-
Notifications
You must be signed in to change notification settings - Fork 272
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
chore: restructure ByTestId queries code by predicate type #608
Merged
Merged
Changes from all commits
Commits
Show all changes
9 commits
Select commit
Hold shift + click to select a range
9871677
refactor: add makeQueries functions
MattAgn 791c5d5
refactor: use new query builders on byTestId
MattAgn ee05201
rebase and rearrange to a bit
thymikee 59a8490
add fixme
thymikee cf5d3d3
refactor: make single function makeQueries
MattAgn f822da0
doc: add TODO to do before end of pr
MattAgn 6f5b08d
fix: fix flow issue
MattAgn cc05823
parametrize Queries
thymikee 47c2fc4
chore: delete duplicated test
MattAgn File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,173 @@ | ||
// @flow | ||
import React from 'react'; | ||
import { View, Text, TextInput, TouchableOpacity, Button } from 'react-native'; | ||
import { render } from '..'; | ||
|
||
const PLACEHOLDER_FRESHNESS = 'Add custom freshness'; | ||
const PLACEHOLDER_CHEF = 'Who inspected freshness?'; | ||
const INPUT_FRESHNESS = 'Custom Freshie'; | ||
const INPUT_CHEF = 'I inspected freshie'; | ||
|
||
class MyButton extends React.Component<any> { | ||
render() { | ||
return ( | ||
<TouchableOpacity onPress={this.props.onPress}> | ||
<Text>{this.props.children}</Text> | ||
</TouchableOpacity> | ||
); | ||
} | ||
} | ||
|
||
class Banana extends React.Component<any, any> { | ||
state = { | ||
fresh: false, | ||
}; | ||
|
||
componentDidUpdate() { | ||
if (this.props.onUpdate) { | ||
this.props.onUpdate(); | ||
} | ||
} | ||
|
||
componentWillUnmount() { | ||
if (this.props.onUnmount) { | ||
this.props.onUnmount(); | ||
} | ||
} | ||
|
||
changeFresh = () => { | ||
this.setState((state) => ({ | ||
fresh: !state.fresh, | ||
})); | ||
}; | ||
|
||
render() { | ||
const test = 0; | ||
return ( | ||
<View> | ||
<Text>Is the banana fresh?</Text> | ||
<Text testID="bananaFresh"> | ||
{this.state.fresh ? 'fresh' : 'not fresh'} | ||
</Text> | ||
<TextInput | ||
testID="bananaCustomFreshness" | ||
placeholder={PLACEHOLDER_FRESHNESS} | ||
value={INPUT_FRESHNESS} | ||
/> | ||
<TextInput | ||
testID="bananaChef" | ||
placeholder={PLACEHOLDER_CHEF} | ||
value={INPUT_CHEF} | ||
/> | ||
<MyButton onPress={this.changeFresh} type="primary"> | ||
Change freshness! | ||
</MyButton> | ||
<Text testID="duplicateText">First Text</Text> | ||
<Text testID="duplicateText">Second Text</Text> | ||
<Text>{test}</Text> | ||
</View> | ||
); | ||
} | ||
} | ||
|
||
const MyComponent = () => { | ||
return <Text>My Component</Text>; | ||
}; | ||
|
||
test('getByTestId returns only native elements', () => { | ||
const { getByTestId, getAllByTestId } = render( | ||
<View> | ||
<Text testID="text">Text</Text> | ||
<TextInput testID="textInput" /> | ||
<View testID="view" /> | ||
<Button testID="button" title="Button" onPress={jest.fn()} /> | ||
<MyComponent testID="myComponent" /> | ||
</View> | ||
); | ||
|
||
expect(getByTestId('text')).toBeTruthy(); | ||
expect(getByTestId('textInput')).toBeTruthy(); | ||
expect(getByTestId('view')).toBeTruthy(); | ||
expect(getByTestId('button')).toBeTruthy(); | ||
|
||
expect(getAllByTestId('text')).toHaveLength(1); | ||
expect(getAllByTestId('textInput')).toHaveLength(1); | ||
expect(getAllByTestId('view')).toHaveLength(1); | ||
expect(getAllByTestId('button')).toHaveLength(1); | ||
|
||
expect(() => getByTestId('myComponent')).toThrowError( | ||
'Unable to find an element with testID: myComponent' | ||
); | ||
expect(() => getAllByTestId('myComponent')).toThrowError( | ||
'Unable to find an element with testID: myComponent' | ||
); | ||
}); | ||
|
||
test('supports a regex matcher', () => { | ||
const { getByTestId, getAllByTestId } = render( | ||
<View> | ||
<Text testID="text">Text</Text> | ||
<TextInput testID="textInput" /> | ||
<View testID="view" /> | ||
<Button testID="button" title="Button" onPress={jest.fn()} /> | ||
<MyComponent testID="myComponent" /> | ||
</View> | ||
); | ||
|
||
expect(getByTestId(/view/)).toBeTruthy(); | ||
expect(getAllByTestId(/text/)).toHaveLength(2); | ||
}); | ||
|
||
test('getByTestId, queryByTestId', () => { | ||
const { getByTestId, queryByTestId } = render(<Banana />); | ||
const component = getByTestId('bananaFresh'); | ||
|
||
expect(component.props.children).toBe('not fresh'); | ||
expect(() => getByTestId('InExistent')).toThrow( | ||
'Unable to find an element with testID: InExistent' | ||
); | ||
|
||
expect(getByTestId('bananaFresh')).toBe(component); | ||
expect(queryByTestId('InExistent')).toBeNull(); | ||
}); | ||
|
||
test('getAllByTestId, queryAllByTestId', () => { | ||
const { getAllByTestId, queryAllByTestId } = render(<Banana />); | ||
const textElements = getAllByTestId('duplicateText'); | ||
|
||
expect(textElements.length).toBe(2); | ||
expect(textElements[0].props.children).toBe('First Text'); | ||
expect(textElements[1].props.children).toBe('Second Text'); | ||
expect(() => getAllByTestId('nonExistentTestId')).toThrow( | ||
'Unable to find an element with testID: nonExistentTestId' | ||
); | ||
|
||
const queriedTextElements = queryAllByTestId('duplicateText'); | ||
|
||
expect(queriedTextElements.length).toBe(2); | ||
expect(queriedTextElements[0]).toBe(textElements[0]); | ||
expect(queriedTextElements[1]).toBe(textElements[1]); | ||
expect(queryAllByTestId('nonExistentTestId')).toHaveLength(0); | ||
}); | ||
|
||
test('findByTestId and findAllByTestId work asynchronously', async () => { | ||
const options = { timeout: 10 }; // Short timeout so that this test runs quickly | ||
const { rerender, findByTestId, findAllByTestId } = render(<View />); | ||
await expect(findByTestId('aTestId', options)).rejects.toBeTruthy(); | ||
await expect(findAllByTestId('aTestId', options)).rejects.toBeTruthy(); | ||
|
||
setTimeout( | ||
() => | ||
rerender( | ||
<View testID="aTestId"> | ||
<Text>Some Text</Text> | ||
<TextInput placeholder="Placeholder Text" /> | ||
<TextInput value="Display Value" /> | ||
</View> | ||
), | ||
20 | ||
); | ||
|
||
await expect(findByTestId('aTestId')).resolves.toBeTruthy(); | ||
await expect(findAllByTestId('aTestId')).resolves.toHaveLength(1); | ||
}, 20000); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,46 @@ | ||
// @flow | ||
import { makeQueries } from './makeQueries'; | ||
import type { Queries } from './makeQueries'; | ||
|
||
const getNodeByTestId = (node, testID) => { | ||
return typeof testID === 'string' | ||
? testID === node.props.testID | ||
: testID.test(node.props.testID); | ||
}; | ||
|
||
const queryAllByTestId = ( | ||
instance: ReactTestInstance | ||
): ((testId: string | RegExp) => Array<ReactTestInstance>) => | ||
function getAllByTestIdFn(testId) { | ||
const results = instance | ||
.findAll((node) => getNodeByTestId(node, testId)) | ||
.filter((element) => typeof element.type === 'string'); | ||
|
||
return results; | ||
}; | ||
|
||
const getMultipleError = (testId) => | ||
`Found multiple elements with testID: ${String(testId)}`; | ||
const getMissingError = (testId) => | ||
`Unable to find an element with testID: ${String(testId)}`; | ||
|
||
const { | ||
getBy: getByTestId, | ||
getAllBy: getAllByTestId, | ||
queryBy: queryByTestId, | ||
findBy: findByTestId, | ||
findAllBy: findAllByTestId, | ||
}: Queries<string | RegExp> = makeQueries( | ||
queryAllByTestId, | ||
getMissingError, | ||
getMultipleError | ||
); | ||
|
||
export { | ||
getByTestId, | ||
getAllByTestId, | ||
queryByTestId, | ||
findByTestId, | ||
findAllByTestId, | ||
queryAllByTestId, | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Consider adding explicit types
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
We can address that in a followup if necessary (I suspect Flow infers that, as it's internal fn)
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I confirm, flow infers it
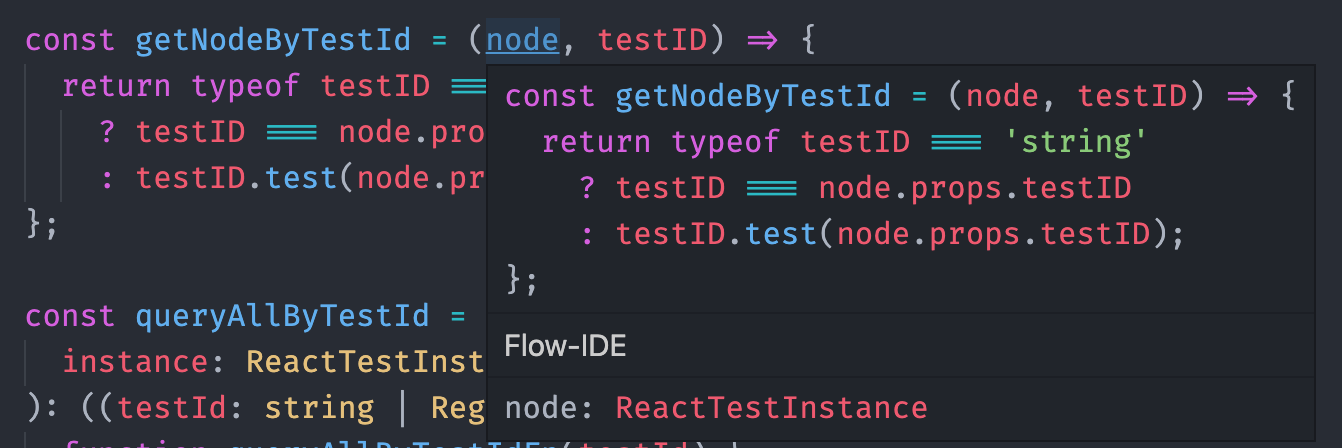