You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Auto merge of rust-lang#11557 - bruno-ortiz:rust-dependencies, r=bruno-ortiz
Creating rust dependencies tree explorer
Hello!
I tried to implement a tree view that shows the dependencies of a project.
It allows to see all dependencies to the project and it uses `cargo tree` for it. Also it allows to click and open the files, the viewtree tries its best to follow the openned file in the editor.
Here is an example:
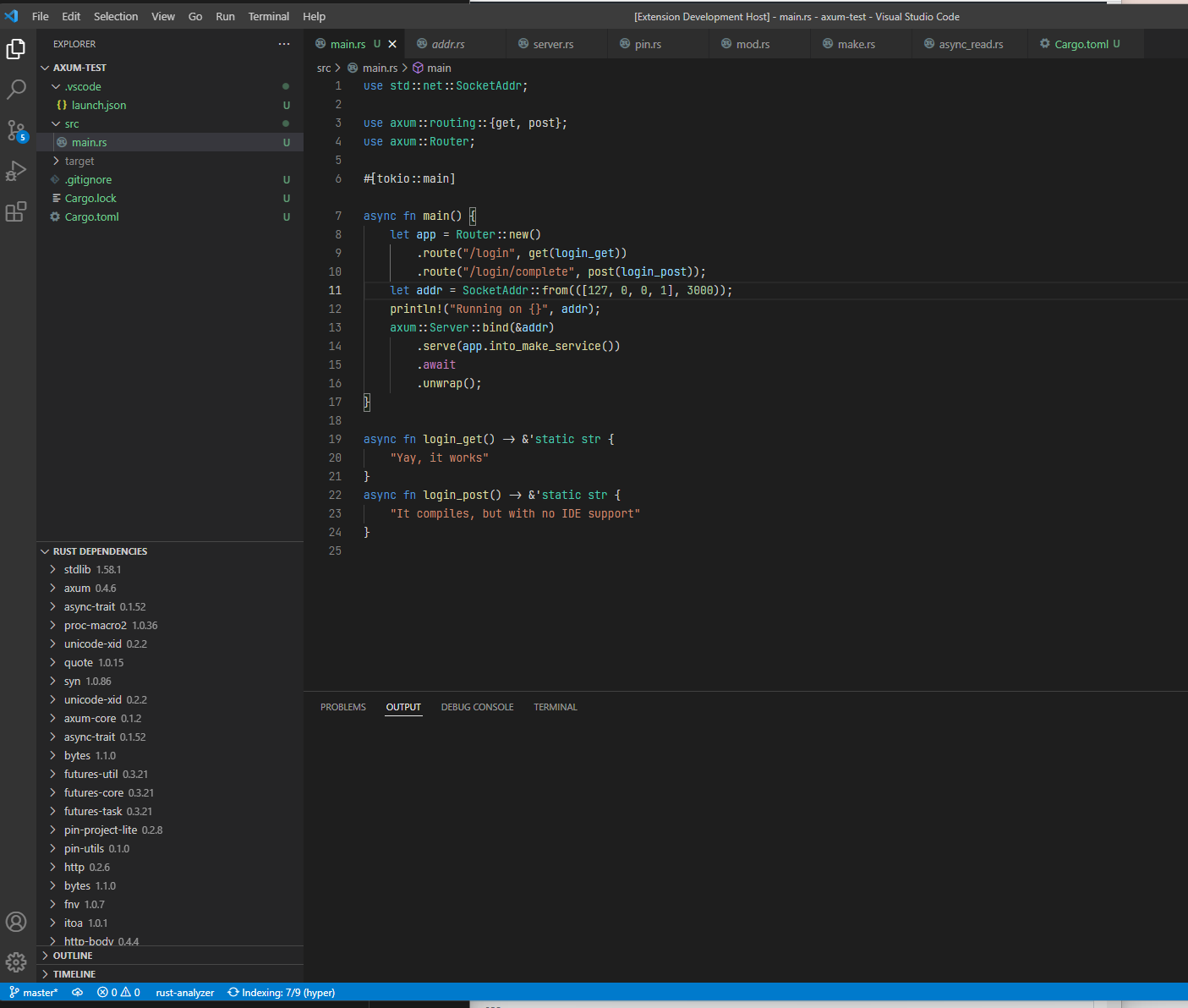
Any feedback is welcome since i have basically no professional experience with TS.
0 commit comments