-
-
Notifications
You must be signed in to change notification settings - Fork 1.1k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Animating Svg component does not work on android #1857
Comments
Did you check if it works on the newest version of the library ( |
I used version |
useAnimatedProps doesn't work (at all?) on Android with 13.x :/ I mentioned it here too: #1845 (comment) |
Some issues with useAnimatedProps seem to have been resolved with 13.2.0, but transform still doesn't work. |
@espenjanson what do you mean by |
Hi @WoLewicki, thanks for trying to fix this! Here's an example of code that worked in 12.4.0 but now doesn't. When I say work, I mean that it doesn't crash - but it does nothing. No transformation happens:
Same thing goes for
I dug around a bit in this repo and found this in src/lib/extract/types.ts:
|
@espenjanson can you provide a simple repo with this code with its all context? It will greatly reduce the time needed to work on it. |
Same problem on iOS with react-content-loader library. https://github.com/danilowoz/react-content-loader/blob/master/src/native/Svg.tsx |
@WoLewicki here is an example of animated component that doesn't work and it causes crash on Android with RN 0.69.5 and latest libs (works fine on iOS):
|
@WoLewicki my issue seems to have been resolved with 13.4.0! However, I'm still getting a type error if I'm not ts-ignoring:
|
@espenjanson can you check if applying #1895 fixes the types issue? |
@maxoschepkov I pasted your example with #1895 merged and it did not crash my app. @iandev096 does it work on earlier versions of the lib? Seems like the native side expects an array with even number of elements on Android for
const animatedProps = useAnimatedProps(() => ({
strokeDashoffset: CIRCLE_LENGTH * (1 - progress.value),
strokeDasharray: [CIRCLE_LENGTH, CIRCLE_LENGTH]
})); Does it fix your issue? |
@iM-GeeKy are you sure |
The error from video should be resolved by adding this line: 8cf4068#diff-73bfcd6b9672fc9694913b10a232038470557fd02a8d688932f64cdc4dc26fbfR111. Can you check if it is present in your project files? It should be there in 13.5.0 |
Upgrading to 13.5.0 did resolve the issue. I appreciate the quick response! 🙌🏻 |
I will close this issue then. Feel free to comment if something is wrong and we can always reopen it then. |
@iM-GeeKy what about incompatible warning from |
@iM-GeeKy thanks 👍 |
Description
I am working on a progress loader component. The animation works on ios, but it doesn't work on android. I have tried it on a couple of different emulators as well as as actual devices, the the animation just does not render
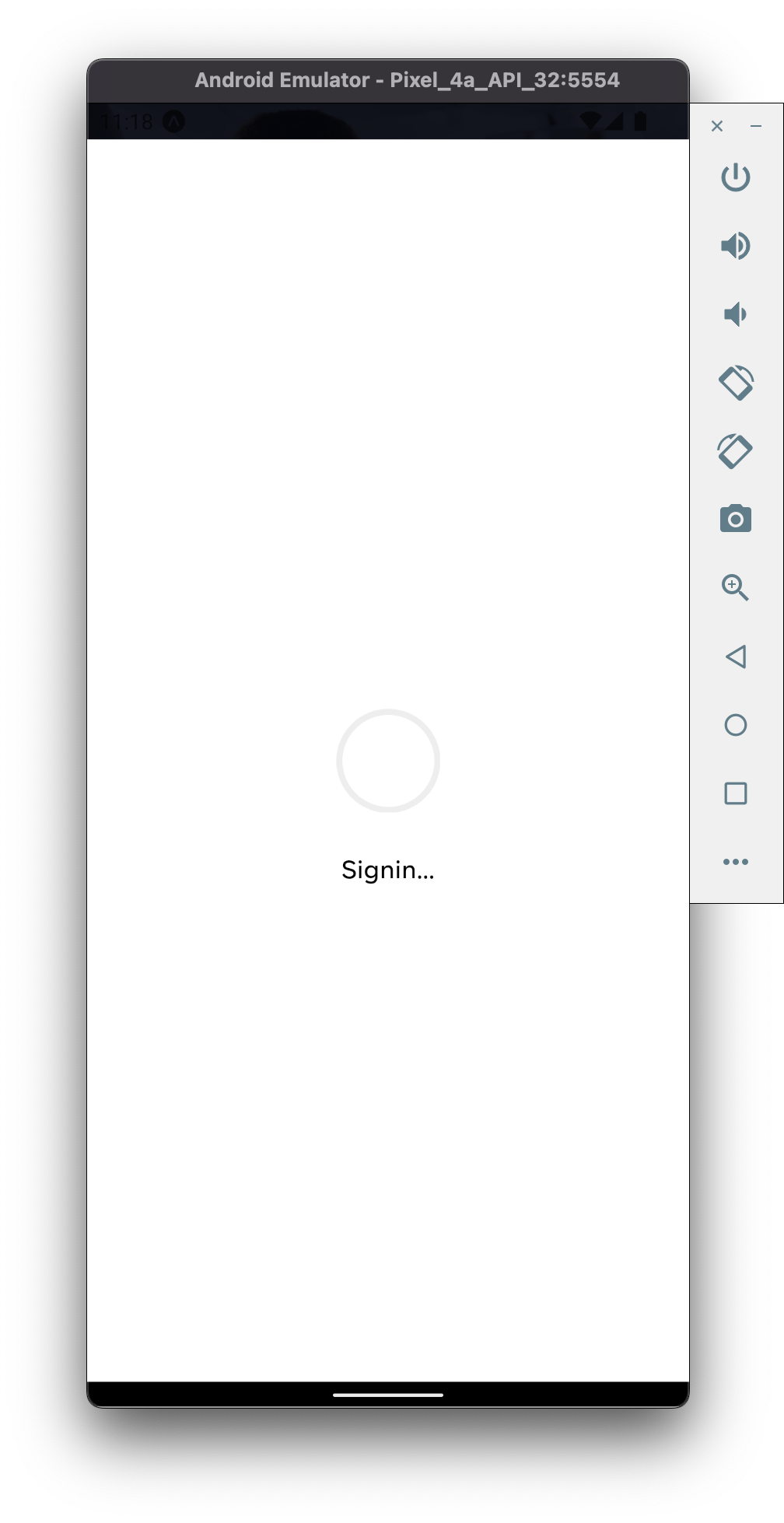
Steps to reproduce
Below is the code for the whole component
Snack or a link to a repository
https://snack.expo.dev/@ianyimiah/reanimated-circular-progress-loader
Reanimated version
2.9.1
React Native version
0.69.5
The text was updated successfully, but these errors were encountered: