-
Notifications
You must be signed in to change notification settings - Fork 9.4k
magento/magento2#20773: Do not throw exception during autoload #20950
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Changes from all commits
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,85 @@ | ||
<?php declare(strict_types=1); | ||
/** | ||
* Copyright © Magento, Inc. All rights reserved. | ||
* See COPYING.txt for license details. | ||
*/ | ||
|
||
namespace Magento\Framework\Code\Generator; | ||
|
||
use Magento\Framework\Code\Generator; | ||
use Magento\Framework\Logger\Monolog as MagentoMonologLogger; | ||
use Magento\TestFramework\ObjectManager; | ||
use PHPUnit\Framework\TestCase; | ||
use PHPUnit_Framework_MockObject_MockObject as MockObject; | ||
use Psr\Log\LoggerInterface; | ||
|
||
class AutoloaderTest extends TestCase | ||
{ | ||
/** | ||
* This method exists to fix the wrong return type hint on \Magento\Framework\App\ObjectManager::getInstance. | ||
* This way the IDE knows it's dealing with an instance of \Magento\TestFramework\ObjectManager and | ||
* not \Magento\Framework\App\ObjectManager. The former has the method addSharedInstance, the latter does not. | ||
* | ||
* @return ObjectManager|\Magento\Framework\App\ObjectManager | ||
* @SuppressWarnings(PHPMD.StaticAccess) | ||
*/ | ||
private function getTestFrameworkObjectManager() | ||
{ | ||
return ObjectManager::getInstance(); | ||
} | ||
|
||
/** | ||
* @before | ||
*/ | ||
public function setupLoggerTestDouble(): void | ||
{ | ||
$loggerTestDouble = $this->createMock(LoggerInterface::class); | ||
$this->getTestFrameworkObjectManager()->addSharedInstance($loggerTestDouble, MagentoMonologLogger::class); | ||
} | ||
|
||
/** | ||
* @after | ||
*/ | ||
public function removeLoggerTestDouble(): void | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Maybe just call them There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. I prefer not to override parent class methods. The There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Such name does not add any value compared to just |
||
{ | ||
$this->getTestFrameworkObjectManager()->removeSharedInstance(MagentoMonologLogger::class); | ||
} | ||
|
||
/** | ||
* @param \RuntimeException $testException | ||
* @return Generator|MockObject | ||
*/ | ||
private function createExceptionThrowingGeneratorTestDouble(\RuntimeException $testException) | ||
{ | ||
/** @var Generator|MockObject $generatorStub */ | ||
$generatorStub = $this->createMock(Generator::class); | ||
$generatorStub->method('generateClass')->willThrowException($testException); | ||
|
||
return $generatorStub; | ||
} | ||
|
||
public function testLogsExceptionDuringGeneration(): void | ||
{ | ||
$exceptionMessage = 'Test exception thrown during generation'; | ||
$testException = new \RuntimeException($exceptionMessage); | ||
|
||
$loggerMock = ObjectManager::getInstance()->get(LoggerInterface::class); | ||
$loggerMock->expects($this->once())->method('debug')->with($exceptionMessage, ['exception' => $testException]); | ||
|
||
$autoloader = new Autoloader($this->createExceptionThrowingGeneratorTestDouble($testException)); | ||
$this->assertNull($autoloader->load(NonExistingClassName::class)); | ||
} | ||
|
||
public function testFiltersDuplicateExceptionMessages(): void | ||
{ | ||
$exceptionMessage = 'Test exception thrown during generation'; | ||
$testException = new \RuntimeException($exceptionMessage); | ||
|
||
$loggerMock = ObjectManager::getInstance()->get(LoggerInterface::class); | ||
$loggerMock->expects($this->once())->method('debug')->with($exceptionMessage, ['exception' => $testException]); | ||
|
||
$autoloader = new Autoloader($this->createExceptionThrowingGeneratorTestDouble($testException)); | ||
$autoloader->load(OneNonExistingClassName::class); | ||
$autoloader->load(AnotherNonExistingClassName::class); | ||
} | ||
} |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Could you please inline this method? I don't see how it improves readability and also
ObjectManager::getInstance()
is used as is at least once.Uh oh!
There was an error while loading. Please reload this page.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
The return type hint fixes the type inference in PHPStorm (see screenshot).
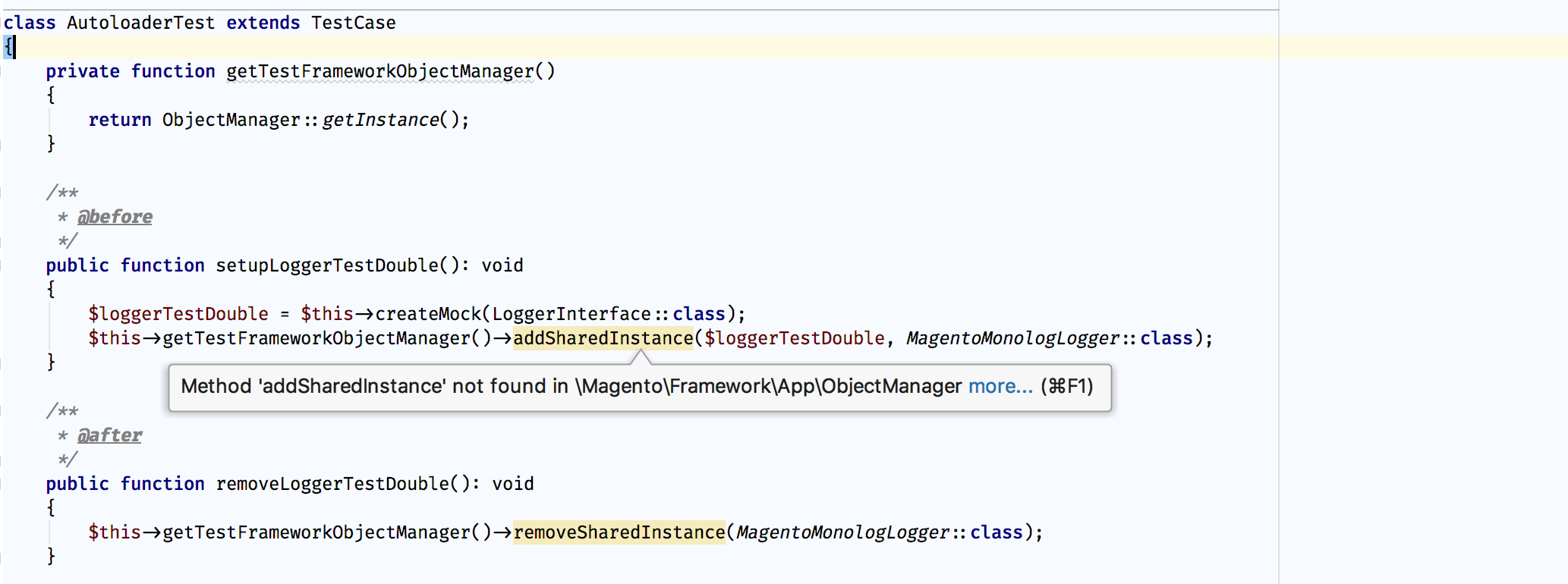
Uh oh!
There was an error while loading. Please reload this page.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Apropos: I should make a PR to fix the return annotation on
getInstance
on the test framework ObjectManager - this has been annoying me for 2.5 years now.There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@Vinai yep, there is no need to bloat each single test.