-
Notifications
You must be signed in to change notification settings - Fork 43
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Avoid a deadlock updating the network D-Bus tree (#966)
## Problem If the network service receives an `Apply` at the same time as any other request, it may get blocked. ## Solution Updating the tree must be done in a separate task. Otherwise, it could fight with an incoming request for the ObjectServer mutex, blocking the actions dispatching. ## Using tokio-console I decided to use [tokio-console](https://docs.rs/tokio-console/latest/tokio_console/) to make it easier to find the problem. This tool collects and displays information about the asynchronous tasks in your program. In the screenshot below you can see that the D-Bus `Set` dispatchers are blocked. 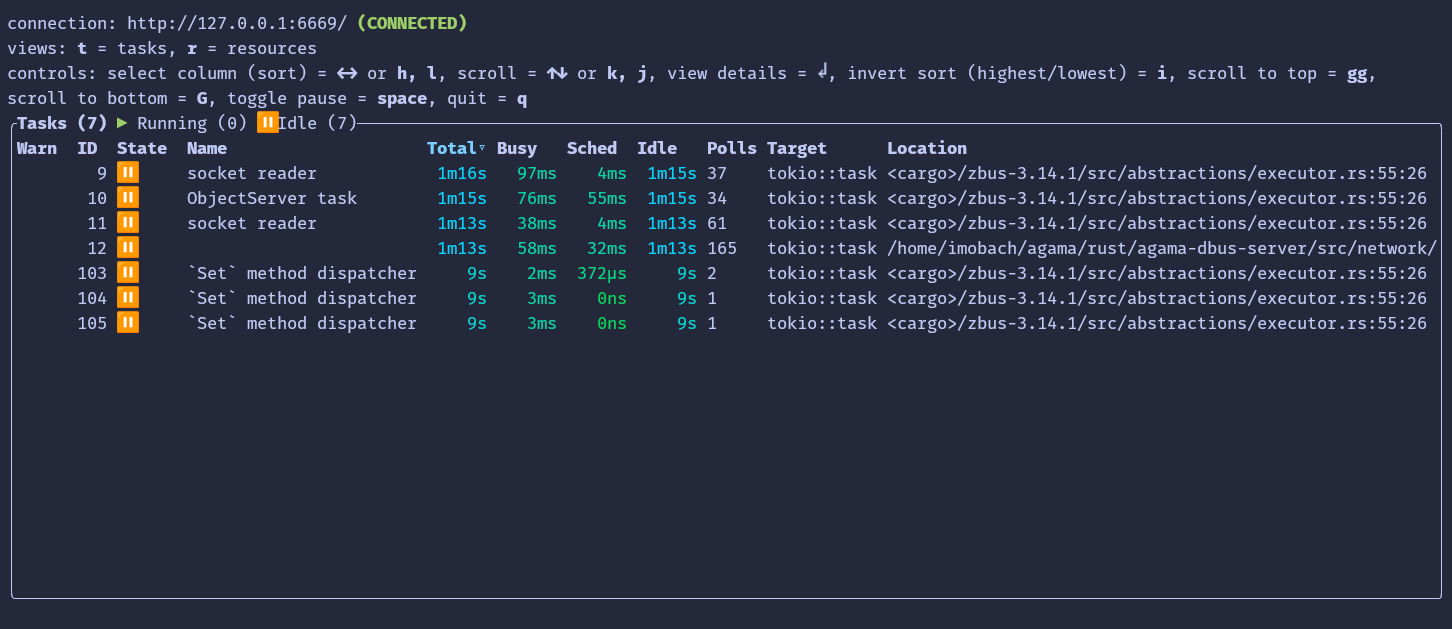 Once you find the problem, it is kind of "obvious", but it may take some time until you figure out what is happening. To use `tokio-console` you need to add some instrumentation to your program and, at this point, it implies adding some unstable Tokio APIs. So if you want to give it a try, you can try the code in the [tokio-console branch](https://github.com/openSUSE/agama/compare/tokio-console).
- Loading branch information
Showing
1 changed file
with
35 additions
and
17 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters