Displaced lane support in traffic lights #1428
Replies: 16 comments 37 replies
-
ArrowDirection vs. LaneDirectionThe basic change that will make displaced lane support possible is to create a new LaneDirection enumeration, which will replace ArrowDirection in traffic light code. This will allow the traffic light code to understand traffic movements other than simple directionality. public enum LaneDirection {
None = 0,
Left = 1,
Forward = 2,
Right = 3,
Turn = 4,
DisplacedLeft = 5,
LeftCrossover = 6,
RightCrossover = 7,
DisplacedRight = 8,
} I have already performed a trial run of creating and using such an enumeration, and in my testing it worked well. It should allow all code not concerned with traffic lights to operate just as it always has. Traffic Light related API and internal methods that calculate this value will need to accept source and target lane indices. The affected code already has this information. Most Traffic Light APIs that use ArrowDirection will be replaced and deprecated. Edit: Existing API methods will not be affected unless a new mode is used, in which case they will interact with it the best that their limitations allow. |
Beta Was this translation helpful? Give feedback.
-
Okay, I'm back on this now, after getting tied up with some other things and distracted for a bit. So I had talked earlier about the LaneDirection enum. Of course this impacts CustomSegmentLight pretty significantly. As I've thought about it the past week, it's occurred to me that I'll already be doing a lot of the work required for lane-based traffic light control, so that's something to keep in mind. When looking at what I want to do to CustomSegmentLight, I think it's worth asking whether the LaneDirection enum really makes sense, or are we better off avoiding the complexity it adds and just going fully lane-based? Here are the highlights of how the class would be revised:
RoadBaseAI.TrafficLightState GetLightState(byte fromLaneIndex, ushort toSegmentId, byte toLaneIndex);
RoadBaseAI.TrafficLightState GetLightState(LaneDirection dir);
Originally, I avoided thinking in terms of controlling traffic lights by lane because @krzychu124 described it as a complete rewrite of the traffic light code. Certainly, it would be a near rewrite of CustomSegmentLight, but my plan already involves tracking lane indices and managing signals by index, so at this point I'm not sure the LaneDirection concept is really buying me anything. |
Beta Was this translation helpful? Give feedback.
-
@krzychu124 @aubergine10 I am an idiot. This was ridiculously easy. stoplights.by.lane.sd.mp4
|
Beta Was this translation helpful? Give feedback.
-
Okay, here is a new plan for grouping displaced lanes separately in CustomSegmentLights.
public enum LaneConfiguration
{
None = 0,
CrossLeft = 1,
CrossRight = 2,
TurnOutOfDisplaced = 4,
TurnIntoDisplaced = 8,
ForwardDisplaced = 16,
}
public record struct VehicleLaneConfiguration(LaneConfiguration laneConfiguration, ExtVehicleType vehicleType)
{
}
That's the high-level view. Here are my proposed info signs: |
Beta Was this translation helpful? Give feedback.
-
Here is a first look at Displaced Lanes. There is still work to do. |
Beta Was this translation helpful? Give feedback.
-
@krzychu124 I am not completely happy with the UI here: Especially the segment coming from the right, the fact that it's a crossover makes it confusing. Here's what I'm thinking as a potential solution:
The net effect of these changes is that in scenarios like the one shown above, the lights governing displaced movements would be in It could be argued that the cross-right movement in this example is really a standard movement, but it is a transition from a displaced to a standard lane. The only way to classify it differently would be through a very complex evaluation and comparison of all the lane connections. |
Beta Was this translation helpful? Give feedback.
-
Beta Was this translation helpful? Give feedback.
-
My apologies if this seems spammy. I wanted to catch everyone who's participated in conversations on this. How does this look? Did I miss anything? @krzychu124 @aubergine10 @kvakvs @chameleon-tbn @kianzarrin Done so far
To Do
|
Beta Was this translation helpful? Give feedback.
-
I don't understand why do we have so many network managers. we have In any case I don't have a strong opinion on this. Both implementations are equal. Do it the way you like it. |
Beta Was this translation helpful? Give feedback.
-
BTW which LaneEnd is Start and which one is End? is it simply based on StartNode of the segment and has nothing to do with the direction of the lane? |
Beta Was this translation helpful? Give feedback.
-
Do you plan to create several small PRs or one very big PR? |
Beta Was this translation helpful? Give feedback.
-
Regarding the remaining to-do items: TTL Copy/Paste - This is basic traffic light functionality that must be included in the initial rollout of this feature. |
Beta Was this translation helpful? Give feedback.
-
#1392 has been rewritten based on this discussion and related development work. |
Beta Was this translation helpful? Give feedback.
-
|
Beta Was this translation helpful? Give feedback.
-
@krzychu124 The more I play with this feature in practice, the more I'm convinced Unfortunately, I've only barely looked at
As an example, here is the alternate logic that would apply when use case 1 is detected: graph TD
Start(Enter Displaced Configuration)
Start-->HowManyIncoming
HowManyIncoming{How many<br/>incoming lanes?}
HowManyIncoming -->|1| Fallback
HowManyIncoming -->|More than 1| LaneCountCheck
LaneCountCheck{Check lane counts}
LaneCountCheck-->|More incoming<br/>than outgoing| Fallback
LaneCountCheck-->|Same incoming<br/>and outgoing| AllDirect
LaneCountCheck-->|More outgoing<br/>than incoming| Allocate
Fallback[Give up and revert</br>to default routing]
Fallback-->End
AllDirect[Make direct crossover<br/>and forward transitions]
AllDirect-->ForwardLaneChanges
Allocate[Allocate crossover and forward lanes<br/>so that orphaned outgoing lanes<br/>of both types are equal]
Allocate-->AllocateDirect
AllocateDirect[Make direct transitions as allocated,<br/>to the innermost lanes]
AllocateDirect-->NewLanes
NewLanes[Make lane-changing transitions<br/>from outermost incoming lanes<br/>to orphaned outgoing lanes]
NewLanes-->ForwardLaneChanges
ForwardLaneChanges[Make lane-changing<br/>forward transitions]
ForwardLaneChanges-->End
End(Done)
|
Beta Was this translation helpful? Give feedback.
-
I have been working on how to add displaced lane support to timed traffic lights. There is code in my fork, but I plan to abandon the current branch and start fresh with the knowledge I've gained so far. Before doing that, I'd like to share what I have so far and get feedback to incorporate into the final version.
This first message will just cover the functionality I have in mind. I'll get into the implementation in later messages.
I have two use cases for displaced lanes. If anyone has more, let me know!
Use Case 1: Displaced Left Turn
Also known as a Continuous Flow Intersection
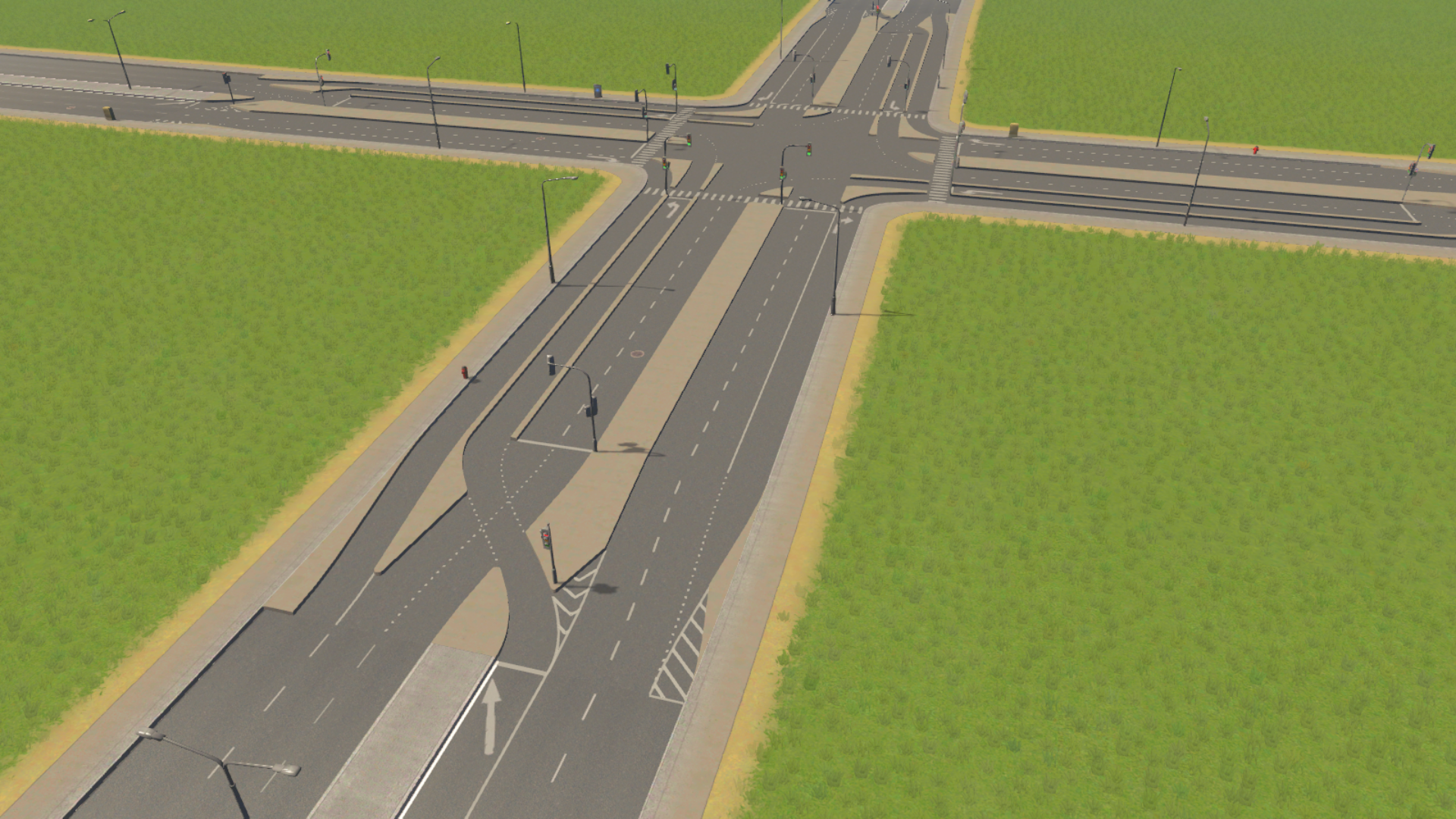
Use Case 2: Turnaround Lane
A slip lane that connects pairs of one-way roads to facilitate U-turns
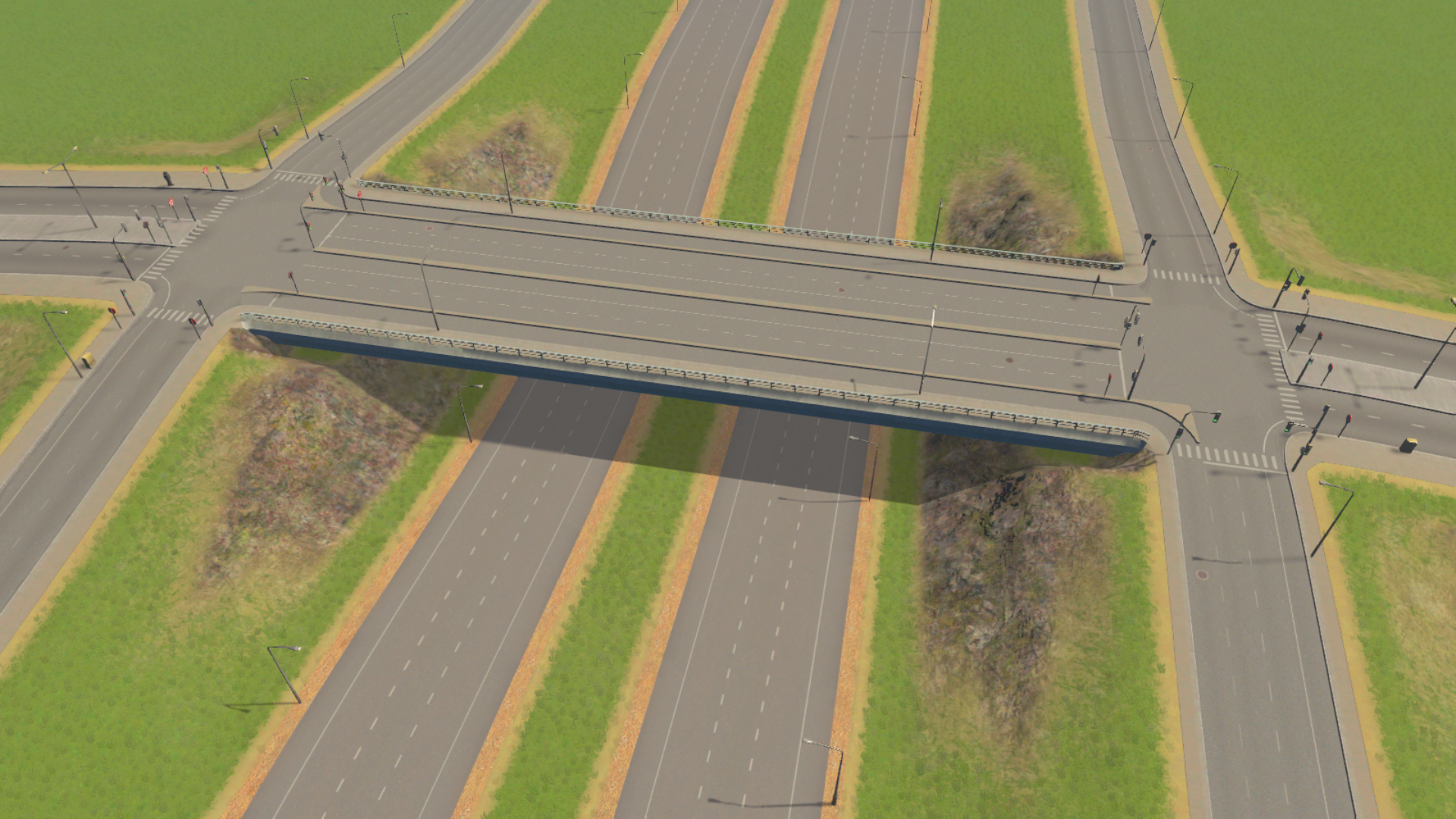
Not a Use Case: Diverging Diamond Interchange
The current traffic light code already supports the diverging diamond interchange, because it does not mix standard and displaced lanes on a single segment.
Functionality Needed
Entry into and exit from displaced lanes needs separate traffic light regulation for these scenarios:
A theoretical condition exists where right-turning traffic turns into or out of a displaced lane. I have not found a useful example of this, but support will probably come naturally since we have to support left-hand traffic.
Proposed Timed Traffic Light Enhancements
Modes
The following modes are currently supported:
The proposal is to add the following modes:
DisplacedTurn Mode
A displaced turn is a left or right turn which either leaves or enters a displaced lane.
In DisplacedTurn mode, the following lights can be controlled. Note that the lights are ordered differently depending on driving direction.
Displaced turns in the direction that is normally a near turn are a questionable use case. On the other hand, there seems to be no reason to omit it, other than to simplify the UI.
Crossover Mode
For left-hand traffic, the definitions of left and right crossover are reversed.
In Crossover mode, the following lights can be controlled. Note that the lights are ordered differently depending on driving direction.
Mode and Light Availability
Availability of the new modes and lights in the UI would be based on the presence of displaced lanes in the involved segments.
Additional Notes
This enhancement affects only the operation of timed traffic lights. It is intentionally limited in scope, and does not impact any other aspects of TMPE's operations except when necessary to facilitate traffic light operation.
Beta Was this translation helpful? Give feedback.
All reactions