-
Notifications
You must be signed in to change notification settings - Fork 7
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Abstract Method NullSafeSet SessionImplementor S3Blob Issue #4
Comments
This is because by default the Heroku buildpack uses Play 1.2 and it looks like this module isn't compatible with Play 1.2. I've tested it with Play 1.4 and JDK 8. To tell heroku to use those versions update your
And create a
When you push to Heroku you should see the build pulling in JDK 1.8 and Play 1.4.5. |
Thanks a bunch James for taking the time to try and figure this out. I
have some interesting results to report.
First, when I require play 1.4.5 in my dependency, the Heroku build fails.
And I've attached a screenshot of that stack trace but it looks like it's
saying 1.4.5 is an unsupported major/minor version.
Interstingly though, when I require version 1.2.5.6, we pass the Heroku
build, and my app opens. When I click the Auctions link, fill out the info
and upload a picture, the code gets passed where it was stuck before, it
sends the photo to AWS, when the successful response returns, it saves all
null values for the item except for the photo, it actually saves the photo
in my database too, which is what I expected.
But I'm not sure why it's saving null values and then crashing on line
I created a system.properties file, placed it in my conf directory and put
that line --- java.runtime.version=1.8 in the file. But I'm still seeing
OpenJDK 1.6 being used in the build. I've pushed the last files I used to
Git so if you want to try and replicate this exactly you can.
I've also created login credentials if you want to visit my app and see the
behavior yourself. You can go to http://java-play-app.herokuapp.com/ and
login with these credentials: Username: ******
Password: *****
After logging in you can click the auction link and create an auction item
and send it. You can open up HeidiSQL and use the credentials you find in
app.conf to look into the database and see the null values and the photo
being stored in the database. I'm going to re-create this application using
C# and .NET core soon, but I really want to put that last bit of icing on
this cake.
I'd appreciate it.
Thanks, CM PS: The app is currently running using 1.2.5.6, since 1.4.5
didn't even get through the Heroku build.
|
Sorry for not being more clear, the |
BTW, I've updated this project to work on Heroku with Play |
Will do.
I'm still new (relative to you likely) to this, so I should have known
where the system.properties file goes.
But thanks for letting me know.
I'll report back what I see happening.
C
…On Mon, Nov 13, 2017 at 9:22 AM, James Ward ***@***.***> wrote:
Sorry for not being more clear, the system.properties file has to be in
the root. Can you try that with 1.4.5 and see how it goes?
—
You are receiving this because you authored the thread.
Reply to this email directly, view it on GitHub
<#4 (comment)>,
or mute the thread
<https://github.com/notifications/unsubscribe-auth/AVggHRCFYIGbV4R-iYIVXt1yEfwzejRnks5s2FCfgaJpZM4QXUGm>
.
|
I'm not sure what you mean.
…On Mon, Nov 13, 2017 at 9:23 AM, James Ward ***@***.***> wrote:
BTW, I've updated this project to work on Heroku with Play 1.4.5 and JDK
8 so you can reference this repo for layout and config.
—
You are receiving this because you authored the thread.
Reply to this email directly, view it on GitHub
<#4 (comment)>,
or mute the thread
<https://github.com/notifications/unsubscribe-auth/AVggHf-AIqqIaWoZ74F2hf0V9Qe6LhBZks5s2FDbgaJpZM4QXUGm>
.
|
This repo is a sample app for the s3blobs module. I've tested that this repo works as expected on Heroku. |
I moved system.properties to the root of the project directory. I changed
the version to 1.4.5
Looks like we're back to it getting stuck on line 56 of Application.java
where the method attempts to save the info to the database.
CM
…On Mon, Nov 13, 2017 at 9:36 AM, James Ward ***@***.***> wrote:
This repo is a sample app for the s3blobs module. I've tested that this
repo works as expected on Heroku.
—
You are receiving this because you authored the thread.
Reply to this email directly, view it on GitHub
<#4 (comment)>,
or mute the thread
<https://github.com/notifications/unsubscribe-auth/AVggHSRQoKp1Vo_VRU_iKsy54T5ZFDWEks5s2FP9gaJpZM4QXUGm>
.
|
And by the way, I saw that the Heroku build did use JDK 1.8 and did use
Play 1.4.5
On Mon, Nov 13, 2017 at 9:39 AM, Chris Mazzochi <cmazzochi81@gmail.com>
wrote:
… I moved system.properties to the root of the project directory. I changed
the version to 1.4.5
Looks like we're back to it getting stuck on line 56 of Application.java
where the method attempts to save the info to the database.
CM
On Mon, Nov 13, 2017 at 9:36 AM, James Ward ***@***.***>
wrote:
> This repo is a sample app for the s3blobs module. I've tested that this
> repo works as expected on Heroku.
>
> —
> You are receiving this because you authored the thread.
> Reply to this email directly, view it on GitHub
> <#4 (comment)>,
> or mute the thread
> <https://github.com/notifications/unsubscribe-auth/AVggHSRQoKp1Vo_VRU_iKsy54T5ZFDWEks5s2FP9gaJpZM4QXUGm>
> .
>
|
Ah, well at least we now know what versions are being used on Heroku. :) There was a Pull Request a while back on the s3blobs project that might be related to this: Think that might be related? If so, I can make that change (it was never merged) and release a new version. |
We are in territory now where I think you are better equipped to answer
that question regarding the pull request you refer to.
I'll have to understand that one a little more first and see but I think
you are better suited to know for sure at this point whether or not that
pull request is related.
Thanks,
C
…On Mon, Nov 13, 2017 at 9:43 AM, James Ward ***@***.***> wrote:
Ah, well at least we now know what versions are being used on Heroku. :)
There was a Pull Request a while back on the s3blobs project that might be
related to this:
jamesward/S3-Blobs-module-for-Play#9
<jamesward/S3-Blobs-module-for-Play#9>
Think that might be related? If so, I can make that change (it was never
merged) and release a new version.
—
You are receiving this because you authored the thread.
Reply to this email directly, view it on GitHub
<#4 (comment)>,
or mute the thread
<https://github.com/notifications/unsubscribe-auth/AVggHem_ywvzgF0nH-7nMM0bxfsg16y4ks5s2FWjgaJpZM4QXUGm>
.
|
I really have no idea what the problem is so I'm totally taking random shots in the dark. :) Next step is to make sure you are using the s3blobs Play module like in https://github.com/jamesward/plays3upload/blob/master/conf/dependencies.yml You don't need the Also, I'm curious what the value of |
This error is occurring when my application is attempting to save information about a photo into a database. Just before it does that it sends the photo to Amazon S3. And that is a successful operation. But when it tries to save the information about the photo into my database, the app crashes.
Can anybody see what I need to do in order to get through this error? I'd appreciate it. Thanks, CM
Here's the stack trace:
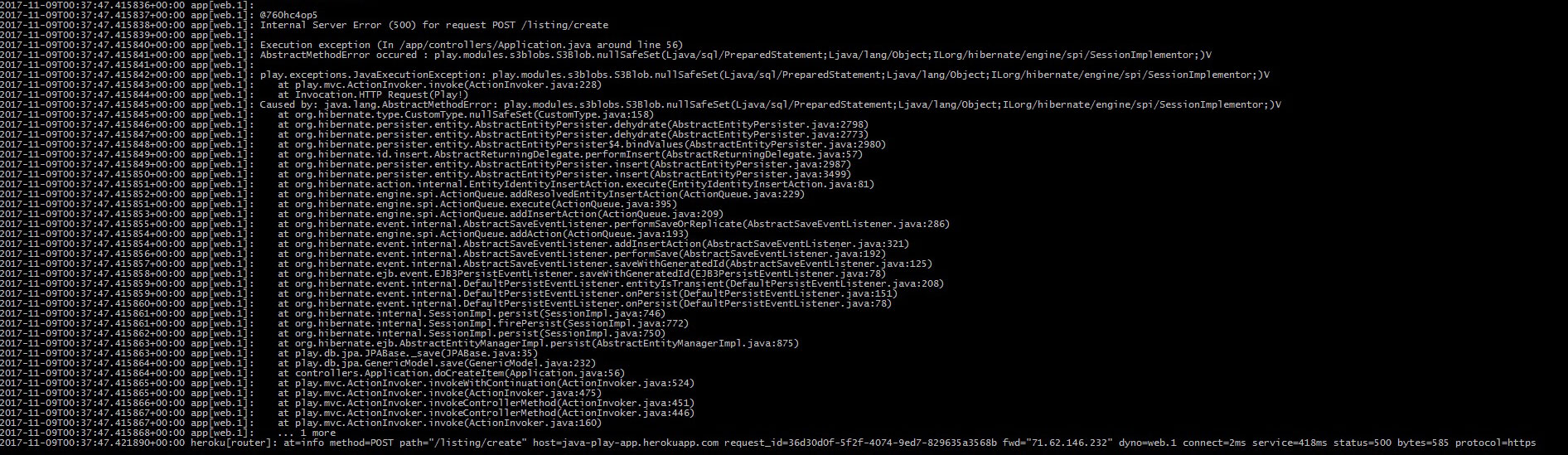
It boils down to these two methods:
Here's that entire class:
Application.java around line 56
The text was updated successfully, but these errors were encountered: