You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
6964: Add full pattern completions for Struct and Variant patterns r=matklad a=Veykril
Just gonna call it full pattern completion as pattern completion is already implemented in a sense by showing idents in pattern position. What this does is basically complete struct and variant patterns where applicable(function params, let statements and refutable pattern locations).
This does not replace just completing the corresponding idents of the structs and variants, instead two completions are shown for these, a completion for the ident itself and a completion for the pattern(if the pattern make sense to be used that is). I figured in some cases one would rather type out the pattern manually if it has a lot of fields but you only care about one since this completion would cause one more work in the end since you would have to delete all the extra matched fields again.
These completions are tagged as `CompletionKind::Snippet`, not sure if that is the right one here.
<details>
<summary>some gifs</summary>
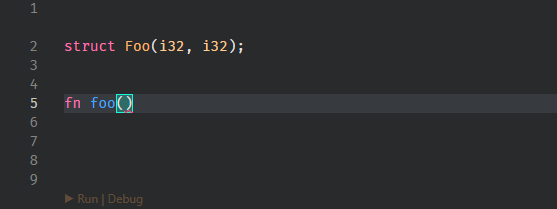
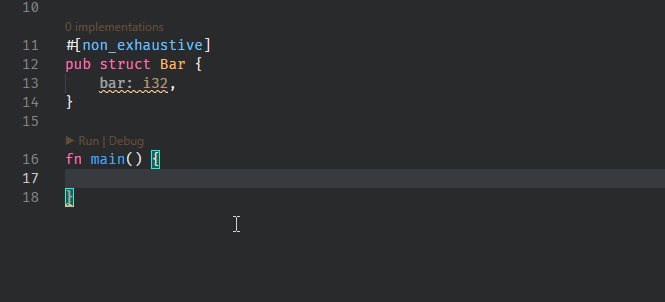
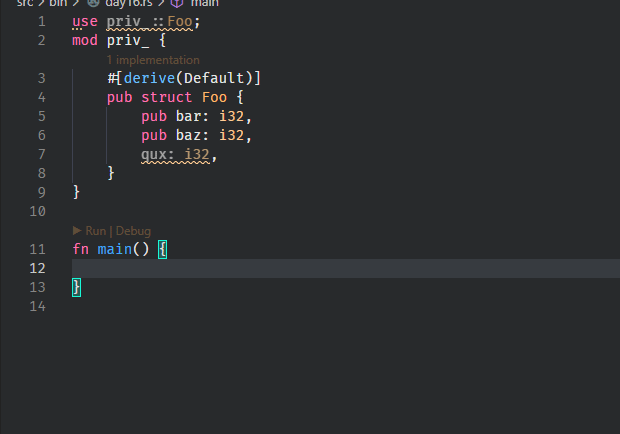
</details>
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
0 commit comments