-
Notifications
You must be signed in to change notification settings - Fork 7
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Refactor structure of OASIS service #352
Comments
handlerHandler accepts oasis.Request and put oasis.Response to certain channel. to do:
selectionstrategy(selector)It accepts `oasis.Request` and generates `oasis.Response`.
It will generate internal `solution` format following such logic:
- detect wether destination is reachable or not
- detect whether charge is needed or not
- detect whether destination is reachable via single charge, find best solution
- best solution for multiple charge
+ SearchAlongRoute
+ ChargeStationBasedStrategy to do:
Package
|
Looks really good! Some questions for discussing:
|
[Perry] Thanks for your feedback, more strength to move on.
[Perry] Also think about to make this change.
[Perry] agree
[Perry] yes, don't over splitting package
[Perry]
[Perry] I saw the name has been used here and here, seems people like to put logic which communicate with external components like message bus, database into a folder called [Perry] Update on 05282020
[Perry] Totally agree. |
Discussion with @wangyoucao577 on 06012020. handler
connectivity
|
Actions:
|
Subtask of #358
To be discussed with @wangyoucao577
// this two paragraphs will be placed in doc :)
OASIS
stands for Optimized Charge Station Selection Service, which mainly supports generating optimal charge station candidates for given query based on user's vehical information. It expects routing engine(like OSRM) to generate electronic spcific cost and only focus on how to choose most optimal charge station combination which balances charging cost(charging time, payment, etc).For example,
OASIS
might suggest user spend 1 hour to charge at station A for x amount of energy then reach destination with 2 hours in total, or charge at station B and station C for 30 minutes each and reach destination with duration of 2 hours 10 minutes.Overview
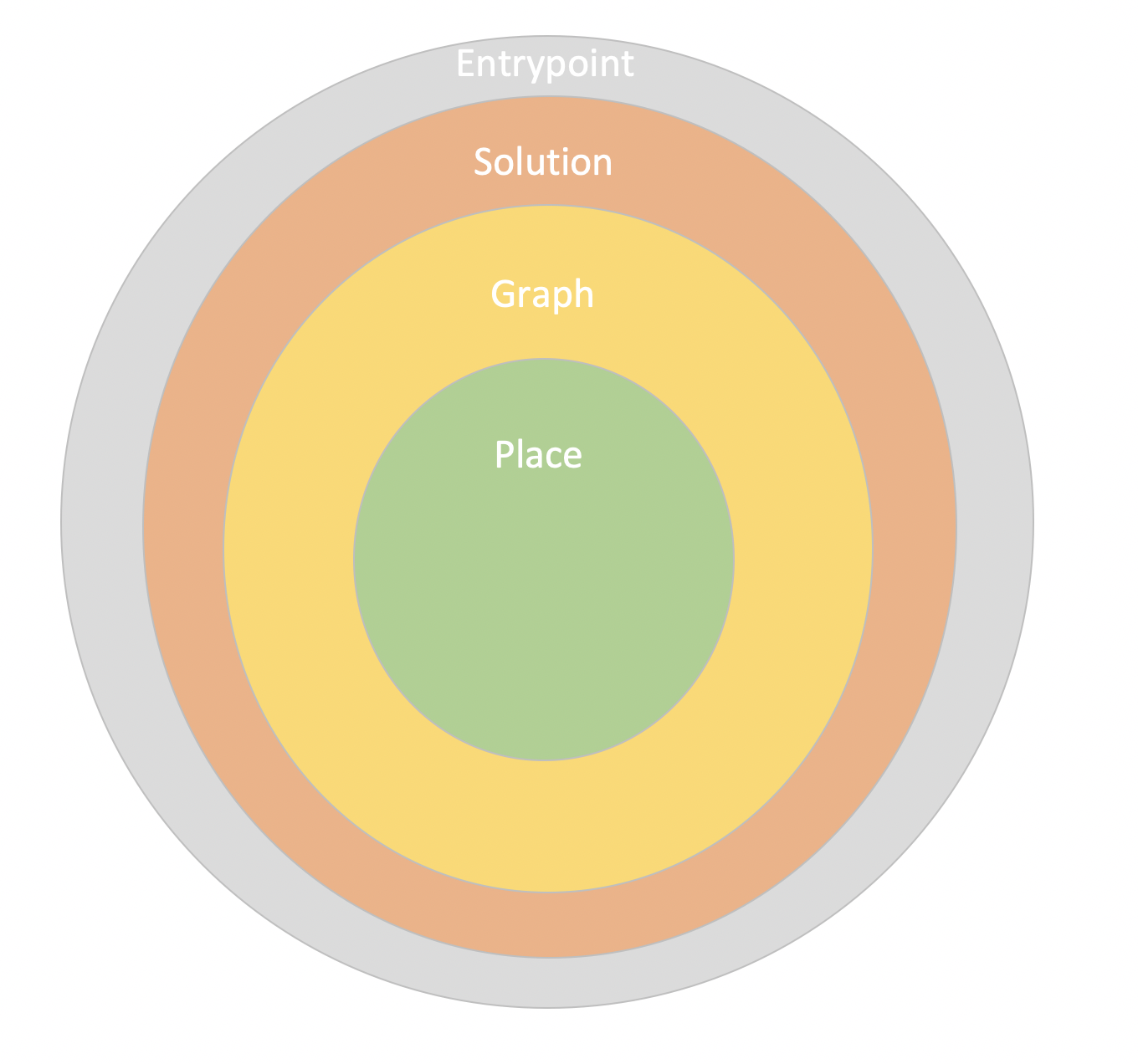
Structure layer
Selector
is the layer contains logic for how to select charge stations, such as* whether need charge or not
* is destination reachable by single charge
* multiple charge station solution finding, which could be
search along route
orcharge station based routing
, implemented by other packagessolution
andGenerateSolution
abstract the interface for how to generate multiple charge station solutionstationgraph
implements theGenerateSolution
interface, and this package representsalgorithm
,graph
in this diagramchargingstrategy
abstract logic ofcharge
which supports calculation instationgraph
chargestations
value object
ofPlace
and operations(usecases) on that objectFile structure
place.go
topological_querier_interface.go
spatial_querier_interface.go
location_querier_interface.go
place_algorithm.go
solution_interface.go
Updated on 07222020: for actions please go to #352 (comment)
The text was updated successfully, but these errors were encountered: