Added viewmodel FOV cvar, added viewmodel xyz offset cvar #107
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
Hello. This closes #26.
Abstract
@execut4ble says:
AFAIK CSGO's
viewmodel_fov
doesn't actually change the viewmodel_FOV at all, it's merely (I THINK) changing the origin from which the "camera" is looking at the gun (or it's actually just keeping the origin the same, but changing how it's "looked at" some other way, but you get the point).This is different to what other source games e.g. Portal/HL2/HL2EP1 do, where viewmodel_fov actually changes the FOV for the viewmodel "camera".
I've implemented cvars for both cases (although the CS:GO offset thing isn't exactly like in CS:GO, obv, you'll see what I mean). See the reference screenshots & videos at the end of this post.
Just for reference here's what the Portal's
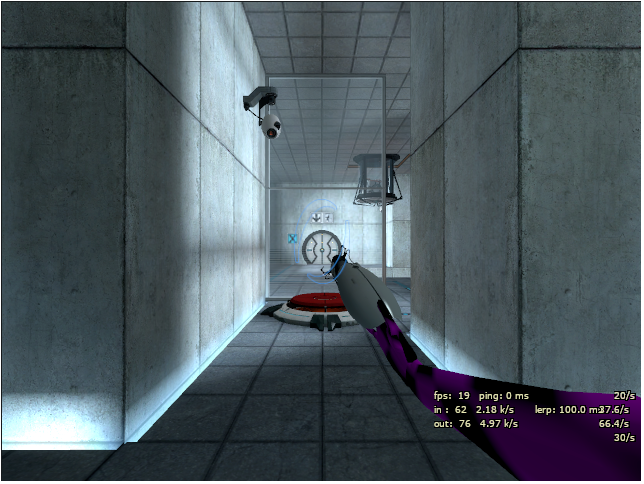
viewmodel_fov
does and whatviewmodel_fov
IMHO should be and therefore what viewmodel_fov in my PR means:Cvars
Both the FOV and offset cvars start with cl_viewmodel for constitency and easy access.
Viewmodel FOV
cl_viewmodel_fov 0
orcl_viewmodel_fov 1-179
- 0 means disabled, 1-179 means the FOV for the gun.default_fov
. (As opposed to for example HL2 where viewmodel_fov defaults to 54 and FOV itself defaults to 75/90)Examples might be easier to understand:
default_fov 120
&cl_viewmodel_fov 0
= this means gun will look like it does on default_fov 120, since cl_viewmodelfov is disabled.default_fov 120
&cl_viewmodel_fov 120
= this means gun will look like it does on default_fov 120, since cl_viewmodelfov is the same value as default_fov -- 120.default_fov 120
&cl_viewmodel_fov 140
= this means gun will look like it would if default_fov was 140, but the rest of the view will still be defaut_fov 120.Therefore if cl_viewmodel_fov is set to 0, the viewmodel FOV will be the same as default game, aka it will be set by
default_fov
like the rest of the view.Viewmodel offset
For the offset variables I've opted for
_right
,_forward
and_up
instead of_y
_x
_z
for the user experience, because technically the_right
variable moves the "camera's" "origin" to the left, but the gun appears more to the right, therefore I've opted for these names.Be aware that these values can be negative. Values that make sense are more or less -100 to 100, they aren't capped, but you usually can't see the gun anymore above 100 or below -100 on basically any FOV.
cl_viewmodel_ofs_right
- 0 means no offset, offsets the origin of the camera to the left, therefore moving the gun to the rightcl_viewmodel_ofs_forward
- 0 means no offset, offsets the origin of the camera to the back, therefore moving the gun forward ("in front of you")cl_viewmodel_ofs_up
- 0 means no offset, offsets the origin of the camera to the bottom, therefore moving the gun up ("above you")Implementation: offsets
These are pretty self-explanatory, they offset the origin from which the "camera" is looking at the viewmodel, this is NOT the same thing as the player's camera. If cl_righthand is 1 (aka gordon becomes lefthanded) the "_ofs_right" offset is multiplied by -1 to get the correct values for the "other side".
Implementation: viewmodel FOV
Uh... where do I start...
StudioModelRenderer.cpp (CStudioModelRenderer)
As the name suggests this classes takes care of parsing studio .mdl models, it sets up bones, adds proper lighting, texture materials, sets up the model body parts and renders them. The code however doesn't tell you much as this file in the client dll module is mostly just using the
IEngineStudio
interface API and the engine takes care of the rest, if I skip the parts that are not important to my implementation:Steps:
<1> --- For us non-important steps like: load model, setup bones, setup lighting, load up textures etc. ---
<2> SetupRenderer - setups the renderer (and sets glStuff to "model rendering" "mode")
<3> Iterates over the bodyparts of the model
mstudiomodel_t
&mstudiobodyparts_t
)<4> Restores the renderer (and therefore sets glStuff back to "non-model rendering" "mode")
Like I've said, this whole process uses the
IEngineStudio
for all these steps so it's not transparent, what however, is transparent is the modelviewer Valve(?) made and the filestudio_render.cpp
that comes with it. Every model viewer (Jed's MV, HLMV-Qt, HLAM) uses the same base from the modelviewer here: https://github.com/ValveSoftware/halflife/tree/master/utils/mdlviewer, although personally I've been using the code of this one as a reference: https://github.com/MoeMod/HLMV-Qt (they all the same anyway, except modelviewer has like no features at all).The code isn't 1:1 to what the engine probably does when the client dll calls
IEngineStudio
but you can get a pretty good idea of what is mostly happening in these IEngineStudio calls. Therefore if you look at this piece of code instudio_render.cpp:DrawModel()
:You can see that it iterates the bodyparts and setups the model, which in our case is what steps 2) and 3) a) are, see here:
You can see that it also calls a
DrawPoints()
function that we also have inStudioModelRenderer.cpp
as an API call:The DrawPoints function is the one that does the final render and therefore does OpenGL calls e.g.:
studio_render.cpp
Therefore this is the exact spot where we should do our OpenGL calls - before the
DrawPoints
function is called and renders the actual model:The Renderer is then restored using an API call
IEngineStudio.RestoreRenderer()
hence why the next render calls (the ones that AREN'T for a viewmodel and therefore won't call our custom OpenGL stuff function) won't have our trickery anymore! \o/The function
SetViewmodelFovProjection()
then calculates the FOV based on the screen resolution (window size) just likedefault_fov
would and sets the custom projection so that the DrawPoints function OpenGL stuff usees that projection instead of the one it usually gets from the engine.When
cl_viewmodel_fov
is set to 0,SetViewmodelFovProjection
isn't called at all and FOV is the same as default game (aka weapon has the same FOV as the whole game set bydefault_fov
)Hopefully this all makes sense, it's almost 4 am and I'm kinda tired. :| Sorry.
Reference screenshots and videos
Video showcasing the cl_viewmodel_fov variable: https://www.youtube.com/watch?v=SujrFh9pnws (sorry for abrupt endng, i had to cut it off because of me mumbling about cl_viewmodel_ofs for too long)
Screenshots, these are self-explanatory, you can see the values used in each screenshot in the console window in the top left corner. 🙂
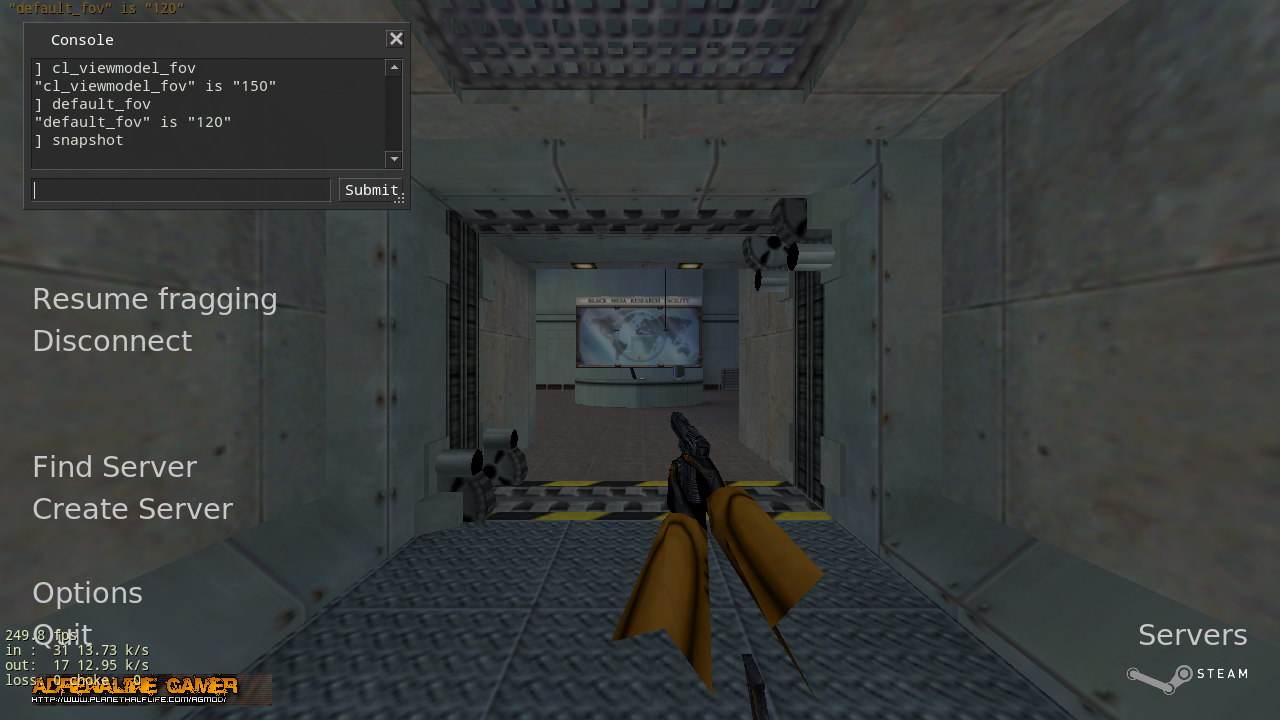
default_fov=120 & cl_viewmodel_fov=0 ↓ ...
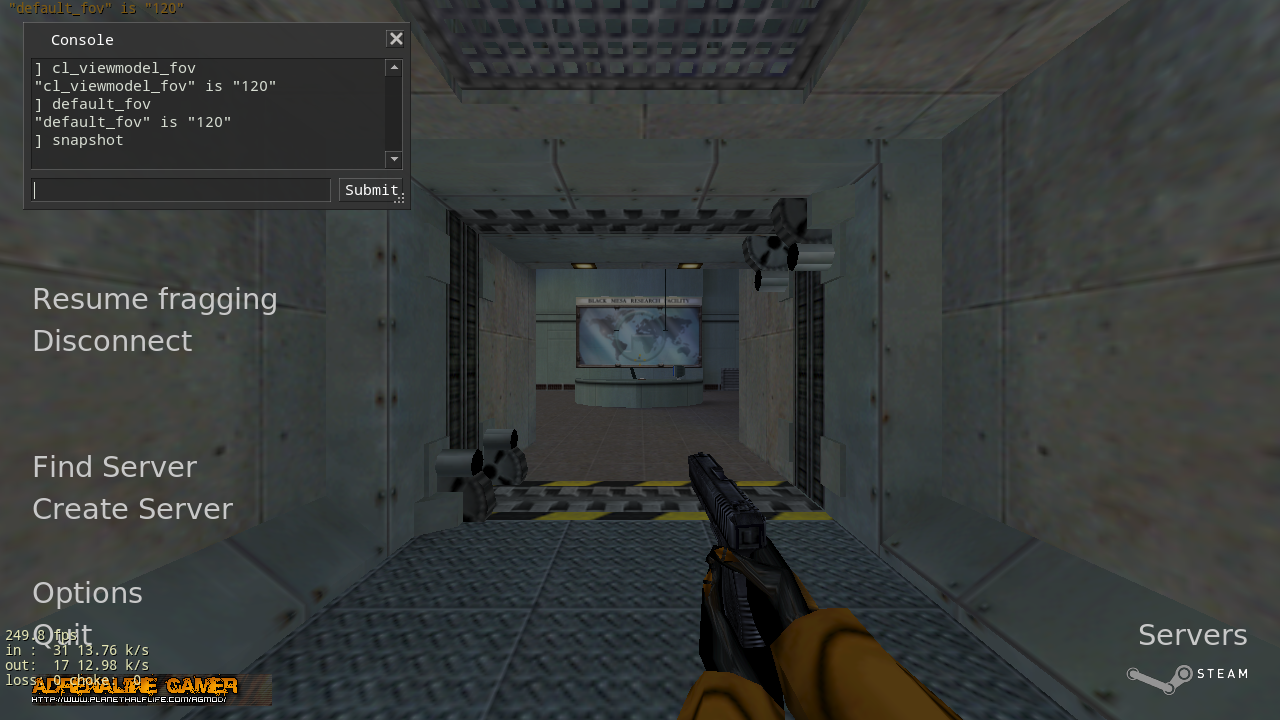
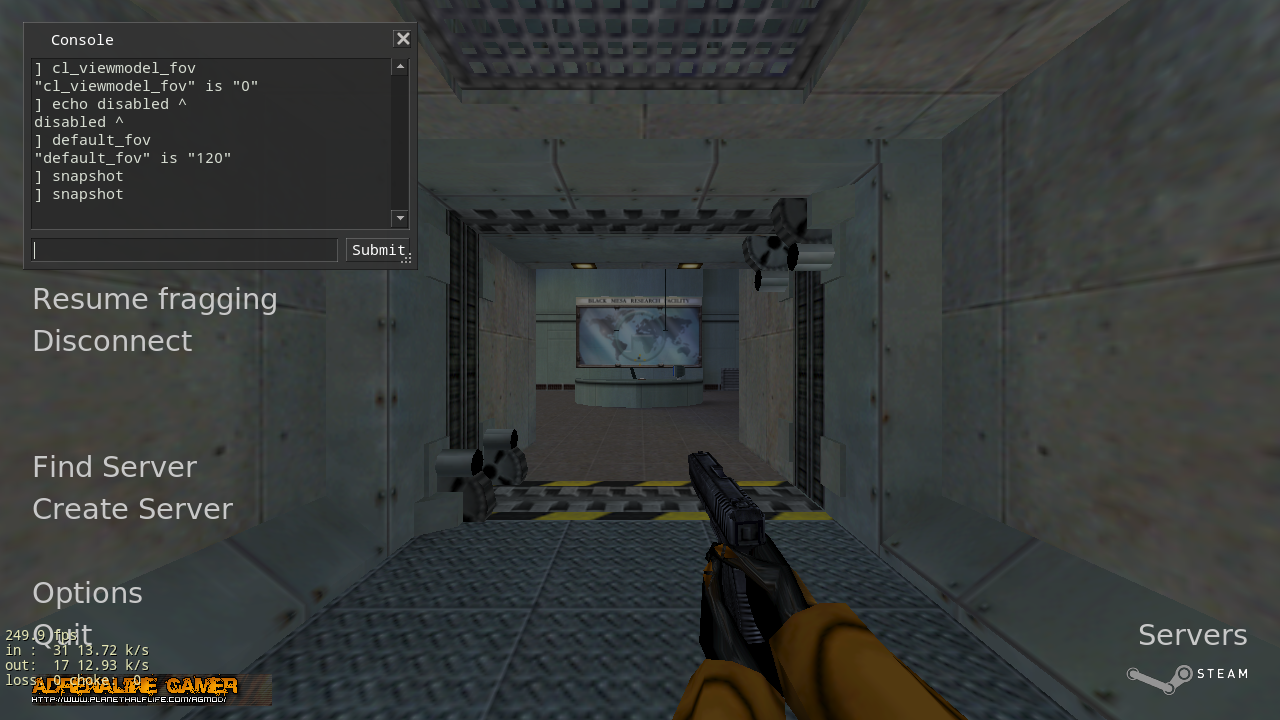
... is the same as default_fov=120 & cl_viewmodel_fov=120 ↓
Very immersive:
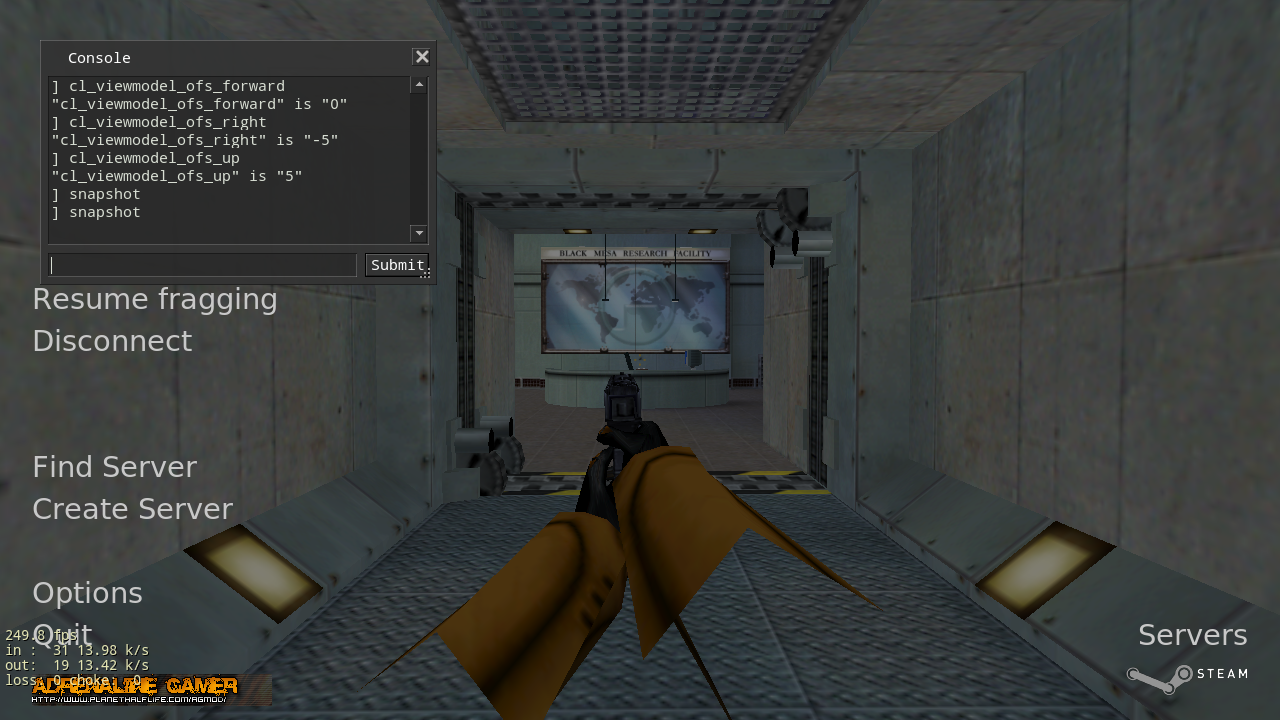
cl_righthand showcase:
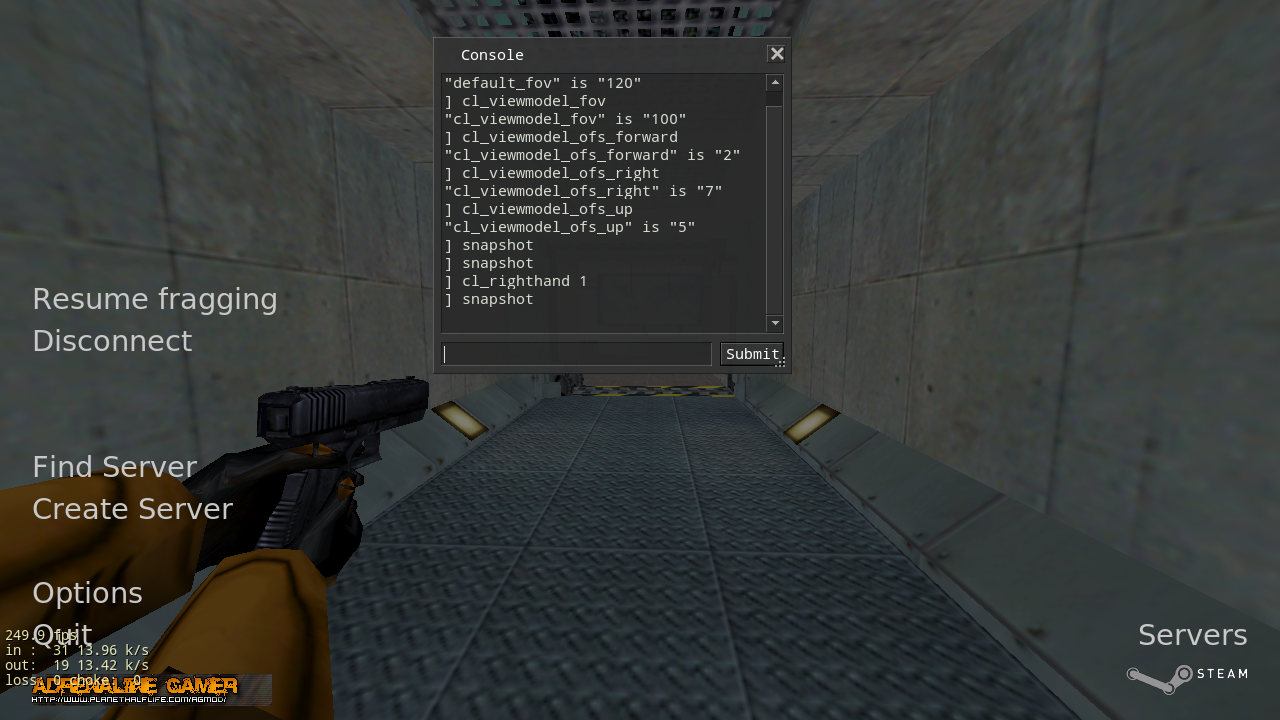
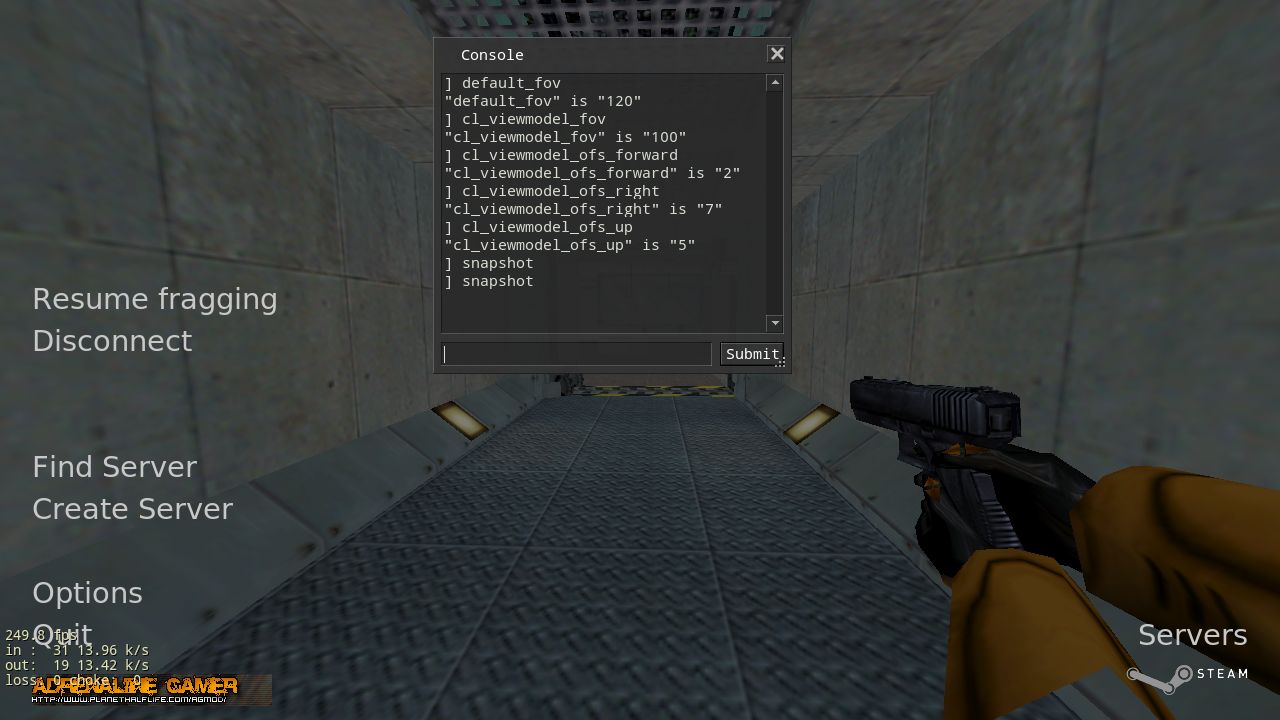
TODO