-
Notifications
You must be signed in to change notification settings - Fork 32
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #10 from diasdavid/reboot
webrtc-explorer reboot
- Loading branch information
Showing
46 changed files
with
14,876 additions
and
602 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,116 +1,125 @@ | ||
webrtc-explorer | ||
======================================= | ||
 | ||
|
||
**tl;dr** `webrtc-explorer` is a [Chord][chord-paper] inspired P2P overlay network designed for the Web platform (browsers), using WebRTC as its transport between peers and WebSockets for Signaling data. Essentially, it enables your peers (browsers) to communicate between each other without the need to have a server as a mediator of messages. | ||
[](https://david-dm.org/diasdavid/webrtc-explorer) | ||
[](https://github.com/diasdavid/WebCompute) | ||
[](https://github.com/feross/standard) | ||
|
||
## Project Information | ||
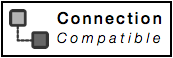 | ||
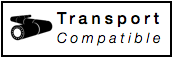 | ||
|
||
> [David Dias MSc in Peer-to-Peer Networks by Technical University of Lisbon](https://github.com/diasdavid/browserCloudjs#research-and-development) | ||
> **tl;dr** `webrtc-explorer` is a [Chord](http://pdos.csail.mit.edu/papers/chord:sigcomm01/chord_sigcomm.pdf) inspired, P2P Network Routing Overlay designed for the Web platform (browsers), using WebRTC as its layer of transport between peers and WebSockets for the exchange of signalling data (setting up a connection and NAT traversal). Essentially, webrtc-explorer enables your peers (browsers) to communicate between each other without the need to have a server to be the mediator. | ||
[](http://www.gsd.inesc-id.pt/) [](http://tecnico.ulisboa.pt/) [](https://github.com/diasdavid/browserCloudjs) | ||
# Usage | ||
|
||
This work was developed by David Dias with supervision by Luís Veiga, all in INESC-ID Lisboa (Distributed Systems Group), Instituto Superior Técnico, Universidade de Lisboa, with the support of Fundação para a Ciência e Tecnologia. | ||
## Install | ||
|
||
More info on the team's work at: | ||
- http://daviddias.me | ||
- http://www.gsd.inesc-id.pt/~lveiga | ||
```sh | ||
> npm install webrtc-explorer | ||
``` | ||
|
||
If you use this project, please acknowledge it in your work by referencing the following document: | ||
If you want to use the Signalling Server that comes with webrtc-explorer, you can use it through your terminal after installing webrtc-explorer globally | ||
|
||
David Dias and Luís Veiga. browserCloud.js A federated community cloud served by a P2P overlay network on top of the web platform. INESC-ID Tec. Rep. 14/2015, Apr. 2015 | ||
```sh | ||
> npm install webrtc-explorer --global | ||
# ... | ||
> sig-server | ||
Signalling Server Started | ||
# now the signalling server is running | ||
``` | ||
|
||
# Badgers | ||
[](https://nodei.co/npm/webrtc-explorer/) | ||
Use [browserify](http://browserify.org) to load transpile your JS code that uses webrtc-explorer, so that all of the dependencies are correctly loaded. | ||
|
||
[](https://gitter.im/diasdavid/webrtc-explorer?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge) | ||
[](https://david-dm.org/diasdavid/webrtc-explorer) | ||
## API | ||
|
||
[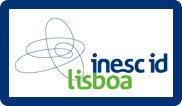](http://www.gsd.inesc-id.pt/) | ||
```javascript | ||
const explorer = require('webrtc-explorer') | ||
``` | ||
|
||
# Properties | ||
#### listen | ||
|
||
- Ids have 48 bits (so that is a multiple of 4 (for hex notation) and doesn't require importing a big-num lib to handle over 53 bits operations) | ||
- The number of fingers of each peer is flexible, however it is recommended to not pass 16 per node (due to browser resource constraints) | ||
- Each peer is responsible for a segment of the hash ring | ||
- The signaling server for webrtc-explorer can be found at: https://github.com/diasdavid/webrtc-explorer-signalling-server | ||
Connects your explorer node to the signalling server, and listens for incomming connections from other peers. | ||
|
||
# Usage | ||
```javascript | ||
const listener = explorer.createListener((socket) => { | ||
// socket with another peer | ||
}) | ||
|
||
listener.listen((err) => { | ||
if (err) { | ||
return console.log('Error listening:', err) | ||
} | ||
console.log('explorer is now listining to incomming connections') | ||
}) | ||
``` | ||
|
||
`webrtc-explorer` uses [browserify](http://browserify.org) | ||
#### dial | ||
|
||
### Create a new peer | ||
Dials into another peer, using the P2P Overlay Routing. | ||
|
||
```javascript | ||
var Explorer = require('webrtc-explorer'); | ||
```JavaScript | ||
const socket = explorer.dial(<peerId> [, <readyCallback>]) | ||
``` | ||
|
||
var config = { | ||
signalingURL: 'http://url-to-webrtc-ring-signaling-server.com' | ||
}; | ||
var peer = new Explorer(config); | ||
Note: since an explorer node routes messages for other peers and itself, it needs first to be ready to 'listen', in order to be able to use the network to send. | ||
|
||
peer.events.on('ready', function () { | ||
// this node is ready | ||
}); | ||
``` | ||
#### updateFinger | ||
|
||
### Register the peer | ||
_not implemented yet_ | ||
|
||
```javascript | ||
peer.events.on('registered', function(data){ | ||
// peer registered with data.peerId | ||
}); | ||
updates a finger on the finger table (if no finger was present on that row, it is added). | ||
|
||
peer.register(); | ||
```JavaScript | ||
explorer.updateFinger(<row>) | ||
``` | ||
|
||
### Send and receive a message | ||
#### updateFingerTable | ||
|
||
peerIds are 48 bits represented in a string using hex format. To send, in one peer: | ||
_not implemented yet_ | ||
|
||
```javascript | ||
var data = 'hey peer, how is it going'; | ||
updates all the rows on the finger table that already had a peer | ||
|
||
peer.send('abcd0f0fdbca', data); | ||
```JavaScript | ||
explorer.updateFingerTable(<row>) | ||
``` | ||
|
||
To receive, in another peer (responsible for that Id) | ||
# Architecture | ||
|
||
```javascript | ||
peer.events.on('message', function(envelope){ | ||
// message from the other peer envelope.data | ||
}); | ||
``` | ||
## Signalling | ||
|
||
### Other options | ||
## Routing | ||
|
||
#### logging | ||
To understand fully webrtc-explorer's core, it is important to be familiar with the [Chord][chord-paper]. | ||
|
||
add the logging flag to your config | ||
## Connections | ||
|
||
```javascript | ||
var config = { | ||
//... | ||
logging: true | ||
}; | ||
``` | ||
## Notes and other properties | ||
|
||
- Ids have 48 bits (so that is a multiple of 4 (for hex notation) and doesn't require importing a big-num lib to handle over 53 bits operations) | ||
- The number of fingers of each peer is flexible, however it is recommended to not pass 16 per node (due to browser resource constraints) | ||
- Each peer is responsible for a segment of the hash ring | ||
|
||
# How does it work | ||
|
||
To understand fully webrtc-explorer's core, it is crucial to be familiar with the [Chord][chord-paper]. webrtc-explorer levarages important battle experience from building webrtc-ring - http://blog.daviddias.me/2014/12/20/webrtc-ring | ||
|
||
* I'll upload my notes and images `soon`, I'm trying to make them as legible as possible. If you have a urgency in one of the parts, please let me know, so that I put that one as a priority. | ||
|
||
## Registering a peer | ||
|
||
## Updating the finger table | ||
|
||
## Signaling between two peers | ||
|
||
## Message routing | ||
|
||
|
||
----------------------------------------------------------------------------- | ||
|
||
## Initial Development and release was supported by INESC-ID (circa Mar 2015) | ||
|
||
> [David Dias MSc in Peer-to-Peer Networks by Technical University of Lisbon](https://github.com/diasdavid/browserCloudjs#research-and-development) | ||
[](http://www.gsd.inesc-id.pt/) [](http://tecnico.ulisboa.pt/) [](https://github.com/diasdavid/browserCloudjs) | ||
|
||
This work was developed by David Dias with supervision by Luís Veiga, all in INESC-ID Lisboa (Distributed Systems Group), Instituto Superior Técnico, Universidade de Lisboa, with the support of Fundação para a Ciência e Tecnologia. | ||
|
||
[chord-paper]: http://pdos.csail.mit.edu/papers/chord:sigcomm01/chord_sigcomm.pdf | ||
More info on the team's work at: | ||
- http://daviddias.me | ||
- http://www.gsd.inesc-id.pt/~lveiga | ||
|
||
If you use this project, please acknowledge it in your work by referencing the following document: | ||
|
||
David Dias and Luís Veiga. browserCloud.js A federated community cloud served by a P2P overlay network on top of the web platform. INESC-ID Tec. Rep. 14/2015, Apr. 2015 |
This file was deleted.
Oops, something went wrong.
Oops, something went wrong.