|
1 |
| -# 题目描述: 两数之和 II - 输入有序数组 |
| 1 | +# 题目描述: 相交链表 |
2 | 2 |
|
3 |
| -给定一个已按照升序排列 的有序数组,找到两个数使得它们相加之和等于目标数。 |
| 3 | +编写一个程序,找到两个单链表相交的起始节点。 |
4 | 4 |
|
5 |
| -函数应该返回这两个下标值 index1 和 index2,其中 index1 必须小于 index2。 |
6 |
| - |
7 |
| -**说明:** |
8 |
| - - 返回的下标值(index1 和 index2)不是从零开始的。 |
9 |
| - - 你可以假设每个输入只对应唯一的答案,而且你不可以重复使用相同的元素。 |
| 5 | +如下面的两个链表: |
| 6 | +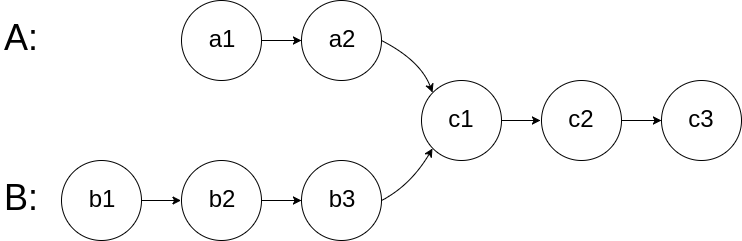 |
| 7 | +在节点 c1 开始相交。 |
10 | 8 |
|
| 9 | +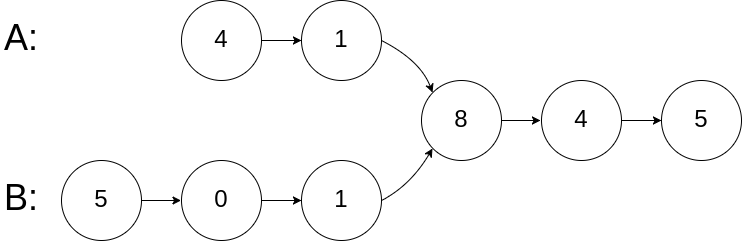 |
11 | 10 |
|
12 | 11 | **示例 1:**
|
13 | 12 | ```
|
14 |
| -输入: numbers = [2, 7, 11, 15], target = 9 |
15 |
| -输出: [1,2] |
16 |
| -解释: 2 与 7 之和等于目标数 9 。因此 index1 = 1, index2 = 2 。 |
| 13 | +输入:intersectVal = 8, listA = [4,1,8,4,5], listB = [5,0,1,8,4,5], skipA = 2, skipB = 3 |
| 14 | +输出:Reference of the node with value = 8 |
| 15 | +输入解释:相交节点的值为 8 (注意,如果两个列表相交则不能为 0)。从各自的表头开始算起,链表 A 为 [4,1,8,4,5],链表 B 为 [5,0,1,8,4,5]。在 A 中,相交节点前有 2 个节点;在 B 中,相交节点前有 3 个节点。 |
17 | 16 | ```
|
18 | 17 |
|
| 18 | + 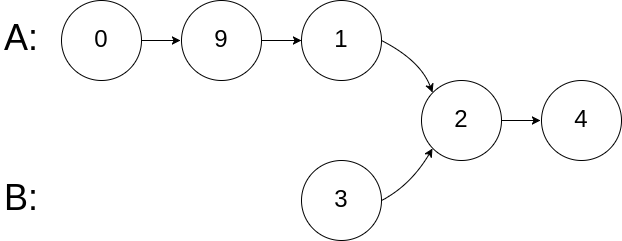 |
| 19 | + |
| 20 | +**示例 2:** |
| 21 | +``` |
| 22 | +输入:intersectVal = 2, listA = [0,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1 |
| 23 | +输出:Reference of the node with value = 2 |
| 24 | +输入解释:相交节点的值为 2 (注意,如果两个列表相交则不能为 0)。从各自的表头开始算起,链表 A 为 [0,9,1,2,4],链表 B 为 [3,2,4]。在 A 中,相交节点前有 3 个节点;在 B 中,相交节点前有 1 个节点。 |
| 25 | +``` |
| 26 | + |
| 27 | + 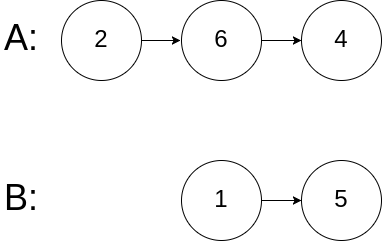 |
| 28 | + |
| 29 | +**示例 3:** |
| 30 | +``` |
| 31 | +输入:intersectVal = 0, listA = [2,6,4], listB = [1,5], skipA = 3, skipB = 2 |
| 32 | +输出:null |
| 33 | +输入解释:从各自的表头开始算起,链表 A 为 [2,6,4],链表 B 为 [1,5]。由于这两个链表不相交,所以 intersectVal 必须为 0,而 skipA 和 skipB 可以是任意值。 |
| 34 | +解释:这两个链表不相交,因此返回 null。 |
| 35 | +``` |
| 36 | + |
| 37 | + |
19 | 38 | # 解题思路:
|
20 |
| -此题与剑指offer第57题题目一类似 |
| 39 | +此题与剑指offer第52题题目一类似 |
21 | 40 |
|
22 | 41 | 方法一:暴力遍历
|
23 | 42 |
|
24 |
| -方法二:哈希表 |
25 |
| - |
26 |
| -方法三:双指针 |
| 43 | +方法二:找出2个链表的长度,然后让长的先走两个链表的长度差,然后再一起走(因为2个链表用公共的尾部)。如果存在共同节点的话,那么从该节点,两个链表之后的元素都是相同的。 也就是说两个链表从尾部往前到某个点,节点都是一样的。 |
27 | 44 |
|
28 | 45 |
|
29 | 46 | # 时间复杂度:
|
30 |
| - 方法一:O(n<sup>2) |
31 |
| - |
32 |
| - 方法二:O(n) |
| 47 | + 方法一:O(nm) |
33 | 48 |
|
34 |
| - 方法三:O(n) |
| 49 | + 方法二:O(n+m) |
35 | 50 | # 空间复杂度
|
36 | 51 | 方法一:O(1)
|
37 | 52 |
|
38 |
| - 方法二:O(n) |
| 53 | + 方法二:O(1) |
39 | 54 |
|
40 |
| - 方法三:O(1) |
41 | 55 |
|
42 | 56 | # 代码
|
43 | 57 |
|
44 | 58 | ## [C++](./Intersection-Of-Two-Linked-Lists.cpp):
|
45 | 59 |
|
46 |
| -### 方法一: 暴力法 |
| 60 | +### 方法一: 暴力遍历法 |
47 | 61 | ```c++
|
| 62 | +/** |
| 63 | + * Definition for singly-linked list. |
| 64 | + * struct ListNode { |
| 65 | + * int val; |
| 66 | + * ListNode *next; |
| 67 | + * ListNode(int x) : val(x), next(NULL) {} |
| 68 | + * }; |
| 69 | + */ |
48 | 70 | class Solution {
|
49 | 71 | public:
|
50 |
| - vector<int> twoSum(vector<int>& numbers, int target) { |
51 |
| - vector <int> res (2,0); |
52 |
| - if (numbers.empty()) |
53 |
| - return res; |
54 |
| - int n = numbers.size(); |
55 |
| - for (int i=0;i<n;i++) |
| 72 | + ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) { |
| 73 | + if (headA==NULL || headB == NULL) return NULL; |
| 74 | + ListNode* p = headA; |
| 75 | + ListNode* q = headB; |
| 76 | + while(p) |
56 | 77 | {
|
57 |
| - for (int j = i+1; j<n;j++) |
| 78 | + q = headB; |
| 79 | + while(q) |
58 | 80 | {
|
59 |
| - if (numbers[i]+numbers[j]==target) |
| 81 | + if (p->val==q->val) |
60 | 82 | {
|
61 |
| - res[0] = numbers[i]; |
62 |
| - res[1] = numbers[j]; |
63 |
| - break; |
| 83 | + return p; |
| 84 | + } |
| 85 | + else |
| 86 | + { |
| 87 | + q = q->next; |
64 | 88 | }
|
65 | 89 | }
|
| 90 | + p = p->next; |
66 | 91 | }
|
67 |
| - return res; |
| 92 | + return NULL; |
68 | 93 | }
|
69 | 94 | };
|
70 | 95 | ```
|
71 | 96 |
|
72 |
| -### 方法二: 哈希表 |
| 97 | +### 方法二: |
73 | 98 | ```c++
|
| 99 | +/** |
| 100 | + * Definition for singly-linked list. |
| 101 | + * struct ListNode { |
| 102 | + * int val; |
| 103 | + * ListNode *next; |
| 104 | + * ListNode(int x) : val(x), next(NULL) {} |
| 105 | + * }; |
| 106 | + */ |
74 | 107 | class Solution {
|
75 | 108 | public:
|
76 |
| - vector<int> twoSum(vector<int>& numbers, int target) { |
77 |
| - vector <int> res (2,0); |
78 |
| - if (numbers.empty()) |
79 |
| - return res; |
80 |
| - int n = numbers.size(); |
81 |
| - unordered_map<int,int> record; |
82 |
| - for (int i=0;i<n;i++) |
| 109 | + ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) { |
| 110 | + if (headA==NULL || headB==NULL) return NULL; |
| 111 | + ListNode* p = headA; |
| 112 | + ListNode* q = headB; |
| 113 | + int n=0,m=0; |
| 114 | + while(p) |
83 | 115 | {
|
84 |
| - int complement = target -numbers[i]; |
85 |
| - if (record.find(complement)!=record.end()) |
86 |
| - { |
87 |
| - res[0] = complement; |
88 |
| - res[1] = nums[i]; |
89 |
| - break; |
90 |
| - } |
91 |
| - record[numbers[i]] = i; |
| 116 | + n++; |
| 117 | + p=p->next; |
92 | 118 | }
|
93 |
| - return res; |
94 |
| - } |
95 |
| -}; |
96 |
| -``` |
97 |
| - |
98 |
| -### 方法三:双指针 |
99 |
| -```c++ |
100 |
| -class Solution { |
101 |
| -public: |
102 |
| - vector<int> twoSum(vector<int>& nums, int target) { |
103 |
| - vector <int> res (2,0); |
104 |
| - if (nums.empty()) |
105 |
| - return res; |
106 |
| - int n = nums.size(); |
107 |
| - int left = 0; |
108 |
| - int right = n-1; |
109 |
| - while (right>left) |
| 119 | + while(q) |
110 | 120 | {
|
111 |
| - if (nums[left]+nums[right]==target) |
112 |
| - { |
113 |
| - res[0] = nums[left]; |
114 |
| - res[1] = nums[right]; |
115 |
| - break; |
116 |
| - } |
117 |
| - if (nums[left]+nums[right]>target) |
118 |
| - { |
119 |
| - right--; |
120 |
| - } |
121 |
| - if (nums[left]+nums[right]<target) |
122 |
| - { |
123 |
| - left++; |
124 |
| - } |
| 121 | + m++; |
| 122 | + q=q->next; |
125 | 123 | }
|
| 124 | + p = headA; |
| 125 | + q = headB; |
| 126 | + int k = 0; |
| 127 | + if (n>=m) |
| 128 | + { |
| 129 | + k=n-m; |
| 130 | + } |
| 131 | + else |
| 132 | + { |
| 133 | + k=m-n; |
| 134 | + p=headB; |
| 135 | + q=headA; |
| 136 | + } |
| 137 | + for (int i=0;i<k;i++) |
| 138 | + { |
| 139 | + p = p->next; |
| 140 | + } |
| 141 | + while(p && q && p!=q) |
| 142 | + { |
| 143 | + p = p->next; |
| 144 | + q = q->next; |
| 145 | + } |
| 146 | + ListNode* res = p; |
126 | 147 | return res;
|
127 | 148 | }
|
128 | 149 | };
|
129 | 150 | ```
|
130 |
| -
|
131 |
| -
|
132 | 151 | ## [Python:](https://github.com/bryceustc/LeetCode_Note/blob/master/python/Intersection-Of-Two-Linked-Lists/Intersection-Of-Two-Linked-Lists.py)
|
133 |
| -### 方法一:暴力法 |
| 152 | +### 方法一:暴力遍历法 |
134 | 153 | ```python
|
| 154 | +# -*- coding:utf-8 -*- |
| 155 | +# class ListNode: |
| 156 | +# def __init__(self, x): |
| 157 | +# self.val = x |
| 158 | +# self.next = None |
135 | 159 | class Solution:
|
136 |
| - def twoSum(self, nums, target): |
| 160 | + def FindFirstCommonNode(self, l1, l2): |
137 | 161 | # write code here
|
138 |
| - res = [] |
139 |
| - n = len(nums) |
140 |
| - if n==0: |
141 |
| - return res |
142 |
| - for i in range(n): |
143 |
| - for j in range(i+1,n): |
144 |
| - if nums[i]+nums[j]==target: |
145 |
| - res.append(nums[i]) |
146 |
| - res.append(nums[j]) |
147 |
| - return res |
148 |
| - return res |
| 162 | + if l1==None or l2 == None: |
| 163 | + return None |
| 164 | + p = l1 |
| 165 | + q = l2 |
| 166 | + while p: |
| 167 | + q = l2 |
| 168 | + while q: |
| 169 | + if p.val==q.val: |
| 170 | + return p |
| 171 | + else: |
| 172 | + q = q.next |
| 173 | + p = p.next |
| 174 | + return None |
149 | 175 | ```
|
150 |
| -### 方法二 :哈希表 |
| 176 | +### 方法二 : |
151 | 177 | ```python
|
152 |
| -class Solution: |
153 |
| - def twoSum(self, nums, target): |
154 |
| - # write code here |
155 |
| - res = [] |
156 |
| - record = {} |
157 |
| - n = len(nums) |
158 |
| - if n==0: |
159 |
| - return res |
160 |
| - for index,num in enumerate (nums): |
161 |
| - complement = target - num |
162 |
| - if complement in record: |
163 |
| - res.append(complement) |
164 |
| - res.append(num) |
165 |
| - return res |
166 |
| - record[num] = index |
167 |
| - return res |
168 |
| -``` |
| 178 | +# Definition for singly-linked list. |
| 179 | +# class ListNode: |
| 180 | +# def __init__(self, x): |
| 181 | +# self.val = x |
| 182 | +# self.next = None |
169 | 183 |
|
170 |
| -### 方法三 : 双指针 |
171 |
| -```python |
172 | 184 | class Solution:
|
173 |
| - def twoSum(self, nums, target): |
174 |
| - # write code here |
175 |
| - res = [] |
176 |
| - n = len(nums) |
177 |
| - left = 0 |
178 |
| - right = n-1 |
179 |
| - while right>left: |
180 |
| - if nums[left]+nums[right]==target: |
181 |
| - res.append(nums[left]) |
182 |
| - res.append(nums[right]) |
183 |
| - return res |
184 |
| - if nums[left]+nums[right]>target: |
185 |
| - right-=1 |
186 |
| - else: |
187 |
| - left+=1 |
| 185 | + def getIntersectionNode(self, headA: ListNode, headB: ListNode) -> ListNode: |
| 186 | + if headA==None or headB == None: |
| 187 | + return None |
| 188 | + p = headA |
| 189 | + q = headB |
| 190 | + n =0 |
| 191 | + m=0 |
| 192 | + while p: |
| 193 | + n+=1 |
| 194 | + p = p.next |
| 195 | + while q: |
| 196 | + m+=1 |
| 197 | + q = q.next |
| 198 | + p = headA |
| 199 | + q = headB |
| 200 | + k = 0 |
| 201 | + if n>=m: |
| 202 | + k = n-m |
| 203 | + else: |
| 204 | + p = headB |
| 205 | + q = headA |
| 206 | + k = m-n |
| 207 | + for i in range(k): |
| 208 | + p = p.next |
| 209 | + while p and q and p!=q: |
| 210 | + p = p.next |
| 211 | + q = q.next |
| 212 | + res = p |
188 | 213 | return res
|
189 | 214 | ```
|
190 | 215 |
|
191 |
| - |
192 |
| - |
193 | 216 | # 参考
|
194 | 217 | - [剑指offer第52题-两个链表的第一个公共节点](https://github.com/bryceustc/CodingInterviews/blob/master/FirstCommonNodesInLists/README.md)
|
195 | 218 |
|
0 commit comments