-
Notifications
You must be signed in to change notification settings - Fork 261
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: More hovering information on the LSP (#3282)
Fixes #3281 Fixes dafny-lang/ide-vscode#335 Fixes dafny-lang/ide-vscode#317 Fixes dafny-lang/ide-vscode#210 Example code: ```dafny predicate P(i: int) { i <= 0 } predicate Q(i: int, j: int) { i == j || -i == j } function method Toast(i: int): int requires P(i) method Test(i: int) returns (j: nat) ensures Q(i, j) { if i < 0 { return -i; } else { return Toast(i); } } ``` | Before: | After | | --------|-------| | Hovering Q(i,j)  | 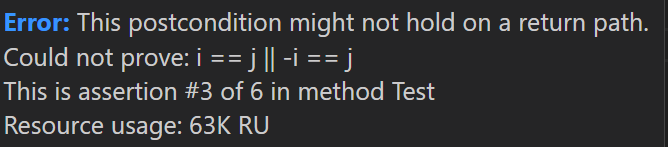 | | Hovering the second return  | 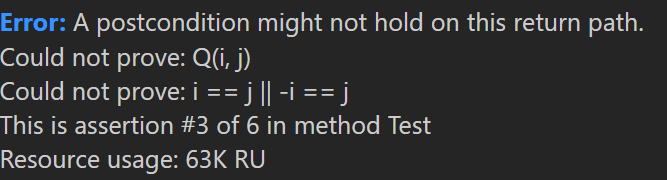 | | Hovering the call to Toast()  | 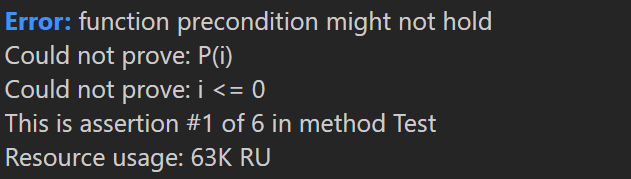 | | Also, on the diagnostics side (and it's clickable!)  |  | | The resolver error appears after all obsolete verification error<br>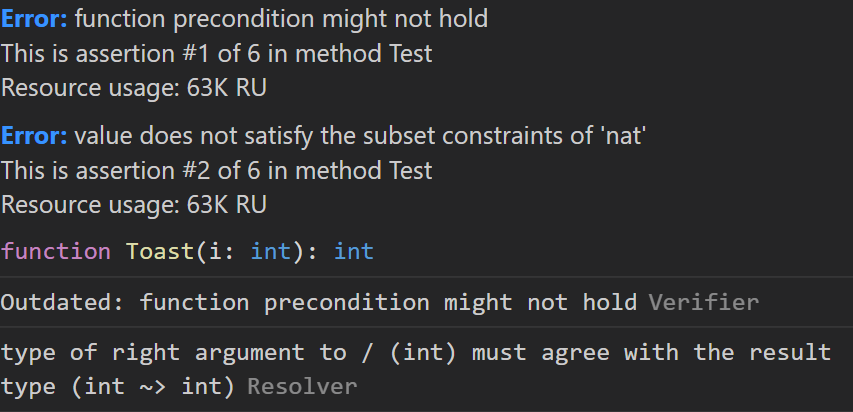 |  | I added language server tests and tested it in VSCode, and it works as expected. <!-- Are you moving a large amount of code? Read CONTRIBUTING.md to learn how to do that while maintaining git history --> <small>By submitting this pull request, I confirm that my contribution is made under the terms of the [MIT license](https://github.com/dafny-lang/dafny/blob/master/LICENSE.txt).</small>
- Loading branch information
1 parent
d0057aa
commit 7e019ba
Showing
15 changed files
with
321 additions
and
27 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.