-
Notifications
You must be signed in to change notification settings - Fork 28
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat(deployment): support Heroku (#38)
* add PORT to start script * upgrade cypress to allow deploy * move universal-cookie to devDependencies * write cacheDirectories in package.json for heroku * add inquirer, prompt for host * add to ci * deploy from GH actions * fix graphql handler * add heroku specific items to CI * updates to support heroku * heroku : dont create apps if user says no * put back commented out things * interpolate prisma env
- Loading branch information
Showing
22 changed files
with
963 additions
and
200 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1 @@ | ||
*ejs* |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
# Set up CI | ||
|
||
This project uses GitHub Actions for CI and should work out of the box. After you've successfully deployed your app, you need to do is set a few ENV vars. Like most CI environments, as you add ENV vars to your app you'll want to also add them in GitHub Secrets. | ||
|
||
## Configure Vercel Deployments | ||
|
||
To deploy to Vercel, you need to set `VERCEL_ORG_ID`, `VERCEL_PROJECT_ID` and `VERCEL_TOKEN` ENV vars. | ||
|
||
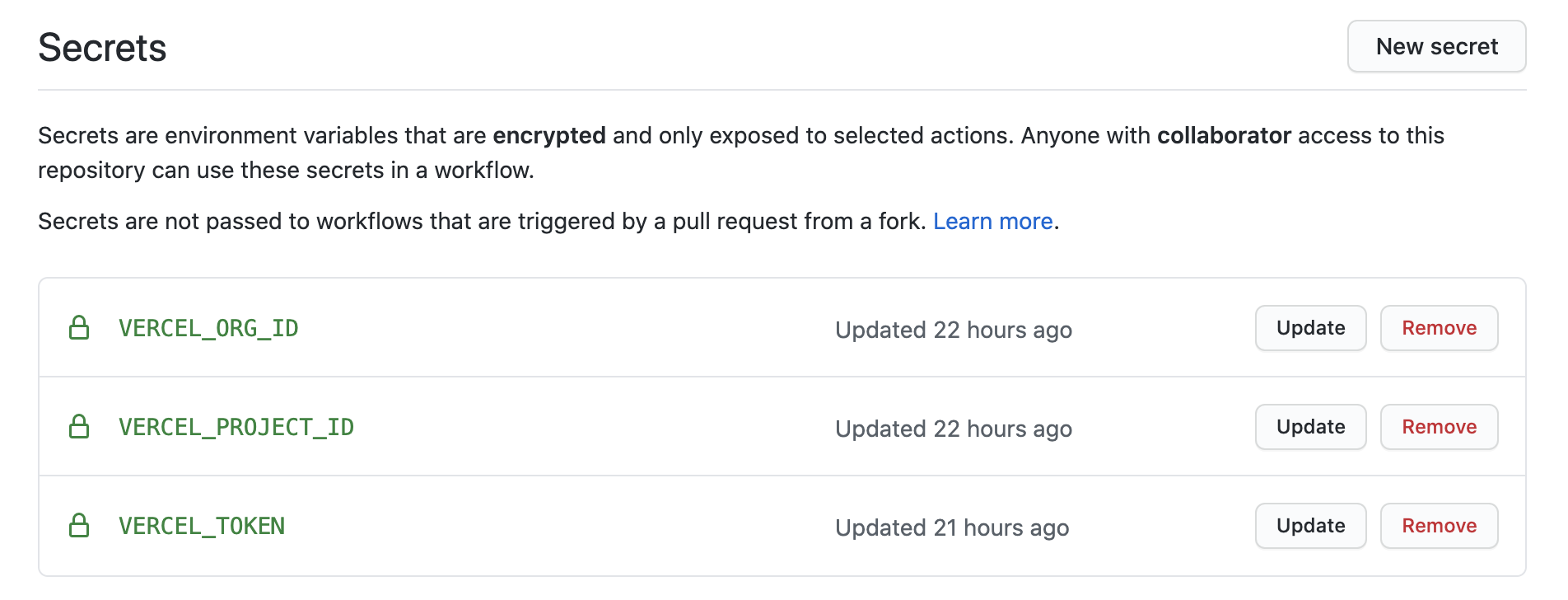 | ||
|
||
The Vercel project and org id, can be copied from `.vercel/project.json`. You can generate a token from https://vercel.com/account/tokens. | ||
|
||
### CI Workflow | ||
|
||
After tests pass, the app will deploy. Every push will create a preview deployment. Merging to the main branch will deploy to staging, and pushing to the production branch will deploy to production. | ||
|
||
If you'd like to change these configurations to a more typical JAMstack flow (where merging to the main branch deploys to production), update the section below in `..github/workflows/main.js.yml`: | ||
|
||
``` | ||
## For a typical JAMstack flow, this should be your default branch. | ||
## For a traditional flow that auto-deploys staging and deploys prod is as needed, keep as is | ||
if: github.ref != 'refs/heads/production' # every branch EXCEPT production | ||
``` | ||
|
||
## Configure Heroku Deployments | ||
|
||
Heroku needs to login as a user in order to create apps and deploy code. While you can do this using your personal Heroku account, we highly recommend creating a "machine user" for better security (for example, ci@echobind.com). The machine user only has access to the required repositories instead of every repository your user account has access to. | ||
|
||
Heroku requires an email and an API Token in order to log in. To get an API token visit your [account settings](https://dashboard.heroku.com/account). | ||
|
||
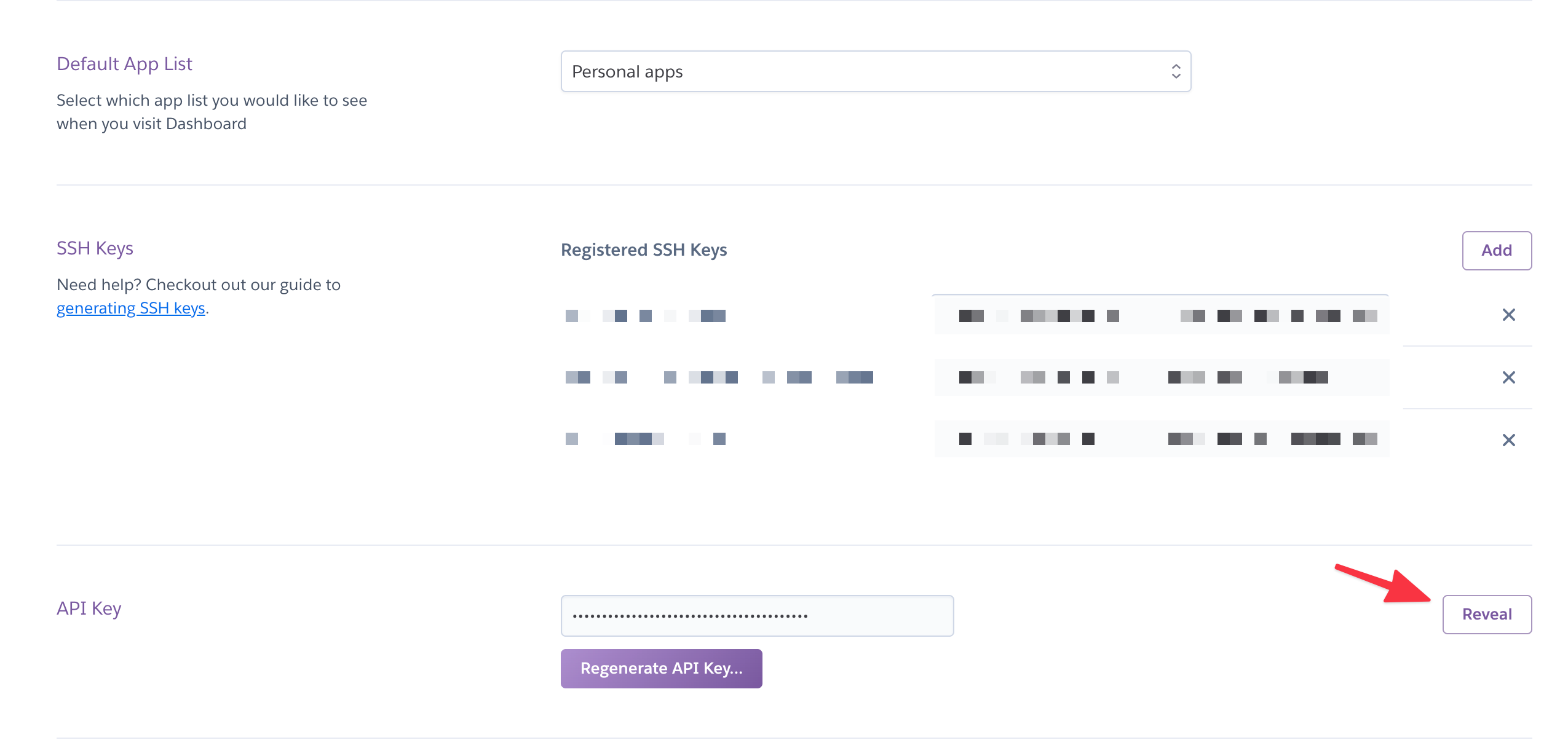 | ||
|
||
Next, add `HEROKU_API_TOKEN` and `HEROKU_EMAIL` to GitHub Secrets: | ||
|
||
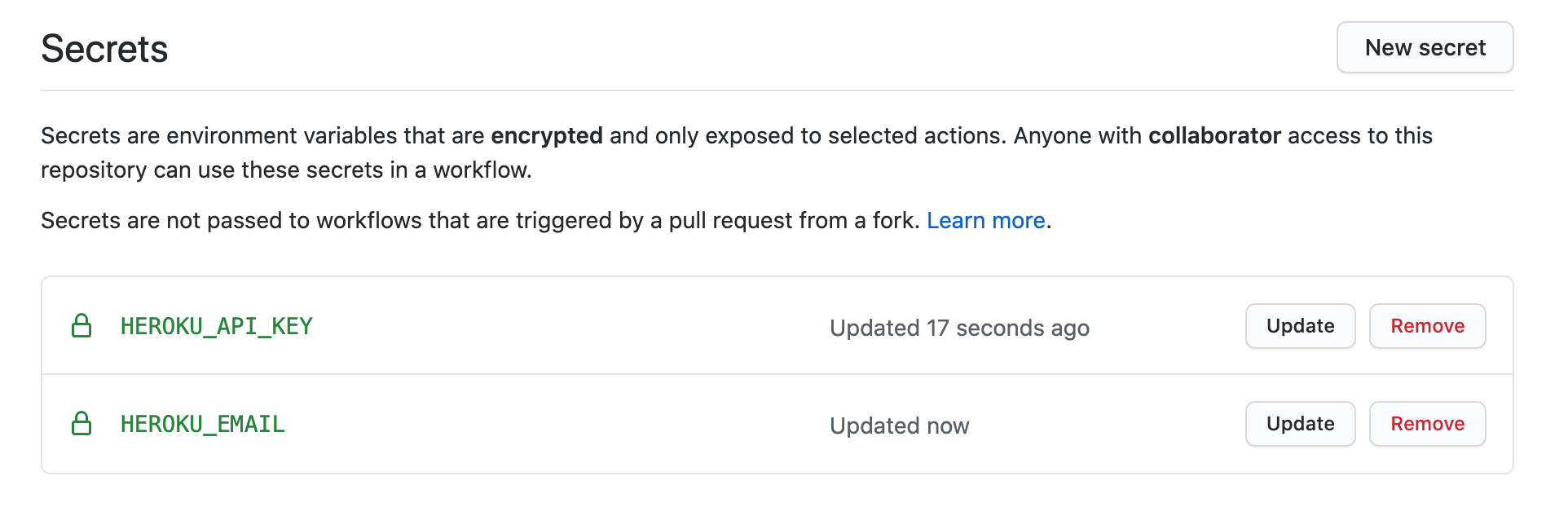 | ||
|
||
After tests pass, the app will deploy. Every push will create a preview deployment. Merging to the main branch will deploy to staging, and pushing to the production branch will deploy to production. | ||
|
||
## You're done! | ||
|
||
Easiest CI configuration ever, right? |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
# Preview / Staging and Production Deployment Setup | ||
|
||
## Deploying to Vercel | ||
|
||
- Create a new Vercel app by running `vercel`. | ||
- Create a staging and production database on Heroku, Digital Ocean, or AWS. For production, you likely want to enable some form of connection pooling so that you don't exhaust database connections. | ||
- Add the appropriate values for the `APP_SECRET` and `DATABASE_URL` ENV vars to the app settings page (https://vercel.com/<org>/<app>/settings/environment-variables). Use the staging URL for preview and the production URL for production. | ||
|
||
Verify things work by running `vercel` again. | ||
|
||
## Deploying to Heroku | ||
|
||
Heroku is not typically used to host Jamstack apps. If possible, you should leverage Vercel or Netlify (coming soon!) as they have some advantages in doing so. That said, we wanted to ensure Bison apps could still be deployed to Heroku if required. Especially since many choose to use Heroku to host their database. | ||
|
||
- Create a new staging app: `heroku apps:create myapp-staging --remote staging` | ||
- Add a value for APP_SECRET. `heroku config:add APP_SECRET=mysecret --remote staging` | ||
- Add a database: `heroku addons:create heroku-postgresql:hobby-dev --remote staging` | ||
- Create a new production app: `heroku apps:create myapp --remote production` | ||
- Add a value for APP_SECRET. `heroku config:add APP_SECRET=mysecret --remote production` | ||
- Add a database: `heroku addons:create heroku-postgresql:standard-0 --remote production`. If you're just testing things out, hobby-dev is sufficient. For production apps, you'll want to use the Standard Tier. | ||
|
||
Verify things work by running `git push staging` and `git push production`. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,65 @@ | ||
# Recommended Dev Workflow | ||
|
||
You're not required to follow this exact workflow, but we've found it gives a good developer experience. | ||
|
||
We start from the API and then create the frontend. The reason for this is that Bison will generate types for your GraphQL operations which you will leverage in your components on the frontend. | ||
|
||
## API | ||
|
||
1. Generate a new GraphQL module using `yarn g:graphql`. | ||
1. Write a type, query, input, or mutation using [Nexus](https://nexusjs.org/guides/schema) | ||
1. Create a new request test using `yarn g:test:request` | ||
1. Run `yarn test` to start the test watcher | ||
1. Add tests cases and update schema code accordingly. The GraphQL playground (localhost:3000/api/graphql) can be helpful to form the proper queries to use in tests. | ||
1. `types.ts` and `api.graphql` should update automatically as you change files. Sometimes it's helpful to open these as a sanity check before moving on to the frontend code. | ||
|
||
## Frontend | ||
|
||
1. Generate a new page using `yarn g:page` | ||
1. Generate a new component using `yarn g:component` | ||
1. If you need to fetch data in your component, use a cell. Generate one using `yarn g:cell` | ||
1. To generate a typed GraphQL query, simply add it to the component or page: | ||
|
||
```ts | ||
export const SIGNUP_MUTATION = gql` | ||
mutation signup($data: SignupInput!) { | ||
signup(data: $data) { | ||
token | ||
user { | ||
id | ||
} | ||
} | ||
} | ||
`; | ||
``` | ||
|
||
5. Use the newly generated hooks from Codegen instead of the typical `useQuery` or `useMutation` hook. For the example above, that would be `useSignupMutation`. You'll now have a fully typed response to work with! | ||
|
||
```tsx | ||
import { User, useMeQuery } from './types'; | ||
|
||
// adding this will auto-generate a custom hook in ./types. | ||
export const ME_QUERY = gql` | ||
query me { | ||
me { | ||
id | ||
} | ||
} | ||
`; | ||
|
||
// an example of taking a user as an argument with optional attributes | ||
function noIdea(user: Partial<User>) { | ||
console.log(user.email); | ||
} | ||
|
||
function fakeCell() { | ||
// use the generated hook | ||
const { data, loading, error } = useMeQuery(); | ||
|
||
if (loading) return <Loading />; | ||
|
||
// data.user will be fully typed | ||
return <Success user={data.user}> | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
# FAQ | ||
|
||
## Where are the generated types? | ||
|
||
TypeScript Types for GraphQL types, queries, and mutations are generated automatically and placed in `./types.ts`. | ||
|
||
## VSCode can't find new types, even though they are in ./types.ts | ||
|
||
Try reopening VSCode. |
Oops, something went wrong.