-
Notifications
You must be signed in to change notification settings - Fork 24.2k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Added filtering to RNTester example screens (#22777)
Summary: This PR adds filtering functionality to individual example screens of RNTester. This is useful for Detox testing of RNTester, since Detox requires elements to be visible on the screen before they can be interacted with. Instead of needing to scroll an arbitrary amount, the test can enter the name of the example to be tested, just as is done on the main screen. This will lead to simpler and more reliable E2E tests for long example screens. This PR doesn't add any automated tests using the filter; those will be added in a separate PR. This is implemented by extracting the existing filtering functionality out of `RNTesterExampleList` into a shared `RNTesterExampleFilter` component that can be used both within `RNTesterExampleList` (the main screen) and `RNTesterExampleContainer` (the example screen). 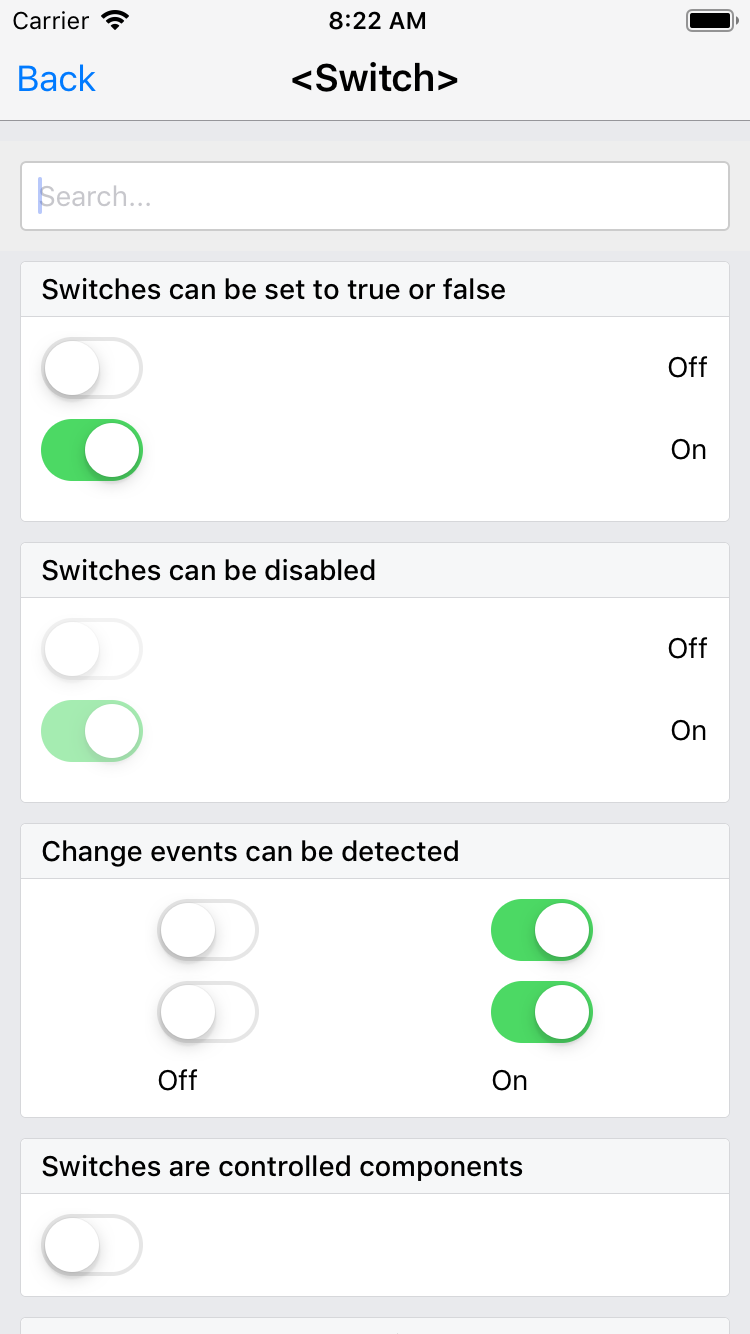 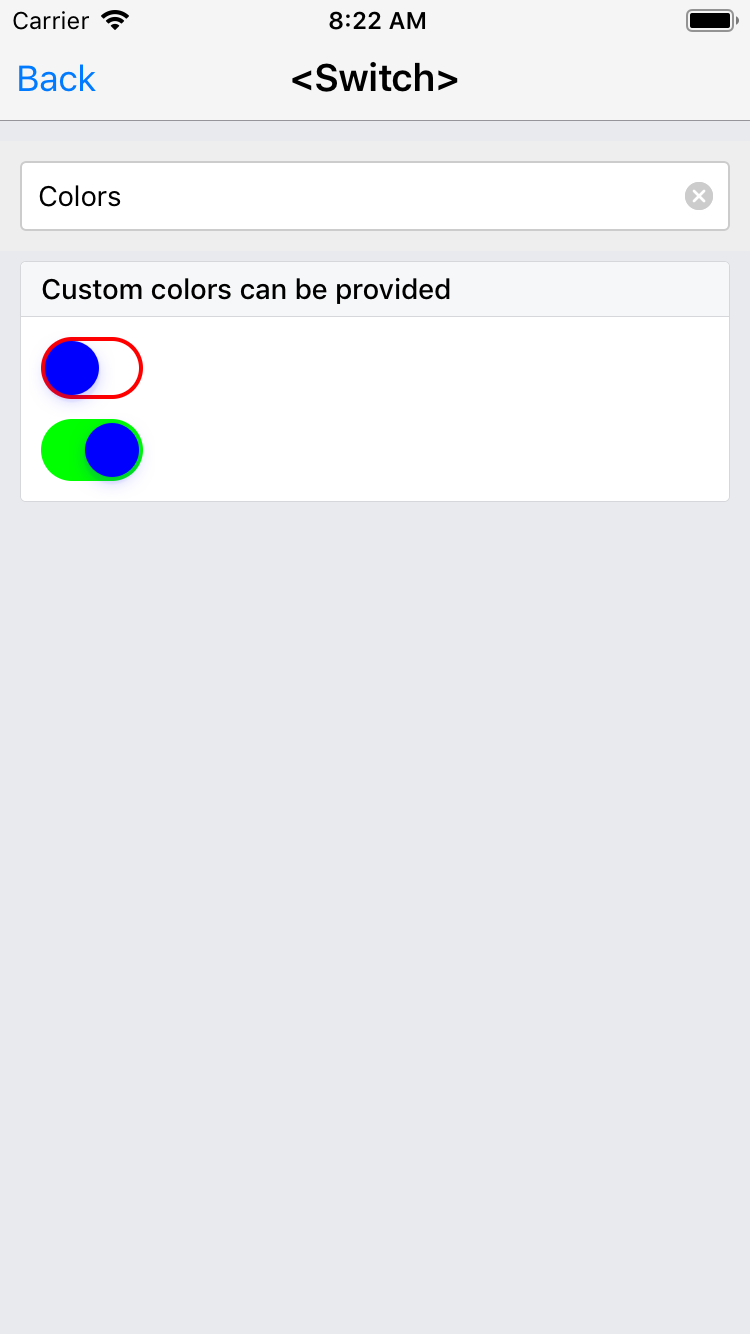 Changelog: ---------- [General] [Added] - Added filtering to RNTester example screens Pull Request resolved: #22777 Reviewed By: TheSavior Differential Revision: D13561744 Pulled By: rickhanlonii fbshipit-source-id: cb120626a8e2b8440f88b871557c0b92fbef5edc
- Loading branch information
1 parent
68f6325
commit bd79303
Showing
3 changed files
with
155 additions
and
77 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,102 @@ | ||
/** | ||
* Copyright (c) Facebook, Inc. and its affiliates. | ||
* | ||
* This source code is licensed under the MIT license found in the | ||
* LICENSE file in the root directory of this source tree. | ||
* | ||
* @format | ||
* @flow | ||
*/ | ||
|
||
'use strict'; | ||
|
||
const React = require('react'); | ||
const StyleSheet = require('StyleSheet'); | ||
const TextInput = require('TextInput'); | ||
const View = require('View'); | ||
|
||
type Props = { | ||
filter: Function, | ||
render: Function, | ||
sections: Object, | ||
disableSearch?: boolean, | ||
}; | ||
|
||
type State = { | ||
filter: string, | ||
}; | ||
|
||
class RNTesterExampleFilter extends React.Component<Props, State> { | ||
state = {filter: ''}; | ||
|
||
render() { | ||
const filterText = this.state.filter; | ||
let filterRegex = /.*/; | ||
|
||
try { | ||
filterRegex = new RegExp(String(filterText), 'i'); | ||
} catch (error) { | ||
console.warn( | ||
'Failed to create RegExp: %s\n%s', | ||
filterText, | ||
error.message, | ||
); | ||
} | ||
|
||
const filter = example => | ||
this.props.disableSearch || this.props.filter({example, filterRegex}); | ||
|
||
const filteredSections = this.props.sections.map(section => ({ | ||
...section, | ||
data: section.data.filter(filter), | ||
})); | ||
|
||
return ( | ||
<View> | ||
{this._renderTextInput()} | ||
{this.props.render({filteredSections})} | ||
</View> | ||
); | ||
} | ||
|
||
_renderTextInput(): ?React.Element<any> { | ||
if (this.props.disableSearch) { | ||
return null; | ||
} | ||
return ( | ||
<View style={styles.searchRow}> | ||
<TextInput | ||
autoCapitalize="none" | ||
autoCorrect={false} | ||
clearButtonMode="always" | ||
onChangeText={text => { | ||
this.setState(() => ({filter: text})); | ||
}} | ||
placeholder="Search..." | ||
underlineColorAndroid="transparent" | ||
style={styles.searchTextInput} | ||
testID="explorer_search" | ||
value={this.state.filter} | ||
/> | ||
</View> | ||
); | ||
} | ||
} | ||
|
||
const styles = StyleSheet.create({ | ||
searchRow: { | ||
backgroundColor: '#eeeeee', | ||
padding: 10, | ||
}, | ||
searchTextInput: { | ||
backgroundColor: 'white', | ||
borderColor: '#cccccc', | ||
borderRadius: 3, | ||
borderWidth: 1, | ||
paddingLeft: 8, | ||
paddingVertical: 0, | ||
height: 35, | ||
}, | ||
}); | ||
|
||
module.exports = RNTesterExampleFilter; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters