forked from 0xPolygon/polygon-edge
-
Notifications
You must be signed in to change notification settings - Fork 22
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[metrics] remove go-kit dependencies (#282)
# Description Now implementation use [go-kit](https://github.com/go-kit/kit) package help build the metrics interface, but there is a performance cost in the implementation of go-kit, this PR replaces go-kit, and directly uses the prometheus metrics interface exist cost code: [prometheus.go#L159](https://github.com/go-kit/kit/blob/e923d5df8514423885b3a6d25cd44ae1d1db6d9d/metrics/prometheus/prometheus.go#L159) `makeLabels` will be called every time the metrics is updated ``` func makeLabels(labelValues ...string) prometheus.Labels { labels := prometheus.Labels{} for i := 0; i < len(labelValues); i += 2 { labels[labelValues[i]] = labelValues[i+1] } return labels } ``` heap pprof results: 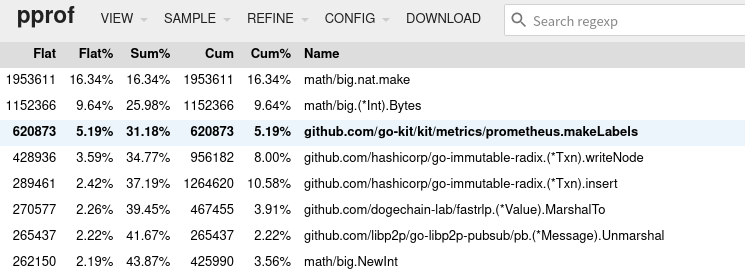 # Changes include - [x] Bugfix (non-breaking change that solves an issue) ## Testing - [x] I have tested this code with the official test suite
- Loading branch information
Showing
19 changed files
with
586 additions
and
544 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,68 +1,75 @@ | ||
package consensus | ||
|
||
import ( | ||
"github.com/go-kit/kit/metrics" | ||
"github.com/go-kit/kit/metrics/discard" | ||
prometheus "github.com/go-kit/kit/metrics/prometheus" | ||
stdprometheus "github.com/prometheus/client_golang/prometheus" | ||
"github.com/dogechain-lab/dogechain/helper/metrics" | ||
"github.com/prometheus/client_golang/prometheus" | ||
) | ||
|
||
// Metrics represents the consensus metrics | ||
type Metrics struct { | ||
// No.of validators | ||
Validators metrics.Gauge | ||
validators prometheus.Gauge | ||
// No.of rounds | ||
Rounds metrics.Gauge | ||
rounds prometheus.Gauge | ||
// No.of transactions in the block | ||
NumTxs metrics.Gauge | ||
|
||
numTxs prometheus.Gauge | ||
//Time between current block and the previous block in seconds | ||
BlockInterval metrics.Gauge | ||
blockInterval prometheus.Gauge | ||
} | ||
|
||
func (m *Metrics) SetValidators(val float64) { | ||
metrics.SetGauge(m.validators, val) | ||
} | ||
|
||
func (m *Metrics) SetRounds(val float64) { | ||
metrics.SetGauge(m.rounds, val) | ||
} | ||
|
||
func (m *Metrics) SetNumTxs(val float64) { | ||
metrics.SetGauge(m.numTxs, val) | ||
} | ||
|
||
func (m *Metrics) SetBlockInterval(val float64) { | ||
metrics.SetGauge(m.blockInterval, val) | ||
} | ||
|
||
// GetPrometheusMetrics return the consensus metrics instance | ||
func GetPrometheusMetrics(namespace string, labelsWithValues ...string) *Metrics { | ||
labels := []string{} | ||
|
||
for i := 0; i < len(labelsWithValues); i += 2 { | ||
labels = append(labels, labelsWithValues[i]) | ||
} | ||
constLabels := metrics.ParseLables(labelsWithValues...) | ||
|
||
return &Metrics{ | ||
Validators: prometheus.NewGaugeFrom(stdprometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "validators", | ||
Help: "Number of validators.", | ||
}, labels).With(labelsWithValues...), | ||
Rounds: prometheus.NewGaugeFrom(stdprometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "rounds", | ||
Help: "Number of rounds.", | ||
}, labels).With(labelsWithValues...), | ||
NumTxs: prometheus.NewGaugeFrom(stdprometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "num_txs", | ||
Help: "Number of transactions.", | ||
}, labels).With(labelsWithValues...), | ||
|
||
BlockInterval: prometheus.NewGaugeFrom(stdprometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "block_interval", | ||
Help: "Time between current block and the previous block in seconds.", | ||
}, labels).With(labelsWithValues...), | ||
validators: prometheus.NewGauge(prometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "validators", | ||
Help: "Number of validators.", | ||
ConstLabels: constLabels, | ||
}), | ||
rounds: prometheus.NewGauge(prometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "rounds", | ||
Help: "Number of rounds.", | ||
ConstLabels: constLabels, | ||
}), | ||
numTxs: prometheus.NewGauge(prometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "num_txs", | ||
Help: "Number of transactions.", | ||
ConstLabels: constLabels, | ||
}), | ||
blockInterval: prometheus.NewGauge(prometheus.GaugeOpts{ | ||
Namespace: namespace, | ||
Subsystem: "consensus", | ||
Name: "block_interval", | ||
Help: "Time between current block and the previous block in seconds.", | ||
ConstLabels: constLabels, | ||
}), | ||
} | ||
} | ||
|
||
// NilMetrics will return the non operational metrics | ||
func NilMetrics() *Metrics { | ||
return &Metrics{ | ||
Validators: discard.NewGauge(), | ||
Rounds: discard.NewGauge(), | ||
NumTxs: discard.NewGauge(), | ||
BlockInterval: discard.NewGauge(), | ||
} | ||
return &Metrics{} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.