forked from rust-lang/rust
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Auto merge of rust-lang#13638 - DesmondWillowbrook:hover-rest-pat-mvp…
…, r=Veykril feat: adds hover hint to ".." in record pattern Hovering on the "rest" pattern in struct destructuring, ```rust struct Baz { a: u32, b: u32, c: u32, d: u32 } let Baz { a, b, ..$0} = Baz { a: 1, b: 2, c: 3, d: 4 }; ``` shows: ``` .., c: u32, d: u32 ``` Currently only works with struct patterns. 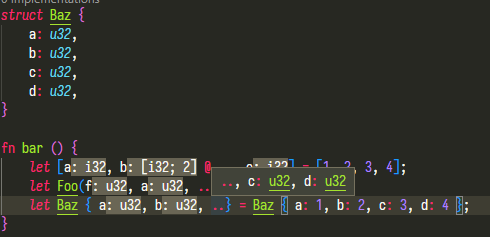
- Loading branch information
Showing
3 changed files
with
140 additions
and
44 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters