-
Notifications
You must be signed in to change notification settings - Fork 545
User Guide
dbluck edited this page Jun 17, 2014
·
11 revisions
The lib is available on Maven Central.
dependencies {
compile 'com.github.dmytrodanylyk.android-process-button:library:1.0.0'
}
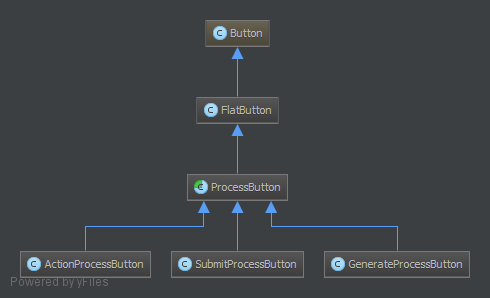
FlatButton
- base class which can be used to display flat button, extends Button
. Configurable by two xml attributes:
<com.dd.processbutton.FlatButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Flat Button"
android:textColor="@android:color/white"
custom:pb_colorNormal="@android:color/holo_blue_light"
custom:pb_colorPressed="@android:color/holo_blue_dark" />
ProcessButton
- abstract class extends FlatButton
, behavior is very similar to android ProgressBar
. Main point is to use setProgress
method to set progress from 0
to 100
.
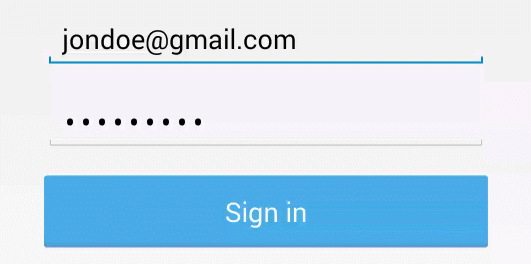
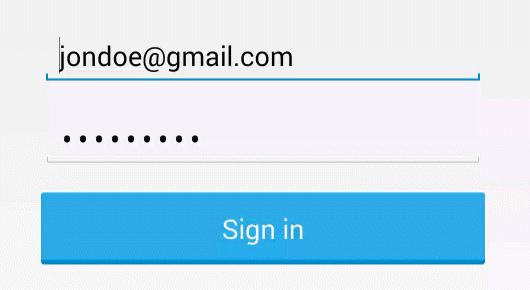
- normal state [0]
- progress state [1-99]
- success state [100]
- error state [-1]
Declare ActionProcessButton
inside your layout
<com.dd.processbutton.iml.ActionProcessButton
android:id="@+id/btnSignIn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="16dp"
android:text="Sign_in"
android:textColor="@android:color/white"
android:textSize="18sp"
custom:pb_colorComplete="@color/green_complete"
custom:pb_colorNormal="@color/blue_normal"
custom:pb_colorPressed="@color/blue_pressed"
custom:pb_colorProgress="@color/purple_progress"
custom:pb_textComplete="Success"
custom:pb_textProgress="Loading"
custom:pb_textError="Error"
custom:pb_colorError="@color/red_error" />
<!--background color which will be displayed when progress is [100]-->
custom:pb_colorComplete
<!--text which will be displayed when progress is [100]-->
custom:pb_textComplete
<!--loading color which will be displayed when progress is [1-99]-->
custom:pb_colorProgress
<!--text which will be displayed when progress is [1-99]-->
custom:pb_textProgress
<!--text which will be displayed when progress is [-1]-->
custom:pb_textError="Error"
<!--background color which will be displayed when progress is [-1]-->
custom:pb_colorComplete
Control it via Java code
ActionProcessButton btnSignIn = (ActionProcessButton) findViewById(R.id.btnSignIn);
btnSignIn.setMode(ActionProcessButton.Mode.PROGRESS);
// no progress
button.setProgress(0);
// progressDrawable cover 50% of button width, progressText is shown
button.setProgress(50);
// progressDrawable cover 75% of button width, progressText is shown
button.setProgress(75);
// completeColor & completeText is shown
button.setProgress(100);
// you can display endless google like progress indicator
btnSignIn.setMode(ActionProcessButton.Mode.ENDLESS);
// set progress > 0 to start progress indicator animation
button.setProgress(1);
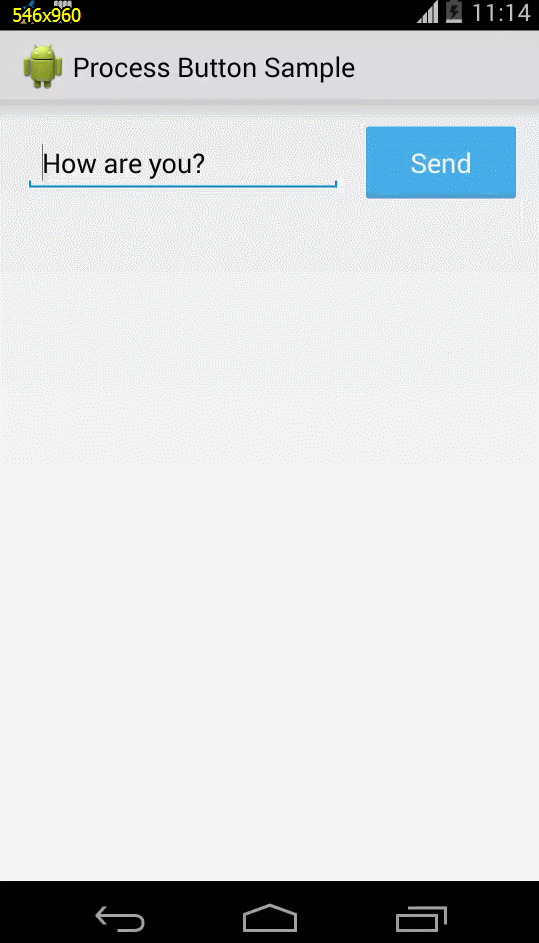
See ActionProcessButton
sample above.
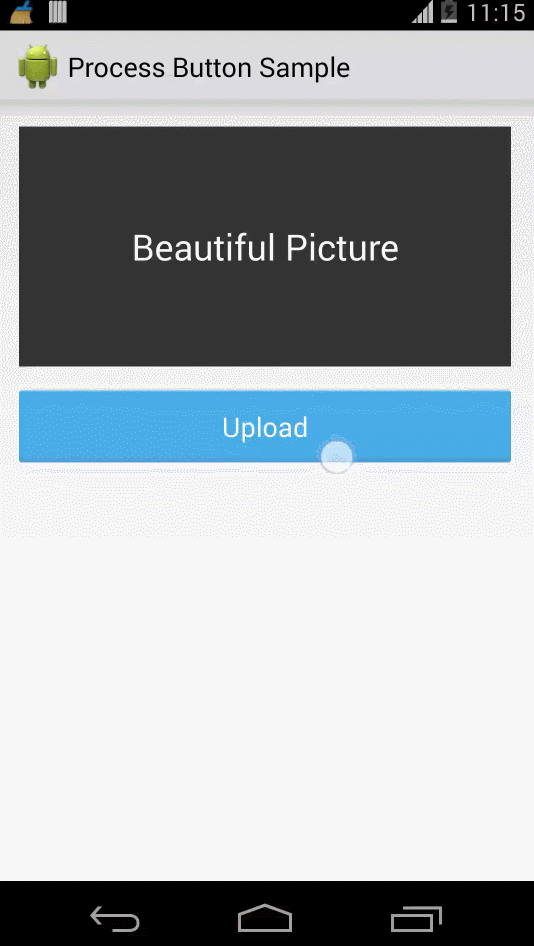
See ActionProcessButton
sample above.