-
Notifications
You must be signed in to change notification settings - Fork 505
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Add fisheye camera model #486
Add fisheye camera model #486
Conversation
This is great and very exciting. All that is left is to hook it up to an ObservationTransformer like we have for Equirect generation. Also need to add tests briefly like we have for stitching equirect. Are there any optimizations that we could use to lessen the number of sensors required btw? Like if the FOV is < 180 is it possible to construct the input from only 5 sensors? That complexity may not be worth it though. Excellent work! |
Thank you for your comment! I added ObservationTransformer and test function for generating fisheye images.
I think it is possible, but I'd rather not do it because it will complicate the process and I'm not sure how much is the improvement. Thank you! |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I see. Good to know I can combine multiple @pytest.mark.parametrize
.
* Add VelocityControl structure for setting desired constant velocity in world and local frame. Interface with PhysicsManager and BulletPhysicsManager. * Add kinematic integrator for velocity control without dynamics. * Added tests for velocity control
Example can not run: Config search path: |
* Add fisheye camera model * Add ObservationTransformer for fisheye camera * Add test for CubeMap2Fisheye * Add comment about future refactor * Update tests Co-authored-by: Aaron Gokaslan <agokaslan@fb.com>
* Add fisheye camera model * Add ObservationTransformer for fisheye camera * Add test for CubeMap2Fisheye * Add comment about future refactor * Update tests Co-authored-by: Aaron Gokaslan <agokaslan@fb.com>
Motivation and Context
It will solve facebookresearch/habitat-sim#794.
Currently, equirectangular images are available aside from perspective images thanks to @Skylion007 . This pull request adds fisheye camera model. The fisheye model is based on the Double Sphere Camera Model. I chose this fisheye camera model because it can handle more than 180 degrees of FoV and the unprojection is fast according to their paper.
I'm not sure if you are interested in generating fisheye images, so currently, it's just draft pull request. Please tell me what you think.
Basically, the structure is the almost same as
Cube2Equirec
except that different projection on a sphere based on camera parameters, taking care of fov, and making sure each point is only assigned to single face.So, when you will marge this camera model, maybe it's better to create a parent class like
Cube2Omni
, andCube2Equirec
andCube2Fisheye
are inhirited from the parent class with different implementation of different projection on a sphere. But, it will require a lot of changes, so I will leave it to you.How Has This Been Tested
You can generate fisheye images by the following code.
The generated fisheye image:
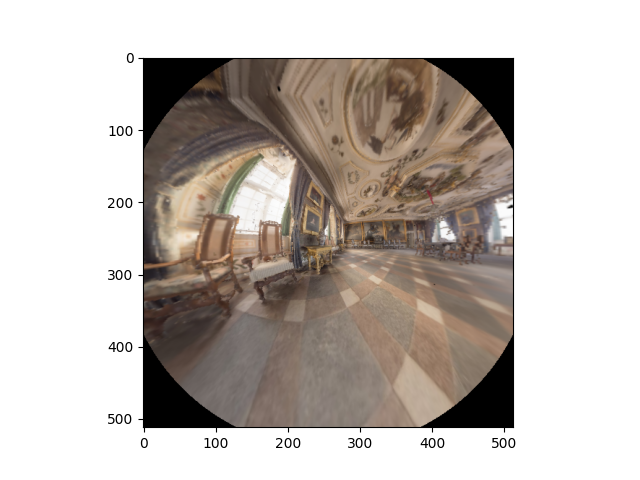
Types of changes
Checklist