-
Notifications
You must be signed in to change notification settings - Fork 34
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
js各种遍历总结(2017.11.19) #13
Comments
for...of... 方法可以搭配keys / entries / values 来使用呀 |
👍 |
var arr = [1,2,3,4,5]; |
@HSQCoollaughing 已改 感谢反馈~~ |
只谈遍历对象的话, for...in的性能是最好的 |
是的 而且你发的文章很棒 😄 感谢分享 |
一 、普通for循环 (只能遍历数组和字符串)
可以break停止循环, 可以读写。
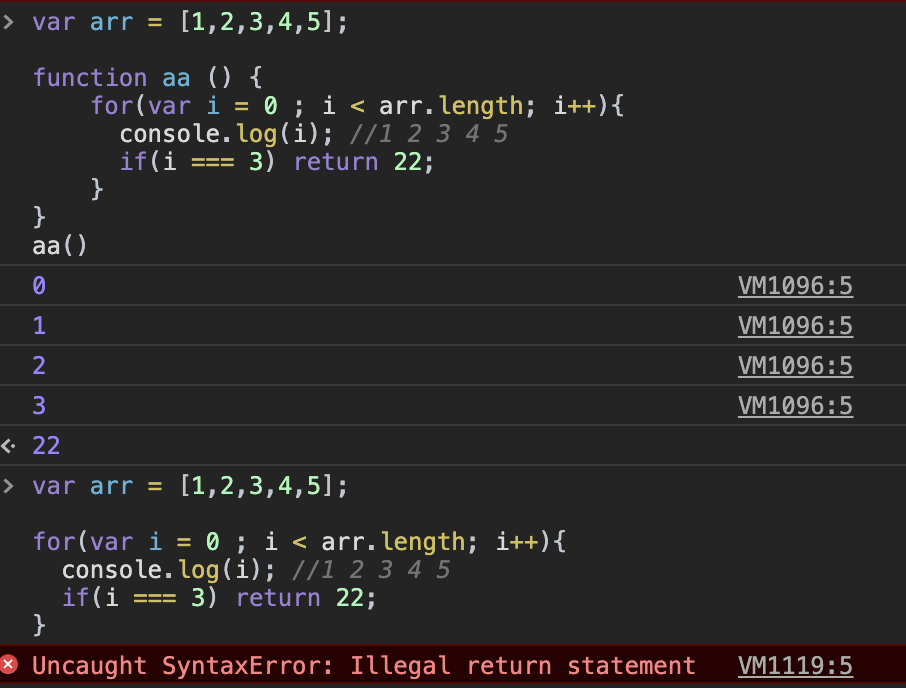
但是用return 需要外面套一层函数, 如图。
不足:重复获取数组长度
使用临时变量,将长度缓存起来,避免重复获取数组长度,当数组较大时优化效果才会比较明显。
这种方法基本上是所有循环遍历方法中性能最高的一种
二、 for..in..循环 (可以遍历字符串、对象、数组)
这个循环很多人爱用,可以用来枚举对象的属性,但实际上,经分析测试,在众多的循环遍历方式中,它的效率是最低的(但在枚举对象属性上性能最好 via @liby的comment),所以一般枚举对象的属性的时候用它
三、 forEach 循环(不能遍历字符串、对象)
不能break停止循环,可以读写。
数组自带的foreach循环,使用频率较高,实际上性能比普通for循环弱
四、 for..of..循环 (需要ES6支持,且不能遍历对象)
对于for...of的循环,可以由break, throw continue 或return终止,但只读不能写。
在数组的用法和forEach挺相似,都是操作数组的元素
打断一下,如果要用forEach 和 for of 遍历对象咋整?
不能直接遍历对象,但是可以结合Object.entries获取index
实际上也是通过 Object.entries去把对象{key, value} 转成[[key, value]], 实际上操作的还是数组
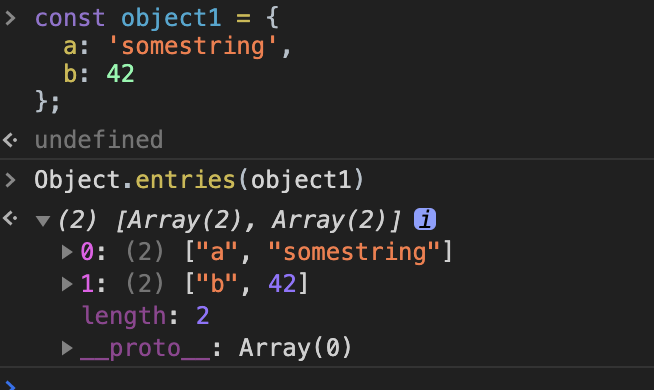
五、map
map是操作每一个arr的元素,如上例子中对数组的每一个元素执行pow方法(需要是个有return的function)。最后的结果仍是数组。
这种方式也是用的比较广泛的,虽然用起来比较优雅,但实际效率还比不上foreach
最后
个人喜欢需要return一个同样长度的数组的就用map,遍历对象用for in,正常其他用普通for循环就好了,性能好能读写能break return,当然不考虑局限性forEach和for of语法会更简洁
js遍历方式总结
JS几种数组遍历方式以及性能分析对比
The text was updated successfully, but these errors were encountered: