- Row end with a superfluous comma when export csv(078d91d)
- Apply a dataFormat column will breaks when hiding(6878e90)
- Setting default sort and then sorting first time always sort descending(ab43542)
- Fix handleSort Function is not changing caret icon(b8ebbb3)
- Fix search fails on columns in data set but not included in table(0ce3eb4)
- Support showing selected row only(a18a463)
- add
showOnlySelected
on selectRow
props
var selectRowProp = {
mode: "checkbox",
showOnlySelected: true
};
- Improve long table performance(564379a)
- Fix the background color of header can't spread to 100%(fa2c827)
- Allow to filter or search data which after formatting(9be42ad)
- Apply
filterFormatted={true}
on <TableHeaderColumn>
to enable filtering formatted data.
- Add class(table-footer-pagination) on pagination for better customization(1ab1662)
- Add class(table-header-wrapper) on table header for better customization(fa2c827)
- Improve search bar too small on small screens (eg. iphone4/5)(674bf95, 351d925)
- Fix condensed table with a overlapping padding on first row(159b8f3)
- Support max height(91bcf2a)
- Assign
maxHeight
to set a max height of table.
- Allow to customize confirmation for row deletion(eb21ec8)
function customConfirm(next){
if (confirm("(It's a custom confirm function)Are you sure you want to delete?")){
//If the confirmation is true, call the function that
//continues the deletion of the record.
next();
}
}
var options = {
handleConfirmDeleteRow: customConfirm
}
<BootstrapTable
data={products}
deleteRow={true}
selectRow={selectRowProp}
options={options}>...
- Tuning the styling of pagination and toolbar panel(3100ee6)
- Upgrade
react-toastr
to 2.3.0
(d9e1c14)
- Upgrade
react
to 0.14.3
(9af1c24)
- Fix Uncaught TypeError: Cannot assign to read only property when edit column after search(689b60f)
- Make the paginatation button disabled appropriately when page change to the end or begin(502cffb)
- Fix a case where current page and pagination size is lost(f01f6ec)(1d57c6d)
- Pagination style tuning(9ccf5ab)
- Support return value from onSelect and onSelectAll handlers(bf27116)
- If return value of this function(onSelect or onSelectAll) is false, the select or deselect action will not be applied.
- Support indeterminate status to select all checkbox(3d9be07)
- Support to change display text when data was empty(d4e16e7)
var options = {
noDataText: 'Your_custom_text'
};
<BootstrapTable
data={products}
options={options}
>...
- Fix column broken when resize to bigger window from smaller(d4b3f87)
- Add selection event of size per page dropdown in pagination(7fbd868)
- Fix import by RequireJS unavailable(3272c45)
- Available to add a custom class on a selection of row(ff06fcd)
var selectRowProp = {
mode: "checkbox",
className: "my-custom-select-class"
};
<BootstrapTable
data={products}
selectRow={selectRowProp}
>...
- Available to insert row By API(a47276a)
- Available to drop row by API(88062b7)
- Available to filter by column through API
- Fix TableDataSet is now available via Window object(b6c065a)
- Fix warning message "Cannot read property 'refs' of undefined_adjustHeaderWidth" when resizing window(fe1910a)
- Fix the NaN value in style(8a6b9b0)
- Fix sizing bugs in 1.2.11 tested in firefox(4d0f7cd)
- Fix csv export bug use on server side rendering(3d46d88)
- Fix #152(ee5e3f5)
- Don't draw a dropdown if one or zero options for pagination size list(881b7cd)
- Fix select row unavailable cause of change in v1.2.11(0dd2dc1)
- Use comma delimited in csv instead of tab delimited(1a219c6)
- Fix missing multiColumnSearch if data reload(cf6a933)
- Fix condensed style bug(4957f55)
- Fix column content exceed user column width definition(88b1368)
- Fix loading toastr css timeout problem(9f592d0)
- Fix Only a ReactOwner can have refs(9e7de02)
- Fix Overflow on column width bug(9ff999e)
- Fix column hidden bug when export csv(7009c39)
- Fix row click also trigger row selection(1cb7dbd)
- Change to page one if data reload(69233b8)
- It's about the issue#125, but not yet fix certainly.
- Support Export CSV
- Set
exportCSV
to true on <BootstrapTable>
, csvFileName
is alternative property for csv file name.
- Support
onRowClick
for after clicking a row(b442d95)
- Add
onRowClick
in options properties on <BootstrapTable>
var options = {
onRowClick: function(row){
}
}
<BootstrapTable
data={products}
options={options}
>...
- Support
afterSearch
and afterColumnFilter
for after searching or column filtering(eccb61d)
- Add
afterSearch
or afterColumnFilter
in options properties on <BootstrapTable>
var options = {
afterSearch: function(searchText, result){
},
afterColumnFilter: function(filterConds, result){
}
}
<BootstrapTable
data={products}
search={true}
columnFilter={true}
options={options}
>...
- fix checkbox default toString() bug(f8ad7a2)
- Support multi search(4874169)
- Add
multiColumnSearch={true}
on <BootstrapTable>
- In search input text, you can use space to split search text, for example: "usa france japan" to search table which contain usa or feance or japan.
- Upgrade to react-toastr@2.2.2(2459c24)
- Fix header and body unalign(3f44200)
- The gap between table and pagination(c1a886b)
- Ensure default checkbox in editor is String(0ef45d0)
- Support
keyField
(2fab4d8)
- Set
keyField
in <BootstrapTable>
to specify which column is key.
- Actually, this attribute is as same as the
isKey
in <TableHeaderColumn>
. So you can choose on to assign which column is key.
function onPageChange(page, sizePerPage){
...
}
var options = {
onPageChange: onPageChange
}
<BootstrapTable
data={products}
pagination={true}
options={options}
>...
}
function onSortChange(sortName, sortOrder){
...
}
var options = {
onSortChange: onSortChange
}
<BootstrapTable
data={products}
options={options}
>...
}
- Split toastr css with react-bootstrap-table css(06defe2)
react-bootstrap-table-all.min.css
include toastr css
react-bootstrap-table.min.css
doesn't include toastr css
- Updat dependencies for node@4.2.1(a3a7b0c)
- Remove deprecated .getDOMNode() calls(37b5c7e)
- Update examples UI and add react-router(4166580)
- use
npm start
or gulp example-server
to watch examples on localhost:3004
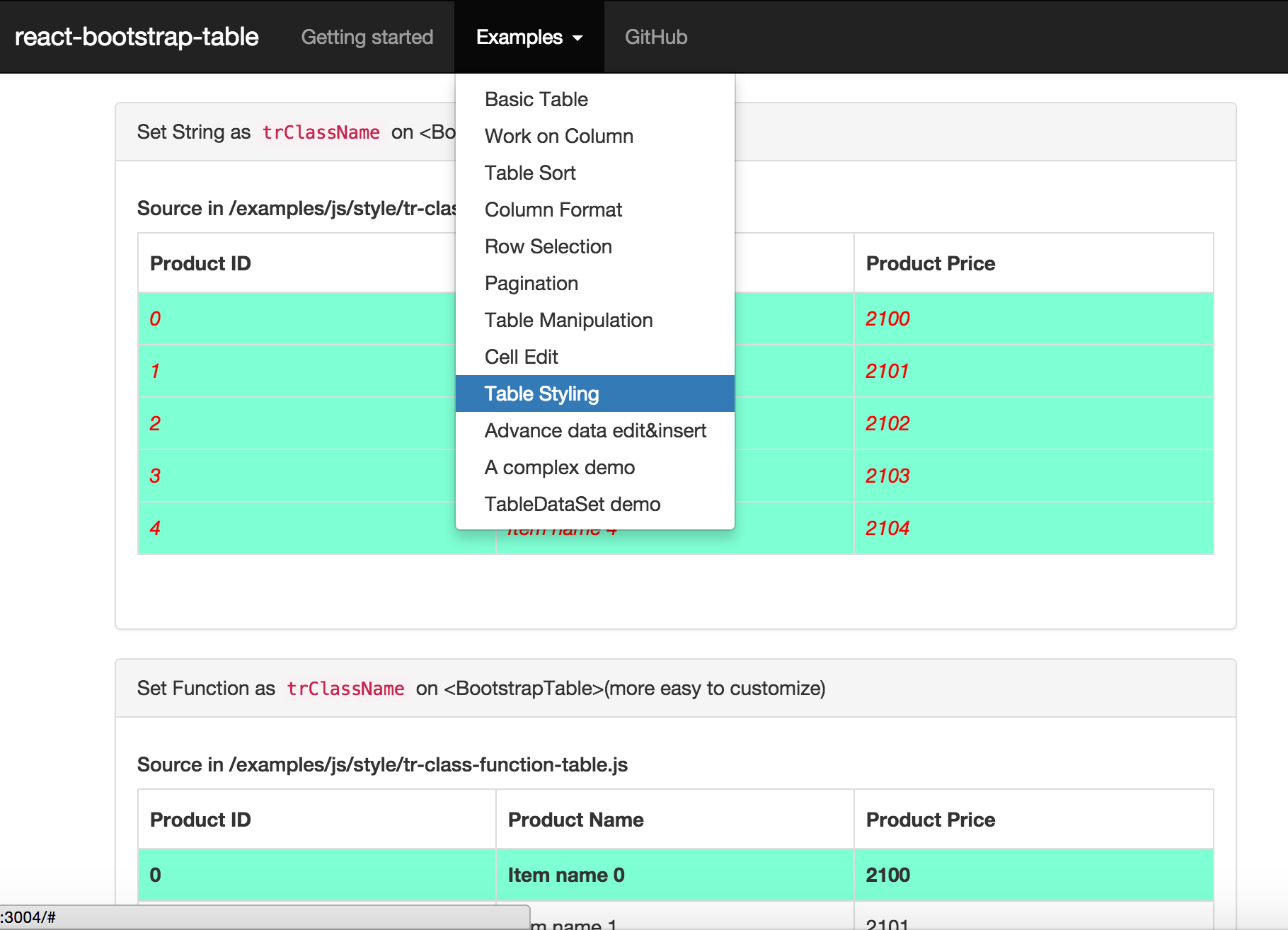
- Update TableDataSet missing pagination(46c93ce)
- Get selected Data only show in table when onSelectAll be called(9d391ee)
function onSelectAll(isSelected, currentDisplayAndSelectedData){
//..
}
- Remove toastr's css hard dependency(28e0b11)
- Fix window is undefinde if use react-bootstrap-table in isomorphic(f5db238)
- Adding Table with borderless feature(43e484f)
- Wrong Dependencies with react-toastr and toastr(bd16999)
- Fix key warning in PaginationList warning(9057e1c)
- Fix default sorting bug(3eb6dbe)
- Separate classname of header and body column
- Set className in <TableHeaderColumn> to define class on header
- Set columnClassName on <TableHeaderColumn> to define class on body's column
- Add cell edit validation and input type(select,checkbox,textarea)
- Add a complete examples Demo
- Run
gulp example-server
and go to localhost:3004/example-list.html
- Give more customize features on Pagination
- Default pagination setting