Commit f0377b2
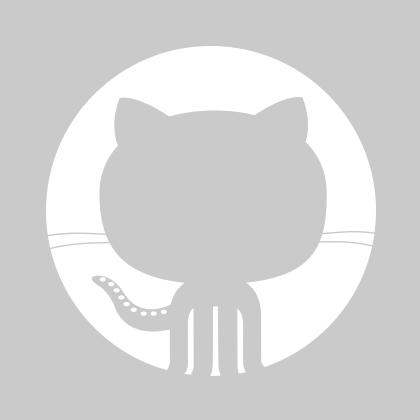
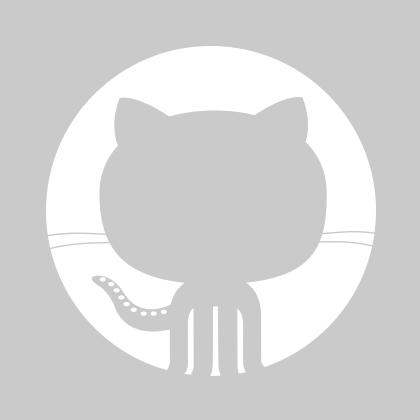
danrubel
commit-bot@chromium.org
1 parent aeb8731 commit f0377b2
File tree
8 files changed
+391
-41
lines changed- pkg
- analyzer
- lib/src
- dart/analysis
- fasta
- test/generated
- front_end
- lib/src/fasta/parser
- test/fasta/parser
8 files changed
+391
-41
lines changedLines changed: 2 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
94 | 94 |
| |
95 | 95 |
| |
96 | 96 |
| |
97 |
| - | |
| 97 | + | |
| 98 | + | |
98 | 99 |
| |
99 | 100 |
| |
100 | 101 |
| |
|
Lines changed: 5 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
967 | 967 |
| |
968 | 968 |
| |
969 | 969 |
| |
970 |
| - | |
| 970 | + | |
971 | 971 |
| |
972 | 972 |
| |
973 | 973 |
| |
| |||
1128 | 1128 |
| |
1129 | 1129 |
| |
1130 | 1130 |
| |
1131 |
| - | |
| 1131 | + | |
1132 | 1132 |
| |
1133 | 1133 |
| |
1134 | 1134 |
| |
| |||
2093 | 2093 |
| |
2094 | 2094 |
| |
2095 | 2095 |
| |
2096 |
| - | |
| 2096 | + | |
2097 | 2097 |
| |
2098 | 2098 |
| |
2099 | 2099 |
| |
2100 | 2100 |
| |
2101 | 2101 |
| |
2102 | 2102 |
| |
2103 | 2103 |
| |
| 2104 | + | |
| 2105 | + | |
2104 | 2106 |
| |
2105 | 2107 |
| |
2106 | 2108 |
| |
|
Lines changed: 70 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
| 17 | + | |
17 | 18 |
| |
18 | 19 |
| |
19 | 20 |
| |
| |||
35 | 36 |
| |
36 | 37 |
| |
37 | 38 |
| |
| 39 | + | |
38 | 40 |
| |
39 | 41 |
| |
40 | 42 |
| |
| |||
1420 | 1422 |
| |
1421 | 1423 |
| |
1422 | 1424 |
| |
| 1425 | + | |
| 1426 | + | |
| 1427 | + | |
| 1428 | + | |
| 1429 | + | |
| 1430 | + | |
| 1431 | + | |
| 1432 | + | |
| 1433 | + | |
| 1434 | + | |
| 1435 | + | |
| 1436 | + | |
| 1437 | + | |
| 1438 | + | |
| 1439 | + | |
| 1440 | + | |
| 1441 | + | |
| 1442 | + | |
| 1443 | + | |
| 1444 | + | |
| 1445 | + | |
| 1446 | + | |
| 1447 | + | |
| 1448 | + | |
| 1449 | + | |
| 1450 | + | |
| 1451 | + | |
| 1452 | + | |
| 1453 | + | |
| 1454 | + | |
| 1455 | + | |
| 1456 | + | |
| 1457 | + | |
| 1458 | + | |
| 1459 | + | |
| 1460 | + | |
| 1461 | + | |
| 1462 | + | |
| 1463 | + | |
| 1464 | + | |
| 1465 | + | |
| 1466 | + | |
| 1467 | + | |
| 1468 | + | |
| 1469 | + | |
| 1470 | + | |
| 1471 | + | |
| 1472 | + | |
| 1473 | + | |
| 1474 | + | |
| 1475 | + | |
| 1476 | + | |
| 1477 | + | |
| 1478 | + | |
1423 | 1479 |
| |
1424 | 1480 |
| |
1425 | 1481 |
| |
| 1482 | + | |
1426 | 1483 |
| |
1427 | 1484 |
| |
1428 | 1485 |
| |
1429 | 1486 |
| |
1430 | 1487 |
| |
1431 | 1488 |
| |
| 1489 | + | |
1432 | 1490 |
| |
1433 | 1491 |
| |
1434 | 1492 |
| |
1435 | 1493 |
| |
| 1494 | + | |
| 1495 | + | |
| 1496 | + | |
| 1497 | + | |
| 1498 | + | |
| 1499 | + | |
1436 | 1500 |
| |
1437 | 1501 |
| |
| 1502 | + | |
1438 | 1503 |
| |
1439 | 1504 |
| |
1440 | 1505 |
| |
| 1506 | + | |
| 1507 | + | |
| 1508 | + | |
| 1509 | + | |
| 1510 | + | |
1441 | 1511 |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1981 | 1981 |
| |
1982 | 1982 |
| |
1983 | 1983 |
| |
1984 |
| - | |
| 1984 | + | |
1985 | 1985 |
| |
1986 | 1986 |
| |
1987 | 1987 |
| |
|
Lines changed: 18 additions & 10 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4500 | 4500 |
| |
4501 | 4501 |
| |
4502 | 4502 |
| |
4503 |
| - | |
4504 |
| - | |
4505 |
| - | |
4506 |
| - | |
4507 |
| - | |
| 4503 | + | |
4508 | 4504 |
| |
4509 | 4505 |
| |
4510 | 4506 |
| |
| |||
4886 | 4882 |
| |
4887 | 4883 |
| |
4888 | 4884 |
| |
4889 |
| - | |
4890 |
| - | |
| 4885 | + | |
| 4886 | + | |
| 4887 | + | |
| 4888 | + | |
4891 | 4889 |
| |
4892 | 4890 |
| |
4893 | 4891 |
| |
| |||
4901 | 4899 |
| |
4902 | 4900 |
| |
4903 | 4901 |
| |
4904 |
| - | |
4905 |
| - | |
| 4902 | + | |
| 4903 | + | |
| 4904 | + | |
| 4905 | + | |
4906 | 4906 |
| |
4907 | 4907 |
| |
4908 | 4908 |
| |
| |||
4921 | 4921 |
| |
4922 | 4922 |
| |
4923 | 4923 |
| |
4924 |
| - | |
| 4924 | + | |
| 4925 | + | |
| 4926 | + | |
| 4927 | + | |
| 4928 | + | |
4925 | 4929 |
| |
4926 | 4930 |
| |
4927 | 4931 |
| |
| |||
5042 | 5046 |
| |
5043 | 5047 |
| |
5044 | 5048 |
| |
| 5049 | + | |
| 5050 | + | |
| 5051 | + | |
| 5052 | + | |
5045 | 5053 |
| |
5046 | 5054 |
| |
5047 | 5055 |
| |
|
Lines changed: 36 additions & 9 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
22 |
| - | |
| 22 | + | |
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
27 |
| - | |
| 26 | + | |
| 27 | + | |
28 | 28 |
| |
29 | 29 |
| |
30 | 30 |
| |
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
44 | 51 |
| |
45 | 52 |
| |
46 | 53 |
| |
| |||
199 | 206 |
| |
200 | 207 |
| |
201 | 208 |
| |
202 |
| - | |
203 |
| - | |
204 |
| - | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
| 212 | + | |
| 213 | + | |
205 | 214 |
| |
206 |
| - | |
207 |
| - | |
| 215 | + | |
| 216 | + | |
| 217 | + | |
| 218 | + | |
208 | 219 |
| |
| 220 | + | |
| 221 | + | |
209 | 222 |
| |
210 | 223 |
| |
211 | 224 |
| |
| |||
255 | 268 |
| |
256 | 269 |
| |
257 | 270 |
| |
258 |
| - | |
| 271 | + | |
| 272 | + | |
| 273 | + | |
| 274 | + | |
| 275 | + | |
| 276 | + | |
| 277 | + | |
| 278 | + | |
| 279 | + | |
| 280 | + | |
| 281 | + | |
| 282 | + | |
| 283 | + | |
| 284 | + | |
| 285 | + | |
259 | 286 |
| |
260 | 287 |
| |
261 | 288 |
| |
|
0 commit comments