-
Notifications
You must be signed in to change notification settings - Fork 27.8k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add type inference for getStaticProps and getServerSideProps (#11842)
This adds `InferredStaticProps` and `InferredServerSideProps` to the typings. - [x] add types for type inference - [x] add explanation to docs - [ ] tests - are there any? 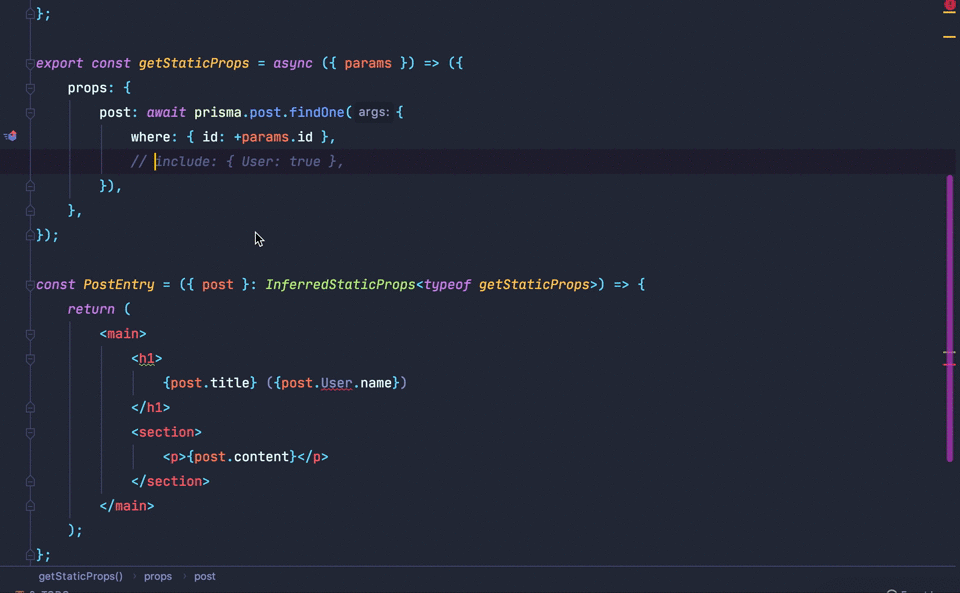 ### What does it do: As an alternative to declaring your Types manually with: ```typescript type Props = { posts: Post[] } export const getStaticProps: GetStaticProps<Props> = () => ({ posts: await fetchMyPosts(), }) export const MyComponent(props: Props) =>( // ... ); ``` we can now also infer the prop types with ```typescript export const getStaticProps = () => ({ // given fetchMyPosts() returns type Post[] posts: await fetchMyPosts(), }) export const MyComponent(props: InferredStaticProps<typeof getStaticProps>) =>( // props.posts will be of type Post[] ); ``` ### help / review wanted - [ ] I am no typescript expert. Although the solution works as intended for me, someone with more knowledge could probably improve the types. Any edge cases I missed? - [ ] are there any tests I should modify/ add?
- Loading branch information
1 parent
37f4353
commit 9f1b4f5
Showing
6 changed files
with
178 additions
and
7 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,46 @@ | ||
import { | ||
InferGetStaticPropsType, | ||
GetStaticPaths, | ||
GetStaticPropsContext, | ||
} from 'next' | ||
|
||
type Post = { | ||
author: string | ||
content: string | ||
} | ||
|
||
export const getStaticPaths: GetStaticPaths = async () => { | ||
return { | ||
paths: [{ params: { post: '1' } }], | ||
fallback: false, | ||
} | ||
} | ||
|
||
export const getStaticProps = async ( | ||
ctx: GetStaticPropsContext<{ post: string }> | ||
) => { | ||
const posts: Post[] = [ | ||
{ | ||
author: 'Vercel', | ||
content: 'hello wolrd', | ||
}, | ||
] | ||
|
||
return { | ||
props: { | ||
posts, | ||
}, | ||
} | ||
} | ||
|
||
function Blog({ posts }: InferGetStaticPropsType<typeof getStaticProps>) { | ||
return ( | ||
<> | ||
{posts.map((post) => ( | ||
<div key={post.author}>{post.author}</div> | ||
))} | ||
</> | ||
) | ||
} | ||
|
||
export default Blog |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
import { InferGetStaticPropsType } from 'next' | ||
|
||
export const getStaticProps = async () => { | ||
return { | ||
props: { data: ['hello', 'world'] }, | ||
unstable_revalidate: false, | ||
} | ||
} | ||
|
||
export default function Page({ | ||
data, | ||
}: InferGetStaticPropsType<typeof getStaticProps>) { | ||
return <h1>{data.join(' ')}</h1> | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
import { InferGetServerSidePropsType, GetServerSidePropsContext } from 'next' | ||
|
||
type Post = { | ||
author: string | ||
content: string | ||
} | ||
|
||
export const getServerSideProps = async ( | ||
ctx: GetServerSidePropsContext<{ post: string }> | ||
) => { | ||
const res = await fetch(`https://.../posts/`) | ||
const posts: Post[] = await res.json() | ||
return { | ||
props: { | ||
posts, | ||
}, | ||
} | ||
} | ||
|
||
function Blog({ | ||
posts, | ||
}: InferGetServerSidePropsType<typeof getServerSideProps>) { | ||
return ( | ||
<> | ||
{posts.map((post) => ( | ||
<div key={post.author}>{post.author}</div> | ||
))} | ||
</> | ||
) | ||
} | ||
|
||
export default Blog |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters