-
Notifications
You must be signed in to change notification settings - Fork 219
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: (LSP) remove unused imports (#6129)
# Description ## Problem Part of #1579 ## Summary This implements another commonly used feature: "remove unused import/s". This is when the entire `use` item is removed: 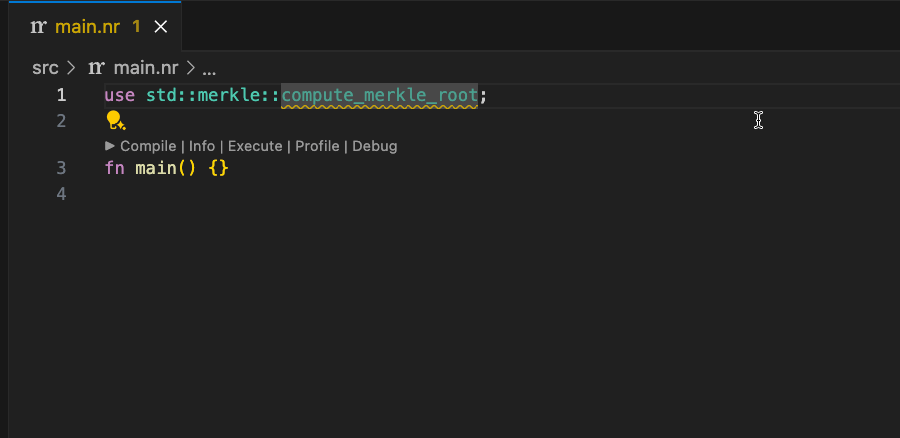 This is when part of the `use` item is removed:  This is when "Remove all the unused imports" is chosen with a selection range: 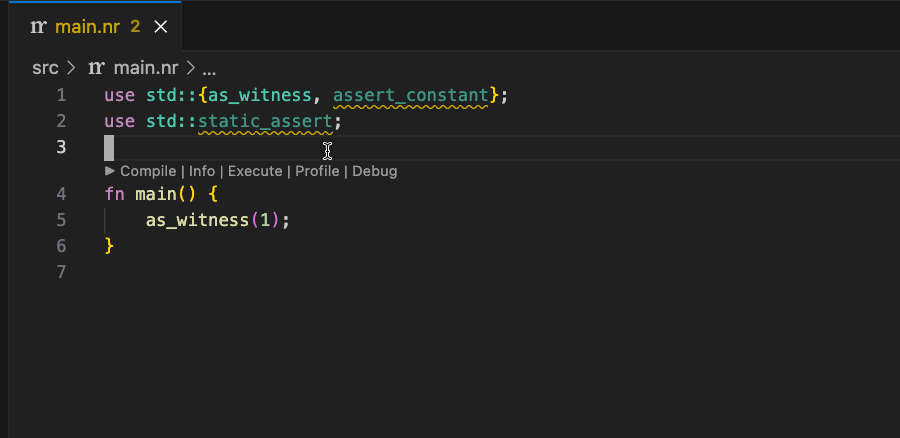 ## Additional Context It works exactly the same as Rust Analyzer: 1. You get a "Remove unused import", "Remove unused imports" or "Remove the entire `use` item" on each `use` item that has unused import(s) 2. You also get a "Remove all the unused imports" code action. This will remove all unused imports in the current selection. I need to clarify 2 because it's something I learned while coding this: a code action is suggested in the selection you have (the selection is the cursor in case there's no selection, though VSCode would sometimes use the current line's error span as the selection). That's why if you have the cursor on an import and choose "Remove all the unused imports" it just removes the imports in the current `use` item. You can get VSCode to actually remove **all** the unused imports if you select all the `use` items, then ask for code actions, then choose "Remove all the unused imports". To be honest this is kind of annoying, but it kind of also makes sense. We _could_ make "Remove all the unused imports" remove all of them regardless of what's the selection (or, well, if at least one selection has unused items) but we could do that as a follow-up. --- Other things to know: 1. This works by creating a new `UseTree` from the original `UseTree` but with imports removed, then converting that to a string (formatting it with `nargo fmt`). What happens if there were comments in the middle of the import? They are lost! But... this is also what Rust Analyzer does. I guess worse case scenario is you revert that code action and manually remove things (comments in these positions are very unlikely to happen). 2. I didn't test the "Remove all the unused imports" action because right now our tests don't support a selection range. But given that the logic for that is relatively simple (just get all the "remove unused import" edits and present that as a new code action) I thought it was okay not to test it (I did try it out, though). We could eventually add tests for this, though. ## Documentation Check one: - [x] No documentation needed. - [ ] Documentation included in this PR. - [ ] **[For Experimental Features]** Documentation to be submitted in a separate PR. # PR Checklist - [x] I have tested the changes locally. - [x] I have formatted the changes with [Prettier](https://prettier.io/) and/or `cargo fmt` on default settings.
- Loading branch information
Showing
8 changed files
with
379 additions
and
26 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.