-
Notifications
You must be signed in to change notification settings - Fork 102
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Update doc link to docs site (#1882)
* Update docs * update readme * update readme
- Loading branch information
Showing
6 changed files
with
16 additions
and
429 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,77 +1,3 @@ | ||
# Customizing Json Serialization in Management SDK | ||
In Management SDK, the method arguments sent to clients are serialized into JSON. We have several ways to customize JSON serialization. We will show all the ways in the order from the most recommended to the least recommended. | ||
|
||
## `ServiceManagerOptions.UseJsonObjectSerializer(ObjectSerializer objectSerializer)` | ||
The most recommended way is to use a general abstract class [`ObjectSerializer`](https://azuresdkdocs.blob.core.windows.net/$web/dotnet/Azure.Core/1.21.0/api/Azure.Core.Serialization/Azure.Core.Serialization.ObjectSerializer.html), because it supports different JSON serialization libraries such as `System.Text.Json` and `Newtonsoft.Json` and it applies to all the transport types. Usually you don't need to implement `ObjectSerializer` yourself, as handy JSON implementations for `System.Text.Json` and `Newtonsoft.Json` are already provided. | ||
|
||
### If you want to use `System.Text.Json` as JSON processing library | ||
The builtin [`JsonObjectSerializer`](https://azuresdkdocs.blob.core.windows.net/$web/dotnet/Azure.Core/1.21.0/api/Azure.Core.Serialization/Azure.Core.Serialization.JsonObjectSerializer.html) uses `System.Text.Json.JsonSerializer` to for serialization/deserialization. Here is a sample to use camel case naming for JSON serialization: | ||
|
||
```cs | ||
var serviceManager = new ServiceManagerBuilder() | ||
.WithOptions(o => | ||
{ | ||
o.ConnectionString = "***"; | ||
o.UseJsonObjectSerializer(new JsonObjectSerializer(new JsonSerializerOptions | ||
{ | ||
PropertyNamingPolicy = JsonNamingPolicy.CamelCase | ||
})); | ||
}) | ||
.BuildServiceManager(); | ||
|
||
``` | ||
|
||
### If you want to use `Newtonsoft.Json` as JSON processing library | ||
First install the package `Microsoft.Azure.Core.NewtonsoftJson` from Nuget using .NET CLI: | ||
```dotnetcli | ||
dotnet add package Microsoft.Azure.Core.NewtonsoftJson | ||
``` | ||
Here is a sample to use camel case naming with [`NewtonsoftJsonObjectSerializer`](https://azuresdkdocs.blob.core.windows.net/$web/dotnet/Microsoft.Azure.Core.NewtonsoftJson/1.0.0/index.html): | ||
|
||
```cs | ||
var serviceManager = new ServiceManagerBuilder() | ||
.WithOptions(o => | ||
{ | ||
o.ConnectionString = "***"; | ||
o.UseJsonObjectSerializer(new NewtonsoftJsonObjectSerializer(new JsonSerializerSettings() | ||
{ | ||
ContractResolver = new CamelCasePropertyNamesContractResolver() | ||
})); | ||
}) | ||
.BuildServiceManager(); | ||
``` | ||
|
||
### If the above choices don't meet your requirements | ||
You can also implement `ObjectSerializer` on your own. The following links might help: | ||
|
||
* [API reference of `ObjectSerializer`](https://azuresdkdocs.blob.core.windows.net/$web/dotnet/Azure.Core/1.21.0/api/Azure.Core.Serialization/Azure.Core.Serialization.ObjectSerializer.html) | ||
|
||
* [Source code of `ObjectSerializer`](https://github.com/Azure/azure-sdk-for-net/blob/main/sdk/core/Azure.Core/src/Serialization/ObjectSerializer.cs) | ||
|
||
* [Source code of `JsonObjectSerializer`](https://github.com/Azure/azure-sdk-for-net/blob/main/sdk/core/Azure.Core/src/Serialization/JsonObjectSerializer.cs) | ||
|
||
|
||
## `ServiceManagerBuilder.WithNewtonsoftJson(Action<NewtonsoftServiceHubProtocolOptions> configure)` | ||
This method is only for `Newtonsoft.Json` users. Here is a sample to use camel case naming: | ||
```cs | ||
var serviceManager = new ServiceManagerBuilder() | ||
.WithNewtonsoftJson(o => | ||
{ | ||
o.PayloadSerializerSettings = new JsonSerializerSettings | ||
{ | ||
ContractResolver = new CamelCasePropertyNamesContractResolver() | ||
}; | ||
}) | ||
.BuildServiceManager(); | ||
``` | ||
|
||
## ~~`ServiceManagerOptions.JsonSerializerSettings`~~ (Deprecated) | ||
This method only applies to transient transport type. Don't use this. | ||
```cs | ||
var serviceManager = new ServiceManagerBuilder() | ||
.WithOptions(o => | ||
{ | ||
o.JsonSerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver(); | ||
}) | ||
.BuildServiceManager(); | ||
``` | ||
This article has been moved to [here](https://learn.microsoft.com/azure/azure-signalr/signalr-howto-use-management-sdk#json-serialization). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,47 +1,3 @@ | ||
# Negotiate | ||
|
||
At the beginning, client will initialize a `POST [endpoint-base]/negotiate` request to establish a connection between the client and the server. | ||
|
||
In the POST request the client sends a query string parameter with the key "negotiateVersion" and the value as the negotiate protocol version it would like to use. If the query string is omitted, the server treats the version as zero. The server will include a "negotiateVersion" property in the json response that says which version it will be using. The version is chosen as described below: | ||
* If the servers minimum supported protocol version is greater than the version requested by the client it will send an error response and close the connection | ||
* If the server supports the request version it will respond with the requested version | ||
* If the requested version is greater than the servers largest supported version the server will respond with its largest supported version | ||
The client may close the connection if the "negotiateVersion" in the response is not acceptable. | ||
|
||
The content type of the response is `application/json` and is a JSON payload containing properties to assist the client in establishing a persistent connection. Extra JSON properties that the client does not know about should be ignored. This allows for future additions without breaking older clients. | ||
|
||
__Now Azure SignalR service supports `Version 0` only__. So client with the "negotiateVersion" greater than zero will get a reponse with `negotiateVersion=0` by design. | ||
|
||
### Version 0 | ||
|
||
When the server and client agree on version 0 the server response will include a "connectionId" property that is used in the "id" query string for the HTTP requests described below. | ||
|
||
A successful negotiate response will look similar to the following payload: | ||
```json | ||
{ | ||
"connectionId":"807809a5-31bf-470d-9e23-afaee35d8a0d", | ||
"negotiateVersion":0, | ||
"availableTransports":[ | ||
{ | ||
"transport": "WebSockets", | ||
"transferFormats": [ "Text", "Binary" ] | ||
}, | ||
{ | ||
"transport": "ServerSentEvents", | ||
"transferFormats": [ "Text" ] | ||
}, | ||
{ | ||
"transport": "LongPolling", | ||
"transferFormats": [ "Text", "Binary" ] | ||
} | ||
] | ||
} | ||
``` | ||
|
||
The payload returned from this endpoint provides the following data: | ||
|
||
* The `connectionId` which is **required** by the Long Polling and Server-Sent Events transports (in order to correlate sends and receives). | ||
* The `negotiateVersion` which is the negotiation protocol version being used between the server and client. | ||
* The `availableTransports` list which describes the transports the server supports. For each transport, the name of the transport (`transport`) is listed, as is a list of "transfer formats" supported by the transport (`transferFormats`) | ||
|
||
Find more details about SignalR transport protocol from [HERE](https://github.com/dotnet/aspnetcore/blob/main/src/SignalR/docs/specs/TransportProtocols.md). | ||
This article has been moved to [here](https://learn.microsoft.com/azure/azure-signalr/signalr-concept-client-negotiation). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,72 +1,3 @@ | ||
# Azure SignalR Local Emulator | ||
|
||
When developing serverless applications, we provide an Azure SignalR Local Emulator to make the local development and integration easier. Please note that the emulator works only for serverless scenarios, for *Default* mode that the Azure SignalR Service acts as a proxy, you can directly use self-host SignalR to do local development. Please also note that emulator only works for *Transient* transport type (the default one) and doesn't work for *Persistent* transport type. | ||
|
||
Features available | ||
------------------ | ||
1. Auth | ||
2. Latest Rest API support | ||
3. Upstream | ||
|
||
Walkthrough | ||
---------------- | ||
Take this serverless sample for example https://github.com/Azure/azure-functions-signalrservice-extension/tree/3e87c3ce277265866ca9d0bf51bb9c7ecea39e14/samples/bidirectional-chat | ||
1. Clone the sample repo to local | ||
``` | ||
git clone https://github.com/Azure/azure-functions-signalrservice-extension.git | ||
cd azure-functions-signalrservice-extension/samples/bidirectional-chat | ||
``` | ||
|
||
2. Install the emulator | ||
``` | ||
dotnet tool install -g Microsoft.Azure.SignalR.Emulator | ||
``` | ||
Or update the emulator to the latest preview version if it is already installed: | ||
``` | ||
dotnet tool update -g Microsoft.Azure.SignalR.Emulator | ||
``` | ||
|
||
3. Run the emulator `asrs-emulator` to list all the available commands: | ||
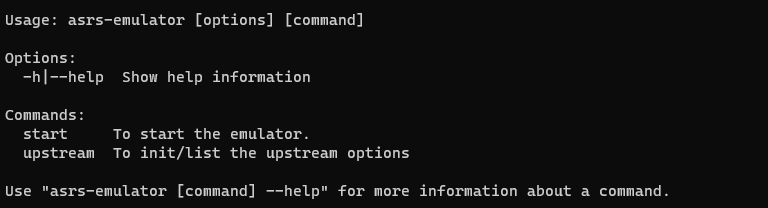 | ||
|
||
4. Init the default upstream settings using: | ||
``` | ||
asrs-emulator upstream init | ||
``` | ||
|
||
It inits a default `settings.json` into the current folder, with a default upstream `UrlTemplate` as `http://localhost:7071/runtime/webhooks/signalr`, which is the URL for SignalR's **local** function trigger: | ||
```json | ||
{ | ||
"UpstreamSettings": { | ||
"Templates": [ | ||
{ | ||
"UrlTemplate": "http://localhost:7071/runtime/webhooks/signalr", | ||
"EventPattern": "*", | ||
"HubPattern": "*", | ||
"CategoryPattern": "*" | ||
} | ||
] | ||
} | ||
} | ||
``` | ||
|
||
You can edit the file to make the pattern more restricted, for example, change `HubPattern` from `*` to `chat`. When the file is modified, its change will be hot loaded into the emulator. | ||
|
||
5. Start the emulator | ||
``` | ||
asrs-emulator start | ||
``` | ||
|
||
After the emulator is successfully started, it generates the ConnectionString to be used later, for example, the ConnectionString is `Endpoint=http://localhost;Port=8888;AccessKey=ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789ABCDEFGH;Version=1.0;` as the below screenshot shows. | ||
|
||
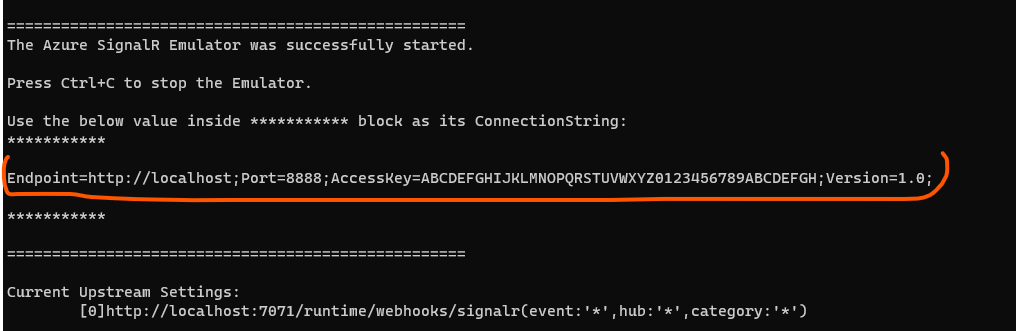 | ||
|
||
The emulator also provides advanced options when start, for example, you can use `asrs-emulator start -p 8999` to customize the port the emulator used. Type `asrs-emulator start --help` to check the options available. | ||
|
||
6. Go into subfolder `csharp` and rename `local.settings.sample.json` to `local.settings.json`, use the ConnectionString generated by the emulator to fill into the value of the **AzureSignalRConnectionString** in your **local.settings.json**. Fill into `AzureWebJobsStorage` your storage connection string, for example, `UseDevelopmentStorage=true` when using storage emulator. Save the file and run the function in the `csharp` subfolder with `func start`. | ||
|
||
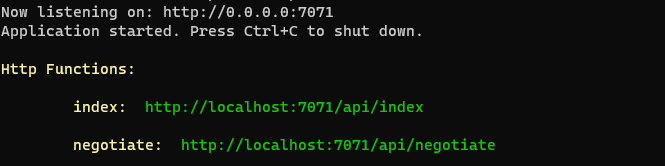 | ||
|
||
7. In the browser, navigate to `http://localhost:7071/api/index` to play with the demo. | ||
|
||
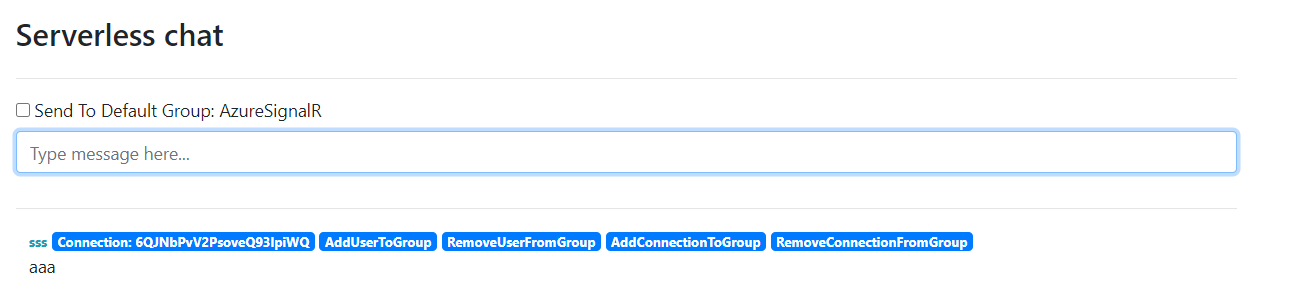 | ||
This article has been moved to [here](https://learn.microsoft.com/azure/azure-signalr/signalr-howto-emulator). |
Oops, something went wrong.