Output errors after setting text red, then normal while in raw mode #8750
Labels
Issue-Question
For questions or discussion
Needs-Tag-Fix
Doesn't match tag requirements
Resolution-Answered
Related to questions that have been answered
Environment
Microsoft Windows [Version 10.0.19041.685]
Windows Terminal Version: 1.4.3243.0
Microsoft Visual Studio Community 2019 Version 16.8.3
Steps to reproduce
Expected behavior
I expect to see as output in all 3 cases:
In the case of the Powershell tab I also expect that when 'exit' is typed it will be echoed to the right of the prompt after the above output.
Actual behavior
In all 3 cases, the final "This text should be normal" line is truncated.
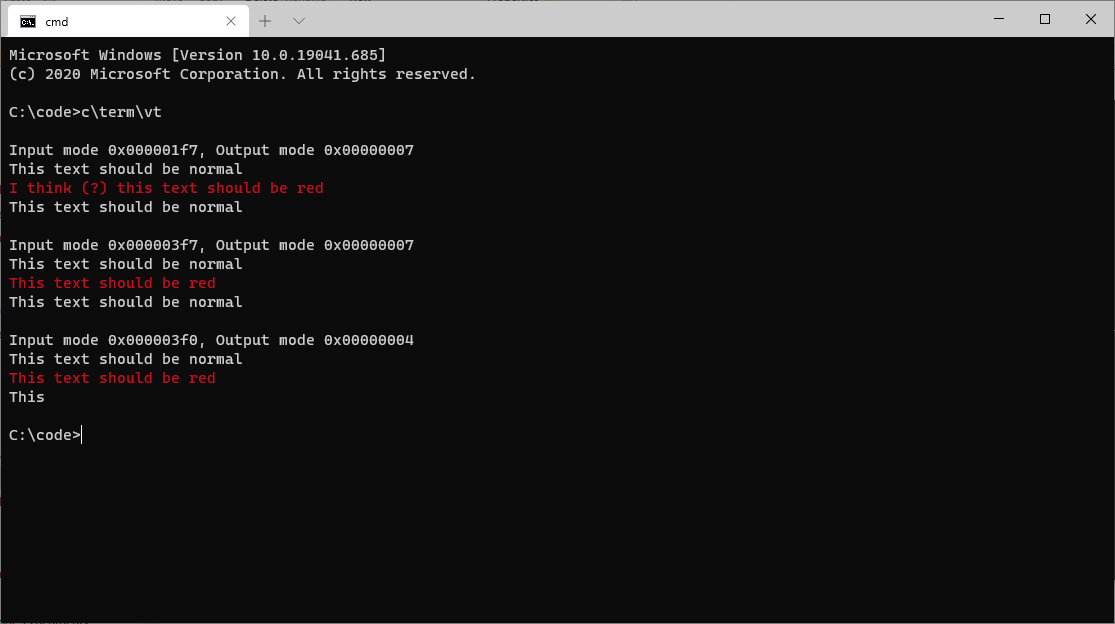
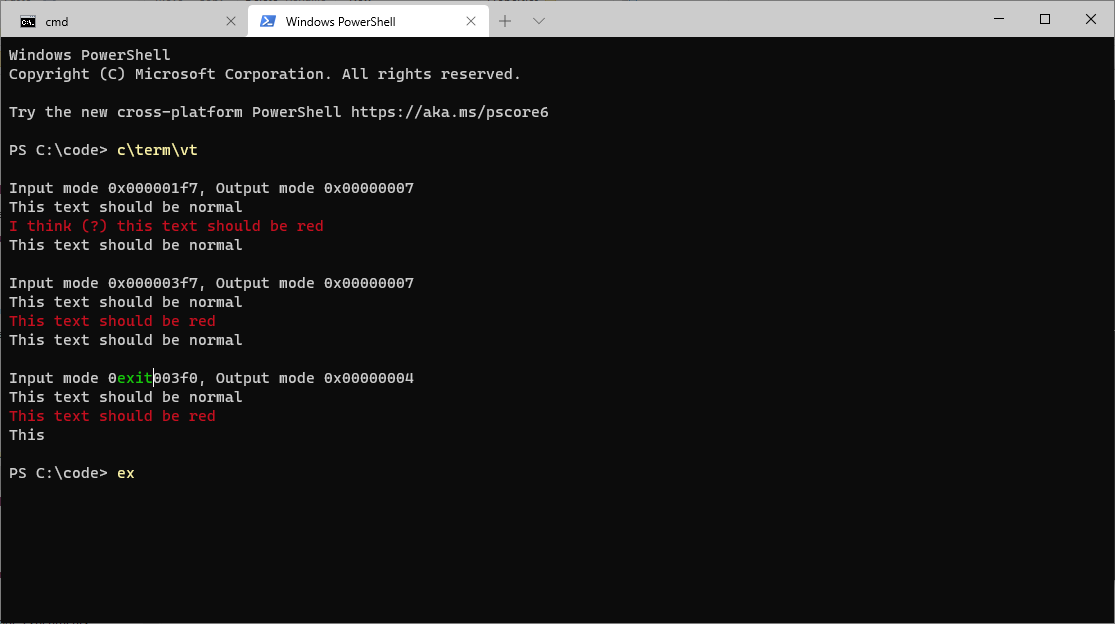
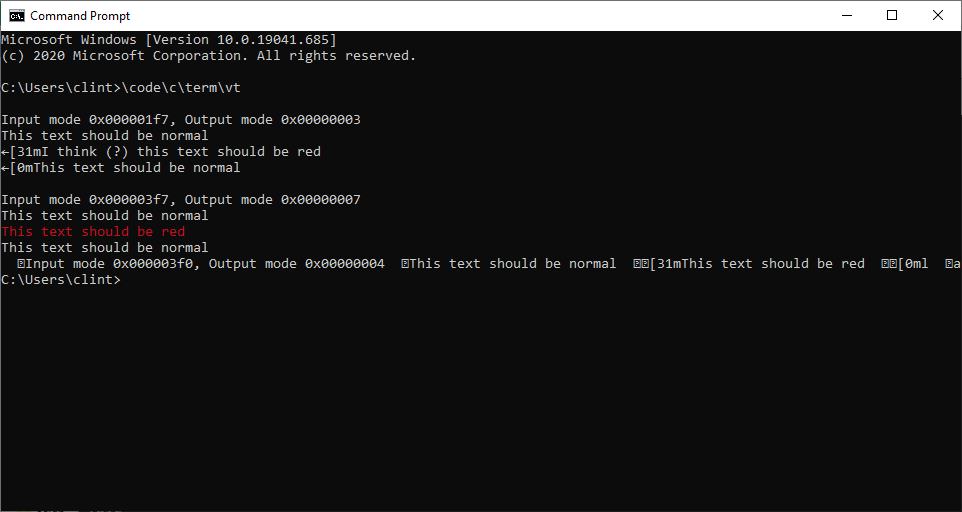
In the Powershell tab when 'exit' is typed, the first 2 characters 'ex' are echoed where they should be, then the cursor jumps up 5 lines and the complete word 'exit' is written there.
Extra notes and questions
Happens whether using printf() or WriteConsoleA()
Am I using the modes properly? I'm just learning about console programming...
Also, is VT output supposed to be disabled when conhost is first started? That surprised me.
Thanks!
The text was updated successfully, but these errors were encountered: