forked from facebook/rocksdb
-
Notifications
You must be signed in to change notification settings - Fork 95
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
This PR optimizes consistency checks performance for the case with a lot of deleted files. When doing `DeleteFilesInRange` with `force_consistency_checks` on for a large range which spans about 16.5K SST files, we found it spent most of the time in `LogAndApply`(the time in red rectangle). 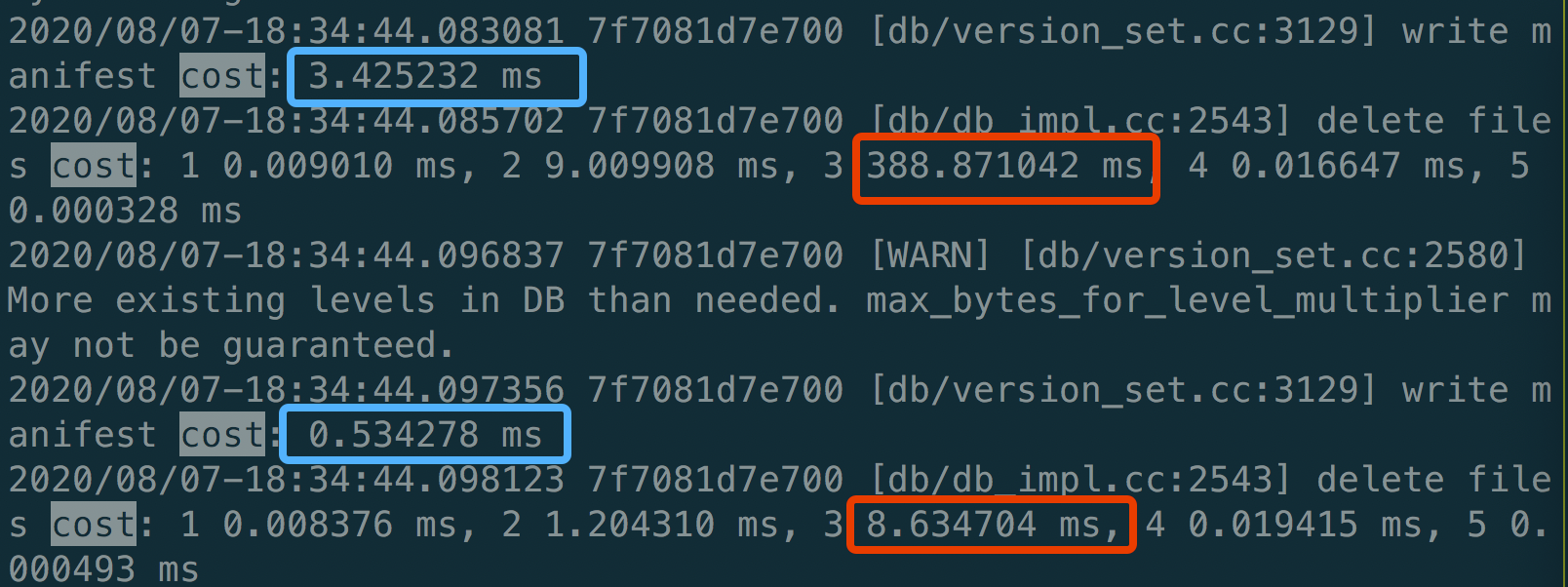 In `CheckConsistencyForDeletes`, it traverses the whole LSM to check if the file existed in the previous version for every deleted file. In the case of a lot of deleted files such as `DeleteFilesInRange`, it would waste too much time on this operation. Even worse, it is done with db mutex held, so it may greatly affect the foreground write performance. After making the check in batch with only one round of traverse of the LSM, the time is greatly reduced. 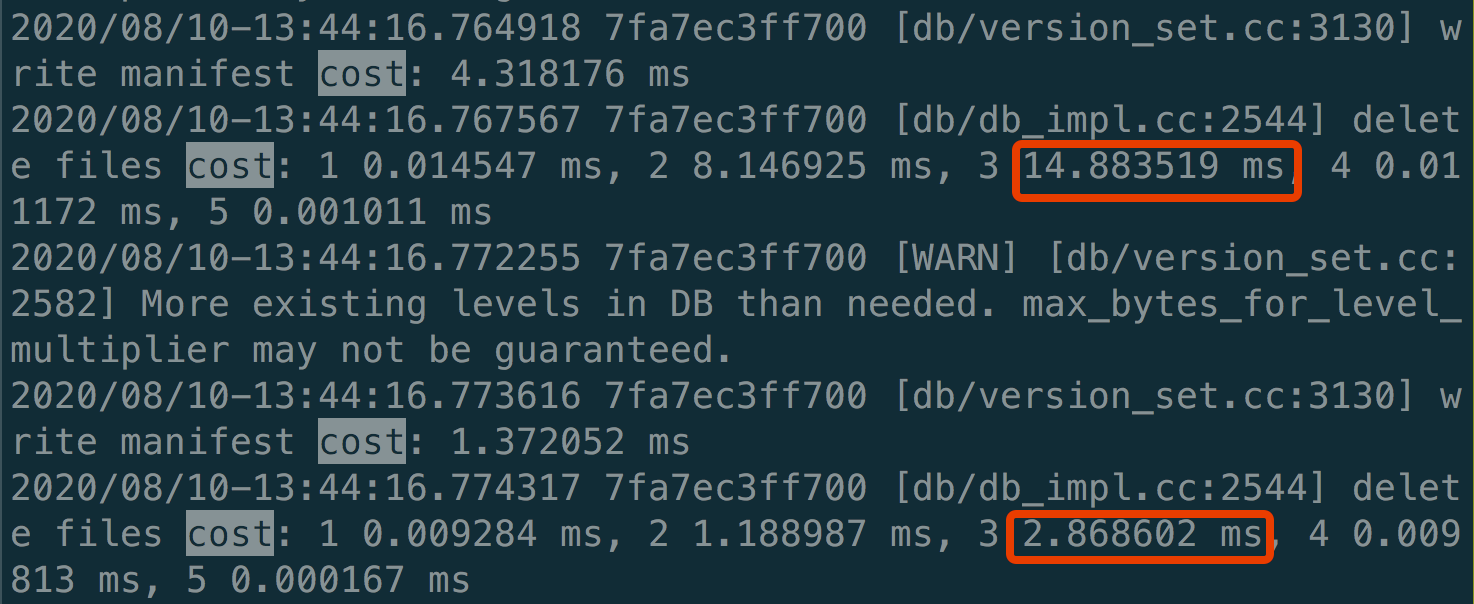 Signed-off-by: Connor1996 <zbk602423539@gmail.com>
- Loading branch information
1 parent
b4321b1
commit 042c90b
Showing
2 changed files
with
56 additions
and
41 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters