The best way to create constraints in code.
- Semantic. Align APIs focus on your goals, not the math behind Auto Layout constraints.
- Powerful. Create multiple constraints with a single line of code.
- Type Safe. Makes it impossible to create invalid constraints, at compile time.
- Fluent. Concise and clear API that follows Swift API Design Guidelines.
- Simple. Stop worrying about
translatesAutoresizingMaskIntoConstraints
and constraints activation.
Example usage:
// Core API
view.anchors.top.equal(superview.top)
view.anchors.width.equal(view.anchors.height * 2)
// Semantic API
view.anchors.edges.pin(insets: 20) // Pins to superview
view.anchors.edges.pin(to: superview.safeAreaLayoutGuide, insets: 20)
view.anchors.width.clamp(to: 10...40)
And here's something a bit more powerful:
view.anchors.edges.pin(insets: 20, alignment: .center)
The documentation for Align is created using DocC and covers all of its APIs in a clear visual way. There is also a cheat sheet available that lists all of the available APIs.
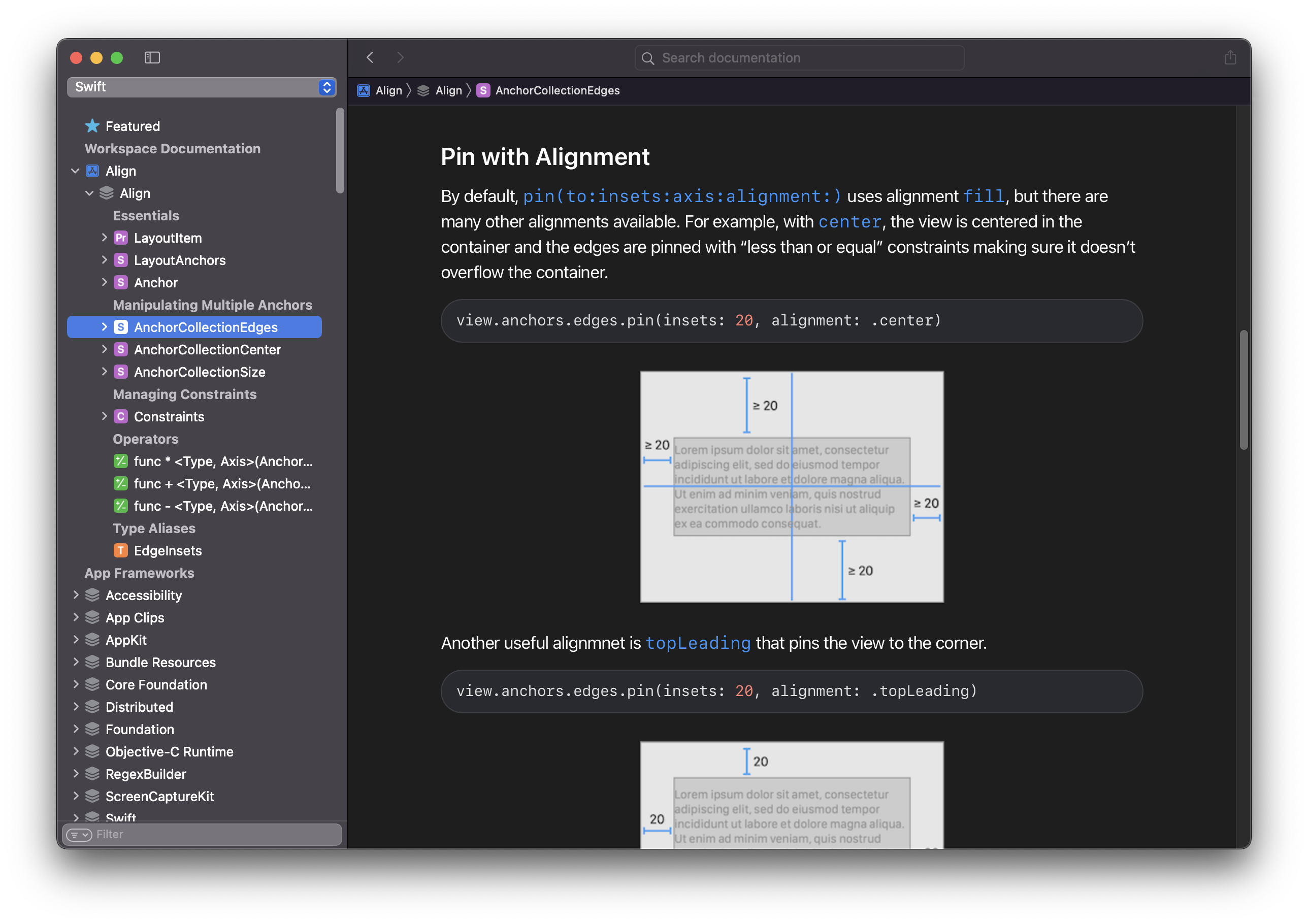
Align | Swift | Xcode | Platforms |
---|---|---|---|
Align 3.3 | Swift 5.10 | Xcode 15.3 | iOS 14, tvOS 14, macOS 10.16 |
Align 3.0 | Swift 5.6 | Xcode 13.3 | iOS 12, tvOS 12, macOS 10.14 |
Align strives for clarity and simplicity by following Swift API Design Guidelines. Although most of the APIs are compact, it is a non-goal to enable the most concise syntax possible.
Align is for someone who:
- Prefers fluent high-level APIs
- Doesn't want to depend on big, complex libraries – Align has only ~330 lines of code
- Prefers to have as little extensions for native classes as possible – Align adds a single property:
anchors
- Doesn't overuse operator overloads, prefers fast compile times
- Likes NSLayoutAnchor but wishes it had simpler API which didn't require manually activating constraints