You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
package generics
type Number interface {
int64 | float64
}
func SumInts(m map[string]int64) int64 {
var s int64
for _, v := range m {
s += v
}
return s
}
func SumFloats(m map[string]float64) float64 {
var s float64
for _, v := range m {
s += v
}
return s
}
func SumIntsOrFloats[K comparable, V int64 | float64](m map[K]V) V {
var s V
for _, v := range m {
s += v
}
return s
}
func SumNumbers[K comparable, V Number](m map[K]V) V {
var s V
for _, v := range m {
s += v
}
return s
}
The generic function is inside the red rectangle. We can see the expected should be like on the green rectangle.
The text was updated successfully, but these errors were encountered:
Users who use vscode-go extension can enable richer syntax highlighting through the semantic tokens provided by gopls. But I think supporting basic syntax highlighting for Go by default (without extra extension installation or running gopls process which isn't supported in web-based IDEs like github.dev or vscode.dev) will be ideal.
Originally from @uudashr in microsoft/vscode#146313
Steps to Reproduce:
math.go
The generic function is inside the red rectangle. We can see the expected should be like on the green rectangle.
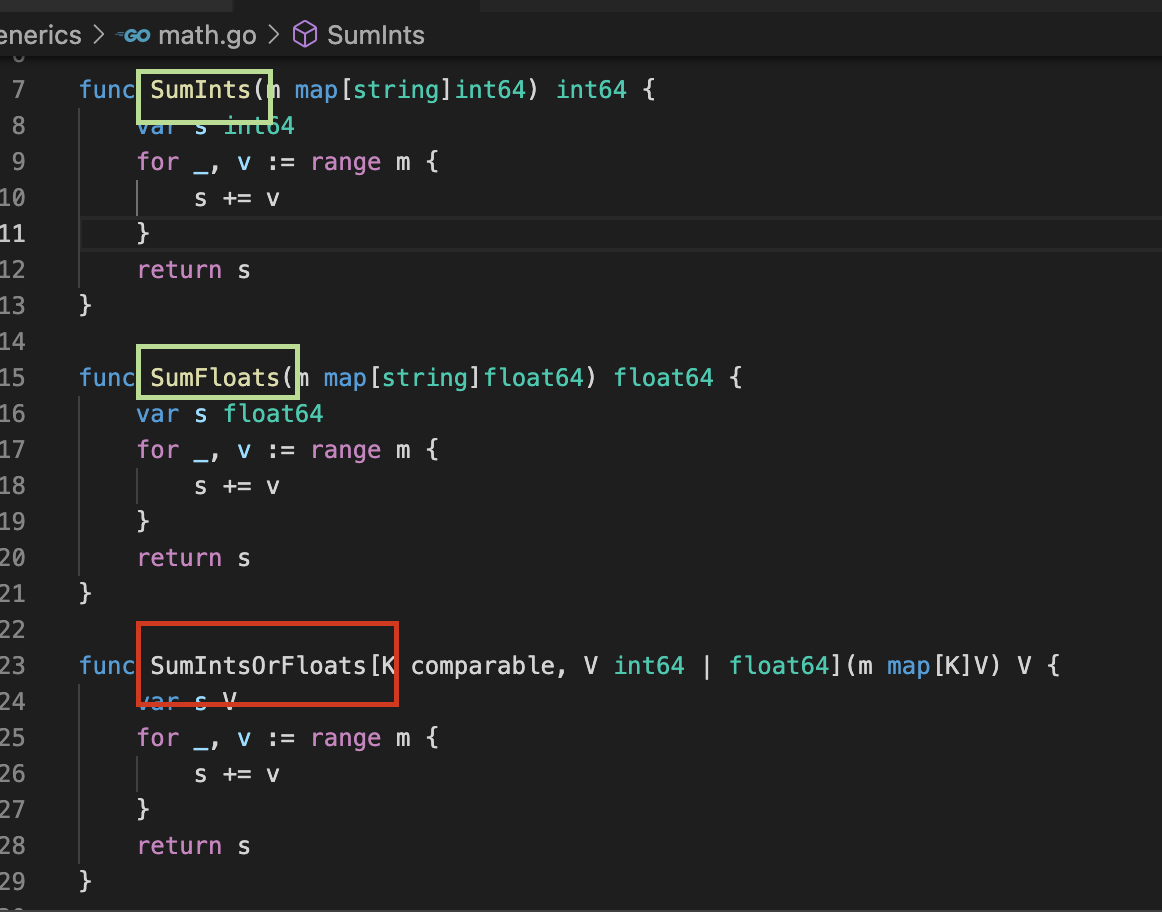
The text was updated successfully, but these errors were encountered: