-
Notifications
You must be signed in to change notification settings - Fork 72
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
36 changed files
with
1,462 additions
and
0 deletions.
There are no files selected for viewing
20 changes: 20 additions & 0 deletions
20
src/main/java/g3301_3400/s3360_stone_removal_game/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,20 @@ | ||
package g3301_3400.s3360_stone_removal_game; | ||
|
||
// #Easy #Math #Simulation #2024_12_03_Time_0_ms_(100.00%)_Space_40.3_MB_(80.86%) | ||
|
||
public class Solution { | ||
public boolean canAliceWin(int n) { | ||
if (n < 10) { | ||
return false; | ||
} | ||
int stonesRemaining = n - 10; | ||
int stonesToBeRemoved = 9; | ||
int i = 1; | ||
while (stonesRemaining >= stonesToBeRemoved) { | ||
stonesRemaining -= stonesToBeRemoved; | ||
i++; | ||
stonesToBeRemoved--; | ||
} | ||
return i % 2 != 0; | ||
} | ||
} |
37 changes: 37 additions & 0 deletions
37
src/main/java/g3301_3400/s3360_stone_removal_game/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
3360\. Stone Removal Game | ||
|
||
Easy | ||
|
||
Alice and Bob are playing a game where they take turns removing stones from a pile, with _Alice going first_. | ||
|
||
* Alice starts by removing **exactly** 10 stones on her first turn. | ||
* For each subsequent turn, each player removes **exactly** 1 fewer stone than the previous opponent. | ||
|
||
The player who cannot make a move loses the game. | ||
|
||
Given a positive integer `n`, return `true` if Alice wins the game and `false` otherwise. | ||
|
||
**Example 1:** | ||
|
||
**Input:** n = 12 | ||
|
||
**Output:** true | ||
|
||
**Explanation:** | ||
|
||
* Alice removes 10 stones on her first turn, leaving 2 stones for Bob. | ||
* Bob cannot remove 9 stones, so Alice wins. | ||
|
||
**Example 2:** | ||
|
||
**Input:** n = 1 | ||
|
||
**Output:** false | ||
|
||
**Explanation:** | ||
|
||
* Alice cannot remove 10 stones, so Alice loses. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= n <= 50` |
40 changes: 40 additions & 0 deletions
40
src/main/java/g3301_3400/s3361_shift_distance_between_two_strings/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
package g3301_3400.s3361_shift_distance_between_two_strings; | ||
|
||
// #Medium #Array #String #Prefix_Sum #2024_12_03_Time_9_ms_(100.00%)_Space_45.8_MB_(36.02%) | ||
|
||
public class Solution { | ||
public long shiftDistance(String s, String t, int[] nextCost, int[] previousCost) { | ||
long[][] costs = new long[26][26]; | ||
long cost; | ||
for (int i = 0; i < 26; i++) { | ||
cost = nextCost[i]; | ||
int j = i == 25 ? 0 : i + 1; | ||
while (j != i) { | ||
costs[i][j] = cost; | ||
cost += nextCost[j]; | ||
if (j == 25) { | ||
j = -1; | ||
} | ||
j++; | ||
} | ||
} | ||
for (int i = 0; i < 26; i++) { | ||
cost = previousCost[i]; | ||
int j = i == 0 ? 25 : i - 1; | ||
while (j != i) { | ||
costs[i][j] = Math.min(costs[i][j], cost); | ||
cost += previousCost[j]; | ||
if (j == 0) { | ||
j = 26; | ||
} | ||
j--; | ||
} | ||
} | ||
int n = s.length(); | ||
long ans = 0; | ||
for (int i = 0; i < n; i++) { | ||
ans += costs[s.charAt(i) - 'a'][t.charAt(i) - 'a']; | ||
} | ||
return ans; | ||
} | ||
} |
47 changes: 47 additions & 0 deletions
47
src/main/java/g3301_3400/s3361_shift_distance_between_two_strings/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
3361\. Shift Distance Between Two Strings | ||
|
||
Medium | ||
|
||
You are given two strings `s` and `t` of the same length, and two integer arrays `nextCost` and `previousCost`. | ||
|
||
In one operation, you can pick any index `i` of `s`, and perform **either one** of the following actions: | ||
|
||
* Shift `s[i]` to the next letter in the alphabet. If `s[i] == 'z'`, you should replace it with `'a'`. This operation costs `nextCost[j]` where `j` is the index of `s[i]` in the alphabet. | ||
* Shift `s[i]` to the previous letter in the alphabet. If `s[i] == 'a'`, you should replace it with `'z'`. This operation costs `previousCost[j]` where `j` is the index of `s[i]` in the alphabet. | ||
|
||
The **shift distance** is the **minimum** total cost of operations required to transform `s` into `t`. | ||
|
||
Return the **shift distance** from `s` to `t`. | ||
|
||
**Example 1:** | ||
|
||
**Input:** s = "abab", t = "baba", nextCost = [100,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0], previousCost = [1,100,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** | ||
|
||
* We choose index `i = 0` and shift `s[0]` 25 times to the previous character for a total cost of 1. | ||
* We choose index `i = 1` and shift `s[1]` 25 times to the next character for a total cost of 0. | ||
* We choose index `i = 2` and shift `s[2]` 25 times to the previous character for a total cost of 1. | ||
* We choose index `i = 3` and shift `s[3]` 25 times to the next character for a total cost of 0. | ||
|
||
**Example 2:** | ||
|
||
**Input:** s = "leet", t = "code", nextCost = [1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1], previousCost = [1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1] | ||
|
||
**Output:** 31 | ||
|
||
**Explanation:** | ||
|
||
* We choose index `i = 0` and shift `s[0]` 9 times to the previous character for a total cost of 9. | ||
* We choose index `i = 1` and shift `s[1]` 10 times to the next character for a total cost of 10. | ||
* We choose index `i = 2` and shift `s[2]` 1 time to the previous character for a total cost of 1. | ||
* We choose index `i = 3` and shift `s[3]` 11 times to the next character for a total cost of 11. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= s.length == t.length <= 10<sup>5</sup></code> | ||
* `s` and `t` consist only of lowercase English letters. | ||
* `nextCost.length == previousCost.length == 26` | ||
* <code>0 <= nextCost[i], previousCost[i] <= 10<sup>9</sup></code> |
32 changes: 32 additions & 0 deletions
32
src/main/java/g3301_3400/s3362_zero_array_transformation_iii/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
package g3301_3400.s3362_zero_array_transformation_iii; | ||
|
||
// #Medium #Array #Sorting #Greedy #Heap_Priority_Queue #Prefix_Sum | ||
// #2024_12_03_Time_68_ms_(91.99%)_Space_93.6_MB_(45.88%) | ||
|
||
import java.util.Arrays; | ||
import java.util.PriorityQueue; | ||
|
||
public class Solution { | ||
public int maxRemoval(int[] nums, int[][] queries) { | ||
Arrays.sort(queries, (a, b) -> a[0] - b[0]); | ||
PriorityQueue<Integer> last = new PriorityQueue<>((a, b) -> b - a); | ||
int[] diffs = new int[nums.length + 1]; | ||
int idx = 0; | ||
int cur = 0; | ||
for (int i = 0; i < nums.length; i++) { | ||
while (idx < queries.length && queries[idx][0] == i) { | ||
last.add(queries[idx][1]); | ||
idx++; | ||
} | ||
cur += diffs[i]; | ||
while (cur < nums[i] && !last.isEmpty() && last.peek() >= i) { | ||
cur++; | ||
diffs[last.poll() + 1]--; | ||
} | ||
if (cur < nums[i]) { | ||
return -1; | ||
} | ||
} | ||
return last.size(); | ||
} | ||
} |
55 changes: 55 additions & 0 deletions
55
src/main/java/g3301_3400/s3362_zero_array_transformation_iii/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,55 @@ | ||
3362\. Zero Array Transformation III | ||
|
||
Medium | ||
|
||
You are given an integer array `nums` of length `n` and a 2D array `queries` where <code>queries[i] = [l<sub>i</sub>, r<sub>i</sub>]</code>. | ||
|
||
Each `queries[i]` represents the following action on `nums`: | ||
|
||
* Decrement the value at each index in the range <code>[l<sub>i</sub>, r<sub>i</sub>]</code> in `nums` by **at most** 1. | ||
* The amount by which the value is decremented can be chosen **independently** for each index. | ||
|
||
A **Zero Array** is an array with all its elements equal to 0. | ||
|
||
Return the **maximum** number of elements that can be removed from `queries`, such that `nums` can still be converted to a **zero array** using the _remaining_ queries. If it is not possible to convert `nums` to a **zero array**, return -1. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [2,0,2], queries = [[0,2],[0,2],[1,1]] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** | ||
|
||
After removing `queries[2]`, `nums` can still be converted to a zero array. | ||
|
||
* Using `queries[0]`, decrement `nums[0]` and `nums[2]` by 1 and `nums[1]` by 0. | ||
* Using `queries[1]`, decrement `nums[0]` and `nums[2]` by 1 and `nums[1]` by 0. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [1,1,1,1], queries = [[1,3],[0,2],[1,3],[1,2]] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** | ||
|
||
We can remove `queries[2]` and `queries[3]`. | ||
|
||
**Example 3:** | ||
|
||
**Input:** nums = [1,2,3,4], queries = [[0,3]] | ||
|
||
**Output:** \-1 | ||
|
||
**Explanation:** | ||
|
||
`nums` cannot be converted to a zero array even after using all the queries. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= nums.length <= 10<sup>5</sup></code> | ||
* <code>0 <= nums[i] <= 10<sup>5</sup></code> | ||
* <code>1 <= queries.length <= 10<sup>5</sup></code> | ||
* `queries[i].length == 2` | ||
* <code>0 <= l<sub>i</sub> <= r<sub>i</sub> < nums.length</code> |
41 changes: 41 additions & 0 deletions
41
src/main/java/g3301_3400/s3363_find_the_maximum_number_of_fruits_collected/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
package g3301_3400.s3363_find_the_maximum_number_of_fruits_collected; | ||
|
||
// #Hard #Array #Dynamic_Programming #Matrix #2024_12_03_Time_12_ms_(91.34%)_Space_142.1_MB_(88.96%) | ||
|
||
public class Solution { | ||
public int maxCollectedFruits(int[][] fruits) { | ||
int n = fruits.length; | ||
// Set inaccessible cells to 0 | ||
for (int i = 0; i < n; ++i) { | ||
for (int j = 0; j < n; ++j) { | ||
if (i < j && j < n - 1 - i) { | ||
fruits[i][j] = 0; | ||
} | ||
if (j < i && i < n - 1 - j) { | ||
fruits[i][j] = 0; | ||
} | ||
} | ||
} | ||
int res = 0; | ||
for (int i = 0; i < n; ++i) { | ||
res += fruits[i][i]; | ||
} | ||
for (int i = 1; i < n; ++i) { | ||
for (int j = i + 1; j < n; ++j) { | ||
fruits[i][j] += | ||
Math.max( | ||
fruits[i - 1][j - 1], | ||
Math.max(fruits[i - 1][j], (j + 1 < n) ? fruits[i - 1][j + 1] : 0)); | ||
} | ||
} | ||
for (int j = 1; j < n; ++j) { | ||
for (int i = j + 1; i < n; ++i) { | ||
fruits[i][j] += | ||
Math.max( | ||
fruits[i - 1][j - 1], | ||
Math.max(fruits[i][j - 1], (i + 1 < n) ? fruits[i + 1][j - 1] : 0)); | ||
} | ||
} | ||
return res + fruits[n - 1][n - 2] + fruits[n - 2][n - 1]; | ||
} | ||
} |
56 changes: 56 additions & 0 deletions
56
...ain/java/g3301_3400/s3363_find_the_maximum_number_of_fruits_collected/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,56 @@ | ||
3363\. Find the Maximum Number of Fruits Collected | ||
|
||
Hard | ||
|
||
There is a game dungeon comprised of `n x n` rooms arranged in a grid. | ||
|
||
You are given a 2D array `fruits` of size `n x n`, where `fruits[i][j]` represents the number of fruits in the room `(i, j)`. Three children will play in the game dungeon, with **initial** positions at the corner rooms `(0, 0)`, `(0, n - 1)`, and `(n - 1, 0)`. | ||
|
||
The children will make **exactly** `n - 1` moves according to the following rules to reach the room `(n - 1, n - 1)`: | ||
|
||
* The child starting from `(0, 0)` must move from their current room `(i, j)` to one of the rooms `(i + 1, j + 1)`, `(i + 1, j)`, and `(i, j + 1)` if the target room exists. | ||
* The child starting from `(0, n - 1)` must move from their current room `(i, j)` to one of the rooms `(i + 1, j - 1)`, `(i + 1, j)`, and `(i + 1, j + 1)` if the target room exists. | ||
* The child starting from `(n - 1, 0)` must move from their current room `(i, j)` to one of the rooms `(i - 1, j + 1)`, `(i, j + 1)`, and `(i + 1, j + 1)` if the target room exists. | ||
|
||
When a child enters a room, they will collect all the fruits there. If two or more children enter the same room, only one child will collect the fruits, and the room will be emptied after they leave. | ||
|
||
Return the **maximum** number of fruits the children can collect from the dungeon. | ||
|
||
**Example 1:** | ||
|
||
**Input:** fruits = [[1,2,3,4],[5,6,8,7],[9,10,11,12],[13,14,15,16]] | ||
|
||
**Output:** 100 | ||
|
||
**Explanation:** | ||
|
||
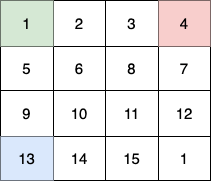 | ||
|
||
In this example: | ||
|
||
* The 1<sup>st</sup> child (green) moves on the path `(0,0) -> (1,1) -> (2,2) -> (3, 3)`. | ||
* The 2<sup>nd</sup> child (red) moves on the path `(0,3) -> (1,2) -> (2,3) -> (3, 3)`. | ||
* The 3<sup>rd</sup> child (blue) moves on the path `(3,0) -> (3,1) -> (3,2) -> (3, 3)`. | ||
|
||
In total they collect `1 + 6 + 11 + 1 + 4 + 8 + 12 + 13 + 14 + 15 = 100` fruits. | ||
|
||
**Example 2:** | ||
|
||
**Input:** fruits = [[1,1],[1,1]] | ||
|
||
**Output:** 4 | ||
|
||
**Explanation:** | ||
|
||
In this example: | ||
|
||
* The 1<sup>st</sup> child moves on the path `(0,0) -> (1,1)`. | ||
* The 2<sup>nd</sup> child moves on the path `(0,1) -> (1,1)`. | ||
* The 3<sup>rd</sup> child moves on the path `(1,0) -> (1,1)`. | ||
|
||
In total they collect `1 + 1 + 1 + 1 = 4` fruits. | ||
|
||
**Constraints:** | ||
|
||
* `2 <= n == fruits.length == fruits[i].length <= 1000` | ||
* `0 <= fruits[i][j] <= 1000` |
25 changes: 25 additions & 0 deletions
25
src/main/java/g3301_3400/s3364_minimum_positive_sum_subarray/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
package g3301_3400.s3364_minimum_positive_sum_subarray; | ||
|
||
// #Easy #Array #Prefix_Sum #Sliding_Window #2024_12_03_Time_1_ms_(100.00%)_Space_44.5_MB_(38.75%) | ||
|
||
import java.util.List; | ||
|
||
public class Solution { | ||
public int minimumSumSubarray(List<Integer> li, int l, int r) { | ||
int n = li.size(); | ||
int min = Integer.MAX_VALUE; | ||
int[] a = new int[n + 1]; | ||
for (int i = 1; i <= n; i++) { | ||
a[i] = a[i - 1] + li.get(i - 1); | ||
} | ||
for (int size = l; size <= r; size++) { | ||
for (int i = size - 1; i < n; i++) { | ||
int sum = a[i + 1] - a[i + 1 - size]; | ||
if (sum > 0) { | ||
min = Math.min(min, sum); | ||
} | ||
} | ||
} | ||
return min == Integer.MAX_VALUE ? -1 : min; | ||
} | ||
} |
Oops, something went wrong.