-
Notifications
You must be signed in to change notification settings - Fork 86
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add code to test notebook code examples (#471)
This PR adds a script to automatically execute notebooks in CI. To keep PRs small and focussed, this only adds the scripts to test notebooks, it doesn't actually fix those notebooks or add anything to CI. <details> <summary>Example: Success</summary> 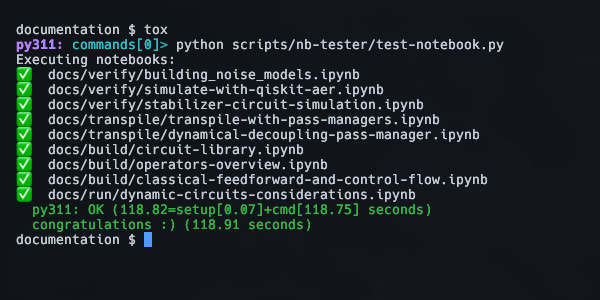 </details> <details> <summary>Example: Failure</summary> 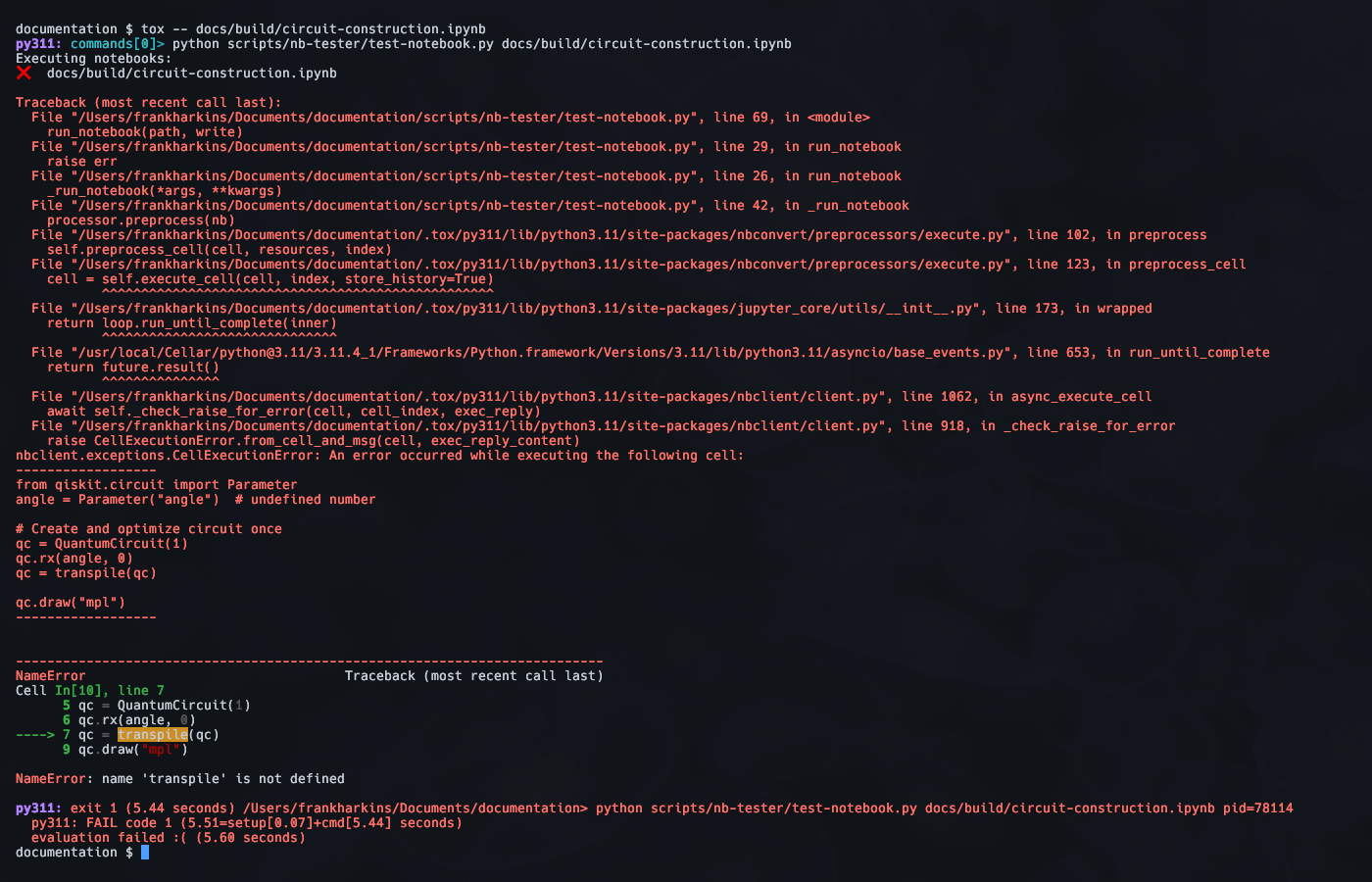 </details> <details> <summary>Why <code>tox</code>?</summary> An important feature is to be able to run a single notebook in the virtual environment. With `tox`, we can use commands like this: ```sh tox -- path/to/notebook ``` Whereas with `nox`, this is the simplest I could get it: ```sh nox --session 'test(path="path/to/notebook")' ``` Note this also doesn't autocomplete the path. The main reason for choosing `nox` seems to be more flexible configuration, but we only need simple configuration in this repo so this isn't a problem. </details> *** Part of #166 --------- Co-authored-by: Eric Arellano <14852634+Eric-Arellano@users.noreply.github.com>
- Loading branch information
1 parent
316dd8b
commit 40ded0a
Showing
5 changed files
with
136 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -2,6 +2,7 @@ | |
.vscode/ | ||
.fleet/ | ||
.venv/ | ||
.tox/ | ||
.envrc | ||
|
||
.ipynb_checkpoints/ | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
# We pin to exact versions for a more reproducible and | ||
# stable build. | ||
nbconvert~=7.11.0 | ||
nbformat~=5.9.2 | ||
ipykernel~=6.27.1 | ||
qiskit[all]~=0.45.1 | ||
qiskit-aer~=0.13.1 | ||
qiskit-ibm-runtime~=0.16.1 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,96 @@ | ||
# This code is a Qiskit project. | ||
# | ||
# (C) Copyright IBM 2023. | ||
# | ||
# This code is licensed under the Apache License, Version 2.0. You may obtain a | ||
# copy of this license in the LICENSE file in the root directory of this source | ||
# tree or at http://www.apache.org/licenses/LICENSE-2.0. | ||
# | ||
# Any modifications or derivative works of this code must retain this copyright | ||
# notice, and modified files need to carry a notice indicating that they have | ||
# been altered from the originals. | ||
|
||
import sys | ||
from pathlib import Path | ||
import nbconvert | ||
import nbformat | ||
|
||
WRITE_FLAG = "--write" | ||
NOTEBOOKS_GLOB = "docs/**/*.ipynb" | ||
NOTEBOOKS_EXCLUDE = [ | ||
"docs/api/**", | ||
"**/.ipynb_checkpoints/**", | ||
# Following notebooks have code errors | ||
"docs/build/circuit-construction.ipynb", | ||
"docs/build/pulse.ipynb", | ||
"docs/transpile/custom-transpiler-pass.ipynb", | ||
"docs/transpile/transpiler-stages.ipynb", | ||
# Following notebook has some non-python dependency I can't figure out | ||
"docs/build/circuit-visualization.ipynb", | ||
# Following notebooks make requests so can't be tested yet | ||
"docs/run/get-backend-information.ipynb", | ||
"docs/start/hello-world.ipynb", | ||
] | ||
|
||
|
||
def execute_notebook(path: Path, write=False) -> bool: | ||
""" | ||
Wrapper function for `_execute_notebook` to print status | ||
""" | ||
print(f"▶️ {path}", end="", flush=True) | ||
try: | ||
_execute_notebook(path, write) | ||
except nbconvert.preprocessors.CellExecutionError as err: | ||
print("\r❌\n") | ||
print(err) | ||
return False | ||
print("\r✅") | ||
return True | ||
|
||
|
||
def _execute_notebook(filepath: Path, write=False) -> None: | ||
""" | ||
Use nbconvert to execute notebook | ||
""" | ||
nb = nbformat.read(filepath, as_version=4) | ||
|
||
processor = nbconvert.preprocessors.ExecutePreprocessor( | ||
timeout=100, | ||
kernel_name="python3", | ||
) | ||
|
||
processor.preprocess(nb) | ||
|
||
if not write: | ||
return | ||
|
||
for cell in nb.cells: | ||
cell.metadata.pop("execution", None) | ||
nbformat.write(nb, filepath) | ||
|
||
|
||
def find_notebooks() -> list[Path]: | ||
""" | ||
Get paths to all notebooks in NOTEBOOKS_GLOB that are not excluded by | ||
NOTEBOOKS_EXCLUDE | ||
""" | ||
all_notebooks = Path(".").rglob(NOTEBOOKS_GLOB) | ||
return [ | ||
path | ||
for path in all_notebooks | ||
if not any(path.match(glob) for glob in NOTEBOOKS_EXCLUDE) | ||
] | ||
|
||
|
||
if __name__ == "__main__": | ||
args = sys.argv[1:] | ||
write = WRITE_FLAG in args | ||
if write: | ||
args.remove(WRITE_FLAG) | ||
|
||
notebook_paths = args or find_notebooks() | ||
print("Executing notebooks:") | ||
results = [execute_notebook(path, write) for path in notebook_paths] | ||
if not all(results): | ||
sys.exit(1) | ||
sys.exit(0) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
[tox] | ||
min_version = 4.0 | ||
env_list = py311 | ||
skipsdist = true | ||
|
||
[testenv] | ||
deps = -r scripts/nb-tester/requirements.txt | ||
setenv = PYDEVD_DISABLE_FILE_VALIDATION=1 | ||
commands = python scripts/nb-tester/test-notebook.py {posargs} |