if err != nil {
- return false, err
- }
-
- if v.Age < 17 || !v.HasDriversLicense {
- return false, nil
- }
-
- for key := range result {
- if strings.Contains(key, "prior_conviction") {
- return false, nil
- }
- }
-
- return true, nil
-}
-```
-
-## API
-
-Marshmallow exposes two main API functions -
-[Unmarshal](https://github.com/PerimeterX/marshmallow/blob/0e0218ab860be8a4b5f57f5ff239f281c250c5da/unmarshal.go#L27)
-and
-[UnmarshalFromJSONMap](https://github.com/PerimeterX/marshmallow/blob/0e0218ab860be8a4b5f57f5ff239f281c250c5da/unmarshal_from_json_map.go#L37).
-While unmarshalling, marshmallow supports the following optional options:
-
-* Setting the mode for handling invalid data using the [WithMode](https://github.com/PerimeterX/marshmallow/blob/0e0218ab860be8a4b5f57f5ff239f281c250c5da/options.go#L30) function.
-* Excluding known fields from the result map using the [WithExcludeKnownFieldsFromMap](https://github.com/PerimeterX/marshmallow/blob/457669ae9973895584f2636eabfc104140d3b700/options.go#L50) function.
-* Skipping struct population to boost performance using the [WithSkipPopulateStruct](https://github.com/PerimeterX/marshmallow/blob/0e0218ab860be8a4b5f57f5ff239f281c250c5da/options.go#L41) function.
-
-In order to capture unknown nested fields, structs must implement [JSONDataHandler](https://github.com/PerimeterX/marshmallow/blob/2d254bf2ed5f9b02cafb8ba6eaa726cba38bc92b/options.go#L65).
-More info [here](https://github.com/PerimeterX/marshmallow/issues/15).
-
-Marshmallow also supports caching of refection information using
-[EnableCache](https://github.com/PerimeterX/marshmallow/blob/d3500aa5b0f330942b178b155da933c035dd3906/cache.go#L40)
-and
-[EnableCustomCache](https://github.com/PerimeterX/marshmallow/blob/d3500aa5b0f330942b178b155da933c035dd3906/cache.go#L35).
-
-**Examples can be found [here](example_test.go)**
-
-# Marshmallow Logo
-
-Marshmallow logo and assets by [Adva Rom](https://www.linkedin.com/in/adva-rom-7a6738127/) are licensed under a Creative Commons Attribution 4.0 International License.
-
-## Contribute
-
-Any type of contribution is warmly welcome and appreciated â¤ï¸
-
-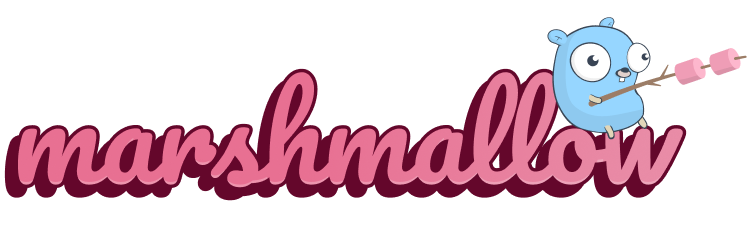
diff --git a/vendor/github.com/perimeterx/marshmallow/cache.go b/vendor/github.com/perimeterx/marshmallow/cache.go
deleted file mode 100644
index a67cea6d..00000000
--- a/vendor/github.com/perimeterx/marshmallow/cache.go
+++ /dev/null
@@ -1,63 +0,0 @@
-// Copyright 2022 PerimeterX. All rights reserved.
-// Use of this source code is governed by a MIT style
-// license that can be found in the LICENSE file.
-
-package marshmallow
-
-import (
- "reflect"
- "sync"
-)
-
-// Cache allows unmarshalling to use a cached version of refection information about types.
-// Cache interface follows the implementation of sync.Map, but you may wrap any cache implementation
-// to match it. This allows you to control max cache size, eviction policies and any other caching aspect.
-type Cache interface {
- // Load returns the value stored in the map for a key, or nil if no value is present.
- // The ok result indicates whether value was found in the map.
- Load(key interface{}) (interface{}, bool)
- // Store sets the value for a key.
- Store(key, value interface{})
-}
-
-// EnableCustomCache enables unmarshalling cache. It allows reuse of refection information about types needed
-// to perform the unmarshalling. A use of such cache can boost up unmarshalling by x1.4.
-// Check out benchmark_test.go for an example.
-//
-// EnableCustomCache is not thread safe! Do not use it while performing unmarshalling, or it will
-// cause an unsafe race condition. Typically, EnableCustomCache should be called once when the process boots.
-//
-// Caching is disabled by default. The use of this function allows enabling it and controlling the
-// behavior of the cache. Typically, the use of sync.Map should be good enough. The caching mechanism
-// stores a single map per struct type. If you plan to unmarshal a huge amount of distinct
-// struct it may get to consume a lot of resources, in which case you have the control to choose
-// the caching implementation you like and its setup.
-func EnableCustomCache(c Cache) {
- cache = c
-}
-
-// EnableCache enables unmarshalling cache with default implementation. More info at EnableCustomCache.
-func EnableCache() {
- EnableCustomCache(&sync.Map{})
-}
-
-var cache Cache
-
-func cacheLookup(t reflect.Type) map[string]reflectionInfo {
- if cache == nil {
- return nil
- }
- value, exists := cache.Load(t)
- if !exists {
- return nil
- }
- result, _ := value.(map[string]reflectionInfo)
- return result
-}
-
-func cacheStore(t reflect.Type, fields map[string]reflectionInfo) {
- if cache == nil {
- return
- }
- cache.Store(t, fields)
-}
diff --git a/vendor/github.com/perimeterx/marshmallow/doc.go b/vendor/github.com/perimeterx/marshmallow/doc.go
deleted file mode 100644
index c179e657..00000000
--- a/vendor/github.com/perimeterx/marshmallow/doc.go
+++ /dev/null
@@ -1,10 +0,0 @@
-/*
-Package marshmallow provides a simple API to perform flexible and performant JSON unmarshalling.
-Unlike other packages, marshmallow supports unmarshalling of some known and some unknown fields
-with zero performance overhead nor extra coding needed. While unmarshalling,
-marshmallow allows fully retaining the original data and access it via a typed struct and a
-dynamic map.
-
-https://github.com/perimeterx/marshmallow
-*/
-package marshmallow
diff --git a/vendor/github.com/perimeterx/marshmallow/errors.go b/vendor/github.com/perimeterx/marshmallow/errors.go
deleted file mode 100644
index c4d341cc..00000000
--- a/vendor/github.com/perimeterx/marshmallow/errors.go
+++ /dev/null
@@ -1,101 +0,0 @@
-// Copyright 2022 PerimeterX. All rights reserved.
-// Use of this source code is governed by a MIT style
-// license that can be found in the LICENSE file.
-
-package marshmallow
-
-import (
- "errors"
- "fmt"
- "github.com/mailru/easyjson/jlexer"
- "reflect"
- "strings"
-)
-
-var (
- // ErrInvalidInput indicates the input JSON is invalid
- ErrInvalidInput = errors.New("invalid JSON input")
-
- // ErrInvalidValue indicates the target struct has invalid type
- ErrInvalidValue = errors.New("unexpected non struct value")
-)
-
-// MultipleLexerError indicates one or more unmarshalling errors during JSON bytes decode
-type MultipleLexerError struct {
- Errors []*jlexer.LexerError
-}
-
-func (m *MultipleLexerError) Error() string {
- errs := make([]string, len(m.Errors))
- for i, lexerError := range m.Errors {
- errs[i] = lexerError.Error()
- }
- return strings.Join(errs, ", ")
-}
-
-// MultipleError indicates one or more unmarshalling errors during JSON map decode
-type MultipleError struct {
- Errors []error
-}
-
-func (m *MultipleError) Error() string {
- errs := make([]string, len(m.Errors))
- for i, lexerError := range m.Errors {
- errs[i] = lexerError.Error()
- }
- return strings.Join(errs, ", ")
-}
-
-// ParseError indicates a JSON map decode error
-type ParseError struct {
- Reason string
- Path string
-}
-
-func (p *ParseError) Error() string {
- return fmt.Sprintf("parse error: %s in %s", p.Reason, p.Path)
-}
-
-func newUnexpectedTypeParseError(expectedType reflect.Type, path []string) *ParseError {
- return &ParseError{
- Reason: fmt.Sprintf("expected type %s", externalTypeName(expectedType)),
- Path: strings.Join(path, "."),
- }
-}
-
-func newUnsupportedTypeParseError(unsupportedType reflect.Type, path []string) *ParseError {
- return &ParseError{
- Reason: fmt.Sprintf("unsupported type %s", externalTypeName(unsupportedType)),
- Path: strings.Join(path, "."),
- }
-}
-
-func addUnexpectedTypeLexerError(lexer *jlexer.Lexer, expectedType reflect.Type) {
- lexer.AddNonFatalError(fmt.Errorf("expected type %s", externalTypeName(expectedType)))
-}
-
-func addUnsupportedTypeLexerError(lexer *jlexer.Lexer, unsupportedType reflect.Type) {
- lexer.AddNonFatalError(fmt.Errorf("unsupported type %s", externalTypeName(unsupportedType)))
-}
-
-func externalTypeName(t reflect.Type) string {
- switch t.Kind() {
- case reflect.String:
- return "string"
- case reflect.Int, reflect.Int8, reflect.Int16, reflect.Int32, reflect.Int64, reflect.Uint,
- reflect.Uint8, reflect.Uint16, reflect.Uint32, reflect.Uint64, reflect.Uintptr, reflect.Float32,
- reflect.Float64, reflect.Complex64, reflect.Complex128:
- return "number"
- case reflect.Bool:
- return "boolean"
- case reflect.Array, reflect.Slice:
- return "array"
- case reflect.Interface:
- return "any"
- case reflect.Map, reflect.Struct:
- return "object"
- case reflect.Ptr:
- return externalTypeName(t.Elem())
- }
- return "invalid"
-}
diff --git a/vendor/github.com/perimeterx/marshmallow/options.go b/vendor/github.com/perimeterx/marshmallow/options.go
deleted file mode 100644
index 423aa9a9..00000000
--- a/vendor/github.com/perimeterx/marshmallow/options.go
+++ /dev/null
@@ -1,77 +0,0 @@
-// Copyright 2022 PerimeterX. All rights reserved.
-// Use of this source code is governed by a MIT style
-// license that can be found in the LICENSE file.
-
-package marshmallow
-
-// Mode dictates the unmarshalling mode.
-// Each mode is self documented below.
-type Mode uint8
-
-const (
- // ModeFailOnFirstError is the default mode. It makes unmarshalling terminate
- // immediately on any kind of error. This error will then be returned.
- ModeFailOnFirstError Mode = iota
-
- // ModeAllowMultipleErrors mode makes unmarshalling keep decoding even if
- // errors are encountered. In case of such error, the erroneous value will be omitted from the result.
- // Eventually, all errors will all be returned, alongside the partial result.
- ModeAllowMultipleErrors
-
- // ModeFailOverToOriginalValue mode makes unmarshalling keep decoding even if
- // errors are encountered. In case of such error, the original external value be placed in the
- // result data, even though it does not meet the schematic requirements.
- // Eventually, all errors will be returned, alongside the full result. Note that the result map
- // will contain values that do not match the struct schema.
- ModeFailOverToOriginalValue
-)
-
-// WithMode is an UnmarshalOption function to set the unmarshalling mode.
-func WithMode(mode Mode) UnmarshalOption {
- return func(options *unmarshalOptions) {
- options.mode = mode
- }
-}
-
-// WithSkipPopulateStruct is an UnmarshalOption function to set the skipPopulateStruct option.
-// Skipping populate struct is set to false by default.
-// If you do not intend to use the struct value once unmarshalling is finished, set this
-// option to true to boost performance. This would mean the struct fields will not be set
-// with values, but rather it will only be used as the target schema when populating the result map.
-func WithSkipPopulateStruct(skipPopulateStruct bool) UnmarshalOption {
- return func(options *unmarshalOptions) {
- options.skipPopulateStruct = skipPopulateStruct
- }
-}
-
-// WithExcludeKnownFieldsFromMap is an UnmarshalOption function to set the excludeKnownFieldsFromMap option.
-// Exclude known fields flag is set to false by default.
-// When the flag is set to true, fields specified in the input struct (known fields) will be excluded from the result map
-func WithExcludeKnownFieldsFromMap(excludeKnownFields bool) UnmarshalOption {
- return func(options *unmarshalOptions) {
- options.excludeKnownFieldsFromMap = excludeKnownFields
- }
-}
-
-type UnmarshalOption func(*unmarshalOptions)
-
-type unmarshalOptions struct {
- mode Mode
- skipPopulateStruct bool
- excludeKnownFieldsFromMap bool
-}
-
-func buildUnmarshalOptions(options []UnmarshalOption) *unmarshalOptions {
- result := &unmarshalOptions{}
- for _, option := range options {
- option(result)
- }
- return result
-}
-
-// JSONDataHandler allow types to handle JSON data as maps.
-// Types should implement this interface if they wish to act on the map representation of parsed JSON input.
-// This is mainly used to allow nested objects to capture unknown fields and leverage marshmallow's abilities.
-type JSONDataHandler interface {
- HandleJSONData(data map[string]interface{})
-}
diff --git a/vendor/github.com/perimeterx/marshmallow/reflection.go b/vendor/github.com/perimeterx/marshmallow/reflection.go
deleted file mode 100644
index 9b7d88ce..00000000
--- a/vendor/github.com/perimeterx/marshmallow/reflection.go
+++ /dev/null
@@ -1,197 +0,0 @@
-// Copyright 2022 PerimeterX. All rights reserved.
-// Use of this source code is governed by a MIT style
-// license that can be found in the LICENSE file.
-
-package marshmallow
-
-import (
- "encoding/json"
- "reflect"
- "strings"
-)
-
-var unmarshalerType = reflect.TypeOf((*json.Unmarshaler)(nil)).Elem()
-
-type reflectionInfo struct {
- path []int
- t reflect.Type
-}
-
-func (r reflectionInfo) field(target reflect.Value) reflect.Value {
- current := target
- for _, i := range r.path {
- current = current.Field(i)
- }
- return current
-}
-
-func mapStructFields(target interface{}) map[string]reflectionInfo {
- t := reflectStructType(target)
- result := cacheLookup(t)
- if result != nil {
- return result
- }
- result = make(map[string]reflectionInfo, t.NumField())
- mapTypeFields(t, result, nil)
- cacheStore(t, result)
- return result
-}
-
-func mapTypeFields(t reflect.Type, result map[string]reflectionInfo, path []int) {
- num := t.NumField()
- for i := 0; i < num; i++ {
- field := t.Field(i)
- fieldPath := append(path, i)
- if field.Anonymous && field.Type.Kind() == reflect.Struct {
- mapTypeFields(field.Type, result, fieldPath)
- continue
- }
- name := field.Tag.Get("json")
- if name == "" || name == "-" {
- continue
- }
- if index := strings.Index(name, ","); index > -1 {
- name = name[:index]
- }
- result[name] = reflectionInfo{
- path: fieldPath,
- t: field.Type,
- }
- }
-}
-
-func reflectStructValue(target interface{}) reflect.Value {
- v := reflect.ValueOf(target)
- for v.Kind() == reflect.Ptr {
- v = v.Elem()
- }
- return v
-}
-
-func reflectStructType(target interface{}) reflect.Type {
- t := reflect.TypeOf(target)
- for t.Kind() == reflect.Ptr {
- t = t.Elem()
- }
- return t
-}
-
-var primitiveConverters = map[reflect.Kind]func(v interface{}) (interface{}, bool){
- reflect.Bool: func(v interface{}) (interface{}, bool) {
- res, ok := v.(bool)
- return res, ok
- },
- reflect.Int: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return int(res), true
- }
- return v, false
- },
- reflect.Int8: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return int8(res), true
- }
- return v, false
- },
- reflect.Int16: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return int16(res), true
- }
- return v, false
- },
- reflect.Int32: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return int32(res), true
- }
- return v, false
- },
- reflect.Int64: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return int64(res), true
- }
- return v, false
- },
- reflect.Uint: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return uint(res), true
- }
- return v, false
- },
- reflect.Uint8: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return uint8(res), true
- }
- return v, false
- },
- reflect.Uint16: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return uint16(res), true
- }
- return v, false
- },
- reflect.Uint32: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return uint32(res), true
- }
- return v, false
- },
- reflect.Uint64: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return uint64(res), true
- }
- return v, false
- },
- reflect.Float32: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return float32(res), true
- }
- return v, false
- },
- reflect.Float64: func(v interface{}) (interface{}, bool) {
- res, ok := v.(float64)
- if ok {
- return res, true
- }
- return v, false
- },
- reflect.Interface: func(v interface{}) (interface{}, bool) {
- return v, true
- },
- reflect.String: func(v interface{}) (interface{}, bool) {
- res, ok := v.(string)
- return res, ok
- },
-}
-
-func assignValue(field reflect.Value, value interface{}) {
- if value == nil {
- return
- }
- reflectValue := reflect.ValueOf(value)
- if reflectValue.Type().AssignableTo(field.Type()) {
- field.Set(reflectValue)
- }
-}
-
-func isValidValue(v interface{}) bool {
- value := reflect.ValueOf(v)
- return value.Kind() == reflect.Ptr && value.Elem().Kind() == reflect.Struct && !value.IsNil()
-}
-
-func safeReflectValue(t reflect.Type, v interface{}) reflect.Value {
- if v == nil {
- return reflect.Zero(t)
- }
- return reflect.ValueOf(v)
-}
diff --git a/vendor/github.com/perimeterx/marshmallow/unmarshal.go b/vendor/github.com/perimeterx/marshmallow/unmarshal.go
deleted file mode 100644
index 4eb13d18..00000000
--- a/vendor/github.com/perimeterx/marshmallow/unmarshal.go
+++ /dev/null
@@ -1,378 +0,0 @@
-// Copyright 2022 PerimeterX. All rights reserved.
-// Use of this source code is governed by a MIT style
-// license that can be found in the LICENSE file.
-
-package marshmallow
-
-import (
- "encoding/json"
- "github.com/mailru/easyjson/jlexer"
- "reflect"
-)
-
-// Unmarshal parses the JSON-encoded object in data and stores the values
-// in the struct pointed to by v and in the returned map.
-// If v is nil or not a pointer to a struct, Unmarshal returns an ErrInvalidValue.
-// If data is not a valid JSON or not a JSON object Unmarshal returns an ErrInvalidInput.
-//
-// Unmarshal follows the rules of json.Unmarshal with the following exceptions:
-// - All input fields are stored in the resulting map, including fields that do not exist in the
-// struct pointed by v.
-// - Unmarshal only operates on JSON object inputs. It will reject all other types of input
-// by returning ErrInvalidInput.
-// - Unmarshal only operates on struct values. It will reject all other types of v by
-// returning ErrInvalidValue.
-// - Unmarshal supports three types of Mode values. Each mode is self documented and affects
-// how Unmarshal behaves.
-func Unmarshal(data []byte, v interface{}, options ...UnmarshalOption) (map[string]interface{}, error) {
- if !isValidValue(v) {
- return nil, ErrInvalidValue
- }
- opts := buildUnmarshalOptions(options)
- useMultipleErrors := opts.mode == ModeAllowMultipleErrors || opts.mode == ModeFailOverToOriginalValue
- d := &decoder{options: opts, lexer: &jlexer.Lexer{Data: data, UseMultipleErrors: useMultipleErrors}}
- result := make(map[string]interface{})
- if d.lexer.IsNull() {
- d.lexer.Skip()
- } else if !d.lexer.IsDelim('{') {
- return nil, ErrInvalidInput
- } else {
- d.populateStruct(false, v, result)
- }
- d.lexer.Consumed()
- if useMultipleErrors {
- errors := d.lexer.GetNonFatalErrors()
- if len(errors) == 0 {
- return result, nil
- }
- return result, &MultipleLexerError{Errors: errors}
- }
- err := d.lexer.Error()
- if err != nil {
- return nil, err
- }
- return result, nil
-}
-
-type decoder struct {
- options *unmarshalOptions
- lexer *jlexer.Lexer
-}
-
-func (d *decoder) populateStruct(forcePopulate bool, structInstance interface{}, result map[string]interface{}) (interface{}, bool) {
- doPopulate := !d.options.skipPopulateStruct || forcePopulate
- var structValue reflect.Value
- if doPopulate {
- structValue = reflectStructValue(structInstance)
- }
- fields := mapStructFields(structInstance)
- var clone map[string]interface{}
- if d.options.mode == ModeFailOverToOriginalValue {
- clone = make(map[string]interface{}, len(fields))
- }
- d.lexer.Delim('{')
- for !d.lexer.IsDelim('}') {
- key := d.lexer.UnsafeFieldName(false)
- d.lexer.WantColon()
- refInfo, exists := fields[key]
- if exists {
- value, isValidType := d.valueByReflectType(refInfo.t)
- if isValidType {
- if value != nil && doPopulate {
- field := refInfo.field(structValue)
- assignValue(field, value)
- }
- if !d.options.excludeKnownFieldsFromMap {
- if result != nil {
- result[key] = value
- }
- if clone != nil {
- clone[key] = value
- }
- }
- } else {
- switch d.options.mode {
- case ModeFailOnFirstError:
- return nil, false
- case ModeFailOverToOriginalValue:
- if !forcePopulate {
- result[key] = value
- } else {
- clone[key] = value
- d.lexer.WantComma()
- d.drainLexerMap(clone)
- return clone, false
- }
- }
- }
- } else {
- value := d.lexer.Interface()
- if result != nil {
- result[key] = value
- }
- if clone != nil {
- clone[key] = value
- }
- }
- d.lexer.WantComma()
- }
- d.lexer.Delim('}')
- return structInstance, true
-}
-
-func (d *decoder) valueByReflectType(t reflect.Type) (interface{}, bool) {
- if t.Implements(unmarshalerType) {
- result := reflect.New(t.Elem()).Interface()
- d.valueFromCustomUnmarshaler(result.(json.Unmarshaler))
- return result, true
- }
- if reflect.PtrTo(t).Implements(unmarshalerType) {
- value := reflect.New(t)
- d.valueFromCustomUnmarshaler(value.Interface().(json.Unmarshaler))
- return value.Elem().Interface(), true
- }
- kind := t.Kind()
- if converter := primitiveConverters[kind]; converter != nil {
- v := d.lexer.Interface()
- if v == nil {
- return nil, true
- }
- converted, ok := converter(v)
- if !ok {
- addUnexpectedTypeLexerError(d.lexer, t)
- return v, false
- }
- return converted, true
- }
- switch kind {
- case reflect.Slice:
- return d.buildSlice(t)
- case reflect.Array:
- return d.buildArray(t)
- case reflect.Map:
- return d.buildMap(t)
- case reflect.Struct:
- value, valid := d.buildStruct(t)
- if value == nil {
- return nil, valid
- }
- if !valid {
- return value, false
- }
- return reflect.ValueOf(value).Elem().Interface(), valid
- case reflect.Ptr:
- if t.Elem().Kind() == reflect.Struct {
- return d.buildStruct(t.Elem())
- }
- value, valid := d.valueByReflectType(t.Elem())
- if value == nil {
- return nil, valid
- }
- if !valid {
- return value, false
- }
- result := reflect.New(reflect.TypeOf(value))
- result.Elem().Set(reflect.ValueOf(value))
- return result.Interface(), valid
- }
- addUnsupportedTypeLexerError(d.lexer, t)
- return nil, false
-}
-
-func (d *decoder) buildSlice(sliceType reflect.Type) (interface{}, bool) {
- if d.lexer.IsNull() {
- d.lexer.Skip()
- return nil, true
- }
- if !d.lexer.IsDelim('[') {
- addUnexpectedTypeLexerError(d.lexer, sliceType)
- return d.lexer.Interface(), false
- }
- elemType := sliceType.Elem()
- d.lexer.Delim('[')
- var sliceValue reflect.Value
- if !d.lexer.IsDelim(']') {
- sliceValue = reflect.MakeSlice(sliceType, 0, 4)
- } else {
- sliceValue = reflect.MakeSlice(sliceType, 0, 0)
- }
- for !d.lexer.IsDelim(']') {
- current, valid := d.valueByReflectType(elemType)
- if !valid {
- if d.options.mode != ModeFailOverToOriginalValue {
- d.drainLexerArray(nil)
- return nil, true
- }
- result := d.cloneReflectArray(sliceValue, -1)
- result = append(result, current)
- return d.drainLexerArray(result), true
- }
- sliceValue = reflect.Append(sliceValue, safeReflectValue(elemType, current))
- d.lexer.WantComma()
- }
- d.lexer.Delim(']')
- return sliceValue.Interface(), true
-}
-
-func (d *decoder) buildArray(arrayType reflect.Type) (interface{}, bool) {
- if d.lexer.IsNull() {
- d.lexer.Skip()
- return nil, true
- }
- if !d.lexer.IsDelim('[') {
- addUnexpectedTypeLexerError(d.lexer, arrayType)
- return d.lexer.Interface(), false
- }
- elemType := arrayType.Elem()
- arrayValue := reflect.New(arrayType).Elem()
- d.lexer.Delim('[')
- for i := 0; !d.lexer.IsDelim(']'); i++ {
- current, valid := d.valueByReflectType(elemType)
- if !valid {
- if d.options.mode != ModeFailOverToOriginalValue {
- d.drainLexerArray(nil)
- return nil, true
- }
- result := d.cloneReflectArray(arrayValue, i)
- result = append(result, current)
- return d.drainLexerArray(result), true
- }
- if current != nil {
- arrayValue.Index(i).Set(reflect.ValueOf(current))
- }
- d.lexer.WantComma()
- }
- d.lexer.Delim(']')
- return arrayValue.Interface(), true
-}
-
-func (d *decoder) buildMap(mapType reflect.Type) (interface{}, bool) {
- if d.lexer.IsNull() {
- d.lexer.Skip()
- return nil, true
- }
- if !d.lexer.IsDelim('{') {
- addUnexpectedTypeLexerError(d.lexer, mapType)
- return d.lexer.Interface(), false
- }
- d.lexer.Delim('{')
- keyType := mapType.Key()
- valueType := mapType.Elem()
- mapValue := reflect.MakeMap(mapType)
- for !d.lexer.IsDelim('}') {
- key, valid := d.valueByReflectType(keyType)
- if !valid {
- if d.options.mode != ModeFailOverToOriginalValue {
- d.lexer.WantColon()
- d.lexer.Interface()
- d.lexer.WantComma()
- d.drainLexerMap(make(map[string]interface{}))
- return nil, true
- }
- strKey, _ := key.(string)
- d.lexer.WantColon()
- value := d.lexer.Interface()
- result := d.cloneReflectMap(mapValue)
- result[strKey] = value
- d.lexer.WantComma()
- d.drainLexerMap(result)
- return result, true
- }
- d.lexer.WantColon()
- value, valid := d.valueByReflectType(valueType)
- if !valid {
- if d.options.mode != ModeFailOverToOriginalValue {
- d.lexer.WantComma()
- d.drainLexerMap(make(map[string]interface{}))
- return nil, true
- }
- strKey, _ := key.(string)
- result := d.cloneReflectMap(mapValue)
- result[strKey] = value
- d.lexer.WantComma()
- d.drainLexerMap(result)
- return result, true
- }
- mapValue.SetMapIndex(safeReflectValue(keyType, key), safeReflectValue(valueType, value))
- d.lexer.WantComma()
- }
- d.lexer.Delim('}')
- return mapValue.Interface(), true
-}
-
-func (d *decoder) buildStruct(structType reflect.Type) (interface{}, bool) {
- if d.lexer.IsNull() {
- d.lexer.Skip()
- return nil, true
- }
- if !d.lexer.IsDelim('{') {
- addUnexpectedTypeLexerError(d.lexer, structType)
- return d.lexer.Interface(), false
- }
- value := reflect.New(structType).Interface()
- handler, ok := value.(JSONDataHandler)
- if !ok {
- return d.populateStruct(true, value, nil)
- }
- data := make(map[string]interface{})
- result, valid := d.populateStruct(true, value, data)
- if valid {
- handler.HandleJSONData(data)
- }
- return result, valid
-}
-
-func (d *decoder) valueFromCustomUnmarshaler(unmarshaler json.Unmarshaler) {
- data := d.lexer.Raw()
- if !d.lexer.Ok() {
- return
- }
- err := unmarshaler.UnmarshalJSON(data)
- if err != nil {
- d.lexer.AddNonFatalError(err)
- }
-}
-
-func (d *decoder) cloneReflectArray(value reflect.Value, length int) []interface{} {
- if length == -1 {
- length = value.Len()
- }
- result := make([]interface{}, length)
- for i := 0; i < length; i++ {
- result[i] = value.Index(i).Interface()
- }
- return result
-}
-
-func (d *decoder) cloneReflectMap(mapValue reflect.Value) map[string]interface{} {
- l := mapValue.Len()
- result := make(map[string]interface{}, l)
- for _, key := range mapValue.MapKeys() {
- value := mapValue.MapIndex(key)
- strKey, _ := key.Interface().(string)
- result[strKey] = value.Interface()
- }
- return result
-}
-
-func (d *decoder) drainLexerArray(target []interface{}) interface{} {
- d.lexer.WantComma()
- for !d.lexer.IsDelim(']') {
- current := d.lexer.Interface()
- target = append(target, current)
- d.lexer.WantComma()
- }
- d.lexer.Delim(']')
- return target
-}
-
-func (d *decoder) drainLexerMap(target map[string]interface{}) {
- for !d.lexer.IsDelim('}') {
- key := d.lexer.String()
- d.lexer.WantColon()
- value := d.lexer.Interface()
- target[key] = value
- d.lexer.WantComma()
- }
- d.lexer.Delim('}')
-}
diff --git a/vendor/github.com/perimeterx/marshmallow/unmarshal_from_json_map.go b/vendor/github.com/perimeterx/marshmallow/unmarshal_from_json_map.go
deleted file mode 100644
index fcf1696f..00000000
--- a/vendor/github.com/perimeterx/marshmallow/unmarshal_from_json_map.go
+++ /dev/null
@@ -1,290 +0,0 @@
-// Copyright 2022 PerimeterX. All rights reserved.
-// Use of this source code is governed by a MIT style
-// license that can be found in the LICENSE file.
-
-package marshmallow
-
-import (
- "reflect"
-)
-
-// UnmarshalerFromJSONMap is the interface implemented by types
-// that can unmarshal a JSON description of themselves.
-// In case you want to implement custom unmarshalling, json.Unmarshaler only supports
-// receiving the data as []byte. However, while unmarshalling from JSON map,
-// the data is not available as a raw []byte and converting to it will significantly
-// hurt performance. Thus, if you wish to implement a custom unmarshalling on a type
-// that is being unmarshalled from a JSON map, you need to implement
-// UnmarshalerFromJSONMap interface.
-type UnmarshalerFromJSONMap interface {
- UnmarshalJSONFromMap(data interface{}) error
-}
-
-// UnmarshalFromJSONMap parses the JSON map data and stores the values
-// in the struct pointed to by v and in the returned map.
-// If v is nil or not a pointer to a struct, UnmarshalFromJSONMap returns an ErrInvalidValue.
-//
-// UnmarshalFromJSONMap follows the rules of json.Unmarshal with the following exceptions:
-// - All input fields are stored in the resulting map, including fields that do not exist in the
-// struct pointed by v.
-// - UnmarshalFromJSONMap receive a JSON map instead of raw bytes. The given input map is assumed
-// to be a JSON map, meaning it should only contain the following types: bool, string, float64,
-// []interface, and map[string]interface{}. Other types will cause decoding to return unexpected results.
-// - UnmarshalFromJSONMap only operates on struct values. It will reject all other types of v by
-// returning ErrInvalidValue.
-// - UnmarshalFromJSONMap supports three types of Mode values. Each mode is self documented and affects
-// how UnmarshalFromJSONMap behaves.
-func UnmarshalFromJSONMap(data map[string]interface{}, v interface{}, options ...UnmarshalOption) (map[string]interface{}, error) {
- if !isValidValue(v) {
- return nil, ErrInvalidValue
- }
- opts := buildUnmarshalOptions(options)
- d := &mapDecoder{options: opts}
- result := make(map[string]interface{})
- if data != nil {
- d.populateStruct(false, nil, data, v, result)
- }
- if opts.mode == ModeAllowMultipleErrors || opts.mode == ModeFailOverToOriginalValue {
- if len(d.errs) == 0 {
- return result, nil
- }
- return result, &MultipleError{Errors: d.errs}
- }
- if d.err != nil {
- return nil, d.err
- }
- return result, nil
-}
-
-var unmarshalerFromJSONMapType = reflect.TypeOf((*UnmarshalerFromJSONMap)(nil)).Elem()
-
-type mapDecoder struct {
- options *unmarshalOptions
- err error
- errs []error
-}
-
-func (m *mapDecoder) populateStruct(forcePopulate bool, path []string, data map[string]interface{}, structInstance interface{}, result map[string]interface{}) (interface{}, bool) {
- doPopulate := !m.options.skipPopulateStruct || forcePopulate
- var structValue reflect.Value
- if doPopulate {
- structValue = reflectStructValue(structInstance)
- }
- fields := mapStructFields(structInstance)
- for key, inputValue := range data {
- refInfo, exists := fields[key]
- if exists {
- value, isValidType := m.valueByReflectType(append(path, key), inputValue, refInfo.t)
- if isValidType {
- if value != nil && doPopulate {
- field := refInfo.field(structValue)
- assignValue(field, value)
- }
- if !m.options.excludeKnownFieldsFromMap {
- if result != nil {
- result[key] = value
- }
- }
- } else {
- switch m.options.mode {
- case ModeFailOnFirstError:
- return nil, false
- case ModeFailOverToOriginalValue:
- if !forcePopulate {
- result[key] = value
- } else {
- return data, false
- }
- }
- }
- } else {
- if result != nil {
- result[key] = inputValue
- }
- }
- }
- return structInstance, true
-}
-
-func (m *mapDecoder) valueByReflectType(path []string, v interface{}, t reflect.Type) (interface{}, bool) {
- if t.Implements(unmarshalerFromJSONMapType) {
- result := reflect.New(t.Elem()).Interface()
- m.valueFromCustomUnmarshaler(v, result.(UnmarshalerFromJSONMap))
- return result, true
- }
- if reflect.PtrTo(t).Implements(unmarshalerFromJSONMapType) {
- value := reflect.New(t)
- m.valueFromCustomUnmarshaler(v, value.Interface().(UnmarshalerFromJSONMap))
- return value.Elem().Interface(), true
- }
- kind := t.Kind()
- if converter := primitiveConverters[kind]; converter != nil {
- if v == nil {
- return nil, true
- }
- converted, ok := converter(v)
- if !ok {
- m.addError(newUnexpectedTypeParseError(t, path))
- return v, false
- }
- return converted, true
- }
- switch kind {
- case reflect.Slice:
- return m.buildSlice(path, v, t)
- case reflect.Array:
- return m.buildArray(path, v, t)
- case reflect.Map:
- return m.buildMap(path, v, t)
- case reflect.Struct:
- value, valid := m.buildStruct(path, v, t)
- if value == nil {
- return nil, valid
- }
- if !valid {
- return value, false
- }
- return reflect.ValueOf(value).Elem().Interface(), valid
- case reflect.Ptr:
- if t.Elem().Kind() == reflect.Struct {
- return m.buildStruct(path, v, t.Elem())
- }
- value, valid := m.valueByReflectType(path, v, t.Elem())
- if value == nil {
- return nil, valid
- }
- if !valid {
- return value, false
- }
- result := reflect.New(reflect.TypeOf(value))
- result.Elem().Set(reflect.ValueOf(value))
- return result.Interface(), valid
- }
- m.addError(newUnsupportedTypeParseError(t, path))
- return nil, false
-}
-
-func (m *mapDecoder) buildSlice(path []string, v interface{}, sliceType reflect.Type) (interface{}, bool) {
- if v == nil {
- return nil, true
- }
- arr, ok := v.([]interface{})
- if !ok {
- m.addError(newUnexpectedTypeParseError(sliceType, path))
- return v, false
- }
- elemType := sliceType.Elem()
- var sliceValue reflect.Value
- if len(arr) > 0 {
- sliceValue = reflect.MakeSlice(sliceType, 0, 4)
- } else {
- sliceValue = reflect.MakeSlice(sliceType, 0, 0)
- }
- for _, element := range arr {
- current, valid := m.valueByReflectType(path, element, elemType)
- if !valid {
- if m.options.mode != ModeFailOverToOriginalValue {
- return nil, true
- }
- return v, true
- }
- sliceValue = reflect.Append(sliceValue, safeReflectValue(elemType, current))
- }
- return sliceValue.Interface(), true
-}
-
-func (m *mapDecoder) buildArray(path []string, v interface{}, arrayType reflect.Type) (interface{}, bool) {
- if v == nil {
- return nil, true
- }
- arr, ok := v.([]interface{})
- if !ok {
- m.addError(newUnexpectedTypeParseError(arrayType, path))
- return v, false
- }
- elemType := arrayType.Elem()
- arrayValue := reflect.New(arrayType).Elem()
- for i, element := range arr {
- current, valid := m.valueByReflectType(path, element, elemType)
- if !valid {
- if m.options.mode != ModeFailOverToOriginalValue {
- return nil, true
- }
- return v, true
- }
- if current != nil {
- arrayValue.Index(i).Set(reflect.ValueOf(current))
- }
- }
- return arrayValue.Interface(), true
-}
-
-func (m *mapDecoder) buildMap(path []string, v interface{}, mapType reflect.Type) (interface{}, bool) {
- if v == nil {
- return nil, true
- }
- mp, ok := v.(map[string]interface{})
- if !ok {
- m.addError(newUnexpectedTypeParseError(mapType, path))
- return v, false
- }
- keyType := mapType.Key()
- valueType := mapType.Elem()
- mapValue := reflect.MakeMap(mapType)
- for inputKey, inputValue := range mp {
- keyPath := append(path, inputKey)
- key, valid := m.valueByReflectType(keyPath, inputKey, keyType)
- if !valid {
- if m.options.mode != ModeFailOverToOriginalValue {
- return nil, true
- }
- return v, true
- }
- value, valid := m.valueByReflectType(keyPath, inputValue, valueType)
- if !valid {
- if m.options.mode != ModeFailOverToOriginalValue {
- return nil, true
- }
- return v, true
- }
- mapValue.SetMapIndex(safeReflectValue(keyType, key), safeReflectValue(valueType, value))
- }
- return mapValue.Interface(), true
-}
-
-func (m *mapDecoder) buildStruct(path []string, v interface{}, structType reflect.Type) (interface{}, bool) {
- if v == nil {
- return nil, true
- }
- mp, ok := v.(map[string]interface{})
- if !ok {
- m.addError(newUnexpectedTypeParseError(structType, path))
- return v, false
- }
- value := reflect.New(structType).Interface()
- handler, ok := value.(JSONDataHandler)
- if !ok {
- return m.populateStruct(true, path, mp, value, nil)
- }
- data := make(map[string]interface{})
- result, valid := m.populateStruct(true, path, mp, value, data)
- if valid {
- handler.HandleJSONData(data)
- }
- return result, valid
-}
-
-func (m *mapDecoder) valueFromCustomUnmarshaler(data interface{}, unmarshaler UnmarshalerFromJSONMap) {
- err := unmarshaler.UnmarshalJSONFromMap(data)
- if err != nil {
- m.addError(err)
- }
-}
-
-func (m *mapDecoder) addError(err error) {
- if m.options.mode == ModeFailOnFirstError {
- m.err = err
- } else {
- m.errs = append(m.errs, err)
- }
-}
diff --git a/vendor/github.com/pkg/errors/.gitignore b/vendor/github.com/pkg/errors/.gitignore
deleted file mode 100644
index daf913b1..00000000
--- a/vendor/github.com/pkg/errors/.gitignore
+++ /dev/null
@@ -1,24 +0,0 @@
-# Compiled Object files, Static and Dynamic libs (Shared Objects)
-*.o
-*.a
-*.so
-
-# Folders
-_obj
-_test
-
-# Architecture specific extensions/prefixes
-*.[568vq]
-[568vq].out
-
-*.cgo1.go
-*.cgo2.c
-_cgo_defun.c
-_cgo_gotypes.go
-_cgo_export.*
-
-_testmain.go
-
-*.exe
-*.test
-*.prof
diff --git a/vendor/github.com/pkg/errors/.travis.yml b/vendor/github.com/pkg/errors/.travis.yml
deleted file mode 100644
index 9159de03..00000000
--- a/vendor/github.com/pkg/errors/.travis.yml
+++ /dev/null
@@ -1,10 +0,0 @@
-language: go
-go_import_path: github.com/pkg/errors
-go:
- - 1.11.x
- - 1.12.x
- - 1.13.x
- - tip
-
-script:
- - make check
diff --git a/vendor/github.com/pkg/errors/LICENSE b/vendor/github.com/pkg/errors/LICENSE
deleted file mode 100644
index 835ba3e7..00000000
--- a/vendor/github.com/pkg/errors/LICENSE
+++ /dev/null
@@ -1,23 +0,0 @@
-Copyright (c) 2015, Dave Cheney
-All rights reserved.
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are met:
-
-* Redistributions of source code must retain the above copyright notice, this
- list of conditions and the following disclaimer.
-
-* Redistributions in binary form must reproduce the above copyright notice,
- this list of conditions and the following disclaimer in the documentation
- and/or other materials provided with the distribution.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/vendor/github.com/pkg/errors/Makefile b/vendor/github.com/pkg/errors/Makefile
deleted file mode 100644
index ce9d7cde..00000000
--- a/vendor/github.com/pkg/errors/Makefile
+++ /dev/null
@@ -1,44 +0,0 @@
-PKGS := github.com/pkg/errors
-SRCDIRS := $(shell go list -f '{{.Dir}}' $(PKGS))
-GO := go
-
-check: test vet gofmt misspell unconvert staticcheck ineffassign unparam
-
-test:
- $(GO) test $(PKGS)
-
-vet: | test
- $(GO) vet $(PKGS)
-
-staticcheck:
- $(GO) get honnef.co/go/tools/cmd/staticcheck
- staticcheck -checks all $(PKGS)
-
-misspell:
- $(GO) get github.com/client9/misspell/cmd/misspell
- misspell \
- -locale GB \
- -error \
- *.md *.go
-
-unconvert:
- $(GO) get github.com/mdempsky/unconvert
- unconvert -v $(PKGS)
-
-ineffassign:
- $(GO) get github.com/gordonklaus/ineffassign
- find $(SRCDIRS) -name '*.go' | xargs ineffassign
-
-pedantic: check errcheck
-
-unparam:
- $(GO) get mvdan.cc/unparam
- unparam ./...
-
-errcheck:
- $(GO) get github.com/kisielk/errcheck
- errcheck $(PKGS)
-
-gofmt:
- @echo Checking code is gofmted
- @test -z "$(shell gofmt -s -l -d -e $(SRCDIRS) | tee /dev/stderr)"
diff --git a/vendor/github.com/pkg/errors/README.md b/vendor/github.com/pkg/errors/README.md
deleted file mode 100644
index 54dfdcb1..00000000
--- a/vendor/github.com/pkg/errors/README.md
+++ /dev/null
@@ -1,59 +0,0 @@
-# errors [](https://travis-ci.org/pkg/errors) [](https://ci.appveyor.com/project/davecheney/errors/branch/master) [](http://godoc.org/github.com/pkg/errors) [](https://goreportcard.com/report/github.com/pkg/errors) [](https://sourcegraph.com/github.com/pkg/errors?badge)
-
-Package errors provides simple error handling primitives.
-
-`go get github.com/pkg/errors`
-
-The traditional error handling idiom in Go is roughly akin to
-```go
-if err != nil {
- return err
-}
-```
-which applied recursively up the call stack results in error reports without context or debugging information. The errors package allows programmers to add context to the failure path in their code in a way that does not destroy the original value of the error.
-
-## Adding context to an error
-
-The errors.Wrap function returns a new error that adds context to the original error. For example
-```go
-_, err := ioutil.ReadAll(r)
-if err != nil {
- return errors.Wrap(err, "read failed")
-}
-```
-## Retrieving the cause of an error
-
-Using `errors.Wrap` constructs a stack of errors, adding context to the preceding error. Depending on the nature of the error it may be necessary to reverse the operation of errors.Wrap to retrieve the original error for inspection. Any error value which implements this interface can be inspected by `errors.Cause`.
-```go
-type causer interface {
- Cause() error
-}
-```
-`errors.Cause` will recursively retrieve the topmost error which does not implement `causer`, which is assumed to be the original cause. For example:
-```go
-switch err := errors.Cause(err).(type) {
-case *MyError:
- // handle specifically
-default:
- // unknown error
-}
-```
-
-[Read the package documentation for more information](https://godoc.org/github.com/pkg/errors).
-
-## Roadmap
-
-With the upcoming [Go2 error proposals](https://go.googlesource.com/proposal/+/master/design/go2draft.md) this package is moving into maintenance mode. The roadmap for a 1.0 release is as follows:
-
-- 0.9. Remove pre Go 1.9 and Go 1.10 support, address outstanding pull requests (if possible)
-- 1.0. Final release.
-
-## Contributing
-
-Because of the Go2 errors changes, this package is not accepting proposals for new functionality. With that said, we welcome pull requests, bug fixes and issue reports.
-
-Before sending a PR, please discuss your change by raising an issue.
-
-## License
-
-BSD-2-Clause
diff --git a/vendor/github.com/pkg/errors/appveyor.yml b/vendor/github.com/pkg/errors/appveyor.yml
deleted file mode 100644
index a932eade..00000000
--- a/vendor/github.com/pkg/errors/appveyor.yml
+++ /dev/null
@@ -1,32 +0,0 @@
-version: build-{build}.{branch}
-
-clone_folder: C:\gopath\src\github.com\pkg\errors
-shallow_clone: true # for startup speed
-
-environment:
- GOPATH: C:\gopath
-
-platform:
- - x64
-
-# http://www.appveyor.com/docs/installed-software
-install:
- # some helpful output for debugging builds
- - go version
- - go env
- # pre-installed MinGW at C:\MinGW is 32bit only
- # but MSYS2 at C:\msys64 has mingw64
- - set PATH=C:\msys64\mingw64\bin;%PATH%
- - gcc --version
- - g++ --version
-
-build_script:
- - go install -v ./...
-
-test_script:
- - set PATH=C:\gopath\bin;%PATH%
- - go test -v ./...
-
-#artifacts:
-# - path: '%GOPATH%\bin\*.exe'
-deploy: off
diff --git a/vendor/github.com/pkg/errors/errors.go b/vendor/github.com/pkg/errors/errors.go
deleted file mode 100644
index 161aea25..00000000
--- a/vendor/github.com/pkg/errors/errors.go
+++ /dev/null
@@ -1,288 +0,0 @@
-// Package errors provides simple error handling primitives.
-//
-// The traditional error handling idiom in Go is roughly akin to
-//
-// if err != nil {
-// return err
-// }
-//
-// which when applied recursively up the call stack results in error reports
-// without context or debugging information. The errors package allows
-// programmers to add context to the failure path in their code in a way
-// that does not destroy the original value of the error.
-//
-// Adding context to an error
-//
-// The errors.Wrap function returns a new error that adds context to the
-// original error by recording a stack trace at the point Wrap is called,
-// together with the supplied message. For example
-//
-// _, err := ioutil.ReadAll(r)
-// if err != nil {
-// return errors.Wrap(err, "read failed")
-// }
-//
-// If additional control is required, the errors.WithStack and
-// errors.WithMessage functions destructure errors.Wrap into its component
-// operations: annotating an error with a stack trace and with a message,
-// respectively.
-//
-// Retrieving the cause of an error
-//
-// Using errors.Wrap constructs a stack of errors, adding context to the
-// preceding error. Depending on the nature of the error it may be necessary
-// to reverse the operation of errors.Wrap to retrieve the original error
-// for inspection. Any error value which implements this interface
-//
-// type causer interface {
-// Cause() error
-// }
-//
-// can be inspected by errors.Cause. errors.Cause will recursively retrieve
-// the topmost error that does not implement causer, which is assumed to be
-// the original cause. For example:
-//
-// switch err := errors.Cause(err).(type) {
-// case *MyError:
-// // handle specifically
-// default:
-// // unknown error
-// }
-//
-// Although the causer interface is not exported by this package, it is
-// considered a part of its stable public interface.
-//
-// Formatted printing of errors
-//
-// All error values returned from this package implement fmt.Formatter and can
-// be formatted by the fmt package. The following verbs are supported:
-//
-// %s print the error. If the error has a Cause it will be
-// printed recursively.
-// %v see %s
-// %+v extended format. Each Frame of the error's StackTrace will
-// be printed in detail.
-//
-// Retrieving the stack trace of an error or wrapper
-//
-// New, Errorf, Wrap, and Wrapf record a stack trace at the point they are
-// invoked. This information can be retrieved with the following interface:
-//
-// type stackTracer interface {
-// StackTrace() errors.StackTrace
-// }
-//
-// The returned errors.StackTrace type is defined as
-//
-// type StackTrace []Frame
-//
-// The Frame type represents a call site in the stack trace. Frame supports
-// the fmt.Formatter interface that can be used for printing information about
-// the stack trace of this error. For example:
-//
-// if err, ok := err.(stackTracer); ok {
-// for _, f := range err.StackTrace() {
-// fmt.Printf("%+s:%d\n", f, f)
-// }
-// }
-//
-// Although the stackTracer interface is not exported by this package, it is
-// considered a part of its stable public interface.
-//
-// See the documentation for Frame.Format for more details.
-package errors
-
-import (
- "fmt"
- "io"
-)
-
-// New returns an error with the supplied message.
-// New also records the stack trace at the point it was called.
-func New(message string) error {
- return &fundamental{
- msg: message,
- stack: callers(),
- }
-}
-
-// Errorf formats according to a format specifier and returns the string
-// as a value that satisfies error.
-// Errorf also records the stack trace at the point it was called.
-func Errorf(format string, args ...interface{}) error {
- return &fundamental{
- msg: fmt.Sprintf(format, args...),
- stack: callers(),
- }
-}
-
-// fundamental is an error that has a message and a stack, but no caller.
-type fundamental struct {
- msg string
- *stack
-}
-
-func (f *fundamental) Error() string { return f.msg }
-
-func (f *fundamental) Format(s fmt.State, verb rune) {
- switch verb {
- case 'v':
- if s.Flag('+') {
- io.WriteString(s, f.msg)
- f.stack.Format(s, verb)
- return
- }
- fallthrough
- case 's':
- io.WriteString(s, f.msg)
- case 'q':
- fmt.Fprintf(s, "%q", f.msg)
- }
-}
-
-// WithStack annotates err with a stack trace at the point WithStack was called.
-// If err is nil, WithStack returns nil.
-func WithStack(err error) error {
- if err == nil {
- return nil
- }
- return &withStack{
- err,
- callers(),
- }
-}
-
-type withStack struct {
- error
- *stack
-}
-
-func (w *withStack) Cause() error { return w.error }
-
-// Unwrap provides compatibility for Go 1.13 error chains.
-func (w *withStack) Unwrap() error { return w.error }
-
-func (w *withStack) Format(s fmt.State, verb rune) {
- switch verb {
- case 'v':
- if s.Flag('+') {
- fmt.Fprintf(s, "%+v", w.Cause())
- w.stack.Format(s, verb)
- return
- }
- fallthrough
- case 's':
- io.WriteString(s, w.Error())
- case 'q':
- fmt.Fprintf(s, "%q", w.Error())
- }
-}
-
-// Wrap returns an error annotating err with a stack trace
-// at the point Wrap is called, and the supplied message.
-// If err is nil, Wrap returns nil.
-func Wrap(err error, message string) error {
- if err == nil {
- return nil
- }
- err = &withMessage{
- cause: err,
- msg: message,
- }
- return &withStack{
- err,
- callers(),
- }
-}
-
-// Wrapf returns an error annotating err with a stack trace
-// at the point Wrapf is called, and the format specifier.
-// If err is nil, Wrapf returns nil.
-func Wrapf(err error, format string, args ...interface{}) error {
- if err == nil {
- return nil
- }
- err = &withMessage{
- cause: err,
- msg: fmt.Sprintf(format, args...),
- }
- return &withStack{
- err,
- callers(),
- }
-}
-
-// WithMessage annotates err with a new message.
-// If err is nil, WithMessage returns nil.
-func WithMessage(err error, message string) error {
- if err == nil {
- return nil
- }
- return &withMessage{
- cause: err,
- msg: message,
- }
-}
-
-// WithMessagef annotates err with the format specifier.
-// If err is nil, WithMessagef returns nil.
-func WithMessagef(err error, format string, args ...interface{}) error {
- if err == nil {
- return nil
- }
- return &withMessage{
- cause: err,
- msg: fmt.Sprintf(format, args...),
- }
-}
-
-type withMessage struct {
- cause error
- msg string
-}
-
-func (w *withMessage) Error() string { return w.msg + ": " + w.cause.Error() }
-func (w *withMessage) Cause() error { return w.cause }
-
-// Unwrap provides compatibility for Go 1.13 error chains.
-func (w *withMessage) Unwrap() error { return w.cause }
-
-func (w *withMessage) Format(s fmt.State, verb rune) {
- switch verb {
- case 'v':
- if s.Flag('+') {
- fmt.Fprintf(s, "%+v\n", w.Cause())
- io.WriteString(s, w.msg)
- return
- }
- fallthrough
- case 's', 'q':
- io.WriteString(s, w.Error())
- }
-}
-
-// Cause returns the underlying cause of the error, if possible.
-// An error value has a cause if it implements the following
-// interface:
-//
-// type causer interface {
-// Cause() error
-// }
-//
-// If the error does not implement Cause, the original error will
-// be returned. If the error is nil, nil will be returned without further
-// investigation.
-func Cause(err error) error {
- type causer interface {
- Cause() error
- }
-
- for err != nil {
- cause, ok := err.(causer)
- if !ok {
- break
- }
- err = cause.Cause()
- }
- return err
-}
diff --git a/vendor/github.com/pkg/errors/go113.go b/vendor/github.com/pkg/errors/go113.go
deleted file mode 100644
index be0d10d0..00000000
--- a/vendor/github.com/pkg/errors/go113.go
+++ /dev/null
@@ -1,38 +0,0 @@
-// +build go1.13
-
-package errors
-
-import (
- stderrors "errors"
-)
-
-// Is reports whether any error in err's chain matches target.
-//
-// The chain consists of err itself followed by the sequence of errors obtained by
-// repeatedly calling Unwrap.
-//
-// An error is considered to match a target if it is equal to that target or if
-// it implements a method Is(error) bool such that Is(target) returns true.
-func Is(err, target error) bool { return stderrors.Is(err, target) }
-
-// As finds the first error in err's chain that matches target, and if so, sets
-// target to that error value and returns true.
-//
-// The chain consists of err itself followed by the sequence of errors obtained by
-// repeatedly calling Unwrap.
-//
-// An error matches target if the error's concrete value is assignable to the value
-// pointed to by target, or if the error has a method As(interface{}) bool such that
-// As(target) returns true. In the latter case, the As method is responsible for
-// setting target.
-//
-// As will panic if target is not a non-nil pointer to either a type that implements
-// error, or to any interface type. As returns false if err is nil.
-func As(err error, target interface{}) bool { return stderrors.As(err, target) }
-
-// Unwrap returns the result of calling the Unwrap method on err, if err's
-// type contains an Unwrap method returning error.
-// Otherwise, Unwrap returns nil.
-func Unwrap(err error) error {
- return stderrors.Unwrap(err)
-}
diff --git a/vendor/github.com/pkg/errors/stack.go b/vendor/github.com/pkg/errors/stack.go
deleted file mode 100644
index 779a8348..00000000
--- a/vendor/github.com/pkg/errors/stack.go
+++ /dev/null
@@ -1,177 +0,0 @@
-package errors
-
-import (
- "fmt"
- "io"
- "path"
- "runtime"
- "strconv"
- "strings"
-)
-
-// Frame represents a program counter inside a stack frame.
-// For historical reasons if Frame is interpreted as a uintptr
-// its value represents the program counter + 1.
-type Frame uintptr
-
-// pc returns the program counter for this frame;
-// multiple frames may have the same PC value.
-func (f Frame) pc() uintptr { return uintptr(f) - 1 }
-
-// file returns the full path to the file that contains the
-// function for this Frame's pc.
-func (f Frame) file() string {
- fn := runtime.FuncForPC(f.pc())
- if fn == nil {
- return "unknown"
- }
- file, _ := fn.FileLine(f.pc())
- return file
-}
-
-// line returns the line number of source code of the
-// function for this Frame's pc.
-func (f Frame) line() int {
- fn := runtime.FuncForPC(f.pc())
- if fn == nil {
- return 0
- }
- _, line := fn.FileLine(f.pc())
- return line
-}
-
-// name returns the name of this function, if known.
-func (f Frame) name() string {
- fn := runtime.FuncForPC(f.pc())
- if fn == nil {
- return "unknown"
- }
- return fn.Name()
-}
-
-// Format formats the frame according to the fmt.Formatter interface.
-//
-// %s source file
-// %d source line
-// %n function name
-// %v equivalent to %s:%d
-//
-// Format accepts flags that alter the printing of some verbs, as follows:
-//
-// %+s function name and path of source file relative to the compile time
-// GOPATH separated by \n\t (\n\t)
-// %+v equivalent to %+s:%d
-func (f Frame) Format(s fmt.State, verb rune) {
- switch verb {
- case 's':
- switch {
- case s.Flag('+'):
- io.WriteString(s, f.name())
- io.WriteString(s, "\n\t")
- io.WriteString(s, f.file())
- default:
- io.WriteString(s, path.Base(f.file()))
- }
- case 'd':
- io.WriteString(s, strconv.Itoa(f.line()))
- case 'n':
- io.WriteString(s, funcname(f.name()))
- case 'v':
- f.Format(s, 's')
- io.WriteString(s, ":")
- f.Format(s, 'd')
- }
-}
-
-// MarshalText formats a stacktrace Frame as a text string. The output is the
-// same as that of fmt.Sprintf("%+v", f), but without newlines or tabs.
-func (f Frame) MarshalText() ([]byte, error) {
- name := f.name()
- if name == "unknown" {
- return []byte(name), nil
- }
- return []byte(fmt.Sprintf("%s %s:%d", name, f.file(), f.line())), nil
-}
-
-// StackTrace is stack of Frames from innermost (newest) to outermost (oldest).
-type StackTrace []Frame
-
-// Format formats the stack of Frames according to the fmt.Formatter interface.
-//
-// %s lists source files for each Frame in the stack
-// %v lists the source file and line number for each Frame in the stack
-//
-// Format accepts flags that alter the printing of some verbs, as follows:
-//
-// %+v Prints filename, function, and line number for each Frame in the stack.
-func (st StackTrace) Format(s fmt.State, verb rune) {
- switch verb {
- case 'v':
- switch {
- case s.Flag('+'):
- for _, f := range st {
- io.WriteString(s, "\n")
- f.Format(s, verb)
- }
- case s.Flag('#'):
- fmt.Fprintf(s, "%#v", []Frame(st))
- default:
- st.formatSlice(s, verb)
- }
- case 's':
- st.formatSlice(s, verb)
- }
-}
-
-// formatSlice will format this StackTrace into the given buffer as a slice of
-// Frame, only valid when called with '%s' or '%v'.
-func (st StackTrace) formatSlice(s fmt.State, verb rune) {
- io.WriteString(s, "[")
- for i, f := range st {
- if i > 0 {
- io.WriteString(s, " ")
- }
- f.Format(s, verb)
- }
- io.WriteString(s, "]")
-}
-
-// stack represents a stack of program counters.
-type stack []uintptr
-
-func (s *stack) Format(st fmt.State, verb rune) {
- switch verb {
- case 'v':
- switch {
- case st.Flag('+'):
- for _, pc := range *s {
- f := Frame(pc)
- fmt.Fprintf(st, "\n%+v", f)
- }
- }
- }
-}
-
-func (s *stack) StackTrace() StackTrace {
- f := make([]Frame, len(*s))
- for i := 0; i < len(f); i++ {
- f[i] = Frame((*s)[i])
- }
- return f
-}
-
-func callers() *stack {
- const depth = 32
- var pcs [depth]uintptr
- n := runtime.Callers(3, pcs[:])
- var st stack = pcs[0:n]
- return &st
-}
-
-// funcname removes the path prefix component of a function's name reported by func.Name().
-func funcname(name string) string {
- i := strings.LastIndex(name, "/")
- name = name[i+1:]
- i = strings.Index(name, ".")
- return name[i+1:]
-}
diff --git a/vendor/github.com/rivo/uniseg/LICENSE.txt b/vendor/github.com/rivo/uniseg/LICENSE.txt
deleted file mode 100644
index 5040f1ef..00000000
--- a/vendor/github.com/rivo/uniseg/LICENSE.txt
+++ /dev/null
@@ -1,21 +0,0 @@
-MIT License
-
-Copyright (c) 2019 Oliver Kuederle
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/vendor/github.com/rivo/uniseg/README.md b/vendor/github.com/rivo/uniseg/README.md
deleted file mode 100644
index 25e93468..00000000
--- a/vendor/github.com/rivo/uniseg/README.md
+++ /dev/null
@@ -1,157 +0,0 @@
-# Unicode Text Segmentation for Go
-
-[](https://pkg.go.dev/github.com/rivo/uniseg)
-[](https://goreportcard.com/report/github.com/rivo/uniseg)
-
-This Go package implements Unicode Text Segmentation according to [Unicode Standard Annex #29](https://unicode.org/reports/tr29/), Unicode Line Breaking according to [Unicode Standard Annex #14](https://unicode.org/reports/tr14/) (Unicode version 14.0.0), and monospace font string width calculation similar to [wcwidth](https://man7.org/linux/man-pages/man3/wcwidth.3.html).
-
-## Background
-
-### Grapheme Clusters
-
-In Go, [strings are read-only slices of bytes](https://go.dev/blog/strings). They can be turned into Unicode code points using the `for` loop or by casting: `[]rune(str)`. However, multiple code points may be combined into one user-perceived character or what the Unicode specification calls "grapheme cluster". Here are some examples:
-
-|String|Bytes (UTF-8)|Code points (runes)|Grapheme clusters|
-|-|-|-|-|
-|Käse|6 bytes: `4b 61 cc 88 73 65`|5 code points: `4b 61 308 73 65`|4 clusters: `[4b],[61 308],[73],[65]`|
-|ðŸ³ï¸â€ðŸŒˆ|14 bytes: `f0 9f 8f b3 ef b8 8f e2 80 8d f0 9f 8c 88`|4 code points: `1f3f3 fe0f 200d 1f308`|1 cluster: `[1f3f3 fe0f 200d 1f308]`|
-|🇩🇪|8 bytes: `f0 9f 87 a9 f0 9f 87 aa`|2 code points: `1f1e9 1f1ea`|1 cluster: `[1f1e9 1f1ea]`|
-
-This package provides tools to iterate over these grapheme clusters. This may be used to determine the number of user-perceived characters, to split strings in their intended places, or to extract individual characters which form a unit.
-
-### Word Boundaries
-
-Word boundaries are used in a number of different contexts. The most familiar ones are selection (double-click mouse selection), cursor movement ("move to next word" control-arrow keys), and the dialog option "Whole Word Search" for search and replace. They are also used in database queries, to determine whether elements are within a certain number of words of one another. Searching may also use word boundaries in determining matching items. This package provides tools to determine word boundaries within strings.
-
-### Sentence Boundaries
-
-Sentence boundaries are often used for triple-click or some other method of selecting or iterating through blocks of text that are larger than single words. They are also used to determine whether words occur within the same sentence in database queries. This package provides tools to determine sentence boundaries within strings.
-
-### Line Breaking
-
-Line breaking, also known as word wrapping, is the process of breaking a section of text into lines such that it will fit in the available width of a page, window or other display area. This package provides tools to determine where a string may or may not be broken and where it must be broken (for example after newline characters).
-
-### Monospace Width
-
-Most terminals or text displays / text editors using a monospace font (for example source code editors) use a fixed width for each character. Some characters such as emojis or characters found in Asian and other languages may take up more than one character cell. This package provides tools to determine the number of cells a string will take up when displayed in a monospace font. See [here](https://pkg.go.dev/github.com/rivo/uniseg#hdr-Monospace_Width) for more information.
-
-## Installation
-
-```bash
-go get github.com/rivo/uniseg
-```
-
-## Examples
-
-### Counting Characters in a String
-
-```go
-n := uniseg.GraphemeClusterCount("🇩🇪ðŸ³ï¸â€ðŸŒˆ")
-fmt.Println(n)
-// 2
-```
-
-### Calculating the Monospace String Width
-
-```go
-width := uniseg.StringWidth("🇩🇪ðŸ³ï¸â€ðŸŒˆ!")
-fmt.Println(width)
-// 5
-```
-
-### Using the [`Graphemes`](https://pkg.go.dev/github.com/rivo/uniseg#Graphemes) Class
-
-This is the most convenient method of iterating over grapheme clusters:
-
-```go
-gr := uniseg.NewGraphemes("ðŸ‘ðŸ¼!")
-for gr.Next() {
- fmt.Printf("%x ", gr.Runes())
-}
-// [1f44d 1f3fc] [21]
-```
-
-### Using the [`Step`](https://pkg.go.dev/github.com/rivo/uniseg#Step) or [`StepString`](https://pkg.go.dev/github.com/rivo/uniseg#StepString) Function
-
-This is orders of magnitude faster than the `Graphemes` class, but it requires the handling of states and boundaries:
-
-```go
-str := "🇩🇪ðŸ³ï¸â€ðŸŒˆ"
-state := -1
-var c string
-for len(str) > 0 {
- c, str, _, state = uniseg.StepString(str, state)
- fmt.Printf("%x ", []rune(c))
-}
-// [1f1e9 1f1ea] [1f3f3 fe0f 200d 1f308]
-```
-
-### Advanced Examples
-
-Breaking into grapheme clusters and evaluating line breaks:
-
-```go
-str := "First line.\nSecond line."
-state := -1
-var (
- c string
- boundaries int
-)
-for len(str) > 0 {
- c, str, boundaries, state = uniseg.StepString(str, state)
- fmt.Print(c)
- if boundaries&uniseg.MaskLine == uniseg.LineCanBreak {
- fmt.Print("|")
- } else if boundaries&uniseg.MaskLine == uniseg.LineMustBreak {
- fmt.Print("‖")
- }
-}
-// First |line.
-// ‖Second |line.‖
-```
-
-If you're only interested in word segmentation, use [`FirstWord`](https://pkg.go.dev/github.com/rivo/uniseg#FirstWord) or [`FirstWordInString`](https://pkg.go.dev/github.com/rivo/uniseg#FirstWordInString):
-
-```go
-str := "Hello, world!"
-state := -1
-var c string
-for len(str) > 0 {
- c, str, state = uniseg.FirstWordInString(str, state)
- fmt.Printf("(%s)\n", c)
-}
-// (Hello)
-// (,)
-// ( )
-// (world)
-// (!)
-```
-
-Similarly, use
-
-- [`FirstGraphemeCluster`](https://pkg.go.dev/github.com/rivo/uniseg#FirstGraphemeCluster) or [`FirstGraphemeClusterInString`](https://pkg.go.dev/github.com/rivo/uniseg#FirstGraphemeClusterInString) for grapheme cluster determination only,
-- [`FirstSentence`](https://pkg.go.dev/github.com/rivo/uniseg#FirstSentence) or [`FirstSentenceInString`](https://pkg.go.dev/github.com/rivo/uniseg#FirstSentenceInString) for sentence segmentation only, and
-- [`FirstLineSegment`](https://pkg.go.dev/github.com/rivo/uniseg#FirstLineSegment) or [`FirstLineSegmentInString`](https://pkg.go.dev/github.com/rivo/uniseg#FirstLineSegmentInString) for line breaking / word wrapping (although using [`Step`](https://pkg.go.dev/github.com/rivo/uniseg#Step) or [`StepString`](https://pkg.go.dev/github.com/rivo/uniseg#StepString) is preferred as it will observe grapheme cluster boundaries).
-
-Finally, if you need to reverse a string while preserving grapheme clusters, use [`ReverseString`](https://pkg.go.dev/github.com/rivo/uniseg#ReverseString):
-
-```go
-fmt.Println(uniseg.ReverseString("🇩🇪ðŸ³ï¸â€ðŸŒˆ"))
-// ðŸ³ï¸â€ðŸŒˆðŸ‡©ðŸ‡ª
-```
-
-## Documentation
-
-Refer to https://pkg.go.dev/github.com/rivo/uniseg for the package's documentation.
-
-## Dependencies
-
-This package does not depend on any packages outside the standard library.
-
-## Sponsor this Project
-
-[Become a Sponsor on GitHub](https://github.com/sponsors/rivo?metadata_source=uniseg_readme) to support this project!
-
-## Your Feedback
-
-Add your issue here on GitHub, preferably before submitting any PR's. Feel free to get in touch if you have any questions.
\ No newline at end of file
diff --git a/vendor/github.com/rivo/uniseg/doc.go b/vendor/github.com/rivo/uniseg/doc.go
deleted file mode 100644
index 11224ae2..00000000
--- a/vendor/github.com/rivo/uniseg/doc.go
+++ /dev/null
@@ -1,108 +0,0 @@
-/*
-Package uniseg implements Unicode Text Segmentation, Unicode Line Breaking, and
-string width calculation for monospace fonts. Unicode Text Segmentation conforms
-to Unicode Standard Annex #29 (https://unicode.org/reports/tr29/) and Unicode
-Line Breaking conforms to Unicode Standard Annex #14
-(https://unicode.org/reports/tr14/).
-
-In short, using this package, you can split a string into grapheme clusters
-(what people would usually refer to as a "character"), into words, and into
-sentences. Or, in its simplest case, this package allows you to count the number
-of characters in a string, especially when it contains complex characters such
-as emojis, combining characters, or characters from Asian, Arabic, Hebrew, or
-other languages. Additionally, you can use it to implement line breaking (or
-"word wrapping"), that is, to determine where text can be broken over to the
-next line when the width of the line is not big enough to fit the entire text.
-Finally, you can use it to calculate the display width of a string for monospace
-fonts.
-
-# Getting Started
-
-If you just want to count the number of characters in a string, you can use
-[GraphemeClusterCount]. If you want to determine the display width of a string,
-you can use [StringWidth]. If you want to iterate over a string, you can use
-[Step], [StepString], or the [Graphemes] class (more convenient but less
-performant). This will provide you with all information: grapheme clusters,
-word boundaries, sentence boundaries, line breaks, and monospace character
-widths. The specialized functions [FirstGraphemeCluster],
-[FirstGraphemeClusterInString], [FirstWord], [FirstWordInString],
-[FirstSentence], and [FirstSentenceInString] can be used if only one type of
-information is needed.
-
-# Grapheme Clusters
-
-Consider the rainbow flag emoji: ðŸ³ï¸â€ðŸŒˆ. On most modern systems, it appears as one
-character. But its string representation actually has 14 bytes, so counting
-bytes (or using len("ðŸ³ï¸â€ðŸŒˆ")) will not work as expected. Counting runes won't,
-either: The flag has 4 Unicode code points, thus 4 runes. The stdlib function
-utf8.RuneCountInString("ðŸ³ï¸â€ðŸŒˆ") and len([]rune("ðŸ³ï¸â€ðŸŒˆ")) will both return 4.
-
-The [GraphemeClusterCount] function will return 1 for the rainbow flag emoji.
-The Graphemes class and a variety of functions in this package will allow you to
-split strings into its grapheme clusters.
-
-# Word Boundaries
-
-Word boundaries are used in a number of different contexts. The most familiar
-ones are selection (double-click mouse selection), cursor movement ("move to
-next word" control-arrow keys), and the dialog option "Whole Word Search" for
-search and replace. This package provides methods for determining word
-boundaries.
-
-# Sentence Boundaries
-
-Sentence boundaries are often used for triple-click or some other method of
-selecting or iterating through blocks of text that are larger than single words.
-They are also used to determine whether words occur within the same sentence in
-database queries. This package provides methods for determining sentence
-boundaries.
-
-# Line Breaking
-
-Line breaking, also known as word wrapping, is the process of breaking a section
-of text into lines such that it will fit in the available width of a page,
-window or other display area. This package provides methods to determine the
-positions in a string where a line must be broken, may be broken, or must not be
-broken.
-
-# Monospace Width
-
-Monospace width, as referred to in this package, is the width of a string in a
-monospace font. This is commonly used in terminal user interfaces or text
-displays or editors that don't support proportional fonts. A width of 1
-corresponds to a single character cell. The C function [wcswidth()] and its
-implementation in other programming languages is in widespread use for the same
-purpose. However, there is no standard for the calculation of such widths, and
-this package differs from wcswidth() in a number of ways, presumably to generate
-more visually pleasing results.
-
-To start, we assume that every code point has a width of 1, with the following
-exceptions:
-
- - Code points with grapheme cluster break properties Control, CR, LF, Extend,
- and ZWJ have a width of 0.
- - U+2E3A, Two-Em Dash, has a width of 3.
- - U+2E3B, Three-Em Dash, has a width of 4.
- - Characters with the East-Asian Width properties "Fullwidth" (F) and "Wide"
- (W) have a width of 2. (Properties "Ambiguous" (A) and "Neutral" (N) both
- have a width of 1.)
- - Code points with grapheme cluster break property Regional Indicator have a
- width of 2.
- - Code points with grapheme cluster break property Extended Pictographic have
- a width of 2, unless their Emoji Presentation flag is "No", in which case
- the width is 1.
-
-For Hangul grapheme clusters composed of conjoining Jamo and for Regional
-Indicators (flags), all code points except the first one have a width of 0. For
-grapheme clusters starting with an Extended Pictographic, any additional code
-point will force a total width of 2, except if the Variation Selector-15
-(U+FE0E) is included, in which case the total width is always 1. Grapheme
-clusters ending with Variation Selector-16 (U+FE0F) have a width of 2.
-
-Note that whether these widths appear correct depends on your application's
-render engine, to which extent it conforms to the Unicode Standard, and its
-choice of font.
-
-[wcswidth()]: https://man7.org/linux/man-pages/man3/wcswidth.3.html
-*/
-package uniseg
diff --git a/vendor/github.com/rivo/uniseg/eastasianwidth.go b/vendor/github.com/rivo/uniseg/eastasianwidth.go
deleted file mode 100644
index 661934ac..00000000
--- a/vendor/github.com/rivo/uniseg/eastasianwidth.go
+++ /dev/null
@@ -1,2556 +0,0 @@
-package uniseg
-
-// Code generated via go generate from gen_properties.go. DO NOT EDIT.
-
-// eastAsianWidth are taken from
-// https://www.unicode.org/Public/14.0.0/ucd/EastAsianWidth.txt
-// and
-// https://unicode.org/Public/14.0.0/ucd/emoji/emoji-data.txt
-// ("Extended_Pictographic" only)
-// on September 10, 2022. See https://www.unicode.org/license.html for the Unicode
-// license agreement.
-var eastAsianWidth = [][3]int{
- {0x0000, 0x001F, prN}, // Cc [32] ..
- {0x0020, 0x0020, prNa}, // Zs SPACE
- {0x0021, 0x0023, prNa}, // Po [3] EXCLAMATION MARK..NUMBER SIGN
- {0x0024, 0x0024, prNa}, // Sc DOLLAR SIGN
- {0x0025, 0x0027, prNa}, // Po [3] PERCENT SIGN..APOSTROPHE
- {0x0028, 0x0028, prNa}, // Ps LEFT PARENTHESIS
- {0x0029, 0x0029, prNa}, // Pe RIGHT PARENTHESIS
- {0x002A, 0x002A, prNa}, // Po ASTERISK
- {0x002B, 0x002B, prNa}, // Sm PLUS SIGN
- {0x002C, 0x002C, prNa}, // Po COMMA
- {0x002D, 0x002D, prNa}, // Pd HYPHEN-MINUS
- {0x002E, 0x002F, prNa}, // Po [2] FULL STOP..SOLIDUS
- {0x0030, 0x0039, prNa}, // Nd [10] DIGIT ZERO..DIGIT NINE
- {0x003A, 0x003B, prNa}, // Po [2] COLON..SEMICOLON
- {0x003C, 0x003E, prNa}, // Sm [3] LESS-THAN SIGN..GREATER-THAN SIGN
- {0x003F, 0x0040, prNa}, // Po [2] QUESTION MARK..COMMERCIAL AT
- {0x0041, 0x005A, prNa}, // Lu [26] LATIN CAPITAL LETTER A..LATIN CAPITAL LETTER Z
- {0x005B, 0x005B, prNa}, // Ps LEFT SQUARE BRACKET
- {0x005C, 0x005C, prNa}, // Po REVERSE SOLIDUS
- {0x005D, 0x005D, prNa}, // Pe RIGHT SQUARE BRACKET
- {0x005E, 0x005E, prNa}, // Sk CIRCUMFLEX ACCENT
- {0x005F, 0x005F, prNa}, // Pc LOW LINE
- {0x0060, 0x0060, prNa}, // Sk GRAVE ACCENT
- {0x0061, 0x007A, prNa}, // Ll [26] LATIN SMALL LETTER A..LATIN SMALL LETTER Z
- {0x007B, 0x007B, prNa}, // Ps LEFT CURLY BRACKET
- {0x007C, 0x007C, prNa}, // Sm VERTICAL LINE
- {0x007D, 0x007D, prNa}, // Pe RIGHT CURLY BRACKET
- {0x007E, 0x007E, prNa}, // Sm TILDE
- {0x007F, 0x007F, prN}, // Cc
- {0x0080, 0x009F, prN}, // Cc [32] ..
- {0x00A0, 0x00A0, prN}, // Zs NO-BREAK SPACE
- {0x00A1, 0x00A1, prA}, // Po INVERTED EXCLAMATION MARK
- {0x00A2, 0x00A3, prNa}, // Sc [2] CENT SIGN..POUND SIGN
- {0x00A4, 0x00A4, prA}, // Sc CURRENCY SIGN
- {0x00A5, 0x00A5, prNa}, // Sc YEN SIGN
- {0x00A6, 0x00A6, prNa}, // So BROKEN BAR
- {0x00A7, 0x00A7, prA}, // Po SECTION SIGN
- {0x00A8, 0x00A8, prA}, // Sk DIAERESIS
- {0x00A9, 0x00A9, prN}, // So COPYRIGHT SIGN
- {0x00AA, 0x00AA, prA}, // Lo FEMININE ORDINAL INDICATOR
- {0x00AB, 0x00AB, prN}, // Pi LEFT-POINTING DOUBLE ANGLE QUOTATION MARK
- {0x00AC, 0x00AC, prNa}, // Sm NOT SIGN
- {0x00AD, 0x00AD, prA}, // Cf SOFT HYPHEN
- {0x00AE, 0x00AE, prA}, // So REGISTERED SIGN
- {0x00AF, 0x00AF, prNa}, // Sk MACRON
- {0x00B0, 0x00B0, prA}, // So DEGREE SIGN
- {0x00B1, 0x00B1, prA}, // Sm PLUS-MINUS SIGN
- {0x00B2, 0x00B3, prA}, // No [2] SUPERSCRIPT TWO..SUPERSCRIPT THREE
- {0x00B4, 0x00B4, prA}, // Sk ACUTE ACCENT
- {0x00B5, 0x00B5, prN}, // Ll MICRO SIGN
- {0x00B6, 0x00B7, prA}, // Po [2] PILCROW SIGN..MIDDLE DOT
- {0x00B8, 0x00B8, prA}, // Sk CEDILLA
- {0x00B9, 0x00B9, prA}, // No SUPERSCRIPT ONE
- {0x00BA, 0x00BA, prA}, // Lo MASCULINE ORDINAL INDICATOR
- {0x00BB, 0x00BB, prN}, // Pf RIGHT-POINTING DOUBLE ANGLE QUOTATION MARK
- {0x00BC, 0x00BE, prA}, // No [3] VULGAR FRACTION ONE QUARTER..VULGAR FRACTION THREE QUARTERS
- {0x00BF, 0x00BF, prA}, // Po INVERTED QUESTION MARK
- {0x00C0, 0x00C5, prN}, // Lu [6] LATIN CAPITAL LETTER A WITH GRAVE..LATIN CAPITAL LETTER A WITH RING ABOVE
- {0x00C6, 0x00C6, prA}, // Lu LATIN CAPITAL LETTER AE
- {0x00C7, 0x00CF, prN}, // Lu [9] LATIN CAPITAL LETTER C WITH CEDILLA..LATIN CAPITAL LETTER I WITH DIAERESIS
- {0x00D0, 0x00D0, prA}, // Lu LATIN CAPITAL LETTER ETH
- {0x00D1, 0x00D6, prN}, // Lu [6] LATIN CAPITAL LETTER N WITH TILDE..LATIN CAPITAL LETTER O WITH DIAERESIS
- {0x00D7, 0x00D7, prA}, // Sm MULTIPLICATION SIGN
- {0x00D8, 0x00D8, prA}, // Lu LATIN CAPITAL LETTER O WITH STROKE
- {0x00D9, 0x00DD, prN}, // Lu [5] LATIN CAPITAL LETTER U WITH GRAVE..LATIN CAPITAL LETTER Y WITH ACUTE
- {0x00DE, 0x00E1, prA}, // L& [4] LATIN CAPITAL LETTER THORN..LATIN SMALL LETTER A WITH ACUTE
- {0x00E2, 0x00E5, prN}, // Ll [4] LATIN SMALL LETTER A WITH CIRCUMFLEX..LATIN SMALL LETTER A WITH RING ABOVE
- {0x00E6, 0x00E6, prA}, // Ll LATIN SMALL LETTER AE
- {0x00E7, 0x00E7, prN}, // Ll LATIN SMALL LETTER C WITH CEDILLA
- {0x00E8, 0x00EA, prA}, // Ll [3] LATIN SMALL LETTER E WITH GRAVE..LATIN SMALL LETTER E WITH CIRCUMFLEX
- {0x00EB, 0x00EB, prN}, // Ll LATIN SMALL LETTER E WITH DIAERESIS
- {0x00EC, 0x00ED, prA}, // Ll [2] LATIN SMALL LETTER I WITH GRAVE..LATIN SMALL LETTER I WITH ACUTE
- {0x00EE, 0x00EF, prN}, // Ll [2] LATIN SMALL LETTER I WITH CIRCUMFLEX..LATIN SMALL LETTER I WITH DIAERESIS
- {0x00F0, 0x00F0, prA}, // Ll LATIN SMALL LETTER ETH
- {0x00F1, 0x00F1, prN}, // Ll LATIN SMALL LETTER N WITH TILDE
- {0x00F2, 0x00F3, prA}, // Ll [2] LATIN SMALL LETTER O WITH GRAVE..LATIN SMALL LETTER O WITH ACUTE
- {0x00F4, 0x00F6, prN}, // Ll [3] LATIN SMALL LETTER O WITH CIRCUMFLEX..LATIN SMALL LETTER O WITH DIAERESIS
- {0x00F7, 0x00F7, prA}, // Sm DIVISION SIGN
- {0x00F8, 0x00FA, prA}, // Ll [3] LATIN SMALL LETTER O WITH STROKE..LATIN SMALL LETTER U WITH ACUTE
- {0x00FB, 0x00FB, prN}, // Ll LATIN SMALL LETTER U WITH CIRCUMFLEX
- {0x00FC, 0x00FC, prA}, // Ll LATIN SMALL LETTER U WITH DIAERESIS
- {0x00FD, 0x00FD, prN}, // Ll LATIN SMALL LETTER Y WITH ACUTE
- {0x00FE, 0x00FE, prA}, // Ll LATIN SMALL LETTER THORN
- {0x00FF, 0x00FF, prN}, // Ll LATIN SMALL LETTER Y WITH DIAERESIS
- {0x0100, 0x0100, prN}, // Lu LATIN CAPITAL LETTER A WITH MACRON
- {0x0101, 0x0101, prA}, // Ll LATIN SMALL LETTER A WITH MACRON
- {0x0102, 0x0110, prN}, // L& [15] LATIN CAPITAL LETTER A WITH BREVE..LATIN CAPITAL LETTER D WITH STROKE
- {0x0111, 0x0111, prA}, // Ll LATIN SMALL LETTER D WITH STROKE
- {0x0112, 0x0112, prN}, // Lu LATIN CAPITAL LETTER E WITH MACRON
- {0x0113, 0x0113, prA}, // Ll LATIN SMALL LETTER E WITH MACRON
- {0x0114, 0x011A, prN}, // L& [7] LATIN CAPITAL LETTER E WITH BREVE..LATIN CAPITAL LETTER E WITH CARON
- {0x011B, 0x011B, prA}, // Ll LATIN SMALL LETTER E WITH CARON
- {0x011C, 0x0125, prN}, // L& [10] LATIN CAPITAL LETTER G WITH CIRCUMFLEX..LATIN SMALL LETTER H WITH CIRCUMFLEX
- {0x0126, 0x0127, prA}, // L& [2] LATIN CAPITAL LETTER H WITH STROKE..LATIN SMALL LETTER H WITH STROKE
- {0x0128, 0x012A, prN}, // L& [3] LATIN CAPITAL LETTER I WITH TILDE..LATIN CAPITAL LETTER I WITH MACRON
- {0x012B, 0x012B, prA}, // Ll LATIN SMALL LETTER I WITH MACRON
- {0x012C, 0x0130, prN}, // L& [5] LATIN CAPITAL LETTER I WITH BREVE..LATIN CAPITAL LETTER I WITH DOT ABOVE
- {0x0131, 0x0133, prA}, // L& [3] LATIN SMALL LETTER DOTLESS I..LATIN SMALL LIGATURE IJ
- {0x0134, 0x0137, prN}, // L& [4] LATIN CAPITAL LETTER J WITH CIRCUMFLEX..LATIN SMALL LETTER K WITH CEDILLA
- {0x0138, 0x0138, prA}, // Ll LATIN SMALL LETTER KRA
- {0x0139, 0x013E, prN}, // L& [6] LATIN CAPITAL LETTER L WITH ACUTE..LATIN SMALL LETTER L WITH CARON
- {0x013F, 0x0142, prA}, // L& [4] LATIN CAPITAL LETTER L WITH MIDDLE DOT..LATIN SMALL LETTER L WITH STROKE
- {0x0143, 0x0143, prN}, // Lu LATIN CAPITAL LETTER N WITH ACUTE
- {0x0144, 0x0144, prA}, // Ll LATIN SMALL LETTER N WITH ACUTE
- {0x0145, 0x0147, prN}, // L& [3] LATIN CAPITAL LETTER N WITH CEDILLA..LATIN CAPITAL LETTER N WITH CARON
- {0x0148, 0x014B, prA}, // L& [4] LATIN SMALL LETTER N WITH CARON..LATIN SMALL LETTER ENG
- {0x014C, 0x014C, prN}, // Lu LATIN CAPITAL LETTER O WITH MACRON
- {0x014D, 0x014D, prA}, // Ll LATIN SMALL LETTER O WITH MACRON
- {0x014E, 0x0151, prN}, // L& [4] LATIN CAPITAL LETTER O WITH BREVE..LATIN SMALL LETTER O WITH DOUBLE ACUTE
- {0x0152, 0x0153, prA}, // L& [2] LATIN CAPITAL LIGATURE OE..LATIN SMALL LIGATURE OE
- {0x0154, 0x0165, prN}, // L& [18] LATIN CAPITAL LETTER R WITH ACUTE..LATIN SMALL LETTER T WITH CARON
- {0x0166, 0x0167, prA}, // L& [2] LATIN CAPITAL LETTER T WITH STROKE..LATIN SMALL LETTER T WITH STROKE
- {0x0168, 0x016A, prN}, // L& [3] LATIN CAPITAL LETTER U WITH TILDE..LATIN CAPITAL LETTER U WITH MACRON
- {0x016B, 0x016B, prA}, // Ll LATIN SMALL LETTER U WITH MACRON
- {0x016C, 0x017F, prN}, // L& [20] LATIN CAPITAL LETTER U WITH BREVE..LATIN SMALL LETTER LONG S
- {0x0180, 0x01BA, prN}, // L& [59] LATIN SMALL LETTER B WITH STROKE..LATIN SMALL LETTER EZH WITH TAIL
- {0x01BB, 0x01BB, prN}, // Lo LATIN LETTER TWO WITH STROKE
- {0x01BC, 0x01BF, prN}, // L& [4] LATIN CAPITAL LETTER TONE FIVE..LATIN LETTER WYNN
- {0x01C0, 0x01C3, prN}, // Lo [4] LATIN LETTER DENTAL CLICK..LATIN LETTER RETROFLEX CLICK
- {0x01C4, 0x01CD, prN}, // L& [10] LATIN CAPITAL LETTER DZ WITH CARON..LATIN CAPITAL LETTER A WITH CARON
- {0x01CE, 0x01CE, prA}, // Ll LATIN SMALL LETTER A WITH CARON
- {0x01CF, 0x01CF, prN}, // Lu LATIN CAPITAL LETTER I WITH CARON
- {0x01D0, 0x01D0, prA}, // Ll LATIN SMALL LETTER I WITH CARON
- {0x01D1, 0x01D1, prN}, // Lu LATIN CAPITAL LETTER O WITH CARON
- {0x01D2, 0x01D2, prA}, // Ll LATIN SMALL LETTER O WITH CARON
- {0x01D3, 0x01D3, prN}, // Lu LATIN CAPITAL LETTER U WITH CARON
- {0x01D4, 0x01D4, prA}, // Ll LATIN SMALL LETTER U WITH CARON
- {0x01D5, 0x01D5, prN}, // Lu LATIN CAPITAL LETTER U WITH DIAERESIS AND MACRON
- {0x01D6, 0x01D6, prA}, // Ll LATIN SMALL LETTER U WITH DIAERESIS AND MACRON
- {0x01D7, 0x01D7, prN}, // Lu LATIN CAPITAL LETTER U WITH DIAERESIS AND ACUTE
- {0x01D8, 0x01D8, prA}, // Ll LATIN SMALL LETTER U WITH DIAERESIS AND ACUTE
- {0x01D9, 0x01D9, prN}, // Lu LATIN CAPITAL LETTER U WITH DIAERESIS AND CARON
- {0x01DA, 0x01DA, prA}, // Ll LATIN SMALL LETTER U WITH DIAERESIS AND CARON
- {0x01DB, 0x01DB, prN}, // Lu LATIN CAPITAL LETTER U WITH DIAERESIS AND GRAVE
- {0x01DC, 0x01DC, prA}, // Ll LATIN SMALL LETTER U WITH DIAERESIS AND GRAVE
- {0x01DD, 0x024F, prN}, // L& [115] LATIN SMALL LETTER TURNED E..LATIN SMALL LETTER Y WITH STROKE
- {0x0250, 0x0250, prN}, // Ll LATIN SMALL LETTER TURNED A
- {0x0251, 0x0251, prA}, // Ll LATIN SMALL LETTER ALPHA
- {0x0252, 0x0260, prN}, // Ll [15] LATIN SMALL LETTER TURNED ALPHA..LATIN SMALL LETTER G WITH HOOK
- {0x0261, 0x0261, prA}, // Ll LATIN SMALL LETTER SCRIPT G
- {0x0262, 0x0293, prN}, // Ll [50] LATIN LETTER SMALL CAPITAL G..LATIN SMALL LETTER EZH WITH CURL
- {0x0294, 0x0294, prN}, // Lo LATIN LETTER GLOTTAL STOP
- {0x0295, 0x02AF, prN}, // Ll [27] LATIN LETTER PHARYNGEAL VOICED FRICATIVE..LATIN SMALL LETTER TURNED H WITH FISHHOOK AND TAIL
- {0x02B0, 0x02C1, prN}, // Lm [18] MODIFIER LETTER SMALL H..MODIFIER LETTER REVERSED GLOTTAL STOP
- {0x02C2, 0x02C3, prN}, // Sk [2] MODIFIER LETTER LEFT ARROWHEAD..MODIFIER LETTER RIGHT ARROWHEAD
- {0x02C4, 0x02C4, prA}, // Sk MODIFIER LETTER UP ARROWHEAD
- {0x02C5, 0x02C5, prN}, // Sk MODIFIER LETTER DOWN ARROWHEAD
- {0x02C6, 0x02C6, prN}, // Lm MODIFIER LETTER CIRCUMFLEX ACCENT
- {0x02C7, 0x02C7, prA}, // Lm CARON
- {0x02C8, 0x02C8, prN}, // Lm MODIFIER LETTER VERTICAL LINE
- {0x02C9, 0x02CB, prA}, // Lm [3] MODIFIER LETTER MACRON..MODIFIER LETTER GRAVE ACCENT
- {0x02CC, 0x02CC, prN}, // Lm MODIFIER LETTER LOW VERTICAL LINE
- {0x02CD, 0x02CD, prA}, // Lm MODIFIER LETTER LOW MACRON
- {0x02CE, 0x02CF, prN}, // Lm [2] MODIFIER LETTER LOW GRAVE ACCENT..MODIFIER LETTER LOW ACUTE ACCENT
- {0x02D0, 0x02D0, prA}, // Lm MODIFIER LETTER TRIANGULAR COLON
- {0x02D1, 0x02D1, prN}, // Lm MODIFIER LETTER HALF TRIANGULAR COLON
- {0x02D2, 0x02D7, prN}, // Sk [6] MODIFIER LETTER CENTRED RIGHT HALF RING..MODIFIER LETTER MINUS SIGN
- {0x02D8, 0x02DB, prA}, // Sk [4] BREVE..OGONEK
- {0x02DC, 0x02DC, prN}, // Sk SMALL TILDE
- {0x02DD, 0x02DD, prA}, // Sk DOUBLE ACUTE ACCENT
- {0x02DE, 0x02DE, prN}, // Sk MODIFIER LETTER RHOTIC HOOK
- {0x02DF, 0x02DF, prA}, // Sk MODIFIER LETTER CROSS ACCENT
- {0x02E0, 0x02E4, prN}, // Lm [5] MODIFIER LETTER SMALL GAMMA..MODIFIER LETTER SMALL REVERSED GLOTTAL STOP
- {0x02E5, 0x02EB, prN}, // Sk [7] MODIFIER LETTER EXTRA-HIGH TONE BAR..MODIFIER LETTER YANG DEPARTING TONE MARK
- {0x02EC, 0x02EC, prN}, // Lm MODIFIER LETTER VOICING
- {0x02ED, 0x02ED, prN}, // Sk MODIFIER LETTER UNASPIRATED
- {0x02EE, 0x02EE, prN}, // Lm MODIFIER LETTER DOUBLE APOSTROPHE
- {0x02EF, 0x02FF, prN}, // Sk [17] MODIFIER LETTER LOW DOWN ARROWHEAD..MODIFIER LETTER LOW LEFT ARROW
- {0x0300, 0x036F, prA}, // Mn [112] COMBINING GRAVE ACCENT..COMBINING LATIN SMALL LETTER X
- {0x0370, 0x0373, prN}, // L& [4] GREEK CAPITAL LETTER HETA..GREEK SMALL LETTER ARCHAIC SAMPI
- {0x0374, 0x0374, prN}, // Lm GREEK NUMERAL SIGN
- {0x0375, 0x0375, prN}, // Sk GREEK LOWER NUMERAL SIGN
- {0x0376, 0x0377, prN}, // L& [2] GREEK CAPITAL LETTER PAMPHYLIAN DIGAMMA..GREEK SMALL LETTER PAMPHYLIAN DIGAMMA
- {0x037A, 0x037A, prN}, // Lm GREEK YPOGEGRAMMENI
- {0x037B, 0x037D, prN}, // Ll [3] GREEK SMALL REVERSED LUNATE SIGMA SYMBOL..GREEK SMALL REVERSED DOTTED LUNATE SIGMA SYMBOL
- {0x037E, 0x037E, prN}, // Po GREEK QUESTION MARK
- {0x037F, 0x037F, prN}, // Lu GREEK CAPITAL LETTER YOT
- {0x0384, 0x0385, prN}, // Sk [2] GREEK TONOS..GREEK DIALYTIKA TONOS
- {0x0386, 0x0386, prN}, // Lu GREEK CAPITAL LETTER ALPHA WITH TONOS
- {0x0387, 0x0387, prN}, // Po GREEK ANO TELEIA
- {0x0388, 0x038A, prN}, // Lu [3] GREEK CAPITAL LETTER EPSILON WITH TONOS..GREEK CAPITAL LETTER IOTA WITH TONOS
- {0x038C, 0x038C, prN}, // Lu GREEK CAPITAL LETTER OMICRON WITH TONOS
- {0x038E, 0x0390, prN}, // L& [3] GREEK CAPITAL LETTER UPSILON WITH TONOS..GREEK SMALL LETTER IOTA WITH DIALYTIKA AND TONOS
- {0x0391, 0x03A1, prA}, // Lu [17] GREEK CAPITAL LETTER ALPHA..GREEK CAPITAL LETTER RHO
- {0x03A3, 0x03A9, prA}, // Lu [7] GREEK CAPITAL LETTER SIGMA..GREEK CAPITAL LETTER OMEGA
- {0x03AA, 0x03B0, prN}, // L& [7] GREEK CAPITAL LETTER IOTA WITH DIALYTIKA..GREEK SMALL LETTER UPSILON WITH DIALYTIKA AND TONOS
- {0x03B1, 0x03C1, prA}, // Ll [17] GREEK SMALL LETTER ALPHA..GREEK SMALL LETTER RHO
- {0x03C2, 0x03C2, prN}, // Ll GREEK SMALL LETTER FINAL SIGMA
- {0x03C3, 0x03C9, prA}, // Ll [7] GREEK SMALL LETTER SIGMA..GREEK SMALL LETTER OMEGA
- {0x03CA, 0x03F5, prN}, // L& [44] GREEK SMALL LETTER IOTA WITH DIALYTIKA..GREEK LUNATE EPSILON SYMBOL
- {0x03F6, 0x03F6, prN}, // Sm GREEK REVERSED LUNATE EPSILON SYMBOL
- {0x03F7, 0x03FF, prN}, // L& [9] GREEK CAPITAL LETTER SHO..GREEK CAPITAL REVERSED DOTTED LUNATE SIGMA SYMBOL
- {0x0400, 0x0400, prN}, // Lu CYRILLIC CAPITAL LETTER IE WITH GRAVE
- {0x0401, 0x0401, prA}, // Lu CYRILLIC CAPITAL LETTER IO
- {0x0402, 0x040F, prN}, // Lu [14] CYRILLIC CAPITAL LETTER DJE..CYRILLIC CAPITAL LETTER DZHE
- {0x0410, 0x044F, prA}, // L& [64] CYRILLIC CAPITAL LETTER A..CYRILLIC SMALL LETTER YA
- {0x0450, 0x0450, prN}, // Ll CYRILLIC SMALL LETTER IE WITH GRAVE
- {0x0451, 0x0451, prA}, // Ll CYRILLIC SMALL LETTER IO
- {0x0452, 0x0481, prN}, // L& [48] CYRILLIC SMALL LETTER DJE..CYRILLIC SMALL LETTER KOPPA
- {0x0482, 0x0482, prN}, // So CYRILLIC THOUSANDS SIGN
- {0x0483, 0x0487, prN}, // Mn [5] COMBINING CYRILLIC TITLO..COMBINING CYRILLIC POKRYTIE
- {0x0488, 0x0489, prN}, // Me [2] COMBINING CYRILLIC HUNDRED THOUSANDS SIGN..COMBINING CYRILLIC MILLIONS SIGN
- {0x048A, 0x04FF, prN}, // L& [118] CYRILLIC CAPITAL LETTER SHORT I WITH TAIL..CYRILLIC SMALL LETTER HA WITH STROKE
- {0x0500, 0x052F, prN}, // L& [48] CYRILLIC CAPITAL LETTER KOMI DE..CYRILLIC SMALL LETTER EL WITH DESCENDER
- {0x0531, 0x0556, prN}, // Lu [38] ARMENIAN CAPITAL LETTER AYB..ARMENIAN CAPITAL LETTER FEH
- {0x0559, 0x0559, prN}, // Lm ARMENIAN MODIFIER LETTER LEFT HALF RING
- {0x055A, 0x055F, prN}, // Po [6] ARMENIAN APOSTROPHE..ARMENIAN ABBREVIATION MARK
- {0x0560, 0x0588, prN}, // Ll [41] ARMENIAN SMALL LETTER TURNED AYB..ARMENIAN SMALL LETTER YI WITH STROKE
- {0x0589, 0x0589, prN}, // Po ARMENIAN FULL STOP
- {0x058A, 0x058A, prN}, // Pd ARMENIAN HYPHEN
- {0x058D, 0x058E, prN}, // So [2] RIGHT-FACING ARMENIAN ETERNITY SIGN..LEFT-FACING ARMENIAN ETERNITY SIGN
- {0x058F, 0x058F, prN}, // Sc ARMENIAN DRAM SIGN
- {0x0591, 0x05BD, prN}, // Mn [45] HEBREW ACCENT ETNAHTA..HEBREW POINT METEG
- {0x05BE, 0x05BE, prN}, // Pd HEBREW PUNCTUATION MAQAF
- {0x05BF, 0x05BF, prN}, // Mn HEBREW POINT RAFE
- {0x05C0, 0x05C0, prN}, // Po HEBREW PUNCTUATION PASEQ
- {0x05C1, 0x05C2, prN}, // Mn [2] HEBREW POINT SHIN DOT..HEBREW POINT SIN DOT
- {0x05C3, 0x05C3, prN}, // Po HEBREW PUNCTUATION SOF PASUQ
- {0x05C4, 0x05C5, prN}, // Mn [2] HEBREW MARK UPPER DOT..HEBREW MARK LOWER DOT
- {0x05C6, 0x05C6, prN}, // Po HEBREW PUNCTUATION NUN HAFUKHA
- {0x05C7, 0x05C7, prN}, // Mn HEBREW POINT QAMATS QATAN
- {0x05D0, 0x05EA, prN}, // Lo [27] HEBREW LETTER ALEF..HEBREW LETTER TAV
- {0x05EF, 0x05F2, prN}, // Lo [4] HEBREW YOD TRIANGLE..HEBREW LIGATURE YIDDISH DOUBLE YOD
- {0x05F3, 0x05F4, prN}, // Po [2] HEBREW PUNCTUATION GERESH..HEBREW PUNCTUATION GERSHAYIM
- {0x0600, 0x0605, prN}, // Cf [6] ARABIC NUMBER SIGN..ARABIC NUMBER MARK ABOVE
- {0x0606, 0x0608, prN}, // Sm [3] ARABIC-INDIC CUBE ROOT..ARABIC RAY
- {0x0609, 0x060A, prN}, // Po [2] ARABIC-INDIC PER MILLE SIGN..ARABIC-INDIC PER TEN THOUSAND SIGN
- {0x060B, 0x060B, prN}, // Sc AFGHANI SIGN
- {0x060C, 0x060D, prN}, // Po [2] ARABIC COMMA..ARABIC DATE SEPARATOR
- {0x060E, 0x060F, prN}, // So [2] ARABIC POETIC VERSE SIGN..ARABIC SIGN MISRA
- {0x0610, 0x061A, prN}, // Mn [11] ARABIC SIGN SALLALLAHOU ALAYHE WASSALLAM..ARABIC SMALL KASRA
- {0x061B, 0x061B, prN}, // Po ARABIC SEMICOLON
- {0x061C, 0x061C, prN}, // Cf ARABIC LETTER MARK
- {0x061D, 0x061F, prN}, // Po [3] ARABIC END OF TEXT MARK..ARABIC QUESTION MARK
- {0x0620, 0x063F, prN}, // Lo [32] ARABIC LETTER KASHMIRI YEH..ARABIC LETTER FARSI YEH WITH THREE DOTS ABOVE
- {0x0640, 0x0640, prN}, // Lm ARABIC TATWEEL
- {0x0641, 0x064A, prN}, // Lo [10] ARABIC LETTER FEH..ARABIC LETTER YEH
- {0x064B, 0x065F, prN}, // Mn [21] ARABIC FATHATAN..ARABIC WAVY HAMZA BELOW
- {0x0660, 0x0669, prN}, // Nd [10] ARABIC-INDIC DIGIT ZERO..ARABIC-INDIC DIGIT NINE
- {0x066A, 0x066D, prN}, // Po [4] ARABIC PERCENT SIGN..ARABIC FIVE POINTED STAR
- {0x066E, 0x066F, prN}, // Lo [2] ARABIC LETTER DOTLESS BEH..ARABIC LETTER DOTLESS QAF
- {0x0670, 0x0670, prN}, // Mn ARABIC LETTER SUPERSCRIPT ALEF
- {0x0671, 0x06D3, prN}, // Lo [99] ARABIC LETTER ALEF WASLA..ARABIC LETTER YEH BARREE WITH HAMZA ABOVE
- {0x06D4, 0x06D4, prN}, // Po ARABIC FULL STOP
- {0x06D5, 0x06D5, prN}, // Lo ARABIC LETTER AE
- {0x06D6, 0x06DC, prN}, // Mn [7] ARABIC SMALL HIGH LIGATURE SAD WITH LAM WITH ALEF MAKSURA..ARABIC SMALL HIGH SEEN
- {0x06DD, 0x06DD, prN}, // Cf ARABIC END OF AYAH
- {0x06DE, 0x06DE, prN}, // So ARABIC START OF RUB EL HIZB
- {0x06DF, 0x06E4, prN}, // Mn [6] ARABIC SMALL HIGH ROUNDED ZERO..ARABIC SMALL HIGH MADDA
- {0x06E5, 0x06E6, prN}, // Lm [2] ARABIC SMALL WAW..ARABIC SMALL YEH
- {0x06E7, 0x06E8, prN}, // Mn [2] ARABIC SMALL HIGH YEH..ARABIC SMALL HIGH NOON
- {0x06E9, 0x06E9, prN}, // So ARABIC PLACE OF SAJDAH
- {0x06EA, 0x06ED, prN}, // Mn [4] ARABIC EMPTY CENTRE LOW STOP..ARABIC SMALL LOW MEEM
- {0x06EE, 0x06EF, prN}, // Lo [2] ARABIC LETTER DAL WITH INVERTED V..ARABIC LETTER REH WITH INVERTED V
- {0x06F0, 0x06F9, prN}, // Nd [10] EXTENDED ARABIC-INDIC DIGIT ZERO..EXTENDED ARABIC-INDIC DIGIT NINE
- {0x06FA, 0x06FC, prN}, // Lo [3] ARABIC LETTER SHEEN WITH DOT BELOW..ARABIC LETTER GHAIN WITH DOT BELOW
- {0x06FD, 0x06FE, prN}, // So [2] ARABIC SIGN SINDHI AMPERSAND..ARABIC SIGN SINDHI POSTPOSITION MEN
- {0x06FF, 0x06FF, prN}, // Lo ARABIC LETTER HEH WITH INVERTED V
- {0x0700, 0x070D, prN}, // Po [14] SYRIAC END OF PARAGRAPH..SYRIAC HARKLEAN ASTERISCUS
- {0x070F, 0x070F, prN}, // Cf SYRIAC ABBREVIATION MARK
- {0x0710, 0x0710, prN}, // Lo SYRIAC LETTER ALAPH
- {0x0711, 0x0711, prN}, // Mn SYRIAC LETTER SUPERSCRIPT ALAPH
- {0x0712, 0x072F, prN}, // Lo [30] SYRIAC LETTER BETH..SYRIAC LETTER PERSIAN DHALATH
- {0x0730, 0x074A, prN}, // Mn [27] SYRIAC PTHAHA ABOVE..SYRIAC BARREKH
- {0x074D, 0x074F, prN}, // Lo [3] SYRIAC LETTER SOGDIAN ZHAIN..SYRIAC LETTER SOGDIAN FE
- {0x0750, 0x077F, prN}, // Lo [48] ARABIC LETTER BEH WITH THREE DOTS HORIZONTALLY BELOW..ARABIC LETTER KAF WITH TWO DOTS ABOVE
- {0x0780, 0x07A5, prN}, // Lo [38] THAANA LETTER HAA..THAANA LETTER WAAVU
- {0x07A6, 0x07B0, prN}, // Mn [11] THAANA ABAFILI..THAANA SUKUN
- {0x07B1, 0x07B1, prN}, // Lo THAANA LETTER NAA
- {0x07C0, 0x07C9, prN}, // Nd [10] NKO DIGIT ZERO..NKO DIGIT NINE
- {0x07CA, 0x07EA, prN}, // Lo [33] NKO LETTER A..NKO LETTER JONA RA
- {0x07EB, 0x07F3, prN}, // Mn [9] NKO COMBINING SHORT HIGH TONE..NKO COMBINING DOUBLE DOT ABOVE
- {0x07F4, 0x07F5, prN}, // Lm [2] NKO HIGH TONE APOSTROPHE..NKO LOW TONE APOSTROPHE
- {0x07F6, 0x07F6, prN}, // So NKO SYMBOL OO DENNEN
- {0x07F7, 0x07F9, prN}, // Po [3] NKO SYMBOL GBAKURUNEN..NKO EXCLAMATION MARK
- {0x07FA, 0x07FA, prN}, // Lm NKO LAJANYALAN
- {0x07FD, 0x07FD, prN}, // Mn NKO DANTAYALAN
- {0x07FE, 0x07FF, prN}, // Sc [2] NKO DOROME SIGN..NKO TAMAN SIGN
- {0x0800, 0x0815, prN}, // Lo [22] SAMARITAN LETTER ALAF..SAMARITAN LETTER TAAF
- {0x0816, 0x0819, prN}, // Mn [4] SAMARITAN MARK IN..SAMARITAN MARK DAGESH
- {0x081A, 0x081A, prN}, // Lm SAMARITAN MODIFIER LETTER EPENTHETIC YUT
- {0x081B, 0x0823, prN}, // Mn [9] SAMARITAN MARK EPENTHETIC YUT..SAMARITAN VOWEL SIGN A
- {0x0824, 0x0824, prN}, // Lm SAMARITAN MODIFIER LETTER SHORT A
- {0x0825, 0x0827, prN}, // Mn [3] SAMARITAN VOWEL SIGN SHORT A..SAMARITAN VOWEL SIGN U
- {0x0828, 0x0828, prN}, // Lm SAMARITAN MODIFIER LETTER I
- {0x0829, 0x082D, prN}, // Mn [5] SAMARITAN VOWEL SIGN LONG I..SAMARITAN MARK NEQUDAA
- {0x0830, 0x083E, prN}, // Po [15] SAMARITAN PUNCTUATION NEQUDAA..SAMARITAN PUNCTUATION ANNAAU
- {0x0840, 0x0858, prN}, // Lo [25] MANDAIC LETTER HALQA..MANDAIC LETTER AIN
- {0x0859, 0x085B, prN}, // Mn [3] MANDAIC AFFRICATION MARK..MANDAIC GEMINATION MARK
- {0x085E, 0x085E, prN}, // Po MANDAIC PUNCTUATION
- {0x0860, 0x086A, prN}, // Lo [11] SYRIAC LETTER MALAYALAM NGA..SYRIAC LETTER MALAYALAM SSA
- {0x0870, 0x0887, prN}, // Lo [24] ARABIC LETTER ALEF WITH ATTACHED FATHA..ARABIC BASELINE ROUND DOT
- {0x0888, 0x0888, prN}, // Sk ARABIC RAISED ROUND DOT
- {0x0889, 0x088E, prN}, // Lo [6] ARABIC LETTER NOON WITH INVERTED SMALL V..ARABIC VERTICAL TAIL
- {0x0890, 0x0891, prN}, // Cf [2] ARABIC POUND MARK ABOVE..ARABIC PIASTRE MARK ABOVE
- {0x0898, 0x089F, prN}, // Mn [8] ARABIC SMALL HIGH WORD AL-JUZ..ARABIC HALF MADDA OVER MADDA
- {0x08A0, 0x08C8, prN}, // Lo [41] ARABIC LETTER BEH WITH SMALL V BELOW..ARABIC LETTER GRAF
- {0x08C9, 0x08C9, prN}, // Lm ARABIC SMALL FARSI YEH
- {0x08CA, 0x08E1, prN}, // Mn [24] ARABIC SMALL HIGH FARSI YEH..ARABIC SMALL HIGH SIGN SAFHA
- {0x08E2, 0x08E2, prN}, // Cf ARABIC DISPUTED END OF AYAH
- {0x08E3, 0x08FF, prN}, // Mn [29] ARABIC TURNED DAMMA BELOW..ARABIC MARK SIDEWAYS NOON GHUNNA
- {0x0900, 0x0902, prN}, // Mn [3] DEVANAGARI SIGN INVERTED CANDRABINDU..DEVANAGARI SIGN ANUSVARA
- {0x0903, 0x0903, prN}, // Mc DEVANAGARI SIGN VISARGA
- {0x0904, 0x0939, prN}, // Lo [54] DEVANAGARI LETTER SHORT A..DEVANAGARI LETTER HA
- {0x093A, 0x093A, prN}, // Mn DEVANAGARI VOWEL SIGN OE
- {0x093B, 0x093B, prN}, // Mc DEVANAGARI VOWEL SIGN OOE
- {0x093C, 0x093C, prN}, // Mn DEVANAGARI SIGN NUKTA
- {0x093D, 0x093D, prN}, // Lo DEVANAGARI SIGN AVAGRAHA
- {0x093E, 0x0940, prN}, // Mc [3] DEVANAGARI VOWEL SIGN AA..DEVANAGARI VOWEL SIGN II
- {0x0941, 0x0948, prN}, // Mn [8] DEVANAGARI VOWEL SIGN U..DEVANAGARI VOWEL SIGN AI
- {0x0949, 0x094C, prN}, // Mc [4] DEVANAGARI VOWEL SIGN CANDRA O..DEVANAGARI VOWEL SIGN AU
- {0x094D, 0x094D, prN}, // Mn DEVANAGARI SIGN VIRAMA
- {0x094E, 0x094F, prN}, // Mc [2] DEVANAGARI VOWEL SIGN PRISHTHAMATRA E..DEVANAGARI VOWEL SIGN AW
- {0x0950, 0x0950, prN}, // Lo DEVANAGARI OM
- {0x0951, 0x0957, prN}, // Mn [7] DEVANAGARI STRESS SIGN UDATTA..DEVANAGARI VOWEL SIGN UUE
- {0x0958, 0x0961, prN}, // Lo [10] DEVANAGARI LETTER QA..DEVANAGARI LETTER VOCALIC LL
- {0x0962, 0x0963, prN}, // Mn [2] DEVANAGARI VOWEL SIGN VOCALIC L..DEVANAGARI VOWEL SIGN VOCALIC LL
- {0x0964, 0x0965, prN}, // Po [2] DEVANAGARI DANDA..DEVANAGARI DOUBLE DANDA
- {0x0966, 0x096F, prN}, // Nd [10] DEVANAGARI DIGIT ZERO..DEVANAGARI DIGIT NINE
- {0x0970, 0x0970, prN}, // Po DEVANAGARI ABBREVIATION SIGN
- {0x0971, 0x0971, prN}, // Lm DEVANAGARI SIGN HIGH SPACING DOT
- {0x0972, 0x097F, prN}, // Lo [14] DEVANAGARI LETTER CANDRA A..DEVANAGARI LETTER BBA
- {0x0980, 0x0980, prN}, // Lo BENGALI ANJI
- {0x0981, 0x0981, prN}, // Mn BENGALI SIGN CANDRABINDU
- {0x0982, 0x0983, prN}, // Mc [2] BENGALI SIGN ANUSVARA..BENGALI SIGN VISARGA
- {0x0985, 0x098C, prN}, // Lo [8] BENGALI LETTER A..BENGALI LETTER VOCALIC L
- {0x098F, 0x0990, prN}, // Lo [2] BENGALI LETTER E..BENGALI LETTER AI
- {0x0993, 0x09A8, prN}, // Lo [22] BENGALI LETTER O..BENGALI LETTER NA
- {0x09AA, 0x09B0, prN}, // Lo [7] BENGALI LETTER PA..BENGALI LETTER RA
- {0x09B2, 0x09B2, prN}, // Lo BENGALI LETTER LA
- {0x09B6, 0x09B9, prN}, // Lo [4] BENGALI LETTER SHA..BENGALI LETTER HA
- {0x09BC, 0x09BC, prN}, // Mn BENGALI SIGN NUKTA
- {0x09BD, 0x09BD, prN}, // Lo BENGALI SIGN AVAGRAHA
- {0x09BE, 0x09C0, prN}, // Mc [3] BENGALI VOWEL SIGN AA..BENGALI VOWEL SIGN II
- {0x09C1, 0x09C4, prN}, // Mn [4] BENGALI VOWEL SIGN U..BENGALI VOWEL SIGN VOCALIC RR
- {0x09C7, 0x09C8, prN}, // Mc [2] BENGALI VOWEL SIGN E..BENGALI VOWEL SIGN AI
- {0x09CB, 0x09CC, prN}, // Mc [2] BENGALI VOWEL SIGN O..BENGALI VOWEL SIGN AU
- {0x09CD, 0x09CD, prN}, // Mn BENGALI SIGN VIRAMA
- {0x09CE, 0x09CE, prN}, // Lo BENGALI LETTER KHANDA TA
- {0x09D7, 0x09D7, prN}, // Mc BENGALI AU LENGTH MARK
- {0x09DC, 0x09DD, prN}, // Lo [2] BENGALI LETTER RRA..BENGALI LETTER RHA
- {0x09DF, 0x09E1, prN}, // Lo [3] BENGALI LETTER YYA..BENGALI LETTER VOCALIC LL
- {0x09E2, 0x09E3, prN}, // Mn [2] BENGALI VOWEL SIGN VOCALIC L..BENGALI VOWEL SIGN VOCALIC LL
- {0x09E6, 0x09EF, prN}, // Nd [10] BENGALI DIGIT ZERO..BENGALI DIGIT NINE
- {0x09F0, 0x09F1, prN}, // Lo [2] BENGALI LETTER RA WITH MIDDLE DIAGONAL..BENGALI LETTER RA WITH LOWER DIAGONAL
- {0x09F2, 0x09F3, prN}, // Sc [2] BENGALI RUPEE MARK..BENGALI RUPEE SIGN
- {0x09F4, 0x09F9, prN}, // No [6] BENGALI CURRENCY NUMERATOR ONE..BENGALI CURRENCY DENOMINATOR SIXTEEN
- {0x09FA, 0x09FA, prN}, // So BENGALI ISSHAR
- {0x09FB, 0x09FB, prN}, // Sc BENGALI GANDA MARK
- {0x09FC, 0x09FC, prN}, // Lo BENGALI LETTER VEDIC ANUSVARA
- {0x09FD, 0x09FD, prN}, // Po BENGALI ABBREVIATION SIGN
- {0x09FE, 0x09FE, prN}, // Mn BENGALI SANDHI MARK
- {0x0A01, 0x0A02, prN}, // Mn [2] GURMUKHI SIGN ADAK BINDI..GURMUKHI SIGN BINDI
- {0x0A03, 0x0A03, prN}, // Mc GURMUKHI SIGN VISARGA
- {0x0A05, 0x0A0A, prN}, // Lo [6] GURMUKHI LETTER A..GURMUKHI LETTER UU
- {0x0A0F, 0x0A10, prN}, // Lo [2] GURMUKHI LETTER EE..GURMUKHI LETTER AI
- {0x0A13, 0x0A28, prN}, // Lo [22] GURMUKHI LETTER OO..GURMUKHI LETTER NA
- {0x0A2A, 0x0A30, prN}, // Lo [7] GURMUKHI LETTER PA..GURMUKHI LETTER RA
- {0x0A32, 0x0A33, prN}, // Lo [2] GURMUKHI LETTER LA..GURMUKHI LETTER LLA
- {0x0A35, 0x0A36, prN}, // Lo [2] GURMUKHI LETTER VA..GURMUKHI LETTER SHA
- {0x0A38, 0x0A39, prN}, // Lo [2] GURMUKHI LETTER SA..GURMUKHI LETTER HA
- {0x0A3C, 0x0A3C, prN}, // Mn GURMUKHI SIGN NUKTA
- {0x0A3E, 0x0A40, prN}, // Mc [3] GURMUKHI VOWEL SIGN AA..GURMUKHI VOWEL SIGN II
- {0x0A41, 0x0A42, prN}, // Mn [2] GURMUKHI VOWEL SIGN U..GURMUKHI VOWEL SIGN UU
- {0x0A47, 0x0A48, prN}, // Mn [2] GURMUKHI VOWEL SIGN EE..GURMUKHI VOWEL SIGN AI
- {0x0A4B, 0x0A4D, prN}, // Mn [3] GURMUKHI VOWEL SIGN OO..GURMUKHI SIGN VIRAMA
- {0x0A51, 0x0A51, prN}, // Mn GURMUKHI SIGN UDAAT
- {0x0A59, 0x0A5C, prN}, // Lo [4] GURMUKHI LETTER KHHA..GURMUKHI LETTER RRA
- {0x0A5E, 0x0A5E, prN}, // Lo GURMUKHI LETTER FA
- {0x0A66, 0x0A6F, prN}, // Nd [10] GURMUKHI DIGIT ZERO..GURMUKHI DIGIT NINE
- {0x0A70, 0x0A71, prN}, // Mn [2] GURMUKHI TIPPI..GURMUKHI ADDAK
- {0x0A72, 0x0A74, prN}, // Lo [3] GURMUKHI IRI..GURMUKHI EK ONKAR
- {0x0A75, 0x0A75, prN}, // Mn GURMUKHI SIGN YAKASH
- {0x0A76, 0x0A76, prN}, // Po GURMUKHI ABBREVIATION SIGN
- {0x0A81, 0x0A82, prN}, // Mn [2] GUJARATI SIGN CANDRABINDU..GUJARATI SIGN ANUSVARA
- {0x0A83, 0x0A83, prN}, // Mc GUJARATI SIGN VISARGA
- {0x0A85, 0x0A8D, prN}, // Lo [9] GUJARATI LETTER A..GUJARATI VOWEL CANDRA E
- {0x0A8F, 0x0A91, prN}, // Lo [3] GUJARATI LETTER E..GUJARATI VOWEL CANDRA O
- {0x0A93, 0x0AA8, prN}, // Lo [22] GUJARATI LETTER O..GUJARATI LETTER NA
- {0x0AAA, 0x0AB0, prN}, // Lo [7] GUJARATI LETTER PA..GUJARATI LETTER RA
- {0x0AB2, 0x0AB3, prN}, // Lo [2] GUJARATI LETTER LA..GUJARATI LETTER LLA
- {0x0AB5, 0x0AB9, prN}, // Lo [5] GUJARATI LETTER VA..GUJARATI LETTER HA
- {0x0ABC, 0x0ABC, prN}, // Mn GUJARATI SIGN NUKTA
- {0x0ABD, 0x0ABD, prN}, // Lo GUJARATI SIGN AVAGRAHA
- {0x0ABE, 0x0AC0, prN}, // Mc [3] GUJARATI VOWEL SIGN AA..GUJARATI VOWEL SIGN II
- {0x0AC1, 0x0AC5, prN}, // Mn [5] GUJARATI VOWEL SIGN U..GUJARATI VOWEL SIGN CANDRA E
- {0x0AC7, 0x0AC8, prN}, // Mn [2] GUJARATI VOWEL SIGN E..GUJARATI VOWEL SIGN AI
- {0x0AC9, 0x0AC9, prN}, // Mc GUJARATI VOWEL SIGN CANDRA O
- {0x0ACB, 0x0ACC, prN}, // Mc [2] GUJARATI VOWEL SIGN O..GUJARATI VOWEL SIGN AU
- {0x0ACD, 0x0ACD, prN}, // Mn GUJARATI SIGN VIRAMA
- {0x0AD0, 0x0AD0, prN}, // Lo GUJARATI OM
- {0x0AE0, 0x0AE1, prN}, // Lo [2] GUJARATI LETTER VOCALIC RR..GUJARATI LETTER VOCALIC LL
- {0x0AE2, 0x0AE3, prN}, // Mn [2] GUJARATI VOWEL SIGN VOCALIC L..GUJARATI VOWEL SIGN VOCALIC LL
- {0x0AE6, 0x0AEF, prN}, // Nd [10] GUJARATI DIGIT ZERO..GUJARATI DIGIT NINE
- {0x0AF0, 0x0AF0, prN}, // Po GUJARATI ABBREVIATION SIGN
- {0x0AF1, 0x0AF1, prN}, // Sc GUJARATI RUPEE SIGN
- {0x0AF9, 0x0AF9, prN}, // Lo GUJARATI LETTER ZHA
- {0x0AFA, 0x0AFF, prN}, // Mn [6] GUJARATI SIGN SUKUN..GUJARATI SIGN TWO-CIRCLE NUKTA ABOVE
- {0x0B01, 0x0B01, prN}, // Mn ORIYA SIGN CANDRABINDU
- {0x0B02, 0x0B03, prN}, // Mc [2] ORIYA SIGN ANUSVARA..ORIYA SIGN VISARGA
- {0x0B05, 0x0B0C, prN}, // Lo [8] ORIYA LETTER A..ORIYA LETTER VOCALIC L
- {0x0B0F, 0x0B10, prN}, // Lo [2] ORIYA LETTER E..ORIYA LETTER AI
- {0x0B13, 0x0B28, prN}, // Lo [22] ORIYA LETTER O..ORIYA LETTER NA
- {0x0B2A, 0x0B30, prN}, // Lo [7] ORIYA LETTER PA..ORIYA LETTER RA
- {0x0B32, 0x0B33, prN}, // Lo [2] ORIYA LETTER LA..ORIYA LETTER LLA
- {0x0B35, 0x0B39, prN}, // Lo [5] ORIYA LETTER VA..ORIYA LETTER HA
- {0x0B3C, 0x0B3C, prN}, // Mn ORIYA SIGN NUKTA
- {0x0B3D, 0x0B3D, prN}, // Lo ORIYA SIGN AVAGRAHA
- {0x0B3E, 0x0B3E, prN}, // Mc ORIYA VOWEL SIGN AA
- {0x0B3F, 0x0B3F, prN}, // Mn ORIYA VOWEL SIGN I
- {0x0B40, 0x0B40, prN}, // Mc ORIYA VOWEL SIGN II
- {0x0B41, 0x0B44, prN}, // Mn [4] ORIYA VOWEL SIGN U..ORIYA VOWEL SIGN VOCALIC RR
- {0x0B47, 0x0B48, prN}, // Mc [2] ORIYA VOWEL SIGN E..ORIYA VOWEL SIGN AI
- {0x0B4B, 0x0B4C, prN}, // Mc [2] ORIYA VOWEL SIGN O..ORIYA VOWEL SIGN AU
- {0x0B4D, 0x0B4D, prN}, // Mn ORIYA SIGN VIRAMA
- {0x0B55, 0x0B56, prN}, // Mn [2] ORIYA SIGN OVERLINE..ORIYA AI LENGTH MARK
- {0x0B57, 0x0B57, prN}, // Mc ORIYA AU LENGTH MARK
- {0x0B5C, 0x0B5D, prN}, // Lo [2] ORIYA LETTER RRA..ORIYA LETTER RHA
- {0x0B5F, 0x0B61, prN}, // Lo [3] ORIYA LETTER YYA..ORIYA LETTER VOCALIC LL
- {0x0B62, 0x0B63, prN}, // Mn [2] ORIYA VOWEL SIGN VOCALIC L..ORIYA VOWEL SIGN VOCALIC LL
- {0x0B66, 0x0B6F, prN}, // Nd [10] ORIYA DIGIT ZERO..ORIYA DIGIT NINE
- {0x0B70, 0x0B70, prN}, // So ORIYA ISSHAR
- {0x0B71, 0x0B71, prN}, // Lo ORIYA LETTER WA
- {0x0B72, 0x0B77, prN}, // No [6] ORIYA FRACTION ONE QUARTER..ORIYA FRACTION THREE SIXTEENTHS
- {0x0B82, 0x0B82, prN}, // Mn TAMIL SIGN ANUSVARA
- {0x0B83, 0x0B83, prN}, // Lo TAMIL SIGN VISARGA
- {0x0B85, 0x0B8A, prN}, // Lo [6] TAMIL LETTER A..TAMIL LETTER UU
- {0x0B8E, 0x0B90, prN}, // Lo [3] TAMIL LETTER E..TAMIL LETTER AI
- {0x0B92, 0x0B95, prN}, // Lo [4] TAMIL LETTER O..TAMIL LETTER KA
- {0x0B99, 0x0B9A, prN}, // Lo [2] TAMIL LETTER NGA..TAMIL LETTER CA
- {0x0B9C, 0x0B9C, prN}, // Lo TAMIL LETTER JA
- {0x0B9E, 0x0B9F, prN}, // Lo [2] TAMIL LETTER NYA..TAMIL LETTER TTA
- {0x0BA3, 0x0BA4, prN}, // Lo [2] TAMIL LETTER NNA..TAMIL LETTER TA
- {0x0BA8, 0x0BAA, prN}, // Lo [3] TAMIL LETTER NA..TAMIL LETTER PA
- {0x0BAE, 0x0BB9, prN}, // Lo [12] TAMIL LETTER MA..TAMIL LETTER HA
- {0x0BBE, 0x0BBF, prN}, // Mc [2] TAMIL VOWEL SIGN AA..TAMIL VOWEL SIGN I
- {0x0BC0, 0x0BC0, prN}, // Mn TAMIL VOWEL SIGN II
- {0x0BC1, 0x0BC2, prN}, // Mc [2] TAMIL VOWEL SIGN U..TAMIL VOWEL SIGN UU
- {0x0BC6, 0x0BC8, prN}, // Mc [3] TAMIL VOWEL SIGN E..TAMIL VOWEL SIGN AI
- {0x0BCA, 0x0BCC, prN}, // Mc [3] TAMIL VOWEL SIGN O..TAMIL VOWEL SIGN AU
- {0x0BCD, 0x0BCD, prN}, // Mn TAMIL SIGN VIRAMA
- {0x0BD0, 0x0BD0, prN}, // Lo TAMIL OM
- {0x0BD7, 0x0BD7, prN}, // Mc TAMIL AU LENGTH MARK
- {0x0BE6, 0x0BEF, prN}, // Nd [10] TAMIL DIGIT ZERO..TAMIL DIGIT NINE
- {0x0BF0, 0x0BF2, prN}, // No [3] TAMIL NUMBER TEN..TAMIL NUMBER ONE THOUSAND
- {0x0BF3, 0x0BF8, prN}, // So [6] TAMIL DAY SIGN..TAMIL AS ABOVE SIGN
- {0x0BF9, 0x0BF9, prN}, // Sc TAMIL RUPEE SIGN
- {0x0BFA, 0x0BFA, prN}, // So TAMIL NUMBER SIGN
- {0x0C00, 0x0C00, prN}, // Mn TELUGU SIGN COMBINING CANDRABINDU ABOVE
- {0x0C01, 0x0C03, prN}, // Mc [3] TELUGU SIGN CANDRABINDU..TELUGU SIGN VISARGA
- {0x0C04, 0x0C04, prN}, // Mn TELUGU SIGN COMBINING ANUSVARA ABOVE
- {0x0C05, 0x0C0C, prN}, // Lo [8] TELUGU LETTER A..TELUGU LETTER VOCALIC L
- {0x0C0E, 0x0C10, prN}, // Lo [3] TELUGU LETTER E..TELUGU LETTER AI
- {0x0C12, 0x0C28, prN}, // Lo [23] TELUGU LETTER O..TELUGU LETTER NA
- {0x0C2A, 0x0C39, prN}, // Lo [16] TELUGU LETTER PA..TELUGU LETTER HA
- {0x0C3C, 0x0C3C, prN}, // Mn TELUGU SIGN NUKTA
- {0x0C3D, 0x0C3D, prN}, // Lo TELUGU SIGN AVAGRAHA
- {0x0C3E, 0x0C40, prN}, // Mn [3] TELUGU VOWEL SIGN AA..TELUGU VOWEL SIGN II
- {0x0C41, 0x0C44, prN}, // Mc [4] TELUGU VOWEL SIGN U..TELUGU VOWEL SIGN VOCALIC RR
- {0x0C46, 0x0C48, prN}, // Mn [3] TELUGU VOWEL SIGN E..TELUGU VOWEL SIGN AI
- {0x0C4A, 0x0C4D, prN}, // Mn [4] TELUGU VOWEL SIGN O..TELUGU SIGN VIRAMA
- {0x0C55, 0x0C56, prN}, // Mn [2] TELUGU LENGTH MARK..TELUGU AI LENGTH MARK
- {0x0C58, 0x0C5A, prN}, // Lo [3] TELUGU LETTER TSA..TELUGU LETTER RRRA
- {0x0C5D, 0x0C5D, prN}, // Lo TELUGU LETTER NAKAARA POLLU
- {0x0C60, 0x0C61, prN}, // Lo [2] TELUGU LETTER VOCALIC RR..TELUGU LETTER VOCALIC LL
- {0x0C62, 0x0C63, prN}, // Mn [2] TELUGU VOWEL SIGN VOCALIC L..TELUGU VOWEL SIGN VOCALIC LL
- {0x0C66, 0x0C6F, prN}, // Nd [10] TELUGU DIGIT ZERO..TELUGU DIGIT NINE
- {0x0C77, 0x0C77, prN}, // Po TELUGU SIGN SIDDHAM
- {0x0C78, 0x0C7E, prN}, // No [7] TELUGU FRACTION DIGIT ZERO FOR ODD POWERS OF FOUR..TELUGU FRACTION DIGIT THREE FOR EVEN POWERS OF FOUR
- {0x0C7F, 0x0C7F, prN}, // So TELUGU SIGN TUUMU
- {0x0C80, 0x0C80, prN}, // Lo KANNADA SIGN SPACING CANDRABINDU
- {0x0C81, 0x0C81, prN}, // Mn KANNADA SIGN CANDRABINDU
- {0x0C82, 0x0C83, prN}, // Mc [2] KANNADA SIGN ANUSVARA..KANNADA SIGN VISARGA
- {0x0C84, 0x0C84, prN}, // Po KANNADA SIGN SIDDHAM
- {0x0C85, 0x0C8C, prN}, // Lo [8] KANNADA LETTER A..KANNADA LETTER VOCALIC L
- {0x0C8E, 0x0C90, prN}, // Lo [3] KANNADA LETTER E..KANNADA LETTER AI
- {0x0C92, 0x0CA8, prN}, // Lo [23] KANNADA LETTER O..KANNADA LETTER NA
- {0x0CAA, 0x0CB3, prN}, // Lo [10] KANNADA LETTER PA..KANNADA LETTER LLA
- {0x0CB5, 0x0CB9, prN}, // Lo [5] KANNADA LETTER VA..KANNADA LETTER HA
- {0x0CBC, 0x0CBC, prN}, // Mn KANNADA SIGN NUKTA
- {0x0CBD, 0x0CBD, prN}, // Lo KANNADA SIGN AVAGRAHA
- {0x0CBE, 0x0CBE, prN}, // Mc KANNADA VOWEL SIGN AA
- {0x0CBF, 0x0CBF, prN}, // Mn KANNADA VOWEL SIGN I
- {0x0CC0, 0x0CC4, prN}, // Mc [5] KANNADA VOWEL SIGN II..KANNADA VOWEL SIGN VOCALIC RR
- {0x0CC6, 0x0CC6, prN}, // Mn KANNADA VOWEL SIGN E
- {0x0CC7, 0x0CC8, prN}, // Mc [2] KANNADA VOWEL SIGN EE..KANNADA VOWEL SIGN AI
- {0x0CCA, 0x0CCB, prN}, // Mc [2] KANNADA VOWEL SIGN O..KANNADA VOWEL SIGN OO
- {0x0CCC, 0x0CCD, prN}, // Mn [2] KANNADA VOWEL SIGN AU..KANNADA SIGN VIRAMA
- {0x0CD5, 0x0CD6, prN}, // Mc [2] KANNADA LENGTH MARK..KANNADA AI LENGTH MARK
- {0x0CDD, 0x0CDE, prN}, // Lo [2] KANNADA LETTER NAKAARA POLLU..KANNADA LETTER FA
- {0x0CE0, 0x0CE1, prN}, // Lo [2] KANNADA LETTER VOCALIC RR..KANNADA LETTER VOCALIC LL
- {0x0CE2, 0x0CE3, prN}, // Mn [2] KANNADA VOWEL SIGN VOCALIC L..KANNADA VOWEL SIGN VOCALIC LL
- {0x0CE6, 0x0CEF, prN}, // Nd [10] KANNADA DIGIT ZERO..KANNADA DIGIT NINE
- {0x0CF1, 0x0CF2, prN}, // Lo [2] KANNADA SIGN JIHVAMULIYA..KANNADA SIGN UPADHMANIYA
- {0x0D00, 0x0D01, prN}, // Mn [2] MALAYALAM SIGN COMBINING ANUSVARA ABOVE..MALAYALAM SIGN CANDRABINDU
- {0x0D02, 0x0D03, prN}, // Mc [2] MALAYALAM SIGN ANUSVARA..MALAYALAM SIGN VISARGA
- {0x0D04, 0x0D0C, prN}, // Lo [9] MALAYALAM LETTER VEDIC ANUSVARA..MALAYALAM LETTER VOCALIC L
- {0x0D0E, 0x0D10, prN}, // Lo [3] MALAYALAM LETTER E..MALAYALAM LETTER AI
- {0x0D12, 0x0D3A, prN}, // Lo [41] MALAYALAM LETTER O..MALAYALAM LETTER TTTA
- {0x0D3B, 0x0D3C, prN}, // Mn [2] MALAYALAM SIGN VERTICAL BAR VIRAMA..MALAYALAM SIGN CIRCULAR VIRAMA
- {0x0D3D, 0x0D3D, prN}, // Lo MALAYALAM SIGN AVAGRAHA
- {0x0D3E, 0x0D40, prN}, // Mc [3] MALAYALAM VOWEL SIGN AA..MALAYALAM VOWEL SIGN II
- {0x0D41, 0x0D44, prN}, // Mn [4] MALAYALAM VOWEL SIGN U..MALAYALAM VOWEL SIGN VOCALIC RR
- {0x0D46, 0x0D48, prN}, // Mc [3] MALAYALAM VOWEL SIGN E..MALAYALAM VOWEL SIGN AI
- {0x0D4A, 0x0D4C, prN}, // Mc [3] MALAYALAM VOWEL SIGN O..MALAYALAM VOWEL SIGN AU
- {0x0D4D, 0x0D4D, prN}, // Mn MALAYALAM SIGN VIRAMA
- {0x0D4E, 0x0D4E, prN}, // Lo MALAYALAM LETTER DOT REPH
- {0x0D4F, 0x0D4F, prN}, // So MALAYALAM SIGN PARA
- {0x0D54, 0x0D56, prN}, // Lo [3] MALAYALAM LETTER CHILLU M..MALAYALAM LETTER CHILLU LLL
- {0x0D57, 0x0D57, prN}, // Mc MALAYALAM AU LENGTH MARK
- {0x0D58, 0x0D5E, prN}, // No [7] MALAYALAM FRACTION ONE ONE-HUNDRED-AND-SIXTIETH..MALAYALAM FRACTION ONE FIFTH
- {0x0D5F, 0x0D61, prN}, // Lo [3] MALAYALAM LETTER ARCHAIC II..MALAYALAM LETTER VOCALIC LL
- {0x0D62, 0x0D63, prN}, // Mn [2] MALAYALAM VOWEL SIGN VOCALIC L..MALAYALAM VOWEL SIGN VOCALIC LL
- {0x0D66, 0x0D6F, prN}, // Nd [10] MALAYALAM DIGIT ZERO..MALAYALAM DIGIT NINE
- {0x0D70, 0x0D78, prN}, // No [9] MALAYALAM NUMBER TEN..MALAYALAM FRACTION THREE SIXTEENTHS
- {0x0D79, 0x0D79, prN}, // So MALAYALAM DATE MARK
- {0x0D7A, 0x0D7F, prN}, // Lo [6] MALAYALAM LETTER CHILLU NN..MALAYALAM LETTER CHILLU K
- {0x0D81, 0x0D81, prN}, // Mn SINHALA SIGN CANDRABINDU
- {0x0D82, 0x0D83, prN}, // Mc [2] SINHALA SIGN ANUSVARAYA..SINHALA SIGN VISARGAYA
- {0x0D85, 0x0D96, prN}, // Lo [18] SINHALA LETTER AYANNA..SINHALA LETTER AUYANNA
- {0x0D9A, 0x0DB1, prN}, // Lo [24] SINHALA LETTER ALPAPRAANA KAYANNA..SINHALA LETTER DANTAJA NAYANNA
- {0x0DB3, 0x0DBB, prN}, // Lo [9] SINHALA LETTER SANYAKA DAYANNA..SINHALA LETTER RAYANNA
- {0x0DBD, 0x0DBD, prN}, // Lo SINHALA LETTER DANTAJA LAYANNA
- {0x0DC0, 0x0DC6, prN}, // Lo [7] SINHALA LETTER VAYANNA..SINHALA LETTER FAYANNA
- {0x0DCA, 0x0DCA, prN}, // Mn SINHALA SIGN AL-LAKUNA
- {0x0DCF, 0x0DD1, prN}, // Mc [3] SINHALA VOWEL SIGN AELA-PILLA..SINHALA VOWEL SIGN DIGA AEDA-PILLA
- {0x0DD2, 0x0DD4, prN}, // Mn [3] SINHALA VOWEL SIGN KETTI IS-PILLA..SINHALA VOWEL SIGN KETTI PAA-PILLA
- {0x0DD6, 0x0DD6, prN}, // Mn SINHALA VOWEL SIGN DIGA PAA-PILLA
- {0x0DD8, 0x0DDF, prN}, // Mc [8] SINHALA VOWEL SIGN GAETTA-PILLA..SINHALA VOWEL SIGN GAYANUKITTA
- {0x0DE6, 0x0DEF, prN}, // Nd [10] SINHALA LITH DIGIT ZERO..SINHALA LITH DIGIT NINE
- {0x0DF2, 0x0DF3, prN}, // Mc [2] SINHALA VOWEL SIGN DIGA GAETTA-PILLA..SINHALA VOWEL SIGN DIGA GAYANUKITTA
- {0x0DF4, 0x0DF4, prN}, // Po SINHALA PUNCTUATION KUNDDALIYA
- {0x0E01, 0x0E30, prN}, // Lo [48] THAI CHARACTER KO KAI..THAI CHARACTER SARA A
- {0x0E31, 0x0E31, prN}, // Mn THAI CHARACTER MAI HAN-AKAT
- {0x0E32, 0x0E33, prN}, // Lo [2] THAI CHARACTER SARA AA..THAI CHARACTER SARA AM
- {0x0E34, 0x0E3A, prN}, // Mn [7] THAI CHARACTER SARA I..THAI CHARACTER PHINTHU
- {0x0E3F, 0x0E3F, prN}, // Sc THAI CURRENCY SYMBOL BAHT
- {0x0E40, 0x0E45, prN}, // Lo [6] THAI CHARACTER SARA E..THAI CHARACTER LAKKHANGYAO
- {0x0E46, 0x0E46, prN}, // Lm THAI CHARACTER MAIYAMOK
- {0x0E47, 0x0E4E, prN}, // Mn [8] THAI CHARACTER MAITAIKHU..THAI CHARACTER YAMAKKAN
- {0x0E4F, 0x0E4F, prN}, // Po THAI CHARACTER FONGMAN
- {0x0E50, 0x0E59, prN}, // Nd [10] THAI DIGIT ZERO..THAI DIGIT NINE
- {0x0E5A, 0x0E5B, prN}, // Po [2] THAI CHARACTER ANGKHANKHU..THAI CHARACTER KHOMUT
- {0x0E81, 0x0E82, prN}, // Lo [2] LAO LETTER KO..LAO LETTER KHO SUNG
- {0x0E84, 0x0E84, prN}, // Lo LAO LETTER KHO TAM
- {0x0E86, 0x0E8A, prN}, // Lo [5] LAO LETTER PALI GHA..LAO LETTER SO TAM
- {0x0E8C, 0x0EA3, prN}, // Lo [24] LAO LETTER PALI JHA..LAO LETTER LO LING
- {0x0EA5, 0x0EA5, prN}, // Lo LAO LETTER LO LOOT
- {0x0EA7, 0x0EB0, prN}, // Lo [10] LAO LETTER WO..LAO VOWEL SIGN A
- {0x0EB1, 0x0EB1, prN}, // Mn LAO VOWEL SIGN MAI KAN
- {0x0EB2, 0x0EB3, prN}, // Lo [2] LAO VOWEL SIGN AA..LAO VOWEL SIGN AM
- {0x0EB4, 0x0EBC, prN}, // Mn [9] LAO VOWEL SIGN I..LAO SEMIVOWEL SIGN LO
- {0x0EBD, 0x0EBD, prN}, // Lo LAO SEMIVOWEL SIGN NYO
- {0x0EC0, 0x0EC4, prN}, // Lo [5] LAO VOWEL SIGN E..LAO VOWEL SIGN AI
- {0x0EC6, 0x0EC6, prN}, // Lm LAO KO LA
- {0x0EC8, 0x0ECD, prN}, // Mn [6] LAO TONE MAI EK..LAO NIGGAHITA
- {0x0ED0, 0x0ED9, prN}, // Nd [10] LAO DIGIT ZERO..LAO DIGIT NINE
- {0x0EDC, 0x0EDF, prN}, // Lo [4] LAO HO NO..LAO LETTER KHMU NYO
- {0x0F00, 0x0F00, prN}, // Lo TIBETAN SYLLABLE OM
- {0x0F01, 0x0F03, prN}, // So [3] TIBETAN MARK GTER YIG MGO TRUNCATED A..TIBETAN MARK GTER YIG MGO -UM GTER TSHEG MA
- {0x0F04, 0x0F12, prN}, // Po [15] TIBETAN MARK INITIAL YIG MGO MDUN MA..TIBETAN MARK RGYA GRAM SHAD
- {0x0F13, 0x0F13, prN}, // So TIBETAN MARK CARET -DZUD RTAGS ME LONG CAN
- {0x0F14, 0x0F14, prN}, // Po TIBETAN MARK GTER TSHEG
- {0x0F15, 0x0F17, prN}, // So [3] TIBETAN LOGOTYPE SIGN CHAD RTAGS..TIBETAN ASTROLOGICAL SIGN SGRA GCAN -CHAR RTAGS
- {0x0F18, 0x0F19, prN}, // Mn [2] TIBETAN ASTROLOGICAL SIGN -KHYUD PA..TIBETAN ASTROLOGICAL SIGN SDONG TSHUGS
- {0x0F1A, 0x0F1F, prN}, // So [6] TIBETAN SIGN RDEL DKAR GCIG..TIBETAN SIGN RDEL DKAR RDEL NAG
- {0x0F20, 0x0F29, prN}, // Nd [10] TIBETAN DIGIT ZERO..TIBETAN DIGIT NINE
- {0x0F2A, 0x0F33, prN}, // No [10] TIBETAN DIGIT HALF ONE..TIBETAN DIGIT HALF ZERO
- {0x0F34, 0x0F34, prN}, // So TIBETAN MARK BSDUS RTAGS
- {0x0F35, 0x0F35, prN}, // Mn TIBETAN MARK NGAS BZUNG NYI ZLA
- {0x0F36, 0x0F36, prN}, // So TIBETAN MARK CARET -DZUD RTAGS BZHI MIG CAN
- {0x0F37, 0x0F37, prN}, // Mn TIBETAN MARK NGAS BZUNG SGOR RTAGS
- {0x0F38, 0x0F38, prN}, // So TIBETAN MARK CHE MGO
- {0x0F39, 0x0F39, prN}, // Mn TIBETAN MARK TSA -PHRU
- {0x0F3A, 0x0F3A, prN}, // Ps TIBETAN MARK GUG RTAGS GYON
- {0x0F3B, 0x0F3B, prN}, // Pe TIBETAN MARK GUG RTAGS GYAS
- {0x0F3C, 0x0F3C, prN}, // Ps TIBETAN MARK ANG KHANG GYON
- {0x0F3D, 0x0F3D, prN}, // Pe TIBETAN MARK ANG KHANG GYAS
- {0x0F3E, 0x0F3F, prN}, // Mc [2] TIBETAN SIGN YAR TSHES..TIBETAN SIGN MAR TSHES
- {0x0F40, 0x0F47, prN}, // Lo [8] TIBETAN LETTER KA..TIBETAN LETTER JA
- {0x0F49, 0x0F6C, prN}, // Lo [36] TIBETAN LETTER NYA..TIBETAN LETTER RRA
- {0x0F71, 0x0F7E, prN}, // Mn [14] TIBETAN VOWEL SIGN AA..TIBETAN SIGN RJES SU NGA RO
- {0x0F7F, 0x0F7F, prN}, // Mc TIBETAN SIGN RNAM BCAD
- {0x0F80, 0x0F84, prN}, // Mn [5] TIBETAN VOWEL SIGN REVERSED I..TIBETAN MARK HALANTA
- {0x0F85, 0x0F85, prN}, // Po TIBETAN MARK PALUTA
- {0x0F86, 0x0F87, prN}, // Mn [2] TIBETAN SIGN LCI RTAGS..TIBETAN SIGN YANG RTAGS
- {0x0F88, 0x0F8C, prN}, // Lo [5] TIBETAN SIGN LCE TSA CAN..TIBETAN SIGN INVERTED MCHU CAN
- {0x0F8D, 0x0F97, prN}, // Mn [11] TIBETAN SUBJOINED SIGN LCE TSA CAN..TIBETAN SUBJOINED LETTER JA
- {0x0F99, 0x0FBC, prN}, // Mn [36] TIBETAN SUBJOINED LETTER NYA..TIBETAN SUBJOINED LETTER FIXED-FORM RA
- {0x0FBE, 0x0FC5, prN}, // So [8] TIBETAN KU RU KHA..TIBETAN SYMBOL RDO RJE
- {0x0FC6, 0x0FC6, prN}, // Mn TIBETAN SYMBOL PADMA GDAN
- {0x0FC7, 0x0FCC, prN}, // So [6] TIBETAN SYMBOL RDO RJE RGYA GRAM..TIBETAN SYMBOL NOR BU BZHI -KHYIL
- {0x0FCE, 0x0FCF, prN}, // So [2] TIBETAN SIGN RDEL NAG RDEL DKAR..TIBETAN SIGN RDEL NAG GSUM
- {0x0FD0, 0x0FD4, prN}, // Po [5] TIBETAN MARK BSKA- SHOG GI MGO RGYAN..TIBETAN MARK CLOSING BRDA RNYING YIG MGO SGAB MA
- {0x0FD5, 0x0FD8, prN}, // So [4] RIGHT-FACING SVASTI SIGN..LEFT-FACING SVASTI SIGN WITH DOTS
- {0x0FD9, 0x0FDA, prN}, // Po [2] TIBETAN MARK LEADING MCHAN RTAGS..TIBETAN MARK TRAILING MCHAN RTAGS
- {0x1000, 0x102A, prN}, // Lo [43] MYANMAR LETTER KA..MYANMAR LETTER AU
- {0x102B, 0x102C, prN}, // Mc [2] MYANMAR VOWEL SIGN TALL AA..MYANMAR VOWEL SIGN AA
- {0x102D, 0x1030, prN}, // Mn [4] MYANMAR VOWEL SIGN I..MYANMAR VOWEL SIGN UU
- {0x1031, 0x1031, prN}, // Mc MYANMAR VOWEL SIGN E
- {0x1032, 0x1037, prN}, // Mn [6] MYANMAR VOWEL SIGN AI..MYANMAR SIGN DOT BELOW
- {0x1038, 0x1038, prN}, // Mc MYANMAR SIGN VISARGA
- {0x1039, 0x103A, prN}, // Mn [2] MYANMAR SIGN VIRAMA..MYANMAR SIGN ASAT
- {0x103B, 0x103C, prN}, // Mc [2] MYANMAR CONSONANT SIGN MEDIAL YA..MYANMAR CONSONANT SIGN MEDIAL RA
- {0x103D, 0x103E, prN}, // Mn [2] MYANMAR CONSONANT SIGN MEDIAL WA..MYANMAR CONSONANT SIGN MEDIAL HA
- {0x103F, 0x103F, prN}, // Lo MYANMAR LETTER GREAT SA
- {0x1040, 0x1049, prN}, // Nd [10] MYANMAR DIGIT ZERO..MYANMAR DIGIT NINE
- {0x104A, 0x104F, prN}, // Po [6] MYANMAR SIGN LITTLE SECTION..MYANMAR SYMBOL GENITIVE
- {0x1050, 0x1055, prN}, // Lo [6] MYANMAR LETTER SHA..MYANMAR LETTER VOCALIC LL
- {0x1056, 0x1057, prN}, // Mc [2] MYANMAR VOWEL SIGN VOCALIC R..MYANMAR VOWEL SIGN VOCALIC RR
- {0x1058, 0x1059, prN}, // Mn [2] MYANMAR VOWEL SIGN VOCALIC L..MYANMAR VOWEL SIGN VOCALIC LL
- {0x105A, 0x105D, prN}, // Lo [4] MYANMAR LETTER MON NGA..MYANMAR LETTER MON BBE
- {0x105E, 0x1060, prN}, // Mn [3] MYANMAR CONSONANT SIGN MON MEDIAL NA..MYANMAR CONSONANT SIGN MON MEDIAL LA
- {0x1061, 0x1061, prN}, // Lo MYANMAR LETTER SGAW KAREN SHA
- {0x1062, 0x1064, prN}, // Mc [3] MYANMAR VOWEL SIGN SGAW KAREN EU..MYANMAR TONE MARK SGAW KAREN KE PHO
- {0x1065, 0x1066, prN}, // Lo [2] MYANMAR LETTER WESTERN PWO KAREN THA..MYANMAR LETTER WESTERN PWO KAREN PWA
- {0x1067, 0x106D, prN}, // Mc [7] MYANMAR VOWEL SIGN WESTERN PWO KAREN EU..MYANMAR SIGN WESTERN PWO KAREN TONE-5
- {0x106E, 0x1070, prN}, // Lo [3] MYANMAR LETTER EASTERN PWO KAREN NNA..MYANMAR LETTER EASTERN PWO KAREN GHWA
- {0x1071, 0x1074, prN}, // Mn [4] MYANMAR VOWEL SIGN GEBA KAREN I..MYANMAR VOWEL SIGN KAYAH EE
- {0x1075, 0x1081, prN}, // Lo [13] MYANMAR LETTER SHAN KA..MYANMAR LETTER SHAN HA
- {0x1082, 0x1082, prN}, // Mn MYANMAR CONSONANT SIGN SHAN MEDIAL WA
- {0x1083, 0x1084, prN}, // Mc [2] MYANMAR VOWEL SIGN SHAN AA..MYANMAR VOWEL SIGN SHAN E
- {0x1085, 0x1086, prN}, // Mn [2] MYANMAR VOWEL SIGN SHAN E ABOVE..MYANMAR VOWEL SIGN SHAN FINAL Y
- {0x1087, 0x108C, prN}, // Mc [6] MYANMAR SIGN SHAN TONE-2..MYANMAR SIGN SHAN COUNCIL TONE-3
- {0x108D, 0x108D, prN}, // Mn MYANMAR SIGN SHAN COUNCIL EMPHATIC TONE
- {0x108E, 0x108E, prN}, // Lo MYANMAR LETTER RUMAI PALAUNG FA
- {0x108F, 0x108F, prN}, // Mc MYANMAR SIGN RUMAI PALAUNG TONE-5
- {0x1090, 0x1099, prN}, // Nd [10] MYANMAR SHAN DIGIT ZERO..MYANMAR SHAN DIGIT NINE
- {0x109A, 0x109C, prN}, // Mc [3] MYANMAR SIGN KHAMTI TONE-1..MYANMAR VOWEL SIGN AITON A
- {0x109D, 0x109D, prN}, // Mn MYANMAR VOWEL SIGN AITON AI
- {0x109E, 0x109F, prN}, // So [2] MYANMAR SYMBOL SHAN ONE..MYANMAR SYMBOL SHAN EXCLAMATION
- {0x10A0, 0x10C5, prN}, // Lu [38] GEORGIAN CAPITAL LETTER AN..GEORGIAN CAPITAL LETTER HOE
- {0x10C7, 0x10C7, prN}, // Lu GEORGIAN CAPITAL LETTER YN
- {0x10CD, 0x10CD, prN}, // Lu GEORGIAN CAPITAL LETTER AEN
- {0x10D0, 0x10FA, prN}, // Ll [43] GEORGIAN LETTER AN..GEORGIAN LETTER AIN
- {0x10FB, 0x10FB, prN}, // Po GEORGIAN PARAGRAPH SEPARATOR
- {0x10FC, 0x10FC, prN}, // Lm MODIFIER LETTER GEORGIAN NAR
- {0x10FD, 0x10FF, prN}, // Ll [3] GEORGIAN LETTER AEN..GEORGIAN LETTER LABIAL SIGN
- {0x1100, 0x115F, prW}, // Lo [96] HANGUL CHOSEONG KIYEOK..HANGUL CHOSEONG FILLER
- {0x1160, 0x11FF, prN}, // Lo [160] HANGUL JUNGSEONG FILLER..HANGUL JONGSEONG SSANGNIEUN
- {0x1200, 0x1248, prN}, // Lo [73] ETHIOPIC SYLLABLE HA..ETHIOPIC SYLLABLE QWA
- {0x124A, 0x124D, prN}, // Lo [4] ETHIOPIC SYLLABLE QWI..ETHIOPIC SYLLABLE QWE
- {0x1250, 0x1256, prN}, // Lo [7] ETHIOPIC SYLLABLE QHA..ETHIOPIC SYLLABLE QHO
- {0x1258, 0x1258, prN}, // Lo ETHIOPIC SYLLABLE QHWA
- {0x125A, 0x125D, prN}, // Lo [4] ETHIOPIC SYLLABLE QHWI..ETHIOPIC SYLLABLE QHWE
- {0x1260, 0x1288, prN}, // Lo [41] ETHIOPIC SYLLABLE BA..ETHIOPIC SYLLABLE XWA
- {0x128A, 0x128D, prN}, // Lo [4] ETHIOPIC SYLLABLE XWI..ETHIOPIC SYLLABLE XWE
- {0x1290, 0x12B0, prN}, // Lo [33] ETHIOPIC SYLLABLE NA..ETHIOPIC SYLLABLE KWA
- {0x12B2, 0x12B5, prN}, // Lo [4] ETHIOPIC SYLLABLE KWI..ETHIOPIC SYLLABLE KWE
- {0x12B8, 0x12BE, prN}, // Lo [7] ETHIOPIC SYLLABLE KXA..ETHIOPIC SYLLABLE KXO
- {0x12C0, 0x12C0, prN}, // Lo ETHIOPIC SYLLABLE KXWA
- {0x12C2, 0x12C5, prN}, // Lo [4] ETHIOPIC SYLLABLE KXWI..ETHIOPIC SYLLABLE KXWE
- {0x12C8, 0x12D6, prN}, // Lo [15] ETHIOPIC SYLLABLE WA..ETHIOPIC SYLLABLE PHARYNGEAL O
- {0x12D8, 0x1310, prN}, // Lo [57] ETHIOPIC SYLLABLE ZA..ETHIOPIC SYLLABLE GWA
- {0x1312, 0x1315, prN}, // Lo [4] ETHIOPIC SYLLABLE GWI..ETHIOPIC SYLLABLE GWE
- {0x1318, 0x135A, prN}, // Lo [67] ETHIOPIC SYLLABLE GGA..ETHIOPIC SYLLABLE FYA
- {0x135D, 0x135F, prN}, // Mn [3] ETHIOPIC COMBINING GEMINATION AND VOWEL LENGTH MARK..ETHIOPIC COMBINING GEMINATION MARK
- {0x1360, 0x1368, prN}, // Po [9] ETHIOPIC SECTION MARK..ETHIOPIC PARAGRAPH SEPARATOR
- {0x1369, 0x137C, prN}, // No [20] ETHIOPIC DIGIT ONE..ETHIOPIC NUMBER TEN THOUSAND
- {0x1380, 0x138F, prN}, // Lo [16] ETHIOPIC SYLLABLE SEBATBEIT MWA..ETHIOPIC SYLLABLE PWE
- {0x1390, 0x1399, prN}, // So [10] ETHIOPIC TONAL MARK YIZET..ETHIOPIC TONAL MARK KURT
- {0x13A0, 0x13F5, prN}, // Lu [86] CHEROKEE LETTER A..CHEROKEE LETTER MV
- {0x13F8, 0x13FD, prN}, // Ll [6] CHEROKEE SMALL LETTER YE..CHEROKEE SMALL LETTER MV
- {0x1400, 0x1400, prN}, // Pd CANADIAN SYLLABICS HYPHEN
- {0x1401, 0x166C, prN}, // Lo [620] CANADIAN SYLLABICS E..CANADIAN SYLLABICS CARRIER TTSA
- {0x166D, 0x166D, prN}, // So CANADIAN SYLLABICS CHI SIGN
- {0x166E, 0x166E, prN}, // Po CANADIAN SYLLABICS FULL STOP
- {0x166F, 0x167F, prN}, // Lo [17] CANADIAN SYLLABICS QAI..CANADIAN SYLLABICS BLACKFOOT W
- {0x1680, 0x1680, prN}, // Zs OGHAM SPACE MARK
- {0x1681, 0x169A, prN}, // Lo [26] OGHAM LETTER BEITH..OGHAM LETTER PEITH
- {0x169B, 0x169B, prN}, // Ps OGHAM FEATHER MARK
- {0x169C, 0x169C, prN}, // Pe OGHAM REVERSED FEATHER MARK
- {0x16A0, 0x16EA, prN}, // Lo [75] RUNIC LETTER FEHU FEOH FE F..RUNIC LETTER X
- {0x16EB, 0x16ED, prN}, // Po [3] RUNIC SINGLE PUNCTUATION..RUNIC CROSS PUNCTUATION
- {0x16EE, 0x16F0, prN}, // Nl [3] RUNIC ARLAUG SYMBOL..RUNIC BELGTHOR SYMBOL
- {0x16F1, 0x16F8, prN}, // Lo [8] RUNIC LETTER K..RUNIC LETTER FRANKS CASKET AESC
- {0x1700, 0x1711, prN}, // Lo [18] TAGALOG LETTER A..TAGALOG LETTER HA
- {0x1712, 0x1714, prN}, // Mn [3] TAGALOG VOWEL SIGN I..TAGALOG SIGN VIRAMA
- {0x1715, 0x1715, prN}, // Mc TAGALOG SIGN PAMUDPOD
- {0x171F, 0x171F, prN}, // Lo TAGALOG LETTER ARCHAIC RA
- {0x1720, 0x1731, prN}, // Lo [18] HANUNOO LETTER A..HANUNOO LETTER HA
- {0x1732, 0x1733, prN}, // Mn [2] HANUNOO VOWEL SIGN I..HANUNOO VOWEL SIGN U
- {0x1734, 0x1734, prN}, // Mc HANUNOO SIGN PAMUDPOD
- {0x1735, 0x1736, prN}, // Po [2] PHILIPPINE SINGLE PUNCTUATION..PHILIPPINE DOUBLE PUNCTUATION
- {0x1740, 0x1751, prN}, // Lo [18] BUHID LETTER A..BUHID LETTER HA
- {0x1752, 0x1753, prN}, // Mn [2] BUHID VOWEL SIGN I..BUHID VOWEL SIGN U
- {0x1760, 0x176C, prN}, // Lo [13] TAGBANWA LETTER A..TAGBANWA LETTER YA
- {0x176E, 0x1770, prN}, // Lo [3] TAGBANWA LETTER LA..TAGBANWA LETTER SA
- {0x1772, 0x1773, prN}, // Mn [2] TAGBANWA VOWEL SIGN I..TAGBANWA VOWEL SIGN U
- {0x1780, 0x17B3, prN}, // Lo [52] KHMER LETTER KA..KHMER INDEPENDENT VOWEL QAU
- {0x17B4, 0x17B5, prN}, // Mn [2] KHMER VOWEL INHERENT AQ..KHMER VOWEL INHERENT AA
- {0x17B6, 0x17B6, prN}, // Mc KHMER VOWEL SIGN AA
- {0x17B7, 0x17BD, prN}, // Mn [7] KHMER VOWEL SIGN I..KHMER VOWEL SIGN UA
- {0x17BE, 0x17C5, prN}, // Mc [8] KHMER VOWEL SIGN OE..KHMER VOWEL SIGN AU
- {0x17C6, 0x17C6, prN}, // Mn KHMER SIGN NIKAHIT
- {0x17C7, 0x17C8, prN}, // Mc [2] KHMER SIGN REAHMUK..KHMER SIGN YUUKALEAPINTU
- {0x17C9, 0x17D3, prN}, // Mn [11] KHMER SIGN MUUSIKATOAN..KHMER SIGN BATHAMASAT
- {0x17D4, 0x17D6, prN}, // Po [3] KHMER SIGN KHAN..KHMER SIGN CAMNUC PII KUUH
- {0x17D7, 0x17D7, prN}, // Lm KHMER SIGN LEK TOO
- {0x17D8, 0x17DA, prN}, // Po [3] KHMER SIGN BEYYAL..KHMER SIGN KOOMUUT
- {0x17DB, 0x17DB, prN}, // Sc KHMER CURRENCY SYMBOL RIEL
- {0x17DC, 0x17DC, prN}, // Lo KHMER SIGN AVAKRAHASANYA
- {0x17DD, 0x17DD, prN}, // Mn KHMER SIGN ATTHACAN
- {0x17E0, 0x17E9, prN}, // Nd [10] KHMER DIGIT ZERO..KHMER DIGIT NINE
- {0x17F0, 0x17F9, prN}, // No [10] KHMER SYMBOL LEK ATTAK SON..KHMER SYMBOL LEK ATTAK PRAM-BUON
- {0x1800, 0x1805, prN}, // Po [6] MONGOLIAN BIRGA..MONGOLIAN FOUR DOTS
- {0x1806, 0x1806, prN}, // Pd MONGOLIAN TODO SOFT HYPHEN
- {0x1807, 0x180A, prN}, // Po [4] MONGOLIAN SIBE SYLLABLE BOUNDARY MARKER..MONGOLIAN NIRUGU
- {0x180B, 0x180D, prN}, // Mn [3] MONGOLIAN FREE VARIATION SELECTOR ONE..MONGOLIAN FREE VARIATION SELECTOR THREE
- {0x180E, 0x180E, prN}, // Cf MONGOLIAN VOWEL SEPARATOR
- {0x180F, 0x180F, prN}, // Mn MONGOLIAN FREE VARIATION SELECTOR FOUR
- {0x1810, 0x1819, prN}, // Nd [10] MONGOLIAN DIGIT ZERO..MONGOLIAN DIGIT NINE
- {0x1820, 0x1842, prN}, // Lo [35] MONGOLIAN LETTER A..MONGOLIAN LETTER CHI
- {0x1843, 0x1843, prN}, // Lm MONGOLIAN LETTER TODO LONG VOWEL SIGN
- {0x1844, 0x1878, prN}, // Lo [53] MONGOLIAN LETTER TODO E..MONGOLIAN LETTER CHA WITH TWO DOTS
- {0x1880, 0x1884, prN}, // Lo [5] MONGOLIAN LETTER ALI GALI ANUSVARA ONE..MONGOLIAN LETTER ALI GALI INVERTED UBADAMA
- {0x1885, 0x1886, prN}, // Mn [2] MONGOLIAN LETTER ALI GALI BALUDA..MONGOLIAN LETTER ALI GALI THREE BALUDA
- {0x1887, 0x18A8, prN}, // Lo [34] MONGOLIAN LETTER ALI GALI A..MONGOLIAN LETTER MANCHU ALI GALI BHA
- {0x18A9, 0x18A9, prN}, // Mn MONGOLIAN LETTER ALI GALI DAGALGA
- {0x18AA, 0x18AA, prN}, // Lo MONGOLIAN LETTER MANCHU ALI GALI LHA
- {0x18B0, 0x18F5, prN}, // Lo [70] CANADIAN SYLLABICS OY..CANADIAN SYLLABICS CARRIER DENTAL S
- {0x1900, 0x191E, prN}, // Lo [31] LIMBU VOWEL-CARRIER LETTER..LIMBU LETTER TRA
- {0x1920, 0x1922, prN}, // Mn [3] LIMBU VOWEL SIGN A..LIMBU VOWEL SIGN U
- {0x1923, 0x1926, prN}, // Mc [4] LIMBU VOWEL SIGN EE..LIMBU VOWEL SIGN AU
- {0x1927, 0x1928, prN}, // Mn [2] LIMBU VOWEL SIGN E..LIMBU VOWEL SIGN O
- {0x1929, 0x192B, prN}, // Mc [3] LIMBU SUBJOINED LETTER YA..LIMBU SUBJOINED LETTER WA
- {0x1930, 0x1931, prN}, // Mc [2] LIMBU SMALL LETTER KA..LIMBU SMALL LETTER NGA
- {0x1932, 0x1932, prN}, // Mn LIMBU SMALL LETTER ANUSVARA
- {0x1933, 0x1938, prN}, // Mc [6] LIMBU SMALL LETTER TA..LIMBU SMALL LETTER LA
- {0x1939, 0x193B, prN}, // Mn [3] LIMBU SIGN MUKPHRENG..LIMBU SIGN SA-I
- {0x1940, 0x1940, prN}, // So LIMBU SIGN LOO
- {0x1944, 0x1945, prN}, // Po [2] LIMBU EXCLAMATION MARK..LIMBU QUESTION MARK
- {0x1946, 0x194F, prN}, // Nd [10] LIMBU DIGIT ZERO..LIMBU DIGIT NINE
- {0x1950, 0x196D, prN}, // Lo [30] TAI LE LETTER KA..TAI LE LETTER AI
- {0x1970, 0x1974, prN}, // Lo [5] TAI LE LETTER TONE-2..TAI LE LETTER TONE-6
- {0x1980, 0x19AB, prN}, // Lo [44] NEW TAI LUE LETTER HIGH QA..NEW TAI LUE LETTER LOW SUA
- {0x19B0, 0x19C9, prN}, // Lo [26] NEW TAI LUE VOWEL SIGN VOWEL SHORTENER..NEW TAI LUE TONE MARK-2
- {0x19D0, 0x19D9, prN}, // Nd [10] NEW TAI LUE DIGIT ZERO..NEW TAI LUE DIGIT NINE
- {0x19DA, 0x19DA, prN}, // No NEW TAI LUE THAM DIGIT ONE
- {0x19DE, 0x19DF, prN}, // So [2] NEW TAI LUE SIGN LAE..NEW TAI LUE SIGN LAEV
- {0x19E0, 0x19FF, prN}, // So [32] KHMER SYMBOL PATHAMASAT..KHMER SYMBOL DAP-PRAM ROC
- {0x1A00, 0x1A16, prN}, // Lo [23] BUGINESE LETTER KA..BUGINESE LETTER HA
- {0x1A17, 0x1A18, prN}, // Mn [2] BUGINESE VOWEL SIGN I..BUGINESE VOWEL SIGN U
- {0x1A19, 0x1A1A, prN}, // Mc [2] BUGINESE VOWEL SIGN E..BUGINESE VOWEL SIGN O
- {0x1A1B, 0x1A1B, prN}, // Mn BUGINESE VOWEL SIGN AE
- {0x1A1E, 0x1A1F, prN}, // Po [2] BUGINESE PALLAWA..BUGINESE END OF SECTION
- {0x1A20, 0x1A54, prN}, // Lo [53] TAI THAM LETTER HIGH KA..TAI THAM LETTER GREAT SA
- {0x1A55, 0x1A55, prN}, // Mc TAI THAM CONSONANT SIGN MEDIAL RA
- {0x1A56, 0x1A56, prN}, // Mn TAI THAM CONSONANT SIGN MEDIAL LA
- {0x1A57, 0x1A57, prN}, // Mc TAI THAM CONSONANT SIGN LA TANG LAI
- {0x1A58, 0x1A5E, prN}, // Mn [7] TAI THAM SIGN MAI KANG LAI..TAI THAM CONSONANT SIGN SA
- {0x1A60, 0x1A60, prN}, // Mn TAI THAM SIGN SAKOT
- {0x1A61, 0x1A61, prN}, // Mc TAI THAM VOWEL SIGN A
- {0x1A62, 0x1A62, prN}, // Mn TAI THAM VOWEL SIGN MAI SAT
- {0x1A63, 0x1A64, prN}, // Mc [2] TAI THAM VOWEL SIGN AA..TAI THAM VOWEL SIGN TALL AA
- {0x1A65, 0x1A6C, prN}, // Mn [8] TAI THAM VOWEL SIGN I..TAI THAM VOWEL SIGN OA BELOW
- {0x1A6D, 0x1A72, prN}, // Mc [6] TAI THAM VOWEL SIGN OY..TAI THAM VOWEL SIGN THAM AI
- {0x1A73, 0x1A7C, prN}, // Mn [10] TAI THAM VOWEL SIGN OA ABOVE..TAI THAM SIGN KHUEN-LUE KARAN
- {0x1A7F, 0x1A7F, prN}, // Mn TAI THAM COMBINING CRYPTOGRAMMIC DOT
- {0x1A80, 0x1A89, prN}, // Nd [10] TAI THAM HORA DIGIT ZERO..TAI THAM HORA DIGIT NINE
- {0x1A90, 0x1A99, prN}, // Nd [10] TAI THAM THAM DIGIT ZERO..TAI THAM THAM DIGIT NINE
- {0x1AA0, 0x1AA6, prN}, // Po [7] TAI THAM SIGN WIANG..TAI THAM SIGN REVERSED ROTATED RANA
- {0x1AA7, 0x1AA7, prN}, // Lm TAI THAM SIGN MAI YAMOK
- {0x1AA8, 0x1AAD, prN}, // Po [6] TAI THAM SIGN KAAN..TAI THAM SIGN CAANG
- {0x1AB0, 0x1ABD, prN}, // Mn [14] COMBINING DOUBLED CIRCUMFLEX ACCENT..COMBINING PARENTHESES BELOW
- {0x1ABE, 0x1ABE, prN}, // Me COMBINING PARENTHESES OVERLAY
- {0x1ABF, 0x1ACE, prN}, // Mn [16] COMBINING LATIN SMALL LETTER W BELOW..COMBINING LATIN SMALL LETTER INSULAR T
- {0x1B00, 0x1B03, prN}, // Mn [4] BALINESE SIGN ULU RICEM..BALINESE SIGN SURANG
- {0x1B04, 0x1B04, prN}, // Mc BALINESE SIGN BISAH
- {0x1B05, 0x1B33, prN}, // Lo [47] BALINESE LETTER AKARA..BALINESE LETTER HA
- {0x1B34, 0x1B34, prN}, // Mn BALINESE SIGN REREKAN
- {0x1B35, 0x1B35, prN}, // Mc BALINESE VOWEL SIGN TEDUNG
- {0x1B36, 0x1B3A, prN}, // Mn [5] BALINESE VOWEL SIGN ULU..BALINESE VOWEL SIGN RA REPA
- {0x1B3B, 0x1B3B, prN}, // Mc BALINESE VOWEL SIGN RA REPA TEDUNG
- {0x1B3C, 0x1B3C, prN}, // Mn BALINESE VOWEL SIGN LA LENGA
- {0x1B3D, 0x1B41, prN}, // Mc [5] BALINESE VOWEL SIGN LA LENGA TEDUNG..BALINESE VOWEL SIGN TALING REPA TEDUNG
- {0x1B42, 0x1B42, prN}, // Mn BALINESE VOWEL SIGN PEPET
- {0x1B43, 0x1B44, prN}, // Mc [2] BALINESE VOWEL SIGN PEPET TEDUNG..BALINESE ADEG ADEG
- {0x1B45, 0x1B4C, prN}, // Lo [8] BALINESE LETTER KAF SASAK..BALINESE LETTER ARCHAIC JNYA
- {0x1B50, 0x1B59, prN}, // Nd [10] BALINESE DIGIT ZERO..BALINESE DIGIT NINE
- {0x1B5A, 0x1B60, prN}, // Po [7] BALINESE PANTI..BALINESE PAMENENG
- {0x1B61, 0x1B6A, prN}, // So [10] BALINESE MUSICAL SYMBOL DONG..BALINESE MUSICAL SYMBOL DANG GEDE
- {0x1B6B, 0x1B73, prN}, // Mn [9] BALINESE MUSICAL SYMBOL COMBINING TEGEH..BALINESE MUSICAL SYMBOL COMBINING GONG
- {0x1B74, 0x1B7C, prN}, // So [9] BALINESE MUSICAL SYMBOL RIGHT-HAND OPEN DUG..BALINESE MUSICAL SYMBOL LEFT-HAND OPEN PING
- {0x1B7D, 0x1B7E, prN}, // Po [2] BALINESE PANTI LANTANG..BALINESE PAMADA LANTANG
- {0x1B80, 0x1B81, prN}, // Mn [2] SUNDANESE SIGN PANYECEK..SUNDANESE SIGN PANGLAYAR
- {0x1B82, 0x1B82, prN}, // Mc SUNDANESE SIGN PANGWISAD
- {0x1B83, 0x1BA0, prN}, // Lo [30] SUNDANESE LETTER A..SUNDANESE LETTER HA
- {0x1BA1, 0x1BA1, prN}, // Mc SUNDANESE CONSONANT SIGN PAMINGKAL
- {0x1BA2, 0x1BA5, prN}, // Mn [4] SUNDANESE CONSONANT SIGN PANYAKRA..SUNDANESE VOWEL SIGN PANYUKU
- {0x1BA6, 0x1BA7, prN}, // Mc [2] SUNDANESE VOWEL SIGN PANAELAENG..SUNDANESE VOWEL SIGN PANOLONG
- {0x1BA8, 0x1BA9, prN}, // Mn [2] SUNDANESE VOWEL SIGN PAMEPET..SUNDANESE VOWEL SIGN PANEULEUNG
- {0x1BAA, 0x1BAA, prN}, // Mc SUNDANESE SIGN PAMAAEH
- {0x1BAB, 0x1BAD, prN}, // Mn [3] SUNDANESE SIGN VIRAMA..SUNDANESE CONSONANT SIGN PASANGAN WA
- {0x1BAE, 0x1BAF, prN}, // Lo [2] SUNDANESE LETTER KHA..SUNDANESE LETTER SYA
- {0x1BB0, 0x1BB9, prN}, // Nd [10] SUNDANESE DIGIT ZERO..SUNDANESE DIGIT NINE
- {0x1BBA, 0x1BBF, prN}, // Lo [6] SUNDANESE AVAGRAHA..SUNDANESE LETTER FINAL M
- {0x1BC0, 0x1BE5, prN}, // Lo [38] BATAK LETTER A..BATAK LETTER U
- {0x1BE6, 0x1BE6, prN}, // Mn BATAK SIGN TOMPI
- {0x1BE7, 0x1BE7, prN}, // Mc BATAK VOWEL SIGN E
- {0x1BE8, 0x1BE9, prN}, // Mn [2] BATAK VOWEL SIGN PAKPAK E..BATAK VOWEL SIGN EE
- {0x1BEA, 0x1BEC, prN}, // Mc [3] BATAK VOWEL SIGN I..BATAK VOWEL SIGN O
- {0x1BED, 0x1BED, prN}, // Mn BATAK VOWEL SIGN KARO O
- {0x1BEE, 0x1BEE, prN}, // Mc BATAK VOWEL SIGN U
- {0x1BEF, 0x1BF1, prN}, // Mn [3] BATAK VOWEL SIGN U FOR SIMALUNGUN SA..BATAK CONSONANT SIGN H
- {0x1BF2, 0x1BF3, prN}, // Mc [2] BATAK PANGOLAT..BATAK PANONGONAN
- {0x1BFC, 0x1BFF, prN}, // Po [4] BATAK SYMBOL BINDU NA METEK..BATAK SYMBOL BINDU PANGOLAT
- {0x1C00, 0x1C23, prN}, // Lo [36] LEPCHA LETTER KA..LEPCHA LETTER A
- {0x1C24, 0x1C2B, prN}, // Mc [8] LEPCHA SUBJOINED LETTER YA..LEPCHA VOWEL SIGN UU
- {0x1C2C, 0x1C33, prN}, // Mn [8] LEPCHA VOWEL SIGN E..LEPCHA CONSONANT SIGN T
- {0x1C34, 0x1C35, prN}, // Mc [2] LEPCHA CONSONANT SIGN NYIN-DO..LEPCHA CONSONANT SIGN KANG
- {0x1C36, 0x1C37, prN}, // Mn [2] LEPCHA SIGN RAN..LEPCHA SIGN NUKTA
- {0x1C3B, 0x1C3F, prN}, // Po [5] LEPCHA PUNCTUATION TA-ROL..LEPCHA PUNCTUATION TSHOOK
- {0x1C40, 0x1C49, prN}, // Nd [10] LEPCHA DIGIT ZERO..LEPCHA DIGIT NINE
- {0x1C4D, 0x1C4F, prN}, // Lo [3] LEPCHA LETTER TTA..LEPCHA LETTER DDA
- {0x1C50, 0x1C59, prN}, // Nd [10] OL CHIKI DIGIT ZERO..OL CHIKI DIGIT NINE
- {0x1C5A, 0x1C77, prN}, // Lo [30] OL CHIKI LETTER LA..OL CHIKI LETTER OH
- {0x1C78, 0x1C7D, prN}, // Lm [6] OL CHIKI MU TTUDDAG..OL CHIKI AHAD
- {0x1C7E, 0x1C7F, prN}, // Po [2] OL CHIKI PUNCTUATION MUCAAD..OL CHIKI PUNCTUATION DOUBLE MUCAAD
- {0x1C80, 0x1C88, prN}, // Ll [9] CYRILLIC SMALL LETTER ROUNDED VE..CYRILLIC SMALL LETTER UNBLENDED UK
- {0x1C90, 0x1CBA, prN}, // Lu [43] GEORGIAN MTAVRULI CAPITAL LETTER AN..GEORGIAN MTAVRULI CAPITAL LETTER AIN
- {0x1CBD, 0x1CBF, prN}, // Lu [3] GEORGIAN MTAVRULI CAPITAL LETTER AEN..GEORGIAN MTAVRULI CAPITAL LETTER LABIAL SIGN
- {0x1CC0, 0x1CC7, prN}, // Po [8] SUNDANESE PUNCTUATION BINDU SURYA..SUNDANESE PUNCTUATION BINDU BA SATANGA
- {0x1CD0, 0x1CD2, prN}, // Mn [3] VEDIC TONE KARSHANA..VEDIC TONE PRENKHA
- {0x1CD3, 0x1CD3, prN}, // Po VEDIC SIGN NIHSHVASA
- {0x1CD4, 0x1CE0, prN}, // Mn [13] VEDIC SIGN YAJURVEDIC MIDLINE SVARITA..VEDIC TONE RIGVEDIC KASHMIRI INDEPENDENT SVARITA
- {0x1CE1, 0x1CE1, prN}, // Mc VEDIC TONE ATHARVAVEDIC INDEPENDENT SVARITA
- {0x1CE2, 0x1CE8, prN}, // Mn [7] VEDIC SIGN VISARGA SVARITA..VEDIC SIGN VISARGA ANUDATTA WITH TAIL
- {0x1CE9, 0x1CEC, prN}, // Lo [4] VEDIC SIGN ANUSVARA ANTARGOMUKHA..VEDIC SIGN ANUSVARA VAMAGOMUKHA WITH TAIL
- {0x1CED, 0x1CED, prN}, // Mn VEDIC SIGN TIRYAK
- {0x1CEE, 0x1CF3, prN}, // Lo [6] VEDIC SIGN HEXIFORM LONG ANUSVARA..VEDIC SIGN ROTATED ARDHAVISARGA
- {0x1CF4, 0x1CF4, prN}, // Mn VEDIC TONE CANDRA ABOVE
- {0x1CF5, 0x1CF6, prN}, // Lo [2] VEDIC SIGN JIHVAMULIYA..VEDIC SIGN UPADHMANIYA
- {0x1CF7, 0x1CF7, prN}, // Mc VEDIC SIGN ATIKRAMA
- {0x1CF8, 0x1CF9, prN}, // Mn [2] VEDIC TONE RING ABOVE..VEDIC TONE DOUBLE RING ABOVE
- {0x1CFA, 0x1CFA, prN}, // Lo VEDIC SIGN DOUBLE ANUSVARA ANTARGOMUKHA
- {0x1D00, 0x1D2B, prN}, // Ll [44] LATIN LETTER SMALL CAPITAL A..CYRILLIC LETTER SMALL CAPITAL EL
- {0x1D2C, 0x1D6A, prN}, // Lm [63] MODIFIER LETTER CAPITAL A..GREEK SUBSCRIPT SMALL LETTER CHI
- {0x1D6B, 0x1D77, prN}, // Ll [13] LATIN SMALL LETTER UE..LATIN SMALL LETTER TURNED G
- {0x1D78, 0x1D78, prN}, // Lm MODIFIER LETTER CYRILLIC EN
- {0x1D79, 0x1D7F, prN}, // Ll [7] LATIN SMALL LETTER INSULAR G..LATIN SMALL LETTER UPSILON WITH STROKE
- {0x1D80, 0x1D9A, prN}, // Ll [27] LATIN SMALL LETTER B WITH PALATAL HOOK..LATIN SMALL LETTER EZH WITH RETROFLEX HOOK
- {0x1D9B, 0x1DBF, prN}, // Lm [37] MODIFIER LETTER SMALL TURNED ALPHA..MODIFIER LETTER SMALL THETA
- {0x1DC0, 0x1DFF, prN}, // Mn [64] COMBINING DOTTED GRAVE ACCENT..COMBINING RIGHT ARROWHEAD AND DOWN ARROWHEAD BELOW
- {0x1E00, 0x1EFF, prN}, // L& [256] LATIN CAPITAL LETTER A WITH RING BELOW..LATIN SMALL LETTER Y WITH LOOP
- {0x1F00, 0x1F15, prN}, // L& [22] GREEK SMALL LETTER ALPHA WITH PSILI..GREEK SMALL LETTER EPSILON WITH DASIA AND OXIA
- {0x1F18, 0x1F1D, prN}, // Lu [6] GREEK CAPITAL LETTER EPSILON WITH PSILI..GREEK CAPITAL LETTER EPSILON WITH DASIA AND OXIA
- {0x1F20, 0x1F45, prN}, // L& [38] GREEK SMALL LETTER ETA WITH PSILI..GREEK SMALL LETTER OMICRON WITH DASIA AND OXIA
- {0x1F48, 0x1F4D, prN}, // Lu [6] GREEK CAPITAL LETTER OMICRON WITH PSILI..GREEK CAPITAL LETTER OMICRON WITH DASIA AND OXIA
- {0x1F50, 0x1F57, prN}, // Ll [8] GREEK SMALL LETTER UPSILON WITH PSILI..GREEK SMALL LETTER UPSILON WITH DASIA AND PERISPOMENI
- {0x1F59, 0x1F59, prN}, // Lu GREEK CAPITAL LETTER UPSILON WITH DASIA
- {0x1F5B, 0x1F5B, prN}, // Lu GREEK CAPITAL LETTER UPSILON WITH DASIA AND VARIA
- {0x1F5D, 0x1F5D, prN}, // Lu GREEK CAPITAL LETTER UPSILON WITH DASIA AND OXIA
- {0x1F5F, 0x1F7D, prN}, // L& [31] GREEK CAPITAL LETTER UPSILON WITH DASIA AND PERISPOMENI..GREEK SMALL LETTER OMEGA WITH OXIA
- {0x1F80, 0x1FB4, prN}, // L& [53] GREEK SMALL LETTER ALPHA WITH PSILI AND YPOGEGRAMMENI..GREEK SMALL LETTER ALPHA WITH OXIA AND YPOGEGRAMMENI
- {0x1FB6, 0x1FBC, prN}, // L& [7] GREEK SMALL LETTER ALPHA WITH PERISPOMENI..GREEK CAPITAL LETTER ALPHA WITH PROSGEGRAMMENI
- {0x1FBD, 0x1FBD, prN}, // Sk GREEK KORONIS
- {0x1FBE, 0x1FBE, prN}, // Ll GREEK PROSGEGRAMMENI
- {0x1FBF, 0x1FC1, prN}, // Sk [3] GREEK PSILI..GREEK DIALYTIKA AND PERISPOMENI
- {0x1FC2, 0x1FC4, prN}, // Ll [3] GREEK SMALL LETTER ETA WITH VARIA AND YPOGEGRAMMENI..GREEK SMALL LETTER ETA WITH OXIA AND YPOGEGRAMMENI
- {0x1FC6, 0x1FCC, prN}, // L& [7] GREEK SMALL LETTER ETA WITH PERISPOMENI..GREEK CAPITAL LETTER ETA WITH PROSGEGRAMMENI
- {0x1FCD, 0x1FCF, prN}, // Sk [3] GREEK PSILI AND VARIA..GREEK PSILI AND PERISPOMENI
- {0x1FD0, 0x1FD3, prN}, // Ll [4] GREEK SMALL LETTER IOTA WITH VRACHY..GREEK SMALL LETTER IOTA WITH DIALYTIKA AND OXIA
- {0x1FD6, 0x1FDB, prN}, // L& [6] GREEK SMALL LETTER IOTA WITH PERISPOMENI..GREEK CAPITAL LETTER IOTA WITH OXIA
- {0x1FDD, 0x1FDF, prN}, // Sk [3] GREEK DASIA AND VARIA..GREEK DASIA AND PERISPOMENI
- {0x1FE0, 0x1FEC, prN}, // L& [13] GREEK SMALL LETTER UPSILON WITH VRACHY..GREEK CAPITAL LETTER RHO WITH DASIA
- {0x1FED, 0x1FEF, prN}, // Sk [3] GREEK DIALYTIKA AND VARIA..GREEK VARIA
- {0x1FF2, 0x1FF4, prN}, // Ll [3] GREEK SMALL LETTER OMEGA WITH VARIA AND YPOGEGRAMMENI..GREEK SMALL LETTER OMEGA WITH OXIA AND YPOGEGRAMMENI
- {0x1FF6, 0x1FFC, prN}, // L& [7] GREEK SMALL LETTER OMEGA WITH PERISPOMENI..GREEK CAPITAL LETTER OMEGA WITH PROSGEGRAMMENI
- {0x1FFD, 0x1FFE, prN}, // Sk [2] GREEK OXIA..GREEK DASIA
- {0x2000, 0x200A, prN}, // Zs [11] EN QUAD..HAIR SPACE
- {0x200B, 0x200F, prN}, // Cf [5] ZERO WIDTH SPACE..RIGHT-TO-LEFT MARK
- {0x2010, 0x2010, prA}, // Pd HYPHEN
- {0x2011, 0x2012, prN}, // Pd [2] NON-BREAKING HYPHEN..FIGURE DASH
- {0x2013, 0x2015, prA}, // Pd [3] EN DASH..HORIZONTAL BAR
- {0x2016, 0x2016, prA}, // Po DOUBLE VERTICAL LINE
- {0x2017, 0x2017, prN}, // Po DOUBLE LOW LINE
- {0x2018, 0x2018, prA}, // Pi LEFT SINGLE QUOTATION MARK
- {0x2019, 0x2019, prA}, // Pf RIGHT SINGLE QUOTATION MARK
- {0x201A, 0x201A, prN}, // Ps SINGLE LOW-9 QUOTATION MARK
- {0x201B, 0x201B, prN}, // Pi SINGLE HIGH-REVERSED-9 QUOTATION MARK
- {0x201C, 0x201C, prA}, // Pi LEFT DOUBLE QUOTATION MARK
- {0x201D, 0x201D, prA}, // Pf RIGHT DOUBLE QUOTATION MARK
- {0x201E, 0x201E, prN}, // Ps DOUBLE LOW-9 QUOTATION MARK
- {0x201F, 0x201F, prN}, // Pi DOUBLE HIGH-REVERSED-9 QUOTATION MARK
- {0x2020, 0x2022, prA}, // Po [3] DAGGER..BULLET
- {0x2023, 0x2023, prN}, // Po TRIANGULAR BULLET
- {0x2024, 0x2027, prA}, // Po [4] ONE DOT LEADER..HYPHENATION POINT
- {0x2028, 0x2028, prN}, // Zl LINE SEPARATOR
- {0x2029, 0x2029, prN}, // Zp PARAGRAPH SEPARATOR
- {0x202A, 0x202E, prN}, // Cf [5] LEFT-TO-RIGHT EMBEDDING..RIGHT-TO-LEFT OVERRIDE
- {0x202F, 0x202F, prN}, // Zs NARROW NO-BREAK SPACE
- {0x2030, 0x2030, prA}, // Po PER MILLE SIGN
- {0x2031, 0x2031, prN}, // Po PER TEN THOUSAND SIGN
- {0x2032, 0x2033, prA}, // Po [2] PRIME..DOUBLE PRIME
- {0x2034, 0x2034, prN}, // Po TRIPLE PRIME
- {0x2035, 0x2035, prA}, // Po REVERSED PRIME
- {0x2036, 0x2038, prN}, // Po [3] REVERSED DOUBLE PRIME..CARET
- {0x2039, 0x2039, prN}, // Pi SINGLE LEFT-POINTING ANGLE QUOTATION MARK
- {0x203A, 0x203A, prN}, // Pf SINGLE RIGHT-POINTING ANGLE QUOTATION MARK
- {0x203B, 0x203B, prA}, // Po REFERENCE MARK
- {0x203C, 0x203D, prN}, // Po [2] DOUBLE EXCLAMATION MARK..INTERROBANG
- {0x203E, 0x203E, prA}, // Po OVERLINE
- {0x203F, 0x2040, prN}, // Pc [2] UNDERTIE..CHARACTER TIE
- {0x2041, 0x2043, prN}, // Po [3] CARET INSERTION POINT..HYPHEN BULLET
- {0x2044, 0x2044, prN}, // Sm FRACTION SLASH
- {0x2045, 0x2045, prN}, // Ps LEFT SQUARE BRACKET WITH QUILL
- {0x2046, 0x2046, prN}, // Pe RIGHT SQUARE BRACKET WITH QUILL
- {0x2047, 0x2051, prN}, // Po [11] DOUBLE QUESTION MARK..TWO ASTERISKS ALIGNED VERTICALLY
- {0x2052, 0x2052, prN}, // Sm COMMERCIAL MINUS SIGN
- {0x2053, 0x2053, prN}, // Po SWUNG DASH
- {0x2054, 0x2054, prN}, // Pc INVERTED UNDERTIE
- {0x2055, 0x205E, prN}, // Po [10] FLOWER PUNCTUATION MARK..VERTICAL FOUR DOTS
- {0x205F, 0x205F, prN}, // Zs MEDIUM MATHEMATICAL SPACE
- {0x2060, 0x2064, prN}, // Cf [5] WORD JOINER..INVISIBLE PLUS
- {0x2066, 0x206F, prN}, // Cf [10] LEFT-TO-RIGHT ISOLATE..NOMINAL DIGIT SHAPES
- {0x2070, 0x2070, prN}, // No SUPERSCRIPT ZERO
- {0x2071, 0x2071, prN}, // Lm SUPERSCRIPT LATIN SMALL LETTER I
- {0x2074, 0x2074, prA}, // No SUPERSCRIPT FOUR
- {0x2075, 0x2079, prN}, // No [5] SUPERSCRIPT FIVE..SUPERSCRIPT NINE
- {0x207A, 0x207C, prN}, // Sm [3] SUPERSCRIPT PLUS SIGN..SUPERSCRIPT EQUALS SIGN
- {0x207D, 0x207D, prN}, // Ps SUPERSCRIPT LEFT PARENTHESIS
- {0x207E, 0x207E, prN}, // Pe SUPERSCRIPT RIGHT PARENTHESIS
- {0x207F, 0x207F, prA}, // Lm SUPERSCRIPT LATIN SMALL LETTER N
- {0x2080, 0x2080, prN}, // No SUBSCRIPT ZERO
- {0x2081, 0x2084, prA}, // No [4] SUBSCRIPT ONE..SUBSCRIPT FOUR
- {0x2085, 0x2089, prN}, // No [5] SUBSCRIPT FIVE..SUBSCRIPT NINE
- {0x208A, 0x208C, prN}, // Sm [3] SUBSCRIPT PLUS SIGN..SUBSCRIPT EQUALS SIGN
- {0x208D, 0x208D, prN}, // Ps SUBSCRIPT LEFT PARENTHESIS
- {0x208E, 0x208E, prN}, // Pe SUBSCRIPT RIGHT PARENTHESIS
- {0x2090, 0x209C, prN}, // Lm [13] LATIN SUBSCRIPT SMALL LETTER A..LATIN SUBSCRIPT SMALL LETTER T
- {0x20A0, 0x20A8, prN}, // Sc [9] EURO-CURRENCY SIGN..RUPEE SIGN
- {0x20A9, 0x20A9, prH}, // Sc WON SIGN
- {0x20AA, 0x20AB, prN}, // Sc [2] NEW SHEQEL SIGN..DONG SIGN
- {0x20AC, 0x20AC, prA}, // Sc EURO SIGN
- {0x20AD, 0x20C0, prN}, // Sc [20] KIP SIGN..SOM SIGN
- {0x20D0, 0x20DC, prN}, // Mn [13] COMBINING LEFT HARPOON ABOVE..COMBINING FOUR DOTS ABOVE
- {0x20DD, 0x20E0, prN}, // Me [4] COMBINING ENCLOSING CIRCLE..COMBINING ENCLOSING CIRCLE BACKSLASH
- {0x20E1, 0x20E1, prN}, // Mn COMBINING LEFT RIGHT ARROW ABOVE
- {0x20E2, 0x20E4, prN}, // Me [3] COMBINING ENCLOSING SCREEN..COMBINING ENCLOSING UPWARD POINTING TRIANGLE
- {0x20E5, 0x20F0, prN}, // Mn [12] COMBINING REVERSE SOLIDUS OVERLAY..COMBINING ASTERISK ABOVE
- {0x2100, 0x2101, prN}, // So [2] ACCOUNT OF..ADDRESSED TO THE SUBJECT
- {0x2102, 0x2102, prN}, // Lu DOUBLE-STRUCK CAPITAL C
- {0x2103, 0x2103, prA}, // So DEGREE CELSIUS
- {0x2104, 0x2104, prN}, // So CENTRE LINE SYMBOL
- {0x2105, 0x2105, prA}, // So CARE OF
- {0x2106, 0x2106, prN}, // So CADA UNA
- {0x2107, 0x2107, prN}, // Lu EULER CONSTANT
- {0x2108, 0x2108, prN}, // So SCRUPLE
- {0x2109, 0x2109, prA}, // So DEGREE FAHRENHEIT
- {0x210A, 0x2112, prN}, // L& [9] SCRIPT SMALL G..SCRIPT CAPITAL L
- {0x2113, 0x2113, prA}, // Ll SCRIPT SMALL L
- {0x2114, 0x2114, prN}, // So L B BAR SYMBOL
- {0x2115, 0x2115, prN}, // Lu DOUBLE-STRUCK CAPITAL N
- {0x2116, 0x2116, prA}, // So NUMERO SIGN
- {0x2117, 0x2117, prN}, // So SOUND RECORDING COPYRIGHT
- {0x2118, 0x2118, prN}, // Sm SCRIPT CAPITAL P
- {0x2119, 0x211D, prN}, // Lu [5] DOUBLE-STRUCK CAPITAL P..DOUBLE-STRUCK CAPITAL R
- {0x211E, 0x2120, prN}, // So [3] PRESCRIPTION TAKE..SERVICE MARK
- {0x2121, 0x2122, prA}, // So [2] TELEPHONE SIGN..TRADE MARK SIGN
- {0x2123, 0x2123, prN}, // So VERSICLE
- {0x2124, 0x2124, prN}, // Lu DOUBLE-STRUCK CAPITAL Z
- {0x2125, 0x2125, prN}, // So OUNCE SIGN
- {0x2126, 0x2126, prA}, // Lu OHM SIGN
- {0x2127, 0x2127, prN}, // So INVERTED OHM SIGN
- {0x2128, 0x2128, prN}, // Lu BLACK-LETTER CAPITAL Z
- {0x2129, 0x2129, prN}, // So TURNED GREEK SMALL LETTER IOTA
- {0x212A, 0x212A, prN}, // Lu KELVIN SIGN
- {0x212B, 0x212B, prA}, // Lu ANGSTROM SIGN
- {0x212C, 0x212D, prN}, // Lu [2] SCRIPT CAPITAL B..BLACK-LETTER CAPITAL C
- {0x212E, 0x212E, prN}, // So ESTIMATED SYMBOL
- {0x212F, 0x2134, prN}, // L& [6] SCRIPT SMALL E..SCRIPT SMALL O
- {0x2135, 0x2138, prN}, // Lo [4] ALEF SYMBOL..DALET SYMBOL
- {0x2139, 0x2139, prN}, // Ll INFORMATION SOURCE
- {0x213A, 0x213B, prN}, // So [2] ROTATED CAPITAL Q..FACSIMILE SIGN
- {0x213C, 0x213F, prN}, // L& [4] DOUBLE-STRUCK SMALL PI..DOUBLE-STRUCK CAPITAL PI
- {0x2140, 0x2144, prN}, // Sm [5] DOUBLE-STRUCK N-ARY SUMMATION..TURNED SANS-SERIF CAPITAL Y
- {0x2145, 0x2149, prN}, // L& [5] DOUBLE-STRUCK ITALIC CAPITAL D..DOUBLE-STRUCK ITALIC SMALL J
- {0x214A, 0x214A, prN}, // So PROPERTY LINE
- {0x214B, 0x214B, prN}, // Sm TURNED AMPERSAND
- {0x214C, 0x214D, prN}, // So [2] PER SIGN..AKTIESELSKAB
- {0x214E, 0x214E, prN}, // Ll TURNED SMALL F
- {0x214F, 0x214F, prN}, // So SYMBOL FOR SAMARITAN SOURCE
- {0x2150, 0x2152, prN}, // No [3] VULGAR FRACTION ONE SEVENTH..VULGAR FRACTION ONE TENTH
- {0x2153, 0x2154, prA}, // No [2] VULGAR FRACTION ONE THIRD..VULGAR FRACTION TWO THIRDS
- {0x2155, 0x215A, prN}, // No [6] VULGAR FRACTION ONE FIFTH..VULGAR FRACTION FIVE SIXTHS
- {0x215B, 0x215E, prA}, // No [4] VULGAR FRACTION ONE EIGHTH..VULGAR FRACTION SEVEN EIGHTHS
- {0x215F, 0x215F, prN}, // No FRACTION NUMERATOR ONE
- {0x2160, 0x216B, prA}, // Nl [12] ROMAN NUMERAL ONE..ROMAN NUMERAL TWELVE
- {0x216C, 0x216F, prN}, // Nl [4] ROMAN NUMERAL FIFTY..ROMAN NUMERAL ONE THOUSAND
- {0x2170, 0x2179, prA}, // Nl [10] SMALL ROMAN NUMERAL ONE..SMALL ROMAN NUMERAL TEN
- {0x217A, 0x2182, prN}, // Nl [9] SMALL ROMAN NUMERAL ELEVEN..ROMAN NUMERAL TEN THOUSAND
- {0x2183, 0x2184, prN}, // L& [2] ROMAN NUMERAL REVERSED ONE HUNDRED..LATIN SMALL LETTER REVERSED C
- {0x2185, 0x2188, prN}, // Nl [4] ROMAN NUMERAL SIX LATE FORM..ROMAN NUMERAL ONE HUNDRED THOUSAND
- {0x2189, 0x2189, prA}, // No VULGAR FRACTION ZERO THIRDS
- {0x218A, 0x218B, prN}, // So [2] TURNED DIGIT TWO..TURNED DIGIT THREE
- {0x2190, 0x2194, prA}, // Sm [5] LEFTWARDS ARROW..LEFT RIGHT ARROW
- {0x2195, 0x2199, prA}, // So [5] UP DOWN ARROW..SOUTH WEST ARROW
- {0x219A, 0x219B, prN}, // Sm [2] LEFTWARDS ARROW WITH STROKE..RIGHTWARDS ARROW WITH STROKE
- {0x219C, 0x219F, prN}, // So [4] LEFTWARDS WAVE ARROW..UPWARDS TWO HEADED ARROW
- {0x21A0, 0x21A0, prN}, // Sm RIGHTWARDS TWO HEADED ARROW
- {0x21A1, 0x21A2, prN}, // So [2] DOWNWARDS TWO HEADED ARROW..LEFTWARDS ARROW WITH TAIL
- {0x21A3, 0x21A3, prN}, // Sm RIGHTWARDS ARROW WITH TAIL
- {0x21A4, 0x21A5, prN}, // So [2] LEFTWARDS ARROW FROM BAR..UPWARDS ARROW FROM BAR
- {0x21A6, 0x21A6, prN}, // Sm RIGHTWARDS ARROW FROM BAR
- {0x21A7, 0x21AD, prN}, // So [7] DOWNWARDS ARROW FROM BAR..LEFT RIGHT WAVE ARROW
- {0x21AE, 0x21AE, prN}, // Sm LEFT RIGHT ARROW WITH STROKE
- {0x21AF, 0x21B7, prN}, // So [9] DOWNWARDS ZIGZAG ARROW..CLOCKWISE TOP SEMICIRCLE ARROW
- {0x21B8, 0x21B9, prA}, // So [2] NORTH WEST ARROW TO LONG BAR..LEFTWARDS ARROW TO BAR OVER RIGHTWARDS ARROW TO BAR
- {0x21BA, 0x21CD, prN}, // So [20] ANTICLOCKWISE OPEN CIRCLE ARROW..LEFTWARDS DOUBLE ARROW WITH STROKE
- {0x21CE, 0x21CF, prN}, // Sm [2] LEFT RIGHT DOUBLE ARROW WITH STROKE..RIGHTWARDS DOUBLE ARROW WITH STROKE
- {0x21D0, 0x21D1, prN}, // So [2] LEFTWARDS DOUBLE ARROW..UPWARDS DOUBLE ARROW
- {0x21D2, 0x21D2, prA}, // Sm RIGHTWARDS DOUBLE ARROW
- {0x21D3, 0x21D3, prN}, // So DOWNWARDS DOUBLE ARROW
- {0x21D4, 0x21D4, prA}, // Sm LEFT RIGHT DOUBLE ARROW
- {0x21D5, 0x21E6, prN}, // So [18] UP DOWN DOUBLE ARROW..LEFTWARDS WHITE ARROW
- {0x21E7, 0x21E7, prA}, // So UPWARDS WHITE ARROW
- {0x21E8, 0x21F3, prN}, // So [12] RIGHTWARDS WHITE ARROW..UP DOWN WHITE ARROW
- {0x21F4, 0x21FF, prN}, // Sm [12] RIGHT ARROW WITH SMALL CIRCLE..LEFT RIGHT OPEN-HEADED ARROW
- {0x2200, 0x2200, prA}, // Sm FOR ALL
- {0x2201, 0x2201, prN}, // Sm COMPLEMENT
- {0x2202, 0x2203, prA}, // Sm [2] PARTIAL DIFFERENTIAL..THERE EXISTS
- {0x2204, 0x2206, prN}, // Sm [3] THERE DOES NOT EXIST..INCREMENT
- {0x2207, 0x2208, prA}, // Sm [2] NABLA..ELEMENT OF
- {0x2209, 0x220A, prN}, // Sm [2] NOT AN ELEMENT OF..SMALL ELEMENT OF
- {0x220B, 0x220B, prA}, // Sm CONTAINS AS MEMBER
- {0x220C, 0x220E, prN}, // Sm [3] DOES NOT CONTAIN AS MEMBER..END OF PROOF
- {0x220F, 0x220F, prA}, // Sm N-ARY PRODUCT
- {0x2210, 0x2210, prN}, // Sm N-ARY COPRODUCT
- {0x2211, 0x2211, prA}, // Sm N-ARY SUMMATION
- {0x2212, 0x2214, prN}, // Sm [3] MINUS SIGN..DOT PLUS
- {0x2215, 0x2215, prA}, // Sm DIVISION SLASH
- {0x2216, 0x2219, prN}, // Sm [4] SET MINUS..BULLET OPERATOR
- {0x221A, 0x221A, prA}, // Sm SQUARE ROOT
- {0x221B, 0x221C, prN}, // Sm [2] CUBE ROOT..FOURTH ROOT
- {0x221D, 0x2220, prA}, // Sm [4] PROPORTIONAL TO..ANGLE
- {0x2221, 0x2222, prN}, // Sm [2] MEASURED ANGLE..SPHERICAL ANGLE
- {0x2223, 0x2223, prA}, // Sm DIVIDES
- {0x2224, 0x2224, prN}, // Sm DOES NOT DIVIDE
- {0x2225, 0x2225, prA}, // Sm PARALLEL TO
- {0x2226, 0x2226, prN}, // Sm NOT PARALLEL TO
- {0x2227, 0x222C, prA}, // Sm [6] LOGICAL AND..DOUBLE INTEGRAL
- {0x222D, 0x222D, prN}, // Sm TRIPLE INTEGRAL
- {0x222E, 0x222E, prA}, // Sm CONTOUR INTEGRAL
- {0x222F, 0x2233, prN}, // Sm [5] SURFACE INTEGRAL..ANTICLOCKWISE CONTOUR INTEGRAL
- {0x2234, 0x2237, prA}, // Sm [4] THEREFORE..PROPORTION
- {0x2238, 0x223B, prN}, // Sm [4] DOT MINUS..HOMOTHETIC
- {0x223C, 0x223D, prA}, // Sm [2] TILDE OPERATOR..REVERSED TILDE
- {0x223E, 0x2247, prN}, // Sm [10] INVERTED LAZY S..NEITHER APPROXIMATELY NOR ACTUALLY EQUAL TO
- {0x2248, 0x2248, prA}, // Sm ALMOST EQUAL TO
- {0x2249, 0x224B, prN}, // Sm [3] NOT ALMOST EQUAL TO..TRIPLE TILDE
- {0x224C, 0x224C, prA}, // Sm ALL EQUAL TO
- {0x224D, 0x2251, prN}, // Sm [5] EQUIVALENT TO..GEOMETRICALLY EQUAL TO
- {0x2252, 0x2252, prA}, // Sm APPROXIMATELY EQUAL TO OR THE IMAGE OF
- {0x2253, 0x225F, prN}, // Sm [13] IMAGE OF OR APPROXIMATELY EQUAL TO..QUESTIONED EQUAL TO
- {0x2260, 0x2261, prA}, // Sm [2] NOT EQUAL TO..IDENTICAL TO
- {0x2262, 0x2263, prN}, // Sm [2] NOT IDENTICAL TO..STRICTLY EQUIVALENT TO
- {0x2264, 0x2267, prA}, // Sm [4] LESS-THAN OR EQUAL TO..GREATER-THAN OVER EQUAL TO
- {0x2268, 0x2269, prN}, // Sm [2] LESS-THAN BUT NOT EQUAL TO..GREATER-THAN BUT NOT EQUAL TO
- {0x226A, 0x226B, prA}, // Sm [2] MUCH LESS-THAN..MUCH GREATER-THAN
- {0x226C, 0x226D, prN}, // Sm [2] BETWEEN..NOT EQUIVALENT TO
- {0x226E, 0x226F, prA}, // Sm [2] NOT LESS-THAN..NOT GREATER-THAN
- {0x2270, 0x2281, prN}, // Sm [18] NEITHER LESS-THAN NOR EQUAL TO..DOES NOT SUCCEED
- {0x2282, 0x2283, prA}, // Sm [2] SUBSET OF..SUPERSET OF
- {0x2284, 0x2285, prN}, // Sm [2] NOT A SUBSET OF..NOT A SUPERSET OF
- {0x2286, 0x2287, prA}, // Sm [2] SUBSET OF OR EQUAL TO..SUPERSET OF OR EQUAL TO
- {0x2288, 0x2294, prN}, // Sm [13] NEITHER A SUBSET OF NOR EQUAL TO..SQUARE CUP
- {0x2295, 0x2295, prA}, // Sm CIRCLED PLUS
- {0x2296, 0x2298, prN}, // Sm [3] CIRCLED MINUS..CIRCLED DIVISION SLASH
- {0x2299, 0x2299, prA}, // Sm CIRCLED DOT OPERATOR
- {0x229A, 0x22A4, prN}, // Sm [11] CIRCLED RING OPERATOR..DOWN TACK
- {0x22A5, 0x22A5, prA}, // Sm UP TACK
- {0x22A6, 0x22BE, prN}, // Sm [25] ASSERTION..RIGHT ANGLE WITH ARC
- {0x22BF, 0x22BF, prA}, // Sm RIGHT TRIANGLE
- {0x22C0, 0x22FF, prN}, // Sm [64] N-ARY LOGICAL AND..Z NOTATION BAG MEMBERSHIP
- {0x2300, 0x2307, prN}, // So [8] DIAMETER SIGN..WAVY LINE
- {0x2308, 0x2308, prN}, // Ps LEFT CEILING
- {0x2309, 0x2309, prN}, // Pe RIGHT CEILING
- {0x230A, 0x230A, prN}, // Ps LEFT FLOOR
- {0x230B, 0x230B, prN}, // Pe RIGHT FLOOR
- {0x230C, 0x2311, prN}, // So [6] BOTTOM RIGHT CROP..SQUARE LOZENGE
- {0x2312, 0x2312, prA}, // So ARC
- {0x2313, 0x2319, prN}, // So [7] SEGMENT..TURNED NOT SIGN
- {0x231A, 0x231B, prW}, // So [2] WATCH..HOURGLASS
- {0x231C, 0x231F, prN}, // So [4] TOP LEFT CORNER..BOTTOM RIGHT CORNER
- {0x2320, 0x2321, prN}, // Sm [2] TOP HALF INTEGRAL..BOTTOM HALF INTEGRAL
- {0x2322, 0x2328, prN}, // So [7] FROWN..KEYBOARD
- {0x2329, 0x2329, prW}, // Ps LEFT-POINTING ANGLE BRACKET
- {0x232A, 0x232A, prW}, // Pe RIGHT-POINTING ANGLE BRACKET
- {0x232B, 0x237B, prN}, // So [81] ERASE TO THE LEFT..NOT CHECK MARK
- {0x237C, 0x237C, prN}, // Sm RIGHT ANGLE WITH DOWNWARDS ZIGZAG ARROW
- {0x237D, 0x239A, prN}, // So [30] SHOULDERED OPEN BOX..CLEAR SCREEN SYMBOL
- {0x239B, 0x23B3, prN}, // Sm [25] LEFT PARENTHESIS UPPER HOOK..SUMMATION BOTTOM
- {0x23B4, 0x23DB, prN}, // So [40] TOP SQUARE BRACKET..FUSE
- {0x23DC, 0x23E1, prN}, // Sm [6] TOP PARENTHESIS..BOTTOM TORTOISE SHELL BRACKET
- {0x23E2, 0x23E8, prN}, // So [7] WHITE TRAPEZIUM..DECIMAL EXPONENT SYMBOL
- {0x23E9, 0x23EC, prW}, // So [4] BLACK RIGHT-POINTING DOUBLE TRIANGLE..BLACK DOWN-POINTING DOUBLE TRIANGLE
- {0x23ED, 0x23EF, prN}, // So [3] BLACK RIGHT-POINTING DOUBLE TRIANGLE WITH VERTICAL BAR..BLACK RIGHT-POINTING TRIANGLE WITH DOUBLE VERTICAL BAR
- {0x23F0, 0x23F0, prW}, // So ALARM CLOCK
- {0x23F1, 0x23F2, prN}, // So [2] STOPWATCH..TIMER CLOCK
- {0x23F3, 0x23F3, prW}, // So HOURGLASS WITH FLOWING SAND
- {0x23F4, 0x23FF, prN}, // So [12] BLACK MEDIUM LEFT-POINTING TRIANGLE..OBSERVER EYE SYMBOL
- {0x2400, 0x2426, prN}, // So [39] SYMBOL FOR NULL..SYMBOL FOR SUBSTITUTE FORM TWO
- {0x2440, 0x244A, prN}, // So [11] OCR HOOK..OCR DOUBLE BACKSLASH
- {0x2460, 0x249B, prA}, // No [60] CIRCLED DIGIT ONE..NUMBER TWENTY FULL STOP
- {0x249C, 0x24E9, prA}, // So [78] PARENTHESIZED LATIN SMALL LETTER A..CIRCLED LATIN SMALL LETTER Z
- {0x24EA, 0x24EA, prN}, // No CIRCLED DIGIT ZERO
- {0x24EB, 0x24FF, prA}, // No [21] NEGATIVE CIRCLED NUMBER ELEVEN..NEGATIVE CIRCLED DIGIT ZERO
- {0x2500, 0x254B, prA}, // So [76] BOX DRAWINGS LIGHT HORIZONTAL..BOX DRAWINGS HEAVY VERTICAL AND HORIZONTAL
- {0x254C, 0x254F, prN}, // So [4] BOX DRAWINGS LIGHT DOUBLE DASH HORIZONTAL..BOX DRAWINGS HEAVY DOUBLE DASH VERTICAL
- {0x2550, 0x2573, prA}, // So [36] BOX DRAWINGS DOUBLE HORIZONTAL..BOX DRAWINGS LIGHT DIAGONAL CROSS
- {0x2574, 0x257F, prN}, // So [12] BOX DRAWINGS LIGHT LEFT..BOX DRAWINGS HEAVY UP AND LIGHT DOWN
- {0x2580, 0x258F, prA}, // So [16] UPPER HALF BLOCK..LEFT ONE EIGHTH BLOCK
- {0x2590, 0x2591, prN}, // So [2] RIGHT HALF BLOCK..LIGHT SHADE
- {0x2592, 0x2595, prA}, // So [4] MEDIUM SHADE..RIGHT ONE EIGHTH BLOCK
- {0x2596, 0x259F, prN}, // So [10] QUADRANT LOWER LEFT..QUADRANT UPPER RIGHT AND LOWER LEFT AND LOWER RIGHT
- {0x25A0, 0x25A1, prA}, // So [2] BLACK SQUARE..WHITE SQUARE
- {0x25A2, 0x25A2, prN}, // So WHITE SQUARE WITH ROUNDED CORNERS
- {0x25A3, 0x25A9, prA}, // So [7] WHITE SQUARE CONTAINING BLACK SMALL SQUARE..SQUARE WITH DIAGONAL CROSSHATCH FILL
- {0x25AA, 0x25B1, prN}, // So [8] BLACK SMALL SQUARE..WHITE PARALLELOGRAM
- {0x25B2, 0x25B3, prA}, // So [2] BLACK UP-POINTING TRIANGLE..WHITE UP-POINTING TRIANGLE
- {0x25B4, 0x25B5, prN}, // So [2] BLACK UP-POINTING SMALL TRIANGLE..WHITE UP-POINTING SMALL TRIANGLE
- {0x25B6, 0x25B6, prA}, // So BLACK RIGHT-POINTING TRIANGLE
- {0x25B7, 0x25B7, prA}, // Sm WHITE RIGHT-POINTING TRIANGLE
- {0x25B8, 0x25BB, prN}, // So [4] BLACK RIGHT-POINTING SMALL TRIANGLE..WHITE RIGHT-POINTING POINTER
- {0x25BC, 0x25BD, prA}, // So [2] BLACK DOWN-POINTING TRIANGLE..WHITE DOWN-POINTING TRIANGLE
- {0x25BE, 0x25BF, prN}, // So [2] BLACK DOWN-POINTING SMALL TRIANGLE..WHITE DOWN-POINTING SMALL TRIANGLE
- {0x25C0, 0x25C0, prA}, // So BLACK LEFT-POINTING TRIANGLE
- {0x25C1, 0x25C1, prA}, // Sm WHITE LEFT-POINTING TRIANGLE
- {0x25C2, 0x25C5, prN}, // So [4] BLACK LEFT-POINTING SMALL TRIANGLE..WHITE LEFT-POINTING POINTER
- {0x25C6, 0x25C8, prA}, // So [3] BLACK DIAMOND..WHITE DIAMOND CONTAINING BLACK SMALL DIAMOND
- {0x25C9, 0x25CA, prN}, // So [2] FISHEYE..LOZENGE
- {0x25CB, 0x25CB, prA}, // So WHITE CIRCLE
- {0x25CC, 0x25CD, prN}, // So [2] DOTTED CIRCLE..CIRCLE WITH VERTICAL FILL
- {0x25CE, 0x25D1, prA}, // So [4] BULLSEYE..CIRCLE WITH RIGHT HALF BLACK
- {0x25D2, 0x25E1, prN}, // So [16] CIRCLE WITH LOWER HALF BLACK..LOWER HALF CIRCLE
- {0x25E2, 0x25E5, prA}, // So [4] BLACK LOWER RIGHT TRIANGLE..BLACK UPPER RIGHT TRIANGLE
- {0x25E6, 0x25EE, prN}, // So [9] WHITE BULLET..UP-POINTING TRIANGLE WITH RIGHT HALF BLACK
- {0x25EF, 0x25EF, prA}, // So LARGE CIRCLE
- {0x25F0, 0x25F7, prN}, // So [8] WHITE SQUARE WITH UPPER LEFT QUADRANT..WHITE CIRCLE WITH UPPER RIGHT QUADRANT
- {0x25F8, 0x25FC, prN}, // Sm [5] UPPER LEFT TRIANGLE..BLACK MEDIUM SQUARE
- {0x25FD, 0x25FE, prW}, // Sm [2] WHITE MEDIUM SMALL SQUARE..BLACK MEDIUM SMALL SQUARE
- {0x25FF, 0x25FF, prN}, // Sm LOWER RIGHT TRIANGLE
- {0x2600, 0x2604, prN}, // So [5] BLACK SUN WITH RAYS..COMET
- {0x2605, 0x2606, prA}, // So [2] BLACK STAR..WHITE STAR
- {0x2607, 0x2608, prN}, // So [2] LIGHTNING..THUNDERSTORM
- {0x2609, 0x2609, prA}, // So SUN
- {0x260A, 0x260D, prN}, // So [4] ASCENDING NODE..OPPOSITION
- {0x260E, 0x260F, prA}, // So [2] BLACK TELEPHONE..WHITE TELEPHONE
- {0x2610, 0x2613, prN}, // So [4] BALLOT BOX..SALTIRE
- {0x2614, 0x2615, prW}, // So [2] UMBRELLA WITH RAIN DROPS..HOT BEVERAGE
- {0x2616, 0x261B, prN}, // So [6] WHITE SHOGI PIECE..BLACK RIGHT POINTING INDEX
- {0x261C, 0x261C, prA}, // So WHITE LEFT POINTING INDEX
- {0x261D, 0x261D, prN}, // So WHITE UP POINTING INDEX
- {0x261E, 0x261E, prA}, // So WHITE RIGHT POINTING INDEX
- {0x261F, 0x263F, prN}, // So [33] WHITE DOWN POINTING INDEX..MERCURY
- {0x2640, 0x2640, prA}, // So FEMALE SIGN
- {0x2641, 0x2641, prN}, // So EARTH
- {0x2642, 0x2642, prA}, // So MALE SIGN
- {0x2643, 0x2647, prN}, // So [5] JUPITER..PLUTO
- {0x2648, 0x2653, prW}, // So [12] ARIES..PISCES
- {0x2654, 0x265F, prN}, // So [12] WHITE CHESS KING..BLACK CHESS PAWN
- {0x2660, 0x2661, prA}, // So [2] BLACK SPADE SUIT..WHITE HEART SUIT
- {0x2662, 0x2662, prN}, // So WHITE DIAMOND SUIT
- {0x2663, 0x2665, prA}, // So [3] BLACK CLUB SUIT..BLACK HEART SUIT
- {0x2666, 0x2666, prN}, // So BLACK DIAMOND SUIT
- {0x2667, 0x266A, prA}, // So [4] WHITE CLUB SUIT..EIGHTH NOTE
- {0x266B, 0x266B, prN}, // So BEAMED EIGHTH NOTES
- {0x266C, 0x266D, prA}, // So [2] BEAMED SIXTEENTH NOTES..MUSIC FLAT SIGN
- {0x266E, 0x266E, prN}, // So MUSIC NATURAL SIGN
- {0x266F, 0x266F, prA}, // Sm MUSIC SHARP SIGN
- {0x2670, 0x267E, prN}, // So [15] WEST SYRIAC CROSS..PERMANENT PAPER SIGN
- {0x267F, 0x267F, prW}, // So WHEELCHAIR SYMBOL
- {0x2680, 0x2692, prN}, // So [19] DIE FACE-1..HAMMER AND PICK
- {0x2693, 0x2693, prW}, // So ANCHOR
- {0x2694, 0x269D, prN}, // So [10] CROSSED SWORDS..OUTLINED WHITE STAR
- {0x269E, 0x269F, prA}, // So [2] THREE LINES CONVERGING RIGHT..THREE LINES CONVERGING LEFT
- {0x26A0, 0x26A0, prN}, // So WARNING SIGN
- {0x26A1, 0x26A1, prW}, // So HIGH VOLTAGE SIGN
- {0x26A2, 0x26A9, prN}, // So [8] DOUBLED FEMALE SIGN..HORIZONTAL MALE WITH STROKE SIGN
- {0x26AA, 0x26AB, prW}, // So [2] MEDIUM WHITE CIRCLE..MEDIUM BLACK CIRCLE
- {0x26AC, 0x26BC, prN}, // So [17] MEDIUM SMALL WHITE CIRCLE..SESQUIQUADRATE
- {0x26BD, 0x26BE, prW}, // So [2] SOCCER BALL..BASEBALL
- {0x26BF, 0x26BF, prA}, // So SQUARED KEY
- {0x26C0, 0x26C3, prN}, // So [4] WHITE DRAUGHTS MAN..BLACK DRAUGHTS KING
- {0x26C4, 0x26C5, prW}, // So [2] SNOWMAN WITHOUT SNOW..SUN BEHIND CLOUD
- {0x26C6, 0x26CD, prA}, // So [8] RAIN..DISABLED CAR
- {0x26CE, 0x26CE, prW}, // So OPHIUCHUS
- {0x26CF, 0x26D3, prA}, // So [5] PICK..CHAINS
- {0x26D4, 0x26D4, prW}, // So NO ENTRY
- {0x26D5, 0x26E1, prA}, // So [13] ALTERNATE ONE-WAY LEFT WAY TRAFFIC..RESTRICTED LEFT ENTRY-2
- {0x26E2, 0x26E2, prN}, // So ASTRONOMICAL SYMBOL FOR URANUS
- {0x26E3, 0x26E3, prA}, // So HEAVY CIRCLE WITH STROKE AND TWO DOTS ABOVE
- {0x26E4, 0x26E7, prN}, // So [4] PENTAGRAM..INVERTED PENTAGRAM
- {0x26E8, 0x26E9, prA}, // So [2] BLACK CROSS ON SHIELD..SHINTO SHRINE
- {0x26EA, 0x26EA, prW}, // So CHURCH
- {0x26EB, 0x26F1, prA}, // So [7] CASTLE..UMBRELLA ON GROUND
- {0x26F2, 0x26F3, prW}, // So [2] FOUNTAIN..FLAG IN HOLE
- {0x26F4, 0x26F4, prA}, // So FERRY
- {0x26F5, 0x26F5, prW}, // So SAILBOAT
- {0x26F6, 0x26F9, prA}, // So [4] SQUARE FOUR CORNERS..PERSON WITH BALL
- {0x26FA, 0x26FA, prW}, // So TENT
- {0x26FB, 0x26FC, prA}, // So [2] JAPANESE BANK SYMBOL..HEADSTONE GRAVEYARD SYMBOL
- {0x26FD, 0x26FD, prW}, // So FUEL PUMP
- {0x26FE, 0x26FF, prA}, // So [2] CUP ON BLACK SQUARE..WHITE FLAG WITH HORIZONTAL MIDDLE BLACK STRIPE
- {0x2700, 0x2704, prN}, // So [5] BLACK SAFETY SCISSORS..WHITE SCISSORS
- {0x2705, 0x2705, prW}, // So WHITE HEAVY CHECK MARK
- {0x2706, 0x2709, prN}, // So [4] TELEPHONE LOCATION SIGN..ENVELOPE
- {0x270A, 0x270B, prW}, // So [2] RAISED FIST..RAISED HAND
- {0x270C, 0x2727, prN}, // So [28] VICTORY HAND..WHITE FOUR POINTED STAR
- {0x2728, 0x2728, prW}, // So SPARKLES
- {0x2729, 0x273C, prN}, // So [20] STRESS OUTLINED WHITE STAR..OPEN CENTRE TEARDROP-SPOKED ASTERISK
- {0x273D, 0x273D, prA}, // So HEAVY TEARDROP-SPOKED ASTERISK
- {0x273E, 0x274B, prN}, // So [14] SIX PETALLED BLACK AND WHITE FLORETTE..HEAVY EIGHT TEARDROP-SPOKED PROPELLER ASTERISK
- {0x274C, 0x274C, prW}, // So CROSS MARK
- {0x274D, 0x274D, prN}, // So SHADOWED WHITE CIRCLE
- {0x274E, 0x274E, prW}, // So NEGATIVE SQUARED CROSS MARK
- {0x274F, 0x2752, prN}, // So [4] LOWER RIGHT DROP-SHADOWED WHITE SQUARE..UPPER RIGHT SHADOWED WHITE SQUARE
- {0x2753, 0x2755, prW}, // So [3] BLACK QUESTION MARK ORNAMENT..WHITE EXCLAMATION MARK ORNAMENT
- {0x2756, 0x2756, prN}, // So BLACK DIAMOND MINUS WHITE X
- {0x2757, 0x2757, prW}, // So HEAVY EXCLAMATION MARK SYMBOL
- {0x2758, 0x2767, prN}, // So [16] LIGHT VERTICAL BAR..ROTATED FLORAL HEART BULLET
- {0x2768, 0x2768, prN}, // Ps MEDIUM LEFT PARENTHESIS ORNAMENT
- {0x2769, 0x2769, prN}, // Pe MEDIUM RIGHT PARENTHESIS ORNAMENT
- {0x276A, 0x276A, prN}, // Ps MEDIUM FLATTENED LEFT PARENTHESIS ORNAMENT
- {0x276B, 0x276B, prN}, // Pe MEDIUM FLATTENED RIGHT PARENTHESIS ORNAMENT
- {0x276C, 0x276C, prN}, // Ps MEDIUM LEFT-POINTING ANGLE BRACKET ORNAMENT
- {0x276D, 0x276D, prN}, // Pe MEDIUM RIGHT-POINTING ANGLE BRACKET ORNAMENT
- {0x276E, 0x276E, prN}, // Ps HEAVY LEFT-POINTING ANGLE QUOTATION MARK ORNAMENT
- {0x276F, 0x276F, prN}, // Pe HEAVY RIGHT-POINTING ANGLE QUOTATION MARK ORNAMENT
- {0x2770, 0x2770, prN}, // Ps HEAVY LEFT-POINTING ANGLE BRACKET ORNAMENT
- {0x2771, 0x2771, prN}, // Pe HEAVY RIGHT-POINTING ANGLE BRACKET ORNAMENT
- {0x2772, 0x2772, prN}, // Ps LIGHT LEFT TORTOISE SHELL BRACKET ORNAMENT
- {0x2773, 0x2773, prN}, // Pe LIGHT RIGHT TORTOISE SHELL BRACKET ORNAMENT
- {0x2774, 0x2774, prN}, // Ps MEDIUM LEFT CURLY BRACKET ORNAMENT
- {0x2775, 0x2775, prN}, // Pe MEDIUM RIGHT CURLY BRACKET ORNAMENT
- {0x2776, 0x277F, prA}, // No [10] DINGBAT NEGATIVE CIRCLED DIGIT ONE..DINGBAT NEGATIVE CIRCLED NUMBER TEN
- {0x2780, 0x2793, prN}, // No [20] DINGBAT CIRCLED SANS-SERIF DIGIT ONE..DINGBAT NEGATIVE CIRCLED SANS-SERIF NUMBER TEN
- {0x2794, 0x2794, prN}, // So HEAVY WIDE-HEADED RIGHTWARDS ARROW
- {0x2795, 0x2797, prW}, // So [3] HEAVY PLUS SIGN..HEAVY DIVISION SIGN
- {0x2798, 0x27AF, prN}, // So [24] HEAVY SOUTH EAST ARROW..NOTCHED LOWER RIGHT-SHADOWED WHITE RIGHTWARDS ARROW
- {0x27B0, 0x27B0, prW}, // So CURLY LOOP
- {0x27B1, 0x27BE, prN}, // So [14] NOTCHED UPPER RIGHT-SHADOWED WHITE RIGHTWARDS ARROW..OPEN-OUTLINED RIGHTWARDS ARROW
- {0x27BF, 0x27BF, prW}, // So DOUBLE CURLY LOOP
- {0x27C0, 0x27C4, prN}, // Sm [5] THREE DIMENSIONAL ANGLE..OPEN SUPERSET
- {0x27C5, 0x27C5, prN}, // Ps LEFT S-SHAPED BAG DELIMITER
- {0x27C6, 0x27C6, prN}, // Pe RIGHT S-SHAPED BAG DELIMITER
- {0x27C7, 0x27E5, prN}, // Sm [31] OR WITH DOT INSIDE..WHITE SQUARE WITH RIGHTWARDS TICK
- {0x27E6, 0x27E6, prNa}, // Ps MATHEMATICAL LEFT WHITE SQUARE BRACKET
- {0x27E7, 0x27E7, prNa}, // Pe MATHEMATICAL RIGHT WHITE SQUARE BRACKET
- {0x27E8, 0x27E8, prNa}, // Ps MATHEMATICAL LEFT ANGLE BRACKET
- {0x27E9, 0x27E9, prNa}, // Pe MATHEMATICAL RIGHT ANGLE BRACKET
- {0x27EA, 0x27EA, prNa}, // Ps MATHEMATICAL LEFT DOUBLE ANGLE BRACKET
- {0x27EB, 0x27EB, prNa}, // Pe MATHEMATICAL RIGHT DOUBLE ANGLE BRACKET
- {0x27EC, 0x27EC, prNa}, // Ps MATHEMATICAL LEFT WHITE TORTOISE SHELL BRACKET
- {0x27ED, 0x27ED, prNa}, // Pe MATHEMATICAL RIGHT WHITE TORTOISE SHELL BRACKET
- {0x27EE, 0x27EE, prN}, // Ps MATHEMATICAL LEFT FLATTENED PARENTHESIS
- {0x27EF, 0x27EF, prN}, // Pe MATHEMATICAL RIGHT FLATTENED PARENTHESIS
- {0x27F0, 0x27FF, prN}, // Sm [16] UPWARDS QUADRUPLE ARROW..LONG RIGHTWARDS SQUIGGLE ARROW
- {0x2800, 0x28FF, prN}, // So [256] BRAILLE PATTERN BLANK..BRAILLE PATTERN DOTS-12345678
- {0x2900, 0x297F, prN}, // Sm [128] RIGHTWARDS TWO-HEADED ARROW WITH VERTICAL STROKE..DOWN FISH TAIL
- {0x2980, 0x2982, prN}, // Sm [3] TRIPLE VERTICAL BAR DELIMITER..Z NOTATION TYPE COLON
- {0x2983, 0x2983, prN}, // Ps LEFT WHITE CURLY BRACKET
- {0x2984, 0x2984, prN}, // Pe RIGHT WHITE CURLY BRACKET
- {0x2985, 0x2985, prNa}, // Ps LEFT WHITE PARENTHESIS
- {0x2986, 0x2986, prNa}, // Pe RIGHT WHITE PARENTHESIS
- {0x2987, 0x2987, prN}, // Ps Z NOTATION LEFT IMAGE BRACKET
- {0x2988, 0x2988, prN}, // Pe Z NOTATION RIGHT IMAGE BRACKET
- {0x2989, 0x2989, prN}, // Ps Z NOTATION LEFT BINDING BRACKET
- {0x298A, 0x298A, prN}, // Pe Z NOTATION RIGHT BINDING BRACKET
- {0x298B, 0x298B, prN}, // Ps LEFT SQUARE BRACKET WITH UNDERBAR
- {0x298C, 0x298C, prN}, // Pe RIGHT SQUARE BRACKET WITH UNDERBAR
- {0x298D, 0x298D, prN}, // Ps LEFT SQUARE BRACKET WITH TICK IN TOP CORNER
- {0x298E, 0x298E, prN}, // Pe RIGHT SQUARE BRACKET WITH TICK IN BOTTOM CORNER
- {0x298F, 0x298F, prN}, // Ps LEFT SQUARE BRACKET WITH TICK IN BOTTOM CORNER
- {0x2990, 0x2990, prN}, // Pe RIGHT SQUARE BRACKET WITH TICK IN TOP CORNER
- {0x2991, 0x2991, prN}, // Ps LEFT ANGLE BRACKET WITH DOT
- {0x2992, 0x2992, prN}, // Pe RIGHT ANGLE BRACKET WITH DOT
- {0x2993, 0x2993, prN}, // Ps LEFT ARC LESS-THAN BRACKET
- {0x2994, 0x2994, prN}, // Pe RIGHT ARC GREATER-THAN BRACKET
- {0x2995, 0x2995, prN}, // Ps DOUBLE LEFT ARC GREATER-THAN BRACKET
- {0x2996, 0x2996, prN}, // Pe DOUBLE RIGHT ARC LESS-THAN BRACKET
- {0x2997, 0x2997, prN}, // Ps LEFT BLACK TORTOISE SHELL BRACKET
- {0x2998, 0x2998, prN}, // Pe RIGHT BLACK TORTOISE SHELL BRACKET
- {0x2999, 0x29D7, prN}, // Sm [63] DOTTED FENCE..BLACK HOURGLASS
- {0x29D8, 0x29D8, prN}, // Ps LEFT WIGGLY FENCE
- {0x29D9, 0x29D9, prN}, // Pe RIGHT WIGGLY FENCE
- {0x29DA, 0x29DA, prN}, // Ps LEFT DOUBLE WIGGLY FENCE
- {0x29DB, 0x29DB, prN}, // Pe RIGHT DOUBLE WIGGLY FENCE
- {0x29DC, 0x29FB, prN}, // Sm [32] INCOMPLETE INFINITY..TRIPLE PLUS
- {0x29FC, 0x29FC, prN}, // Ps LEFT-POINTING CURVED ANGLE BRACKET
- {0x29FD, 0x29FD, prN}, // Pe RIGHT-POINTING CURVED ANGLE BRACKET
- {0x29FE, 0x29FF, prN}, // Sm [2] TINY..MINY
- {0x2A00, 0x2AFF, prN}, // Sm [256] N-ARY CIRCLED DOT OPERATOR..N-ARY WHITE VERTICAL BAR
- {0x2B00, 0x2B1A, prN}, // So [27] NORTH EAST WHITE ARROW..DOTTED SQUARE
- {0x2B1B, 0x2B1C, prW}, // So [2] BLACK LARGE SQUARE..WHITE LARGE SQUARE
- {0x2B1D, 0x2B2F, prN}, // So [19] BLACK VERY SMALL SQUARE..WHITE VERTICAL ELLIPSE
- {0x2B30, 0x2B44, prN}, // Sm [21] LEFT ARROW WITH SMALL CIRCLE..RIGHTWARDS ARROW THROUGH SUPERSET
- {0x2B45, 0x2B46, prN}, // So [2] LEFTWARDS QUADRUPLE ARROW..RIGHTWARDS QUADRUPLE ARROW
- {0x2B47, 0x2B4C, prN}, // Sm [6] REVERSE TILDE OPERATOR ABOVE RIGHTWARDS ARROW..RIGHTWARDS ARROW ABOVE REVERSE TILDE OPERATOR
- {0x2B4D, 0x2B4F, prN}, // So [3] DOWNWARDS TRIANGLE-HEADED ZIGZAG ARROW..SHORT BACKSLANTED SOUTH ARROW
- {0x2B50, 0x2B50, prW}, // So WHITE MEDIUM STAR
- {0x2B51, 0x2B54, prN}, // So [4] BLACK SMALL STAR..WHITE RIGHT-POINTING PENTAGON
- {0x2B55, 0x2B55, prW}, // So HEAVY LARGE CIRCLE
- {0x2B56, 0x2B59, prA}, // So [4] HEAVY OVAL WITH OVAL INSIDE..HEAVY CIRCLED SALTIRE
- {0x2B5A, 0x2B73, prN}, // So [26] SLANTED NORTH ARROW WITH HOOKED HEAD..DOWNWARDS TRIANGLE-HEADED ARROW TO BAR
- {0x2B76, 0x2B95, prN}, // So [32] NORTH WEST TRIANGLE-HEADED ARROW TO BAR..RIGHTWARDS BLACK ARROW
- {0x2B97, 0x2BFF, prN}, // So [105] SYMBOL FOR TYPE A ELECTRONICS..HELLSCHREIBER PAUSE SYMBOL
- {0x2C00, 0x2C5F, prN}, // L& [96] GLAGOLITIC CAPITAL LETTER AZU..GLAGOLITIC SMALL LETTER CAUDATE CHRIVI
- {0x2C60, 0x2C7B, prN}, // L& [28] LATIN CAPITAL LETTER L WITH DOUBLE BAR..LATIN LETTER SMALL CAPITAL TURNED E
- {0x2C7C, 0x2C7D, prN}, // Lm [2] LATIN SUBSCRIPT SMALL LETTER J..MODIFIER LETTER CAPITAL V
- {0x2C7E, 0x2C7F, prN}, // Lu [2] LATIN CAPITAL LETTER S WITH SWASH TAIL..LATIN CAPITAL LETTER Z WITH SWASH TAIL
- {0x2C80, 0x2CE4, prN}, // L& [101] COPTIC CAPITAL LETTER ALFA..COPTIC SYMBOL KAI
- {0x2CE5, 0x2CEA, prN}, // So [6] COPTIC SYMBOL MI RO..COPTIC SYMBOL SHIMA SIMA
- {0x2CEB, 0x2CEE, prN}, // L& [4] COPTIC CAPITAL LETTER CRYPTOGRAMMIC SHEI..COPTIC SMALL LETTER CRYPTOGRAMMIC GANGIA
- {0x2CEF, 0x2CF1, prN}, // Mn [3] COPTIC COMBINING NI ABOVE..COPTIC COMBINING SPIRITUS LENIS
- {0x2CF2, 0x2CF3, prN}, // L& [2] COPTIC CAPITAL LETTER BOHAIRIC KHEI..COPTIC SMALL LETTER BOHAIRIC KHEI
- {0x2CF9, 0x2CFC, prN}, // Po [4] COPTIC OLD NUBIAN FULL STOP..COPTIC OLD NUBIAN VERSE DIVIDER
- {0x2CFD, 0x2CFD, prN}, // No COPTIC FRACTION ONE HALF
- {0x2CFE, 0x2CFF, prN}, // Po [2] COPTIC FULL STOP..COPTIC MORPHOLOGICAL DIVIDER
- {0x2D00, 0x2D25, prN}, // Ll [38] GEORGIAN SMALL LETTER AN..GEORGIAN SMALL LETTER HOE
- {0x2D27, 0x2D27, prN}, // Ll GEORGIAN SMALL LETTER YN
- {0x2D2D, 0x2D2D, prN}, // Ll GEORGIAN SMALL LETTER AEN
- {0x2D30, 0x2D67, prN}, // Lo [56] TIFINAGH LETTER YA..TIFINAGH LETTER YO
- {0x2D6F, 0x2D6F, prN}, // Lm TIFINAGH MODIFIER LETTER LABIALIZATION MARK
- {0x2D70, 0x2D70, prN}, // Po TIFINAGH SEPARATOR MARK
- {0x2D7F, 0x2D7F, prN}, // Mn TIFINAGH CONSONANT JOINER
- {0x2D80, 0x2D96, prN}, // Lo [23] ETHIOPIC SYLLABLE LOA..ETHIOPIC SYLLABLE GGWE
- {0x2DA0, 0x2DA6, prN}, // Lo [7] ETHIOPIC SYLLABLE SSA..ETHIOPIC SYLLABLE SSO
- {0x2DA8, 0x2DAE, prN}, // Lo [7] ETHIOPIC SYLLABLE CCA..ETHIOPIC SYLLABLE CCO
- {0x2DB0, 0x2DB6, prN}, // Lo [7] ETHIOPIC SYLLABLE ZZA..ETHIOPIC SYLLABLE ZZO
- {0x2DB8, 0x2DBE, prN}, // Lo [7] ETHIOPIC SYLLABLE CCHA..ETHIOPIC SYLLABLE CCHO
- {0x2DC0, 0x2DC6, prN}, // Lo [7] ETHIOPIC SYLLABLE QYA..ETHIOPIC SYLLABLE QYO
- {0x2DC8, 0x2DCE, prN}, // Lo [7] ETHIOPIC SYLLABLE KYA..ETHIOPIC SYLLABLE KYO
- {0x2DD0, 0x2DD6, prN}, // Lo [7] ETHIOPIC SYLLABLE XYA..ETHIOPIC SYLLABLE XYO
- {0x2DD8, 0x2DDE, prN}, // Lo [7] ETHIOPIC SYLLABLE GYA..ETHIOPIC SYLLABLE GYO
- {0x2DE0, 0x2DFF, prN}, // Mn [32] COMBINING CYRILLIC LETTER BE..COMBINING CYRILLIC LETTER IOTIFIED BIG YUS
- {0x2E00, 0x2E01, prN}, // Po [2] RIGHT ANGLE SUBSTITUTION MARKER..RIGHT ANGLE DOTTED SUBSTITUTION MARKER
- {0x2E02, 0x2E02, prN}, // Pi LEFT SUBSTITUTION BRACKET
- {0x2E03, 0x2E03, prN}, // Pf RIGHT SUBSTITUTION BRACKET
- {0x2E04, 0x2E04, prN}, // Pi LEFT DOTTED SUBSTITUTION BRACKET
- {0x2E05, 0x2E05, prN}, // Pf RIGHT DOTTED SUBSTITUTION BRACKET
- {0x2E06, 0x2E08, prN}, // Po [3] RAISED INTERPOLATION MARKER..DOTTED TRANSPOSITION MARKER
- {0x2E09, 0x2E09, prN}, // Pi LEFT TRANSPOSITION BRACKET
- {0x2E0A, 0x2E0A, prN}, // Pf RIGHT TRANSPOSITION BRACKET
- {0x2E0B, 0x2E0B, prN}, // Po RAISED SQUARE
- {0x2E0C, 0x2E0C, prN}, // Pi LEFT RAISED OMISSION BRACKET
- {0x2E0D, 0x2E0D, prN}, // Pf RIGHT RAISED OMISSION BRACKET
- {0x2E0E, 0x2E16, prN}, // Po [9] EDITORIAL CORONIS..DOTTED RIGHT-POINTING ANGLE
- {0x2E17, 0x2E17, prN}, // Pd DOUBLE OBLIQUE HYPHEN
- {0x2E18, 0x2E19, prN}, // Po [2] INVERTED INTERROBANG..PALM BRANCH
- {0x2E1A, 0x2E1A, prN}, // Pd HYPHEN WITH DIAERESIS
- {0x2E1B, 0x2E1B, prN}, // Po TILDE WITH RING ABOVE
- {0x2E1C, 0x2E1C, prN}, // Pi LEFT LOW PARAPHRASE BRACKET
- {0x2E1D, 0x2E1D, prN}, // Pf RIGHT LOW PARAPHRASE BRACKET
- {0x2E1E, 0x2E1F, prN}, // Po [2] TILDE WITH DOT ABOVE..TILDE WITH DOT BELOW
- {0x2E20, 0x2E20, prN}, // Pi LEFT VERTICAL BAR WITH QUILL
- {0x2E21, 0x2E21, prN}, // Pf RIGHT VERTICAL BAR WITH QUILL
- {0x2E22, 0x2E22, prN}, // Ps TOP LEFT HALF BRACKET
- {0x2E23, 0x2E23, prN}, // Pe TOP RIGHT HALF BRACKET
- {0x2E24, 0x2E24, prN}, // Ps BOTTOM LEFT HALF BRACKET
- {0x2E25, 0x2E25, prN}, // Pe BOTTOM RIGHT HALF BRACKET
- {0x2E26, 0x2E26, prN}, // Ps LEFT SIDEWAYS U BRACKET
- {0x2E27, 0x2E27, prN}, // Pe RIGHT SIDEWAYS U BRACKET
- {0x2E28, 0x2E28, prN}, // Ps LEFT DOUBLE PARENTHESIS
- {0x2E29, 0x2E29, prN}, // Pe RIGHT DOUBLE PARENTHESIS
- {0x2E2A, 0x2E2E, prN}, // Po [5] TWO DOTS OVER ONE DOT PUNCTUATION..REVERSED QUESTION MARK
- {0x2E2F, 0x2E2F, prN}, // Lm VERTICAL TILDE
- {0x2E30, 0x2E39, prN}, // Po [10] RING POINT..TOP HALF SECTION SIGN
- {0x2E3A, 0x2E3B, prN}, // Pd [2] TWO-EM DASH..THREE-EM DASH
- {0x2E3C, 0x2E3F, prN}, // Po [4] STENOGRAPHIC FULL STOP..CAPITULUM
- {0x2E40, 0x2E40, prN}, // Pd DOUBLE HYPHEN
- {0x2E41, 0x2E41, prN}, // Po REVERSED COMMA
- {0x2E42, 0x2E42, prN}, // Ps DOUBLE LOW-REVERSED-9 QUOTATION MARK
- {0x2E43, 0x2E4F, prN}, // Po [13] DASH WITH LEFT UPTURN..CORNISH VERSE DIVIDER
- {0x2E50, 0x2E51, prN}, // So [2] CROSS PATTY WITH RIGHT CROSSBAR..CROSS PATTY WITH LEFT CROSSBAR
- {0x2E52, 0x2E54, prN}, // Po [3] TIRONIAN SIGN CAPITAL ET..MEDIEVAL QUESTION MARK
- {0x2E55, 0x2E55, prN}, // Ps LEFT SQUARE BRACKET WITH STROKE
- {0x2E56, 0x2E56, prN}, // Pe RIGHT SQUARE BRACKET WITH STROKE
- {0x2E57, 0x2E57, prN}, // Ps LEFT SQUARE BRACKET WITH DOUBLE STROKE
- {0x2E58, 0x2E58, prN}, // Pe RIGHT SQUARE BRACKET WITH DOUBLE STROKE
- {0x2E59, 0x2E59, prN}, // Ps TOP HALF LEFT PARENTHESIS
- {0x2E5A, 0x2E5A, prN}, // Pe TOP HALF RIGHT PARENTHESIS
- {0x2E5B, 0x2E5B, prN}, // Ps BOTTOM HALF LEFT PARENTHESIS
- {0x2E5C, 0x2E5C, prN}, // Pe BOTTOM HALF RIGHT PARENTHESIS
- {0x2E5D, 0x2E5D, prN}, // Pd OBLIQUE HYPHEN
- {0x2E80, 0x2E99, prW}, // So [26] CJK RADICAL REPEAT..CJK RADICAL RAP
- {0x2E9B, 0x2EF3, prW}, // So [89] CJK RADICAL CHOKE..CJK RADICAL C-SIMPLIFIED TURTLE
- {0x2F00, 0x2FD5, prW}, // So [214] KANGXI RADICAL ONE..KANGXI RADICAL FLUTE
- {0x2FF0, 0x2FFB, prW}, // So [12] IDEOGRAPHIC DESCRIPTION CHARACTER LEFT TO RIGHT..IDEOGRAPHIC DESCRIPTION CHARACTER OVERLAID
- {0x3000, 0x3000, prF}, // Zs IDEOGRAPHIC SPACE
- {0x3001, 0x3003, prW}, // Po [3] IDEOGRAPHIC COMMA..DITTO MARK
- {0x3004, 0x3004, prW}, // So JAPANESE INDUSTRIAL STANDARD SYMBOL
- {0x3005, 0x3005, prW}, // Lm IDEOGRAPHIC ITERATION MARK
- {0x3006, 0x3006, prW}, // Lo IDEOGRAPHIC CLOSING MARK
- {0x3007, 0x3007, prW}, // Nl IDEOGRAPHIC NUMBER ZERO
- {0x3008, 0x3008, prW}, // Ps LEFT ANGLE BRACKET
- {0x3009, 0x3009, prW}, // Pe RIGHT ANGLE BRACKET
- {0x300A, 0x300A, prW}, // Ps LEFT DOUBLE ANGLE BRACKET
- {0x300B, 0x300B, prW}, // Pe RIGHT DOUBLE ANGLE BRACKET
- {0x300C, 0x300C, prW}, // Ps LEFT CORNER BRACKET
- {0x300D, 0x300D, prW}, // Pe RIGHT CORNER BRACKET
- {0x300E, 0x300E, prW}, // Ps LEFT WHITE CORNER BRACKET
- {0x300F, 0x300F, prW}, // Pe RIGHT WHITE CORNER BRACKET
- {0x3010, 0x3010, prW}, // Ps LEFT BLACK LENTICULAR BRACKET
- {0x3011, 0x3011, prW}, // Pe RIGHT BLACK LENTICULAR BRACKET
- {0x3012, 0x3013, prW}, // So [2] POSTAL MARK..GETA MARK
- {0x3014, 0x3014, prW}, // Ps LEFT TORTOISE SHELL BRACKET
- {0x3015, 0x3015, prW}, // Pe RIGHT TORTOISE SHELL BRACKET
- {0x3016, 0x3016, prW}, // Ps LEFT WHITE LENTICULAR BRACKET
- {0x3017, 0x3017, prW}, // Pe RIGHT WHITE LENTICULAR BRACKET
- {0x3018, 0x3018, prW}, // Ps LEFT WHITE TORTOISE SHELL BRACKET
- {0x3019, 0x3019, prW}, // Pe RIGHT WHITE TORTOISE SHELL BRACKET
- {0x301A, 0x301A, prW}, // Ps LEFT WHITE SQUARE BRACKET
- {0x301B, 0x301B, prW}, // Pe RIGHT WHITE SQUARE BRACKET
- {0x301C, 0x301C, prW}, // Pd WAVE DASH
- {0x301D, 0x301D, prW}, // Ps REVERSED DOUBLE PRIME QUOTATION MARK
- {0x301E, 0x301F, prW}, // Pe [2] DOUBLE PRIME QUOTATION MARK..LOW DOUBLE PRIME QUOTATION MARK
- {0x3020, 0x3020, prW}, // So POSTAL MARK FACE
- {0x3021, 0x3029, prW}, // Nl [9] HANGZHOU NUMERAL ONE..HANGZHOU NUMERAL NINE
- {0x302A, 0x302D, prW}, // Mn [4] IDEOGRAPHIC LEVEL TONE MARK..IDEOGRAPHIC ENTERING TONE MARK
- {0x302E, 0x302F, prW}, // Mc [2] HANGUL SINGLE DOT TONE MARK..HANGUL DOUBLE DOT TONE MARK
- {0x3030, 0x3030, prW}, // Pd WAVY DASH
- {0x3031, 0x3035, prW}, // Lm [5] VERTICAL KANA REPEAT MARK..VERTICAL KANA REPEAT MARK LOWER HALF
- {0x3036, 0x3037, prW}, // So [2] CIRCLED POSTAL MARK..IDEOGRAPHIC TELEGRAPH LINE FEED SEPARATOR SYMBOL
- {0x3038, 0x303A, prW}, // Nl [3] HANGZHOU NUMERAL TEN..HANGZHOU NUMERAL THIRTY
- {0x303B, 0x303B, prW}, // Lm VERTICAL IDEOGRAPHIC ITERATION MARK
- {0x303C, 0x303C, prW}, // Lo MASU MARK
- {0x303D, 0x303D, prW}, // Po PART ALTERNATION MARK
- {0x303E, 0x303E, prW}, // So IDEOGRAPHIC VARIATION INDICATOR
- {0x303F, 0x303F, prN}, // So IDEOGRAPHIC HALF FILL SPACE
- {0x3041, 0x3096, prW}, // Lo [86] HIRAGANA LETTER SMALL A..HIRAGANA LETTER SMALL KE
- {0x3099, 0x309A, prW}, // Mn [2] COMBINING KATAKANA-HIRAGANA VOICED SOUND MARK..COMBINING KATAKANA-HIRAGANA SEMI-VOICED SOUND MARK
- {0x309B, 0x309C, prW}, // Sk [2] KATAKANA-HIRAGANA VOICED SOUND MARK..KATAKANA-HIRAGANA SEMI-VOICED SOUND MARK
- {0x309D, 0x309E, prW}, // Lm [2] HIRAGANA ITERATION MARK..HIRAGANA VOICED ITERATION MARK
- {0x309F, 0x309F, prW}, // Lo HIRAGANA DIGRAPH YORI
- {0x30A0, 0x30A0, prW}, // Pd KATAKANA-HIRAGANA DOUBLE HYPHEN
- {0x30A1, 0x30FA, prW}, // Lo [90] KATAKANA LETTER SMALL A..KATAKANA LETTER VO
- {0x30FB, 0x30FB, prW}, // Po KATAKANA MIDDLE DOT
- {0x30FC, 0x30FE, prW}, // Lm [3] KATAKANA-HIRAGANA PROLONGED SOUND MARK..KATAKANA VOICED ITERATION MARK
- {0x30FF, 0x30FF, prW}, // Lo KATAKANA DIGRAPH KOTO
- {0x3105, 0x312F, prW}, // Lo [43] BOPOMOFO LETTER B..BOPOMOFO LETTER NN
- {0x3131, 0x318E, prW}, // Lo [94] HANGUL LETTER KIYEOK..HANGUL LETTER ARAEAE
- {0x3190, 0x3191, prW}, // So [2] IDEOGRAPHIC ANNOTATION LINKING MARK..IDEOGRAPHIC ANNOTATION REVERSE MARK
- {0x3192, 0x3195, prW}, // No [4] IDEOGRAPHIC ANNOTATION ONE MARK..IDEOGRAPHIC ANNOTATION FOUR MARK
- {0x3196, 0x319F, prW}, // So [10] IDEOGRAPHIC ANNOTATION TOP MARK..IDEOGRAPHIC ANNOTATION MAN MARK
- {0x31A0, 0x31BF, prW}, // Lo [32] BOPOMOFO LETTER BU..BOPOMOFO LETTER AH
- {0x31C0, 0x31E3, prW}, // So [36] CJK STROKE T..CJK STROKE Q
- {0x31F0, 0x31FF, prW}, // Lo [16] KATAKANA LETTER SMALL KU..KATAKANA LETTER SMALL RO
- {0x3200, 0x321E, prW}, // So [31] PARENTHESIZED HANGUL KIYEOK..PARENTHESIZED KOREAN CHARACTER O HU
- {0x3220, 0x3229, prW}, // No [10] PARENTHESIZED IDEOGRAPH ONE..PARENTHESIZED IDEOGRAPH TEN
- {0x322A, 0x3247, prW}, // So [30] PARENTHESIZED IDEOGRAPH MOON..CIRCLED IDEOGRAPH KOTO
- {0x3248, 0x324F, prA}, // No [8] CIRCLED NUMBER TEN ON BLACK SQUARE..CIRCLED NUMBER EIGHTY ON BLACK SQUARE
- {0x3250, 0x3250, prW}, // So PARTNERSHIP SIGN
- {0x3251, 0x325F, prW}, // No [15] CIRCLED NUMBER TWENTY ONE..CIRCLED NUMBER THIRTY FIVE
- {0x3260, 0x327F, prW}, // So [32] CIRCLED HANGUL KIYEOK..KOREAN STANDARD SYMBOL
- {0x3280, 0x3289, prW}, // No [10] CIRCLED IDEOGRAPH ONE..CIRCLED IDEOGRAPH TEN
- {0x328A, 0x32B0, prW}, // So [39] CIRCLED IDEOGRAPH MOON..CIRCLED IDEOGRAPH NIGHT
- {0x32B1, 0x32BF, prW}, // No [15] CIRCLED NUMBER THIRTY SIX..CIRCLED NUMBER FIFTY
- {0x32C0, 0x32FF, prW}, // So [64] IDEOGRAPHIC TELEGRAPH SYMBOL FOR JANUARY..SQUARE ERA NAME REIWA
- {0x3300, 0x33FF, prW}, // So [256] SQUARE APAATO..SQUARE GAL
- {0x3400, 0x4DBF, prW}, // Lo [6592] CJK UNIFIED IDEOGRAPH-3400..CJK UNIFIED IDEOGRAPH-4DBF
- {0x4DC0, 0x4DFF, prN}, // So [64] HEXAGRAM FOR THE CREATIVE HEAVEN..HEXAGRAM FOR BEFORE COMPLETION
- {0x4E00, 0x9FFF, prW}, // Lo [20992] CJK UNIFIED IDEOGRAPH-4E00..CJK UNIFIED IDEOGRAPH-9FFF
- {0xA000, 0xA014, prW}, // Lo [21] YI SYLLABLE IT..YI SYLLABLE E
- {0xA015, 0xA015, prW}, // Lm YI SYLLABLE WU
- {0xA016, 0xA48C, prW}, // Lo [1143] YI SYLLABLE BIT..YI SYLLABLE YYR
- {0xA490, 0xA4C6, prW}, // So [55] YI RADICAL QOT..YI RADICAL KE
- {0xA4D0, 0xA4F7, prN}, // Lo [40] LISU LETTER BA..LISU LETTER OE
- {0xA4F8, 0xA4FD, prN}, // Lm [6] LISU LETTER TONE MYA TI..LISU LETTER TONE MYA JEU
- {0xA4FE, 0xA4FF, prN}, // Po [2] LISU PUNCTUATION COMMA..LISU PUNCTUATION FULL STOP
- {0xA500, 0xA60B, prN}, // Lo [268] VAI SYLLABLE EE..VAI SYLLABLE NG
- {0xA60C, 0xA60C, prN}, // Lm VAI SYLLABLE LENGTHENER
- {0xA60D, 0xA60F, prN}, // Po [3] VAI COMMA..VAI QUESTION MARK
- {0xA610, 0xA61F, prN}, // Lo [16] VAI SYLLABLE NDOLE FA..VAI SYMBOL JONG
- {0xA620, 0xA629, prN}, // Nd [10] VAI DIGIT ZERO..VAI DIGIT NINE
- {0xA62A, 0xA62B, prN}, // Lo [2] VAI SYLLABLE NDOLE MA..VAI SYLLABLE NDOLE DO
- {0xA640, 0xA66D, prN}, // L& [46] CYRILLIC CAPITAL LETTER ZEMLYA..CYRILLIC SMALL LETTER DOUBLE MONOCULAR O
- {0xA66E, 0xA66E, prN}, // Lo CYRILLIC LETTER MULTIOCULAR O
- {0xA66F, 0xA66F, prN}, // Mn COMBINING CYRILLIC VZMET
- {0xA670, 0xA672, prN}, // Me [3] COMBINING CYRILLIC TEN MILLIONS SIGN..COMBINING CYRILLIC THOUSAND MILLIONS SIGN
- {0xA673, 0xA673, prN}, // Po SLAVONIC ASTERISK
- {0xA674, 0xA67D, prN}, // Mn [10] COMBINING CYRILLIC LETTER UKRAINIAN IE..COMBINING CYRILLIC PAYEROK
- {0xA67E, 0xA67E, prN}, // Po CYRILLIC KAVYKA
- {0xA67F, 0xA67F, prN}, // Lm CYRILLIC PAYEROK
- {0xA680, 0xA69B, prN}, // L& [28] CYRILLIC CAPITAL LETTER DWE..CYRILLIC SMALL LETTER CROSSED O
- {0xA69C, 0xA69D, prN}, // Lm [2] MODIFIER LETTER CYRILLIC HARD SIGN..MODIFIER LETTER CYRILLIC SOFT SIGN
- {0xA69E, 0xA69F, prN}, // Mn [2] COMBINING CYRILLIC LETTER EF..COMBINING CYRILLIC LETTER IOTIFIED E
- {0xA6A0, 0xA6E5, prN}, // Lo [70] BAMUM LETTER A..BAMUM LETTER KI
- {0xA6E6, 0xA6EF, prN}, // Nl [10] BAMUM LETTER MO..BAMUM LETTER KOGHOM
- {0xA6F0, 0xA6F1, prN}, // Mn [2] BAMUM COMBINING MARK KOQNDON..BAMUM COMBINING MARK TUKWENTIS
- {0xA6F2, 0xA6F7, prN}, // Po [6] BAMUM NJAEMLI..BAMUM QUESTION MARK
- {0xA700, 0xA716, prN}, // Sk [23] MODIFIER LETTER CHINESE TONE YIN PING..MODIFIER LETTER EXTRA-LOW LEFT-STEM TONE BAR
- {0xA717, 0xA71F, prN}, // Lm [9] MODIFIER LETTER DOT VERTICAL BAR..MODIFIER LETTER LOW INVERTED EXCLAMATION MARK
- {0xA720, 0xA721, prN}, // Sk [2] MODIFIER LETTER STRESS AND HIGH TONE..MODIFIER LETTER STRESS AND LOW TONE
- {0xA722, 0xA76F, prN}, // L& [78] LATIN CAPITAL LETTER EGYPTOLOGICAL ALEF..LATIN SMALL LETTER CON
- {0xA770, 0xA770, prN}, // Lm MODIFIER LETTER US
- {0xA771, 0xA787, prN}, // L& [23] LATIN SMALL LETTER DUM..LATIN SMALL LETTER INSULAR T
- {0xA788, 0xA788, prN}, // Lm MODIFIER LETTER LOW CIRCUMFLEX ACCENT
- {0xA789, 0xA78A, prN}, // Sk [2] MODIFIER LETTER COLON..MODIFIER LETTER SHORT EQUALS SIGN
- {0xA78B, 0xA78E, prN}, // L& [4] LATIN CAPITAL LETTER SALTILLO..LATIN SMALL LETTER L WITH RETROFLEX HOOK AND BELT
- {0xA78F, 0xA78F, prN}, // Lo LATIN LETTER SINOLOGICAL DOT
- {0xA790, 0xA7CA, prN}, // L& [59] LATIN CAPITAL LETTER N WITH DESCENDER..LATIN SMALL LETTER S WITH SHORT STROKE OVERLAY
- {0xA7D0, 0xA7D1, prN}, // L& [2] LATIN CAPITAL LETTER CLOSED INSULAR G..LATIN SMALL LETTER CLOSED INSULAR G
- {0xA7D3, 0xA7D3, prN}, // Ll LATIN SMALL LETTER DOUBLE THORN
- {0xA7D5, 0xA7D9, prN}, // L& [5] LATIN SMALL LETTER DOUBLE WYNN..LATIN SMALL LETTER SIGMOID S
- {0xA7F2, 0xA7F4, prN}, // Lm [3] MODIFIER LETTER CAPITAL C..MODIFIER LETTER CAPITAL Q
- {0xA7F5, 0xA7F6, prN}, // L& [2] LATIN CAPITAL LETTER REVERSED HALF H..LATIN SMALL LETTER REVERSED HALF H
- {0xA7F7, 0xA7F7, prN}, // Lo LATIN EPIGRAPHIC LETTER SIDEWAYS I
- {0xA7F8, 0xA7F9, prN}, // Lm [2] MODIFIER LETTER CAPITAL H WITH STROKE..MODIFIER LETTER SMALL LIGATURE OE
- {0xA7FA, 0xA7FA, prN}, // Ll LATIN LETTER SMALL CAPITAL TURNED M
- {0xA7FB, 0xA7FF, prN}, // Lo [5] LATIN EPIGRAPHIC LETTER REVERSED F..LATIN EPIGRAPHIC LETTER ARCHAIC M
- {0xA800, 0xA801, prN}, // Lo [2] SYLOTI NAGRI LETTER A..SYLOTI NAGRI LETTER I
- {0xA802, 0xA802, prN}, // Mn SYLOTI NAGRI SIGN DVISVARA
- {0xA803, 0xA805, prN}, // Lo [3] SYLOTI NAGRI LETTER U..SYLOTI NAGRI LETTER O
- {0xA806, 0xA806, prN}, // Mn SYLOTI NAGRI SIGN HASANTA
- {0xA807, 0xA80A, prN}, // Lo [4] SYLOTI NAGRI LETTER KO..SYLOTI NAGRI LETTER GHO
- {0xA80B, 0xA80B, prN}, // Mn SYLOTI NAGRI SIGN ANUSVARA
- {0xA80C, 0xA822, prN}, // Lo [23] SYLOTI NAGRI LETTER CO..SYLOTI NAGRI LETTER HO
- {0xA823, 0xA824, prN}, // Mc [2] SYLOTI NAGRI VOWEL SIGN A..SYLOTI NAGRI VOWEL SIGN I
- {0xA825, 0xA826, prN}, // Mn [2] SYLOTI NAGRI VOWEL SIGN U..SYLOTI NAGRI VOWEL SIGN E
- {0xA827, 0xA827, prN}, // Mc SYLOTI NAGRI VOWEL SIGN OO
- {0xA828, 0xA82B, prN}, // So [4] SYLOTI NAGRI POETRY MARK-1..SYLOTI NAGRI POETRY MARK-4
- {0xA82C, 0xA82C, prN}, // Mn SYLOTI NAGRI SIGN ALTERNATE HASANTA
- {0xA830, 0xA835, prN}, // No [6] NORTH INDIC FRACTION ONE QUARTER..NORTH INDIC FRACTION THREE SIXTEENTHS
- {0xA836, 0xA837, prN}, // So [2] NORTH INDIC QUARTER MARK..NORTH INDIC PLACEHOLDER MARK
- {0xA838, 0xA838, prN}, // Sc NORTH INDIC RUPEE MARK
- {0xA839, 0xA839, prN}, // So NORTH INDIC QUANTITY MARK
- {0xA840, 0xA873, prN}, // Lo [52] PHAGS-PA LETTER KA..PHAGS-PA LETTER CANDRABINDU
- {0xA874, 0xA877, prN}, // Po [4] PHAGS-PA SINGLE HEAD MARK..PHAGS-PA MARK DOUBLE SHAD
- {0xA880, 0xA881, prN}, // Mc [2] SAURASHTRA SIGN ANUSVARA..SAURASHTRA SIGN VISARGA
- {0xA882, 0xA8B3, prN}, // Lo [50] SAURASHTRA LETTER A..SAURASHTRA LETTER LLA
- {0xA8B4, 0xA8C3, prN}, // Mc [16] SAURASHTRA CONSONANT SIGN HAARU..SAURASHTRA VOWEL SIGN AU
- {0xA8C4, 0xA8C5, prN}, // Mn [2] SAURASHTRA SIGN VIRAMA..SAURASHTRA SIGN CANDRABINDU
- {0xA8CE, 0xA8CF, prN}, // Po [2] SAURASHTRA DANDA..SAURASHTRA DOUBLE DANDA
- {0xA8D0, 0xA8D9, prN}, // Nd [10] SAURASHTRA DIGIT ZERO..SAURASHTRA DIGIT NINE
- {0xA8E0, 0xA8F1, prN}, // Mn [18] COMBINING DEVANAGARI DIGIT ZERO..COMBINING DEVANAGARI SIGN AVAGRAHA
- {0xA8F2, 0xA8F7, prN}, // Lo [6] DEVANAGARI SIGN SPACING CANDRABINDU..DEVANAGARI SIGN CANDRABINDU AVAGRAHA
- {0xA8F8, 0xA8FA, prN}, // Po [3] DEVANAGARI SIGN PUSHPIKA..DEVANAGARI CARET
- {0xA8FB, 0xA8FB, prN}, // Lo DEVANAGARI HEADSTROKE
- {0xA8FC, 0xA8FC, prN}, // Po DEVANAGARI SIGN SIDDHAM
- {0xA8FD, 0xA8FE, prN}, // Lo [2] DEVANAGARI JAIN OM..DEVANAGARI LETTER AY
- {0xA8FF, 0xA8FF, prN}, // Mn DEVANAGARI VOWEL SIGN AY
- {0xA900, 0xA909, prN}, // Nd [10] KAYAH LI DIGIT ZERO..KAYAH LI DIGIT NINE
- {0xA90A, 0xA925, prN}, // Lo [28] KAYAH LI LETTER KA..KAYAH LI LETTER OO
- {0xA926, 0xA92D, prN}, // Mn [8] KAYAH LI VOWEL UE..KAYAH LI TONE CALYA PLOPHU
- {0xA92E, 0xA92F, prN}, // Po [2] KAYAH LI SIGN CWI..KAYAH LI SIGN SHYA
- {0xA930, 0xA946, prN}, // Lo [23] REJANG LETTER KA..REJANG LETTER A
- {0xA947, 0xA951, prN}, // Mn [11] REJANG VOWEL SIGN I..REJANG CONSONANT SIGN R
- {0xA952, 0xA953, prN}, // Mc [2] REJANG CONSONANT SIGN H..REJANG VIRAMA
- {0xA95F, 0xA95F, prN}, // Po REJANG SECTION MARK
- {0xA960, 0xA97C, prW}, // Lo [29] HANGUL CHOSEONG TIKEUT-MIEUM..HANGUL CHOSEONG SSANGYEORINHIEUH
- {0xA980, 0xA982, prN}, // Mn [3] JAVANESE SIGN PANYANGGA..JAVANESE SIGN LAYAR
- {0xA983, 0xA983, prN}, // Mc JAVANESE SIGN WIGNYAN
- {0xA984, 0xA9B2, prN}, // Lo [47] JAVANESE LETTER A..JAVANESE LETTER HA
- {0xA9B3, 0xA9B3, prN}, // Mn JAVANESE SIGN CECAK TELU
- {0xA9B4, 0xA9B5, prN}, // Mc [2] JAVANESE VOWEL SIGN TARUNG..JAVANESE VOWEL SIGN TOLONG
- {0xA9B6, 0xA9B9, prN}, // Mn [4] JAVANESE VOWEL SIGN WULU..JAVANESE VOWEL SIGN SUKU MENDUT
- {0xA9BA, 0xA9BB, prN}, // Mc [2] JAVANESE VOWEL SIGN TALING..JAVANESE VOWEL SIGN DIRGA MURE
- {0xA9BC, 0xA9BD, prN}, // Mn [2] JAVANESE VOWEL SIGN PEPET..JAVANESE CONSONANT SIGN KERET
- {0xA9BE, 0xA9C0, prN}, // Mc [3] JAVANESE CONSONANT SIGN PENGKAL..JAVANESE PANGKON
- {0xA9C1, 0xA9CD, prN}, // Po [13] JAVANESE LEFT RERENGGAN..JAVANESE TURNED PADA PISELEH
- {0xA9CF, 0xA9CF, prN}, // Lm JAVANESE PANGRANGKEP
- {0xA9D0, 0xA9D9, prN}, // Nd [10] JAVANESE DIGIT ZERO..JAVANESE DIGIT NINE
- {0xA9DE, 0xA9DF, prN}, // Po [2] JAVANESE PADA TIRTA TUMETES..JAVANESE PADA ISEN-ISEN
- {0xA9E0, 0xA9E4, prN}, // Lo [5] MYANMAR LETTER SHAN GHA..MYANMAR LETTER SHAN BHA
- {0xA9E5, 0xA9E5, prN}, // Mn MYANMAR SIGN SHAN SAW
- {0xA9E6, 0xA9E6, prN}, // Lm MYANMAR MODIFIER LETTER SHAN REDUPLICATION
- {0xA9E7, 0xA9EF, prN}, // Lo [9] MYANMAR LETTER TAI LAING NYA..MYANMAR LETTER TAI LAING NNA
- {0xA9F0, 0xA9F9, prN}, // Nd [10] MYANMAR TAI LAING DIGIT ZERO..MYANMAR TAI LAING DIGIT NINE
- {0xA9FA, 0xA9FE, prN}, // Lo [5] MYANMAR LETTER TAI LAING LLA..MYANMAR LETTER TAI LAING BHA
- {0xAA00, 0xAA28, prN}, // Lo [41] CHAM LETTER A..CHAM LETTER HA
- {0xAA29, 0xAA2E, prN}, // Mn [6] CHAM VOWEL SIGN AA..CHAM VOWEL SIGN OE
- {0xAA2F, 0xAA30, prN}, // Mc [2] CHAM VOWEL SIGN O..CHAM VOWEL SIGN AI
- {0xAA31, 0xAA32, prN}, // Mn [2] CHAM VOWEL SIGN AU..CHAM VOWEL SIGN UE
- {0xAA33, 0xAA34, prN}, // Mc [2] CHAM CONSONANT SIGN YA..CHAM CONSONANT SIGN RA
- {0xAA35, 0xAA36, prN}, // Mn [2] CHAM CONSONANT SIGN LA..CHAM CONSONANT SIGN WA
- {0xAA40, 0xAA42, prN}, // Lo [3] CHAM LETTER FINAL K..CHAM LETTER FINAL NG
- {0xAA43, 0xAA43, prN}, // Mn CHAM CONSONANT SIGN FINAL NG
- {0xAA44, 0xAA4B, prN}, // Lo [8] CHAM LETTER FINAL CH..CHAM LETTER FINAL SS
- {0xAA4C, 0xAA4C, prN}, // Mn CHAM CONSONANT SIGN FINAL M
- {0xAA4D, 0xAA4D, prN}, // Mc CHAM CONSONANT SIGN FINAL H
- {0xAA50, 0xAA59, prN}, // Nd [10] CHAM DIGIT ZERO..CHAM DIGIT NINE
- {0xAA5C, 0xAA5F, prN}, // Po [4] CHAM PUNCTUATION SPIRAL..CHAM PUNCTUATION TRIPLE DANDA
- {0xAA60, 0xAA6F, prN}, // Lo [16] MYANMAR LETTER KHAMTI GA..MYANMAR LETTER KHAMTI FA
- {0xAA70, 0xAA70, prN}, // Lm MYANMAR MODIFIER LETTER KHAMTI REDUPLICATION
- {0xAA71, 0xAA76, prN}, // Lo [6] MYANMAR LETTER KHAMTI XA..MYANMAR LOGOGRAM KHAMTI HM
- {0xAA77, 0xAA79, prN}, // So [3] MYANMAR SYMBOL AITON EXCLAMATION..MYANMAR SYMBOL AITON TWO
- {0xAA7A, 0xAA7A, prN}, // Lo MYANMAR LETTER AITON RA
- {0xAA7B, 0xAA7B, prN}, // Mc MYANMAR SIGN PAO KAREN TONE
- {0xAA7C, 0xAA7C, prN}, // Mn MYANMAR SIGN TAI LAING TONE-2
- {0xAA7D, 0xAA7D, prN}, // Mc MYANMAR SIGN TAI LAING TONE-5
- {0xAA7E, 0xAA7F, prN}, // Lo [2] MYANMAR LETTER SHWE PALAUNG CHA..MYANMAR LETTER SHWE PALAUNG SHA
- {0xAA80, 0xAAAF, prN}, // Lo [48] TAI VIET LETTER LOW KO..TAI VIET LETTER HIGH O
- {0xAAB0, 0xAAB0, prN}, // Mn TAI VIET MAI KANG
- {0xAAB1, 0xAAB1, prN}, // Lo TAI VIET VOWEL AA
- {0xAAB2, 0xAAB4, prN}, // Mn [3] TAI VIET VOWEL I..TAI VIET VOWEL U
- {0xAAB5, 0xAAB6, prN}, // Lo [2] TAI VIET VOWEL E..TAI VIET VOWEL O
- {0xAAB7, 0xAAB8, prN}, // Mn [2] TAI VIET MAI KHIT..TAI VIET VOWEL IA
- {0xAAB9, 0xAABD, prN}, // Lo [5] TAI VIET VOWEL UEA..TAI VIET VOWEL AN
- {0xAABE, 0xAABF, prN}, // Mn [2] TAI VIET VOWEL AM..TAI VIET TONE MAI EK
- {0xAAC0, 0xAAC0, prN}, // Lo TAI VIET TONE MAI NUENG
- {0xAAC1, 0xAAC1, prN}, // Mn TAI VIET TONE MAI THO
- {0xAAC2, 0xAAC2, prN}, // Lo TAI VIET TONE MAI SONG
- {0xAADB, 0xAADC, prN}, // Lo [2] TAI VIET SYMBOL KON..TAI VIET SYMBOL NUENG
- {0xAADD, 0xAADD, prN}, // Lm TAI VIET SYMBOL SAM
- {0xAADE, 0xAADF, prN}, // Po [2] TAI VIET SYMBOL HO HOI..TAI VIET SYMBOL KOI KOI
- {0xAAE0, 0xAAEA, prN}, // Lo [11] MEETEI MAYEK LETTER E..MEETEI MAYEK LETTER SSA
- {0xAAEB, 0xAAEB, prN}, // Mc MEETEI MAYEK VOWEL SIGN II
- {0xAAEC, 0xAAED, prN}, // Mn [2] MEETEI MAYEK VOWEL SIGN UU..MEETEI MAYEK VOWEL SIGN AAI
- {0xAAEE, 0xAAEF, prN}, // Mc [2] MEETEI MAYEK VOWEL SIGN AU..MEETEI MAYEK VOWEL SIGN AAU
- {0xAAF0, 0xAAF1, prN}, // Po [2] MEETEI MAYEK CHEIKHAN..MEETEI MAYEK AHANG KHUDAM
- {0xAAF2, 0xAAF2, prN}, // Lo MEETEI MAYEK ANJI
- {0xAAF3, 0xAAF4, prN}, // Lm [2] MEETEI MAYEK SYLLABLE REPETITION MARK..MEETEI MAYEK WORD REPETITION MARK
- {0xAAF5, 0xAAF5, prN}, // Mc MEETEI MAYEK VOWEL SIGN VISARGA
- {0xAAF6, 0xAAF6, prN}, // Mn MEETEI MAYEK VIRAMA
- {0xAB01, 0xAB06, prN}, // Lo [6] ETHIOPIC SYLLABLE TTHU..ETHIOPIC SYLLABLE TTHO
- {0xAB09, 0xAB0E, prN}, // Lo [6] ETHIOPIC SYLLABLE DDHU..ETHIOPIC SYLLABLE DDHO
- {0xAB11, 0xAB16, prN}, // Lo [6] ETHIOPIC SYLLABLE DZU..ETHIOPIC SYLLABLE DZO
- {0xAB20, 0xAB26, prN}, // Lo [7] ETHIOPIC SYLLABLE CCHHA..ETHIOPIC SYLLABLE CCHHO
- {0xAB28, 0xAB2E, prN}, // Lo [7] ETHIOPIC SYLLABLE BBA..ETHIOPIC SYLLABLE BBO
- {0xAB30, 0xAB5A, prN}, // Ll [43] LATIN SMALL LETTER BARRED ALPHA..LATIN SMALL LETTER Y WITH SHORT RIGHT LEG
- {0xAB5B, 0xAB5B, prN}, // Sk MODIFIER BREVE WITH INVERTED BREVE
- {0xAB5C, 0xAB5F, prN}, // Lm [4] MODIFIER LETTER SMALL HENG..MODIFIER LETTER SMALL U WITH LEFT HOOK
- {0xAB60, 0xAB68, prN}, // Ll [9] LATIN SMALL LETTER SAKHA YAT..LATIN SMALL LETTER TURNED R WITH MIDDLE TILDE
- {0xAB69, 0xAB69, prN}, // Lm MODIFIER LETTER SMALL TURNED W
- {0xAB6A, 0xAB6B, prN}, // Sk [2] MODIFIER LETTER LEFT TACK..MODIFIER LETTER RIGHT TACK
- {0xAB70, 0xABBF, prN}, // Ll [80] CHEROKEE SMALL LETTER A..CHEROKEE SMALL LETTER YA
- {0xABC0, 0xABE2, prN}, // Lo [35] MEETEI MAYEK LETTER KOK..MEETEI MAYEK LETTER I LONSUM
- {0xABE3, 0xABE4, prN}, // Mc [2] MEETEI MAYEK VOWEL SIGN ONAP..MEETEI MAYEK VOWEL SIGN INAP
- {0xABE5, 0xABE5, prN}, // Mn MEETEI MAYEK VOWEL SIGN ANAP
- {0xABE6, 0xABE7, prN}, // Mc [2] MEETEI MAYEK VOWEL SIGN YENAP..MEETEI MAYEK VOWEL SIGN SOUNAP
- {0xABE8, 0xABE8, prN}, // Mn MEETEI MAYEK VOWEL SIGN UNAP
- {0xABE9, 0xABEA, prN}, // Mc [2] MEETEI MAYEK VOWEL SIGN CHEINAP..MEETEI MAYEK VOWEL SIGN NUNG
- {0xABEB, 0xABEB, prN}, // Po MEETEI MAYEK CHEIKHEI
- {0xABEC, 0xABEC, prN}, // Mc MEETEI MAYEK LUM IYEK
- {0xABED, 0xABED, prN}, // Mn MEETEI MAYEK APUN IYEK
- {0xABF0, 0xABF9, prN}, // Nd [10] MEETEI MAYEK DIGIT ZERO..MEETEI MAYEK DIGIT NINE
- {0xAC00, 0xD7A3, prW}, // Lo [11172] HANGUL SYLLABLE GA..HANGUL SYLLABLE HIH
- {0xD7B0, 0xD7C6, prN}, // Lo [23] HANGUL JUNGSEONG O-YEO..HANGUL JUNGSEONG ARAEA-E
- {0xD7CB, 0xD7FB, prN}, // Lo [49] HANGUL JONGSEONG NIEUN-RIEUL..HANGUL JONGSEONG PHIEUPH-THIEUTH
- {0xD800, 0xDB7F, prN}, // Cs [896] ..
- {0xDB80, 0xDBFF, prN}, // Cs [128] ..
- {0xDC00, 0xDFFF, prN}, // Cs [1024] ..
- {0xE000, 0xF8FF, prA}, // Co [6400] ..
- {0xF900, 0xFA6D, prW}, // Lo [366] CJK COMPATIBILITY IDEOGRAPH-F900..CJK COMPATIBILITY IDEOGRAPH-FA6D
- {0xFA6E, 0xFA6F, prW}, // Cn [2] ..
- {0xFA70, 0xFAD9, prW}, // Lo [106] CJK COMPATIBILITY IDEOGRAPH-FA70..CJK COMPATIBILITY IDEOGRAPH-FAD9
- {0xFADA, 0xFAFF, prW}, // Cn [38] ..
- {0xFB00, 0xFB06, prN}, // Ll [7] LATIN SMALL LIGATURE FF..LATIN SMALL LIGATURE ST
- {0xFB13, 0xFB17, prN}, // Ll [5] ARMENIAN SMALL LIGATURE MEN NOW..ARMENIAN SMALL LIGATURE MEN XEH
- {0xFB1D, 0xFB1D, prN}, // Lo HEBREW LETTER YOD WITH HIRIQ
- {0xFB1E, 0xFB1E, prN}, // Mn HEBREW POINT JUDEO-SPANISH VARIKA
- {0xFB1F, 0xFB28, prN}, // Lo [10] HEBREW LIGATURE YIDDISH YOD YOD PATAH..HEBREW LETTER WIDE TAV
- {0xFB29, 0xFB29, prN}, // Sm HEBREW LETTER ALTERNATIVE PLUS SIGN
- {0xFB2A, 0xFB36, prN}, // Lo [13] HEBREW LETTER SHIN WITH SHIN DOT..HEBREW LETTER ZAYIN WITH DAGESH
- {0xFB38, 0xFB3C, prN}, // Lo [5] HEBREW LETTER TET WITH DAGESH..HEBREW LETTER LAMED WITH DAGESH
- {0xFB3E, 0xFB3E, prN}, // Lo HEBREW LETTER MEM WITH DAGESH
- {0xFB40, 0xFB41, prN}, // Lo [2] HEBREW LETTER NUN WITH DAGESH..HEBREW LETTER SAMEKH WITH DAGESH
- {0xFB43, 0xFB44, prN}, // Lo [2] HEBREW LETTER FINAL PE WITH DAGESH..HEBREW LETTER PE WITH DAGESH
- {0xFB46, 0xFB4F, prN}, // Lo [10] HEBREW LETTER TSADI WITH DAGESH..HEBREW LIGATURE ALEF LAMED
- {0xFB50, 0xFBB1, prN}, // Lo [98] ARABIC LETTER ALEF WASLA ISOLATED FORM..ARABIC LETTER YEH BARREE WITH HAMZA ABOVE FINAL FORM
- {0xFBB2, 0xFBC2, prN}, // Sk [17] ARABIC SYMBOL DOT ABOVE..ARABIC SYMBOL WASLA ABOVE
- {0xFBD3, 0xFD3D, prN}, // Lo [363] ARABIC LETTER NG ISOLATED FORM..ARABIC LIGATURE ALEF WITH FATHATAN ISOLATED FORM
- {0xFD3E, 0xFD3E, prN}, // Pe ORNATE LEFT PARENTHESIS
- {0xFD3F, 0xFD3F, prN}, // Ps ORNATE RIGHT PARENTHESIS
- {0xFD40, 0xFD4F, prN}, // So [16] ARABIC LIGATURE RAHIMAHU ALLAAH..ARABIC LIGATURE RAHIMAHUM ALLAAH
- {0xFD50, 0xFD8F, prN}, // Lo [64] ARABIC LIGATURE TEH WITH JEEM WITH MEEM INITIAL FORM..ARABIC LIGATURE MEEM WITH KHAH WITH MEEM INITIAL FORM
- {0xFD92, 0xFDC7, prN}, // Lo [54] ARABIC LIGATURE MEEM WITH JEEM WITH KHAH INITIAL FORM..ARABIC LIGATURE NOON WITH JEEM WITH YEH FINAL FORM
- {0xFDCF, 0xFDCF, prN}, // So ARABIC LIGATURE SALAAMUHU ALAYNAA
- {0xFDF0, 0xFDFB, prN}, // Lo [12] ARABIC LIGATURE SALLA USED AS KORANIC STOP SIGN ISOLATED FORM..ARABIC LIGATURE JALLAJALALOUHOU
- {0xFDFC, 0xFDFC, prN}, // Sc RIAL SIGN
- {0xFDFD, 0xFDFF, prN}, // So [3] ARABIC LIGATURE BISMILLAH AR-RAHMAN AR-RAHEEM..ARABIC LIGATURE AZZA WA JALL
- {0xFE00, 0xFE0F, prA}, // Mn [16] VARIATION SELECTOR-1..VARIATION SELECTOR-16
- {0xFE10, 0xFE16, prW}, // Po [7] PRESENTATION FORM FOR VERTICAL COMMA..PRESENTATION FORM FOR VERTICAL QUESTION MARK
- {0xFE17, 0xFE17, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT WHITE LENTICULAR BRACKET
- {0xFE18, 0xFE18, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT WHITE LENTICULAR BRAKCET
- {0xFE19, 0xFE19, prW}, // Po PRESENTATION FORM FOR VERTICAL HORIZONTAL ELLIPSIS
- {0xFE20, 0xFE2F, prN}, // Mn [16] COMBINING LIGATURE LEFT HALF..COMBINING CYRILLIC TITLO RIGHT HALF
- {0xFE30, 0xFE30, prW}, // Po PRESENTATION FORM FOR VERTICAL TWO DOT LEADER
- {0xFE31, 0xFE32, prW}, // Pd [2] PRESENTATION FORM FOR VERTICAL EM DASH..PRESENTATION FORM FOR VERTICAL EN DASH
- {0xFE33, 0xFE34, prW}, // Pc [2] PRESENTATION FORM FOR VERTICAL LOW LINE..PRESENTATION FORM FOR VERTICAL WAVY LOW LINE
- {0xFE35, 0xFE35, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT PARENTHESIS
- {0xFE36, 0xFE36, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT PARENTHESIS
- {0xFE37, 0xFE37, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT CURLY BRACKET
- {0xFE38, 0xFE38, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT CURLY BRACKET
- {0xFE39, 0xFE39, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT TORTOISE SHELL BRACKET
- {0xFE3A, 0xFE3A, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT TORTOISE SHELL BRACKET
- {0xFE3B, 0xFE3B, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT BLACK LENTICULAR BRACKET
- {0xFE3C, 0xFE3C, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT BLACK LENTICULAR BRACKET
- {0xFE3D, 0xFE3D, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT DOUBLE ANGLE BRACKET
- {0xFE3E, 0xFE3E, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT DOUBLE ANGLE BRACKET
- {0xFE3F, 0xFE3F, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT ANGLE BRACKET
- {0xFE40, 0xFE40, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT ANGLE BRACKET
- {0xFE41, 0xFE41, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT CORNER BRACKET
- {0xFE42, 0xFE42, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT CORNER BRACKET
- {0xFE43, 0xFE43, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT WHITE CORNER BRACKET
- {0xFE44, 0xFE44, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT WHITE CORNER BRACKET
- {0xFE45, 0xFE46, prW}, // Po [2] SESAME DOT..WHITE SESAME DOT
- {0xFE47, 0xFE47, prW}, // Ps PRESENTATION FORM FOR VERTICAL LEFT SQUARE BRACKET
- {0xFE48, 0xFE48, prW}, // Pe PRESENTATION FORM FOR VERTICAL RIGHT SQUARE BRACKET
- {0xFE49, 0xFE4C, prW}, // Po [4] DASHED OVERLINE..DOUBLE WAVY OVERLINE
- {0xFE4D, 0xFE4F, prW}, // Pc [3] DASHED LOW LINE..WAVY LOW LINE
- {0xFE50, 0xFE52, prW}, // Po [3] SMALL COMMA..SMALL FULL STOP
- {0xFE54, 0xFE57, prW}, // Po [4] SMALL SEMICOLON..SMALL EXCLAMATION MARK
- {0xFE58, 0xFE58, prW}, // Pd SMALL EM DASH
- {0xFE59, 0xFE59, prW}, // Ps SMALL LEFT PARENTHESIS
- {0xFE5A, 0xFE5A, prW}, // Pe SMALL RIGHT PARENTHESIS
- {0xFE5B, 0xFE5B, prW}, // Ps SMALL LEFT CURLY BRACKET
- {0xFE5C, 0xFE5C, prW}, // Pe SMALL RIGHT CURLY BRACKET
- {0xFE5D, 0xFE5D, prW}, // Ps SMALL LEFT TORTOISE SHELL BRACKET
- {0xFE5E, 0xFE5E, prW}, // Pe SMALL RIGHT TORTOISE SHELL BRACKET
- {0xFE5F, 0xFE61, prW}, // Po [3] SMALL NUMBER SIGN..SMALL ASTERISK
- {0xFE62, 0xFE62, prW}, // Sm SMALL PLUS SIGN
- {0xFE63, 0xFE63, prW}, // Pd SMALL HYPHEN-MINUS
- {0xFE64, 0xFE66, prW}, // Sm [3] SMALL LESS-THAN SIGN..SMALL EQUALS SIGN
- {0xFE68, 0xFE68, prW}, // Po SMALL REVERSE SOLIDUS
- {0xFE69, 0xFE69, prW}, // Sc SMALL DOLLAR SIGN
- {0xFE6A, 0xFE6B, prW}, // Po [2] SMALL PERCENT SIGN..SMALL COMMERCIAL AT
- {0xFE70, 0xFE74, prN}, // Lo [5] ARABIC FATHATAN ISOLATED FORM..ARABIC KASRATAN ISOLATED FORM
- {0xFE76, 0xFEFC, prN}, // Lo [135] ARABIC FATHA ISOLATED FORM..ARABIC LIGATURE LAM WITH ALEF FINAL FORM
- {0xFEFF, 0xFEFF, prN}, // Cf ZERO WIDTH NO-BREAK SPACE
- {0xFF01, 0xFF03, prF}, // Po [3] FULLWIDTH EXCLAMATION MARK..FULLWIDTH NUMBER SIGN
- {0xFF04, 0xFF04, prF}, // Sc FULLWIDTH DOLLAR SIGN
- {0xFF05, 0xFF07, prF}, // Po [3] FULLWIDTH PERCENT SIGN..FULLWIDTH APOSTROPHE
- {0xFF08, 0xFF08, prF}, // Ps FULLWIDTH LEFT PARENTHESIS
- {0xFF09, 0xFF09, prF}, // Pe FULLWIDTH RIGHT PARENTHESIS
- {0xFF0A, 0xFF0A, prF}, // Po FULLWIDTH ASTERISK
- {0xFF0B, 0xFF0B, prF}, // Sm FULLWIDTH PLUS SIGN
- {0xFF0C, 0xFF0C, prF}, // Po FULLWIDTH COMMA
- {0xFF0D, 0xFF0D, prF}, // Pd FULLWIDTH HYPHEN-MINUS
- {0xFF0E, 0xFF0F, prF}, // Po [2] FULLWIDTH FULL STOP..FULLWIDTH SOLIDUS
- {0xFF10, 0xFF19, prF}, // Nd [10] FULLWIDTH DIGIT ZERO..FULLWIDTH DIGIT NINE
- {0xFF1A, 0xFF1B, prF}, // Po [2] FULLWIDTH COLON..FULLWIDTH SEMICOLON
- {0xFF1C, 0xFF1E, prF}, // Sm [3] FULLWIDTH LESS-THAN SIGN..FULLWIDTH GREATER-THAN SIGN
- {0xFF1F, 0xFF20, prF}, // Po [2] FULLWIDTH QUESTION MARK..FULLWIDTH COMMERCIAL AT
- {0xFF21, 0xFF3A, prF}, // Lu [26] FULLWIDTH LATIN CAPITAL LETTER A..FULLWIDTH LATIN CAPITAL LETTER Z
- {0xFF3B, 0xFF3B, prF}, // Ps FULLWIDTH LEFT SQUARE BRACKET
- {0xFF3C, 0xFF3C, prF}, // Po FULLWIDTH REVERSE SOLIDUS
- {0xFF3D, 0xFF3D, prF}, // Pe FULLWIDTH RIGHT SQUARE BRACKET
- {0xFF3E, 0xFF3E, prF}, // Sk FULLWIDTH CIRCUMFLEX ACCENT
- {0xFF3F, 0xFF3F, prF}, // Pc FULLWIDTH LOW LINE
- {0xFF40, 0xFF40, prF}, // Sk FULLWIDTH GRAVE ACCENT
- {0xFF41, 0xFF5A, prF}, // Ll [26] FULLWIDTH LATIN SMALL LETTER A..FULLWIDTH LATIN SMALL LETTER Z
- {0xFF5B, 0xFF5B, prF}, // Ps FULLWIDTH LEFT CURLY BRACKET
- {0xFF5C, 0xFF5C, prF}, // Sm FULLWIDTH VERTICAL LINE
- {0xFF5D, 0xFF5D, prF}, // Pe FULLWIDTH RIGHT CURLY BRACKET
- {0xFF5E, 0xFF5E, prF}, // Sm FULLWIDTH TILDE
- {0xFF5F, 0xFF5F, prF}, // Ps FULLWIDTH LEFT WHITE PARENTHESIS
- {0xFF60, 0xFF60, prF}, // Pe FULLWIDTH RIGHT WHITE PARENTHESIS
- {0xFF61, 0xFF61, prH}, // Po HALFWIDTH IDEOGRAPHIC FULL STOP
- {0xFF62, 0xFF62, prH}, // Ps HALFWIDTH LEFT CORNER BRACKET
- {0xFF63, 0xFF63, prH}, // Pe HALFWIDTH RIGHT CORNER BRACKET
- {0xFF64, 0xFF65, prH}, // Po [2] HALFWIDTH IDEOGRAPHIC COMMA..HALFWIDTH KATAKANA MIDDLE DOT
- {0xFF66, 0xFF6F, prH}, // Lo [10] HALFWIDTH KATAKANA LETTER WO..HALFWIDTH KATAKANA LETTER SMALL TU
- {0xFF70, 0xFF70, prH}, // Lm HALFWIDTH KATAKANA-HIRAGANA PROLONGED SOUND MARK
- {0xFF71, 0xFF9D, prH}, // Lo [45] HALFWIDTH KATAKANA LETTER A..HALFWIDTH KATAKANA LETTER N
- {0xFF9E, 0xFF9F, prH}, // Lm [2] HALFWIDTH KATAKANA VOICED SOUND MARK..HALFWIDTH KATAKANA SEMI-VOICED SOUND MARK
- {0xFFA0, 0xFFBE, prH}, // Lo [31] HALFWIDTH HANGUL FILLER..HALFWIDTH HANGUL LETTER HIEUH
- {0xFFC2, 0xFFC7, prH}, // Lo [6] HALFWIDTH HANGUL LETTER A..HALFWIDTH HANGUL LETTER E
- {0xFFCA, 0xFFCF, prH}, // Lo [6] HALFWIDTH HANGUL LETTER YEO..HALFWIDTH HANGUL LETTER OE
- {0xFFD2, 0xFFD7, prH}, // Lo [6] HALFWIDTH HANGUL LETTER YO..HALFWIDTH HANGUL LETTER YU
- {0xFFDA, 0xFFDC, prH}, // Lo [3] HALFWIDTH HANGUL LETTER EU..HALFWIDTH HANGUL LETTER I
- {0xFFE0, 0xFFE1, prF}, // Sc [2] FULLWIDTH CENT SIGN..FULLWIDTH POUND SIGN
- {0xFFE2, 0xFFE2, prF}, // Sm FULLWIDTH NOT SIGN
- {0xFFE3, 0xFFE3, prF}, // Sk FULLWIDTH MACRON
- {0xFFE4, 0xFFE4, prF}, // So FULLWIDTH BROKEN BAR
- {0xFFE5, 0xFFE6, prF}, // Sc [2] FULLWIDTH YEN SIGN..FULLWIDTH WON SIGN
- {0xFFE8, 0xFFE8, prH}, // So HALFWIDTH FORMS LIGHT VERTICAL
- {0xFFE9, 0xFFEC, prH}, // Sm [4] HALFWIDTH LEFTWARDS ARROW..HALFWIDTH DOWNWARDS ARROW
- {0xFFED, 0xFFEE, prH}, // So [2] HALFWIDTH BLACK SQUARE..HALFWIDTH WHITE CIRCLE
- {0xFFF9, 0xFFFB, prN}, // Cf [3] INTERLINEAR ANNOTATION ANCHOR..INTERLINEAR ANNOTATION TERMINATOR
- {0xFFFC, 0xFFFC, prN}, // So OBJECT REPLACEMENT CHARACTER
- {0xFFFD, 0xFFFD, prA}, // So REPLACEMENT CHARACTER
- {0x10000, 0x1000B, prN}, // Lo [12] LINEAR B SYLLABLE B008 A..LINEAR B SYLLABLE B046 JE
- {0x1000D, 0x10026, prN}, // Lo [26] LINEAR B SYLLABLE B036 JO..LINEAR B SYLLABLE B032 QO
- {0x10028, 0x1003A, prN}, // Lo [19] LINEAR B SYLLABLE B060 RA..LINEAR B SYLLABLE B042 WO
- {0x1003C, 0x1003D, prN}, // Lo [2] LINEAR B SYLLABLE B017 ZA..LINEAR B SYLLABLE B074 ZE
- {0x1003F, 0x1004D, prN}, // Lo [15] LINEAR B SYLLABLE B020 ZO..LINEAR B SYLLABLE B091 TWO
- {0x10050, 0x1005D, prN}, // Lo [14] LINEAR B SYMBOL B018..LINEAR B SYMBOL B089
- {0x10080, 0x100FA, prN}, // Lo [123] LINEAR B IDEOGRAM B100 MAN..LINEAR B IDEOGRAM VESSEL B305
- {0x10100, 0x10102, prN}, // Po [3] AEGEAN WORD SEPARATOR LINE..AEGEAN CHECK MARK
- {0x10107, 0x10133, prN}, // No [45] AEGEAN NUMBER ONE..AEGEAN NUMBER NINETY THOUSAND
- {0x10137, 0x1013F, prN}, // So [9] AEGEAN WEIGHT BASE UNIT..AEGEAN MEASURE THIRD SUBUNIT
- {0x10140, 0x10174, prN}, // Nl [53] GREEK ACROPHONIC ATTIC ONE QUARTER..GREEK ACROPHONIC STRATIAN FIFTY MNAS
- {0x10175, 0x10178, prN}, // No [4] GREEK ONE HALF SIGN..GREEK THREE QUARTERS SIGN
- {0x10179, 0x10189, prN}, // So [17] GREEK YEAR SIGN..GREEK TRYBLION BASE SIGN
- {0x1018A, 0x1018B, prN}, // No [2] GREEK ZERO SIGN..GREEK ONE QUARTER SIGN
- {0x1018C, 0x1018E, prN}, // So [3] GREEK SINUSOID SIGN..NOMISMA SIGN
- {0x10190, 0x1019C, prN}, // So [13] ROMAN SEXTANS SIGN..ASCIA SYMBOL
- {0x101A0, 0x101A0, prN}, // So GREEK SYMBOL TAU RHO
- {0x101D0, 0x101FC, prN}, // So [45] PHAISTOS DISC SIGN PEDESTRIAN..PHAISTOS DISC SIGN WAVY BAND
- {0x101FD, 0x101FD, prN}, // Mn PHAISTOS DISC SIGN COMBINING OBLIQUE STROKE
- {0x10280, 0x1029C, prN}, // Lo [29] LYCIAN LETTER A..LYCIAN LETTER X
- {0x102A0, 0x102D0, prN}, // Lo [49] CARIAN LETTER A..CARIAN LETTER UUU3
- {0x102E0, 0x102E0, prN}, // Mn COPTIC EPACT THOUSANDS MARK
- {0x102E1, 0x102FB, prN}, // No [27] COPTIC EPACT DIGIT ONE..COPTIC EPACT NUMBER NINE HUNDRED
- {0x10300, 0x1031F, prN}, // Lo [32] OLD ITALIC LETTER A..OLD ITALIC LETTER ESS
- {0x10320, 0x10323, prN}, // No [4] OLD ITALIC NUMERAL ONE..OLD ITALIC NUMERAL FIFTY
- {0x1032D, 0x1032F, prN}, // Lo [3] OLD ITALIC LETTER YE..OLD ITALIC LETTER SOUTHERN TSE
- {0x10330, 0x10340, prN}, // Lo [17] GOTHIC LETTER AHSA..GOTHIC LETTER PAIRTHRA
- {0x10341, 0x10341, prN}, // Nl GOTHIC LETTER NINETY
- {0x10342, 0x10349, prN}, // Lo [8] GOTHIC LETTER RAIDA..GOTHIC LETTER OTHAL
- {0x1034A, 0x1034A, prN}, // Nl GOTHIC LETTER NINE HUNDRED
- {0x10350, 0x10375, prN}, // Lo [38] OLD PERMIC LETTER AN..OLD PERMIC LETTER IA
- {0x10376, 0x1037A, prN}, // Mn [5] COMBINING OLD PERMIC LETTER AN..COMBINING OLD PERMIC LETTER SII
- {0x10380, 0x1039D, prN}, // Lo [30] UGARITIC LETTER ALPA..UGARITIC LETTER SSU
- {0x1039F, 0x1039F, prN}, // Po UGARITIC WORD DIVIDER
- {0x103A0, 0x103C3, prN}, // Lo [36] OLD PERSIAN SIGN A..OLD PERSIAN SIGN HA
- {0x103C8, 0x103CF, prN}, // Lo [8] OLD PERSIAN SIGN AURAMAZDAA..OLD PERSIAN SIGN BUUMISH
- {0x103D0, 0x103D0, prN}, // Po OLD PERSIAN WORD DIVIDER
- {0x103D1, 0x103D5, prN}, // Nl [5] OLD PERSIAN NUMBER ONE..OLD PERSIAN NUMBER HUNDRED
- {0x10400, 0x1044F, prN}, // L& [80] DESERET CAPITAL LETTER LONG I..DESERET SMALL LETTER EW
- {0x10450, 0x1047F, prN}, // Lo [48] SHAVIAN LETTER PEEP..SHAVIAN LETTER YEW
- {0x10480, 0x1049D, prN}, // Lo [30] OSMANYA LETTER ALEF..OSMANYA LETTER OO
- {0x104A0, 0x104A9, prN}, // Nd [10] OSMANYA DIGIT ZERO..OSMANYA DIGIT NINE
- {0x104B0, 0x104D3, prN}, // Lu [36] OSAGE CAPITAL LETTER A..OSAGE CAPITAL LETTER ZHA
- {0x104D8, 0x104FB, prN}, // Ll [36] OSAGE SMALL LETTER A..OSAGE SMALL LETTER ZHA
- {0x10500, 0x10527, prN}, // Lo [40] ELBASAN LETTER A..ELBASAN LETTER KHE
- {0x10530, 0x10563, prN}, // Lo [52] CAUCASIAN ALBANIAN LETTER ALT..CAUCASIAN ALBANIAN LETTER KIW
- {0x1056F, 0x1056F, prN}, // Po CAUCASIAN ALBANIAN CITATION MARK
- {0x10570, 0x1057A, prN}, // Lu [11] VITHKUQI CAPITAL LETTER A..VITHKUQI CAPITAL LETTER GA
- {0x1057C, 0x1058A, prN}, // Lu [15] VITHKUQI CAPITAL LETTER HA..VITHKUQI CAPITAL LETTER RE
- {0x1058C, 0x10592, prN}, // Lu [7] VITHKUQI CAPITAL LETTER SE..VITHKUQI CAPITAL LETTER XE
- {0x10594, 0x10595, prN}, // Lu [2] VITHKUQI CAPITAL LETTER Y..VITHKUQI CAPITAL LETTER ZE
- {0x10597, 0x105A1, prN}, // Ll [11] VITHKUQI SMALL LETTER A..VITHKUQI SMALL LETTER GA
- {0x105A3, 0x105B1, prN}, // Ll [15] VITHKUQI SMALL LETTER HA..VITHKUQI SMALL LETTER RE
- {0x105B3, 0x105B9, prN}, // Ll [7] VITHKUQI SMALL LETTER SE..VITHKUQI SMALL LETTER XE
- {0x105BB, 0x105BC, prN}, // Ll [2] VITHKUQI SMALL LETTER Y..VITHKUQI SMALL LETTER ZE
- {0x10600, 0x10736, prN}, // Lo [311] LINEAR A SIGN AB001..LINEAR A SIGN A664
- {0x10740, 0x10755, prN}, // Lo [22] LINEAR A SIGN A701 A..LINEAR A SIGN A732 JE
- {0x10760, 0x10767, prN}, // Lo [8] LINEAR A SIGN A800..LINEAR A SIGN A807
- {0x10780, 0x10785, prN}, // Lm [6] MODIFIER LETTER SMALL CAPITAL AA..MODIFIER LETTER SMALL B WITH HOOK
- {0x10787, 0x107B0, prN}, // Lm [42] MODIFIER LETTER SMALL DZ DIGRAPH..MODIFIER LETTER SMALL V WITH RIGHT HOOK
- {0x107B2, 0x107BA, prN}, // Lm [9] MODIFIER LETTER SMALL CAPITAL Y..MODIFIER LETTER SMALL S WITH CURL
- {0x10800, 0x10805, prN}, // Lo [6] CYPRIOT SYLLABLE A..CYPRIOT SYLLABLE JA
- {0x10808, 0x10808, prN}, // Lo CYPRIOT SYLLABLE JO
- {0x1080A, 0x10835, prN}, // Lo [44] CYPRIOT SYLLABLE KA..CYPRIOT SYLLABLE WO
- {0x10837, 0x10838, prN}, // Lo [2] CYPRIOT SYLLABLE XA..CYPRIOT SYLLABLE XE
- {0x1083C, 0x1083C, prN}, // Lo CYPRIOT SYLLABLE ZA
- {0x1083F, 0x1083F, prN}, // Lo CYPRIOT SYLLABLE ZO
- {0x10840, 0x10855, prN}, // Lo [22] IMPERIAL ARAMAIC LETTER ALEPH..IMPERIAL ARAMAIC LETTER TAW
- {0x10857, 0x10857, prN}, // Po IMPERIAL ARAMAIC SECTION SIGN
- {0x10858, 0x1085F, prN}, // No [8] IMPERIAL ARAMAIC NUMBER ONE..IMPERIAL ARAMAIC NUMBER TEN THOUSAND
- {0x10860, 0x10876, prN}, // Lo [23] PALMYRENE LETTER ALEPH..PALMYRENE LETTER TAW
- {0x10877, 0x10878, prN}, // So [2] PALMYRENE LEFT-POINTING FLEURON..PALMYRENE RIGHT-POINTING FLEURON
- {0x10879, 0x1087F, prN}, // No [7] PALMYRENE NUMBER ONE..PALMYRENE NUMBER TWENTY
- {0x10880, 0x1089E, prN}, // Lo [31] NABATAEAN LETTER FINAL ALEPH..NABATAEAN LETTER TAW
- {0x108A7, 0x108AF, prN}, // No [9] NABATAEAN NUMBER ONE..NABATAEAN NUMBER ONE HUNDRED
- {0x108E0, 0x108F2, prN}, // Lo [19] HATRAN LETTER ALEPH..HATRAN LETTER QOPH
- {0x108F4, 0x108F5, prN}, // Lo [2] HATRAN LETTER SHIN..HATRAN LETTER TAW
- {0x108FB, 0x108FF, prN}, // No [5] HATRAN NUMBER ONE..HATRAN NUMBER ONE HUNDRED
- {0x10900, 0x10915, prN}, // Lo [22] PHOENICIAN LETTER ALF..PHOENICIAN LETTER TAU
- {0x10916, 0x1091B, prN}, // No [6] PHOENICIAN NUMBER ONE..PHOENICIAN NUMBER THREE
- {0x1091F, 0x1091F, prN}, // Po PHOENICIAN WORD SEPARATOR
- {0x10920, 0x10939, prN}, // Lo [26] LYDIAN LETTER A..LYDIAN LETTER C
- {0x1093F, 0x1093F, prN}, // Po LYDIAN TRIANGULAR MARK
- {0x10980, 0x1099F, prN}, // Lo [32] MEROITIC HIEROGLYPHIC LETTER A..MEROITIC HIEROGLYPHIC SYMBOL VIDJ-2
- {0x109A0, 0x109B7, prN}, // Lo [24] MEROITIC CURSIVE LETTER A..MEROITIC CURSIVE LETTER DA
- {0x109BC, 0x109BD, prN}, // No [2] MEROITIC CURSIVE FRACTION ELEVEN TWELFTHS..MEROITIC CURSIVE FRACTION ONE HALF
- {0x109BE, 0x109BF, prN}, // Lo [2] MEROITIC CURSIVE LOGOGRAM RMT..MEROITIC CURSIVE LOGOGRAM IMN
- {0x109C0, 0x109CF, prN}, // No [16] MEROITIC CURSIVE NUMBER ONE..MEROITIC CURSIVE NUMBER SEVENTY
- {0x109D2, 0x109FF, prN}, // No [46] MEROITIC CURSIVE NUMBER ONE HUNDRED..MEROITIC CURSIVE FRACTION TEN TWELFTHS
- {0x10A00, 0x10A00, prN}, // Lo KHAROSHTHI LETTER A
- {0x10A01, 0x10A03, prN}, // Mn [3] KHAROSHTHI VOWEL SIGN I..KHAROSHTHI VOWEL SIGN VOCALIC R
- {0x10A05, 0x10A06, prN}, // Mn [2] KHAROSHTHI VOWEL SIGN E..KHAROSHTHI VOWEL SIGN O
- {0x10A0C, 0x10A0F, prN}, // Mn [4] KHAROSHTHI VOWEL LENGTH MARK..KHAROSHTHI SIGN VISARGA
- {0x10A10, 0x10A13, prN}, // Lo [4] KHAROSHTHI LETTER KA..KHAROSHTHI LETTER GHA
- {0x10A15, 0x10A17, prN}, // Lo [3] KHAROSHTHI LETTER CA..KHAROSHTHI LETTER JA
- {0x10A19, 0x10A35, prN}, // Lo [29] KHAROSHTHI LETTER NYA..KHAROSHTHI LETTER VHA
- {0x10A38, 0x10A3A, prN}, // Mn [3] KHAROSHTHI SIGN BAR ABOVE..KHAROSHTHI SIGN DOT BELOW
- {0x10A3F, 0x10A3F, prN}, // Mn KHAROSHTHI VIRAMA
- {0x10A40, 0x10A48, prN}, // No [9] KHAROSHTHI DIGIT ONE..KHAROSHTHI FRACTION ONE HALF
- {0x10A50, 0x10A58, prN}, // Po [9] KHAROSHTHI PUNCTUATION DOT..KHAROSHTHI PUNCTUATION LINES
- {0x10A60, 0x10A7C, prN}, // Lo [29] OLD SOUTH ARABIAN LETTER HE..OLD SOUTH ARABIAN LETTER THETH
- {0x10A7D, 0x10A7E, prN}, // No [2] OLD SOUTH ARABIAN NUMBER ONE..OLD SOUTH ARABIAN NUMBER FIFTY
- {0x10A7F, 0x10A7F, prN}, // Po OLD SOUTH ARABIAN NUMERIC INDICATOR
- {0x10A80, 0x10A9C, prN}, // Lo [29] OLD NORTH ARABIAN LETTER HEH..OLD NORTH ARABIAN LETTER ZAH
- {0x10A9D, 0x10A9F, prN}, // No [3] OLD NORTH ARABIAN NUMBER ONE..OLD NORTH ARABIAN NUMBER TWENTY
- {0x10AC0, 0x10AC7, prN}, // Lo [8] MANICHAEAN LETTER ALEPH..MANICHAEAN LETTER WAW
- {0x10AC8, 0x10AC8, prN}, // So MANICHAEAN SIGN UD
- {0x10AC9, 0x10AE4, prN}, // Lo [28] MANICHAEAN LETTER ZAYIN..MANICHAEAN LETTER TAW
- {0x10AE5, 0x10AE6, prN}, // Mn [2] MANICHAEAN ABBREVIATION MARK ABOVE..MANICHAEAN ABBREVIATION MARK BELOW
- {0x10AEB, 0x10AEF, prN}, // No [5] MANICHAEAN NUMBER ONE..MANICHAEAN NUMBER ONE HUNDRED
- {0x10AF0, 0x10AF6, prN}, // Po [7] MANICHAEAN PUNCTUATION STAR..MANICHAEAN PUNCTUATION LINE FILLER
- {0x10B00, 0x10B35, prN}, // Lo [54] AVESTAN LETTER A..AVESTAN LETTER HE
- {0x10B39, 0x10B3F, prN}, // Po [7] AVESTAN ABBREVIATION MARK..LARGE ONE RING OVER TWO RINGS PUNCTUATION
- {0x10B40, 0x10B55, prN}, // Lo [22] INSCRIPTIONAL PARTHIAN LETTER ALEPH..INSCRIPTIONAL PARTHIAN LETTER TAW
- {0x10B58, 0x10B5F, prN}, // No [8] INSCRIPTIONAL PARTHIAN NUMBER ONE..INSCRIPTIONAL PARTHIAN NUMBER ONE THOUSAND
- {0x10B60, 0x10B72, prN}, // Lo [19] INSCRIPTIONAL PAHLAVI LETTER ALEPH..INSCRIPTIONAL PAHLAVI LETTER TAW
- {0x10B78, 0x10B7F, prN}, // No [8] INSCRIPTIONAL PAHLAVI NUMBER ONE..INSCRIPTIONAL PAHLAVI NUMBER ONE THOUSAND
- {0x10B80, 0x10B91, prN}, // Lo [18] PSALTER PAHLAVI LETTER ALEPH..PSALTER PAHLAVI LETTER TAW
- {0x10B99, 0x10B9C, prN}, // Po [4] PSALTER PAHLAVI SECTION MARK..PSALTER PAHLAVI FOUR DOTS WITH DOT
- {0x10BA9, 0x10BAF, prN}, // No [7] PSALTER PAHLAVI NUMBER ONE..PSALTER PAHLAVI NUMBER ONE HUNDRED
- {0x10C00, 0x10C48, prN}, // Lo [73] OLD TURKIC LETTER ORKHON A..OLD TURKIC LETTER ORKHON BASH
- {0x10C80, 0x10CB2, prN}, // Lu [51] OLD HUNGARIAN CAPITAL LETTER A..OLD HUNGARIAN CAPITAL LETTER US
- {0x10CC0, 0x10CF2, prN}, // Ll [51] OLD HUNGARIAN SMALL LETTER A..OLD HUNGARIAN SMALL LETTER US
- {0x10CFA, 0x10CFF, prN}, // No [6] OLD HUNGARIAN NUMBER ONE..OLD HUNGARIAN NUMBER ONE THOUSAND
- {0x10D00, 0x10D23, prN}, // Lo [36] HANIFI ROHINGYA LETTER A..HANIFI ROHINGYA MARK NA KHONNA
- {0x10D24, 0x10D27, prN}, // Mn [4] HANIFI ROHINGYA SIGN HARBAHAY..HANIFI ROHINGYA SIGN TASSI
- {0x10D30, 0x10D39, prN}, // Nd [10] HANIFI ROHINGYA DIGIT ZERO..HANIFI ROHINGYA DIGIT NINE
- {0x10E60, 0x10E7E, prN}, // No [31] RUMI DIGIT ONE..RUMI FRACTION TWO THIRDS
- {0x10E80, 0x10EA9, prN}, // Lo [42] YEZIDI LETTER ELIF..YEZIDI LETTER ET
- {0x10EAB, 0x10EAC, prN}, // Mn [2] YEZIDI COMBINING HAMZA MARK..YEZIDI COMBINING MADDA MARK
- {0x10EAD, 0x10EAD, prN}, // Pd YEZIDI HYPHENATION MARK
- {0x10EB0, 0x10EB1, prN}, // Lo [2] YEZIDI LETTER LAM WITH DOT ABOVE..YEZIDI LETTER YOT WITH CIRCUMFLEX ABOVE
- {0x10F00, 0x10F1C, prN}, // Lo [29] OLD SOGDIAN LETTER ALEPH..OLD SOGDIAN LETTER FINAL TAW WITH VERTICAL TAIL
- {0x10F1D, 0x10F26, prN}, // No [10] OLD SOGDIAN NUMBER ONE..OLD SOGDIAN FRACTION ONE HALF
- {0x10F27, 0x10F27, prN}, // Lo OLD SOGDIAN LIGATURE AYIN-DALETH
- {0x10F30, 0x10F45, prN}, // Lo [22] SOGDIAN LETTER ALEPH..SOGDIAN INDEPENDENT SHIN
- {0x10F46, 0x10F50, prN}, // Mn [11] SOGDIAN COMBINING DOT BELOW..SOGDIAN COMBINING STROKE BELOW
- {0x10F51, 0x10F54, prN}, // No [4] SOGDIAN NUMBER ONE..SOGDIAN NUMBER ONE HUNDRED
- {0x10F55, 0x10F59, prN}, // Po [5] SOGDIAN PUNCTUATION TWO VERTICAL BARS..SOGDIAN PUNCTUATION HALF CIRCLE WITH DOT
- {0x10F70, 0x10F81, prN}, // Lo [18] OLD UYGHUR LETTER ALEPH..OLD UYGHUR LETTER LESH
- {0x10F82, 0x10F85, prN}, // Mn [4] OLD UYGHUR COMBINING DOT ABOVE..OLD UYGHUR COMBINING TWO DOTS BELOW
- {0x10F86, 0x10F89, prN}, // Po [4] OLD UYGHUR PUNCTUATION BAR..OLD UYGHUR PUNCTUATION FOUR DOTS
- {0x10FB0, 0x10FC4, prN}, // Lo [21] CHORASMIAN LETTER ALEPH..CHORASMIAN LETTER TAW
- {0x10FC5, 0x10FCB, prN}, // No [7] CHORASMIAN NUMBER ONE..CHORASMIAN NUMBER ONE HUNDRED
- {0x10FE0, 0x10FF6, prN}, // Lo [23] ELYMAIC LETTER ALEPH..ELYMAIC LIGATURE ZAYIN-YODH
- {0x11000, 0x11000, prN}, // Mc BRAHMI SIGN CANDRABINDU
- {0x11001, 0x11001, prN}, // Mn BRAHMI SIGN ANUSVARA
- {0x11002, 0x11002, prN}, // Mc BRAHMI SIGN VISARGA
- {0x11003, 0x11037, prN}, // Lo [53] BRAHMI SIGN JIHVAMULIYA..BRAHMI LETTER OLD TAMIL NNNA
- {0x11038, 0x11046, prN}, // Mn [15] BRAHMI VOWEL SIGN AA..BRAHMI VIRAMA
- {0x11047, 0x1104D, prN}, // Po [7] BRAHMI DANDA..BRAHMI PUNCTUATION LOTUS
- {0x11052, 0x11065, prN}, // No [20] BRAHMI NUMBER ONE..BRAHMI NUMBER ONE THOUSAND
- {0x11066, 0x1106F, prN}, // Nd [10] BRAHMI DIGIT ZERO..BRAHMI DIGIT NINE
- {0x11070, 0x11070, prN}, // Mn BRAHMI SIGN OLD TAMIL VIRAMA
- {0x11071, 0x11072, prN}, // Lo [2] BRAHMI LETTER OLD TAMIL SHORT E..BRAHMI LETTER OLD TAMIL SHORT O
- {0x11073, 0x11074, prN}, // Mn [2] BRAHMI VOWEL SIGN OLD TAMIL SHORT E..BRAHMI VOWEL SIGN OLD TAMIL SHORT O
- {0x11075, 0x11075, prN}, // Lo BRAHMI LETTER OLD TAMIL LLA
- {0x1107F, 0x1107F, prN}, // Mn BRAHMI NUMBER JOINER
- {0x11080, 0x11081, prN}, // Mn [2] KAITHI SIGN CANDRABINDU..KAITHI SIGN ANUSVARA
- {0x11082, 0x11082, prN}, // Mc KAITHI SIGN VISARGA
- {0x11083, 0x110AF, prN}, // Lo [45] KAITHI LETTER A..KAITHI LETTER HA
- {0x110B0, 0x110B2, prN}, // Mc [3] KAITHI VOWEL SIGN AA..KAITHI VOWEL SIGN II
- {0x110B3, 0x110B6, prN}, // Mn [4] KAITHI VOWEL SIGN U..KAITHI VOWEL SIGN AI
- {0x110B7, 0x110B8, prN}, // Mc [2] KAITHI VOWEL SIGN O..KAITHI VOWEL SIGN AU
- {0x110B9, 0x110BA, prN}, // Mn [2] KAITHI SIGN VIRAMA..KAITHI SIGN NUKTA
- {0x110BB, 0x110BC, prN}, // Po [2] KAITHI ABBREVIATION SIGN..KAITHI ENUMERATION SIGN
- {0x110BD, 0x110BD, prN}, // Cf KAITHI NUMBER SIGN
- {0x110BE, 0x110C1, prN}, // Po [4] KAITHI SECTION MARK..KAITHI DOUBLE DANDA
- {0x110C2, 0x110C2, prN}, // Mn KAITHI VOWEL SIGN VOCALIC R
- {0x110CD, 0x110CD, prN}, // Cf KAITHI NUMBER SIGN ABOVE
- {0x110D0, 0x110E8, prN}, // Lo [25] SORA SOMPENG LETTER SAH..SORA SOMPENG LETTER MAE
- {0x110F0, 0x110F9, prN}, // Nd [10] SORA SOMPENG DIGIT ZERO..SORA SOMPENG DIGIT NINE
- {0x11100, 0x11102, prN}, // Mn [3] CHAKMA SIGN CANDRABINDU..CHAKMA SIGN VISARGA
- {0x11103, 0x11126, prN}, // Lo [36] CHAKMA LETTER AA..CHAKMA LETTER HAA
- {0x11127, 0x1112B, prN}, // Mn [5] CHAKMA VOWEL SIGN A..CHAKMA VOWEL SIGN UU
- {0x1112C, 0x1112C, prN}, // Mc CHAKMA VOWEL SIGN E
- {0x1112D, 0x11134, prN}, // Mn [8] CHAKMA VOWEL SIGN AI..CHAKMA MAAYYAA
- {0x11136, 0x1113F, prN}, // Nd [10] CHAKMA DIGIT ZERO..CHAKMA DIGIT NINE
- {0x11140, 0x11143, prN}, // Po [4] CHAKMA SECTION MARK..CHAKMA QUESTION MARK
- {0x11144, 0x11144, prN}, // Lo CHAKMA LETTER LHAA
- {0x11145, 0x11146, prN}, // Mc [2] CHAKMA VOWEL SIGN AA..CHAKMA VOWEL SIGN EI
- {0x11147, 0x11147, prN}, // Lo CHAKMA LETTER VAA
- {0x11150, 0x11172, prN}, // Lo [35] MAHAJANI LETTER A..MAHAJANI LETTER RRA
- {0x11173, 0x11173, prN}, // Mn MAHAJANI SIGN NUKTA
- {0x11174, 0x11175, prN}, // Po [2] MAHAJANI ABBREVIATION SIGN..MAHAJANI SECTION MARK
- {0x11176, 0x11176, prN}, // Lo MAHAJANI LIGATURE SHRI
- {0x11180, 0x11181, prN}, // Mn [2] SHARADA SIGN CANDRABINDU..SHARADA SIGN ANUSVARA
- {0x11182, 0x11182, prN}, // Mc SHARADA SIGN VISARGA
- {0x11183, 0x111B2, prN}, // Lo [48] SHARADA LETTER A..SHARADA LETTER HA
- {0x111B3, 0x111B5, prN}, // Mc [3] SHARADA VOWEL SIGN AA..SHARADA VOWEL SIGN II
- {0x111B6, 0x111BE, prN}, // Mn [9] SHARADA VOWEL SIGN U..SHARADA VOWEL SIGN O
- {0x111BF, 0x111C0, prN}, // Mc [2] SHARADA VOWEL SIGN AU..SHARADA SIGN VIRAMA
- {0x111C1, 0x111C4, prN}, // Lo [4] SHARADA SIGN AVAGRAHA..SHARADA OM
- {0x111C5, 0x111C8, prN}, // Po [4] SHARADA DANDA..SHARADA SEPARATOR
- {0x111C9, 0x111CC, prN}, // Mn [4] SHARADA SANDHI MARK..SHARADA EXTRA SHORT VOWEL MARK
- {0x111CD, 0x111CD, prN}, // Po SHARADA SUTRA MARK
- {0x111CE, 0x111CE, prN}, // Mc SHARADA VOWEL SIGN PRISHTHAMATRA E
- {0x111CF, 0x111CF, prN}, // Mn SHARADA SIGN INVERTED CANDRABINDU
- {0x111D0, 0x111D9, prN}, // Nd [10] SHARADA DIGIT ZERO..SHARADA DIGIT NINE
- {0x111DA, 0x111DA, prN}, // Lo SHARADA EKAM
- {0x111DB, 0x111DB, prN}, // Po SHARADA SIGN SIDDHAM
- {0x111DC, 0x111DC, prN}, // Lo SHARADA HEADSTROKE
- {0x111DD, 0x111DF, prN}, // Po [3] SHARADA CONTINUATION SIGN..SHARADA SECTION MARK-2
- {0x111E1, 0x111F4, prN}, // No [20] SINHALA ARCHAIC DIGIT ONE..SINHALA ARCHAIC NUMBER ONE THOUSAND
- {0x11200, 0x11211, prN}, // Lo [18] KHOJKI LETTER A..KHOJKI LETTER JJA
- {0x11213, 0x1122B, prN}, // Lo [25] KHOJKI LETTER NYA..KHOJKI LETTER LLA
- {0x1122C, 0x1122E, prN}, // Mc [3] KHOJKI VOWEL SIGN AA..KHOJKI VOWEL SIGN II
- {0x1122F, 0x11231, prN}, // Mn [3] KHOJKI VOWEL SIGN U..KHOJKI VOWEL SIGN AI
- {0x11232, 0x11233, prN}, // Mc [2] KHOJKI VOWEL SIGN O..KHOJKI VOWEL SIGN AU
- {0x11234, 0x11234, prN}, // Mn KHOJKI SIGN ANUSVARA
- {0x11235, 0x11235, prN}, // Mc KHOJKI SIGN VIRAMA
- {0x11236, 0x11237, prN}, // Mn [2] KHOJKI SIGN NUKTA..KHOJKI SIGN SHADDA
- {0x11238, 0x1123D, prN}, // Po [6] KHOJKI DANDA..KHOJKI ABBREVIATION SIGN
- {0x1123E, 0x1123E, prN}, // Mn KHOJKI SIGN SUKUN
- {0x11280, 0x11286, prN}, // Lo [7] MULTANI LETTER A..MULTANI LETTER GA
- {0x11288, 0x11288, prN}, // Lo MULTANI LETTER GHA
- {0x1128A, 0x1128D, prN}, // Lo [4] MULTANI LETTER CA..MULTANI LETTER JJA
- {0x1128F, 0x1129D, prN}, // Lo [15] MULTANI LETTER NYA..MULTANI LETTER BA
- {0x1129F, 0x112A8, prN}, // Lo [10] MULTANI LETTER BHA..MULTANI LETTER RHA
- {0x112A9, 0x112A9, prN}, // Po MULTANI SECTION MARK
- {0x112B0, 0x112DE, prN}, // Lo [47] KHUDAWADI LETTER A..KHUDAWADI LETTER HA
- {0x112DF, 0x112DF, prN}, // Mn KHUDAWADI SIGN ANUSVARA
- {0x112E0, 0x112E2, prN}, // Mc [3] KHUDAWADI VOWEL SIGN AA..KHUDAWADI VOWEL SIGN II
- {0x112E3, 0x112EA, prN}, // Mn [8] KHUDAWADI VOWEL SIGN U..KHUDAWADI SIGN VIRAMA
- {0x112F0, 0x112F9, prN}, // Nd [10] KHUDAWADI DIGIT ZERO..KHUDAWADI DIGIT NINE
- {0x11300, 0x11301, prN}, // Mn [2] GRANTHA SIGN COMBINING ANUSVARA ABOVE..GRANTHA SIGN CANDRABINDU
- {0x11302, 0x11303, prN}, // Mc [2] GRANTHA SIGN ANUSVARA..GRANTHA SIGN VISARGA
- {0x11305, 0x1130C, prN}, // Lo [8] GRANTHA LETTER A..GRANTHA LETTER VOCALIC L
- {0x1130F, 0x11310, prN}, // Lo [2] GRANTHA LETTER EE..GRANTHA LETTER AI
- {0x11313, 0x11328, prN}, // Lo [22] GRANTHA LETTER OO..GRANTHA LETTER NA
- {0x1132A, 0x11330, prN}, // Lo [7] GRANTHA LETTER PA..GRANTHA LETTER RA
- {0x11332, 0x11333, prN}, // Lo [2] GRANTHA LETTER LA..GRANTHA LETTER LLA
- {0x11335, 0x11339, prN}, // Lo [5] GRANTHA LETTER VA..GRANTHA LETTER HA
- {0x1133B, 0x1133C, prN}, // Mn [2] COMBINING BINDU BELOW..GRANTHA SIGN NUKTA
- {0x1133D, 0x1133D, prN}, // Lo GRANTHA SIGN AVAGRAHA
- {0x1133E, 0x1133F, prN}, // Mc [2] GRANTHA VOWEL SIGN AA..GRANTHA VOWEL SIGN I
- {0x11340, 0x11340, prN}, // Mn GRANTHA VOWEL SIGN II
- {0x11341, 0x11344, prN}, // Mc [4] GRANTHA VOWEL SIGN U..GRANTHA VOWEL SIGN VOCALIC RR
- {0x11347, 0x11348, prN}, // Mc [2] GRANTHA VOWEL SIGN EE..GRANTHA VOWEL SIGN AI
- {0x1134B, 0x1134D, prN}, // Mc [3] GRANTHA VOWEL SIGN OO..GRANTHA SIGN VIRAMA
- {0x11350, 0x11350, prN}, // Lo GRANTHA OM
- {0x11357, 0x11357, prN}, // Mc GRANTHA AU LENGTH MARK
- {0x1135D, 0x11361, prN}, // Lo [5] GRANTHA SIGN PLUTA..GRANTHA LETTER VOCALIC LL
- {0x11362, 0x11363, prN}, // Mc [2] GRANTHA VOWEL SIGN VOCALIC L..GRANTHA VOWEL SIGN VOCALIC LL
- {0x11366, 0x1136C, prN}, // Mn [7] COMBINING GRANTHA DIGIT ZERO..COMBINING GRANTHA DIGIT SIX
- {0x11370, 0x11374, prN}, // Mn [5] COMBINING GRANTHA LETTER A..COMBINING GRANTHA LETTER PA
- {0x11400, 0x11434, prN}, // Lo [53] NEWA LETTER A..NEWA LETTER HA
- {0x11435, 0x11437, prN}, // Mc [3] NEWA VOWEL SIGN AA..NEWA VOWEL SIGN II
- {0x11438, 0x1143F, prN}, // Mn [8] NEWA VOWEL SIGN U..NEWA VOWEL SIGN AI
- {0x11440, 0x11441, prN}, // Mc [2] NEWA VOWEL SIGN O..NEWA VOWEL SIGN AU
- {0x11442, 0x11444, prN}, // Mn [3] NEWA SIGN VIRAMA..NEWA SIGN ANUSVARA
- {0x11445, 0x11445, prN}, // Mc NEWA SIGN VISARGA
- {0x11446, 0x11446, prN}, // Mn NEWA SIGN NUKTA
- {0x11447, 0x1144A, prN}, // Lo [4] NEWA SIGN AVAGRAHA..NEWA SIDDHI
- {0x1144B, 0x1144F, prN}, // Po [5] NEWA DANDA..NEWA ABBREVIATION SIGN
- {0x11450, 0x11459, prN}, // Nd [10] NEWA DIGIT ZERO..NEWA DIGIT NINE
- {0x1145A, 0x1145B, prN}, // Po [2] NEWA DOUBLE COMMA..NEWA PLACEHOLDER MARK
- {0x1145D, 0x1145D, prN}, // Po NEWA INSERTION SIGN
- {0x1145E, 0x1145E, prN}, // Mn NEWA SANDHI MARK
- {0x1145F, 0x11461, prN}, // Lo [3] NEWA LETTER VEDIC ANUSVARA..NEWA SIGN UPADHMANIYA
- {0x11480, 0x114AF, prN}, // Lo [48] TIRHUTA ANJI..TIRHUTA LETTER HA
- {0x114B0, 0x114B2, prN}, // Mc [3] TIRHUTA VOWEL SIGN AA..TIRHUTA VOWEL SIGN II
- {0x114B3, 0x114B8, prN}, // Mn [6] TIRHUTA VOWEL SIGN U..TIRHUTA VOWEL SIGN VOCALIC LL
- {0x114B9, 0x114B9, prN}, // Mc TIRHUTA VOWEL SIGN E
- {0x114BA, 0x114BA, prN}, // Mn TIRHUTA VOWEL SIGN SHORT E
- {0x114BB, 0x114BE, prN}, // Mc [4] TIRHUTA VOWEL SIGN AI..TIRHUTA VOWEL SIGN AU
- {0x114BF, 0x114C0, prN}, // Mn [2] TIRHUTA SIGN CANDRABINDU..TIRHUTA SIGN ANUSVARA
- {0x114C1, 0x114C1, prN}, // Mc TIRHUTA SIGN VISARGA
- {0x114C2, 0x114C3, prN}, // Mn [2] TIRHUTA SIGN VIRAMA..TIRHUTA SIGN NUKTA
- {0x114C4, 0x114C5, prN}, // Lo [2] TIRHUTA SIGN AVAGRAHA..TIRHUTA GVANG
- {0x114C6, 0x114C6, prN}, // Po TIRHUTA ABBREVIATION SIGN
- {0x114C7, 0x114C7, prN}, // Lo TIRHUTA OM
- {0x114D0, 0x114D9, prN}, // Nd [10] TIRHUTA DIGIT ZERO..TIRHUTA DIGIT NINE
- {0x11580, 0x115AE, prN}, // Lo [47] SIDDHAM LETTER A..SIDDHAM LETTER HA
- {0x115AF, 0x115B1, prN}, // Mc [3] SIDDHAM VOWEL SIGN AA..SIDDHAM VOWEL SIGN II
- {0x115B2, 0x115B5, prN}, // Mn [4] SIDDHAM VOWEL SIGN U..SIDDHAM VOWEL SIGN VOCALIC RR
- {0x115B8, 0x115BB, prN}, // Mc [4] SIDDHAM VOWEL SIGN E..SIDDHAM VOWEL SIGN AU
- {0x115BC, 0x115BD, prN}, // Mn [2] SIDDHAM SIGN CANDRABINDU..SIDDHAM SIGN ANUSVARA
- {0x115BE, 0x115BE, prN}, // Mc SIDDHAM SIGN VISARGA
- {0x115BF, 0x115C0, prN}, // Mn [2] SIDDHAM SIGN VIRAMA..SIDDHAM SIGN NUKTA
- {0x115C1, 0x115D7, prN}, // Po [23] SIDDHAM SIGN SIDDHAM..SIDDHAM SECTION MARK WITH CIRCLES AND FOUR ENCLOSURES
- {0x115D8, 0x115DB, prN}, // Lo [4] SIDDHAM LETTER THREE-CIRCLE ALTERNATE I..SIDDHAM LETTER ALTERNATE U
- {0x115DC, 0x115DD, prN}, // Mn [2] SIDDHAM VOWEL SIGN ALTERNATE U..SIDDHAM VOWEL SIGN ALTERNATE UU
- {0x11600, 0x1162F, prN}, // Lo [48] MODI LETTER A..MODI LETTER LLA
- {0x11630, 0x11632, prN}, // Mc [3] MODI VOWEL SIGN AA..MODI VOWEL SIGN II
- {0x11633, 0x1163A, prN}, // Mn [8] MODI VOWEL SIGN U..MODI VOWEL SIGN AI
- {0x1163B, 0x1163C, prN}, // Mc [2] MODI VOWEL SIGN O..MODI VOWEL SIGN AU
- {0x1163D, 0x1163D, prN}, // Mn MODI SIGN ANUSVARA
- {0x1163E, 0x1163E, prN}, // Mc MODI SIGN VISARGA
- {0x1163F, 0x11640, prN}, // Mn [2] MODI SIGN VIRAMA..MODI SIGN ARDHACANDRA
- {0x11641, 0x11643, prN}, // Po [3] MODI DANDA..MODI ABBREVIATION SIGN
- {0x11644, 0x11644, prN}, // Lo MODI SIGN HUVA
- {0x11650, 0x11659, prN}, // Nd [10] MODI DIGIT ZERO..MODI DIGIT NINE
- {0x11660, 0x1166C, prN}, // Po [13] MONGOLIAN BIRGA WITH ORNAMENT..MONGOLIAN TURNED SWIRL BIRGA WITH DOUBLE ORNAMENT
- {0x11680, 0x116AA, prN}, // Lo [43] TAKRI LETTER A..TAKRI LETTER RRA
- {0x116AB, 0x116AB, prN}, // Mn TAKRI SIGN ANUSVARA
- {0x116AC, 0x116AC, prN}, // Mc TAKRI SIGN VISARGA
- {0x116AD, 0x116AD, prN}, // Mn TAKRI VOWEL SIGN AA
- {0x116AE, 0x116AF, prN}, // Mc [2] TAKRI VOWEL SIGN I..TAKRI VOWEL SIGN II
- {0x116B0, 0x116B5, prN}, // Mn [6] TAKRI VOWEL SIGN U..TAKRI VOWEL SIGN AU
- {0x116B6, 0x116B6, prN}, // Mc TAKRI SIGN VIRAMA
- {0x116B7, 0x116B7, prN}, // Mn TAKRI SIGN NUKTA
- {0x116B8, 0x116B8, prN}, // Lo TAKRI LETTER ARCHAIC KHA
- {0x116B9, 0x116B9, prN}, // Po TAKRI ABBREVIATION SIGN
- {0x116C0, 0x116C9, prN}, // Nd [10] TAKRI DIGIT ZERO..TAKRI DIGIT NINE
- {0x11700, 0x1171A, prN}, // Lo [27] AHOM LETTER KA..AHOM LETTER ALTERNATE BA
- {0x1171D, 0x1171F, prN}, // Mn [3] AHOM CONSONANT SIGN MEDIAL LA..AHOM CONSONANT SIGN MEDIAL LIGATING RA
- {0x11720, 0x11721, prN}, // Mc [2] AHOM VOWEL SIGN A..AHOM VOWEL SIGN AA
- {0x11722, 0x11725, prN}, // Mn [4] AHOM VOWEL SIGN I..AHOM VOWEL SIGN UU
- {0x11726, 0x11726, prN}, // Mc AHOM VOWEL SIGN E
- {0x11727, 0x1172B, prN}, // Mn [5] AHOM VOWEL SIGN AW..AHOM SIGN KILLER
- {0x11730, 0x11739, prN}, // Nd [10] AHOM DIGIT ZERO..AHOM DIGIT NINE
- {0x1173A, 0x1173B, prN}, // No [2] AHOM NUMBER TEN..AHOM NUMBER TWENTY
- {0x1173C, 0x1173E, prN}, // Po [3] AHOM SIGN SMALL SECTION..AHOM SIGN RULAI
- {0x1173F, 0x1173F, prN}, // So AHOM SYMBOL VI
- {0x11740, 0x11746, prN}, // Lo [7] AHOM LETTER CA..AHOM LETTER LLA
- {0x11800, 0x1182B, prN}, // Lo [44] DOGRA LETTER A..DOGRA LETTER RRA
- {0x1182C, 0x1182E, prN}, // Mc [3] DOGRA VOWEL SIGN AA..DOGRA VOWEL SIGN II
- {0x1182F, 0x11837, prN}, // Mn [9] DOGRA VOWEL SIGN U..DOGRA SIGN ANUSVARA
- {0x11838, 0x11838, prN}, // Mc DOGRA SIGN VISARGA
- {0x11839, 0x1183A, prN}, // Mn [2] DOGRA SIGN VIRAMA..DOGRA SIGN NUKTA
- {0x1183B, 0x1183B, prN}, // Po DOGRA ABBREVIATION SIGN
- {0x118A0, 0x118DF, prN}, // L& [64] WARANG CITI CAPITAL LETTER NGAA..WARANG CITI SMALL LETTER VIYO
- {0x118E0, 0x118E9, prN}, // Nd [10] WARANG CITI DIGIT ZERO..WARANG CITI DIGIT NINE
- {0x118EA, 0x118F2, prN}, // No [9] WARANG CITI NUMBER TEN..WARANG CITI NUMBER NINETY
- {0x118FF, 0x118FF, prN}, // Lo WARANG CITI OM
- {0x11900, 0x11906, prN}, // Lo [7] DIVES AKURU LETTER A..DIVES AKURU LETTER E
- {0x11909, 0x11909, prN}, // Lo DIVES AKURU LETTER O
- {0x1190C, 0x11913, prN}, // Lo [8] DIVES AKURU LETTER KA..DIVES AKURU LETTER JA
- {0x11915, 0x11916, prN}, // Lo [2] DIVES AKURU LETTER NYA..DIVES AKURU LETTER TTA
- {0x11918, 0x1192F, prN}, // Lo [24] DIVES AKURU LETTER DDA..DIVES AKURU LETTER ZA
- {0x11930, 0x11935, prN}, // Mc [6] DIVES AKURU VOWEL SIGN AA..DIVES AKURU VOWEL SIGN E
- {0x11937, 0x11938, prN}, // Mc [2] DIVES AKURU VOWEL SIGN AI..DIVES AKURU VOWEL SIGN O
- {0x1193B, 0x1193C, prN}, // Mn [2] DIVES AKURU SIGN ANUSVARA..DIVES AKURU SIGN CANDRABINDU
- {0x1193D, 0x1193D, prN}, // Mc DIVES AKURU SIGN HALANTA
- {0x1193E, 0x1193E, prN}, // Mn DIVES AKURU VIRAMA
- {0x1193F, 0x1193F, prN}, // Lo DIVES AKURU PREFIXED NASAL SIGN
- {0x11940, 0x11940, prN}, // Mc DIVES AKURU MEDIAL YA
- {0x11941, 0x11941, prN}, // Lo DIVES AKURU INITIAL RA
- {0x11942, 0x11942, prN}, // Mc DIVES AKURU MEDIAL RA
- {0x11943, 0x11943, prN}, // Mn DIVES AKURU SIGN NUKTA
- {0x11944, 0x11946, prN}, // Po [3] DIVES AKURU DOUBLE DANDA..DIVES AKURU END OF TEXT MARK
- {0x11950, 0x11959, prN}, // Nd [10] DIVES AKURU DIGIT ZERO..DIVES AKURU DIGIT NINE
- {0x119A0, 0x119A7, prN}, // Lo [8] NANDINAGARI LETTER A..NANDINAGARI LETTER VOCALIC RR
- {0x119AA, 0x119D0, prN}, // Lo [39] NANDINAGARI LETTER E..NANDINAGARI LETTER RRA
- {0x119D1, 0x119D3, prN}, // Mc [3] NANDINAGARI VOWEL SIGN AA..NANDINAGARI VOWEL SIGN II
- {0x119D4, 0x119D7, prN}, // Mn [4] NANDINAGARI VOWEL SIGN U..NANDINAGARI VOWEL SIGN VOCALIC RR
- {0x119DA, 0x119DB, prN}, // Mn [2] NANDINAGARI VOWEL SIGN E..NANDINAGARI VOWEL SIGN AI
- {0x119DC, 0x119DF, prN}, // Mc [4] NANDINAGARI VOWEL SIGN O..NANDINAGARI SIGN VISARGA
- {0x119E0, 0x119E0, prN}, // Mn NANDINAGARI SIGN VIRAMA
- {0x119E1, 0x119E1, prN}, // Lo NANDINAGARI SIGN AVAGRAHA
- {0x119E2, 0x119E2, prN}, // Po NANDINAGARI SIGN SIDDHAM
- {0x119E3, 0x119E3, prN}, // Lo NANDINAGARI HEADSTROKE
- {0x119E4, 0x119E4, prN}, // Mc NANDINAGARI VOWEL SIGN PRISHTHAMATRA E
- {0x11A00, 0x11A00, prN}, // Lo ZANABAZAR SQUARE LETTER A
- {0x11A01, 0x11A0A, prN}, // Mn [10] ZANABAZAR SQUARE VOWEL SIGN I..ZANABAZAR SQUARE VOWEL LENGTH MARK
- {0x11A0B, 0x11A32, prN}, // Lo [40] ZANABAZAR SQUARE LETTER KA..ZANABAZAR SQUARE LETTER KSSA
- {0x11A33, 0x11A38, prN}, // Mn [6] ZANABAZAR SQUARE FINAL CONSONANT MARK..ZANABAZAR SQUARE SIGN ANUSVARA
- {0x11A39, 0x11A39, prN}, // Mc ZANABAZAR SQUARE SIGN VISARGA
- {0x11A3A, 0x11A3A, prN}, // Lo ZANABAZAR SQUARE CLUSTER-INITIAL LETTER RA
- {0x11A3B, 0x11A3E, prN}, // Mn [4] ZANABAZAR SQUARE CLUSTER-FINAL LETTER YA..ZANABAZAR SQUARE CLUSTER-FINAL LETTER VA
- {0x11A3F, 0x11A46, prN}, // Po [8] ZANABAZAR SQUARE INITIAL HEAD MARK..ZANABAZAR SQUARE CLOSING DOUBLE-LINED HEAD MARK
- {0x11A47, 0x11A47, prN}, // Mn ZANABAZAR SQUARE SUBJOINER
- {0x11A50, 0x11A50, prN}, // Lo SOYOMBO LETTER A
- {0x11A51, 0x11A56, prN}, // Mn [6] SOYOMBO VOWEL SIGN I..SOYOMBO VOWEL SIGN OE
- {0x11A57, 0x11A58, prN}, // Mc [2] SOYOMBO VOWEL SIGN AI..SOYOMBO VOWEL SIGN AU
- {0x11A59, 0x11A5B, prN}, // Mn [3] SOYOMBO VOWEL SIGN VOCALIC R..SOYOMBO VOWEL LENGTH MARK
- {0x11A5C, 0x11A89, prN}, // Lo [46] SOYOMBO LETTER KA..SOYOMBO CLUSTER-INITIAL LETTER SA
- {0x11A8A, 0x11A96, prN}, // Mn [13] SOYOMBO FINAL CONSONANT SIGN G..SOYOMBO SIGN ANUSVARA
- {0x11A97, 0x11A97, prN}, // Mc SOYOMBO SIGN VISARGA
- {0x11A98, 0x11A99, prN}, // Mn [2] SOYOMBO GEMINATION MARK..SOYOMBO SUBJOINER
- {0x11A9A, 0x11A9C, prN}, // Po [3] SOYOMBO MARK TSHEG..SOYOMBO MARK DOUBLE SHAD
- {0x11A9D, 0x11A9D, prN}, // Lo SOYOMBO MARK PLUTA
- {0x11A9E, 0x11AA2, prN}, // Po [5] SOYOMBO HEAD MARK WITH MOON AND SUN AND TRIPLE FLAME..SOYOMBO TERMINAL MARK-2
- {0x11AB0, 0x11ABF, prN}, // Lo [16] CANADIAN SYLLABICS NATTILIK HI..CANADIAN SYLLABICS SPA
- {0x11AC0, 0x11AF8, prN}, // Lo [57] PAU CIN HAU LETTER PA..PAU CIN HAU GLOTTAL STOP FINAL
- {0x11C00, 0x11C08, prN}, // Lo [9] BHAIKSUKI LETTER A..BHAIKSUKI LETTER VOCALIC L
- {0x11C0A, 0x11C2E, prN}, // Lo [37] BHAIKSUKI LETTER E..BHAIKSUKI LETTER HA
- {0x11C2F, 0x11C2F, prN}, // Mc BHAIKSUKI VOWEL SIGN AA
- {0x11C30, 0x11C36, prN}, // Mn [7] BHAIKSUKI VOWEL SIGN I..BHAIKSUKI VOWEL SIGN VOCALIC L
- {0x11C38, 0x11C3D, prN}, // Mn [6] BHAIKSUKI VOWEL SIGN E..BHAIKSUKI SIGN ANUSVARA
- {0x11C3E, 0x11C3E, prN}, // Mc BHAIKSUKI SIGN VISARGA
- {0x11C3F, 0x11C3F, prN}, // Mn BHAIKSUKI SIGN VIRAMA
- {0x11C40, 0x11C40, prN}, // Lo BHAIKSUKI SIGN AVAGRAHA
- {0x11C41, 0x11C45, prN}, // Po [5] BHAIKSUKI DANDA..BHAIKSUKI GAP FILLER-2
- {0x11C50, 0x11C59, prN}, // Nd [10] BHAIKSUKI DIGIT ZERO..BHAIKSUKI DIGIT NINE
- {0x11C5A, 0x11C6C, prN}, // No [19] BHAIKSUKI NUMBER ONE..BHAIKSUKI HUNDREDS UNIT MARK
- {0x11C70, 0x11C71, prN}, // Po [2] MARCHEN HEAD MARK..MARCHEN MARK SHAD
- {0x11C72, 0x11C8F, prN}, // Lo [30] MARCHEN LETTER KA..MARCHEN LETTER A
- {0x11C92, 0x11CA7, prN}, // Mn [22] MARCHEN SUBJOINED LETTER KA..MARCHEN SUBJOINED LETTER ZA
- {0x11CA9, 0x11CA9, prN}, // Mc MARCHEN SUBJOINED LETTER YA
- {0x11CAA, 0x11CB0, prN}, // Mn [7] MARCHEN SUBJOINED LETTER RA..MARCHEN VOWEL SIGN AA
- {0x11CB1, 0x11CB1, prN}, // Mc MARCHEN VOWEL SIGN I
- {0x11CB2, 0x11CB3, prN}, // Mn [2] MARCHEN VOWEL SIGN U..MARCHEN VOWEL SIGN E
- {0x11CB4, 0x11CB4, prN}, // Mc MARCHEN VOWEL SIGN O
- {0x11CB5, 0x11CB6, prN}, // Mn [2] MARCHEN SIGN ANUSVARA..MARCHEN SIGN CANDRABINDU
- {0x11D00, 0x11D06, prN}, // Lo [7] MASARAM GONDI LETTER A..MASARAM GONDI LETTER E
- {0x11D08, 0x11D09, prN}, // Lo [2] MASARAM GONDI LETTER AI..MASARAM GONDI LETTER O
- {0x11D0B, 0x11D30, prN}, // Lo [38] MASARAM GONDI LETTER AU..MASARAM GONDI LETTER TRA
- {0x11D31, 0x11D36, prN}, // Mn [6] MASARAM GONDI VOWEL SIGN AA..MASARAM GONDI VOWEL SIGN VOCALIC R
- {0x11D3A, 0x11D3A, prN}, // Mn MASARAM GONDI VOWEL SIGN E
- {0x11D3C, 0x11D3D, prN}, // Mn [2] MASARAM GONDI VOWEL SIGN AI..MASARAM GONDI VOWEL SIGN O
- {0x11D3F, 0x11D45, prN}, // Mn [7] MASARAM GONDI VOWEL SIGN AU..MASARAM GONDI VIRAMA
- {0x11D46, 0x11D46, prN}, // Lo MASARAM GONDI REPHA
- {0x11D47, 0x11D47, prN}, // Mn MASARAM GONDI RA-KARA
- {0x11D50, 0x11D59, prN}, // Nd [10] MASARAM GONDI DIGIT ZERO..MASARAM GONDI DIGIT NINE
- {0x11D60, 0x11D65, prN}, // Lo [6] GUNJALA GONDI LETTER A..GUNJALA GONDI LETTER UU
- {0x11D67, 0x11D68, prN}, // Lo [2] GUNJALA GONDI LETTER EE..GUNJALA GONDI LETTER AI
- {0x11D6A, 0x11D89, prN}, // Lo [32] GUNJALA GONDI LETTER OO..GUNJALA GONDI LETTER SA
- {0x11D8A, 0x11D8E, prN}, // Mc [5] GUNJALA GONDI VOWEL SIGN AA..GUNJALA GONDI VOWEL SIGN UU
- {0x11D90, 0x11D91, prN}, // Mn [2] GUNJALA GONDI VOWEL SIGN EE..GUNJALA GONDI VOWEL SIGN AI
- {0x11D93, 0x11D94, prN}, // Mc [2] GUNJALA GONDI VOWEL SIGN OO..GUNJALA GONDI VOWEL SIGN AU
- {0x11D95, 0x11D95, prN}, // Mn GUNJALA GONDI SIGN ANUSVARA
- {0x11D96, 0x11D96, prN}, // Mc GUNJALA GONDI SIGN VISARGA
- {0x11D97, 0x11D97, prN}, // Mn GUNJALA GONDI VIRAMA
- {0x11D98, 0x11D98, prN}, // Lo GUNJALA GONDI OM
- {0x11DA0, 0x11DA9, prN}, // Nd [10] GUNJALA GONDI DIGIT ZERO..GUNJALA GONDI DIGIT NINE
- {0x11EE0, 0x11EF2, prN}, // Lo [19] MAKASAR LETTER KA..MAKASAR ANGKA
- {0x11EF3, 0x11EF4, prN}, // Mn [2] MAKASAR VOWEL SIGN I..MAKASAR VOWEL SIGN U
- {0x11EF5, 0x11EF6, prN}, // Mc [2] MAKASAR VOWEL SIGN E..MAKASAR VOWEL SIGN O
- {0x11EF7, 0x11EF8, prN}, // Po [2] MAKASAR PASSIMBANG..MAKASAR END OF SECTION
- {0x11FB0, 0x11FB0, prN}, // Lo LISU LETTER YHA
- {0x11FC0, 0x11FD4, prN}, // No [21] TAMIL FRACTION ONE THREE-HUNDRED-AND-TWENTIETH..TAMIL FRACTION DOWNSCALING FACTOR KIIZH
- {0x11FD5, 0x11FDC, prN}, // So [8] TAMIL SIGN NEL..TAMIL SIGN MUKKURUNI
- {0x11FDD, 0x11FE0, prN}, // Sc [4] TAMIL SIGN KAACU..TAMIL SIGN VARAAKAN
- {0x11FE1, 0x11FF1, prN}, // So [17] TAMIL SIGN PAARAM..TAMIL SIGN VAKAIYARAA
- {0x11FFF, 0x11FFF, prN}, // Po TAMIL PUNCTUATION END OF TEXT
- {0x12000, 0x12399, prN}, // Lo [922] CUNEIFORM SIGN A..CUNEIFORM SIGN U U
- {0x12400, 0x1246E, prN}, // Nl [111] CUNEIFORM NUMERIC SIGN TWO ASH..CUNEIFORM NUMERIC SIGN NINE U VARIANT FORM
- {0x12470, 0x12474, prN}, // Po [5] CUNEIFORM PUNCTUATION SIGN OLD ASSYRIAN WORD DIVIDER..CUNEIFORM PUNCTUATION SIGN DIAGONAL QUADCOLON
- {0x12480, 0x12543, prN}, // Lo [196] CUNEIFORM SIGN AB TIMES NUN TENU..CUNEIFORM SIGN ZU5 TIMES THREE DISH TENU
- {0x12F90, 0x12FF0, prN}, // Lo [97] CYPRO-MINOAN SIGN CM001..CYPRO-MINOAN SIGN CM114
- {0x12FF1, 0x12FF2, prN}, // Po [2] CYPRO-MINOAN SIGN CM301..CYPRO-MINOAN SIGN CM302
- {0x13000, 0x1342E, prN}, // Lo [1071] EGYPTIAN HIEROGLYPH A001..EGYPTIAN HIEROGLYPH AA032
- {0x13430, 0x13438, prN}, // Cf [9] EGYPTIAN HIEROGLYPH VERTICAL JOINER..EGYPTIAN HIEROGLYPH END SEGMENT
- {0x14400, 0x14646, prN}, // Lo [583] ANATOLIAN HIEROGLYPH A001..ANATOLIAN HIEROGLYPH A530
- {0x16800, 0x16A38, prN}, // Lo [569] BAMUM LETTER PHASE-A NGKUE MFON..BAMUM LETTER PHASE-F VUEQ
- {0x16A40, 0x16A5E, prN}, // Lo [31] MRO LETTER TA..MRO LETTER TEK
- {0x16A60, 0x16A69, prN}, // Nd [10] MRO DIGIT ZERO..MRO DIGIT NINE
- {0x16A6E, 0x16A6F, prN}, // Po [2] MRO DANDA..MRO DOUBLE DANDA
- {0x16A70, 0x16ABE, prN}, // Lo [79] TANGSA LETTER OZ..TANGSA LETTER ZA
- {0x16AC0, 0x16AC9, prN}, // Nd [10] TANGSA DIGIT ZERO..TANGSA DIGIT NINE
- {0x16AD0, 0x16AED, prN}, // Lo [30] BASSA VAH LETTER ENNI..BASSA VAH LETTER I
- {0x16AF0, 0x16AF4, prN}, // Mn [5] BASSA VAH COMBINING HIGH TONE..BASSA VAH COMBINING HIGH-LOW TONE
- {0x16AF5, 0x16AF5, prN}, // Po BASSA VAH FULL STOP
- {0x16B00, 0x16B2F, prN}, // Lo [48] PAHAWH HMONG VOWEL KEEB..PAHAWH HMONG CONSONANT CAU
- {0x16B30, 0x16B36, prN}, // Mn [7] PAHAWH HMONG MARK CIM TUB..PAHAWH HMONG MARK CIM TAUM
- {0x16B37, 0x16B3B, prN}, // Po [5] PAHAWH HMONG SIGN VOS THOM..PAHAWH HMONG SIGN VOS FEEM
- {0x16B3C, 0x16B3F, prN}, // So [4] PAHAWH HMONG SIGN XYEEM NTXIV..PAHAWH HMONG SIGN XYEEM FAIB
- {0x16B40, 0x16B43, prN}, // Lm [4] PAHAWH HMONG SIGN VOS SEEV..PAHAWH HMONG SIGN IB YAM
- {0x16B44, 0x16B44, prN}, // Po PAHAWH HMONG SIGN XAUS
- {0x16B45, 0x16B45, prN}, // So PAHAWH HMONG SIGN CIM TSOV ROG
- {0x16B50, 0x16B59, prN}, // Nd [10] PAHAWH HMONG DIGIT ZERO..PAHAWH HMONG DIGIT NINE
- {0x16B5B, 0x16B61, prN}, // No [7] PAHAWH HMONG NUMBER TENS..PAHAWH HMONG NUMBER TRILLIONS
- {0x16B63, 0x16B77, prN}, // Lo [21] PAHAWH HMONG SIGN VOS LUB..PAHAWH HMONG SIGN CIM NRES TOS
- {0x16B7D, 0x16B8F, prN}, // Lo [19] PAHAWH HMONG CLAN SIGN TSHEEJ..PAHAWH HMONG CLAN SIGN VWJ
- {0x16E40, 0x16E7F, prN}, // L& [64] MEDEFAIDRIN CAPITAL LETTER M..MEDEFAIDRIN SMALL LETTER Y
- {0x16E80, 0x16E96, prN}, // No [23] MEDEFAIDRIN DIGIT ZERO..MEDEFAIDRIN DIGIT THREE ALTERNATE FORM
- {0x16E97, 0x16E9A, prN}, // Po [4] MEDEFAIDRIN COMMA..MEDEFAIDRIN EXCLAMATION OH
- {0x16F00, 0x16F4A, prN}, // Lo [75] MIAO LETTER PA..MIAO LETTER RTE
- {0x16F4F, 0x16F4F, prN}, // Mn MIAO SIGN CONSONANT MODIFIER BAR
- {0x16F50, 0x16F50, prN}, // Lo MIAO LETTER NASALIZATION
- {0x16F51, 0x16F87, prN}, // Mc [55] MIAO SIGN ASPIRATION..MIAO VOWEL SIGN UI
- {0x16F8F, 0x16F92, prN}, // Mn [4] MIAO TONE RIGHT..MIAO TONE BELOW
- {0x16F93, 0x16F9F, prN}, // Lm [13] MIAO LETTER TONE-2..MIAO LETTER REFORMED TONE-8
- {0x16FE0, 0x16FE1, prW}, // Lm [2] TANGUT ITERATION MARK..NUSHU ITERATION MARK
- {0x16FE2, 0x16FE2, prW}, // Po OLD CHINESE HOOK MARK
- {0x16FE3, 0x16FE3, prW}, // Lm OLD CHINESE ITERATION MARK
- {0x16FE4, 0x16FE4, prW}, // Mn KHITAN SMALL SCRIPT FILLER
- {0x16FF0, 0x16FF1, prW}, // Mc [2] VIETNAMESE ALTERNATE READING MARK CA..VIETNAMESE ALTERNATE READING MARK NHAY
- {0x17000, 0x187F7, prW}, // Lo [6136] TANGUT IDEOGRAPH-17000..TANGUT IDEOGRAPH-187F7
- {0x18800, 0x18AFF, prW}, // Lo [768] TANGUT COMPONENT-001..TANGUT COMPONENT-768
- {0x18B00, 0x18CD5, prW}, // Lo [470] KHITAN SMALL SCRIPT CHARACTER-18B00..KHITAN SMALL SCRIPT CHARACTER-18CD5
- {0x18D00, 0x18D08, prW}, // Lo [9] TANGUT IDEOGRAPH-18D00..TANGUT IDEOGRAPH-18D08
- {0x1AFF0, 0x1AFF3, prW}, // Lm [4] KATAKANA LETTER MINNAN TONE-2..KATAKANA LETTER MINNAN TONE-5
- {0x1AFF5, 0x1AFFB, prW}, // Lm [7] KATAKANA LETTER MINNAN TONE-7..KATAKANA LETTER MINNAN NASALIZED TONE-5
- {0x1AFFD, 0x1AFFE, prW}, // Lm [2] KATAKANA LETTER MINNAN NASALIZED TONE-7..KATAKANA LETTER MINNAN NASALIZED TONE-8
- {0x1B000, 0x1B0FF, prW}, // Lo [256] KATAKANA LETTER ARCHAIC E..HENTAIGANA LETTER RE-2
- {0x1B100, 0x1B122, prW}, // Lo [35] HENTAIGANA LETTER RE-3..KATAKANA LETTER ARCHAIC WU
- {0x1B150, 0x1B152, prW}, // Lo [3] HIRAGANA LETTER SMALL WI..HIRAGANA LETTER SMALL WO
- {0x1B164, 0x1B167, prW}, // Lo [4] KATAKANA LETTER SMALL WI..KATAKANA LETTER SMALL N
- {0x1B170, 0x1B2FB, prW}, // Lo [396] NUSHU CHARACTER-1B170..NUSHU CHARACTER-1B2FB
- {0x1BC00, 0x1BC6A, prN}, // Lo [107] DUPLOYAN LETTER H..DUPLOYAN LETTER VOCALIC M
- {0x1BC70, 0x1BC7C, prN}, // Lo [13] DUPLOYAN AFFIX LEFT HORIZONTAL SECANT..DUPLOYAN AFFIX ATTACHED TANGENT HOOK
- {0x1BC80, 0x1BC88, prN}, // Lo [9] DUPLOYAN AFFIX HIGH ACUTE..DUPLOYAN AFFIX HIGH VERTICAL
- {0x1BC90, 0x1BC99, prN}, // Lo [10] DUPLOYAN AFFIX LOW ACUTE..DUPLOYAN AFFIX LOW ARROW
- {0x1BC9C, 0x1BC9C, prN}, // So DUPLOYAN SIGN O WITH CROSS
- {0x1BC9D, 0x1BC9E, prN}, // Mn [2] DUPLOYAN THICK LETTER SELECTOR..DUPLOYAN DOUBLE MARK
- {0x1BC9F, 0x1BC9F, prN}, // Po DUPLOYAN PUNCTUATION CHINOOK FULL STOP
- {0x1BCA0, 0x1BCA3, prN}, // Cf [4] SHORTHAND FORMAT LETTER OVERLAP..SHORTHAND FORMAT UP STEP
- {0x1CF00, 0x1CF2D, prN}, // Mn [46] ZNAMENNY COMBINING MARK GORAZDO NIZKO S KRYZHEM ON LEFT..ZNAMENNY COMBINING MARK KRYZH ON LEFT
- {0x1CF30, 0x1CF46, prN}, // Mn [23] ZNAMENNY COMBINING TONAL RANGE MARK MRACHNO..ZNAMENNY PRIZNAK MODIFIER ROG
- {0x1CF50, 0x1CFC3, prN}, // So [116] ZNAMENNY NEUME KRYUK..ZNAMENNY NEUME PAUK
- {0x1D000, 0x1D0F5, prN}, // So [246] BYZANTINE MUSICAL SYMBOL PSILI..BYZANTINE MUSICAL SYMBOL GORGON NEO KATO
- {0x1D100, 0x1D126, prN}, // So [39] MUSICAL SYMBOL SINGLE BARLINE..MUSICAL SYMBOL DRUM CLEF-2
- {0x1D129, 0x1D164, prN}, // So [60] MUSICAL SYMBOL MULTIPLE MEASURE REST..MUSICAL SYMBOL ONE HUNDRED TWENTY-EIGHTH NOTE
- {0x1D165, 0x1D166, prN}, // Mc [2] MUSICAL SYMBOL COMBINING STEM..MUSICAL SYMBOL COMBINING SPRECHGESANG STEM
- {0x1D167, 0x1D169, prN}, // Mn [3] MUSICAL SYMBOL COMBINING TREMOLO-1..MUSICAL SYMBOL COMBINING TREMOLO-3
- {0x1D16A, 0x1D16C, prN}, // So [3] MUSICAL SYMBOL FINGERED TREMOLO-1..MUSICAL SYMBOL FINGERED TREMOLO-3
- {0x1D16D, 0x1D172, prN}, // Mc [6] MUSICAL SYMBOL COMBINING AUGMENTATION DOT..MUSICAL SYMBOL COMBINING FLAG-5
- {0x1D173, 0x1D17A, prN}, // Cf [8] MUSICAL SYMBOL BEGIN BEAM..MUSICAL SYMBOL END PHRASE
- {0x1D17B, 0x1D182, prN}, // Mn [8] MUSICAL SYMBOL COMBINING ACCENT..MUSICAL SYMBOL COMBINING LOURE
- {0x1D183, 0x1D184, prN}, // So [2] MUSICAL SYMBOL ARPEGGIATO UP..MUSICAL SYMBOL ARPEGGIATO DOWN
- {0x1D185, 0x1D18B, prN}, // Mn [7] MUSICAL SYMBOL COMBINING DOIT..MUSICAL SYMBOL COMBINING TRIPLE TONGUE
- {0x1D18C, 0x1D1A9, prN}, // So [30] MUSICAL SYMBOL RINFORZANDO..MUSICAL SYMBOL DEGREE SLASH
- {0x1D1AA, 0x1D1AD, prN}, // Mn [4] MUSICAL SYMBOL COMBINING DOWN BOW..MUSICAL SYMBOL COMBINING SNAP PIZZICATO
- {0x1D1AE, 0x1D1EA, prN}, // So [61] MUSICAL SYMBOL PEDAL MARK..MUSICAL SYMBOL KORON
- {0x1D200, 0x1D241, prN}, // So [66] GREEK VOCAL NOTATION SYMBOL-1..GREEK INSTRUMENTAL NOTATION SYMBOL-54
- {0x1D242, 0x1D244, prN}, // Mn [3] COMBINING GREEK MUSICAL TRISEME..COMBINING GREEK MUSICAL PENTASEME
- {0x1D245, 0x1D245, prN}, // So GREEK MUSICAL LEIMMA
- {0x1D2E0, 0x1D2F3, prN}, // No [20] MAYAN NUMERAL ZERO..MAYAN NUMERAL NINETEEN
- {0x1D300, 0x1D356, prN}, // So [87] MONOGRAM FOR EARTH..TETRAGRAM FOR FOSTERING
- {0x1D360, 0x1D378, prN}, // No [25] COUNTING ROD UNIT DIGIT ONE..TALLY MARK FIVE
- {0x1D400, 0x1D454, prN}, // L& [85] MATHEMATICAL BOLD CAPITAL A..MATHEMATICAL ITALIC SMALL G
- {0x1D456, 0x1D49C, prN}, // L& [71] MATHEMATICAL ITALIC SMALL I..MATHEMATICAL SCRIPT CAPITAL A
- {0x1D49E, 0x1D49F, prN}, // Lu [2] MATHEMATICAL SCRIPT CAPITAL C..MATHEMATICAL SCRIPT CAPITAL D
- {0x1D4A2, 0x1D4A2, prN}, // Lu MATHEMATICAL SCRIPT CAPITAL G
- {0x1D4A5, 0x1D4A6, prN}, // Lu [2] MATHEMATICAL SCRIPT CAPITAL J..MATHEMATICAL SCRIPT CAPITAL K
- {0x1D4A9, 0x1D4AC, prN}, // Lu [4] MATHEMATICAL SCRIPT CAPITAL N..MATHEMATICAL SCRIPT CAPITAL Q
- {0x1D4AE, 0x1D4B9, prN}, // L& [12] MATHEMATICAL SCRIPT CAPITAL S..MATHEMATICAL SCRIPT SMALL D
- {0x1D4BB, 0x1D4BB, prN}, // Ll MATHEMATICAL SCRIPT SMALL F
- {0x1D4BD, 0x1D4C3, prN}, // Ll [7] MATHEMATICAL SCRIPT SMALL H..MATHEMATICAL SCRIPT SMALL N
- {0x1D4C5, 0x1D505, prN}, // L& [65] MATHEMATICAL SCRIPT SMALL P..MATHEMATICAL FRAKTUR CAPITAL B
- {0x1D507, 0x1D50A, prN}, // Lu [4] MATHEMATICAL FRAKTUR CAPITAL D..MATHEMATICAL FRAKTUR CAPITAL G
- {0x1D50D, 0x1D514, prN}, // Lu [8] MATHEMATICAL FRAKTUR CAPITAL J..MATHEMATICAL FRAKTUR CAPITAL Q
- {0x1D516, 0x1D51C, prN}, // Lu [7] MATHEMATICAL FRAKTUR CAPITAL S..MATHEMATICAL FRAKTUR CAPITAL Y
- {0x1D51E, 0x1D539, prN}, // L& [28] MATHEMATICAL FRAKTUR SMALL A..MATHEMATICAL DOUBLE-STRUCK CAPITAL B
- {0x1D53B, 0x1D53E, prN}, // Lu [4] MATHEMATICAL DOUBLE-STRUCK CAPITAL D..MATHEMATICAL DOUBLE-STRUCK CAPITAL G
- {0x1D540, 0x1D544, prN}, // Lu [5] MATHEMATICAL DOUBLE-STRUCK CAPITAL I..MATHEMATICAL DOUBLE-STRUCK CAPITAL M
- {0x1D546, 0x1D546, prN}, // Lu MATHEMATICAL DOUBLE-STRUCK CAPITAL O
- {0x1D54A, 0x1D550, prN}, // Lu [7] MATHEMATICAL DOUBLE-STRUCK CAPITAL S..MATHEMATICAL DOUBLE-STRUCK CAPITAL Y
- {0x1D552, 0x1D6A5, prN}, // L& [340] MATHEMATICAL DOUBLE-STRUCK SMALL A..MATHEMATICAL ITALIC SMALL DOTLESS J
- {0x1D6A8, 0x1D6C0, prN}, // Lu [25] MATHEMATICAL BOLD CAPITAL ALPHA..MATHEMATICAL BOLD CAPITAL OMEGA
- {0x1D6C1, 0x1D6C1, prN}, // Sm MATHEMATICAL BOLD NABLA
- {0x1D6C2, 0x1D6DA, prN}, // Ll [25] MATHEMATICAL BOLD SMALL ALPHA..MATHEMATICAL BOLD SMALL OMEGA
- {0x1D6DB, 0x1D6DB, prN}, // Sm MATHEMATICAL BOLD PARTIAL DIFFERENTIAL
- {0x1D6DC, 0x1D6FA, prN}, // L& [31] MATHEMATICAL BOLD EPSILON SYMBOL..MATHEMATICAL ITALIC CAPITAL OMEGA
- {0x1D6FB, 0x1D6FB, prN}, // Sm MATHEMATICAL ITALIC NABLA
- {0x1D6FC, 0x1D714, prN}, // Ll [25] MATHEMATICAL ITALIC SMALL ALPHA..MATHEMATICAL ITALIC SMALL OMEGA
- {0x1D715, 0x1D715, prN}, // Sm MATHEMATICAL ITALIC PARTIAL DIFFERENTIAL
- {0x1D716, 0x1D734, prN}, // L& [31] MATHEMATICAL ITALIC EPSILON SYMBOL..MATHEMATICAL BOLD ITALIC CAPITAL OMEGA
- {0x1D735, 0x1D735, prN}, // Sm MATHEMATICAL BOLD ITALIC NABLA
- {0x1D736, 0x1D74E, prN}, // Ll [25] MATHEMATICAL BOLD ITALIC SMALL ALPHA..MATHEMATICAL BOLD ITALIC SMALL OMEGA
- {0x1D74F, 0x1D74F, prN}, // Sm MATHEMATICAL BOLD ITALIC PARTIAL DIFFERENTIAL
- {0x1D750, 0x1D76E, prN}, // L& [31] MATHEMATICAL BOLD ITALIC EPSILON SYMBOL..MATHEMATICAL SANS-SERIF BOLD CAPITAL OMEGA
- {0x1D76F, 0x1D76F, prN}, // Sm MATHEMATICAL SANS-SERIF BOLD NABLA
- {0x1D770, 0x1D788, prN}, // Ll [25] MATHEMATICAL SANS-SERIF BOLD SMALL ALPHA..MATHEMATICAL SANS-SERIF BOLD SMALL OMEGA
- {0x1D789, 0x1D789, prN}, // Sm MATHEMATICAL SANS-SERIF BOLD PARTIAL DIFFERENTIAL
- {0x1D78A, 0x1D7A8, prN}, // L& [31] MATHEMATICAL SANS-SERIF BOLD EPSILON SYMBOL..MATHEMATICAL SANS-SERIF BOLD ITALIC CAPITAL OMEGA
- {0x1D7A9, 0x1D7A9, prN}, // Sm MATHEMATICAL SANS-SERIF BOLD ITALIC NABLA
- {0x1D7AA, 0x1D7C2, prN}, // Ll [25] MATHEMATICAL SANS-SERIF BOLD ITALIC SMALL ALPHA..MATHEMATICAL SANS-SERIF BOLD ITALIC SMALL OMEGA
- {0x1D7C3, 0x1D7C3, prN}, // Sm MATHEMATICAL SANS-SERIF BOLD ITALIC PARTIAL DIFFERENTIAL
- {0x1D7C4, 0x1D7CB, prN}, // L& [8] MATHEMATICAL SANS-SERIF BOLD ITALIC EPSILON SYMBOL..MATHEMATICAL BOLD SMALL DIGAMMA
- {0x1D7CE, 0x1D7FF, prN}, // Nd [50] MATHEMATICAL BOLD DIGIT ZERO..MATHEMATICAL MONOSPACE DIGIT NINE
- {0x1D800, 0x1D9FF, prN}, // So [512] SIGNWRITING HAND-FIST INDEX..SIGNWRITING HEAD
- {0x1DA00, 0x1DA36, prN}, // Mn [55] SIGNWRITING HEAD RIM..SIGNWRITING AIR SUCKING IN
- {0x1DA37, 0x1DA3A, prN}, // So [4] SIGNWRITING AIR BLOW SMALL ROTATIONS..SIGNWRITING BREATH EXHALE
- {0x1DA3B, 0x1DA6C, prN}, // Mn [50] SIGNWRITING MOUTH CLOSED NEUTRAL..SIGNWRITING EXCITEMENT
- {0x1DA6D, 0x1DA74, prN}, // So [8] SIGNWRITING SHOULDER HIP SPINE..SIGNWRITING TORSO-FLOORPLANE TWISTING
- {0x1DA75, 0x1DA75, prN}, // Mn SIGNWRITING UPPER BODY TILTING FROM HIP JOINTS
- {0x1DA76, 0x1DA83, prN}, // So [14] SIGNWRITING LIMB COMBINATION..SIGNWRITING LOCATION DEPTH
- {0x1DA84, 0x1DA84, prN}, // Mn SIGNWRITING LOCATION HEAD NECK
- {0x1DA85, 0x1DA86, prN}, // So [2] SIGNWRITING LOCATION TORSO..SIGNWRITING LOCATION LIMBS DIGITS
- {0x1DA87, 0x1DA8B, prN}, // Po [5] SIGNWRITING COMMA..SIGNWRITING PARENTHESIS
- {0x1DA9B, 0x1DA9F, prN}, // Mn [5] SIGNWRITING FILL MODIFIER-2..SIGNWRITING FILL MODIFIER-6
- {0x1DAA1, 0x1DAAF, prN}, // Mn [15] SIGNWRITING ROTATION MODIFIER-2..SIGNWRITING ROTATION MODIFIER-16
- {0x1DF00, 0x1DF09, prN}, // Ll [10] LATIN SMALL LETTER FENG DIGRAPH WITH TRILL..LATIN SMALL LETTER T WITH HOOK AND RETROFLEX HOOK
- {0x1DF0A, 0x1DF0A, prN}, // Lo LATIN LETTER RETROFLEX CLICK WITH RETROFLEX HOOK
- {0x1DF0B, 0x1DF1E, prN}, // Ll [20] LATIN SMALL LETTER ESH WITH DOUBLE BAR..LATIN SMALL LETTER S WITH CURL
- {0x1E000, 0x1E006, prN}, // Mn [7] COMBINING GLAGOLITIC LETTER AZU..COMBINING GLAGOLITIC LETTER ZHIVETE
- {0x1E008, 0x1E018, prN}, // Mn [17] COMBINING GLAGOLITIC LETTER ZEMLJA..COMBINING GLAGOLITIC LETTER HERU
- {0x1E01B, 0x1E021, prN}, // Mn [7] COMBINING GLAGOLITIC LETTER SHTA..COMBINING GLAGOLITIC LETTER YATI
- {0x1E023, 0x1E024, prN}, // Mn [2] COMBINING GLAGOLITIC LETTER YU..COMBINING GLAGOLITIC LETTER SMALL YUS
- {0x1E026, 0x1E02A, prN}, // Mn [5] COMBINING GLAGOLITIC LETTER YO..COMBINING GLAGOLITIC LETTER FITA
- {0x1E100, 0x1E12C, prN}, // Lo [45] NYIAKENG PUACHUE HMONG LETTER MA..NYIAKENG PUACHUE HMONG LETTER W
- {0x1E130, 0x1E136, prN}, // Mn [7] NYIAKENG PUACHUE HMONG TONE-B..NYIAKENG PUACHUE HMONG TONE-D
- {0x1E137, 0x1E13D, prN}, // Lm [7] NYIAKENG PUACHUE HMONG SIGN FOR PERSON..NYIAKENG PUACHUE HMONG SYLLABLE LENGTHENER
- {0x1E140, 0x1E149, prN}, // Nd [10] NYIAKENG PUACHUE HMONG DIGIT ZERO..NYIAKENG PUACHUE HMONG DIGIT NINE
- {0x1E14E, 0x1E14E, prN}, // Lo NYIAKENG PUACHUE HMONG LOGOGRAM NYAJ
- {0x1E14F, 0x1E14F, prN}, // So NYIAKENG PUACHUE HMONG CIRCLED CA
- {0x1E290, 0x1E2AD, prN}, // Lo [30] TOTO LETTER PA..TOTO LETTER A
- {0x1E2AE, 0x1E2AE, prN}, // Mn TOTO SIGN RISING TONE
- {0x1E2C0, 0x1E2EB, prN}, // Lo [44] WANCHO LETTER AA..WANCHO LETTER YIH
- {0x1E2EC, 0x1E2EF, prN}, // Mn [4] WANCHO TONE TUP..WANCHO TONE KOINI
- {0x1E2F0, 0x1E2F9, prN}, // Nd [10] WANCHO DIGIT ZERO..WANCHO DIGIT NINE
- {0x1E2FF, 0x1E2FF, prN}, // Sc WANCHO NGUN SIGN
- {0x1E7E0, 0x1E7E6, prN}, // Lo [7] ETHIOPIC SYLLABLE HHYA..ETHIOPIC SYLLABLE HHYO
- {0x1E7E8, 0x1E7EB, prN}, // Lo [4] ETHIOPIC SYLLABLE GURAGE HHWA..ETHIOPIC SYLLABLE HHWE
- {0x1E7ED, 0x1E7EE, prN}, // Lo [2] ETHIOPIC SYLLABLE GURAGE MWI..ETHIOPIC SYLLABLE GURAGE MWEE
- {0x1E7F0, 0x1E7FE, prN}, // Lo [15] ETHIOPIC SYLLABLE GURAGE QWI..ETHIOPIC SYLLABLE GURAGE PWEE
- {0x1E800, 0x1E8C4, prN}, // Lo [197] MENDE KIKAKUI SYLLABLE M001 KI..MENDE KIKAKUI SYLLABLE M060 NYON
- {0x1E8C7, 0x1E8CF, prN}, // No [9] MENDE KIKAKUI DIGIT ONE..MENDE KIKAKUI DIGIT NINE
- {0x1E8D0, 0x1E8D6, prN}, // Mn [7] MENDE KIKAKUI COMBINING NUMBER TEENS..MENDE KIKAKUI COMBINING NUMBER MILLIONS
- {0x1E900, 0x1E943, prN}, // L& [68] ADLAM CAPITAL LETTER ALIF..ADLAM SMALL LETTER SHA
- {0x1E944, 0x1E94A, prN}, // Mn [7] ADLAM ALIF LENGTHENER..ADLAM NUKTA
- {0x1E94B, 0x1E94B, prN}, // Lm ADLAM NASALIZATION MARK
- {0x1E950, 0x1E959, prN}, // Nd [10] ADLAM DIGIT ZERO..ADLAM DIGIT NINE
- {0x1E95E, 0x1E95F, prN}, // Po [2] ADLAM INITIAL EXCLAMATION MARK..ADLAM INITIAL QUESTION MARK
- {0x1EC71, 0x1ECAB, prN}, // No [59] INDIC SIYAQ NUMBER ONE..INDIC SIYAQ NUMBER PREFIXED NINE
- {0x1ECAC, 0x1ECAC, prN}, // So INDIC SIYAQ PLACEHOLDER
- {0x1ECAD, 0x1ECAF, prN}, // No [3] INDIC SIYAQ FRACTION ONE QUARTER..INDIC SIYAQ FRACTION THREE QUARTERS
- {0x1ECB0, 0x1ECB0, prN}, // Sc INDIC SIYAQ RUPEE MARK
- {0x1ECB1, 0x1ECB4, prN}, // No [4] INDIC SIYAQ NUMBER ALTERNATE ONE..INDIC SIYAQ ALTERNATE LAKH MARK
- {0x1ED01, 0x1ED2D, prN}, // No [45] OTTOMAN SIYAQ NUMBER ONE..OTTOMAN SIYAQ NUMBER NINETY THOUSAND
- {0x1ED2E, 0x1ED2E, prN}, // So OTTOMAN SIYAQ MARRATAN
- {0x1ED2F, 0x1ED3D, prN}, // No [15] OTTOMAN SIYAQ ALTERNATE NUMBER TWO..OTTOMAN SIYAQ FRACTION ONE SIXTH
- {0x1EE00, 0x1EE03, prN}, // Lo [4] ARABIC MATHEMATICAL ALEF..ARABIC MATHEMATICAL DAL
- {0x1EE05, 0x1EE1F, prN}, // Lo [27] ARABIC MATHEMATICAL WAW..ARABIC MATHEMATICAL DOTLESS QAF
- {0x1EE21, 0x1EE22, prN}, // Lo [2] ARABIC MATHEMATICAL INITIAL BEH..ARABIC MATHEMATICAL INITIAL JEEM
- {0x1EE24, 0x1EE24, prN}, // Lo ARABIC MATHEMATICAL INITIAL HEH
- {0x1EE27, 0x1EE27, prN}, // Lo ARABIC MATHEMATICAL INITIAL HAH
- {0x1EE29, 0x1EE32, prN}, // Lo [10] ARABIC MATHEMATICAL INITIAL YEH..ARABIC MATHEMATICAL INITIAL QAF
- {0x1EE34, 0x1EE37, prN}, // Lo [4] ARABIC MATHEMATICAL INITIAL SHEEN..ARABIC MATHEMATICAL INITIAL KHAH
- {0x1EE39, 0x1EE39, prN}, // Lo ARABIC MATHEMATICAL INITIAL DAD
- {0x1EE3B, 0x1EE3B, prN}, // Lo ARABIC MATHEMATICAL INITIAL GHAIN
- {0x1EE42, 0x1EE42, prN}, // Lo ARABIC MATHEMATICAL TAILED JEEM
- {0x1EE47, 0x1EE47, prN}, // Lo ARABIC MATHEMATICAL TAILED HAH
- {0x1EE49, 0x1EE49, prN}, // Lo ARABIC MATHEMATICAL TAILED YEH
- {0x1EE4B, 0x1EE4B, prN}, // Lo ARABIC MATHEMATICAL TAILED LAM
- {0x1EE4D, 0x1EE4F, prN}, // Lo [3] ARABIC MATHEMATICAL TAILED NOON..ARABIC MATHEMATICAL TAILED AIN
- {0x1EE51, 0x1EE52, prN}, // Lo [2] ARABIC MATHEMATICAL TAILED SAD..ARABIC MATHEMATICAL TAILED QAF
- {0x1EE54, 0x1EE54, prN}, // Lo ARABIC MATHEMATICAL TAILED SHEEN
- {0x1EE57, 0x1EE57, prN}, // Lo ARABIC MATHEMATICAL TAILED KHAH
- {0x1EE59, 0x1EE59, prN}, // Lo ARABIC MATHEMATICAL TAILED DAD
- {0x1EE5B, 0x1EE5B, prN}, // Lo ARABIC MATHEMATICAL TAILED GHAIN
- {0x1EE5D, 0x1EE5D, prN}, // Lo ARABIC MATHEMATICAL TAILED DOTLESS NOON
- {0x1EE5F, 0x1EE5F, prN}, // Lo ARABIC MATHEMATICAL TAILED DOTLESS QAF
- {0x1EE61, 0x1EE62, prN}, // Lo [2] ARABIC MATHEMATICAL STRETCHED BEH..ARABIC MATHEMATICAL STRETCHED JEEM
- {0x1EE64, 0x1EE64, prN}, // Lo ARABIC MATHEMATICAL STRETCHED HEH
- {0x1EE67, 0x1EE6A, prN}, // Lo [4] ARABIC MATHEMATICAL STRETCHED HAH..ARABIC MATHEMATICAL STRETCHED KAF
- {0x1EE6C, 0x1EE72, prN}, // Lo [7] ARABIC MATHEMATICAL STRETCHED MEEM..ARABIC MATHEMATICAL STRETCHED QAF
- {0x1EE74, 0x1EE77, prN}, // Lo [4] ARABIC MATHEMATICAL STRETCHED SHEEN..ARABIC MATHEMATICAL STRETCHED KHAH
- {0x1EE79, 0x1EE7C, prN}, // Lo [4] ARABIC MATHEMATICAL STRETCHED DAD..ARABIC MATHEMATICAL STRETCHED DOTLESS BEH
- {0x1EE7E, 0x1EE7E, prN}, // Lo ARABIC MATHEMATICAL STRETCHED DOTLESS FEH
- {0x1EE80, 0x1EE89, prN}, // Lo [10] ARABIC MATHEMATICAL LOOPED ALEF..ARABIC MATHEMATICAL LOOPED YEH
- {0x1EE8B, 0x1EE9B, prN}, // Lo [17] ARABIC MATHEMATICAL LOOPED LAM..ARABIC MATHEMATICAL LOOPED GHAIN
- {0x1EEA1, 0x1EEA3, prN}, // Lo [3] ARABIC MATHEMATICAL DOUBLE-STRUCK BEH..ARABIC MATHEMATICAL DOUBLE-STRUCK DAL
- {0x1EEA5, 0x1EEA9, prN}, // Lo [5] ARABIC MATHEMATICAL DOUBLE-STRUCK WAW..ARABIC MATHEMATICAL DOUBLE-STRUCK YEH
- {0x1EEAB, 0x1EEBB, prN}, // Lo [17] ARABIC MATHEMATICAL DOUBLE-STRUCK LAM..ARABIC MATHEMATICAL DOUBLE-STRUCK GHAIN
- {0x1EEF0, 0x1EEF1, prN}, // Sm [2] ARABIC MATHEMATICAL OPERATOR MEEM WITH HAH WITH TATWEEL..ARABIC MATHEMATICAL OPERATOR HAH WITH DAL
- {0x1F000, 0x1F003, prN}, // So [4] MAHJONG TILE EAST WIND..MAHJONG TILE NORTH WIND
- {0x1F004, 0x1F004, prW}, // So MAHJONG TILE RED DRAGON
- {0x1F005, 0x1F02B, prN}, // So [39] MAHJONG TILE GREEN DRAGON..MAHJONG TILE BACK
- {0x1F030, 0x1F093, prN}, // So [100] DOMINO TILE HORIZONTAL BACK..DOMINO TILE VERTICAL-06-06
- {0x1F0A0, 0x1F0AE, prN}, // So [15] PLAYING CARD BACK..PLAYING CARD KING OF SPADES
- {0x1F0B1, 0x1F0BF, prN}, // So [15] PLAYING CARD ACE OF HEARTS..PLAYING CARD RED JOKER
- {0x1F0C1, 0x1F0CE, prN}, // So [14] PLAYING CARD ACE OF DIAMONDS..PLAYING CARD KING OF DIAMONDS
- {0x1F0CF, 0x1F0CF, prW}, // So PLAYING CARD BLACK JOKER
- {0x1F0D1, 0x1F0F5, prN}, // So [37] PLAYING CARD ACE OF CLUBS..PLAYING CARD TRUMP-21
- {0x1F100, 0x1F10A, prA}, // No [11] DIGIT ZERO FULL STOP..DIGIT NINE COMMA
- {0x1F10B, 0x1F10C, prN}, // No [2] DINGBAT CIRCLED SANS-SERIF DIGIT ZERO..DINGBAT NEGATIVE CIRCLED SANS-SERIF DIGIT ZERO
- {0x1F10D, 0x1F10F, prN}, // So [3] CIRCLED ZERO WITH SLASH..CIRCLED DOLLAR SIGN WITH OVERLAID BACKSLASH
- {0x1F110, 0x1F12D, prA}, // So [30] PARENTHESIZED LATIN CAPITAL LETTER A..CIRCLED CD
- {0x1F12E, 0x1F12F, prN}, // So [2] CIRCLED WZ..COPYLEFT SYMBOL
- {0x1F130, 0x1F169, prA}, // So [58] SQUARED LATIN CAPITAL LETTER A..NEGATIVE CIRCLED LATIN CAPITAL LETTER Z
- {0x1F16A, 0x1F16F, prN}, // So [6] RAISED MC SIGN..CIRCLED HUMAN FIGURE
- {0x1F170, 0x1F18D, prA}, // So [30] NEGATIVE SQUARED LATIN CAPITAL LETTER A..NEGATIVE SQUARED SA
- {0x1F18E, 0x1F18E, prW}, // So NEGATIVE SQUARED AB
- {0x1F18F, 0x1F190, prA}, // So [2] NEGATIVE SQUARED WC..SQUARE DJ
- {0x1F191, 0x1F19A, prW}, // So [10] SQUARED CL..SQUARED VS
- {0x1F19B, 0x1F1AC, prA}, // So [18] SQUARED THREE D..SQUARED VOD
- {0x1F1AD, 0x1F1AD, prN}, // So MASK WORK SYMBOL
- {0x1F1E6, 0x1F1FF, prN}, // So [26] REGIONAL INDICATOR SYMBOL LETTER A..REGIONAL INDICATOR SYMBOL LETTER Z
- {0x1F200, 0x1F202, prW}, // So [3] SQUARE HIRAGANA HOKA..SQUARED KATAKANA SA
- {0x1F210, 0x1F23B, prW}, // So [44] SQUARED CJK UNIFIED IDEOGRAPH-624B..SQUARED CJK UNIFIED IDEOGRAPH-914D
- {0x1F240, 0x1F248, prW}, // So [9] TORTOISE SHELL BRACKETED CJK UNIFIED IDEOGRAPH-672C..TORTOISE SHELL BRACKETED CJK UNIFIED IDEOGRAPH-6557
- {0x1F250, 0x1F251, prW}, // So [2] CIRCLED IDEOGRAPH ADVANTAGE..CIRCLED IDEOGRAPH ACCEPT
- {0x1F260, 0x1F265, prW}, // So [6] ROUNDED SYMBOL FOR FU..ROUNDED SYMBOL FOR CAI
- {0x1F300, 0x1F320, prW}, // So [33] CYCLONE..SHOOTING STAR
- {0x1F321, 0x1F32C, prN}, // So [12] THERMOMETER..WIND BLOWING FACE
- {0x1F32D, 0x1F335, prW}, // So [9] HOT DOG..CACTUS
- {0x1F336, 0x1F336, prN}, // So HOT PEPPER
- {0x1F337, 0x1F37C, prW}, // So [70] TULIP..BABY BOTTLE
- {0x1F37D, 0x1F37D, prN}, // So FORK AND KNIFE WITH PLATE
- {0x1F37E, 0x1F393, prW}, // So [22] BOTTLE WITH POPPING CORK..GRADUATION CAP
- {0x1F394, 0x1F39F, prN}, // So [12] HEART WITH TIP ON THE LEFT..ADMISSION TICKETS
- {0x1F3A0, 0x1F3CA, prW}, // So [43] CAROUSEL HORSE..SWIMMER
- {0x1F3CB, 0x1F3CE, prN}, // So [4] WEIGHT LIFTER..RACING CAR
- {0x1F3CF, 0x1F3D3, prW}, // So [5] CRICKET BAT AND BALL..TABLE TENNIS PADDLE AND BALL
- {0x1F3D4, 0x1F3DF, prN}, // So [12] SNOW CAPPED MOUNTAIN..STADIUM
- {0x1F3E0, 0x1F3F0, prW}, // So [17] HOUSE BUILDING..EUROPEAN CASTLE
- {0x1F3F1, 0x1F3F3, prN}, // So [3] WHITE PENNANT..WAVING WHITE FLAG
- {0x1F3F4, 0x1F3F4, prW}, // So WAVING BLACK FLAG
- {0x1F3F5, 0x1F3F7, prN}, // So [3] ROSETTE..LABEL
- {0x1F3F8, 0x1F3FA, prW}, // So [3] BADMINTON RACQUET AND SHUTTLECOCK..AMPHORA
- {0x1F3FB, 0x1F3FF, prW}, // Sk [5] EMOJI MODIFIER FITZPATRICK TYPE-1-2..EMOJI MODIFIER FITZPATRICK TYPE-6
- {0x1F400, 0x1F43E, prW}, // So [63] RAT..PAW PRINTS
- {0x1F43F, 0x1F43F, prN}, // So CHIPMUNK
- {0x1F440, 0x1F440, prW}, // So EYES
- {0x1F441, 0x1F441, prN}, // So EYE
- {0x1F442, 0x1F4FC, prW}, // So [187] EAR..VIDEOCASSETTE
- {0x1F4FD, 0x1F4FE, prN}, // So [2] FILM PROJECTOR..PORTABLE STEREO
- {0x1F4FF, 0x1F53D, prW}, // So [63] PRAYER BEADS..DOWN-POINTING SMALL RED TRIANGLE
- {0x1F53E, 0x1F54A, prN}, // So [13] LOWER RIGHT SHADOWED WHITE CIRCLE..DOVE OF PEACE
- {0x1F54B, 0x1F54E, prW}, // So [4] KAABA..MENORAH WITH NINE BRANCHES
- {0x1F54F, 0x1F54F, prN}, // So BOWL OF HYGIEIA
- {0x1F550, 0x1F567, prW}, // So [24] CLOCK FACE ONE OCLOCK..CLOCK FACE TWELVE-THIRTY
- {0x1F568, 0x1F579, prN}, // So [18] RIGHT SPEAKER..JOYSTICK
- {0x1F57A, 0x1F57A, prW}, // So MAN DANCING
- {0x1F57B, 0x1F594, prN}, // So [26] LEFT HAND TELEPHONE RECEIVER..REVERSED VICTORY HAND
- {0x1F595, 0x1F596, prW}, // So [2] REVERSED HAND WITH MIDDLE FINGER EXTENDED..RAISED HAND WITH PART BETWEEN MIDDLE AND RING FINGERS
- {0x1F597, 0x1F5A3, prN}, // So [13] WHITE DOWN POINTING LEFT HAND INDEX..BLACK DOWN POINTING BACKHAND INDEX
- {0x1F5A4, 0x1F5A4, prW}, // So BLACK HEART
- {0x1F5A5, 0x1F5FA, prN}, // So [86] DESKTOP COMPUTER..WORLD MAP
- {0x1F5FB, 0x1F5FF, prW}, // So [5] MOUNT FUJI..MOYAI
- {0x1F600, 0x1F64F, prW}, // So [80] GRINNING FACE..PERSON WITH FOLDED HANDS
- {0x1F650, 0x1F67F, prN}, // So [48] NORTH WEST POINTING LEAF..REVERSE CHECKER BOARD
- {0x1F680, 0x1F6C5, prW}, // So [70] ROCKET..LEFT LUGGAGE
- {0x1F6C6, 0x1F6CB, prN}, // So [6] TRIANGLE WITH ROUNDED CORNERS..COUCH AND LAMP
- {0x1F6CC, 0x1F6CC, prW}, // So SLEEPING ACCOMMODATION
- {0x1F6CD, 0x1F6CF, prN}, // So [3] SHOPPING BAGS..BED
- {0x1F6D0, 0x1F6D2, prW}, // So [3] PLACE OF WORSHIP..SHOPPING TROLLEY
- {0x1F6D3, 0x1F6D4, prN}, // So [2] STUPA..PAGODA
- {0x1F6D5, 0x1F6D7, prW}, // So [3] HINDU TEMPLE..ELEVATOR
- {0x1F6DD, 0x1F6DF, prW}, // So [3] PLAYGROUND SLIDE..RING BUOY
- {0x1F6E0, 0x1F6EA, prN}, // So [11] HAMMER AND WRENCH..NORTHEAST-POINTING AIRPLANE
- {0x1F6EB, 0x1F6EC, prW}, // So [2] AIRPLANE DEPARTURE..AIRPLANE ARRIVING
- {0x1F6F0, 0x1F6F3, prN}, // So [4] SATELLITE..PASSENGER SHIP
- {0x1F6F4, 0x1F6FC, prW}, // So [9] SCOOTER..ROLLER SKATE
- {0x1F700, 0x1F773, prN}, // So [116] ALCHEMICAL SYMBOL FOR QUINTESSENCE..ALCHEMICAL SYMBOL FOR HALF OUNCE
- {0x1F780, 0x1F7D8, prN}, // So [89] BLACK LEFT-POINTING ISOSCELES RIGHT TRIANGLE..NEGATIVE CIRCLED SQUARE
- {0x1F7E0, 0x1F7EB, prW}, // So [12] LARGE ORANGE CIRCLE..LARGE BROWN SQUARE
- {0x1F7F0, 0x1F7F0, prW}, // So HEAVY EQUALS SIGN
- {0x1F800, 0x1F80B, prN}, // So [12] LEFTWARDS ARROW WITH SMALL TRIANGLE ARROWHEAD..DOWNWARDS ARROW WITH LARGE TRIANGLE ARROWHEAD
- {0x1F810, 0x1F847, prN}, // So [56] LEFTWARDS ARROW WITH SMALL EQUILATERAL ARROWHEAD..DOWNWARDS HEAVY ARROW
- {0x1F850, 0x1F859, prN}, // So [10] LEFTWARDS SANS-SERIF ARROW..UP DOWN SANS-SERIF ARROW
- {0x1F860, 0x1F887, prN}, // So [40] WIDE-HEADED LEFTWARDS LIGHT BARB ARROW..WIDE-HEADED SOUTH WEST VERY HEAVY BARB ARROW
- {0x1F890, 0x1F8AD, prN}, // So [30] LEFTWARDS TRIANGLE ARROWHEAD..WHITE ARROW SHAFT WIDTH TWO THIRDS
- {0x1F8B0, 0x1F8B1, prN}, // So [2] ARROW POINTING UPWARDS THEN NORTH WEST..ARROW POINTING RIGHTWARDS THEN CURVING SOUTH WEST
- {0x1F900, 0x1F90B, prN}, // So [12] CIRCLED CROSS FORMEE WITH FOUR DOTS..DOWNWARD FACING NOTCHED HOOK WITH DOT
- {0x1F90C, 0x1F93A, prW}, // So [47] PINCHED FINGERS..FENCER
- {0x1F93B, 0x1F93B, prN}, // So MODERN PENTATHLON
- {0x1F93C, 0x1F945, prW}, // So [10] WRESTLERS..GOAL NET
- {0x1F946, 0x1F946, prN}, // So RIFLE
- {0x1F947, 0x1F9FF, prW}, // So [185] FIRST PLACE MEDAL..NAZAR AMULET
- {0x1FA00, 0x1FA53, prN}, // So [84] NEUTRAL CHESS KING..BLACK CHESS KNIGHT-BISHOP
- {0x1FA60, 0x1FA6D, prN}, // So [14] XIANGQI RED GENERAL..XIANGQI BLACK SOLDIER
- {0x1FA70, 0x1FA74, prW}, // So [5] BALLET SHOES..THONG SANDAL
- {0x1FA78, 0x1FA7C, prW}, // So [5] DROP OF BLOOD..CRUTCH
- {0x1FA80, 0x1FA86, prW}, // So [7] YO-YO..NESTING DOLLS
- {0x1FA90, 0x1FAAC, prW}, // So [29] RINGED PLANET..HAMSA
- {0x1FAB0, 0x1FABA, prW}, // So [11] FLY..NEST WITH EGGS
- {0x1FAC0, 0x1FAC5, prW}, // So [6] ANATOMICAL HEART..PERSON WITH CROWN
- {0x1FAD0, 0x1FAD9, prW}, // So [10] BLUEBERRIES..JAR
- {0x1FAE0, 0x1FAE7, prW}, // So [8] MELTING FACE..BUBBLES
- {0x1FAF0, 0x1FAF6, prW}, // So [7] HAND WITH INDEX FINGER AND THUMB CROSSED..HEART HANDS
- {0x1FB00, 0x1FB92, prN}, // So [147] BLOCK SEXTANT-1..UPPER HALF INVERSE MEDIUM SHADE AND LOWER HALF BLOCK
- {0x1FB94, 0x1FBCA, prN}, // So [55] LEFT HALF INVERSE MEDIUM SHADE AND RIGHT HALF BLOCK..WHITE UP-POINTING CHEVRON
- {0x1FBF0, 0x1FBF9, prN}, // Nd [10] SEGMENTED DIGIT ZERO..SEGMENTED DIGIT NINE
- {0x20000, 0x2A6DF, prW}, // Lo [42720] CJK UNIFIED IDEOGRAPH-20000..CJK UNIFIED IDEOGRAPH-2A6DF
- {0x2A6E0, 0x2A6FF, prW}, // Cn [32] ..
- {0x2A700, 0x2B738, prW}, // Lo [4153] CJK UNIFIED IDEOGRAPH-2A700..CJK UNIFIED IDEOGRAPH-2B738
- {0x2B739, 0x2B73F, prW}, // Cn [7] ..
- {0x2B740, 0x2B81D, prW}, // Lo [222] CJK UNIFIED IDEOGRAPH-2B740..CJK UNIFIED IDEOGRAPH-2B81D
- {0x2B81E, 0x2B81F, prW}, // Cn [2] ..
- {0x2B820, 0x2CEA1, prW}, // Lo [5762] CJK UNIFIED IDEOGRAPH-2B820..CJK UNIFIED IDEOGRAPH-2CEA1
- {0x2CEA2, 0x2CEAF, prW}, // Cn [14] ..
- {0x2CEB0, 0x2EBE0, prW}, // Lo [7473] CJK UNIFIED IDEOGRAPH-2CEB0..CJK UNIFIED IDEOGRAPH-2EBE0
- {0x2EBE1, 0x2F7FF, prW}, // Cn [3103] ..
- {0x2F800, 0x2FA1D, prW}, // Lo [542] CJK COMPATIBILITY IDEOGRAPH-2F800..CJK COMPATIBILITY IDEOGRAPH-2FA1D
- {0x2FA1E, 0x2FA1F, prW}, // Cn [2] ..
- {0x2FA20, 0x2FFFD, prW}, // Cn [1502] ..
- {0x30000, 0x3134A, prW}, // Lo [4939] CJK UNIFIED IDEOGRAPH-30000..CJK UNIFIED IDEOGRAPH-3134A
- {0x3134B, 0x3FFFD, prW}, // Cn [60595] ..
- {0xE0001, 0xE0001, prN}, // Cf LANGUAGE TAG
- {0xE0020, 0xE007F, prN}, // Cf [96] TAG SPACE..CANCEL TAG
- {0xE0100, 0xE01EF, prA}, // Mn [240] VARIATION SELECTOR-17..VARIATION SELECTOR-256
- {0xF0000, 0xFFFFD, prA}, // Co [65534] ..
- {0x100000, 0x10FFFD, prA}, // Co [65534] ..
-}
diff --git a/vendor/github.com/rivo/uniseg/emojipresentation.go b/vendor/github.com/rivo/uniseg/emojipresentation.go
deleted file mode 100644
index fd0f7451..00000000
--- a/vendor/github.com/rivo/uniseg/emojipresentation.go
+++ /dev/null
@@ -1,285 +0,0 @@
-package uniseg
-
-// Code generated via go generate from gen_properties.go. DO NOT EDIT.
-
-// emojiPresentation are taken from
-//
-// and
-// https://unicode.org/Public/14.0.0/ucd/emoji/emoji-data.txt
-// ("Extended_Pictographic" only)
-// on September 10, 2022. See https://www.unicode.org/license.html for the Unicode
-// license agreement.
-var emojiPresentation = [][3]int{
- {0x231A, 0x231B, prEmojiPresentation}, // E0.6 [2] (⌚..⌛) watch..hourglass done
- {0x23E9, 0x23EC, prEmojiPresentation}, // E0.6 [4] (â©..â¬) fast-forward button..fast down button
- {0x23F0, 0x23F0, prEmojiPresentation}, // E0.6 [1] (â°) alarm clock
- {0x23F3, 0x23F3, prEmojiPresentation}, // E0.6 [1] (â³) hourglass not done
- {0x25FD, 0x25FE, prEmojiPresentation}, // E0.6 [2] (â—½..â—¾) white medium-small square..black medium-small square
- {0x2614, 0x2615, prEmojiPresentation}, // E0.6 [2] (☔..☕) umbrella with rain drops..hot beverage
- {0x2648, 0x2653, prEmojiPresentation}, // E0.6 [12] (♈..♓) Aries..Pisces
- {0x267F, 0x267F, prEmojiPresentation}, // E0.6 [1] (♿) wheelchair symbol
- {0x2693, 0x2693, prEmojiPresentation}, // E0.6 [1] (âš“) anchor
- {0x26A1, 0x26A1, prEmojiPresentation}, // E0.6 [1] (âš¡) high voltage
- {0x26AA, 0x26AB, prEmojiPresentation}, // E0.6 [2] (⚪..⚫) white circle..black circle
- {0x26BD, 0x26BE, prEmojiPresentation}, // E0.6 [2] (âš½..âš¾) soccer ball..baseball
- {0x26C4, 0x26C5, prEmojiPresentation}, // E0.6 [2] (⛄..⛅) snowman without snow..sun behind cloud
- {0x26CE, 0x26CE, prEmojiPresentation}, // E0.6 [1] (⛎) Ophiuchus
- {0x26D4, 0x26D4, prEmojiPresentation}, // E0.6 [1] (â›”) no entry
- {0x26EA, 0x26EA, prEmojiPresentation}, // E0.6 [1] (⛪) church
- {0x26F2, 0x26F3, prEmojiPresentation}, // E0.6 [2] (⛲..⛳) fountain..flag in hole
- {0x26F5, 0x26F5, prEmojiPresentation}, // E0.6 [1] (⛵) sailboat
- {0x26FA, 0x26FA, prEmojiPresentation}, // E0.6 [1] (⛺) tent
- {0x26FD, 0x26FD, prEmojiPresentation}, // E0.6 [1] (⛽) fuel pump
- {0x2705, 0x2705, prEmojiPresentation}, // E0.6 [1] (✅) check mark button
- {0x270A, 0x270B, prEmojiPresentation}, // E0.6 [2] (✊..✋) raised fist..raised hand
- {0x2728, 0x2728, prEmojiPresentation}, // E0.6 [1] (✨) sparkles
- {0x274C, 0x274C, prEmojiPresentation}, // E0.6 [1] (âŒ) cross mark
- {0x274E, 0x274E, prEmojiPresentation}, // E0.6 [1] (âŽ) cross mark button
- {0x2753, 0x2755, prEmojiPresentation}, // E0.6 [3] (â“..â•) red question mark..white exclamation mark
- {0x2757, 0x2757, prEmojiPresentation}, // E0.6 [1] (â—) red exclamation mark
- {0x2795, 0x2797, prEmojiPresentation}, // E0.6 [3] (âž•..âž—) plus..divide
- {0x27B0, 0x27B0, prEmojiPresentation}, // E0.6 [1] (âž°) curly loop
- {0x27BF, 0x27BF, prEmojiPresentation}, // E1.0 [1] (âž¿) double curly loop
- {0x2B1B, 0x2B1C, prEmojiPresentation}, // E0.6 [2] (⬛..⬜) black large square..white large square
- {0x2B50, 0x2B50, prEmojiPresentation}, // E0.6 [1] (â) star
- {0x2B55, 0x2B55, prEmojiPresentation}, // E0.6 [1] (â•) hollow red circle
- {0x1F004, 0x1F004, prEmojiPresentation}, // E0.6 [1] (🀄) mahjong red dragon
- {0x1F0CF, 0x1F0CF, prEmojiPresentation}, // E0.6 [1] (ðŸƒ) joker
- {0x1F18E, 0x1F18E, prEmojiPresentation}, // E0.6 [1] (🆎) AB button (blood type)
- {0x1F191, 0x1F19A, prEmojiPresentation}, // E0.6 [10] (🆑..🆚) CL button..VS button
- {0x1F1E6, 0x1F1FF, prEmojiPresentation}, // E0.0 [26] (🇦..🇿) regional indicator symbol letter a..regional indicator symbol letter z
- {0x1F201, 0x1F201, prEmojiPresentation}, // E0.6 [1] (ðŸˆ) Japanese “here†button
- {0x1F21A, 0x1F21A, prEmojiPresentation}, // E0.6 [1] (🈚) Japanese “free of charge†button
- {0x1F22F, 0x1F22F, prEmojiPresentation}, // E0.6 [1] (🈯) Japanese “reserved†button
- {0x1F232, 0x1F236, prEmojiPresentation}, // E0.6 [5] (🈲..🈶) Japanese “prohibited†button..Japanese “not free of charge†button
- {0x1F238, 0x1F23A, prEmojiPresentation}, // E0.6 [3] (🈸..🈺) Japanese “application†button..Japanese “open for business†button
- {0x1F250, 0x1F251, prEmojiPresentation}, // E0.6 [2] (ðŸ‰..🉑) Japanese “bargain†button..Japanese “acceptable†button
- {0x1F300, 0x1F30C, prEmojiPresentation}, // E0.6 [13] (🌀..🌌) cyclone..milky way
- {0x1F30D, 0x1F30E, prEmojiPresentation}, // E0.7 [2] (ðŸŒ..🌎) globe showing Europe-Africa..globe showing Americas
- {0x1F30F, 0x1F30F, prEmojiPresentation}, // E0.6 [1] (ðŸŒ) globe showing Asia-Australia
- {0x1F310, 0x1F310, prEmojiPresentation}, // E1.0 [1] (ðŸŒ) globe with meridians
- {0x1F311, 0x1F311, prEmojiPresentation}, // E0.6 [1] (🌑) new moon
- {0x1F312, 0x1F312, prEmojiPresentation}, // E1.0 [1] (🌒) waxing crescent moon
- {0x1F313, 0x1F315, prEmojiPresentation}, // E0.6 [3] (🌓..🌕) first quarter moon..full moon
- {0x1F316, 0x1F318, prEmojiPresentation}, // E1.0 [3] (🌖..🌘) waning gibbous moon..waning crescent moon
- {0x1F319, 0x1F319, prEmojiPresentation}, // E0.6 [1] (🌙) crescent moon
- {0x1F31A, 0x1F31A, prEmojiPresentation}, // E1.0 [1] (🌚) new moon face
- {0x1F31B, 0x1F31B, prEmojiPresentation}, // E0.6 [1] (🌛) first quarter moon face
- {0x1F31C, 0x1F31C, prEmojiPresentation}, // E0.7 [1] (🌜) last quarter moon face
- {0x1F31D, 0x1F31E, prEmojiPresentation}, // E1.0 [2] (ðŸŒ..🌞) full moon face..sun with face
- {0x1F31F, 0x1F320, prEmojiPresentation}, // E0.6 [2] (🌟..🌠) glowing star..shooting star
- {0x1F32D, 0x1F32F, prEmojiPresentation}, // E1.0 [3] (ðŸŒ..🌯) hot dog..burrito
- {0x1F330, 0x1F331, prEmojiPresentation}, // E0.6 [2] (🌰..🌱) chestnut..seedling
- {0x1F332, 0x1F333, prEmojiPresentation}, // E1.0 [2] (🌲..🌳) evergreen tree..deciduous tree
- {0x1F334, 0x1F335, prEmojiPresentation}, // E0.6 [2] (🌴..🌵) palm tree..cactus
- {0x1F337, 0x1F34A, prEmojiPresentation}, // E0.6 [20] (🌷..ðŸŠ) tulip..tangerine
- {0x1F34B, 0x1F34B, prEmojiPresentation}, // E1.0 [1] (ðŸ‹) lemon
- {0x1F34C, 0x1F34F, prEmojiPresentation}, // E0.6 [4] (ðŸŒ..ðŸ) banana..green apple
- {0x1F350, 0x1F350, prEmojiPresentation}, // E1.0 [1] (ðŸ) pear
- {0x1F351, 0x1F37B, prEmojiPresentation}, // E0.6 [43] (ðŸ‘..ðŸ») peach..clinking beer mugs
- {0x1F37C, 0x1F37C, prEmojiPresentation}, // E1.0 [1] (ðŸ¼) baby bottle
- {0x1F37E, 0x1F37F, prEmojiPresentation}, // E1.0 [2] (ðŸ¾..ðŸ¿) bottle with popping cork..popcorn
- {0x1F380, 0x1F393, prEmojiPresentation}, // E0.6 [20] (🎀..🎓) ribbon..graduation cap
- {0x1F3A0, 0x1F3C4, prEmojiPresentation}, // E0.6 [37] (🎠..ðŸ„) carousel horse..person surfing
- {0x1F3C5, 0x1F3C5, prEmojiPresentation}, // E1.0 [1] (ðŸ…) sports medal
- {0x1F3C6, 0x1F3C6, prEmojiPresentation}, // E0.6 [1] (ðŸ†) trophy
- {0x1F3C7, 0x1F3C7, prEmojiPresentation}, // E1.0 [1] (ðŸ‡) horse racing
- {0x1F3C8, 0x1F3C8, prEmojiPresentation}, // E0.6 [1] (ðŸˆ) american football
- {0x1F3C9, 0x1F3C9, prEmojiPresentation}, // E1.0 [1] (ðŸ‰) rugby football
- {0x1F3CA, 0x1F3CA, prEmojiPresentation}, // E0.6 [1] (ðŸŠ) person swimming
- {0x1F3CF, 0x1F3D3, prEmojiPresentation}, // E1.0 [5] (ðŸ..ðŸ“) cricket game..ping pong
- {0x1F3E0, 0x1F3E3, prEmojiPresentation}, // E0.6 [4] (ðŸ ..ðŸ£) house..Japanese post office
- {0x1F3E4, 0x1F3E4, prEmojiPresentation}, // E1.0 [1] (ðŸ¤) post office
- {0x1F3E5, 0x1F3F0, prEmojiPresentation}, // E0.6 [12] (ðŸ¥..ðŸ°) hospital..castle
- {0x1F3F4, 0x1F3F4, prEmojiPresentation}, // E1.0 [1] (ðŸ´) black flag
- {0x1F3F8, 0x1F407, prEmojiPresentation}, // E1.0 [16] (ðŸ¸..ðŸ‡) badminton..rabbit
- {0x1F408, 0x1F408, prEmojiPresentation}, // E0.7 [1] (ðŸˆ) cat
- {0x1F409, 0x1F40B, prEmojiPresentation}, // E1.0 [3] (ðŸ‰..ðŸ‹) dragon..whale
- {0x1F40C, 0x1F40E, prEmojiPresentation}, // E0.6 [3] (ðŸŒ..ðŸŽ) snail..horse
- {0x1F40F, 0x1F410, prEmojiPresentation}, // E1.0 [2] (ðŸ..ðŸ) ram..goat
- {0x1F411, 0x1F412, prEmojiPresentation}, // E0.6 [2] (ðŸ‘..ðŸ’) ewe..monkey
- {0x1F413, 0x1F413, prEmojiPresentation}, // E1.0 [1] (ðŸ“) rooster
- {0x1F414, 0x1F414, prEmojiPresentation}, // E0.6 [1] (ðŸ”) chicken
- {0x1F415, 0x1F415, prEmojiPresentation}, // E0.7 [1] (ðŸ•) dog
- {0x1F416, 0x1F416, prEmojiPresentation}, // E1.0 [1] (ðŸ–) pig
- {0x1F417, 0x1F429, prEmojiPresentation}, // E0.6 [19] (ðŸ—..ðŸ©) boar..poodle
- {0x1F42A, 0x1F42A, prEmojiPresentation}, // E1.0 [1] (ðŸª) camel
- {0x1F42B, 0x1F43E, prEmojiPresentation}, // E0.6 [20] (ðŸ«..ðŸ¾) two-hump camel..paw prints
- {0x1F440, 0x1F440, prEmojiPresentation}, // E0.6 [1] (👀) eyes
- {0x1F442, 0x1F464, prEmojiPresentation}, // E0.6 [35] (👂..👤) ear..bust in silhouette
- {0x1F465, 0x1F465, prEmojiPresentation}, // E1.0 [1] (👥) busts in silhouette
- {0x1F466, 0x1F46B, prEmojiPresentation}, // E0.6 [6] (👦..👫) boy..woman and man holding hands
- {0x1F46C, 0x1F46D, prEmojiPresentation}, // E1.0 [2] (👬..ðŸ‘) men holding hands..women holding hands
- {0x1F46E, 0x1F4AC, prEmojiPresentation}, // E0.6 [63] (👮..💬) police officer..speech balloon
- {0x1F4AD, 0x1F4AD, prEmojiPresentation}, // E1.0 [1] (ðŸ’) thought balloon
- {0x1F4AE, 0x1F4B5, prEmojiPresentation}, // E0.6 [8] (💮..💵) white flower..dollar banknote
- {0x1F4B6, 0x1F4B7, prEmojiPresentation}, // E1.0 [2] (💶..💷) euro banknote..pound banknote
- {0x1F4B8, 0x1F4EB, prEmojiPresentation}, // E0.6 [52] (💸..📫) money with wings..closed mailbox with raised flag
- {0x1F4EC, 0x1F4ED, prEmojiPresentation}, // E0.7 [2] (📬..ðŸ“) open mailbox with raised flag..open mailbox with lowered flag
- {0x1F4EE, 0x1F4EE, prEmojiPresentation}, // E0.6 [1] (📮) postbox
- {0x1F4EF, 0x1F4EF, prEmojiPresentation}, // E1.0 [1] (📯) postal horn
- {0x1F4F0, 0x1F4F4, prEmojiPresentation}, // E0.6 [5] (📰..📴) newspaper..mobile phone off
- {0x1F4F5, 0x1F4F5, prEmojiPresentation}, // E1.0 [1] (📵) no mobile phones
- {0x1F4F6, 0x1F4F7, prEmojiPresentation}, // E0.6 [2] (📶..📷) antenna bars..camera
- {0x1F4F8, 0x1F4F8, prEmojiPresentation}, // E1.0 [1] (📸) camera with flash
- {0x1F4F9, 0x1F4FC, prEmojiPresentation}, // E0.6 [4] (📹..📼) video camera..videocassette
- {0x1F4FF, 0x1F502, prEmojiPresentation}, // E1.0 [4] (📿..🔂) prayer beads..repeat single button
- {0x1F503, 0x1F503, prEmojiPresentation}, // E0.6 [1] (🔃) clockwise vertical arrows
- {0x1F504, 0x1F507, prEmojiPresentation}, // E1.0 [4] (🔄..🔇) counterclockwise arrows button..muted speaker
- {0x1F508, 0x1F508, prEmojiPresentation}, // E0.7 [1] (🔈) speaker low volume
- {0x1F509, 0x1F509, prEmojiPresentation}, // E1.0 [1] (🔉) speaker medium volume
- {0x1F50A, 0x1F514, prEmojiPresentation}, // E0.6 [11] (🔊..🔔) speaker high volume..bell
- {0x1F515, 0x1F515, prEmojiPresentation}, // E1.0 [1] (🔕) bell with slash
- {0x1F516, 0x1F52B, prEmojiPresentation}, // E0.6 [22] (🔖..🔫) bookmark..water pistol
- {0x1F52C, 0x1F52D, prEmojiPresentation}, // E1.0 [2] (🔬..ðŸ”) microscope..telescope
- {0x1F52E, 0x1F53D, prEmojiPresentation}, // E0.6 [16] (🔮..🔽) crystal ball..downwards button
- {0x1F54B, 0x1F54E, prEmojiPresentation}, // E1.0 [4] (🕋..🕎) kaaba..menorah
- {0x1F550, 0x1F55B, prEmojiPresentation}, // E0.6 [12] (ðŸ•..🕛) one o’clock..twelve o’clock
- {0x1F55C, 0x1F567, prEmojiPresentation}, // E0.7 [12] (🕜..🕧) one-thirty..twelve-thirty
- {0x1F57A, 0x1F57A, prEmojiPresentation}, // E3.0 [1] (🕺) man dancing
- {0x1F595, 0x1F596, prEmojiPresentation}, // E1.0 [2] (🖕..🖖) middle finger..vulcan salute
- {0x1F5A4, 0x1F5A4, prEmojiPresentation}, // E3.0 [1] (🖤) black heart
- {0x1F5FB, 0x1F5FF, prEmojiPresentation}, // E0.6 [5] (🗻..🗿) mount fuji..moai
- {0x1F600, 0x1F600, prEmojiPresentation}, // E1.0 [1] (😀) grinning face
- {0x1F601, 0x1F606, prEmojiPresentation}, // E0.6 [6] (ðŸ˜..😆) beaming face with smiling eyes..grinning squinting face
- {0x1F607, 0x1F608, prEmojiPresentation}, // E1.0 [2] (😇..😈) smiling face with halo..smiling face with horns
- {0x1F609, 0x1F60D, prEmojiPresentation}, // E0.6 [5] (😉..ðŸ˜) winking face..smiling face with heart-eyes
- {0x1F60E, 0x1F60E, prEmojiPresentation}, // E1.0 [1] (😎) smiling face with sunglasses
- {0x1F60F, 0x1F60F, prEmojiPresentation}, // E0.6 [1] (ðŸ˜) smirking face
- {0x1F610, 0x1F610, prEmojiPresentation}, // E0.7 [1] (ðŸ˜) neutral face
- {0x1F611, 0x1F611, prEmojiPresentation}, // E1.0 [1] (😑) expressionless face
- {0x1F612, 0x1F614, prEmojiPresentation}, // E0.6 [3] (😒..😔) unamused face..pensive face
- {0x1F615, 0x1F615, prEmojiPresentation}, // E1.0 [1] (😕) confused face
- {0x1F616, 0x1F616, prEmojiPresentation}, // E0.6 [1] (😖) confounded face
- {0x1F617, 0x1F617, prEmojiPresentation}, // E1.0 [1] (😗) kissing face
- {0x1F618, 0x1F618, prEmojiPresentation}, // E0.6 [1] (😘) face blowing a kiss
- {0x1F619, 0x1F619, prEmojiPresentation}, // E1.0 [1] (😙) kissing face with smiling eyes
- {0x1F61A, 0x1F61A, prEmojiPresentation}, // E0.6 [1] (😚) kissing face with closed eyes
- {0x1F61B, 0x1F61B, prEmojiPresentation}, // E1.0 [1] (😛) face with tongue
- {0x1F61C, 0x1F61E, prEmojiPresentation}, // E0.6 [3] (😜..😞) winking face with tongue..disappointed face
- {0x1F61F, 0x1F61F, prEmojiPresentation}, // E1.0 [1] (😟) worried face
- {0x1F620, 0x1F625, prEmojiPresentation}, // E0.6 [6] (😠..😥) angry face..sad but relieved face
- {0x1F626, 0x1F627, prEmojiPresentation}, // E1.0 [2] (😦..😧) frowning face with open mouth..anguished face
- {0x1F628, 0x1F62B, prEmojiPresentation}, // E0.6 [4] (😨..😫) fearful face..tired face
- {0x1F62C, 0x1F62C, prEmojiPresentation}, // E1.0 [1] (😬) grimacing face
- {0x1F62D, 0x1F62D, prEmojiPresentation}, // E0.6 [1] (ðŸ˜) loudly crying face
- {0x1F62E, 0x1F62F, prEmojiPresentation}, // E1.0 [2] (😮..😯) face with open mouth..hushed face
- {0x1F630, 0x1F633, prEmojiPresentation}, // E0.6 [4] (😰..😳) anxious face with sweat..flushed face
- {0x1F634, 0x1F634, prEmojiPresentation}, // E1.0 [1] (😴) sleeping face
- {0x1F635, 0x1F635, prEmojiPresentation}, // E0.6 [1] (😵) face with crossed-out eyes
- {0x1F636, 0x1F636, prEmojiPresentation}, // E1.0 [1] (😶) face without mouth
- {0x1F637, 0x1F640, prEmojiPresentation}, // E0.6 [10] (😷..🙀) face with medical mask..weary cat
- {0x1F641, 0x1F644, prEmojiPresentation}, // E1.0 [4] (ðŸ™..🙄) slightly frowning face..face with rolling eyes
- {0x1F645, 0x1F64F, prEmojiPresentation}, // E0.6 [11] (🙅..ðŸ™) person gesturing NO..folded hands
- {0x1F680, 0x1F680, prEmojiPresentation}, // E0.6 [1] (🚀) rocket
- {0x1F681, 0x1F682, prEmojiPresentation}, // E1.0 [2] (ðŸš..🚂) helicopter..locomotive
- {0x1F683, 0x1F685, prEmojiPresentation}, // E0.6 [3] (🚃..🚅) railway car..bullet train
- {0x1F686, 0x1F686, prEmojiPresentation}, // E1.0 [1] (🚆) train
- {0x1F687, 0x1F687, prEmojiPresentation}, // E0.6 [1] (🚇) metro
- {0x1F688, 0x1F688, prEmojiPresentation}, // E1.0 [1] (🚈) light rail
- {0x1F689, 0x1F689, prEmojiPresentation}, // E0.6 [1] (🚉) station
- {0x1F68A, 0x1F68B, prEmojiPresentation}, // E1.0 [2] (🚊..🚋) tram..tram car
- {0x1F68C, 0x1F68C, prEmojiPresentation}, // E0.6 [1] (🚌) bus
- {0x1F68D, 0x1F68D, prEmojiPresentation}, // E0.7 [1] (ðŸš) oncoming bus
- {0x1F68E, 0x1F68E, prEmojiPresentation}, // E1.0 [1] (🚎) trolleybus
- {0x1F68F, 0x1F68F, prEmojiPresentation}, // E0.6 [1] (ðŸš) bus stop
- {0x1F690, 0x1F690, prEmojiPresentation}, // E1.0 [1] (ðŸš) minibus
- {0x1F691, 0x1F693, prEmojiPresentation}, // E0.6 [3] (🚑..🚓) ambulance..police car
- {0x1F694, 0x1F694, prEmojiPresentation}, // E0.7 [1] (🚔) oncoming police car
- {0x1F695, 0x1F695, prEmojiPresentation}, // E0.6 [1] (🚕) taxi
- {0x1F696, 0x1F696, prEmojiPresentation}, // E1.0 [1] (🚖) oncoming taxi
- {0x1F697, 0x1F697, prEmojiPresentation}, // E0.6 [1] (🚗) automobile
- {0x1F698, 0x1F698, prEmojiPresentation}, // E0.7 [1] (🚘) oncoming automobile
- {0x1F699, 0x1F69A, prEmojiPresentation}, // E0.6 [2] (🚙..🚚) sport utility vehicle..delivery truck
- {0x1F69B, 0x1F6A1, prEmojiPresentation}, // E1.0 [7] (🚛..🚡) articulated lorry..aerial tramway
- {0x1F6A2, 0x1F6A2, prEmojiPresentation}, // E0.6 [1] (🚢) ship
- {0x1F6A3, 0x1F6A3, prEmojiPresentation}, // E1.0 [1] (🚣) person rowing boat
- {0x1F6A4, 0x1F6A5, prEmojiPresentation}, // E0.6 [2] (🚤..🚥) speedboat..horizontal traffic light
- {0x1F6A6, 0x1F6A6, prEmojiPresentation}, // E1.0 [1] (🚦) vertical traffic light
- {0x1F6A7, 0x1F6AD, prEmojiPresentation}, // E0.6 [7] (🚧..ðŸš) construction..no smoking
- {0x1F6AE, 0x1F6B1, prEmojiPresentation}, // E1.0 [4] (🚮..🚱) litter in bin sign..non-potable water
- {0x1F6B2, 0x1F6B2, prEmojiPresentation}, // E0.6 [1] (🚲) bicycle
- {0x1F6B3, 0x1F6B5, prEmojiPresentation}, // E1.0 [3] (🚳..🚵) no bicycles..person mountain biking
- {0x1F6B6, 0x1F6B6, prEmojiPresentation}, // E0.6 [1] (🚶) person walking
- {0x1F6B7, 0x1F6B8, prEmojiPresentation}, // E1.0 [2] (🚷..🚸) no pedestrians..children crossing
- {0x1F6B9, 0x1F6BE, prEmojiPresentation}, // E0.6 [6] (🚹..🚾) men’s room..water closet
- {0x1F6BF, 0x1F6BF, prEmojiPresentation}, // E1.0 [1] (🚿) shower
- {0x1F6C0, 0x1F6C0, prEmojiPresentation}, // E0.6 [1] (🛀) person taking bath
- {0x1F6C1, 0x1F6C5, prEmojiPresentation}, // E1.0 [5] (ðŸ›..🛅) bathtub..left luggage
- {0x1F6CC, 0x1F6CC, prEmojiPresentation}, // E1.0 [1] (🛌) person in bed
- {0x1F6D0, 0x1F6D0, prEmojiPresentation}, // E1.0 [1] (ðŸ›) place of worship
- {0x1F6D1, 0x1F6D2, prEmojiPresentation}, // E3.0 [2] (🛑..🛒) stop sign..shopping cart
- {0x1F6D5, 0x1F6D5, prEmojiPresentation}, // E12.0 [1] (🛕) hindu temple
- {0x1F6D6, 0x1F6D7, prEmojiPresentation}, // E13.0 [2] (🛖..🛗) hut..elevator
- {0x1F6DD, 0x1F6DF, prEmojiPresentation}, // E14.0 [3] (ðŸ›..🛟) playground slide..ring buoy
- {0x1F6EB, 0x1F6EC, prEmojiPresentation}, // E1.0 [2] (🛫..🛬) airplane departure..airplane arrival
- {0x1F6F4, 0x1F6F6, prEmojiPresentation}, // E3.0 [3] (🛴..🛶) kick scooter..canoe
- {0x1F6F7, 0x1F6F8, prEmojiPresentation}, // E5.0 [2] (🛷..🛸) sled..flying saucer
- {0x1F6F9, 0x1F6F9, prEmojiPresentation}, // E11.0 [1] (🛹) skateboard
- {0x1F6FA, 0x1F6FA, prEmojiPresentation}, // E12.0 [1] (🛺) auto rickshaw
- {0x1F6FB, 0x1F6FC, prEmojiPresentation}, // E13.0 [2] (🛻..🛼) pickup truck..roller skate
- {0x1F7E0, 0x1F7EB, prEmojiPresentation}, // E12.0 [12] (🟠..🟫) orange circle..brown square
- {0x1F7F0, 0x1F7F0, prEmojiPresentation}, // E14.0 [1] (🟰) heavy equals sign
- {0x1F90C, 0x1F90C, prEmojiPresentation}, // E13.0 [1] (🤌) pinched fingers
- {0x1F90D, 0x1F90F, prEmojiPresentation}, // E12.0 [3] (ðŸ¤..ðŸ¤) white heart..pinching hand
- {0x1F910, 0x1F918, prEmojiPresentation}, // E1.0 [9] (ðŸ¤..🤘) zipper-mouth face..sign of the horns
- {0x1F919, 0x1F91E, prEmojiPresentation}, // E3.0 [6] (🤙..🤞) call me hand..crossed fingers
- {0x1F91F, 0x1F91F, prEmojiPresentation}, // E5.0 [1] (🤟) love-you gesture
- {0x1F920, 0x1F927, prEmojiPresentation}, // E3.0 [8] (🤠..🤧) cowboy hat face..sneezing face
- {0x1F928, 0x1F92F, prEmojiPresentation}, // E5.0 [8] (🤨..🤯) face with raised eyebrow..exploding head
- {0x1F930, 0x1F930, prEmojiPresentation}, // E3.0 [1] (🤰) pregnant woman
- {0x1F931, 0x1F932, prEmojiPresentation}, // E5.0 [2] (🤱..🤲) breast-feeding..palms up together
- {0x1F933, 0x1F93A, prEmojiPresentation}, // E3.0 [8] (🤳..🤺) selfie..person fencing
- {0x1F93C, 0x1F93E, prEmojiPresentation}, // E3.0 [3] (🤼..🤾) people wrestling..person playing handball
- {0x1F93F, 0x1F93F, prEmojiPresentation}, // E12.0 [1] (🤿) diving mask
- {0x1F940, 0x1F945, prEmojiPresentation}, // E3.0 [6] (🥀..🥅) wilted flower..goal net
- {0x1F947, 0x1F94B, prEmojiPresentation}, // E3.0 [5] (🥇..🥋) 1st place medal..martial arts uniform
- {0x1F94C, 0x1F94C, prEmojiPresentation}, // E5.0 [1] (🥌) curling stone
- {0x1F94D, 0x1F94F, prEmojiPresentation}, // E11.0 [3] (ðŸ¥..ðŸ¥) lacrosse..flying disc
- {0x1F950, 0x1F95E, prEmojiPresentation}, // E3.0 [15] (ðŸ¥..🥞) croissant..pancakes
- {0x1F95F, 0x1F96B, prEmojiPresentation}, // E5.0 [13] (🥟..🥫) dumpling..canned food
- {0x1F96C, 0x1F970, prEmojiPresentation}, // E11.0 [5] (🥬..🥰) leafy green..smiling face with hearts
- {0x1F971, 0x1F971, prEmojiPresentation}, // E12.0 [1] (🥱) yawning face
- {0x1F972, 0x1F972, prEmojiPresentation}, // E13.0 [1] (🥲) smiling face with tear
- {0x1F973, 0x1F976, prEmojiPresentation}, // E11.0 [4] (🥳..🥶) partying face..cold face
- {0x1F977, 0x1F978, prEmojiPresentation}, // E13.0 [2] (🥷..🥸) ninja..disguised face
- {0x1F979, 0x1F979, prEmojiPresentation}, // E14.0 [1] (🥹) face holding back tears
- {0x1F97A, 0x1F97A, prEmojiPresentation}, // E11.0 [1] (🥺) pleading face
- {0x1F97B, 0x1F97B, prEmojiPresentation}, // E12.0 [1] (🥻) sari
- {0x1F97C, 0x1F97F, prEmojiPresentation}, // E11.0 [4] (🥼..🥿) lab coat..flat shoe
- {0x1F980, 0x1F984, prEmojiPresentation}, // E1.0 [5] (🦀..🦄) crab..unicorn
- {0x1F985, 0x1F991, prEmojiPresentation}, // E3.0 [13] (🦅..🦑) eagle..squid
- {0x1F992, 0x1F997, prEmojiPresentation}, // E5.0 [6] (🦒..🦗) giraffe..cricket
- {0x1F998, 0x1F9A2, prEmojiPresentation}, // E11.0 [11] (🦘..🦢) kangaroo..swan
- {0x1F9A3, 0x1F9A4, prEmojiPresentation}, // E13.0 [2] (🦣..🦤) mammoth..dodo
- {0x1F9A5, 0x1F9AA, prEmojiPresentation}, // E12.0 [6] (🦥..🦪) sloth..oyster
- {0x1F9AB, 0x1F9AD, prEmojiPresentation}, // E13.0 [3] (🦫..ðŸ¦) beaver..seal
- {0x1F9AE, 0x1F9AF, prEmojiPresentation}, // E12.0 [2] (🦮..🦯) guide dog..white cane
- {0x1F9B0, 0x1F9B9, prEmojiPresentation}, // E11.0 [10] (🦰..🦹) red hair..supervillain
- {0x1F9BA, 0x1F9BF, prEmojiPresentation}, // E12.0 [6] (🦺..🦿) safety vest..mechanical leg
- {0x1F9C0, 0x1F9C0, prEmojiPresentation}, // E1.0 [1] (🧀) cheese wedge
- {0x1F9C1, 0x1F9C2, prEmojiPresentation}, // E11.0 [2] (ðŸ§..🧂) cupcake..salt
- {0x1F9C3, 0x1F9CA, prEmojiPresentation}, // E12.0 [8] (🧃..🧊) beverage box..ice
- {0x1F9CB, 0x1F9CB, prEmojiPresentation}, // E13.0 [1] (🧋) bubble tea
- {0x1F9CC, 0x1F9CC, prEmojiPresentation}, // E14.0 [1] (🧌) troll
- {0x1F9CD, 0x1F9CF, prEmojiPresentation}, // E12.0 [3] (ðŸ§..ðŸ§) person standing..deaf person
- {0x1F9D0, 0x1F9E6, prEmojiPresentation}, // E5.0 [23] (ðŸ§..🧦) face with monocle..socks
- {0x1F9E7, 0x1F9FF, prEmojiPresentation}, // E11.0 [25] (🧧..🧿) red envelope..nazar amulet
- {0x1FA70, 0x1FA73, prEmojiPresentation}, // E12.0 [4] (🩰..🩳) ballet shoes..shorts
- {0x1FA74, 0x1FA74, prEmojiPresentation}, // E13.0 [1] (🩴) thong sandal
- {0x1FA78, 0x1FA7A, prEmojiPresentation}, // E12.0 [3] (🩸..🩺) drop of blood..stethoscope
- {0x1FA7B, 0x1FA7C, prEmojiPresentation}, // E14.0 [2] (🩻..🩼) x-ray..crutch
- {0x1FA80, 0x1FA82, prEmojiPresentation}, // E12.0 [3] (🪀..🪂) yo-yo..parachute
- {0x1FA83, 0x1FA86, prEmojiPresentation}, // E13.0 [4] (🪃..🪆) boomerang..nesting dolls
- {0x1FA90, 0x1FA95, prEmojiPresentation}, // E12.0 [6] (ðŸª..🪕) ringed planet..banjo
- {0x1FA96, 0x1FAA8, prEmojiPresentation}, // E13.0 [19] (🪖..🪨) military helmet..rock
- {0x1FAA9, 0x1FAAC, prEmojiPresentation}, // E14.0 [4] (🪩..🪬) mirror ball..hamsa
- {0x1FAB0, 0x1FAB6, prEmojiPresentation}, // E13.0 [7] (🪰..🪶) fly..feather
- {0x1FAB7, 0x1FABA, prEmojiPresentation}, // E14.0 [4] (🪷..🪺) lotus..nest with eggs
- {0x1FAC0, 0x1FAC2, prEmojiPresentation}, // E13.0 [3] (🫀..🫂) anatomical heart..people hugging
- {0x1FAC3, 0x1FAC5, prEmojiPresentation}, // E14.0 [3] (🫃..🫅) pregnant man..person with crown
- {0x1FAD0, 0x1FAD6, prEmojiPresentation}, // E13.0 [7] (ðŸ«..🫖) blueberries..teapot
- {0x1FAD7, 0x1FAD9, prEmojiPresentation}, // E14.0 [3] (🫗..🫙) pouring liquid..jar
- {0x1FAE0, 0x1FAE7, prEmojiPresentation}, // E14.0 [8] (🫠..🫧) melting face..bubbles
- {0x1FAF0, 0x1FAF6, prEmojiPresentation}, // E14.0 [7] (🫰..🫶) hand with index finger and thumb crossed..heart hands
-}
diff --git a/vendor/github.com/rivo/uniseg/gen_breaktest.go b/vendor/github.com/rivo/uniseg/gen_breaktest.go
deleted file mode 100644
index e613c4cd..00000000
--- a/vendor/github.com/rivo/uniseg/gen_breaktest.go
+++ /dev/null
@@ -1,213 +0,0 @@
-//go:build generate
-
-// This program generates a Go containing a slice of test cases based on the
-// Unicode Character Database auxiliary data files. The command line arguments
-// are as follows:
-//
-// 1. The name of the Unicode data file (just the filename, without extension).
-// 2. The name of the locally generated Go file.
-// 3. The name of the slice containing the test cases.
-// 4. The name of the generator, for logging purposes.
-//
-//go:generate go run gen_breaktest.go GraphemeBreakTest graphemebreak_test.go graphemeBreakTestCases graphemes
-//go:generate go run gen_breaktest.go WordBreakTest wordbreak_test.go wordBreakTestCases words
-//go:generate go run gen_breaktest.go SentenceBreakTest sentencebreak_test.go sentenceBreakTestCases sentences
-//go:generate go run gen_breaktest.go LineBreakTest linebreak_test.go lineBreakTestCases lines
-
-package main
-
-import (
- "bufio"
- "bytes"
- "errors"
- "fmt"
- "go/format"
- "io/ioutil"
- "log"
- "net/http"
- "os"
- "time"
-)
-
-// We want to test against a specific version rather than the latest. When the
-// package is upgraded to a new version, change these to generate new tests.
-const (
- testCaseURL = `https://www.unicode.org/Public/14.0.0/ucd/auxiliary/%s.txt`
-)
-
-func main() {
- if len(os.Args) < 5 {
- fmt.Println("Not enough arguments, see code for details")
- os.Exit(1)
- }
-
- log.SetPrefix("gen_breaktest (" + os.Args[4] + "): ")
- log.SetFlags(0)
-
- // Read text of testcases and parse into Go source code.
- src, err := parse(fmt.Sprintf(testCaseURL, os.Args[1]))
- if err != nil {
- log.Fatal(err)
- }
-
- // Format the Go code.
- formatted, err := format.Source(src)
- if err != nil {
- log.Fatalln("gofmt:", err)
- }
-
- // Write it out.
- log.Print("Writing to ", os.Args[2])
- if err := ioutil.WriteFile(os.Args[2], formatted, 0644); err != nil {
- log.Fatal(err)
- }
-}
-
-// parse reads a break text file, either from a local file or from a URL. It
-// parses the file data into Go source code representing the test cases.
-func parse(url string) ([]byte, error) {
- log.Printf("Parsing %s", url)
- res, err := http.Get(url)
- if err != nil {
- return nil, err
- }
- body := res.Body
- defer body.Close()
-
- buf := new(bytes.Buffer)
- buf.Grow(120 << 10)
- buf.WriteString(`package uniseg
-
-// Code generated via go generate from gen_breaktest.go. DO NOT EDIT.
-
-// ` + os.Args[3] + ` are Grapheme testcases taken from
-// ` + url + `
-// on ` + time.Now().Format("January 2, 2006") + `. See
-// https://www.unicode.org/license.html for the Unicode license agreement.
-var ` + os.Args[3] + ` = []testCase {
-`)
-
- sc := bufio.NewScanner(body)
- num := 1
- var line []byte
- original := make([]byte, 0, 64)
- expected := make([]byte, 0, 64)
- for sc.Scan() {
- num++
- line = sc.Bytes()
- if len(line) == 0 || line[0] == '#' {
- continue
- }
- var comment []byte
- if i := bytes.IndexByte(line, '#'); i >= 0 {
- comment = bytes.TrimSpace(line[i+1:])
- line = bytes.TrimSpace(line[:i])
- }
- original, expected, err := parseRuneSequence(line, original[:0], expected[:0])
- if err != nil {
- return nil, fmt.Errorf(`line %d: %v: %q`, num, err, line)
- }
- fmt.Fprintf(buf, "\t{original: \"%s\", expected: %s}, // %s\n", original, expected, comment)
- }
- if err := sc.Err(); err != nil {
- return nil, err
- }
-
- // Check for final "# EOF", useful check if we're streaming via HTTP
- if !bytes.Equal(line, []byte("# EOF")) {
- return nil, fmt.Errorf(`line %d: exected "# EOF" as final line, got %q`, num, line)
- }
- buf.WriteString("}\n")
- return buf.Bytes(), nil
-}
-
-// Used by parseRuneSequence to match input via bytes.HasPrefix.
-var (
- prefixBreak = []byte("÷ ")
- prefixDontBreak = []byte("× ")
- breakOk = []byte("÷")
- breakNo = []byte("×")
-)
-
-// parseRuneSequence parses a rune + breaking opportunity sequence from b
-// and appends the Go code for testcase.original to orig
-// and appends the Go code for testcase.expected to exp.
-// It retuns the new orig and exp slices.
-//
-// E.g. for the input b="÷ 0020 × 0308 ÷ 1F1E6 ÷"
-// it will append
-// "\u0020\u0308\U0001F1E6"
-// and "[][]rune{{0x0020,0x0308},{0x1F1E6},}"
-// to orig and exp respectively.
-//
-// The formatting of exp is expected to be cleaned up by gofmt or format.Source.
-// Note we explicitly require the sequence to start with ÷ and we implicitly
-// require it to end with ÷.
-func parseRuneSequence(b, orig, exp []byte) ([]byte, []byte, error) {
- // Check for and remove first ÷ or ×.
- if !bytes.HasPrefix(b, prefixBreak) && !bytes.HasPrefix(b, prefixDontBreak) {
- return nil, nil, errors.New("expected ÷ or × as first character")
- }
- if bytes.HasPrefix(b, prefixBreak) {
- b = b[len(prefixBreak):]
- } else {
- b = b[len(prefixDontBreak):]
- }
-
- boundary := true
- exp = append(exp, "[][]rune{"...)
- for len(b) > 0 {
- if boundary {
- exp = append(exp, '{')
- }
- exp = append(exp, "0x"...)
- // Find end of hex digits.
- var i int
- for i = 0; i < len(b) && b[i] != ' '; i++ {
- if d := b[i]; ('0' <= d || d <= '9') ||
- ('A' <= d || d <= 'F') ||
- ('a' <= d || d <= 'f') {
- continue
- }
- return nil, nil, errors.New("bad hex digit")
- }
- switch i {
- case 4:
- orig = append(orig, "\\u"...)
- case 5:
- orig = append(orig, "\\U000"...)
- default:
- return nil, nil, errors.New("unsupport code point hex length")
- }
- orig = append(orig, b[:i]...)
- exp = append(exp, b[:i]...)
- b = b[i:]
-
- // Check for space between hex and ÷ or ×.
- if len(b) < 1 || b[0] != ' ' {
- return nil, nil, errors.New("bad input")
- }
- b = b[1:]
-
- // Check for next boundary.
- switch {
- case bytes.HasPrefix(b, breakOk):
- boundary = true
- b = b[len(breakOk):]
- case bytes.HasPrefix(b, breakNo):
- boundary = false
- b = b[len(breakNo):]
- default:
- return nil, nil, errors.New("missing ÷ or ×")
- }
- if boundary {
- exp = append(exp, '}')
- }
- exp = append(exp, ',')
- if len(b) > 0 && b[0] == ' ' {
- b = b[1:]
- }
- }
- exp = append(exp, '}')
- return orig, exp, nil
-}
diff --git a/vendor/github.com/rivo/uniseg/gen_properties.go b/vendor/github.com/rivo/uniseg/gen_properties.go
deleted file mode 100644
index 999d5efd..00000000
--- a/vendor/github.com/rivo/uniseg/gen_properties.go
+++ /dev/null
@@ -1,256 +0,0 @@
-//go:build generate
-
-// This program generates a property file in Go file from Unicode Character
-// Database auxiliary data files. The command line arguments are as follows:
-//
-// 1. The name of the Unicode data file (just the filename, without extension).
-// Can be "-" (to skip) if the emoji flag is included.
-// 2. The name of the locally generated Go file.
-// 3. The name of the slice mapping code points to properties.
-// 4. The name of the generator, for logging purposes.
-// 5. (Optional) Flags, comma-separated. The following flags are available:
-// - "emojis=": include the specified emoji properties (e.g.
-// "Extended_Pictographic").
-// - "gencat": include general category properties.
-//
-//go:generate go run gen_properties.go auxiliary/GraphemeBreakProperty graphemeproperties.go graphemeCodePoints graphemes emojis=Extended_Pictographic
-//go:generate go run gen_properties.go auxiliary/WordBreakProperty wordproperties.go workBreakCodePoints words emojis=Extended_Pictographic
-//go:generate go run gen_properties.go auxiliary/SentenceBreakProperty sentenceproperties.go sentenceBreakCodePoints sentences
-//go:generate go run gen_properties.go LineBreak lineproperties.go lineBreakCodePoints lines gencat
-//go:generate go run gen_properties.go EastAsianWidth eastasianwidth.go eastAsianWidth eastasianwidth
-//go:generate go run gen_properties.go - emojipresentation.go emojiPresentation emojipresentation emojis=Emoji_Presentation
-package main
-
-import (
- "bufio"
- "bytes"
- "errors"
- "fmt"
- "go/format"
- "io/ioutil"
- "log"
- "net/http"
- "os"
- "regexp"
- "sort"
- "strconv"
- "strings"
- "time"
-)
-
-// We want to test against a specific version rather than the latest. When the
-// package is upgraded to a new version, change these to generate new tests.
-const (
- propertyURL = `https://www.unicode.org/Public/14.0.0/ucd/%s.txt`
- emojiURL = `https://unicode.org/Public/14.0.0/ucd/emoji/emoji-data.txt`
-)
-
-// The regular expression for a line containing a code point range property.
-var propertyPattern = regexp.MustCompile(`^([0-9A-F]{4,6})(\.\.([0-9A-F]{4,6}))?\s*;\s*([A-Za-z0-9_]+)\s*#\s(.+)$`)
-
-func main() {
- if len(os.Args) < 5 {
- fmt.Println("Not enough arguments, see code for details")
- os.Exit(1)
- }
-
- log.SetPrefix("gen_properties (" + os.Args[4] + "): ")
- log.SetFlags(0)
-
- // Parse flags.
- flags := make(map[string]string)
- if len(os.Args) >= 6 {
- for _, flag := range strings.Split(os.Args[5], ",") {
- flagFields := strings.Split(flag, "=")
- if len(flagFields) == 1 {
- flags[flagFields[0]] = "yes"
- } else {
- flags[flagFields[0]] = flagFields[1]
- }
- }
- }
-
- // Parse the text file and generate Go source code from it.
- _, includeGeneralCategory := flags["gencat"]
- var mainURL string
- if os.Args[1] != "-" {
- mainURL = fmt.Sprintf(propertyURL, os.Args[1])
- }
- src, err := parse(mainURL, flags["emojis"], includeGeneralCategory)
- if err != nil {
- log.Fatal(err)
- }
-
- // Format the Go code.
- formatted, err := format.Source([]byte(src))
- if err != nil {
- log.Fatal("gofmt:", err)
- }
-
- // Save it to the (local) target file.
- log.Print("Writing to ", os.Args[2])
- if err := ioutil.WriteFile(os.Args[2], formatted, 0644); err != nil {
- log.Fatal(err)
- }
-}
-
-// parse parses the Unicode Properties text files located at the given URLs and
-// returns their equivalent Go source code to be used in the uniseg package. If
-// "emojiProperty" is not an empty string, emoji code points for that emoji
-// property (e.g. "Extended_Pictographic") will be included. In those cases, you
-// may pass an empty "propertyURL" to skip parsing the main properties file. If
-// "includeGeneralCategory" is true, the Unicode General Category property will
-// be extracted from the comments and included in the output.
-func parse(propertyURL, emojiProperty string, includeGeneralCategory bool) (string, error) {
- if propertyURL == "" && emojiProperty == "" {
- return "", errors.New("no properties to parse")
- }
-
- // Temporary buffer to hold properties.
- var properties [][4]string
-
- // Open the first URL.
- if propertyURL != "" {
- log.Printf("Parsing %s", propertyURL)
- res, err := http.Get(propertyURL)
- if err != nil {
- return "", err
- }
- in1 := res.Body
- defer in1.Close()
-
- // Parse it.
- scanner := bufio.NewScanner(in1)
- num := 0
- for scanner.Scan() {
- num++
- line := strings.TrimSpace(scanner.Text())
-
- // Skip comments and empty lines.
- if strings.HasPrefix(line, "#") || line == "" {
- continue
- }
-
- // Everything else must be a code point range, a property and a comment.
- from, to, property, comment, err := parseProperty(line)
- if err != nil {
- return "", fmt.Errorf("%s line %d: %v", os.Args[4], num, err)
- }
- properties = append(properties, [4]string{from, to, property, comment})
- }
- if err := scanner.Err(); err != nil {
- return "", err
- }
- }
-
- // Open the second URL.
- if emojiProperty != "" {
- log.Printf("Parsing %s", emojiURL)
- res, err := http.Get(emojiURL)
- if err != nil {
- return "", err
- }
- in2 := res.Body
- defer in2.Close()
-
- // Parse it.
- scanner := bufio.NewScanner(in2)
- num := 0
- for scanner.Scan() {
- num++
- line := scanner.Text()
-
- // Skip comments, empty lines, and everything not containing
- // "Extended_Pictographic".
- if strings.HasPrefix(line, "#") || line == "" || !strings.Contains(line, emojiProperty) {
- continue
- }
-
- // Everything else must be a code point range, a property and a comment.
- from, to, property, comment, err := parseProperty(line)
- if err != nil {
- return "", fmt.Errorf("emojis line %d: %v", num, err)
- }
- properties = append(properties, [4]string{from, to, property, comment})
- }
- if err := scanner.Err(); err != nil {
- return "", err
- }
- }
-
- // Sort properties.
- sort.Slice(properties, func(i, j int) bool {
- left, _ := strconv.ParseUint(properties[i][0], 16, 64)
- right, _ := strconv.ParseUint(properties[j][0], 16, 64)
- return left < right
- })
-
- // Header.
- var (
- buf bytes.Buffer
- emojiComment string
- )
- columns := 3
- if includeGeneralCategory {
- columns = 4
- }
- if emojiURL != "" {
- emojiComment = `
-// and
-// ` + emojiURL + `
-// ("Extended_Pictographic" only)`
- }
- buf.WriteString(`package uniseg
-
-// Code generated via go generate from gen_properties.go. DO NOT EDIT.
-
-// ` + os.Args[3] + ` are taken from
-// ` + propertyURL + emojiComment + `
-// on ` + time.Now().Format("January 2, 2006") + `. See https://www.unicode.org/license.html for the Unicode
-// license agreement.
-var ` + os.Args[3] + ` = [][` + strconv.Itoa(columns) + `]int{
- `)
-
- // Properties.
- for _, prop := range properties {
- if includeGeneralCategory {
- generalCategory := "gc" + prop[3][:2]
- if generalCategory == "gcL&" {
- generalCategory = "gcLC"
- }
- prop[3] = prop[3][3:]
- fmt.Fprintf(&buf, "{0x%s,0x%s,%s,%s}, // %s\n", prop[0], prop[1], translateProperty("pr", prop[2]), generalCategory, prop[3])
- } else {
- fmt.Fprintf(&buf, "{0x%s,0x%s,%s}, // %s\n", prop[0], prop[1], translateProperty("pr", prop[2]), prop[3])
- }
- }
-
- // Tail.
- buf.WriteString("}")
-
- return buf.String(), nil
-}
-
-// parseProperty parses a line of the Unicode properties text file containing a
-// property for a code point range and returns it along with its comment.
-func parseProperty(line string) (from, to, property, comment string, err error) {
- fields := propertyPattern.FindStringSubmatch(line)
- if fields == nil {
- err = errors.New("no property found")
- return
- }
- from = fields[1]
- to = fields[3]
- if to == "" {
- to = from
- }
- property = fields[4]
- comment = fields[5]
- return
-}
-
-// translateProperty translates a property name as used in the Unicode data file
-// to a variable used in the Go code.
-func translateProperty(prefix, property string) string {
- return prefix + strings.ReplaceAll(property, "_", "")
-}
diff --git a/vendor/github.com/rivo/uniseg/grapheme.go b/vendor/github.com/rivo/uniseg/grapheme.go
deleted file mode 100644
index d5d4c09e..00000000
--- a/vendor/github.com/rivo/uniseg/grapheme.go
+++ /dev/null
@@ -1,332 +0,0 @@
-package uniseg
-
-import "unicode/utf8"
-
-// Graphemes implements an iterator over Unicode grapheme clusters, or
-// user-perceived characters. While iterating, it also provides information
-// about word boundaries, sentence boundaries, line breaks, and monospace
-// character widths.
-//
-// After constructing the class via [NewGraphemes] for a given string "str",
-// [Graphemes.Next] is called for every grapheme cluster in a loop until it
-// returns false. Inside the loop, information about the grapheme cluster as
-// well as boundary information and character width is available via the various
-// methods (see examples below).
-//
-// Using this class to iterate over a string is convenient but it is much slower
-// than using this package's [Step] or [StepString] functions or any of the
-// other specialized functions starting with "First".
-type Graphemes struct {
- // The original string.
- original string
-
- // The remaining string to be parsed.
- remaining string
-
- // The current grapheme cluster.
- cluster string
-
- // The byte offset of the current grapheme cluster relative to the original
- // string.
- offset int
-
- // The current boundary information of the [Step] parser.
- boundaries int
-
- // The current state of the [Step] parser.
- state int
-}
-
-// NewGraphemes returns a new grapheme cluster iterator.
-func NewGraphemes(str string) *Graphemes {
- return &Graphemes{
- original: str,
- remaining: str,
- state: -1,
- }
-}
-
-// Next advances the iterator by one grapheme cluster and returns false if no
-// clusters are left. This function must be called before the first cluster is
-// accessed.
-func (g *Graphemes) Next() bool {
- if len(g.remaining) == 0 {
- // We're already past the end.
- g.state = -2
- g.cluster = ""
- return false
- }
- g.offset += len(g.cluster)
- g.cluster, g.remaining, g.boundaries, g.state = StepString(g.remaining, g.state)
- return true
-}
-
-// Runes returns a slice of runes (code points) which corresponds to the current
-// grapheme cluster. If the iterator is already past the end or [Graphemes.Next]
-// has not yet been called, nil is returned.
-func (g *Graphemes) Runes() []rune {
- if g.state < 0 {
- return nil
- }
- return []rune(g.cluster)
-}
-
-// Str returns a substring of the original string which corresponds to the
-// current grapheme cluster. If the iterator is already past the end or
-// [Graphemes.Next] has not yet been called, an empty string is returned.
-func (g *Graphemes) Str() string {
- return g.cluster
-}
-
-// Bytes returns a byte slice which corresponds to the current grapheme cluster.
-// If the iterator is already past the end or [Graphemes.Next] has not yet been
-// called, nil is returned.
-func (g *Graphemes) Bytes() []byte {
- if g.state < 0 {
- return nil
- }
- return []byte(g.cluster)
-}
-
-// Positions returns the interval of the current grapheme cluster as byte
-// positions into the original string. The first returned value "from" indexes
-// the first byte and the second returned value "to" indexes the first byte that
-// is not included anymore, i.e. str[from:to] is the current grapheme cluster of
-// the original string "str". If [Graphemes.Next] has not yet been called, both
-// values are 0. If the iterator is already past the end, both values are 1.
-func (g *Graphemes) Positions() (int, int) {
- if g.state == -1 {
- return 0, 0
- } else if g.state == -2 {
- return 1, 1
- }
- return g.offset, g.offset + len(g.cluster)
-}
-
-// IsWordBoundary returns true if a word ends after the current grapheme
-// cluster.
-func (g *Graphemes) IsWordBoundary() bool {
- if g.state < 0 {
- return true
- }
- return g.boundaries&MaskWord != 0
-}
-
-// IsSentenceBoundary returns true if a sentence ends after the current
-// grapheme cluster.
-func (g *Graphemes) IsSentenceBoundary() bool {
- if g.state < 0 {
- return true
- }
- return g.boundaries&MaskSentence != 0
-}
-
-// LineBreak returns whether the line can be broken after the current grapheme
-// cluster. A value of [LineDontBreak] means the line may not be broken, a value
-// of [LineMustBreak] means the line must be broken, and a value of
-// [LineCanBreak] means the line may or may not be broken.
-func (g *Graphemes) LineBreak() int {
- if g.state == -1 {
- return LineDontBreak
- }
- if g.state == -2 {
- return LineMustBreak
- }
- return g.boundaries & MaskLine
-}
-
-// Width returns the monospace width of the current grapheme cluster.
-func (g *Graphemes) Width() int {
- if g.state < 0 {
- return 0
- }
- return g.boundaries >> ShiftWidth
-}
-
-// Reset puts the iterator into its initial state such that the next call to
-// [Graphemes.Next] sets it to the first grapheme cluster again.
-func (g *Graphemes) Reset() {
- g.state = -1
- g.offset = 0
- g.cluster = ""
- g.remaining = g.original
-}
-
-// GraphemeClusterCount returns the number of user-perceived characters
-// (grapheme clusters) for the given string.
-func GraphemeClusterCount(s string) (n int) {
- state := -1
- for len(s) > 0 {
- _, s, _, state = FirstGraphemeClusterInString(s, state)
- n++
- }
- return
-}
-
-// ReverseString reverses the given string while observing grapheme cluster
-// boundaries.
-func ReverseString(s string) string {
- str := []byte(s)
- reversed := make([]byte, len(str))
- state := -1
- index := len(str)
- for len(str) > 0 {
- var cluster []byte
- cluster, str, _, state = FirstGraphemeCluster(str, state)
- index -= len(cluster)
- copy(reversed[index:], cluster)
- if index <= len(str)/2 {
- break
- }
- }
- return string(reversed)
-}
-
-// The number of bits the grapheme property must be shifted to make place for
-// grapheme states.
-const shiftGraphemePropState = 4
-
-// FirstGraphemeCluster returns the first grapheme cluster found in the given
-// byte slice according to the rules of Unicode Standard Annex #29, Grapheme
-// Cluster Boundaries. This function can be called continuously to extract all
-// grapheme clusters from a byte slice, as illustrated in the example below.
-//
-// If you don't know the current state, for example when calling the function
-// for the first time, you must pass -1. For consecutive calls, pass the state
-// and rest slice returned by the previous call.
-//
-// The "rest" slice is the sub-slice of the original byte slice "b" starting
-// after the last byte of the identified grapheme cluster. If the length of the
-// "rest" slice is 0, the entire byte slice "b" has been processed. The
-// "cluster" byte slice is the sub-slice of the input slice containing the
-// identified grapheme cluster.
-//
-// The returned width is the width of the grapheme cluster for most monospace
-// fonts where a value of 1 represents one character cell.
-//
-// Given an empty byte slice "b", the function returns nil values.
-//
-// While slightly less convenient than using the Graphemes class, this function
-// has much better performance and makes no allocations. It lends itself well to
-// large byte slices.
-func FirstGraphemeCluster(b []byte, state int) (cluster, rest []byte, width, newState int) {
- // An empty byte slice returns nothing.
- if len(b) == 0 {
- return
- }
-
- // Extract the first rune.
- r, length := utf8.DecodeRune(b)
- if len(b) <= length { // If we're already past the end, there is nothing else to parse.
- var prop int
- if state < 0 {
- prop = property(graphemeCodePoints, r)
- } else {
- prop = state >> shiftGraphemePropState
- }
- return b, nil, runeWidth(r, prop), grAny | (prop << shiftGraphemePropState)
- }
-
- // If we don't know the state, determine it now.
- var firstProp int
- if state < 0 {
- state, firstProp, _ = transitionGraphemeState(state, r)
- } else {
- firstProp = state >> shiftGraphemePropState
- }
- width += runeWidth(r, firstProp)
-
- // Transition until we find a boundary.
- for {
- var (
- prop int
- boundary bool
- )
-
- r, l := utf8.DecodeRune(b[length:])
- state, prop, boundary = transitionGraphemeState(state&maskGraphemeState, r)
-
- if boundary {
- return b[:length], b[length:], width, state | (prop << shiftGraphemePropState)
- }
-
- if r == vs16 {
- width = 2
- } else if firstProp != prExtendedPictographic && firstProp != prRegionalIndicator && firstProp != prL {
- width += runeWidth(r, prop)
- } else if firstProp == prExtendedPictographic {
- if r == vs15 {
- width = 1
- } else {
- width = 2
- }
- }
-
- length += l
- if len(b) <= length {
- return b, nil, width, grAny | (prop << shiftGraphemePropState)
- }
- }
-}
-
-// FirstGraphemeClusterInString is like [FirstGraphemeCluster] but its input and
-// outputs are strings.
-func FirstGraphemeClusterInString(str string, state int) (cluster, rest string, width, newState int) {
- // An empty string returns nothing.
- if len(str) == 0 {
- return
- }
-
- // Extract the first rune.
- r, length := utf8.DecodeRuneInString(str)
- if len(str) <= length { // If we're already past the end, there is nothing else to parse.
- var prop int
- if state < 0 {
- prop = property(graphemeCodePoints, r)
- } else {
- prop = state >> shiftGraphemePropState
- }
- return str, "", runeWidth(r, prop), grAny | (prop << shiftGraphemePropState)
- }
-
- // If we don't know the state, determine it now.
- var firstProp int
- if state < 0 {
- state, firstProp, _ = transitionGraphemeState(state, r)
- } else {
- firstProp = state >> shiftGraphemePropState
- }
- width += runeWidth(r, firstProp)
-
- // Transition until we find a boundary.
- for {
- var (
- prop int
- boundary bool
- )
-
- r, l := utf8.DecodeRuneInString(str[length:])
- state, prop, boundary = transitionGraphemeState(state&maskGraphemeState, r)
-
- if boundary {
- return str[:length], str[length:], width, state | (prop << shiftGraphemePropState)
- }
-
- if r == vs16 {
- width = 2
- } else if firstProp != prExtendedPictographic && firstProp != prRegionalIndicator && firstProp != prL {
- width += runeWidth(r, prop)
- } else if firstProp == prExtendedPictographic {
- if r == vs15 {
- width = 1
- } else {
- width = 2
- }
- }
-
- length += l
- if len(str) <= length {
- return str, "", width, grAny | (prop << shiftGraphemePropState)
- }
- }
-}
diff --git a/vendor/github.com/rivo/uniseg/graphemeproperties.go b/vendor/github.com/rivo/uniseg/graphemeproperties.go
deleted file mode 100644
index a87d140b..00000000
--- a/vendor/github.com/rivo/uniseg/graphemeproperties.go
+++ /dev/null
@@ -1,1891 +0,0 @@
-package uniseg
-
-// Code generated via go generate from gen_properties.go. DO NOT EDIT.
-
-// graphemeCodePoints are taken from
-// https://www.unicode.org/Public/14.0.0/ucd/auxiliary/GraphemeBreakProperty.txt
-// and
-// https://unicode.org/Public/14.0.0/ucd/emoji/emoji-data.txt
-// ("Extended_Pictographic" only)
-// on September 10, 2022. See https://www.unicode.org/license.html for the Unicode
-// license agreement.
-var graphemeCodePoints = [][3]int{
- {0x0000, 0x0009, prControl}, // Cc [10] ..
- {0x000A, 0x000A, prLF}, // Cc
- {0x000B, 0x000C, prControl}, // Cc [2] ..
- {0x000D, 0x000D, prCR}, // Cc
- {0x000E, 0x001F, prControl}, // Cc [18] ..
- {0x007F, 0x009F, prControl}, // Cc [33] ..
- {0x00A9, 0x00A9, prExtendedPictographic}, // E0.6 [1] (©ï¸) copyright
- {0x00AD, 0x00AD, prControl}, // Cf SOFT HYPHEN
- {0x00AE, 0x00AE, prExtendedPictographic}, // E0.6 [1] (®ï¸) registered
- {0x0300, 0x036F, prExtend}, // Mn [112] COMBINING GRAVE ACCENT..COMBINING LATIN SMALL LETTER X
- {0x0483, 0x0487, prExtend}, // Mn [5] COMBINING CYRILLIC TITLO..COMBINING CYRILLIC POKRYTIE
- {0x0488, 0x0489, prExtend}, // Me [2] COMBINING CYRILLIC HUNDRED THOUSANDS SIGN..COMBINING CYRILLIC MILLIONS SIGN
- {0x0591, 0x05BD, prExtend}, // Mn [45] HEBREW ACCENT ETNAHTA..HEBREW POINT METEG
- {0x05BF, 0x05BF, prExtend}, // Mn HEBREW POINT RAFE
- {0x05C1, 0x05C2, prExtend}, // Mn [2] HEBREW POINT SHIN DOT..HEBREW POINT SIN DOT
- {0x05C4, 0x05C5, prExtend}, // Mn [2] HEBREW MARK UPPER DOT..HEBREW MARK LOWER DOT
- {0x05C7, 0x05C7, prExtend}, // Mn HEBREW POINT QAMATS QATAN
- {0x0600, 0x0605, prPrepend}, // Cf [6] ARABIC NUMBER SIGN..ARABIC NUMBER MARK ABOVE
- {0x0610, 0x061A, prExtend}, // Mn [11] ARABIC SIGN SALLALLAHOU ALAYHE WASSALLAM..ARABIC SMALL KASRA
- {0x061C, 0x061C, prControl}, // Cf ARABIC LETTER MARK
- {0x064B, 0x065F, prExtend}, // Mn [21] ARABIC FATHATAN..ARABIC WAVY HAMZA BELOW
- {0x0670, 0x0670, prExtend}, // Mn ARABIC LETTER SUPERSCRIPT ALEF
- {0x06D6, 0x06DC, prExtend}, // Mn [7] ARABIC SMALL HIGH LIGATURE SAD WITH LAM WITH ALEF MAKSURA..ARABIC SMALL HIGH SEEN
- {0x06DD, 0x06DD, prPrepend}, // Cf ARABIC END OF AYAH
- {0x06DF, 0x06E4, prExtend}, // Mn [6] ARABIC SMALL HIGH ROUNDED ZERO..ARABIC SMALL HIGH MADDA
- {0x06E7, 0x06E8, prExtend}, // Mn [2] ARABIC SMALL HIGH YEH..ARABIC SMALL HIGH NOON
- {0x06EA, 0x06ED, prExtend}, // Mn [4] ARABIC EMPTY CENTRE LOW STOP..ARABIC SMALL LOW MEEM
- {0x070F, 0x070F, prPrepend}, // Cf SYRIAC ABBREVIATION MARK
- {0x0711, 0x0711, prExtend}, // Mn SYRIAC LETTER SUPERSCRIPT ALAPH
- {0x0730, 0x074A, prExtend}, // Mn [27] SYRIAC PTHAHA ABOVE..SYRIAC BARREKH
- {0x07A6, 0x07B0, prExtend}, // Mn [11] THAANA ABAFILI..THAANA SUKUN
- {0x07EB, 0x07F3, prExtend}, // Mn [9] NKO COMBINING SHORT HIGH TONE..NKO COMBINING DOUBLE DOT ABOVE
- {0x07FD, 0x07FD, prExtend}, // Mn NKO DANTAYALAN
- {0x0816, 0x0819, prExtend}, // Mn [4] SAMARITAN MARK IN..SAMARITAN MARK DAGESH
- {0x081B, 0x0823, prExtend}, // Mn [9] SAMARITAN MARK EPENTHETIC YUT..SAMARITAN VOWEL SIGN A
- {0x0825, 0x0827, prExtend}, // Mn [3] SAMARITAN VOWEL SIGN SHORT A..SAMARITAN VOWEL SIGN U
- {0x0829, 0x082D, prExtend}, // Mn [5] SAMARITAN VOWEL SIGN LONG I..SAMARITAN MARK NEQUDAA
- {0x0859, 0x085B, prExtend}, // Mn [3] MANDAIC AFFRICATION MARK..MANDAIC GEMINATION MARK
- {0x0890, 0x0891, prPrepend}, // Cf [2] ARABIC POUND MARK ABOVE..ARABIC PIASTRE MARK ABOVE
- {0x0898, 0x089F, prExtend}, // Mn [8] ARABIC SMALL HIGH WORD AL-JUZ..ARABIC HALF MADDA OVER MADDA
- {0x08CA, 0x08E1, prExtend}, // Mn [24] ARABIC SMALL HIGH FARSI YEH..ARABIC SMALL HIGH SIGN SAFHA
- {0x08E2, 0x08E2, prPrepend}, // Cf ARABIC DISPUTED END OF AYAH
- {0x08E3, 0x0902, prExtend}, // Mn [32] ARABIC TURNED DAMMA BELOW..DEVANAGARI SIGN ANUSVARA
- {0x0903, 0x0903, prSpacingMark}, // Mc DEVANAGARI SIGN VISARGA
- {0x093A, 0x093A, prExtend}, // Mn DEVANAGARI VOWEL SIGN OE
- {0x093B, 0x093B, prSpacingMark}, // Mc DEVANAGARI VOWEL SIGN OOE
- {0x093C, 0x093C, prExtend}, // Mn DEVANAGARI SIGN NUKTA
- {0x093E, 0x0940, prSpacingMark}, // Mc [3] DEVANAGARI VOWEL SIGN AA..DEVANAGARI VOWEL SIGN II
- {0x0941, 0x0948, prExtend}, // Mn [8] DEVANAGARI VOWEL SIGN U..DEVANAGARI VOWEL SIGN AI
- {0x0949, 0x094C, prSpacingMark}, // Mc [4] DEVANAGARI VOWEL SIGN CANDRA O..DEVANAGARI VOWEL SIGN AU
- {0x094D, 0x094D, prExtend}, // Mn DEVANAGARI SIGN VIRAMA
- {0x094E, 0x094F, prSpacingMark}, // Mc [2] DEVANAGARI VOWEL SIGN PRISHTHAMATRA E..DEVANAGARI VOWEL SIGN AW
- {0x0951, 0x0957, prExtend}, // Mn [7] DEVANAGARI STRESS SIGN UDATTA..DEVANAGARI VOWEL SIGN UUE
- {0x0962, 0x0963, prExtend}, // Mn [2] DEVANAGARI VOWEL SIGN VOCALIC L..DEVANAGARI VOWEL SIGN VOCALIC LL
- {0x0981, 0x0981, prExtend}, // Mn BENGALI SIGN CANDRABINDU
- {0x0982, 0x0983, prSpacingMark}, // Mc [2] BENGALI SIGN ANUSVARA..BENGALI SIGN VISARGA
- {0x09BC, 0x09BC, prExtend}, // Mn BENGALI SIGN NUKTA
- {0x09BE, 0x09BE, prExtend}, // Mc BENGALI VOWEL SIGN AA
- {0x09BF, 0x09C0, prSpacingMark}, // Mc [2] BENGALI VOWEL SIGN I..BENGALI VOWEL SIGN II
- {0x09C1, 0x09C4, prExtend}, // Mn [4] BENGALI VOWEL SIGN U..BENGALI VOWEL SIGN VOCALIC RR
- {0x09C7, 0x09C8, prSpacingMark}, // Mc [2] BENGALI VOWEL SIGN E..BENGALI VOWEL SIGN AI
- {0x09CB, 0x09CC, prSpacingMark}, // Mc [2] BENGALI VOWEL SIGN O..BENGALI VOWEL SIGN AU
- {0x09CD, 0x09CD, prExtend}, // Mn BENGALI SIGN VIRAMA
- {0x09D7, 0x09D7, prExtend}, // Mc BENGALI AU LENGTH MARK
- {0x09E2, 0x09E3, prExtend}, // Mn [2] BENGALI VOWEL SIGN VOCALIC L..BENGALI VOWEL SIGN VOCALIC LL
- {0x09FE, 0x09FE, prExtend}, // Mn BENGALI SANDHI MARK
- {0x0A01, 0x0A02, prExtend}, // Mn [2] GURMUKHI SIGN ADAK BINDI..GURMUKHI SIGN BINDI
- {0x0A03, 0x0A03, prSpacingMark}, // Mc GURMUKHI SIGN VISARGA
- {0x0A3C, 0x0A3C, prExtend}, // Mn GURMUKHI SIGN NUKTA
- {0x0A3E, 0x0A40, prSpacingMark}, // Mc [3] GURMUKHI VOWEL SIGN AA..GURMUKHI VOWEL SIGN II
- {0x0A41, 0x0A42, prExtend}, // Mn [2] GURMUKHI VOWEL SIGN U..GURMUKHI VOWEL SIGN UU
- {0x0A47, 0x0A48, prExtend}, // Mn [2] GURMUKHI VOWEL SIGN EE..GURMUKHI VOWEL SIGN AI
- {0x0A4B, 0x0A4D, prExtend}, // Mn [3] GURMUKHI VOWEL SIGN OO..GURMUKHI SIGN VIRAMA
- {0x0A51, 0x0A51, prExtend}, // Mn GURMUKHI SIGN UDAAT
- {0x0A70, 0x0A71, prExtend}, // Mn [2] GURMUKHI TIPPI..GURMUKHI ADDAK
- {0x0A75, 0x0A75, prExtend}, // Mn GURMUKHI SIGN YAKASH
- {0x0A81, 0x0A82, prExtend}, // Mn [2] GUJARATI SIGN CANDRABINDU..GUJARATI SIGN ANUSVARA
- {0x0A83, 0x0A83, prSpacingMark}, // Mc GUJARATI SIGN VISARGA
- {0x0ABC, 0x0ABC, prExtend}, // Mn GUJARATI SIGN NUKTA
- {0x0ABE, 0x0AC0, prSpacingMark}, // Mc [3] GUJARATI VOWEL SIGN AA..GUJARATI VOWEL SIGN II
- {0x0AC1, 0x0AC5, prExtend}, // Mn [5] GUJARATI VOWEL SIGN U..GUJARATI VOWEL SIGN CANDRA E
- {0x0AC7, 0x0AC8, prExtend}, // Mn [2] GUJARATI VOWEL SIGN E..GUJARATI VOWEL SIGN AI
- {0x0AC9, 0x0AC9, prSpacingMark}, // Mc GUJARATI VOWEL SIGN CANDRA O
- {0x0ACB, 0x0ACC, prSpacingMark}, // Mc [2] GUJARATI VOWEL SIGN O..GUJARATI VOWEL SIGN AU
- {0x0ACD, 0x0ACD, prExtend}, // Mn GUJARATI SIGN VIRAMA
- {0x0AE2, 0x0AE3, prExtend}, // Mn [2] GUJARATI VOWEL SIGN VOCALIC L..GUJARATI VOWEL SIGN VOCALIC LL
- {0x0AFA, 0x0AFF, prExtend}, // Mn [6] GUJARATI SIGN SUKUN..GUJARATI SIGN TWO-CIRCLE NUKTA ABOVE
- {0x0B01, 0x0B01, prExtend}, // Mn ORIYA SIGN CANDRABINDU
- {0x0B02, 0x0B03, prSpacingMark}, // Mc [2] ORIYA SIGN ANUSVARA..ORIYA SIGN VISARGA
- {0x0B3C, 0x0B3C, prExtend}, // Mn ORIYA SIGN NUKTA
- {0x0B3E, 0x0B3E, prExtend}, // Mc ORIYA VOWEL SIGN AA
- {0x0B3F, 0x0B3F, prExtend}, // Mn ORIYA VOWEL SIGN I
- {0x0B40, 0x0B40, prSpacingMark}, // Mc ORIYA VOWEL SIGN II
- {0x0B41, 0x0B44, prExtend}, // Mn [4] ORIYA VOWEL SIGN U..ORIYA VOWEL SIGN VOCALIC RR
- {0x0B47, 0x0B48, prSpacingMark}, // Mc [2] ORIYA VOWEL SIGN E..ORIYA VOWEL SIGN AI
- {0x0B4B, 0x0B4C, prSpacingMark}, // Mc [2] ORIYA VOWEL SIGN O..ORIYA VOWEL SIGN AU
- {0x0B4D, 0x0B4D, prExtend}, // Mn ORIYA SIGN VIRAMA
- {0x0B55, 0x0B56, prExtend}, // Mn [2] ORIYA SIGN OVERLINE..ORIYA AI LENGTH MARK
- {0x0B57, 0x0B57, prExtend}, // Mc ORIYA AU LENGTH MARK
- {0x0B62, 0x0B63, prExtend}, // Mn [2] ORIYA VOWEL SIGN VOCALIC L..ORIYA VOWEL SIGN VOCALIC LL
- {0x0B82, 0x0B82, prExtend}, // Mn TAMIL SIGN ANUSVARA
- {0x0BBE, 0x0BBE, prExtend}, // Mc TAMIL VOWEL SIGN AA
- {0x0BBF, 0x0BBF, prSpacingMark}, // Mc TAMIL VOWEL SIGN I
- {0x0BC0, 0x0BC0, prExtend}, // Mn TAMIL VOWEL SIGN II
- {0x0BC1, 0x0BC2, prSpacingMark}, // Mc [2] TAMIL VOWEL SIGN U..TAMIL VOWEL SIGN UU
- {0x0BC6, 0x0BC8, prSpacingMark}, // Mc [3] TAMIL VOWEL SIGN E..TAMIL VOWEL SIGN AI
- {0x0BCA, 0x0BCC, prSpacingMark}, // Mc [3] TAMIL VOWEL SIGN O..TAMIL VOWEL SIGN AU
- {0x0BCD, 0x0BCD, prExtend}, // Mn TAMIL SIGN VIRAMA
- {0x0BD7, 0x0BD7, prExtend}, // Mc TAMIL AU LENGTH MARK
- {0x0C00, 0x0C00, prExtend}, // Mn TELUGU SIGN COMBINING CANDRABINDU ABOVE
- {0x0C01, 0x0C03, prSpacingMark}, // Mc [3] TELUGU SIGN CANDRABINDU..TELUGU SIGN VISARGA
- {0x0C04, 0x0C04, prExtend}, // Mn TELUGU SIGN COMBINING ANUSVARA ABOVE
- {0x0C3C, 0x0C3C, prExtend}, // Mn TELUGU SIGN NUKTA
- {0x0C3E, 0x0C40, prExtend}, // Mn [3] TELUGU VOWEL SIGN AA..TELUGU VOWEL SIGN II
- {0x0C41, 0x0C44, prSpacingMark}, // Mc [4] TELUGU VOWEL SIGN U..TELUGU VOWEL SIGN VOCALIC RR
- {0x0C46, 0x0C48, prExtend}, // Mn [3] TELUGU VOWEL SIGN E..TELUGU VOWEL SIGN AI
- {0x0C4A, 0x0C4D, prExtend}, // Mn [4] TELUGU VOWEL SIGN O..TELUGU SIGN VIRAMA
- {0x0C55, 0x0C56, prExtend}, // Mn [2] TELUGU LENGTH MARK..TELUGU AI LENGTH MARK
- {0x0C62, 0x0C63, prExtend}, // Mn [2] TELUGU VOWEL SIGN VOCALIC L..TELUGU VOWEL SIGN VOCALIC LL
- {0x0C81, 0x0C81, prExtend}, // Mn KANNADA SIGN CANDRABINDU
- {0x0C82, 0x0C83, prSpacingMark}, // Mc [2] KANNADA SIGN ANUSVARA..KANNADA SIGN VISARGA
- {0x0CBC, 0x0CBC, prExtend}, // Mn KANNADA SIGN NUKTA
- {0x0CBE, 0x0CBE, prSpacingMark}, // Mc KANNADA VOWEL SIGN AA
- {0x0CBF, 0x0CBF, prExtend}, // Mn KANNADA VOWEL SIGN I
- {0x0CC0, 0x0CC1, prSpacingMark}, // Mc [2] KANNADA VOWEL SIGN II..KANNADA VOWEL SIGN U
- {0x0CC2, 0x0CC2, prExtend}, // Mc KANNADA VOWEL SIGN UU
- {0x0CC3, 0x0CC4, prSpacingMark}, // Mc [2] KANNADA VOWEL SIGN VOCALIC R..KANNADA VOWEL SIGN VOCALIC RR
- {0x0CC6, 0x0CC6, prExtend}, // Mn KANNADA VOWEL SIGN E
- {0x0CC7, 0x0CC8, prSpacingMark}, // Mc [2] KANNADA VOWEL SIGN EE..KANNADA VOWEL SIGN AI
- {0x0CCA, 0x0CCB, prSpacingMark}, // Mc [2] KANNADA VOWEL SIGN O..KANNADA VOWEL SIGN OO
- {0x0CCC, 0x0CCD, prExtend}, // Mn [2] KANNADA VOWEL SIGN AU..KANNADA SIGN VIRAMA
- {0x0CD5, 0x0CD6, prExtend}, // Mc [2] KANNADA LENGTH MARK..KANNADA AI LENGTH MARK
- {0x0CE2, 0x0CE3, prExtend}, // Mn [2] KANNADA VOWEL SIGN VOCALIC L..KANNADA VOWEL SIGN VOCALIC LL
- {0x0D00, 0x0D01, prExtend}, // Mn [2] MALAYALAM SIGN COMBINING ANUSVARA ABOVE..MALAYALAM SIGN CANDRABINDU
- {0x0D02, 0x0D03, prSpacingMark}, // Mc [2] MALAYALAM SIGN ANUSVARA..MALAYALAM SIGN VISARGA
- {0x0D3B, 0x0D3C, prExtend}, // Mn [2] MALAYALAM SIGN VERTICAL BAR VIRAMA..MALAYALAM SIGN CIRCULAR VIRAMA
- {0x0D3E, 0x0D3E, prExtend}, // Mc MALAYALAM VOWEL SIGN AA
- {0x0D3F, 0x0D40, prSpacingMark}, // Mc [2] MALAYALAM VOWEL SIGN I..MALAYALAM VOWEL SIGN II
- {0x0D41, 0x0D44, prExtend}, // Mn [4] MALAYALAM VOWEL SIGN U..MALAYALAM VOWEL SIGN VOCALIC RR
- {0x0D46, 0x0D48, prSpacingMark}, // Mc [3] MALAYALAM VOWEL SIGN E..MALAYALAM VOWEL SIGN AI
- {0x0D4A, 0x0D4C, prSpacingMark}, // Mc [3] MALAYALAM VOWEL SIGN O..MALAYALAM VOWEL SIGN AU
- {0x0D4D, 0x0D4D, prExtend}, // Mn MALAYALAM SIGN VIRAMA
- {0x0D4E, 0x0D4E, prPrepend}, // Lo MALAYALAM LETTER DOT REPH
- {0x0D57, 0x0D57, prExtend}, // Mc MALAYALAM AU LENGTH MARK
- {0x0D62, 0x0D63, prExtend}, // Mn [2] MALAYALAM VOWEL SIGN VOCALIC L..MALAYALAM VOWEL SIGN VOCALIC LL
- {0x0D81, 0x0D81, prExtend}, // Mn SINHALA SIGN CANDRABINDU
- {0x0D82, 0x0D83, prSpacingMark}, // Mc [2] SINHALA SIGN ANUSVARAYA..SINHALA SIGN VISARGAYA
- {0x0DCA, 0x0DCA, prExtend}, // Mn SINHALA SIGN AL-LAKUNA
- {0x0DCF, 0x0DCF, prExtend}, // Mc SINHALA VOWEL SIGN AELA-PILLA
- {0x0DD0, 0x0DD1, prSpacingMark}, // Mc [2] SINHALA VOWEL SIGN KETTI AEDA-PILLA..SINHALA VOWEL SIGN DIGA AEDA-PILLA
- {0x0DD2, 0x0DD4, prExtend}, // Mn [3] SINHALA VOWEL SIGN KETTI IS-PILLA..SINHALA VOWEL SIGN KETTI PAA-PILLA
- {0x0DD6, 0x0DD6, prExtend}, // Mn SINHALA VOWEL SIGN DIGA PAA-PILLA
- {0x0DD8, 0x0DDE, prSpacingMark}, // Mc [7] SINHALA VOWEL SIGN GAETTA-PILLA..SINHALA VOWEL SIGN KOMBUVA HAA GAYANUKITTA
- {0x0DDF, 0x0DDF, prExtend}, // Mc SINHALA VOWEL SIGN GAYANUKITTA
- {0x0DF2, 0x0DF3, prSpacingMark}, // Mc [2] SINHALA VOWEL SIGN DIGA GAETTA-PILLA..SINHALA VOWEL SIGN DIGA GAYANUKITTA
- {0x0E31, 0x0E31, prExtend}, // Mn THAI CHARACTER MAI HAN-AKAT
- {0x0E33, 0x0E33, prSpacingMark}, // Lo THAI CHARACTER SARA AM
- {0x0E34, 0x0E3A, prExtend}, // Mn [7] THAI CHARACTER SARA I..THAI CHARACTER PHINTHU
- {0x0E47, 0x0E4E, prExtend}, // Mn [8] THAI CHARACTER MAITAIKHU..THAI CHARACTER YAMAKKAN
- {0x0EB1, 0x0EB1, prExtend}, // Mn LAO VOWEL SIGN MAI KAN
- {0x0EB3, 0x0EB3, prSpacingMark}, // Lo LAO VOWEL SIGN AM
- {0x0EB4, 0x0EBC, prExtend}, // Mn [9] LAO VOWEL SIGN I..LAO SEMIVOWEL SIGN LO
- {0x0EC8, 0x0ECD, prExtend}, // Mn [6] LAO TONE MAI EK..LAO NIGGAHITA
- {0x0F18, 0x0F19, prExtend}, // Mn [2] TIBETAN ASTROLOGICAL SIGN -KHYUD PA..TIBETAN ASTROLOGICAL SIGN SDONG TSHUGS
- {0x0F35, 0x0F35, prExtend}, // Mn TIBETAN MARK NGAS BZUNG NYI ZLA
- {0x0F37, 0x0F37, prExtend}, // Mn TIBETAN MARK NGAS BZUNG SGOR RTAGS
- {0x0F39, 0x0F39, prExtend}, // Mn TIBETAN MARK TSA -PHRU
- {0x0F3E, 0x0F3F, prSpacingMark}, // Mc [2] TIBETAN SIGN YAR TSHES..TIBETAN SIGN MAR TSHES
- {0x0F71, 0x0F7E, prExtend}, // Mn [14] TIBETAN VOWEL SIGN AA..TIBETAN SIGN RJES SU NGA RO
- {0x0F7F, 0x0F7F, prSpacingMark}, // Mc TIBETAN SIGN RNAM BCAD
- {0x0F80, 0x0F84, prExtend}, // Mn [5] TIBETAN VOWEL SIGN REVERSED I..TIBETAN MARK HALANTA
- {0x0F86, 0x0F87, prExtend}, // Mn [2] TIBETAN SIGN LCI RTAGS..TIBETAN SIGN YANG RTAGS
- {0x0F8D, 0x0F97, prExtend}, // Mn [11] TIBETAN SUBJOINED SIGN LCE TSA CAN..TIBETAN SUBJOINED LETTER JA
- {0x0F99, 0x0FBC, prExtend}, // Mn [36] TIBETAN SUBJOINED LETTER NYA..TIBETAN SUBJOINED LETTER FIXED-FORM RA
- {0x0FC6, 0x0FC6, prExtend}, // Mn TIBETAN SYMBOL PADMA GDAN
- {0x102D, 0x1030, prExtend}, // Mn [4] MYANMAR VOWEL SIGN I..MYANMAR VOWEL SIGN UU
- {0x1031, 0x1031, prSpacingMark}, // Mc MYANMAR VOWEL SIGN E
- {0x1032, 0x1037, prExtend}, // Mn [6] MYANMAR VOWEL SIGN AI..MYANMAR SIGN DOT BELOW
- {0x1039, 0x103A, prExtend}, // Mn [2] MYANMAR SIGN VIRAMA..MYANMAR SIGN ASAT
- {0x103B, 0x103C, prSpacingMark}, // Mc [2] MYANMAR CONSONANT SIGN MEDIAL YA..MYANMAR CONSONANT SIGN MEDIAL RA
- {0x103D, 0x103E, prExtend}, // Mn [2] MYANMAR CONSONANT SIGN MEDIAL WA..MYANMAR CONSONANT SIGN MEDIAL HA
- {0x1056, 0x1057, prSpacingMark}, // Mc [2] MYANMAR VOWEL SIGN VOCALIC R..MYANMAR VOWEL SIGN VOCALIC RR
- {0x1058, 0x1059, prExtend}, // Mn [2] MYANMAR VOWEL SIGN VOCALIC L..MYANMAR VOWEL SIGN VOCALIC LL
- {0x105E, 0x1060, prExtend}, // Mn [3] MYANMAR CONSONANT SIGN MON MEDIAL NA..MYANMAR CONSONANT SIGN MON MEDIAL LA
- {0x1071, 0x1074, prExtend}, // Mn [4] MYANMAR VOWEL SIGN GEBA KAREN I..MYANMAR VOWEL SIGN KAYAH EE
- {0x1082, 0x1082, prExtend}, // Mn MYANMAR CONSONANT SIGN SHAN MEDIAL WA
- {0x1084, 0x1084, prSpacingMark}, // Mc MYANMAR VOWEL SIGN SHAN E
- {0x1085, 0x1086, prExtend}, // Mn [2] MYANMAR VOWEL SIGN SHAN E ABOVE..MYANMAR VOWEL SIGN SHAN FINAL Y
- {0x108D, 0x108D, prExtend}, // Mn MYANMAR SIGN SHAN COUNCIL EMPHATIC TONE
- {0x109D, 0x109D, prExtend}, // Mn MYANMAR VOWEL SIGN AITON AI
- {0x1100, 0x115F, prL}, // Lo [96] HANGUL CHOSEONG KIYEOK..HANGUL CHOSEONG FILLER
- {0x1160, 0x11A7, prV}, // Lo [72] HANGUL JUNGSEONG FILLER..HANGUL JUNGSEONG O-YAE
- {0x11A8, 0x11FF, prT}, // Lo [88] HANGUL JONGSEONG KIYEOK..HANGUL JONGSEONG SSANGNIEUN
- {0x135D, 0x135F, prExtend}, // Mn [3] ETHIOPIC COMBINING GEMINATION AND VOWEL LENGTH MARK..ETHIOPIC COMBINING GEMINATION MARK
- {0x1712, 0x1714, prExtend}, // Mn [3] TAGALOG VOWEL SIGN I..TAGALOG SIGN VIRAMA
- {0x1715, 0x1715, prSpacingMark}, // Mc TAGALOG SIGN PAMUDPOD
- {0x1732, 0x1733, prExtend}, // Mn [2] HANUNOO VOWEL SIGN I..HANUNOO VOWEL SIGN U
- {0x1734, 0x1734, prSpacingMark}, // Mc HANUNOO SIGN PAMUDPOD
- {0x1752, 0x1753, prExtend}, // Mn [2] BUHID VOWEL SIGN I..BUHID VOWEL SIGN U
- {0x1772, 0x1773, prExtend}, // Mn [2] TAGBANWA VOWEL SIGN I..TAGBANWA VOWEL SIGN U
- {0x17B4, 0x17B5, prExtend}, // Mn [2] KHMER VOWEL INHERENT AQ..KHMER VOWEL INHERENT AA
- {0x17B6, 0x17B6, prSpacingMark}, // Mc KHMER VOWEL SIGN AA
- {0x17B7, 0x17BD, prExtend}, // Mn [7] KHMER VOWEL SIGN I..KHMER VOWEL SIGN UA
- {0x17BE, 0x17C5, prSpacingMark}, // Mc [8] KHMER VOWEL SIGN OE..KHMER VOWEL SIGN AU
- {0x17C6, 0x17C6, prExtend}, // Mn KHMER SIGN NIKAHIT
- {0x17C7, 0x17C8, prSpacingMark}, // Mc [2] KHMER SIGN REAHMUK..KHMER SIGN YUUKALEAPINTU
- {0x17C9, 0x17D3, prExtend}, // Mn [11] KHMER SIGN MUUSIKATOAN..KHMER SIGN BATHAMASAT
- {0x17DD, 0x17DD, prExtend}, // Mn KHMER SIGN ATTHACAN
- {0x180B, 0x180D, prExtend}, // Mn [3] MONGOLIAN FREE VARIATION SELECTOR ONE..MONGOLIAN FREE VARIATION SELECTOR THREE
- {0x180E, 0x180E, prControl}, // Cf MONGOLIAN VOWEL SEPARATOR
- {0x180F, 0x180F, prExtend}, // Mn MONGOLIAN FREE VARIATION SELECTOR FOUR
- {0x1885, 0x1886, prExtend}, // Mn [2] MONGOLIAN LETTER ALI GALI BALUDA..MONGOLIAN LETTER ALI GALI THREE BALUDA
- {0x18A9, 0x18A9, prExtend}, // Mn MONGOLIAN LETTER ALI GALI DAGALGA
- {0x1920, 0x1922, prExtend}, // Mn [3] LIMBU VOWEL SIGN A..LIMBU VOWEL SIGN U
- {0x1923, 0x1926, prSpacingMark}, // Mc [4] LIMBU VOWEL SIGN EE..LIMBU VOWEL SIGN AU
- {0x1927, 0x1928, prExtend}, // Mn [2] LIMBU VOWEL SIGN E..LIMBU VOWEL SIGN O
- {0x1929, 0x192B, prSpacingMark}, // Mc [3] LIMBU SUBJOINED LETTER YA..LIMBU SUBJOINED LETTER WA
- {0x1930, 0x1931, prSpacingMark}, // Mc [2] LIMBU SMALL LETTER KA..LIMBU SMALL LETTER NGA
- {0x1932, 0x1932, prExtend}, // Mn LIMBU SMALL LETTER ANUSVARA
- {0x1933, 0x1938, prSpacingMark}, // Mc [6] LIMBU SMALL LETTER TA..LIMBU SMALL LETTER LA
- {0x1939, 0x193B, prExtend}, // Mn [3] LIMBU SIGN MUKPHRENG..LIMBU SIGN SA-I
- {0x1A17, 0x1A18, prExtend}, // Mn [2] BUGINESE VOWEL SIGN I..BUGINESE VOWEL SIGN U
- {0x1A19, 0x1A1A, prSpacingMark}, // Mc [2] BUGINESE VOWEL SIGN E..BUGINESE VOWEL SIGN O
- {0x1A1B, 0x1A1B, prExtend}, // Mn BUGINESE VOWEL SIGN AE
- {0x1A55, 0x1A55, prSpacingMark}, // Mc TAI THAM CONSONANT SIGN MEDIAL RA
- {0x1A56, 0x1A56, prExtend}, // Mn TAI THAM CONSONANT SIGN MEDIAL LA
- {0x1A57, 0x1A57, prSpacingMark}, // Mc TAI THAM CONSONANT SIGN LA TANG LAI
- {0x1A58, 0x1A5E, prExtend}, // Mn [7] TAI THAM SIGN MAI KANG LAI..TAI THAM CONSONANT SIGN SA
- {0x1A60, 0x1A60, prExtend}, // Mn TAI THAM SIGN SAKOT
- {0x1A62, 0x1A62, prExtend}, // Mn TAI THAM VOWEL SIGN MAI SAT
- {0x1A65, 0x1A6C, prExtend}, // Mn [8] TAI THAM VOWEL SIGN I..TAI THAM VOWEL SIGN OA BELOW
- {0x1A6D, 0x1A72, prSpacingMark}, // Mc [6] TAI THAM VOWEL SIGN OY..TAI THAM VOWEL SIGN THAM AI
- {0x1A73, 0x1A7C, prExtend}, // Mn [10] TAI THAM VOWEL SIGN OA ABOVE..TAI THAM SIGN KHUEN-LUE KARAN
- {0x1A7F, 0x1A7F, prExtend}, // Mn TAI THAM COMBINING CRYPTOGRAMMIC DOT
- {0x1AB0, 0x1ABD, prExtend}, // Mn [14] COMBINING DOUBLED CIRCUMFLEX ACCENT..COMBINING PARENTHESES BELOW
- {0x1ABE, 0x1ABE, prExtend}, // Me COMBINING PARENTHESES OVERLAY
- {0x1ABF, 0x1ACE, prExtend}, // Mn [16] COMBINING LATIN SMALL LETTER W BELOW..COMBINING LATIN SMALL LETTER INSULAR T
- {0x1B00, 0x1B03, prExtend}, // Mn [4] BALINESE SIGN ULU RICEM..BALINESE SIGN SURANG
- {0x1B04, 0x1B04, prSpacingMark}, // Mc BALINESE SIGN BISAH
- {0x1B34, 0x1B34, prExtend}, // Mn BALINESE SIGN REREKAN
- {0x1B35, 0x1B35, prExtend}, // Mc BALINESE VOWEL SIGN TEDUNG
- {0x1B36, 0x1B3A, prExtend}, // Mn [5] BALINESE VOWEL SIGN ULU..BALINESE VOWEL SIGN RA REPA
- {0x1B3B, 0x1B3B, prSpacingMark}, // Mc BALINESE VOWEL SIGN RA REPA TEDUNG
- {0x1B3C, 0x1B3C, prExtend}, // Mn BALINESE VOWEL SIGN LA LENGA
- {0x1B3D, 0x1B41, prSpacingMark}, // Mc [5] BALINESE VOWEL SIGN LA LENGA TEDUNG..BALINESE VOWEL SIGN TALING REPA TEDUNG
- {0x1B42, 0x1B42, prExtend}, // Mn BALINESE VOWEL SIGN PEPET
- {0x1B43, 0x1B44, prSpacingMark}, // Mc [2] BALINESE VOWEL SIGN PEPET TEDUNG..BALINESE ADEG ADEG
- {0x1B6B, 0x1B73, prExtend}, // Mn [9] BALINESE MUSICAL SYMBOL COMBINING TEGEH..BALINESE MUSICAL SYMBOL COMBINING GONG
- {0x1B80, 0x1B81, prExtend}, // Mn [2] SUNDANESE SIGN PANYECEK..SUNDANESE SIGN PANGLAYAR
- {0x1B82, 0x1B82, prSpacingMark}, // Mc SUNDANESE SIGN PANGWISAD
- {0x1BA1, 0x1BA1, prSpacingMark}, // Mc SUNDANESE CONSONANT SIGN PAMINGKAL
- {0x1BA2, 0x1BA5, prExtend}, // Mn [4] SUNDANESE CONSONANT SIGN PANYAKRA..SUNDANESE VOWEL SIGN PANYUKU
- {0x1BA6, 0x1BA7, prSpacingMark}, // Mc [2] SUNDANESE VOWEL SIGN PANAELAENG..SUNDANESE VOWEL SIGN PANOLONG
- {0x1BA8, 0x1BA9, prExtend}, // Mn [2] SUNDANESE VOWEL SIGN PAMEPET..SUNDANESE VOWEL SIGN PANEULEUNG
- {0x1BAA, 0x1BAA, prSpacingMark}, // Mc SUNDANESE SIGN PAMAAEH
- {0x1BAB, 0x1BAD, prExtend}, // Mn [3] SUNDANESE SIGN VIRAMA..SUNDANESE CONSONANT SIGN PASANGAN WA
- {0x1BE6, 0x1BE6, prExtend}, // Mn BATAK SIGN TOMPI
- {0x1BE7, 0x1BE7, prSpacingMark}, // Mc BATAK VOWEL SIGN E
- {0x1BE8, 0x1BE9, prExtend}, // Mn [2] BATAK VOWEL SIGN PAKPAK E..BATAK VOWEL SIGN EE
- {0x1BEA, 0x1BEC, prSpacingMark}, // Mc [3] BATAK VOWEL SIGN I..BATAK VOWEL SIGN O
- {0x1BED, 0x1BED, prExtend}, // Mn BATAK VOWEL SIGN KARO O
- {0x1BEE, 0x1BEE, prSpacingMark}, // Mc BATAK VOWEL SIGN U
- {0x1BEF, 0x1BF1, prExtend}, // Mn [3] BATAK VOWEL SIGN U FOR SIMALUNGUN SA..BATAK CONSONANT SIGN H
- {0x1BF2, 0x1BF3, prSpacingMark}, // Mc [2] BATAK PANGOLAT..BATAK PANONGONAN
- {0x1C24, 0x1C2B, prSpacingMark}, // Mc [8] LEPCHA SUBJOINED LETTER YA..LEPCHA VOWEL SIGN UU
- {0x1C2C, 0x1C33, prExtend}, // Mn [8] LEPCHA VOWEL SIGN E..LEPCHA CONSONANT SIGN T
- {0x1C34, 0x1C35, prSpacingMark}, // Mc [2] LEPCHA CONSONANT SIGN NYIN-DO..LEPCHA CONSONANT SIGN KANG
- {0x1C36, 0x1C37, prExtend}, // Mn [2] LEPCHA SIGN RAN..LEPCHA SIGN NUKTA
- {0x1CD0, 0x1CD2, prExtend}, // Mn [3] VEDIC TONE KARSHANA..VEDIC TONE PRENKHA
- {0x1CD4, 0x1CE0, prExtend}, // Mn [13] VEDIC SIGN YAJURVEDIC MIDLINE SVARITA..VEDIC TONE RIGVEDIC KASHMIRI INDEPENDENT SVARITA
- {0x1CE1, 0x1CE1, prSpacingMark}, // Mc VEDIC TONE ATHARVAVEDIC INDEPENDENT SVARITA
- {0x1CE2, 0x1CE8, prExtend}, // Mn [7] VEDIC SIGN VISARGA SVARITA..VEDIC SIGN VISARGA ANUDATTA WITH TAIL
- {0x1CED, 0x1CED, prExtend}, // Mn VEDIC SIGN TIRYAK
- {0x1CF4, 0x1CF4, prExtend}, // Mn VEDIC TONE CANDRA ABOVE
- {0x1CF7, 0x1CF7, prSpacingMark}, // Mc VEDIC SIGN ATIKRAMA
- {0x1CF8, 0x1CF9, prExtend}, // Mn [2] VEDIC TONE RING ABOVE..VEDIC TONE DOUBLE RING ABOVE
- {0x1DC0, 0x1DFF, prExtend}, // Mn [64] COMBINING DOTTED GRAVE ACCENT..COMBINING RIGHT ARROWHEAD AND DOWN ARROWHEAD BELOW
- {0x200B, 0x200B, prControl}, // Cf ZERO WIDTH SPACE
- {0x200C, 0x200C, prExtend}, // Cf ZERO WIDTH NON-JOINER
- {0x200D, 0x200D, prZWJ}, // Cf ZERO WIDTH JOINER
- {0x200E, 0x200F, prControl}, // Cf [2] LEFT-TO-RIGHT MARK..RIGHT-TO-LEFT MARK
- {0x2028, 0x2028, prControl}, // Zl LINE SEPARATOR
- {0x2029, 0x2029, prControl}, // Zp PARAGRAPH SEPARATOR
- {0x202A, 0x202E, prControl}, // Cf [5] LEFT-TO-RIGHT EMBEDDING..RIGHT-TO-LEFT OVERRIDE
- {0x203C, 0x203C, prExtendedPictographic}, // E0.6 [1] (‼ï¸) double exclamation mark
- {0x2049, 0x2049, prExtendedPictographic}, // E0.6 [1] (â‰ï¸) exclamation question mark
- {0x2060, 0x2064, prControl}, // Cf [5] WORD JOINER..INVISIBLE PLUS
- {0x2065, 0x2065, prControl}, // Cn
- {0x2066, 0x206F, prControl}, // Cf [10] LEFT-TO-RIGHT ISOLATE..NOMINAL DIGIT SHAPES
- {0x20D0, 0x20DC, prExtend}, // Mn [13] COMBINING LEFT HARPOON ABOVE..COMBINING FOUR DOTS ABOVE
- {0x20DD, 0x20E0, prExtend}, // Me [4] COMBINING ENCLOSING CIRCLE..COMBINING ENCLOSING CIRCLE BACKSLASH
- {0x20E1, 0x20E1, prExtend}, // Mn COMBINING LEFT RIGHT ARROW ABOVE
- {0x20E2, 0x20E4, prExtend}, // Me [3] COMBINING ENCLOSING SCREEN..COMBINING ENCLOSING UPWARD POINTING TRIANGLE
- {0x20E5, 0x20F0, prExtend}, // Mn [12] COMBINING REVERSE SOLIDUS OVERLAY..COMBINING ASTERISK ABOVE
- {0x2122, 0x2122, prExtendedPictographic}, // E0.6 [1] (â„¢ï¸) trade mark
- {0x2139, 0x2139, prExtendedPictographic}, // E0.6 [1] (ℹï¸) information
- {0x2194, 0x2199, prExtendedPictographic}, // E0.6 [6] (↔ï¸..↙ï¸) left-right arrow..down-left arrow
- {0x21A9, 0x21AA, prExtendedPictographic}, // E0.6 [2] (↩ï¸..↪ï¸) right arrow curving left..left arrow curving right
- {0x231A, 0x231B, prExtendedPictographic}, // E0.6 [2] (⌚..⌛) watch..hourglass done
- {0x2328, 0x2328, prExtendedPictographic}, // E1.0 [1] (⌨ï¸) keyboard
- {0x2388, 0x2388, prExtendedPictographic}, // E0.0 [1] (⎈) HELM SYMBOL
- {0x23CF, 0x23CF, prExtendedPictographic}, // E1.0 [1] (âï¸) eject button
- {0x23E9, 0x23EC, prExtendedPictographic}, // E0.6 [4] (â©..â¬) fast-forward button..fast down button
- {0x23ED, 0x23EE, prExtendedPictographic}, // E0.7 [2] (âï¸..â®ï¸) next track button..last track button
- {0x23EF, 0x23EF, prExtendedPictographic}, // E1.0 [1] (â¯ï¸) play or pause button
- {0x23F0, 0x23F0, prExtendedPictographic}, // E0.6 [1] (â°) alarm clock
- {0x23F1, 0x23F2, prExtendedPictographic}, // E1.0 [2] (â±ï¸..â²ï¸) stopwatch..timer clock
- {0x23F3, 0x23F3, prExtendedPictographic}, // E0.6 [1] (â³) hourglass not done
- {0x23F8, 0x23FA, prExtendedPictographic}, // E0.7 [3] (â¸ï¸..âºï¸) pause button..record button
- {0x24C2, 0x24C2, prExtendedPictographic}, // E0.6 [1] (â“‚ï¸) circled M
- {0x25AA, 0x25AB, prExtendedPictographic}, // E0.6 [2] (â–ªï¸..â–«ï¸) black small square..white small square
- {0x25B6, 0x25B6, prExtendedPictographic}, // E0.6 [1] (â–¶ï¸) play button
- {0x25C0, 0x25C0, prExtendedPictographic}, // E0.6 [1] (â—€ï¸) reverse button
- {0x25FB, 0x25FE, prExtendedPictographic}, // E0.6 [4] (â—»ï¸..â—¾) white medium square..black medium-small square
- {0x2600, 0x2601, prExtendedPictographic}, // E0.6 [2] (☀ï¸..â˜ï¸) sun..cloud
- {0x2602, 0x2603, prExtendedPictographic}, // E0.7 [2] (☂ï¸..☃ï¸) umbrella..snowman
- {0x2604, 0x2604, prExtendedPictographic}, // E1.0 [1] (☄ï¸) comet
- {0x2605, 0x2605, prExtendedPictographic}, // E0.0 [1] (★) BLACK STAR
- {0x2607, 0x260D, prExtendedPictographic}, // E0.0 [7] (☇..â˜) LIGHTNING..OPPOSITION
- {0x260E, 0x260E, prExtendedPictographic}, // E0.6 [1] (☎ï¸) telephone
- {0x260F, 0x2610, prExtendedPictographic}, // E0.0 [2] (â˜..â˜) WHITE TELEPHONE..BALLOT BOX
- {0x2611, 0x2611, prExtendedPictographic}, // E0.6 [1] (☑ï¸) check box with check
- {0x2612, 0x2612, prExtendedPictographic}, // E0.0 [1] (☒) BALLOT BOX WITH X
- {0x2614, 0x2615, prExtendedPictographic}, // E0.6 [2] (☔..☕) umbrella with rain drops..hot beverage
- {0x2616, 0x2617, prExtendedPictographic}, // E0.0 [2] (☖..☗) WHITE SHOGI PIECE..BLACK SHOGI PIECE
- {0x2618, 0x2618, prExtendedPictographic}, // E1.0 [1] (☘ï¸) shamrock
- {0x2619, 0x261C, prExtendedPictographic}, // E0.0 [4] (☙..☜) REVERSED ROTATED FLORAL HEART BULLET..WHITE LEFT POINTING INDEX
- {0x261D, 0x261D, prExtendedPictographic}, // E0.6 [1] (â˜ï¸) index pointing up
- {0x261E, 0x261F, prExtendedPictographic}, // E0.0 [2] (☞..☟) WHITE RIGHT POINTING INDEX..WHITE DOWN POINTING INDEX
- {0x2620, 0x2620, prExtendedPictographic}, // E1.0 [1] (☠ï¸) skull and crossbones
- {0x2621, 0x2621, prExtendedPictographic}, // E0.0 [1] (☡) CAUTION SIGN
- {0x2622, 0x2623, prExtendedPictographic}, // E1.0 [2] (☢ï¸..☣ï¸) radioactive..biohazard
- {0x2624, 0x2625, prExtendedPictographic}, // E0.0 [2] (☤..☥) CADUCEUS..ANKH
- {0x2626, 0x2626, prExtendedPictographic}, // E1.0 [1] (☦ï¸) orthodox cross
- {0x2627, 0x2629, prExtendedPictographic}, // E0.0 [3] (☧..☩) CHI RHO..CROSS OF JERUSALEM
- {0x262A, 0x262A, prExtendedPictographic}, // E0.7 [1] (☪ï¸) star and crescent
- {0x262B, 0x262D, prExtendedPictographic}, // E0.0 [3] (☫..â˜) FARSI SYMBOL..HAMMER AND SICKLE
- {0x262E, 0x262E, prExtendedPictographic}, // E1.0 [1] (☮ï¸) peace symbol
- {0x262F, 0x262F, prExtendedPictographic}, // E0.7 [1] (☯ï¸) yin yang
- {0x2630, 0x2637, prExtendedPictographic}, // E0.0 [8] (☰..☷) TRIGRAM FOR HEAVEN..TRIGRAM FOR EARTH
- {0x2638, 0x2639, prExtendedPictographic}, // E0.7 [2] (☸ï¸..☹ï¸) wheel of dharma..frowning face
- {0x263A, 0x263A, prExtendedPictographic}, // E0.6 [1] (☺ï¸) smiling face
- {0x263B, 0x263F, prExtendedPictographic}, // E0.0 [5] (☻..☿) BLACK SMILING FACE..MERCURY
- {0x2640, 0x2640, prExtendedPictographic}, // E4.0 [1] (♀ï¸) female sign
- {0x2641, 0x2641, prExtendedPictographic}, // E0.0 [1] (â™) EARTH
- {0x2642, 0x2642, prExtendedPictographic}, // E4.0 [1] (♂ï¸) male sign
- {0x2643, 0x2647, prExtendedPictographic}, // E0.0 [5] (♃..♇) JUPITER..PLUTO
- {0x2648, 0x2653, prExtendedPictographic}, // E0.6 [12] (♈..♓) Aries..Pisces
- {0x2654, 0x265E, prExtendedPictographic}, // E0.0 [11] (♔..♞) WHITE CHESS KING..BLACK CHESS KNIGHT
- {0x265F, 0x265F, prExtendedPictographic}, // E11.0 [1] (♟ï¸) chess pawn
- {0x2660, 0x2660, prExtendedPictographic}, // E0.6 [1] (â™ ï¸) spade suit
- {0x2661, 0x2662, prExtendedPictographic}, // E0.0 [2] (♡..♢) WHITE HEART SUIT..WHITE DIAMOND SUIT
- {0x2663, 0x2663, prExtendedPictographic}, // E0.6 [1] (♣ï¸) club suit
- {0x2664, 0x2664, prExtendedPictographic}, // E0.0 [1] (♤) WHITE SPADE SUIT
- {0x2665, 0x2666, prExtendedPictographic}, // E0.6 [2] (♥ï¸..♦ï¸) heart suit..diamond suit
- {0x2667, 0x2667, prExtendedPictographic}, // E0.0 [1] (â™§) WHITE CLUB SUIT
- {0x2668, 0x2668, prExtendedPictographic}, // E0.6 [1] (♨ï¸) hot springs
- {0x2669, 0x267A, prExtendedPictographic}, // E0.0 [18] (♩..♺) QUARTER NOTE..RECYCLING SYMBOL FOR GENERIC MATERIALS
- {0x267B, 0x267B, prExtendedPictographic}, // E0.6 [1] (â™»ï¸) recycling symbol
- {0x267C, 0x267D, prExtendedPictographic}, // E0.0 [2] (♼..♽) RECYCLED PAPER SYMBOL..PARTIALLY-RECYCLED PAPER SYMBOL
- {0x267E, 0x267E, prExtendedPictographic}, // E11.0 [1] (♾ï¸) infinity
- {0x267F, 0x267F, prExtendedPictographic}, // E0.6 [1] (♿) wheelchair symbol
- {0x2680, 0x2685, prExtendedPictographic}, // E0.0 [6] (⚀..⚅) DIE FACE-1..DIE FACE-6
- {0x2690, 0x2691, prExtendedPictographic}, // E0.0 [2] (âš..âš‘) WHITE FLAG..BLACK FLAG
- {0x2692, 0x2692, prExtendedPictographic}, // E1.0 [1] (âš’ï¸) hammer and pick
- {0x2693, 0x2693, prExtendedPictographic}, // E0.6 [1] (âš“) anchor
- {0x2694, 0x2694, prExtendedPictographic}, // E1.0 [1] (âš”ï¸) crossed swords
- {0x2695, 0x2695, prExtendedPictographic}, // E4.0 [1] (âš•ï¸) medical symbol
- {0x2696, 0x2697, prExtendedPictographic}, // E1.0 [2] (âš–ï¸..âš—ï¸) balance scale..alembic
- {0x2698, 0x2698, prExtendedPictographic}, // E0.0 [1] (⚘) FLOWER
- {0x2699, 0x2699, prExtendedPictographic}, // E1.0 [1] (âš™ï¸) gear
- {0x269A, 0x269A, prExtendedPictographic}, // E0.0 [1] (âšš) STAFF OF HERMES
- {0x269B, 0x269C, prExtendedPictographic}, // E1.0 [2] (âš›ï¸..âšœï¸) atom symbol..fleur-de-lis
- {0x269D, 0x269F, prExtendedPictographic}, // E0.0 [3] (âš..⚟) OUTLINED WHITE STAR..THREE LINES CONVERGING LEFT
- {0x26A0, 0x26A1, prExtendedPictographic}, // E0.6 [2] (âš ï¸..âš¡) warning..high voltage
- {0x26A2, 0x26A6, prExtendedPictographic}, // E0.0 [5] (⚢..⚦) DOUBLED FEMALE SIGN..MALE WITH STROKE SIGN
- {0x26A7, 0x26A7, prExtendedPictographic}, // E13.0 [1] (âš§ï¸) transgender symbol
- {0x26A8, 0x26A9, prExtendedPictographic}, // E0.0 [2] (⚨..⚩) VERTICAL MALE WITH STROKE SIGN..HORIZONTAL MALE WITH STROKE SIGN
- {0x26AA, 0x26AB, prExtendedPictographic}, // E0.6 [2] (⚪..⚫) white circle..black circle
- {0x26AC, 0x26AF, prExtendedPictographic}, // E0.0 [4] (⚬..⚯) MEDIUM SMALL WHITE CIRCLE..UNMARRIED PARTNERSHIP SYMBOL
- {0x26B0, 0x26B1, prExtendedPictographic}, // E1.0 [2] (âš°ï¸..âš±ï¸) coffin..funeral urn
- {0x26B2, 0x26BC, prExtendedPictographic}, // E0.0 [11] (âš²..âš¼) NEUTER..SESQUIQUADRATE
- {0x26BD, 0x26BE, prExtendedPictographic}, // E0.6 [2] (âš½..âš¾) soccer ball..baseball
- {0x26BF, 0x26C3, prExtendedPictographic}, // E0.0 [5] (⚿..⛃) SQUARED KEY..BLACK DRAUGHTS KING
- {0x26C4, 0x26C5, prExtendedPictographic}, // E0.6 [2] (⛄..⛅) snowman without snow..sun behind cloud
- {0x26C6, 0x26C7, prExtendedPictographic}, // E0.0 [2] (⛆..⛇) RAIN..BLACK SNOWMAN
- {0x26C8, 0x26C8, prExtendedPictographic}, // E0.7 [1] (⛈ï¸) cloud with lightning and rain
- {0x26C9, 0x26CD, prExtendedPictographic}, // E0.0 [5] (⛉..â›) TURNED WHITE SHOGI PIECE..DISABLED CAR
- {0x26CE, 0x26CE, prExtendedPictographic}, // E0.6 [1] (⛎) Ophiuchus
- {0x26CF, 0x26CF, prExtendedPictographic}, // E0.7 [1] (â›ï¸) pick
- {0x26D0, 0x26D0, prExtendedPictographic}, // E0.0 [1] (â›) CAR SLIDING
- {0x26D1, 0x26D1, prExtendedPictographic}, // E0.7 [1] (⛑ï¸) rescue worker’s helmet
- {0x26D2, 0x26D2, prExtendedPictographic}, // E0.0 [1] (â›’) CIRCLED CROSSING LANES
- {0x26D3, 0x26D3, prExtendedPictographic}, // E0.7 [1] (⛓ï¸) chains
- {0x26D4, 0x26D4, prExtendedPictographic}, // E0.6 [1] (â›”) no entry
- {0x26D5, 0x26E8, prExtendedPictographic}, // E0.0 [20] (⛕..⛨) ALTERNATE ONE-WAY LEFT WAY TRAFFIC..BLACK CROSS ON SHIELD
- {0x26E9, 0x26E9, prExtendedPictographic}, // E0.7 [1] (⛩ï¸) shinto shrine
- {0x26EA, 0x26EA, prExtendedPictographic}, // E0.6 [1] (⛪) church
- {0x26EB, 0x26EF, prExtendedPictographic}, // E0.0 [5] (⛫..⛯) CASTLE..MAP SYMBOL FOR LIGHTHOUSE
- {0x26F0, 0x26F1, prExtendedPictographic}, // E0.7 [2] (â›°ï¸..â›±ï¸) mountain..umbrella on ground
- {0x26F2, 0x26F3, prExtendedPictographic}, // E0.6 [2] (⛲..⛳) fountain..flag in hole
- {0x26F4, 0x26F4, prExtendedPictographic}, // E0.7 [1] (â›´ï¸) ferry
- {0x26F5, 0x26F5, prExtendedPictographic}, // E0.6 [1] (⛵) sailboat
- {0x26F6, 0x26F6, prExtendedPictographic}, // E0.0 [1] (â›¶) SQUARE FOUR CORNERS
- {0x26F7, 0x26F9, prExtendedPictographic}, // E0.7 [3] (â›·ï¸..⛹ï¸) skier..person bouncing ball
- {0x26FA, 0x26FA, prExtendedPictographic}, // E0.6 [1] (⛺) tent
- {0x26FB, 0x26FC, prExtendedPictographic}, // E0.0 [2] (⛻..⛼) JAPANESE BANK SYMBOL..HEADSTONE GRAVEYARD SYMBOL
- {0x26FD, 0x26FD, prExtendedPictographic}, // E0.6 [1] (⛽) fuel pump
- {0x26FE, 0x2701, prExtendedPictographic}, // E0.0 [4] (⛾..âœ) CUP ON BLACK SQUARE..UPPER BLADE SCISSORS
- {0x2702, 0x2702, prExtendedPictographic}, // E0.6 [1] (✂ï¸) scissors
- {0x2703, 0x2704, prExtendedPictographic}, // E0.0 [2] (✃..✄) LOWER BLADE SCISSORS..WHITE SCISSORS
- {0x2705, 0x2705, prExtendedPictographic}, // E0.6 [1] (✅) check mark button
- {0x2708, 0x270C, prExtendedPictographic}, // E0.6 [5] (✈ï¸..✌ï¸) airplane..victory hand
- {0x270D, 0x270D, prExtendedPictographic}, // E0.7 [1] (âœï¸) writing hand
- {0x270E, 0x270E, prExtendedPictographic}, // E0.0 [1] (✎) LOWER RIGHT PENCIL
- {0x270F, 0x270F, prExtendedPictographic}, // E0.6 [1] (âœï¸) pencil
- {0x2710, 0x2711, prExtendedPictographic}, // E0.0 [2] (âœ..✑) UPPER RIGHT PENCIL..WHITE NIB
- {0x2712, 0x2712, prExtendedPictographic}, // E0.6 [1] (✒ï¸) black nib
- {0x2714, 0x2714, prExtendedPictographic}, // E0.6 [1] (✔ï¸) check mark
- {0x2716, 0x2716, prExtendedPictographic}, // E0.6 [1] (✖ï¸) multiply
- {0x271D, 0x271D, prExtendedPictographic}, // E0.7 [1] (âœï¸) latin cross
- {0x2721, 0x2721, prExtendedPictographic}, // E0.7 [1] (✡ï¸) star of David
- {0x2728, 0x2728, prExtendedPictographic}, // E0.6 [1] (✨) sparkles
- {0x2733, 0x2734, prExtendedPictographic}, // E0.6 [2] (✳ï¸..✴ï¸) eight-spoked asterisk..eight-pointed star
- {0x2744, 0x2744, prExtendedPictographic}, // E0.6 [1] (â„ï¸) snowflake
- {0x2747, 0x2747, prExtendedPictographic}, // E0.6 [1] (â‡ï¸) sparkle
- {0x274C, 0x274C, prExtendedPictographic}, // E0.6 [1] (âŒ) cross mark
- {0x274E, 0x274E, prExtendedPictographic}, // E0.6 [1] (âŽ) cross mark button
- {0x2753, 0x2755, prExtendedPictographic}, // E0.6 [3] (â“..â•) red question mark..white exclamation mark
- {0x2757, 0x2757, prExtendedPictographic}, // E0.6 [1] (â—) red exclamation mark
- {0x2763, 0x2763, prExtendedPictographic}, // E1.0 [1] (â£ï¸) heart exclamation
- {0x2764, 0x2764, prExtendedPictographic}, // E0.6 [1] (â¤ï¸) red heart
- {0x2765, 0x2767, prExtendedPictographic}, // E0.0 [3] (â¥..â§) ROTATED HEAVY BLACK HEART BULLET..ROTATED FLORAL HEART BULLET
- {0x2795, 0x2797, prExtendedPictographic}, // E0.6 [3] (âž•..âž—) plus..divide
- {0x27A1, 0x27A1, prExtendedPictographic}, // E0.6 [1] (âž¡ï¸) right arrow
- {0x27B0, 0x27B0, prExtendedPictographic}, // E0.6 [1] (âž°) curly loop
- {0x27BF, 0x27BF, prExtendedPictographic}, // E1.0 [1] (âž¿) double curly loop
- {0x2934, 0x2935, prExtendedPictographic}, // E0.6 [2] (⤴ï¸..⤵ï¸) right arrow curving up..right arrow curving down
- {0x2B05, 0x2B07, prExtendedPictographic}, // E0.6 [3] (⬅ï¸..⬇ï¸) left arrow..down arrow
- {0x2B1B, 0x2B1C, prExtendedPictographic}, // E0.6 [2] (⬛..⬜) black large square..white large square
- {0x2B50, 0x2B50, prExtendedPictographic}, // E0.6 [1] (â) star
- {0x2B55, 0x2B55, prExtendedPictographic}, // E0.6 [1] (â•) hollow red circle
- {0x2CEF, 0x2CF1, prExtend}, // Mn [3] COPTIC COMBINING NI ABOVE..COPTIC COMBINING SPIRITUS LENIS
- {0x2D7F, 0x2D7F, prExtend}, // Mn TIFINAGH CONSONANT JOINER
- {0x2DE0, 0x2DFF, prExtend}, // Mn [32] COMBINING CYRILLIC LETTER BE..COMBINING CYRILLIC LETTER IOTIFIED BIG YUS
- {0x302A, 0x302D, prExtend}, // Mn [4] IDEOGRAPHIC LEVEL TONE MARK..IDEOGRAPHIC ENTERING TONE MARK
- {0x302E, 0x302F, prExtend}, // Mc [2] HANGUL SINGLE DOT TONE MARK..HANGUL DOUBLE DOT TONE MARK
- {0x3030, 0x3030, prExtendedPictographic}, // E0.6 [1] (〰ï¸) wavy dash
- {0x303D, 0x303D, prExtendedPictographic}, // E0.6 [1] (〽ï¸) part alternation mark
- {0x3099, 0x309A, prExtend}, // Mn [2] COMBINING KATAKANA-HIRAGANA VOICED SOUND MARK..COMBINING KATAKANA-HIRAGANA SEMI-VOICED SOUND MARK
- {0x3297, 0x3297, prExtendedPictographic}, // E0.6 [1] (㊗ï¸) Japanese “congratulations†button
- {0x3299, 0x3299, prExtendedPictographic}, // E0.6 [1] (㊙ï¸) Japanese “secret†button
- {0xA66F, 0xA66F, prExtend}, // Mn COMBINING CYRILLIC VZMET
- {0xA670, 0xA672, prExtend}, // Me [3] COMBINING CYRILLIC TEN MILLIONS SIGN..COMBINING CYRILLIC THOUSAND MILLIONS SIGN
- {0xA674, 0xA67D, prExtend}, // Mn [10] COMBINING CYRILLIC LETTER UKRAINIAN IE..COMBINING CYRILLIC PAYEROK
- {0xA69E, 0xA69F, prExtend}, // Mn [2] COMBINING CYRILLIC LETTER EF..COMBINING CYRILLIC LETTER IOTIFIED E
- {0xA6F0, 0xA6F1, prExtend}, // Mn [2] BAMUM COMBINING MARK KOQNDON..BAMUM COMBINING MARK TUKWENTIS
- {0xA802, 0xA802, prExtend}, // Mn SYLOTI NAGRI SIGN DVISVARA
- {0xA806, 0xA806, prExtend}, // Mn SYLOTI NAGRI SIGN HASANTA
- {0xA80B, 0xA80B, prExtend}, // Mn SYLOTI NAGRI SIGN ANUSVARA
- {0xA823, 0xA824, prSpacingMark}, // Mc [2] SYLOTI NAGRI VOWEL SIGN A..SYLOTI NAGRI VOWEL SIGN I
- {0xA825, 0xA826, prExtend}, // Mn [2] SYLOTI NAGRI VOWEL SIGN U..SYLOTI NAGRI VOWEL SIGN E
- {0xA827, 0xA827, prSpacingMark}, // Mc SYLOTI NAGRI VOWEL SIGN OO
- {0xA82C, 0xA82C, prExtend}, // Mn SYLOTI NAGRI SIGN ALTERNATE HASANTA
- {0xA880, 0xA881, prSpacingMark}, // Mc [2] SAURASHTRA SIGN ANUSVARA..SAURASHTRA SIGN VISARGA
- {0xA8B4, 0xA8C3, prSpacingMark}, // Mc [16] SAURASHTRA CONSONANT SIGN HAARU..SAURASHTRA VOWEL SIGN AU
- {0xA8C4, 0xA8C5, prExtend}, // Mn [2] SAURASHTRA SIGN VIRAMA..SAURASHTRA SIGN CANDRABINDU
- {0xA8E0, 0xA8F1, prExtend}, // Mn [18] COMBINING DEVANAGARI DIGIT ZERO..COMBINING DEVANAGARI SIGN AVAGRAHA
- {0xA8FF, 0xA8FF, prExtend}, // Mn DEVANAGARI VOWEL SIGN AY
- {0xA926, 0xA92D, prExtend}, // Mn [8] KAYAH LI VOWEL UE..KAYAH LI TONE CALYA PLOPHU
- {0xA947, 0xA951, prExtend}, // Mn [11] REJANG VOWEL SIGN I..REJANG CONSONANT SIGN R
- {0xA952, 0xA953, prSpacingMark}, // Mc [2] REJANG CONSONANT SIGN H..REJANG VIRAMA
- {0xA960, 0xA97C, prL}, // Lo [29] HANGUL CHOSEONG TIKEUT-MIEUM..HANGUL CHOSEONG SSANGYEORINHIEUH
- {0xA980, 0xA982, prExtend}, // Mn [3] JAVANESE SIGN PANYANGGA..JAVANESE SIGN LAYAR
- {0xA983, 0xA983, prSpacingMark}, // Mc JAVANESE SIGN WIGNYAN
- {0xA9B3, 0xA9B3, prExtend}, // Mn JAVANESE SIGN CECAK TELU
- {0xA9B4, 0xA9B5, prSpacingMark}, // Mc [2] JAVANESE VOWEL SIGN TARUNG..JAVANESE VOWEL SIGN TOLONG
- {0xA9B6, 0xA9B9, prExtend}, // Mn [4] JAVANESE VOWEL SIGN WULU..JAVANESE VOWEL SIGN SUKU MENDUT
- {0xA9BA, 0xA9BB, prSpacingMark}, // Mc [2] JAVANESE VOWEL SIGN TALING..JAVANESE VOWEL SIGN DIRGA MURE
- {0xA9BC, 0xA9BD, prExtend}, // Mn [2] JAVANESE VOWEL SIGN PEPET..JAVANESE CONSONANT SIGN KERET
- {0xA9BE, 0xA9C0, prSpacingMark}, // Mc [3] JAVANESE CONSONANT SIGN PENGKAL..JAVANESE PANGKON
- {0xA9E5, 0xA9E5, prExtend}, // Mn MYANMAR SIGN SHAN SAW
- {0xAA29, 0xAA2E, prExtend}, // Mn [6] CHAM VOWEL SIGN AA..CHAM VOWEL SIGN OE
- {0xAA2F, 0xAA30, prSpacingMark}, // Mc [2] CHAM VOWEL SIGN O..CHAM VOWEL SIGN AI
- {0xAA31, 0xAA32, prExtend}, // Mn [2] CHAM VOWEL SIGN AU..CHAM VOWEL SIGN UE
- {0xAA33, 0xAA34, prSpacingMark}, // Mc [2] CHAM CONSONANT SIGN YA..CHAM CONSONANT SIGN RA
- {0xAA35, 0xAA36, prExtend}, // Mn [2] CHAM CONSONANT SIGN LA..CHAM CONSONANT SIGN WA
- {0xAA43, 0xAA43, prExtend}, // Mn CHAM CONSONANT SIGN FINAL NG
- {0xAA4C, 0xAA4C, prExtend}, // Mn CHAM CONSONANT SIGN FINAL M
- {0xAA4D, 0xAA4D, prSpacingMark}, // Mc CHAM CONSONANT SIGN FINAL H
- {0xAA7C, 0xAA7C, prExtend}, // Mn MYANMAR SIGN TAI LAING TONE-2
- {0xAAB0, 0xAAB0, prExtend}, // Mn TAI VIET MAI KANG
- {0xAAB2, 0xAAB4, prExtend}, // Mn [3] TAI VIET VOWEL I..TAI VIET VOWEL U
- {0xAAB7, 0xAAB8, prExtend}, // Mn [2] TAI VIET MAI KHIT..TAI VIET VOWEL IA
- {0xAABE, 0xAABF, prExtend}, // Mn [2] TAI VIET VOWEL AM..TAI VIET TONE MAI EK
- {0xAAC1, 0xAAC1, prExtend}, // Mn TAI VIET TONE MAI THO
- {0xAAEB, 0xAAEB, prSpacingMark}, // Mc MEETEI MAYEK VOWEL SIGN II
- {0xAAEC, 0xAAED, prExtend}, // Mn [2] MEETEI MAYEK VOWEL SIGN UU..MEETEI MAYEK VOWEL SIGN AAI
- {0xAAEE, 0xAAEF, prSpacingMark}, // Mc [2] MEETEI MAYEK VOWEL SIGN AU..MEETEI MAYEK VOWEL SIGN AAU
- {0xAAF5, 0xAAF5, prSpacingMark}, // Mc MEETEI MAYEK VOWEL SIGN VISARGA
- {0xAAF6, 0xAAF6, prExtend}, // Mn MEETEI MAYEK VIRAMA
- {0xABE3, 0xABE4, prSpacingMark}, // Mc [2] MEETEI MAYEK VOWEL SIGN ONAP..MEETEI MAYEK VOWEL SIGN INAP
- {0xABE5, 0xABE5, prExtend}, // Mn MEETEI MAYEK VOWEL SIGN ANAP
- {0xABE6, 0xABE7, prSpacingMark}, // Mc [2] MEETEI MAYEK VOWEL SIGN YENAP..MEETEI MAYEK VOWEL SIGN SOUNAP
- {0xABE8, 0xABE8, prExtend}, // Mn MEETEI MAYEK VOWEL SIGN UNAP
- {0xABE9, 0xABEA, prSpacingMark}, // Mc [2] MEETEI MAYEK VOWEL SIGN CHEINAP..MEETEI MAYEK VOWEL SIGN NUNG
- {0xABEC, 0xABEC, prSpacingMark}, // Mc MEETEI MAYEK LUM IYEK
- {0xABED, 0xABED, prExtend}, // Mn MEETEI MAYEK APUN IYEK
- {0xAC00, 0xAC00, prLV}, // Lo HANGUL SYLLABLE GA
- {0xAC01, 0xAC1B, prLVT}, // Lo [27] HANGUL SYLLABLE GAG..HANGUL SYLLABLE GAH
- {0xAC1C, 0xAC1C, prLV}, // Lo HANGUL SYLLABLE GAE
- {0xAC1D, 0xAC37, prLVT}, // Lo [27] HANGUL SYLLABLE GAEG..HANGUL SYLLABLE GAEH
- {0xAC38, 0xAC38, prLV}, // Lo HANGUL SYLLABLE GYA
- {0xAC39, 0xAC53, prLVT}, // Lo [27] HANGUL SYLLABLE GYAG..HANGUL SYLLABLE GYAH
- {0xAC54, 0xAC54, prLV}, // Lo HANGUL SYLLABLE GYAE
- {0xAC55, 0xAC6F, prLVT}, // Lo [27] HANGUL SYLLABLE GYAEG..HANGUL SYLLABLE GYAEH
- {0xAC70, 0xAC70, prLV}, // Lo HANGUL SYLLABLE GEO
- {0xAC71, 0xAC8B, prLVT}, // Lo [27] HANGUL SYLLABLE GEOG..HANGUL SYLLABLE GEOH
- {0xAC8C, 0xAC8C, prLV}, // Lo HANGUL SYLLABLE GE
- {0xAC8D, 0xACA7, prLVT}, // Lo [27] HANGUL SYLLABLE GEG..HANGUL SYLLABLE GEH
- {0xACA8, 0xACA8, prLV}, // Lo HANGUL SYLLABLE GYEO
- {0xACA9, 0xACC3, prLVT}, // Lo [27] HANGUL SYLLABLE GYEOG..HANGUL SYLLABLE GYEOH
- {0xACC4, 0xACC4, prLV}, // Lo HANGUL SYLLABLE GYE
- {0xACC5, 0xACDF, prLVT}, // Lo [27] HANGUL SYLLABLE GYEG..HANGUL SYLLABLE GYEH
- {0xACE0, 0xACE0, prLV}, // Lo HANGUL SYLLABLE GO
- {0xACE1, 0xACFB, prLVT}, // Lo [27] HANGUL SYLLABLE GOG..HANGUL SYLLABLE GOH
- {0xACFC, 0xACFC, prLV}, // Lo HANGUL SYLLABLE GWA
- {0xACFD, 0xAD17, prLVT}, // Lo [27] HANGUL SYLLABLE GWAG..HANGUL SYLLABLE GWAH
- {0xAD18, 0xAD18, prLV}, // Lo HANGUL SYLLABLE GWAE
- {0xAD19, 0xAD33, prLVT}, // Lo [27] HANGUL SYLLABLE GWAEG..HANGUL SYLLABLE GWAEH
- {0xAD34, 0xAD34, prLV}, // Lo HANGUL SYLLABLE GOE
- {0xAD35, 0xAD4F, prLVT}, // Lo [27] HANGUL SYLLABLE GOEG..HANGUL SYLLABLE GOEH
- {0xAD50, 0xAD50, prLV}, // Lo HANGUL SYLLABLE GYO
- {0xAD51, 0xAD6B, prLVT}, // Lo [27] HANGUL SYLLABLE GYOG..HANGUL SYLLABLE GYOH
- {0xAD6C, 0xAD6C, prLV}, // Lo HANGUL SYLLABLE GU
- {0xAD6D, 0xAD87, prLVT}, // Lo [27] HANGUL SYLLABLE GUG..HANGUL SYLLABLE GUH
- {0xAD88, 0xAD88, prLV}, // Lo HANGUL SYLLABLE GWEO
- {0xAD89, 0xADA3, prLVT}, // Lo [27] HANGUL SYLLABLE GWEOG..HANGUL SYLLABLE GWEOH
- {0xADA4, 0xADA4, prLV}, // Lo HANGUL SYLLABLE GWE
- {0xADA5, 0xADBF, prLVT}, // Lo [27] HANGUL SYLLABLE GWEG..HANGUL SYLLABLE GWEH
- {0xADC0, 0xADC0, prLV}, // Lo HANGUL SYLLABLE GWI
- {0xADC1, 0xADDB, prLVT}, // Lo [27] HANGUL SYLLABLE GWIG..HANGUL SYLLABLE GWIH
- {0xADDC, 0xADDC, prLV}, // Lo HANGUL SYLLABLE GYU
- {0xADDD, 0xADF7, prLVT}, // Lo [27] HANGUL SYLLABLE GYUG..HANGUL SYLLABLE GYUH
- {0xADF8, 0xADF8, prLV}, // Lo HANGUL SYLLABLE GEU
- {0xADF9, 0xAE13, prLVT}, // Lo [27] HANGUL SYLLABLE GEUG..HANGUL SYLLABLE GEUH
- {0xAE14, 0xAE14, prLV}, // Lo HANGUL SYLLABLE GYI
- {0xAE15, 0xAE2F, prLVT}, // Lo [27] HANGUL SYLLABLE GYIG..HANGUL SYLLABLE GYIH
- {0xAE30, 0xAE30, prLV}, // Lo HANGUL SYLLABLE GI
- {0xAE31, 0xAE4B, prLVT}, // Lo [27] HANGUL SYLLABLE GIG..HANGUL SYLLABLE GIH
- {0xAE4C, 0xAE4C, prLV}, // Lo HANGUL SYLLABLE GGA
- {0xAE4D, 0xAE67, prLVT}, // Lo [27] HANGUL SYLLABLE GGAG..HANGUL SYLLABLE GGAH
- {0xAE68, 0xAE68, prLV}, // Lo HANGUL SYLLABLE GGAE
- {0xAE69, 0xAE83, prLVT}, // Lo [27] HANGUL SYLLABLE GGAEG..HANGUL SYLLABLE GGAEH
- {0xAE84, 0xAE84, prLV}, // Lo HANGUL SYLLABLE GGYA
- {0xAE85, 0xAE9F, prLVT}, // Lo [27] HANGUL SYLLABLE GGYAG..HANGUL SYLLABLE GGYAH
- {0xAEA0, 0xAEA0, prLV}, // Lo HANGUL SYLLABLE GGYAE
- {0xAEA1, 0xAEBB, prLVT}, // Lo [27] HANGUL SYLLABLE GGYAEG..HANGUL SYLLABLE GGYAEH
- {0xAEBC, 0xAEBC, prLV}, // Lo HANGUL SYLLABLE GGEO
- {0xAEBD, 0xAED7, prLVT}, // Lo [27] HANGUL SYLLABLE GGEOG..HANGUL SYLLABLE GGEOH
- {0xAED8, 0xAED8, prLV}, // Lo HANGUL SYLLABLE GGE
- {0xAED9, 0xAEF3, prLVT}, // Lo [27] HANGUL SYLLABLE GGEG..HANGUL SYLLABLE GGEH
- {0xAEF4, 0xAEF4, prLV}, // Lo HANGUL SYLLABLE GGYEO
- {0xAEF5, 0xAF0F, prLVT}, // Lo [27] HANGUL SYLLABLE GGYEOG..HANGUL SYLLABLE GGYEOH
- {0xAF10, 0xAF10, prLV}, // Lo HANGUL SYLLABLE GGYE
- {0xAF11, 0xAF2B, prLVT}, // Lo [27] HANGUL SYLLABLE GGYEG..HANGUL SYLLABLE GGYEH
- {0xAF2C, 0xAF2C, prLV}, // Lo HANGUL SYLLABLE GGO
- {0xAF2D, 0xAF47, prLVT}, // Lo [27] HANGUL SYLLABLE GGOG..HANGUL SYLLABLE GGOH
- {0xAF48, 0xAF48, prLV}, // Lo HANGUL SYLLABLE GGWA
- {0xAF49, 0xAF63, prLVT}, // Lo [27] HANGUL SYLLABLE GGWAG..HANGUL SYLLABLE GGWAH
- {0xAF64, 0xAF64, prLV}, // Lo HANGUL SYLLABLE GGWAE
- {0xAF65, 0xAF7F, prLVT}, // Lo [27] HANGUL SYLLABLE GGWAEG..HANGUL SYLLABLE GGWAEH
- {0xAF80, 0xAF80, prLV}, // Lo HANGUL SYLLABLE GGOE
- {0xAF81, 0xAF9B, prLVT}, // Lo [27] HANGUL SYLLABLE GGOEG..HANGUL SYLLABLE GGOEH
- {0xAF9C, 0xAF9C, prLV}, // Lo HANGUL SYLLABLE GGYO
- {0xAF9D, 0xAFB7, prLVT}, // Lo [27] HANGUL SYLLABLE GGYOG..HANGUL SYLLABLE GGYOH
- {0xAFB8, 0xAFB8, prLV}, // Lo HANGUL SYLLABLE GGU
- {0xAFB9, 0xAFD3, prLVT}, // Lo [27] HANGUL SYLLABLE GGUG..HANGUL SYLLABLE GGUH
- {0xAFD4, 0xAFD4, prLV}, // Lo HANGUL SYLLABLE GGWEO
- {0xAFD5, 0xAFEF, prLVT}, // Lo [27] HANGUL SYLLABLE GGWEOG..HANGUL SYLLABLE GGWEOH
- {0xAFF0, 0xAFF0, prLV}, // Lo HANGUL SYLLABLE GGWE
- {0xAFF1, 0xB00B, prLVT}, // Lo [27] HANGUL SYLLABLE GGWEG..HANGUL SYLLABLE GGWEH
- {0xB00C, 0xB00C, prLV}, // Lo HANGUL SYLLABLE GGWI
- {0xB00D, 0xB027, prLVT}, // Lo [27] HANGUL SYLLABLE GGWIG..HANGUL SYLLABLE GGWIH
- {0xB028, 0xB028, prLV}, // Lo HANGUL SYLLABLE GGYU
- {0xB029, 0xB043, prLVT}, // Lo [27] HANGUL SYLLABLE GGYUG..HANGUL SYLLABLE GGYUH
- {0xB044, 0xB044, prLV}, // Lo HANGUL SYLLABLE GGEU
- {0xB045, 0xB05F, prLVT}, // Lo [27] HANGUL SYLLABLE GGEUG..HANGUL SYLLABLE GGEUH
- {0xB060, 0xB060, prLV}, // Lo HANGUL SYLLABLE GGYI
- {0xB061, 0xB07B, prLVT}, // Lo [27] HANGUL SYLLABLE GGYIG..HANGUL SYLLABLE GGYIH
- {0xB07C, 0xB07C, prLV}, // Lo HANGUL SYLLABLE GGI
- {0xB07D, 0xB097, prLVT}, // Lo [27] HANGUL SYLLABLE GGIG..HANGUL SYLLABLE GGIH
- {0xB098, 0xB098, prLV}, // Lo HANGUL SYLLABLE NA
- {0xB099, 0xB0B3, prLVT}, // Lo [27] HANGUL SYLLABLE NAG..HANGUL SYLLABLE NAH
- {0xB0B4, 0xB0B4, prLV}, // Lo HANGUL SYLLABLE NAE
- {0xB0B5, 0xB0CF, prLVT}, // Lo [27] HANGUL SYLLABLE NAEG..HANGUL SYLLABLE NAEH
- {0xB0D0, 0xB0D0, prLV}, // Lo HANGUL SYLLABLE NYA
- {0xB0D1, 0xB0EB, prLVT}, // Lo [27] HANGUL SYLLABLE NYAG..HANGUL SYLLABLE NYAH
- {0xB0EC, 0xB0EC, prLV}, // Lo HANGUL SYLLABLE NYAE
- {0xB0ED, 0xB107, prLVT}, // Lo [27] HANGUL SYLLABLE NYAEG..HANGUL SYLLABLE NYAEH
- {0xB108, 0xB108, prLV}, // Lo HANGUL SYLLABLE NEO
- {0xB109, 0xB123, prLVT}, // Lo [27] HANGUL SYLLABLE NEOG..HANGUL SYLLABLE NEOH
- {0xB124, 0xB124, prLV}, // Lo HANGUL SYLLABLE NE
- {0xB125, 0xB13F, prLVT}, // Lo [27] HANGUL SYLLABLE NEG..HANGUL SYLLABLE NEH
- {0xB140, 0xB140, prLV}, // Lo HANGUL SYLLABLE NYEO
- {0xB141, 0xB15B, prLVT}, // Lo [27] HANGUL SYLLABLE NYEOG..HANGUL SYLLABLE NYEOH
- {0xB15C, 0xB15C, prLV}, // Lo HANGUL SYLLABLE NYE
- {0xB15D, 0xB177, prLVT}, // Lo [27] HANGUL SYLLABLE NYEG..HANGUL SYLLABLE NYEH
- {0xB178, 0xB178, prLV}, // Lo HANGUL SYLLABLE NO
- {0xB179, 0xB193, prLVT}, // Lo [27] HANGUL SYLLABLE NOG..HANGUL SYLLABLE NOH
- {0xB194, 0xB194, prLV}, // Lo HANGUL SYLLABLE NWA
- {0xB195, 0xB1AF, prLVT}, // Lo [27] HANGUL SYLLABLE NWAG..HANGUL SYLLABLE NWAH
- {0xB1B0, 0xB1B0, prLV}, // Lo HANGUL SYLLABLE NWAE
- {0xB1B1, 0xB1CB, prLVT}, // Lo [27] HANGUL SYLLABLE NWAEG..HANGUL SYLLABLE NWAEH
- {0xB1CC, 0xB1CC, prLV}, // Lo HANGUL SYLLABLE NOE
- {0xB1CD, 0xB1E7, prLVT}, // Lo [27] HANGUL SYLLABLE NOEG..HANGUL SYLLABLE NOEH
- {0xB1E8, 0xB1E8, prLV}, // Lo HANGUL SYLLABLE NYO
- {0xB1E9, 0xB203, prLVT}, // Lo [27] HANGUL SYLLABLE NYOG..HANGUL SYLLABLE NYOH
- {0xB204, 0xB204, prLV}, // Lo HANGUL SYLLABLE NU
- {0xB205, 0xB21F, prLVT}, // Lo [27] HANGUL SYLLABLE NUG..HANGUL SYLLABLE NUH
- {0xB220, 0xB220, prLV}, // Lo HANGUL SYLLABLE NWEO
- {0xB221, 0xB23B, prLVT}, // Lo [27] HANGUL SYLLABLE NWEOG..HANGUL SYLLABLE NWEOH
- {0xB23C, 0xB23C, prLV}, // Lo HANGUL SYLLABLE NWE
- {0xB23D, 0xB257, prLVT}, // Lo [27] HANGUL SYLLABLE NWEG..HANGUL SYLLABLE NWEH
- {0xB258, 0xB258, prLV}, // Lo HANGUL SYLLABLE NWI
- {0xB259, 0xB273, prLVT}, // Lo [27] HANGUL SYLLABLE NWIG..HANGUL SYLLABLE NWIH
- {0xB274, 0xB274, prLV}, // Lo HANGUL SYLLABLE NYU
- {0xB275, 0xB28F, prLVT}, // Lo [27] HANGUL SYLLABLE NYUG..HANGUL SYLLABLE NYUH
- {0xB290, 0xB290, prLV}, // Lo HANGUL SYLLABLE NEU
- {0xB291, 0xB2AB, prLVT}, // Lo [27] HANGUL SYLLABLE NEUG..HANGUL SYLLABLE NEUH
- {0xB2AC, 0xB2AC, prLV}, // Lo HANGUL SYLLABLE NYI
- {0xB2AD, 0xB2C7, prLVT}, // Lo [27] HANGUL SYLLABLE NYIG..HANGUL SYLLABLE NYIH
- {0xB2C8, 0xB2C8, prLV}, // Lo HANGUL SYLLABLE NI
- {0xB2C9, 0xB2E3, prLVT}, // Lo [27] HANGUL SYLLABLE NIG..HANGUL SYLLABLE NIH
- {0xB2E4, 0xB2E4, prLV}, // Lo HANGUL SYLLABLE DA
- {0xB2E5, 0xB2FF, prLVT}, // Lo [27] HANGUL SYLLABLE DAG..HANGUL SYLLABLE DAH
- {0xB300, 0xB300, prLV}, // Lo HANGUL SYLLABLE DAE
- {0xB301, 0xB31B, prLVT}, // Lo [27] HANGUL SYLLABLE DAEG..HANGUL SYLLABLE DAEH
- {0xB31C, 0xB31C, prLV}, // Lo HANGUL SYLLABLE DYA
- {0xB31D, 0xB337, prLVT}, // Lo [27] HANGUL SYLLABLE DYAG..HANGUL SYLLABLE DYAH
- {0xB338, 0xB338, prLV}, // Lo HANGUL SYLLABLE DYAE
- {0xB339, 0xB353, prLVT}, // Lo [27] HANGUL SYLLABLE DYAEG..HANGUL SYLLABLE DYAEH
- {0xB354, 0xB354, prLV}, // Lo HANGUL SYLLABLE DEO
- {0xB355, 0xB36F, prLVT}, // Lo [27] HANGUL SYLLABLE DEOG..HANGUL SYLLABLE DEOH
- {0xB370, 0xB370, prLV}, // Lo HANGUL SYLLABLE DE
- {0xB371, 0xB38B, prLVT}, // Lo [27] HANGUL SYLLABLE DEG..HANGUL SYLLABLE DEH
- {0xB38C, 0xB38C, prLV}, // Lo HANGUL SYLLABLE DYEO
- {0xB38D, 0xB3A7, prLVT}, // Lo [27] HANGUL SYLLABLE DYEOG..HANGUL SYLLABLE DYEOH
- {0xB3A8, 0xB3A8, prLV}, // Lo HANGUL SYLLABLE DYE
- {0xB3A9, 0xB3C3, prLVT}, // Lo [27] HANGUL SYLLABLE DYEG..HANGUL SYLLABLE DYEH
- {0xB3C4, 0xB3C4, prLV}, // Lo HANGUL SYLLABLE DO
- {0xB3C5, 0xB3DF, prLVT}, // Lo [27] HANGUL SYLLABLE DOG..HANGUL SYLLABLE DOH
- {0xB3E0, 0xB3E0, prLV}, // Lo HANGUL SYLLABLE DWA
- {0xB3E1, 0xB3FB, prLVT}, // Lo [27] HANGUL SYLLABLE DWAG..HANGUL SYLLABLE DWAH
- {0xB3FC, 0xB3FC, prLV}, // Lo HANGUL SYLLABLE DWAE
- {0xB3FD, 0xB417, prLVT}, // Lo [27] HANGUL SYLLABLE DWAEG..HANGUL SYLLABLE DWAEH
- {0xB418, 0xB418, prLV}, // Lo HANGUL SYLLABLE DOE
- {0xB419, 0xB433, prLVT}, // Lo [27] HANGUL SYLLABLE DOEG..HANGUL SYLLABLE DOEH
- {0xB434, 0xB434, prLV}, // Lo HANGUL SYLLABLE DYO
- {0xB435, 0xB44F, prLVT}, // Lo [27] HANGUL SYLLABLE DYOG..HANGUL SYLLABLE DYOH
- {0xB450, 0xB450, prLV}, // Lo HANGUL SYLLABLE DU
- {0xB451, 0xB46B, prLVT}, // Lo [27] HANGUL SYLLABLE DUG..HANGUL SYLLABLE DUH
- {0xB46C, 0xB46C, prLV}, // Lo HANGUL SYLLABLE DWEO
- {0xB46D, 0xB487, prLVT}, // Lo [27] HANGUL SYLLABLE DWEOG..HANGUL SYLLABLE DWEOH
- {0xB488, 0xB488, prLV}, // Lo HANGUL SYLLABLE DWE
- {0xB489, 0xB4A3, prLVT}, // Lo [27] HANGUL SYLLABLE DWEG..HANGUL SYLLABLE DWEH
- {0xB4A4, 0xB4A4, prLV}, // Lo HANGUL SYLLABLE DWI
- {0xB4A5, 0xB4BF, prLVT}, // Lo [27] HANGUL SYLLABLE DWIG..HANGUL SYLLABLE DWIH
- {0xB4C0, 0xB4C0, prLV}, // Lo HANGUL SYLLABLE DYU
- {0xB4C1, 0xB4DB, prLVT}, // Lo [27] HANGUL SYLLABLE DYUG..HANGUL SYLLABLE DYUH
- {0xB4DC, 0xB4DC, prLV}, // Lo HANGUL SYLLABLE DEU
- {0xB4DD, 0xB4F7, prLVT}, // Lo [27] HANGUL SYLLABLE DEUG..HANGUL SYLLABLE DEUH
- {0xB4F8, 0xB4F8, prLV}, // Lo HANGUL SYLLABLE DYI
- {0xB4F9, 0xB513, prLVT}, // Lo [27] HANGUL SYLLABLE DYIG..HANGUL SYLLABLE DYIH
- {0xB514, 0xB514, prLV}, // Lo HANGUL SYLLABLE DI
- {0xB515, 0xB52F, prLVT}, // Lo [27] HANGUL SYLLABLE DIG..HANGUL SYLLABLE DIH
- {0xB530, 0xB530, prLV}, // Lo HANGUL SYLLABLE DDA
- {0xB531, 0xB54B, prLVT}, // Lo [27] HANGUL SYLLABLE DDAG..HANGUL SYLLABLE DDAH
- {0xB54C, 0xB54C, prLV}, // Lo HANGUL SYLLABLE DDAE
- {0xB54D, 0xB567, prLVT}, // Lo [27] HANGUL SYLLABLE DDAEG..HANGUL SYLLABLE DDAEH
- {0xB568, 0xB568, prLV}, // Lo HANGUL SYLLABLE DDYA
- {0xB569, 0xB583, prLVT}, // Lo [27] HANGUL SYLLABLE DDYAG..HANGUL SYLLABLE DDYAH
- {0xB584, 0xB584, prLV}, // Lo HANGUL SYLLABLE DDYAE
- {0xB585, 0xB59F, prLVT}, // Lo [27] HANGUL SYLLABLE DDYAEG..HANGUL SYLLABLE DDYAEH
- {0xB5A0, 0xB5A0, prLV}, // Lo HANGUL SYLLABLE DDEO
- {0xB5A1, 0xB5BB, prLVT}, // Lo [27] HANGUL SYLLABLE DDEOG..HANGUL SYLLABLE DDEOH
- {0xB5BC, 0xB5BC, prLV}, // Lo HANGUL SYLLABLE DDE
- {0xB5BD, 0xB5D7, prLVT}, // Lo [27] HANGUL SYLLABLE DDEG..HANGUL SYLLABLE DDEH
- {0xB5D8, 0xB5D8, prLV}, // Lo HANGUL SYLLABLE DDYEO
- {0xB5D9, 0xB5F3, prLVT}, // Lo [27] HANGUL SYLLABLE DDYEOG..HANGUL SYLLABLE DDYEOH
- {0xB5F4, 0xB5F4, prLV}, // Lo HANGUL SYLLABLE DDYE
- {0xB5F5, 0xB60F, prLVT}, // Lo [27] HANGUL SYLLABLE DDYEG..HANGUL SYLLABLE DDYEH
- {0xB610, 0xB610, prLV}, // Lo HANGUL SYLLABLE DDO
- {0xB611, 0xB62B, prLVT}, // Lo [27] HANGUL SYLLABLE DDOG..HANGUL SYLLABLE DDOH
- {0xB62C, 0xB62C, prLV}, // Lo HANGUL SYLLABLE DDWA
- {0xB62D, 0xB647, prLVT}, // Lo [27] HANGUL SYLLABLE DDWAG..HANGUL SYLLABLE DDWAH
- {0xB648, 0xB648, prLV}, // Lo HANGUL SYLLABLE DDWAE
- {0xB649, 0xB663, prLVT}, // Lo [27] HANGUL SYLLABLE DDWAEG..HANGUL SYLLABLE DDWAEH
- {0xB664, 0xB664, prLV}, // Lo HANGUL SYLLABLE DDOE
- {0xB665, 0xB67F, prLVT}, // Lo [27] HANGUL SYLLABLE DDOEG..HANGUL SYLLABLE DDOEH
- {0xB680, 0xB680, prLV}, // Lo HANGUL SYLLABLE DDYO
- {0xB681, 0xB69B, prLVT}, // Lo [27] HANGUL SYLLABLE DDYOG..HANGUL SYLLABLE DDYOH
- {0xB69C, 0xB69C, prLV}, // Lo HANGUL SYLLABLE DDU
- {0xB69D, 0xB6B7, prLVT}, // Lo [27] HANGUL SYLLABLE DDUG..HANGUL SYLLABLE DDUH
- {0xB6B8, 0xB6B8, prLV}, // Lo HANGUL SYLLABLE DDWEO
- {0xB6B9, 0xB6D3, prLVT}, // Lo [27] HANGUL SYLLABLE DDWEOG..HANGUL SYLLABLE DDWEOH
- {0xB6D4, 0xB6D4, prLV}, // Lo HANGUL SYLLABLE DDWE
- {0xB6D5, 0xB6EF, prLVT}, // Lo [27] HANGUL SYLLABLE DDWEG..HANGUL SYLLABLE DDWEH
- {0xB6F0, 0xB6F0, prLV}, // Lo HANGUL SYLLABLE DDWI
- {0xB6F1, 0xB70B, prLVT}, // Lo [27] HANGUL SYLLABLE DDWIG..HANGUL SYLLABLE DDWIH
- {0xB70C, 0xB70C, prLV}, // Lo HANGUL SYLLABLE DDYU
- {0xB70D, 0xB727, prLVT}, // Lo [27] HANGUL SYLLABLE DDYUG..HANGUL SYLLABLE DDYUH
- {0xB728, 0xB728, prLV}, // Lo HANGUL SYLLABLE DDEU
- {0xB729, 0xB743, prLVT}, // Lo [27] HANGUL SYLLABLE DDEUG..HANGUL SYLLABLE DDEUH
- {0xB744, 0xB744, prLV}, // Lo HANGUL SYLLABLE DDYI
- {0xB745, 0xB75F, prLVT}, // Lo [27] HANGUL SYLLABLE DDYIG..HANGUL SYLLABLE DDYIH
- {0xB760, 0xB760, prLV}, // Lo HANGUL SYLLABLE DDI
- {0xB761, 0xB77B, prLVT}, // Lo [27] HANGUL SYLLABLE DDIG..HANGUL SYLLABLE DDIH
- {0xB77C, 0xB77C, prLV}, // Lo HANGUL SYLLABLE RA
- {0xB77D, 0xB797, prLVT}, // Lo [27] HANGUL SYLLABLE RAG..HANGUL SYLLABLE RAH
- {0xB798, 0xB798, prLV}, // Lo HANGUL SYLLABLE RAE
- {0xB799, 0xB7B3, prLVT}, // Lo [27] HANGUL SYLLABLE RAEG..HANGUL SYLLABLE RAEH
- {0xB7B4, 0xB7B4, prLV}, // Lo HANGUL SYLLABLE RYA
- {0xB7B5, 0xB7CF, prLVT}, // Lo [27] HANGUL SYLLABLE RYAG..HANGUL SYLLABLE RYAH
- {0xB7D0, 0xB7D0, prLV}, // Lo HANGUL SYLLABLE RYAE
- {0xB7D1, 0xB7EB, prLVT}, // Lo [27] HANGUL SYLLABLE RYAEG..HANGUL SYLLABLE RYAEH
- {0xB7EC, 0xB7EC, prLV}, // Lo HANGUL SYLLABLE REO
- {0xB7ED, 0xB807, prLVT}, // Lo [27] HANGUL SYLLABLE REOG..HANGUL SYLLABLE REOH
- {0xB808, 0xB808, prLV}, // Lo HANGUL SYLLABLE RE
- {0xB809, 0xB823, prLVT}, // Lo [27] HANGUL SYLLABLE REG..HANGUL SYLLABLE REH
- {0xB824, 0xB824, prLV}, // Lo HANGUL SYLLABLE RYEO
- {0xB825, 0xB83F, prLVT}, // Lo [27] HANGUL SYLLABLE RYEOG..HANGUL SYLLABLE RYEOH
- {0xB840, 0xB840, prLV}, // Lo HANGUL SYLLABLE RYE
- {0xB841, 0xB85B, prLVT}, // Lo [27] HANGUL SYLLABLE RYEG..HANGUL SYLLABLE RYEH
- {0xB85C, 0xB85C, prLV}, // Lo HANGUL SYLLABLE RO
- {0xB85D, 0xB877, prLVT}, // Lo [27] HANGUL SYLLABLE ROG..HANGUL SYLLABLE ROH
- {0xB878, 0xB878, prLV}, // Lo HANGUL SYLLABLE RWA
- {0xB879, 0xB893, prLVT}, // Lo [27] HANGUL SYLLABLE RWAG..HANGUL SYLLABLE RWAH
- {0xB894, 0xB894, prLV}, // Lo HANGUL SYLLABLE RWAE
- {0xB895, 0xB8AF, prLVT}, // Lo [27] HANGUL SYLLABLE RWAEG..HANGUL SYLLABLE RWAEH
- {0xB8B0, 0xB8B0, prLV}, // Lo HANGUL SYLLABLE ROE
- {0xB8B1, 0xB8CB, prLVT}, // Lo [27] HANGUL SYLLABLE ROEG..HANGUL SYLLABLE ROEH
- {0xB8CC, 0xB8CC, prLV}, // Lo HANGUL SYLLABLE RYO
- {0xB8CD, 0xB8E7, prLVT}, // Lo [27] HANGUL SYLLABLE RYOG..HANGUL SYLLABLE RYOH
- {0xB8E8, 0xB8E8, prLV}, // Lo HANGUL SYLLABLE RU
- {0xB8E9, 0xB903, prLVT}, // Lo [27] HANGUL SYLLABLE RUG..HANGUL SYLLABLE RUH
- {0xB904, 0xB904, prLV}, // Lo HANGUL SYLLABLE RWEO
- {0xB905, 0xB91F, prLVT}, // Lo [27] HANGUL SYLLABLE RWEOG..HANGUL SYLLABLE RWEOH
- {0xB920, 0xB920, prLV}, // Lo HANGUL SYLLABLE RWE
- {0xB921, 0xB93B, prLVT}, // Lo [27] HANGUL SYLLABLE RWEG..HANGUL SYLLABLE RWEH
- {0xB93C, 0xB93C, prLV}, // Lo HANGUL SYLLABLE RWI
- {0xB93D, 0xB957, prLVT}, // Lo [27] HANGUL SYLLABLE RWIG..HANGUL SYLLABLE RWIH
- {0xB958, 0xB958, prLV}, // Lo HANGUL SYLLABLE RYU
- {0xB959, 0xB973, prLVT}, // Lo [27] HANGUL SYLLABLE RYUG..HANGUL SYLLABLE RYUH
- {0xB974, 0xB974, prLV}, // Lo HANGUL SYLLABLE REU
- {0xB975, 0xB98F, prLVT}, // Lo [27] HANGUL SYLLABLE REUG..HANGUL SYLLABLE REUH
- {0xB990, 0xB990, prLV}, // Lo HANGUL SYLLABLE RYI
- {0xB991, 0xB9AB, prLVT}, // Lo [27] HANGUL SYLLABLE RYIG..HANGUL SYLLABLE RYIH
- {0xB9AC, 0xB9AC, prLV}, // Lo HANGUL SYLLABLE RI
- {0xB9AD, 0xB9C7, prLVT}, // Lo [27] HANGUL SYLLABLE RIG..HANGUL SYLLABLE RIH
- {0xB9C8, 0xB9C8, prLV}, // Lo HANGUL SYLLABLE MA
- {0xB9C9, 0xB9E3, prLVT}, // Lo [27] HANGUL SYLLABLE MAG..HANGUL SYLLABLE MAH
- {0xB9E4, 0xB9E4, prLV}, // Lo HANGUL SYLLABLE MAE
- {0xB9E5, 0xB9FF, prLVT}, // Lo [27] HANGUL SYLLABLE MAEG..HANGUL SYLLABLE MAEH
- {0xBA00, 0xBA00, prLV}, // Lo HANGUL SYLLABLE MYA
- {0xBA01, 0xBA1B, prLVT}, // Lo [27] HANGUL SYLLABLE MYAG..HANGUL SYLLABLE MYAH
- {0xBA1C, 0xBA1C, prLV}, // Lo HANGUL SYLLABLE MYAE
- {0xBA1D, 0xBA37, prLVT}, // Lo [27] HANGUL SYLLABLE MYAEG..HANGUL SYLLABLE MYAEH
- {0xBA38, 0xBA38, prLV}, // Lo HANGUL SYLLABLE MEO
- {0xBA39, 0xBA53, prLVT}, // Lo [27] HANGUL SYLLABLE MEOG..HANGUL SYLLABLE MEOH
- {0xBA54, 0xBA54, prLV}, // Lo HANGUL SYLLABLE ME
- {0xBA55, 0xBA6F, prLVT}, // Lo [27] HANGUL SYLLABLE MEG..HANGUL SYLLABLE MEH
- {0xBA70, 0xBA70, prLV}, // Lo HANGUL SYLLABLE MYEO
- {0xBA71, 0xBA8B, prLVT}, // Lo [27] HANGUL SYLLABLE MYEOG..HANGUL SYLLABLE MYEOH
- {0xBA8C, 0xBA8C, prLV}, // Lo HANGUL SYLLABLE MYE
- {0xBA8D, 0xBAA7, prLVT}, // Lo [27] HANGUL SYLLABLE MYEG..HANGUL SYLLABLE MYEH
- {0xBAA8, 0xBAA8, prLV}, // Lo HANGUL SYLLABLE MO
- {0xBAA9, 0xBAC3, prLVT}, // Lo [27] HANGUL SYLLABLE MOG..HANGUL SYLLABLE MOH
- {0xBAC4, 0xBAC4, prLV}, // Lo HANGUL SYLLABLE MWA
- {0xBAC5, 0xBADF, prLVT}, // Lo [27] HANGUL SYLLABLE MWAG..HANGUL SYLLABLE MWAH
- {0xBAE0, 0xBAE0, prLV}, // Lo HANGUL SYLLABLE MWAE
- {0xBAE1, 0xBAFB, prLVT}, // Lo [27] HANGUL SYLLABLE MWAEG..HANGUL SYLLABLE MWAEH
- {0xBAFC, 0xBAFC, prLV}, // Lo HANGUL SYLLABLE MOE
- {0xBAFD, 0xBB17, prLVT}, // Lo [27] HANGUL SYLLABLE MOEG..HANGUL SYLLABLE MOEH
- {0xBB18, 0xBB18, prLV}, // Lo HANGUL SYLLABLE MYO
- {0xBB19, 0xBB33, prLVT}, // Lo [27] HANGUL SYLLABLE MYOG..HANGUL SYLLABLE MYOH
- {0xBB34, 0xBB34, prLV}, // Lo HANGUL SYLLABLE MU
- {0xBB35, 0xBB4F, prLVT}, // Lo [27] HANGUL SYLLABLE MUG..HANGUL SYLLABLE MUH
- {0xBB50, 0xBB50, prLV}, // Lo HANGUL SYLLABLE MWEO
- {0xBB51, 0xBB6B, prLVT}, // Lo [27] HANGUL SYLLABLE MWEOG..HANGUL SYLLABLE MWEOH
- {0xBB6C, 0xBB6C, prLV}, // Lo HANGUL SYLLABLE MWE
- {0xBB6D, 0xBB87, prLVT}, // Lo [27] HANGUL SYLLABLE MWEG..HANGUL SYLLABLE MWEH
- {0xBB88, 0xBB88, prLV}, // Lo HANGUL SYLLABLE MWI
- {0xBB89, 0xBBA3, prLVT}, // Lo [27] HANGUL SYLLABLE MWIG..HANGUL SYLLABLE MWIH
- {0xBBA4, 0xBBA4, prLV}, // Lo HANGUL SYLLABLE MYU
- {0xBBA5, 0xBBBF, prLVT}, // Lo [27] HANGUL SYLLABLE MYUG..HANGUL SYLLABLE MYUH
- {0xBBC0, 0xBBC0, prLV}, // Lo HANGUL SYLLABLE MEU
- {0xBBC1, 0xBBDB, prLVT}, // Lo [27] HANGUL SYLLABLE MEUG..HANGUL SYLLABLE MEUH
- {0xBBDC, 0xBBDC, prLV}, // Lo HANGUL SYLLABLE MYI
- {0xBBDD, 0xBBF7, prLVT}, // Lo [27] HANGUL SYLLABLE MYIG..HANGUL SYLLABLE MYIH
- {0xBBF8, 0xBBF8, prLV}, // Lo HANGUL SYLLABLE MI
- {0xBBF9, 0xBC13, prLVT}, // Lo [27] HANGUL SYLLABLE MIG..HANGUL SYLLABLE MIH
- {0xBC14, 0xBC14, prLV}, // Lo HANGUL SYLLABLE BA
- {0xBC15, 0xBC2F, prLVT}, // Lo [27] HANGUL SYLLABLE BAG..HANGUL SYLLABLE BAH
- {0xBC30, 0xBC30, prLV}, // Lo HANGUL SYLLABLE BAE
- {0xBC31, 0xBC4B, prLVT}, // Lo [27] HANGUL SYLLABLE BAEG..HANGUL SYLLABLE BAEH
- {0xBC4C, 0xBC4C, prLV}, // Lo HANGUL SYLLABLE BYA
- {0xBC4D, 0xBC67, prLVT}, // Lo [27] HANGUL SYLLABLE BYAG..HANGUL SYLLABLE BYAH
- {0xBC68, 0xBC68, prLV}, // Lo HANGUL SYLLABLE BYAE
- {0xBC69, 0xBC83, prLVT}, // Lo [27] HANGUL SYLLABLE BYAEG..HANGUL SYLLABLE BYAEH
- {0xBC84, 0xBC84, prLV}, // Lo HANGUL SYLLABLE BEO
- {0xBC85, 0xBC9F, prLVT}, // Lo [27] HANGUL SYLLABLE BEOG..HANGUL SYLLABLE BEOH
- {0xBCA0, 0xBCA0, prLV}, // Lo HANGUL SYLLABLE BE
- {0xBCA1, 0xBCBB, prLVT}, // Lo [27] HANGUL SYLLABLE BEG..HANGUL SYLLABLE BEH
- {0xBCBC, 0xBCBC, prLV}, // Lo HANGUL SYLLABLE BYEO
- {0xBCBD, 0xBCD7, prLVT}, // Lo [27] HANGUL SYLLABLE BYEOG..HANGUL SYLLABLE BYEOH
- {0xBCD8, 0xBCD8, prLV}, // Lo HANGUL SYLLABLE BYE
- {0xBCD9, 0xBCF3, prLVT}, // Lo [27] HANGUL SYLLABLE BYEG..HANGUL SYLLABLE BYEH
- {0xBCF4, 0xBCF4, prLV}, // Lo HANGUL SYLLABLE BO
- {0xBCF5, 0xBD0F, prLVT}, // Lo [27] HANGUL SYLLABLE BOG..HANGUL SYLLABLE BOH
- {0xBD10, 0xBD10, prLV}, // Lo HANGUL SYLLABLE BWA
- {0xBD11, 0xBD2B, prLVT}, // Lo [27] HANGUL SYLLABLE BWAG..HANGUL SYLLABLE BWAH
- {0xBD2C, 0xBD2C, prLV}, // Lo HANGUL SYLLABLE BWAE
- {0xBD2D, 0xBD47, prLVT}, // Lo [27] HANGUL SYLLABLE BWAEG..HANGUL SYLLABLE BWAEH
- {0xBD48, 0xBD48, prLV}, // Lo HANGUL SYLLABLE BOE
- {0xBD49, 0xBD63, prLVT}, // Lo [27] HANGUL SYLLABLE BOEG..HANGUL SYLLABLE BOEH
- {0xBD64, 0xBD64, prLV}, // Lo HANGUL SYLLABLE BYO
- {0xBD65, 0xBD7F, prLVT}, // Lo [27] HANGUL SYLLABLE BYOG..HANGUL SYLLABLE BYOH
- {0xBD80, 0xBD80, prLV}, // Lo HANGUL SYLLABLE BU
- {0xBD81, 0xBD9B, prLVT}, // Lo [27] HANGUL SYLLABLE BUG..HANGUL SYLLABLE BUH
- {0xBD9C, 0xBD9C, prLV}, // Lo HANGUL SYLLABLE BWEO
- {0xBD9D, 0xBDB7, prLVT}, // Lo [27] HANGUL SYLLABLE BWEOG..HANGUL SYLLABLE BWEOH
- {0xBDB8, 0xBDB8, prLV}, // Lo HANGUL SYLLABLE BWE
- {0xBDB9, 0xBDD3, prLVT}, // Lo [27] HANGUL SYLLABLE BWEG..HANGUL SYLLABLE BWEH
- {0xBDD4, 0xBDD4, prLV}, // Lo HANGUL SYLLABLE BWI
- {0xBDD5, 0xBDEF, prLVT}, // Lo [27] HANGUL SYLLABLE BWIG..HANGUL SYLLABLE BWIH
- {0xBDF0, 0xBDF0, prLV}, // Lo HANGUL SYLLABLE BYU
- {0xBDF1, 0xBE0B, prLVT}, // Lo [27] HANGUL SYLLABLE BYUG..HANGUL SYLLABLE BYUH
- {0xBE0C, 0xBE0C, prLV}, // Lo HANGUL SYLLABLE BEU
- {0xBE0D, 0xBE27, prLVT}, // Lo [27] HANGUL SYLLABLE BEUG..HANGUL SYLLABLE BEUH
- {0xBE28, 0xBE28, prLV}, // Lo HANGUL SYLLABLE BYI
- {0xBE29, 0xBE43, prLVT}, // Lo [27] HANGUL SYLLABLE BYIG..HANGUL SYLLABLE BYIH
- {0xBE44, 0xBE44, prLV}, // Lo HANGUL SYLLABLE BI
- {0xBE45, 0xBE5F, prLVT}, // Lo [27] HANGUL SYLLABLE BIG..HANGUL SYLLABLE BIH
- {0xBE60, 0xBE60, prLV}, // Lo HANGUL SYLLABLE BBA
- {0xBE61, 0xBE7B, prLVT}, // Lo [27] HANGUL SYLLABLE BBAG..HANGUL SYLLABLE BBAH
- {0xBE7C, 0xBE7C, prLV}, // Lo HANGUL SYLLABLE BBAE
- {0xBE7D, 0xBE97, prLVT}, // Lo [27] HANGUL SYLLABLE BBAEG..HANGUL SYLLABLE BBAEH
- {0xBE98, 0xBE98, prLV}, // Lo HANGUL SYLLABLE BBYA
- {0xBE99, 0xBEB3, prLVT}, // Lo [27] HANGUL SYLLABLE BBYAG..HANGUL SYLLABLE BBYAH
- {0xBEB4, 0xBEB4, prLV}, // Lo HANGUL SYLLABLE BBYAE
- {0xBEB5, 0xBECF, prLVT}, // Lo [27] HANGUL SYLLABLE BBYAEG..HANGUL SYLLABLE BBYAEH
- {0xBED0, 0xBED0, prLV}, // Lo HANGUL SYLLABLE BBEO
- {0xBED1, 0xBEEB, prLVT}, // Lo [27] HANGUL SYLLABLE BBEOG..HANGUL SYLLABLE BBEOH
- {0xBEEC, 0xBEEC, prLV}, // Lo HANGUL SYLLABLE BBE
- {0xBEED, 0xBF07, prLVT}, // Lo [27] HANGUL SYLLABLE BBEG..HANGUL SYLLABLE BBEH
- {0xBF08, 0xBF08, prLV}, // Lo HANGUL SYLLABLE BBYEO
- {0xBF09, 0xBF23, prLVT}, // Lo [27] HANGUL SYLLABLE BBYEOG..HANGUL SYLLABLE BBYEOH
- {0xBF24, 0xBF24, prLV}, // Lo HANGUL SYLLABLE BBYE
- {0xBF25, 0xBF3F, prLVT}, // Lo [27] HANGUL SYLLABLE BBYEG..HANGUL SYLLABLE BBYEH
- {0xBF40, 0xBF40, prLV}, // Lo HANGUL SYLLABLE BBO
- {0xBF41, 0xBF5B, prLVT}, // Lo [27] HANGUL SYLLABLE BBOG..HANGUL SYLLABLE BBOH
- {0xBF5C, 0xBF5C, prLV}, // Lo HANGUL SYLLABLE BBWA
- {0xBF5D, 0xBF77, prLVT}, // Lo [27] HANGUL SYLLABLE BBWAG..HANGUL SYLLABLE BBWAH
- {0xBF78, 0xBF78, prLV}, // Lo HANGUL SYLLABLE BBWAE
- {0xBF79, 0xBF93, prLVT}, // Lo [27] HANGUL SYLLABLE BBWAEG..HANGUL SYLLABLE BBWAEH
- {0xBF94, 0xBF94, prLV}, // Lo HANGUL SYLLABLE BBOE
- {0xBF95, 0xBFAF, prLVT}, // Lo [27] HANGUL SYLLABLE BBOEG..HANGUL SYLLABLE BBOEH
- {0xBFB0, 0xBFB0, prLV}, // Lo HANGUL SYLLABLE BBYO
- {0xBFB1, 0xBFCB, prLVT}, // Lo [27] HANGUL SYLLABLE BBYOG..HANGUL SYLLABLE BBYOH
- {0xBFCC, 0xBFCC, prLV}, // Lo HANGUL SYLLABLE BBU
- {0xBFCD, 0xBFE7, prLVT}, // Lo [27] HANGUL SYLLABLE BBUG..HANGUL SYLLABLE BBUH
- {0xBFE8, 0xBFE8, prLV}, // Lo HANGUL SYLLABLE BBWEO
- {0xBFE9, 0xC003, prLVT}, // Lo [27] HANGUL SYLLABLE BBWEOG..HANGUL SYLLABLE BBWEOH
- {0xC004, 0xC004, prLV}, // Lo HANGUL SYLLABLE BBWE
- {0xC005, 0xC01F, prLVT}, // Lo [27] HANGUL SYLLABLE BBWEG..HANGUL SYLLABLE BBWEH
- {0xC020, 0xC020, prLV}, // Lo HANGUL SYLLABLE BBWI
- {0xC021, 0xC03B, prLVT}, // Lo [27] HANGUL SYLLABLE BBWIG..HANGUL SYLLABLE BBWIH
- {0xC03C, 0xC03C, prLV}, // Lo HANGUL SYLLABLE BBYU
- {0xC03D, 0xC057, prLVT}, // Lo [27] HANGUL SYLLABLE BBYUG..HANGUL SYLLABLE BBYUH
- {0xC058, 0xC058, prLV}, // Lo HANGUL SYLLABLE BBEU
- {0xC059, 0xC073, prLVT}, // Lo [27] HANGUL SYLLABLE BBEUG..HANGUL SYLLABLE BBEUH
- {0xC074, 0xC074, prLV}, // Lo HANGUL SYLLABLE BBYI
- {0xC075, 0xC08F, prLVT}, // Lo [27] HANGUL SYLLABLE BBYIG..HANGUL SYLLABLE BBYIH
- {0xC090, 0xC090, prLV}, // Lo HANGUL SYLLABLE BBI
- {0xC091, 0xC0AB, prLVT}, // Lo [27] HANGUL SYLLABLE BBIG..HANGUL SYLLABLE BBIH
- {0xC0AC, 0xC0AC, prLV}, // Lo HANGUL SYLLABLE SA
- {0xC0AD, 0xC0C7, prLVT}, // Lo [27] HANGUL SYLLABLE SAG..HANGUL SYLLABLE SAH
- {0xC0C8, 0xC0C8, prLV}, // Lo HANGUL SYLLABLE SAE
- {0xC0C9, 0xC0E3, prLVT}, // Lo [27] HANGUL SYLLABLE SAEG..HANGUL SYLLABLE SAEH
- {0xC0E4, 0xC0E4, prLV}, // Lo HANGUL SYLLABLE SYA
- {0xC0E5, 0xC0FF, prLVT}, // Lo [27] HANGUL SYLLABLE SYAG..HANGUL SYLLABLE SYAH
- {0xC100, 0xC100, prLV}, // Lo HANGUL SYLLABLE SYAE
- {0xC101, 0xC11B, prLVT}, // Lo [27] HANGUL SYLLABLE SYAEG..HANGUL SYLLABLE SYAEH
- {0xC11C, 0xC11C, prLV}, // Lo HANGUL SYLLABLE SEO
- {0xC11D, 0xC137, prLVT}, // Lo [27] HANGUL SYLLABLE SEOG..HANGUL SYLLABLE SEOH
- {0xC138, 0xC138, prLV}, // Lo HANGUL SYLLABLE SE
- {0xC139, 0xC153, prLVT}, // Lo [27] HANGUL SYLLABLE SEG..HANGUL SYLLABLE SEH
- {0xC154, 0xC154, prLV}, // Lo HANGUL SYLLABLE SYEO
- {0xC155, 0xC16F, prLVT}, // Lo [27] HANGUL SYLLABLE SYEOG..HANGUL SYLLABLE SYEOH
- {0xC170, 0xC170, prLV}, // Lo HANGUL SYLLABLE SYE
- {0xC171, 0xC18B, prLVT}, // Lo [27] HANGUL SYLLABLE SYEG..HANGUL SYLLABLE SYEH
- {0xC18C, 0xC18C, prLV}, // Lo HANGUL SYLLABLE SO
- {0xC18D, 0xC1A7, prLVT}, // Lo [27] HANGUL SYLLABLE SOG..HANGUL SYLLABLE SOH
- {0xC1A8, 0xC1A8, prLV}, // Lo HANGUL SYLLABLE SWA
- {0xC1A9, 0xC1C3, prLVT}, // Lo [27] HANGUL SYLLABLE SWAG..HANGUL SYLLABLE SWAH
- {0xC1C4, 0xC1C4, prLV}, // Lo HANGUL SYLLABLE SWAE
- {0xC1C5, 0xC1DF, prLVT}, // Lo [27] HANGUL SYLLABLE SWAEG..HANGUL SYLLABLE SWAEH
- {0xC1E0, 0xC1E0, prLV}, // Lo HANGUL SYLLABLE SOE
- {0xC1E1, 0xC1FB, prLVT}, // Lo [27] HANGUL SYLLABLE SOEG..HANGUL SYLLABLE SOEH
- {0xC1FC, 0xC1FC, prLV}, // Lo HANGUL SYLLABLE SYO
- {0xC1FD, 0xC217, prLVT}, // Lo [27] HANGUL SYLLABLE SYOG..HANGUL SYLLABLE SYOH
- {0xC218, 0xC218, prLV}, // Lo HANGUL SYLLABLE SU
- {0xC219, 0xC233, prLVT}, // Lo [27] HANGUL SYLLABLE SUG..HANGUL SYLLABLE SUH
- {0xC234, 0xC234, prLV}, // Lo HANGUL SYLLABLE SWEO
- {0xC235, 0xC24F, prLVT}, // Lo [27] HANGUL SYLLABLE SWEOG..HANGUL SYLLABLE SWEOH
- {0xC250, 0xC250, prLV}, // Lo HANGUL SYLLABLE SWE
- {0xC251, 0xC26B, prLVT}, // Lo [27] HANGUL SYLLABLE SWEG..HANGUL SYLLABLE SWEH
- {0xC26C, 0xC26C, prLV}, // Lo HANGUL SYLLABLE SWI
- {0xC26D, 0xC287, prLVT}, // Lo [27] HANGUL SYLLABLE SWIG..HANGUL SYLLABLE SWIH
- {0xC288, 0xC288, prLV}, // Lo HANGUL SYLLABLE SYU
- {0xC289, 0xC2A3, prLVT}, // Lo [27] HANGUL SYLLABLE SYUG..HANGUL SYLLABLE SYUH
- {0xC2A4, 0xC2A4, prLV}, // Lo HANGUL SYLLABLE SEU
- {0xC2A5, 0xC2BF, prLVT}, // Lo [27] HANGUL SYLLABLE SEUG..HANGUL SYLLABLE SEUH
- {0xC2C0, 0xC2C0, prLV}, // Lo HANGUL SYLLABLE SYI
- {0xC2C1, 0xC2DB, prLVT}, // Lo [27] HANGUL SYLLABLE SYIG..HANGUL SYLLABLE SYIH
- {0xC2DC, 0xC2DC, prLV}, // Lo HANGUL SYLLABLE SI
- {0xC2DD, 0xC2F7, prLVT}, // Lo [27] HANGUL SYLLABLE SIG..HANGUL SYLLABLE SIH
- {0xC2F8, 0xC2F8, prLV}, // Lo HANGUL SYLLABLE SSA
- {0xC2F9, 0xC313, prLVT}, // Lo [27] HANGUL SYLLABLE SSAG..HANGUL SYLLABLE SSAH
- {0xC314, 0xC314, prLV}, // Lo HANGUL SYLLABLE SSAE
- {0xC315, 0xC32F, prLVT}, // Lo [27] HANGUL SYLLABLE SSAEG..HANGUL SYLLABLE SSAEH
- {0xC330, 0xC330, prLV}, // Lo HANGUL SYLLABLE SSYA
- {0xC331, 0xC34B, prLVT}, // Lo [27] HANGUL SYLLABLE SSYAG..HANGUL SYLLABLE SSYAH
- {0xC34C, 0xC34C, prLV}, // Lo HANGUL SYLLABLE SSYAE
- {0xC34D, 0xC367, prLVT}, // Lo [27] HANGUL SYLLABLE SSYAEG..HANGUL SYLLABLE SSYAEH
- {0xC368, 0xC368, prLV}, // Lo HANGUL SYLLABLE SSEO
- {0xC369, 0xC383, prLVT}, // Lo [27] HANGUL SYLLABLE SSEOG..HANGUL SYLLABLE SSEOH
- {0xC384, 0xC384, prLV}, // Lo HANGUL SYLLABLE SSE
- {0xC385, 0xC39F, prLVT}, // Lo [27] HANGUL SYLLABLE SSEG..HANGUL SYLLABLE SSEH
- {0xC3A0, 0xC3A0, prLV}, // Lo HANGUL SYLLABLE SSYEO
- {0xC3A1, 0xC3BB, prLVT}, // Lo [27] HANGUL SYLLABLE SSYEOG..HANGUL SYLLABLE SSYEOH
- {0xC3BC, 0xC3BC, prLV}, // Lo HANGUL SYLLABLE SSYE
- {0xC3BD, 0xC3D7, prLVT}, // Lo [27] HANGUL SYLLABLE SSYEG..HANGUL SYLLABLE SSYEH
- {0xC3D8, 0xC3D8, prLV}, // Lo HANGUL SYLLABLE SSO
- {0xC3D9, 0xC3F3, prLVT}, // Lo [27] HANGUL SYLLABLE SSOG..HANGUL SYLLABLE SSOH
- {0xC3F4, 0xC3F4, prLV}, // Lo HANGUL SYLLABLE SSWA
- {0xC3F5, 0xC40F, prLVT}, // Lo [27] HANGUL SYLLABLE SSWAG..HANGUL SYLLABLE SSWAH
- {0xC410, 0xC410, prLV}, // Lo HANGUL SYLLABLE SSWAE
- {0xC411, 0xC42B, prLVT}, // Lo [27] HANGUL SYLLABLE SSWAEG..HANGUL SYLLABLE SSWAEH
- {0xC42C, 0xC42C, prLV}, // Lo HANGUL SYLLABLE SSOE
- {0xC42D, 0xC447, prLVT}, // Lo [27] HANGUL SYLLABLE SSOEG..HANGUL SYLLABLE SSOEH
- {0xC448, 0xC448, prLV}, // Lo HANGUL SYLLABLE SSYO
- {0xC449, 0xC463, prLVT}, // Lo [27] HANGUL SYLLABLE SSYOG..HANGUL SYLLABLE SSYOH
- {0xC464, 0xC464, prLV}, // Lo HANGUL SYLLABLE SSU
- {0xC465, 0xC47F, prLVT}, // Lo [27] HANGUL SYLLABLE SSUG..HANGUL SYLLABLE SSUH
- {0xC480, 0xC480, prLV}, // Lo HANGUL SYLLABLE SSWEO
- {0xC481, 0xC49B, prLVT}, // Lo [27] HANGUL SYLLABLE SSWEOG..HANGUL SYLLABLE SSWEOH
- {0xC49C, 0xC49C, prLV}, // Lo HANGUL SYLLABLE SSWE
- {0xC49D, 0xC4B7, prLVT}, // Lo [27] HANGUL SYLLABLE SSWEG..HANGUL SYLLABLE SSWEH
- {0xC4B8, 0xC4B8, prLV}, // Lo HANGUL SYLLABLE SSWI
- {0xC4B9, 0xC4D3, prLVT}, // Lo [27] HANGUL SYLLABLE SSWIG..HANGUL SYLLABLE SSWIH
- {0xC4D4, 0xC4D4, prLV}, // Lo HANGUL SYLLABLE SSYU
- {0xC4D5, 0xC4EF, prLVT}, // Lo [27] HANGUL SYLLABLE SSYUG..HANGUL SYLLABLE SSYUH
- {0xC4F0, 0xC4F0, prLV}, // Lo HANGUL SYLLABLE SSEU
- {0xC4F1, 0xC50B, prLVT}, // Lo [27] HANGUL SYLLABLE SSEUG..HANGUL SYLLABLE SSEUH
- {0xC50C, 0xC50C, prLV}, // Lo HANGUL SYLLABLE SSYI
- {0xC50D, 0xC527, prLVT}, // Lo [27] HANGUL SYLLABLE SSYIG..HANGUL SYLLABLE SSYIH
- {0xC528, 0xC528, prLV}, // Lo HANGUL SYLLABLE SSI
- {0xC529, 0xC543, prLVT}, // Lo [27] HANGUL SYLLABLE SSIG..HANGUL SYLLABLE SSIH
- {0xC544, 0xC544, prLV}, // Lo HANGUL SYLLABLE A
- {0xC545, 0xC55F, prLVT}, // Lo [27] HANGUL SYLLABLE AG..HANGUL SYLLABLE AH
- {0xC560, 0xC560, prLV}, // Lo HANGUL SYLLABLE AE
- {0xC561, 0xC57B, prLVT}, // Lo [27] HANGUL SYLLABLE AEG..HANGUL SYLLABLE AEH
- {0xC57C, 0xC57C, prLV}, // Lo HANGUL SYLLABLE YA
- {0xC57D, 0xC597, prLVT}, // Lo [27] HANGUL SYLLABLE YAG..HANGUL SYLLABLE YAH
- {0xC598, 0xC598, prLV}, // Lo HANGUL SYLLABLE YAE
- {0xC599, 0xC5B3, prLVT}, // Lo [27] HANGUL SYLLABLE YAEG..HANGUL SYLLABLE YAEH
- {0xC5B4, 0xC5B4, prLV}, // Lo HANGUL SYLLABLE EO
- {0xC5B5, 0xC5CF, prLVT}, // Lo [27] HANGUL SYLLABLE EOG..HANGUL SYLLABLE EOH
- {0xC5D0, 0xC5D0, prLV}, // Lo HANGUL SYLLABLE E
- {0xC5D1, 0xC5EB, prLVT}, // Lo [27] HANGUL SYLLABLE EG..HANGUL SYLLABLE EH
- {0xC5EC, 0xC5EC, prLV}, // Lo HANGUL SYLLABLE YEO
- {0xC5ED, 0xC607, prLVT}, // Lo [27] HANGUL SYLLABLE YEOG..HANGUL SYLLABLE YEOH
- {0xC608, 0xC608, prLV}, // Lo HANGUL SYLLABLE YE
- {0xC609, 0xC623, prLVT}, // Lo [27] HANGUL SYLLABLE YEG..HANGUL SYLLABLE YEH
- {0xC624, 0xC624, prLV}, // Lo HANGUL SYLLABLE O
- {0xC625, 0xC63F, prLVT}, // Lo [27] HANGUL SYLLABLE OG..HANGUL SYLLABLE OH
- {0xC640, 0xC640, prLV}, // Lo HANGUL SYLLABLE WA
- {0xC641, 0xC65B, prLVT}, // Lo [27] HANGUL SYLLABLE WAG..HANGUL SYLLABLE WAH
- {0xC65C, 0xC65C, prLV}, // Lo HANGUL SYLLABLE WAE
- {0xC65D, 0xC677, prLVT}, // Lo [27] HANGUL SYLLABLE WAEG..HANGUL SYLLABLE WAEH
- {0xC678, 0xC678, prLV}, // Lo HANGUL SYLLABLE OE
- {0xC679, 0xC693, prLVT}, // Lo [27] HANGUL SYLLABLE OEG..HANGUL SYLLABLE OEH
- {0xC694, 0xC694, prLV}, // Lo HANGUL SYLLABLE YO
- {0xC695, 0xC6AF, prLVT}, // Lo [27] HANGUL SYLLABLE YOG..HANGUL SYLLABLE YOH
- {0xC6B0, 0xC6B0, prLV}, // Lo HANGUL SYLLABLE U
- {0xC6B1, 0xC6CB, prLVT}, // Lo [27] HANGUL SYLLABLE UG..HANGUL SYLLABLE UH
- {0xC6CC, 0xC6CC, prLV}, // Lo HANGUL SYLLABLE WEO
- {0xC6CD, 0xC6E7, prLVT}, // Lo [27] HANGUL SYLLABLE WEOG..HANGUL SYLLABLE WEOH
- {0xC6E8, 0xC6E8, prLV}, // Lo HANGUL SYLLABLE WE
- {0xC6E9, 0xC703, prLVT}, // Lo [27] HANGUL SYLLABLE WEG..HANGUL SYLLABLE WEH
- {0xC704, 0xC704, prLV}, // Lo HANGUL SYLLABLE WI
- {0xC705, 0xC71F, prLVT}, // Lo [27] HANGUL SYLLABLE WIG..HANGUL SYLLABLE WIH
- {0xC720, 0xC720, prLV}, // Lo HANGUL SYLLABLE YU
- {0xC721, 0xC73B, prLVT}, // Lo [27] HANGUL SYLLABLE YUG..HANGUL SYLLABLE YUH
- {0xC73C, 0xC73C, prLV}, // Lo HANGUL SYLLABLE EU
- {0xC73D, 0xC757, prLVT}, // Lo [27] HANGUL SYLLABLE EUG..HANGUL SYLLABLE EUH
- {0xC758, 0xC758, prLV}, // Lo HANGUL SYLLABLE YI
- {0xC759, 0xC773, prLVT}, // Lo [27] HANGUL SYLLABLE YIG..HANGUL SYLLABLE YIH
- {0xC774, 0xC774, prLV}, // Lo HANGUL SYLLABLE I
- {0xC775, 0xC78F, prLVT}, // Lo [27] HANGUL SYLLABLE IG..HANGUL SYLLABLE IH
- {0xC790, 0xC790, prLV}, // Lo HANGUL SYLLABLE JA
- {0xC791, 0xC7AB, prLVT}, // Lo [27] HANGUL SYLLABLE JAG..HANGUL SYLLABLE JAH
- {0xC7AC, 0xC7AC, prLV}, // Lo HANGUL SYLLABLE JAE
- {0xC7AD, 0xC7C7, prLVT}, // Lo [27] HANGUL SYLLABLE JAEG..HANGUL SYLLABLE JAEH
- {0xC7C8, 0xC7C8, prLV}, // Lo HANGUL SYLLABLE JYA
- {0xC7C9, 0xC7E3, prLVT}, // Lo [27] HANGUL SYLLABLE JYAG..HANGUL SYLLABLE JYAH
- {0xC7E4, 0xC7E4, prLV}, // Lo HANGUL SYLLABLE JYAE
- {0xC7E5, 0xC7FF, prLVT}, // Lo [27] HANGUL SYLLABLE JYAEG..HANGUL SYLLABLE JYAEH
- {0xC800, 0xC800, prLV}, // Lo HANGUL SYLLABLE JEO
- {0xC801, 0xC81B, prLVT}, // Lo [27] HANGUL SYLLABLE JEOG..HANGUL SYLLABLE JEOH
- {0xC81C, 0xC81C, prLV}, // Lo HANGUL SYLLABLE JE
- {0xC81D, 0xC837, prLVT}, // Lo [27] HANGUL SYLLABLE JEG..HANGUL SYLLABLE JEH
- {0xC838, 0xC838, prLV}, // Lo HANGUL SYLLABLE JYEO
- {0xC839, 0xC853, prLVT}, // Lo [27] HANGUL SYLLABLE JYEOG..HANGUL SYLLABLE JYEOH
- {0xC854, 0xC854, prLV}, // Lo HANGUL SYLLABLE JYE
- {0xC855, 0xC86F, prLVT}, // Lo [27] HANGUL SYLLABLE JYEG..HANGUL SYLLABLE JYEH
- {0xC870, 0xC870, prLV}, // Lo HANGUL SYLLABLE JO
- {0xC871, 0xC88B, prLVT}, // Lo [27] HANGUL SYLLABLE JOG..HANGUL SYLLABLE JOH
- {0xC88C, 0xC88C, prLV}, // Lo HANGUL SYLLABLE JWA
- {0xC88D, 0xC8A7, prLVT}, // Lo [27] HANGUL SYLLABLE JWAG..HANGUL SYLLABLE JWAH
- {0xC8A8, 0xC8A8, prLV}, // Lo HANGUL SYLLABLE JWAE
- {0xC8A9, 0xC8C3, prLVT}, // Lo [27] HANGUL SYLLABLE JWAEG..HANGUL SYLLABLE JWAEH
- {0xC8C4, 0xC8C4, prLV}, // Lo HANGUL SYLLABLE JOE
- {0xC8C5, 0xC8DF, prLVT}, // Lo [27] HANGUL SYLLABLE JOEG..HANGUL SYLLABLE JOEH
- {0xC8E0, 0xC8E0, prLV}, // Lo HANGUL SYLLABLE JYO
- {0xC8E1, 0xC8FB, prLVT}, // Lo [27] HANGUL SYLLABLE JYOG..HANGUL SYLLABLE JYOH
- {0xC8FC, 0xC8FC, prLV}, // Lo HANGUL SYLLABLE JU
- {0xC8FD, 0xC917, prLVT}, // Lo [27] HANGUL SYLLABLE JUG..HANGUL SYLLABLE JUH
- {0xC918, 0xC918, prLV}, // Lo HANGUL SYLLABLE JWEO
- {0xC919, 0xC933, prLVT}, // Lo [27] HANGUL SYLLABLE JWEOG..HANGUL SYLLABLE JWEOH
- {0xC934, 0xC934, prLV}, // Lo HANGUL SYLLABLE JWE
- {0xC935, 0xC94F, prLVT}, // Lo [27] HANGUL SYLLABLE JWEG..HANGUL SYLLABLE JWEH
- {0xC950, 0xC950, prLV}, // Lo HANGUL SYLLABLE JWI
- {0xC951, 0xC96B, prLVT}, // Lo [27] HANGUL SYLLABLE JWIG..HANGUL SYLLABLE JWIH
- {0xC96C, 0xC96C, prLV}, // Lo HANGUL SYLLABLE JYU
- {0xC96D, 0xC987, prLVT}, // Lo [27] HANGUL SYLLABLE JYUG..HANGUL SYLLABLE JYUH
- {0xC988, 0xC988, prLV}, // Lo HANGUL SYLLABLE JEU
- {0xC989, 0xC9A3, prLVT}, // Lo [27] HANGUL SYLLABLE JEUG..HANGUL SYLLABLE JEUH
- {0xC9A4, 0xC9A4, prLV}, // Lo HANGUL SYLLABLE JYI
- {0xC9A5, 0xC9BF, prLVT}, // Lo [27] HANGUL SYLLABLE JYIG..HANGUL SYLLABLE JYIH
- {0xC9C0, 0xC9C0, prLV}, // Lo HANGUL SYLLABLE JI
- {0xC9C1, 0xC9DB, prLVT}, // Lo [27] HANGUL SYLLABLE JIG..HANGUL SYLLABLE JIH
- {0xC9DC, 0xC9DC, prLV}, // Lo HANGUL SYLLABLE JJA
- {0xC9DD, 0xC9F7, prLVT}, // Lo [27] HANGUL SYLLABLE JJAG..HANGUL SYLLABLE JJAH
- {0xC9F8, 0xC9F8, prLV}, // Lo HANGUL SYLLABLE JJAE
- {0xC9F9, 0xCA13, prLVT}, // Lo [27] HANGUL SYLLABLE JJAEG..HANGUL SYLLABLE JJAEH
- {0xCA14, 0xCA14, prLV}, // Lo HANGUL SYLLABLE JJYA
- {0xCA15, 0xCA2F, prLVT}, // Lo [27] HANGUL SYLLABLE JJYAG..HANGUL SYLLABLE JJYAH
- {0xCA30, 0xCA30, prLV}, // Lo HANGUL SYLLABLE JJYAE
- {0xCA31, 0xCA4B, prLVT}, // Lo [27] HANGUL SYLLABLE JJYAEG..HANGUL SYLLABLE JJYAEH
- {0xCA4C, 0xCA4C, prLV}, // Lo HANGUL SYLLABLE JJEO
- {0xCA4D, 0xCA67, prLVT}, // Lo [27] HANGUL SYLLABLE JJEOG..HANGUL SYLLABLE JJEOH
- {0xCA68, 0xCA68, prLV}, // Lo HANGUL SYLLABLE JJE
- {0xCA69, 0xCA83, prLVT}, // Lo [27] HANGUL SYLLABLE JJEG..HANGUL SYLLABLE JJEH
- {0xCA84, 0xCA84, prLV}, // Lo HANGUL SYLLABLE JJYEO
- {0xCA85, 0xCA9F, prLVT}, // Lo [27] HANGUL SYLLABLE JJYEOG..HANGUL SYLLABLE JJYEOH
- {0xCAA0, 0xCAA0, prLV}, // Lo HANGUL SYLLABLE JJYE
- {0xCAA1, 0xCABB, prLVT}, // Lo [27] HANGUL SYLLABLE JJYEG..HANGUL SYLLABLE JJYEH
- {0xCABC, 0xCABC, prLV}, // Lo HANGUL SYLLABLE JJO
- {0xCABD, 0xCAD7, prLVT}, // Lo [27] HANGUL SYLLABLE JJOG..HANGUL SYLLABLE JJOH
- {0xCAD8, 0xCAD8, prLV}, // Lo HANGUL SYLLABLE JJWA
- {0xCAD9, 0xCAF3, prLVT}, // Lo [27] HANGUL SYLLABLE JJWAG..HANGUL SYLLABLE JJWAH
- {0xCAF4, 0xCAF4, prLV}, // Lo HANGUL SYLLABLE JJWAE
- {0xCAF5, 0xCB0F, prLVT}, // Lo [27] HANGUL SYLLABLE JJWAEG..HANGUL SYLLABLE JJWAEH
- {0xCB10, 0xCB10, prLV}, // Lo HANGUL SYLLABLE JJOE
- {0xCB11, 0xCB2B, prLVT}, // Lo [27] HANGUL SYLLABLE JJOEG..HANGUL SYLLABLE JJOEH
- {0xCB2C, 0xCB2C, prLV}, // Lo HANGUL SYLLABLE JJYO
- {0xCB2D, 0xCB47, prLVT}, // Lo [27] HANGUL SYLLABLE JJYOG..HANGUL SYLLABLE JJYOH
- {0xCB48, 0xCB48, prLV}, // Lo HANGUL SYLLABLE JJU
- {0xCB49, 0xCB63, prLVT}, // Lo [27] HANGUL SYLLABLE JJUG..HANGUL SYLLABLE JJUH
- {0xCB64, 0xCB64, prLV}, // Lo HANGUL SYLLABLE JJWEO
- {0xCB65, 0xCB7F, prLVT}, // Lo [27] HANGUL SYLLABLE JJWEOG..HANGUL SYLLABLE JJWEOH
- {0xCB80, 0xCB80, prLV}, // Lo HANGUL SYLLABLE JJWE
- {0xCB81, 0xCB9B, prLVT}, // Lo [27] HANGUL SYLLABLE JJWEG..HANGUL SYLLABLE JJWEH
- {0xCB9C, 0xCB9C, prLV}, // Lo HANGUL SYLLABLE JJWI
- {0xCB9D, 0xCBB7, prLVT}, // Lo [27] HANGUL SYLLABLE JJWIG..HANGUL SYLLABLE JJWIH
- {0xCBB8, 0xCBB8, prLV}, // Lo HANGUL SYLLABLE JJYU
- {0xCBB9, 0xCBD3, prLVT}, // Lo [27] HANGUL SYLLABLE JJYUG..HANGUL SYLLABLE JJYUH
- {0xCBD4, 0xCBD4, prLV}, // Lo HANGUL SYLLABLE JJEU
- {0xCBD5, 0xCBEF, prLVT}, // Lo [27] HANGUL SYLLABLE JJEUG..HANGUL SYLLABLE JJEUH
- {0xCBF0, 0xCBF0, prLV}, // Lo HANGUL SYLLABLE JJYI
- {0xCBF1, 0xCC0B, prLVT}, // Lo [27] HANGUL SYLLABLE JJYIG..HANGUL SYLLABLE JJYIH
- {0xCC0C, 0xCC0C, prLV}, // Lo HANGUL SYLLABLE JJI
- {0xCC0D, 0xCC27, prLVT}, // Lo [27] HANGUL SYLLABLE JJIG..HANGUL SYLLABLE JJIH
- {0xCC28, 0xCC28, prLV}, // Lo HANGUL SYLLABLE CA
- {0xCC29, 0xCC43, prLVT}, // Lo [27] HANGUL SYLLABLE CAG..HANGUL SYLLABLE CAH
- {0xCC44, 0xCC44, prLV}, // Lo HANGUL SYLLABLE CAE
- {0xCC45, 0xCC5F, prLVT}, // Lo [27] HANGUL SYLLABLE CAEG..HANGUL SYLLABLE CAEH
- {0xCC60, 0xCC60, prLV}, // Lo HANGUL SYLLABLE CYA
- {0xCC61, 0xCC7B, prLVT}, // Lo [27] HANGUL SYLLABLE CYAG..HANGUL SYLLABLE CYAH
- {0xCC7C, 0xCC7C, prLV}, // Lo HANGUL SYLLABLE CYAE
- {0xCC7D, 0xCC97, prLVT}, // Lo [27] HANGUL SYLLABLE CYAEG..HANGUL SYLLABLE CYAEH
- {0xCC98, 0xCC98, prLV}, // Lo HANGUL SYLLABLE CEO
- {0xCC99, 0xCCB3, prLVT}, // Lo [27] HANGUL SYLLABLE CEOG..HANGUL SYLLABLE CEOH
- {0xCCB4, 0xCCB4, prLV}, // Lo HANGUL SYLLABLE CE
- {0xCCB5, 0xCCCF, prLVT}, // Lo [27] HANGUL SYLLABLE CEG..HANGUL SYLLABLE CEH
- {0xCCD0, 0xCCD0, prLV}, // Lo HANGUL SYLLABLE CYEO
- {0xCCD1, 0xCCEB, prLVT}, // Lo [27] HANGUL SYLLABLE CYEOG..HANGUL SYLLABLE CYEOH
- {0xCCEC, 0xCCEC, prLV}, // Lo HANGUL SYLLABLE CYE
- {0xCCED, 0xCD07, prLVT}, // Lo [27] HANGUL SYLLABLE CYEG..HANGUL SYLLABLE CYEH
- {0xCD08, 0xCD08, prLV}, // Lo HANGUL SYLLABLE CO
- {0xCD09, 0xCD23, prLVT}, // Lo [27] HANGUL SYLLABLE COG..HANGUL SYLLABLE COH
- {0xCD24, 0xCD24, prLV}, // Lo HANGUL SYLLABLE CWA
- {0xCD25, 0xCD3F, prLVT}, // Lo [27] HANGUL SYLLABLE CWAG..HANGUL SYLLABLE CWAH
- {0xCD40, 0xCD40, prLV}, // Lo HANGUL SYLLABLE CWAE
- {0xCD41, 0xCD5B, prLVT}, // Lo [27] HANGUL SYLLABLE CWAEG..HANGUL SYLLABLE CWAEH
- {0xCD5C, 0xCD5C, prLV}, // Lo HANGUL SYLLABLE COE
- {0xCD5D, 0xCD77, prLVT}, // Lo [27] HANGUL SYLLABLE COEG..HANGUL SYLLABLE COEH
- {0xCD78, 0xCD78, prLV}, // Lo HANGUL SYLLABLE CYO
- {0xCD79, 0xCD93, prLVT}, // Lo [27] HANGUL SYLLABLE CYOG..HANGUL SYLLABLE CYOH
- {0xCD94, 0xCD94, prLV}, // Lo HANGUL SYLLABLE CU
- {0xCD95, 0xCDAF, prLVT}, // Lo [27] HANGUL SYLLABLE CUG..HANGUL SYLLABLE CUH
- {0xCDB0, 0xCDB0, prLV}, // Lo HANGUL SYLLABLE CWEO
- {0xCDB1, 0xCDCB, prLVT}, // Lo [27] HANGUL SYLLABLE CWEOG..HANGUL SYLLABLE CWEOH
- {0xCDCC, 0xCDCC, prLV}, // Lo HANGUL SYLLABLE CWE
- {0xCDCD, 0xCDE7, prLVT}, // Lo [27] HANGUL SYLLABLE CWEG..HANGUL SYLLABLE CWEH
- {0xCDE8, 0xCDE8, prLV}, // Lo HANGUL SYLLABLE CWI
- {0xCDE9, 0xCE03, prLVT}, // Lo [27] HANGUL SYLLABLE CWIG..HANGUL SYLLABLE CWIH
- {0xCE04, 0xCE04, prLV}, // Lo HANGUL SYLLABLE CYU
- {0xCE05, 0xCE1F, prLVT}, // Lo [27] HANGUL SYLLABLE CYUG..HANGUL SYLLABLE CYUH
- {0xCE20, 0xCE20, prLV}, // Lo HANGUL SYLLABLE CEU
- {0xCE21, 0xCE3B, prLVT}, // Lo [27] HANGUL SYLLABLE CEUG..HANGUL SYLLABLE CEUH
- {0xCE3C, 0xCE3C, prLV}, // Lo HANGUL SYLLABLE CYI
- {0xCE3D, 0xCE57, prLVT}, // Lo [27] HANGUL SYLLABLE CYIG..HANGUL SYLLABLE CYIH
- {0xCE58, 0xCE58, prLV}, // Lo HANGUL SYLLABLE CI
- {0xCE59, 0xCE73, prLVT}, // Lo [27] HANGUL SYLLABLE CIG..HANGUL SYLLABLE CIH
- {0xCE74, 0xCE74, prLV}, // Lo HANGUL SYLLABLE KA
- {0xCE75, 0xCE8F, prLVT}, // Lo [27] HANGUL SYLLABLE KAG..HANGUL SYLLABLE KAH
- {0xCE90, 0xCE90, prLV}, // Lo HANGUL SYLLABLE KAE
- {0xCE91, 0xCEAB, prLVT}, // Lo [27] HANGUL SYLLABLE KAEG..HANGUL SYLLABLE KAEH
- {0xCEAC, 0xCEAC, prLV}, // Lo HANGUL SYLLABLE KYA
- {0xCEAD, 0xCEC7, prLVT}, // Lo [27] HANGUL SYLLABLE KYAG..HANGUL SYLLABLE KYAH
- {0xCEC8, 0xCEC8, prLV}, // Lo HANGUL SYLLABLE KYAE
- {0xCEC9, 0xCEE3, prLVT}, // Lo [27] HANGUL SYLLABLE KYAEG..HANGUL SYLLABLE KYAEH
- {0xCEE4, 0xCEE4, prLV}, // Lo HANGUL SYLLABLE KEO
- {0xCEE5, 0xCEFF, prLVT}, // Lo [27] HANGUL SYLLABLE KEOG..HANGUL SYLLABLE KEOH
- {0xCF00, 0xCF00, prLV}, // Lo HANGUL SYLLABLE KE
- {0xCF01, 0xCF1B, prLVT}, // Lo [27] HANGUL SYLLABLE KEG..HANGUL SYLLABLE KEH
- {0xCF1C, 0xCF1C, prLV}, // Lo HANGUL SYLLABLE KYEO
- {0xCF1D, 0xCF37, prLVT}, // Lo [27] HANGUL SYLLABLE KYEOG..HANGUL SYLLABLE KYEOH
- {0xCF38, 0xCF38, prLV}, // Lo HANGUL SYLLABLE KYE
- {0xCF39, 0xCF53, prLVT}, // Lo [27] HANGUL SYLLABLE KYEG..HANGUL SYLLABLE KYEH
- {0xCF54, 0xCF54, prLV}, // Lo HANGUL SYLLABLE KO
- {0xCF55, 0xCF6F, prLVT}, // Lo [27] HANGUL SYLLABLE KOG..HANGUL SYLLABLE KOH
- {0xCF70, 0xCF70, prLV}, // Lo HANGUL SYLLABLE KWA
- {0xCF71, 0xCF8B, prLVT}, // Lo [27] HANGUL SYLLABLE KWAG..HANGUL SYLLABLE KWAH
- {0xCF8C, 0xCF8C, prLV}, // Lo HANGUL SYLLABLE KWAE
- {0xCF8D, 0xCFA7, prLVT}, // Lo [27] HANGUL SYLLABLE KWAEG..HANGUL SYLLABLE KWAEH
- {0xCFA8, 0xCFA8, prLV}, // Lo HANGUL SYLLABLE KOE
- {0xCFA9, 0xCFC3, prLVT}, // Lo [27] HANGUL SYLLABLE KOEG..HANGUL SYLLABLE KOEH
- {0xCFC4, 0xCFC4, prLV}, // Lo HANGUL SYLLABLE KYO
- {0xCFC5, 0xCFDF, prLVT}, // Lo [27] HANGUL SYLLABLE KYOG..HANGUL SYLLABLE KYOH
- {0xCFE0, 0xCFE0, prLV}, // Lo HANGUL SYLLABLE KU
- {0xCFE1, 0xCFFB, prLVT}, // Lo [27] HANGUL SYLLABLE KUG..HANGUL SYLLABLE KUH
- {0xCFFC, 0xCFFC, prLV}, // Lo HANGUL SYLLABLE KWEO
- {0xCFFD, 0xD017, prLVT}, // Lo [27] HANGUL SYLLABLE KWEOG..HANGUL SYLLABLE KWEOH
- {0xD018, 0xD018, prLV}, // Lo HANGUL SYLLABLE KWE
- {0xD019, 0xD033, prLVT}, // Lo [27] HANGUL SYLLABLE KWEG..HANGUL SYLLABLE KWEH
- {0xD034, 0xD034, prLV}, // Lo HANGUL SYLLABLE KWI
- {0xD035, 0xD04F, prLVT}, // Lo [27] HANGUL SYLLABLE KWIG..HANGUL SYLLABLE KWIH
- {0xD050, 0xD050, prLV}, // Lo HANGUL SYLLABLE KYU
- {0xD051, 0xD06B, prLVT}, // Lo [27] HANGUL SYLLABLE KYUG..HANGUL SYLLABLE KYUH
- {0xD06C, 0xD06C, prLV}, // Lo HANGUL SYLLABLE KEU
- {0xD06D, 0xD087, prLVT}, // Lo [27] HANGUL SYLLABLE KEUG..HANGUL SYLLABLE KEUH
- {0xD088, 0xD088, prLV}, // Lo HANGUL SYLLABLE KYI
- {0xD089, 0xD0A3, prLVT}, // Lo [27] HANGUL SYLLABLE KYIG..HANGUL SYLLABLE KYIH
- {0xD0A4, 0xD0A4, prLV}, // Lo HANGUL SYLLABLE KI
- {0xD0A5, 0xD0BF, prLVT}, // Lo [27] HANGUL SYLLABLE KIG..HANGUL SYLLABLE KIH
- {0xD0C0, 0xD0C0, prLV}, // Lo HANGUL SYLLABLE TA
- {0xD0C1, 0xD0DB, prLVT}, // Lo [27] HANGUL SYLLABLE TAG..HANGUL SYLLABLE TAH
- {0xD0DC, 0xD0DC, prLV}, // Lo HANGUL SYLLABLE TAE
- {0xD0DD, 0xD0F7, prLVT}, // Lo [27] HANGUL SYLLABLE TAEG..HANGUL SYLLABLE TAEH
- {0xD0F8, 0xD0F8, prLV}, // Lo HANGUL SYLLABLE TYA
- {0xD0F9, 0xD113, prLVT}, // Lo [27] HANGUL SYLLABLE TYAG..HANGUL SYLLABLE TYAH
- {0xD114, 0xD114, prLV}, // Lo HANGUL SYLLABLE TYAE
- {0xD115, 0xD12F, prLVT}, // Lo [27] HANGUL SYLLABLE TYAEG..HANGUL SYLLABLE TYAEH
- {0xD130, 0xD130, prLV}, // Lo HANGUL SYLLABLE TEO
- {0xD131, 0xD14B, prLVT}, // Lo [27] HANGUL SYLLABLE TEOG..HANGUL SYLLABLE TEOH
- {0xD14C, 0xD14C, prLV}, // Lo HANGUL SYLLABLE TE
- {0xD14D, 0xD167, prLVT}, // Lo [27] HANGUL SYLLABLE TEG..HANGUL SYLLABLE TEH
- {0xD168, 0xD168, prLV}, // Lo HANGUL SYLLABLE TYEO
- {0xD169, 0xD183, prLVT}, // Lo [27] HANGUL SYLLABLE TYEOG..HANGUL SYLLABLE TYEOH
- {0xD184, 0xD184, prLV}, // Lo HANGUL SYLLABLE TYE
- {0xD185, 0xD19F, prLVT}, // Lo [27] HANGUL SYLLABLE TYEG..HANGUL SYLLABLE TYEH
- {0xD1A0, 0xD1A0, prLV}, // Lo HANGUL SYLLABLE TO
- {0xD1A1, 0xD1BB, prLVT}, // Lo [27] HANGUL SYLLABLE TOG..HANGUL SYLLABLE TOH
- {0xD1BC, 0xD1BC, prLV}, // Lo HANGUL SYLLABLE TWA
- {0xD1BD, 0xD1D7, prLVT}, // Lo [27] HANGUL SYLLABLE TWAG..HANGUL SYLLABLE TWAH
- {0xD1D8, 0xD1D8, prLV}, // Lo HANGUL SYLLABLE TWAE
- {0xD1D9, 0xD1F3, prLVT}, // Lo [27] HANGUL SYLLABLE TWAEG..HANGUL SYLLABLE TWAEH
- {0xD1F4, 0xD1F4, prLV}, // Lo HANGUL SYLLABLE TOE
- {0xD1F5, 0xD20F, prLVT}, // Lo [27] HANGUL SYLLABLE TOEG..HANGUL SYLLABLE TOEH
- {0xD210, 0xD210, prLV}, // Lo HANGUL SYLLABLE TYO
- {0xD211, 0xD22B, prLVT}, // Lo [27] HANGUL SYLLABLE TYOG..HANGUL SYLLABLE TYOH
- {0xD22C, 0xD22C, prLV}, // Lo HANGUL SYLLABLE TU
- {0xD22D, 0xD247, prLVT}, // Lo [27] HANGUL SYLLABLE TUG..HANGUL SYLLABLE TUH
- {0xD248, 0xD248, prLV}, // Lo HANGUL SYLLABLE TWEO
- {0xD249, 0xD263, prLVT}, // Lo [27] HANGUL SYLLABLE TWEOG..HANGUL SYLLABLE TWEOH
- {0xD264, 0xD264, prLV}, // Lo HANGUL SYLLABLE TWE
- {0xD265, 0xD27F, prLVT}, // Lo [27] HANGUL SYLLABLE TWEG..HANGUL SYLLABLE TWEH
- {0xD280, 0xD280, prLV}, // Lo HANGUL SYLLABLE TWI
- {0xD281, 0xD29B, prLVT}, // Lo [27] HANGUL SYLLABLE TWIG..HANGUL SYLLABLE TWIH
- {0xD29C, 0xD29C, prLV}, // Lo HANGUL SYLLABLE TYU
- {0xD29D, 0xD2B7, prLVT}, // Lo [27] HANGUL SYLLABLE TYUG..HANGUL SYLLABLE TYUH
- {0xD2B8, 0xD2B8, prLV}, // Lo HANGUL SYLLABLE TEU
- {0xD2B9, 0xD2D3, prLVT}, // Lo [27] HANGUL SYLLABLE TEUG..HANGUL SYLLABLE TEUH
- {0xD2D4, 0xD2D4, prLV}, // Lo HANGUL SYLLABLE TYI
- {0xD2D5, 0xD2EF, prLVT}, // Lo [27] HANGUL SYLLABLE TYIG..HANGUL SYLLABLE TYIH
- {0xD2F0, 0xD2F0, prLV}, // Lo HANGUL SYLLABLE TI
- {0xD2F1, 0xD30B, prLVT}, // Lo [27] HANGUL SYLLABLE TIG..HANGUL SYLLABLE TIH
- {0xD30C, 0xD30C, prLV}, // Lo HANGUL SYLLABLE PA
- {0xD30D, 0xD327, prLVT}, // Lo [27] HANGUL SYLLABLE PAG..HANGUL SYLLABLE PAH
- {0xD328, 0xD328, prLV}, // Lo HANGUL SYLLABLE PAE
- {0xD329, 0xD343, prLVT}, // Lo [27] HANGUL SYLLABLE PAEG..HANGUL SYLLABLE PAEH
- {0xD344, 0xD344, prLV}, // Lo HANGUL SYLLABLE PYA
- {0xD345, 0xD35F, prLVT}, // Lo [27] HANGUL SYLLABLE PYAG..HANGUL SYLLABLE PYAH
- {0xD360, 0xD360, prLV}, // Lo HANGUL SYLLABLE PYAE
- {0xD361, 0xD37B, prLVT}, // Lo [27] HANGUL SYLLABLE PYAEG..HANGUL SYLLABLE PYAEH
- {0xD37C, 0xD37C, prLV}, // Lo HANGUL SYLLABLE PEO
- {0xD37D, 0xD397, prLVT}, // Lo [27] HANGUL SYLLABLE PEOG..HANGUL SYLLABLE PEOH
- {0xD398, 0xD398, prLV}, // Lo HANGUL SYLLABLE PE
- {0xD399, 0xD3B3, prLVT}, // Lo [27] HANGUL SYLLABLE PEG..HANGUL SYLLABLE PEH
- {0xD3B4, 0xD3B4, prLV}, // Lo HANGUL SYLLABLE PYEO
- {0xD3B5, 0xD3CF, prLVT}, // Lo [27] HANGUL SYLLABLE PYEOG..HANGUL SYLLABLE PYEOH
- {0xD3D0, 0xD3D0, prLV}, // Lo HANGUL SYLLABLE PYE
- {0xD3D1, 0xD3EB, prLVT}, // Lo [27] HANGUL SYLLABLE PYEG..HANGUL SYLLABLE PYEH
- {0xD3EC, 0xD3EC, prLV}, // Lo HANGUL SYLLABLE PO
- {0xD3ED, 0xD407, prLVT}, // Lo [27] HANGUL SYLLABLE POG..HANGUL SYLLABLE POH
- {0xD408, 0xD408, prLV}, // Lo HANGUL SYLLABLE PWA
- {0xD409, 0xD423, prLVT}, // Lo [27] HANGUL SYLLABLE PWAG..HANGUL SYLLABLE PWAH
- {0xD424, 0xD424, prLV}, // Lo HANGUL SYLLABLE PWAE
- {0xD425, 0xD43F, prLVT}, // Lo [27] HANGUL SYLLABLE PWAEG..HANGUL SYLLABLE PWAEH
- {0xD440, 0xD440, prLV}, // Lo HANGUL SYLLABLE POE
- {0xD441, 0xD45B, prLVT}, // Lo [27] HANGUL SYLLABLE POEG..HANGUL SYLLABLE POEH
- {0xD45C, 0xD45C, prLV}, // Lo HANGUL SYLLABLE PYO
- {0xD45D, 0xD477, prLVT}, // Lo [27] HANGUL SYLLABLE PYOG..HANGUL SYLLABLE PYOH
- {0xD478, 0xD478, prLV}, // Lo HANGUL SYLLABLE PU
- {0xD479, 0xD493, prLVT}, // Lo [27] HANGUL SYLLABLE PUG..HANGUL SYLLABLE PUH
- {0xD494, 0xD494, prLV}, // Lo HANGUL SYLLABLE PWEO
- {0xD495, 0xD4AF, prLVT}, // Lo [27] HANGUL SYLLABLE PWEOG..HANGUL SYLLABLE PWEOH
- {0xD4B0, 0xD4B0, prLV}, // Lo HANGUL SYLLABLE PWE
- {0xD4B1, 0xD4CB, prLVT}, // Lo [27] HANGUL SYLLABLE PWEG..HANGUL SYLLABLE PWEH
- {0xD4CC, 0xD4CC, prLV}, // Lo HANGUL SYLLABLE PWI
- {0xD4CD, 0xD4E7, prLVT}, // Lo [27] HANGUL SYLLABLE PWIG..HANGUL SYLLABLE PWIH
- {0xD4E8, 0xD4E8, prLV}, // Lo HANGUL SYLLABLE PYU
- {0xD4E9, 0xD503, prLVT}, // Lo [27] HANGUL SYLLABLE PYUG..HANGUL SYLLABLE PYUH
- {0xD504, 0xD504, prLV}, // Lo HANGUL SYLLABLE PEU
- {0xD505, 0xD51F, prLVT}, // Lo [27] HANGUL SYLLABLE PEUG..HANGUL SYLLABLE PEUH
- {0xD520, 0xD520, prLV}, // Lo HANGUL SYLLABLE PYI
- {0xD521, 0xD53B, prLVT}, // Lo [27] HANGUL SYLLABLE PYIG..HANGUL SYLLABLE PYIH
- {0xD53C, 0xD53C, prLV}, // Lo HANGUL SYLLABLE PI
- {0xD53D, 0xD557, prLVT}, // Lo [27] HANGUL SYLLABLE PIG..HANGUL SYLLABLE PIH
- {0xD558, 0xD558, prLV}, // Lo HANGUL SYLLABLE HA
- {0xD559, 0xD573, prLVT}, // Lo [27] HANGUL SYLLABLE HAG..HANGUL SYLLABLE HAH
- {0xD574, 0xD574, prLV}, // Lo HANGUL SYLLABLE HAE
- {0xD575, 0xD58F, prLVT}, // Lo [27] HANGUL SYLLABLE HAEG..HANGUL SYLLABLE HAEH
- {0xD590, 0xD590, prLV}, // Lo HANGUL SYLLABLE HYA
- {0xD591, 0xD5AB, prLVT}, // Lo [27] HANGUL SYLLABLE HYAG..HANGUL SYLLABLE HYAH
- {0xD5AC, 0xD5AC, prLV}, // Lo HANGUL SYLLABLE HYAE
- {0xD5AD, 0xD5C7, prLVT}, // Lo [27] HANGUL SYLLABLE HYAEG..HANGUL SYLLABLE HYAEH
- {0xD5C8, 0xD5C8, prLV}, // Lo HANGUL SYLLABLE HEO
- {0xD5C9, 0xD5E3, prLVT}, // Lo [27] HANGUL SYLLABLE HEOG..HANGUL SYLLABLE HEOH
- {0xD5E4, 0xD5E4, prLV}, // Lo HANGUL SYLLABLE HE
- {0xD5E5, 0xD5FF, prLVT}, // Lo [27] HANGUL SYLLABLE HEG..HANGUL SYLLABLE HEH
- {0xD600, 0xD600, prLV}, // Lo HANGUL SYLLABLE HYEO
- {0xD601, 0xD61B, prLVT}, // Lo [27] HANGUL SYLLABLE HYEOG..HANGUL SYLLABLE HYEOH
- {0xD61C, 0xD61C, prLV}, // Lo HANGUL SYLLABLE HYE
- {0xD61D, 0xD637, prLVT}, // Lo [27] HANGUL SYLLABLE HYEG..HANGUL SYLLABLE HYEH
- {0xD638, 0xD638, prLV}, // Lo HANGUL SYLLABLE HO
- {0xD639, 0xD653, prLVT}, // Lo [27] HANGUL SYLLABLE HOG..HANGUL SYLLABLE HOH
- {0xD654, 0xD654, prLV}, // Lo HANGUL SYLLABLE HWA
- {0xD655, 0xD66F, prLVT}, // Lo [27] HANGUL SYLLABLE HWAG..HANGUL SYLLABLE HWAH
- {0xD670, 0xD670, prLV}, // Lo HANGUL SYLLABLE HWAE
- {0xD671, 0xD68B, prLVT}, // Lo [27] HANGUL SYLLABLE HWAEG..HANGUL SYLLABLE HWAEH
- {0xD68C, 0xD68C, prLV}, // Lo HANGUL SYLLABLE HOE
- {0xD68D, 0xD6A7, prLVT}, // Lo [27] HANGUL SYLLABLE HOEG..HANGUL SYLLABLE HOEH
- {0xD6A8, 0xD6A8, prLV}, // Lo HANGUL SYLLABLE HYO
- {0xD6A9, 0xD6C3, prLVT}, // Lo [27] HANGUL SYLLABLE HYOG..HANGUL SYLLABLE HYOH
- {0xD6C4, 0xD6C4, prLV}, // Lo HANGUL SYLLABLE HU
- {0xD6C5, 0xD6DF, prLVT}, // Lo [27] HANGUL SYLLABLE HUG..HANGUL SYLLABLE HUH
- {0xD6E0, 0xD6E0, prLV}, // Lo HANGUL SYLLABLE HWEO
- {0xD6E1, 0xD6FB, prLVT}, // Lo [27] HANGUL SYLLABLE HWEOG..HANGUL SYLLABLE HWEOH
- {0xD6FC, 0xD6FC, prLV}, // Lo HANGUL SYLLABLE HWE
- {0xD6FD, 0xD717, prLVT}, // Lo [27] HANGUL SYLLABLE HWEG..HANGUL SYLLABLE HWEH
- {0xD718, 0xD718, prLV}, // Lo HANGUL SYLLABLE HWI
- {0xD719, 0xD733, prLVT}, // Lo [27] HANGUL SYLLABLE HWIG..HANGUL SYLLABLE HWIH
- {0xD734, 0xD734, prLV}, // Lo HANGUL SYLLABLE HYU
- {0xD735, 0xD74F, prLVT}, // Lo [27] HANGUL SYLLABLE HYUG..HANGUL SYLLABLE HYUH
- {0xD750, 0xD750, prLV}, // Lo HANGUL SYLLABLE HEU
- {0xD751, 0xD76B, prLVT}, // Lo [27] HANGUL SYLLABLE HEUG..HANGUL SYLLABLE HEUH
- {0xD76C, 0xD76C, prLV}, // Lo HANGUL SYLLABLE HYI
- {0xD76D, 0xD787, prLVT}, // Lo [27] HANGUL SYLLABLE HYIG..HANGUL SYLLABLE HYIH
- {0xD788, 0xD788, prLV}, // Lo HANGUL SYLLABLE HI
- {0xD789, 0xD7A3, prLVT}, // Lo [27] HANGUL SYLLABLE HIG..HANGUL SYLLABLE HIH
- {0xD7B0, 0xD7C6, prV}, // Lo [23] HANGUL JUNGSEONG O-YEO..HANGUL JUNGSEONG ARAEA-E
- {0xD7CB, 0xD7FB, prT}, // Lo [49] HANGUL JONGSEONG NIEUN-RIEUL..HANGUL JONGSEONG PHIEUPH-THIEUTH
- {0xFB1E, 0xFB1E, prExtend}, // Mn HEBREW POINT JUDEO-SPANISH VARIKA
- {0xFE00, 0xFE0F, prExtend}, // Mn [16] VARIATION SELECTOR-1..VARIATION SELECTOR-16
- {0xFE20, 0xFE2F, prExtend}, // Mn [16] COMBINING LIGATURE LEFT HALF..COMBINING CYRILLIC TITLO RIGHT HALF
- {0xFEFF, 0xFEFF, prControl}, // Cf ZERO WIDTH NO-BREAK SPACE
- {0xFF9E, 0xFF9F, prExtend}, // Lm [2] HALFWIDTH KATAKANA VOICED SOUND MARK..HALFWIDTH KATAKANA SEMI-VOICED SOUND MARK
- {0xFFF0, 0xFFF8, prControl}, // Cn [9] ..
- {0xFFF9, 0xFFFB, prControl}, // Cf [3] INTERLINEAR ANNOTATION ANCHOR..INTERLINEAR ANNOTATION TERMINATOR
- {0x101FD, 0x101FD, prExtend}, // Mn PHAISTOS DISC SIGN COMBINING OBLIQUE STROKE
- {0x102E0, 0x102E0, prExtend}, // Mn COPTIC EPACT THOUSANDS MARK
- {0x10376, 0x1037A, prExtend}, // Mn [5] COMBINING OLD PERMIC LETTER AN..COMBINING OLD PERMIC LETTER SII
- {0x10A01, 0x10A03, prExtend}, // Mn [3] KHAROSHTHI VOWEL SIGN I..KHAROSHTHI VOWEL SIGN VOCALIC R
- {0x10A05, 0x10A06, prExtend}, // Mn [2] KHAROSHTHI VOWEL SIGN E..KHAROSHTHI VOWEL SIGN O
- {0x10A0C, 0x10A0F, prExtend}, // Mn [4] KHAROSHTHI VOWEL LENGTH MARK..KHAROSHTHI SIGN VISARGA
- {0x10A38, 0x10A3A, prExtend}, // Mn [3] KHAROSHTHI SIGN BAR ABOVE..KHAROSHTHI SIGN DOT BELOW
- {0x10A3F, 0x10A3F, prExtend}, // Mn KHAROSHTHI VIRAMA
- {0x10AE5, 0x10AE6, prExtend}, // Mn [2] MANICHAEAN ABBREVIATION MARK ABOVE..MANICHAEAN ABBREVIATION MARK BELOW
- {0x10D24, 0x10D27, prExtend}, // Mn [4] HANIFI ROHINGYA SIGN HARBAHAY..HANIFI ROHINGYA SIGN TASSI
- {0x10EAB, 0x10EAC, prExtend}, // Mn [2] YEZIDI COMBINING HAMZA MARK..YEZIDI COMBINING MADDA MARK
- {0x10F46, 0x10F50, prExtend}, // Mn [11] SOGDIAN COMBINING DOT BELOW..SOGDIAN COMBINING STROKE BELOW
- {0x10F82, 0x10F85, prExtend}, // Mn [4] OLD UYGHUR COMBINING DOT ABOVE..OLD UYGHUR COMBINING TWO DOTS BELOW
- {0x11000, 0x11000, prSpacingMark}, // Mc BRAHMI SIGN CANDRABINDU
- {0x11001, 0x11001, prExtend}, // Mn BRAHMI SIGN ANUSVARA
- {0x11002, 0x11002, prSpacingMark}, // Mc BRAHMI SIGN VISARGA
- {0x11038, 0x11046, prExtend}, // Mn [15] BRAHMI VOWEL SIGN AA..BRAHMI VIRAMA
- {0x11070, 0x11070, prExtend}, // Mn BRAHMI SIGN OLD TAMIL VIRAMA
- {0x11073, 0x11074, prExtend}, // Mn [2] BRAHMI VOWEL SIGN OLD TAMIL SHORT E..BRAHMI VOWEL SIGN OLD TAMIL SHORT O
- {0x1107F, 0x11081, prExtend}, // Mn [3] BRAHMI NUMBER JOINER..KAITHI SIGN ANUSVARA
- {0x11082, 0x11082, prSpacingMark}, // Mc KAITHI SIGN VISARGA
- {0x110B0, 0x110B2, prSpacingMark}, // Mc [3] KAITHI VOWEL SIGN AA..KAITHI VOWEL SIGN II
- {0x110B3, 0x110B6, prExtend}, // Mn [4] KAITHI VOWEL SIGN U..KAITHI VOWEL SIGN AI
- {0x110B7, 0x110B8, prSpacingMark}, // Mc [2] KAITHI VOWEL SIGN O..KAITHI VOWEL SIGN AU
- {0x110B9, 0x110BA, prExtend}, // Mn [2] KAITHI SIGN VIRAMA..KAITHI SIGN NUKTA
- {0x110BD, 0x110BD, prPrepend}, // Cf KAITHI NUMBER SIGN
- {0x110C2, 0x110C2, prExtend}, // Mn KAITHI VOWEL SIGN VOCALIC R
- {0x110CD, 0x110CD, prPrepend}, // Cf KAITHI NUMBER SIGN ABOVE
- {0x11100, 0x11102, prExtend}, // Mn [3] CHAKMA SIGN CANDRABINDU..CHAKMA SIGN VISARGA
- {0x11127, 0x1112B, prExtend}, // Mn [5] CHAKMA VOWEL SIGN A..CHAKMA VOWEL SIGN UU
- {0x1112C, 0x1112C, prSpacingMark}, // Mc CHAKMA VOWEL SIGN E
- {0x1112D, 0x11134, prExtend}, // Mn [8] CHAKMA VOWEL SIGN AI..CHAKMA MAAYYAA
- {0x11145, 0x11146, prSpacingMark}, // Mc [2] CHAKMA VOWEL SIGN AA..CHAKMA VOWEL SIGN EI
- {0x11173, 0x11173, prExtend}, // Mn MAHAJANI SIGN NUKTA
- {0x11180, 0x11181, prExtend}, // Mn [2] SHARADA SIGN CANDRABINDU..SHARADA SIGN ANUSVARA
- {0x11182, 0x11182, prSpacingMark}, // Mc SHARADA SIGN VISARGA
- {0x111B3, 0x111B5, prSpacingMark}, // Mc [3] SHARADA VOWEL SIGN AA..SHARADA VOWEL SIGN II
- {0x111B6, 0x111BE, prExtend}, // Mn [9] SHARADA VOWEL SIGN U..SHARADA VOWEL SIGN O
- {0x111BF, 0x111C0, prSpacingMark}, // Mc [2] SHARADA VOWEL SIGN AU..SHARADA SIGN VIRAMA
- {0x111C2, 0x111C3, prPrepend}, // Lo [2] SHARADA SIGN JIHVAMULIYA..SHARADA SIGN UPADHMANIYA
- {0x111C9, 0x111CC, prExtend}, // Mn [4] SHARADA SANDHI MARK..SHARADA EXTRA SHORT VOWEL MARK
- {0x111CE, 0x111CE, prSpacingMark}, // Mc SHARADA VOWEL SIGN PRISHTHAMATRA E
- {0x111CF, 0x111CF, prExtend}, // Mn SHARADA SIGN INVERTED CANDRABINDU
- {0x1122C, 0x1122E, prSpacingMark}, // Mc [3] KHOJKI VOWEL SIGN AA..KHOJKI VOWEL SIGN II
- {0x1122F, 0x11231, prExtend}, // Mn [3] KHOJKI VOWEL SIGN U..KHOJKI VOWEL SIGN AI
- {0x11232, 0x11233, prSpacingMark}, // Mc [2] KHOJKI VOWEL SIGN O..KHOJKI VOWEL SIGN AU
- {0x11234, 0x11234, prExtend}, // Mn KHOJKI SIGN ANUSVARA
- {0x11235, 0x11235, prSpacingMark}, // Mc KHOJKI SIGN VIRAMA
- {0x11236, 0x11237, prExtend}, // Mn [2] KHOJKI SIGN NUKTA..KHOJKI SIGN SHADDA
- {0x1123E, 0x1123E, prExtend}, // Mn KHOJKI SIGN SUKUN
- {0x112DF, 0x112DF, prExtend}, // Mn KHUDAWADI SIGN ANUSVARA
- {0x112E0, 0x112E2, prSpacingMark}, // Mc [3] KHUDAWADI VOWEL SIGN AA..KHUDAWADI VOWEL SIGN II
- {0x112E3, 0x112EA, prExtend}, // Mn [8] KHUDAWADI VOWEL SIGN U..KHUDAWADI SIGN VIRAMA
- {0x11300, 0x11301, prExtend}, // Mn [2] GRANTHA SIGN COMBINING ANUSVARA ABOVE..GRANTHA SIGN CANDRABINDU
- {0x11302, 0x11303, prSpacingMark}, // Mc [2] GRANTHA SIGN ANUSVARA..GRANTHA SIGN VISARGA
- {0x1133B, 0x1133C, prExtend}, // Mn [2] COMBINING BINDU BELOW..GRANTHA SIGN NUKTA
- {0x1133E, 0x1133E, prExtend}, // Mc GRANTHA VOWEL SIGN AA
- {0x1133F, 0x1133F, prSpacingMark}, // Mc GRANTHA VOWEL SIGN I
- {0x11340, 0x11340, prExtend}, // Mn GRANTHA VOWEL SIGN II
- {0x11341, 0x11344, prSpacingMark}, // Mc [4] GRANTHA VOWEL SIGN U..GRANTHA VOWEL SIGN VOCALIC RR
- {0x11347, 0x11348, prSpacingMark}, // Mc [2] GRANTHA VOWEL SIGN EE..GRANTHA VOWEL SIGN AI
- {0x1134B, 0x1134D, prSpacingMark}, // Mc [3] GRANTHA VOWEL SIGN OO..GRANTHA SIGN VIRAMA
- {0x11357, 0x11357, prExtend}, // Mc GRANTHA AU LENGTH MARK
- {0x11362, 0x11363, prSpacingMark}, // Mc [2] GRANTHA VOWEL SIGN VOCALIC L..GRANTHA VOWEL SIGN VOCALIC LL
- {0x11366, 0x1136C, prExtend}, // Mn [7] COMBINING GRANTHA DIGIT ZERO..COMBINING GRANTHA DIGIT SIX
- {0x11370, 0x11374, prExtend}, // Mn [5] COMBINING GRANTHA LETTER A..COMBINING GRANTHA LETTER PA
- {0x11435, 0x11437, prSpacingMark}, // Mc [3] NEWA VOWEL SIGN AA..NEWA VOWEL SIGN II
- {0x11438, 0x1143F, prExtend}, // Mn [8] NEWA VOWEL SIGN U..NEWA VOWEL SIGN AI
- {0x11440, 0x11441, prSpacingMark}, // Mc [2] NEWA VOWEL SIGN O..NEWA VOWEL SIGN AU
- {0x11442, 0x11444, prExtend}, // Mn [3] NEWA SIGN VIRAMA..NEWA SIGN ANUSVARA
- {0x11445, 0x11445, prSpacingMark}, // Mc NEWA SIGN VISARGA
- {0x11446, 0x11446, prExtend}, // Mn NEWA SIGN NUKTA
- {0x1145E, 0x1145E, prExtend}, // Mn NEWA SANDHI MARK
- {0x114B0, 0x114B0, prExtend}, // Mc TIRHUTA VOWEL SIGN AA
- {0x114B1, 0x114B2, prSpacingMark}, // Mc [2] TIRHUTA VOWEL SIGN I..TIRHUTA VOWEL SIGN II
- {0x114B3, 0x114B8, prExtend}, // Mn [6] TIRHUTA VOWEL SIGN U..TIRHUTA VOWEL SIGN VOCALIC LL
- {0x114B9, 0x114B9, prSpacingMark}, // Mc TIRHUTA VOWEL SIGN E
- {0x114BA, 0x114BA, prExtend}, // Mn TIRHUTA VOWEL SIGN SHORT E
- {0x114BB, 0x114BC, prSpacingMark}, // Mc [2] TIRHUTA VOWEL SIGN AI..TIRHUTA VOWEL SIGN O
- {0x114BD, 0x114BD, prExtend}, // Mc TIRHUTA VOWEL SIGN SHORT O
- {0x114BE, 0x114BE, prSpacingMark}, // Mc TIRHUTA VOWEL SIGN AU
- {0x114BF, 0x114C0, prExtend}, // Mn [2] TIRHUTA SIGN CANDRABINDU..TIRHUTA SIGN ANUSVARA
- {0x114C1, 0x114C1, prSpacingMark}, // Mc TIRHUTA SIGN VISARGA
- {0x114C2, 0x114C3, prExtend}, // Mn [2] TIRHUTA SIGN VIRAMA..TIRHUTA SIGN NUKTA
- {0x115AF, 0x115AF, prExtend}, // Mc SIDDHAM VOWEL SIGN AA
- {0x115B0, 0x115B1, prSpacingMark}, // Mc [2] SIDDHAM VOWEL SIGN I..SIDDHAM VOWEL SIGN II
- {0x115B2, 0x115B5, prExtend}, // Mn [4] SIDDHAM VOWEL SIGN U..SIDDHAM VOWEL SIGN VOCALIC RR
- {0x115B8, 0x115BB, prSpacingMark}, // Mc [4] SIDDHAM VOWEL SIGN E..SIDDHAM VOWEL SIGN AU
- {0x115BC, 0x115BD, prExtend}, // Mn [2] SIDDHAM SIGN CANDRABINDU..SIDDHAM SIGN ANUSVARA
- {0x115BE, 0x115BE, prSpacingMark}, // Mc SIDDHAM SIGN VISARGA
- {0x115BF, 0x115C0, prExtend}, // Mn [2] SIDDHAM SIGN VIRAMA..SIDDHAM SIGN NUKTA
- {0x115DC, 0x115DD, prExtend}, // Mn [2] SIDDHAM VOWEL SIGN ALTERNATE U..SIDDHAM VOWEL SIGN ALTERNATE UU
- {0x11630, 0x11632, prSpacingMark}, // Mc [3] MODI VOWEL SIGN AA..MODI VOWEL SIGN II
- {0x11633, 0x1163A, prExtend}, // Mn [8] MODI VOWEL SIGN U..MODI VOWEL SIGN AI
- {0x1163B, 0x1163C, prSpacingMark}, // Mc [2] MODI VOWEL SIGN O..MODI VOWEL SIGN AU
- {0x1163D, 0x1163D, prExtend}, // Mn MODI SIGN ANUSVARA
- {0x1163E, 0x1163E, prSpacingMark}, // Mc MODI SIGN VISARGA
- {0x1163F, 0x11640, prExtend}, // Mn [2] MODI SIGN VIRAMA..MODI SIGN ARDHACANDRA
- {0x116AB, 0x116AB, prExtend}, // Mn TAKRI SIGN ANUSVARA
- {0x116AC, 0x116AC, prSpacingMark}, // Mc TAKRI SIGN VISARGA
- {0x116AD, 0x116AD, prExtend}, // Mn TAKRI VOWEL SIGN AA
- {0x116AE, 0x116AF, prSpacingMark}, // Mc [2] TAKRI VOWEL SIGN I..TAKRI VOWEL SIGN II
- {0x116B0, 0x116B5, prExtend}, // Mn [6] TAKRI VOWEL SIGN U..TAKRI VOWEL SIGN AU
- {0x116B6, 0x116B6, prSpacingMark}, // Mc TAKRI SIGN VIRAMA
- {0x116B7, 0x116B7, prExtend}, // Mn TAKRI SIGN NUKTA
- {0x1171D, 0x1171F, prExtend}, // Mn [3] AHOM CONSONANT SIGN MEDIAL LA..AHOM CONSONANT SIGN MEDIAL LIGATING RA
- {0x11722, 0x11725, prExtend}, // Mn [4] AHOM VOWEL SIGN I..AHOM VOWEL SIGN UU
- {0x11726, 0x11726, prSpacingMark}, // Mc AHOM VOWEL SIGN E
- {0x11727, 0x1172B, prExtend}, // Mn [5] AHOM VOWEL SIGN AW..AHOM SIGN KILLER
- {0x1182C, 0x1182E, prSpacingMark}, // Mc [3] DOGRA VOWEL SIGN AA..DOGRA VOWEL SIGN II
- {0x1182F, 0x11837, prExtend}, // Mn [9] DOGRA VOWEL SIGN U..DOGRA SIGN ANUSVARA
- {0x11838, 0x11838, prSpacingMark}, // Mc DOGRA SIGN VISARGA
- {0x11839, 0x1183A, prExtend}, // Mn [2] DOGRA SIGN VIRAMA..DOGRA SIGN NUKTA
- {0x11930, 0x11930, prExtend}, // Mc DIVES AKURU VOWEL SIGN AA
- {0x11931, 0x11935, prSpacingMark}, // Mc [5] DIVES AKURU VOWEL SIGN I..DIVES AKURU VOWEL SIGN E
- {0x11937, 0x11938, prSpacingMark}, // Mc [2] DIVES AKURU VOWEL SIGN AI..DIVES AKURU VOWEL SIGN O
- {0x1193B, 0x1193C, prExtend}, // Mn [2] DIVES AKURU SIGN ANUSVARA..DIVES AKURU SIGN CANDRABINDU
- {0x1193D, 0x1193D, prSpacingMark}, // Mc DIVES AKURU SIGN HALANTA
- {0x1193E, 0x1193E, prExtend}, // Mn DIVES AKURU VIRAMA
- {0x1193F, 0x1193F, prPrepend}, // Lo DIVES AKURU PREFIXED NASAL SIGN
- {0x11940, 0x11940, prSpacingMark}, // Mc DIVES AKURU MEDIAL YA
- {0x11941, 0x11941, prPrepend}, // Lo DIVES AKURU INITIAL RA
- {0x11942, 0x11942, prSpacingMark}, // Mc DIVES AKURU MEDIAL RA
- {0x11943, 0x11943, prExtend}, // Mn DIVES AKURU SIGN NUKTA
- {0x119D1, 0x119D3, prSpacingMark}, // Mc [3] NANDINAGARI VOWEL SIGN AA..NANDINAGARI VOWEL SIGN II
- {0x119D4, 0x119D7, prExtend}, // Mn [4] NANDINAGARI VOWEL SIGN U..NANDINAGARI VOWEL SIGN VOCALIC RR
- {0x119DA, 0x119DB, prExtend}, // Mn [2] NANDINAGARI VOWEL SIGN E..NANDINAGARI VOWEL SIGN AI
- {0x119DC, 0x119DF, prSpacingMark}, // Mc [4] NANDINAGARI VOWEL SIGN O..NANDINAGARI SIGN VISARGA
- {0x119E0, 0x119E0, prExtend}, // Mn NANDINAGARI SIGN VIRAMA
- {0x119E4, 0x119E4, prSpacingMark}, // Mc NANDINAGARI VOWEL SIGN PRISHTHAMATRA E
- {0x11A01, 0x11A0A, prExtend}, // Mn [10] ZANABAZAR SQUARE VOWEL SIGN I..ZANABAZAR SQUARE VOWEL LENGTH MARK
- {0x11A33, 0x11A38, prExtend}, // Mn [6] ZANABAZAR SQUARE FINAL CONSONANT MARK..ZANABAZAR SQUARE SIGN ANUSVARA
- {0x11A39, 0x11A39, prSpacingMark}, // Mc ZANABAZAR SQUARE SIGN VISARGA
- {0x11A3A, 0x11A3A, prPrepend}, // Lo ZANABAZAR SQUARE CLUSTER-INITIAL LETTER RA
- {0x11A3B, 0x11A3E, prExtend}, // Mn [4] ZANABAZAR SQUARE CLUSTER-FINAL LETTER YA..ZANABAZAR SQUARE CLUSTER-FINAL LETTER VA
- {0x11A47, 0x11A47, prExtend}, // Mn ZANABAZAR SQUARE SUBJOINER
- {0x11A51, 0x11A56, prExtend}, // Mn [6] SOYOMBO VOWEL SIGN I..SOYOMBO VOWEL SIGN OE
- {0x11A57, 0x11A58, prSpacingMark}, // Mc [2] SOYOMBO VOWEL SIGN AI..SOYOMBO VOWEL SIGN AU
- {0x11A59, 0x11A5B, prExtend}, // Mn [3] SOYOMBO VOWEL SIGN VOCALIC R..SOYOMBO VOWEL LENGTH MARK
- {0x11A84, 0x11A89, prPrepend}, // Lo [6] SOYOMBO SIGN JIHVAMULIYA..SOYOMBO CLUSTER-INITIAL LETTER SA
- {0x11A8A, 0x11A96, prExtend}, // Mn [13] SOYOMBO FINAL CONSONANT SIGN G..SOYOMBO SIGN ANUSVARA
- {0x11A97, 0x11A97, prSpacingMark}, // Mc SOYOMBO SIGN VISARGA
- {0x11A98, 0x11A99, prExtend}, // Mn [2] SOYOMBO GEMINATION MARK..SOYOMBO SUBJOINER
- {0x11C2F, 0x11C2F, prSpacingMark}, // Mc BHAIKSUKI VOWEL SIGN AA
- {0x11C30, 0x11C36, prExtend}, // Mn [7] BHAIKSUKI VOWEL SIGN I..BHAIKSUKI VOWEL SIGN VOCALIC L
- {0x11C38, 0x11C3D, prExtend}, // Mn [6] BHAIKSUKI VOWEL SIGN E..BHAIKSUKI SIGN ANUSVARA
- {0x11C3E, 0x11C3E, prSpacingMark}, // Mc BHAIKSUKI SIGN VISARGA
- {0x11C3F, 0x11C3F, prExtend}, // Mn BHAIKSUKI SIGN VIRAMA
- {0x11C92, 0x11CA7, prExtend}, // Mn [22] MARCHEN SUBJOINED LETTER KA..MARCHEN SUBJOINED LETTER ZA
- {0x11CA9, 0x11CA9, prSpacingMark}, // Mc MARCHEN SUBJOINED LETTER YA
- {0x11CAA, 0x11CB0, prExtend}, // Mn [7] MARCHEN SUBJOINED LETTER RA..MARCHEN VOWEL SIGN AA
- {0x11CB1, 0x11CB1, prSpacingMark}, // Mc MARCHEN VOWEL SIGN I
- {0x11CB2, 0x11CB3, prExtend}, // Mn [2] MARCHEN VOWEL SIGN U..MARCHEN VOWEL SIGN E
- {0x11CB4, 0x11CB4, prSpacingMark}, // Mc MARCHEN VOWEL SIGN O
- {0x11CB5, 0x11CB6, prExtend}, // Mn [2] MARCHEN SIGN ANUSVARA..MARCHEN SIGN CANDRABINDU
- {0x11D31, 0x11D36, prExtend}, // Mn [6] MASARAM GONDI VOWEL SIGN AA..MASARAM GONDI VOWEL SIGN VOCALIC R
- {0x11D3A, 0x11D3A, prExtend}, // Mn MASARAM GONDI VOWEL SIGN E
- {0x11D3C, 0x11D3D, prExtend}, // Mn [2] MASARAM GONDI VOWEL SIGN AI..MASARAM GONDI VOWEL SIGN O
- {0x11D3F, 0x11D45, prExtend}, // Mn [7] MASARAM GONDI VOWEL SIGN AU..MASARAM GONDI VIRAMA
- {0x11D46, 0x11D46, prPrepend}, // Lo MASARAM GONDI REPHA
- {0x11D47, 0x11D47, prExtend}, // Mn MASARAM GONDI RA-KARA
- {0x11D8A, 0x11D8E, prSpacingMark}, // Mc [5] GUNJALA GONDI VOWEL SIGN AA..GUNJALA GONDI VOWEL SIGN UU
- {0x11D90, 0x11D91, prExtend}, // Mn [2] GUNJALA GONDI VOWEL SIGN EE..GUNJALA GONDI VOWEL SIGN AI
- {0x11D93, 0x11D94, prSpacingMark}, // Mc [2] GUNJALA GONDI VOWEL SIGN OO..GUNJALA GONDI VOWEL SIGN AU
- {0x11D95, 0x11D95, prExtend}, // Mn GUNJALA GONDI SIGN ANUSVARA
- {0x11D96, 0x11D96, prSpacingMark}, // Mc GUNJALA GONDI SIGN VISARGA
- {0x11D97, 0x11D97, prExtend}, // Mn GUNJALA GONDI VIRAMA
- {0x11EF3, 0x11EF4, prExtend}, // Mn [2] MAKASAR VOWEL SIGN I..MAKASAR VOWEL SIGN U
- {0x11EF5, 0x11EF6, prSpacingMark}, // Mc [2] MAKASAR VOWEL SIGN E..MAKASAR VOWEL SIGN O
- {0x13430, 0x13438, prControl}, // Cf [9] EGYPTIAN HIEROGLYPH VERTICAL JOINER..EGYPTIAN HIEROGLYPH END SEGMENT
- {0x16AF0, 0x16AF4, prExtend}, // Mn [5] BASSA VAH COMBINING HIGH TONE..BASSA VAH COMBINING HIGH-LOW TONE
- {0x16B30, 0x16B36, prExtend}, // Mn [7] PAHAWH HMONG MARK CIM TUB..PAHAWH HMONG MARK CIM TAUM
- {0x16F4F, 0x16F4F, prExtend}, // Mn MIAO SIGN CONSONANT MODIFIER BAR
- {0x16F51, 0x16F87, prSpacingMark}, // Mc [55] MIAO SIGN ASPIRATION..MIAO VOWEL SIGN UI
- {0x16F8F, 0x16F92, prExtend}, // Mn [4] MIAO TONE RIGHT..MIAO TONE BELOW
- {0x16FE4, 0x16FE4, prExtend}, // Mn KHITAN SMALL SCRIPT FILLER
- {0x16FF0, 0x16FF1, prSpacingMark}, // Mc [2] VIETNAMESE ALTERNATE READING MARK CA..VIETNAMESE ALTERNATE READING MARK NHAY
- {0x1BC9D, 0x1BC9E, prExtend}, // Mn [2] DUPLOYAN THICK LETTER SELECTOR..DUPLOYAN DOUBLE MARK
- {0x1BCA0, 0x1BCA3, prControl}, // Cf [4] SHORTHAND FORMAT LETTER OVERLAP..SHORTHAND FORMAT UP STEP
- {0x1CF00, 0x1CF2D, prExtend}, // Mn [46] ZNAMENNY COMBINING MARK GORAZDO NIZKO S KRYZHEM ON LEFT..ZNAMENNY COMBINING MARK KRYZH ON LEFT
- {0x1CF30, 0x1CF46, prExtend}, // Mn [23] ZNAMENNY COMBINING TONAL RANGE MARK MRACHNO..ZNAMENNY PRIZNAK MODIFIER ROG
- {0x1D165, 0x1D165, prExtend}, // Mc MUSICAL SYMBOL COMBINING STEM
- {0x1D166, 0x1D166, prSpacingMark}, // Mc MUSICAL SYMBOL COMBINING SPRECHGESANG STEM
- {0x1D167, 0x1D169, prExtend}, // Mn [3] MUSICAL SYMBOL COMBINING TREMOLO-1..MUSICAL SYMBOL COMBINING TREMOLO-3
- {0x1D16D, 0x1D16D, prSpacingMark}, // Mc MUSICAL SYMBOL COMBINING AUGMENTATION DOT
- {0x1D16E, 0x1D172, prExtend}, // Mc [5] MUSICAL SYMBOL COMBINING FLAG-1..MUSICAL SYMBOL COMBINING FLAG-5
- {0x1D173, 0x1D17A, prControl}, // Cf [8] MUSICAL SYMBOL BEGIN BEAM..MUSICAL SYMBOL END PHRASE
- {0x1D17B, 0x1D182, prExtend}, // Mn [8] MUSICAL SYMBOL COMBINING ACCENT..MUSICAL SYMBOL COMBINING LOURE
- {0x1D185, 0x1D18B, prExtend}, // Mn [7] MUSICAL SYMBOL COMBINING DOIT..MUSICAL SYMBOL COMBINING TRIPLE TONGUE
- {0x1D1AA, 0x1D1AD, prExtend}, // Mn [4] MUSICAL SYMBOL COMBINING DOWN BOW..MUSICAL SYMBOL COMBINING SNAP PIZZICATO
- {0x1D242, 0x1D244, prExtend}, // Mn [3] COMBINING GREEK MUSICAL TRISEME..COMBINING GREEK MUSICAL PENTASEME
- {0x1DA00, 0x1DA36, prExtend}, // Mn [55] SIGNWRITING HEAD RIM..SIGNWRITING AIR SUCKING IN
- {0x1DA3B, 0x1DA6C, prExtend}, // Mn [50] SIGNWRITING MOUTH CLOSED NEUTRAL..SIGNWRITING EXCITEMENT
- {0x1DA75, 0x1DA75, prExtend}, // Mn SIGNWRITING UPPER BODY TILTING FROM HIP JOINTS
- {0x1DA84, 0x1DA84, prExtend}, // Mn SIGNWRITING LOCATION HEAD NECK
- {0x1DA9B, 0x1DA9F, prExtend}, // Mn [5] SIGNWRITING FILL MODIFIER-2..SIGNWRITING FILL MODIFIER-6
- {0x1DAA1, 0x1DAAF, prExtend}, // Mn [15] SIGNWRITING ROTATION MODIFIER-2..SIGNWRITING ROTATION MODIFIER-16
- {0x1E000, 0x1E006, prExtend}, // Mn [7] COMBINING GLAGOLITIC LETTER AZU..COMBINING GLAGOLITIC LETTER ZHIVETE
- {0x1E008, 0x1E018, prExtend}, // Mn [17] COMBINING GLAGOLITIC LETTER ZEMLJA..COMBINING GLAGOLITIC LETTER HERU
- {0x1E01B, 0x1E021, prExtend}, // Mn [7] COMBINING GLAGOLITIC LETTER SHTA..COMBINING GLAGOLITIC LETTER YATI
- {0x1E023, 0x1E024, prExtend}, // Mn [2] COMBINING GLAGOLITIC LETTER YU..COMBINING GLAGOLITIC LETTER SMALL YUS
- {0x1E026, 0x1E02A, prExtend}, // Mn [5] COMBINING GLAGOLITIC LETTER YO..COMBINING GLAGOLITIC LETTER FITA
- {0x1E130, 0x1E136, prExtend}, // Mn [7] NYIAKENG PUACHUE HMONG TONE-B..NYIAKENG PUACHUE HMONG TONE-D
- {0x1E2AE, 0x1E2AE, prExtend}, // Mn TOTO SIGN RISING TONE
- {0x1E2EC, 0x1E2EF, prExtend}, // Mn [4] WANCHO TONE TUP..WANCHO TONE KOINI
- {0x1E8D0, 0x1E8D6, prExtend}, // Mn [7] MENDE KIKAKUI COMBINING NUMBER TEENS..MENDE KIKAKUI COMBINING NUMBER MILLIONS
- {0x1E944, 0x1E94A, prExtend}, // Mn [7] ADLAM ALIF LENGTHENER..ADLAM NUKTA
- {0x1F000, 0x1F003, prExtendedPictographic}, // E0.0 [4] (🀀..🀃) MAHJONG TILE EAST WIND..MAHJONG TILE NORTH WIND
- {0x1F004, 0x1F004, prExtendedPictographic}, // E0.6 [1] (🀄) mahjong red dragon
- {0x1F005, 0x1F0CE, prExtendedPictographic}, // E0.0 [202] (🀅..🃎) MAHJONG TILE GREEN DRAGON..PLAYING CARD KING OF DIAMONDS
- {0x1F0CF, 0x1F0CF, prExtendedPictographic}, // E0.6 [1] (ðŸƒ) joker
- {0x1F0D0, 0x1F0FF, prExtendedPictographic}, // E0.0 [48] (ðŸƒ..🃿) ..
- {0x1F10D, 0x1F10F, prExtendedPictographic}, // E0.0 [3] (ðŸ„..ðŸ„) CIRCLED ZERO WITH SLASH..CIRCLED DOLLAR SIGN WITH OVERLAID BACKSLASH
- {0x1F12F, 0x1F12F, prExtendedPictographic}, // E0.0 [1] (🄯) COPYLEFT SYMBOL
- {0x1F16C, 0x1F16F, prExtendedPictographic}, // E0.0 [4] (🅬..🅯) RAISED MR SIGN..CIRCLED HUMAN FIGURE
- {0x1F170, 0x1F171, prExtendedPictographic}, // E0.6 [2] (🅰ï¸..🅱ï¸) A button (blood type)..B button (blood type)
- {0x1F17E, 0x1F17F, prExtendedPictographic}, // E0.6 [2] (🅾ï¸..🅿ï¸) O button (blood type)..P button
- {0x1F18E, 0x1F18E, prExtendedPictographic}, // E0.6 [1] (🆎) AB button (blood type)
- {0x1F191, 0x1F19A, prExtendedPictographic}, // E0.6 [10] (🆑..🆚) CL button..VS button
- {0x1F1AD, 0x1F1E5, prExtendedPictographic}, // E0.0 [57] (ðŸ†..🇥) MASK WORK SYMBOL..
- {0x1F1E6, 0x1F1FF, prRegionalIndicator}, // So [26] REGIONAL INDICATOR SYMBOL LETTER A..REGIONAL INDICATOR SYMBOL LETTER Z
- {0x1F201, 0x1F202, prExtendedPictographic}, // E0.6 [2] (ðŸˆ..🈂ï¸) Japanese “here†button..Japanese “service charge†button
- {0x1F203, 0x1F20F, prExtendedPictographic}, // E0.0 [13] (🈃..ðŸˆ) ..
- {0x1F21A, 0x1F21A, prExtendedPictographic}, // E0.6 [1] (🈚) Japanese “free of charge†button
- {0x1F22F, 0x1F22F, prExtendedPictographic}, // E0.6 [1] (🈯) Japanese “reserved†button
- {0x1F232, 0x1F23A, prExtendedPictographic}, // E0.6 [9] (🈲..🈺) Japanese “prohibited†button..Japanese “open for business†button
- {0x1F23C, 0x1F23F, prExtendedPictographic}, // E0.0 [4] (🈼..🈿) ..
- {0x1F249, 0x1F24F, prExtendedPictographic}, // E0.0 [7] (🉉..ðŸ‰) ..
- {0x1F250, 0x1F251, prExtendedPictographic}, // E0.6 [2] (ðŸ‰..🉑) Japanese “bargain†button..Japanese “acceptable†button
- {0x1F252, 0x1F2FF, prExtendedPictographic}, // E0.0 [174] (🉒..🋿) ..
- {0x1F300, 0x1F30C, prExtendedPictographic}, // E0.6 [13] (🌀..🌌) cyclone..milky way
- {0x1F30D, 0x1F30E, prExtendedPictographic}, // E0.7 [2] (ðŸŒ..🌎) globe showing Europe-Africa..globe showing Americas
- {0x1F30F, 0x1F30F, prExtendedPictographic}, // E0.6 [1] (ðŸŒ) globe showing Asia-Australia
- {0x1F310, 0x1F310, prExtendedPictographic}, // E1.0 [1] (ðŸŒ) globe with meridians
- {0x1F311, 0x1F311, prExtendedPictographic}, // E0.6 [1] (🌑) new moon
- {0x1F312, 0x1F312, prExtendedPictographic}, // E1.0 [1] (🌒) waxing crescent moon
- {0x1F313, 0x1F315, prExtendedPictographic}, // E0.6 [3] (🌓..🌕) first quarter moon..full moon
- {0x1F316, 0x1F318, prExtendedPictographic}, // E1.0 [3] (🌖..🌘) waning gibbous moon..waning crescent moon
- {0x1F319, 0x1F319, prExtendedPictographic}, // E0.6 [1] (🌙) crescent moon
- {0x1F31A, 0x1F31A, prExtendedPictographic}, // E1.0 [1] (🌚) new moon face
- {0x1F31B, 0x1F31B, prExtendedPictographic}, // E0.6 [1] (🌛) first quarter moon face
- {0x1F31C, 0x1F31C, prExtendedPictographic}, // E0.7 [1] (🌜) last quarter moon face
- {0x1F31D, 0x1F31E, prExtendedPictographic}, // E1.0 [2] (ðŸŒ..🌞) full moon face..sun with face
- {0x1F31F, 0x1F320, prExtendedPictographic}, // E0.6 [2] (🌟..🌠) glowing star..shooting star
- {0x1F321, 0x1F321, prExtendedPictographic}, // E0.7 [1] (🌡ï¸) thermometer
- {0x1F322, 0x1F323, prExtendedPictographic}, // E0.0 [2] (🌢..🌣) BLACK DROPLET..WHITE SUN
- {0x1F324, 0x1F32C, prExtendedPictographic}, // E0.7 [9] (🌤ï¸..🌬ï¸) sun behind small cloud..wind face
- {0x1F32D, 0x1F32F, prExtendedPictographic}, // E1.0 [3] (ðŸŒ..🌯) hot dog..burrito
- {0x1F330, 0x1F331, prExtendedPictographic}, // E0.6 [2] (🌰..🌱) chestnut..seedling
- {0x1F332, 0x1F333, prExtendedPictographic}, // E1.0 [2] (🌲..🌳) evergreen tree..deciduous tree
- {0x1F334, 0x1F335, prExtendedPictographic}, // E0.6 [2] (🌴..🌵) palm tree..cactus
- {0x1F336, 0x1F336, prExtendedPictographic}, // E0.7 [1] (🌶ï¸) hot pepper
- {0x1F337, 0x1F34A, prExtendedPictographic}, // E0.6 [20] (🌷..ðŸŠ) tulip..tangerine
- {0x1F34B, 0x1F34B, prExtendedPictographic}, // E1.0 [1] (ðŸ‹) lemon
- {0x1F34C, 0x1F34F, prExtendedPictographic}, // E0.6 [4] (ðŸŒ..ðŸ) banana..green apple
- {0x1F350, 0x1F350, prExtendedPictographic}, // E1.0 [1] (ðŸ) pear
- {0x1F351, 0x1F37B, prExtendedPictographic}, // E0.6 [43] (ðŸ‘..ðŸ») peach..clinking beer mugs
- {0x1F37C, 0x1F37C, prExtendedPictographic}, // E1.0 [1] (ðŸ¼) baby bottle
- {0x1F37D, 0x1F37D, prExtendedPictographic}, // E0.7 [1] (ðŸ½ï¸) fork and knife with plate
- {0x1F37E, 0x1F37F, prExtendedPictographic}, // E1.0 [2] (ðŸ¾..ðŸ¿) bottle with popping cork..popcorn
- {0x1F380, 0x1F393, prExtendedPictographic}, // E0.6 [20] (🎀..🎓) ribbon..graduation cap
- {0x1F394, 0x1F395, prExtendedPictographic}, // E0.0 [2] (🎔..🎕) HEART WITH TIP ON THE LEFT..BOUQUET OF FLOWERS
- {0x1F396, 0x1F397, prExtendedPictographic}, // E0.7 [2] (🎖ï¸..🎗ï¸) military medal..reminder ribbon
- {0x1F398, 0x1F398, prExtendedPictographic}, // E0.0 [1] (🎘) MUSICAL KEYBOARD WITH JACKS
- {0x1F399, 0x1F39B, prExtendedPictographic}, // E0.7 [3] (🎙ï¸..🎛ï¸) studio microphone..control knobs
- {0x1F39C, 0x1F39D, prExtendedPictographic}, // E0.0 [2] (🎜..ðŸŽ) BEAMED ASCENDING MUSICAL NOTES..BEAMED DESCENDING MUSICAL NOTES
- {0x1F39E, 0x1F39F, prExtendedPictographic}, // E0.7 [2] (🎞ï¸..🎟ï¸) film frames..admission tickets
- {0x1F3A0, 0x1F3C4, prExtendedPictographic}, // E0.6 [37] (🎠..ðŸ„) carousel horse..person surfing
- {0x1F3C5, 0x1F3C5, prExtendedPictographic}, // E1.0 [1] (ðŸ…) sports medal
- {0x1F3C6, 0x1F3C6, prExtendedPictographic}, // E0.6 [1] (ðŸ†) trophy
- {0x1F3C7, 0x1F3C7, prExtendedPictographic}, // E1.0 [1] (ðŸ‡) horse racing
- {0x1F3C8, 0x1F3C8, prExtendedPictographic}, // E0.6 [1] (ðŸˆ) american football
- {0x1F3C9, 0x1F3C9, prExtendedPictographic}, // E1.0 [1] (ðŸ‰) rugby football
- {0x1F3CA, 0x1F3CA, prExtendedPictographic}, // E0.6 [1] (ðŸŠ) person swimming
- {0x1F3CB, 0x1F3CE, prExtendedPictographic}, // E0.7 [4] (ðŸ‹ï¸..ðŸŽï¸) person lifting weights..racing car
- {0x1F3CF, 0x1F3D3, prExtendedPictographic}, // E1.0 [5] (ðŸ..ðŸ“) cricket game..ping pong
- {0x1F3D4, 0x1F3DF, prExtendedPictographic}, // E0.7 [12] (ðŸ”ï¸..ðŸŸï¸) snow-capped mountain..stadium
- {0x1F3E0, 0x1F3E3, prExtendedPictographic}, // E0.6 [4] (ðŸ ..ðŸ£) house..Japanese post office
- {0x1F3E4, 0x1F3E4, prExtendedPictographic}, // E1.0 [1] (ðŸ¤) post office
- {0x1F3E5, 0x1F3F0, prExtendedPictographic}, // E0.6 [12] (ðŸ¥..ðŸ°) hospital..castle
- {0x1F3F1, 0x1F3F2, prExtendedPictographic}, // E0.0 [2] (ðŸ±..ðŸ²) WHITE PENNANT..BLACK PENNANT
- {0x1F3F3, 0x1F3F3, prExtendedPictographic}, // E0.7 [1] (ðŸ³ï¸) white flag
- {0x1F3F4, 0x1F3F4, prExtendedPictographic}, // E1.0 [1] (ðŸ´) black flag
- {0x1F3F5, 0x1F3F5, prExtendedPictographic}, // E0.7 [1] (ðŸµï¸) rosette
- {0x1F3F6, 0x1F3F6, prExtendedPictographic}, // E0.0 [1] (ðŸ¶) BLACK ROSETTE
- {0x1F3F7, 0x1F3F7, prExtendedPictographic}, // E0.7 [1] (ðŸ·ï¸) label
- {0x1F3F8, 0x1F3FA, prExtendedPictographic}, // E1.0 [3] (ðŸ¸..ðŸº) badminton..amphora
- {0x1F3FB, 0x1F3FF, prExtend}, // Sk [5] EMOJI MODIFIER FITZPATRICK TYPE-1-2..EMOJI MODIFIER FITZPATRICK TYPE-6
- {0x1F400, 0x1F407, prExtendedPictographic}, // E1.0 [8] (ðŸ€..ðŸ‡) rat..rabbit
- {0x1F408, 0x1F408, prExtendedPictographic}, // E0.7 [1] (ðŸˆ) cat
- {0x1F409, 0x1F40B, prExtendedPictographic}, // E1.0 [3] (ðŸ‰..ðŸ‹) dragon..whale
- {0x1F40C, 0x1F40E, prExtendedPictographic}, // E0.6 [3] (ðŸŒ..ðŸŽ) snail..horse
- {0x1F40F, 0x1F410, prExtendedPictographic}, // E1.0 [2] (ðŸ..ðŸ) ram..goat
- {0x1F411, 0x1F412, prExtendedPictographic}, // E0.6 [2] (ðŸ‘..ðŸ’) ewe..monkey
- {0x1F413, 0x1F413, prExtendedPictographic}, // E1.0 [1] (ðŸ“) rooster
- {0x1F414, 0x1F414, prExtendedPictographic}, // E0.6 [1] (ðŸ”) chicken
- {0x1F415, 0x1F415, prExtendedPictographic}, // E0.7 [1] (ðŸ•) dog
- {0x1F416, 0x1F416, prExtendedPictographic}, // E1.0 [1] (ðŸ–) pig
- {0x1F417, 0x1F429, prExtendedPictographic}, // E0.6 [19] (ðŸ—..ðŸ©) boar..poodle
- {0x1F42A, 0x1F42A, prExtendedPictographic}, // E1.0 [1] (ðŸª) camel
- {0x1F42B, 0x1F43E, prExtendedPictographic}, // E0.6 [20] (ðŸ«..ðŸ¾) two-hump camel..paw prints
- {0x1F43F, 0x1F43F, prExtendedPictographic}, // E0.7 [1] (ðŸ¿ï¸) chipmunk
- {0x1F440, 0x1F440, prExtendedPictographic}, // E0.6 [1] (👀) eyes
- {0x1F441, 0x1F441, prExtendedPictographic}, // E0.7 [1] (ðŸ‘ï¸) eye
- {0x1F442, 0x1F464, prExtendedPictographic}, // E0.6 [35] (👂..👤) ear..bust in silhouette
- {0x1F465, 0x1F465, prExtendedPictographic}, // E1.0 [1] (👥) busts in silhouette
- {0x1F466, 0x1F46B, prExtendedPictographic}, // E0.6 [6] (👦..👫) boy..woman and man holding hands
- {0x1F46C, 0x1F46D, prExtendedPictographic}, // E1.0 [2] (👬..ðŸ‘) men holding hands..women holding hands
- {0x1F46E, 0x1F4AC, prExtendedPictographic}, // E0.6 [63] (👮..💬) police officer..speech balloon
- {0x1F4AD, 0x1F4AD, prExtendedPictographic}, // E1.0 [1] (ðŸ’) thought balloon
- {0x1F4AE, 0x1F4B5, prExtendedPictographic}, // E0.6 [8] (💮..💵) white flower..dollar banknote
- {0x1F4B6, 0x1F4B7, prExtendedPictographic}, // E1.0 [2] (💶..💷) euro banknote..pound banknote
- {0x1F4B8, 0x1F4EB, prExtendedPictographic}, // E0.6 [52] (💸..📫) money with wings..closed mailbox with raised flag
- {0x1F4EC, 0x1F4ED, prExtendedPictographic}, // E0.7 [2] (📬..ðŸ“) open mailbox with raised flag..open mailbox with lowered flag
- {0x1F4EE, 0x1F4EE, prExtendedPictographic}, // E0.6 [1] (📮) postbox
- {0x1F4EF, 0x1F4EF, prExtendedPictographic}, // E1.0 [1] (📯) postal horn
- {0x1F4F0, 0x1F4F4, prExtendedPictographic}, // E0.6 [5] (📰..📴) newspaper..mobile phone off
- {0x1F4F5, 0x1F4F5, prExtendedPictographic}, // E1.0 [1] (📵) no mobile phones
- {0x1F4F6, 0x1F4F7, prExtendedPictographic}, // E0.6 [2] (📶..📷) antenna bars..camera
- {0x1F4F8, 0x1F4F8, prExtendedPictographic}, // E1.0 [1] (📸) camera with flash
- {0x1F4F9, 0x1F4FC, prExtendedPictographic}, // E0.6 [4] (📹..📼) video camera..videocassette
- {0x1F4FD, 0x1F4FD, prExtendedPictographic}, // E0.7 [1] (📽ï¸) film projector
- {0x1F4FE, 0x1F4FE, prExtendedPictographic}, // E0.0 [1] (📾) PORTABLE STEREO
- {0x1F4FF, 0x1F502, prExtendedPictographic}, // E1.0 [4] (📿..🔂) prayer beads..repeat single button
- {0x1F503, 0x1F503, prExtendedPictographic}, // E0.6 [1] (🔃) clockwise vertical arrows
- {0x1F504, 0x1F507, prExtendedPictographic}, // E1.0 [4] (🔄..🔇) counterclockwise arrows button..muted speaker
- {0x1F508, 0x1F508, prExtendedPictographic}, // E0.7 [1] (🔈) speaker low volume
- {0x1F509, 0x1F509, prExtendedPictographic}, // E1.0 [1] (🔉) speaker medium volume
- {0x1F50A, 0x1F514, prExtendedPictographic}, // E0.6 [11] (🔊..🔔) speaker high volume..bell
- {0x1F515, 0x1F515, prExtendedPictographic}, // E1.0 [1] (🔕) bell with slash
- {0x1F516, 0x1F52B, prExtendedPictographic}, // E0.6 [22] (🔖..🔫) bookmark..water pistol
- {0x1F52C, 0x1F52D, prExtendedPictographic}, // E1.0 [2] (🔬..ðŸ”) microscope..telescope
- {0x1F52E, 0x1F53D, prExtendedPictographic}, // E0.6 [16] (🔮..🔽) crystal ball..downwards button
- {0x1F546, 0x1F548, prExtendedPictographic}, // E0.0 [3] (🕆..🕈) WHITE LATIN CROSS..CELTIC CROSS
- {0x1F549, 0x1F54A, prExtendedPictographic}, // E0.7 [2] (🕉ï¸..🕊ï¸) om..dove
- {0x1F54B, 0x1F54E, prExtendedPictographic}, // E1.0 [4] (🕋..🕎) kaaba..menorah
- {0x1F54F, 0x1F54F, prExtendedPictographic}, // E0.0 [1] (ðŸ•) BOWL OF HYGIEIA
- {0x1F550, 0x1F55B, prExtendedPictographic}, // E0.6 [12] (ðŸ•..🕛) one o’clock..twelve o’clock
- {0x1F55C, 0x1F567, prExtendedPictographic}, // E0.7 [12] (🕜..🕧) one-thirty..twelve-thirty
- {0x1F568, 0x1F56E, prExtendedPictographic}, // E0.0 [7] (🕨..🕮) RIGHT SPEAKER..BOOK
- {0x1F56F, 0x1F570, prExtendedPictographic}, // E0.7 [2] (🕯ï¸..🕰ï¸) candle..mantelpiece clock
- {0x1F571, 0x1F572, prExtendedPictographic}, // E0.0 [2] (🕱..🕲) BLACK SKULL AND CROSSBONES..NO PIRACY
- {0x1F573, 0x1F579, prExtendedPictographic}, // E0.7 [7] (🕳ï¸..🕹ï¸) hole..joystick
- {0x1F57A, 0x1F57A, prExtendedPictographic}, // E3.0 [1] (🕺) man dancing
- {0x1F57B, 0x1F586, prExtendedPictographic}, // E0.0 [12] (🕻..🖆) LEFT HAND TELEPHONE RECEIVER..PEN OVER STAMPED ENVELOPE
- {0x1F587, 0x1F587, prExtendedPictographic}, // E0.7 [1] (🖇ï¸) linked paperclips
- {0x1F588, 0x1F589, prExtendedPictographic}, // E0.0 [2] (🖈..🖉) BLACK PUSHPIN..LOWER LEFT PENCIL
- {0x1F58A, 0x1F58D, prExtendedPictographic}, // E0.7 [4] (🖊ï¸..ðŸ–ï¸) pen..crayon
- {0x1F58E, 0x1F58F, prExtendedPictographic}, // E0.0 [2] (🖎..ðŸ–) LEFT WRITING HAND..TURNED OK HAND SIGN
- {0x1F590, 0x1F590, prExtendedPictographic}, // E0.7 [1] (ðŸ–ï¸) hand with fingers splayed
- {0x1F591, 0x1F594, prExtendedPictographic}, // E0.0 [4] (🖑..🖔) REVERSED RAISED HAND WITH FINGERS SPLAYED..REVERSED VICTORY HAND
- {0x1F595, 0x1F596, prExtendedPictographic}, // E1.0 [2] (🖕..🖖) middle finger..vulcan salute
- {0x1F597, 0x1F5A3, prExtendedPictographic}, // E0.0 [13] (🖗..🖣) WHITE DOWN POINTING LEFT HAND INDEX..BLACK DOWN POINTING BACKHAND INDEX
- {0x1F5A4, 0x1F5A4, prExtendedPictographic}, // E3.0 [1] (🖤) black heart
- {0x1F5A5, 0x1F5A5, prExtendedPictographic}, // E0.7 [1] (🖥ï¸) desktop computer
- {0x1F5A6, 0x1F5A7, prExtendedPictographic}, // E0.0 [2] (🖦..🖧) KEYBOARD AND MOUSE..THREE NETWORKED COMPUTERS
- {0x1F5A8, 0x1F5A8, prExtendedPictographic}, // E0.7 [1] (🖨ï¸) printer
- {0x1F5A9, 0x1F5B0, prExtendedPictographic}, // E0.0 [8] (🖩..🖰) POCKET CALCULATOR..TWO BUTTON MOUSE
- {0x1F5B1, 0x1F5B2, prExtendedPictographic}, // E0.7 [2] (🖱ï¸..🖲ï¸) computer mouse..trackball
- {0x1F5B3, 0x1F5BB, prExtendedPictographic}, // E0.0 [9] (🖳..🖻) OLD PERSONAL COMPUTER..DOCUMENT WITH PICTURE
- {0x1F5BC, 0x1F5BC, prExtendedPictographic}, // E0.7 [1] (🖼ï¸) framed picture
- {0x1F5BD, 0x1F5C1, prExtendedPictographic}, // E0.0 [5] (🖽..ðŸ—) FRAME WITH TILES..OPEN FOLDER
- {0x1F5C2, 0x1F5C4, prExtendedPictographic}, // E0.7 [3] (🗂ï¸..🗄ï¸) card index dividers..file cabinet
- {0x1F5C5, 0x1F5D0, prExtendedPictographic}, // E0.0 [12] (🗅..ðŸ—) EMPTY NOTE..PAGES
- {0x1F5D1, 0x1F5D3, prExtendedPictographic}, // E0.7 [3] (🗑ï¸..🗓ï¸) wastebasket..spiral calendar
- {0x1F5D4, 0x1F5DB, prExtendedPictographic}, // E0.0 [8] (🗔..🗛) DESKTOP WINDOW..DECREASE FONT SIZE SYMBOL
- {0x1F5DC, 0x1F5DE, prExtendedPictographic}, // E0.7 [3] (🗜ï¸..🗞ï¸) clamp..rolled-up newspaper
- {0x1F5DF, 0x1F5E0, prExtendedPictographic}, // E0.0 [2] (🗟..🗠) PAGE WITH CIRCLED TEXT..STOCK CHART
- {0x1F5E1, 0x1F5E1, prExtendedPictographic}, // E0.7 [1] (🗡ï¸) dagger
- {0x1F5E2, 0x1F5E2, prExtendedPictographic}, // E0.0 [1] (🗢) LIPS
- {0x1F5E3, 0x1F5E3, prExtendedPictographic}, // E0.7 [1] (🗣ï¸) speaking head
- {0x1F5E4, 0x1F5E7, prExtendedPictographic}, // E0.0 [4] (🗤..🗧) THREE RAYS ABOVE..THREE RAYS RIGHT
- {0x1F5E8, 0x1F5E8, prExtendedPictographic}, // E2.0 [1] (🗨ï¸) left speech bubble
- {0x1F5E9, 0x1F5EE, prExtendedPictographic}, // E0.0 [6] (🗩..🗮) RIGHT SPEECH BUBBLE..LEFT ANGER BUBBLE
- {0x1F5EF, 0x1F5EF, prExtendedPictographic}, // E0.7 [1] (🗯ï¸) right anger bubble
- {0x1F5F0, 0x1F5F2, prExtendedPictographic}, // E0.0 [3] (🗰..🗲) MOOD BUBBLE..LIGHTNING MOOD
- {0x1F5F3, 0x1F5F3, prExtendedPictographic}, // E0.7 [1] (🗳ï¸) ballot box with ballot
- {0x1F5F4, 0x1F5F9, prExtendedPictographic}, // E0.0 [6] (🗴..🗹) BALLOT SCRIPT X..BALLOT BOX WITH BOLD CHECK
- {0x1F5FA, 0x1F5FA, prExtendedPictographic}, // E0.7 [1] (🗺ï¸) world map
- {0x1F5FB, 0x1F5FF, prExtendedPictographic}, // E0.6 [5] (🗻..🗿) mount fuji..moai
- {0x1F600, 0x1F600, prExtendedPictographic}, // E1.0 [1] (😀) grinning face
- {0x1F601, 0x1F606, prExtendedPictographic}, // E0.6 [6] (ðŸ˜..😆) beaming face with smiling eyes..grinning squinting face
- {0x1F607, 0x1F608, prExtendedPictographic}, // E1.0 [2] (😇..😈) smiling face with halo..smiling face with horns
- {0x1F609, 0x1F60D, prExtendedPictographic}, // E0.6 [5] (😉..ðŸ˜) winking face..smiling face with heart-eyes
- {0x1F60E, 0x1F60E, prExtendedPictographic}, // E1.0 [1] (😎) smiling face with sunglasses
- {0x1F60F, 0x1F60F, prExtendedPictographic}, // E0.6 [1] (ðŸ˜) smirking face
- {0x1F610, 0x1F610, prExtendedPictographic}, // E0.7 [1] (ðŸ˜) neutral face
- {0x1F611, 0x1F611, prExtendedPictographic}, // E1.0 [1] (😑) expressionless face
- {0x1F612, 0x1F614, prExtendedPictographic}, // E0.6 [3] (😒..😔) unamused face..pensive face
- {0x1F615, 0x1F615, prExtendedPictographic}, // E1.0 [1] (😕) confused face
- {0x1F616, 0x1F616, prExtendedPictographic}, // E0.6 [1] (😖) confounded face
- {0x1F617, 0x1F617, prExtendedPictographic}, // E1.0 [1] (😗) kissing face
- {0x1F618, 0x1F618, prExtendedPictographic}, // E0.6 [1] (😘) face blowing a kiss
- {0x1F619, 0x1F619, prExtendedPictographic}, // E1.0 [1] (😙) kissing face with smiling eyes
- {0x1F61A, 0x1F61A, prExtendedPictographic}, // E0.6 [1] (😚) kissing face with closed eyes
- {0x1F61B, 0x1F61B, prExtendedPictographic}, // E1.0 [1] (😛) face with tongue
- {0x1F61C, 0x1F61E, prExtendedPictographic}, // E0.6 [3] (😜..😞) winking face with tongue..disappointed face
- {0x1F61F, 0x1F61F, prExtendedPictographic}, // E1.0 [1] (😟) worried face
- {0x1F620, 0x1F625, prExtendedPictographic}, // E0.6 [6] (😠..😥) angry face..sad but relieved face
- {0x1F626, 0x1F627, prExtendedPictographic}, // E1.0 [2] (😦..😧) frowning face with open mouth..anguished face
- {0x1F628, 0x1F62B, prExtendedPictographic}, // E0.6 [4] (😨..😫) fearful face..tired face
- {0x1F62C, 0x1F62C, prExtendedPictographic}, // E1.0 [1] (😬) grimacing face
- {0x1F62D, 0x1F62D, prExtendedPictographic}, // E0.6 [1] (ðŸ˜) loudly crying face
- {0x1F62E, 0x1F62F, prExtendedPictographic}, // E1.0 [2] (😮..😯) face with open mouth..hushed face
- {0x1F630, 0x1F633, prExtendedPictographic}, // E0.6 [4] (😰..😳) anxious face with sweat..flushed face
- {0x1F634, 0x1F634, prExtendedPictographic}, // E1.0 [1] (😴) sleeping face
- {0x1F635, 0x1F635, prExtendedPictographic}, // E0.6 [1] (😵) face with crossed-out eyes
- {0x1F636, 0x1F636, prExtendedPictographic}, // E1.0 [1] (😶) face without mouth
- {0x1F637, 0x1F640, prExtendedPictographic}, // E0.6 [10] (😷..🙀) face with medical mask..weary cat
- {0x1F641, 0x1F644, prExtendedPictographic}, // E1.0 [4] (ðŸ™..🙄) slightly frowning face..face with rolling eyes
- {0x1F645, 0x1F64F, prExtendedPictographic}, // E0.6 [11] (🙅..ðŸ™) person gesturing NO..folded hands
- {0x1F680, 0x1F680, prExtendedPictographic}, // E0.6 [1] (🚀) rocket
- {0x1F681, 0x1F682, prExtendedPictographic}, // E1.0 [2] (ðŸš..🚂) helicopter..locomotive
- {0x1F683, 0x1F685, prExtendedPictographic}, // E0.6 [3] (🚃..🚅) railway car..bullet train
- {0x1F686, 0x1F686, prExtendedPictographic}, // E1.0 [1] (🚆) train
- {0x1F687, 0x1F687, prExtendedPictographic}, // E0.6 [1] (🚇) metro
- {0x1F688, 0x1F688, prExtendedPictographic}, // E1.0 [1] (🚈) light rail
- {0x1F689, 0x1F689, prExtendedPictographic}, // E0.6 [1] (🚉) station
- {0x1F68A, 0x1F68B, prExtendedPictographic}, // E1.0 [2] (🚊..🚋) tram..tram car
- {0x1F68C, 0x1F68C, prExtendedPictographic}, // E0.6 [1] (🚌) bus
- {0x1F68D, 0x1F68D, prExtendedPictographic}, // E0.7 [1] (ðŸš) oncoming bus
- {0x1F68E, 0x1F68E, prExtendedPictographic}, // E1.0 [1] (🚎) trolleybus
- {0x1F68F, 0x1F68F, prExtendedPictographic}, // E0.6 [1] (ðŸš) bus stop
- {0x1F690, 0x1F690, prExtendedPictographic}, // E1.0 [1] (ðŸš) minibus
- {0x1F691, 0x1F693, prExtendedPictographic}, // E0.6 [3] (🚑..🚓) ambulance..police car
- {0x1F694, 0x1F694, prExtendedPictographic}, // E0.7 [1] (🚔) oncoming police car
- {0x1F695, 0x1F695, prExtendedPictographic}, // E0.6 [1] (🚕) taxi
- {0x1F696, 0x1F696, prExtendedPictographic}, // E1.0 [1] (🚖) oncoming taxi
- {0x1F697, 0x1F697, prExtendedPictographic}, // E0.6 [1] (🚗) automobile
- {0x1F698, 0x1F698, prExtendedPictographic}, // E0.7 [1] (🚘) oncoming automobile
- {0x1F699, 0x1F69A, prExtendedPictographic}, // E0.6 [2] (🚙..🚚) sport utility vehicle..delivery truck
- {0x1F69B, 0x1F6A1, prExtendedPictographic}, // E1.0 [7] (🚛..🚡) articulated lorry..aerial tramway
- {0x1F6A2, 0x1F6A2, prExtendedPictographic}, // E0.6 [1] (🚢) ship
- {0x1F6A3, 0x1F6A3, prExtendedPictographic}, // E1.0 [1] (🚣) person rowing boat
- {0x1F6A4, 0x1F6A5, prExtendedPictographic}, // E0.6 [2] (🚤..🚥) speedboat..horizontal traffic light
- {0x1F6A6, 0x1F6A6, prExtendedPictographic}, // E1.0 [1] (🚦) vertical traffic light
- {0x1F6A7, 0x1F6AD, prExtendedPictographic}, // E0.6 [7] (🚧..ðŸš) construction..no smoking
- {0x1F6AE, 0x1F6B1, prExtendedPictographic}, // E1.0 [4] (🚮..🚱) litter in bin sign..non-potable water
- {0x1F6B2, 0x1F6B2, prExtendedPictographic}, // E0.6 [1] (🚲) bicycle
- {0x1F6B3, 0x1F6B5, prExtendedPictographic}, // E1.0 [3] (🚳..🚵) no bicycles..person mountain biking
- {0x1F6B6, 0x1F6B6, prExtendedPictographic}, // E0.6 [1] (🚶) person walking
- {0x1F6B7, 0x1F6B8, prExtendedPictographic}, // E1.0 [2] (🚷..🚸) no pedestrians..children crossing
- {0x1F6B9, 0x1F6BE, prExtendedPictographic}, // E0.6 [6] (🚹..🚾) men’s room..water closet
- {0x1F6BF, 0x1F6BF, prExtendedPictographic}, // E1.0 [1] (🚿) shower
- {0x1F6C0, 0x1F6C0, prExtendedPictographic}, // E0.6 [1] (🛀) person taking bath
- {0x1F6C1, 0x1F6C5, prExtendedPictographic}, // E1.0 [5] (ðŸ›..🛅) bathtub..left luggage
- {0x1F6C6, 0x1F6CA, prExtendedPictographic}, // E0.0 [5] (🛆..🛊) TRIANGLE WITH ROUNDED CORNERS..GIRLS SYMBOL
- {0x1F6CB, 0x1F6CB, prExtendedPictographic}, // E0.7 [1] (🛋ï¸) couch and lamp
- {0x1F6CC, 0x1F6CC, prExtendedPictographic}, // E1.0 [1] (🛌) person in bed
- {0x1F6CD, 0x1F6CF, prExtendedPictographic}, // E0.7 [3] (ðŸ›ï¸..ðŸ›ï¸) shopping bags..bed
- {0x1F6D0, 0x1F6D0, prExtendedPictographic}, // E1.0 [1] (ðŸ›) place of worship
- {0x1F6D1, 0x1F6D2, prExtendedPictographic}, // E3.0 [2] (🛑..🛒) stop sign..shopping cart
- {0x1F6D3, 0x1F6D4, prExtendedPictographic}, // E0.0 [2] (🛓..🛔) STUPA..PAGODA
- {0x1F6D5, 0x1F6D5, prExtendedPictographic}, // E12.0 [1] (🛕) hindu temple
- {0x1F6D6, 0x1F6D7, prExtendedPictographic}, // E13.0 [2] (🛖..🛗) hut..elevator
- {0x1F6D8, 0x1F6DC, prExtendedPictographic}, // E0.0 [5] (🛘..🛜) ..
- {0x1F6DD, 0x1F6DF, prExtendedPictographic}, // E14.0 [3] (ðŸ›..🛟) playground slide..ring buoy
- {0x1F6E0, 0x1F6E5, prExtendedPictographic}, // E0.7 [6] (🛠ï¸..🛥ï¸) hammer and wrench..motor boat
- {0x1F6E6, 0x1F6E8, prExtendedPictographic}, // E0.0 [3] (🛦..🛨) UP-POINTING MILITARY AIRPLANE..UP-POINTING SMALL AIRPLANE
- {0x1F6E9, 0x1F6E9, prExtendedPictographic}, // E0.7 [1] (🛩ï¸) small airplane
- {0x1F6EA, 0x1F6EA, prExtendedPictographic}, // E0.0 [1] (🛪) NORTHEAST-POINTING AIRPLANE
- {0x1F6EB, 0x1F6EC, prExtendedPictographic}, // E1.0 [2] (🛫..🛬) airplane departure..airplane arrival
- {0x1F6ED, 0x1F6EF, prExtendedPictographic}, // E0.0 [3] (ðŸ›..🛯) ..
- {0x1F6F0, 0x1F6F0, prExtendedPictographic}, // E0.7 [1] (🛰ï¸) satellite
- {0x1F6F1, 0x1F6F2, prExtendedPictographic}, // E0.0 [2] (🛱..🛲) ONCOMING FIRE ENGINE..DIESEL LOCOMOTIVE
- {0x1F6F3, 0x1F6F3, prExtendedPictographic}, // E0.7 [1] (🛳ï¸) passenger ship
- {0x1F6F4, 0x1F6F6, prExtendedPictographic}, // E3.0 [3] (🛴..🛶) kick scooter..canoe
- {0x1F6F7, 0x1F6F8, prExtendedPictographic}, // E5.0 [2] (🛷..🛸) sled..flying saucer
- {0x1F6F9, 0x1F6F9, prExtendedPictographic}, // E11.0 [1] (🛹) skateboard
- {0x1F6FA, 0x1F6FA, prExtendedPictographic}, // E12.0 [1] (🛺) auto rickshaw
- {0x1F6FB, 0x1F6FC, prExtendedPictographic}, // E13.0 [2] (🛻..🛼) pickup truck..roller skate
- {0x1F6FD, 0x1F6FF, prExtendedPictographic}, // E0.0 [3] (🛽..🛿) ..
- {0x1F774, 0x1F77F, prExtendedPictographic}, // E0.0 [12] (ðŸ´..ðŸ¿) ..
- {0x1F7D5, 0x1F7DF, prExtendedPictographic}, // E0.0 [11] (🟕..🟟) CIRCLED TRIANGLE..
- {0x1F7E0, 0x1F7EB, prExtendedPictographic}, // E12.0 [12] (🟠..🟫) orange circle..brown square
- {0x1F7EC, 0x1F7EF, prExtendedPictographic}, // E0.0 [4] (🟬..🟯) ..
- {0x1F7F0, 0x1F7F0, prExtendedPictographic}, // E14.0 [1] (🟰) heavy equals sign
- {0x1F7F1, 0x1F7FF, prExtendedPictographic}, // E0.0 [15] (🟱..🟿) ..
- {0x1F80C, 0x1F80F, prExtendedPictographic}, // E0.0 [4] (🠌..ðŸ ) ..
- {0x1F848, 0x1F84F, prExtendedPictographic}, // E0.0 [8] (🡈..ðŸ¡) ..
- {0x1F85A, 0x1F85F, prExtendedPictographic}, // E0.0 [6] (🡚..🡟) ..
- {0x1F888, 0x1F88F, prExtendedPictographic}, // E0.0 [8] (🢈..ðŸ¢) ..
- {0x1F8AE, 0x1F8FF, prExtendedPictographic}, // E0.0 [82] (🢮..🣿) ..
- {0x1F90C, 0x1F90C, prExtendedPictographic}, // E13.0 [1] (🤌) pinched fingers
- {0x1F90D, 0x1F90F, prExtendedPictographic}, // E12.0 [3] (ðŸ¤..ðŸ¤) white heart..pinching hand
- {0x1F910, 0x1F918, prExtendedPictographic}, // E1.0 [9] (ðŸ¤..🤘) zipper-mouth face..sign of the horns
- {0x1F919, 0x1F91E, prExtendedPictographic}, // E3.0 [6] (🤙..🤞) call me hand..crossed fingers
- {0x1F91F, 0x1F91F, prExtendedPictographic}, // E5.0 [1] (🤟) love-you gesture
- {0x1F920, 0x1F927, prExtendedPictographic}, // E3.0 [8] (🤠..🤧) cowboy hat face..sneezing face
- {0x1F928, 0x1F92F, prExtendedPictographic}, // E5.0 [8] (🤨..🤯) face with raised eyebrow..exploding head
- {0x1F930, 0x1F930, prExtendedPictographic}, // E3.0 [1] (🤰) pregnant woman
- {0x1F931, 0x1F932, prExtendedPictographic}, // E5.0 [2] (🤱..🤲) breast-feeding..palms up together
- {0x1F933, 0x1F93A, prExtendedPictographic}, // E3.0 [8] (🤳..🤺) selfie..person fencing
- {0x1F93C, 0x1F93E, prExtendedPictographic}, // E3.0 [3] (🤼..🤾) people wrestling..person playing handball
- {0x1F93F, 0x1F93F, prExtendedPictographic}, // E12.0 [1] (🤿) diving mask
- {0x1F940, 0x1F945, prExtendedPictographic}, // E3.0 [6] (🥀..🥅) wilted flower..goal net
- {0x1F947, 0x1F94B, prExtendedPictographic}, // E3.0 [5] (🥇..🥋) 1st place medal..martial arts uniform
- {0x1F94C, 0x1F94C, prExtendedPictographic}, // E5.0 [1] (🥌) curling stone
- {0x1F94D, 0x1F94F, prExtendedPictographic}, // E11.0 [3] (ðŸ¥..ðŸ¥) lacrosse..flying disc
- {0x1F950, 0x1F95E, prExtendedPictographic}, // E3.0 [15] (ðŸ¥..🥞) croissant..pancakes
- {0x1F95F, 0x1F96B, prExtendedPictographic}, // E5.0 [13] (🥟..🥫) dumpling..canned food
- {0x1F96C, 0x1F970, prExtendedPictographic}, // E11.0 [5] (🥬..🥰) leafy green..smiling face with hearts
- {0x1F971, 0x1F971, prExtendedPictographic}, // E12.0 [1] (🥱) yawning face
- {0x1F972, 0x1F972, prExtendedPictographic}, // E13.0 [1] (🥲) smiling face with tear
- {0x1F973, 0x1F976, prExtendedPictographic}, // E11.0 [4] (🥳..🥶) partying face..cold face
- {0x1F977, 0x1F978, prExtendedPictographic}, // E13.0 [2] (🥷..🥸) ninja..disguised face
- {0x1F979, 0x1F979, prExtendedPictographic}, // E14.0 [1] (🥹) face holding back tears
- {0x1F97A, 0x1F97A, prExtendedPictographic}, // E11.0 [1] (🥺) pleading face
- {0x1F97B, 0x1F97B, prExtendedPictographic}, // E12.0 [1] (🥻) sari
- {0x1F97C, 0x1F97F, prExtendedPictographic}, // E11.0 [4] (🥼..🥿) lab coat..flat shoe
- {0x1F980, 0x1F984, prExtendedPictographic}, // E1.0 [5] (🦀..🦄) crab..unicorn
- {0x1F985, 0x1F991, prExtendedPictographic}, // E3.0 [13] (🦅..🦑) eagle..squid
- {0x1F992, 0x1F997, prExtendedPictographic}, // E5.0 [6] (🦒..🦗) giraffe..cricket
- {0x1F998, 0x1F9A2, prExtendedPictographic}, // E11.0 [11] (🦘..🦢) kangaroo..swan
- {0x1F9A3, 0x1F9A4, prExtendedPictographic}, // E13.0 [2] (🦣..🦤) mammoth..dodo
- {0x1F9A5, 0x1F9AA, prExtendedPictographic}, // E12.0 [6] (🦥..🦪) sloth..oyster
- {0x1F9AB, 0x1F9AD, prExtendedPictographic}, // E13.0 [3] (🦫..ðŸ¦) beaver..seal
- {0x1F9AE, 0x1F9AF, prExtendedPictographic}, // E12.0 [2] (🦮..🦯) guide dog..white cane
- {0x1F9B0, 0x1F9B9, prExtendedPictographic}, // E11.0 [10] (🦰..🦹) red hair..supervillain
- {0x1F9BA, 0x1F9BF, prExtendedPictographic}, // E12.0 [6] (🦺..🦿) safety vest..mechanical leg
- {0x1F9C0, 0x1F9C0, prExtendedPictographic}, // E1.0 [1] (🧀) cheese wedge
- {0x1F9C1, 0x1F9C2, prExtendedPictographic}, // E11.0 [2] (ðŸ§..🧂) cupcake..salt
- {0x1F9C3, 0x1F9CA, prExtendedPictographic}, // E12.0 [8] (🧃..🧊) beverage box..ice
- {0x1F9CB, 0x1F9CB, prExtendedPictographic}, // E13.0 [1] (🧋) bubble tea
- {0x1F9CC, 0x1F9CC, prExtendedPictographic}, // E14.0 [1] (🧌) troll
- {0x1F9CD, 0x1F9CF, prExtendedPictographic}, // E12.0 [3] (ðŸ§..ðŸ§) person standing..deaf person
- {0x1F9D0, 0x1F9E6, prExtendedPictographic}, // E5.0 [23] (ðŸ§..🧦) face with monocle..socks
- {0x1F9E7, 0x1F9FF, prExtendedPictographic}, // E11.0 [25] (🧧..🧿) red envelope..nazar amulet
- {0x1FA00, 0x1FA6F, prExtendedPictographic}, // E0.0 [112] (🨀..🩯) NEUTRAL CHESS KING..
- {0x1FA70, 0x1FA73, prExtendedPictographic}, // E12.0 [4] (🩰..🩳) ballet shoes..shorts
- {0x1FA74, 0x1FA74, prExtendedPictographic}, // E13.0 [1] (🩴) thong sandal
- {0x1FA75, 0x1FA77, prExtendedPictographic}, // E0.0 [3] (🩵..🩷) ..
- {0x1FA78, 0x1FA7A, prExtendedPictographic}, // E12.0 [3] (🩸..🩺) drop of blood..stethoscope
- {0x1FA7B, 0x1FA7C, prExtendedPictographic}, // E14.0 [2] (🩻..🩼) x-ray..crutch
- {0x1FA7D, 0x1FA7F, prExtendedPictographic}, // E0.0 [3] (🩽..🩿) ..
- {0x1FA80, 0x1FA82, prExtendedPictographic}, // E12.0 [3] (🪀..🪂) yo-yo..parachute
- {0x1FA83, 0x1FA86, prExtendedPictographic}, // E13.0 [4] (🪃..🪆) boomerang..nesting dolls
- {0x1FA87, 0x1FA8F, prExtendedPictographic}, // E0.0 [9] (🪇..ðŸª) ..
- {0x1FA90, 0x1FA95, prExtendedPictographic}, // E12.0 [6] (ðŸª..🪕) ringed planet..banjo
- {0x1FA96, 0x1FAA8, prExtendedPictographic}, // E13.0 [19] (🪖..🪨) military helmet..rock
- {0x1FAA9, 0x1FAAC, prExtendedPictographic}, // E14.0 [4] (🪩..🪬) mirror ball..hamsa
- {0x1FAAD, 0x1FAAF, prExtendedPictographic}, // E0.0 [3] (ðŸª..🪯) ..
- {0x1FAB0, 0x1FAB6, prExtendedPictographic}, // E13.0 [7] (🪰..🪶) fly..feather
- {0x1FAB7, 0x1FABA, prExtendedPictographic}, // E14.0 [4] (🪷..🪺) lotus..nest with eggs
- {0x1FABB, 0x1FABF, prExtendedPictographic}, // E0.0 [5] (🪻..🪿) ..
- {0x1FAC0, 0x1FAC2, prExtendedPictographic}, // E13.0 [3] (🫀..🫂) anatomical heart..people hugging
- {0x1FAC3, 0x1FAC5, prExtendedPictographic}, // E14.0 [3] (🫃..🫅) pregnant man..person with crown
- {0x1FAC6, 0x1FACF, prExtendedPictographic}, // E0.0 [10] (🫆..ðŸ«) ..
- {0x1FAD0, 0x1FAD6, prExtendedPictographic}, // E13.0 [7] (ðŸ«..🫖) blueberries..teapot
- {0x1FAD7, 0x1FAD9, prExtendedPictographic}, // E14.0 [3] (🫗..🫙) pouring liquid..jar
- {0x1FADA, 0x1FADF, prExtendedPictographic}, // E0.0 [6] (🫚..🫟) ..
- {0x1FAE0, 0x1FAE7, prExtendedPictographic}, // E14.0 [8] (🫠..🫧) melting face..bubbles
- {0x1FAE8, 0x1FAEF, prExtendedPictographic}, // E0.0 [8] (🫨..🫯) ..
- {0x1FAF0, 0x1FAF6, prExtendedPictographic}, // E14.0 [7] (🫰..🫶) hand with index finger and thumb crossed..heart hands
- {0x1FAF7, 0x1FAFF, prExtendedPictographic}, // E0.0 [9] (🫷..🫿) ..
- {0x1FC00, 0x1FFFD, prExtendedPictographic}, // E0.0[1022] (🰀..🿽) ..
- {0xE0000, 0xE0000, prControl}, // Cn
- {0xE0001, 0xE0001, prControl}, // Cf LANGUAGE TAG
- {0xE0002, 0xE001F, prControl}, // Cn [30] ..
- {0xE0020, 0xE007F, prExtend}, // Cf [96] TAG SPACE..CANCEL TAG
- {0xE0080, 0xE00FF, prControl}, // Cn [128] ..
- {0xE0100, 0xE01EF, prExtend}, // Mn [240] VARIATION SELECTOR-17..VARIATION SELECTOR-256
- {0xE01F0, 0xE0FFF, prControl}, // Cn [3600] ..
-}
diff --git a/vendor/github.com/rivo/uniseg/graphemerules.go b/vendor/github.com/rivo/uniseg/graphemerules.go
deleted file mode 100644
index 907b30bd..00000000
--- a/vendor/github.com/rivo/uniseg/graphemerules.go
+++ /dev/null
@@ -1,138 +0,0 @@
-package uniseg
-
-// The states of the grapheme cluster parser.
-const (
- grAny = iota
- grCR
- grControlLF
- grL
- grLVV
- grLVTT
- grPrepend
- grExtendedPictographic
- grExtendedPictographicZWJ
- grRIOdd
- grRIEven
-)
-
-// The grapheme cluster parser's breaking instructions.
-const (
- grNoBoundary = iota
- grBoundary
-)
-
-// The grapheme cluster parser's state transitions. Maps (state, property) to
-// (new state, breaking instruction, rule number). The breaking instruction
-// always refers to the boundary between the last and next code point.
-//
-// This map is queried as follows:
-//
-// 1. Find specific state + specific property. Stop if found.
-// 2. Find specific state + any property.
-// 3. Find any state + specific property.
-// 4. If only (2) or (3) (but not both) was found, stop.
-// 5. If both (2) and (3) were found, use state from (3) and breaking instruction
-// from the transition with the lower rule number, prefer (3) if rule numbers
-// are equal. Stop.
-// 6. Assume grAny and grBoundary.
-//
-// Unicode version 14.0.0.
-var grTransitions = map[[2]int][3]int{
- // GB5
- {grAny, prCR}: {grCR, grBoundary, 50},
- {grAny, prLF}: {grControlLF, grBoundary, 50},
- {grAny, prControl}: {grControlLF, grBoundary, 50},
-
- // GB4
- {grCR, prAny}: {grAny, grBoundary, 40},
- {grControlLF, prAny}: {grAny, grBoundary, 40},
-
- // GB3.
- {grCR, prLF}: {grAny, grNoBoundary, 30},
-
- // GB6.
- {grAny, prL}: {grL, grBoundary, 9990},
- {grL, prL}: {grL, grNoBoundary, 60},
- {grL, prV}: {grLVV, grNoBoundary, 60},
- {grL, prLV}: {grLVV, grNoBoundary, 60},
- {grL, prLVT}: {grLVTT, grNoBoundary, 60},
-
- // GB7.
- {grAny, prLV}: {grLVV, grBoundary, 9990},
- {grAny, prV}: {grLVV, grBoundary, 9990},
- {grLVV, prV}: {grLVV, grNoBoundary, 70},
- {grLVV, prT}: {grLVTT, grNoBoundary, 70},
-
- // GB8.
- {grAny, prLVT}: {grLVTT, grBoundary, 9990},
- {grAny, prT}: {grLVTT, grBoundary, 9990},
- {grLVTT, prT}: {grLVTT, grNoBoundary, 80},
-
- // GB9.
- {grAny, prExtend}: {grAny, grNoBoundary, 90},
- {grAny, prZWJ}: {grAny, grNoBoundary, 90},
-
- // GB9a.
- {grAny, prSpacingMark}: {grAny, grNoBoundary, 91},
-
- // GB9b.
- {grAny, prPrepend}: {grPrepend, grBoundary, 9990},
- {grPrepend, prAny}: {grAny, grNoBoundary, 92},
-
- // GB11.
- {grAny, prExtendedPictographic}: {grExtendedPictographic, grBoundary, 9990},
- {grExtendedPictographic, prExtend}: {grExtendedPictographic, grNoBoundary, 110},
- {grExtendedPictographic, prZWJ}: {grExtendedPictographicZWJ, grNoBoundary, 110},
- {grExtendedPictographicZWJ, prExtendedPictographic}: {grExtendedPictographic, grNoBoundary, 110},
-
- // GB12 / GB13.
- {grAny, prRegionalIndicator}: {grRIOdd, grBoundary, 9990},
- {grRIOdd, prRegionalIndicator}: {grRIEven, grNoBoundary, 120},
- {grRIEven, prRegionalIndicator}: {grRIOdd, grBoundary, 120},
-}
-
-// transitionGraphemeState determines the new state of the grapheme cluster
-// parser given the current state and the next code point. It also returns the
-// code point's grapheme property (the value mapped by the [graphemeCodePoints]
-// table) and whether a cluster boundary was detected.
-func transitionGraphemeState(state int, r rune) (newState, prop int, boundary bool) {
- // Determine the property of the next character.
- prop = property(graphemeCodePoints, r)
-
- // Find the applicable transition.
- transition, ok := grTransitions[[2]int{state, prop}]
- if ok {
- // We have a specific transition. We'll use it.
- return transition[0], prop, transition[1] == grBoundary
- }
-
- // No specific transition found. Try the less specific ones.
- transAnyProp, okAnyProp := grTransitions[[2]int{state, prAny}]
- transAnyState, okAnyState := grTransitions[[2]int{grAny, prop}]
- if okAnyProp && okAnyState {
- // Both apply. We'll use a mix (see comments for grTransitions).
- newState = transAnyState[0]
- boundary = transAnyState[1] == grBoundary
- if transAnyProp[2] < transAnyState[2] {
- boundary = transAnyProp[1] == grBoundary
- }
- return
- }
-
- if okAnyProp {
- // We only have a specific state.
- return transAnyProp[0], prop, transAnyProp[1] == grBoundary
- // This branch will probably never be reached because okAnyState will
- // always be true given the current transition map. But we keep it here
- // for future modifications to the transition map where this may not be
- // true anymore.
- }
-
- if okAnyState {
- // We only have a specific property.
- return transAnyState[0], prop, transAnyState[1] == grBoundary
- }
-
- // No known transition. GB999: Any ÷ Any.
- return grAny, prop, true
-}
diff --git a/vendor/github.com/rivo/uniseg/line.go b/vendor/github.com/rivo/uniseg/line.go
deleted file mode 100644
index c0398cac..00000000
--- a/vendor/github.com/rivo/uniseg/line.go
+++ /dev/null
@@ -1,131 +0,0 @@
-package uniseg
-
-import "unicode/utf8"
-
-// FirstLineSegment returns the prefix of the given byte slice after which a
-// decision to break the string over to the next line can or must be made,
-// according to the rules of Unicode Standard Annex #14. This is used to
-// implement line breaking.
-//
-// Line breaking, also known as word wrapping, is the process of breaking a
-// section of text into lines such that it will fit in the available width of a
-// page, window or other display area.
-//
-// The returned "segment" may not be broken into smaller parts, unless no other
-// breaking opportunities present themselves, in which case you may break by
-// grapheme clusters (using the [FirstGraphemeCluster] function to determine the
-// grapheme clusters).
-//
-// The "mustBreak" flag indicates whether you MUST break the line after the
-// given segment (true), for example after newline characters, or you MAY break
-// the line after the given segment (false).
-//
-// This function can be called continuously to extract all non-breaking sub-sets
-// from a byte slice, as illustrated in the example below.
-//
-// If you don't know the current state, for example when calling the function
-// for the first time, you must pass -1. For consecutive calls, pass the state
-// and rest slice returned by the previous call.
-//
-// The "rest" slice is the sub-slice of the original byte slice "b" starting
-// after the last byte of the identified line segment. If the length of the
-// "rest" slice is 0, the entire byte slice "b" has been processed. The
-// "segment" byte slice is the sub-slice of the input slice containing the
-// identified line segment.
-//
-// Given an empty byte slice "b", the function returns nil values.
-//
-// Note that in accordance with UAX #14 LB3, the final segment will end with
-// "mustBreak" set to true. You can choose to ignore this by checking if the
-// length of the "rest" slice is 0 and calling [HasTrailingLineBreak] or
-// [HasTrailingLineBreakInString] on the last rune.
-//
-// Note also that this algorithm may break within grapheme clusters. This is
-// addressed in Section 8.2 Example 6 of UAX #14. To avoid this, you can use
-// the [Step] function instead.
-func FirstLineSegment(b []byte, state int) (segment, rest []byte, mustBreak bool, newState int) {
- // An empty byte slice returns nothing.
- if len(b) == 0 {
- return
- }
-
- // Extract the first rune.
- r, length := utf8.DecodeRune(b)
- if len(b) <= length { // If we're already past the end, there is nothing else to parse.
- return b, nil, true, lbAny // LB3.
- }
-
- // If we don't know the state, determine it now.
- if state < 0 {
- state, _ = transitionLineBreakState(state, r, b[length:], "")
- }
-
- // Transition until we find a boundary.
- var boundary int
- for {
- r, l := utf8.DecodeRune(b[length:])
- state, boundary = transitionLineBreakState(state, r, b[length+l:], "")
-
- if boundary != LineDontBreak {
- return b[:length], b[length:], boundary == LineMustBreak, state
- }
-
- length += l
- if len(b) <= length {
- return b, nil, true, lbAny // LB3
- }
- }
-}
-
-// FirstLineSegmentInString is like FirstLineSegment() but its input and outputs
-// are strings.
-func FirstLineSegmentInString(str string, state int) (segment, rest string, mustBreak bool, newState int) {
- // An empty byte slice returns nothing.
- if len(str) == 0 {
- return
- }
-
- // Extract the first rune.
- r, length := utf8.DecodeRuneInString(str)
- if len(str) <= length { // If we're already past the end, there is nothing else to parse.
- return str, "", true, lbAny // LB3.
- }
-
- // If we don't know the state, determine it now.
- if state < 0 {
- state, _ = transitionLineBreakState(state, r, nil, str[length:])
- }
-
- // Transition until we find a boundary.
- var boundary int
- for {
- r, l := utf8.DecodeRuneInString(str[length:])
- state, boundary = transitionLineBreakState(state, r, nil, str[length+l:])
-
- if boundary != LineDontBreak {
- return str[:length], str[length:], boundary == LineMustBreak, state
- }
-
- length += l
- if len(str) <= length {
- return str, "", true, lbAny // LB3.
- }
- }
-}
-
-// HasTrailingLineBreak returns true if the last rune in the given byte slice is
-// one of the hard line break code points defined in LB4 and LB5 of [UAX #14].
-//
-// [UAX #14]: https://www.unicode.org/reports/tr14/#Algorithm
-func HasTrailingLineBreak(b []byte) bool {
- r, _ := utf8.DecodeLastRune(b)
- property, _ := propertyWithGenCat(lineBreakCodePoints, r)
- return property == lbBK || property == lbCR || property == lbLF || property == lbNL
-}
-
-// HasTrailingLineBreakInString is like [HasTrailingLineBreak] but for a string.
-func HasTrailingLineBreakInString(str string) bool {
- r, _ := utf8.DecodeLastRuneInString(str)
- property, _ := propertyWithGenCat(lineBreakCodePoints, r)
- return property == lbBK || property == lbCR || property == lbLF || property == lbNL
-}
diff --git a/vendor/github.com/rivo/uniseg/lineproperties.go b/vendor/github.com/rivo/uniseg/lineproperties.go
deleted file mode 100644
index 32169306..00000000
--- a/vendor/github.com/rivo/uniseg/lineproperties.go
+++ /dev/null
@@ -1,3513 +0,0 @@
-package uniseg
-
-// Code generated via go generate from gen_properties.go. DO NOT EDIT.
-
-// lineBreakCodePoints are taken from
-// https://www.unicode.org/Public/14.0.0/ucd/LineBreak.txt
-// and
-// https://unicode.org/Public/14.0.0/ucd/emoji/emoji-data.txt
-// ("Extended_Pictographic" only)
-// on September 10, 2022. See https://www.unicode.org/license.html for the Unicode
-// license agreement.
-var lineBreakCodePoints = [][4]int{
- {0x0000, 0x0008, prCM, gcCc}, // [9] ..
- {0x0009, 0x0009, prBA, gcCc}, //
- {0x000A, 0x000A, prLF, gcCc}, //
- {0x000B, 0x000C, prBK, gcCc}, // [2] ..
- {0x000D, 0x000D, prCR, gcCc}, //
- {0x000E, 0x001F, prCM, gcCc}, // [18] ..
- {0x0020, 0x0020, prSP, gcZs}, // SPACE
- {0x0021, 0x0021, prEX, gcPo}, // EXCLAMATION MARK
- {0x0022, 0x0022, prQU, gcPo}, // QUOTATION MARK
- {0x0023, 0x0023, prAL, gcPo}, // NUMBER SIGN
- {0x0024, 0x0024, prPR, gcSc}, // DOLLAR SIGN
- {0x0025, 0x0025, prPO, gcPo}, // PERCENT SIGN
- {0x0026, 0x0026, prAL, gcPo}, // AMPERSAND
- {0x0027, 0x0027, prQU, gcPo}, // APOSTROPHE
- {0x0028, 0x0028, prOP, gcPs}, // LEFT PARENTHESIS
- {0x0029, 0x0029, prCP, gcPe}, // RIGHT PARENTHESIS
- {0x002A, 0x002A, prAL, gcPo}, // ASTERISK
- {0x002B, 0x002B, prPR, gcSm}, // PLUS SIGN
- {0x002C, 0x002C, prIS, gcPo}, // COMMA
- {0x002D, 0x002D, prHY, gcPd}, // HYPHEN-MINUS
- {0x002E, 0x002E, prIS, gcPo}, // FULL STOP
- {0x002F, 0x002F, prSY, gcPo}, // SOLIDUS
- {0x0030, 0x0039, prNU, gcNd}, // [10] DIGIT ZERO..DIGIT NINE
- {0x003A, 0x003B, prIS, gcPo}, // [2] COLON..SEMICOLON
- {0x003C, 0x003E, prAL, gcSm}, // [3] LESS-THAN SIGN..GREATER-THAN SIGN
- {0x003F, 0x003F, prEX, gcPo}, // QUESTION MARK
- {0x0040, 0x0040, prAL, gcPo}, // COMMERCIAL AT
- {0x0041, 0x005A, prAL, gcLu}, // [26] LATIN CAPITAL LETTER A..LATIN CAPITAL LETTER Z
- {0x005B, 0x005B, prOP, gcPs}, // LEFT SQUARE BRACKET
- {0x005C, 0x005C, prPR, gcPo}, // REVERSE SOLIDUS
- {0x005D, 0x005D, prCP, gcPe}, // RIGHT SQUARE BRACKET
- {0x005E, 0x005E, prAL, gcSk}, // CIRCUMFLEX ACCENT
- {0x005F, 0x005F, prAL, gcPc}, // LOW LINE
- {0x0060, 0x0060, prAL, gcSk}, // GRAVE ACCENT
- {0x0061, 0x007A, prAL, gcLl}, // [26] LATIN SMALL LETTER A..LATIN SMALL LETTER Z
- {0x007B, 0x007B, prOP, gcPs}, // LEFT CURLY BRACKET
- {0x007C, 0x007C, prBA, gcSm}, // VERTICAL LINE
- {0x007D, 0x007D, prCL, gcPe}, // RIGHT CURLY BRACKET
- {0x007E, 0x007E, prAL, gcSm}, // TILDE
- {0x007F, 0x007F, prCM, gcCc}, //
- {0x0080, 0x0084, prCM, gcCc}, // [5] ..
- {0x0085, 0x0085, prNL, gcCc}, //
- {0x0086, 0x009F, prCM, gcCc}, // [26] ..
- {0x00A0, 0x00A0, prGL, gcZs}, // NO-BREAK SPACE
- {0x00A1, 0x00A1, prOP, gcPo}, // INVERTED EXCLAMATION MARK
- {0x00A2, 0x00A2, prPO, gcSc}, // CENT SIGN
- {0x00A3, 0x00A5, prPR, gcSc}, // [3] POUND SIGN..YEN SIGN
- {0x00A6, 0x00A6, prAL, gcSo}, // BROKEN BAR
- {0x00A7, 0x00A7, prAI, gcPo}, // SECTION SIGN
- {0x00A8, 0x00A8, prAI, gcSk}, // DIAERESIS
- {0x00A9, 0x00A9, prAL, gcSo}, // COPYRIGHT SIGN
- {0x00AA, 0x00AA, prAI, gcLo}, // FEMININE ORDINAL INDICATOR
- {0x00AB, 0x00AB, prQU, gcPi}, // LEFT-POINTING DOUBLE ANGLE QUOTATION MARK
- {0x00AC, 0x00AC, prAL, gcSm}, // NOT SIGN
- {0x00AD, 0x00AD, prBA, gcCf}, // SOFT HYPHEN
- {0x00AE, 0x00AE, prAL, gcSo}, // REGISTERED SIGN
- {0x00AF, 0x00AF, prAL, gcSk}, // MACRON
- {0x00B0, 0x00B0, prPO, gcSo}, // DEGREE SIGN
- {0x00B1, 0x00B1, prPR, gcSm}, // PLUS-MINUS SIGN
- {0x00B2, 0x00B3, prAI, gcNo}, // [2] SUPERSCRIPT TWO..SUPERSCRIPT THREE
- {0x00B4, 0x00B4, prBB, gcSk}, // ACUTE ACCENT
- {0x00B5, 0x00B5, prAL, gcLl}, // MICRO SIGN
- {0x00B6, 0x00B7, prAI, gcPo}, // [2] PILCROW SIGN..MIDDLE DOT
- {0x00B8, 0x00B8, prAI, gcSk}, // CEDILLA
- {0x00B9, 0x00B9, prAI, gcNo}, // SUPERSCRIPT ONE
- {0x00BA, 0x00BA, prAI, gcLo}, // MASCULINE ORDINAL INDICATOR
- {0x00BB, 0x00BB, prQU, gcPf}, // RIGHT-POINTING DOUBLE ANGLE QUOTATION MARK
- {0x00BC, 0x00BE, prAI, gcNo}, // [3] VULGAR FRACTION ONE QUARTER..VULGAR FRACTION THREE QUARTERS
- {0x00BF, 0x00BF, prOP, gcPo}, // INVERTED QUESTION MARK
- {0x00C0, 0x00D6, prAL, gcLu}, // [23] LATIN CAPITAL LETTER A WITH GRAVE..LATIN CAPITAL LETTER O WITH DIAERESIS
- {0x00D7, 0x00D7, prAI, gcSm}, // MULTIPLICATION SIGN
- {0x00D8, 0x00F6, prAL, gcLC}, // [31] LATIN CAPITAL LETTER O WITH STROKE..LATIN SMALL LETTER O WITH DIAERESIS
- {0x00F7, 0x00F7, prAI, gcSm}, // DIVISION SIGN
- {0x00F8, 0x00FF, prAL, gcLl}, // [8] LATIN SMALL LETTER O WITH STROKE..LATIN SMALL LETTER Y WITH DIAERESIS
- {0x0100, 0x017F, prAL, gcLC}, // [128] LATIN CAPITAL LETTER A WITH MACRON..LATIN SMALL LETTER LONG S
- {0x0180, 0x01BA, prAL, gcLC}, // [59] LATIN SMALL LETTER B WITH STROKE..LATIN SMALL LETTER EZH WITH TAIL
- {0x01BB, 0x01BB, prAL, gcLo}, // LATIN LETTER TWO WITH STROKE
- {0x01BC, 0x01BF, prAL, gcLC}, // [4] LATIN CAPITAL LETTER TONE FIVE..LATIN LETTER WYNN
- {0x01C0, 0x01C3, prAL, gcLo}, // [4] LATIN LETTER DENTAL CLICK..LATIN LETTER RETROFLEX CLICK
- {0x01C4, 0x024F, prAL, gcLC}, // [140] LATIN CAPITAL LETTER DZ WITH CARON..LATIN SMALL LETTER Y WITH STROKE
- {0x0250, 0x0293, prAL, gcLl}, // [68] LATIN SMALL LETTER TURNED A..LATIN SMALL LETTER EZH WITH CURL
- {0x0294, 0x0294, prAL, gcLo}, // LATIN LETTER GLOTTAL STOP
- {0x0295, 0x02AF, prAL, gcLl}, // [27] LATIN LETTER PHARYNGEAL VOICED FRICATIVE..LATIN SMALL LETTER TURNED H WITH FISHHOOK AND TAIL
- {0x02B0, 0x02C1, prAL, gcLm}, // [18] MODIFIER LETTER SMALL H..MODIFIER LETTER REVERSED GLOTTAL STOP
- {0x02C2, 0x02C5, prAL, gcSk}, // [4] MODIFIER LETTER LEFT ARROWHEAD..MODIFIER LETTER DOWN ARROWHEAD
- {0x02C6, 0x02C6, prAL, gcLm}, // MODIFIER LETTER CIRCUMFLEX ACCENT
- {0x02C7, 0x02C7, prAI, gcLm}, // CARON
- {0x02C8, 0x02C8, prBB, gcLm}, // MODIFIER LETTER VERTICAL LINE
- {0x02C9, 0x02CB, prAI, gcLm}, // [3] MODIFIER LETTER MACRON..MODIFIER LETTER GRAVE ACCENT
- {0x02CC, 0x02CC, prBB, gcLm}, // MODIFIER LETTER LOW VERTICAL LINE
- {0x02CD, 0x02CD, prAI, gcLm}, // MODIFIER LETTER LOW MACRON
- {0x02CE, 0x02CF, prAL, gcLm}, // [2] MODIFIER LETTER LOW GRAVE ACCENT..MODIFIER LETTER LOW ACUTE ACCENT
- {0x02D0, 0x02D0, prAI, gcLm}, // MODIFIER LETTER TRIANGULAR COLON
- {0x02D1, 0x02D1, prAL, gcLm}, // MODIFIER LETTER HALF TRIANGULAR COLON
- {0x02D2, 0x02D7, prAL, gcSk}, // [6] MODIFIER LETTER CENTRED RIGHT HALF RING..MODIFIER LETTER MINUS SIGN
- {0x02D8, 0x02DB, prAI, gcSk}, // [4] BREVE..OGONEK
- {0x02DC, 0x02DC, prAL, gcSk}, // SMALL TILDE
- {0x02DD, 0x02DD, prAI, gcSk}, // DOUBLE ACUTE ACCENT
- {0x02DE, 0x02DE, prAL, gcSk}, // MODIFIER LETTER RHOTIC HOOK
- {0x02DF, 0x02DF, prBB, gcSk}, // MODIFIER LETTER CROSS ACCENT
- {0x02E0, 0x02E4, prAL, gcLm}, // [5] MODIFIER LETTER SMALL GAMMA..MODIFIER LETTER SMALL REVERSED GLOTTAL STOP
- {0x02E5, 0x02EB, prAL, gcSk}, // [7] MODIFIER LETTER EXTRA-HIGH TONE BAR..MODIFIER LETTER YANG DEPARTING TONE MARK
- {0x02EC, 0x02EC, prAL, gcLm}, // MODIFIER LETTER VOICING
- {0x02ED, 0x02ED, prAL, gcSk}, // MODIFIER LETTER UNASPIRATED
- {0x02EE, 0x02EE, prAL, gcLm}, // MODIFIER LETTER DOUBLE APOSTROPHE
- {0x02EF, 0x02FF, prAL, gcSk}, // [17] MODIFIER LETTER LOW DOWN ARROWHEAD..MODIFIER LETTER LOW LEFT ARROW
- {0x0300, 0x034E, prCM, gcMn}, // [79] COMBINING GRAVE ACCENT..COMBINING UPWARDS ARROW BELOW
- {0x034F, 0x034F, prGL, gcMn}, // COMBINING GRAPHEME JOINER
- {0x0350, 0x035B, prCM, gcMn}, // [12] COMBINING RIGHT ARROWHEAD ABOVE..COMBINING ZIGZAG ABOVE
- {0x035C, 0x0362, prGL, gcMn}, // [7] COMBINING DOUBLE BREVE BELOW..COMBINING DOUBLE RIGHTWARDS ARROW BELOW
- {0x0363, 0x036F, prCM, gcMn}, // [13] COMBINING LATIN SMALL LETTER A..COMBINING LATIN SMALL LETTER X
- {0x0370, 0x0373, prAL, gcLC}, // [4] GREEK CAPITAL LETTER HETA..GREEK SMALL LETTER ARCHAIC SAMPI
- {0x0374, 0x0374, prAL, gcLm}, // GREEK NUMERAL SIGN
- {0x0375, 0x0375, prAL, gcSk}, // GREEK LOWER NUMERAL SIGN
- {0x0376, 0x0377, prAL, gcLC}, // [2] GREEK CAPITAL LETTER PAMPHYLIAN DIGAMMA..GREEK SMALL LETTER PAMPHYLIAN DIGAMMA
- {0x037A, 0x037A, prAL, gcLm}, // GREEK YPOGEGRAMMENI
- {0x037B, 0x037D, prAL, gcLl}, // [3] GREEK SMALL REVERSED LUNATE SIGMA SYMBOL..GREEK SMALL REVERSED DOTTED LUNATE SIGMA SYMBOL
- {0x037E, 0x037E, prIS, gcPo}, // GREEK QUESTION MARK
- {0x037F, 0x037F, prAL, gcLu}, // GREEK CAPITAL LETTER YOT
- {0x0384, 0x0385, prAL, gcSk}, // [2] GREEK TONOS..GREEK DIALYTIKA TONOS
- {0x0386, 0x0386, prAL, gcLu}, // GREEK CAPITAL LETTER ALPHA WITH TONOS
- {0x0387, 0x0387, prAL, gcPo}, // GREEK ANO TELEIA
- {0x0388, 0x038A, prAL, gcLu}, // [3] GREEK CAPITAL LETTER EPSILON WITH TONOS..GREEK CAPITAL LETTER IOTA WITH TONOS
- {0x038C, 0x038C, prAL, gcLu}, // GREEK CAPITAL LETTER OMICRON WITH TONOS
- {0x038E, 0x03A1, prAL, gcLC}, // [20] GREEK CAPITAL LETTER UPSILON WITH TONOS..GREEK CAPITAL LETTER RHO
- {0x03A3, 0x03F5, prAL, gcLC}, // [83] GREEK CAPITAL LETTER SIGMA..GREEK LUNATE EPSILON SYMBOL
- {0x03F6, 0x03F6, prAL, gcSm}, // GREEK REVERSED LUNATE EPSILON SYMBOL
- {0x03F7, 0x03FF, prAL, gcLC}, // [9] GREEK CAPITAL LETTER SHO..GREEK CAPITAL REVERSED DOTTED LUNATE SIGMA SYMBOL
- {0x0400, 0x0481, prAL, gcLC}, // [130] CYRILLIC CAPITAL LETTER IE WITH GRAVE..CYRILLIC SMALL LETTER KOPPA
- {0x0482, 0x0482, prAL, gcSo}, // CYRILLIC THOUSANDS SIGN
- {0x0483, 0x0487, prCM, gcMn}, // [5] COMBINING CYRILLIC TITLO..COMBINING CYRILLIC POKRYTIE
- {0x0488, 0x0489, prCM, gcMe}, // [2] COMBINING CYRILLIC HUNDRED THOUSANDS SIGN..COMBINING CYRILLIC MILLIONS SIGN
- {0x048A, 0x04FF, prAL, gcLC}, // [118] CYRILLIC CAPITAL LETTER SHORT I WITH TAIL..CYRILLIC SMALL LETTER HA WITH STROKE
- {0x0500, 0x052F, prAL, gcLC}, // [48] CYRILLIC CAPITAL LETTER KOMI DE..CYRILLIC SMALL LETTER EL WITH DESCENDER
- {0x0531, 0x0556, prAL, gcLu}, // [38] ARMENIAN CAPITAL LETTER AYB..ARMENIAN CAPITAL LETTER FEH
- {0x0559, 0x0559, prAL, gcLm}, // ARMENIAN MODIFIER LETTER LEFT HALF RING
- {0x055A, 0x055F, prAL, gcPo}, // [6] ARMENIAN APOSTROPHE..ARMENIAN ABBREVIATION MARK
- {0x0560, 0x0588, prAL, gcLl}, // [41] ARMENIAN SMALL LETTER TURNED AYB..ARMENIAN SMALL LETTER YI WITH STROKE
- {0x0589, 0x0589, prIS, gcPo}, // ARMENIAN FULL STOP
- {0x058A, 0x058A, prBA, gcPd}, // ARMENIAN HYPHEN
- {0x058D, 0x058E, prAL, gcSo}, // [2] RIGHT-FACING ARMENIAN ETERNITY SIGN..LEFT-FACING ARMENIAN ETERNITY SIGN
- {0x058F, 0x058F, prPR, gcSc}, // ARMENIAN DRAM SIGN
- {0x0591, 0x05BD, prCM, gcMn}, // [45] HEBREW ACCENT ETNAHTA..HEBREW POINT METEG
- {0x05BE, 0x05BE, prBA, gcPd}, // HEBREW PUNCTUATION MAQAF
- {0x05BF, 0x05BF, prCM, gcMn}, // HEBREW POINT RAFE
- {0x05C0, 0x05C0, prAL, gcPo}, // HEBREW PUNCTUATION PASEQ
- {0x05C1, 0x05C2, prCM, gcMn}, // [2] HEBREW POINT SHIN DOT..HEBREW POINT SIN DOT
- {0x05C3, 0x05C3, prAL, gcPo}, // HEBREW PUNCTUATION SOF PASUQ
- {0x05C4, 0x05C5, prCM, gcMn}, // [2] HEBREW MARK UPPER DOT..HEBREW MARK LOWER DOT
- {0x05C6, 0x05C6, prEX, gcPo}, // HEBREW PUNCTUATION NUN HAFUKHA
- {0x05C7, 0x05C7, prCM, gcMn}, // HEBREW POINT QAMATS QATAN
- {0x05D0, 0x05EA, prHL, gcLo}, // [27] HEBREW LETTER ALEF..HEBREW LETTER TAV
- {0x05EF, 0x05F2, prHL, gcLo}, // [4] HEBREW YOD TRIANGLE..HEBREW LIGATURE YIDDISH DOUBLE YOD
- {0x05F3, 0x05F4, prAL, gcPo}, // [2] HEBREW PUNCTUATION GERESH..HEBREW PUNCTUATION GERSHAYIM
- {0x0600, 0x0605, prAL, gcCf}, // [6] ARABIC NUMBER SIGN..ARABIC NUMBER MARK ABOVE
- {0x0606, 0x0608, prAL, gcSm}, // [3] ARABIC-INDIC CUBE ROOT..ARABIC RAY
- {0x0609, 0x060A, prPO, gcPo}, // [2] ARABIC-INDIC PER MILLE SIGN..ARABIC-INDIC PER TEN THOUSAND SIGN
- {0x060B, 0x060B, prPO, gcSc}, // AFGHANI SIGN
- {0x060C, 0x060D, prIS, gcPo}, // [2] ARABIC COMMA..ARABIC DATE SEPARATOR
- {0x060E, 0x060F, prAL, gcSo}, // [2] ARABIC POETIC VERSE SIGN..ARABIC SIGN MISRA
- {0x0610, 0x061A, prCM, gcMn}, // [11] ARABIC SIGN SALLALLAHOU ALAYHE WASSALLAM..ARABIC SMALL KASRA
- {0x061B, 0x061B, prEX, gcPo}, // ARABIC SEMICOLON
- {0x061C, 0x061C, prCM, gcCf}, // ARABIC LETTER MARK
- {0x061D, 0x061F, prEX, gcPo}, // [3] ARABIC END OF TEXT MARK..ARABIC QUESTION MARK
- {0x0620, 0x063F, prAL, gcLo}, // [32] ARABIC LETTER KASHMIRI YEH..ARABIC LETTER FARSI YEH WITH THREE DOTS ABOVE
- {0x0640, 0x0640, prAL, gcLm}, // ARABIC TATWEEL
- {0x0641, 0x064A, prAL, gcLo}, // [10] ARABIC LETTER FEH..ARABIC LETTER YEH
- {0x064B, 0x065F, prCM, gcMn}, // [21] ARABIC FATHATAN..ARABIC WAVY HAMZA BELOW
- {0x0660, 0x0669, prNU, gcNd}, // [10] ARABIC-INDIC DIGIT ZERO..ARABIC-INDIC DIGIT NINE
- {0x066A, 0x066A, prPO, gcPo}, // ARABIC PERCENT SIGN
- {0x066B, 0x066C, prNU, gcPo}, // [2] ARABIC DECIMAL SEPARATOR..ARABIC THOUSANDS SEPARATOR
- {0x066D, 0x066D, prAL, gcPo}, // ARABIC FIVE POINTED STAR
- {0x066E, 0x066F, prAL, gcLo}, // [2] ARABIC LETTER DOTLESS BEH..ARABIC LETTER DOTLESS QAF
- {0x0670, 0x0670, prCM, gcMn}, // ARABIC LETTER SUPERSCRIPT ALEF
- {0x0671, 0x06D3, prAL, gcLo}, // [99] ARABIC LETTER ALEF WASLA..ARABIC LETTER YEH BARREE WITH HAMZA ABOVE
- {0x06D4, 0x06D4, prEX, gcPo}, // ARABIC FULL STOP
- {0x06D5, 0x06D5, prAL, gcLo}, // ARABIC LETTER AE
- {0x06D6, 0x06DC, prCM, gcMn}, // [7] ARABIC SMALL HIGH LIGATURE SAD WITH LAM WITH ALEF MAKSURA..ARABIC SMALL HIGH SEEN
- {0x06DD, 0x06DD, prAL, gcCf}, // ARABIC END OF AYAH
- {0x06DE, 0x06DE, prAL, gcSo}, // ARABIC START OF RUB EL HIZB
- {0x06DF, 0x06E4, prCM, gcMn}, // [6] ARABIC SMALL HIGH ROUNDED ZERO..ARABIC SMALL HIGH MADDA
- {0x06E5, 0x06E6, prAL, gcLm}, // [2] ARABIC SMALL WAW..ARABIC SMALL YEH
- {0x06E7, 0x06E8, prCM, gcMn}, // [2] ARABIC SMALL HIGH YEH..ARABIC SMALL HIGH NOON
- {0x06E9, 0x06E9, prAL, gcSo}, // ARABIC PLACE OF SAJDAH
- {0x06EA, 0x06ED, prCM, gcMn}, // [4] ARABIC EMPTY CENTRE LOW STOP..ARABIC SMALL LOW MEEM
- {0x06EE, 0x06EF, prAL, gcLo}, // [2] ARABIC LETTER DAL WITH INVERTED V..ARABIC LETTER REH WITH INVERTED V
- {0x06F0, 0x06F9, prNU, gcNd}, // [10] EXTENDED ARABIC-INDIC DIGIT ZERO..EXTENDED ARABIC-INDIC DIGIT NINE
- {0x06FA, 0x06FC, prAL, gcLo}, // [3] ARABIC LETTER SHEEN WITH DOT BELOW..ARABIC LETTER GHAIN WITH DOT BELOW
- {0x06FD, 0x06FE, prAL, gcSo}, // [2] ARABIC SIGN SINDHI AMPERSAND..ARABIC SIGN SINDHI POSTPOSITION MEN
- {0x06FF, 0x06FF, prAL, gcLo}, // ARABIC LETTER HEH WITH INVERTED V
- {0x0700, 0x070D, prAL, gcPo}, // [14] SYRIAC END OF PARAGRAPH..SYRIAC HARKLEAN ASTERISCUS
- {0x070F, 0x070F, prAL, gcCf}, // SYRIAC ABBREVIATION MARK
- {0x0710, 0x0710, prAL, gcLo}, // SYRIAC LETTER ALAPH
- {0x0711, 0x0711, prCM, gcMn}, // SYRIAC LETTER SUPERSCRIPT ALAPH
- {0x0712, 0x072F, prAL, gcLo}, // [30] SYRIAC LETTER BETH..SYRIAC LETTER PERSIAN DHALATH
- {0x0730, 0x074A, prCM, gcMn}, // [27] SYRIAC PTHAHA ABOVE..SYRIAC BARREKH
- {0x074D, 0x074F, prAL, gcLo}, // [3] SYRIAC LETTER SOGDIAN ZHAIN..SYRIAC LETTER SOGDIAN FE
- {0x0750, 0x077F, prAL, gcLo}, // [48] ARABIC LETTER BEH WITH THREE DOTS HORIZONTALLY BELOW..ARABIC LETTER KAF WITH TWO DOTS ABOVE
- {0x0780, 0x07A5, prAL, gcLo}, // [38] THAANA LETTER HAA..THAANA LETTER WAAVU
- {0x07A6, 0x07B0, prCM, gcMn}, // [11] THAANA ABAFILI..THAANA SUKUN
- {0x07B1, 0x07B1, prAL, gcLo}, // THAANA LETTER NAA
- {0x07C0, 0x07C9, prNU, gcNd}, // [10] NKO DIGIT ZERO..NKO DIGIT NINE
- {0x07CA, 0x07EA, prAL, gcLo}, // [33] NKO LETTER A..NKO LETTER JONA RA
- {0x07EB, 0x07F3, prCM, gcMn}, // [9] NKO COMBINING SHORT HIGH TONE..NKO COMBINING DOUBLE DOT ABOVE
- {0x07F4, 0x07F5, prAL, gcLm}, // [2] NKO HIGH TONE APOSTROPHE..NKO LOW TONE APOSTROPHE
- {0x07F6, 0x07F6, prAL, gcSo}, // NKO SYMBOL OO DENNEN
- {0x07F7, 0x07F7, prAL, gcPo}, // NKO SYMBOL GBAKURUNEN
- {0x07F8, 0x07F8, prIS, gcPo}, // NKO COMMA
- {0x07F9, 0x07F9, prEX, gcPo}, // NKO EXCLAMATION MARK
- {0x07FA, 0x07FA, prAL, gcLm}, // NKO LAJANYALAN
- {0x07FD, 0x07FD, prCM, gcMn}, // NKO DANTAYALAN
- {0x07FE, 0x07FF, prPR, gcSc}, // [2] NKO DOROME SIGN..NKO TAMAN SIGN
- {0x0800, 0x0815, prAL, gcLo}, // [22] SAMARITAN LETTER ALAF..SAMARITAN LETTER TAAF
- {0x0816, 0x0819, prCM, gcMn}, // [4] SAMARITAN MARK IN..SAMARITAN MARK DAGESH
- {0x081A, 0x081A, prAL, gcLm}, // SAMARITAN MODIFIER LETTER EPENTHETIC YUT
- {0x081B, 0x0823, prCM, gcMn}, // [9] SAMARITAN MARK EPENTHETIC YUT..SAMARITAN VOWEL SIGN A
- {0x0824, 0x0824, prAL, gcLm}, // SAMARITAN MODIFIER LETTER SHORT A
- {0x0825, 0x0827, prCM, gcMn}, // [3] SAMARITAN VOWEL SIGN SHORT A..SAMARITAN VOWEL SIGN U
- {0x0828, 0x0828, prAL, gcLm}, // SAMARITAN MODIFIER LETTER I
- {0x0829, 0x082D, prCM, gcMn}, // [5] SAMARITAN VOWEL SIGN LONG I..SAMARITAN MARK NEQUDAA
- {0x0830, 0x083E, prAL, gcPo}, // [15] SAMARITAN PUNCTUATION NEQUDAA..SAMARITAN PUNCTUATION ANNAAU
- {0x0840, 0x0858, prAL, gcLo}, // [25] MANDAIC LETTER HALQA..MANDAIC LETTER AIN
- {0x0859, 0x085B, prCM, gcMn}, // [3] MANDAIC AFFRICATION MARK..MANDAIC GEMINATION MARK
- {0x085E, 0x085E, prAL, gcPo}, // MANDAIC PUNCTUATION
- {0x0860, 0x086A, prAL, gcLo}, // [11] SYRIAC LETTER MALAYALAM NGA..SYRIAC LETTER MALAYALAM SSA
- {0x0870, 0x0887, prAL, gcLo}, // [24] ARABIC LETTER ALEF WITH ATTACHED FATHA..ARABIC BASELINE ROUND DOT
- {0x0888, 0x0888, prAL, gcSk}, // ARABIC RAISED ROUND DOT
- {0x0889, 0x088E, prAL, gcLo}, // [6] ARABIC LETTER NOON WITH INVERTED SMALL V..ARABIC VERTICAL TAIL
- {0x0890, 0x0891, prAL, gcCf}, // [2] ARABIC POUND MARK ABOVE..ARABIC PIASTRE MARK ABOVE
- {0x0898, 0x089F, prCM, gcMn}, // [8] ARABIC SMALL HIGH WORD AL-JUZ..ARABIC HALF MADDA OVER MADDA
- {0x08A0, 0x08C8, prAL, gcLo}, // [41] ARABIC LETTER BEH WITH SMALL V BELOW..ARABIC LETTER GRAF
- {0x08C9, 0x08C9, prAL, gcLm}, // ARABIC SMALL FARSI YEH
- {0x08CA, 0x08E1, prCM, gcMn}, // [24] ARABIC SMALL HIGH FARSI YEH..ARABIC SMALL HIGH SIGN SAFHA
- {0x08E2, 0x08E2, prAL, gcCf}, // ARABIC DISPUTED END OF AYAH
- {0x08E3, 0x08FF, prCM, gcMn}, // [29] ARABIC TURNED DAMMA BELOW..ARABIC MARK SIDEWAYS NOON GHUNNA
- {0x0900, 0x0902, prCM, gcMn}, // [3] DEVANAGARI SIGN INVERTED CANDRABINDU..DEVANAGARI SIGN ANUSVARA
- {0x0903, 0x0903, prCM, gcMc}, // DEVANAGARI SIGN VISARGA
- {0x0904, 0x0939, prAL, gcLo}, // [54] DEVANAGARI LETTER SHORT A..DEVANAGARI LETTER HA
- {0x093A, 0x093A, prCM, gcMn}, // DEVANAGARI VOWEL SIGN OE
- {0x093B, 0x093B, prCM, gcMc}, // DEVANAGARI VOWEL SIGN OOE
- {0x093C, 0x093C, prCM, gcMn}, // DEVANAGARI SIGN NUKTA
- {0x093D, 0x093D, prAL, gcLo}, // DEVANAGARI SIGN AVAGRAHA
- {0x093E, 0x0940, prCM, gcMc}, // [3] DEVANAGARI VOWEL SIGN AA..DEVANAGARI VOWEL SIGN II
- {0x0941, 0x0948, prCM, gcMn}, // [8] DEVANAGARI VOWEL SIGN U..DEVANAGARI VOWEL SIGN AI
- {0x0949, 0x094C, prCM, gcMc}, // [4] DEVANAGARI VOWEL SIGN CANDRA O..DEVANAGARI VOWEL SIGN AU
- {0x094D, 0x094D, prCM, gcMn}, // DEVANAGARI SIGN VIRAMA
- {0x094E, 0x094F, prCM, gcMc}, // [2] DEVANAGARI VOWEL SIGN PRISHTHAMATRA E..DEVANAGARI VOWEL SIGN AW
- {0x0950, 0x0950, prAL, gcLo}, // DEVANAGARI OM
- {0x0951, 0x0957, prCM, gcMn}, // [7] DEVANAGARI STRESS SIGN UDATTA..DEVANAGARI VOWEL SIGN UUE
- {0x0958, 0x0961, prAL, gcLo}, // [10] DEVANAGARI LETTER QA..DEVANAGARI LETTER VOCALIC LL
- {0x0962, 0x0963, prCM, gcMn}, // [2] DEVANAGARI VOWEL SIGN VOCALIC L..DEVANAGARI VOWEL SIGN VOCALIC LL
- {0x0964, 0x0965, prBA, gcPo}, // [2] DEVANAGARI DANDA..DEVANAGARI DOUBLE DANDA
- {0x0966, 0x096F, prNU, gcNd}, // [10] DEVANAGARI DIGIT ZERO..DEVANAGARI DIGIT NINE
- {0x0970, 0x0970, prAL, gcPo}, // DEVANAGARI ABBREVIATION SIGN
- {0x0971, 0x0971, prAL, gcLm}, // DEVANAGARI SIGN HIGH SPACING DOT
- {0x0972, 0x097F, prAL, gcLo}, // [14] DEVANAGARI LETTER CANDRA A..DEVANAGARI LETTER BBA
- {0x0980, 0x0980, prAL, gcLo}, // BENGALI ANJI
- {0x0981, 0x0981, prCM, gcMn}, // BENGALI SIGN CANDRABINDU
- {0x0982, 0x0983, prCM, gcMc}, // [2] BENGALI SIGN ANUSVARA..BENGALI SIGN VISARGA
- {0x0985, 0x098C, prAL, gcLo}, // [8] BENGALI LETTER A..BENGALI LETTER VOCALIC L
- {0x098F, 0x0990, prAL, gcLo}, // [2] BENGALI LETTER E..BENGALI LETTER AI
- {0x0993, 0x09A8, prAL, gcLo}, // [22] BENGALI LETTER O..BENGALI LETTER NA
- {0x09AA, 0x09B0, prAL, gcLo}, // [7] BENGALI LETTER PA..BENGALI LETTER RA
- {0x09B2, 0x09B2, prAL, gcLo}, // BENGALI LETTER LA
- {0x09B6, 0x09B9, prAL, gcLo}, // [4] BENGALI LETTER SHA..BENGALI LETTER HA
- {0x09BC, 0x09BC, prCM, gcMn}, // BENGALI SIGN NUKTA
- {0x09BD, 0x09BD, prAL, gcLo}, // BENGALI SIGN AVAGRAHA
- {0x09BE, 0x09C0, prCM, gcMc}, // [3] BENGALI VOWEL SIGN AA..BENGALI VOWEL SIGN II
- {0x09C1, 0x09C4, prCM, gcMn}, // [4] BENGALI VOWEL SIGN U..BENGALI VOWEL SIGN VOCALIC RR
- {0x09C7, 0x09C8, prCM, gcMc}, // [2] BENGALI VOWEL SIGN E..BENGALI VOWEL SIGN AI
- {0x09CB, 0x09CC, prCM, gcMc}, // [2] BENGALI VOWEL SIGN O..BENGALI VOWEL SIGN AU
- {0x09CD, 0x09CD, prCM, gcMn}, // BENGALI SIGN VIRAMA
- {0x09CE, 0x09CE, prAL, gcLo}, // BENGALI LETTER KHANDA TA
- {0x09D7, 0x09D7, prCM, gcMc}, // BENGALI AU LENGTH MARK
- {0x09DC, 0x09DD, prAL, gcLo}, // [2] BENGALI LETTER RRA..BENGALI LETTER RHA
- {0x09DF, 0x09E1, prAL, gcLo}, // [3] BENGALI LETTER YYA..BENGALI LETTER VOCALIC LL
- {0x09E2, 0x09E3, prCM, gcMn}, // [2] BENGALI VOWEL SIGN VOCALIC L..BENGALI VOWEL SIGN VOCALIC LL
- {0x09E6, 0x09EF, prNU, gcNd}, // [10] BENGALI DIGIT ZERO..BENGALI DIGIT NINE
- {0x09F0, 0x09F1, prAL, gcLo}, // [2] BENGALI LETTER RA WITH MIDDLE DIAGONAL..BENGALI LETTER RA WITH LOWER DIAGONAL
- {0x09F2, 0x09F3, prPO, gcSc}, // [2] BENGALI RUPEE MARK..BENGALI RUPEE SIGN
- {0x09F4, 0x09F8, prAL, gcNo}, // [5] BENGALI CURRENCY NUMERATOR ONE..BENGALI CURRENCY NUMERATOR ONE LESS THAN THE DENOMINATOR
- {0x09F9, 0x09F9, prPO, gcNo}, // BENGALI CURRENCY DENOMINATOR SIXTEEN
- {0x09FA, 0x09FA, prAL, gcSo}, // BENGALI ISSHAR
- {0x09FB, 0x09FB, prPR, gcSc}, // BENGALI GANDA MARK
- {0x09FC, 0x09FC, prAL, gcLo}, // BENGALI LETTER VEDIC ANUSVARA
- {0x09FD, 0x09FD, prAL, gcPo}, // BENGALI ABBREVIATION SIGN
- {0x09FE, 0x09FE, prCM, gcMn}, // BENGALI SANDHI MARK
- {0x0A01, 0x0A02, prCM, gcMn}, // [2] GURMUKHI SIGN ADAK BINDI..GURMUKHI SIGN BINDI
- {0x0A03, 0x0A03, prCM, gcMc}, // GURMUKHI SIGN VISARGA
- {0x0A05, 0x0A0A, prAL, gcLo}, // [6] GURMUKHI LETTER A..GURMUKHI LETTER UU
- {0x0A0F, 0x0A10, prAL, gcLo}, // [2] GURMUKHI LETTER EE..GURMUKHI LETTER AI
- {0x0A13, 0x0A28, prAL, gcLo}, // [22] GURMUKHI LETTER OO..GURMUKHI LETTER NA
- {0x0A2A, 0x0A30, prAL, gcLo}, // [7] GURMUKHI LETTER PA..GURMUKHI LETTER RA
- {0x0A32, 0x0A33, prAL, gcLo}, // [2] GURMUKHI LETTER LA..GURMUKHI LETTER LLA
- {0x0A35, 0x0A36, prAL, gcLo}, // [2] GURMUKHI LETTER VA..GURMUKHI LETTER SHA
- {0x0A38, 0x0A39, prAL, gcLo}, // [2] GURMUKHI LETTER SA..GURMUKHI LETTER HA
- {0x0A3C, 0x0A3C, prCM, gcMn}, // GURMUKHI SIGN NUKTA
- {0x0A3E, 0x0A40, prCM, gcMc}, // [3] GURMUKHI VOWEL SIGN AA..GURMUKHI VOWEL SIGN II
- {0x0A41, 0x0A42, prCM, gcMn}, // [2] GURMUKHI VOWEL SIGN U..GURMUKHI VOWEL SIGN UU
- {0x0A47, 0x0A48, prCM, gcMn}, // [2] GURMUKHI VOWEL SIGN EE..GURMUKHI VOWEL SIGN AI
- {0x0A4B, 0x0A4D, prCM, gcMn}, // [3] GURMUKHI VOWEL SIGN OO..GURMUKHI SIGN VIRAMA
- {0x0A51, 0x0A51, prCM, gcMn}, // GURMUKHI SIGN UDAAT
- {0x0A59, 0x0A5C, prAL, gcLo}, // [4] GURMUKHI LETTER KHHA..GURMUKHI LETTER RRA
- {0x0A5E, 0x0A5E, prAL, gcLo}, // GURMUKHI LETTER FA
- {0x0A66, 0x0A6F, prNU, gcNd}, // [10] GURMUKHI DIGIT ZERO..GURMUKHI DIGIT NINE
- {0x0A70, 0x0A71, prCM, gcMn}, // [2] GURMUKHI TIPPI..GURMUKHI ADDAK
- {0x0A72, 0x0A74, prAL, gcLo}, // [3] GURMUKHI IRI..GURMUKHI EK ONKAR
- {0x0A75, 0x0A75, prCM, gcMn}, // GURMUKHI SIGN YAKASH
- {0x0A76, 0x0A76, prAL, gcPo}, // GURMUKHI ABBREVIATION SIGN
- {0x0A81, 0x0A82, prCM, gcMn}, // [2] GUJARATI SIGN CANDRABINDU..GUJARATI SIGN ANUSVARA
- {0x0A83, 0x0A83, prCM, gcMc}, // GUJARATI SIGN VISARGA
- {0x0A85, 0x0A8D, prAL, gcLo}, // [9] GUJARATI LETTER A..GUJARATI VOWEL CANDRA E
- {0x0A8F, 0x0A91, prAL, gcLo}, // [3] GUJARATI LETTER E..GUJARATI VOWEL CANDRA O
- {0x0A93, 0x0AA8, prAL, gcLo}, // [22] GUJARATI LETTER O..GUJARATI LETTER NA
- {0x0AAA, 0x0AB0, prAL, gcLo}, // [7] GUJARATI LETTER PA..GUJARATI LETTER RA
- {0x0AB2, 0x0AB3, prAL, gcLo}, // [2] GUJARATI LETTER LA..GUJARATI LETTER LLA
- {0x0AB5, 0x0AB9, prAL, gcLo}, // [5] GUJARATI LETTER VA..GUJARATI LETTER HA
- {0x0ABC, 0x0ABC, prCM, gcMn}, // GUJARATI SIGN NUKTA
- {0x0ABD, 0x0ABD, prAL, gcLo}, // GUJARATI SIGN AVAGRAHA
- {0x0ABE, 0x0AC0, prCM, gcMc}, // [3] GUJARATI VOWEL SIGN AA..GUJARATI VOWEL SIGN II
- {0x0AC1, 0x0AC5, prCM, gcMn}, // [5] GUJARATI VOWEL SIGN U..GUJARATI VOWEL SIGN CANDRA E
- {0x0AC7, 0x0AC8, prCM, gcMn}, // [2] GUJARATI VOWEL SIGN E..GUJARATI VOWEL SIGN AI
- {0x0AC9, 0x0AC9, prCM, gcMc}, // GUJARATI VOWEL SIGN CANDRA O
- {0x0ACB, 0x0ACC, prCM, gcMc}, // [2] GUJARATI VOWEL SIGN O..GUJARATI VOWEL SIGN AU
- {0x0ACD, 0x0ACD, prCM, gcMn}, // GUJARATI SIGN VIRAMA
- {0x0AD0, 0x0AD0, prAL, gcLo}, // GUJARATI OM
- {0x0AE0, 0x0AE1, prAL, gcLo}, // [2] GUJARATI LETTER VOCALIC RR..GUJARATI LETTER VOCALIC LL
- {0x0AE2, 0x0AE3, prCM, gcMn}, // [2] GUJARATI VOWEL SIGN VOCALIC L..GUJARATI VOWEL SIGN VOCALIC LL
- {0x0AE6, 0x0AEF, prNU, gcNd}, // [10] GUJARATI DIGIT ZERO..GUJARATI DIGIT NINE
- {0x0AF0, 0x0AF0, prAL, gcPo}, // GUJARATI ABBREVIATION SIGN
- {0x0AF1, 0x0AF1, prPR, gcSc}, // GUJARATI RUPEE SIGN
- {0x0AF9, 0x0AF9, prAL, gcLo}, // GUJARATI LETTER ZHA
- {0x0AFA, 0x0AFF, prCM, gcMn}, // [6] GUJARATI SIGN SUKUN..GUJARATI SIGN TWO-CIRCLE NUKTA ABOVE
- {0x0B01, 0x0B01, prCM, gcMn}, // ORIYA SIGN CANDRABINDU
- {0x0B02, 0x0B03, prCM, gcMc}, // [2] ORIYA SIGN ANUSVARA..ORIYA SIGN VISARGA
- {0x0B05, 0x0B0C, prAL, gcLo}, // [8] ORIYA LETTER A..ORIYA LETTER VOCALIC L
- {0x0B0F, 0x0B10, prAL, gcLo}, // [2] ORIYA LETTER E..ORIYA LETTER AI
- {0x0B13, 0x0B28, prAL, gcLo}, // [22] ORIYA LETTER O..ORIYA LETTER NA
- {0x0B2A, 0x0B30, prAL, gcLo}, // [7] ORIYA LETTER PA..ORIYA LETTER RA
- {0x0B32, 0x0B33, prAL, gcLo}, // [2] ORIYA LETTER LA..ORIYA LETTER LLA
- {0x0B35, 0x0B39, prAL, gcLo}, // [5] ORIYA LETTER VA..ORIYA LETTER HA
- {0x0B3C, 0x0B3C, prCM, gcMn}, // ORIYA SIGN NUKTA
- {0x0B3D, 0x0B3D, prAL, gcLo}, // ORIYA SIGN AVAGRAHA
- {0x0B3E, 0x0B3E, prCM, gcMc}, // ORIYA VOWEL SIGN AA
- {0x0B3F, 0x0B3F, prCM, gcMn}, // ORIYA VOWEL SIGN I
- {0x0B40, 0x0B40, prCM, gcMc}, // ORIYA VOWEL SIGN II
- {0x0B41, 0x0B44, prCM, gcMn}, // [4] ORIYA VOWEL SIGN U..ORIYA VOWEL SIGN VOCALIC RR
- {0x0B47, 0x0B48, prCM, gcMc}, // [2] ORIYA VOWEL SIGN E..ORIYA VOWEL SIGN AI
- {0x0B4B, 0x0B4C, prCM, gcMc}, // [2] ORIYA VOWEL SIGN O..ORIYA VOWEL SIGN AU
- {0x0B4D, 0x0B4D, prCM, gcMn}, // ORIYA SIGN VIRAMA
- {0x0B55, 0x0B56, prCM, gcMn}, // [2] ORIYA SIGN OVERLINE..ORIYA AI LENGTH MARK
- {0x0B57, 0x0B57, prCM, gcMc}, // ORIYA AU LENGTH MARK
- {0x0B5C, 0x0B5D, prAL, gcLo}, // [2] ORIYA LETTER RRA..ORIYA LETTER RHA
- {0x0B5F, 0x0B61, prAL, gcLo}, // [3] ORIYA LETTER YYA..ORIYA LETTER VOCALIC LL
- {0x0B62, 0x0B63, prCM, gcMn}, // [2] ORIYA VOWEL SIGN VOCALIC L..ORIYA VOWEL SIGN VOCALIC LL
- {0x0B66, 0x0B6F, prNU, gcNd}, // [10] ORIYA DIGIT ZERO..ORIYA DIGIT NINE
- {0x0B70, 0x0B70, prAL, gcSo}, // ORIYA ISSHAR
- {0x0B71, 0x0B71, prAL, gcLo}, // ORIYA LETTER WA
- {0x0B72, 0x0B77, prAL, gcNo}, // [6] ORIYA FRACTION ONE QUARTER..ORIYA FRACTION THREE SIXTEENTHS
- {0x0B82, 0x0B82, prCM, gcMn}, // TAMIL SIGN ANUSVARA
- {0x0B83, 0x0B83, prAL, gcLo}, // TAMIL SIGN VISARGA
- {0x0B85, 0x0B8A, prAL, gcLo}, // [6] TAMIL LETTER A..TAMIL LETTER UU
- {0x0B8E, 0x0B90, prAL, gcLo}, // [3] TAMIL LETTER E..TAMIL LETTER AI
- {0x0B92, 0x0B95, prAL, gcLo}, // [4] TAMIL LETTER O..TAMIL LETTER KA
- {0x0B99, 0x0B9A, prAL, gcLo}, // [2] TAMIL LETTER NGA..TAMIL LETTER CA
- {0x0B9C, 0x0B9C, prAL, gcLo}, // TAMIL LETTER JA
- {0x0B9E, 0x0B9F, prAL, gcLo}, // [2] TAMIL LETTER NYA..TAMIL LETTER TTA
- {0x0BA3, 0x0BA4, prAL, gcLo}, // [2] TAMIL LETTER NNA..TAMIL LETTER TA
- {0x0BA8, 0x0BAA, prAL, gcLo}, // [3] TAMIL LETTER NA..TAMIL LETTER PA
- {0x0BAE, 0x0BB9, prAL, gcLo}, // [12] TAMIL LETTER MA..TAMIL LETTER HA
- {0x0BBE, 0x0BBF, prCM, gcMc}, // [2] TAMIL VOWEL SIGN AA..TAMIL VOWEL SIGN I
- {0x0BC0, 0x0BC0, prCM, gcMn}, // TAMIL VOWEL SIGN II
- {0x0BC1, 0x0BC2, prCM, gcMc}, // [2] TAMIL VOWEL SIGN U..TAMIL VOWEL SIGN UU
- {0x0BC6, 0x0BC8, prCM, gcMc}, // [3] TAMIL VOWEL SIGN E..TAMIL VOWEL SIGN AI
- {0x0BCA, 0x0BCC, prCM, gcMc}, // [3] TAMIL VOWEL SIGN O..TAMIL VOWEL SIGN AU
- {0x0BCD, 0x0BCD, prCM, gcMn}, // TAMIL SIGN VIRAMA
- {0x0BD0, 0x0BD0, prAL, gcLo}, // TAMIL OM
- {0x0BD7, 0x0BD7, prCM, gcMc}, // TAMIL AU LENGTH MARK
- {0x0BE6, 0x0BEF, prNU, gcNd}, // [10] TAMIL DIGIT ZERO..TAMIL DIGIT NINE
- {0x0BF0, 0x0BF2, prAL, gcNo}, // [3] TAMIL NUMBER TEN..TAMIL NUMBER ONE THOUSAND
- {0x0BF3, 0x0BF8, prAL, gcSo}, // [6] TAMIL DAY SIGN..TAMIL AS ABOVE SIGN
- {0x0BF9, 0x0BF9, prPR, gcSc}, // TAMIL RUPEE SIGN
- {0x0BFA, 0x0BFA, prAL, gcSo}, // TAMIL NUMBER SIGN
- {0x0C00, 0x0C00, prCM, gcMn}, // TELUGU SIGN COMBINING CANDRABINDU ABOVE
- {0x0C01, 0x0C03, prCM, gcMc}, // [3] TELUGU SIGN CANDRABINDU..TELUGU SIGN VISARGA
- {0x0C04, 0x0C04, prCM, gcMn}, // TELUGU SIGN COMBINING ANUSVARA ABOVE
- {0x0C05, 0x0C0C, prAL, gcLo}, // [8] TELUGU LETTER A..TELUGU LETTER VOCALIC L
- {0x0C0E, 0x0C10, prAL, gcLo}, // [3] TELUGU LETTER E..TELUGU LETTER AI
- {0x0C12, 0x0C28, prAL, gcLo}, // [23] TELUGU LETTER O..TELUGU LETTER NA
- {0x0C2A, 0x0C39, prAL, gcLo}, // [16] TELUGU LETTER PA..TELUGU LETTER HA
- {0x0C3C, 0x0C3C, prCM, gcMn}, // TELUGU SIGN NUKTA
- {0x0C3D, 0x0C3D, prAL, gcLo}, // TELUGU SIGN AVAGRAHA
- {0x0C3E, 0x0C40, prCM, gcMn}, // [3] TELUGU VOWEL SIGN AA..TELUGU VOWEL SIGN II
- {0x0C41, 0x0C44, prCM, gcMc}, // [4] TELUGU VOWEL SIGN U..TELUGU VOWEL SIGN VOCALIC RR
- {0x0C46, 0x0C48, prCM, gcMn}, // [3] TELUGU VOWEL SIGN E..TELUGU VOWEL SIGN AI
- {0x0C4A, 0x0C4D, prCM, gcMn}, // [4] TELUGU VOWEL SIGN O..TELUGU SIGN VIRAMA
- {0x0C55, 0x0C56, prCM, gcMn}, // [2] TELUGU LENGTH MARK..TELUGU AI LENGTH MARK
- {0x0C58, 0x0C5A, prAL, gcLo}, // [3] TELUGU LETTER TSA..TELUGU LETTER RRRA
- {0x0C5D, 0x0C5D, prAL, gcLo}, // TELUGU LETTER NAKAARA POLLU
- {0x0C60, 0x0C61, prAL, gcLo}, // [2] TELUGU LETTER VOCALIC RR..TELUGU LETTER VOCALIC LL
- {0x0C62, 0x0C63, prCM, gcMn}, // [2] TELUGU VOWEL SIGN VOCALIC L..TELUGU VOWEL SIGN VOCALIC LL
- {0x0C66, 0x0C6F, prNU, gcNd}, // [10] TELUGU DIGIT ZERO..TELUGU DIGIT NINE
- {0x0C77, 0x0C77, prBB, gcPo}, // TELUGU SIGN SIDDHAM
- {0x0C78, 0x0C7E, prAL, gcNo}, // [7] TELUGU FRACTION DIGIT ZERO FOR ODD POWERS OF FOUR..TELUGU FRACTION DIGIT THREE FOR EVEN POWERS OF FOUR
- {0x0C7F, 0x0C7F, prAL, gcSo}, // TELUGU SIGN TUUMU
- {0x0C80, 0x0C80, prAL, gcLo}, // KANNADA SIGN SPACING CANDRABINDU
- {0x0C81, 0x0C81, prCM, gcMn}, // KANNADA SIGN CANDRABINDU
- {0x0C82, 0x0C83, prCM, gcMc}, // [2] KANNADA SIGN ANUSVARA..KANNADA SIGN VISARGA
- {0x0C84, 0x0C84, prBB, gcPo}, // KANNADA SIGN SIDDHAM
- {0x0C85, 0x0C8C, prAL, gcLo}, // [8] KANNADA LETTER A..KANNADA LETTER VOCALIC L
- {0x0C8E, 0x0C90, prAL, gcLo}, // [3] KANNADA LETTER E..KANNADA LETTER AI
- {0x0C92, 0x0CA8, prAL, gcLo}, // [23] KANNADA LETTER O..KANNADA LETTER NA
- {0x0CAA, 0x0CB3, prAL, gcLo}, // [10] KANNADA LETTER PA..KANNADA LETTER LLA
- {0x0CB5, 0x0CB9, prAL, gcLo}, // [5] KANNADA LETTER VA..KANNADA LETTER HA
- {0x0CBC, 0x0CBC, prCM, gcMn}, // KANNADA SIGN NUKTA
- {0x0CBD, 0x0CBD, prAL, gcLo}, // KANNADA SIGN AVAGRAHA
- {0x0CBE, 0x0CBE, prCM, gcMc}, // KANNADA VOWEL SIGN AA
- {0x0CBF, 0x0CBF, prCM, gcMn}, // KANNADA VOWEL SIGN I
- {0x0CC0, 0x0CC4, prCM, gcMc}, // [5] KANNADA VOWEL SIGN II..KANNADA VOWEL SIGN VOCALIC RR
- {0x0CC6, 0x0CC6, prCM, gcMn}, // KANNADA VOWEL SIGN E
- {0x0CC7, 0x0CC8, prCM, gcMc}, // [2] KANNADA VOWEL SIGN EE..KANNADA VOWEL SIGN AI
- {0x0CCA, 0x0CCB, prCM, gcMc}, // [2] KANNADA VOWEL SIGN O..KANNADA VOWEL SIGN OO
- {0x0CCC, 0x0CCD, prCM, gcMn}, // [2] KANNADA VOWEL SIGN AU..KANNADA SIGN VIRAMA
- {0x0CD5, 0x0CD6, prCM, gcMc}, // [2] KANNADA LENGTH MARK..KANNADA AI LENGTH MARK
- {0x0CDD, 0x0CDE, prAL, gcLo}, // [2] KANNADA LETTER NAKAARA POLLU..KANNADA LETTER FA
- {0x0CE0, 0x0CE1, prAL, gcLo}, // [2] KANNADA LETTER VOCALIC RR..KANNADA LETTER VOCALIC LL
- {0x0CE2, 0x0CE3, prCM, gcMn}, // [2] KANNADA VOWEL SIGN VOCALIC L..KANNADA VOWEL SIGN VOCALIC LL
- {0x0CE6, 0x0CEF, prNU, gcNd}, // [10] KANNADA DIGIT ZERO..KANNADA DIGIT NINE
- {0x0CF1, 0x0CF2, prAL, gcLo}, // [2] KANNADA SIGN JIHVAMULIYA..KANNADA SIGN UPADHMANIYA
- {0x0D00, 0x0D01, prCM, gcMn}, // [2] MALAYALAM SIGN COMBINING ANUSVARA ABOVE..MALAYALAM SIGN CANDRABINDU
- {0x0D02, 0x0D03, prCM, gcMc}, // [2] MALAYALAM SIGN ANUSVARA..MALAYALAM SIGN VISARGA
- {0x0D04, 0x0D0C, prAL, gcLo}, // [9] MALAYALAM LETTER VEDIC ANUSVARA..MALAYALAM LETTER VOCALIC L
- {0x0D0E, 0x0D10, prAL, gcLo}, // [3] MALAYALAM LETTER E..MALAYALAM LETTER AI
- {0x0D12, 0x0D3A, prAL, gcLo}, // [41] MALAYALAM LETTER O..MALAYALAM LETTER TTTA
- {0x0D3B, 0x0D3C, prCM, gcMn}, // [2] MALAYALAM SIGN VERTICAL BAR VIRAMA..MALAYALAM SIGN CIRCULAR VIRAMA
- {0x0D3D, 0x0D3D, prAL, gcLo}, // MALAYALAM SIGN AVAGRAHA
- {0x0D3E, 0x0D40, prCM, gcMc}, // [3] MALAYALAM VOWEL SIGN AA..MALAYALAM VOWEL SIGN II
- {0x0D41, 0x0D44, prCM, gcMn}, // [4] MALAYALAM VOWEL SIGN U..MALAYALAM VOWEL SIGN VOCALIC RR
- {0x0D46, 0x0D48, prCM, gcMc}, // [3] MALAYALAM VOWEL SIGN E..MALAYALAM VOWEL SIGN AI
- {0x0D4A, 0x0D4C, prCM, gcMc}, // [3] MALAYALAM VOWEL SIGN O..MALAYALAM VOWEL SIGN AU
- {0x0D4D, 0x0D4D, prCM, gcMn}, // MALAYALAM SIGN VIRAMA
- {0x0D4E, 0x0D4E, prAL, gcLo}, // MALAYALAM LETTER DOT REPH
- {0x0D4F, 0x0D4F, prAL, gcSo}, // MALAYALAM SIGN PARA
- {0x0D54, 0x0D56, prAL, gcLo}, // [3] MALAYALAM LETTER CHILLU M..MALAYALAM LETTER CHILLU LLL
- {0x0D57, 0x0D57, prCM, gcMc}, // MALAYALAM AU LENGTH MARK
- {0x0D58, 0x0D5E, prAL, gcNo}, // [7] MALAYALAM FRACTION ONE ONE-HUNDRED-AND-SIXTIETH..MALAYALAM FRACTION ONE FIFTH
- {0x0D5F, 0x0D61, prAL, gcLo}, // [3] MALAYALAM LETTER ARCHAIC II..MALAYALAM LETTER VOCALIC LL
- {0x0D62, 0x0D63, prCM, gcMn}, // [2] MALAYALAM VOWEL SIGN VOCALIC L..MALAYALAM VOWEL SIGN VOCALIC LL
- {0x0D66, 0x0D6F, prNU, gcNd}, // [10] MALAYALAM DIGIT ZERO..MALAYALAM DIGIT NINE
- {0x0D70, 0x0D78, prAL, gcNo}, // [9] MALAYALAM NUMBER TEN..MALAYALAM FRACTION THREE SIXTEENTHS
- {0x0D79, 0x0D79, prPO, gcSo}, // MALAYALAM DATE MARK
- {0x0D7A, 0x0D7F, prAL, gcLo}, // [6] MALAYALAM LETTER CHILLU NN..MALAYALAM LETTER CHILLU K
- {0x0D81, 0x0D81, prCM, gcMn}, // SINHALA SIGN CANDRABINDU
- {0x0D82, 0x0D83, prCM, gcMc}, // [2] SINHALA SIGN ANUSVARAYA..SINHALA SIGN VISARGAYA
- {0x0D85, 0x0D96, prAL, gcLo}, // [18] SINHALA LETTER AYANNA..SINHALA LETTER AUYANNA
- {0x0D9A, 0x0DB1, prAL, gcLo}, // [24] SINHALA LETTER ALPAPRAANA KAYANNA..SINHALA LETTER DANTAJA NAYANNA
- {0x0DB3, 0x0DBB, prAL, gcLo}, // [9] SINHALA LETTER SANYAKA DAYANNA..SINHALA LETTER RAYANNA
- {0x0DBD, 0x0DBD, prAL, gcLo}, // SINHALA LETTER DANTAJA LAYANNA
- {0x0DC0, 0x0DC6, prAL, gcLo}, // [7] SINHALA LETTER VAYANNA..SINHALA LETTER FAYANNA
- {0x0DCA, 0x0DCA, prCM, gcMn}, // SINHALA SIGN AL-LAKUNA
- {0x0DCF, 0x0DD1, prCM, gcMc}, // [3] SINHALA VOWEL SIGN AELA-PILLA..SINHALA VOWEL SIGN DIGA AEDA-PILLA
- {0x0DD2, 0x0DD4, prCM, gcMn}, // [3] SINHALA VOWEL SIGN KETTI IS-PILLA..SINHALA VOWEL SIGN KETTI PAA-PILLA
- {0x0DD6, 0x0DD6, prCM, gcMn}, // SINHALA VOWEL SIGN DIGA PAA-PILLA
- {0x0DD8, 0x0DDF, prCM, gcMc}, // [8] SINHALA VOWEL SIGN GAETTA-PILLA..SINHALA VOWEL SIGN GAYANUKITTA
- {0x0DE6, 0x0DEF, prNU, gcNd}, // [10] SINHALA LITH DIGIT ZERO..SINHALA LITH DIGIT NINE
- {0x0DF2, 0x0DF3, prCM, gcMc}, // [2] SINHALA VOWEL SIGN DIGA GAETTA-PILLA..SINHALA VOWEL SIGN DIGA GAYANUKITTA
- {0x0DF4, 0x0DF4, prAL, gcPo}, // SINHALA PUNCTUATION KUNDDALIYA
- {0x0E01, 0x0E30, prSA, gcLo}, // [48] THAI CHARACTER KO KAI..THAI CHARACTER SARA A
- {0x0E31, 0x0E31, prSA, gcMn}, // THAI CHARACTER MAI HAN-AKAT
- {0x0E32, 0x0E33, prSA, gcLo}, // [2] THAI CHARACTER SARA AA..THAI CHARACTER SARA AM
- {0x0E34, 0x0E3A, prSA, gcMn}, // [7] THAI CHARACTER SARA I..THAI CHARACTER PHINTHU
- {0x0E3F, 0x0E3F, prPR, gcSc}, // THAI CURRENCY SYMBOL BAHT
- {0x0E40, 0x0E45, prSA, gcLo}, // [6] THAI CHARACTER SARA E..THAI CHARACTER LAKKHANGYAO
- {0x0E46, 0x0E46, prSA, gcLm}, // THAI CHARACTER MAIYAMOK
- {0x0E47, 0x0E4E, prSA, gcMn}, // [8] THAI CHARACTER MAITAIKHU..THAI CHARACTER YAMAKKAN
- {0x0E4F, 0x0E4F, prAL, gcPo}, // THAI CHARACTER FONGMAN
- {0x0E50, 0x0E59, prNU, gcNd}, // [10] THAI DIGIT ZERO..THAI DIGIT NINE
- {0x0E5A, 0x0E5B, prBA, gcPo}, // [2] THAI CHARACTER ANGKHANKHU..THAI CHARACTER KHOMUT
- {0x0E81, 0x0E82, prSA, gcLo}, // [2] LAO LETTER KO..LAO LETTER KHO SUNG
- {0x0E84, 0x0E84, prSA, gcLo}, // LAO LETTER KHO TAM
- {0x0E86, 0x0E8A, prSA, gcLo}, // [5] LAO LETTER PALI GHA..LAO LETTER SO TAM
- {0x0E8C, 0x0EA3, prSA, gcLo}, // [24] LAO LETTER PALI JHA..LAO LETTER LO LING
- {0x0EA5, 0x0EA5, prSA, gcLo}, // LAO LETTER LO LOOT
- {0x0EA7, 0x0EB0, prSA, gcLo}, // [10] LAO LETTER WO..LAO VOWEL SIGN A
- {0x0EB1, 0x0EB1, prSA, gcMn}, // LAO VOWEL SIGN MAI KAN
- {0x0EB2, 0x0EB3, prSA, gcLo}, // [2] LAO VOWEL SIGN AA..LAO VOWEL SIGN AM
- {0x0EB4, 0x0EBC, prSA, gcMn}, // [9] LAO VOWEL SIGN I..LAO SEMIVOWEL SIGN LO
- {0x0EBD, 0x0EBD, prSA, gcLo}, // LAO SEMIVOWEL SIGN NYO
- {0x0EC0, 0x0EC4, prSA, gcLo}, // [5] LAO VOWEL SIGN E..LAO VOWEL SIGN AI
- {0x0EC6, 0x0EC6, prSA, gcLm}, // LAO KO LA
- {0x0EC8, 0x0ECD, prSA, gcMn}, // [6] LAO TONE MAI EK..LAO NIGGAHITA
- {0x0ED0, 0x0ED9, prNU, gcNd}, // [10] LAO DIGIT ZERO..LAO DIGIT NINE
- {0x0EDC, 0x0EDF, prSA, gcLo}, // [4] LAO HO NO..LAO LETTER KHMU NYO
- {0x0F00, 0x0F00, prAL, gcLo}, // TIBETAN SYLLABLE OM
- {0x0F01, 0x0F03, prBB, gcSo}, // [3] TIBETAN MARK GTER YIG MGO TRUNCATED A..TIBETAN MARK GTER YIG MGO -UM GTER TSHEG MA
- {0x0F04, 0x0F04, prBB, gcPo}, // TIBETAN MARK INITIAL YIG MGO MDUN MA
- {0x0F05, 0x0F05, prAL, gcPo}, // TIBETAN MARK CLOSING YIG MGO SGAB MA
- {0x0F06, 0x0F07, prBB, gcPo}, // [2] TIBETAN MARK CARET YIG MGO PHUR SHAD MA..TIBETAN MARK YIG MGO TSHEG SHAD MA
- {0x0F08, 0x0F08, prGL, gcPo}, // TIBETAN MARK SBRUL SHAD
- {0x0F09, 0x0F0A, prBB, gcPo}, // [2] TIBETAN MARK BSKUR YIG MGO..TIBETAN MARK BKA- SHOG YIG MGO
- {0x0F0B, 0x0F0B, prBA, gcPo}, // TIBETAN MARK INTERSYLLABIC TSHEG
- {0x0F0C, 0x0F0C, prGL, gcPo}, // TIBETAN MARK DELIMITER TSHEG BSTAR
- {0x0F0D, 0x0F11, prEX, gcPo}, // [5] TIBETAN MARK SHAD..TIBETAN MARK RIN CHEN SPUNGS SHAD
- {0x0F12, 0x0F12, prGL, gcPo}, // TIBETAN MARK RGYA GRAM SHAD
- {0x0F13, 0x0F13, prAL, gcSo}, // TIBETAN MARK CARET -DZUD RTAGS ME LONG CAN
- {0x0F14, 0x0F14, prEX, gcPo}, // TIBETAN MARK GTER TSHEG
- {0x0F15, 0x0F17, prAL, gcSo}, // [3] TIBETAN LOGOTYPE SIGN CHAD RTAGS..TIBETAN ASTROLOGICAL SIGN SGRA GCAN -CHAR RTAGS
- {0x0F18, 0x0F19, prCM, gcMn}, // [2] TIBETAN ASTROLOGICAL SIGN -KHYUD PA..TIBETAN ASTROLOGICAL SIGN SDONG TSHUGS
- {0x0F1A, 0x0F1F, prAL, gcSo}, // [6] TIBETAN SIGN RDEL DKAR GCIG..TIBETAN SIGN RDEL DKAR RDEL NAG
- {0x0F20, 0x0F29, prNU, gcNd}, // [10] TIBETAN DIGIT ZERO..TIBETAN DIGIT NINE
- {0x0F2A, 0x0F33, prAL, gcNo}, // [10] TIBETAN DIGIT HALF ONE..TIBETAN DIGIT HALF ZERO
- {0x0F34, 0x0F34, prBA, gcSo}, // TIBETAN MARK BSDUS RTAGS
- {0x0F35, 0x0F35, prCM, gcMn}, // TIBETAN MARK NGAS BZUNG NYI ZLA
- {0x0F36, 0x0F36, prAL, gcSo}, // TIBETAN MARK CARET -DZUD RTAGS BZHI MIG CAN
- {0x0F37, 0x0F37, prCM, gcMn}, // TIBETAN MARK NGAS BZUNG SGOR RTAGS
- {0x0F38, 0x0F38, prAL, gcSo}, // TIBETAN MARK CHE MGO
- {0x0F39, 0x0F39, prCM, gcMn}, // TIBETAN MARK TSA -PHRU
- {0x0F3A, 0x0F3A, prOP, gcPs}, // TIBETAN MARK GUG RTAGS GYON
- {0x0F3B, 0x0F3B, prCL, gcPe}, // TIBETAN MARK GUG RTAGS GYAS
- {0x0F3C, 0x0F3C, prOP, gcPs}, // TIBETAN MARK ANG KHANG GYON
- {0x0F3D, 0x0F3D, prCL, gcPe}, // TIBETAN MARK ANG KHANG GYAS
- {0x0F3E, 0x0F3F, prCM, gcMc}, // [2] TIBETAN SIGN YAR TSHES..TIBETAN SIGN MAR TSHES
- {0x0F40, 0x0F47, prAL, gcLo}, // [8] TIBETAN LETTER KA..TIBETAN LETTER JA
- {0x0F49, 0x0F6C, prAL, gcLo}, // [36] TIBETAN LETTER NYA..TIBETAN LETTER RRA
- {0x0F71, 0x0F7E, prCM, gcMn}, // [14] TIBETAN VOWEL SIGN AA..TIBETAN SIGN RJES SU NGA RO
- {0x0F7F, 0x0F7F, prBA, gcMc}, // TIBETAN SIGN RNAM BCAD
- {0x0F80, 0x0F84, prCM, gcMn}, // [5] TIBETAN VOWEL SIGN REVERSED I..TIBETAN MARK HALANTA
- {0x0F85, 0x0F85, prBA, gcPo}, // TIBETAN MARK PALUTA
- {0x0F86, 0x0F87, prCM, gcMn}, // [2] TIBETAN SIGN LCI RTAGS..TIBETAN SIGN YANG RTAGS
- {0x0F88, 0x0F8C, prAL, gcLo}, // [5] TIBETAN SIGN LCE TSA CAN..TIBETAN SIGN INVERTED MCHU CAN
- {0x0F8D, 0x0F97, prCM, gcMn}, // [11] TIBETAN SUBJOINED SIGN LCE TSA CAN..TIBETAN SUBJOINED LETTER JA
- {0x0F99, 0x0FBC, prCM, gcMn}, // [36] TIBETAN SUBJOINED LETTER NYA..TIBETAN SUBJOINED LETTER FIXED-FORM RA
- {0x0FBE, 0x0FBF, prBA, gcSo}, // [2] TIBETAN KU RU KHA..TIBETAN KU RU KHA BZHI MIG CAN
- {0x0FC0, 0x0FC5, prAL, gcSo}, // [6] TIBETAN CANTILLATION SIGN HEAVY BEAT..TIBETAN SYMBOL RDO RJE
- {0x0FC6, 0x0FC6, prCM, gcMn}, // TIBETAN SYMBOL PADMA GDAN
- {0x0FC7, 0x0FCC, prAL, gcSo}, // [6] TIBETAN SYMBOL RDO RJE RGYA GRAM..TIBETAN SYMBOL NOR BU BZHI -KHYIL
- {0x0FCE, 0x0FCF, prAL, gcSo}, // [2] TIBETAN SIGN RDEL NAG RDEL DKAR..TIBETAN SIGN RDEL NAG GSUM
- {0x0FD0, 0x0FD1, prBB, gcPo}, // [2] TIBETAN MARK BSKA- SHOG GI MGO RGYAN..TIBETAN MARK MNYAM YIG GI MGO RGYAN
- {0x0FD2, 0x0FD2, prBA, gcPo}, // TIBETAN MARK NYIS TSHEG
- {0x0FD3, 0x0FD3, prBB, gcPo}, // TIBETAN MARK INITIAL BRDA RNYING YIG MGO MDUN MA
- {0x0FD4, 0x0FD4, prAL, gcPo}, // TIBETAN MARK CLOSING BRDA RNYING YIG MGO SGAB MA
- {0x0FD5, 0x0FD8, prAL, gcSo}, // [4] RIGHT-FACING SVASTI SIGN..LEFT-FACING SVASTI SIGN WITH DOTS
- {0x0FD9, 0x0FDA, prGL, gcPo}, // [2] TIBETAN MARK LEADING MCHAN RTAGS..TIBETAN MARK TRAILING MCHAN RTAGS
- {0x1000, 0x102A, prSA, gcLo}, // [43] MYANMAR LETTER KA..MYANMAR LETTER AU
- {0x102B, 0x102C, prSA, gcMc}, // [2] MYANMAR VOWEL SIGN TALL AA..MYANMAR VOWEL SIGN AA
- {0x102D, 0x1030, prSA, gcMn}, // [4] MYANMAR VOWEL SIGN I..MYANMAR VOWEL SIGN UU
- {0x1031, 0x1031, prSA, gcMc}, // MYANMAR VOWEL SIGN E
- {0x1032, 0x1037, prSA, gcMn}, // [6] MYANMAR VOWEL SIGN AI..MYANMAR SIGN DOT BELOW
- {0x1038, 0x1038, prSA, gcMc}, // MYANMAR SIGN VISARGA
- {0x1039, 0x103A, prSA, gcMn}, // [2] MYANMAR SIGN VIRAMA..MYANMAR SIGN ASAT
- {0x103B, 0x103C, prSA, gcMc}, // [2] MYANMAR CONSONANT SIGN MEDIAL YA..MYANMAR CONSONANT SIGN MEDIAL RA
- {0x103D, 0x103E, prSA, gcMn}, // [2] MYANMAR CONSONANT SIGN MEDIAL WA..MYANMAR CONSONANT SIGN MEDIAL HA
- {0x103F, 0x103F, prSA, gcLo}, // MYANMAR LETTER GREAT SA
- {0x1040, 0x1049, prNU, gcNd}, // [10] MYANMAR DIGIT ZERO..MYANMAR DIGIT NINE
- {0x104A, 0x104B, prBA, gcPo}, // [2] MYANMAR SIGN LITTLE SECTION..MYANMAR SIGN SECTION
- {0x104C, 0x104F, prAL, gcPo}, // [4] MYANMAR SYMBOL LOCATIVE..MYANMAR SYMBOL GENITIVE
- {0x1050, 0x1055, prSA, gcLo}, // [6] MYANMAR LETTER SHA..MYANMAR LETTER VOCALIC LL
- {0x1056, 0x1057, prSA, gcMc}, // [2] MYANMAR VOWEL SIGN VOCALIC R..MYANMAR VOWEL SIGN VOCALIC RR
- {0x1058, 0x1059, prSA, gcMn}, // [2] MYANMAR VOWEL SIGN VOCALIC L..MYANMAR VOWEL SIGN VOCALIC LL
- {0x105A, 0x105D, prSA, gcLo}, // [4] MYANMAR LETTER MON NGA..MYANMAR LETTER MON BBE
- {0x105E, 0x1060, prSA, gcMn}, // [3] MYANMAR CONSONANT SIGN MON MEDIAL NA..MYANMAR CONSONANT SIGN MON MEDIAL LA
- {0x1061, 0x1061, prSA, gcLo}, // MYANMAR LETTER SGAW KAREN SHA
- {0x1062, 0x1064, prSA, gcMc}, // [3] MYANMAR VOWEL SIGN SGAW KAREN EU..MYANMAR TONE MARK SGAW KAREN KE PHO
- {0x1065, 0x1066, prSA, gcLo}, // [2] MYANMAR LETTER WESTERN PWO KAREN THA..MYANMAR LETTER WESTERN PWO KAREN PWA
- {0x1067, 0x106D, prSA, gcMc}, // [7] MYANMAR VOWEL SIGN WESTERN PWO KAREN EU..MYANMAR SIGN WESTERN PWO KAREN TONE-5
- {0x106E, 0x1070, prSA, gcLo}, // [3] MYANMAR LETTER EASTERN PWO KAREN NNA..MYANMAR LETTER EASTERN PWO KAREN GHWA
- {0x1071, 0x1074, prSA, gcMn}, // [4] MYANMAR VOWEL SIGN GEBA KAREN I..MYANMAR VOWEL SIGN KAYAH EE
- {0x1075, 0x1081, prSA, gcLo}, // [13] MYANMAR LETTER SHAN KA..MYANMAR LETTER SHAN HA
- {0x1082, 0x1082, prSA, gcMn}, // MYANMAR CONSONANT SIGN SHAN MEDIAL WA
- {0x1083, 0x1084, prSA, gcMc}, // [2] MYANMAR VOWEL SIGN SHAN AA..MYANMAR VOWEL SIGN SHAN E
- {0x1085, 0x1086, prSA, gcMn}, // [2] MYANMAR VOWEL SIGN SHAN E ABOVE..MYANMAR VOWEL SIGN SHAN FINAL Y
- {0x1087, 0x108C, prSA, gcMc}, // [6] MYANMAR SIGN SHAN TONE-2..MYANMAR SIGN SHAN COUNCIL TONE-3
- {0x108D, 0x108D, prSA, gcMn}, // MYANMAR SIGN SHAN COUNCIL EMPHATIC TONE
- {0x108E, 0x108E, prSA, gcLo}, // MYANMAR LETTER RUMAI PALAUNG FA
- {0x108F, 0x108F, prSA, gcMc}, // MYANMAR SIGN RUMAI PALAUNG TONE-5
- {0x1090, 0x1099, prNU, gcNd}, // [10] MYANMAR SHAN DIGIT ZERO..MYANMAR SHAN DIGIT NINE
- {0x109A, 0x109C, prSA, gcMc}, // [3] MYANMAR SIGN KHAMTI TONE-1..MYANMAR VOWEL SIGN AITON A
- {0x109D, 0x109D, prSA, gcMn}, // MYANMAR VOWEL SIGN AITON AI
- {0x109E, 0x109F, prSA, gcSo}, // [2] MYANMAR SYMBOL SHAN ONE..MYANMAR SYMBOL SHAN EXCLAMATION
- {0x10A0, 0x10C5, prAL, gcLu}, // [38] GEORGIAN CAPITAL LETTER AN..GEORGIAN CAPITAL LETTER HOE
- {0x10C7, 0x10C7, prAL, gcLu}, // GEORGIAN CAPITAL LETTER YN
- {0x10CD, 0x10CD, prAL, gcLu}, // GEORGIAN CAPITAL LETTER AEN
- {0x10D0, 0x10FA, prAL, gcLl}, // [43] GEORGIAN LETTER AN..GEORGIAN LETTER AIN
- {0x10FB, 0x10FB, prAL, gcPo}, // GEORGIAN PARAGRAPH SEPARATOR
- {0x10FC, 0x10FC, prAL, gcLm}, // MODIFIER LETTER GEORGIAN NAR
- {0x10FD, 0x10FF, prAL, gcLl}, // [3] GEORGIAN LETTER AEN..GEORGIAN LETTER LABIAL SIGN
- {0x1100, 0x115F, prJL, gcLo}, // [96] HANGUL CHOSEONG KIYEOK..HANGUL CHOSEONG FILLER
- {0x1160, 0x11A7, prJV, gcLo}, // [72] HANGUL JUNGSEONG FILLER..HANGUL JUNGSEONG O-YAE
- {0x11A8, 0x11FF, prJT, gcLo}, // [88] HANGUL JONGSEONG KIYEOK..HANGUL JONGSEONG SSANGNIEUN
- {0x1200, 0x1248, prAL, gcLo}, // [73] ETHIOPIC SYLLABLE HA..ETHIOPIC SYLLABLE QWA
- {0x124A, 0x124D, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE QWI..ETHIOPIC SYLLABLE QWE
- {0x1250, 0x1256, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE QHA..ETHIOPIC SYLLABLE QHO
- {0x1258, 0x1258, prAL, gcLo}, // ETHIOPIC SYLLABLE QHWA
- {0x125A, 0x125D, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE QHWI..ETHIOPIC SYLLABLE QHWE
- {0x1260, 0x1288, prAL, gcLo}, // [41] ETHIOPIC SYLLABLE BA..ETHIOPIC SYLLABLE XWA
- {0x128A, 0x128D, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE XWI..ETHIOPIC SYLLABLE XWE
- {0x1290, 0x12B0, prAL, gcLo}, // [33] ETHIOPIC SYLLABLE NA..ETHIOPIC SYLLABLE KWA
- {0x12B2, 0x12B5, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE KWI..ETHIOPIC SYLLABLE KWE
- {0x12B8, 0x12BE, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE KXA..ETHIOPIC SYLLABLE KXO
- {0x12C0, 0x12C0, prAL, gcLo}, // ETHIOPIC SYLLABLE KXWA
- {0x12C2, 0x12C5, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE KXWI..ETHIOPIC SYLLABLE KXWE
- {0x12C8, 0x12D6, prAL, gcLo}, // [15] ETHIOPIC SYLLABLE WA..ETHIOPIC SYLLABLE PHARYNGEAL O
- {0x12D8, 0x1310, prAL, gcLo}, // [57] ETHIOPIC SYLLABLE ZA..ETHIOPIC SYLLABLE GWA
- {0x1312, 0x1315, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE GWI..ETHIOPIC SYLLABLE GWE
- {0x1318, 0x135A, prAL, gcLo}, // [67] ETHIOPIC SYLLABLE GGA..ETHIOPIC SYLLABLE FYA
- {0x135D, 0x135F, prCM, gcMn}, // [3] ETHIOPIC COMBINING GEMINATION AND VOWEL LENGTH MARK..ETHIOPIC COMBINING GEMINATION MARK
- {0x1360, 0x1360, prAL, gcPo}, // ETHIOPIC SECTION MARK
- {0x1361, 0x1361, prBA, gcPo}, // ETHIOPIC WORDSPACE
- {0x1362, 0x1368, prAL, gcPo}, // [7] ETHIOPIC FULL STOP..ETHIOPIC PARAGRAPH SEPARATOR
- {0x1369, 0x137C, prAL, gcNo}, // [20] ETHIOPIC DIGIT ONE..ETHIOPIC NUMBER TEN THOUSAND
- {0x1380, 0x138F, prAL, gcLo}, // [16] ETHIOPIC SYLLABLE SEBATBEIT MWA..ETHIOPIC SYLLABLE PWE
- {0x1390, 0x1399, prAL, gcSo}, // [10] ETHIOPIC TONAL MARK YIZET..ETHIOPIC TONAL MARK KURT
- {0x13A0, 0x13F5, prAL, gcLu}, // [86] CHEROKEE LETTER A..CHEROKEE LETTER MV
- {0x13F8, 0x13FD, prAL, gcLl}, // [6] CHEROKEE SMALL LETTER YE..CHEROKEE SMALL LETTER MV
- {0x1400, 0x1400, prBA, gcPd}, // CANADIAN SYLLABICS HYPHEN
- {0x1401, 0x166C, prAL, gcLo}, // [620] CANADIAN SYLLABICS E..CANADIAN SYLLABICS CARRIER TTSA
- {0x166D, 0x166D, prAL, gcSo}, // CANADIAN SYLLABICS CHI SIGN
- {0x166E, 0x166E, prAL, gcPo}, // CANADIAN SYLLABICS FULL STOP
- {0x166F, 0x167F, prAL, gcLo}, // [17] CANADIAN SYLLABICS QAI..CANADIAN SYLLABICS BLACKFOOT W
- {0x1680, 0x1680, prBA, gcZs}, // OGHAM SPACE MARK
- {0x1681, 0x169A, prAL, gcLo}, // [26] OGHAM LETTER BEITH..OGHAM LETTER PEITH
- {0x169B, 0x169B, prOP, gcPs}, // OGHAM FEATHER MARK
- {0x169C, 0x169C, prCL, gcPe}, // OGHAM REVERSED FEATHER MARK
- {0x16A0, 0x16EA, prAL, gcLo}, // [75] RUNIC LETTER FEHU FEOH FE F..RUNIC LETTER X
- {0x16EB, 0x16ED, prBA, gcPo}, // [3] RUNIC SINGLE PUNCTUATION..RUNIC CROSS PUNCTUATION
- {0x16EE, 0x16F0, prAL, gcNl}, // [3] RUNIC ARLAUG SYMBOL..RUNIC BELGTHOR SYMBOL
- {0x16F1, 0x16F8, prAL, gcLo}, // [8] RUNIC LETTER K..RUNIC LETTER FRANKS CASKET AESC
- {0x1700, 0x1711, prAL, gcLo}, // [18] TAGALOG LETTER A..TAGALOG LETTER HA
- {0x1712, 0x1714, prCM, gcMn}, // [3] TAGALOG VOWEL SIGN I..TAGALOG SIGN VIRAMA
- {0x1715, 0x1715, prCM, gcMc}, // TAGALOG SIGN PAMUDPOD
- {0x171F, 0x171F, prAL, gcLo}, // TAGALOG LETTER ARCHAIC RA
- {0x1720, 0x1731, prAL, gcLo}, // [18] HANUNOO LETTER A..HANUNOO LETTER HA
- {0x1732, 0x1733, prCM, gcMn}, // [2] HANUNOO VOWEL SIGN I..HANUNOO VOWEL SIGN U
- {0x1734, 0x1734, prCM, gcMc}, // HANUNOO SIGN PAMUDPOD
- {0x1735, 0x1736, prBA, gcPo}, // [2] PHILIPPINE SINGLE PUNCTUATION..PHILIPPINE DOUBLE PUNCTUATION
- {0x1740, 0x1751, prAL, gcLo}, // [18] BUHID LETTER A..BUHID LETTER HA
- {0x1752, 0x1753, prCM, gcMn}, // [2] BUHID VOWEL SIGN I..BUHID VOWEL SIGN U
- {0x1760, 0x176C, prAL, gcLo}, // [13] TAGBANWA LETTER A..TAGBANWA LETTER YA
- {0x176E, 0x1770, prAL, gcLo}, // [3] TAGBANWA LETTER LA..TAGBANWA LETTER SA
- {0x1772, 0x1773, prCM, gcMn}, // [2] TAGBANWA VOWEL SIGN I..TAGBANWA VOWEL SIGN U
- {0x1780, 0x17B3, prSA, gcLo}, // [52] KHMER LETTER KA..KHMER INDEPENDENT VOWEL QAU
- {0x17B4, 0x17B5, prSA, gcMn}, // [2] KHMER VOWEL INHERENT AQ..KHMER VOWEL INHERENT AA
- {0x17B6, 0x17B6, prSA, gcMc}, // KHMER VOWEL SIGN AA
- {0x17B7, 0x17BD, prSA, gcMn}, // [7] KHMER VOWEL SIGN I..KHMER VOWEL SIGN UA
- {0x17BE, 0x17C5, prSA, gcMc}, // [8] KHMER VOWEL SIGN OE..KHMER VOWEL SIGN AU
- {0x17C6, 0x17C6, prSA, gcMn}, // KHMER SIGN NIKAHIT
- {0x17C7, 0x17C8, prSA, gcMc}, // [2] KHMER SIGN REAHMUK..KHMER SIGN YUUKALEAPINTU
- {0x17C9, 0x17D3, prSA, gcMn}, // [11] KHMER SIGN MUUSIKATOAN..KHMER SIGN BATHAMASAT
- {0x17D4, 0x17D5, prBA, gcPo}, // [2] KHMER SIGN KHAN..KHMER SIGN BARIYOOSAN
- {0x17D6, 0x17D6, prNS, gcPo}, // KHMER SIGN CAMNUC PII KUUH
- {0x17D7, 0x17D7, prSA, gcLm}, // KHMER SIGN LEK TOO
- {0x17D8, 0x17D8, prBA, gcPo}, // KHMER SIGN BEYYAL
- {0x17D9, 0x17D9, prAL, gcPo}, // KHMER SIGN PHNAEK MUAN
- {0x17DA, 0x17DA, prBA, gcPo}, // KHMER SIGN KOOMUUT
- {0x17DB, 0x17DB, prPR, gcSc}, // KHMER CURRENCY SYMBOL RIEL
- {0x17DC, 0x17DC, prSA, gcLo}, // KHMER SIGN AVAKRAHASANYA
- {0x17DD, 0x17DD, prSA, gcMn}, // KHMER SIGN ATTHACAN
- {0x17E0, 0x17E9, prNU, gcNd}, // [10] KHMER DIGIT ZERO..KHMER DIGIT NINE
- {0x17F0, 0x17F9, prAL, gcNo}, // [10] KHMER SYMBOL LEK ATTAK SON..KHMER SYMBOL LEK ATTAK PRAM-BUON
- {0x1800, 0x1801, prAL, gcPo}, // [2] MONGOLIAN BIRGA..MONGOLIAN ELLIPSIS
- {0x1802, 0x1803, prEX, gcPo}, // [2] MONGOLIAN COMMA..MONGOLIAN FULL STOP
- {0x1804, 0x1805, prBA, gcPo}, // [2] MONGOLIAN COLON..MONGOLIAN FOUR DOTS
- {0x1806, 0x1806, prBB, gcPd}, // MONGOLIAN TODO SOFT HYPHEN
- {0x1807, 0x1807, prAL, gcPo}, // MONGOLIAN SIBE SYLLABLE BOUNDARY MARKER
- {0x1808, 0x1809, prEX, gcPo}, // [2] MONGOLIAN MANCHU COMMA..MONGOLIAN MANCHU FULL STOP
- {0x180A, 0x180A, prAL, gcPo}, // MONGOLIAN NIRUGU
- {0x180B, 0x180D, prCM, gcMn}, // [3] MONGOLIAN FREE VARIATION SELECTOR ONE..MONGOLIAN FREE VARIATION SELECTOR THREE
- {0x180E, 0x180E, prGL, gcCf}, // MONGOLIAN VOWEL SEPARATOR
- {0x180F, 0x180F, prCM, gcMn}, // MONGOLIAN FREE VARIATION SELECTOR FOUR
- {0x1810, 0x1819, prNU, gcNd}, // [10] MONGOLIAN DIGIT ZERO..MONGOLIAN DIGIT NINE
- {0x1820, 0x1842, prAL, gcLo}, // [35] MONGOLIAN LETTER A..MONGOLIAN LETTER CHI
- {0x1843, 0x1843, prAL, gcLm}, // MONGOLIAN LETTER TODO LONG VOWEL SIGN
- {0x1844, 0x1878, prAL, gcLo}, // [53] MONGOLIAN LETTER TODO E..MONGOLIAN LETTER CHA WITH TWO DOTS
- {0x1880, 0x1884, prAL, gcLo}, // [5] MONGOLIAN LETTER ALI GALI ANUSVARA ONE..MONGOLIAN LETTER ALI GALI INVERTED UBADAMA
- {0x1885, 0x1886, prCM, gcMn}, // [2] MONGOLIAN LETTER ALI GALI BALUDA..MONGOLIAN LETTER ALI GALI THREE BALUDA
- {0x1887, 0x18A8, prAL, gcLo}, // [34] MONGOLIAN LETTER ALI GALI A..MONGOLIAN LETTER MANCHU ALI GALI BHA
- {0x18A9, 0x18A9, prCM, gcMn}, // MONGOLIAN LETTER ALI GALI DAGALGA
- {0x18AA, 0x18AA, prAL, gcLo}, // MONGOLIAN LETTER MANCHU ALI GALI LHA
- {0x18B0, 0x18F5, prAL, gcLo}, // [70] CANADIAN SYLLABICS OY..CANADIAN SYLLABICS CARRIER DENTAL S
- {0x1900, 0x191E, prAL, gcLo}, // [31] LIMBU VOWEL-CARRIER LETTER..LIMBU LETTER TRA
- {0x1920, 0x1922, prCM, gcMn}, // [3] LIMBU VOWEL SIGN A..LIMBU VOWEL SIGN U
- {0x1923, 0x1926, prCM, gcMc}, // [4] LIMBU VOWEL SIGN EE..LIMBU VOWEL SIGN AU
- {0x1927, 0x1928, prCM, gcMn}, // [2] LIMBU VOWEL SIGN E..LIMBU VOWEL SIGN O
- {0x1929, 0x192B, prCM, gcMc}, // [3] LIMBU SUBJOINED LETTER YA..LIMBU SUBJOINED LETTER WA
- {0x1930, 0x1931, prCM, gcMc}, // [2] LIMBU SMALL LETTER KA..LIMBU SMALL LETTER NGA
- {0x1932, 0x1932, prCM, gcMn}, // LIMBU SMALL LETTER ANUSVARA
- {0x1933, 0x1938, prCM, gcMc}, // [6] LIMBU SMALL LETTER TA..LIMBU SMALL LETTER LA
- {0x1939, 0x193B, prCM, gcMn}, // [3] LIMBU SIGN MUKPHRENG..LIMBU SIGN SA-I
- {0x1940, 0x1940, prAL, gcSo}, // LIMBU SIGN LOO
- {0x1944, 0x1945, prEX, gcPo}, // [2] LIMBU EXCLAMATION MARK..LIMBU QUESTION MARK
- {0x1946, 0x194F, prNU, gcNd}, // [10] LIMBU DIGIT ZERO..LIMBU DIGIT NINE
- {0x1950, 0x196D, prSA, gcLo}, // [30] TAI LE LETTER KA..TAI LE LETTER AI
- {0x1970, 0x1974, prSA, gcLo}, // [5] TAI LE LETTER TONE-2..TAI LE LETTER TONE-6
- {0x1980, 0x19AB, prSA, gcLo}, // [44] NEW TAI LUE LETTER HIGH QA..NEW TAI LUE LETTER LOW SUA
- {0x19B0, 0x19C9, prSA, gcLo}, // [26] NEW TAI LUE VOWEL SIGN VOWEL SHORTENER..NEW TAI LUE TONE MARK-2
- {0x19D0, 0x19D9, prNU, gcNd}, // [10] NEW TAI LUE DIGIT ZERO..NEW TAI LUE DIGIT NINE
- {0x19DA, 0x19DA, prSA, gcNo}, // NEW TAI LUE THAM DIGIT ONE
- {0x19DE, 0x19DF, prSA, gcSo}, // [2] NEW TAI LUE SIGN LAE..NEW TAI LUE SIGN LAEV
- {0x19E0, 0x19FF, prAL, gcSo}, // [32] KHMER SYMBOL PATHAMASAT..KHMER SYMBOL DAP-PRAM ROC
- {0x1A00, 0x1A16, prAL, gcLo}, // [23] BUGINESE LETTER KA..BUGINESE LETTER HA
- {0x1A17, 0x1A18, prCM, gcMn}, // [2] BUGINESE VOWEL SIGN I..BUGINESE VOWEL SIGN U
- {0x1A19, 0x1A1A, prCM, gcMc}, // [2] BUGINESE VOWEL SIGN E..BUGINESE VOWEL SIGN O
- {0x1A1B, 0x1A1B, prCM, gcMn}, // BUGINESE VOWEL SIGN AE
- {0x1A1E, 0x1A1F, prAL, gcPo}, // [2] BUGINESE PALLAWA..BUGINESE END OF SECTION
- {0x1A20, 0x1A54, prSA, gcLo}, // [53] TAI THAM LETTER HIGH KA..TAI THAM LETTER GREAT SA
- {0x1A55, 0x1A55, prSA, gcMc}, // TAI THAM CONSONANT SIGN MEDIAL RA
- {0x1A56, 0x1A56, prSA, gcMn}, // TAI THAM CONSONANT SIGN MEDIAL LA
- {0x1A57, 0x1A57, prSA, gcMc}, // TAI THAM CONSONANT SIGN LA TANG LAI
- {0x1A58, 0x1A5E, prSA, gcMn}, // [7] TAI THAM SIGN MAI KANG LAI..TAI THAM CONSONANT SIGN SA
- {0x1A60, 0x1A60, prSA, gcMn}, // TAI THAM SIGN SAKOT
- {0x1A61, 0x1A61, prSA, gcMc}, // TAI THAM VOWEL SIGN A
- {0x1A62, 0x1A62, prSA, gcMn}, // TAI THAM VOWEL SIGN MAI SAT
- {0x1A63, 0x1A64, prSA, gcMc}, // [2] TAI THAM VOWEL SIGN AA..TAI THAM VOWEL SIGN TALL AA
- {0x1A65, 0x1A6C, prSA, gcMn}, // [8] TAI THAM VOWEL SIGN I..TAI THAM VOWEL SIGN OA BELOW
- {0x1A6D, 0x1A72, prSA, gcMc}, // [6] TAI THAM VOWEL SIGN OY..TAI THAM VOWEL SIGN THAM AI
- {0x1A73, 0x1A7C, prSA, gcMn}, // [10] TAI THAM VOWEL SIGN OA ABOVE..TAI THAM SIGN KHUEN-LUE KARAN
- {0x1A7F, 0x1A7F, prCM, gcMn}, // TAI THAM COMBINING CRYPTOGRAMMIC DOT
- {0x1A80, 0x1A89, prNU, gcNd}, // [10] TAI THAM HORA DIGIT ZERO..TAI THAM HORA DIGIT NINE
- {0x1A90, 0x1A99, prNU, gcNd}, // [10] TAI THAM THAM DIGIT ZERO..TAI THAM THAM DIGIT NINE
- {0x1AA0, 0x1AA6, prSA, gcPo}, // [7] TAI THAM SIGN WIANG..TAI THAM SIGN REVERSED ROTATED RANA
- {0x1AA7, 0x1AA7, prSA, gcLm}, // TAI THAM SIGN MAI YAMOK
- {0x1AA8, 0x1AAD, prSA, gcPo}, // [6] TAI THAM SIGN KAAN..TAI THAM SIGN CAANG
- {0x1AB0, 0x1ABD, prCM, gcMn}, // [14] COMBINING DOUBLED CIRCUMFLEX ACCENT..COMBINING PARENTHESES BELOW
- {0x1ABE, 0x1ABE, prCM, gcMe}, // COMBINING PARENTHESES OVERLAY
- {0x1ABF, 0x1ACE, prCM, gcMn}, // [16] COMBINING LATIN SMALL LETTER W BELOW..COMBINING LATIN SMALL LETTER INSULAR T
- {0x1B00, 0x1B03, prCM, gcMn}, // [4] BALINESE SIGN ULU RICEM..BALINESE SIGN SURANG
- {0x1B04, 0x1B04, prCM, gcMc}, // BALINESE SIGN BISAH
- {0x1B05, 0x1B33, prAL, gcLo}, // [47] BALINESE LETTER AKARA..BALINESE LETTER HA
- {0x1B34, 0x1B34, prCM, gcMn}, // BALINESE SIGN REREKAN
- {0x1B35, 0x1B35, prCM, gcMc}, // BALINESE VOWEL SIGN TEDUNG
- {0x1B36, 0x1B3A, prCM, gcMn}, // [5] BALINESE VOWEL SIGN ULU..BALINESE VOWEL SIGN RA REPA
- {0x1B3B, 0x1B3B, prCM, gcMc}, // BALINESE VOWEL SIGN RA REPA TEDUNG
- {0x1B3C, 0x1B3C, prCM, gcMn}, // BALINESE VOWEL SIGN LA LENGA
- {0x1B3D, 0x1B41, prCM, gcMc}, // [5] BALINESE VOWEL SIGN LA LENGA TEDUNG..BALINESE VOWEL SIGN TALING REPA TEDUNG
- {0x1B42, 0x1B42, prCM, gcMn}, // BALINESE VOWEL SIGN PEPET
- {0x1B43, 0x1B44, prCM, gcMc}, // [2] BALINESE VOWEL SIGN PEPET TEDUNG..BALINESE ADEG ADEG
- {0x1B45, 0x1B4C, prAL, gcLo}, // [8] BALINESE LETTER KAF SASAK..BALINESE LETTER ARCHAIC JNYA
- {0x1B50, 0x1B59, prNU, gcNd}, // [10] BALINESE DIGIT ZERO..BALINESE DIGIT NINE
- {0x1B5A, 0x1B5B, prBA, gcPo}, // [2] BALINESE PANTI..BALINESE PAMADA
- {0x1B5C, 0x1B5C, prAL, gcPo}, // BALINESE WINDU
- {0x1B5D, 0x1B60, prBA, gcPo}, // [4] BALINESE CARIK PAMUNGKAH..BALINESE PAMENENG
- {0x1B61, 0x1B6A, prAL, gcSo}, // [10] BALINESE MUSICAL SYMBOL DONG..BALINESE MUSICAL SYMBOL DANG GEDE
- {0x1B6B, 0x1B73, prCM, gcMn}, // [9] BALINESE MUSICAL SYMBOL COMBINING TEGEH..BALINESE MUSICAL SYMBOL COMBINING GONG
- {0x1B74, 0x1B7C, prAL, gcSo}, // [9] BALINESE MUSICAL SYMBOL RIGHT-HAND OPEN DUG..BALINESE MUSICAL SYMBOL LEFT-HAND OPEN PING
- {0x1B7D, 0x1B7E, prBA, gcPo}, // [2] BALINESE PANTI LANTANG..BALINESE PAMADA LANTANG
- {0x1B80, 0x1B81, prCM, gcMn}, // [2] SUNDANESE SIGN PANYECEK..SUNDANESE SIGN PANGLAYAR
- {0x1B82, 0x1B82, prCM, gcMc}, // SUNDANESE SIGN PANGWISAD
- {0x1B83, 0x1BA0, prAL, gcLo}, // [30] SUNDANESE LETTER A..SUNDANESE LETTER HA
- {0x1BA1, 0x1BA1, prCM, gcMc}, // SUNDANESE CONSONANT SIGN PAMINGKAL
- {0x1BA2, 0x1BA5, prCM, gcMn}, // [4] SUNDANESE CONSONANT SIGN PANYAKRA..SUNDANESE VOWEL SIGN PANYUKU
- {0x1BA6, 0x1BA7, prCM, gcMc}, // [2] SUNDANESE VOWEL SIGN PANAELAENG..SUNDANESE VOWEL SIGN PANOLONG
- {0x1BA8, 0x1BA9, prCM, gcMn}, // [2] SUNDANESE VOWEL SIGN PAMEPET..SUNDANESE VOWEL SIGN PANEULEUNG
- {0x1BAA, 0x1BAA, prCM, gcMc}, // SUNDANESE SIGN PAMAAEH
- {0x1BAB, 0x1BAD, prCM, gcMn}, // [3] SUNDANESE SIGN VIRAMA..SUNDANESE CONSONANT SIGN PASANGAN WA
- {0x1BAE, 0x1BAF, prAL, gcLo}, // [2] SUNDANESE LETTER KHA..SUNDANESE LETTER SYA
- {0x1BB0, 0x1BB9, prNU, gcNd}, // [10] SUNDANESE DIGIT ZERO..SUNDANESE DIGIT NINE
- {0x1BBA, 0x1BBF, prAL, gcLo}, // [6] SUNDANESE AVAGRAHA..SUNDANESE LETTER FINAL M
- {0x1BC0, 0x1BE5, prAL, gcLo}, // [38] BATAK LETTER A..BATAK LETTER U
- {0x1BE6, 0x1BE6, prCM, gcMn}, // BATAK SIGN TOMPI
- {0x1BE7, 0x1BE7, prCM, gcMc}, // BATAK VOWEL SIGN E
- {0x1BE8, 0x1BE9, prCM, gcMn}, // [2] BATAK VOWEL SIGN PAKPAK E..BATAK VOWEL SIGN EE
- {0x1BEA, 0x1BEC, prCM, gcMc}, // [3] BATAK VOWEL SIGN I..BATAK VOWEL SIGN O
- {0x1BED, 0x1BED, prCM, gcMn}, // BATAK VOWEL SIGN KARO O
- {0x1BEE, 0x1BEE, prCM, gcMc}, // BATAK VOWEL SIGN U
- {0x1BEF, 0x1BF1, prCM, gcMn}, // [3] BATAK VOWEL SIGN U FOR SIMALUNGUN SA..BATAK CONSONANT SIGN H
- {0x1BF2, 0x1BF3, prCM, gcMc}, // [2] BATAK PANGOLAT..BATAK PANONGONAN
- {0x1BFC, 0x1BFF, prAL, gcPo}, // [4] BATAK SYMBOL BINDU NA METEK..BATAK SYMBOL BINDU PANGOLAT
- {0x1C00, 0x1C23, prAL, gcLo}, // [36] LEPCHA LETTER KA..LEPCHA LETTER A
- {0x1C24, 0x1C2B, prCM, gcMc}, // [8] LEPCHA SUBJOINED LETTER YA..LEPCHA VOWEL SIGN UU
- {0x1C2C, 0x1C33, prCM, gcMn}, // [8] LEPCHA VOWEL SIGN E..LEPCHA CONSONANT SIGN T
- {0x1C34, 0x1C35, prCM, gcMc}, // [2] LEPCHA CONSONANT SIGN NYIN-DO..LEPCHA CONSONANT SIGN KANG
- {0x1C36, 0x1C37, prCM, gcMn}, // [2] LEPCHA SIGN RAN..LEPCHA SIGN NUKTA
- {0x1C3B, 0x1C3F, prBA, gcPo}, // [5] LEPCHA PUNCTUATION TA-ROL..LEPCHA PUNCTUATION TSHOOK
- {0x1C40, 0x1C49, prNU, gcNd}, // [10] LEPCHA DIGIT ZERO..LEPCHA DIGIT NINE
- {0x1C4D, 0x1C4F, prAL, gcLo}, // [3] LEPCHA LETTER TTA..LEPCHA LETTER DDA
- {0x1C50, 0x1C59, prNU, gcNd}, // [10] OL CHIKI DIGIT ZERO..OL CHIKI DIGIT NINE
- {0x1C5A, 0x1C77, prAL, gcLo}, // [30] OL CHIKI LETTER LA..OL CHIKI LETTER OH
- {0x1C78, 0x1C7D, prAL, gcLm}, // [6] OL CHIKI MU TTUDDAG..OL CHIKI AHAD
- {0x1C7E, 0x1C7F, prBA, gcPo}, // [2] OL CHIKI PUNCTUATION MUCAAD..OL CHIKI PUNCTUATION DOUBLE MUCAAD
- {0x1C80, 0x1C88, prAL, gcLl}, // [9] CYRILLIC SMALL LETTER ROUNDED VE..CYRILLIC SMALL LETTER UNBLENDED UK
- {0x1C90, 0x1CBA, prAL, gcLu}, // [43] GEORGIAN MTAVRULI CAPITAL LETTER AN..GEORGIAN MTAVRULI CAPITAL LETTER AIN
- {0x1CBD, 0x1CBF, prAL, gcLu}, // [3] GEORGIAN MTAVRULI CAPITAL LETTER AEN..GEORGIAN MTAVRULI CAPITAL LETTER LABIAL SIGN
- {0x1CC0, 0x1CC7, prAL, gcPo}, // [8] SUNDANESE PUNCTUATION BINDU SURYA..SUNDANESE PUNCTUATION BINDU BA SATANGA
- {0x1CD0, 0x1CD2, prCM, gcMn}, // [3] VEDIC TONE KARSHANA..VEDIC TONE PRENKHA
- {0x1CD3, 0x1CD3, prAL, gcPo}, // VEDIC SIGN NIHSHVASA
- {0x1CD4, 0x1CE0, prCM, gcMn}, // [13] VEDIC SIGN YAJURVEDIC MIDLINE SVARITA..VEDIC TONE RIGVEDIC KASHMIRI INDEPENDENT SVARITA
- {0x1CE1, 0x1CE1, prCM, gcMc}, // VEDIC TONE ATHARVAVEDIC INDEPENDENT SVARITA
- {0x1CE2, 0x1CE8, prCM, gcMn}, // [7] VEDIC SIGN VISARGA SVARITA..VEDIC SIGN VISARGA ANUDATTA WITH TAIL
- {0x1CE9, 0x1CEC, prAL, gcLo}, // [4] VEDIC SIGN ANUSVARA ANTARGOMUKHA..VEDIC SIGN ANUSVARA VAMAGOMUKHA WITH TAIL
- {0x1CED, 0x1CED, prCM, gcMn}, // VEDIC SIGN TIRYAK
- {0x1CEE, 0x1CF3, prAL, gcLo}, // [6] VEDIC SIGN HEXIFORM LONG ANUSVARA..VEDIC SIGN ROTATED ARDHAVISARGA
- {0x1CF4, 0x1CF4, prCM, gcMn}, // VEDIC TONE CANDRA ABOVE
- {0x1CF5, 0x1CF6, prAL, gcLo}, // [2] VEDIC SIGN JIHVAMULIYA..VEDIC SIGN UPADHMANIYA
- {0x1CF7, 0x1CF7, prCM, gcMc}, // VEDIC SIGN ATIKRAMA
- {0x1CF8, 0x1CF9, prCM, gcMn}, // [2] VEDIC TONE RING ABOVE..VEDIC TONE DOUBLE RING ABOVE
- {0x1CFA, 0x1CFA, prAL, gcLo}, // VEDIC SIGN DOUBLE ANUSVARA ANTARGOMUKHA
- {0x1D00, 0x1D2B, prAL, gcLl}, // [44] LATIN LETTER SMALL CAPITAL A..CYRILLIC LETTER SMALL CAPITAL EL
- {0x1D2C, 0x1D6A, prAL, gcLm}, // [63] MODIFIER LETTER CAPITAL A..GREEK SUBSCRIPT SMALL LETTER CHI
- {0x1D6B, 0x1D77, prAL, gcLl}, // [13] LATIN SMALL LETTER UE..LATIN SMALL LETTER TURNED G
- {0x1D78, 0x1D78, prAL, gcLm}, // MODIFIER LETTER CYRILLIC EN
- {0x1D79, 0x1D7F, prAL, gcLl}, // [7] LATIN SMALL LETTER INSULAR G..LATIN SMALL LETTER UPSILON WITH STROKE
- {0x1D80, 0x1D9A, prAL, gcLl}, // [27] LATIN SMALL LETTER B WITH PALATAL HOOK..LATIN SMALL LETTER EZH WITH RETROFLEX HOOK
- {0x1D9B, 0x1DBF, prAL, gcLm}, // [37] MODIFIER LETTER SMALL TURNED ALPHA..MODIFIER LETTER SMALL THETA
- {0x1DC0, 0x1DFF, prCM, gcMn}, // [64] COMBINING DOTTED GRAVE ACCENT..COMBINING RIGHT ARROWHEAD AND DOWN ARROWHEAD BELOW
- {0x1E00, 0x1EFF, prAL, gcLC}, // [256] LATIN CAPITAL LETTER A WITH RING BELOW..LATIN SMALL LETTER Y WITH LOOP
- {0x1F00, 0x1F15, prAL, gcLC}, // [22] GREEK SMALL LETTER ALPHA WITH PSILI..GREEK SMALL LETTER EPSILON WITH DASIA AND OXIA
- {0x1F18, 0x1F1D, prAL, gcLu}, // [6] GREEK CAPITAL LETTER EPSILON WITH PSILI..GREEK CAPITAL LETTER EPSILON WITH DASIA AND OXIA
- {0x1F20, 0x1F45, prAL, gcLC}, // [38] GREEK SMALL LETTER ETA WITH PSILI..GREEK SMALL LETTER OMICRON WITH DASIA AND OXIA
- {0x1F48, 0x1F4D, prAL, gcLu}, // [6] GREEK CAPITAL LETTER OMICRON WITH PSILI..GREEK CAPITAL LETTER OMICRON WITH DASIA AND OXIA
- {0x1F50, 0x1F57, prAL, gcLl}, // [8] GREEK SMALL LETTER UPSILON WITH PSILI..GREEK SMALL LETTER UPSILON WITH DASIA AND PERISPOMENI
- {0x1F59, 0x1F59, prAL, gcLu}, // GREEK CAPITAL LETTER UPSILON WITH DASIA
- {0x1F5B, 0x1F5B, prAL, gcLu}, // GREEK CAPITAL LETTER UPSILON WITH DASIA AND VARIA
- {0x1F5D, 0x1F5D, prAL, gcLu}, // GREEK CAPITAL LETTER UPSILON WITH DASIA AND OXIA
- {0x1F5F, 0x1F7D, prAL, gcLC}, // [31] GREEK CAPITAL LETTER UPSILON WITH DASIA AND PERISPOMENI..GREEK SMALL LETTER OMEGA WITH OXIA
- {0x1F80, 0x1FB4, prAL, gcLC}, // [53] GREEK SMALL LETTER ALPHA WITH PSILI AND YPOGEGRAMMENI..GREEK SMALL LETTER ALPHA WITH OXIA AND YPOGEGRAMMENI
- {0x1FB6, 0x1FBC, prAL, gcLC}, // [7] GREEK SMALL LETTER ALPHA WITH PERISPOMENI..GREEK CAPITAL LETTER ALPHA WITH PROSGEGRAMMENI
- {0x1FBD, 0x1FBD, prAL, gcSk}, // GREEK KORONIS
- {0x1FBE, 0x1FBE, prAL, gcLl}, // GREEK PROSGEGRAMMENI
- {0x1FBF, 0x1FC1, prAL, gcSk}, // [3] GREEK PSILI..GREEK DIALYTIKA AND PERISPOMENI
- {0x1FC2, 0x1FC4, prAL, gcLl}, // [3] GREEK SMALL LETTER ETA WITH VARIA AND YPOGEGRAMMENI..GREEK SMALL LETTER ETA WITH OXIA AND YPOGEGRAMMENI
- {0x1FC6, 0x1FCC, prAL, gcLC}, // [7] GREEK SMALL LETTER ETA WITH PERISPOMENI..GREEK CAPITAL LETTER ETA WITH PROSGEGRAMMENI
- {0x1FCD, 0x1FCF, prAL, gcSk}, // [3] GREEK PSILI AND VARIA..GREEK PSILI AND PERISPOMENI
- {0x1FD0, 0x1FD3, prAL, gcLl}, // [4] GREEK SMALL LETTER IOTA WITH VRACHY..GREEK SMALL LETTER IOTA WITH DIALYTIKA AND OXIA
- {0x1FD6, 0x1FDB, prAL, gcLC}, // [6] GREEK SMALL LETTER IOTA WITH PERISPOMENI..GREEK CAPITAL LETTER IOTA WITH OXIA
- {0x1FDD, 0x1FDF, prAL, gcSk}, // [3] GREEK DASIA AND VARIA..GREEK DASIA AND PERISPOMENI
- {0x1FE0, 0x1FEC, prAL, gcLC}, // [13] GREEK SMALL LETTER UPSILON WITH VRACHY..GREEK CAPITAL LETTER RHO WITH DASIA
- {0x1FED, 0x1FEF, prAL, gcSk}, // [3] GREEK DIALYTIKA AND VARIA..GREEK VARIA
- {0x1FF2, 0x1FF4, prAL, gcLl}, // [3] GREEK SMALL LETTER OMEGA WITH VARIA AND YPOGEGRAMMENI..GREEK SMALL LETTER OMEGA WITH OXIA AND YPOGEGRAMMENI
- {0x1FF6, 0x1FFC, prAL, gcLC}, // [7] GREEK SMALL LETTER OMEGA WITH PERISPOMENI..GREEK CAPITAL LETTER OMEGA WITH PROSGEGRAMMENI
- {0x1FFD, 0x1FFD, prBB, gcSk}, // GREEK OXIA
- {0x1FFE, 0x1FFE, prAL, gcSk}, // GREEK DASIA
- {0x2000, 0x2006, prBA, gcZs}, // [7] EN QUAD..SIX-PER-EM SPACE
- {0x2007, 0x2007, prGL, gcZs}, // FIGURE SPACE
- {0x2008, 0x200A, prBA, gcZs}, // [3] PUNCTUATION SPACE..HAIR SPACE
- {0x200B, 0x200B, prZW, gcCf}, // ZERO WIDTH SPACE
- {0x200C, 0x200C, prCM, gcCf}, // ZERO WIDTH NON-JOINER
- {0x200D, 0x200D, prZWJ, gcCf}, // ZERO WIDTH JOINER
- {0x200E, 0x200F, prCM, gcCf}, // [2] LEFT-TO-RIGHT MARK..RIGHT-TO-LEFT MARK
- {0x2010, 0x2010, prBA, gcPd}, // HYPHEN
- {0x2011, 0x2011, prGL, gcPd}, // NON-BREAKING HYPHEN
- {0x2012, 0x2013, prBA, gcPd}, // [2] FIGURE DASH..EN DASH
- {0x2014, 0x2014, prB2, gcPd}, // EM DASH
- {0x2015, 0x2015, prAI, gcPd}, // HORIZONTAL BAR
- {0x2016, 0x2016, prAI, gcPo}, // DOUBLE VERTICAL LINE
- {0x2017, 0x2017, prAL, gcPo}, // DOUBLE LOW LINE
- {0x2018, 0x2018, prQU, gcPi}, // LEFT SINGLE QUOTATION MARK
- {0x2019, 0x2019, prQU, gcPf}, // RIGHT SINGLE QUOTATION MARK
- {0x201A, 0x201A, prOP, gcPs}, // SINGLE LOW-9 QUOTATION MARK
- {0x201B, 0x201C, prQU, gcPi}, // [2] SINGLE HIGH-REVERSED-9 QUOTATION MARK..LEFT DOUBLE QUOTATION MARK
- {0x201D, 0x201D, prQU, gcPf}, // RIGHT DOUBLE QUOTATION MARK
- {0x201E, 0x201E, prOP, gcPs}, // DOUBLE LOW-9 QUOTATION MARK
- {0x201F, 0x201F, prQU, gcPi}, // DOUBLE HIGH-REVERSED-9 QUOTATION MARK
- {0x2020, 0x2021, prAI, gcPo}, // [2] DAGGER..DOUBLE DAGGER
- {0x2022, 0x2023, prAL, gcPo}, // [2] BULLET..TRIANGULAR BULLET
- {0x2024, 0x2026, prIN, gcPo}, // [3] ONE DOT LEADER..HORIZONTAL ELLIPSIS
- {0x2027, 0x2027, prBA, gcPo}, // HYPHENATION POINT
- {0x2028, 0x2028, prBK, gcZl}, // LINE SEPARATOR
- {0x2029, 0x2029, prBK, gcZp}, // PARAGRAPH SEPARATOR
- {0x202A, 0x202E, prCM, gcCf}, // [5] LEFT-TO-RIGHT EMBEDDING..RIGHT-TO-LEFT OVERRIDE
- {0x202F, 0x202F, prGL, gcZs}, // NARROW NO-BREAK SPACE
- {0x2030, 0x2037, prPO, gcPo}, // [8] PER MILLE SIGN..REVERSED TRIPLE PRIME
- {0x2038, 0x2038, prAL, gcPo}, // CARET
- {0x2039, 0x2039, prQU, gcPi}, // SINGLE LEFT-POINTING ANGLE QUOTATION MARK
- {0x203A, 0x203A, prQU, gcPf}, // SINGLE RIGHT-POINTING ANGLE QUOTATION MARK
- {0x203B, 0x203B, prAI, gcPo}, // REFERENCE MARK
- {0x203C, 0x203D, prNS, gcPo}, // [2] DOUBLE EXCLAMATION MARK..INTERROBANG
- {0x203E, 0x203E, prAL, gcPo}, // OVERLINE
- {0x203F, 0x2040, prAL, gcPc}, // [2] UNDERTIE..CHARACTER TIE
- {0x2041, 0x2043, prAL, gcPo}, // [3] CARET INSERTION POINT..HYPHEN BULLET
- {0x2044, 0x2044, prIS, gcSm}, // FRACTION SLASH
- {0x2045, 0x2045, prOP, gcPs}, // LEFT SQUARE BRACKET WITH QUILL
- {0x2046, 0x2046, prCL, gcPe}, // RIGHT SQUARE BRACKET WITH QUILL
- {0x2047, 0x2049, prNS, gcPo}, // [3] DOUBLE QUESTION MARK..EXCLAMATION QUESTION MARK
- {0x204A, 0x2051, prAL, gcPo}, // [8] TIRONIAN SIGN ET..TWO ASTERISKS ALIGNED VERTICALLY
- {0x2052, 0x2052, prAL, gcSm}, // COMMERCIAL MINUS SIGN
- {0x2053, 0x2053, prAL, gcPo}, // SWUNG DASH
- {0x2054, 0x2054, prAL, gcPc}, // INVERTED UNDERTIE
- {0x2055, 0x2055, prAL, gcPo}, // FLOWER PUNCTUATION MARK
- {0x2056, 0x2056, prBA, gcPo}, // THREE DOT PUNCTUATION
- {0x2057, 0x2057, prAL, gcPo}, // QUADRUPLE PRIME
- {0x2058, 0x205B, prBA, gcPo}, // [4] FOUR DOT PUNCTUATION..FOUR DOT MARK
- {0x205C, 0x205C, prAL, gcPo}, // DOTTED CROSS
- {0x205D, 0x205E, prBA, gcPo}, // [2] TRICOLON..VERTICAL FOUR DOTS
- {0x205F, 0x205F, prBA, gcZs}, // MEDIUM MATHEMATICAL SPACE
- {0x2060, 0x2060, prWJ, gcCf}, // WORD JOINER
- {0x2061, 0x2064, prAL, gcCf}, // [4] FUNCTION APPLICATION..INVISIBLE PLUS
- {0x2066, 0x206F, prCM, gcCf}, // [10] LEFT-TO-RIGHT ISOLATE..NOMINAL DIGIT SHAPES
- {0x2070, 0x2070, prAL, gcNo}, // SUPERSCRIPT ZERO
- {0x2071, 0x2071, prAL, gcLm}, // SUPERSCRIPT LATIN SMALL LETTER I
- {0x2074, 0x2074, prAI, gcNo}, // SUPERSCRIPT FOUR
- {0x2075, 0x2079, prAL, gcNo}, // [5] SUPERSCRIPT FIVE..SUPERSCRIPT NINE
- {0x207A, 0x207C, prAL, gcSm}, // [3] SUPERSCRIPT PLUS SIGN..SUPERSCRIPT EQUALS SIGN
- {0x207D, 0x207D, prOP, gcPs}, // SUPERSCRIPT LEFT PARENTHESIS
- {0x207E, 0x207E, prCL, gcPe}, // SUPERSCRIPT RIGHT PARENTHESIS
- {0x207F, 0x207F, prAI, gcLm}, // SUPERSCRIPT LATIN SMALL LETTER N
- {0x2080, 0x2080, prAL, gcNo}, // SUBSCRIPT ZERO
- {0x2081, 0x2084, prAI, gcNo}, // [4] SUBSCRIPT ONE..SUBSCRIPT FOUR
- {0x2085, 0x2089, prAL, gcNo}, // [5] SUBSCRIPT FIVE..SUBSCRIPT NINE
- {0x208A, 0x208C, prAL, gcSm}, // [3] SUBSCRIPT PLUS SIGN..SUBSCRIPT EQUALS SIGN
- {0x208D, 0x208D, prOP, gcPs}, // SUBSCRIPT LEFT PARENTHESIS
- {0x208E, 0x208E, prCL, gcPe}, // SUBSCRIPT RIGHT PARENTHESIS
- {0x2090, 0x209C, prAL, gcLm}, // [13] LATIN SUBSCRIPT SMALL LETTER A..LATIN SUBSCRIPT SMALL LETTER T
- {0x20A0, 0x20A6, prPR, gcSc}, // [7] EURO-CURRENCY SIGN..NAIRA SIGN
- {0x20A7, 0x20A7, prPO, gcSc}, // PESETA SIGN
- {0x20A8, 0x20B5, prPR, gcSc}, // [14] RUPEE SIGN..CEDI SIGN
- {0x20B6, 0x20B6, prPO, gcSc}, // LIVRE TOURNOIS SIGN
- {0x20B7, 0x20BA, prPR, gcSc}, // [4] SPESMILO SIGN..TURKISH LIRA SIGN
- {0x20BB, 0x20BB, prPO, gcSc}, // NORDIC MARK SIGN
- {0x20BC, 0x20BD, prPR, gcSc}, // [2] MANAT SIGN..RUBLE SIGN
- {0x20BE, 0x20BE, prPO, gcSc}, // LARI SIGN
- {0x20BF, 0x20BF, prPR, gcSc}, // BITCOIN SIGN
- {0x20C0, 0x20C0, prPO, gcSc}, // SOM SIGN
- {0x20C1, 0x20CF, prPR, gcCn}, // [15] ..
- {0x20D0, 0x20DC, prCM, gcMn}, // [13] COMBINING LEFT HARPOON ABOVE..COMBINING FOUR DOTS ABOVE
- {0x20DD, 0x20E0, prCM, gcMe}, // [4] COMBINING ENCLOSING CIRCLE..COMBINING ENCLOSING CIRCLE BACKSLASH
- {0x20E1, 0x20E1, prCM, gcMn}, // COMBINING LEFT RIGHT ARROW ABOVE
- {0x20E2, 0x20E4, prCM, gcMe}, // [3] COMBINING ENCLOSING SCREEN..COMBINING ENCLOSING UPWARD POINTING TRIANGLE
- {0x20E5, 0x20F0, prCM, gcMn}, // [12] COMBINING REVERSE SOLIDUS OVERLAY..COMBINING ASTERISK ABOVE
- {0x2100, 0x2101, prAL, gcSo}, // [2] ACCOUNT OF..ADDRESSED TO THE SUBJECT
- {0x2102, 0x2102, prAL, gcLu}, // DOUBLE-STRUCK CAPITAL C
- {0x2103, 0x2103, prPO, gcSo}, // DEGREE CELSIUS
- {0x2104, 0x2104, prAL, gcSo}, // CENTRE LINE SYMBOL
- {0x2105, 0x2105, prAI, gcSo}, // CARE OF
- {0x2106, 0x2106, prAL, gcSo}, // CADA UNA
- {0x2107, 0x2107, prAL, gcLu}, // EULER CONSTANT
- {0x2108, 0x2108, prAL, gcSo}, // SCRUPLE
- {0x2109, 0x2109, prPO, gcSo}, // DEGREE FAHRENHEIT
- {0x210A, 0x2112, prAL, gcLC}, // [9] SCRIPT SMALL G..SCRIPT CAPITAL L
- {0x2113, 0x2113, prAI, gcLl}, // SCRIPT SMALL L
- {0x2114, 0x2114, prAL, gcSo}, // L B BAR SYMBOL
- {0x2115, 0x2115, prAL, gcLu}, // DOUBLE-STRUCK CAPITAL N
- {0x2116, 0x2116, prPR, gcSo}, // NUMERO SIGN
- {0x2117, 0x2117, prAL, gcSo}, // SOUND RECORDING COPYRIGHT
- {0x2118, 0x2118, prAL, gcSm}, // SCRIPT CAPITAL P
- {0x2119, 0x211D, prAL, gcLu}, // [5] DOUBLE-STRUCK CAPITAL P..DOUBLE-STRUCK CAPITAL R
- {0x211E, 0x2120, prAL, gcSo}, // [3] PRESCRIPTION TAKE..SERVICE MARK
- {0x2121, 0x2122, prAI, gcSo}, // [2] TELEPHONE SIGN..TRADE MARK SIGN
- {0x2123, 0x2123, prAL, gcSo}, // VERSICLE
- {0x2124, 0x2124, prAL, gcLu}, // DOUBLE-STRUCK CAPITAL Z
- {0x2125, 0x2125, prAL, gcSo}, // OUNCE SIGN
- {0x2126, 0x2126, prAL, gcLu}, // OHM SIGN
- {0x2127, 0x2127, prAL, gcSo}, // INVERTED OHM SIGN
- {0x2128, 0x2128, prAL, gcLu}, // BLACK-LETTER CAPITAL Z
- {0x2129, 0x2129, prAL, gcSo}, // TURNED GREEK SMALL LETTER IOTA
- {0x212A, 0x212A, prAL, gcLu}, // KELVIN SIGN
- {0x212B, 0x212B, prAI, gcLu}, // ANGSTROM SIGN
- {0x212C, 0x212D, prAL, gcLu}, // [2] SCRIPT CAPITAL B..BLACK-LETTER CAPITAL C
- {0x212E, 0x212E, prAL, gcSo}, // ESTIMATED SYMBOL
- {0x212F, 0x2134, prAL, gcLC}, // [6] SCRIPT SMALL E..SCRIPT SMALL O
- {0x2135, 0x2138, prAL, gcLo}, // [4] ALEF SYMBOL..DALET SYMBOL
- {0x2139, 0x2139, prAL, gcLl}, // INFORMATION SOURCE
- {0x213A, 0x213B, prAL, gcSo}, // [2] ROTATED CAPITAL Q..FACSIMILE SIGN
- {0x213C, 0x213F, prAL, gcLC}, // [4] DOUBLE-STRUCK SMALL PI..DOUBLE-STRUCK CAPITAL PI
- {0x2140, 0x2144, prAL, gcSm}, // [5] DOUBLE-STRUCK N-ARY SUMMATION..TURNED SANS-SERIF CAPITAL Y
- {0x2145, 0x2149, prAL, gcLC}, // [5] DOUBLE-STRUCK ITALIC CAPITAL D..DOUBLE-STRUCK ITALIC SMALL J
- {0x214A, 0x214A, prAL, gcSo}, // PROPERTY LINE
- {0x214B, 0x214B, prAL, gcSm}, // TURNED AMPERSAND
- {0x214C, 0x214D, prAL, gcSo}, // [2] PER SIGN..AKTIESELSKAB
- {0x214E, 0x214E, prAL, gcLl}, // TURNED SMALL F
- {0x214F, 0x214F, prAL, gcSo}, // SYMBOL FOR SAMARITAN SOURCE
- {0x2150, 0x2153, prAL, gcNo}, // [4] VULGAR FRACTION ONE SEVENTH..VULGAR FRACTION ONE THIRD
- {0x2154, 0x2155, prAI, gcNo}, // [2] VULGAR FRACTION TWO THIRDS..VULGAR FRACTION ONE FIFTH
- {0x2156, 0x215A, prAL, gcNo}, // [5] VULGAR FRACTION TWO FIFTHS..VULGAR FRACTION FIVE SIXTHS
- {0x215B, 0x215B, prAI, gcNo}, // VULGAR FRACTION ONE EIGHTH
- {0x215C, 0x215D, prAL, gcNo}, // [2] VULGAR FRACTION THREE EIGHTHS..VULGAR FRACTION FIVE EIGHTHS
- {0x215E, 0x215E, prAI, gcNo}, // VULGAR FRACTION SEVEN EIGHTHS
- {0x215F, 0x215F, prAL, gcNo}, // FRACTION NUMERATOR ONE
- {0x2160, 0x216B, prAI, gcNl}, // [12] ROMAN NUMERAL ONE..ROMAN NUMERAL TWELVE
- {0x216C, 0x216F, prAL, gcNl}, // [4] ROMAN NUMERAL FIFTY..ROMAN NUMERAL ONE THOUSAND
- {0x2170, 0x2179, prAI, gcNl}, // [10] SMALL ROMAN NUMERAL ONE..SMALL ROMAN NUMERAL TEN
- {0x217A, 0x2182, prAL, gcNl}, // [9] SMALL ROMAN NUMERAL ELEVEN..ROMAN NUMERAL TEN THOUSAND
- {0x2183, 0x2184, prAL, gcLC}, // [2] ROMAN NUMERAL REVERSED ONE HUNDRED..LATIN SMALL LETTER REVERSED C
- {0x2185, 0x2188, prAL, gcNl}, // [4] ROMAN NUMERAL SIX LATE FORM..ROMAN NUMERAL ONE HUNDRED THOUSAND
- {0x2189, 0x2189, prAI, gcNo}, // VULGAR FRACTION ZERO THIRDS
- {0x218A, 0x218B, prAL, gcSo}, // [2] TURNED DIGIT TWO..TURNED DIGIT THREE
- {0x2190, 0x2194, prAI, gcSm}, // [5] LEFTWARDS ARROW..LEFT RIGHT ARROW
- {0x2195, 0x2199, prAI, gcSo}, // [5] UP DOWN ARROW..SOUTH WEST ARROW
- {0x219A, 0x219B, prAL, gcSm}, // [2] LEFTWARDS ARROW WITH STROKE..RIGHTWARDS ARROW WITH STROKE
- {0x219C, 0x219F, prAL, gcSo}, // [4] LEFTWARDS WAVE ARROW..UPWARDS TWO HEADED ARROW
- {0x21A0, 0x21A0, prAL, gcSm}, // RIGHTWARDS TWO HEADED ARROW
- {0x21A1, 0x21A2, prAL, gcSo}, // [2] DOWNWARDS TWO HEADED ARROW..LEFTWARDS ARROW WITH TAIL
- {0x21A3, 0x21A3, prAL, gcSm}, // RIGHTWARDS ARROW WITH TAIL
- {0x21A4, 0x21A5, prAL, gcSo}, // [2] LEFTWARDS ARROW FROM BAR..UPWARDS ARROW FROM BAR
- {0x21A6, 0x21A6, prAL, gcSm}, // RIGHTWARDS ARROW FROM BAR
- {0x21A7, 0x21AD, prAL, gcSo}, // [7] DOWNWARDS ARROW FROM BAR..LEFT RIGHT WAVE ARROW
- {0x21AE, 0x21AE, prAL, gcSm}, // LEFT RIGHT ARROW WITH STROKE
- {0x21AF, 0x21CD, prAL, gcSo}, // [31] DOWNWARDS ZIGZAG ARROW..LEFTWARDS DOUBLE ARROW WITH STROKE
- {0x21CE, 0x21CF, prAL, gcSm}, // [2] LEFT RIGHT DOUBLE ARROW WITH STROKE..RIGHTWARDS DOUBLE ARROW WITH STROKE
- {0x21D0, 0x21D1, prAL, gcSo}, // [2] LEFTWARDS DOUBLE ARROW..UPWARDS DOUBLE ARROW
- {0x21D2, 0x21D2, prAI, gcSm}, // RIGHTWARDS DOUBLE ARROW
- {0x21D3, 0x21D3, prAL, gcSo}, // DOWNWARDS DOUBLE ARROW
- {0x21D4, 0x21D4, prAI, gcSm}, // LEFT RIGHT DOUBLE ARROW
- {0x21D5, 0x21F3, prAL, gcSo}, // [31] UP DOWN DOUBLE ARROW..UP DOWN WHITE ARROW
- {0x21F4, 0x21FF, prAL, gcSm}, // [12] RIGHT ARROW WITH SMALL CIRCLE..LEFT RIGHT OPEN-HEADED ARROW
- {0x2200, 0x2200, prAI, gcSm}, // FOR ALL
- {0x2201, 0x2201, prAL, gcSm}, // COMPLEMENT
- {0x2202, 0x2203, prAI, gcSm}, // [2] PARTIAL DIFFERENTIAL..THERE EXISTS
- {0x2204, 0x2206, prAL, gcSm}, // [3] THERE DOES NOT EXIST..INCREMENT
- {0x2207, 0x2208, prAI, gcSm}, // [2] NABLA..ELEMENT OF
- {0x2209, 0x220A, prAL, gcSm}, // [2] NOT AN ELEMENT OF..SMALL ELEMENT OF
- {0x220B, 0x220B, prAI, gcSm}, // CONTAINS AS MEMBER
- {0x220C, 0x220E, prAL, gcSm}, // [3] DOES NOT CONTAIN AS MEMBER..END OF PROOF
- {0x220F, 0x220F, prAI, gcSm}, // N-ARY PRODUCT
- {0x2210, 0x2210, prAL, gcSm}, // N-ARY COPRODUCT
- {0x2211, 0x2211, prAI, gcSm}, // N-ARY SUMMATION
- {0x2212, 0x2213, prPR, gcSm}, // [2] MINUS SIGN..MINUS-OR-PLUS SIGN
- {0x2214, 0x2214, prAL, gcSm}, // DOT PLUS
- {0x2215, 0x2215, prAI, gcSm}, // DIVISION SLASH
- {0x2216, 0x2219, prAL, gcSm}, // [4] SET MINUS..BULLET OPERATOR
- {0x221A, 0x221A, prAI, gcSm}, // SQUARE ROOT
- {0x221B, 0x221C, prAL, gcSm}, // [2] CUBE ROOT..FOURTH ROOT
- {0x221D, 0x2220, prAI, gcSm}, // [4] PROPORTIONAL TO..ANGLE
- {0x2221, 0x2222, prAL, gcSm}, // [2] MEASURED ANGLE..SPHERICAL ANGLE
- {0x2223, 0x2223, prAI, gcSm}, // DIVIDES
- {0x2224, 0x2224, prAL, gcSm}, // DOES NOT DIVIDE
- {0x2225, 0x2225, prAI, gcSm}, // PARALLEL TO
- {0x2226, 0x2226, prAL, gcSm}, // NOT PARALLEL TO
- {0x2227, 0x222C, prAI, gcSm}, // [6] LOGICAL AND..DOUBLE INTEGRAL
- {0x222D, 0x222D, prAL, gcSm}, // TRIPLE INTEGRAL
- {0x222E, 0x222E, prAI, gcSm}, // CONTOUR INTEGRAL
- {0x222F, 0x2233, prAL, gcSm}, // [5] SURFACE INTEGRAL..ANTICLOCKWISE CONTOUR INTEGRAL
- {0x2234, 0x2237, prAI, gcSm}, // [4] THEREFORE..PROPORTION
- {0x2238, 0x223B, prAL, gcSm}, // [4] DOT MINUS..HOMOTHETIC
- {0x223C, 0x223D, prAI, gcSm}, // [2] TILDE OPERATOR..REVERSED TILDE
- {0x223E, 0x2247, prAL, gcSm}, // [10] INVERTED LAZY S..NEITHER APPROXIMATELY NOR ACTUALLY EQUAL TO
- {0x2248, 0x2248, prAI, gcSm}, // ALMOST EQUAL TO
- {0x2249, 0x224B, prAL, gcSm}, // [3] NOT ALMOST EQUAL TO..TRIPLE TILDE
- {0x224C, 0x224C, prAI, gcSm}, // ALL EQUAL TO
- {0x224D, 0x2251, prAL, gcSm}, // [5] EQUIVALENT TO..GEOMETRICALLY EQUAL TO
- {0x2252, 0x2252, prAI, gcSm}, // APPROXIMATELY EQUAL TO OR THE IMAGE OF
- {0x2253, 0x225F, prAL, gcSm}, // [13] IMAGE OF OR APPROXIMATELY EQUAL TO..QUESTIONED EQUAL TO
- {0x2260, 0x2261, prAI, gcSm}, // [2] NOT EQUAL TO..IDENTICAL TO
- {0x2262, 0x2263, prAL, gcSm}, // [2] NOT IDENTICAL TO..STRICTLY EQUIVALENT TO
- {0x2264, 0x2267, prAI, gcSm}, // [4] LESS-THAN OR EQUAL TO..GREATER-THAN OVER EQUAL TO
- {0x2268, 0x2269, prAL, gcSm}, // [2] LESS-THAN BUT NOT EQUAL TO..GREATER-THAN BUT NOT EQUAL TO
- {0x226A, 0x226B, prAI, gcSm}, // [2] MUCH LESS-THAN..MUCH GREATER-THAN
- {0x226C, 0x226D, prAL, gcSm}, // [2] BETWEEN..NOT EQUIVALENT TO
- {0x226E, 0x226F, prAI, gcSm}, // [2] NOT LESS-THAN..NOT GREATER-THAN
- {0x2270, 0x2281, prAL, gcSm}, // [18] NEITHER LESS-THAN NOR EQUAL TO..DOES NOT SUCCEED
- {0x2282, 0x2283, prAI, gcSm}, // [2] SUBSET OF..SUPERSET OF
- {0x2284, 0x2285, prAL, gcSm}, // [2] NOT A SUBSET OF..NOT A SUPERSET OF
- {0x2286, 0x2287, prAI, gcSm}, // [2] SUBSET OF OR EQUAL TO..SUPERSET OF OR EQUAL TO
- {0x2288, 0x2294, prAL, gcSm}, // [13] NEITHER A SUBSET OF NOR EQUAL TO..SQUARE CUP
- {0x2295, 0x2295, prAI, gcSm}, // CIRCLED PLUS
- {0x2296, 0x2298, prAL, gcSm}, // [3] CIRCLED MINUS..CIRCLED DIVISION SLASH
- {0x2299, 0x2299, prAI, gcSm}, // CIRCLED DOT OPERATOR
- {0x229A, 0x22A4, prAL, gcSm}, // [11] CIRCLED RING OPERATOR..DOWN TACK
- {0x22A5, 0x22A5, prAI, gcSm}, // UP TACK
- {0x22A6, 0x22BE, prAL, gcSm}, // [25] ASSERTION..RIGHT ANGLE WITH ARC
- {0x22BF, 0x22BF, prAI, gcSm}, // RIGHT TRIANGLE
- {0x22C0, 0x22EE, prAL, gcSm}, // [47] N-ARY LOGICAL AND..VERTICAL ELLIPSIS
- {0x22EF, 0x22EF, prIN, gcSm}, // MIDLINE HORIZONTAL ELLIPSIS
- {0x22F0, 0x22FF, prAL, gcSm}, // [16] UP RIGHT DIAGONAL ELLIPSIS..Z NOTATION BAG MEMBERSHIP
- {0x2300, 0x2307, prAL, gcSo}, // [8] DIAMETER SIGN..WAVY LINE
- {0x2308, 0x2308, prOP, gcPs}, // LEFT CEILING
- {0x2309, 0x2309, prCL, gcPe}, // RIGHT CEILING
- {0x230A, 0x230A, prOP, gcPs}, // LEFT FLOOR
- {0x230B, 0x230B, prCL, gcPe}, // RIGHT FLOOR
- {0x230C, 0x2311, prAL, gcSo}, // [6] BOTTOM RIGHT CROP..SQUARE LOZENGE
- {0x2312, 0x2312, prAI, gcSo}, // ARC
- {0x2313, 0x2319, prAL, gcSo}, // [7] SEGMENT..TURNED NOT SIGN
- {0x231A, 0x231B, prID, gcSo}, // [2] WATCH..HOURGLASS
- {0x231C, 0x231F, prAL, gcSo}, // [4] TOP LEFT CORNER..BOTTOM RIGHT CORNER
- {0x2320, 0x2321, prAL, gcSm}, // [2] TOP HALF INTEGRAL..BOTTOM HALF INTEGRAL
- {0x2322, 0x2328, prAL, gcSo}, // [7] FROWN..KEYBOARD
- {0x2329, 0x2329, prOP, gcPs}, // LEFT-POINTING ANGLE BRACKET
- {0x232A, 0x232A, prCL, gcPe}, // RIGHT-POINTING ANGLE BRACKET
- {0x232B, 0x237B, prAL, gcSo}, // [81] ERASE TO THE LEFT..NOT CHECK MARK
- {0x237C, 0x237C, prAL, gcSm}, // RIGHT ANGLE WITH DOWNWARDS ZIGZAG ARROW
- {0x237D, 0x239A, prAL, gcSo}, // [30] SHOULDERED OPEN BOX..CLEAR SCREEN SYMBOL
- {0x239B, 0x23B3, prAL, gcSm}, // [25] LEFT PARENTHESIS UPPER HOOK..SUMMATION BOTTOM
- {0x23B4, 0x23DB, prAL, gcSo}, // [40] TOP SQUARE BRACKET..FUSE
- {0x23DC, 0x23E1, prAL, gcSm}, // [6] TOP PARENTHESIS..BOTTOM TORTOISE SHELL BRACKET
- {0x23E2, 0x23EF, prAL, gcSo}, // [14] WHITE TRAPEZIUM..BLACK RIGHT-POINTING TRIANGLE WITH DOUBLE VERTICAL BAR
- {0x23F0, 0x23F3, prID, gcSo}, // [4] ALARM CLOCK..HOURGLASS WITH FLOWING SAND
- {0x23F4, 0x23FF, prAL, gcSo}, // [12] BLACK MEDIUM LEFT-POINTING TRIANGLE..OBSERVER EYE SYMBOL
- {0x2400, 0x2426, prAL, gcSo}, // [39] SYMBOL FOR NULL..SYMBOL FOR SUBSTITUTE FORM TWO
- {0x2440, 0x244A, prAL, gcSo}, // [11] OCR HOOK..OCR DOUBLE BACKSLASH
- {0x2460, 0x249B, prAI, gcNo}, // [60] CIRCLED DIGIT ONE..NUMBER TWENTY FULL STOP
- {0x249C, 0x24E9, prAI, gcSo}, // [78] PARENTHESIZED LATIN SMALL LETTER A..CIRCLED LATIN SMALL LETTER Z
- {0x24EA, 0x24FE, prAI, gcNo}, // [21] CIRCLED DIGIT ZERO..DOUBLE CIRCLED NUMBER TEN
- {0x24FF, 0x24FF, prAL, gcNo}, // NEGATIVE CIRCLED DIGIT ZERO
- {0x2500, 0x254B, prAI, gcSo}, // [76] BOX DRAWINGS LIGHT HORIZONTAL..BOX DRAWINGS HEAVY VERTICAL AND HORIZONTAL
- {0x254C, 0x254F, prAL, gcSo}, // [4] BOX DRAWINGS LIGHT DOUBLE DASH HORIZONTAL..BOX DRAWINGS HEAVY DOUBLE DASH VERTICAL
- {0x2550, 0x2574, prAI, gcSo}, // [37] BOX DRAWINGS DOUBLE HORIZONTAL..BOX DRAWINGS LIGHT LEFT
- {0x2575, 0x257F, prAL, gcSo}, // [11] BOX DRAWINGS LIGHT UP..BOX DRAWINGS HEAVY UP AND LIGHT DOWN
- {0x2580, 0x258F, prAI, gcSo}, // [16] UPPER HALF BLOCK..LEFT ONE EIGHTH BLOCK
- {0x2590, 0x2591, prAL, gcSo}, // [2] RIGHT HALF BLOCK..LIGHT SHADE
- {0x2592, 0x2595, prAI, gcSo}, // [4] MEDIUM SHADE..RIGHT ONE EIGHTH BLOCK
- {0x2596, 0x259F, prAL, gcSo}, // [10] QUADRANT LOWER LEFT..QUADRANT UPPER RIGHT AND LOWER LEFT AND LOWER RIGHT
- {0x25A0, 0x25A1, prAI, gcSo}, // [2] BLACK SQUARE..WHITE SQUARE
- {0x25A2, 0x25A2, prAL, gcSo}, // WHITE SQUARE WITH ROUNDED CORNERS
- {0x25A3, 0x25A9, prAI, gcSo}, // [7] WHITE SQUARE CONTAINING BLACK SMALL SQUARE..SQUARE WITH DIAGONAL CROSSHATCH FILL
- {0x25AA, 0x25B1, prAL, gcSo}, // [8] BLACK SMALL SQUARE..WHITE PARALLELOGRAM
- {0x25B2, 0x25B3, prAI, gcSo}, // [2] BLACK UP-POINTING TRIANGLE..WHITE UP-POINTING TRIANGLE
- {0x25B4, 0x25B5, prAL, gcSo}, // [2] BLACK UP-POINTING SMALL TRIANGLE..WHITE UP-POINTING SMALL TRIANGLE
- {0x25B6, 0x25B6, prAI, gcSo}, // BLACK RIGHT-POINTING TRIANGLE
- {0x25B7, 0x25B7, prAI, gcSm}, // WHITE RIGHT-POINTING TRIANGLE
- {0x25B8, 0x25BB, prAL, gcSo}, // [4] BLACK RIGHT-POINTING SMALL TRIANGLE..WHITE RIGHT-POINTING POINTER
- {0x25BC, 0x25BD, prAI, gcSo}, // [2] BLACK DOWN-POINTING TRIANGLE..WHITE DOWN-POINTING TRIANGLE
- {0x25BE, 0x25BF, prAL, gcSo}, // [2] BLACK DOWN-POINTING SMALL TRIANGLE..WHITE DOWN-POINTING SMALL TRIANGLE
- {0x25C0, 0x25C0, prAI, gcSo}, // BLACK LEFT-POINTING TRIANGLE
- {0x25C1, 0x25C1, prAI, gcSm}, // WHITE LEFT-POINTING TRIANGLE
- {0x25C2, 0x25C5, prAL, gcSo}, // [4] BLACK LEFT-POINTING SMALL TRIANGLE..WHITE LEFT-POINTING POINTER
- {0x25C6, 0x25C8, prAI, gcSo}, // [3] BLACK DIAMOND..WHITE DIAMOND CONTAINING BLACK SMALL DIAMOND
- {0x25C9, 0x25CA, prAL, gcSo}, // [2] FISHEYE..LOZENGE
- {0x25CB, 0x25CB, prAI, gcSo}, // WHITE CIRCLE
- {0x25CC, 0x25CD, prAL, gcSo}, // [2] DOTTED CIRCLE..CIRCLE WITH VERTICAL FILL
- {0x25CE, 0x25D1, prAI, gcSo}, // [4] BULLSEYE..CIRCLE WITH RIGHT HALF BLACK
- {0x25D2, 0x25E1, prAL, gcSo}, // [16] CIRCLE WITH LOWER HALF BLACK..LOWER HALF CIRCLE
- {0x25E2, 0x25E5, prAI, gcSo}, // [4] BLACK LOWER RIGHT TRIANGLE..BLACK UPPER RIGHT TRIANGLE
- {0x25E6, 0x25EE, prAL, gcSo}, // [9] WHITE BULLET..UP-POINTING TRIANGLE WITH RIGHT HALF BLACK
- {0x25EF, 0x25EF, prAI, gcSo}, // LARGE CIRCLE
- {0x25F0, 0x25F7, prAL, gcSo}, // [8] WHITE SQUARE WITH UPPER LEFT QUADRANT..WHITE CIRCLE WITH UPPER RIGHT QUADRANT
- {0x25F8, 0x25FF, prAL, gcSm}, // [8] UPPER LEFT TRIANGLE..LOWER RIGHT TRIANGLE
- {0x2600, 0x2603, prID, gcSo}, // [4] BLACK SUN WITH RAYS..SNOWMAN
- {0x2604, 0x2604, prAL, gcSo}, // COMET
- {0x2605, 0x2606, prAI, gcSo}, // [2] BLACK STAR..WHITE STAR
- {0x2607, 0x2608, prAL, gcSo}, // [2] LIGHTNING..THUNDERSTORM
- {0x2609, 0x2609, prAI, gcSo}, // SUN
- {0x260A, 0x260D, prAL, gcSo}, // [4] ASCENDING NODE..OPPOSITION
- {0x260E, 0x260F, prAI, gcSo}, // [2] BLACK TELEPHONE..WHITE TELEPHONE
- {0x2610, 0x2613, prAL, gcSo}, // [4] BALLOT BOX..SALTIRE
- {0x2614, 0x2615, prID, gcSo}, // [2] UMBRELLA WITH RAIN DROPS..HOT BEVERAGE
- {0x2616, 0x2617, prAI, gcSo}, // [2] WHITE SHOGI PIECE..BLACK SHOGI PIECE
- {0x2618, 0x2618, prID, gcSo}, // SHAMROCK
- {0x2619, 0x2619, prAL, gcSo}, // REVERSED ROTATED FLORAL HEART BULLET
- {0x261A, 0x261C, prID, gcSo}, // [3] BLACK LEFT POINTING INDEX..WHITE LEFT POINTING INDEX
- {0x261D, 0x261D, prEB, gcSo}, // WHITE UP POINTING INDEX
- {0x261E, 0x261F, prID, gcSo}, // [2] WHITE RIGHT POINTING INDEX..WHITE DOWN POINTING INDEX
- {0x2620, 0x2638, prAL, gcSo}, // [25] SKULL AND CROSSBONES..WHEEL OF DHARMA
- {0x2639, 0x263B, prID, gcSo}, // [3] WHITE FROWNING FACE..BLACK SMILING FACE
- {0x263C, 0x263F, prAL, gcSo}, // [4] WHITE SUN WITH RAYS..MERCURY
- {0x2640, 0x2640, prAI, gcSo}, // FEMALE SIGN
- {0x2641, 0x2641, prAL, gcSo}, // EARTH
- {0x2642, 0x2642, prAI, gcSo}, // MALE SIGN
- {0x2643, 0x265F, prAL, gcSo}, // [29] JUPITER..BLACK CHESS PAWN
- {0x2660, 0x2661, prAI, gcSo}, // [2] BLACK SPADE SUIT..WHITE HEART SUIT
- {0x2662, 0x2662, prAL, gcSo}, // WHITE DIAMOND SUIT
- {0x2663, 0x2665, prAI, gcSo}, // [3] BLACK CLUB SUIT..BLACK HEART SUIT
- {0x2666, 0x2666, prAL, gcSo}, // BLACK DIAMOND SUIT
- {0x2667, 0x2667, prAI, gcSo}, // WHITE CLUB SUIT
- {0x2668, 0x2668, prID, gcSo}, // HOT SPRINGS
- {0x2669, 0x266A, prAI, gcSo}, // [2] QUARTER NOTE..EIGHTH NOTE
- {0x266B, 0x266B, prAL, gcSo}, // BEAMED EIGHTH NOTES
- {0x266C, 0x266D, prAI, gcSo}, // [2] BEAMED SIXTEENTH NOTES..MUSIC FLAT SIGN
- {0x266E, 0x266E, prAL, gcSo}, // MUSIC NATURAL SIGN
- {0x266F, 0x266F, prAI, gcSm}, // MUSIC SHARP SIGN
- {0x2670, 0x267E, prAL, gcSo}, // [15] WEST SYRIAC CROSS..PERMANENT PAPER SIGN
- {0x267F, 0x267F, prID, gcSo}, // WHEELCHAIR SYMBOL
- {0x2680, 0x269D, prAL, gcSo}, // [30] DIE FACE-1..OUTLINED WHITE STAR
- {0x269E, 0x269F, prAI, gcSo}, // [2] THREE LINES CONVERGING RIGHT..THREE LINES CONVERGING LEFT
- {0x26A0, 0x26BC, prAL, gcSo}, // [29] WARNING SIGN..SESQUIQUADRATE
- {0x26BD, 0x26C8, prID, gcSo}, // [12] SOCCER BALL..THUNDER CLOUD AND RAIN
- {0x26C9, 0x26CC, prAI, gcSo}, // [4] TURNED WHITE SHOGI PIECE..CROSSING LANES
- {0x26CD, 0x26CD, prID, gcSo}, // DISABLED CAR
- {0x26CE, 0x26CE, prAL, gcSo}, // OPHIUCHUS
- {0x26CF, 0x26D1, prID, gcSo}, // [3] PICK..HELMET WITH WHITE CROSS
- {0x26D2, 0x26D2, prAI, gcSo}, // CIRCLED CROSSING LANES
- {0x26D3, 0x26D4, prID, gcSo}, // [2] CHAINS..NO ENTRY
- {0x26D5, 0x26D7, prAI, gcSo}, // [3] ALTERNATE ONE-WAY LEFT WAY TRAFFIC..WHITE TWO-WAY LEFT WAY TRAFFIC
- {0x26D8, 0x26D9, prID, gcSo}, // [2] BLACK LEFT LANE MERGE..WHITE LEFT LANE MERGE
- {0x26DA, 0x26DB, prAI, gcSo}, // [2] DRIVE SLOW SIGN..HEAVY WHITE DOWN-POINTING TRIANGLE
- {0x26DC, 0x26DC, prID, gcSo}, // LEFT CLOSED ENTRY
- {0x26DD, 0x26DE, prAI, gcSo}, // [2] SQUARED SALTIRE..FALLING DIAGONAL IN WHITE CIRCLE IN BLACK SQUARE
- {0x26DF, 0x26E1, prID, gcSo}, // [3] BLACK TRUCK..RESTRICTED LEFT ENTRY-2
- {0x26E2, 0x26E2, prAL, gcSo}, // ASTRONOMICAL SYMBOL FOR URANUS
- {0x26E3, 0x26E3, prAI, gcSo}, // HEAVY CIRCLE WITH STROKE AND TWO DOTS ABOVE
- {0x26E4, 0x26E7, prAL, gcSo}, // [4] PENTAGRAM..INVERTED PENTAGRAM
- {0x26E8, 0x26E9, prAI, gcSo}, // [2] BLACK CROSS ON SHIELD..SHINTO SHRINE
- {0x26EA, 0x26EA, prID, gcSo}, // CHURCH
- {0x26EB, 0x26F0, prAI, gcSo}, // [6] CASTLE..MOUNTAIN
- {0x26F1, 0x26F5, prID, gcSo}, // [5] UMBRELLA ON GROUND..SAILBOAT
- {0x26F6, 0x26F6, prAI, gcSo}, // SQUARE FOUR CORNERS
- {0x26F7, 0x26F8, prID, gcSo}, // [2] SKIER..ICE SKATE
- {0x26F9, 0x26F9, prEB, gcSo}, // PERSON WITH BALL
- {0x26FA, 0x26FA, prID, gcSo}, // TENT
- {0x26FB, 0x26FC, prAI, gcSo}, // [2] JAPANESE BANK SYMBOL..HEADSTONE GRAVEYARD SYMBOL
- {0x26FD, 0x26FF, prID, gcSo}, // [3] FUEL PUMP..WHITE FLAG WITH HORIZONTAL MIDDLE BLACK STRIPE
- {0x2700, 0x2704, prID, gcSo}, // [5] BLACK SAFETY SCISSORS..WHITE SCISSORS
- {0x2705, 0x2707, prAL, gcSo}, // [3] WHITE HEAVY CHECK MARK..TAPE DRIVE
- {0x2708, 0x2709, prID, gcSo}, // [2] AIRPLANE..ENVELOPE
- {0x270A, 0x270D, prEB, gcSo}, // [4] RAISED FIST..WRITING HAND
- {0x270E, 0x2756, prAL, gcSo}, // [73] LOWER RIGHT PENCIL..BLACK DIAMOND MINUS WHITE X
- {0x2757, 0x2757, prAI, gcSo}, // HEAVY EXCLAMATION MARK SYMBOL
- {0x2758, 0x275A, prAL, gcSo}, // [3] LIGHT VERTICAL BAR..HEAVY VERTICAL BAR
- {0x275B, 0x2760, prQU, gcSo}, // [6] HEAVY SINGLE TURNED COMMA QUOTATION MARK ORNAMENT..HEAVY LOW DOUBLE COMMA QUOTATION MARK ORNAMENT
- {0x2761, 0x2761, prAL, gcSo}, // CURVED STEM PARAGRAPH SIGN ORNAMENT
- {0x2762, 0x2763, prEX, gcSo}, // [2] HEAVY EXCLAMATION MARK ORNAMENT..HEAVY HEART EXCLAMATION MARK ORNAMENT
- {0x2764, 0x2764, prID, gcSo}, // HEAVY BLACK HEART
- {0x2765, 0x2767, prAL, gcSo}, // [3] ROTATED HEAVY BLACK HEART BULLET..ROTATED FLORAL HEART BULLET
- {0x2768, 0x2768, prOP, gcPs}, // MEDIUM LEFT PARENTHESIS ORNAMENT
- {0x2769, 0x2769, prCL, gcPe}, // MEDIUM RIGHT PARENTHESIS ORNAMENT
- {0x276A, 0x276A, prOP, gcPs}, // MEDIUM FLATTENED LEFT PARENTHESIS ORNAMENT
- {0x276B, 0x276B, prCL, gcPe}, // MEDIUM FLATTENED RIGHT PARENTHESIS ORNAMENT
- {0x276C, 0x276C, prOP, gcPs}, // MEDIUM LEFT-POINTING ANGLE BRACKET ORNAMENT
- {0x276D, 0x276D, prCL, gcPe}, // MEDIUM RIGHT-POINTING ANGLE BRACKET ORNAMENT
- {0x276E, 0x276E, prOP, gcPs}, // HEAVY LEFT-POINTING ANGLE QUOTATION MARK ORNAMENT
- {0x276F, 0x276F, prCL, gcPe}, // HEAVY RIGHT-POINTING ANGLE QUOTATION MARK ORNAMENT
- {0x2770, 0x2770, prOP, gcPs}, // HEAVY LEFT-POINTING ANGLE BRACKET ORNAMENT
- {0x2771, 0x2771, prCL, gcPe}, // HEAVY RIGHT-POINTING ANGLE BRACKET ORNAMENT
- {0x2772, 0x2772, prOP, gcPs}, // LIGHT LEFT TORTOISE SHELL BRACKET ORNAMENT
- {0x2773, 0x2773, prCL, gcPe}, // LIGHT RIGHT TORTOISE SHELL BRACKET ORNAMENT
- {0x2774, 0x2774, prOP, gcPs}, // MEDIUM LEFT CURLY BRACKET ORNAMENT
- {0x2775, 0x2775, prCL, gcPe}, // MEDIUM RIGHT CURLY BRACKET ORNAMENT
- {0x2776, 0x2793, prAI, gcNo}, // [30] DINGBAT NEGATIVE CIRCLED DIGIT ONE..DINGBAT NEGATIVE CIRCLED SANS-SERIF NUMBER TEN
- {0x2794, 0x27BF, prAL, gcSo}, // [44] HEAVY WIDE-HEADED RIGHTWARDS ARROW..DOUBLE CURLY LOOP
- {0x27C0, 0x27C4, prAL, gcSm}, // [5] THREE DIMENSIONAL ANGLE..OPEN SUPERSET
- {0x27C5, 0x27C5, prOP, gcPs}, // LEFT S-SHAPED BAG DELIMITER
- {0x27C6, 0x27C6, prCL, gcPe}, // RIGHT S-SHAPED BAG DELIMITER
- {0x27C7, 0x27E5, prAL, gcSm}, // [31] OR WITH DOT INSIDE..WHITE SQUARE WITH RIGHTWARDS TICK
- {0x27E6, 0x27E6, prOP, gcPs}, // MATHEMATICAL LEFT WHITE SQUARE BRACKET
- {0x27E7, 0x27E7, prCL, gcPe}, // MATHEMATICAL RIGHT WHITE SQUARE BRACKET
- {0x27E8, 0x27E8, prOP, gcPs}, // MATHEMATICAL LEFT ANGLE BRACKET
- {0x27E9, 0x27E9, prCL, gcPe}, // MATHEMATICAL RIGHT ANGLE BRACKET
- {0x27EA, 0x27EA, prOP, gcPs}, // MATHEMATICAL LEFT DOUBLE ANGLE BRACKET
- {0x27EB, 0x27EB, prCL, gcPe}, // MATHEMATICAL RIGHT DOUBLE ANGLE BRACKET
- {0x27EC, 0x27EC, prOP, gcPs}, // MATHEMATICAL LEFT WHITE TORTOISE SHELL BRACKET
- {0x27ED, 0x27ED, prCL, gcPe}, // MATHEMATICAL RIGHT WHITE TORTOISE SHELL BRACKET
- {0x27EE, 0x27EE, prOP, gcPs}, // MATHEMATICAL LEFT FLATTENED PARENTHESIS
- {0x27EF, 0x27EF, prCL, gcPe}, // MATHEMATICAL RIGHT FLATTENED PARENTHESIS
- {0x27F0, 0x27FF, prAL, gcSm}, // [16] UPWARDS QUADRUPLE ARROW..LONG RIGHTWARDS SQUIGGLE ARROW
- {0x2800, 0x28FF, prAL, gcSo}, // [256] BRAILLE PATTERN BLANK..BRAILLE PATTERN DOTS-12345678
- {0x2900, 0x297F, prAL, gcSm}, // [128] RIGHTWARDS TWO-HEADED ARROW WITH VERTICAL STROKE..DOWN FISH TAIL
- {0x2980, 0x2982, prAL, gcSm}, // [3] TRIPLE VERTICAL BAR DELIMITER..Z NOTATION TYPE COLON
- {0x2983, 0x2983, prOP, gcPs}, // LEFT WHITE CURLY BRACKET
- {0x2984, 0x2984, prCL, gcPe}, // RIGHT WHITE CURLY BRACKET
- {0x2985, 0x2985, prOP, gcPs}, // LEFT WHITE PARENTHESIS
- {0x2986, 0x2986, prCL, gcPe}, // RIGHT WHITE PARENTHESIS
- {0x2987, 0x2987, prOP, gcPs}, // Z NOTATION LEFT IMAGE BRACKET
- {0x2988, 0x2988, prCL, gcPe}, // Z NOTATION RIGHT IMAGE BRACKET
- {0x2989, 0x2989, prOP, gcPs}, // Z NOTATION LEFT BINDING BRACKET
- {0x298A, 0x298A, prCL, gcPe}, // Z NOTATION RIGHT BINDING BRACKET
- {0x298B, 0x298B, prOP, gcPs}, // LEFT SQUARE BRACKET WITH UNDERBAR
- {0x298C, 0x298C, prCL, gcPe}, // RIGHT SQUARE BRACKET WITH UNDERBAR
- {0x298D, 0x298D, prOP, gcPs}, // LEFT SQUARE BRACKET WITH TICK IN TOP CORNER
- {0x298E, 0x298E, prCL, gcPe}, // RIGHT SQUARE BRACKET WITH TICK IN BOTTOM CORNER
- {0x298F, 0x298F, prOP, gcPs}, // LEFT SQUARE BRACKET WITH TICK IN BOTTOM CORNER
- {0x2990, 0x2990, prCL, gcPe}, // RIGHT SQUARE BRACKET WITH TICK IN TOP CORNER
- {0x2991, 0x2991, prOP, gcPs}, // LEFT ANGLE BRACKET WITH DOT
- {0x2992, 0x2992, prCL, gcPe}, // RIGHT ANGLE BRACKET WITH DOT
- {0x2993, 0x2993, prOP, gcPs}, // LEFT ARC LESS-THAN BRACKET
- {0x2994, 0x2994, prCL, gcPe}, // RIGHT ARC GREATER-THAN BRACKET
- {0x2995, 0x2995, prOP, gcPs}, // DOUBLE LEFT ARC GREATER-THAN BRACKET
- {0x2996, 0x2996, prCL, gcPe}, // DOUBLE RIGHT ARC LESS-THAN BRACKET
- {0x2997, 0x2997, prOP, gcPs}, // LEFT BLACK TORTOISE SHELL BRACKET
- {0x2998, 0x2998, prCL, gcPe}, // RIGHT BLACK TORTOISE SHELL BRACKET
- {0x2999, 0x29D7, prAL, gcSm}, // [63] DOTTED FENCE..BLACK HOURGLASS
- {0x29D8, 0x29D8, prOP, gcPs}, // LEFT WIGGLY FENCE
- {0x29D9, 0x29D9, prCL, gcPe}, // RIGHT WIGGLY FENCE
- {0x29DA, 0x29DA, prOP, gcPs}, // LEFT DOUBLE WIGGLY FENCE
- {0x29DB, 0x29DB, prCL, gcPe}, // RIGHT DOUBLE WIGGLY FENCE
- {0x29DC, 0x29FB, prAL, gcSm}, // [32] INCOMPLETE INFINITY..TRIPLE PLUS
- {0x29FC, 0x29FC, prOP, gcPs}, // LEFT-POINTING CURVED ANGLE BRACKET
- {0x29FD, 0x29FD, prCL, gcPe}, // RIGHT-POINTING CURVED ANGLE BRACKET
- {0x29FE, 0x29FF, prAL, gcSm}, // [2] TINY..MINY
- {0x2A00, 0x2AFF, prAL, gcSm}, // [256] N-ARY CIRCLED DOT OPERATOR..N-ARY WHITE VERTICAL BAR
- {0x2B00, 0x2B2F, prAL, gcSo}, // [48] NORTH EAST WHITE ARROW..WHITE VERTICAL ELLIPSE
- {0x2B30, 0x2B44, prAL, gcSm}, // [21] LEFT ARROW WITH SMALL CIRCLE..RIGHTWARDS ARROW THROUGH SUPERSET
- {0x2B45, 0x2B46, prAL, gcSo}, // [2] LEFTWARDS QUADRUPLE ARROW..RIGHTWARDS QUADRUPLE ARROW
- {0x2B47, 0x2B4C, prAL, gcSm}, // [6] REVERSE TILDE OPERATOR ABOVE RIGHTWARDS ARROW..RIGHTWARDS ARROW ABOVE REVERSE TILDE OPERATOR
- {0x2B4D, 0x2B54, prAL, gcSo}, // [8] DOWNWARDS TRIANGLE-HEADED ZIGZAG ARROW..WHITE RIGHT-POINTING PENTAGON
- {0x2B55, 0x2B59, prAI, gcSo}, // [5] HEAVY LARGE CIRCLE..HEAVY CIRCLED SALTIRE
- {0x2B5A, 0x2B73, prAL, gcSo}, // [26] SLANTED NORTH ARROW WITH HOOKED HEAD..DOWNWARDS TRIANGLE-HEADED ARROW TO BAR
- {0x2B76, 0x2B95, prAL, gcSo}, // [32] NORTH WEST TRIANGLE-HEADED ARROW TO BAR..RIGHTWARDS BLACK ARROW
- {0x2B97, 0x2BFF, prAL, gcSo}, // [105] SYMBOL FOR TYPE A ELECTRONICS..HELLSCHREIBER PAUSE SYMBOL
- {0x2C00, 0x2C5F, prAL, gcLC}, // [96] GLAGOLITIC CAPITAL LETTER AZU..GLAGOLITIC SMALL LETTER CAUDATE CHRIVI
- {0x2C60, 0x2C7B, prAL, gcLC}, // [28] LATIN CAPITAL LETTER L WITH DOUBLE BAR..LATIN LETTER SMALL CAPITAL TURNED E
- {0x2C7C, 0x2C7D, prAL, gcLm}, // [2] LATIN SUBSCRIPT SMALL LETTER J..MODIFIER LETTER CAPITAL V
- {0x2C7E, 0x2C7F, prAL, gcLu}, // [2] LATIN CAPITAL LETTER S WITH SWASH TAIL..LATIN CAPITAL LETTER Z WITH SWASH TAIL
- {0x2C80, 0x2CE4, prAL, gcLC}, // [101] COPTIC CAPITAL LETTER ALFA..COPTIC SYMBOL KAI
- {0x2CE5, 0x2CEA, prAL, gcSo}, // [6] COPTIC SYMBOL MI RO..COPTIC SYMBOL SHIMA SIMA
- {0x2CEB, 0x2CEE, prAL, gcLC}, // [4] COPTIC CAPITAL LETTER CRYPTOGRAMMIC SHEI..COPTIC SMALL LETTER CRYPTOGRAMMIC GANGIA
- {0x2CEF, 0x2CF1, prCM, gcMn}, // [3] COPTIC COMBINING NI ABOVE..COPTIC COMBINING SPIRITUS LENIS
- {0x2CF2, 0x2CF3, prAL, gcLC}, // [2] COPTIC CAPITAL LETTER BOHAIRIC KHEI..COPTIC SMALL LETTER BOHAIRIC KHEI
- {0x2CF9, 0x2CF9, prEX, gcPo}, // COPTIC OLD NUBIAN FULL STOP
- {0x2CFA, 0x2CFC, prBA, gcPo}, // [3] COPTIC OLD NUBIAN DIRECT QUESTION MARK..COPTIC OLD NUBIAN VERSE DIVIDER
- {0x2CFD, 0x2CFD, prAL, gcNo}, // COPTIC FRACTION ONE HALF
- {0x2CFE, 0x2CFE, prEX, gcPo}, // COPTIC FULL STOP
- {0x2CFF, 0x2CFF, prBA, gcPo}, // COPTIC MORPHOLOGICAL DIVIDER
- {0x2D00, 0x2D25, prAL, gcLl}, // [38] GEORGIAN SMALL LETTER AN..GEORGIAN SMALL LETTER HOE
- {0x2D27, 0x2D27, prAL, gcLl}, // GEORGIAN SMALL LETTER YN
- {0x2D2D, 0x2D2D, prAL, gcLl}, // GEORGIAN SMALL LETTER AEN
- {0x2D30, 0x2D67, prAL, gcLo}, // [56] TIFINAGH LETTER YA..TIFINAGH LETTER YO
- {0x2D6F, 0x2D6F, prAL, gcLm}, // TIFINAGH MODIFIER LETTER LABIALIZATION MARK
- {0x2D70, 0x2D70, prBA, gcPo}, // TIFINAGH SEPARATOR MARK
- {0x2D7F, 0x2D7F, prCM, gcMn}, // TIFINAGH CONSONANT JOINER
- {0x2D80, 0x2D96, prAL, gcLo}, // [23] ETHIOPIC SYLLABLE LOA..ETHIOPIC SYLLABLE GGWE
- {0x2DA0, 0x2DA6, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE SSA..ETHIOPIC SYLLABLE SSO
- {0x2DA8, 0x2DAE, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE CCA..ETHIOPIC SYLLABLE CCO
- {0x2DB0, 0x2DB6, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE ZZA..ETHIOPIC SYLLABLE ZZO
- {0x2DB8, 0x2DBE, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE CCHA..ETHIOPIC SYLLABLE CCHO
- {0x2DC0, 0x2DC6, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE QYA..ETHIOPIC SYLLABLE QYO
- {0x2DC8, 0x2DCE, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE KYA..ETHIOPIC SYLLABLE KYO
- {0x2DD0, 0x2DD6, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE XYA..ETHIOPIC SYLLABLE XYO
- {0x2DD8, 0x2DDE, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE GYA..ETHIOPIC SYLLABLE GYO
- {0x2DE0, 0x2DFF, prCM, gcMn}, // [32] COMBINING CYRILLIC LETTER BE..COMBINING CYRILLIC LETTER IOTIFIED BIG YUS
- {0x2E00, 0x2E01, prQU, gcPo}, // [2] RIGHT ANGLE SUBSTITUTION MARKER..RIGHT ANGLE DOTTED SUBSTITUTION MARKER
- {0x2E02, 0x2E02, prQU, gcPi}, // LEFT SUBSTITUTION BRACKET
- {0x2E03, 0x2E03, prQU, gcPf}, // RIGHT SUBSTITUTION BRACKET
- {0x2E04, 0x2E04, prQU, gcPi}, // LEFT DOTTED SUBSTITUTION BRACKET
- {0x2E05, 0x2E05, prQU, gcPf}, // RIGHT DOTTED SUBSTITUTION BRACKET
- {0x2E06, 0x2E08, prQU, gcPo}, // [3] RAISED INTERPOLATION MARKER..DOTTED TRANSPOSITION MARKER
- {0x2E09, 0x2E09, prQU, gcPi}, // LEFT TRANSPOSITION BRACKET
- {0x2E0A, 0x2E0A, prQU, gcPf}, // RIGHT TRANSPOSITION BRACKET
- {0x2E0B, 0x2E0B, prQU, gcPo}, // RAISED SQUARE
- {0x2E0C, 0x2E0C, prQU, gcPi}, // LEFT RAISED OMISSION BRACKET
- {0x2E0D, 0x2E0D, prQU, gcPf}, // RIGHT RAISED OMISSION BRACKET
- {0x2E0E, 0x2E15, prBA, gcPo}, // [8] EDITORIAL CORONIS..UPWARDS ANCORA
- {0x2E16, 0x2E16, prAL, gcPo}, // DOTTED RIGHT-POINTING ANGLE
- {0x2E17, 0x2E17, prBA, gcPd}, // DOUBLE OBLIQUE HYPHEN
- {0x2E18, 0x2E18, prOP, gcPo}, // INVERTED INTERROBANG
- {0x2E19, 0x2E19, prBA, gcPo}, // PALM BRANCH
- {0x2E1A, 0x2E1A, prAL, gcPd}, // HYPHEN WITH DIAERESIS
- {0x2E1B, 0x2E1B, prAL, gcPo}, // TILDE WITH RING ABOVE
- {0x2E1C, 0x2E1C, prQU, gcPi}, // LEFT LOW PARAPHRASE BRACKET
- {0x2E1D, 0x2E1D, prQU, gcPf}, // RIGHT LOW PARAPHRASE BRACKET
- {0x2E1E, 0x2E1F, prAL, gcPo}, // [2] TILDE WITH DOT ABOVE..TILDE WITH DOT BELOW
- {0x2E20, 0x2E20, prQU, gcPi}, // LEFT VERTICAL BAR WITH QUILL
- {0x2E21, 0x2E21, prQU, gcPf}, // RIGHT VERTICAL BAR WITH QUILL
- {0x2E22, 0x2E22, prOP, gcPs}, // TOP LEFT HALF BRACKET
- {0x2E23, 0x2E23, prCL, gcPe}, // TOP RIGHT HALF BRACKET
- {0x2E24, 0x2E24, prOP, gcPs}, // BOTTOM LEFT HALF BRACKET
- {0x2E25, 0x2E25, prCL, gcPe}, // BOTTOM RIGHT HALF BRACKET
- {0x2E26, 0x2E26, prOP, gcPs}, // LEFT SIDEWAYS U BRACKET
- {0x2E27, 0x2E27, prCL, gcPe}, // RIGHT SIDEWAYS U BRACKET
- {0x2E28, 0x2E28, prOP, gcPs}, // LEFT DOUBLE PARENTHESIS
- {0x2E29, 0x2E29, prCL, gcPe}, // RIGHT DOUBLE PARENTHESIS
- {0x2E2A, 0x2E2D, prBA, gcPo}, // [4] TWO DOTS OVER ONE DOT PUNCTUATION..FIVE DOT MARK
- {0x2E2E, 0x2E2E, prEX, gcPo}, // REVERSED QUESTION MARK
- {0x2E2F, 0x2E2F, prAL, gcLm}, // VERTICAL TILDE
- {0x2E30, 0x2E31, prBA, gcPo}, // [2] RING POINT..WORD SEPARATOR MIDDLE DOT
- {0x2E32, 0x2E32, prAL, gcPo}, // TURNED COMMA
- {0x2E33, 0x2E34, prBA, gcPo}, // [2] RAISED DOT..RAISED COMMA
- {0x2E35, 0x2E39, prAL, gcPo}, // [5] TURNED SEMICOLON..TOP HALF SECTION SIGN
- {0x2E3A, 0x2E3B, prB2, gcPd}, // [2] TWO-EM DASH..THREE-EM DASH
- {0x2E3C, 0x2E3E, prBA, gcPo}, // [3] STENOGRAPHIC FULL STOP..WIGGLY VERTICAL LINE
- {0x2E3F, 0x2E3F, prAL, gcPo}, // CAPITULUM
- {0x2E40, 0x2E40, prBA, gcPd}, // DOUBLE HYPHEN
- {0x2E41, 0x2E41, prBA, gcPo}, // REVERSED COMMA
- {0x2E42, 0x2E42, prOP, gcPs}, // DOUBLE LOW-REVERSED-9 QUOTATION MARK
- {0x2E43, 0x2E4A, prBA, gcPo}, // [8] DASH WITH LEFT UPTURN..DOTTED SOLIDUS
- {0x2E4B, 0x2E4B, prAL, gcPo}, // TRIPLE DAGGER
- {0x2E4C, 0x2E4C, prBA, gcPo}, // MEDIEVAL COMMA
- {0x2E4D, 0x2E4D, prAL, gcPo}, // PARAGRAPHUS MARK
- {0x2E4E, 0x2E4F, prBA, gcPo}, // [2] PUNCTUS ELEVATUS MARK..CORNISH VERSE DIVIDER
- {0x2E50, 0x2E51, prAL, gcSo}, // [2] CROSS PATTY WITH RIGHT CROSSBAR..CROSS PATTY WITH LEFT CROSSBAR
- {0x2E52, 0x2E52, prAL, gcPo}, // TIRONIAN SIGN CAPITAL ET
- {0x2E53, 0x2E54, prEX, gcPo}, // [2] MEDIEVAL EXCLAMATION MARK..MEDIEVAL QUESTION MARK
- {0x2E55, 0x2E55, prOP, gcPs}, // LEFT SQUARE BRACKET WITH STROKE
- {0x2E56, 0x2E56, prCL, gcPe}, // RIGHT SQUARE BRACKET WITH STROKE
- {0x2E57, 0x2E57, prOP, gcPs}, // LEFT SQUARE BRACKET WITH DOUBLE STROKE
- {0x2E58, 0x2E58, prCL, gcPe}, // RIGHT SQUARE BRACKET WITH DOUBLE STROKE
- {0x2E59, 0x2E59, prOP, gcPs}, // TOP HALF LEFT PARENTHESIS
- {0x2E5A, 0x2E5A, prCL, gcPe}, // TOP HALF RIGHT PARENTHESIS
- {0x2E5B, 0x2E5B, prOP, gcPs}, // BOTTOM HALF LEFT PARENTHESIS
- {0x2E5C, 0x2E5C, prCL, gcPe}, // BOTTOM HALF RIGHT PARENTHESIS
- {0x2E5D, 0x2E5D, prBA, gcPd}, // OBLIQUE HYPHEN
- {0x2E80, 0x2E99, prID, gcSo}, // [26] CJK RADICAL REPEAT..CJK RADICAL RAP
- {0x2E9B, 0x2EF3, prID, gcSo}, // [89] CJK RADICAL CHOKE..CJK RADICAL C-SIMPLIFIED TURTLE
- {0x2F00, 0x2FD5, prID, gcSo}, // [214] KANGXI RADICAL ONE..KANGXI RADICAL FLUTE
- {0x2FF0, 0x2FFB, prID, gcSo}, // [12] IDEOGRAPHIC DESCRIPTION CHARACTER LEFT TO RIGHT..IDEOGRAPHIC DESCRIPTION CHARACTER OVERLAID
- {0x3000, 0x3000, prBA, gcZs}, // IDEOGRAPHIC SPACE
- {0x3001, 0x3002, prCL, gcPo}, // [2] IDEOGRAPHIC COMMA..IDEOGRAPHIC FULL STOP
- {0x3003, 0x3003, prID, gcPo}, // DITTO MARK
- {0x3004, 0x3004, prID, gcSo}, // JAPANESE INDUSTRIAL STANDARD SYMBOL
- {0x3005, 0x3005, prNS, gcLm}, // IDEOGRAPHIC ITERATION MARK
- {0x3006, 0x3006, prID, gcLo}, // IDEOGRAPHIC CLOSING MARK
- {0x3007, 0x3007, prID, gcNl}, // IDEOGRAPHIC NUMBER ZERO
- {0x3008, 0x3008, prOP, gcPs}, // LEFT ANGLE BRACKET
- {0x3009, 0x3009, prCL, gcPe}, // RIGHT ANGLE BRACKET
- {0x300A, 0x300A, prOP, gcPs}, // LEFT DOUBLE ANGLE BRACKET
- {0x300B, 0x300B, prCL, gcPe}, // RIGHT DOUBLE ANGLE BRACKET
- {0x300C, 0x300C, prOP, gcPs}, // LEFT CORNER BRACKET
- {0x300D, 0x300D, prCL, gcPe}, // RIGHT CORNER BRACKET
- {0x300E, 0x300E, prOP, gcPs}, // LEFT WHITE CORNER BRACKET
- {0x300F, 0x300F, prCL, gcPe}, // RIGHT WHITE CORNER BRACKET
- {0x3010, 0x3010, prOP, gcPs}, // LEFT BLACK LENTICULAR BRACKET
- {0x3011, 0x3011, prCL, gcPe}, // RIGHT BLACK LENTICULAR BRACKET
- {0x3012, 0x3013, prID, gcSo}, // [2] POSTAL MARK..GETA MARK
- {0x3014, 0x3014, prOP, gcPs}, // LEFT TORTOISE SHELL BRACKET
- {0x3015, 0x3015, prCL, gcPe}, // RIGHT TORTOISE SHELL BRACKET
- {0x3016, 0x3016, prOP, gcPs}, // LEFT WHITE LENTICULAR BRACKET
- {0x3017, 0x3017, prCL, gcPe}, // RIGHT WHITE LENTICULAR BRACKET
- {0x3018, 0x3018, prOP, gcPs}, // LEFT WHITE TORTOISE SHELL BRACKET
- {0x3019, 0x3019, prCL, gcPe}, // RIGHT WHITE TORTOISE SHELL BRACKET
- {0x301A, 0x301A, prOP, gcPs}, // LEFT WHITE SQUARE BRACKET
- {0x301B, 0x301B, prCL, gcPe}, // RIGHT WHITE SQUARE BRACKET
- {0x301C, 0x301C, prNS, gcPd}, // WAVE DASH
- {0x301D, 0x301D, prOP, gcPs}, // REVERSED DOUBLE PRIME QUOTATION MARK
- {0x301E, 0x301F, prCL, gcPe}, // [2] DOUBLE PRIME QUOTATION MARK..LOW DOUBLE PRIME QUOTATION MARK
- {0x3020, 0x3020, prID, gcSo}, // POSTAL MARK FACE
- {0x3021, 0x3029, prID, gcNl}, // [9] HANGZHOU NUMERAL ONE..HANGZHOU NUMERAL NINE
- {0x302A, 0x302D, prCM, gcMn}, // [4] IDEOGRAPHIC LEVEL TONE MARK..IDEOGRAPHIC ENTERING TONE MARK
- {0x302E, 0x302F, prCM, gcMc}, // [2] HANGUL SINGLE DOT TONE MARK..HANGUL DOUBLE DOT TONE MARK
- {0x3030, 0x3030, prID, gcPd}, // WAVY DASH
- {0x3031, 0x3034, prID, gcLm}, // [4] VERTICAL KANA REPEAT MARK..VERTICAL KANA REPEAT WITH VOICED SOUND MARK UPPER HALF
- {0x3035, 0x3035, prCM, gcLm}, // VERTICAL KANA REPEAT MARK LOWER HALF
- {0x3036, 0x3037, prID, gcSo}, // [2] CIRCLED POSTAL MARK..IDEOGRAPHIC TELEGRAPH LINE FEED SEPARATOR SYMBOL
- {0x3038, 0x303A, prID, gcNl}, // [3] HANGZHOU NUMERAL TEN..HANGZHOU NUMERAL THIRTY
- {0x303B, 0x303B, prNS, gcLm}, // VERTICAL IDEOGRAPHIC ITERATION MARK
- {0x303C, 0x303C, prNS, gcLo}, // MASU MARK
- {0x303D, 0x303D, prID, gcPo}, // PART ALTERNATION MARK
- {0x303E, 0x303F, prID, gcSo}, // [2] IDEOGRAPHIC VARIATION INDICATOR..IDEOGRAPHIC HALF FILL SPACE
- {0x3041, 0x3041, prCJ, gcLo}, // HIRAGANA LETTER SMALL A
- {0x3042, 0x3042, prID, gcLo}, // HIRAGANA LETTER A
- {0x3043, 0x3043, prCJ, gcLo}, // HIRAGANA LETTER SMALL I
- {0x3044, 0x3044, prID, gcLo}, // HIRAGANA LETTER I
- {0x3045, 0x3045, prCJ, gcLo}, // HIRAGANA LETTER SMALL U
- {0x3046, 0x3046, prID, gcLo}, // HIRAGANA LETTER U
- {0x3047, 0x3047, prCJ, gcLo}, // HIRAGANA LETTER SMALL E
- {0x3048, 0x3048, prID, gcLo}, // HIRAGANA LETTER E
- {0x3049, 0x3049, prCJ, gcLo}, // HIRAGANA LETTER SMALL O
- {0x304A, 0x3062, prID, gcLo}, // [25] HIRAGANA LETTER O..HIRAGANA LETTER DI
- {0x3063, 0x3063, prCJ, gcLo}, // HIRAGANA LETTER SMALL TU
- {0x3064, 0x3082, prID, gcLo}, // [31] HIRAGANA LETTER TU..HIRAGANA LETTER MO
- {0x3083, 0x3083, prCJ, gcLo}, // HIRAGANA LETTER SMALL YA
- {0x3084, 0x3084, prID, gcLo}, // HIRAGANA LETTER YA
- {0x3085, 0x3085, prCJ, gcLo}, // HIRAGANA LETTER SMALL YU
- {0x3086, 0x3086, prID, gcLo}, // HIRAGANA LETTER YU
- {0x3087, 0x3087, prCJ, gcLo}, // HIRAGANA LETTER SMALL YO
- {0x3088, 0x308D, prID, gcLo}, // [6] HIRAGANA LETTER YO..HIRAGANA LETTER RO
- {0x308E, 0x308E, prCJ, gcLo}, // HIRAGANA LETTER SMALL WA
- {0x308F, 0x3094, prID, gcLo}, // [6] HIRAGANA LETTER WA..HIRAGANA LETTER VU
- {0x3095, 0x3096, prCJ, gcLo}, // [2] HIRAGANA LETTER SMALL KA..HIRAGANA LETTER SMALL KE
- {0x3099, 0x309A, prCM, gcMn}, // [2] COMBINING KATAKANA-HIRAGANA VOICED SOUND MARK..COMBINING KATAKANA-HIRAGANA SEMI-VOICED SOUND MARK
- {0x309B, 0x309C, prNS, gcSk}, // [2] KATAKANA-HIRAGANA VOICED SOUND MARK..KATAKANA-HIRAGANA SEMI-VOICED SOUND MARK
- {0x309D, 0x309E, prNS, gcLm}, // [2] HIRAGANA ITERATION MARK..HIRAGANA VOICED ITERATION MARK
- {0x309F, 0x309F, prID, gcLo}, // HIRAGANA DIGRAPH YORI
- {0x30A0, 0x30A0, prNS, gcPd}, // KATAKANA-HIRAGANA DOUBLE HYPHEN
- {0x30A1, 0x30A1, prCJ, gcLo}, // KATAKANA LETTER SMALL A
- {0x30A2, 0x30A2, prID, gcLo}, // KATAKANA LETTER A
- {0x30A3, 0x30A3, prCJ, gcLo}, // KATAKANA LETTER SMALL I
- {0x30A4, 0x30A4, prID, gcLo}, // KATAKANA LETTER I
- {0x30A5, 0x30A5, prCJ, gcLo}, // KATAKANA LETTER SMALL U
- {0x30A6, 0x30A6, prID, gcLo}, // KATAKANA LETTER U
- {0x30A7, 0x30A7, prCJ, gcLo}, // KATAKANA LETTER SMALL E
- {0x30A8, 0x30A8, prID, gcLo}, // KATAKANA LETTER E
- {0x30A9, 0x30A9, prCJ, gcLo}, // KATAKANA LETTER SMALL O
- {0x30AA, 0x30C2, prID, gcLo}, // [25] KATAKANA LETTER O..KATAKANA LETTER DI
- {0x30C3, 0x30C3, prCJ, gcLo}, // KATAKANA LETTER SMALL TU
- {0x30C4, 0x30E2, prID, gcLo}, // [31] KATAKANA LETTER TU..KATAKANA LETTER MO
- {0x30E3, 0x30E3, prCJ, gcLo}, // KATAKANA LETTER SMALL YA
- {0x30E4, 0x30E4, prID, gcLo}, // KATAKANA LETTER YA
- {0x30E5, 0x30E5, prCJ, gcLo}, // KATAKANA LETTER SMALL YU
- {0x30E6, 0x30E6, prID, gcLo}, // KATAKANA LETTER YU
- {0x30E7, 0x30E7, prCJ, gcLo}, // KATAKANA LETTER SMALL YO
- {0x30E8, 0x30ED, prID, gcLo}, // [6] KATAKANA LETTER YO..KATAKANA LETTER RO
- {0x30EE, 0x30EE, prCJ, gcLo}, // KATAKANA LETTER SMALL WA
- {0x30EF, 0x30F4, prID, gcLo}, // [6] KATAKANA LETTER WA..KATAKANA LETTER VU
- {0x30F5, 0x30F6, prCJ, gcLo}, // [2] KATAKANA LETTER SMALL KA..KATAKANA LETTER SMALL KE
- {0x30F7, 0x30FA, prID, gcLo}, // [4] KATAKANA LETTER VA..KATAKANA LETTER VO
- {0x30FB, 0x30FB, prNS, gcPo}, // KATAKANA MIDDLE DOT
- {0x30FC, 0x30FC, prCJ, gcLm}, // KATAKANA-HIRAGANA PROLONGED SOUND MARK
- {0x30FD, 0x30FE, prNS, gcLm}, // [2] KATAKANA ITERATION MARK..KATAKANA VOICED ITERATION MARK
- {0x30FF, 0x30FF, prID, gcLo}, // KATAKANA DIGRAPH KOTO
- {0x3105, 0x312F, prID, gcLo}, // [43] BOPOMOFO LETTER B..BOPOMOFO LETTER NN
- {0x3131, 0x318E, prID, gcLo}, // [94] HANGUL LETTER KIYEOK..HANGUL LETTER ARAEAE
- {0x3190, 0x3191, prID, gcSo}, // [2] IDEOGRAPHIC ANNOTATION LINKING MARK..IDEOGRAPHIC ANNOTATION REVERSE MARK
- {0x3192, 0x3195, prID, gcNo}, // [4] IDEOGRAPHIC ANNOTATION ONE MARK..IDEOGRAPHIC ANNOTATION FOUR MARK
- {0x3196, 0x319F, prID, gcSo}, // [10] IDEOGRAPHIC ANNOTATION TOP MARK..IDEOGRAPHIC ANNOTATION MAN MARK
- {0x31A0, 0x31BF, prID, gcLo}, // [32] BOPOMOFO LETTER BU..BOPOMOFO LETTER AH
- {0x31C0, 0x31E3, prID, gcSo}, // [36] CJK STROKE T..CJK STROKE Q
- {0x31F0, 0x31FF, prCJ, gcLo}, // [16] KATAKANA LETTER SMALL KU..KATAKANA LETTER SMALL RO
- {0x3200, 0x321E, prID, gcSo}, // [31] PARENTHESIZED HANGUL KIYEOK..PARENTHESIZED KOREAN CHARACTER O HU
- {0x3220, 0x3229, prID, gcNo}, // [10] PARENTHESIZED IDEOGRAPH ONE..PARENTHESIZED IDEOGRAPH TEN
- {0x322A, 0x3247, prID, gcSo}, // [30] PARENTHESIZED IDEOGRAPH MOON..CIRCLED IDEOGRAPH KOTO
- {0x3248, 0x324F, prAI, gcNo}, // [8] CIRCLED NUMBER TEN ON BLACK SQUARE..CIRCLED NUMBER EIGHTY ON BLACK SQUARE
- {0x3250, 0x3250, prID, gcSo}, // PARTNERSHIP SIGN
- {0x3251, 0x325F, prID, gcNo}, // [15] CIRCLED NUMBER TWENTY ONE..CIRCLED NUMBER THIRTY FIVE
- {0x3260, 0x327F, prID, gcSo}, // [32] CIRCLED HANGUL KIYEOK..KOREAN STANDARD SYMBOL
- {0x3280, 0x3289, prID, gcNo}, // [10] CIRCLED IDEOGRAPH ONE..CIRCLED IDEOGRAPH TEN
- {0x328A, 0x32B0, prID, gcSo}, // [39] CIRCLED IDEOGRAPH MOON..CIRCLED IDEOGRAPH NIGHT
- {0x32B1, 0x32BF, prID, gcNo}, // [15] CIRCLED NUMBER THIRTY SIX..CIRCLED NUMBER FIFTY
- {0x32C0, 0x32FF, prID, gcSo}, // [64] IDEOGRAPHIC TELEGRAPH SYMBOL FOR JANUARY..SQUARE ERA NAME REIWA
- {0x3300, 0x33FF, prID, gcSo}, // [256] SQUARE APAATO..SQUARE GAL
- {0x3400, 0x4DBF, prID, gcLo}, // [6592] CJK UNIFIED IDEOGRAPH-3400..CJK UNIFIED IDEOGRAPH-4DBF
- {0x4DC0, 0x4DFF, prAL, gcSo}, // [64] HEXAGRAM FOR THE CREATIVE HEAVEN..HEXAGRAM FOR BEFORE COMPLETION
- {0x4E00, 0x9FFF, prID, gcLo}, // [20992] CJK UNIFIED IDEOGRAPH-4E00..CJK UNIFIED IDEOGRAPH-9FFF
- {0xA000, 0xA014, prID, gcLo}, // [21] YI SYLLABLE IT..YI SYLLABLE E
- {0xA015, 0xA015, prNS, gcLm}, // YI SYLLABLE WU
- {0xA016, 0xA48C, prID, gcLo}, // [1143] YI SYLLABLE BIT..YI SYLLABLE YYR
- {0xA490, 0xA4C6, prID, gcSo}, // [55] YI RADICAL QOT..YI RADICAL KE
- {0xA4D0, 0xA4F7, prAL, gcLo}, // [40] LISU LETTER BA..LISU LETTER OE
- {0xA4F8, 0xA4FD, prAL, gcLm}, // [6] LISU LETTER TONE MYA TI..LISU LETTER TONE MYA JEU
- {0xA4FE, 0xA4FF, prBA, gcPo}, // [2] LISU PUNCTUATION COMMA..LISU PUNCTUATION FULL STOP
- {0xA500, 0xA60B, prAL, gcLo}, // [268] VAI SYLLABLE EE..VAI SYLLABLE NG
- {0xA60C, 0xA60C, prAL, gcLm}, // VAI SYLLABLE LENGTHENER
- {0xA60D, 0xA60D, prBA, gcPo}, // VAI COMMA
- {0xA60E, 0xA60E, prEX, gcPo}, // VAI FULL STOP
- {0xA60F, 0xA60F, prBA, gcPo}, // VAI QUESTION MARK
- {0xA610, 0xA61F, prAL, gcLo}, // [16] VAI SYLLABLE NDOLE FA..VAI SYMBOL JONG
- {0xA620, 0xA629, prNU, gcNd}, // [10] VAI DIGIT ZERO..VAI DIGIT NINE
- {0xA62A, 0xA62B, prAL, gcLo}, // [2] VAI SYLLABLE NDOLE MA..VAI SYLLABLE NDOLE DO
- {0xA640, 0xA66D, prAL, gcLC}, // [46] CYRILLIC CAPITAL LETTER ZEMLYA..CYRILLIC SMALL LETTER DOUBLE MONOCULAR O
- {0xA66E, 0xA66E, prAL, gcLo}, // CYRILLIC LETTER MULTIOCULAR O
- {0xA66F, 0xA66F, prCM, gcMn}, // COMBINING CYRILLIC VZMET
- {0xA670, 0xA672, prCM, gcMe}, // [3] COMBINING CYRILLIC TEN MILLIONS SIGN..COMBINING CYRILLIC THOUSAND MILLIONS SIGN
- {0xA673, 0xA673, prAL, gcPo}, // SLAVONIC ASTERISK
- {0xA674, 0xA67D, prCM, gcMn}, // [10] COMBINING CYRILLIC LETTER UKRAINIAN IE..COMBINING CYRILLIC PAYEROK
- {0xA67E, 0xA67E, prAL, gcPo}, // CYRILLIC KAVYKA
- {0xA67F, 0xA67F, prAL, gcLm}, // CYRILLIC PAYEROK
- {0xA680, 0xA69B, prAL, gcLC}, // [28] CYRILLIC CAPITAL LETTER DWE..CYRILLIC SMALL LETTER CROSSED O
- {0xA69C, 0xA69D, prAL, gcLm}, // [2] MODIFIER LETTER CYRILLIC HARD SIGN..MODIFIER LETTER CYRILLIC SOFT SIGN
- {0xA69E, 0xA69F, prCM, gcMn}, // [2] COMBINING CYRILLIC LETTER EF..COMBINING CYRILLIC LETTER IOTIFIED E
- {0xA6A0, 0xA6E5, prAL, gcLo}, // [70] BAMUM LETTER A..BAMUM LETTER KI
- {0xA6E6, 0xA6EF, prAL, gcNl}, // [10] BAMUM LETTER MO..BAMUM LETTER KOGHOM
- {0xA6F0, 0xA6F1, prCM, gcMn}, // [2] BAMUM COMBINING MARK KOQNDON..BAMUM COMBINING MARK TUKWENTIS
- {0xA6F2, 0xA6F2, prAL, gcPo}, // BAMUM NJAEMLI
- {0xA6F3, 0xA6F7, prBA, gcPo}, // [5] BAMUM FULL STOP..BAMUM QUESTION MARK
- {0xA700, 0xA716, prAL, gcSk}, // [23] MODIFIER LETTER CHINESE TONE YIN PING..MODIFIER LETTER EXTRA-LOW LEFT-STEM TONE BAR
- {0xA717, 0xA71F, prAL, gcLm}, // [9] MODIFIER LETTER DOT VERTICAL BAR..MODIFIER LETTER LOW INVERTED EXCLAMATION MARK
- {0xA720, 0xA721, prAL, gcSk}, // [2] MODIFIER LETTER STRESS AND HIGH TONE..MODIFIER LETTER STRESS AND LOW TONE
- {0xA722, 0xA76F, prAL, gcLC}, // [78] LATIN CAPITAL LETTER EGYPTOLOGICAL ALEF..LATIN SMALL LETTER CON
- {0xA770, 0xA770, prAL, gcLm}, // MODIFIER LETTER US
- {0xA771, 0xA787, prAL, gcLC}, // [23] LATIN SMALL LETTER DUM..LATIN SMALL LETTER INSULAR T
- {0xA788, 0xA788, prAL, gcLm}, // MODIFIER LETTER LOW CIRCUMFLEX ACCENT
- {0xA789, 0xA78A, prAL, gcSk}, // [2] MODIFIER LETTER COLON..MODIFIER LETTER SHORT EQUALS SIGN
- {0xA78B, 0xA78E, prAL, gcLC}, // [4] LATIN CAPITAL LETTER SALTILLO..LATIN SMALL LETTER L WITH RETROFLEX HOOK AND BELT
- {0xA78F, 0xA78F, prAL, gcLo}, // LATIN LETTER SINOLOGICAL DOT
- {0xA790, 0xA7CA, prAL, gcLC}, // [59] LATIN CAPITAL LETTER N WITH DESCENDER..LATIN SMALL LETTER S WITH SHORT STROKE OVERLAY
- {0xA7D0, 0xA7D1, prAL, gcLC}, // [2] LATIN CAPITAL LETTER CLOSED INSULAR G..LATIN SMALL LETTER CLOSED INSULAR G
- {0xA7D3, 0xA7D3, prAL, gcLl}, // LATIN SMALL LETTER DOUBLE THORN
- {0xA7D5, 0xA7D9, prAL, gcLC}, // [5] LATIN SMALL LETTER DOUBLE WYNN..LATIN SMALL LETTER SIGMOID S
- {0xA7F2, 0xA7F4, prAL, gcLm}, // [3] MODIFIER LETTER CAPITAL C..MODIFIER LETTER CAPITAL Q
- {0xA7F5, 0xA7F6, prAL, gcLC}, // [2] LATIN CAPITAL LETTER REVERSED HALF H..LATIN SMALL LETTER REVERSED HALF H
- {0xA7F7, 0xA7F7, prAL, gcLo}, // LATIN EPIGRAPHIC LETTER SIDEWAYS I
- {0xA7F8, 0xA7F9, prAL, gcLm}, // [2] MODIFIER LETTER CAPITAL H WITH STROKE..MODIFIER LETTER SMALL LIGATURE OE
- {0xA7FA, 0xA7FA, prAL, gcLl}, // LATIN LETTER SMALL CAPITAL TURNED M
- {0xA7FB, 0xA7FF, prAL, gcLo}, // [5] LATIN EPIGRAPHIC LETTER REVERSED F..LATIN EPIGRAPHIC LETTER ARCHAIC M
- {0xA800, 0xA801, prAL, gcLo}, // [2] SYLOTI NAGRI LETTER A..SYLOTI NAGRI LETTER I
- {0xA802, 0xA802, prCM, gcMn}, // SYLOTI NAGRI SIGN DVISVARA
- {0xA803, 0xA805, prAL, gcLo}, // [3] SYLOTI NAGRI LETTER U..SYLOTI NAGRI LETTER O
- {0xA806, 0xA806, prCM, gcMn}, // SYLOTI NAGRI SIGN HASANTA
- {0xA807, 0xA80A, prAL, gcLo}, // [4] SYLOTI NAGRI LETTER KO..SYLOTI NAGRI LETTER GHO
- {0xA80B, 0xA80B, prCM, gcMn}, // SYLOTI NAGRI SIGN ANUSVARA
- {0xA80C, 0xA822, prAL, gcLo}, // [23] SYLOTI NAGRI LETTER CO..SYLOTI NAGRI LETTER HO
- {0xA823, 0xA824, prCM, gcMc}, // [2] SYLOTI NAGRI VOWEL SIGN A..SYLOTI NAGRI VOWEL SIGN I
- {0xA825, 0xA826, prCM, gcMn}, // [2] SYLOTI NAGRI VOWEL SIGN U..SYLOTI NAGRI VOWEL SIGN E
- {0xA827, 0xA827, prCM, gcMc}, // SYLOTI NAGRI VOWEL SIGN OO
- {0xA828, 0xA82B, prAL, gcSo}, // [4] SYLOTI NAGRI POETRY MARK-1..SYLOTI NAGRI POETRY MARK-4
- {0xA82C, 0xA82C, prCM, gcMn}, // SYLOTI NAGRI SIGN ALTERNATE HASANTA
- {0xA830, 0xA835, prAL, gcNo}, // [6] NORTH INDIC FRACTION ONE QUARTER..NORTH INDIC FRACTION THREE SIXTEENTHS
- {0xA836, 0xA837, prAL, gcSo}, // [2] NORTH INDIC QUARTER MARK..NORTH INDIC PLACEHOLDER MARK
- {0xA838, 0xA838, prPO, gcSc}, // NORTH INDIC RUPEE MARK
- {0xA839, 0xA839, prAL, gcSo}, // NORTH INDIC QUANTITY MARK
- {0xA840, 0xA873, prAL, gcLo}, // [52] PHAGS-PA LETTER KA..PHAGS-PA LETTER CANDRABINDU
- {0xA874, 0xA875, prBB, gcPo}, // [2] PHAGS-PA SINGLE HEAD MARK..PHAGS-PA DOUBLE HEAD MARK
- {0xA876, 0xA877, prEX, gcPo}, // [2] PHAGS-PA MARK SHAD..PHAGS-PA MARK DOUBLE SHAD
- {0xA880, 0xA881, prCM, gcMc}, // [2] SAURASHTRA SIGN ANUSVARA..SAURASHTRA SIGN VISARGA
- {0xA882, 0xA8B3, prAL, gcLo}, // [50] SAURASHTRA LETTER A..SAURASHTRA LETTER LLA
- {0xA8B4, 0xA8C3, prCM, gcMc}, // [16] SAURASHTRA CONSONANT SIGN HAARU..SAURASHTRA VOWEL SIGN AU
- {0xA8C4, 0xA8C5, prCM, gcMn}, // [2] SAURASHTRA SIGN VIRAMA..SAURASHTRA SIGN CANDRABINDU
- {0xA8CE, 0xA8CF, prBA, gcPo}, // [2] SAURASHTRA DANDA..SAURASHTRA DOUBLE DANDA
- {0xA8D0, 0xA8D9, prNU, gcNd}, // [10] SAURASHTRA DIGIT ZERO..SAURASHTRA DIGIT NINE
- {0xA8E0, 0xA8F1, prCM, gcMn}, // [18] COMBINING DEVANAGARI DIGIT ZERO..COMBINING DEVANAGARI SIGN AVAGRAHA
- {0xA8F2, 0xA8F7, prAL, gcLo}, // [6] DEVANAGARI SIGN SPACING CANDRABINDU..DEVANAGARI SIGN CANDRABINDU AVAGRAHA
- {0xA8F8, 0xA8FA, prAL, gcPo}, // [3] DEVANAGARI SIGN PUSHPIKA..DEVANAGARI CARET
- {0xA8FB, 0xA8FB, prAL, gcLo}, // DEVANAGARI HEADSTROKE
- {0xA8FC, 0xA8FC, prBB, gcPo}, // DEVANAGARI SIGN SIDDHAM
- {0xA8FD, 0xA8FE, prAL, gcLo}, // [2] DEVANAGARI JAIN OM..DEVANAGARI LETTER AY
- {0xA8FF, 0xA8FF, prCM, gcMn}, // DEVANAGARI VOWEL SIGN AY
- {0xA900, 0xA909, prNU, gcNd}, // [10] KAYAH LI DIGIT ZERO..KAYAH LI DIGIT NINE
- {0xA90A, 0xA925, prAL, gcLo}, // [28] KAYAH LI LETTER KA..KAYAH LI LETTER OO
- {0xA926, 0xA92D, prCM, gcMn}, // [8] KAYAH LI VOWEL UE..KAYAH LI TONE CALYA PLOPHU
- {0xA92E, 0xA92F, prBA, gcPo}, // [2] KAYAH LI SIGN CWI..KAYAH LI SIGN SHYA
- {0xA930, 0xA946, prAL, gcLo}, // [23] REJANG LETTER KA..REJANG LETTER A
- {0xA947, 0xA951, prCM, gcMn}, // [11] REJANG VOWEL SIGN I..REJANG CONSONANT SIGN R
- {0xA952, 0xA953, prCM, gcMc}, // [2] REJANG CONSONANT SIGN H..REJANG VIRAMA
- {0xA95F, 0xA95F, prAL, gcPo}, // REJANG SECTION MARK
- {0xA960, 0xA97C, prJL, gcLo}, // [29] HANGUL CHOSEONG TIKEUT-MIEUM..HANGUL CHOSEONG SSANGYEORINHIEUH
- {0xA980, 0xA982, prCM, gcMn}, // [3] JAVANESE SIGN PANYANGGA..JAVANESE SIGN LAYAR
- {0xA983, 0xA983, prCM, gcMc}, // JAVANESE SIGN WIGNYAN
- {0xA984, 0xA9B2, prAL, gcLo}, // [47] JAVANESE LETTER A..JAVANESE LETTER HA
- {0xA9B3, 0xA9B3, prCM, gcMn}, // JAVANESE SIGN CECAK TELU
- {0xA9B4, 0xA9B5, prCM, gcMc}, // [2] JAVANESE VOWEL SIGN TARUNG..JAVANESE VOWEL SIGN TOLONG
- {0xA9B6, 0xA9B9, prCM, gcMn}, // [4] JAVANESE VOWEL SIGN WULU..JAVANESE VOWEL SIGN SUKU MENDUT
- {0xA9BA, 0xA9BB, prCM, gcMc}, // [2] JAVANESE VOWEL SIGN TALING..JAVANESE VOWEL SIGN DIRGA MURE
- {0xA9BC, 0xA9BD, prCM, gcMn}, // [2] JAVANESE VOWEL SIGN PEPET..JAVANESE CONSONANT SIGN KERET
- {0xA9BE, 0xA9C0, prCM, gcMc}, // [3] JAVANESE CONSONANT SIGN PENGKAL..JAVANESE PANGKON
- {0xA9C1, 0xA9C6, prAL, gcPo}, // [6] JAVANESE LEFT RERENGGAN..JAVANESE PADA WINDU
- {0xA9C7, 0xA9C9, prBA, gcPo}, // [3] JAVANESE PADA PANGKAT..JAVANESE PADA LUNGSI
- {0xA9CA, 0xA9CD, prAL, gcPo}, // [4] JAVANESE PADA ADEG..JAVANESE TURNED PADA PISELEH
- {0xA9CF, 0xA9CF, prAL, gcLm}, // JAVANESE PANGRANGKEP
- {0xA9D0, 0xA9D9, prNU, gcNd}, // [10] JAVANESE DIGIT ZERO..JAVANESE DIGIT NINE
- {0xA9DE, 0xA9DF, prAL, gcPo}, // [2] JAVANESE PADA TIRTA TUMETES..JAVANESE PADA ISEN-ISEN
- {0xA9E0, 0xA9E4, prSA, gcLo}, // [5] MYANMAR LETTER SHAN GHA..MYANMAR LETTER SHAN BHA
- {0xA9E5, 0xA9E5, prSA, gcMn}, // MYANMAR SIGN SHAN SAW
- {0xA9E6, 0xA9E6, prSA, gcLm}, // MYANMAR MODIFIER LETTER SHAN REDUPLICATION
- {0xA9E7, 0xA9EF, prSA, gcLo}, // [9] MYANMAR LETTER TAI LAING NYA..MYANMAR LETTER TAI LAING NNA
- {0xA9F0, 0xA9F9, prNU, gcNd}, // [10] MYANMAR TAI LAING DIGIT ZERO..MYANMAR TAI LAING DIGIT NINE
- {0xA9FA, 0xA9FE, prSA, gcLo}, // [5] MYANMAR LETTER TAI LAING LLA..MYANMAR LETTER TAI LAING BHA
- {0xAA00, 0xAA28, prAL, gcLo}, // [41] CHAM LETTER A..CHAM LETTER HA
- {0xAA29, 0xAA2E, prCM, gcMn}, // [6] CHAM VOWEL SIGN AA..CHAM VOWEL SIGN OE
- {0xAA2F, 0xAA30, prCM, gcMc}, // [2] CHAM VOWEL SIGN O..CHAM VOWEL SIGN AI
- {0xAA31, 0xAA32, prCM, gcMn}, // [2] CHAM VOWEL SIGN AU..CHAM VOWEL SIGN UE
- {0xAA33, 0xAA34, prCM, gcMc}, // [2] CHAM CONSONANT SIGN YA..CHAM CONSONANT SIGN RA
- {0xAA35, 0xAA36, prCM, gcMn}, // [2] CHAM CONSONANT SIGN LA..CHAM CONSONANT SIGN WA
- {0xAA40, 0xAA42, prAL, gcLo}, // [3] CHAM LETTER FINAL K..CHAM LETTER FINAL NG
- {0xAA43, 0xAA43, prCM, gcMn}, // CHAM CONSONANT SIGN FINAL NG
- {0xAA44, 0xAA4B, prAL, gcLo}, // [8] CHAM LETTER FINAL CH..CHAM LETTER FINAL SS
- {0xAA4C, 0xAA4C, prCM, gcMn}, // CHAM CONSONANT SIGN FINAL M
- {0xAA4D, 0xAA4D, prCM, gcMc}, // CHAM CONSONANT SIGN FINAL H
- {0xAA50, 0xAA59, prNU, gcNd}, // [10] CHAM DIGIT ZERO..CHAM DIGIT NINE
- {0xAA5C, 0xAA5C, prAL, gcPo}, // CHAM PUNCTUATION SPIRAL
- {0xAA5D, 0xAA5F, prBA, gcPo}, // [3] CHAM PUNCTUATION DANDA..CHAM PUNCTUATION TRIPLE DANDA
- {0xAA60, 0xAA6F, prSA, gcLo}, // [16] MYANMAR LETTER KHAMTI GA..MYANMAR LETTER KHAMTI FA
- {0xAA70, 0xAA70, prSA, gcLm}, // MYANMAR MODIFIER LETTER KHAMTI REDUPLICATION
- {0xAA71, 0xAA76, prSA, gcLo}, // [6] MYANMAR LETTER KHAMTI XA..MYANMAR LOGOGRAM KHAMTI HM
- {0xAA77, 0xAA79, prSA, gcSo}, // [3] MYANMAR SYMBOL AITON EXCLAMATION..MYANMAR SYMBOL AITON TWO
- {0xAA7A, 0xAA7A, prSA, gcLo}, // MYANMAR LETTER AITON RA
- {0xAA7B, 0xAA7B, prSA, gcMc}, // MYANMAR SIGN PAO KAREN TONE
- {0xAA7C, 0xAA7C, prSA, gcMn}, // MYANMAR SIGN TAI LAING TONE-2
- {0xAA7D, 0xAA7D, prSA, gcMc}, // MYANMAR SIGN TAI LAING TONE-5
- {0xAA7E, 0xAA7F, prSA, gcLo}, // [2] MYANMAR LETTER SHWE PALAUNG CHA..MYANMAR LETTER SHWE PALAUNG SHA
- {0xAA80, 0xAAAF, prSA, gcLo}, // [48] TAI VIET LETTER LOW KO..TAI VIET LETTER HIGH O
- {0xAAB0, 0xAAB0, prSA, gcMn}, // TAI VIET MAI KANG
- {0xAAB1, 0xAAB1, prSA, gcLo}, // TAI VIET VOWEL AA
- {0xAAB2, 0xAAB4, prSA, gcMn}, // [3] TAI VIET VOWEL I..TAI VIET VOWEL U
- {0xAAB5, 0xAAB6, prSA, gcLo}, // [2] TAI VIET VOWEL E..TAI VIET VOWEL O
- {0xAAB7, 0xAAB8, prSA, gcMn}, // [2] TAI VIET MAI KHIT..TAI VIET VOWEL IA
- {0xAAB9, 0xAABD, prSA, gcLo}, // [5] TAI VIET VOWEL UEA..TAI VIET VOWEL AN
- {0xAABE, 0xAABF, prSA, gcMn}, // [2] TAI VIET VOWEL AM..TAI VIET TONE MAI EK
- {0xAAC0, 0xAAC0, prSA, gcLo}, // TAI VIET TONE MAI NUENG
- {0xAAC1, 0xAAC1, prSA, gcMn}, // TAI VIET TONE MAI THO
- {0xAAC2, 0xAAC2, prSA, gcLo}, // TAI VIET TONE MAI SONG
- {0xAADB, 0xAADC, prSA, gcLo}, // [2] TAI VIET SYMBOL KON..TAI VIET SYMBOL NUENG
- {0xAADD, 0xAADD, prSA, gcLm}, // TAI VIET SYMBOL SAM
- {0xAADE, 0xAADF, prSA, gcPo}, // [2] TAI VIET SYMBOL HO HOI..TAI VIET SYMBOL KOI KOI
- {0xAAE0, 0xAAEA, prAL, gcLo}, // [11] MEETEI MAYEK LETTER E..MEETEI MAYEK LETTER SSA
- {0xAAEB, 0xAAEB, prCM, gcMc}, // MEETEI MAYEK VOWEL SIGN II
- {0xAAEC, 0xAAED, prCM, gcMn}, // [2] MEETEI MAYEK VOWEL SIGN UU..MEETEI MAYEK VOWEL SIGN AAI
- {0xAAEE, 0xAAEF, prCM, gcMc}, // [2] MEETEI MAYEK VOWEL SIGN AU..MEETEI MAYEK VOWEL SIGN AAU
- {0xAAF0, 0xAAF1, prBA, gcPo}, // [2] MEETEI MAYEK CHEIKHAN..MEETEI MAYEK AHANG KHUDAM
- {0xAAF2, 0xAAF2, prAL, gcLo}, // MEETEI MAYEK ANJI
- {0xAAF3, 0xAAF4, prAL, gcLm}, // [2] MEETEI MAYEK SYLLABLE REPETITION MARK..MEETEI MAYEK WORD REPETITION MARK
- {0xAAF5, 0xAAF5, prCM, gcMc}, // MEETEI MAYEK VOWEL SIGN VISARGA
- {0xAAF6, 0xAAF6, prCM, gcMn}, // MEETEI MAYEK VIRAMA
- {0xAB01, 0xAB06, prAL, gcLo}, // [6] ETHIOPIC SYLLABLE TTHU..ETHIOPIC SYLLABLE TTHO
- {0xAB09, 0xAB0E, prAL, gcLo}, // [6] ETHIOPIC SYLLABLE DDHU..ETHIOPIC SYLLABLE DDHO
- {0xAB11, 0xAB16, prAL, gcLo}, // [6] ETHIOPIC SYLLABLE DZU..ETHIOPIC SYLLABLE DZO
- {0xAB20, 0xAB26, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE CCHHA..ETHIOPIC SYLLABLE CCHHO
- {0xAB28, 0xAB2E, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE BBA..ETHIOPIC SYLLABLE BBO
- {0xAB30, 0xAB5A, prAL, gcLl}, // [43] LATIN SMALL LETTER BARRED ALPHA..LATIN SMALL LETTER Y WITH SHORT RIGHT LEG
- {0xAB5B, 0xAB5B, prAL, gcSk}, // MODIFIER BREVE WITH INVERTED BREVE
- {0xAB5C, 0xAB5F, prAL, gcLm}, // [4] MODIFIER LETTER SMALL HENG..MODIFIER LETTER SMALL U WITH LEFT HOOK
- {0xAB60, 0xAB68, prAL, gcLl}, // [9] LATIN SMALL LETTER SAKHA YAT..LATIN SMALL LETTER TURNED R WITH MIDDLE TILDE
- {0xAB69, 0xAB69, prAL, gcLm}, // MODIFIER LETTER SMALL TURNED W
- {0xAB6A, 0xAB6B, prAL, gcSk}, // [2] MODIFIER LETTER LEFT TACK..MODIFIER LETTER RIGHT TACK
- {0xAB70, 0xABBF, prAL, gcLl}, // [80] CHEROKEE SMALL LETTER A..CHEROKEE SMALL LETTER YA
- {0xABC0, 0xABE2, prAL, gcLo}, // [35] MEETEI MAYEK LETTER KOK..MEETEI MAYEK LETTER I LONSUM
- {0xABE3, 0xABE4, prCM, gcMc}, // [2] MEETEI MAYEK VOWEL SIGN ONAP..MEETEI MAYEK VOWEL SIGN INAP
- {0xABE5, 0xABE5, prCM, gcMn}, // MEETEI MAYEK VOWEL SIGN ANAP
- {0xABE6, 0xABE7, prCM, gcMc}, // [2] MEETEI MAYEK VOWEL SIGN YENAP..MEETEI MAYEK VOWEL SIGN SOUNAP
- {0xABE8, 0xABE8, prCM, gcMn}, // MEETEI MAYEK VOWEL SIGN UNAP
- {0xABE9, 0xABEA, prCM, gcMc}, // [2] MEETEI MAYEK VOWEL SIGN CHEINAP..MEETEI MAYEK VOWEL SIGN NUNG
- {0xABEB, 0xABEB, prBA, gcPo}, // MEETEI MAYEK CHEIKHEI
- {0xABEC, 0xABEC, prCM, gcMc}, // MEETEI MAYEK LUM IYEK
- {0xABED, 0xABED, prCM, gcMn}, // MEETEI MAYEK APUN IYEK
- {0xABF0, 0xABF9, prNU, gcNd}, // [10] MEETEI MAYEK DIGIT ZERO..MEETEI MAYEK DIGIT NINE
- {0xAC00, 0xAC00, prH2, gcLo}, // HANGUL SYLLABLE GA
- {0xAC01, 0xAC1B, prH3, gcLo}, // [27] HANGUL SYLLABLE GAG..HANGUL SYLLABLE GAH
- {0xAC1C, 0xAC1C, prH2, gcLo}, // HANGUL SYLLABLE GAE
- {0xAC1D, 0xAC37, prH3, gcLo}, // [27] HANGUL SYLLABLE GAEG..HANGUL SYLLABLE GAEH
- {0xAC38, 0xAC38, prH2, gcLo}, // HANGUL SYLLABLE GYA
- {0xAC39, 0xAC53, prH3, gcLo}, // [27] HANGUL SYLLABLE GYAG..HANGUL SYLLABLE GYAH
- {0xAC54, 0xAC54, prH2, gcLo}, // HANGUL SYLLABLE GYAE
- {0xAC55, 0xAC6F, prH3, gcLo}, // [27] HANGUL SYLLABLE GYAEG..HANGUL SYLLABLE GYAEH
- {0xAC70, 0xAC70, prH2, gcLo}, // HANGUL SYLLABLE GEO
- {0xAC71, 0xAC8B, prH3, gcLo}, // [27] HANGUL SYLLABLE GEOG..HANGUL SYLLABLE GEOH
- {0xAC8C, 0xAC8C, prH2, gcLo}, // HANGUL SYLLABLE GE
- {0xAC8D, 0xACA7, prH3, gcLo}, // [27] HANGUL SYLLABLE GEG..HANGUL SYLLABLE GEH
- {0xACA8, 0xACA8, prH2, gcLo}, // HANGUL SYLLABLE GYEO
- {0xACA9, 0xACC3, prH3, gcLo}, // [27] HANGUL SYLLABLE GYEOG..HANGUL SYLLABLE GYEOH
- {0xACC4, 0xACC4, prH2, gcLo}, // HANGUL SYLLABLE GYE
- {0xACC5, 0xACDF, prH3, gcLo}, // [27] HANGUL SYLLABLE GYEG..HANGUL SYLLABLE GYEH
- {0xACE0, 0xACE0, prH2, gcLo}, // HANGUL SYLLABLE GO
- {0xACE1, 0xACFB, prH3, gcLo}, // [27] HANGUL SYLLABLE GOG..HANGUL SYLLABLE GOH
- {0xACFC, 0xACFC, prH2, gcLo}, // HANGUL SYLLABLE GWA
- {0xACFD, 0xAD17, prH3, gcLo}, // [27] HANGUL SYLLABLE GWAG..HANGUL SYLLABLE GWAH
- {0xAD18, 0xAD18, prH2, gcLo}, // HANGUL SYLLABLE GWAE
- {0xAD19, 0xAD33, prH3, gcLo}, // [27] HANGUL SYLLABLE GWAEG..HANGUL SYLLABLE GWAEH
- {0xAD34, 0xAD34, prH2, gcLo}, // HANGUL SYLLABLE GOE
- {0xAD35, 0xAD4F, prH3, gcLo}, // [27] HANGUL SYLLABLE GOEG..HANGUL SYLLABLE GOEH
- {0xAD50, 0xAD50, prH2, gcLo}, // HANGUL SYLLABLE GYO
- {0xAD51, 0xAD6B, prH3, gcLo}, // [27] HANGUL SYLLABLE GYOG..HANGUL SYLLABLE GYOH
- {0xAD6C, 0xAD6C, prH2, gcLo}, // HANGUL SYLLABLE GU
- {0xAD6D, 0xAD87, prH3, gcLo}, // [27] HANGUL SYLLABLE GUG..HANGUL SYLLABLE GUH
- {0xAD88, 0xAD88, prH2, gcLo}, // HANGUL SYLLABLE GWEO
- {0xAD89, 0xADA3, prH3, gcLo}, // [27] HANGUL SYLLABLE GWEOG..HANGUL SYLLABLE GWEOH
- {0xADA4, 0xADA4, prH2, gcLo}, // HANGUL SYLLABLE GWE
- {0xADA5, 0xADBF, prH3, gcLo}, // [27] HANGUL SYLLABLE GWEG..HANGUL SYLLABLE GWEH
- {0xADC0, 0xADC0, prH2, gcLo}, // HANGUL SYLLABLE GWI
- {0xADC1, 0xADDB, prH3, gcLo}, // [27] HANGUL SYLLABLE GWIG..HANGUL SYLLABLE GWIH
- {0xADDC, 0xADDC, prH2, gcLo}, // HANGUL SYLLABLE GYU
- {0xADDD, 0xADF7, prH3, gcLo}, // [27] HANGUL SYLLABLE GYUG..HANGUL SYLLABLE GYUH
- {0xADF8, 0xADF8, prH2, gcLo}, // HANGUL SYLLABLE GEU
- {0xADF9, 0xAE13, prH3, gcLo}, // [27] HANGUL SYLLABLE GEUG..HANGUL SYLLABLE GEUH
- {0xAE14, 0xAE14, prH2, gcLo}, // HANGUL SYLLABLE GYI
- {0xAE15, 0xAE2F, prH3, gcLo}, // [27] HANGUL SYLLABLE GYIG..HANGUL SYLLABLE GYIH
- {0xAE30, 0xAE30, prH2, gcLo}, // HANGUL SYLLABLE GI
- {0xAE31, 0xAE4B, prH3, gcLo}, // [27] HANGUL SYLLABLE GIG..HANGUL SYLLABLE GIH
- {0xAE4C, 0xAE4C, prH2, gcLo}, // HANGUL SYLLABLE GGA
- {0xAE4D, 0xAE67, prH3, gcLo}, // [27] HANGUL SYLLABLE GGAG..HANGUL SYLLABLE GGAH
- {0xAE68, 0xAE68, prH2, gcLo}, // HANGUL SYLLABLE GGAE
- {0xAE69, 0xAE83, prH3, gcLo}, // [27] HANGUL SYLLABLE GGAEG..HANGUL SYLLABLE GGAEH
- {0xAE84, 0xAE84, prH2, gcLo}, // HANGUL SYLLABLE GGYA
- {0xAE85, 0xAE9F, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYAG..HANGUL SYLLABLE GGYAH
- {0xAEA0, 0xAEA0, prH2, gcLo}, // HANGUL SYLLABLE GGYAE
- {0xAEA1, 0xAEBB, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYAEG..HANGUL SYLLABLE GGYAEH
- {0xAEBC, 0xAEBC, prH2, gcLo}, // HANGUL SYLLABLE GGEO
- {0xAEBD, 0xAED7, prH3, gcLo}, // [27] HANGUL SYLLABLE GGEOG..HANGUL SYLLABLE GGEOH
- {0xAED8, 0xAED8, prH2, gcLo}, // HANGUL SYLLABLE GGE
- {0xAED9, 0xAEF3, prH3, gcLo}, // [27] HANGUL SYLLABLE GGEG..HANGUL SYLLABLE GGEH
- {0xAEF4, 0xAEF4, prH2, gcLo}, // HANGUL SYLLABLE GGYEO
- {0xAEF5, 0xAF0F, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYEOG..HANGUL SYLLABLE GGYEOH
- {0xAF10, 0xAF10, prH2, gcLo}, // HANGUL SYLLABLE GGYE
- {0xAF11, 0xAF2B, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYEG..HANGUL SYLLABLE GGYEH
- {0xAF2C, 0xAF2C, prH2, gcLo}, // HANGUL SYLLABLE GGO
- {0xAF2D, 0xAF47, prH3, gcLo}, // [27] HANGUL SYLLABLE GGOG..HANGUL SYLLABLE GGOH
- {0xAF48, 0xAF48, prH2, gcLo}, // HANGUL SYLLABLE GGWA
- {0xAF49, 0xAF63, prH3, gcLo}, // [27] HANGUL SYLLABLE GGWAG..HANGUL SYLLABLE GGWAH
- {0xAF64, 0xAF64, prH2, gcLo}, // HANGUL SYLLABLE GGWAE
- {0xAF65, 0xAF7F, prH3, gcLo}, // [27] HANGUL SYLLABLE GGWAEG..HANGUL SYLLABLE GGWAEH
- {0xAF80, 0xAF80, prH2, gcLo}, // HANGUL SYLLABLE GGOE
- {0xAF81, 0xAF9B, prH3, gcLo}, // [27] HANGUL SYLLABLE GGOEG..HANGUL SYLLABLE GGOEH
- {0xAF9C, 0xAF9C, prH2, gcLo}, // HANGUL SYLLABLE GGYO
- {0xAF9D, 0xAFB7, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYOG..HANGUL SYLLABLE GGYOH
- {0xAFB8, 0xAFB8, prH2, gcLo}, // HANGUL SYLLABLE GGU
- {0xAFB9, 0xAFD3, prH3, gcLo}, // [27] HANGUL SYLLABLE GGUG..HANGUL SYLLABLE GGUH
- {0xAFD4, 0xAFD4, prH2, gcLo}, // HANGUL SYLLABLE GGWEO
- {0xAFD5, 0xAFEF, prH3, gcLo}, // [27] HANGUL SYLLABLE GGWEOG..HANGUL SYLLABLE GGWEOH
- {0xAFF0, 0xAFF0, prH2, gcLo}, // HANGUL SYLLABLE GGWE
- {0xAFF1, 0xB00B, prH3, gcLo}, // [27] HANGUL SYLLABLE GGWEG..HANGUL SYLLABLE GGWEH
- {0xB00C, 0xB00C, prH2, gcLo}, // HANGUL SYLLABLE GGWI
- {0xB00D, 0xB027, prH3, gcLo}, // [27] HANGUL SYLLABLE GGWIG..HANGUL SYLLABLE GGWIH
- {0xB028, 0xB028, prH2, gcLo}, // HANGUL SYLLABLE GGYU
- {0xB029, 0xB043, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYUG..HANGUL SYLLABLE GGYUH
- {0xB044, 0xB044, prH2, gcLo}, // HANGUL SYLLABLE GGEU
- {0xB045, 0xB05F, prH3, gcLo}, // [27] HANGUL SYLLABLE GGEUG..HANGUL SYLLABLE GGEUH
- {0xB060, 0xB060, prH2, gcLo}, // HANGUL SYLLABLE GGYI
- {0xB061, 0xB07B, prH3, gcLo}, // [27] HANGUL SYLLABLE GGYIG..HANGUL SYLLABLE GGYIH
- {0xB07C, 0xB07C, prH2, gcLo}, // HANGUL SYLLABLE GGI
- {0xB07D, 0xB097, prH3, gcLo}, // [27] HANGUL SYLLABLE GGIG..HANGUL SYLLABLE GGIH
- {0xB098, 0xB098, prH2, gcLo}, // HANGUL SYLLABLE NA
- {0xB099, 0xB0B3, prH3, gcLo}, // [27] HANGUL SYLLABLE NAG..HANGUL SYLLABLE NAH
- {0xB0B4, 0xB0B4, prH2, gcLo}, // HANGUL SYLLABLE NAE
- {0xB0B5, 0xB0CF, prH3, gcLo}, // [27] HANGUL SYLLABLE NAEG..HANGUL SYLLABLE NAEH
- {0xB0D0, 0xB0D0, prH2, gcLo}, // HANGUL SYLLABLE NYA
- {0xB0D1, 0xB0EB, prH3, gcLo}, // [27] HANGUL SYLLABLE NYAG..HANGUL SYLLABLE NYAH
- {0xB0EC, 0xB0EC, prH2, gcLo}, // HANGUL SYLLABLE NYAE
- {0xB0ED, 0xB107, prH3, gcLo}, // [27] HANGUL SYLLABLE NYAEG..HANGUL SYLLABLE NYAEH
- {0xB108, 0xB108, prH2, gcLo}, // HANGUL SYLLABLE NEO
- {0xB109, 0xB123, prH3, gcLo}, // [27] HANGUL SYLLABLE NEOG..HANGUL SYLLABLE NEOH
- {0xB124, 0xB124, prH2, gcLo}, // HANGUL SYLLABLE NE
- {0xB125, 0xB13F, prH3, gcLo}, // [27] HANGUL SYLLABLE NEG..HANGUL SYLLABLE NEH
- {0xB140, 0xB140, prH2, gcLo}, // HANGUL SYLLABLE NYEO
- {0xB141, 0xB15B, prH3, gcLo}, // [27] HANGUL SYLLABLE NYEOG..HANGUL SYLLABLE NYEOH
- {0xB15C, 0xB15C, prH2, gcLo}, // HANGUL SYLLABLE NYE
- {0xB15D, 0xB177, prH3, gcLo}, // [27] HANGUL SYLLABLE NYEG..HANGUL SYLLABLE NYEH
- {0xB178, 0xB178, prH2, gcLo}, // HANGUL SYLLABLE NO
- {0xB179, 0xB193, prH3, gcLo}, // [27] HANGUL SYLLABLE NOG..HANGUL SYLLABLE NOH
- {0xB194, 0xB194, prH2, gcLo}, // HANGUL SYLLABLE NWA
- {0xB195, 0xB1AF, prH3, gcLo}, // [27] HANGUL SYLLABLE NWAG..HANGUL SYLLABLE NWAH
- {0xB1B0, 0xB1B0, prH2, gcLo}, // HANGUL SYLLABLE NWAE
- {0xB1B1, 0xB1CB, prH3, gcLo}, // [27] HANGUL SYLLABLE NWAEG..HANGUL SYLLABLE NWAEH
- {0xB1CC, 0xB1CC, prH2, gcLo}, // HANGUL SYLLABLE NOE
- {0xB1CD, 0xB1E7, prH3, gcLo}, // [27] HANGUL SYLLABLE NOEG..HANGUL SYLLABLE NOEH
- {0xB1E8, 0xB1E8, prH2, gcLo}, // HANGUL SYLLABLE NYO
- {0xB1E9, 0xB203, prH3, gcLo}, // [27] HANGUL SYLLABLE NYOG..HANGUL SYLLABLE NYOH
- {0xB204, 0xB204, prH2, gcLo}, // HANGUL SYLLABLE NU
- {0xB205, 0xB21F, prH3, gcLo}, // [27] HANGUL SYLLABLE NUG..HANGUL SYLLABLE NUH
- {0xB220, 0xB220, prH2, gcLo}, // HANGUL SYLLABLE NWEO
- {0xB221, 0xB23B, prH3, gcLo}, // [27] HANGUL SYLLABLE NWEOG..HANGUL SYLLABLE NWEOH
- {0xB23C, 0xB23C, prH2, gcLo}, // HANGUL SYLLABLE NWE
- {0xB23D, 0xB257, prH3, gcLo}, // [27] HANGUL SYLLABLE NWEG..HANGUL SYLLABLE NWEH
- {0xB258, 0xB258, prH2, gcLo}, // HANGUL SYLLABLE NWI
- {0xB259, 0xB273, prH3, gcLo}, // [27] HANGUL SYLLABLE NWIG..HANGUL SYLLABLE NWIH
- {0xB274, 0xB274, prH2, gcLo}, // HANGUL SYLLABLE NYU
- {0xB275, 0xB28F, prH3, gcLo}, // [27] HANGUL SYLLABLE NYUG..HANGUL SYLLABLE NYUH
- {0xB290, 0xB290, prH2, gcLo}, // HANGUL SYLLABLE NEU
- {0xB291, 0xB2AB, prH3, gcLo}, // [27] HANGUL SYLLABLE NEUG..HANGUL SYLLABLE NEUH
- {0xB2AC, 0xB2AC, prH2, gcLo}, // HANGUL SYLLABLE NYI
- {0xB2AD, 0xB2C7, prH3, gcLo}, // [27] HANGUL SYLLABLE NYIG..HANGUL SYLLABLE NYIH
- {0xB2C8, 0xB2C8, prH2, gcLo}, // HANGUL SYLLABLE NI
- {0xB2C9, 0xB2E3, prH3, gcLo}, // [27] HANGUL SYLLABLE NIG..HANGUL SYLLABLE NIH
- {0xB2E4, 0xB2E4, prH2, gcLo}, // HANGUL SYLLABLE DA
- {0xB2E5, 0xB2FF, prH3, gcLo}, // [27] HANGUL SYLLABLE DAG..HANGUL SYLLABLE DAH
- {0xB300, 0xB300, prH2, gcLo}, // HANGUL SYLLABLE DAE
- {0xB301, 0xB31B, prH3, gcLo}, // [27] HANGUL SYLLABLE DAEG..HANGUL SYLLABLE DAEH
- {0xB31C, 0xB31C, prH2, gcLo}, // HANGUL SYLLABLE DYA
- {0xB31D, 0xB337, prH3, gcLo}, // [27] HANGUL SYLLABLE DYAG..HANGUL SYLLABLE DYAH
- {0xB338, 0xB338, prH2, gcLo}, // HANGUL SYLLABLE DYAE
- {0xB339, 0xB353, prH3, gcLo}, // [27] HANGUL SYLLABLE DYAEG..HANGUL SYLLABLE DYAEH
- {0xB354, 0xB354, prH2, gcLo}, // HANGUL SYLLABLE DEO
- {0xB355, 0xB36F, prH3, gcLo}, // [27] HANGUL SYLLABLE DEOG..HANGUL SYLLABLE DEOH
- {0xB370, 0xB370, prH2, gcLo}, // HANGUL SYLLABLE DE
- {0xB371, 0xB38B, prH3, gcLo}, // [27] HANGUL SYLLABLE DEG..HANGUL SYLLABLE DEH
- {0xB38C, 0xB38C, prH2, gcLo}, // HANGUL SYLLABLE DYEO
- {0xB38D, 0xB3A7, prH3, gcLo}, // [27] HANGUL SYLLABLE DYEOG..HANGUL SYLLABLE DYEOH
- {0xB3A8, 0xB3A8, prH2, gcLo}, // HANGUL SYLLABLE DYE
- {0xB3A9, 0xB3C3, prH3, gcLo}, // [27] HANGUL SYLLABLE DYEG..HANGUL SYLLABLE DYEH
- {0xB3C4, 0xB3C4, prH2, gcLo}, // HANGUL SYLLABLE DO
- {0xB3C5, 0xB3DF, prH3, gcLo}, // [27] HANGUL SYLLABLE DOG..HANGUL SYLLABLE DOH
- {0xB3E0, 0xB3E0, prH2, gcLo}, // HANGUL SYLLABLE DWA
- {0xB3E1, 0xB3FB, prH3, gcLo}, // [27] HANGUL SYLLABLE DWAG..HANGUL SYLLABLE DWAH
- {0xB3FC, 0xB3FC, prH2, gcLo}, // HANGUL SYLLABLE DWAE
- {0xB3FD, 0xB417, prH3, gcLo}, // [27] HANGUL SYLLABLE DWAEG..HANGUL SYLLABLE DWAEH
- {0xB418, 0xB418, prH2, gcLo}, // HANGUL SYLLABLE DOE
- {0xB419, 0xB433, prH3, gcLo}, // [27] HANGUL SYLLABLE DOEG..HANGUL SYLLABLE DOEH
- {0xB434, 0xB434, prH2, gcLo}, // HANGUL SYLLABLE DYO
- {0xB435, 0xB44F, prH3, gcLo}, // [27] HANGUL SYLLABLE DYOG..HANGUL SYLLABLE DYOH
- {0xB450, 0xB450, prH2, gcLo}, // HANGUL SYLLABLE DU
- {0xB451, 0xB46B, prH3, gcLo}, // [27] HANGUL SYLLABLE DUG..HANGUL SYLLABLE DUH
- {0xB46C, 0xB46C, prH2, gcLo}, // HANGUL SYLLABLE DWEO
- {0xB46D, 0xB487, prH3, gcLo}, // [27] HANGUL SYLLABLE DWEOG..HANGUL SYLLABLE DWEOH
- {0xB488, 0xB488, prH2, gcLo}, // HANGUL SYLLABLE DWE
- {0xB489, 0xB4A3, prH3, gcLo}, // [27] HANGUL SYLLABLE DWEG..HANGUL SYLLABLE DWEH
- {0xB4A4, 0xB4A4, prH2, gcLo}, // HANGUL SYLLABLE DWI
- {0xB4A5, 0xB4BF, prH3, gcLo}, // [27] HANGUL SYLLABLE DWIG..HANGUL SYLLABLE DWIH
- {0xB4C0, 0xB4C0, prH2, gcLo}, // HANGUL SYLLABLE DYU
- {0xB4C1, 0xB4DB, prH3, gcLo}, // [27] HANGUL SYLLABLE DYUG..HANGUL SYLLABLE DYUH
- {0xB4DC, 0xB4DC, prH2, gcLo}, // HANGUL SYLLABLE DEU
- {0xB4DD, 0xB4F7, prH3, gcLo}, // [27] HANGUL SYLLABLE DEUG..HANGUL SYLLABLE DEUH
- {0xB4F8, 0xB4F8, prH2, gcLo}, // HANGUL SYLLABLE DYI
- {0xB4F9, 0xB513, prH3, gcLo}, // [27] HANGUL SYLLABLE DYIG..HANGUL SYLLABLE DYIH
- {0xB514, 0xB514, prH2, gcLo}, // HANGUL SYLLABLE DI
- {0xB515, 0xB52F, prH3, gcLo}, // [27] HANGUL SYLLABLE DIG..HANGUL SYLLABLE DIH
- {0xB530, 0xB530, prH2, gcLo}, // HANGUL SYLLABLE DDA
- {0xB531, 0xB54B, prH3, gcLo}, // [27] HANGUL SYLLABLE DDAG..HANGUL SYLLABLE DDAH
- {0xB54C, 0xB54C, prH2, gcLo}, // HANGUL SYLLABLE DDAE
- {0xB54D, 0xB567, prH3, gcLo}, // [27] HANGUL SYLLABLE DDAEG..HANGUL SYLLABLE DDAEH
- {0xB568, 0xB568, prH2, gcLo}, // HANGUL SYLLABLE DDYA
- {0xB569, 0xB583, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYAG..HANGUL SYLLABLE DDYAH
- {0xB584, 0xB584, prH2, gcLo}, // HANGUL SYLLABLE DDYAE
- {0xB585, 0xB59F, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYAEG..HANGUL SYLLABLE DDYAEH
- {0xB5A0, 0xB5A0, prH2, gcLo}, // HANGUL SYLLABLE DDEO
- {0xB5A1, 0xB5BB, prH3, gcLo}, // [27] HANGUL SYLLABLE DDEOG..HANGUL SYLLABLE DDEOH
- {0xB5BC, 0xB5BC, prH2, gcLo}, // HANGUL SYLLABLE DDE
- {0xB5BD, 0xB5D7, prH3, gcLo}, // [27] HANGUL SYLLABLE DDEG..HANGUL SYLLABLE DDEH
- {0xB5D8, 0xB5D8, prH2, gcLo}, // HANGUL SYLLABLE DDYEO
- {0xB5D9, 0xB5F3, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYEOG..HANGUL SYLLABLE DDYEOH
- {0xB5F4, 0xB5F4, prH2, gcLo}, // HANGUL SYLLABLE DDYE
- {0xB5F5, 0xB60F, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYEG..HANGUL SYLLABLE DDYEH
- {0xB610, 0xB610, prH2, gcLo}, // HANGUL SYLLABLE DDO
- {0xB611, 0xB62B, prH3, gcLo}, // [27] HANGUL SYLLABLE DDOG..HANGUL SYLLABLE DDOH
- {0xB62C, 0xB62C, prH2, gcLo}, // HANGUL SYLLABLE DDWA
- {0xB62D, 0xB647, prH3, gcLo}, // [27] HANGUL SYLLABLE DDWAG..HANGUL SYLLABLE DDWAH
- {0xB648, 0xB648, prH2, gcLo}, // HANGUL SYLLABLE DDWAE
- {0xB649, 0xB663, prH3, gcLo}, // [27] HANGUL SYLLABLE DDWAEG..HANGUL SYLLABLE DDWAEH
- {0xB664, 0xB664, prH2, gcLo}, // HANGUL SYLLABLE DDOE
- {0xB665, 0xB67F, prH3, gcLo}, // [27] HANGUL SYLLABLE DDOEG..HANGUL SYLLABLE DDOEH
- {0xB680, 0xB680, prH2, gcLo}, // HANGUL SYLLABLE DDYO
- {0xB681, 0xB69B, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYOG..HANGUL SYLLABLE DDYOH
- {0xB69C, 0xB69C, prH2, gcLo}, // HANGUL SYLLABLE DDU
- {0xB69D, 0xB6B7, prH3, gcLo}, // [27] HANGUL SYLLABLE DDUG..HANGUL SYLLABLE DDUH
- {0xB6B8, 0xB6B8, prH2, gcLo}, // HANGUL SYLLABLE DDWEO
- {0xB6B9, 0xB6D3, prH3, gcLo}, // [27] HANGUL SYLLABLE DDWEOG..HANGUL SYLLABLE DDWEOH
- {0xB6D4, 0xB6D4, prH2, gcLo}, // HANGUL SYLLABLE DDWE
- {0xB6D5, 0xB6EF, prH3, gcLo}, // [27] HANGUL SYLLABLE DDWEG..HANGUL SYLLABLE DDWEH
- {0xB6F0, 0xB6F0, prH2, gcLo}, // HANGUL SYLLABLE DDWI
- {0xB6F1, 0xB70B, prH3, gcLo}, // [27] HANGUL SYLLABLE DDWIG..HANGUL SYLLABLE DDWIH
- {0xB70C, 0xB70C, prH2, gcLo}, // HANGUL SYLLABLE DDYU
- {0xB70D, 0xB727, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYUG..HANGUL SYLLABLE DDYUH
- {0xB728, 0xB728, prH2, gcLo}, // HANGUL SYLLABLE DDEU
- {0xB729, 0xB743, prH3, gcLo}, // [27] HANGUL SYLLABLE DDEUG..HANGUL SYLLABLE DDEUH
- {0xB744, 0xB744, prH2, gcLo}, // HANGUL SYLLABLE DDYI
- {0xB745, 0xB75F, prH3, gcLo}, // [27] HANGUL SYLLABLE DDYIG..HANGUL SYLLABLE DDYIH
- {0xB760, 0xB760, prH2, gcLo}, // HANGUL SYLLABLE DDI
- {0xB761, 0xB77B, prH3, gcLo}, // [27] HANGUL SYLLABLE DDIG..HANGUL SYLLABLE DDIH
- {0xB77C, 0xB77C, prH2, gcLo}, // HANGUL SYLLABLE RA
- {0xB77D, 0xB797, prH3, gcLo}, // [27] HANGUL SYLLABLE RAG..HANGUL SYLLABLE RAH
- {0xB798, 0xB798, prH2, gcLo}, // HANGUL SYLLABLE RAE
- {0xB799, 0xB7B3, prH3, gcLo}, // [27] HANGUL SYLLABLE RAEG..HANGUL SYLLABLE RAEH
- {0xB7B4, 0xB7B4, prH2, gcLo}, // HANGUL SYLLABLE RYA
- {0xB7B5, 0xB7CF, prH3, gcLo}, // [27] HANGUL SYLLABLE RYAG..HANGUL SYLLABLE RYAH
- {0xB7D0, 0xB7D0, prH2, gcLo}, // HANGUL SYLLABLE RYAE
- {0xB7D1, 0xB7EB, prH3, gcLo}, // [27] HANGUL SYLLABLE RYAEG..HANGUL SYLLABLE RYAEH
- {0xB7EC, 0xB7EC, prH2, gcLo}, // HANGUL SYLLABLE REO
- {0xB7ED, 0xB807, prH3, gcLo}, // [27] HANGUL SYLLABLE REOG..HANGUL SYLLABLE REOH
- {0xB808, 0xB808, prH2, gcLo}, // HANGUL SYLLABLE RE
- {0xB809, 0xB823, prH3, gcLo}, // [27] HANGUL SYLLABLE REG..HANGUL SYLLABLE REH
- {0xB824, 0xB824, prH2, gcLo}, // HANGUL SYLLABLE RYEO
- {0xB825, 0xB83F, prH3, gcLo}, // [27] HANGUL SYLLABLE RYEOG..HANGUL SYLLABLE RYEOH
- {0xB840, 0xB840, prH2, gcLo}, // HANGUL SYLLABLE RYE
- {0xB841, 0xB85B, prH3, gcLo}, // [27] HANGUL SYLLABLE RYEG..HANGUL SYLLABLE RYEH
- {0xB85C, 0xB85C, prH2, gcLo}, // HANGUL SYLLABLE RO
- {0xB85D, 0xB877, prH3, gcLo}, // [27] HANGUL SYLLABLE ROG..HANGUL SYLLABLE ROH
- {0xB878, 0xB878, prH2, gcLo}, // HANGUL SYLLABLE RWA
- {0xB879, 0xB893, prH3, gcLo}, // [27] HANGUL SYLLABLE RWAG..HANGUL SYLLABLE RWAH
- {0xB894, 0xB894, prH2, gcLo}, // HANGUL SYLLABLE RWAE
- {0xB895, 0xB8AF, prH3, gcLo}, // [27] HANGUL SYLLABLE RWAEG..HANGUL SYLLABLE RWAEH
- {0xB8B0, 0xB8B0, prH2, gcLo}, // HANGUL SYLLABLE ROE
- {0xB8B1, 0xB8CB, prH3, gcLo}, // [27] HANGUL SYLLABLE ROEG..HANGUL SYLLABLE ROEH
- {0xB8CC, 0xB8CC, prH2, gcLo}, // HANGUL SYLLABLE RYO
- {0xB8CD, 0xB8E7, prH3, gcLo}, // [27] HANGUL SYLLABLE RYOG..HANGUL SYLLABLE RYOH
- {0xB8E8, 0xB8E8, prH2, gcLo}, // HANGUL SYLLABLE RU
- {0xB8E9, 0xB903, prH3, gcLo}, // [27] HANGUL SYLLABLE RUG..HANGUL SYLLABLE RUH
- {0xB904, 0xB904, prH2, gcLo}, // HANGUL SYLLABLE RWEO
- {0xB905, 0xB91F, prH3, gcLo}, // [27] HANGUL SYLLABLE RWEOG..HANGUL SYLLABLE RWEOH
- {0xB920, 0xB920, prH2, gcLo}, // HANGUL SYLLABLE RWE
- {0xB921, 0xB93B, prH3, gcLo}, // [27] HANGUL SYLLABLE RWEG..HANGUL SYLLABLE RWEH
- {0xB93C, 0xB93C, prH2, gcLo}, // HANGUL SYLLABLE RWI
- {0xB93D, 0xB957, prH3, gcLo}, // [27] HANGUL SYLLABLE RWIG..HANGUL SYLLABLE RWIH
- {0xB958, 0xB958, prH2, gcLo}, // HANGUL SYLLABLE RYU
- {0xB959, 0xB973, prH3, gcLo}, // [27] HANGUL SYLLABLE RYUG..HANGUL SYLLABLE RYUH
- {0xB974, 0xB974, prH2, gcLo}, // HANGUL SYLLABLE REU
- {0xB975, 0xB98F, prH3, gcLo}, // [27] HANGUL SYLLABLE REUG..HANGUL SYLLABLE REUH
- {0xB990, 0xB990, prH2, gcLo}, // HANGUL SYLLABLE RYI
- {0xB991, 0xB9AB, prH3, gcLo}, // [27] HANGUL SYLLABLE RYIG..HANGUL SYLLABLE RYIH
- {0xB9AC, 0xB9AC, prH2, gcLo}, // HANGUL SYLLABLE RI
- {0xB9AD, 0xB9C7, prH3, gcLo}, // [27] HANGUL SYLLABLE RIG..HANGUL SYLLABLE RIH
- {0xB9C8, 0xB9C8, prH2, gcLo}, // HANGUL SYLLABLE MA
- {0xB9C9, 0xB9E3, prH3, gcLo}, // [27] HANGUL SYLLABLE MAG..HANGUL SYLLABLE MAH
- {0xB9E4, 0xB9E4, prH2, gcLo}, // HANGUL SYLLABLE MAE
- {0xB9E5, 0xB9FF, prH3, gcLo}, // [27] HANGUL SYLLABLE MAEG..HANGUL SYLLABLE MAEH
- {0xBA00, 0xBA00, prH2, gcLo}, // HANGUL SYLLABLE MYA
- {0xBA01, 0xBA1B, prH3, gcLo}, // [27] HANGUL SYLLABLE MYAG..HANGUL SYLLABLE MYAH
- {0xBA1C, 0xBA1C, prH2, gcLo}, // HANGUL SYLLABLE MYAE
- {0xBA1D, 0xBA37, prH3, gcLo}, // [27] HANGUL SYLLABLE MYAEG..HANGUL SYLLABLE MYAEH
- {0xBA38, 0xBA38, prH2, gcLo}, // HANGUL SYLLABLE MEO
- {0xBA39, 0xBA53, prH3, gcLo}, // [27] HANGUL SYLLABLE MEOG..HANGUL SYLLABLE MEOH
- {0xBA54, 0xBA54, prH2, gcLo}, // HANGUL SYLLABLE ME
- {0xBA55, 0xBA6F, prH3, gcLo}, // [27] HANGUL SYLLABLE MEG..HANGUL SYLLABLE MEH
- {0xBA70, 0xBA70, prH2, gcLo}, // HANGUL SYLLABLE MYEO
- {0xBA71, 0xBA8B, prH3, gcLo}, // [27] HANGUL SYLLABLE MYEOG..HANGUL SYLLABLE MYEOH
- {0xBA8C, 0xBA8C, prH2, gcLo}, // HANGUL SYLLABLE MYE
- {0xBA8D, 0xBAA7, prH3, gcLo}, // [27] HANGUL SYLLABLE MYEG..HANGUL SYLLABLE MYEH
- {0xBAA8, 0xBAA8, prH2, gcLo}, // HANGUL SYLLABLE MO
- {0xBAA9, 0xBAC3, prH3, gcLo}, // [27] HANGUL SYLLABLE MOG..HANGUL SYLLABLE MOH
- {0xBAC4, 0xBAC4, prH2, gcLo}, // HANGUL SYLLABLE MWA
- {0xBAC5, 0xBADF, prH3, gcLo}, // [27] HANGUL SYLLABLE MWAG..HANGUL SYLLABLE MWAH
- {0xBAE0, 0xBAE0, prH2, gcLo}, // HANGUL SYLLABLE MWAE
- {0xBAE1, 0xBAFB, prH3, gcLo}, // [27] HANGUL SYLLABLE MWAEG..HANGUL SYLLABLE MWAEH
- {0xBAFC, 0xBAFC, prH2, gcLo}, // HANGUL SYLLABLE MOE
- {0xBAFD, 0xBB17, prH3, gcLo}, // [27] HANGUL SYLLABLE MOEG..HANGUL SYLLABLE MOEH
- {0xBB18, 0xBB18, prH2, gcLo}, // HANGUL SYLLABLE MYO
- {0xBB19, 0xBB33, prH3, gcLo}, // [27] HANGUL SYLLABLE MYOG..HANGUL SYLLABLE MYOH
- {0xBB34, 0xBB34, prH2, gcLo}, // HANGUL SYLLABLE MU
- {0xBB35, 0xBB4F, prH3, gcLo}, // [27] HANGUL SYLLABLE MUG..HANGUL SYLLABLE MUH
- {0xBB50, 0xBB50, prH2, gcLo}, // HANGUL SYLLABLE MWEO
- {0xBB51, 0xBB6B, prH3, gcLo}, // [27] HANGUL SYLLABLE MWEOG..HANGUL SYLLABLE MWEOH
- {0xBB6C, 0xBB6C, prH2, gcLo}, // HANGUL SYLLABLE MWE
- {0xBB6D, 0xBB87, prH3, gcLo}, // [27] HANGUL SYLLABLE MWEG..HANGUL SYLLABLE MWEH
- {0xBB88, 0xBB88, prH2, gcLo}, // HANGUL SYLLABLE MWI
- {0xBB89, 0xBBA3, prH3, gcLo}, // [27] HANGUL SYLLABLE MWIG..HANGUL SYLLABLE MWIH
- {0xBBA4, 0xBBA4, prH2, gcLo}, // HANGUL SYLLABLE MYU
- {0xBBA5, 0xBBBF, prH3, gcLo}, // [27] HANGUL SYLLABLE MYUG..HANGUL SYLLABLE MYUH
- {0xBBC0, 0xBBC0, prH2, gcLo}, // HANGUL SYLLABLE MEU
- {0xBBC1, 0xBBDB, prH3, gcLo}, // [27] HANGUL SYLLABLE MEUG..HANGUL SYLLABLE MEUH
- {0xBBDC, 0xBBDC, prH2, gcLo}, // HANGUL SYLLABLE MYI
- {0xBBDD, 0xBBF7, prH3, gcLo}, // [27] HANGUL SYLLABLE MYIG..HANGUL SYLLABLE MYIH
- {0xBBF8, 0xBBF8, prH2, gcLo}, // HANGUL SYLLABLE MI
- {0xBBF9, 0xBC13, prH3, gcLo}, // [27] HANGUL SYLLABLE MIG..HANGUL SYLLABLE MIH
- {0xBC14, 0xBC14, prH2, gcLo}, // HANGUL SYLLABLE BA
- {0xBC15, 0xBC2F, prH3, gcLo}, // [27] HANGUL SYLLABLE BAG..HANGUL SYLLABLE BAH
- {0xBC30, 0xBC30, prH2, gcLo}, // HANGUL SYLLABLE BAE
- {0xBC31, 0xBC4B, prH3, gcLo}, // [27] HANGUL SYLLABLE BAEG..HANGUL SYLLABLE BAEH
- {0xBC4C, 0xBC4C, prH2, gcLo}, // HANGUL SYLLABLE BYA
- {0xBC4D, 0xBC67, prH3, gcLo}, // [27] HANGUL SYLLABLE BYAG..HANGUL SYLLABLE BYAH
- {0xBC68, 0xBC68, prH2, gcLo}, // HANGUL SYLLABLE BYAE
- {0xBC69, 0xBC83, prH3, gcLo}, // [27] HANGUL SYLLABLE BYAEG..HANGUL SYLLABLE BYAEH
- {0xBC84, 0xBC84, prH2, gcLo}, // HANGUL SYLLABLE BEO
- {0xBC85, 0xBC9F, prH3, gcLo}, // [27] HANGUL SYLLABLE BEOG..HANGUL SYLLABLE BEOH
- {0xBCA0, 0xBCA0, prH2, gcLo}, // HANGUL SYLLABLE BE
- {0xBCA1, 0xBCBB, prH3, gcLo}, // [27] HANGUL SYLLABLE BEG..HANGUL SYLLABLE BEH
- {0xBCBC, 0xBCBC, prH2, gcLo}, // HANGUL SYLLABLE BYEO
- {0xBCBD, 0xBCD7, prH3, gcLo}, // [27] HANGUL SYLLABLE BYEOG..HANGUL SYLLABLE BYEOH
- {0xBCD8, 0xBCD8, prH2, gcLo}, // HANGUL SYLLABLE BYE
- {0xBCD9, 0xBCF3, prH3, gcLo}, // [27] HANGUL SYLLABLE BYEG..HANGUL SYLLABLE BYEH
- {0xBCF4, 0xBCF4, prH2, gcLo}, // HANGUL SYLLABLE BO
- {0xBCF5, 0xBD0F, prH3, gcLo}, // [27] HANGUL SYLLABLE BOG..HANGUL SYLLABLE BOH
- {0xBD10, 0xBD10, prH2, gcLo}, // HANGUL SYLLABLE BWA
- {0xBD11, 0xBD2B, prH3, gcLo}, // [27] HANGUL SYLLABLE BWAG..HANGUL SYLLABLE BWAH
- {0xBD2C, 0xBD2C, prH2, gcLo}, // HANGUL SYLLABLE BWAE
- {0xBD2D, 0xBD47, prH3, gcLo}, // [27] HANGUL SYLLABLE BWAEG..HANGUL SYLLABLE BWAEH
- {0xBD48, 0xBD48, prH2, gcLo}, // HANGUL SYLLABLE BOE
- {0xBD49, 0xBD63, prH3, gcLo}, // [27] HANGUL SYLLABLE BOEG..HANGUL SYLLABLE BOEH
- {0xBD64, 0xBD64, prH2, gcLo}, // HANGUL SYLLABLE BYO
- {0xBD65, 0xBD7F, prH3, gcLo}, // [27] HANGUL SYLLABLE BYOG..HANGUL SYLLABLE BYOH
- {0xBD80, 0xBD80, prH2, gcLo}, // HANGUL SYLLABLE BU
- {0xBD81, 0xBD9B, prH3, gcLo}, // [27] HANGUL SYLLABLE BUG..HANGUL SYLLABLE BUH
- {0xBD9C, 0xBD9C, prH2, gcLo}, // HANGUL SYLLABLE BWEO
- {0xBD9D, 0xBDB7, prH3, gcLo}, // [27] HANGUL SYLLABLE BWEOG..HANGUL SYLLABLE BWEOH
- {0xBDB8, 0xBDB8, prH2, gcLo}, // HANGUL SYLLABLE BWE
- {0xBDB9, 0xBDD3, prH3, gcLo}, // [27] HANGUL SYLLABLE BWEG..HANGUL SYLLABLE BWEH
- {0xBDD4, 0xBDD4, prH2, gcLo}, // HANGUL SYLLABLE BWI
- {0xBDD5, 0xBDEF, prH3, gcLo}, // [27] HANGUL SYLLABLE BWIG..HANGUL SYLLABLE BWIH
- {0xBDF0, 0xBDF0, prH2, gcLo}, // HANGUL SYLLABLE BYU
- {0xBDF1, 0xBE0B, prH3, gcLo}, // [27] HANGUL SYLLABLE BYUG..HANGUL SYLLABLE BYUH
- {0xBE0C, 0xBE0C, prH2, gcLo}, // HANGUL SYLLABLE BEU
- {0xBE0D, 0xBE27, prH3, gcLo}, // [27] HANGUL SYLLABLE BEUG..HANGUL SYLLABLE BEUH
- {0xBE28, 0xBE28, prH2, gcLo}, // HANGUL SYLLABLE BYI
- {0xBE29, 0xBE43, prH3, gcLo}, // [27] HANGUL SYLLABLE BYIG..HANGUL SYLLABLE BYIH
- {0xBE44, 0xBE44, prH2, gcLo}, // HANGUL SYLLABLE BI
- {0xBE45, 0xBE5F, prH3, gcLo}, // [27] HANGUL SYLLABLE BIG..HANGUL SYLLABLE BIH
- {0xBE60, 0xBE60, prH2, gcLo}, // HANGUL SYLLABLE BBA
- {0xBE61, 0xBE7B, prH3, gcLo}, // [27] HANGUL SYLLABLE BBAG..HANGUL SYLLABLE BBAH
- {0xBE7C, 0xBE7C, prH2, gcLo}, // HANGUL SYLLABLE BBAE
- {0xBE7D, 0xBE97, prH3, gcLo}, // [27] HANGUL SYLLABLE BBAEG..HANGUL SYLLABLE BBAEH
- {0xBE98, 0xBE98, prH2, gcLo}, // HANGUL SYLLABLE BBYA
- {0xBE99, 0xBEB3, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYAG..HANGUL SYLLABLE BBYAH
- {0xBEB4, 0xBEB4, prH2, gcLo}, // HANGUL SYLLABLE BBYAE
- {0xBEB5, 0xBECF, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYAEG..HANGUL SYLLABLE BBYAEH
- {0xBED0, 0xBED0, prH2, gcLo}, // HANGUL SYLLABLE BBEO
- {0xBED1, 0xBEEB, prH3, gcLo}, // [27] HANGUL SYLLABLE BBEOG..HANGUL SYLLABLE BBEOH
- {0xBEEC, 0xBEEC, prH2, gcLo}, // HANGUL SYLLABLE BBE
- {0xBEED, 0xBF07, prH3, gcLo}, // [27] HANGUL SYLLABLE BBEG..HANGUL SYLLABLE BBEH
- {0xBF08, 0xBF08, prH2, gcLo}, // HANGUL SYLLABLE BBYEO
- {0xBF09, 0xBF23, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYEOG..HANGUL SYLLABLE BBYEOH
- {0xBF24, 0xBF24, prH2, gcLo}, // HANGUL SYLLABLE BBYE
- {0xBF25, 0xBF3F, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYEG..HANGUL SYLLABLE BBYEH
- {0xBF40, 0xBF40, prH2, gcLo}, // HANGUL SYLLABLE BBO
- {0xBF41, 0xBF5B, prH3, gcLo}, // [27] HANGUL SYLLABLE BBOG..HANGUL SYLLABLE BBOH
- {0xBF5C, 0xBF5C, prH2, gcLo}, // HANGUL SYLLABLE BBWA
- {0xBF5D, 0xBF77, prH3, gcLo}, // [27] HANGUL SYLLABLE BBWAG..HANGUL SYLLABLE BBWAH
- {0xBF78, 0xBF78, prH2, gcLo}, // HANGUL SYLLABLE BBWAE
- {0xBF79, 0xBF93, prH3, gcLo}, // [27] HANGUL SYLLABLE BBWAEG..HANGUL SYLLABLE BBWAEH
- {0xBF94, 0xBF94, prH2, gcLo}, // HANGUL SYLLABLE BBOE
- {0xBF95, 0xBFAF, prH3, gcLo}, // [27] HANGUL SYLLABLE BBOEG..HANGUL SYLLABLE BBOEH
- {0xBFB0, 0xBFB0, prH2, gcLo}, // HANGUL SYLLABLE BBYO
- {0xBFB1, 0xBFCB, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYOG..HANGUL SYLLABLE BBYOH
- {0xBFCC, 0xBFCC, prH2, gcLo}, // HANGUL SYLLABLE BBU
- {0xBFCD, 0xBFE7, prH3, gcLo}, // [27] HANGUL SYLLABLE BBUG..HANGUL SYLLABLE BBUH
- {0xBFE8, 0xBFE8, prH2, gcLo}, // HANGUL SYLLABLE BBWEO
- {0xBFE9, 0xC003, prH3, gcLo}, // [27] HANGUL SYLLABLE BBWEOG..HANGUL SYLLABLE BBWEOH
- {0xC004, 0xC004, prH2, gcLo}, // HANGUL SYLLABLE BBWE
- {0xC005, 0xC01F, prH3, gcLo}, // [27] HANGUL SYLLABLE BBWEG..HANGUL SYLLABLE BBWEH
- {0xC020, 0xC020, prH2, gcLo}, // HANGUL SYLLABLE BBWI
- {0xC021, 0xC03B, prH3, gcLo}, // [27] HANGUL SYLLABLE BBWIG..HANGUL SYLLABLE BBWIH
- {0xC03C, 0xC03C, prH2, gcLo}, // HANGUL SYLLABLE BBYU
- {0xC03D, 0xC057, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYUG..HANGUL SYLLABLE BBYUH
- {0xC058, 0xC058, prH2, gcLo}, // HANGUL SYLLABLE BBEU
- {0xC059, 0xC073, prH3, gcLo}, // [27] HANGUL SYLLABLE BBEUG..HANGUL SYLLABLE BBEUH
- {0xC074, 0xC074, prH2, gcLo}, // HANGUL SYLLABLE BBYI
- {0xC075, 0xC08F, prH3, gcLo}, // [27] HANGUL SYLLABLE BBYIG..HANGUL SYLLABLE BBYIH
- {0xC090, 0xC090, prH2, gcLo}, // HANGUL SYLLABLE BBI
- {0xC091, 0xC0AB, prH3, gcLo}, // [27] HANGUL SYLLABLE BBIG..HANGUL SYLLABLE BBIH
- {0xC0AC, 0xC0AC, prH2, gcLo}, // HANGUL SYLLABLE SA
- {0xC0AD, 0xC0C7, prH3, gcLo}, // [27] HANGUL SYLLABLE SAG..HANGUL SYLLABLE SAH
- {0xC0C8, 0xC0C8, prH2, gcLo}, // HANGUL SYLLABLE SAE
- {0xC0C9, 0xC0E3, prH3, gcLo}, // [27] HANGUL SYLLABLE SAEG..HANGUL SYLLABLE SAEH
- {0xC0E4, 0xC0E4, prH2, gcLo}, // HANGUL SYLLABLE SYA
- {0xC0E5, 0xC0FF, prH3, gcLo}, // [27] HANGUL SYLLABLE SYAG..HANGUL SYLLABLE SYAH
- {0xC100, 0xC100, prH2, gcLo}, // HANGUL SYLLABLE SYAE
- {0xC101, 0xC11B, prH3, gcLo}, // [27] HANGUL SYLLABLE SYAEG..HANGUL SYLLABLE SYAEH
- {0xC11C, 0xC11C, prH2, gcLo}, // HANGUL SYLLABLE SEO
- {0xC11D, 0xC137, prH3, gcLo}, // [27] HANGUL SYLLABLE SEOG..HANGUL SYLLABLE SEOH
- {0xC138, 0xC138, prH2, gcLo}, // HANGUL SYLLABLE SE
- {0xC139, 0xC153, prH3, gcLo}, // [27] HANGUL SYLLABLE SEG..HANGUL SYLLABLE SEH
- {0xC154, 0xC154, prH2, gcLo}, // HANGUL SYLLABLE SYEO
- {0xC155, 0xC16F, prH3, gcLo}, // [27] HANGUL SYLLABLE SYEOG..HANGUL SYLLABLE SYEOH
- {0xC170, 0xC170, prH2, gcLo}, // HANGUL SYLLABLE SYE
- {0xC171, 0xC18B, prH3, gcLo}, // [27] HANGUL SYLLABLE SYEG..HANGUL SYLLABLE SYEH
- {0xC18C, 0xC18C, prH2, gcLo}, // HANGUL SYLLABLE SO
- {0xC18D, 0xC1A7, prH3, gcLo}, // [27] HANGUL SYLLABLE SOG..HANGUL SYLLABLE SOH
- {0xC1A8, 0xC1A8, prH2, gcLo}, // HANGUL SYLLABLE SWA
- {0xC1A9, 0xC1C3, prH3, gcLo}, // [27] HANGUL SYLLABLE SWAG..HANGUL SYLLABLE SWAH
- {0xC1C4, 0xC1C4, prH2, gcLo}, // HANGUL SYLLABLE SWAE
- {0xC1C5, 0xC1DF, prH3, gcLo}, // [27] HANGUL SYLLABLE SWAEG..HANGUL SYLLABLE SWAEH
- {0xC1E0, 0xC1E0, prH2, gcLo}, // HANGUL SYLLABLE SOE
- {0xC1E1, 0xC1FB, prH3, gcLo}, // [27] HANGUL SYLLABLE SOEG..HANGUL SYLLABLE SOEH
- {0xC1FC, 0xC1FC, prH2, gcLo}, // HANGUL SYLLABLE SYO
- {0xC1FD, 0xC217, prH3, gcLo}, // [27] HANGUL SYLLABLE SYOG..HANGUL SYLLABLE SYOH
- {0xC218, 0xC218, prH2, gcLo}, // HANGUL SYLLABLE SU
- {0xC219, 0xC233, prH3, gcLo}, // [27] HANGUL SYLLABLE SUG..HANGUL SYLLABLE SUH
- {0xC234, 0xC234, prH2, gcLo}, // HANGUL SYLLABLE SWEO
- {0xC235, 0xC24F, prH3, gcLo}, // [27] HANGUL SYLLABLE SWEOG..HANGUL SYLLABLE SWEOH
- {0xC250, 0xC250, prH2, gcLo}, // HANGUL SYLLABLE SWE
- {0xC251, 0xC26B, prH3, gcLo}, // [27] HANGUL SYLLABLE SWEG..HANGUL SYLLABLE SWEH
- {0xC26C, 0xC26C, prH2, gcLo}, // HANGUL SYLLABLE SWI
- {0xC26D, 0xC287, prH3, gcLo}, // [27] HANGUL SYLLABLE SWIG..HANGUL SYLLABLE SWIH
- {0xC288, 0xC288, prH2, gcLo}, // HANGUL SYLLABLE SYU
- {0xC289, 0xC2A3, prH3, gcLo}, // [27] HANGUL SYLLABLE SYUG..HANGUL SYLLABLE SYUH
- {0xC2A4, 0xC2A4, prH2, gcLo}, // HANGUL SYLLABLE SEU
- {0xC2A5, 0xC2BF, prH3, gcLo}, // [27] HANGUL SYLLABLE SEUG..HANGUL SYLLABLE SEUH
- {0xC2C0, 0xC2C0, prH2, gcLo}, // HANGUL SYLLABLE SYI
- {0xC2C1, 0xC2DB, prH3, gcLo}, // [27] HANGUL SYLLABLE SYIG..HANGUL SYLLABLE SYIH
- {0xC2DC, 0xC2DC, prH2, gcLo}, // HANGUL SYLLABLE SI
- {0xC2DD, 0xC2F7, prH3, gcLo}, // [27] HANGUL SYLLABLE SIG..HANGUL SYLLABLE SIH
- {0xC2F8, 0xC2F8, prH2, gcLo}, // HANGUL SYLLABLE SSA
- {0xC2F9, 0xC313, prH3, gcLo}, // [27] HANGUL SYLLABLE SSAG..HANGUL SYLLABLE SSAH
- {0xC314, 0xC314, prH2, gcLo}, // HANGUL SYLLABLE SSAE
- {0xC315, 0xC32F, prH3, gcLo}, // [27] HANGUL SYLLABLE SSAEG..HANGUL SYLLABLE SSAEH
- {0xC330, 0xC330, prH2, gcLo}, // HANGUL SYLLABLE SSYA
- {0xC331, 0xC34B, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYAG..HANGUL SYLLABLE SSYAH
- {0xC34C, 0xC34C, prH2, gcLo}, // HANGUL SYLLABLE SSYAE
- {0xC34D, 0xC367, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYAEG..HANGUL SYLLABLE SSYAEH
- {0xC368, 0xC368, prH2, gcLo}, // HANGUL SYLLABLE SSEO
- {0xC369, 0xC383, prH3, gcLo}, // [27] HANGUL SYLLABLE SSEOG..HANGUL SYLLABLE SSEOH
- {0xC384, 0xC384, prH2, gcLo}, // HANGUL SYLLABLE SSE
- {0xC385, 0xC39F, prH3, gcLo}, // [27] HANGUL SYLLABLE SSEG..HANGUL SYLLABLE SSEH
- {0xC3A0, 0xC3A0, prH2, gcLo}, // HANGUL SYLLABLE SSYEO
- {0xC3A1, 0xC3BB, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYEOG..HANGUL SYLLABLE SSYEOH
- {0xC3BC, 0xC3BC, prH2, gcLo}, // HANGUL SYLLABLE SSYE
- {0xC3BD, 0xC3D7, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYEG..HANGUL SYLLABLE SSYEH
- {0xC3D8, 0xC3D8, prH2, gcLo}, // HANGUL SYLLABLE SSO
- {0xC3D9, 0xC3F3, prH3, gcLo}, // [27] HANGUL SYLLABLE SSOG..HANGUL SYLLABLE SSOH
- {0xC3F4, 0xC3F4, prH2, gcLo}, // HANGUL SYLLABLE SSWA
- {0xC3F5, 0xC40F, prH3, gcLo}, // [27] HANGUL SYLLABLE SSWAG..HANGUL SYLLABLE SSWAH
- {0xC410, 0xC410, prH2, gcLo}, // HANGUL SYLLABLE SSWAE
- {0xC411, 0xC42B, prH3, gcLo}, // [27] HANGUL SYLLABLE SSWAEG..HANGUL SYLLABLE SSWAEH
- {0xC42C, 0xC42C, prH2, gcLo}, // HANGUL SYLLABLE SSOE
- {0xC42D, 0xC447, prH3, gcLo}, // [27] HANGUL SYLLABLE SSOEG..HANGUL SYLLABLE SSOEH
- {0xC448, 0xC448, prH2, gcLo}, // HANGUL SYLLABLE SSYO
- {0xC449, 0xC463, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYOG..HANGUL SYLLABLE SSYOH
- {0xC464, 0xC464, prH2, gcLo}, // HANGUL SYLLABLE SSU
- {0xC465, 0xC47F, prH3, gcLo}, // [27] HANGUL SYLLABLE SSUG..HANGUL SYLLABLE SSUH
- {0xC480, 0xC480, prH2, gcLo}, // HANGUL SYLLABLE SSWEO
- {0xC481, 0xC49B, prH3, gcLo}, // [27] HANGUL SYLLABLE SSWEOG..HANGUL SYLLABLE SSWEOH
- {0xC49C, 0xC49C, prH2, gcLo}, // HANGUL SYLLABLE SSWE
- {0xC49D, 0xC4B7, prH3, gcLo}, // [27] HANGUL SYLLABLE SSWEG..HANGUL SYLLABLE SSWEH
- {0xC4B8, 0xC4B8, prH2, gcLo}, // HANGUL SYLLABLE SSWI
- {0xC4B9, 0xC4D3, prH3, gcLo}, // [27] HANGUL SYLLABLE SSWIG..HANGUL SYLLABLE SSWIH
- {0xC4D4, 0xC4D4, prH2, gcLo}, // HANGUL SYLLABLE SSYU
- {0xC4D5, 0xC4EF, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYUG..HANGUL SYLLABLE SSYUH
- {0xC4F0, 0xC4F0, prH2, gcLo}, // HANGUL SYLLABLE SSEU
- {0xC4F1, 0xC50B, prH3, gcLo}, // [27] HANGUL SYLLABLE SSEUG..HANGUL SYLLABLE SSEUH
- {0xC50C, 0xC50C, prH2, gcLo}, // HANGUL SYLLABLE SSYI
- {0xC50D, 0xC527, prH3, gcLo}, // [27] HANGUL SYLLABLE SSYIG..HANGUL SYLLABLE SSYIH
- {0xC528, 0xC528, prH2, gcLo}, // HANGUL SYLLABLE SSI
- {0xC529, 0xC543, prH3, gcLo}, // [27] HANGUL SYLLABLE SSIG..HANGUL SYLLABLE SSIH
- {0xC544, 0xC544, prH2, gcLo}, // HANGUL SYLLABLE A
- {0xC545, 0xC55F, prH3, gcLo}, // [27] HANGUL SYLLABLE AG..HANGUL SYLLABLE AH
- {0xC560, 0xC560, prH2, gcLo}, // HANGUL SYLLABLE AE
- {0xC561, 0xC57B, prH3, gcLo}, // [27] HANGUL SYLLABLE AEG..HANGUL SYLLABLE AEH
- {0xC57C, 0xC57C, prH2, gcLo}, // HANGUL SYLLABLE YA
- {0xC57D, 0xC597, prH3, gcLo}, // [27] HANGUL SYLLABLE YAG..HANGUL SYLLABLE YAH
- {0xC598, 0xC598, prH2, gcLo}, // HANGUL SYLLABLE YAE
- {0xC599, 0xC5B3, prH3, gcLo}, // [27] HANGUL SYLLABLE YAEG..HANGUL SYLLABLE YAEH
- {0xC5B4, 0xC5B4, prH2, gcLo}, // HANGUL SYLLABLE EO
- {0xC5B5, 0xC5CF, prH3, gcLo}, // [27] HANGUL SYLLABLE EOG..HANGUL SYLLABLE EOH
- {0xC5D0, 0xC5D0, prH2, gcLo}, // HANGUL SYLLABLE E
- {0xC5D1, 0xC5EB, prH3, gcLo}, // [27] HANGUL SYLLABLE EG..HANGUL SYLLABLE EH
- {0xC5EC, 0xC5EC, prH2, gcLo}, // HANGUL SYLLABLE YEO
- {0xC5ED, 0xC607, prH3, gcLo}, // [27] HANGUL SYLLABLE YEOG..HANGUL SYLLABLE YEOH
- {0xC608, 0xC608, prH2, gcLo}, // HANGUL SYLLABLE YE
- {0xC609, 0xC623, prH3, gcLo}, // [27] HANGUL SYLLABLE YEG..HANGUL SYLLABLE YEH
- {0xC624, 0xC624, prH2, gcLo}, // HANGUL SYLLABLE O
- {0xC625, 0xC63F, prH3, gcLo}, // [27] HANGUL SYLLABLE OG..HANGUL SYLLABLE OH
- {0xC640, 0xC640, prH2, gcLo}, // HANGUL SYLLABLE WA
- {0xC641, 0xC65B, prH3, gcLo}, // [27] HANGUL SYLLABLE WAG..HANGUL SYLLABLE WAH
- {0xC65C, 0xC65C, prH2, gcLo}, // HANGUL SYLLABLE WAE
- {0xC65D, 0xC677, prH3, gcLo}, // [27] HANGUL SYLLABLE WAEG..HANGUL SYLLABLE WAEH
- {0xC678, 0xC678, prH2, gcLo}, // HANGUL SYLLABLE OE
- {0xC679, 0xC693, prH3, gcLo}, // [27] HANGUL SYLLABLE OEG..HANGUL SYLLABLE OEH
- {0xC694, 0xC694, prH2, gcLo}, // HANGUL SYLLABLE YO
- {0xC695, 0xC6AF, prH3, gcLo}, // [27] HANGUL SYLLABLE YOG..HANGUL SYLLABLE YOH
- {0xC6B0, 0xC6B0, prH2, gcLo}, // HANGUL SYLLABLE U
- {0xC6B1, 0xC6CB, prH3, gcLo}, // [27] HANGUL SYLLABLE UG..HANGUL SYLLABLE UH
- {0xC6CC, 0xC6CC, prH2, gcLo}, // HANGUL SYLLABLE WEO
- {0xC6CD, 0xC6E7, prH3, gcLo}, // [27] HANGUL SYLLABLE WEOG..HANGUL SYLLABLE WEOH
- {0xC6E8, 0xC6E8, prH2, gcLo}, // HANGUL SYLLABLE WE
- {0xC6E9, 0xC703, prH3, gcLo}, // [27] HANGUL SYLLABLE WEG..HANGUL SYLLABLE WEH
- {0xC704, 0xC704, prH2, gcLo}, // HANGUL SYLLABLE WI
- {0xC705, 0xC71F, prH3, gcLo}, // [27] HANGUL SYLLABLE WIG..HANGUL SYLLABLE WIH
- {0xC720, 0xC720, prH2, gcLo}, // HANGUL SYLLABLE YU
- {0xC721, 0xC73B, prH3, gcLo}, // [27] HANGUL SYLLABLE YUG..HANGUL SYLLABLE YUH
- {0xC73C, 0xC73C, prH2, gcLo}, // HANGUL SYLLABLE EU
- {0xC73D, 0xC757, prH3, gcLo}, // [27] HANGUL SYLLABLE EUG..HANGUL SYLLABLE EUH
- {0xC758, 0xC758, prH2, gcLo}, // HANGUL SYLLABLE YI
- {0xC759, 0xC773, prH3, gcLo}, // [27] HANGUL SYLLABLE YIG..HANGUL SYLLABLE YIH
- {0xC774, 0xC774, prH2, gcLo}, // HANGUL SYLLABLE I
- {0xC775, 0xC78F, prH3, gcLo}, // [27] HANGUL SYLLABLE IG..HANGUL SYLLABLE IH
- {0xC790, 0xC790, prH2, gcLo}, // HANGUL SYLLABLE JA
- {0xC791, 0xC7AB, prH3, gcLo}, // [27] HANGUL SYLLABLE JAG..HANGUL SYLLABLE JAH
- {0xC7AC, 0xC7AC, prH2, gcLo}, // HANGUL SYLLABLE JAE
- {0xC7AD, 0xC7C7, prH3, gcLo}, // [27] HANGUL SYLLABLE JAEG..HANGUL SYLLABLE JAEH
- {0xC7C8, 0xC7C8, prH2, gcLo}, // HANGUL SYLLABLE JYA
- {0xC7C9, 0xC7E3, prH3, gcLo}, // [27] HANGUL SYLLABLE JYAG..HANGUL SYLLABLE JYAH
- {0xC7E4, 0xC7E4, prH2, gcLo}, // HANGUL SYLLABLE JYAE
- {0xC7E5, 0xC7FF, prH3, gcLo}, // [27] HANGUL SYLLABLE JYAEG..HANGUL SYLLABLE JYAEH
- {0xC800, 0xC800, prH2, gcLo}, // HANGUL SYLLABLE JEO
- {0xC801, 0xC81B, prH3, gcLo}, // [27] HANGUL SYLLABLE JEOG..HANGUL SYLLABLE JEOH
- {0xC81C, 0xC81C, prH2, gcLo}, // HANGUL SYLLABLE JE
- {0xC81D, 0xC837, prH3, gcLo}, // [27] HANGUL SYLLABLE JEG..HANGUL SYLLABLE JEH
- {0xC838, 0xC838, prH2, gcLo}, // HANGUL SYLLABLE JYEO
- {0xC839, 0xC853, prH3, gcLo}, // [27] HANGUL SYLLABLE JYEOG..HANGUL SYLLABLE JYEOH
- {0xC854, 0xC854, prH2, gcLo}, // HANGUL SYLLABLE JYE
- {0xC855, 0xC86F, prH3, gcLo}, // [27] HANGUL SYLLABLE JYEG..HANGUL SYLLABLE JYEH
- {0xC870, 0xC870, prH2, gcLo}, // HANGUL SYLLABLE JO
- {0xC871, 0xC88B, prH3, gcLo}, // [27] HANGUL SYLLABLE JOG..HANGUL SYLLABLE JOH
- {0xC88C, 0xC88C, prH2, gcLo}, // HANGUL SYLLABLE JWA
- {0xC88D, 0xC8A7, prH3, gcLo}, // [27] HANGUL SYLLABLE JWAG..HANGUL SYLLABLE JWAH
- {0xC8A8, 0xC8A8, prH2, gcLo}, // HANGUL SYLLABLE JWAE
- {0xC8A9, 0xC8C3, prH3, gcLo}, // [27] HANGUL SYLLABLE JWAEG..HANGUL SYLLABLE JWAEH
- {0xC8C4, 0xC8C4, prH2, gcLo}, // HANGUL SYLLABLE JOE
- {0xC8C5, 0xC8DF, prH3, gcLo}, // [27] HANGUL SYLLABLE JOEG..HANGUL SYLLABLE JOEH
- {0xC8E0, 0xC8E0, prH2, gcLo}, // HANGUL SYLLABLE JYO
- {0xC8E1, 0xC8FB, prH3, gcLo}, // [27] HANGUL SYLLABLE JYOG..HANGUL SYLLABLE JYOH
- {0xC8FC, 0xC8FC, prH2, gcLo}, // HANGUL SYLLABLE JU
- {0xC8FD, 0xC917, prH3, gcLo}, // [27] HANGUL SYLLABLE JUG..HANGUL SYLLABLE JUH
- {0xC918, 0xC918, prH2, gcLo}, // HANGUL SYLLABLE JWEO
- {0xC919, 0xC933, prH3, gcLo}, // [27] HANGUL SYLLABLE JWEOG..HANGUL SYLLABLE JWEOH
- {0xC934, 0xC934, prH2, gcLo}, // HANGUL SYLLABLE JWE
- {0xC935, 0xC94F, prH3, gcLo}, // [27] HANGUL SYLLABLE JWEG..HANGUL SYLLABLE JWEH
- {0xC950, 0xC950, prH2, gcLo}, // HANGUL SYLLABLE JWI
- {0xC951, 0xC96B, prH3, gcLo}, // [27] HANGUL SYLLABLE JWIG..HANGUL SYLLABLE JWIH
- {0xC96C, 0xC96C, prH2, gcLo}, // HANGUL SYLLABLE JYU
- {0xC96D, 0xC987, prH3, gcLo}, // [27] HANGUL SYLLABLE JYUG..HANGUL SYLLABLE JYUH
- {0xC988, 0xC988, prH2, gcLo}, // HANGUL SYLLABLE JEU
- {0xC989, 0xC9A3, prH3, gcLo}, // [27] HANGUL SYLLABLE JEUG..HANGUL SYLLABLE JEUH
- {0xC9A4, 0xC9A4, prH2, gcLo}, // HANGUL SYLLABLE JYI
- {0xC9A5, 0xC9BF, prH3, gcLo}, // [27] HANGUL SYLLABLE JYIG..HANGUL SYLLABLE JYIH
- {0xC9C0, 0xC9C0, prH2, gcLo}, // HANGUL SYLLABLE JI
- {0xC9C1, 0xC9DB, prH3, gcLo}, // [27] HANGUL SYLLABLE JIG..HANGUL SYLLABLE JIH
- {0xC9DC, 0xC9DC, prH2, gcLo}, // HANGUL SYLLABLE JJA
- {0xC9DD, 0xC9F7, prH3, gcLo}, // [27] HANGUL SYLLABLE JJAG..HANGUL SYLLABLE JJAH
- {0xC9F8, 0xC9F8, prH2, gcLo}, // HANGUL SYLLABLE JJAE
- {0xC9F9, 0xCA13, prH3, gcLo}, // [27] HANGUL SYLLABLE JJAEG..HANGUL SYLLABLE JJAEH
- {0xCA14, 0xCA14, prH2, gcLo}, // HANGUL SYLLABLE JJYA
- {0xCA15, 0xCA2F, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYAG..HANGUL SYLLABLE JJYAH
- {0xCA30, 0xCA30, prH2, gcLo}, // HANGUL SYLLABLE JJYAE
- {0xCA31, 0xCA4B, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYAEG..HANGUL SYLLABLE JJYAEH
- {0xCA4C, 0xCA4C, prH2, gcLo}, // HANGUL SYLLABLE JJEO
- {0xCA4D, 0xCA67, prH3, gcLo}, // [27] HANGUL SYLLABLE JJEOG..HANGUL SYLLABLE JJEOH
- {0xCA68, 0xCA68, prH2, gcLo}, // HANGUL SYLLABLE JJE
- {0xCA69, 0xCA83, prH3, gcLo}, // [27] HANGUL SYLLABLE JJEG..HANGUL SYLLABLE JJEH
- {0xCA84, 0xCA84, prH2, gcLo}, // HANGUL SYLLABLE JJYEO
- {0xCA85, 0xCA9F, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYEOG..HANGUL SYLLABLE JJYEOH
- {0xCAA0, 0xCAA0, prH2, gcLo}, // HANGUL SYLLABLE JJYE
- {0xCAA1, 0xCABB, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYEG..HANGUL SYLLABLE JJYEH
- {0xCABC, 0xCABC, prH2, gcLo}, // HANGUL SYLLABLE JJO
- {0xCABD, 0xCAD7, prH3, gcLo}, // [27] HANGUL SYLLABLE JJOG..HANGUL SYLLABLE JJOH
- {0xCAD8, 0xCAD8, prH2, gcLo}, // HANGUL SYLLABLE JJWA
- {0xCAD9, 0xCAF3, prH3, gcLo}, // [27] HANGUL SYLLABLE JJWAG..HANGUL SYLLABLE JJWAH
- {0xCAF4, 0xCAF4, prH2, gcLo}, // HANGUL SYLLABLE JJWAE
- {0xCAF5, 0xCB0F, prH3, gcLo}, // [27] HANGUL SYLLABLE JJWAEG..HANGUL SYLLABLE JJWAEH
- {0xCB10, 0xCB10, prH2, gcLo}, // HANGUL SYLLABLE JJOE
- {0xCB11, 0xCB2B, prH3, gcLo}, // [27] HANGUL SYLLABLE JJOEG..HANGUL SYLLABLE JJOEH
- {0xCB2C, 0xCB2C, prH2, gcLo}, // HANGUL SYLLABLE JJYO
- {0xCB2D, 0xCB47, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYOG..HANGUL SYLLABLE JJYOH
- {0xCB48, 0xCB48, prH2, gcLo}, // HANGUL SYLLABLE JJU
- {0xCB49, 0xCB63, prH3, gcLo}, // [27] HANGUL SYLLABLE JJUG..HANGUL SYLLABLE JJUH
- {0xCB64, 0xCB64, prH2, gcLo}, // HANGUL SYLLABLE JJWEO
- {0xCB65, 0xCB7F, prH3, gcLo}, // [27] HANGUL SYLLABLE JJWEOG..HANGUL SYLLABLE JJWEOH
- {0xCB80, 0xCB80, prH2, gcLo}, // HANGUL SYLLABLE JJWE
- {0xCB81, 0xCB9B, prH3, gcLo}, // [27] HANGUL SYLLABLE JJWEG..HANGUL SYLLABLE JJWEH
- {0xCB9C, 0xCB9C, prH2, gcLo}, // HANGUL SYLLABLE JJWI
- {0xCB9D, 0xCBB7, prH3, gcLo}, // [27] HANGUL SYLLABLE JJWIG..HANGUL SYLLABLE JJWIH
- {0xCBB8, 0xCBB8, prH2, gcLo}, // HANGUL SYLLABLE JJYU
- {0xCBB9, 0xCBD3, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYUG..HANGUL SYLLABLE JJYUH
- {0xCBD4, 0xCBD4, prH2, gcLo}, // HANGUL SYLLABLE JJEU
- {0xCBD5, 0xCBEF, prH3, gcLo}, // [27] HANGUL SYLLABLE JJEUG..HANGUL SYLLABLE JJEUH
- {0xCBF0, 0xCBF0, prH2, gcLo}, // HANGUL SYLLABLE JJYI
- {0xCBF1, 0xCC0B, prH3, gcLo}, // [27] HANGUL SYLLABLE JJYIG..HANGUL SYLLABLE JJYIH
- {0xCC0C, 0xCC0C, prH2, gcLo}, // HANGUL SYLLABLE JJI
- {0xCC0D, 0xCC27, prH3, gcLo}, // [27] HANGUL SYLLABLE JJIG..HANGUL SYLLABLE JJIH
- {0xCC28, 0xCC28, prH2, gcLo}, // HANGUL SYLLABLE CA
- {0xCC29, 0xCC43, prH3, gcLo}, // [27] HANGUL SYLLABLE CAG..HANGUL SYLLABLE CAH
- {0xCC44, 0xCC44, prH2, gcLo}, // HANGUL SYLLABLE CAE
- {0xCC45, 0xCC5F, prH3, gcLo}, // [27] HANGUL SYLLABLE CAEG..HANGUL SYLLABLE CAEH
- {0xCC60, 0xCC60, prH2, gcLo}, // HANGUL SYLLABLE CYA
- {0xCC61, 0xCC7B, prH3, gcLo}, // [27] HANGUL SYLLABLE CYAG..HANGUL SYLLABLE CYAH
- {0xCC7C, 0xCC7C, prH2, gcLo}, // HANGUL SYLLABLE CYAE
- {0xCC7D, 0xCC97, prH3, gcLo}, // [27] HANGUL SYLLABLE CYAEG..HANGUL SYLLABLE CYAEH
- {0xCC98, 0xCC98, prH2, gcLo}, // HANGUL SYLLABLE CEO
- {0xCC99, 0xCCB3, prH3, gcLo}, // [27] HANGUL SYLLABLE CEOG..HANGUL SYLLABLE CEOH
- {0xCCB4, 0xCCB4, prH2, gcLo}, // HANGUL SYLLABLE CE
- {0xCCB5, 0xCCCF, prH3, gcLo}, // [27] HANGUL SYLLABLE CEG..HANGUL SYLLABLE CEH
- {0xCCD0, 0xCCD0, prH2, gcLo}, // HANGUL SYLLABLE CYEO
- {0xCCD1, 0xCCEB, prH3, gcLo}, // [27] HANGUL SYLLABLE CYEOG..HANGUL SYLLABLE CYEOH
- {0xCCEC, 0xCCEC, prH2, gcLo}, // HANGUL SYLLABLE CYE
- {0xCCED, 0xCD07, prH3, gcLo}, // [27] HANGUL SYLLABLE CYEG..HANGUL SYLLABLE CYEH
- {0xCD08, 0xCD08, prH2, gcLo}, // HANGUL SYLLABLE CO
- {0xCD09, 0xCD23, prH3, gcLo}, // [27] HANGUL SYLLABLE COG..HANGUL SYLLABLE COH
- {0xCD24, 0xCD24, prH2, gcLo}, // HANGUL SYLLABLE CWA
- {0xCD25, 0xCD3F, prH3, gcLo}, // [27] HANGUL SYLLABLE CWAG..HANGUL SYLLABLE CWAH
- {0xCD40, 0xCD40, prH2, gcLo}, // HANGUL SYLLABLE CWAE
- {0xCD41, 0xCD5B, prH3, gcLo}, // [27] HANGUL SYLLABLE CWAEG..HANGUL SYLLABLE CWAEH
- {0xCD5C, 0xCD5C, prH2, gcLo}, // HANGUL SYLLABLE COE
- {0xCD5D, 0xCD77, prH3, gcLo}, // [27] HANGUL SYLLABLE COEG..HANGUL SYLLABLE COEH
- {0xCD78, 0xCD78, prH2, gcLo}, // HANGUL SYLLABLE CYO
- {0xCD79, 0xCD93, prH3, gcLo}, // [27] HANGUL SYLLABLE CYOG..HANGUL SYLLABLE CYOH
- {0xCD94, 0xCD94, prH2, gcLo}, // HANGUL SYLLABLE CU
- {0xCD95, 0xCDAF, prH3, gcLo}, // [27] HANGUL SYLLABLE CUG..HANGUL SYLLABLE CUH
- {0xCDB0, 0xCDB0, prH2, gcLo}, // HANGUL SYLLABLE CWEO
- {0xCDB1, 0xCDCB, prH3, gcLo}, // [27] HANGUL SYLLABLE CWEOG..HANGUL SYLLABLE CWEOH
- {0xCDCC, 0xCDCC, prH2, gcLo}, // HANGUL SYLLABLE CWE
- {0xCDCD, 0xCDE7, prH3, gcLo}, // [27] HANGUL SYLLABLE CWEG..HANGUL SYLLABLE CWEH
- {0xCDE8, 0xCDE8, prH2, gcLo}, // HANGUL SYLLABLE CWI
- {0xCDE9, 0xCE03, prH3, gcLo}, // [27] HANGUL SYLLABLE CWIG..HANGUL SYLLABLE CWIH
- {0xCE04, 0xCE04, prH2, gcLo}, // HANGUL SYLLABLE CYU
- {0xCE05, 0xCE1F, prH3, gcLo}, // [27] HANGUL SYLLABLE CYUG..HANGUL SYLLABLE CYUH
- {0xCE20, 0xCE20, prH2, gcLo}, // HANGUL SYLLABLE CEU
- {0xCE21, 0xCE3B, prH3, gcLo}, // [27] HANGUL SYLLABLE CEUG..HANGUL SYLLABLE CEUH
- {0xCE3C, 0xCE3C, prH2, gcLo}, // HANGUL SYLLABLE CYI
- {0xCE3D, 0xCE57, prH3, gcLo}, // [27] HANGUL SYLLABLE CYIG..HANGUL SYLLABLE CYIH
- {0xCE58, 0xCE58, prH2, gcLo}, // HANGUL SYLLABLE CI
- {0xCE59, 0xCE73, prH3, gcLo}, // [27] HANGUL SYLLABLE CIG..HANGUL SYLLABLE CIH
- {0xCE74, 0xCE74, prH2, gcLo}, // HANGUL SYLLABLE KA
- {0xCE75, 0xCE8F, prH3, gcLo}, // [27] HANGUL SYLLABLE KAG..HANGUL SYLLABLE KAH
- {0xCE90, 0xCE90, prH2, gcLo}, // HANGUL SYLLABLE KAE
- {0xCE91, 0xCEAB, prH3, gcLo}, // [27] HANGUL SYLLABLE KAEG..HANGUL SYLLABLE KAEH
- {0xCEAC, 0xCEAC, prH2, gcLo}, // HANGUL SYLLABLE KYA
- {0xCEAD, 0xCEC7, prH3, gcLo}, // [27] HANGUL SYLLABLE KYAG..HANGUL SYLLABLE KYAH
- {0xCEC8, 0xCEC8, prH2, gcLo}, // HANGUL SYLLABLE KYAE
- {0xCEC9, 0xCEE3, prH3, gcLo}, // [27] HANGUL SYLLABLE KYAEG..HANGUL SYLLABLE KYAEH
- {0xCEE4, 0xCEE4, prH2, gcLo}, // HANGUL SYLLABLE KEO
- {0xCEE5, 0xCEFF, prH3, gcLo}, // [27] HANGUL SYLLABLE KEOG..HANGUL SYLLABLE KEOH
- {0xCF00, 0xCF00, prH2, gcLo}, // HANGUL SYLLABLE KE
- {0xCF01, 0xCF1B, prH3, gcLo}, // [27] HANGUL SYLLABLE KEG..HANGUL SYLLABLE KEH
- {0xCF1C, 0xCF1C, prH2, gcLo}, // HANGUL SYLLABLE KYEO
- {0xCF1D, 0xCF37, prH3, gcLo}, // [27] HANGUL SYLLABLE KYEOG..HANGUL SYLLABLE KYEOH
- {0xCF38, 0xCF38, prH2, gcLo}, // HANGUL SYLLABLE KYE
- {0xCF39, 0xCF53, prH3, gcLo}, // [27] HANGUL SYLLABLE KYEG..HANGUL SYLLABLE KYEH
- {0xCF54, 0xCF54, prH2, gcLo}, // HANGUL SYLLABLE KO
- {0xCF55, 0xCF6F, prH3, gcLo}, // [27] HANGUL SYLLABLE KOG..HANGUL SYLLABLE KOH
- {0xCF70, 0xCF70, prH2, gcLo}, // HANGUL SYLLABLE KWA
- {0xCF71, 0xCF8B, prH3, gcLo}, // [27] HANGUL SYLLABLE KWAG..HANGUL SYLLABLE KWAH
- {0xCF8C, 0xCF8C, prH2, gcLo}, // HANGUL SYLLABLE KWAE
- {0xCF8D, 0xCFA7, prH3, gcLo}, // [27] HANGUL SYLLABLE KWAEG..HANGUL SYLLABLE KWAEH
- {0xCFA8, 0xCFA8, prH2, gcLo}, // HANGUL SYLLABLE KOE
- {0xCFA9, 0xCFC3, prH3, gcLo}, // [27] HANGUL SYLLABLE KOEG..HANGUL SYLLABLE KOEH
- {0xCFC4, 0xCFC4, prH2, gcLo}, // HANGUL SYLLABLE KYO
- {0xCFC5, 0xCFDF, prH3, gcLo}, // [27] HANGUL SYLLABLE KYOG..HANGUL SYLLABLE KYOH
- {0xCFE0, 0xCFE0, prH2, gcLo}, // HANGUL SYLLABLE KU
- {0xCFE1, 0xCFFB, prH3, gcLo}, // [27] HANGUL SYLLABLE KUG..HANGUL SYLLABLE KUH
- {0xCFFC, 0xCFFC, prH2, gcLo}, // HANGUL SYLLABLE KWEO
- {0xCFFD, 0xD017, prH3, gcLo}, // [27] HANGUL SYLLABLE KWEOG..HANGUL SYLLABLE KWEOH
- {0xD018, 0xD018, prH2, gcLo}, // HANGUL SYLLABLE KWE
- {0xD019, 0xD033, prH3, gcLo}, // [27] HANGUL SYLLABLE KWEG..HANGUL SYLLABLE KWEH
- {0xD034, 0xD034, prH2, gcLo}, // HANGUL SYLLABLE KWI
- {0xD035, 0xD04F, prH3, gcLo}, // [27] HANGUL SYLLABLE KWIG..HANGUL SYLLABLE KWIH
- {0xD050, 0xD050, prH2, gcLo}, // HANGUL SYLLABLE KYU
- {0xD051, 0xD06B, prH3, gcLo}, // [27] HANGUL SYLLABLE KYUG..HANGUL SYLLABLE KYUH
- {0xD06C, 0xD06C, prH2, gcLo}, // HANGUL SYLLABLE KEU
- {0xD06D, 0xD087, prH3, gcLo}, // [27] HANGUL SYLLABLE KEUG..HANGUL SYLLABLE KEUH
- {0xD088, 0xD088, prH2, gcLo}, // HANGUL SYLLABLE KYI
- {0xD089, 0xD0A3, prH3, gcLo}, // [27] HANGUL SYLLABLE KYIG..HANGUL SYLLABLE KYIH
- {0xD0A4, 0xD0A4, prH2, gcLo}, // HANGUL SYLLABLE KI
- {0xD0A5, 0xD0BF, prH3, gcLo}, // [27] HANGUL SYLLABLE KIG..HANGUL SYLLABLE KIH
- {0xD0C0, 0xD0C0, prH2, gcLo}, // HANGUL SYLLABLE TA
- {0xD0C1, 0xD0DB, prH3, gcLo}, // [27] HANGUL SYLLABLE TAG..HANGUL SYLLABLE TAH
- {0xD0DC, 0xD0DC, prH2, gcLo}, // HANGUL SYLLABLE TAE
- {0xD0DD, 0xD0F7, prH3, gcLo}, // [27] HANGUL SYLLABLE TAEG..HANGUL SYLLABLE TAEH
- {0xD0F8, 0xD0F8, prH2, gcLo}, // HANGUL SYLLABLE TYA
- {0xD0F9, 0xD113, prH3, gcLo}, // [27] HANGUL SYLLABLE TYAG..HANGUL SYLLABLE TYAH
- {0xD114, 0xD114, prH2, gcLo}, // HANGUL SYLLABLE TYAE
- {0xD115, 0xD12F, prH3, gcLo}, // [27] HANGUL SYLLABLE TYAEG..HANGUL SYLLABLE TYAEH
- {0xD130, 0xD130, prH2, gcLo}, // HANGUL SYLLABLE TEO
- {0xD131, 0xD14B, prH3, gcLo}, // [27] HANGUL SYLLABLE TEOG..HANGUL SYLLABLE TEOH
- {0xD14C, 0xD14C, prH2, gcLo}, // HANGUL SYLLABLE TE
- {0xD14D, 0xD167, prH3, gcLo}, // [27] HANGUL SYLLABLE TEG..HANGUL SYLLABLE TEH
- {0xD168, 0xD168, prH2, gcLo}, // HANGUL SYLLABLE TYEO
- {0xD169, 0xD183, prH3, gcLo}, // [27] HANGUL SYLLABLE TYEOG..HANGUL SYLLABLE TYEOH
- {0xD184, 0xD184, prH2, gcLo}, // HANGUL SYLLABLE TYE
- {0xD185, 0xD19F, prH3, gcLo}, // [27] HANGUL SYLLABLE TYEG..HANGUL SYLLABLE TYEH
- {0xD1A0, 0xD1A0, prH2, gcLo}, // HANGUL SYLLABLE TO
- {0xD1A1, 0xD1BB, prH3, gcLo}, // [27] HANGUL SYLLABLE TOG..HANGUL SYLLABLE TOH
- {0xD1BC, 0xD1BC, prH2, gcLo}, // HANGUL SYLLABLE TWA
- {0xD1BD, 0xD1D7, prH3, gcLo}, // [27] HANGUL SYLLABLE TWAG..HANGUL SYLLABLE TWAH
- {0xD1D8, 0xD1D8, prH2, gcLo}, // HANGUL SYLLABLE TWAE
- {0xD1D9, 0xD1F3, prH3, gcLo}, // [27] HANGUL SYLLABLE TWAEG..HANGUL SYLLABLE TWAEH
- {0xD1F4, 0xD1F4, prH2, gcLo}, // HANGUL SYLLABLE TOE
- {0xD1F5, 0xD20F, prH3, gcLo}, // [27] HANGUL SYLLABLE TOEG..HANGUL SYLLABLE TOEH
- {0xD210, 0xD210, prH2, gcLo}, // HANGUL SYLLABLE TYO
- {0xD211, 0xD22B, prH3, gcLo}, // [27] HANGUL SYLLABLE TYOG..HANGUL SYLLABLE TYOH
- {0xD22C, 0xD22C, prH2, gcLo}, // HANGUL SYLLABLE TU
- {0xD22D, 0xD247, prH3, gcLo}, // [27] HANGUL SYLLABLE TUG..HANGUL SYLLABLE TUH
- {0xD248, 0xD248, prH2, gcLo}, // HANGUL SYLLABLE TWEO
- {0xD249, 0xD263, prH3, gcLo}, // [27] HANGUL SYLLABLE TWEOG..HANGUL SYLLABLE TWEOH
- {0xD264, 0xD264, prH2, gcLo}, // HANGUL SYLLABLE TWE
- {0xD265, 0xD27F, prH3, gcLo}, // [27] HANGUL SYLLABLE TWEG..HANGUL SYLLABLE TWEH
- {0xD280, 0xD280, prH2, gcLo}, // HANGUL SYLLABLE TWI
- {0xD281, 0xD29B, prH3, gcLo}, // [27] HANGUL SYLLABLE TWIG..HANGUL SYLLABLE TWIH
- {0xD29C, 0xD29C, prH2, gcLo}, // HANGUL SYLLABLE TYU
- {0xD29D, 0xD2B7, prH3, gcLo}, // [27] HANGUL SYLLABLE TYUG..HANGUL SYLLABLE TYUH
- {0xD2B8, 0xD2B8, prH2, gcLo}, // HANGUL SYLLABLE TEU
- {0xD2B9, 0xD2D3, prH3, gcLo}, // [27] HANGUL SYLLABLE TEUG..HANGUL SYLLABLE TEUH
- {0xD2D4, 0xD2D4, prH2, gcLo}, // HANGUL SYLLABLE TYI
- {0xD2D5, 0xD2EF, prH3, gcLo}, // [27] HANGUL SYLLABLE TYIG..HANGUL SYLLABLE TYIH
- {0xD2F0, 0xD2F0, prH2, gcLo}, // HANGUL SYLLABLE TI
- {0xD2F1, 0xD30B, prH3, gcLo}, // [27] HANGUL SYLLABLE TIG..HANGUL SYLLABLE TIH
- {0xD30C, 0xD30C, prH2, gcLo}, // HANGUL SYLLABLE PA
- {0xD30D, 0xD327, prH3, gcLo}, // [27] HANGUL SYLLABLE PAG..HANGUL SYLLABLE PAH
- {0xD328, 0xD328, prH2, gcLo}, // HANGUL SYLLABLE PAE
- {0xD329, 0xD343, prH3, gcLo}, // [27] HANGUL SYLLABLE PAEG..HANGUL SYLLABLE PAEH
- {0xD344, 0xD344, prH2, gcLo}, // HANGUL SYLLABLE PYA
- {0xD345, 0xD35F, prH3, gcLo}, // [27] HANGUL SYLLABLE PYAG..HANGUL SYLLABLE PYAH
- {0xD360, 0xD360, prH2, gcLo}, // HANGUL SYLLABLE PYAE
- {0xD361, 0xD37B, prH3, gcLo}, // [27] HANGUL SYLLABLE PYAEG..HANGUL SYLLABLE PYAEH
- {0xD37C, 0xD37C, prH2, gcLo}, // HANGUL SYLLABLE PEO
- {0xD37D, 0xD397, prH3, gcLo}, // [27] HANGUL SYLLABLE PEOG..HANGUL SYLLABLE PEOH
- {0xD398, 0xD398, prH2, gcLo}, // HANGUL SYLLABLE PE
- {0xD399, 0xD3B3, prH3, gcLo}, // [27] HANGUL SYLLABLE PEG..HANGUL SYLLABLE PEH
- {0xD3B4, 0xD3B4, prH2, gcLo}, // HANGUL SYLLABLE PYEO
- {0xD3B5, 0xD3CF, prH3, gcLo}, // [27] HANGUL SYLLABLE PYEOG..HANGUL SYLLABLE PYEOH
- {0xD3D0, 0xD3D0, prH2, gcLo}, // HANGUL SYLLABLE PYE
- {0xD3D1, 0xD3EB, prH3, gcLo}, // [27] HANGUL SYLLABLE PYEG..HANGUL SYLLABLE PYEH
- {0xD3EC, 0xD3EC, prH2, gcLo}, // HANGUL SYLLABLE PO
- {0xD3ED, 0xD407, prH3, gcLo}, // [27] HANGUL SYLLABLE POG..HANGUL SYLLABLE POH
- {0xD408, 0xD408, prH2, gcLo}, // HANGUL SYLLABLE PWA
- {0xD409, 0xD423, prH3, gcLo}, // [27] HANGUL SYLLABLE PWAG..HANGUL SYLLABLE PWAH
- {0xD424, 0xD424, prH2, gcLo}, // HANGUL SYLLABLE PWAE
- {0xD425, 0xD43F, prH3, gcLo}, // [27] HANGUL SYLLABLE PWAEG..HANGUL SYLLABLE PWAEH
- {0xD440, 0xD440, prH2, gcLo}, // HANGUL SYLLABLE POE
- {0xD441, 0xD45B, prH3, gcLo}, // [27] HANGUL SYLLABLE POEG..HANGUL SYLLABLE POEH
- {0xD45C, 0xD45C, prH2, gcLo}, // HANGUL SYLLABLE PYO
- {0xD45D, 0xD477, prH3, gcLo}, // [27] HANGUL SYLLABLE PYOG..HANGUL SYLLABLE PYOH
- {0xD478, 0xD478, prH2, gcLo}, // HANGUL SYLLABLE PU
- {0xD479, 0xD493, prH3, gcLo}, // [27] HANGUL SYLLABLE PUG..HANGUL SYLLABLE PUH
- {0xD494, 0xD494, prH2, gcLo}, // HANGUL SYLLABLE PWEO
- {0xD495, 0xD4AF, prH3, gcLo}, // [27] HANGUL SYLLABLE PWEOG..HANGUL SYLLABLE PWEOH
- {0xD4B0, 0xD4B0, prH2, gcLo}, // HANGUL SYLLABLE PWE
- {0xD4B1, 0xD4CB, prH3, gcLo}, // [27] HANGUL SYLLABLE PWEG..HANGUL SYLLABLE PWEH
- {0xD4CC, 0xD4CC, prH2, gcLo}, // HANGUL SYLLABLE PWI
- {0xD4CD, 0xD4E7, prH3, gcLo}, // [27] HANGUL SYLLABLE PWIG..HANGUL SYLLABLE PWIH
- {0xD4E8, 0xD4E8, prH2, gcLo}, // HANGUL SYLLABLE PYU
- {0xD4E9, 0xD503, prH3, gcLo}, // [27] HANGUL SYLLABLE PYUG..HANGUL SYLLABLE PYUH
- {0xD504, 0xD504, prH2, gcLo}, // HANGUL SYLLABLE PEU
- {0xD505, 0xD51F, prH3, gcLo}, // [27] HANGUL SYLLABLE PEUG..HANGUL SYLLABLE PEUH
- {0xD520, 0xD520, prH2, gcLo}, // HANGUL SYLLABLE PYI
- {0xD521, 0xD53B, prH3, gcLo}, // [27] HANGUL SYLLABLE PYIG..HANGUL SYLLABLE PYIH
- {0xD53C, 0xD53C, prH2, gcLo}, // HANGUL SYLLABLE PI
- {0xD53D, 0xD557, prH3, gcLo}, // [27] HANGUL SYLLABLE PIG..HANGUL SYLLABLE PIH
- {0xD558, 0xD558, prH2, gcLo}, // HANGUL SYLLABLE HA
- {0xD559, 0xD573, prH3, gcLo}, // [27] HANGUL SYLLABLE HAG..HANGUL SYLLABLE HAH
- {0xD574, 0xD574, prH2, gcLo}, // HANGUL SYLLABLE HAE
- {0xD575, 0xD58F, prH3, gcLo}, // [27] HANGUL SYLLABLE HAEG..HANGUL SYLLABLE HAEH
- {0xD590, 0xD590, prH2, gcLo}, // HANGUL SYLLABLE HYA
- {0xD591, 0xD5AB, prH3, gcLo}, // [27] HANGUL SYLLABLE HYAG..HANGUL SYLLABLE HYAH
- {0xD5AC, 0xD5AC, prH2, gcLo}, // HANGUL SYLLABLE HYAE
- {0xD5AD, 0xD5C7, prH3, gcLo}, // [27] HANGUL SYLLABLE HYAEG..HANGUL SYLLABLE HYAEH
- {0xD5C8, 0xD5C8, prH2, gcLo}, // HANGUL SYLLABLE HEO
- {0xD5C9, 0xD5E3, prH3, gcLo}, // [27] HANGUL SYLLABLE HEOG..HANGUL SYLLABLE HEOH
- {0xD5E4, 0xD5E4, prH2, gcLo}, // HANGUL SYLLABLE HE
- {0xD5E5, 0xD5FF, prH3, gcLo}, // [27] HANGUL SYLLABLE HEG..HANGUL SYLLABLE HEH
- {0xD600, 0xD600, prH2, gcLo}, // HANGUL SYLLABLE HYEO
- {0xD601, 0xD61B, prH3, gcLo}, // [27] HANGUL SYLLABLE HYEOG..HANGUL SYLLABLE HYEOH
- {0xD61C, 0xD61C, prH2, gcLo}, // HANGUL SYLLABLE HYE
- {0xD61D, 0xD637, prH3, gcLo}, // [27] HANGUL SYLLABLE HYEG..HANGUL SYLLABLE HYEH
- {0xD638, 0xD638, prH2, gcLo}, // HANGUL SYLLABLE HO
- {0xD639, 0xD653, prH3, gcLo}, // [27] HANGUL SYLLABLE HOG..HANGUL SYLLABLE HOH
- {0xD654, 0xD654, prH2, gcLo}, // HANGUL SYLLABLE HWA
- {0xD655, 0xD66F, prH3, gcLo}, // [27] HANGUL SYLLABLE HWAG..HANGUL SYLLABLE HWAH
- {0xD670, 0xD670, prH2, gcLo}, // HANGUL SYLLABLE HWAE
- {0xD671, 0xD68B, prH3, gcLo}, // [27] HANGUL SYLLABLE HWAEG..HANGUL SYLLABLE HWAEH
- {0xD68C, 0xD68C, prH2, gcLo}, // HANGUL SYLLABLE HOE
- {0xD68D, 0xD6A7, prH3, gcLo}, // [27] HANGUL SYLLABLE HOEG..HANGUL SYLLABLE HOEH
- {0xD6A8, 0xD6A8, prH2, gcLo}, // HANGUL SYLLABLE HYO
- {0xD6A9, 0xD6C3, prH3, gcLo}, // [27] HANGUL SYLLABLE HYOG..HANGUL SYLLABLE HYOH
- {0xD6C4, 0xD6C4, prH2, gcLo}, // HANGUL SYLLABLE HU
- {0xD6C5, 0xD6DF, prH3, gcLo}, // [27] HANGUL SYLLABLE HUG..HANGUL SYLLABLE HUH
- {0xD6E0, 0xD6E0, prH2, gcLo}, // HANGUL SYLLABLE HWEO
- {0xD6E1, 0xD6FB, prH3, gcLo}, // [27] HANGUL SYLLABLE HWEOG..HANGUL SYLLABLE HWEOH
- {0xD6FC, 0xD6FC, prH2, gcLo}, // HANGUL SYLLABLE HWE
- {0xD6FD, 0xD717, prH3, gcLo}, // [27] HANGUL SYLLABLE HWEG..HANGUL SYLLABLE HWEH
- {0xD718, 0xD718, prH2, gcLo}, // HANGUL SYLLABLE HWI
- {0xD719, 0xD733, prH3, gcLo}, // [27] HANGUL SYLLABLE HWIG..HANGUL SYLLABLE HWIH
- {0xD734, 0xD734, prH2, gcLo}, // HANGUL SYLLABLE HYU
- {0xD735, 0xD74F, prH3, gcLo}, // [27] HANGUL SYLLABLE HYUG..HANGUL SYLLABLE HYUH
- {0xD750, 0xD750, prH2, gcLo}, // HANGUL SYLLABLE HEU
- {0xD751, 0xD76B, prH3, gcLo}, // [27] HANGUL SYLLABLE HEUG..HANGUL SYLLABLE HEUH
- {0xD76C, 0xD76C, prH2, gcLo}, // HANGUL SYLLABLE HYI
- {0xD76D, 0xD787, prH3, gcLo}, // [27] HANGUL SYLLABLE HYIG..HANGUL SYLLABLE HYIH
- {0xD788, 0xD788, prH2, gcLo}, // HANGUL SYLLABLE HI
- {0xD789, 0xD7A3, prH3, gcLo}, // [27] HANGUL SYLLABLE HIG..HANGUL SYLLABLE HIH
- {0xD7B0, 0xD7C6, prJV, gcLo}, // [23] HANGUL JUNGSEONG O-YEO..HANGUL JUNGSEONG ARAEA-E
- {0xD7CB, 0xD7FB, prJT, gcLo}, // [49] HANGUL JONGSEONG NIEUN-RIEUL..HANGUL JONGSEONG PHIEUPH-THIEUTH
- {0xD800, 0xDB7F, prSG, gcCs}, // [896] ..
- {0xDB80, 0xDBFF, prSG, gcCs}, // [128] ..
- {0xDC00, 0xDFFF, prSG, gcCs}, // [1024] ..
- {0xE000, 0xF8FF, prXX, gcCo}, // [6400] ..
- {0xF900, 0xFA6D, prID, gcLo}, // [366] CJK COMPATIBILITY IDEOGRAPH-F900..CJK COMPATIBILITY IDEOGRAPH-FA6D
- {0xFA6E, 0xFA6F, prID, gcCn}, // [2] ..
- {0xFA70, 0xFAD9, prID, gcLo}, // [106] CJK COMPATIBILITY IDEOGRAPH-FA70..CJK COMPATIBILITY IDEOGRAPH-FAD9
- {0xFADA, 0xFAFF, prID, gcCn}, // [38] ..
- {0xFB00, 0xFB06, prAL, gcLl}, // [7] LATIN SMALL LIGATURE FF..LATIN SMALL LIGATURE ST
- {0xFB13, 0xFB17, prAL, gcLl}, // [5] ARMENIAN SMALL LIGATURE MEN NOW..ARMENIAN SMALL LIGATURE MEN XEH
- {0xFB1D, 0xFB1D, prHL, gcLo}, // HEBREW LETTER YOD WITH HIRIQ
- {0xFB1E, 0xFB1E, prCM, gcMn}, // HEBREW POINT JUDEO-SPANISH VARIKA
- {0xFB1F, 0xFB28, prHL, gcLo}, // [10] HEBREW LIGATURE YIDDISH YOD YOD PATAH..HEBREW LETTER WIDE TAV
- {0xFB29, 0xFB29, prAL, gcSm}, // HEBREW LETTER ALTERNATIVE PLUS SIGN
- {0xFB2A, 0xFB36, prHL, gcLo}, // [13] HEBREW LETTER SHIN WITH SHIN DOT..HEBREW LETTER ZAYIN WITH DAGESH
- {0xFB38, 0xFB3C, prHL, gcLo}, // [5] HEBREW LETTER TET WITH DAGESH..HEBREW LETTER LAMED WITH DAGESH
- {0xFB3E, 0xFB3E, prHL, gcLo}, // HEBREW LETTER MEM WITH DAGESH
- {0xFB40, 0xFB41, prHL, gcLo}, // [2] HEBREW LETTER NUN WITH DAGESH..HEBREW LETTER SAMEKH WITH DAGESH
- {0xFB43, 0xFB44, prHL, gcLo}, // [2] HEBREW LETTER FINAL PE WITH DAGESH..HEBREW LETTER PE WITH DAGESH
- {0xFB46, 0xFB4F, prHL, gcLo}, // [10] HEBREW LETTER TSADI WITH DAGESH..HEBREW LIGATURE ALEF LAMED
- {0xFB50, 0xFBB1, prAL, gcLo}, // [98] ARABIC LETTER ALEF WASLA ISOLATED FORM..ARABIC LETTER YEH BARREE WITH HAMZA ABOVE FINAL FORM
- {0xFBB2, 0xFBC2, prAL, gcSk}, // [17] ARABIC SYMBOL DOT ABOVE..ARABIC SYMBOL WASLA ABOVE
- {0xFBD3, 0xFD3D, prAL, gcLo}, // [363] ARABIC LETTER NG ISOLATED FORM..ARABIC LIGATURE ALEF WITH FATHATAN ISOLATED FORM
- {0xFD3E, 0xFD3E, prCL, gcPe}, // ORNATE LEFT PARENTHESIS
- {0xFD3F, 0xFD3F, prOP, gcPs}, // ORNATE RIGHT PARENTHESIS
- {0xFD40, 0xFD4F, prAL, gcSo}, // [16] ARABIC LIGATURE RAHIMAHU ALLAAH..ARABIC LIGATURE RAHIMAHUM ALLAAH
- {0xFD50, 0xFD8F, prAL, gcLo}, // [64] ARABIC LIGATURE TEH WITH JEEM WITH MEEM INITIAL FORM..ARABIC LIGATURE MEEM WITH KHAH WITH MEEM INITIAL FORM
- {0xFD92, 0xFDC7, prAL, gcLo}, // [54] ARABIC LIGATURE MEEM WITH JEEM WITH KHAH INITIAL FORM..ARABIC LIGATURE NOON WITH JEEM WITH YEH FINAL FORM
- {0xFDCF, 0xFDCF, prAL, gcSo}, // ARABIC LIGATURE SALAAMUHU ALAYNAA
- {0xFDF0, 0xFDFB, prAL, gcLo}, // [12] ARABIC LIGATURE SALLA USED AS KORANIC STOP SIGN ISOLATED FORM..ARABIC LIGATURE JALLAJALALOUHOU
- {0xFDFC, 0xFDFC, prPO, gcSc}, // RIAL SIGN
- {0xFDFD, 0xFDFF, prAL, gcSo}, // [3] ARABIC LIGATURE BISMILLAH AR-RAHMAN AR-RAHEEM..ARABIC LIGATURE AZZA WA JALL
- {0xFE00, 0xFE0F, prCM, gcMn}, // [16] VARIATION SELECTOR-1..VARIATION SELECTOR-16
- {0xFE10, 0xFE10, prIS, gcPo}, // PRESENTATION FORM FOR VERTICAL COMMA
- {0xFE11, 0xFE12, prCL, gcPo}, // [2] PRESENTATION FORM FOR VERTICAL IDEOGRAPHIC COMMA..PRESENTATION FORM FOR VERTICAL IDEOGRAPHIC FULL STOP
- {0xFE13, 0xFE14, prIS, gcPo}, // [2] PRESENTATION FORM FOR VERTICAL COLON..PRESENTATION FORM FOR VERTICAL SEMICOLON
- {0xFE15, 0xFE16, prEX, gcPo}, // [2] PRESENTATION FORM FOR VERTICAL EXCLAMATION MARK..PRESENTATION FORM FOR VERTICAL QUESTION MARK
- {0xFE17, 0xFE17, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT WHITE LENTICULAR BRACKET
- {0xFE18, 0xFE18, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT WHITE LENTICULAR BRAKCET
- {0xFE19, 0xFE19, prIN, gcPo}, // PRESENTATION FORM FOR VERTICAL HORIZONTAL ELLIPSIS
- {0xFE20, 0xFE2F, prCM, gcMn}, // [16] COMBINING LIGATURE LEFT HALF..COMBINING CYRILLIC TITLO RIGHT HALF
- {0xFE30, 0xFE30, prID, gcPo}, // PRESENTATION FORM FOR VERTICAL TWO DOT LEADER
- {0xFE31, 0xFE32, prID, gcPd}, // [2] PRESENTATION FORM FOR VERTICAL EM DASH..PRESENTATION FORM FOR VERTICAL EN DASH
- {0xFE33, 0xFE34, prID, gcPc}, // [2] PRESENTATION FORM FOR VERTICAL LOW LINE..PRESENTATION FORM FOR VERTICAL WAVY LOW LINE
- {0xFE35, 0xFE35, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT PARENTHESIS
- {0xFE36, 0xFE36, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT PARENTHESIS
- {0xFE37, 0xFE37, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT CURLY BRACKET
- {0xFE38, 0xFE38, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT CURLY BRACKET
- {0xFE39, 0xFE39, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT TORTOISE SHELL BRACKET
- {0xFE3A, 0xFE3A, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT TORTOISE SHELL BRACKET
- {0xFE3B, 0xFE3B, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT BLACK LENTICULAR BRACKET
- {0xFE3C, 0xFE3C, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT BLACK LENTICULAR BRACKET
- {0xFE3D, 0xFE3D, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT DOUBLE ANGLE BRACKET
- {0xFE3E, 0xFE3E, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT DOUBLE ANGLE BRACKET
- {0xFE3F, 0xFE3F, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT ANGLE BRACKET
- {0xFE40, 0xFE40, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT ANGLE BRACKET
- {0xFE41, 0xFE41, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT CORNER BRACKET
- {0xFE42, 0xFE42, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT CORNER BRACKET
- {0xFE43, 0xFE43, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT WHITE CORNER BRACKET
- {0xFE44, 0xFE44, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT WHITE CORNER BRACKET
- {0xFE45, 0xFE46, prID, gcPo}, // [2] SESAME DOT..WHITE SESAME DOT
- {0xFE47, 0xFE47, prOP, gcPs}, // PRESENTATION FORM FOR VERTICAL LEFT SQUARE BRACKET
- {0xFE48, 0xFE48, prCL, gcPe}, // PRESENTATION FORM FOR VERTICAL RIGHT SQUARE BRACKET
- {0xFE49, 0xFE4C, prID, gcPo}, // [4] DASHED OVERLINE..DOUBLE WAVY OVERLINE
- {0xFE4D, 0xFE4F, prID, gcPc}, // [3] DASHED LOW LINE..WAVY LOW LINE
- {0xFE50, 0xFE50, prCL, gcPo}, // SMALL COMMA
- {0xFE51, 0xFE51, prID, gcPo}, // SMALL IDEOGRAPHIC COMMA
- {0xFE52, 0xFE52, prCL, gcPo}, // SMALL FULL STOP
- {0xFE54, 0xFE55, prNS, gcPo}, // [2] SMALL SEMICOLON..SMALL COLON
- {0xFE56, 0xFE57, prEX, gcPo}, // [2] SMALL QUESTION MARK..SMALL EXCLAMATION MARK
- {0xFE58, 0xFE58, prID, gcPd}, // SMALL EM DASH
- {0xFE59, 0xFE59, prOP, gcPs}, // SMALL LEFT PARENTHESIS
- {0xFE5A, 0xFE5A, prCL, gcPe}, // SMALL RIGHT PARENTHESIS
- {0xFE5B, 0xFE5B, prOP, gcPs}, // SMALL LEFT CURLY BRACKET
- {0xFE5C, 0xFE5C, prCL, gcPe}, // SMALL RIGHT CURLY BRACKET
- {0xFE5D, 0xFE5D, prOP, gcPs}, // SMALL LEFT TORTOISE SHELL BRACKET
- {0xFE5E, 0xFE5E, prCL, gcPe}, // SMALL RIGHT TORTOISE SHELL BRACKET
- {0xFE5F, 0xFE61, prID, gcPo}, // [3] SMALL NUMBER SIGN..SMALL ASTERISK
- {0xFE62, 0xFE62, prID, gcSm}, // SMALL PLUS SIGN
- {0xFE63, 0xFE63, prID, gcPd}, // SMALL HYPHEN-MINUS
- {0xFE64, 0xFE66, prID, gcSm}, // [3] SMALL LESS-THAN SIGN..SMALL EQUALS SIGN
- {0xFE68, 0xFE68, prID, gcPo}, // SMALL REVERSE SOLIDUS
- {0xFE69, 0xFE69, prPR, gcSc}, // SMALL DOLLAR SIGN
- {0xFE6A, 0xFE6A, prPO, gcPo}, // SMALL PERCENT SIGN
- {0xFE6B, 0xFE6B, prID, gcPo}, // SMALL COMMERCIAL AT
- {0xFE70, 0xFE74, prAL, gcLo}, // [5] ARABIC FATHATAN ISOLATED FORM..ARABIC KASRATAN ISOLATED FORM
- {0xFE76, 0xFEFC, prAL, gcLo}, // [135] ARABIC FATHA ISOLATED FORM..ARABIC LIGATURE LAM WITH ALEF FINAL FORM
- {0xFEFF, 0xFEFF, prWJ, gcCf}, // ZERO WIDTH NO-BREAK SPACE
- {0xFF01, 0xFF01, prEX, gcPo}, // FULLWIDTH EXCLAMATION MARK
- {0xFF02, 0xFF03, prID, gcPo}, // [2] FULLWIDTH QUOTATION MARK..FULLWIDTH NUMBER SIGN
- {0xFF04, 0xFF04, prPR, gcSc}, // FULLWIDTH DOLLAR SIGN
- {0xFF05, 0xFF05, prPO, gcPo}, // FULLWIDTH PERCENT SIGN
- {0xFF06, 0xFF07, prID, gcPo}, // [2] FULLWIDTH AMPERSAND..FULLWIDTH APOSTROPHE
- {0xFF08, 0xFF08, prOP, gcPs}, // FULLWIDTH LEFT PARENTHESIS
- {0xFF09, 0xFF09, prCL, gcPe}, // FULLWIDTH RIGHT PARENTHESIS
- {0xFF0A, 0xFF0A, prID, gcPo}, // FULLWIDTH ASTERISK
- {0xFF0B, 0xFF0B, prID, gcSm}, // FULLWIDTH PLUS SIGN
- {0xFF0C, 0xFF0C, prCL, gcPo}, // FULLWIDTH COMMA
- {0xFF0D, 0xFF0D, prID, gcPd}, // FULLWIDTH HYPHEN-MINUS
- {0xFF0E, 0xFF0E, prCL, gcPo}, // FULLWIDTH FULL STOP
- {0xFF0F, 0xFF0F, prID, gcPo}, // FULLWIDTH SOLIDUS
- {0xFF10, 0xFF19, prID, gcNd}, // [10] FULLWIDTH DIGIT ZERO..FULLWIDTH DIGIT NINE
- {0xFF1A, 0xFF1B, prNS, gcPo}, // [2] FULLWIDTH COLON..FULLWIDTH SEMICOLON
- {0xFF1C, 0xFF1E, prID, gcSm}, // [3] FULLWIDTH LESS-THAN SIGN..FULLWIDTH GREATER-THAN SIGN
- {0xFF1F, 0xFF1F, prEX, gcPo}, // FULLWIDTH QUESTION MARK
- {0xFF20, 0xFF20, prID, gcPo}, // FULLWIDTH COMMERCIAL AT
- {0xFF21, 0xFF3A, prID, gcLu}, // [26] FULLWIDTH LATIN CAPITAL LETTER A..FULLWIDTH LATIN CAPITAL LETTER Z
- {0xFF3B, 0xFF3B, prOP, gcPs}, // FULLWIDTH LEFT SQUARE BRACKET
- {0xFF3C, 0xFF3C, prID, gcPo}, // FULLWIDTH REVERSE SOLIDUS
- {0xFF3D, 0xFF3D, prCL, gcPe}, // FULLWIDTH RIGHT SQUARE BRACKET
- {0xFF3E, 0xFF3E, prID, gcSk}, // FULLWIDTH CIRCUMFLEX ACCENT
- {0xFF3F, 0xFF3F, prID, gcPc}, // FULLWIDTH LOW LINE
- {0xFF40, 0xFF40, prID, gcSk}, // FULLWIDTH GRAVE ACCENT
- {0xFF41, 0xFF5A, prID, gcLl}, // [26] FULLWIDTH LATIN SMALL LETTER A..FULLWIDTH LATIN SMALL LETTER Z
- {0xFF5B, 0xFF5B, prOP, gcPs}, // FULLWIDTH LEFT CURLY BRACKET
- {0xFF5C, 0xFF5C, prID, gcSm}, // FULLWIDTH VERTICAL LINE
- {0xFF5D, 0xFF5D, prCL, gcPe}, // FULLWIDTH RIGHT CURLY BRACKET
- {0xFF5E, 0xFF5E, prID, gcSm}, // FULLWIDTH TILDE
- {0xFF5F, 0xFF5F, prOP, gcPs}, // FULLWIDTH LEFT WHITE PARENTHESIS
- {0xFF60, 0xFF60, prCL, gcPe}, // FULLWIDTH RIGHT WHITE PARENTHESIS
- {0xFF61, 0xFF61, prCL, gcPo}, // HALFWIDTH IDEOGRAPHIC FULL STOP
- {0xFF62, 0xFF62, prOP, gcPs}, // HALFWIDTH LEFT CORNER BRACKET
- {0xFF63, 0xFF63, prCL, gcPe}, // HALFWIDTH RIGHT CORNER BRACKET
- {0xFF64, 0xFF64, prCL, gcPo}, // HALFWIDTH IDEOGRAPHIC COMMA
- {0xFF65, 0xFF65, prNS, gcPo}, // HALFWIDTH KATAKANA MIDDLE DOT
- {0xFF66, 0xFF66, prID, gcLo}, // HALFWIDTH KATAKANA LETTER WO
- {0xFF67, 0xFF6F, prCJ, gcLo}, // [9] HALFWIDTH KATAKANA LETTER SMALL A..HALFWIDTH KATAKANA LETTER SMALL TU
- {0xFF70, 0xFF70, prCJ, gcLm}, // HALFWIDTH KATAKANA-HIRAGANA PROLONGED SOUND MARK
- {0xFF71, 0xFF9D, prID, gcLo}, // [45] HALFWIDTH KATAKANA LETTER A..HALFWIDTH KATAKANA LETTER N
- {0xFF9E, 0xFF9F, prNS, gcLm}, // [2] HALFWIDTH KATAKANA VOICED SOUND MARK..HALFWIDTH KATAKANA SEMI-VOICED SOUND MARK
- {0xFFA0, 0xFFBE, prID, gcLo}, // [31] HALFWIDTH HANGUL FILLER..HALFWIDTH HANGUL LETTER HIEUH
- {0xFFC2, 0xFFC7, prID, gcLo}, // [6] HALFWIDTH HANGUL LETTER A..HALFWIDTH HANGUL LETTER E
- {0xFFCA, 0xFFCF, prID, gcLo}, // [6] HALFWIDTH HANGUL LETTER YEO..HALFWIDTH HANGUL LETTER OE
- {0xFFD2, 0xFFD7, prID, gcLo}, // [6] HALFWIDTH HANGUL LETTER YO..HALFWIDTH HANGUL LETTER YU
- {0xFFDA, 0xFFDC, prID, gcLo}, // [3] HALFWIDTH HANGUL LETTER EU..HALFWIDTH HANGUL LETTER I
- {0xFFE0, 0xFFE0, prPO, gcSc}, // FULLWIDTH CENT SIGN
- {0xFFE1, 0xFFE1, prPR, gcSc}, // FULLWIDTH POUND SIGN
- {0xFFE2, 0xFFE2, prID, gcSm}, // FULLWIDTH NOT SIGN
- {0xFFE3, 0xFFE3, prID, gcSk}, // FULLWIDTH MACRON
- {0xFFE4, 0xFFE4, prID, gcSo}, // FULLWIDTH BROKEN BAR
- {0xFFE5, 0xFFE6, prPR, gcSc}, // [2] FULLWIDTH YEN SIGN..FULLWIDTH WON SIGN
- {0xFFE8, 0xFFE8, prAL, gcSo}, // HALFWIDTH FORMS LIGHT VERTICAL
- {0xFFE9, 0xFFEC, prAL, gcSm}, // [4] HALFWIDTH LEFTWARDS ARROW..HALFWIDTH DOWNWARDS ARROW
- {0xFFED, 0xFFEE, prAL, gcSo}, // [2] HALFWIDTH BLACK SQUARE..HALFWIDTH WHITE CIRCLE
- {0xFFF9, 0xFFFB, prCM, gcCf}, // [3] INTERLINEAR ANNOTATION ANCHOR..INTERLINEAR ANNOTATION TERMINATOR
- {0xFFFC, 0xFFFC, prCB, gcSo}, // OBJECT REPLACEMENT CHARACTER
- {0xFFFD, 0xFFFD, prAI, gcSo}, // REPLACEMENT CHARACTER
- {0x10000, 0x1000B, prAL, gcLo}, // [12] LINEAR B SYLLABLE B008 A..LINEAR B SYLLABLE B046 JE
- {0x1000D, 0x10026, prAL, gcLo}, // [26] LINEAR B SYLLABLE B036 JO..LINEAR B SYLLABLE B032 QO
- {0x10028, 0x1003A, prAL, gcLo}, // [19] LINEAR B SYLLABLE B060 RA..LINEAR B SYLLABLE B042 WO
- {0x1003C, 0x1003D, prAL, gcLo}, // [2] LINEAR B SYLLABLE B017 ZA..LINEAR B SYLLABLE B074 ZE
- {0x1003F, 0x1004D, prAL, gcLo}, // [15] LINEAR B SYLLABLE B020 ZO..LINEAR B SYLLABLE B091 TWO
- {0x10050, 0x1005D, prAL, gcLo}, // [14] LINEAR B SYMBOL B018..LINEAR B SYMBOL B089
- {0x10080, 0x100FA, prAL, gcLo}, // [123] LINEAR B IDEOGRAM B100 MAN..LINEAR B IDEOGRAM VESSEL B305
- {0x10100, 0x10102, prBA, gcPo}, // [3] AEGEAN WORD SEPARATOR LINE..AEGEAN CHECK MARK
- {0x10107, 0x10133, prAL, gcNo}, // [45] AEGEAN NUMBER ONE..AEGEAN NUMBER NINETY THOUSAND
- {0x10137, 0x1013F, prAL, gcSo}, // [9] AEGEAN WEIGHT BASE UNIT..AEGEAN MEASURE THIRD SUBUNIT
- {0x10140, 0x10174, prAL, gcNl}, // [53] GREEK ACROPHONIC ATTIC ONE QUARTER..GREEK ACROPHONIC STRATIAN FIFTY MNAS
- {0x10175, 0x10178, prAL, gcNo}, // [4] GREEK ONE HALF SIGN..GREEK THREE QUARTERS SIGN
- {0x10179, 0x10189, prAL, gcSo}, // [17] GREEK YEAR SIGN..GREEK TRYBLION BASE SIGN
- {0x1018A, 0x1018B, prAL, gcNo}, // [2] GREEK ZERO SIGN..GREEK ONE QUARTER SIGN
- {0x1018C, 0x1018E, prAL, gcSo}, // [3] GREEK SINUSOID SIGN..NOMISMA SIGN
- {0x10190, 0x1019C, prAL, gcSo}, // [13] ROMAN SEXTANS SIGN..ASCIA SYMBOL
- {0x101A0, 0x101A0, prAL, gcSo}, // GREEK SYMBOL TAU RHO
- {0x101D0, 0x101FC, prAL, gcSo}, // [45] PHAISTOS DISC SIGN PEDESTRIAN..PHAISTOS DISC SIGN WAVY BAND
- {0x101FD, 0x101FD, prCM, gcMn}, // PHAISTOS DISC SIGN COMBINING OBLIQUE STROKE
- {0x10280, 0x1029C, prAL, gcLo}, // [29] LYCIAN LETTER A..LYCIAN LETTER X
- {0x102A0, 0x102D0, prAL, gcLo}, // [49] CARIAN LETTER A..CARIAN LETTER UUU3
- {0x102E0, 0x102E0, prCM, gcMn}, // COPTIC EPACT THOUSANDS MARK
- {0x102E1, 0x102FB, prAL, gcNo}, // [27] COPTIC EPACT DIGIT ONE..COPTIC EPACT NUMBER NINE HUNDRED
- {0x10300, 0x1031F, prAL, gcLo}, // [32] OLD ITALIC LETTER A..OLD ITALIC LETTER ESS
- {0x10320, 0x10323, prAL, gcNo}, // [4] OLD ITALIC NUMERAL ONE..OLD ITALIC NUMERAL FIFTY
- {0x1032D, 0x1032F, prAL, gcLo}, // [3] OLD ITALIC LETTER YE..OLD ITALIC LETTER SOUTHERN TSE
- {0x10330, 0x10340, prAL, gcLo}, // [17] GOTHIC LETTER AHSA..GOTHIC LETTER PAIRTHRA
- {0x10341, 0x10341, prAL, gcNl}, // GOTHIC LETTER NINETY
- {0x10342, 0x10349, prAL, gcLo}, // [8] GOTHIC LETTER RAIDA..GOTHIC LETTER OTHAL
- {0x1034A, 0x1034A, prAL, gcNl}, // GOTHIC LETTER NINE HUNDRED
- {0x10350, 0x10375, prAL, gcLo}, // [38] OLD PERMIC LETTER AN..OLD PERMIC LETTER IA
- {0x10376, 0x1037A, prCM, gcMn}, // [5] COMBINING OLD PERMIC LETTER AN..COMBINING OLD PERMIC LETTER SII
- {0x10380, 0x1039D, prAL, gcLo}, // [30] UGARITIC LETTER ALPA..UGARITIC LETTER SSU
- {0x1039F, 0x1039F, prBA, gcPo}, // UGARITIC WORD DIVIDER
- {0x103A0, 0x103C3, prAL, gcLo}, // [36] OLD PERSIAN SIGN A..OLD PERSIAN SIGN HA
- {0x103C8, 0x103CF, prAL, gcLo}, // [8] OLD PERSIAN SIGN AURAMAZDAA..OLD PERSIAN SIGN BUUMISH
- {0x103D0, 0x103D0, prBA, gcPo}, // OLD PERSIAN WORD DIVIDER
- {0x103D1, 0x103D5, prAL, gcNl}, // [5] OLD PERSIAN NUMBER ONE..OLD PERSIAN NUMBER HUNDRED
- {0x10400, 0x1044F, prAL, gcLC}, // [80] DESERET CAPITAL LETTER LONG I..DESERET SMALL LETTER EW
- {0x10450, 0x1047F, prAL, gcLo}, // [48] SHAVIAN LETTER PEEP..SHAVIAN LETTER YEW
- {0x10480, 0x1049D, prAL, gcLo}, // [30] OSMANYA LETTER ALEF..OSMANYA LETTER OO
- {0x104A0, 0x104A9, prNU, gcNd}, // [10] OSMANYA DIGIT ZERO..OSMANYA DIGIT NINE
- {0x104B0, 0x104D3, prAL, gcLu}, // [36] OSAGE CAPITAL LETTER A..OSAGE CAPITAL LETTER ZHA
- {0x104D8, 0x104FB, prAL, gcLl}, // [36] OSAGE SMALL LETTER A..OSAGE SMALL LETTER ZHA
- {0x10500, 0x10527, prAL, gcLo}, // [40] ELBASAN LETTER A..ELBASAN LETTER KHE
- {0x10530, 0x10563, prAL, gcLo}, // [52] CAUCASIAN ALBANIAN LETTER ALT..CAUCASIAN ALBANIAN LETTER KIW
- {0x1056F, 0x1056F, prAL, gcPo}, // CAUCASIAN ALBANIAN CITATION MARK
- {0x10570, 0x1057A, prAL, gcLu}, // [11] VITHKUQI CAPITAL LETTER A..VITHKUQI CAPITAL LETTER GA
- {0x1057C, 0x1058A, prAL, gcLu}, // [15] VITHKUQI CAPITAL LETTER HA..VITHKUQI CAPITAL LETTER RE
- {0x1058C, 0x10592, prAL, gcLu}, // [7] VITHKUQI CAPITAL LETTER SE..VITHKUQI CAPITAL LETTER XE
- {0x10594, 0x10595, prAL, gcLu}, // [2] VITHKUQI CAPITAL LETTER Y..VITHKUQI CAPITAL LETTER ZE
- {0x10597, 0x105A1, prAL, gcLl}, // [11] VITHKUQI SMALL LETTER A..VITHKUQI SMALL LETTER GA
- {0x105A3, 0x105B1, prAL, gcLl}, // [15] VITHKUQI SMALL LETTER HA..VITHKUQI SMALL LETTER RE
- {0x105B3, 0x105B9, prAL, gcLl}, // [7] VITHKUQI SMALL LETTER SE..VITHKUQI SMALL LETTER XE
- {0x105BB, 0x105BC, prAL, gcLl}, // [2] VITHKUQI SMALL LETTER Y..VITHKUQI SMALL LETTER ZE
- {0x10600, 0x10736, prAL, gcLo}, // [311] LINEAR A SIGN AB001..LINEAR A SIGN A664
- {0x10740, 0x10755, prAL, gcLo}, // [22] LINEAR A SIGN A701 A..LINEAR A SIGN A732 JE
- {0x10760, 0x10767, prAL, gcLo}, // [8] LINEAR A SIGN A800..LINEAR A SIGN A807
- {0x10780, 0x10785, prAL, gcLm}, // [6] MODIFIER LETTER SMALL CAPITAL AA..MODIFIER LETTER SMALL B WITH HOOK
- {0x10787, 0x107B0, prAL, gcLm}, // [42] MODIFIER LETTER SMALL DZ DIGRAPH..MODIFIER LETTER SMALL V WITH RIGHT HOOK
- {0x107B2, 0x107BA, prAL, gcLm}, // [9] MODIFIER LETTER SMALL CAPITAL Y..MODIFIER LETTER SMALL S WITH CURL
- {0x10800, 0x10805, prAL, gcLo}, // [6] CYPRIOT SYLLABLE A..CYPRIOT SYLLABLE JA
- {0x10808, 0x10808, prAL, gcLo}, // CYPRIOT SYLLABLE JO
- {0x1080A, 0x10835, prAL, gcLo}, // [44] CYPRIOT SYLLABLE KA..CYPRIOT SYLLABLE WO
- {0x10837, 0x10838, prAL, gcLo}, // [2] CYPRIOT SYLLABLE XA..CYPRIOT SYLLABLE XE
- {0x1083C, 0x1083C, prAL, gcLo}, // CYPRIOT SYLLABLE ZA
- {0x1083F, 0x1083F, prAL, gcLo}, // CYPRIOT SYLLABLE ZO
- {0x10840, 0x10855, prAL, gcLo}, // [22] IMPERIAL ARAMAIC LETTER ALEPH..IMPERIAL ARAMAIC LETTER TAW
- {0x10857, 0x10857, prBA, gcPo}, // IMPERIAL ARAMAIC SECTION SIGN
- {0x10858, 0x1085F, prAL, gcNo}, // [8] IMPERIAL ARAMAIC NUMBER ONE..IMPERIAL ARAMAIC NUMBER TEN THOUSAND
- {0x10860, 0x10876, prAL, gcLo}, // [23] PALMYRENE LETTER ALEPH..PALMYRENE LETTER TAW
- {0x10877, 0x10878, prAL, gcSo}, // [2] PALMYRENE LEFT-POINTING FLEURON..PALMYRENE RIGHT-POINTING FLEURON
- {0x10879, 0x1087F, prAL, gcNo}, // [7] PALMYRENE NUMBER ONE..PALMYRENE NUMBER TWENTY
- {0x10880, 0x1089E, prAL, gcLo}, // [31] NABATAEAN LETTER FINAL ALEPH..NABATAEAN LETTER TAW
- {0x108A7, 0x108AF, prAL, gcNo}, // [9] NABATAEAN NUMBER ONE..NABATAEAN NUMBER ONE HUNDRED
- {0x108E0, 0x108F2, prAL, gcLo}, // [19] HATRAN LETTER ALEPH..HATRAN LETTER QOPH
- {0x108F4, 0x108F5, prAL, gcLo}, // [2] HATRAN LETTER SHIN..HATRAN LETTER TAW
- {0x108FB, 0x108FF, prAL, gcNo}, // [5] HATRAN NUMBER ONE..HATRAN NUMBER ONE HUNDRED
- {0x10900, 0x10915, prAL, gcLo}, // [22] PHOENICIAN LETTER ALF..PHOENICIAN LETTER TAU
- {0x10916, 0x1091B, prAL, gcNo}, // [6] PHOENICIAN NUMBER ONE..PHOENICIAN NUMBER THREE
- {0x1091F, 0x1091F, prBA, gcPo}, // PHOENICIAN WORD SEPARATOR
- {0x10920, 0x10939, prAL, gcLo}, // [26] LYDIAN LETTER A..LYDIAN LETTER C
- {0x1093F, 0x1093F, prAL, gcPo}, // LYDIAN TRIANGULAR MARK
- {0x10980, 0x1099F, prAL, gcLo}, // [32] MEROITIC HIEROGLYPHIC LETTER A..MEROITIC HIEROGLYPHIC SYMBOL VIDJ-2
- {0x109A0, 0x109B7, prAL, gcLo}, // [24] MEROITIC CURSIVE LETTER A..MEROITIC CURSIVE LETTER DA
- {0x109BC, 0x109BD, prAL, gcNo}, // [2] MEROITIC CURSIVE FRACTION ELEVEN TWELFTHS..MEROITIC CURSIVE FRACTION ONE HALF
- {0x109BE, 0x109BF, prAL, gcLo}, // [2] MEROITIC CURSIVE LOGOGRAM RMT..MEROITIC CURSIVE LOGOGRAM IMN
- {0x109C0, 0x109CF, prAL, gcNo}, // [16] MEROITIC CURSIVE NUMBER ONE..MEROITIC CURSIVE NUMBER SEVENTY
- {0x109D2, 0x109FF, prAL, gcNo}, // [46] MEROITIC CURSIVE NUMBER ONE HUNDRED..MEROITIC CURSIVE FRACTION TEN TWELFTHS
- {0x10A00, 0x10A00, prAL, gcLo}, // KHAROSHTHI LETTER A
- {0x10A01, 0x10A03, prCM, gcMn}, // [3] KHAROSHTHI VOWEL SIGN I..KHAROSHTHI VOWEL SIGN VOCALIC R
- {0x10A05, 0x10A06, prCM, gcMn}, // [2] KHAROSHTHI VOWEL SIGN E..KHAROSHTHI VOWEL SIGN O
- {0x10A0C, 0x10A0F, prCM, gcMn}, // [4] KHAROSHTHI VOWEL LENGTH MARK..KHAROSHTHI SIGN VISARGA
- {0x10A10, 0x10A13, prAL, gcLo}, // [4] KHAROSHTHI LETTER KA..KHAROSHTHI LETTER GHA
- {0x10A15, 0x10A17, prAL, gcLo}, // [3] KHAROSHTHI LETTER CA..KHAROSHTHI LETTER JA
- {0x10A19, 0x10A35, prAL, gcLo}, // [29] KHAROSHTHI LETTER NYA..KHAROSHTHI LETTER VHA
- {0x10A38, 0x10A3A, prCM, gcMn}, // [3] KHAROSHTHI SIGN BAR ABOVE..KHAROSHTHI SIGN DOT BELOW
- {0x10A3F, 0x10A3F, prCM, gcMn}, // KHAROSHTHI VIRAMA
- {0x10A40, 0x10A48, prAL, gcNo}, // [9] KHAROSHTHI DIGIT ONE..KHAROSHTHI FRACTION ONE HALF
- {0x10A50, 0x10A57, prBA, gcPo}, // [8] KHAROSHTHI PUNCTUATION DOT..KHAROSHTHI PUNCTUATION DOUBLE DANDA
- {0x10A58, 0x10A58, prAL, gcPo}, // KHAROSHTHI PUNCTUATION LINES
- {0x10A60, 0x10A7C, prAL, gcLo}, // [29] OLD SOUTH ARABIAN LETTER HE..OLD SOUTH ARABIAN LETTER THETH
- {0x10A7D, 0x10A7E, prAL, gcNo}, // [2] OLD SOUTH ARABIAN NUMBER ONE..OLD SOUTH ARABIAN NUMBER FIFTY
- {0x10A7F, 0x10A7F, prAL, gcPo}, // OLD SOUTH ARABIAN NUMERIC INDICATOR
- {0x10A80, 0x10A9C, prAL, gcLo}, // [29] OLD NORTH ARABIAN LETTER HEH..OLD NORTH ARABIAN LETTER ZAH
- {0x10A9D, 0x10A9F, prAL, gcNo}, // [3] OLD NORTH ARABIAN NUMBER ONE..OLD NORTH ARABIAN NUMBER TWENTY
- {0x10AC0, 0x10AC7, prAL, gcLo}, // [8] MANICHAEAN LETTER ALEPH..MANICHAEAN LETTER WAW
- {0x10AC8, 0x10AC8, prAL, gcSo}, // MANICHAEAN SIGN UD
- {0x10AC9, 0x10AE4, prAL, gcLo}, // [28] MANICHAEAN LETTER ZAYIN..MANICHAEAN LETTER TAW
- {0x10AE5, 0x10AE6, prCM, gcMn}, // [2] MANICHAEAN ABBREVIATION MARK ABOVE..MANICHAEAN ABBREVIATION MARK BELOW
- {0x10AEB, 0x10AEF, prAL, gcNo}, // [5] MANICHAEAN NUMBER ONE..MANICHAEAN NUMBER ONE HUNDRED
- {0x10AF0, 0x10AF5, prBA, gcPo}, // [6] MANICHAEAN PUNCTUATION STAR..MANICHAEAN PUNCTUATION TWO DOTS
- {0x10AF6, 0x10AF6, prIN, gcPo}, // MANICHAEAN PUNCTUATION LINE FILLER
- {0x10B00, 0x10B35, prAL, gcLo}, // [54] AVESTAN LETTER A..AVESTAN LETTER HE
- {0x10B39, 0x10B3F, prBA, gcPo}, // [7] AVESTAN ABBREVIATION MARK..LARGE ONE RING OVER TWO RINGS PUNCTUATION
- {0x10B40, 0x10B55, prAL, gcLo}, // [22] INSCRIPTIONAL PARTHIAN LETTER ALEPH..INSCRIPTIONAL PARTHIAN LETTER TAW
- {0x10B58, 0x10B5F, prAL, gcNo}, // [8] INSCRIPTIONAL PARTHIAN NUMBER ONE..INSCRIPTIONAL PARTHIAN NUMBER ONE THOUSAND
- {0x10B60, 0x10B72, prAL, gcLo}, // [19] INSCRIPTIONAL PAHLAVI LETTER ALEPH..INSCRIPTIONAL PAHLAVI LETTER TAW
- {0x10B78, 0x10B7F, prAL, gcNo}, // [8] INSCRIPTIONAL PAHLAVI NUMBER ONE..INSCRIPTIONAL PAHLAVI NUMBER ONE THOUSAND
- {0x10B80, 0x10B91, prAL, gcLo}, // [18] PSALTER PAHLAVI LETTER ALEPH..PSALTER PAHLAVI LETTER TAW
- {0x10B99, 0x10B9C, prAL, gcPo}, // [4] PSALTER PAHLAVI SECTION MARK..PSALTER PAHLAVI FOUR DOTS WITH DOT
- {0x10BA9, 0x10BAF, prAL, gcNo}, // [7] PSALTER PAHLAVI NUMBER ONE..PSALTER PAHLAVI NUMBER ONE HUNDRED
- {0x10C00, 0x10C48, prAL, gcLo}, // [73] OLD TURKIC LETTER ORKHON A..OLD TURKIC LETTER ORKHON BASH
- {0x10C80, 0x10CB2, prAL, gcLu}, // [51] OLD HUNGARIAN CAPITAL LETTER A..OLD HUNGARIAN CAPITAL LETTER US
- {0x10CC0, 0x10CF2, prAL, gcLl}, // [51] OLD HUNGARIAN SMALL LETTER A..OLD HUNGARIAN SMALL LETTER US
- {0x10CFA, 0x10CFF, prAL, gcNo}, // [6] OLD HUNGARIAN NUMBER ONE..OLD HUNGARIAN NUMBER ONE THOUSAND
- {0x10D00, 0x10D23, prAL, gcLo}, // [36] HANIFI ROHINGYA LETTER A..HANIFI ROHINGYA MARK NA KHONNA
- {0x10D24, 0x10D27, prCM, gcMn}, // [4] HANIFI ROHINGYA SIGN HARBAHAY..HANIFI ROHINGYA SIGN TASSI
- {0x10D30, 0x10D39, prNU, gcNd}, // [10] HANIFI ROHINGYA DIGIT ZERO..HANIFI ROHINGYA DIGIT NINE
- {0x10E60, 0x10E7E, prAL, gcNo}, // [31] RUMI DIGIT ONE..RUMI FRACTION TWO THIRDS
- {0x10E80, 0x10EA9, prAL, gcLo}, // [42] YEZIDI LETTER ELIF..YEZIDI LETTER ET
- {0x10EAB, 0x10EAC, prCM, gcMn}, // [2] YEZIDI COMBINING HAMZA MARK..YEZIDI COMBINING MADDA MARK
- {0x10EAD, 0x10EAD, prBA, gcPd}, // YEZIDI HYPHENATION MARK
- {0x10EB0, 0x10EB1, prAL, gcLo}, // [2] YEZIDI LETTER LAM WITH DOT ABOVE..YEZIDI LETTER YOT WITH CIRCUMFLEX ABOVE
- {0x10F00, 0x10F1C, prAL, gcLo}, // [29] OLD SOGDIAN LETTER ALEPH..OLD SOGDIAN LETTER FINAL TAW WITH VERTICAL TAIL
- {0x10F1D, 0x10F26, prAL, gcNo}, // [10] OLD SOGDIAN NUMBER ONE..OLD SOGDIAN FRACTION ONE HALF
- {0x10F27, 0x10F27, prAL, gcLo}, // OLD SOGDIAN LIGATURE AYIN-DALETH
- {0x10F30, 0x10F45, prAL, gcLo}, // [22] SOGDIAN LETTER ALEPH..SOGDIAN INDEPENDENT SHIN
- {0x10F46, 0x10F50, prCM, gcMn}, // [11] SOGDIAN COMBINING DOT BELOW..SOGDIAN COMBINING STROKE BELOW
- {0x10F51, 0x10F54, prAL, gcNo}, // [4] SOGDIAN NUMBER ONE..SOGDIAN NUMBER ONE HUNDRED
- {0x10F55, 0x10F59, prAL, gcPo}, // [5] SOGDIAN PUNCTUATION TWO VERTICAL BARS..SOGDIAN PUNCTUATION HALF CIRCLE WITH DOT
- {0x10F70, 0x10F81, prAL, gcLo}, // [18] OLD UYGHUR LETTER ALEPH..OLD UYGHUR LETTER LESH
- {0x10F82, 0x10F85, prCM, gcMn}, // [4] OLD UYGHUR COMBINING DOT ABOVE..OLD UYGHUR COMBINING TWO DOTS BELOW
- {0x10F86, 0x10F89, prAL, gcPo}, // [4] OLD UYGHUR PUNCTUATION BAR..OLD UYGHUR PUNCTUATION FOUR DOTS
- {0x10FB0, 0x10FC4, prAL, gcLo}, // [21] CHORASMIAN LETTER ALEPH..CHORASMIAN LETTER TAW
- {0x10FC5, 0x10FCB, prAL, gcNo}, // [7] CHORASMIAN NUMBER ONE..CHORASMIAN NUMBER ONE HUNDRED
- {0x10FE0, 0x10FF6, prAL, gcLo}, // [23] ELYMAIC LETTER ALEPH..ELYMAIC LIGATURE ZAYIN-YODH
- {0x11000, 0x11000, prCM, gcMc}, // BRAHMI SIGN CANDRABINDU
- {0x11001, 0x11001, prCM, gcMn}, // BRAHMI SIGN ANUSVARA
- {0x11002, 0x11002, prCM, gcMc}, // BRAHMI SIGN VISARGA
- {0x11003, 0x11037, prAL, gcLo}, // [53] BRAHMI SIGN JIHVAMULIYA..BRAHMI LETTER OLD TAMIL NNNA
- {0x11038, 0x11046, prCM, gcMn}, // [15] BRAHMI VOWEL SIGN AA..BRAHMI VIRAMA
- {0x11047, 0x11048, prBA, gcPo}, // [2] BRAHMI DANDA..BRAHMI DOUBLE DANDA
- {0x11049, 0x1104D, prAL, gcPo}, // [5] BRAHMI PUNCTUATION DOT..BRAHMI PUNCTUATION LOTUS
- {0x11052, 0x11065, prAL, gcNo}, // [20] BRAHMI NUMBER ONE..BRAHMI NUMBER ONE THOUSAND
- {0x11066, 0x1106F, prNU, gcNd}, // [10] BRAHMI DIGIT ZERO..BRAHMI DIGIT NINE
- {0x11070, 0x11070, prCM, gcMn}, // BRAHMI SIGN OLD TAMIL VIRAMA
- {0x11071, 0x11072, prAL, gcLo}, // [2] BRAHMI LETTER OLD TAMIL SHORT E..BRAHMI LETTER OLD TAMIL SHORT O
- {0x11073, 0x11074, prCM, gcMn}, // [2] BRAHMI VOWEL SIGN OLD TAMIL SHORT E..BRAHMI VOWEL SIGN OLD TAMIL SHORT O
- {0x11075, 0x11075, prAL, gcLo}, // BRAHMI LETTER OLD TAMIL LLA
- {0x1107F, 0x1107F, prCM, gcMn}, // BRAHMI NUMBER JOINER
- {0x11080, 0x11081, prCM, gcMn}, // [2] KAITHI SIGN CANDRABINDU..KAITHI SIGN ANUSVARA
- {0x11082, 0x11082, prCM, gcMc}, // KAITHI SIGN VISARGA
- {0x11083, 0x110AF, prAL, gcLo}, // [45] KAITHI LETTER A..KAITHI LETTER HA
- {0x110B0, 0x110B2, prCM, gcMc}, // [3] KAITHI VOWEL SIGN AA..KAITHI VOWEL SIGN II
- {0x110B3, 0x110B6, prCM, gcMn}, // [4] KAITHI VOWEL SIGN U..KAITHI VOWEL SIGN AI
- {0x110B7, 0x110B8, prCM, gcMc}, // [2] KAITHI VOWEL SIGN O..KAITHI VOWEL SIGN AU
- {0x110B9, 0x110BA, prCM, gcMn}, // [2] KAITHI SIGN VIRAMA..KAITHI SIGN NUKTA
- {0x110BB, 0x110BC, prAL, gcPo}, // [2] KAITHI ABBREVIATION SIGN..KAITHI ENUMERATION SIGN
- {0x110BD, 0x110BD, prAL, gcCf}, // KAITHI NUMBER SIGN
- {0x110BE, 0x110C1, prBA, gcPo}, // [4] KAITHI SECTION MARK..KAITHI DOUBLE DANDA
- {0x110C2, 0x110C2, prCM, gcMn}, // KAITHI VOWEL SIGN VOCALIC R
- {0x110CD, 0x110CD, prAL, gcCf}, // KAITHI NUMBER SIGN ABOVE
- {0x110D0, 0x110E8, prAL, gcLo}, // [25] SORA SOMPENG LETTER SAH..SORA SOMPENG LETTER MAE
- {0x110F0, 0x110F9, prNU, gcNd}, // [10] SORA SOMPENG DIGIT ZERO..SORA SOMPENG DIGIT NINE
- {0x11100, 0x11102, prCM, gcMn}, // [3] CHAKMA SIGN CANDRABINDU..CHAKMA SIGN VISARGA
- {0x11103, 0x11126, prAL, gcLo}, // [36] CHAKMA LETTER AA..CHAKMA LETTER HAA
- {0x11127, 0x1112B, prCM, gcMn}, // [5] CHAKMA VOWEL SIGN A..CHAKMA VOWEL SIGN UU
- {0x1112C, 0x1112C, prCM, gcMc}, // CHAKMA VOWEL SIGN E
- {0x1112D, 0x11134, prCM, gcMn}, // [8] CHAKMA VOWEL SIGN AI..CHAKMA MAAYYAA
- {0x11136, 0x1113F, prNU, gcNd}, // [10] CHAKMA DIGIT ZERO..CHAKMA DIGIT NINE
- {0x11140, 0x11143, prBA, gcPo}, // [4] CHAKMA SECTION MARK..CHAKMA QUESTION MARK
- {0x11144, 0x11144, prAL, gcLo}, // CHAKMA LETTER LHAA
- {0x11145, 0x11146, prCM, gcMc}, // [2] CHAKMA VOWEL SIGN AA..CHAKMA VOWEL SIGN EI
- {0x11147, 0x11147, prAL, gcLo}, // CHAKMA LETTER VAA
- {0x11150, 0x11172, prAL, gcLo}, // [35] MAHAJANI LETTER A..MAHAJANI LETTER RRA
- {0x11173, 0x11173, prCM, gcMn}, // MAHAJANI SIGN NUKTA
- {0x11174, 0x11174, prAL, gcPo}, // MAHAJANI ABBREVIATION SIGN
- {0x11175, 0x11175, prBB, gcPo}, // MAHAJANI SECTION MARK
- {0x11176, 0x11176, prAL, gcLo}, // MAHAJANI LIGATURE SHRI
- {0x11180, 0x11181, prCM, gcMn}, // [2] SHARADA SIGN CANDRABINDU..SHARADA SIGN ANUSVARA
- {0x11182, 0x11182, prCM, gcMc}, // SHARADA SIGN VISARGA
- {0x11183, 0x111B2, prAL, gcLo}, // [48] SHARADA LETTER A..SHARADA LETTER HA
- {0x111B3, 0x111B5, prCM, gcMc}, // [3] SHARADA VOWEL SIGN AA..SHARADA VOWEL SIGN II
- {0x111B6, 0x111BE, prCM, gcMn}, // [9] SHARADA VOWEL SIGN U..SHARADA VOWEL SIGN O
- {0x111BF, 0x111C0, prCM, gcMc}, // [2] SHARADA VOWEL SIGN AU..SHARADA SIGN VIRAMA
- {0x111C1, 0x111C4, prAL, gcLo}, // [4] SHARADA SIGN AVAGRAHA..SHARADA OM
- {0x111C5, 0x111C6, prBA, gcPo}, // [2] SHARADA DANDA..SHARADA DOUBLE DANDA
- {0x111C7, 0x111C7, prAL, gcPo}, // SHARADA ABBREVIATION SIGN
- {0x111C8, 0x111C8, prBA, gcPo}, // SHARADA SEPARATOR
- {0x111C9, 0x111CC, prCM, gcMn}, // [4] SHARADA SANDHI MARK..SHARADA EXTRA SHORT VOWEL MARK
- {0x111CD, 0x111CD, prAL, gcPo}, // SHARADA SUTRA MARK
- {0x111CE, 0x111CE, prCM, gcMc}, // SHARADA VOWEL SIGN PRISHTHAMATRA E
- {0x111CF, 0x111CF, prCM, gcMn}, // SHARADA SIGN INVERTED CANDRABINDU
- {0x111D0, 0x111D9, prNU, gcNd}, // [10] SHARADA DIGIT ZERO..SHARADA DIGIT NINE
- {0x111DA, 0x111DA, prAL, gcLo}, // SHARADA EKAM
- {0x111DB, 0x111DB, prBB, gcPo}, // SHARADA SIGN SIDDHAM
- {0x111DC, 0x111DC, prAL, gcLo}, // SHARADA HEADSTROKE
- {0x111DD, 0x111DF, prBA, gcPo}, // [3] SHARADA CONTINUATION SIGN..SHARADA SECTION MARK-2
- {0x111E1, 0x111F4, prAL, gcNo}, // [20] SINHALA ARCHAIC DIGIT ONE..SINHALA ARCHAIC NUMBER ONE THOUSAND
- {0x11200, 0x11211, prAL, gcLo}, // [18] KHOJKI LETTER A..KHOJKI LETTER JJA
- {0x11213, 0x1122B, prAL, gcLo}, // [25] KHOJKI LETTER NYA..KHOJKI LETTER LLA
- {0x1122C, 0x1122E, prCM, gcMc}, // [3] KHOJKI VOWEL SIGN AA..KHOJKI VOWEL SIGN II
- {0x1122F, 0x11231, prCM, gcMn}, // [3] KHOJKI VOWEL SIGN U..KHOJKI VOWEL SIGN AI
- {0x11232, 0x11233, prCM, gcMc}, // [2] KHOJKI VOWEL SIGN O..KHOJKI VOWEL SIGN AU
- {0x11234, 0x11234, prCM, gcMn}, // KHOJKI SIGN ANUSVARA
- {0x11235, 0x11235, prCM, gcMc}, // KHOJKI SIGN VIRAMA
- {0x11236, 0x11237, prCM, gcMn}, // [2] KHOJKI SIGN NUKTA..KHOJKI SIGN SHADDA
- {0x11238, 0x11239, prBA, gcPo}, // [2] KHOJKI DANDA..KHOJKI DOUBLE DANDA
- {0x1123A, 0x1123A, prAL, gcPo}, // KHOJKI WORD SEPARATOR
- {0x1123B, 0x1123C, prBA, gcPo}, // [2] KHOJKI SECTION MARK..KHOJKI DOUBLE SECTION MARK
- {0x1123D, 0x1123D, prAL, gcPo}, // KHOJKI ABBREVIATION SIGN
- {0x1123E, 0x1123E, prCM, gcMn}, // KHOJKI SIGN SUKUN
- {0x11280, 0x11286, prAL, gcLo}, // [7] MULTANI LETTER A..MULTANI LETTER GA
- {0x11288, 0x11288, prAL, gcLo}, // MULTANI LETTER GHA
- {0x1128A, 0x1128D, prAL, gcLo}, // [4] MULTANI LETTER CA..MULTANI LETTER JJA
- {0x1128F, 0x1129D, prAL, gcLo}, // [15] MULTANI LETTER NYA..MULTANI LETTER BA
- {0x1129F, 0x112A8, prAL, gcLo}, // [10] MULTANI LETTER BHA..MULTANI LETTER RHA
- {0x112A9, 0x112A9, prBA, gcPo}, // MULTANI SECTION MARK
- {0x112B0, 0x112DE, prAL, gcLo}, // [47] KHUDAWADI LETTER A..KHUDAWADI LETTER HA
- {0x112DF, 0x112DF, prCM, gcMn}, // KHUDAWADI SIGN ANUSVARA
- {0x112E0, 0x112E2, prCM, gcMc}, // [3] KHUDAWADI VOWEL SIGN AA..KHUDAWADI VOWEL SIGN II
- {0x112E3, 0x112EA, prCM, gcMn}, // [8] KHUDAWADI VOWEL SIGN U..KHUDAWADI SIGN VIRAMA
- {0x112F0, 0x112F9, prNU, gcNd}, // [10] KHUDAWADI DIGIT ZERO..KHUDAWADI DIGIT NINE
- {0x11300, 0x11301, prCM, gcMn}, // [2] GRANTHA SIGN COMBINING ANUSVARA ABOVE..GRANTHA SIGN CANDRABINDU
- {0x11302, 0x11303, prCM, gcMc}, // [2] GRANTHA SIGN ANUSVARA..GRANTHA SIGN VISARGA
- {0x11305, 0x1130C, prAL, gcLo}, // [8] GRANTHA LETTER A..GRANTHA LETTER VOCALIC L
- {0x1130F, 0x11310, prAL, gcLo}, // [2] GRANTHA LETTER EE..GRANTHA LETTER AI
- {0x11313, 0x11328, prAL, gcLo}, // [22] GRANTHA LETTER OO..GRANTHA LETTER NA
- {0x1132A, 0x11330, prAL, gcLo}, // [7] GRANTHA LETTER PA..GRANTHA LETTER RA
- {0x11332, 0x11333, prAL, gcLo}, // [2] GRANTHA LETTER LA..GRANTHA LETTER LLA
- {0x11335, 0x11339, prAL, gcLo}, // [5] GRANTHA LETTER VA..GRANTHA LETTER HA
- {0x1133B, 0x1133C, prCM, gcMn}, // [2] COMBINING BINDU BELOW..GRANTHA SIGN NUKTA
- {0x1133D, 0x1133D, prAL, gcLo}, // GRANTHA SIGN AVAGRAHA
- {0x1133E, 0x1133F, prCM, gcMc}, // [2] GRANTHA VOWEL SIGN AA..GRANTHA VOWEL SIGN I
- {0x11340, 0x11340, prCM, gcMn}, // GRANTHA VOWEL SIGN II
- {0x11341, 0x11344, prCM, gcMc}, // [4] GRANTHA VOWEL SIGN U..GRANTHA VOWEL SIGN VOCALIC RR
- {0x11347, 0x11348, prCM, gcMc}, // [2] GRANTHA VOWEL SIGN EE..GRANTHA VOWEL SIGN AI
- {0x1134B, 0x1134D, prCM, gcMc}, // [3] GRANTHA VOWEL SIGN OO..GRANTHA SIGN VIRAMA
- {0x11350, 0x11350, prAL, gcLo}, // GRANTHA OM
- {0x11357, 0x11357, prCM, gcMc}, // GRANTHA AU LENGTH MARK
- {0x1135D, 0x11361, prAL, gcLo}, // [5] GRANTHA SIGN PLUTA..GRANTHA LETTER VOCALIC LL
- {0x11362, 0x11363, prCM, gcMc}, // [2] GRANTHA VOWEL SIGN VOCALIC L..GRANTHA VOWEL SIGN VOCALIC LL
- {0x11366, 0x1136C, prCM, gcMn}, // [7] COMBINING GRANTHA DIGIT ZERO..COMBINING GRANTHA DIGIT SIX
- {0x11370, 0x11374, prCM, gcMn}, // [5] COMBINING GRANTHA LETTER A..COMBINING GRANTHA LETTER PA
- {0x11400, 0x11434, prAL, gcLo}, // [53] NEWA LETTER A..NEWA LETTER HA
- {0x11435, 0x11437, prCM, gcMc}, // [3] NEWA VOWEL SIGN AA..NEWA VOWEL SIGN II
- {0x11438, 0x1143F, prCM, gcMn}, // [8] NEWA VOWEL SIGN U..NEWA VOWEL SIGN AI
- {0x11440, 0x11441, prCM, gcMc}, // [2] NEWA VOWEL SIGN O..NEWA VOWEL SIGN AU
- {0x11442, 0x11444, prCM, gcMn}, // [3] NEWA SIGN VIRAMA..NEWA SIGN ANUSVARA
- {0x11445, 0x11445, prCM, gcMc}, // NEWA SIGN VISARGA
- {0x11446, 0x11446, prCM, gcMn}, // NEWA SIGN NUKTA
- {0x11447, 0x1144A, prAL, gcLo}, // [4] NEWA SIGN AVAGRAHA..NEWA SIDDHI
- {0x1144B, 0x1144E, prBA, gcPo}, // [4] NEWA DANDA..NEWA GAP FILLER
- {0x1144F, 0x1144F, prAL, gcPo}, // NEWA ABBREVIATION SIGN
- {0x11450, 0x11459, prNU, gcNd}, // [10] NEWA DIGIT ZERO..NEWA DIGIT NINE
- {0x1145A, 0x1145B, prBA, gcPo}, // [2] NEWA DOUBLE COMMA..NEWA PLACEHOLDER MARK
- {0x1145D, 0x1145D, prAL, gcPo}, // NEWA INSERTION SIGN
- {0x1145E, 0x1145E, prCM, gcMn}, // NEWA SANDHI MARK
- {0x1145F, 0x11461, prAL, gcLo}, // [3] NEWA LETTER VEDIC ANUSVARA..NEWA SIGN UPADHMANIYA
- {0x11480, 0x114AF, prAL, gcLo}, // [48] TIRHUTA ANJI..TIRHUTA LETTER HA
- {0x114B0, 0x114B2, prCM, gcMc}, // [3] TIRHUTA VOWEL SIGN AA..TIRHUTA VOWEL SIGN II
- {0x114B3, 0x114B8, prCM, gcMn}, // [6] TIRHUTA VOWEL SIGN U..TIRHUTA VOWEL SIGN VOCALIC LL
- {0x114B9, 0x114B9, prCM, gcMc}, // TIRHUTA VOWEL SIGN E
- {0x114BA, 0x114BA, prCM, gcMn}, // TIRHUTA VOWEL SIGN SHORT E
- {0x114BB, 0x114BE, prCM, gcMc}, // [4] TIRHUTA VOWEL SIGN AI..TIRHUTA VOWEL SIGN AU
- {0x114BF, 0x114C0, prCM, gcMn}, // [2] TIRHUTA SIGN CANDRABINDU..TIRHUTA SIGN ANUSVARA
- {0x114C1, 0x114C1, prCM, gcMc}, // TIRHUTA SIGN VISARGA
- {0x114C2, 0x114C3, prCM, gcMn}, // [2] TIRHUTA SIGN VIRAMA..TIRHUTA SIGN NUKTA
- {0x114C4, 0x114C5, prAL, gcLo}, // [2] TIRHUTA SIGN AVAGRAHA..TIRHUTA GVANG
- {0x114C6, 0x114C6, prAL, gcPo}, // TIRHUTA ABBREVIATION SIGN
- {0x114C7, 0x114C7, prAL, gcLo}, // TIRHUTA OM
- {0x114D0, 0x114D9, prNU, gcNd}, // [10] TIRHUTA DIGIT ZERO..TIRHUTA DIGIT NINE
- {0x11580, 0x115AE, prAL, gcLo}, // [47] SIDDHAM LETTER A..SIDDHAM LETTER HA
- {0x115AF, 0x115B1, prCM, gcMc}, // [3] SIDDHAM VOWEL SIGN AA..SIDDHAM VOWEL SIGN II
- {0x115B2, 0x115B5, prCM, gcMn}, // [4] SIDDHAM VOWEL SIGN U..SIDDHAM VOWEL SIGN VOCALIC RR
- {0x115B8, 0x115BB, prCM, gcMc}, // [4] SIDDHAM VOWEL SIGN E..SIDDHAM VOWEL SIGN AU
- {0x115BC, 0x115BD, prCM, gcMn}, // [2] SIDDHAM SIGN CANDRABINDU..SIDDHAM SIGN ANUSVARA
- {0x115BE, 0x115BE, prCM, gcMc}, // SIDDHAM SIGN VISARGA
- {0x115BF, 0x115C0, prCM, gcMn}, // [2] SIDDHAM SIGN VIRAMA..SIDDHAM SIGN NUKTA
- {0x115C1, 0x115C1, prBB, gcPo}, // SIDDHAM SIGN SIDDHAM
- {0x115C2, 0x115C3, prBA, gcPo}, // [2] SIDDHAM DANDA..SIDDHAM DOUBLE DANDA
- {0x115C4, 0x115C5, prEX, gcPo}, // [2] SIDDHAM SEPARATOR DOT..SIDDHAM SEPARATOR BAR
- {0x115C6, 0x115C8, prAL, gcPo}, // [3] SIDDHAM REPETITION MARK-1..SIDDHAM REPETITION MARK-3
- {0x115C9, 0x115D7, prBA, gcPo}, // [15] SIDDHAM END OF TEXT MARK..SIDDHAM SECTION MARK WITH CIRCLES AND FOUR ENCLOSURES
- {0x115D8, 0x115DB, prAL, gcLo}, // [4] SIDDHAM LETTER THREE-CIRCLE ALTERNATE I..SIDDHAM LETTER ALTERNATE U
- {0x115DC, 0x115DD, prCM, gcMn}, // [2] SIDDHAM VOWEL SIGN ALTERNATE U..SIDDHAM VOWEL SIGN ALTERNATE UU
- {0x11600, 0x1162F, prAL, gcLo}, // [48] MODI LETTER A..MODI LETTER LLA
- {0x11630, 0x11632, prCM, gcMc}, // [3] MODI VOWEL SIGN AA..MODI VOWEL SIGN II
- {0x11633, 0x1163A, prCM, gcMn}, // [8] MODI VOWEL SIGN U..MODI VOWEL SIGN AI
- {0x1163B, 0x1163C, prCM, gcMc}, // [2] MODI VOWEL SIGN O..MODI VOWEL SIGN AU
- {0x1163D, 0x1163D, prCM, gcMn}, // MODI SIGN ANUSVARA
- {0x1163E, 0x1163E, prCM, gcMc}, // MODI SIGN VISARGA
- {0x1163F, 0x11640, prCM, gcMn}, // [2] MODI SIGN VIRAMA..MODI SIGN ARDHACANDRA
- {0x11641, 0x11642, prBA, gcPo}, // [2] MODI DANDA..MODI DOUBLE DANDA
- {0x11643, 0x11643, prAL, gcPo}, // MODI ABBREVIATION SIGN
- {0x11644, 0x11644, prAL, gcLo}, // MODI SIGN HUVA
- {0x11650, 0x11659, prNU, gcNd}, // [10] MODI DIGIT ZERO..MODI DIGIT NINE
- {0x11660, 0x1166C, prBB, gcPo}, // [13] MONGOLIAN BIRGA WITH ORNAMENT..MONGOLIAN TURNED SWIRL BIRGA WITH DOUBLE ORNAMENT
- {0x11680, 0x116AA, prAL, gcLo}, // [43] TAKRI LETTER A..TAKRI LETTER RRA
- {0x116AB, 0x116AB, prCM, gcMn}, // TAKRI SIGN ANUSVARA
- {0x116AC, 0x116AC, prCM, gcMc}, // TAKRI SIGN VISARGA
- {0x116AD, 0x116AD, prCM, gcMn}, // TAKRI VOWEL SIGN AA
- {0x116AE, 0x116AF, prCM, gcMc}, // [2] TAKRI VOWEL SIGN I..TAKRI VOWEL SIGN II
- {0x116B0, 0x116B5, prCM, gcMn}, // [6] TAKRI VOWEL SIGN U..TAKRI VOWEL SIGN AU
- {0x116B6, 0x116B6, prCM, gcMc}, // TAKRI SIGN VIRAMA
- {0x116B7, 0x116B7, prCM, gcMn}, // TAKRI SIGN NUKTA
- {0x116B8, 0x116B8, prAL, gcLo}, // TAKRI LETTER ARCHAIC KHA
- {0x116B9, 0x116B9, prAL, gcPo}, // TAKRI ABBREVIATION SIGN
- {0x116C0, 0x116C9, prNU, gcNd}, // [10] TAKRI DIGIT ZERO..TAKRI DIGIT NINE
- {0x11700, 0x1171A, prSA, gcLo}, // [27] AHOM LETTER KA..AHOM LETTER ALTERNATE BA
- {0x1171D, 0x1171F, prSA, gcMn}, // [3] AHOM CONSONANT SIGN MEDIAL LA..AHOM CONSONANT SIGN MEDIAL LIGATING RA
- {0x11720, 0x11721, prSA, gcMc}, // [2] AHOM VOWEL SIGN A..AHOM VOWEL SIGN AA
- {0x11722, 0x11725, prSA, gcMn}, // [4] AHOM VOWEL SIGN I..AHOM VOWEL SIGN UU
- {0x11726, 0x11726, prSA, gcMc}, // AHOM VOWEL SIGN E
- {0x11727, 0x1172B, prSA, gcMn}, // [5] AHOM VOWEL SIGN AW..AHOM SIGN KILLER
- {0x11730, 0x11739, prNU, gcNd}, // [10] AHOM DIGIT ZERO..AHOM DIGIT NINE
- {0x1173A, 0x1173B, prSA, gcNo}, // [2] AHOM NUMBER TEN..AHOM NUMBER TWENTY
- {0x1173C, 0x1173E, prBA, gcPo}, // [3] AHOM SIGN SMALL SECTION..AHOM SIGN RULAI
- {0x1173F, 0x1173F, prSA, gcSo}, // AHOM SYMBOL VI
- {0x11740, 0x11746, prSA, gcLo}, // [7] AHOM LETTER CA..AHOM LETTER LLA
- {0x11800, 0x1182B, prAL, gcLo}, // [44] DOGRA LETTER A..DOGRA LETTER RRA
- {0x1182C, 0x1182E, prCM, gcMc}, // [3] DOGRA VOWEL SIGN AA..DOGRA VOWEL SIGN II
- {0x1182F, 0x11837, prCM, gcMn}, // [9] DOGRA VOWEL SIGN U..DOGRA SIGN ANUSVARA
- {0x11838, 0x11838, prCM, gcMc}, // DOGRA SIGN VISARGA
- {0x11839, 0x1183A, prCM, gcMn}, // [2] DOGRA SIGN VIRAMA..DOGRA SIGN NUKTA
- {0x1183B, 0x1183B, prAL, gcPo}, // DOGRA ABBREVIATION SIGN
- {0x118A0, 0x118DF, prAL, gcLC}, // [64] WARANG CITI CAPITAL LETTER NGAA..WARANG CITI SMALL LETTER VIYO
- {0x118E0, 0x118E9, prNU, gcNd}, // [10] WARANG CITI DIGIT ZERO..WARANG CITI DIGIT NINE
- {0x118EA, 0x118F2, prAL, gcNo}, // [9] WARANG CITI NUMBER TEN..WARANG CITI NUMBER NINETY
- {0x118FF, 0x118FF, prAL, gcLo}, // WARANG CITI OM
- {0x11900, 0x11906, prAL, gcLo}, // [7] DIVES AKURU LETTER A..DIVES AKURU LETTER E
- {0x11909, 0x11909, prAL, gcLo}, // DIVES AKURU LETTER O
- {0x1190C, 0x11913, prAL, gcLo}, // [8] DIVES AKURU LETTER KA..DIVES AKURU LETTER JA
- {0x11915, 0x11916, prAL, gcLo}, // [2] DIVES AKURU LETTER NYA..DIVES AKURU LETTER TTA
- {0x11918, 0x1192F, prAL, gcLo}, // [24] DIVES AKURU LETTER DDA..DIVES AKURU LETTER ZA
- {0x11930, 0x11935, prCM, gcMc}, // [6] DIVES AKURU VOWEL SIGN AA..DIVES AKURU VOWEL SIGN E
- {0x11937, 0x11938, prCM, gcMc}, // [2] DIVES AKURU VOWEL SIGN AI..DIVES AKURU VOWEL SIGN O
- {0x1193B, 0x1193C, prCM, gcMn}, // [2] DIVES AKURU SIGN ANUSVARA..DIVES AKURU SIGN CANDRABINDU
- {0x1193D, 0x1193D, prCM, gcMc}, // DIVES AKURU SIGN HALANTA
- {0x1193E, 0x1193E, prCM, gcMn}, // DIVES AKURU VIRAMA
- {0x1193F, 0x1193F, prAL, gcLo}, // DIVES AKURU PREFIXED NASAL SIGN
- {0x11940, 0x11940, prCM, gcMc}, // DIVES AKURU MEDIAL YA
- {0x11941, 0x11941, prAL, gcLo}, // DIVES AKURU INITIAL RA
- {0x11942, 0x11942, prCM, gcMc}, // DIVES AKURU MEDIAL RA
- {0x11943, 0x11943, prCM, gcMn}, // DIVES AKURU SIGN NUKTA
- {0x11944, 0x11946, prBA, gcPo}, // [3] DIVES AKURU DOUBLE DANDA..DIVES AKURU END OF TEXT MARK
- {0x11950, 0x11959, prNU, gcNd}, // [10] DIVES AKURU DIGIT ZERO..DIVES AKURU DIGIT NINE
- {0x119A0, 0x119A7, prAL, gcLo}, // [8] NANDINAGARI LETTER A..NANDINAGARI LETTER VOCALIC RR
- {0x119AA, 0x119D0, prAL, gcLo}, // [39] NANDINAGARI LETTER E..NANDINAGARI LETTER RRA
- {0x119D1, 0x119D3, prCM, gcMc}, // [3] NANDINAGARI VOWEL SIGN AA..NANDINAGARI VOWEL SIGN II
- {0x119D4, 0x119D7, prCM, gcMn}, // [4] NANDINAGARI VOWEL SIGN U..NANDINAGARI VOWEL SIGN VOCALIC RR
- {0x119DA, 0x119DB, prCM, gcMn}, // [2] NANDINAGARI VOWEL SIGN E..NANDINAGARI VOWEL SIGN AI
- {0x119DC, 0x119DF, prCM, gcMc}, // [4] NANDINAGARI VOWEL SIGN O..NANDINAGARI SIGN VISARGA
- {0x119E0, 0x119E0, prCM, gcMn}, // NANDINAGARI SIGN VIRAMA
- {0x119E1, 0x119E1, prAL, gcLo}, // NANDINAGARI SIGN AVAGRAHA
- {0x119E2, 0x119E2, prBB, gcPo}, // NANDINAGARI SIGN SIDDHAM
- {0x119E3, 0x119E3, prAL, gcLo}, // NANDINAGARI HEADSTROKE
- {0x119E4, 0x119E4, prCM, gcMc}, // NANDINAGARI VOWEL SIGN PRISHTHAMATRA E
- {0x11A00, 0x11A00, prAL, gcLo}, // ZANABAZAR SQUARE LETTER A
- {0x11A01, 0x11A0A, prCM, gcMn}, // [10] ZANABAZAR SQUARE VOWEL SIGN I..ZANABAZAR SQUARE VOWEL LENGTH MARK
- {0x11A0B, 0x11A32, prAL, gcLo}, // [40] ZANABAZAR SQUARE LETTER KA..ZANABAZAR SQUARE LETTER KSSA
- {0x11A33, 0x11A38, prCM, gcMn}, // [6] ZANABAZAR SQUARE FINAL CONSONANT MARK..ZANABAZAR SQUARE SIGN ANUSVARA
- {0x11A39, 0x11A39, prCM, gcMc}, // ZANABAZAR SQUARE SIGN VISARGA
- {0x11A3A, 0x11A3A, prAL, gcLo}, // ZANABAZAR SQUARE CLUSTER-INITIAL LETTER RA
- {0x11A3B, 0x11A3E, prCM, gcMn}, // [4] ZANABAZAR SQUARE CLUSTER-FINAL LETTER YA..ZANABAZAR SQUARE CLUSTER-FINAL LETTER VA
- {0x11A3F, 0x11A3F, prBB, gcPo}, // ZANABAZAR SQUARE INITIAL HEAD MARK
- {0x11A40, 0x11A40, prAL, gcPo}, // ZANABAZAR SQUARE CLOSING HEAD MARK
- {0x11A41, 0x11A44, prBA, gcPo}, // [4] ZANABAZAR SQUARE MARK TSHEG..ZANABAZAR SQUARE MARK LONG TSHEG
- {0x11A45, 0x11A45, prBB, gcPo}, // ZANABAZAR SQUARE INITIAL DOUBLE-LINED HEAD MARK
- {0x11A46, 0x11A46, prAL, gcPo}, // ZANABAZAR SQUARE CLOSING DOUBLE-LINED HEAD MARK
- {0x11A47, 0x11A47, prCM, gcMn}, // ZANABAZAR SQUARE SUBJOINER
- {0x11A50, 0x11A50, prAL, gcLo}, // SOYOMBO LETTER A
- {0x11A51, 0x11A56, prCM, gcMn}, // [6] SOYOMBO VOWEL SIGN I..SOYOMBO VOWEL SIGN OE
- {0x11A57, 0x11A58, prCM, gcMc}, // [2] SOYOMBO VOWEL SIGN AI..SOYOMBO VOWEL SIGN AU
- {0x11A59, 0x11A5B, prCM, gcMn}, // [3] SOYOMBO VOWEL SIGN VOCALIC R..SOYOMBO VOWEL LENGTH MARK
- {0x11A5C, 0x11A89, prAL, gcLo}, // [46] SOYOMBO LETTER KA..SOYOMBO CLUSTER-INITIAL LETTER SA
- {0x11A8A, 0x11A96, prCM, gcMn}, // [13] SOYOMBO FINAL CONSONANT SIGN G..SOYOMBO SIGN ANUSVARA
- {0x11A97, 0x11A97, prCM, gcMc}, // SOYOMBO SIGN VISARGA
- {0x11A98, 0x11A99, prCM, gcMn}, // [2] SOYOMBO GEMINATION MARK..SOYOMBO SUBJOINER
- {0x11A9A, 0x11A9C, prBA, gcPo}, // [3] SOYOMBO MARK TSHEG..SOYOMBO MARK DOUBLE SHAD
- {0x11A9D, 0x11A9D, prAL, gcLo}, // SOYOMBO MARK PLUTA
- {0x11A9E, 0x11AA0, prBB, gcPo}, // [3] SOYOMBO HEAD MARK WITH MOON AND SUN AND TRIPLE FLAME..SOYOMBO HEAD MARK WITH MOON AND SUN
- {0x11AA1, 0x11AA2, prBA, gcPo}, // [2] SOYOMBO TERMINAL MARK-1..SOYOMBO TERMINAL MARK-2
- {0x11AB0, 0x11ABF, prAL, gcLo}, // [16] CANADIAN SYLLABICS NATTILIK HI..CANADIAN SYLLABICS SPA
- {0x11AC0, 0x11AF8, prAL, gcLo}, // [57] PAU CIN HAU LETTER PA..PAU CIN HAU GLOTTAL STOP FINAL
- {0x11C00, 0x11C08, prAL, gcLo}, // [9] BHAIKSUKI LETTER A..BHAIKSUKI LETTER VOCALIC L
- {0x11C0A, 0x11C2E, prAL, gcLo}, // [37] BHAIKSUKI LETTER E..BHAIKSUKI LETTER HA
- {0x11C2F, 0x11C2F, prCM, gcMc}, // BHAIKSUKI VOWEL SIGN AA
- {0x11C30, 0x11C36, prCM, gcMn}, // [7] BHAIKSUKI VOWEL SIGN I..BHAIKSUKI VOWEL SIGN VOCALIC L
- {0x11C38, 0x11C3D, prCM, gcMn}, // [6] BHAIKSUKI VOWEL SIGN E..BHAIKSUKI SIGN ANUSVARA
- {0x11C3E, 0x11C3E, prCM, gcMc}, // BHAIKSUKI SIGN VISARGA
- {0x11C3F, 0x11C3F, prCM, gcMn}, // BHAIKSUKI SIGN VIRAMA
- {0x11C40, 0x11C40, prAL, gcLo}, // BHAIKSUKI SIGN AVAGRAHA
- {0x11C41, 0x11C45, prBA, gcPo}, // [5] BHAIKSUKI DANDA..BHAIKSUKI GAP FILLER-2
- {0x11C50, 0x11C59, prNU, gcNd}, // [10] BHAIKSUKI DIGIT ZERO..BHAIKSUKI DIGIT NINE
- {0x11C5A, 0x11C6C, prAL, gcNo}, // [19] BHAIKSUKI NUMBER ONE..BHAIKSUKI HUNDREDS UNIT MARK
- {0x11C70, 0x11C70, prBB, gcPo}, // MARCHEN HEAD MARK
- {0x11C71, 0x11C71, prEX, gcPo}, // MARCHEN MARK SHAD
- {0x11C72, 0x11C8F, prAL, gcLo}, // [30] MARCHEN LETTER KA..MARCHEN LETTER A
- {0x11C92, 0x11CA7, prCM, gcMn}, // [22] MARCHEN SUBJOINED LETTER KA..MARCHEN SUBJOINED LETTER ZA
- {0x11CA9, 0x11CA9, prCM, gcMc}, // MARCHEN SUBJOINED LETTER YA
- {0x11CAA, 0x11CB0, prCM, gcMn}, // [7] MARCHEN SUBJOINED LETTER RA..MARCHEN VOWEL SIGN AA
- {0x11CB1, 0x11CB1, prCM, gcMc}, // MARCHEN VOWEL SIGN I
- {0x11CB2, 0x11CB3, prCM, gcMn}, // [2] MARCHEN VOWEL SIGN U..MARCHEN VOWEL SIGN E
- {0x11CB4, 0x11CB4, prCM, gcMc}, // MARCHEN VOWEL SIGN O
- {0x11CB5, 0x11CB6, prCM, gcMn}, // [2] MARCHEN SIGN ANUSVARA..MARCHEN SIGN CANDRABINDU
- {0x11D00, 0x11D06, prAL, gcLo}, // [7] MASARAM GONDI LETTER A..MASARAM GONDI LETTER E
- {0x11D08, 0x11D09, prAL, gcLo}, // [2] MASARAM GONDI LETTER AI..MASARAM GONDI LETTER O
- {0x11D0B, 0x11D30, prAL, gcLo}, // [38] MASARAM GONDI LETTER AU..MASARAM GONDI LETTER TRA
- {0x11D31, 0x11D36, prCM, gcMn}, // [6] MASARAM GONDI VOWEL SIGN AA..MASARAM GONDI VOWEL SIGN VOCALIC R
- {0x11D3A, 0x11D3A, prCM, gcMn}, // MASARAM GONDI VOWEL SIGN E
- {0x11D3C, 0x11D3D, prCM, gcMn}, // [2] MASARAM GONDI VOWEL SIGN AI..MASARAM GONDI VOWEL SIGN O
- {0x11D3F, 0x11D45, prCM, gcMn}, // [7] MASARAM GONDI VOWEL SIGN AU..MASARAM GONDI VIRAMA
- {0x11D46, 0x11D46, prAL, gcLo}, // MASARAM GONDI REPHA
- {0x11D47, 0x11D47, prCM, gcMn}, // MASARAM GONDI RA-KARA
- {0x11D50, 0x11D59, prNU, gcNd}, // [10] MASARAM GONDI DIGIT ZERO..MASARAM GONDI DIGIT NINE
- {0x11D60, 0x11D65, prAL, gcLo}, // [6] GUNJALA GONDI LETTER A..GUNJALA GONDI LETTER UU
- {0x11D67, 0x11D68, prAL, gcLo}, // [2] GUNJALA GONDI LETTER EE..GUNJALA GONDI LETTER AI
- {0x11D6A, 0x11D89, prAL, gcLo}, // [32] GUNJALA GONDI LETTER OO..GUNJALA GONDI LETTER SA
- {0x11D8A, 0x11D8E, prCM, gcMc}, // [5] GUNJALA GONDI VOWEL SIGN AA..GUNJALA GONDI VOWEL SIGN UU
- {0x11D90, 0x11D91, prCM, gcMn}, // [2] GUNJALA GONDI VOWEL SIGN EE..GUNJALA GONDI VOWEL SIGN AI
- {0x11D93, 0x11D94, prCM, gcMc}, // [2] GUNJALA GONDI VOWEL SIGN OO..GUNJALA GONDI VOWEL SIGN AU
- {0x11D95, 0x11D95, prCM, gcMn}, // GUNJALA GONDI SIGN ANUSVARA
- {0x11D96, 0x11D96, prCM, gcMc}, // GUNJALA GONDI SIGN VISARGA
- {0x11D97, 0x11D97, prCM, gcMn}, // GUNJALA GONDI VIRAMA
- {0x11D98, 0x11D98, prAL, gcLo}, // GUNJALA GONDI OM
- {0x11DA0, 0x11DA9, prNU, gcNd}, // [10] GUNJALA GONDI DIGIT ZERO..GUNJALA GONDI DIGIT NINE
- {0x11EE0, 0x11EF2, prAL, gcLo}, // [19] MAKASAR LETTER KA..MAKASAR ANGKA
- {0x11EF3, 0x11EF4, prCM, gcMn}, // [2] MAKASAR VOWEL SIGN I..MAKASAR VOWEL SIGN U
- {0x11EF5, 0x11EF6, prCM, gcMc}, // [2] MAKASAR VOWEL SIGN E..MAKASAR VOWEL SIGN O
- {0x11EF7, 0x11EF8, prAL, gcPo}, // [2] MAKASAR PASSIMBANG..MAKASAR END OF SECTION
- {0x11FB0, 0x11FB0, prAL, gcLo}, // LISU LETTER YHA
- {0x11FC0, 0x11FD4, prAL, gcNo}, // [21] TAMIL FRACTION ONE THREE-HUNDRED-AND-TWENTIETH..TAMIL FRACTION DOWNSCALING FACTOR KIIZH
- {0x11FD5, 0x11FDC, prAL, gcSo}, // [8] TAMIL SIGN NEL..TAMIL SIGN MUKKURUNI
- {0x11FDD, 0x11FE0, prPO, gcSc}, // [4] TAMIL SIGN KAACU..TAMIL SIGN VARAAKAN
- {0x11FE1, 0x11FF1, prAL, gcSo}, // [17] TAMIL SIGN PAARAM..TAMIL SIGN VAKAIYARAA
- {0x11FFF, 0x11FFF, prBA, gcPo}, // TAMIL PUNCTUATION END OF TEXT
- {0x12000, 0x12399, prAL, gcLo}, // [922] CUNEIFORM SIGN A..CUNEIFORM SIGN U U
- {0x12400, 0x1246E, prAL, gcNl}, // [111] CUNEIFORM NUMERIC SIGN TWO ASH..CUNEIFORM NUMERIC SIGN NINE U VARIANT FORM
- {0x12470, 0x12474, prBA, gcPo}, // [5] CUNEIFORM PUNCTUATION SIGN OLD ASSYRIAN WORD DIVIDER..CUNEIFORM PUNCTUATION SIGN DIAGONAL QUADCOLON
- {0x12480, 0x12543, prAL, gcLo}, // [196] CUNEIFORM SIGN AB TIMES NUN TENU..CUNEIFORM SIGN ZU5 TIMES THREE DISH TENU
- {0x12F90, 0x12FF0, prAL, gcLo}, // [97] CYPRO-MINOAN SIGN CM001..CYPRO-MINOAN SIGN CM114
- {0x12FF1, 0x12FF2, prAL, gcPo}, // [2] CYPRO-MINOAN SIGN CM301..CYPRO-MINOAN SIGN CM302
- {0x13000, 0x13257, prAL, gcLo}, // [600] EGYPTIAN HIEROGLYPH A001..EGYPTIAN HIEROGLYPH O006
- {0x13258, 0x1325A, prOP, gcLo}, // [3] EGYPTIAN HIEROGLYPH O006A..EGYPTIAN HIEROGLYPH O006C
- {0x1325B, 0x1325D, prCL, gcLo}, // [3] EGYPTIAN HIEROGLYPH O006D..EGYPTIAN HIEROGLYPH O006F
- {0x1325E, 0x13281, prAL, gcLo}, // [36] EGYPTIAN HIEROGLYPH O007..EGYPTIAN HIEROGLYPH O033
- {0x13282, 0x13282, prCL, gcLo}, // EGYPTIAN HIEROGLYPH O033A
- {0x13283, 0x13285, prAL, gcLo}, // [3] EGYPTIAN HIEROGLYPH O034..EGYPTIAN HIEROGLYPH O036
- {0x13286, 0x13286, prOP, gcLo}, // EGYPTIAN HIEROGLYPH O036A
- {0x13287, 0x13287, prCL, gcLo}, // EGYPTIAN HIEROGLYPH O036B
- {0x13288, 0x13288, prOP, gcLo}, // EGYPTIAN HIEROGLYPH O036C
- {0x13289, 0x13289, prCL, gcLo}, // EGYPTIAN HIEROGLYPH O036D
- {0x1328A, 0x13378, prAL, gcLo}, // [239] EGYPTIAN HIEROGLYPH O037..EGYPTIAN HIEROGLYPH V011
- {0x13379, 0x13379, prOP, gcLo}, // EGYPTIAN HIEROGLYPH V011A
- {0x1337A, 0x1337B, prCL, gcLo}, // [2] EGYPTIAN HIEROGLYPH V011B..EGYPTIAN HIEROGLYPH V011C
- {0x1337C, 0x1342E, prAL, gcLo}, // [179] EGYPTIAN HIEROGLYPH V012..EGYPTIAN HIEROGLYPH AA032
- {0x13430, 0x13436, prGL, gcCf}, // [7] EGYPTIAN HIEROGLYPH VERTICAL JOINER..EGYPTIAN HIEROGLYPH OVERLAY MIDDLE
- {0x13437, 0x13437, prOP, gcCf}, // EGYPTIAN HIEROGLYPH BEGIN SEGMENT
- {0x13438, 0x13438, prCL, gcCf}, // EGYPTIAN HIEROGLYPH END SEGMENT
- {0x14400, 0x145CD, prAL, gcLo}, // [462] ANATOLIAN HIEROGLYPH A001..ANATOLIAN HIEROGLYPH A409
- {0x145CE, 0x145CE, prOP, gcLo}, // ANATOLIAN HIEROGLYPH A410 BEGIN LOGOGRAM MARK
- {0x145CF, 0x145CF, prCL, gcLo}, // ANATOLIAN HIEROGLYPH A410A END LOGOGRAM MARK
- {0x145D0, 0x14646, prAL, gcLo}, // [119] ANATOLIAN HIEROGLYPH A411..ANATOLIAN HIEROGLYPH A530
- {0x16800, 0x16A38, prAL, gcLo}, // [569] BAMUM LETTER PHASE-A NGKUE MFON..BAMUM LETTER PHASE-F VUEQ
- {0x16A40, 0x16A5E, prAL, gcLo}, // [31] MRO LETTER TA..MRO LETTER TEK
- {0x16A60, 0x16A69, prNU, gcNd}, // [10] MRO DIGIT ZERO..MRO DIGIT NINE
- {0x16A6E, 0x16A6F, prBA, gcPo}, // [2] MRO DANDA..MRO DOUBLE DANDA
- {0x16A70, 0x16ABE, prAL, gcLo}, // [79] TANGSA LETTER OZ..TANGSA LETTER ZA
- {0x16AC0, 0x16AC9, prNU, gcNd}, // [10] TANGSA DIGIT ZERO..TANGSA DIGIT NINE
- {0x16AD0, 0x16AED, prAL, gcLo}, // [30] BASSA VAH LETTER ENNI..BASSA VAH LETTER I
- {0x16AF0, 0x16AF4, prCM, gcMn}, // [5] BASSA VAH COMBINING HIGH TONE..BASSA VAH COMBINING HIGH-LOW TONE
- {0x16AF5, 0x16AF5, prBA, gcPo}, // BASSA VAH FULL STOP
- {0x16B00, 0x16B2F, prAL, gcLo}, // [48] PAHAWH HMONG VOWEL KEEB..PAHAWH HMONG CONSONANT CAU
- {0x16B30, 0x16B36, prCM, gcMn}, // [7] PAHAWH HMONG MARK CIM TUB..PAHAWH HMONG MARK CIM TAUM
- {0x16B37, 0x16B39, prBA, gcPo}, // [3] PAHAWH HMONG SIGN VOS THOM..PAHAWH HMONG SIGN CIM CHEEM
- {0x16B3A, 0x16B3B, prAL, gcPo}, // [2] PAHAWH HMONG SIGN VOS THIAB..PAHAWH HMONG SIGN VOS FEEM
- {0x16B3C, 0x16B3F, prAL, gcSo}, // [4] PAHAWH HMONG SIGN XYEEM NTXIV..PAHAWH HMONG SIGN XYEEM FAIB
- {0x16B40, 0x16B43, prAL, gcLm}, // [4] PAHAWH HMONG SIGN VOS SEEV..PAHAWH HMONG SIGN IB YAM
- {0x16B44, 0x16B44, prBA, gcPo}, // PAHAWH HMONG SIGN XAUS
- {0x16B45, 0x16B45, prAL, gcSo}, // PAHAWH HMONG SIGN CIM TSOV ROG
- {0x16B50, 0x16B59, prNU, gcNd}, // [10] PAHAWH HMONG DIGIT ZERO..PAHAWH HMONG DIGIT NINE
- {0x16B5B, 0x16B61, prAL, gcNo}, // [7] PAHAWH HMONG NUMBER TENS..PAHAWH HMONG NUMBER TRILLIONS
- {0x16B63, 0x16B77, prAL, gcLo}, // [21] PAHAWH HMONG SIGN VOS LUB..PAHAWH HMONG SIGN CIM NRES TOS
- {0x16B7D, 0x16B8F, prAL, gcLo}, // [19] PAHAWH HMONG CLAN SIGN TSHEEJ..PAHAWH HMONG CLAN SIGN VWJ
- {0x16E40, 0x16E7F, prAL, gcLC}, // [64] MEDEFAIDRIN CAPITAL LETTER M..MEDEFAIDRIN SMALL LETTER Y
- {0x16E80, 0x16E96, prAL, gcNo}, // [23] MEDEFAIDRIN DIGIT ZERO..MEDEFAIDRIN DIGIT THREE ALTERNATE FORM
- {0x16E97, 0x16E98, prBA, gcPo}, // [2] MEDEFAIDRIN COMMA..MEDEFAIDRIN FULL STOP
- {0x16E99, 0x16E9A, prAL, gcPo}, // [2] MEDEFAIDRIN SYMBOL AIVA..MEDEFAIDRIN EXCLAMATION OH
- {0x16F00, 0x16F4A, prAL, gcLo}, // [75] MIAO LETTER PA..MIAO LETTER RTE
- {0x16F4F, 0x16F4F, prCM, gcMn}, // MIAO SIGN CONSONANT MODIFIER BAR
- {0x16F50, 0x16F50, prAL, gcLo}, // MIAO LETTER NASALIZATION
- {0x16F51, 0x16F87, prCM, gcMc}, // [55] MIAO SIGN ASPIRATION..MIAO VOWEL SIGN UI
- {0x16F8F, 0x16F92, prCM, gcMn}, // [4] MIAO TONE RIGHT..MIAO TONE BELOW
- {0x16F93, 0x16F9F, prAL, gcLm}, // [13] MIAO LETTER TONE-2..MIAO LETTER REFORMED TONE-8
- {0x16FE0, 0x16FE1, prNS, gcLm}, // [2] TANGUT ITERATION MARK..NUSHU ITERATION MARK
- {0x16FE2, 0x16FE2, prNS, gcPo}, // OLD CHINESE HOOK MARK
- {0x16FE3, 0x16FE3, prNS, gcLm}, // OLD CHINESE ITERATION MARK
- {0x16FE4, 0x16FE4, prGL, gcMn}, // KHITAN SMALL SCRIPT FILLER
- {0x16FF0, 0x16FF1, prCM, gcMc}, // [2] VIETNAMESE ALTERNATE READING MARK CA..VIETNAMESE ALTERNATE READING MARK NHAY
- {0x17000, 0x187F7, prID, gcLo}, // [6136] TANGUT IDEOGRAPH-17000..TANGUT IDEOGRAPH-187F7
- {0x18800, 0x18AFF, prID, gcLo}, // [768] TANGUT COMPONENT-001..TANGUT COMPONENT-768
- {0x18B00, 0x18CD5, prAL, gcLo}, // [470] KHITAN SMALL SCRIPT CHARACTER-18B00..KHITAN SMALL SCRIPT CHARACTER-18CD5
- {0x18D00, 0x18D08, prID, gcLo}, // [9] TANGUT IDEOGRAPH-18D00..TANGUT IDEOGRAPH-18D08
- {0x1AFF0, 0x1AFF3, prAL, gcLm}, // [4] KATAKANA LETTER MINNAN TONE-2..KATAKANA LETTER MINNAN TONE-5
- {0x1AFF5, 0x1AFFB, prAL, gcLm}, // [7] KATAKANA LETTER MINNAN TONE-7..KATAKANA LETTER MINNAN NASALIZED TONE-5
- {0x1AFFD, 0x1AFFE, prAL, gcLm}, // [2] KATAKANA LETTER MINNAN NASALIZED TONE-7..KATAKANA LETTER MINNAN NASALIZED TONE-8
- {0x1B000, 0x1B0FF, prID, gcLo}, // [256] KATAKANA LETTER ARCHAIC E..HENTAIGANA LETTER RE-2
- {0x1B100, 0x1B122, prID, gcLo}, // [35] HENTAIGANA LETTER RE-3..KATAKANA LETTER ARCHAIC WU
- {0x1B150, 0x1B152, prCJ, gcLo}, // [3] HIRAGANA LETTER SMALL WI..HIRAGANA LETTER SMALL WO
- {0x1B164, 0x1B167, prCJ, gcLo}, // [4] KATAKANA LETTER SMALL WI..KATAKANA LETTER SMALL N
- {0x1B170, 0x1B2FB, prID, gcLo}, // [396] NUSHU CHARACTER-1B170..NUSHU CHARACTER-1B2FB
- {0x1BC00, 0x1BC6A, prAL, gcLo}, // [107] DUPLOYAN LETTER H..DUPLOYAN LETTER VOCALIC M
- {0x1BC70, 0x1BC7C, prAL, gcLo}, // [13] DUPLOYAN AFFIX LEFT HORIZONTAL SECANT..DUPLOYAN AFFIX ATTACHED TANGENT HOOK
- {0x1BC80, 0x1BC88, prAL, gcLo}, // [9] DUPLOYAN AFFIX HIGH ACUTE..DUPLOYAN AFFIX HIGH VERTICAL
- {0x1BC90, 0x1BC99, prAL, gcLo}, // [10] DUPLOYAN AFFIX LOW ACUTE..DUPLOYAN AFFIX LOW ARROW
- {0x1BC9C, 0x1BC9C, prAL, gcSo}, // DUPLOYAN SIGN O WITH CROSS
- {0x1BC9D, 0x1BC9E, prCM, gcMn}, // [2] DUPLOYAN THICK LETTER SELECTOR..DUPLOYAN DOUBLE MARK
- {0x1BC9F, 0x1BC9F, prBA, gcPo}, // DUPLOYAN PUNCTUATION CHINOOK FULL STOP
- {0x1BCA0, 0x1BCA3, prCM, gcCf}, // [4] SHORTHAND FORMAT LETTER OVERLAP..SHORTHAND FORMAT UP STEP
- {0x1CF00, 0x1CF2D, prCM, gcMn}, // [46] ZNAMENNY COMBINING MARK GORAZDO NIZKO S KRYZHEM ON LEFT..ZNAMENNY COMBINING MARK KRYZH ON LEFT
- {0x1CF30, 0x1CF46, prCM, gcMn}, // [23] ZNAMENNY COMBINING TONAL RANGE MARK MRACHNO..ZNAMENNY PRIZNAK MODIFIER ROG
- {0x1CF50, 0x1CFC3, prAL, gcSo}, // [116] ZNAMENNY NEUME KRYUK..ZNAMENNY NEUME PAUK
- {0x1D000, 0x1D0F5, prAL, gcSo}, // [246] BYZANTINE MUSICAL SYMBOL PSILI..BYZANTINE MUSICAL SYMBOL GORGON NEO KATO
- {0x1D100, 0x1D126, prAL, gcSo}, // [39] MUSICAL SYMBOL SINGLE BARLINE..MUSICAL SYMBOL DRUM CLEF-2
- {0x1D129, 0x1D164, prAL, gcSo}, // [60] MUSICAL SYMBOL MULTIPLE MEASURE REST..MUSICAL SYMBOL ONE HUNDRED TWENTY-EIGHTH NOTE
- {0x1D165, 0x1D166, prCM, gcMc}, // [2] MUSICAL SYMBOL COMBINING STEM..MUSICAL SYMBOL COMBINING SPRECHGESANG STEM
- {0x1D167, 0x1D169, prCM, gcMn}, // [3] MUSICAL SYMBOL COMBINING TREMOLO-1..MUSICAL SYMBOL COMBINING TREMOLO-3
- {0x1D16A, 0x1D16C, prAL, gcSo}, // [3] MUSICAL SYMBOL FINGERED TREMOLO-1..MUSICAL SYMBOL FINGERED TREMOLO-3
- {0x1D16D, 0x1D172, prCM, gcMc}, // [6] MUSICAL SYMBOL COMBINING AUGMENTATION DOT..MUSICAL SYMBOL COMBINING FLAG-5
- {0x1D173, 0x1D17A, prCM, gcCf}, // [8] MUSICAL SYMBOL BEGIN BEAM..MUSICAL SYMBOL END PHRASE
- {0x1D17B, 0x1D182, prCM, gcMn}, // [8] MUSICAL SYMBOL COMBINING ACCENT..MUSICAL SYMBOL COMBINING LOURE
- {0x1D183, 0x1D184, prAL, gcSo}, // [2] MUSICAL SYMBOL ARPEGGIATO UP..MUSICAL SYMBOL ARPEGGIATO DOWN
- {0x1D185, 0x1D18B, prCM, gcMn}, // [7] MUSICAL SYMBOL COMBINING DOIT..MUSICAL SYMBOL COMBINING TRIPLE TONGUE
- {0x1D18C, 0x1D1A9, prAL, gcSo}, // [30] MUSICAL SYMBOL RINFORZANDO..MUSICAL SYMBOL DEGREE SLASH
- {0x1D1AA, 0x1D1AD, prCM, gcMn}, // [4] MUSICAL SYMBOL COMBINING DOWN BOW..MUSICAL SYMBOL COMBINING SNAP PIZZICATO
- {0x1D1AE, 0x1D1EA, prAL, gcSo}, // [61] MUSICAL SYMBOL PEDAL MARK..MUSICAL SYMBOL KORON
- {0x1D200, 0x1D241, prAL, gcSo}, // [66] GREEK VOCAL NOTATION SYMBOL-1..GREEK INSTRUMENTAL NOTATION SYMBOL-54
- {0x1D242, 0x1D244, prCM, gcMn}, // [3] COMBINING GREEK MUSICAL TRISEME..COMBINING GREEK MUSICAL PENTASEME
- {0x1D245, 0x1D245, prAL, gcSo}, // GREEK MUSICAL LEIMMA
- {0x1D2E0, 0x1D2F3, prAL, gcNo}, // [20] MAYAN NUMERAL ZERO..MAYAN NUMERAL NINETEEN
- {0x1D300, 0x1D356, prAL, gcSo}, // [87] MONOGRAM FOR EARTH..TETRAGRAM FOR FOSTERING
- {0x1D360, 0x1D378, prAL, gcNo}, // [25] COUNTING ROD UNIT DIGIT ONE..TALLY MARK FIVE
- {0x1D400, 0x1D454, prAL, gcLC}, // [85] MATHEMATICAL BOLD CAPITAL A..MATHEMATICAL ITALIC SMALL G
- {0x1D456, 0x1D49C, prAL, gcLC}, // [71] MATHEMATICAL ITALIC SMALL I..MATHEMATICAL SCRIPT CAPITAL A
- {0x1D49E, 0x1D49F, prAL, gcLu}, // [2] MATHEMATICAL SCRIPT CAPITAL C..MATHEMATICAL SCRIPT CAPITAL D
- {0x1D4A2, 0x1D4A2, prAL, gcLu}, // MATHEMATICAL SCRIPT CAPITAL G
- {0x1D4A5, 0x1D4A6, prAL, gcLu}, // [2] MATHEMATICAL SCRIPT CAPITAL J..MATHEMATICAL SCRIPT CAPITAL K
- {0x1D4A9, 0x1D4AC, prAL, gcLu}, // [4] MATHEMATICAL SCRIPT CAPITAL N..MATHEMATICAL SCRIPT CAPITAL Q
- {0x1D4AE, 0x1D4B9, prAL, gcLC}, // [12] MATHEMATICAL SCRIPT CAPITAL S..MATHEMATICAL SCRIPT SMALL D
- {0x1D4BB, 0x1D4BB, prAL, gcLl}, // MATHEMATICAL SCRIPT SMALL F
- {0x1D4BD, 0x1D4C3, prAL, gcLl}, // [7] MATHEMATICAL SCRIPT SMALL H..MATHEMATICAL SCRIPT SMALL N
- {0x1D4C5, 0x1D505, prAL, gcLC}, // [65] MATHEMATICAL SCRIPT SMALL P..MATHEMATICAL FRAKTUR CAPITAL B
- {0x1D507, 0x1D50A, prAL, gcLu}, // [4] MATHEMATICAL FRAKTUR CAPITAL D..MATHEMATICAL FRAKTUR CAPITAL G
- {0x1D50D, 0x1D514, prAL, gcLu}, // [8] MATHEMATICAL FRAKTUR CAPITAL J..MATHEMATICAL FRAKTUR CAPITAL Q
- {0x1D516, 0x1D51C, prAL, gcLu}, // [7] MATHEMATICAL FRAKTUR CAPITAL S..MATHEMATICAL FRAKTUR CAPITAL Y
- {0x1D51E, 0x1D539, prAL, gcLC}, // [28] MATHEMATICAL FRAKTUR SMALL A..MATHEMATICAL DOUBLE-STRUCK CAPITAL B
- {0x1D53B, 0x1D53E, prAL, gcLu}, // [4] MATHEMATICAL DOUBLE-STRUCK CAPITAL D..MATHEMATICAL DOUBLE-STRUCK CAPITAL G
- {0x1D540, 0x1D544, prAL, gcLu}, // [5] MATHEMATICAL DOUBLE-STRUCK CAPITAL I..MATHEMATICAL DOUBLE-STRUCK CAPITAL M
- {0x1D546, 0x1D546, prAL, gcLu}, // MATHEMATICAL DOUBLE-STRUCK CAPITAL O
- {0x1D54A, 0x1D550, prAL, gcLu}, // [7] MATHEMATICAL DOUBLE-STRUCK CAPITAL S..MATHEMATICAL DOUBLE-STRUCK CAPITAL Y
- {0x1D552, 0x1D6A5, prAL, gcLC}, // [340] MATHEMATICAL DOUBLE-STRUCK SMALL A..MATHEMATICAL ITALIC SMALL DOTLESS J
- {0x1D6A8, 0x1D6C0, prAL, gcLu}, // [25] MATHEMATICAL BOLD CAPITAL ALPHA..MATHEMATICAL BOLD CAPITAL OMEGA
- {0x1D6C1, 0x1D6C1, prAL, gcSm}, // MATHEMATICAL BOLD NABLA
- {0x1D6C2, 0x1D6DA, prAL, gcLl}, // [25] MATHEMATICAL BOLD SMALL ALPHA..MATHEMATICAL BOLD SMALL OMEGA
- {0x1D6DB, 0x1D6DB, prAL, gcSm}, // MATHEMATICAL BOLD PARTIAL DIFFERENTIAL
- {0x1D6DC, 0x1D6FA, prAL, gcLC}, // [31] MATHEMATICAL BOLD EPSILON SYMBOL..MATHEMATICAL ITALIC CAPITAL OMEGA
- {0x1D6FB, 0x1D6FB, prAL, gcSm}, // MATHEMATICAL ITALIC NABLA
- {0x1D6FC, 0x1D714, prAL, gcLl}, // [25] MATHEMATICAL ITALIC SMALL ALPHA..MATHEMATICAL ITALIC SMALL OMEGA
- {0x1D715, 0x1D715, prAL, gcSm}, // MATHEMATICAL ITALIC PARTIAL DIFFERENTIAL
- {0x1D716, 0x1D734, prAL, gcLC}, // [31] MATHEMATICAL ITALIC EPSILON SYMBOL..MATHEMATICAL BOLD ITALIC CAPITAL OMEGA
- {0x1D735, 0x1D735, prAL, gcSm}, // MATHEMATICAL BOLD ITALIC NABLA
- {0x1D736, 0x1D74E, prAL, gcLl}, // [25] MATHEMATICAL BOLD ITALIC SMALL ALPHA..MATHEMATICAL BOLD ITALIC SMALL OMEGA
- {0x1D74F, 0x1D74F, prAL, gcSm}, // MATHEMATICAL BOLD ITALIC PARTIAL DIFFERENTIAL
- {0x1D750, 0x1D76E, prAL, gcLC}, // [31] MATHEMATICAL BOLD ITALIC EPSILON SYMBOL..MATHEMATICAL SANS-SERIF BOLD CAPITAL OMEGA
- {0x1D76F, 0x1D76F, prAL, gcSm}, // MATHEMATICAL SANS-SERIF BOLD NABLA
- {0x1D770, 0x1D788, prAL, gcLl}, // [25] MATHEMATICAL SANS-SERIF BOLD SMALL ALPHA..MATHEMATICAL SANS-SERIF BOLD SMALL OMEGA
- {0x1D789, 0x1D789, prAL, gcSm}, // MATHEMATICAL SANS-SERIF BOLD PARTIAL DIFFERENTIAL
- {0x1D78A, 0x1D7A8, prAL, gcLC}, // [31] MATHEMATICAL SANS-SERIF BOLD EPSILON SYMBOL..MATHEMATICAL SANS-SERIF BOLD ITALIC CAPITAL OMEGA
- {0x1D7A9, 0x1D7A9, prAL, gcSm}, // MATHEMATICAL SANS-SERIF BOLD ITALIC NABLA
- {0x1D7AA, 0x1D7C2, prAL, gcLl}, // [25] MATHEMATICAL SANS-SERIF BOLD ITALIC SMALL ALPHA..MATHEMATICAL SANS-SERIF BOLD ITALIC SMALL OMEGA
- {0x1D7C3, 0x1D7C3, prAL, gcSm}, // MATHEMATICAL SANS-SERIF BOLD ITALIC PARTIAL DIFFERENTIAL
- {0x1D7C4, 0x1D7CB, prAL, gcLC}, // [8] MATHEMATICAL SANS-SERIF BOLD ITALIC EPSILON SYMBOL..MATHEMATICAL BOLD SMALL DIGAMMA
- {0x1D7CE, 0x1D7FF, prNU, gcNd}, // [50] MATHEMATICAL BOLD DIGIT ZERO..MATHEMATICAL MONOSPACE DIGIT NINE
- {0x1D800, 0x1D9FF, prAL, gcSo}, // [512] SIGNWRITING HAND-FIST INDEX..SIGNWRITING HEAD
- {0x1DA00, 0x1DA36, prCM, gcMn}, // [55] SIGNWRITING HEAD RIM..SIGNWRITING AIR SUCKING IN
- {0x1DA37, 0x1DA3A, prAL, gcSo}, // [4] SIGNWRITING AIR BLOW SMALL ROTATIONS..SIGNWRITING BREATH EXHALE
- {0x1DA3B, 0x1DA6C, prCM, gcMn}, // [50] SIGNWRITING MOUTH CLOSED NEUTRAL..SIGNWRITING EXCITEMENT
- {0x1DA6D, 0x1DA74, prAL, gcSo}, // [8] SIGNWRITING SHOULDER HIP SPINE..SIGNWRITING TORSO-FLOORPLANE TWISTING
- {0x1DA75, 0x1DA75, prCM, gcMn}, // SIGNWRITING UPPER BODY TILTING FROM HIP JOINTS
- {0x1DA76, 0x1DA83, prAL, gcSo}, // [14] SIGNWRITING LIMB COMBINATION..SIGNWRITING LOCATION DEPTH
- {0x1DA84, 0x1DA84, prCM, gcMn}, // SIGNWRITING LOCATION HEAD NECK
- {0x1DA85, 0x1DA86, prAL, gcSo}, // [2] SIGNWRITING LOCATION TORSO..SIGNWRITING LOCATION LIMBS DIGITS
- {0x1DA87, 0x1DA8A, prBA, gcPo}, // [4] SIGNWRITING COMMA..SIGNWRITING COLON
- {0x1DA8B, 0x1DA8B, prAL, gcPo}, // SIGNWRITING PARENTHESIS
- {0x1DA9B, 0x1DA9F, prCM, gcMn}, // [5] SIGNWRITING FILL MODIFIER-2..SIGNWRITING FILL MODIFIER-6
- {0x1DAA1, 0x1DAAF, prCM, gcMn}, // [15] SIGNWRITING ROTATION MODIFIER-2..SIGNWRITING ROTATION MODIFIER-16
- {0x1DF00, 0x1DF09, prAL, gcLl}, // [10] LATIN SMALL LETTER FENG DIGRAPH WITH TRILL..LATIN SMALL LETTER T WITH HOOK AND RETROFLEX HOOK
- {0x1DF0A, 0x1DF0A, prAL, gcLo}, // LATIN LETTER RETROFLEX CLICK WITH RETROFLEX HOOK
- {0x1DF0B, 0x1DF1E, prAL, gcLl}, // [20] LATIN SMALL LETTER ESH WITH DOUBLE BAR..LATIN SMALL LETTER S WITH CURL
- {0x1E000, 0x1E006, prCM, gcMn}, // [7] COMBINING GLAGOLITIC LETTER AZU..COMBINING GLAGOLITIC LETTER ZHIVETE
- {0x1E008, 0x1E018, prCM, gcMn}, // [17] COMBINING GLAGOLITIC LETTER ZEMLJA..COMBINING GLAGOLITIC LETTER HERU
- {0x1E01B, 0x1E021, prCM, gcMn}, // [7] COMBINING GLAGOLITIC LETTER SHTA..COMBINING GLAGOLITIC LETTER YATI
- {0x1E023, 0x1E024, prCM, gcMn}, // [2] COMBINING GLAGOLITIC LETTER YU..COMBINING GLAGOLITIC LETTER SMALL YUS
- {0x1E026, 0x1E02A, prCM, gcMn}, // [5] COMBINING GLAGOLITIC LETTER YO..COMBINING GLAGOLITIC LETTER FITA
- {0x1E100, 0x1E12C, prAL, gcLo}, // [45] NYIAKENG PUACHUE HMONG LETTER MA..NYIAKENG PUACHUE HMONG LETTER W
- {0x1E130, 0x1E136, prCM, gcMn}, // [7] NYIAKENG PUACHUE HMONG TONE-B..NYIAKENG PUACHUE HMONG TONE-D
- {0x1E137, 0x1E13D, prAL, gcLm}, // [7] NYIAKENG PUACHUE HMONG SIGN FOR PERSON..NYIAKENG PUACHUE HMONG SYLLABLE LENGTHENER
- {0x1E140, 0x1E149, prNU, gcNd}, // [10] NYIAKENG PUACHUE HMONG DIGIT ZERO..NYIAKENG PUACHUE HMONG DIGIT NINE
- {0x1E14E, 0x1E14E, prAL, gcLo}, // NYIAKENG PUACHUE HMONG LOGOGRAM NYAJ
- {0x1E14F, 0x1E14F, prAL, gcSo}, // NYIAKENG PUACHUE HMONG CIRCLED CA
- {0x1E290, 0x1E2AD, prAL, gcLo}, // [30] TOTO LETTER PA..TOTO LETTER A
- {0x1E2AE, 0x1E2AE, prCM, gcMn}, // TOTO SIGN RISING TONE
- {0x1E2C0, 0x1E2EB, prAL, gcLo}, // [44] WANCHO LETTER AA..WANCHO LETTER YIH
- {0x1E2EC, 0x1E2EF, prCM, gcMn}, // [4] WANCHO TONE TUP..WANCHO TONE KOINI
- {0x1E2F0, 0x1E2F9, prNU, gcNd}, // [10] WANCHO DIGIT ZERO..WANCHO DIGIT NINE
- {0x1E2FF, 0x1E2FF, prPR, gcSc}, // WANCHO NGUN SIGN
- {0x1E7E0, 0x1E7E6, prAL, gcLo}, // [7] ETHIOPIC SYLLABLE HHYA..ETHIOPIC SYLLABLE HHYO
- {0x1E7E8, 0x1E7EB, prAL, gcLo}, // [4] ETHIOPIC SYLLABLE GURAGE HHWA..ETHIOPIC SYLLABLE HHWE
- {0x1E7ED, 0x1E7EE, prAL, gcLo}, // [2] ETHIOPIC SYLLABLE GURAGE MWI..ETHIOPIC SYLLABLE GURAGE MWEE
- {0x1E7F0, 0x1E7FE, prAL, gcLo}, // [15] ETHIOPIC SYLLABLE GURAGE QWI..ETHIOPIC SYLLABLE GURAGE PWEE
- {0x1E800, 0x1E8C4, prAL, gcLo}, // [197] MENDE KIKAKUI SYLLABLE M001 KI..MENDE KIKAKUI SYLLABLE M060 NYON
- {0x1E8C7, 0x1E8CF, prAL, gcNo}, // [9] MENDE KIKAKUI DIGIT ONE..MENDE KIKAKUI DIGIT NINE
- {0x1E8D0, 0x1E8D6, prCM, gcMn}, // [7] MENDE KIKAKUI COMBINING NUMBER TEENS..MENDE KIKAKUI COMBINING NUMBER MILLIONS
- {0x1E900, 0x1E943, prAL, gcLC}, // [68] ADLAM CAPITAL LETTER ALIF..ADLAM SMALL LETTER SHA
- {0x1E944, 0x1E94A, prCM, gcMn}, // [7] ADLAM ALIF LENGTHENER..ADLAM NUKTA
- {0x1E94B, 0x1E94B, prAL, gcLm}, // ADLAM NASALIZATION MARK
- {0x1E950, 0x1E959, prNU, gcNd}, // [10] ADLAM DIGIT ZERO..ADLAM DIGIT NINE
- {0x1E95E, 0x1E95F, prOP, gcPo}, // [2] ADLAM INITIAL EXCLAMATION MARK..ADLAM INITIAL QUESTION MARK
- {0x1EC71, 0x1ECAB, prAL, gcNo}, // [59] INDIC SIYAQ NUMBER ONE..INDIC SIYAQ NUMBER PREFIXED NINE
- {0x1ECAC, 0x1ECAC, prPO, gcSo}, // INDIC SIYAQ PLACEHOLDER
- {0x1ECAD, 0x1ECAF, prAL, gcNo}, // [3] INDIC SIYAQ FRACTION ONE QUARTER..INDIC SIYAQ FRACTION THREE QUARTERS
- {0x1ECB0, 0x1ECB0, prPO, gcSc}, // INDIC SIYAQ RUPEE MARK
- {0x1ECB1, 0x1ECB4, prAL, gcNo}, // [4] INDIC SIYAQ NUMBER ALTERNATE ONE..INDIC SIYAQ ALTERNATE LAKH MARK
- {0x1ED01, 0x1ED2D, prAL, gcNo}, // [45] OTTOMAN SIYAQ NUMBER ONE..OTTOMAN SIYAQ NUMBER NINETY THOUSAND
- {0x1ED2E, 0x1ED2E, prAL, gcSo}, // OTTOMAN SIYAQ MARRATAN
- {0x1ED2F, 0x1ED3D, prAL, gcNo}, // [15] OTTOMAN SIYAQ ALTERNATE NUMBER TWO..OTTOMAN SIYAQ FRACTION ONE SIXTH
- {0x1EE00, 0x1EE03, prAL, gcLo}, // [4] ARABIC MATHEMATICAL ALEF..ARABIC MATHEMATICAL DAL
- {0x1EE05, 0x1EE1F, prAL, gcLo}, // [27] ARABIC MATHEMATICAL WAW..ARABIC MATHEMATICAL DOTLESS QAF
- {0x1EE21, 0x1EE22, prAL, gcLo}, // [2] ARABIC MATHEMATICAL INITIAL BEH..ARABIC MATHEMATICAL INITIAL JEEM
- {0x1EE24, 0x1EE24, prAL, gcLo}, // ARABIC MATHEMATICAL INITIAL HEH
- {0x1EE27, 0x1EE27, prAL, gcLo}, // ARABIC MATHEMATICAL INITIAL HAH
- {0x1EE29, 0x1EE32, prAL, gcLo}, // [10] ARABIC MATHEMATICAL INITIAL YEH..ARABIC MATHEMATICAL INITIAL QAF
- {0x1EE34, 0x1EE37, prAL, gcLo}, // [4] ARABIC MATHEMATICAL INITIAL SHEEN..ARABIC MATHEMATICAL INITIAL KHAH
- {0x1EE39, 0x1EE39, prAL, gcLo}, // ARABIC MATHEMATICAL INITIAL DAD
- {0x1EE3B, 0x1EE3B, prAL, gcLo}, // ARABIC MATHEMATICAL INITIAL GHAIN
- {0x1EE42, 0x1EE42, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED JEEM
- {0x1EE47, 0x1EE47, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED HAH
- {0x1EE49, 0x1EE49, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED YEH
- {0x1EE4B, 0x1EE4B, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED LAM
- {0x1EE4D, 0x1EE4F, prAL, gcLo}, // [3] ARABIC MATHEMATICAL TAILED NOON..ARABIC MATHEMATICAL TAILED AIN
- {0x1EE51, 0x1EE52, prAL, gcLo}, // [2] ARABIC MATHEMATICAL TAILED SAD..ARABIC MATHEMATICAL TAILED QAF
- {0x1EE54, 0x1EE54, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED SHEEN
- {0x1EE57, 0x1EE57, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED KHAH
- {0x1EE59, 0x1EE59, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED DAD
- {0x1EE5B, 0x1EE5B, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED GHAIN
- {0x1EE5D, 0x1EE5D, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED DOTLESS NOON
- {0x1EE5F, 0x1EE5F, prAL, gcLo}, // ARABIC MATHEMATICAL TAILED DOTLESS QAF
- {0x1EE61, 0x1EE62, prAL, gcLo}, // [2] ARABIC MATHEMATICAL STRETCHED BEH..ARABIC MATHEMATICAL STRETCHED JEEM
- {0x1EE64, 0x1EE64, prAL, gcLo}, // ARABIC MATHEMATICAL STRETCHED HEH
- {0x1EE67, 0x1EE6A, prAL, gcLo}, // [4] ARABIC MATHEMATICAL STRETCHED HAH..ARABIC MATHEMATICAL STRETCHED KAF
- {0x1EE6C, 0x1EE72, prAL, gcLo}, // [7] ARABIC MATHEMATICAL STRETCHED MEEM..ARABIC MATHEMATICAL STRETCHED QAF
- {0x1EE74, 0x1EE77, prAL, gcLo}, // [4] ARABIC MATHEMATICAL STRETCHED SHEEN..ARABIC MATHEMATICAL STRETCHED KHAH
- {0x1EE79, 0x1EE7C, prAL, gcLo}, // [4] ARABIC MATHEMATICAL STRETCHED DAD..ARABIC MATHEMATICAL STRETCHED DOTLESS BEH
- {0x1EE7E, 0x1EE7E, prAL, gcLo}, // ARABIC MATHEMATICAL STRETCHED DOTLESS FEH
- {0x1EE80, 0x1EE89, prAL, gcLo}, // [10] ARABIC MATHEMATICAL LOOPED ALEF..ARABIC MATHEMATICAL LOOPED YEH
- {0x1EE8B, 0x1EE9B, prAL, gcLo}, // [17] ARABIC MATHEMATICAL LOOPED LAM..ARABIC MATHEMATICAL LOOPED GHAIN
- {0x1EEA1, 0x1EEA3, prAL, gcLo}, // [3] ARABIC MATHEMATICAL DOUBLE-STRUCK BEH..ARABIC MATHEMATICAL DOUBLE-STRUCK DAL
- {0x1EEA5, 0x1EEA9, prAL, gcLo}, // [5] ARABIC MATHEMATICAL DOUBLE-STRUCK WAW..ARABIC MATHEMATICAL DOUBLE-STRUCK YEH
- {0x1EEAB, 0x1EEBB, prAL, gcLo}, // [17] ARABIC MATHEMATICAL DOUBLE-STRUCK LAM..ARABIC MATHEMATICAL DOUBLE-STRUCK GHAIN
- {0x1EEF0, 0x1EEF1, prAL, gcSm}, // [2] ARABIC MATHEMATICAL OPERATOR MEEM WITH HAH WITH TATWEEL..ARABIC MATHEMATICAL OPERATOR HAH WITH DAL
- {0x1F000, 0x1F02B, prID, gcSo}, // [44] MAHJONG TILE EAST WIND..MAHJONG TILE BACK
- {0x1F02C, 0x1F02F, prID, gcCn}, // [4] ..
- {0x1F030, 0x1F093, prID, gcSo}, // [100] DOMINO TILE HORIZONTAL BACK..DOMINO TILE VERTICAL-06-06
- {0x1F094, 0x1F09F, prID, gcCn}, // [12] ..
- {0x1F0A0, 0x1F0AE, prID, gcSo}, // [15] PLAYING CARD BACK..PLAYING CARD KING OF SPADES
- {0x1F0AF, 0x1F0B0, prID, gcCn}, // [2] ..
- {0x1F0B1, 0x1F0BF, prID, gcSo}, // [15] PLAYING CARD ACE OF HEARTS..PLAYING CARD RED JOKER
- {0x1F0C0, 0x1F0C0, prID, gcCn}, //
- {0x1F0C1, 0x1F0CF, prID, gcSo}, // [15] PLAYING CARD ACE OF DIAMONDS..PLAYING CARD BLACK JOKER
- {0x1F0D0, 0x1F0D0, prID, gcCn}, //
- {0x1F0D1, 0x1F0F5, prID, gcSo}, // [37] PLAYING CARD ACE OF CLUBS..PLAYING CARD TRUMP-21
- {0x1F0F6, 0x1F0FF, prID, gcCn}, // [10] ..
- {0x1F100, 0x1F10C, prAI, gcNo}, // [13] DIGIT ZERO FULL STOP..DINGBAT NEGATIVE CIRCLED SANS-SERIF DIGIT ZERO
- {0x1F10D, 0x1F10F, prID, gcSo}, // [3] CIRCLED ZERO WITH SLASH..CIRCLED DOLLAR SIGN WITH OVERLAID BACKSLASH
- {0x1F110, 0x1F12D, prAI, gcSo}, // [30] PARENTHESIZED LATIN CAPITAL LETTER A..CIRCLED CD
- {0x1F12E, 0x1F12F, prAL, gcSo}, // [2] CIRCLED WZ..COPYLEFT SYMBOL
- {0x1F130, 0x1F169, prAI, gcSo}, // [58] SQUARED LATIN CAPITAL LETTER A..NEGATIVE CIRCLED LATIN CAPITAL LETTER Z
- {0x1F16A, 0x1F16C, prAL, gcSo}, // [3] RAISED MC SIGN..RAISED MR SIGN
- {0x1F16D, 0x1F16F, prID, gcSo}, // [3] CIRCLED CC..CIRCLED HUMAN FIGURE
- {0x1F170, 0x1F1AC, prAI, gcSo}, // [61] NEGATIVE SQUARED LATIN CAPITAL LETTER A..SQUARED VOD
- {0x1F1AD, 0x1F1AD, prID, gcSo}, // MASK WORK SYMBOL
- {0x1F1AE, 0x1F1E5, prID, gcCn}, // [56] ..
- {0x1F1E6, 0x1F1FF, prRI, gcSo}, // [26] REGIONAL INDICATOR SYMBOL LETTER A..REGIONAL INDICATOR SYMBOL LETTER Z
- {0x1F200, 0x1F202, prID, gcSo}, // [3] SQUARE HIRAGANA HOKA..SQUARED KATAKANA SA
- {0x1F203, 0x1F20F, prID, gcCn}, // [13] ..
- {0x1F210, 0x1F23B, prID, gcSo}, // [44] SQUARED CJK UNIFIED IDEOGRAPH-624B..SQUARED CJK UNIFIED IDEOGRAPH-914D
- {0x1F23C, 0x1F23F, prID, gcCn}, // [4] ..
- {0x1F240, 0x1F248, prID, gcSo}, // [9] TORTOISE SHELL BRACKETED CJK UNIFIED IDEOGRAPH-672C..TORTOISE SHELL BRACKETED CJK UNIFIED IDEOGRAPH-6557
- {0x1F249, 0x1F24F, prID, gcCn}, // [7] ..
- {0x1F250, 0x1F251, prID, gcSo}, // [2] CIRCLED IDEOGRAPH ADVANTAGE..CIRCLED IDEOGRAPH ACCEPT
- {0x1F252, 0x1F25F, prID, gcCn}, // [14] ..
- {0x1F260, 0x1F265, prID, gcSo}, // [6] ROUNDED SYMBOL FOR FU..ROUNDED SYMBOL FOR CAI
- {0x1F266, 0x1F2FF, prID, gcCn}, // [154] ..
- {0x1F300, 0x1F384, prID, gcSo}, // [133] CYCLONE..CHRISTMAS TREE
- {0x1F385, 0x1F385, prEB, gcSo}, // FATHER CHRISTMAS
- {0x1F386, 0x1F39B, prID, gcSo}, // [22] FIREWORKS..CONTROL KNOBS
- {0x1F39C, 0x1F39D, prAL, gcSo}, // [2] BEAMED ASCENDING MUSICAL NOTES..BEAMED DESCENDING MUSICAL NOTES
- {0x1F39E, 0x1F3B4, prID, gcSo}, // [23] FILM FRAMES..FLOWER PLAYING CARDS
- {0x1F3B5, 0x1F3B6, prAL, gcSo}, // [2] MUSICAL NOTE..MULTIPLE MUSICAL NOTES
- {0x1F3B7, 0x1F3BB, prID, gcSo}, // [5] SAXOPHONE..VIOLIN
- {0x1F3BC, 0x1F3BC, prAL, gcSo}, // MUSICAL SCORE
- {0x1F3BD, 0x1F3C1, prID, gcSo}, // [5] RUNNING SHIRT WITH SASH..CHEQUERED FLAG
- {0x1F3C2, 0x1F3C4, prEB, gcSo}, // [3] SNOWBOARDER..SURFER
- {0x1F3C5, 0x1F3C6, prID, gcSo}, // [2] SPORTS MEDAL..TROPHY
- {0x1F3C7, 0x1F3C7, prEB, gcSo}, // HORSE RACING
- {0x1F3C8, 0x1F3C9, prID, gcSo}, // [2] AMERICAN FOOTBALL..RUGBY FOOTBALL
- {0x1F3CA, 0x1F3CC, prEB, gcSo}, // [3] SWIMMER..GOLFER
- {0x1F3CD, 0x1F3FA, prID, gcSo}, // [46] RACING MOTORCYCLE..AMPHORA
- {0x1F3FB, 0x1F3FF, prEM, gcSk}, // [5] EMOJI MODIFIER FITZPATRICK TYPE-1-2..EMOJI MODIFIER FITZPATRICK TYPE-6
- {0x1F400, 0x1F441, prID, gcSo}, // [66] RAT..EYE
- {0x1F442, 0x1F443, prEB, gcSo}, // [2] EAR..NOSE
- {0x1F444, 0x1F445, prID, gcSo}, // [2] MOUTH..TONGUE
- {0x1F446, 0x1F450, prEB, gcSo}, // [11] WHITE UP POINTING BACKHAND INDEX..OPEN HANDS SIGN
- {0x1F451, 0x1F465, prID, gcSo}, // [21] CROWN..BUSTS IN SILHOUETTE
- {0x1F466, 0x1F478, prEB, gcSo}, // [19] BOY..PRINCESS
- {0x1F479, 0x1F47B, prID, gcSo}, // [3] JAPANESE OGRE..GHOST
- {0x1F47C, 0x1F47C, prEB, gcSo}, // BABY ANGEL
- {0x1F47D, 0x1F480, prID, gcSo}, // [4] EXTRATERRESTRIAL ALIEN..SKULL
- {0x1F481, 0x1F483, prEB, gcSo}, // [3] INFORMATION DESK PERSON..DANCER
- {0x1F484, 0x1F484, prID, gcSo}, // LIPSTICK
- {0x1F485, 0x1F487, prEB, gcSo}, // [3] NAIL POLISH..HAIRCUT
- {0x1F488, 0x1F48E, prID, gcSo}, // [7] BARBER POLE..GEM STONE
- {0x1F48F, 0x1F48F, prEB, gcSo}, // KISS
- {0x1F490, 0x1F490, prID, gcSo}, // BOUQUET
- {0x1F491, 0x1F491, prEB, gcSo}, // COUPLE WITH HEART
- {0x1F492, 0x1F49F, prID, gcSo}, // [14] WEDDING..HEART DECORATION
- {0x1F4A0, 0x1F4A0, prAL, gcSo}, // DIAMOND SHAPE WITH A DOT INSIDE
- {0x1F4A1, 0x1F4A1, prID, gcSo}, // ELECTRIC LIGHT BULB
- {0x1F4A2, 0x1F4A2, prAL, gcSo}, // ANGER SYMBOL
- {0x1F4A3, 0x1F4A3, prID, gcSo}, // BOMB
- {0x1F4A4, 0x1F4A4, prAL, gcSo}, // SLEEPING SYMBOL
- {0x1F4A5, 0x1F4A9, prID, gcSo}, // [5] COLLISION SYMBOL..PILE OF POO
- {0x1F4AA, 0x1F4AA, prEB, gcSo}, // FLEXED BICEPS
- {0x1F4AB, 0x1F4AE, prID, gcSo}, // [4] DIZZY SYMBOL..WHITE FLOWER
- {0x1F4AF, 0x1F4AF, prAL, gcSo}, // HUNDRED POINTS SYMBOL
- {0x1F4B0, 0x1F4B0, prID, gcSo}, // MONEY BAG
- {0x1F4B1, 0x1F4B2, prAL, gcSo}, // [2] CURRENCY EXCHANGE..HEAVY DOLLAR SIGN
- {0x1F4B3, 0x1F4FF, prID, gcSo}, // [77] CREDIT CARD..PRAYER BEADS
- {0x1F500, 0x1F506, prAL, gcSo}, // [7] TWISTED RIGHTWARDS ARROWS..HIGH BRIGHTNESS SYMBOL
- {0x1F507, 0x1F516, prID, gcSo}, // [16] SPEAKER WITH CANCELLATION STROKE..BOOKMARK
- {0x1F517, 0x1F524, prAL, gcSo}, // [14] LINK SYMBOL..INPUT SYMBOL FOR LATIN LETTERS
- {0x1F525, 0x1F531, prID, gcSo}, // [13] FIRE..TRIDENT EMBLEM
- {0x1F532, 0x1F549, prAL, gcSo}, // [24] BLACK SQUARE BUTTON..OM SYMBOL
- {0x1F54A, 0x1F573, prID, gcSo}, // [42] DOVE OF PEACE..HOLE
- {0x1F574, 0x1F575, prEB, gcSo}, // [2] MAN IN BUSINESS SUIT LEVITATING..SLEUTH OR SPY
- {0x1F576, 0x1F579, prID, gcSo}, // [4] DARK SUNGLASSES..JOYSTICK
- {0x1F57A, 0x1F57A, prEB, gcSo}, // MAN DANCING
- {0x1F57B, 0x1F58F, prID, gcSo}, // [21] LEFT HAND TELEPHONE RECEIVER..TURNED OK HAND SIGN
- {0x1F590, 0x1F590, prEB, gcSo}, // RAISED HAND WITH FINGERS SPLAYED
- {0x1F591, 0x1F594, prID, gcSo}, // [4] REVERSED RAISED HAND WITH FINGERS SPLAYED..REVERSED VICTORY HAND
- {0x1F595, 0x1F596, prEB, gcSo}, // [2] REVERSED HAND WITH MIDDLE FINGER EXTENDED..RAISED HAND WITH PART BETWEEN MIDDLE AND RING FINGERS
- {0x1F597, 0x1F5D3, prID, gcSo}, // [61] WHITE DOWN POINTING LEFT HAND INDEX..SPIRAL CALENDAR PAD
- {0x1F5D4, 0x1F5DB, prAL, gcSo}, // [8] DESKTOP WINDOW..DECREASE FONT SIZE SYMBOL
- {0x1F5DC, 0x1F5F3, prID, gcSo}, // [24] COMPRESSION..BALLOT BOX WITH BALLOT
- {0x1F5F4, 0x1F5F9, prAL, gcSo}, // [6] BALLOT SCRIPT X..BALLOT BOX WITH BOLD CHECK
- {0x1F5FA, 0x1F5FF, prID, gcSo}, // [6] WORLD MAP..MOYAI
- {0x1F600, 0x1F644, prID, gcSo}, // [69] GRINNING FACE..FACE WITH ROLLING EYES
- {0x1F645, 0x1F647, prEB, gcSo}, // [3] FACE WITH NO GOOD GESTURE..PERSON BOWING DEEPLY
- {0x1F648, 0x1F64A, prID, gcSo}, // [3] SEE-NO-EVIL MONKEY..SPEAK-NO-EVIL MONKEY
- {0x1F64B, 0x1F64F, prEB, gcSo}, // [5] HAPPY PERSON RAISING ONE HAND..PERSON WITH FOLDED HANDS
- {0x1F650, 0x1F675, prAL, gcSo}, // [38] NORTH WEST POINTING LEAF..SWASH AMPERSAND ORNAMENT
- {0x1F676, 0x1F678, prQU, gcSo}, // [3] SANS-SERIF HEAVY DOUBLE TURNED COMMA QUOTATION MARK ORNAMENT..SANS-SERIF HEAVY LOW DOUBLE COMMA QUOTATION MARK ORNAMENT
- {0x1F679, 0x1F67B, prNS, gcSo}, // [3] HEAVY INTERROBANG ORNAMENT..HEAVY SANS-SERIF INTERROBANG ORNAMENT
- {0x1F67C, 0x1F67F, prAL, gcSo}, // [4] VERY HEAVY SOLIDUS..REVERSE CHECKER BOARD
- {0x1F680, 0x1F6A2, prID, gcSo}, // [35] ROCKET..SHIP
- {0x1F6A3, 0x1F6A3, prEB, gcSo}, // ROWBOAT
- {0x1F6A4, 0x1F6B3, prID, gcSo}, // [16] SPEEDBOAT..NO BICYCLES
- {0x1F6B4, 0x1F6B6, prEB, gcSo}, // [3] BICYCLIST..PEDESTRIAN
- {0x1F6B7, 0x1F6BF, prID, gcSo}, // [9] NO PEDESTRIANS..SHOWER
- {0x1F6C0, 0x1F6C0, prEB, gcSo}, // BATH
- {0x1F6C1, 0x1F6CB, prID, gcSo}, // [11] BATHTUB..COUCH AND LAMP
- {0x1F6CC, 0x1F6CC, prEB, gcSo}, // SLEEPING ACCOMMODATION
- {0x1F6CD, 0x1F6D7, prID, gcSo}, // [11] SHOPPING BAGS..ELEVATOR
- {0x1F6D8, 0x1F6DC, prID, gcCn}, // [5] ..
- {0x1F6DD, 0x1F6EC, prID, gcSo}, // [16] PLAYGROUND SLIDE..AIRPLANE ARRIVING
- {0x1F6ED, 0x1F6EF, prID, gcCn}, // [3] ..
- {0x1F6F0, 0x1F6FC, prID, gcSo}, // [13] SATELLITE..ROLLER SKATE
- {0x1F6FD, 0x1F6FF, prID, gcCn}, // [3] ..
- {0x1F700, 0x1F773, prAL, gcSo}, // [116] ALCHEMICAL SYMBOL FOR QUINTESSENCE..ALCHEMICAL SYMBOL FOR HALF OUNCE
- {0x1F774, 0x1F77F, prID, gcCn}, // [12]