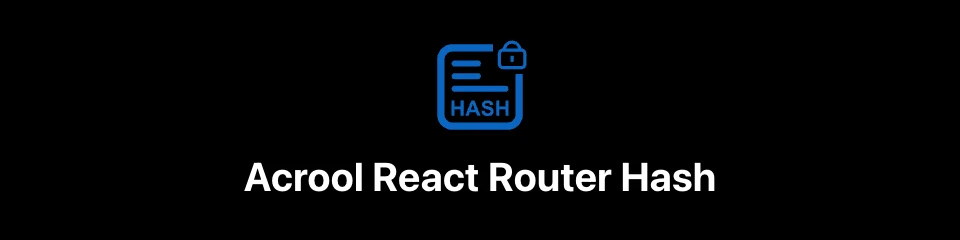
Hash Route + History Route Additional based for React Route v6
with react-router-dom version 6.x
^3.2.0 support react >=18.0.0 <20.0.0
- With react-router-dom version 6.x
- In CSR, it is easy to implement the light box routing function
- Modified and enhanced HashRouter function by react-router-dom, supports path params
- Extract the shared optical box to the router to separate dependencies
- With @acrool/react-modal to support persistent lightbox
yarn add @acrool/react-router-hash
import {Route, Routes, useLocation} from 'react-router-dom';
import {HashRoutes, HashRoute} from '@acrool/react-router-hash';
import {unstable_HistoryRouter as Router} from "react-router-dom";
const history = createBrowserHistory({window});
const MainRouter = () => {
return <Router history={history}>
{/* Base pathname */}
<Routes>
<Route path="/" element={<Dashboard/>} />
<Route path="*" element={<NotFound/>}/>
</Routes>
{/* Hash pathname*/}
<HashRoutes>
<HashRoute path="login" element={<Login/>}/>
<HashRoute path="control/*" element={<EditLayout/>}>
<HashRoute path="editAccount/:id" element={<EditAccount/>}/>
<HashRoute path="editPassword" element={<EditPassword/>}/>
</HashRoute>
</HashRoutes>
</Router>
};
import {useHashParams} from '@acrool/react-router-hash';
const Dashboard = () => {
const navigate = useNavigate();
return <div>
<h2>Dashboard</h2>
<p>This page dashboard.</p>
<button type="button" onClick={() => navigate({hash: '/control/editAccount/1'})}>EditAccount HashModal</button>
<button type="button" onClick={() => navigate({hash: '/control/editPassword'})}>EditPassword HashModal</button>
</div>;
};
const EditAccount = () => {
const {id} = useHashParams<{id: string}>();
const navigate = useNavigate();
const pathname = useHashPathname();
return <>
<p>hash pathname: {pathname}</p>
<p>useHashParams id: {id}</p>
<button type="button" onClick={() => navigate({hash: undefined})}>Close HashModal</button>
</>;
};
There is also a example that you can play with it: