Fix memory leak in pipe loop reference implementation #968
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
The reference implementation of
ReadableStream.pipeTo
leaks memory when piping a lot of chunks.Steps to reproduce
npm i heapdump
leak.js
containing the code snippet belownode --expose_gc leak.js
before.heapsnapshot
andafter.heapsnapshot
) in Chrome DevToolsafter
profile and switch to the Comparison viewleak.js
Expected results
Promise
instances (# Delta
) is zero.Actual results
Promise
instances is 10,000.Root cause analysis
The cause of this memory leak is due to
pipeLoop
creating a long promise chain using recursion:Every time a chunk is piped through, the promise chain is extended. This chain keeps a ton of promises alive, and cannot be garbage collected until the pipe ends (i.e. when either end closes or errors).
Proposed solution
This PR fixes the memory leak by creating a single
Promise
for the return value ofpipeLoop
, and manually resolving/rejecting it.With this fix, the leak is gone:
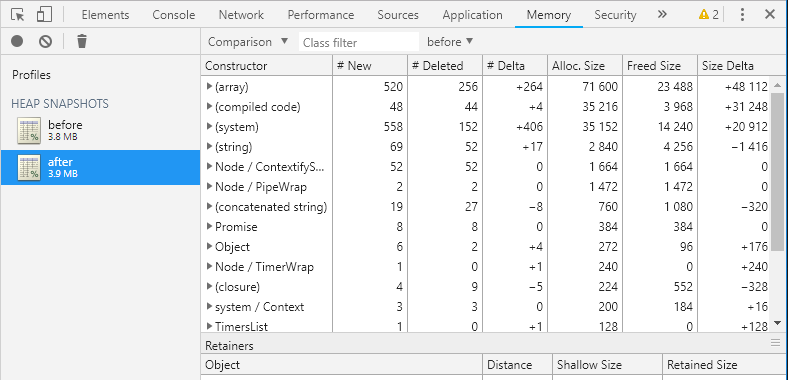
Environment