-
Notifications
You must be signed in to change notification settings - Fork 7
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Brian Joseph Petro
committed
Sep 18, 2024
1 parent
714451c
commit ca3aae7
Showing
129 changed files
with
7,533 additions
and
3,356 deletions.
There are no files selected for viewing
Large diffs are not rendered by default.
Oops, something went wrong.
File renamed without changes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,166 @@ | ||
# Smart Chat Model | ||
Used to integrate OpenAI GPT-4, Antrhopic Claude, Google Gemini & Cohere Command-r in [Smart Connections](https://github.com/brianpetro/obsidian-smart-connections). | ||
|
||
Try the [demo](https://brianpetro.github.io/jsbrains/sample-web) or browse the [demo code here](https://github.com/brianpetro/jsbrains/tree/main/assets/sample-web.html). | ||
|
||
[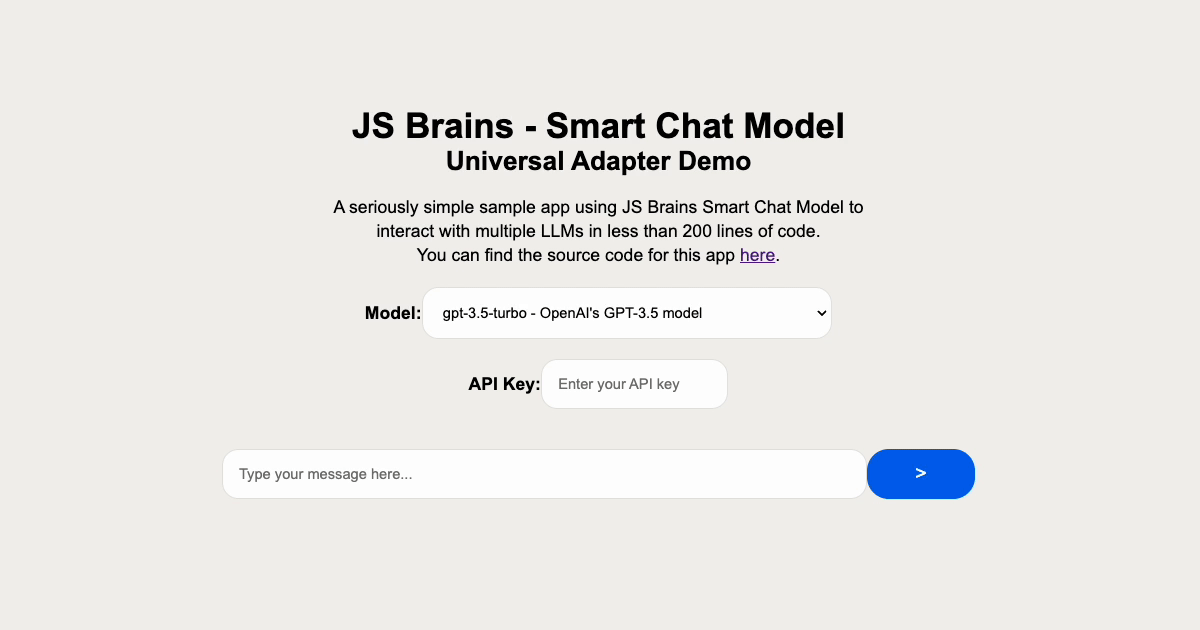](https://brianpetro.github.io/jsbrains/sample-web) | ||
|
||
## SmartChatModel Usage Guide | ||
```bash | ||
npm install smart-chat-model | ||
``` | ||
|
||
`SmartChatModel` is a comprehensive class designed to facilitate interactions with chat models, supporting features like streaming responses, handling tool calls, and customizing requests through adapters. It's built to be adaptable for various chat model configurations and platforms. | ||
The SmartChatModel class provides a flexible and powerful way to interact with chat models, supporting advanced features like streaming, tool calls, and request customization. By following this guide, you should be able to integrate and use SmartChatModel effectively in your projects. | ||
|
||
### Initialization | ||
To initialize a SmartChatModel instance, you need to provide the main environment object, a model key to select the configuration from chat_models.json, and optional user-defined options to override the default model configuration. | ||
|
||
```js | ||
const { SmartChatModel } = require('./chat.js'); | ||
|
||
const mainEnv = {}; // Your main environment object | ||
|
||
const modelKey = 'your_model_key'; // Key from chat_models.json | ||
|
||
const options = {}; // Optional overrides | ||
|
||
const chatModel = new SmartChatModel(mainEnv, modelKey, options); | ||
``` | ||
|
||
### Making Requests | ||
To make a request to the chat model, use the complete method. This method allows for customization of the request through options and can handle both streaming and non-streaming responses. | ||
|
||
```js | ||
const requestOptions = { | ||
|
||
messages: [{ role: "user", content: "Hello" }], | ||
|
||
temperature: 0.3, | ||
|
||
max_tokens: 100, | ||
|
||
stream: false, // Set to true for streaming responses | ||
|
||
}; | ||
|
||
chatModel.complete(requestOptions) | ||
|
||
.then(response => { | ||
|
||
console.log("Response:", response); | ||
|
||
}) | ||
|
||
.catch(error => { | ||
|
||
console.error("Error:", error); | ||
|
||
}); | ||
``` | ||
|
||
|
||
### Streaming Responses | ||
If the chat model supports streaming and it's enabled in the configuration, you can set stream: true in the request options to receive streaming responses. The SmartChatModel will handle the streaming logic internally, including starting and stopping the stream as needed. | ||
|
||
### Handling Tool Calls | ||
The SmartChatModel can automatically detect and handle tool calls within responses. It uses the configured adapters to interpret the tool call data and executes the corresponding tool handler if a valid tool call is detected. | ||
|
||
### Customization with Adapters | ||
Adapters allow for customization of request and response handling. If your use case requires specific handling logic, you can create a custom adapter and specify it in the model configuration. The SmartChatModel will use this adapter for preparing requests, parsing responses, and handling tool calls. | ||
|
||
### Counting Tokens | ||
The count_tokens and estimate_tokens methods are available for estimating the number of tokens in a given input. This can be useful for managing request sizes and understanding the complexity of inputs. | ||
|
||
```js | ||
const input = "Hello, world!"; | ||
|
||
chatModel.count_tokens(input) | ||
|
||
.then(tokenCount => { | ||
|
||
console.log("Token count:", tokenCount); | ||
|
||
}); | ||
``` | ||
|
||
### Testing API Key | ||
The test_api_key method allows you to verify if the provided API key is valid by making a test request to the chat model. | ||
|
||
```js | ||
chatModel.test_api_key() | ||
|
||
.then(isValid => { | ||
|
||
console.log("API key valid:", isValid); | ||
|
||
}); | ||
``` | ||
|
||
|
||
|
||
|
||
## Adapter Pattern for Platform Compatibility | ||
The `SmartChatModel` class is designed to be extensible and compatible with various chat platforms. This is achieved through the use of the adapter pattern, which abstracts platform-specific logic into separate adapter classes. Each adapter implements a consistent interface that `SmartChatModel` can interact with, regardless of the underlying platform differences. | ||
|
||
### Purpose | ||
The adapter pattern allows for the easy integration of new platforms by encapsulating the API-specific logic within adapters. This ensures that the core logic of `SmartChatModel` remains clean and focused, while still being able to support a wide range of platforms. | ||
|
||
### Key Adapter Methods | ||
Adapters must implement the following methods to be compatible with SmartChatModel: | ||
|
||
##### Converting options into the platform-specific request format | ||
- `prepare_request_body(opts)`: | ||
|
||
##### Extracting the message content from the platform's response. | ||
- `get_message(resp_json)` | ||
- `get_message_content(resp_json)` | ||
|
||
##### Methods for extracting and handling tool call information from the response. | ||
- `get_tool_call(resp_json)` | ||
- `get_tool_name(tool_call)` | ||
- `get_tool_call_content(tool_call)` | ||
|
||
##### Handling streaming responses. | ||
- `get_text_chunk_from_stream(event)` | ||
- `is_end_of_stream(event)`: | ||
|
||
##### Providing token counting for input validation or estimation. | ||
- `async count_tokens(input)` | ||
- `estimate_tokens(input)` | ||
|
||
### Implementing a New Adapter | ||
To add support for a new platform, follow these steps: | ||
##### Create Adapter Class | ||
1. Create a new file in the adapters directory for the platform, e.g., `new_platform.js`. | ||
2. Define a class `NewPlatformAdapter` that implements the required methods. | ||
|
||
```js | ||
class NewPlatformAdapter { | ||
prepare_request_body(opts) { /* Implementation */ } | ||
get_message_content(json) { /* Implementation */ } | ||
// Implement other required methods... | ||
} | ||
|
||
exports.NewPlatformAdapter = NewPlatformAdapter; | ||
``` | ||
##### Integrate Adapter | ||
1. Add the new adapter to `adapters.js` which exports all of the adapters. | ||
2. Ensure `SmartChatModel` initializes it based on the `config.adapter`. | ||
|
||
### Using Adapters in SmartChatModel | ||
`SmartChatModel` interacts with adapters by checking for the implementation of specific methods and calling them. This allows for flexible handling of different platform behaviors without modifying the core logic. | ||
|
||
For example, to prepare the request body: | ||
|
||
```js | ||
req.body = JSON.stringify(this.adapter?.prepare_request_body ? this.adapter.prepare_request_body(body) : body); | ||
``` | ||
And to handle streaming responses: | ||
```js | ||
let text_chunk = this.adapter?.get_text_chunk_from_stream ? this.adapter.get_text_chunk_from_stream(event) : defaultTextChunkHandling(event); | ||
``` | ||
This pattern ensures that `SmartChatModel` remains adaptable and can easily extend support to new platforms by adding corresponding adapters. |
File renamed without changes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,158 @@ | ||
/** | ||
* AnthropicAdapter class provides methods to adapt the chat model interactions specifically for the Anthropic model. | ||
* It includes methods to prepare request bodies, count and estimate tokens, and handle tool calls and messages. | ||
*/ | ||
class AnthropicAdapter { | ||
/** | ||
* Prepares the request body for the Anthropic API by converting ChatML format to a format compatible with Anthropic. | ||
* @param {Object} opts - The options object containing messages and other parameters in ChatML format. | ||
* @returns {Object} The request body formatted for the Anthropic API. | ||
*/ | ||
prepare_request_body(opts) { return chatml_to_anthropic(opts); } | ||
/** | ||
* Counts the tokens in the input by estimating them, as the Anthropic model does not provide a direct method. | ||
* @param {string|Object} input - The input text or object to count tokens in. | ||
* @returns {Promise<number>} The estimated number of tokens in the input. | ||
*/ | ||
async count_tokens(input) { | ||
// Currently, the Anthropic model does not provide a way to count tokens | ||
return this.estimate_tokens(input); | ||
} | ||
/** | ||
* Estimates the number of tokens in the input based on a rough average token size. | ||
* @param {string|Object} input - The input text or object to estimate tokens in. | ||
* @returns {number} The estimated number of tokens. | ||
*/ | ||
estimate_tokens(input){ | ||
if(typeof input === 'object') input = JSON.stringify(input); | ||
// Note: The division by 6 is a rough estimate based on observed average token size. | ||
return Math.ceil(input.length / 6); // Use Math.ceil for a more accurate count | ||
} | ||
/** | ||
* Extracts the first tool call from the JSON response content. | ||
* @param {Object} json - The JSON response from which to extract the tool call. | ||
* @returns {Object|null} The first tool call found, or null if none exist. | ||
*/ | ||
get_tool_call(json){ | ||
return json.content.find(msg => msg.type === 'tool_use'); | ||
} | ||
/** | ||
* Retrieves the input content of a tool call. | ||
* @param {Object} tool_call - The tool call object from which to extract the input. | ||
* @returns {Object} The input of the tool call. | ||
*/ | ||
get_tool_call_content(tool_call){ | ||
return tool_call.input; | ||
} | ||
/** | ||
* Retrieves the name of the tool from a tool call object. | ||
* @param {Object} tool_call - The tool call object from which to extract the name. | ||
* @returns {string} The name of the tool. | ||
*/ | ||
get_tool_name(tool_call){ | ||
return tool_call.name; | ||
} | ||
/** | ||
* Extracts the first message from the JSON response content. | ||
* @param {Object} json - The JSON response from which to extract the message. | ||
* @returns {Object|null} The first message found, or null if none exist. | ||
*/ | ||
get_message(json){ return json.content?.[0]; } | ||
/** | ||
* Retrieves the content of the first message from the JSON response. | ||
* @param {Object} json - The JSON response from which to extract the message content. | ||
* @returns {string|null} The content of the first message, or null if no message is found. | ||
*/ | ||
get_message_content(json) { return this.get_message(json)?.[this.get_message(json)?.type]; } | ||
} | ||
exports.AnthropicAdapter = AnthropicAdapter; | ||
// https://docs.anthropic.com/claude/reference/messages_post | ||
/** | ||
* Convert a ChatML object to an Anthropic object | ||
* @param {Object} opts - The ChatML object | ||
* @description This function converts a ChatML object to an Anthropic object. It filters out system messages and adds a system message prior to the last user message. | ||
* @returns {Object} - The Anthropic object | ||
*/ | ||
function chatml_to_anthropic(opts) { | ||
let tool_counter = 0; | ||
const messages = opts.messages | ||
.filter(msg => msg.role !== 'system') | ||
.map(m => { | ||
if(m.role === 'tool'){ | ||
return { role: 'user', content: [ | ||
{ | ||
type: 'tool_result', | ||
tool_use_id: `tool-${tool_counter}`, | ||
content: m.content | ||
} | ||
]}; | ||
} | ||
if(m.role === 'assistant' && m.tool_calls){ | ||
tool_counter++; | ||
const out = { | ||
role: m.role, | ||
content: m.tool_calls.map(c => ({ | ||
type: 'tool_use', | ||
id: `tool-${tool_counter}`, | ||
name: c.function.name, | ||
input: (typeof c.function.arguments === 'string') ? JSON.parse(c.function.arguments) : c.function.arguments | ||
})) | ||
}; | ||
if(m.content){ | ||
if(typeof m.content === 'string') out.content.push({type: 'text', text: m.content}); | ||
else m.content.forEach(c => out.content.push(c)); | ||
} | ||
return out; | ||
} | ||
if(typeof m.content === 'string') return { role: m.role, content: m.content }; | ||
if(Array.isArray(m.content)){ | ||
const content = m.content.map(c => { | ||
if(c.type === 'text') return {type: 'text', text: c.text}; | ||
if(c.type === 'image_url'){ | ||
const image_url = c.image_url.url; | ||
let media_type = image_url.split(":")[1].split(";")[0]; | ||
if(media_type === 'image/jpg') media_type = 'image/jpeg'; | ||
return {type: 'image', source: {type: 'base64', media_type: media_type, data: image_url.split(",")[1]}}; | ||
} | ||
}); | ||
return { role: m.role, content }; | ||
} | ||
return m; | ||
}) | ||
; | ||
const { model, max_tokens, temperature, tools, tool_choice } = opts; | ||
// DO: handled better (Smart Connections specific) | ||
// get index of last system message | ||
const last_system_idx = opts.messages.findLastIndex(msg => msg.role === 'system' && msg.content.includes('---BEGIN')); | ||
if (last_system_idx > -1) { | ||
const system_prompt = '<context>\n' + opts.messages[last_system_idx].content + '\n</context>\n'; | ||
messages[messages.length - 1].content = system_prompt + messages[messages.length - 1].content; | ||
} | ||
const out = { | ||
messages, | ||
model, | ||
max_tokens, | ||
temperature, | ||
} | ||
if(tools){ | ||
out.tools = tools.map(tool => ({ | ||
name: tool.function.name, | ||
description: tool.function.description, | ||
input_schema: tool.function.parameters, | ||
})); | ||
if(tool_choice?.type === 'function'){ | ||
// add "Use the ${tool.name} tool" to the last user message | ||
const tool_prompt = `Use the "${tool_choice.function.name}" tool!`; | ||
const last_user_idx = out.messages.findLastIndex(msg => msg.role === 'user'); | ||
out.messages[last_user_idx].content += '\n' + tool_prompt; | ||
out.system = `Required: use the "${tool_choice.function.name}" tool!`; | ||
} | ||
} | ||
// DO: handled better (Smart Connections specific) | ||
// if system message exists prior to last_system_idx AND does not include "---BEGIN" then add to body.system | ||
const last_non_context_system_idx = opts.messages.findLastIndex(msg => msg.role === 'system' && !msg.content.includes('---BEGIN')); | ||
if(last_non_context_system_idx > -1) out.system = opts.messages[last_non_context_system_idx].content; | ||
return out; | ||
} | ||
exports.chatml_to_anthropic = chatml_to_anthropic; | ||
|
Oops, something went wrong.