This repository was archived by the owner on Nov 20, 2018. It is now read-only.
Commit 2f83855
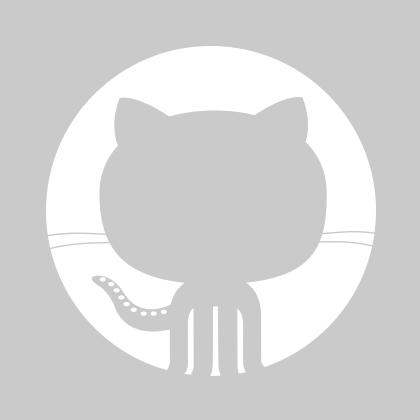
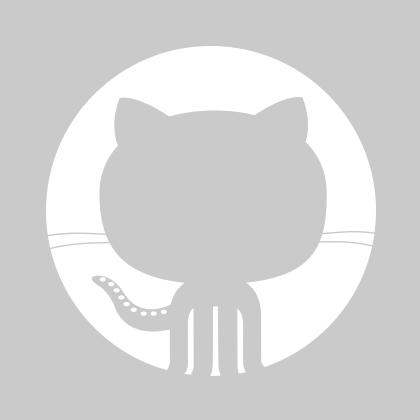
Justin Kotalik
Justin Kotalik
1 parent e320755 commit 2f83855
File tree
8 files changed
+247
-8
lines changed- src
- Microsoft.AspNetCore.Http.Abstractions
- Microsoft.AspNetCore.Http.Extensions
- test
- Microsoft.AspNetCore.Http.Abstractions.Tests
- Microsoft.AspNetCore.Http.Extensions.Tests
8 files changed
+247
-8
lines changedLines changed: 7 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
107 | 107 |
| |
108 | 108 |
| |
109 | 109 |
| |
110 |
| - | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
111 | 115 |
| |
112 | 116 |
| |
113 | 117 |
| |
114 | 118 |
| |
115 | 119 |
| |
116 | 120 |
| |
117 |
| - | |
| 121 | + | |
118 | 122 |
| |
119 | 123 |
| |
120 | 124 |
| |
121 | 125 |
| |
122 | 126 |
| |
123 | 127 |
| |
124 |
| - | |
| 128 | + | |
125 | 129 |
| |
126 | 130 |
| |
127 | 131 |
| |
|
Lines changed: 6 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
205 | 205 |
| |
206 | 206 |
| |
207 | 207 |
| |
| 208 | + | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
208 | 212 |
| |
209 | 213 |
| |
210 | 214 |
| |
| |||
217 | 221 |
| |
218 | 222 |
| |
219 | 223 |
| |
220 |
| - | |
| 224 | + | |
221 | 225 |
| |
222 | 226 |
| |
223 | 227 |
| |
| |||
228 | 232 |
| |
229 | 233 |
| |
230 | 234 |
| |
231 |
| - | |
| 235 | + | |
232 | 236 |
| |
233 | 237 |
| |
234 | 238 |
| |
|
Lines changed: 7 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
215 | 215 |
| |
216 | 216 |
| |
217 | 217 |
| |
218 |
| - | |
| 218 | + | |
| 219 | + | |
| 220 | + | |
| 221 | + | |
| 222 | + | |
219 | 223 |
| |
220 | 224 |
| |
221 | 225 |
| |
222 | 226 |
| |
223 | 227 |
| |
224 | 228 |
| |
225 |
| - | |
| 229 | + | |
226 | 230 |
| |
227 | 231 |
| |
228 | 232 |
| |
229 | 233 |
| |
230 | 234 |
| |
231 | 235 |
| |
232 |
| - | |
| 236 | + | |
233 | 237 |
| |
234 | 238 |
| |
235 | 239 |
| |
|
Lines changed: 64 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
11 | 11 |
| |
12 | 12 |
| |
13 | 13 |
| |
| 14 | + | |
| 15 | + | |
| 16 | + | |
14 | 17 |
| |
15 | 18 |
| |
16 | 19 |
| |
| |||
70 | 73 |
| |
71 | 74 |
| |
72 | 75 |
| |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
73 | 137 |
| |
74 | 138 |
| |
75 | 139 |
| |
|
Lines changed: 40 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + |
Lines changed: 30 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
92 | 92 |
| |
93 | 93 |
| |
94 | 94 |
| |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
95 | 125 |
| |
96 | 126 |
|
Lines changed: 30 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
105 | 105 |
| |
106 | 106 |
| |
107 | 107 |
| |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
| 137 | + | |
108 | 138 |
| |
109 | 139 |
|
Lines changed: 63 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
66 | 66 |
| |
67 | 67 |
| |
68 | 68 |
| |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
69 | 132 |
| |
70 | 133 |
|
0 commit comments