-
Notifications
You must be signed in to change notification settings - Fork 37
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[glTF] Load scenes with UINT8 indices (#482)
Load scenes that contain 8-bit index buffers. How the index buffer ends up being packed depends on the capabilities of the device: - On Vulkan with `VK_KHR_index_type_uint8` extension, the UINT8 indices can be packed into a `INDEX_TYPE_UINT8` buffer. This is the optimal case as it doesn't require additional memory. - Otherwise, the UINT8 indices need to be repacked into UINT16 indices. This comes at the cost of a larger GPU buffer. This allows us to load more Khronos [glTF-Sample-Assets](https://github.com/KhronosGroup/glTF-Sample-Assets) like [TextureCoordinateTest](https://github.com/KhronosGroup/glTF-Sample-Assets/tree/main/Models/TextureCoordinateTest). 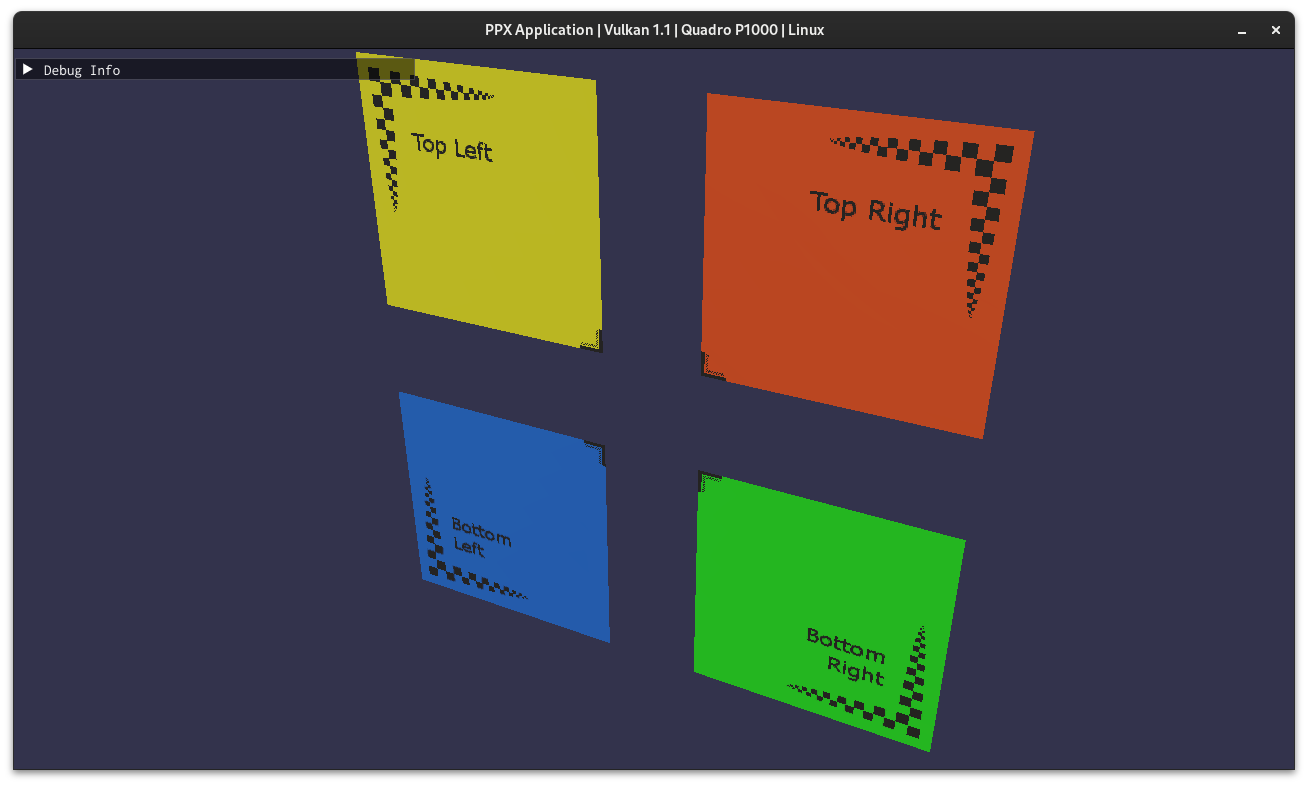 Fixes #453
- Loading branch information
1 parent
e50fbc8
commit 5aa9f65
Showing
1 changed file
with
139 additions
and
52 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters