Replies: 2 comments 3 replies
-
It works in JS, but TS can't infer types. So, we don't recommend it for TS users. |
Beta Was this translation helpful? Give feedback.
3 replies
-
import { atom, useAtom } from "jotai";
const numberAtom = atom(10, (get, set, arg: string) => {
const number = parseInt(arg, 10);
// won't accept number
set(numberAtom, number);
// accepts string
set(numberAtom, "");
});
const setNumberAtom = atom(null, (get, set) => {
// do you want it to accept number?
set(numberAtom, 11);
// string is fine
set(numberAtom, "11");
});
const number2Atom = atom(10, (get, set, arg: string | number) => {
const number = typeof arg === "string" ? parseInt(arg, 10) : arg;
// it can accept number
set(number2Atom, number);
// accepts string
set(number2Atom, "");
});
const setNumber2Atom = atom(null, (get, set) => {
// but then this accepts too
set(number2Atom, 11);
// string is fine
set(number2Atom, "11");
}); I assume you want the first to pass and the second to fail. My suggestion is a type assertion: https://tsplay.dev/mpnBaw import { atom, WritableAtom } from "jotai";
const number3Atom = atom(10, (get, set, arg: string) => {
const number = parseInt(arg, 10);
// accepts number with assertion
set(number3Atom as WritableAtom<number, string | number>, number);
// accepts string
set(number3Atom, "");
});
const setNumber3Atom = atom(null, (get, set) => {
// it does not accept number
set(number3Atom, 11);
// string is fine
set(number3Atom, "11");
}); |
Beta Was this translation helpful? Give feedback.
0 replies
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
-
Is this a bug?
I created a number atom, then created an updater function that accepts a string that would convert the string to a number, then assign it to the atom. Hover, the set function won't accept a number, but will let me assign a string to the atom with type number.
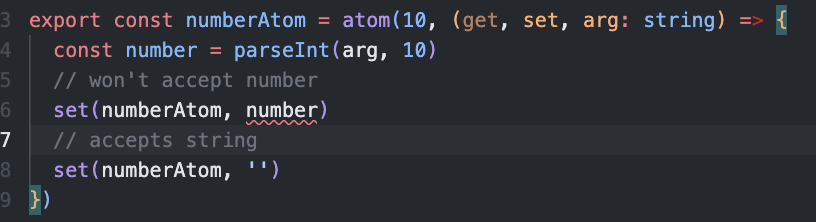
And when I use the atom in a component, I get no indicator that the type is like

number | string
, it still thinks it's just a number, even though it's letting me write a string to it.Here's the full code:
Beta Was this translation helpful? Give feedback.
All reactions