-
Notifications
You must be signed in to change notification settings - Fork 36
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: [WD-16972] TLS user management spike. (#1026)
## Done - Created "Create Identity" Button - Create modal to create new TLS fine grained identities. - A few new functions and a new LXDTrustToken type. ## User Management Spike [✔️] A user needs to be able to create a TLS user (Creates a pending user) [✔️] A user needs to be able to destroy a TLS user ([WD-16894](#1008)) Fixes: - Inability to create TLS fine grained tokens through the UI. ## QA 1. Run the LXD-UI: - On the demo server via the link posted by @webteam-app below. This is only available for PRs created by collaborators of the repo. Ask @mas-who or @edlerd for access. - With a local copy of this branch, [build and run as described in the docs](../CONTRIBUTING.md#setting-up-for-development). 2. Perform the following QA steps: - [List the steps to QA the new feature(s) or prove that a bug has been resolved] ## Screenshots 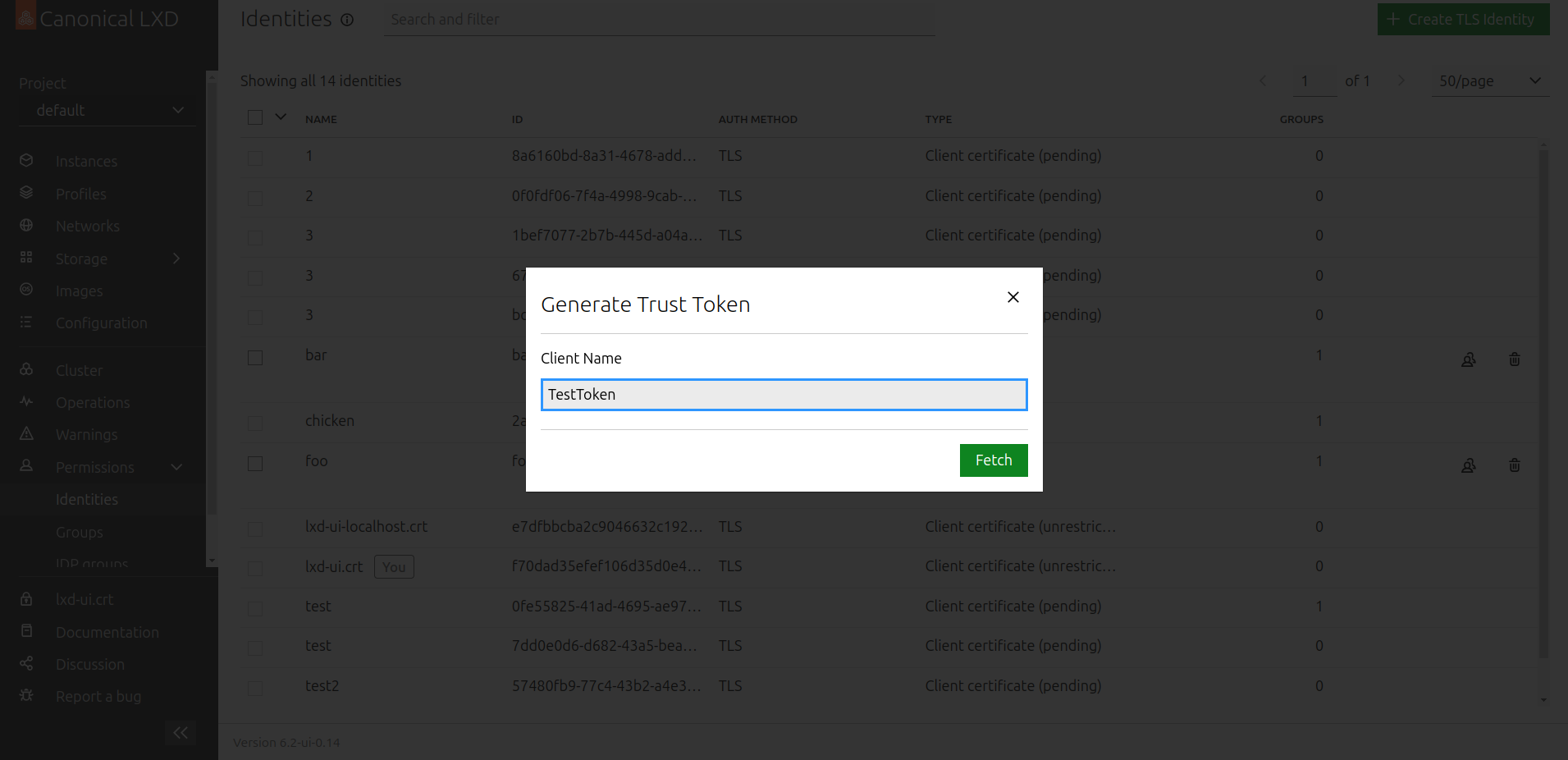  [WD-16894]: https://warthogs.atlassian.net/browse/WD-16894?atlOrigin=eyJpIjoiNWRkNTljNzYxNjVmNDY3MDlhMDU5Y2ZhYzA5YTRkZjUiLCJwIjoiZ2l0aHViLWNvbS1KU1cifQ
- Loading branch information
Showing
9 changed files
with
246 additions
and
12 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,145 @@ | ||
import { FC, useState } from "react"; | ||
import { | ||
ActionButton, | ||
Button, | ||
Icon, | ||
Input, | ||
Modal, | ||
Notification, | ||
} from "@canonical/react-components"; | ||
import { useFormik } from "formik"; | ||
import { createFineGrainedTlsIdentity } from "api/auth-identities"; | ||
import { base64EncodeObject } from "util/helpers"; | ||
import { useQueryClient } from "@tanstack/react-query"; | ||
import { queryKeys } from "util/queryKeys"; | ||
|
||
interface Props { | ||
onClose: () => void; | ||
} | ||
|
||
const CreateIdentityModal: FC<Props> = ({ onClose }) => { | ||
const [token, setToken] = useState<string | null>(null); | ||
const [error, setError] = useState(false); | ||
const [copied, setCopied] = useState(false); | ||
const queryClient = useQueryClient(); | ||
|
||
const formik = useFormik({ | ||
initialValues: { | ||
identityName: "", | ||
}, | ||
onSubmit: (values) => { | ||
setError(false); | ||
createFineGrainedTlsIdentity(values.identityName) | ||
.then((response) => { | ||
const encodedToken = base64EncodeObject(response); | ||
setToken(encodedToken); | ||
|
||
void queryClient.invalidateQueries({ | ||
queryKey: [queryKeys.identities], | ||
}); | ||
}) | ||
.catch(() => { | ||
setError(true); | ||
}); | ||
}, | ||
}); | ||
|
||
const handleCopy = async () => { | ||
if (token) { | ||
try { | ||
await navigator.clipboard.writeText(token); | ||
setCopied(true); | ||
|
||
setTimeout(() => { | ||
setCopied(false); | ||
}, 5000); | ||
} catch (error) { | ||
console.error(error); | ||
} | ||
} | ||
}; | ||
|
||
return ( | ||
<Modal | ||
close={onClose} | ||
className="create-tls-identity" | ||
title={token ? "Identity has been created" : "Generate trust token"} | ||
buttonRow={ | ||
<> | ||
{token && ( | ||
<> | ||
<Button | ||
aria-label={ | ||
copied ? "Copied to clipboard" : "Copy to clipboard" | ||
} | ||
title="Copy token" | ||
className="u-no-margin--bottom" | ||
onClick={() => handleCopy()} | ||
type="button" | ||
hasIcon | ||
> | ||
<Icon name={copied ? "task-outstanding" : "copy"} /> | ||
</Button> | ||
|
||
<Button | ||
aria-label="Close" | ||
className="u-no-margin--bottom" | ||
onClick={onClose} | ||
type="button" | ||
> | ||
Close | ||
</Button> | ||
</> | ||
)} | ||
{!token && ( | ||
<ActionButton | ||
aria-label="Generate token" | ||
appearance="positive" | ||
className="u-no-margin--bottom" | ||
onClick={() => void formik.submitForm()} | ||
disabled={ | ||
formik.values.identityName.length === 0 || formik.isSubmitting | ||
} | ||
loading={formik.isSubmitting} | ||
> | ||
Generate token | ||
</ActionButton> | ||
)} | ||
</> | ||
} | ||
> | ||
{error && ( | ||
<Notification | ||
severity="negative" | ||
title="Token creation failed" | ||
></Notification> | ||
)} | ||
{!token && ( | ||
<Input | ||
id="identityName" | ||
type="text" | ||
label="Identity Name" | ||
onBlur={formik.handleBlur} | ||
onChange={formik.handleChange} | ||
value={formik.values.identityName} | ||
/> | ||
)} | ||
{token && ( | ||
<> | ||
<p>The token below can be used to log in as the new identity.</p> | ||
|
||
<Notification | ||
severity="caution" | ||
title="Make sure to copy the token now as it will not be shown again." | ||
></Notification> | ||
|
||
<div className="token-code-block"> | ||
<code>{token}</code> | ||
</div> | ||
</> | ||
)} | ||
</Modal> | ||
); | ||
}; | ||
|
||
export default CreateIdentityModal; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
import { Button, Icon } from "@canonical/react-components"; | ||
import { useSmallScreen } from "context/useSmallScreen"; | ||
import { FC } from "react"; | ||
import usePortal from "react-useportal"; | ||
import CreateIdentityModal from "./CreateIdentityModal"; | ||
|
||
const CreateTlsIdentityBtn: FC = () => { | ||
const isSmallScreen = useSmallScreen(); | ||
const { openPortal, closePortal, isOpen, Portal } = usePortal(); | ||
|
||
return ( | ||
<> | ||
{isOpen && ( | ||
<Portal> | ||
<CreateIdentityModal onClose={closePortal} /> | ||
</Portal> | ||
)} | ||
<Button | ||
appearance="positive" | ||
className="u-float-right u-no-margin--bottom" | ||
onClick={openPortal} | ||
hasIcon={!isSmallScreen} | ||
> | ||
{!isSmallScreen && <Icon name="plus" light />} | ||
<span>Create TLS Identity</span> | ||
</Button> | ||
</> | ||
); | ||
}; | ||
|
||
export default CreateTlsIdentityBtn; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters