Commit 6518079
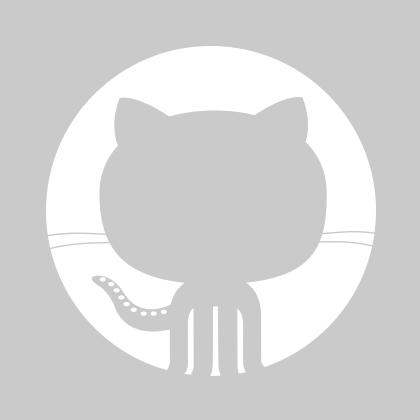
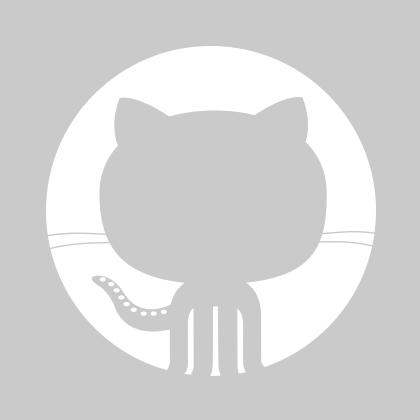
Jack Carrig
Jack Carrig
1 parent 5bd643e commit 6518079
File tree
7 files changed
+208
-70
lines changed- public
- javascripts
- stylesheets
- views
7 files changed
+208
-70
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
| 2 | + |
+62-41
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
34 | 34 |
| |
35 | 35 |
| |
36 | 36 |
| |
| 37 | + | |
37 | 38 |
| |
38 | 39 |
| |
39 |
| - | |
40 |
| - | |
| 40 | + | |
| 41 | + | |
41 | 42 |
| |
42 |
| - | |
| 43 | + | |
43 | 44 |
| |
44 | 45 |
| |
45 | 46 |
| |
46 |
| - | |
47 |
| - | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
48 | 53 |
| |
49 | 54 |
| |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 |
| - | |
| 55 | + | |
82 | 56 |
| |
83 | 57 |
| |
84 | 58 |
| |
85 | 59 |
| |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
86 | 104 |
| |
87 |
| - | |
| 105 | + | |
88 | 106 |
| |
89 | 107 |
| |
90 |
| - | |
| 108 | + | |
91 | 109 |
| |
92 |
| - | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
93 | 114 |
| |
94 | 115 |
| |
95 | 116 |
| |
96 | 117 |
| |
97 | 118 |
| |
98 | 119 |
| |
99 |
| - | |
| 120 | + | |
100 | 121 |
| |
101 | 122 |
| |
102 | 123 |
| |
|
+19-13
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
52 | 52 |
| |
53 | 53 |
| |
54 | 54 |
| |
55 |
| - | |
| 55 | + | |
56 | 56 |
| |
57 | 57 |
| |
58 | 58 |
| |
59 | 59 |
| |
60 | 60 |
| |
61 | 61 |
| |
62 |
| - | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
63 | 69 |
| |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
74 | 80 |
| |
75 | 81 |
| |
76 |
| - | |
| 82 | + | |
77 | 83 |
| |
78 | 84 |
| |
79 | 85 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
6 | 35 |
| |
7 | 36 |
| |
8 | 37 |
| |
|
Some generated files are not rendered by default. Learn more about customizing how changed files appear on GitHub.
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
3 | 8 |
| |
| 9 | + | |
4 | 10 |
| |
5 | 11 |
| |
6 | 12 |
| |
7 | 13 |
| |
| 14 | + | |
| 15 | + | |
| 16 | + | |
8 | 17 |
| |
9 | 18 |
| |
10 | 19 |
| |
11 | 20 |
| |
12 | 21 |
| |
13 | 22 |
| |
| 23 | + | |
| 24 | + | |
14 | 25 |
| |
15 | 26 |
| |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
16 | 76 |
| |
17 | 77 |
| |
18 | 78 |
| |
| |||
42 | 102 |
| |
43 | 103 |
| |
44 | 104 |
| |
45 |
| - | |
46 | 105 |
| |
47 | 106 |
| |
48 | 107 |
| |
49 |
| - | |
50 | 108 |
| |
51 | 109 |
| |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
52 | 115 |
| |
53 |
| - | |
54 | 116 |
| |
55 | 117 |
| |
56 | 118 |
| |
57 | 119 |
| |
58 | 120 |
| |
59 | 121 |
| |
| 122 | + |
+15-10
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + |
0 commit comments