|
14 | 14 |
|
15 | 15 | package apijson.demo;
|
16 | 16 |
|
| 17 | +import apijson.JSON; |
| 18 | +import apijson.RequestMethod; |
17 | 19 | import com.alibaba.druid.pool.DruidDataSource;
|
| 20 | +import com.alibaba.fastjson.JSONObject; |
| 21 | +import com.alibaba.fastjson.support.spring.FastJsonRedisSerializer; |
18 | 22 | import com.vesoft.nebula.jdbc.impl.NebulaDriver;
|
19 | 23 | import com.zaxxer.hikari.HikariDataSource;
|
20 | 24 |
|
| 25 | +import java.io.Serializable; |
21 | 26 | import java.sql.Connection;
|
22 |
| -import java.sql.DriverManager; |
| 27 | +import java.util.List; |
23 | 28 | import java.util.Map;
|
24 | 29 | import java.util.Properties;
|
| 30 | +import java.util.concurrent.TimeUnit; |
25 | 31 |
|
26 | 32 | import javax.sql.DataSource;
|
27 | 33 |
|
28 | 34 | import apijson.Log;
|
29 | 35 | import apijson.boot.DemoApplication;
|
30 | 36 | import apijson.framework.APIJSONSQLExecutor;
|
31 | 37 | import apijson.orm.SQLConfig;
|
| 38 | +import org.springframework.data.redis.connection.RedisStandaloneConfiguration; |
| 39 | +import org.springframework.data.redis.connection.jedis.JedisConnectionFactory; |
| 40 | +import org.springframework.data.redis.core.RedisTemplate; |
| 41 | +import org.springframework.data.redis.serializer.GenericToStringSerializer; |
| 42 | +import org.springframework.data.redis.serializer.StringRedisSerializer; |
| 43 | + |
| 44 | +import static apijson.framework.APIJSONConstant.PRIVACY_; |
| 45 | +import static apijson.framework.APIJSONConstant.USER_; |
32 | 46 |
|
33 | 47 |
|
34 | 48 | /**SQL 执行器,支持连接池及多数据源
|
|
38 | 52 | public class DemoSQLExecutor extends APIJSONSQLExecutor {
|
39 | 53 | public static final String TAG = "DemoSQLExecutor";
|
40 | 54 |
|
| 55 | + // Redis 缓存 <<<<<<<<<<<<<<<<<<<<<<< |
| 56 | + public static final RedisTemplate<String, String> REDIS_TEMPLATE; |
| 57 | + static { |
| 58 | + REDIS_TEMPLATE = new RedisTemplate<>(); |
| 59 | + REDIS_TEMPLATE.setConnectionFactory(new JedisConnectionFactory(new RedisStandaloneConfiguration("127.0.0.1", 6379))); |
| 60 | + REDIS_TEMPLATE.setKeySerializer(new StringRedisSerializer()); |
| 61 | + REDIS_TEMPLATE.setHashValueSerializer(new GenericToStringSerializer<>(Serializable.class)); |
| 62 | + REDIS_TEMPLATE.setValueSerializer(new GenericToStringSerializer<>(Serializable.class)); |
| 63 | +// REDIS_TEMPLATE.setValueSerializer(new FastJsonRedisSerializer<List<JSONObject>>(List.class)); |
| 64 | + REDIS_TEMPLATE.afterPropertiesSet(); |
| 65 | + } |
| 66 | + |
41 | 67 | // 可重写以下方法,支持 Redis 等单机全局缓存或分布式缓存
|
42 |
| - // @Override |
43 |
| - // public List<JSONObject> getCache(String sql, int type) { |
44 |
| - // return super.getCache(sql, type); |
45 |
| - // } |
46 |
| - // @Override |
47 |
| - // public synchronized void putCache(String sql, List<JSONObject> list, int type) { |
48 |
| - // super.putCache(sql, list, type); |
49 |
| - // } |
50 |
| - // @Override |
51 |
| - // public synchronized void removeCache(String sql, int type) { |
52 |
| - // super.removeCache(sql, type); |
53 |
| - // } |
| 68 | + @Override |
| 69 | + public List<JSONObject> getCache(String sql, SQLConfig config) { |
| 70 | + List<JSONObject> list = super.getCache(sql, config); |
| 71 | + if (list == null) { |
| 72 | + list = JSON.parseArray(REDIS_TEMPLATE.opsForValue().get(sql), JSONObject.class); |
| 73 | + } |
| 74 | + return list; |
| 75 | + } |
| 76 | + @Override |
| 77 | + public synchronized void putCache(String sql, List<JSONObject> list, SQLConfig config) { |
| 78 | + super.putCache(sql, list, config); |
| 79 | + if (config != null && config.isMain()) { |
| 80 | + if (config.isExplain() || RequestMethod.isHeadMethod(config.getMethod(), true)) { |
| 81 | + REDIS_TEMPLATE.opsForValue().set(sql, JSON.toJSONString(list), 10*60, TimeUnit.SECONDS); |
| 82 | + } else { |
| 83 | + String table = config.getTable(); |
| 84 | + REDIS_TEMPLATE.opsForValue().set(sql, JSON.toJSONString(list), USER_.equals(table) || PRIVACY_.equals(table) ? 10*60 : 60, TimeUnit.SECONDS); |
| 85 | + } |
| 86 | + } |
| 87 | + } |
| 88 | + @Override |
| 89 | + public synchronized void removeCache(String sql, SQLConfig config) { |
| 90 | + super.removeCache(sql, config); |
| 91 | + if (config.getMethod() == RequestMethod.DELETE) { // 避免缓存击穿 |
| 92 | + REDIS_TEMPLATE.expire(sql, 60, TimeUnit.SECONDS); |
| 93 | + } else { |
| 94 | + REDIS_TEMPLATE.delete(sql); |
| 95 | + } |
| 96 | + } |
| 97 | + |
| 98 | + @Override |
| 99 | + public JSONObject execute(SQLConfig config, boolean unknownType) throws Exception { |
| 100 | + JSONObject result = super.execute(config, unknownType); |
| 101 | + RequestMethod method = config.getMethod(); |
| 102 | + if (method == RequestMethod.POST) { // 没必要,直接查就行了 |
| 103 | +// Object id = result.get(config.getIdKey()); |
| 104 | +// Object idIn = result.get(config.getIdKey() + "[]"); |
| 105 | +// SQLConfig cacheConfig = APIJSONRouterApplication.DEFAULT_APIJSON_CREATOR.createSQLConfig(); |
| 106 | +// cacheConfig.setMethod(RequestMethod.GET); |
| 107 | +// |
| 108 | + } |
| 109 | + else if (method == RequestMethod.PUT || method == RequestMethod.DELETE) { // RequestMethod.isQueryMethod(method) == false) { |
| 110 | + config.setMethod(RequestMethod.GET); |
| 111 | + boolean isPrepared = config.isPrepared(); |
| 112 | + removeCache(config.getSQL(false), config); |
| 113 | + config.setPrepared(isPrepared); |
| 114 | + config.setMethod(method); |
| 115 | + } |
| 116 | + return result; |
| 117 | + } |
| 118 | + |
| 119 | + // Redis 缓存 >>>>>>>>>>>>>>>>>>>>>>>>>>>>>> |
54 | 120 |
|
55 | 121 | // 适配连接池,如果这里能拿到连接池的有效 Connection,则 SQLConfig 不需要配置 dbVersion, dbUri, dbAccount, dbPassword
|
56 | 122 | @Override
|
|
2 commit comments
TommyLemon commentedon Jan 5, 2023
这段可以封装到 APIJSONORM 中的父类方法
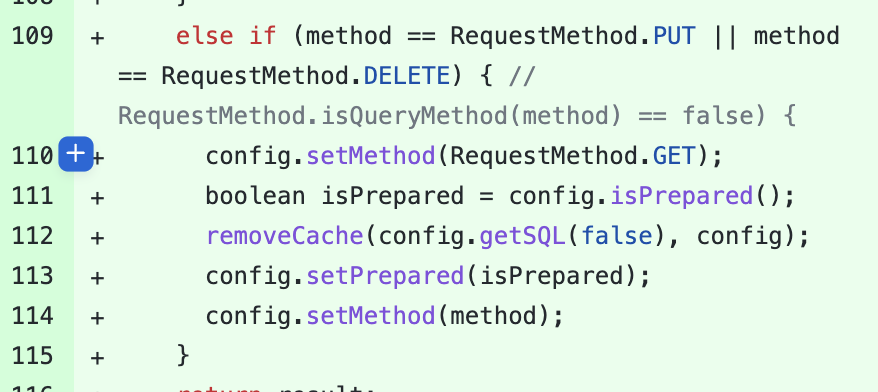
060a10e#diff-8dfe5875f3d4605d5cbe632993b210e70ebe5111367746fe1bc6449338841087R109-R115
TommyLemon commentedon Jan 6, 2023
已完成