-
Notifications
You must be signed in to change notification settings - Fork 22
/
Copy path3745.brightest-position-on-street.py
65 lines (62 loc) · 2.09 KB
/
3745.brightest-position-on-street.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
# Tag: Prefix Sum Array, Array, Line Sweep
# Time: O(N)
# Space: O(N)
# Ref: Leetcode-2237
# Note: -
# A street has a number of streetlights whose coordinates are given by an array of `lights`, each `lights[i] = [position, range]` denoting the streetlight whose coordinates are at `position` and which can illuminate the range `[position - range, position + range]` including the boundary points.
#
# When a position `p`, exists that is illuminated by more than one streetlight, this position is a little brighter, now given `lights`, return the **brightest horizontal coordinate position**, and **if more than one position with the same brightness exists, return the one with the smallest coordinates.**
#
# Example 1:
# ```
# Input:
# lights = [[-3, 2], [1, 2], [3, 2]]
# Output:
# -1
# Explanation:
# The first streetlight illuminates the range [-5, -1]
# The second streetlight illuminates the range [-1, 3]
# The third streetlight illuminates the range [1, 5]
# Positions -1, 1, 2, 3 are all illuminated by two streetlights
# So we return the smallest coordinate, -1
# ```
#
# 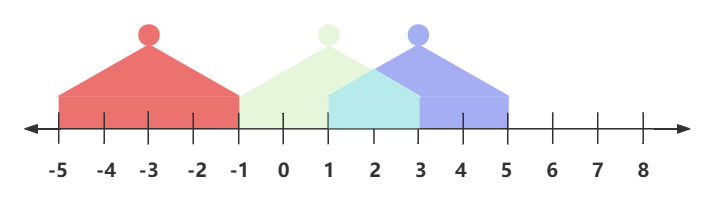
#
# Example 2:
# ```
# Input:
# lights = [[1, 0], [0, 1]]
# Output:
# 1
# ```
#
# $1 \leq lights.length \leq 10^5$
# $lights[i].length == 2$
# $-10^8 \leq position \leq 10^8$
# $0 \leq range \leq 10^8$
from typing import (
List,
)
from collections import defaultdict
class Solution:
"""
@param lights: Location and extent of illumination of street lights
@return: The brightest position
"""
def brightest_position(self, lights: List[List[int]]) -> int:
# write your code here
lines = defaultdict(int)
for p, r in lights:
lines[p - r] += 1
lines[p + r + 1] -= 1
print(lines)
prefix = 0
brightest = 0
res = 0
for pos in sorted(lines.keys()):
prefix += lines[pos]
if prefix > brightest:
brightest = prefix
res = pos
return res